for Loop ICS 111 Introduction to Computer Science
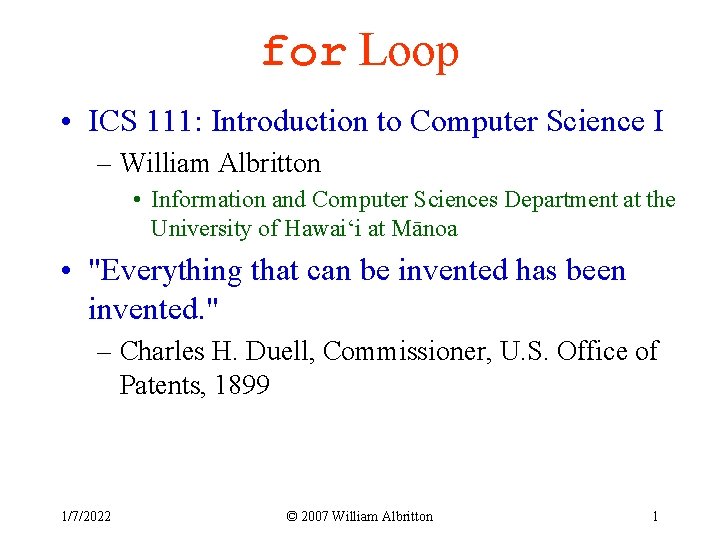
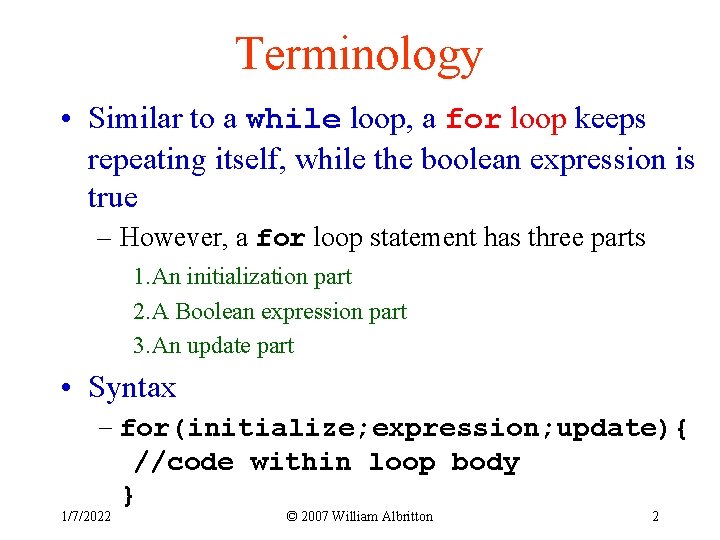
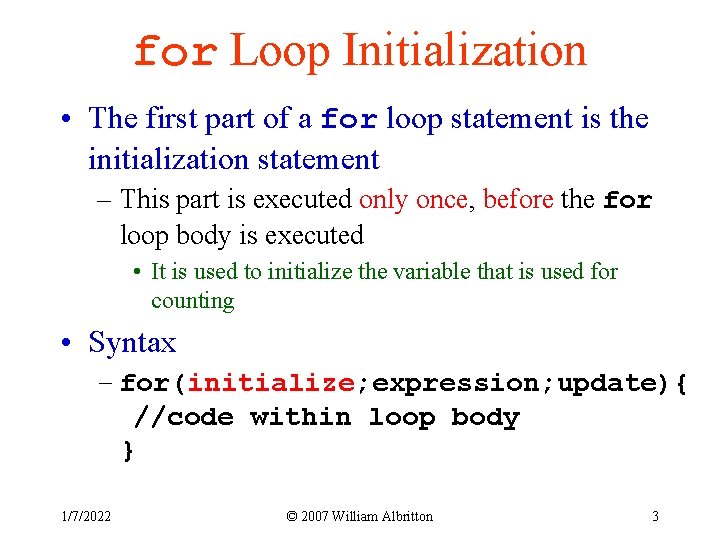
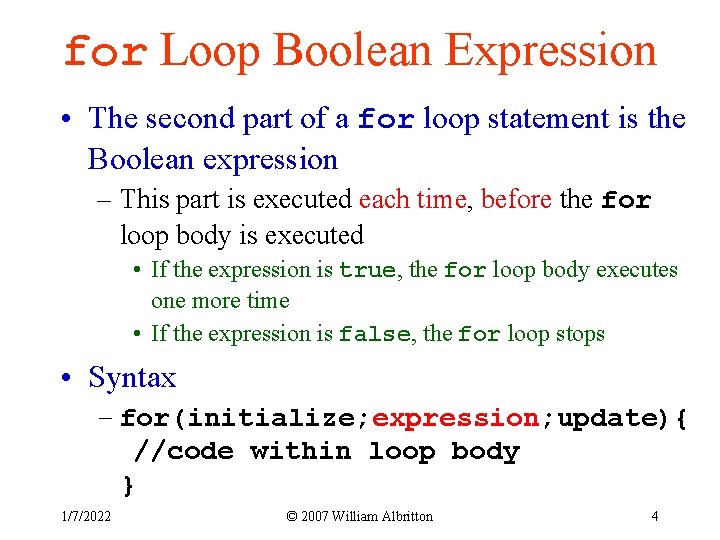
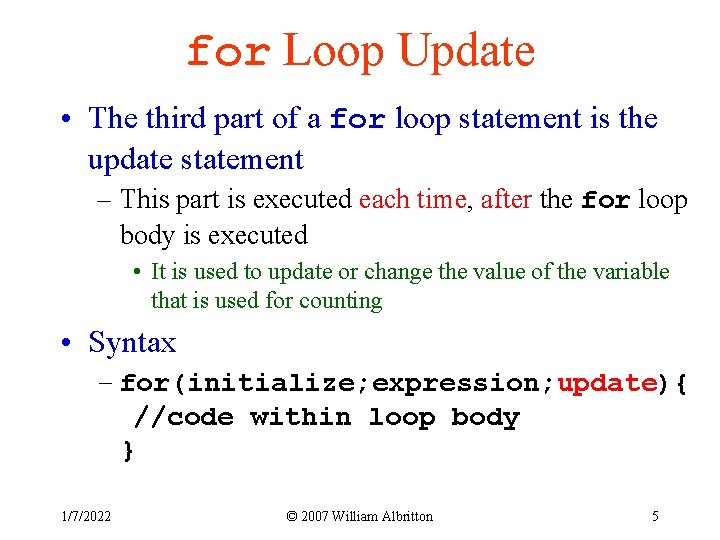
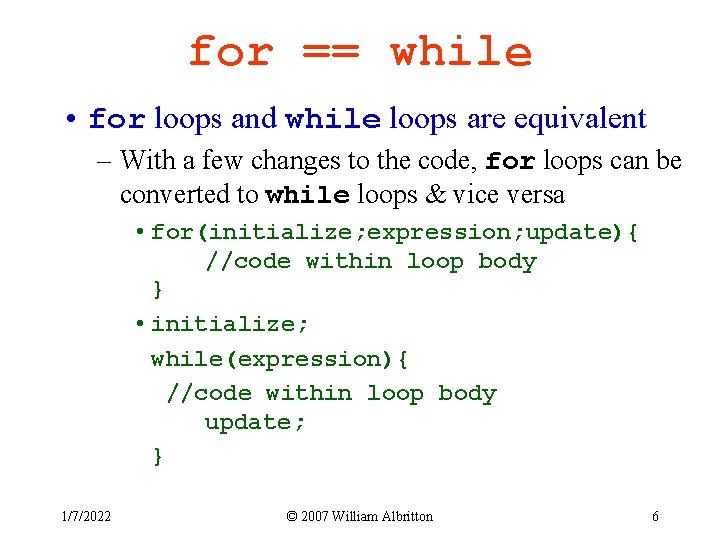
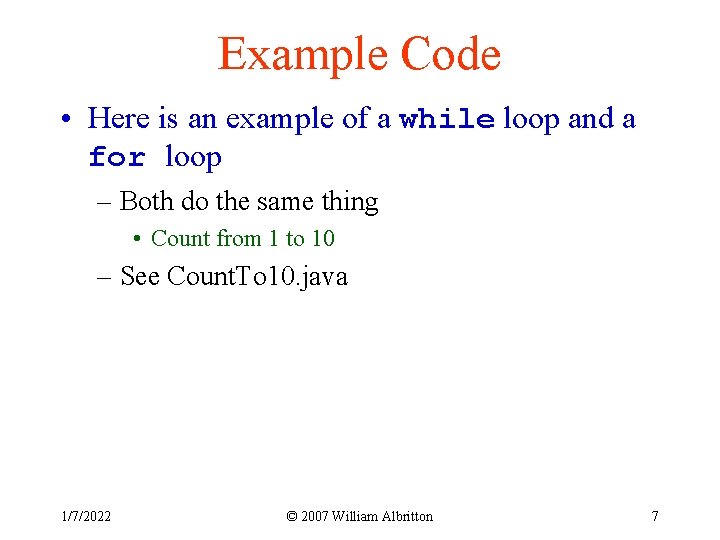
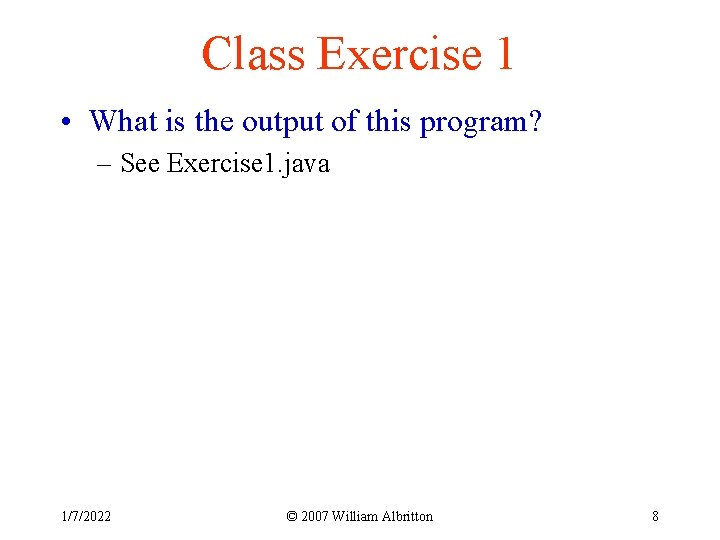
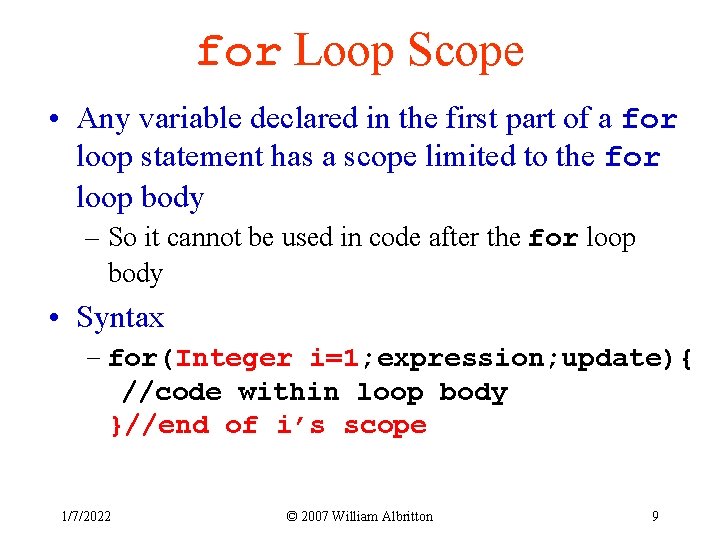
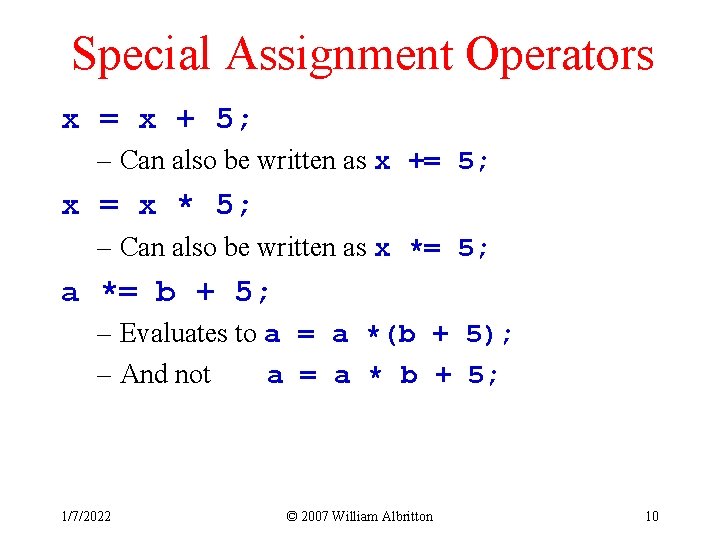
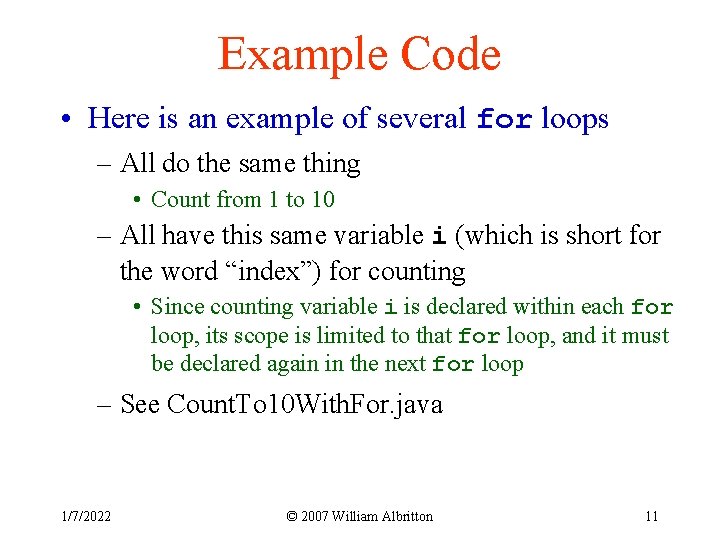
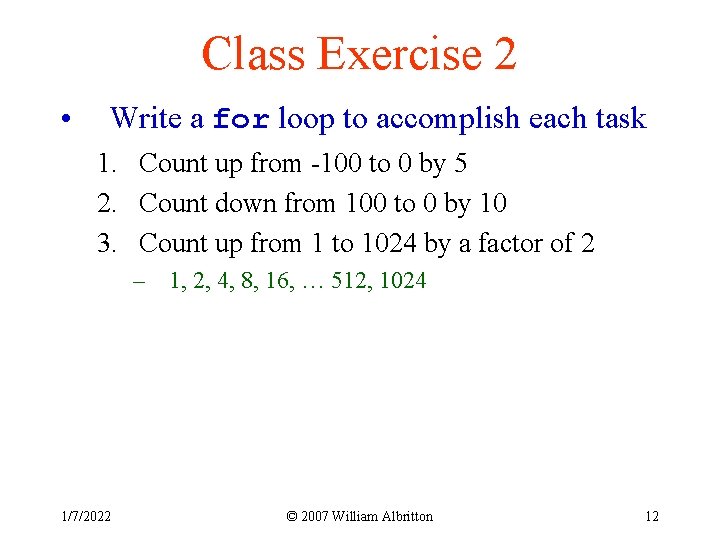
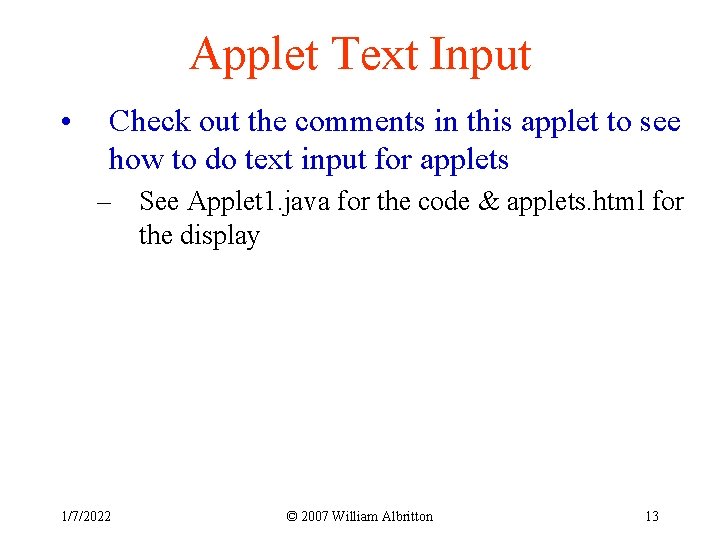
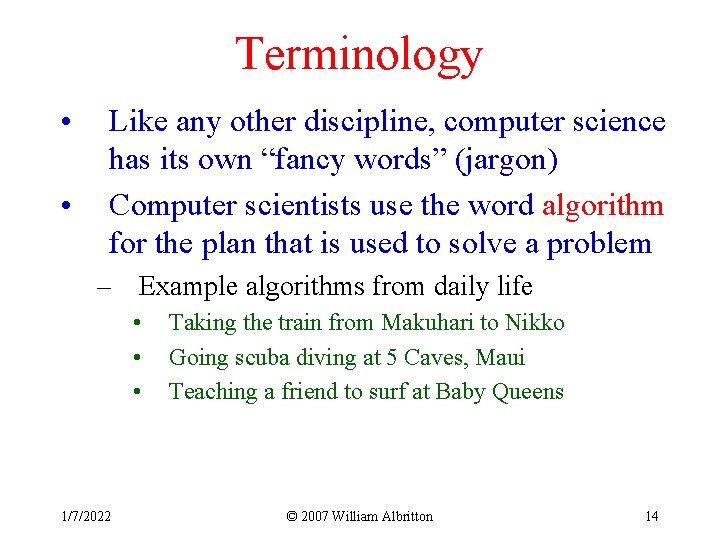
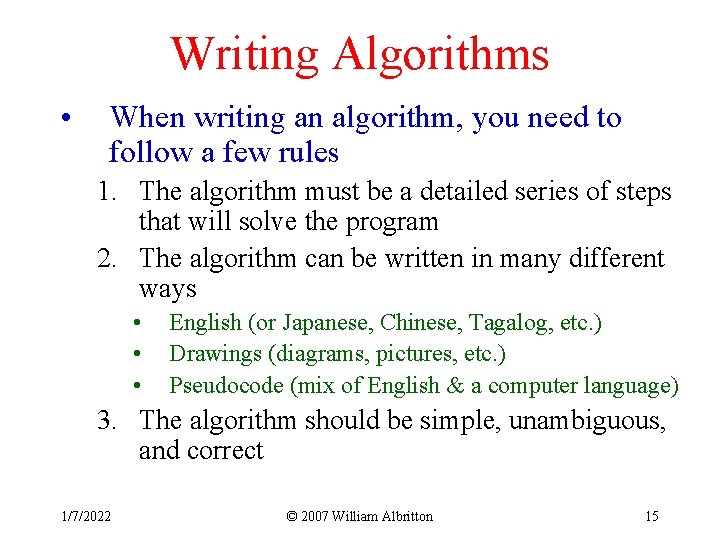
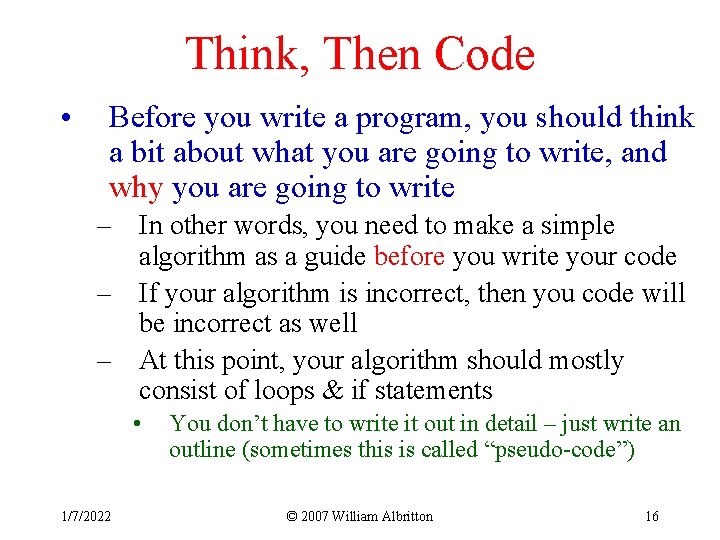
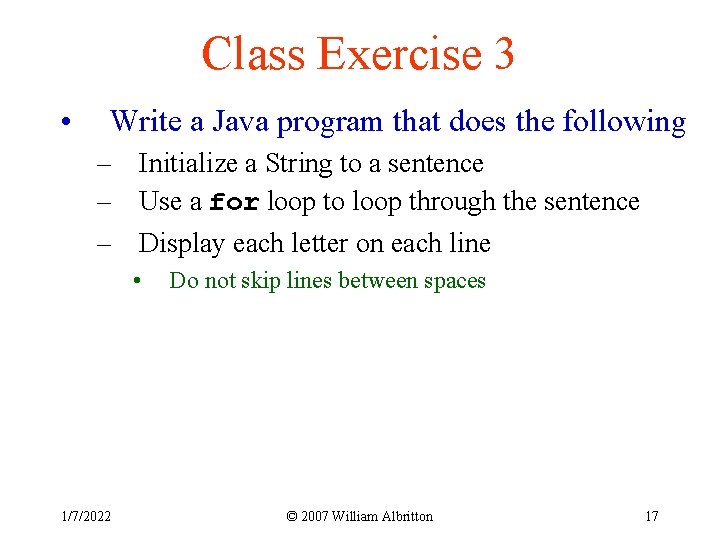
- Slides: 17
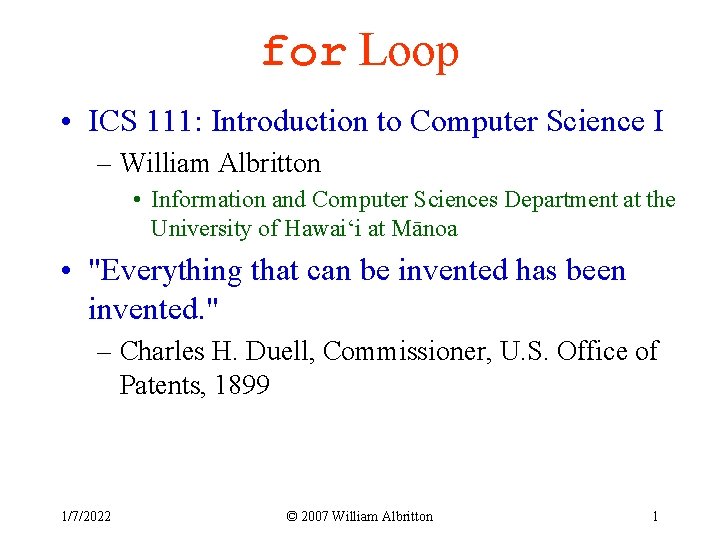
