Exceptions ICS 111 Introduction to Computer Science I
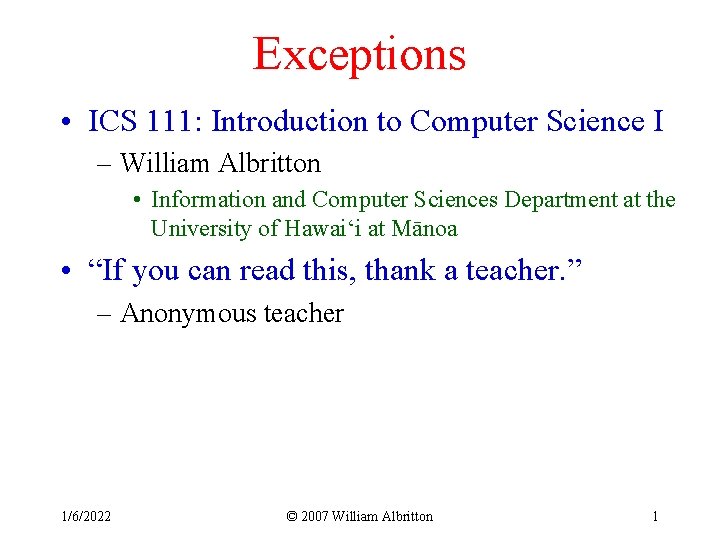
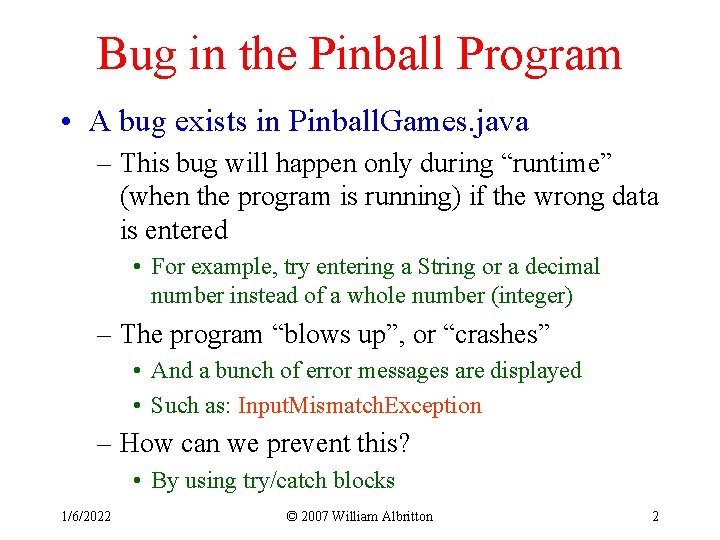
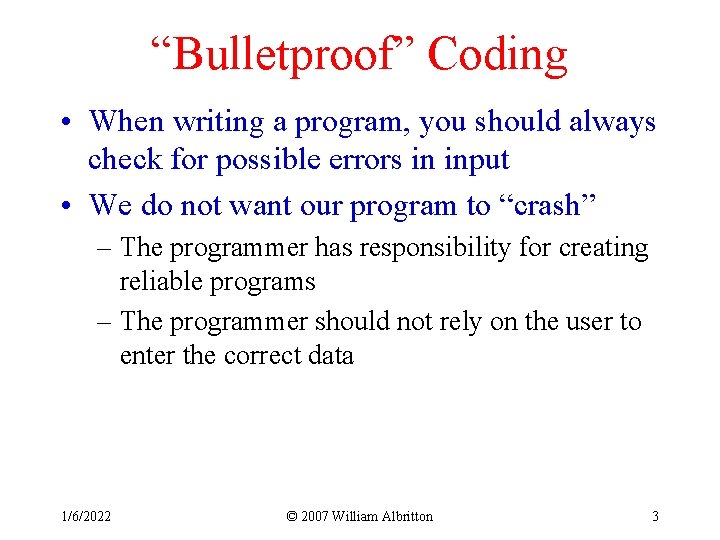
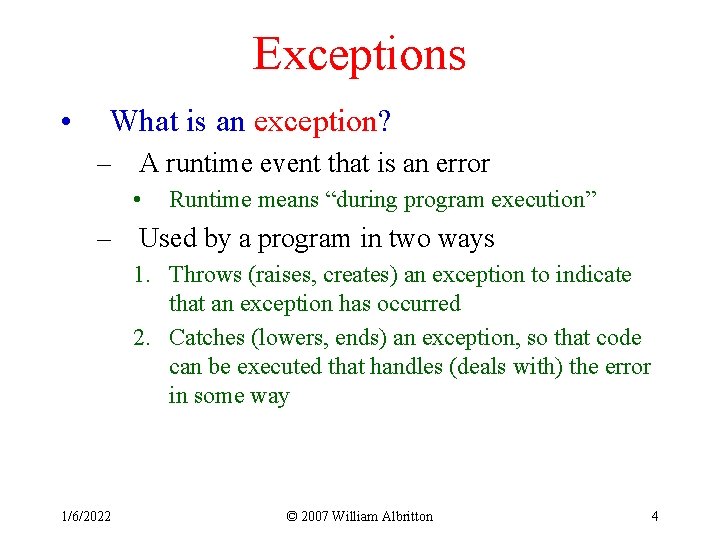
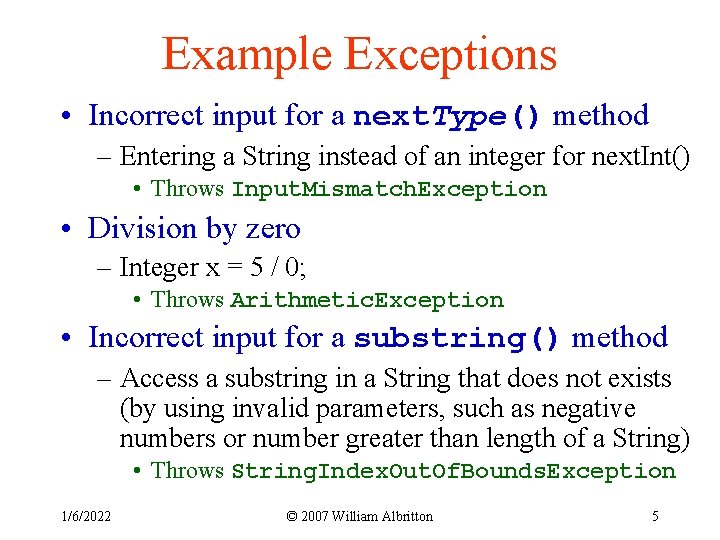
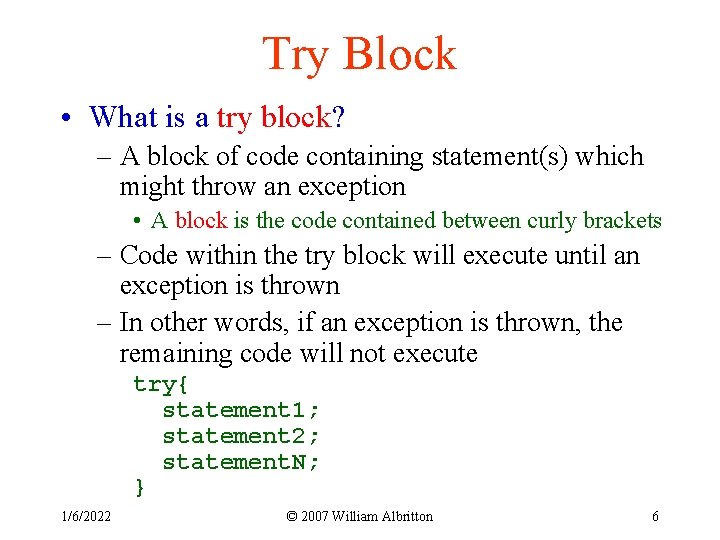