for Loop • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • "Everything that can be invented has been invented. " – Charles H. Duell, Commissioner, U. S. Office of Patents, 1899 1/7/2022 © 2007 William Albritton 1
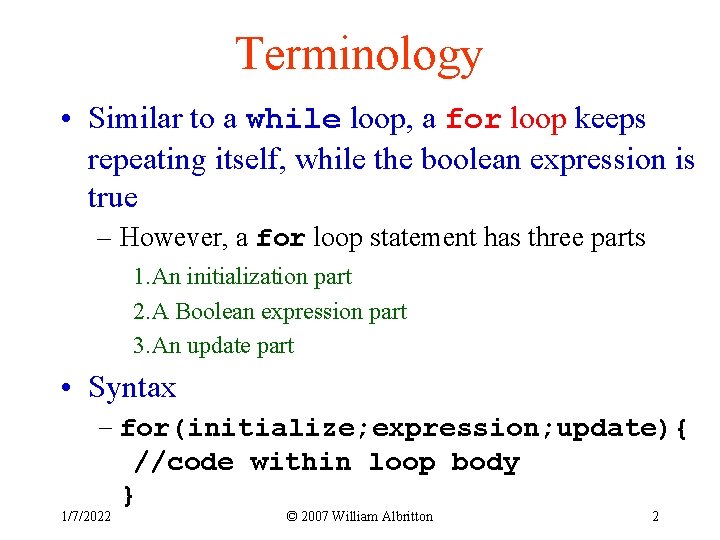
Terminology • Similar to a while loop, a for loop keeps repeating itself, while the boolean expression is true – However, a for loop statement has three parts 1. An initialization part 2. A Boolean expression part 3. An update part • Syntax – for(initialize; expression; update){ //code within loop body } 1/7/2022 © 2007 William Albritton 2
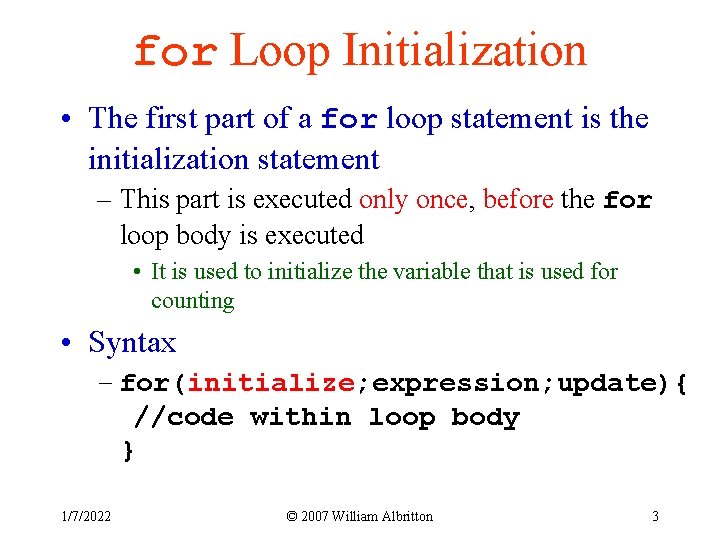
for Loop Initialization • The first part of a for loop statement is the initialization statement – This part is executed only once, before the for loop body is executed • It is used to initialize the variable that is used for counting • Syntax – for(initialize; expression; update){ //code within loop body } 1/7/2022 © 2007 William Albritton 3