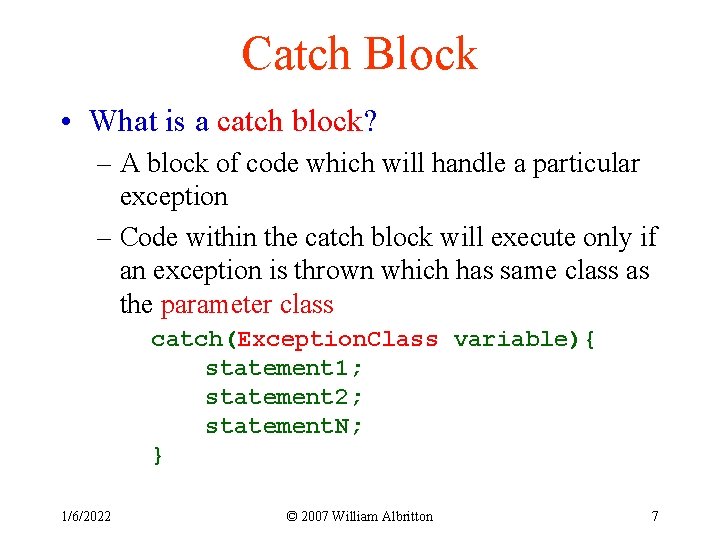
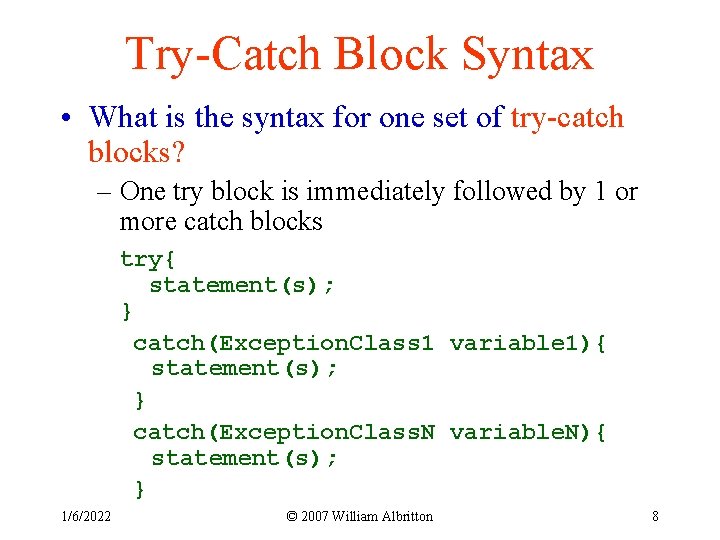
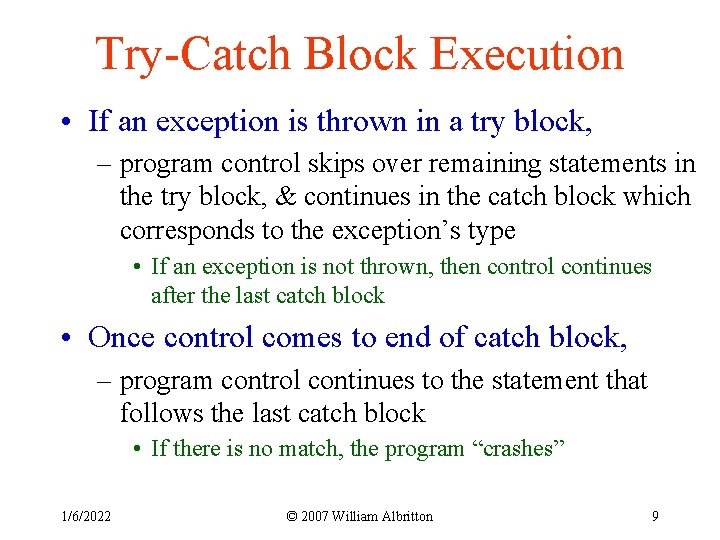
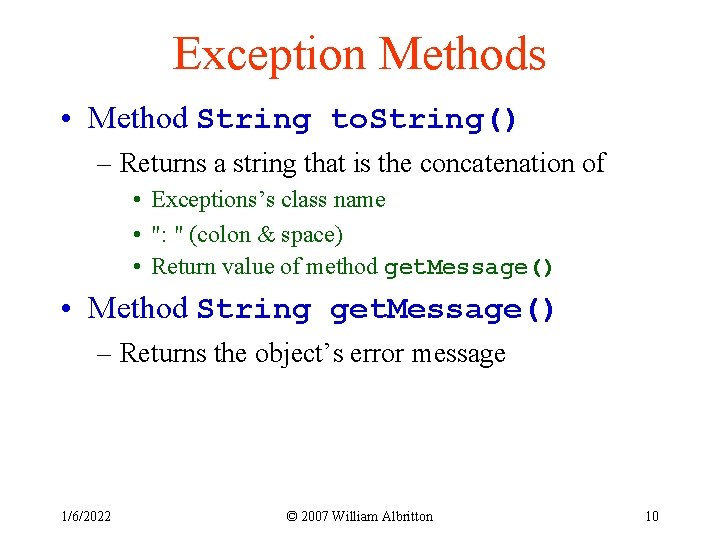
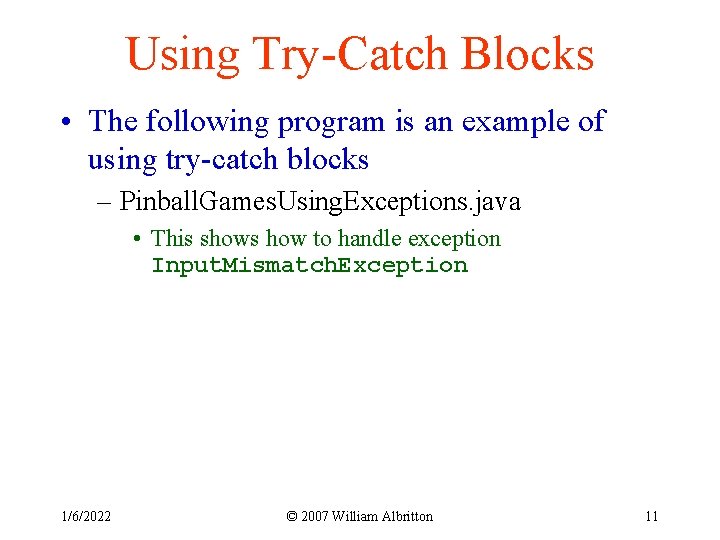
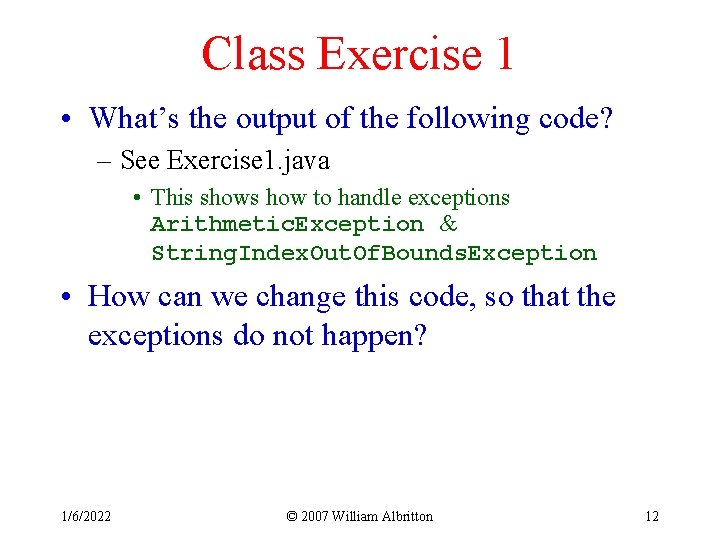