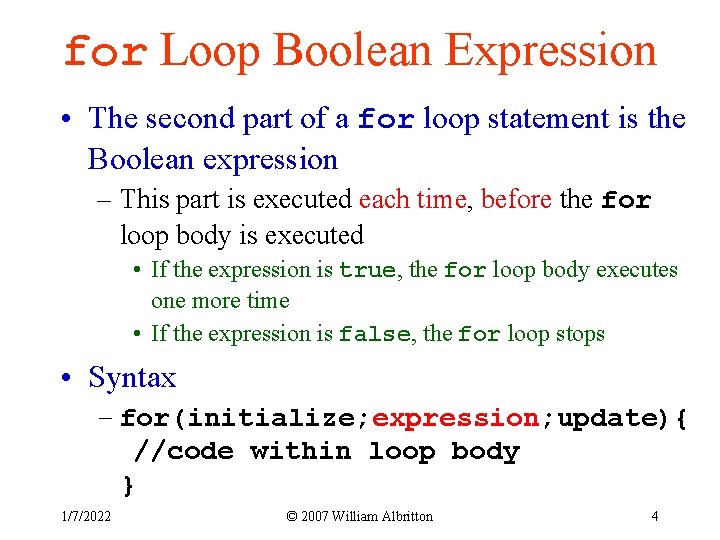
for Loop Boolean Expression • The second part of a for loop statement is the Boolean expression – This part is executed each time, before the for loop body is executed • If the expression is true, the for loop body executes one more time • If the expression is false, the for loop stops • Syntax – for(initialize; expression; update){ //code within loop body } 1/7/2022 © 2007 William Albritton 4
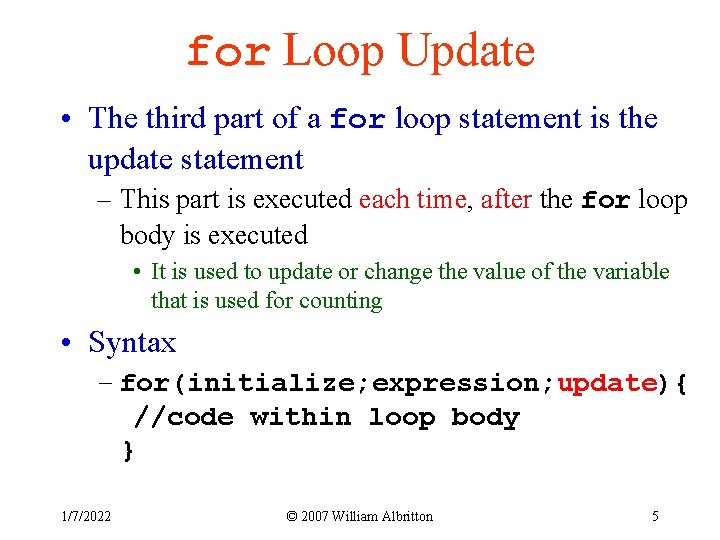
for Loop Update • The third part of a for loop statement is the update statement – This part is executed each time, after the for loop body is executed • It is used to update or change the value of the variable that is used for counting • Syntax – for(initialize; expression; update){ //code within loop body } 1/7/2022 © 2007 William Albritton 5
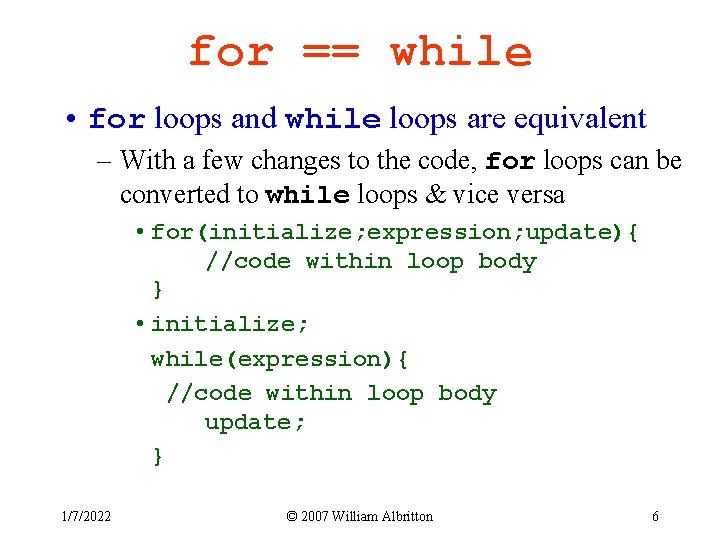
for == while • for loops and while loops are equivalent – With a few changes to the code, for loops can be converted to while loops & vice versa • for(initialize; expression; update){ //code within loop body } • initialize; while(expression){ //code within loop body update; } 1/7/2022 © 2007 William Albritton 6
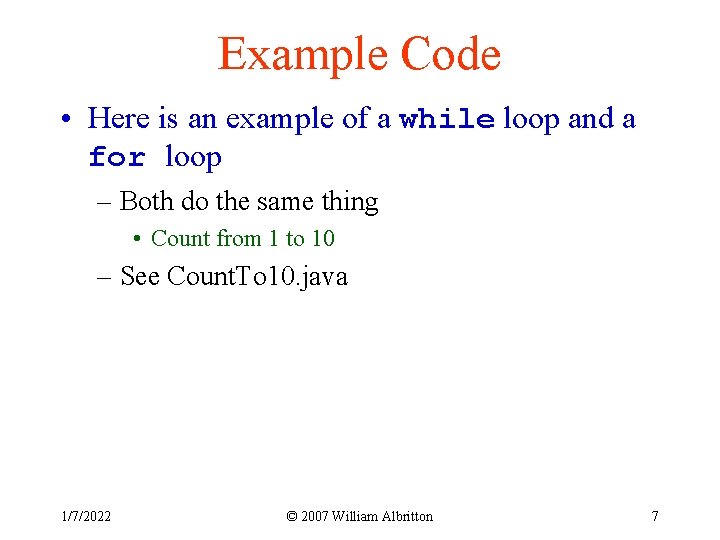
Example Code • Here is an example of a while loop and a for loop – Both do the same thing • Count from 1 to 10 – See Count. To 10. java 1/7/2022 © 2007 William Albritton 7
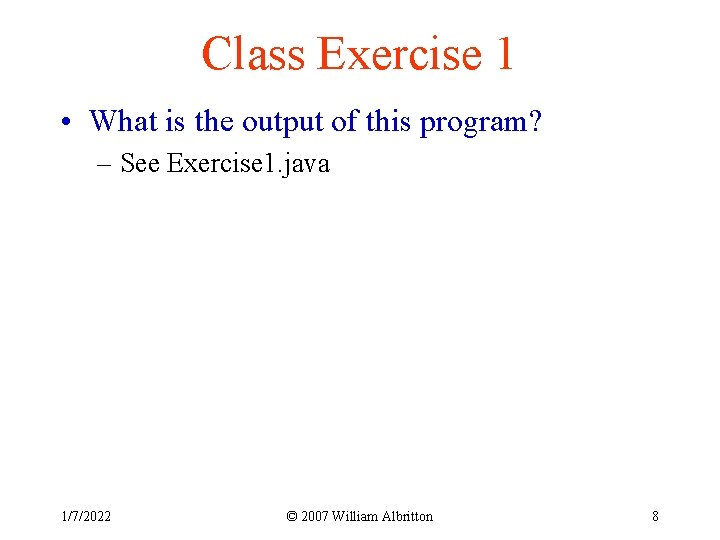
Class Exercise 1 • What is the output of this program? – See Exercise 1. java 1/7/2022 © 2007 William Albritton 8
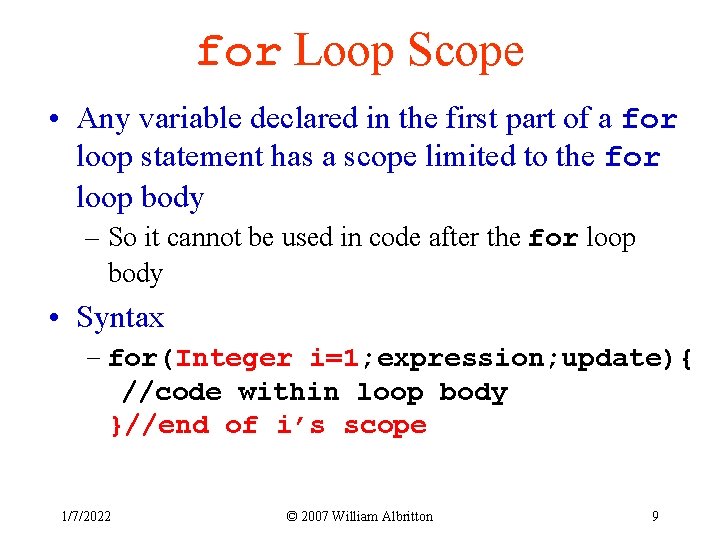
for Loop Scope • Any variable declared in the first part of a for loop statement has a scope limited to the for loop body – So it cannot be used in code after the for loop body • Syntax – for(Integer i=1; expression; update){ //code within loop body }//end of i’s scope 1/7/2022 © 2007 William Albritton 9