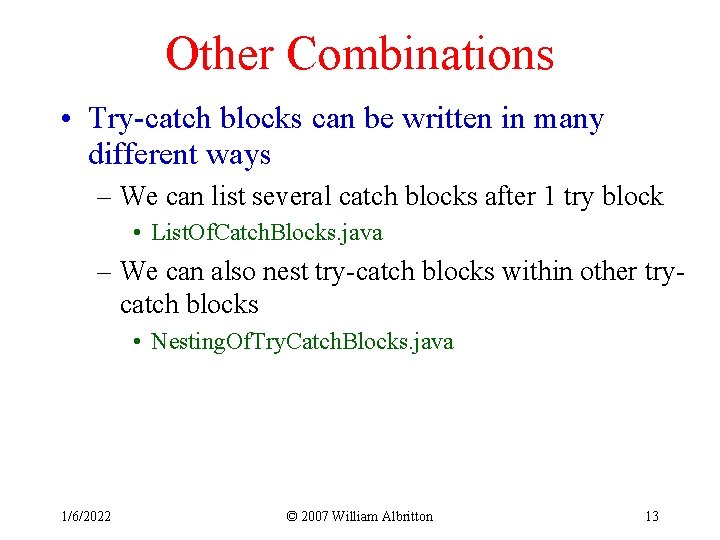
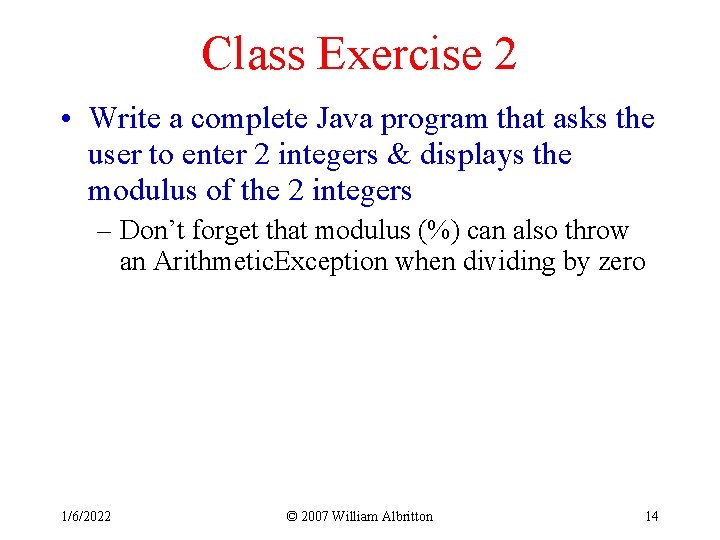
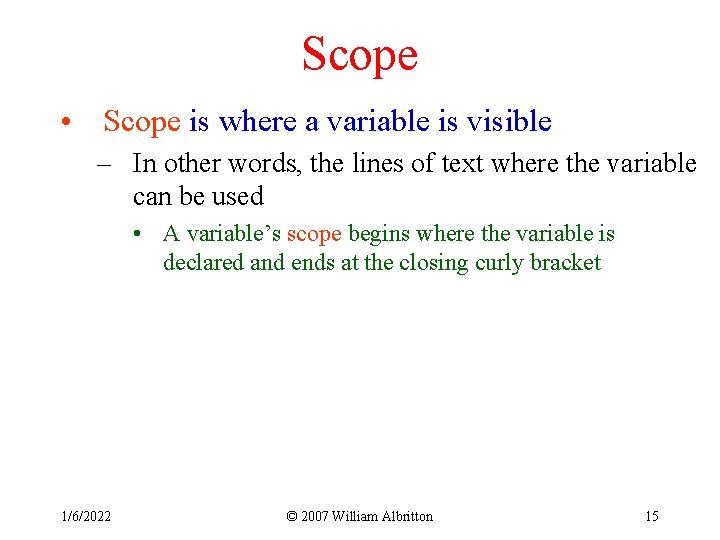
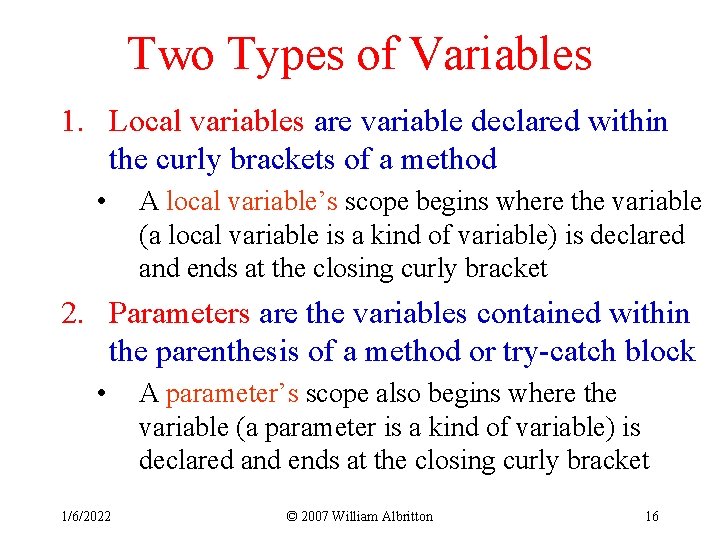