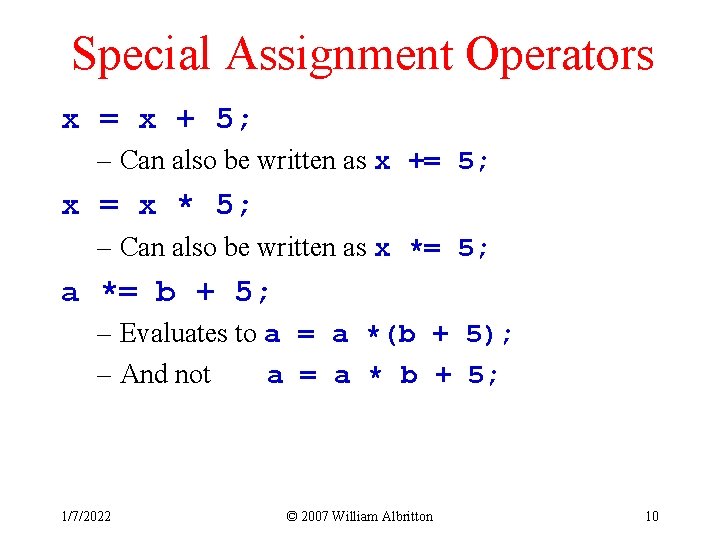
Special Assignment Operators x = x + 5; – Can also be written as x += 5; x = x * 5; – Can also be written as x *= 5; a *= b + 5; – Evaluates to a = a *(b + 5); – And not a = a * b + 5; 1/7/2022 © 2007 William Albritton 10
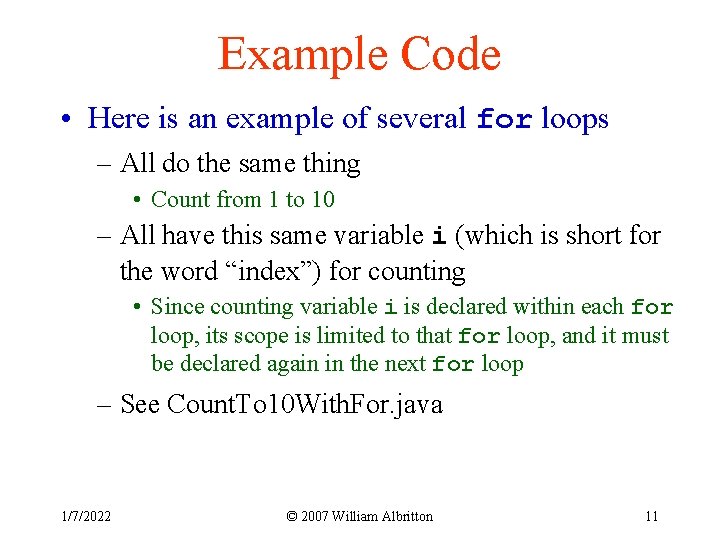
Example Code • Here is an example of several for loops – All do the same thing • Count from 1 to 10 – All have this same variable i (which is short for the word “index”) for counting • Since counting variable i is declared within each for loop, its scope is limited to that for loop, and it must be declared again in the next for loop – See Count. To 10 With. For. java 1/7/2022 © 2007 William Albritton 11
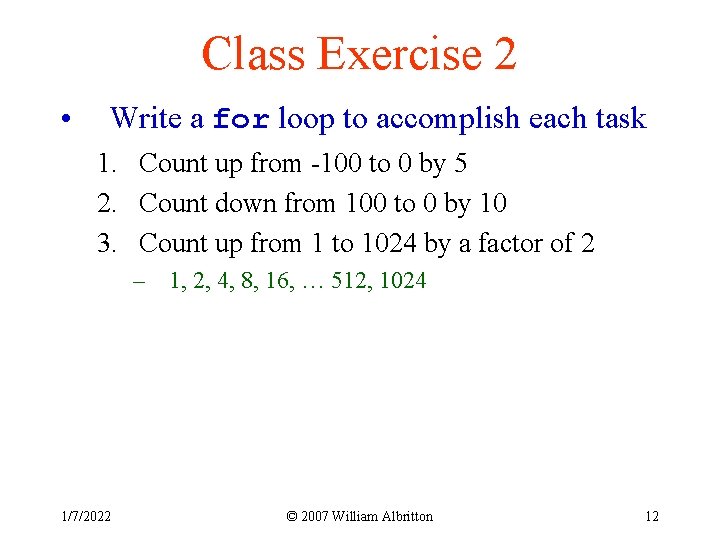
Class Exercise 2 • Write a for loop to accomplish each task 1. Count up from -100 to 0 by 5 2. Count down from 100 to 0 by 10 3. Count up from 1 to 1024 by a factor of 2 – 1, 2, 4, 8, 16, … 512, 1024 1/7/2022 © 2007 William Albritton 12
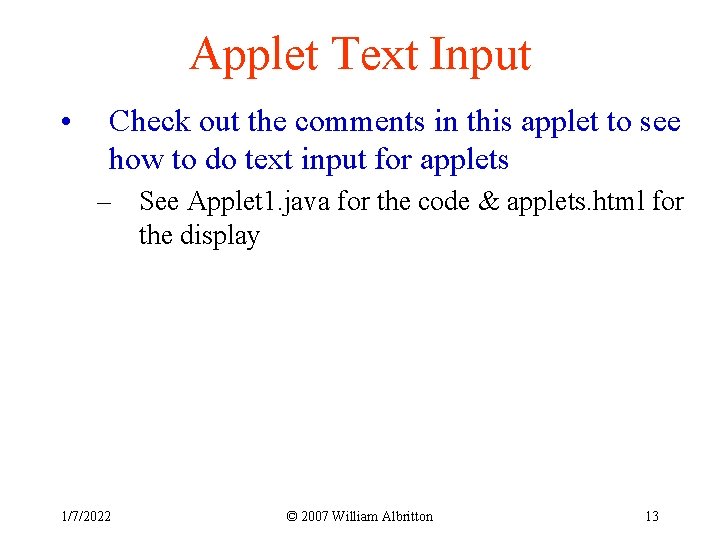
Applet Text Input • Check out the comments in this applet to see how to do text input for applets – See Applet 1. java for the code & applets. html for the display 1/7/2022 © 2007 William Albritton 13
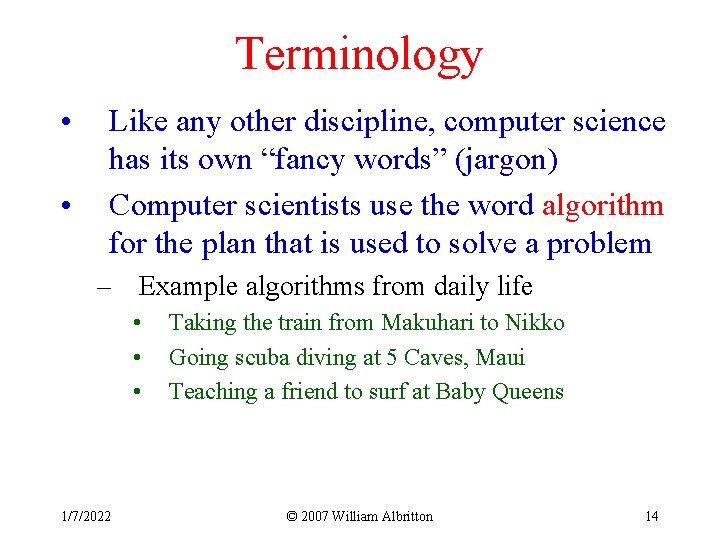
Terminology • • Like any other discipline, computer science has its own “fancy words” (jargon) Computer scientists use the word algorithm for the plan that is used to solve a problem – Example algorithms from daily life • • • 1/7/2022 Taking the train from Makuhari to Nikko Going scuba diving at 5 Caves, Maui Teaching a friend to surf at Baby Queens © 2007 William Albritton 14
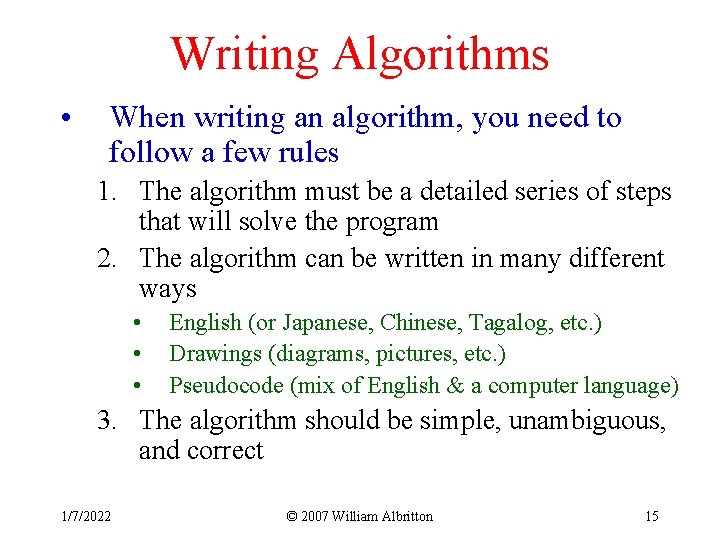
Writing Algorithms • When writing an algorithm, you need to follow a few rules 1. The algorithm must be a detailed series of steps that will solve the program 2. The algorithm can be written in many different ways • • • English (or Japanese, Chinese, Tagalog, etc. ) Drawings (diagrams, pictures, etc. ) Pseudocode (mix of English & a computer language) 3. The algorithm should be simple, unambiguous, and correct 1/7/2022 © 2007 William Albritton 15
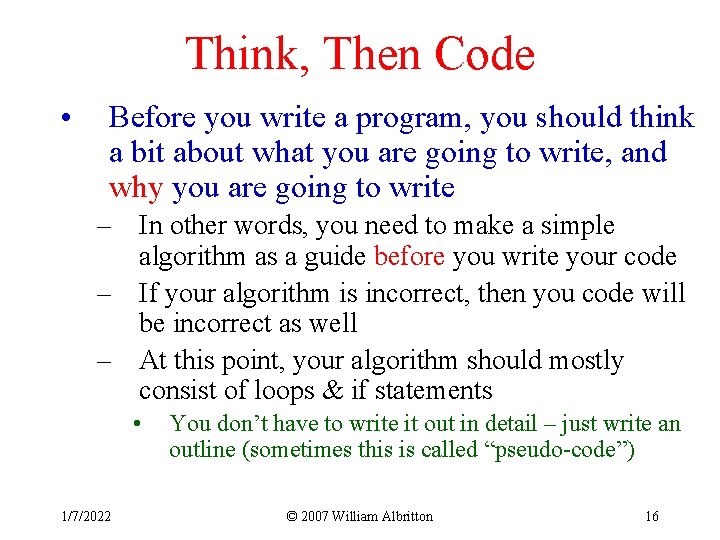
Think, Then Code • Before you write a program, you should think a bit about what you are going to write, and why you are going to write – In other words, you need to make a simple algorithm as a guide before you write your code – If your algorithm is incorrect, then you code will be incorrect as well – At this point, your algorithm should mostly consist of loops & if statements • 1/7/2022 You don’t have to write it out in detail – just write an outline (sometimes this is called “pseudo-code”) © 2007 William Albritton 16
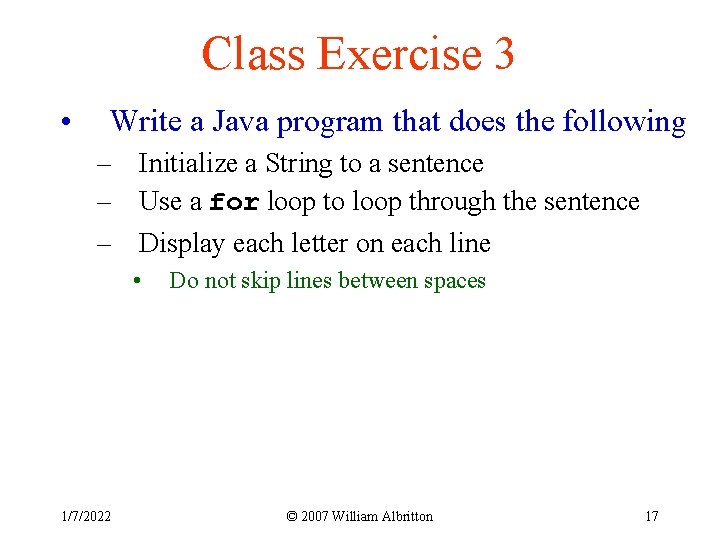
Class Exercise 3 • Write a Java program that does the following – Initialize a String to a sentence – Use a for loop to loop through the sentence – Display each letter on each line • 1/7/2022 Do not skip lines between spaces © 2007 William Albritton 17
100 011
Ics 111
Ics 33 midterm
Ics 111
Advanced incident reporting system
What kind of science
It 111 introduction to computing
Kompor lpg termasuk open loop atau close loop
Fifth gear loop the loop
Open loop vs closed loop in cars
Manakah yang lebih baik open loop atau close loop system
Do while loop adalah
L
Multi loop pid controller regolatore pid multi loop
Wwwk-6.thinkcentral
Introduction to computer science midterm exam
Introduction to computer science midterm exam test
C++ code