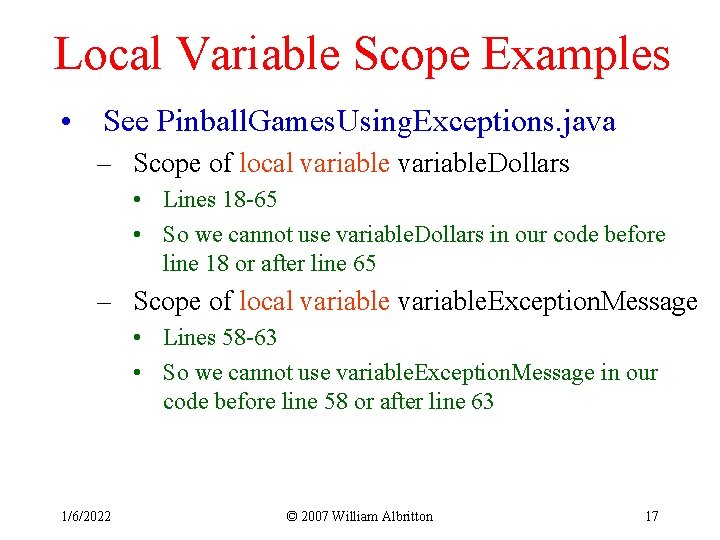
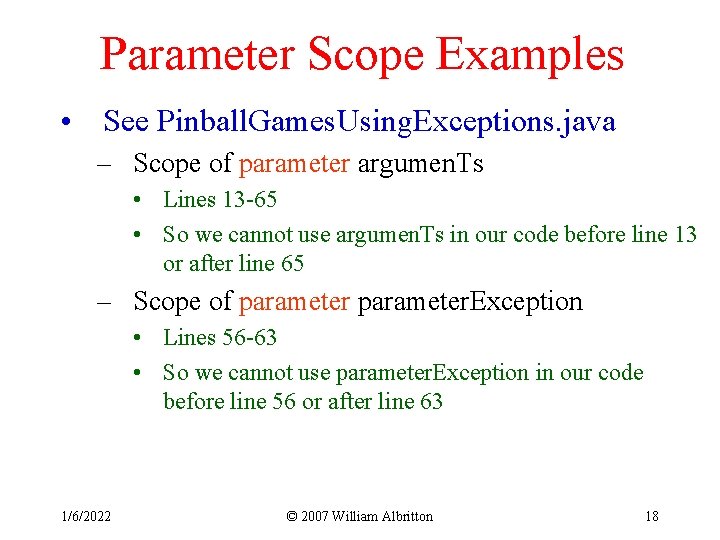
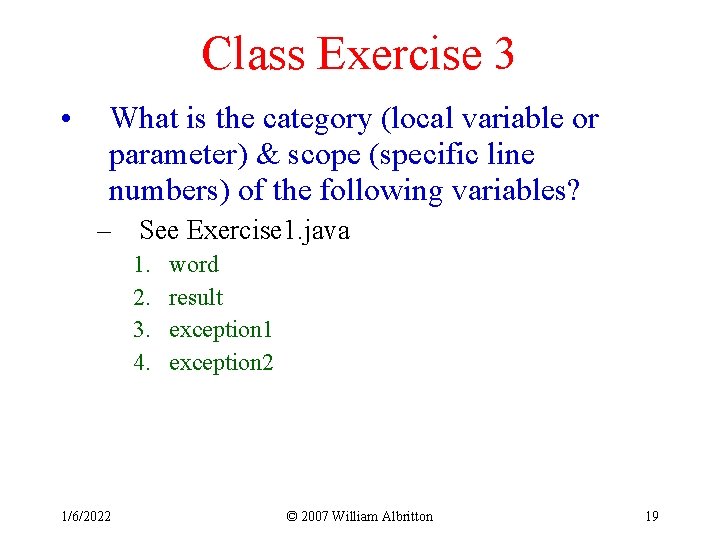
- Slides: 19
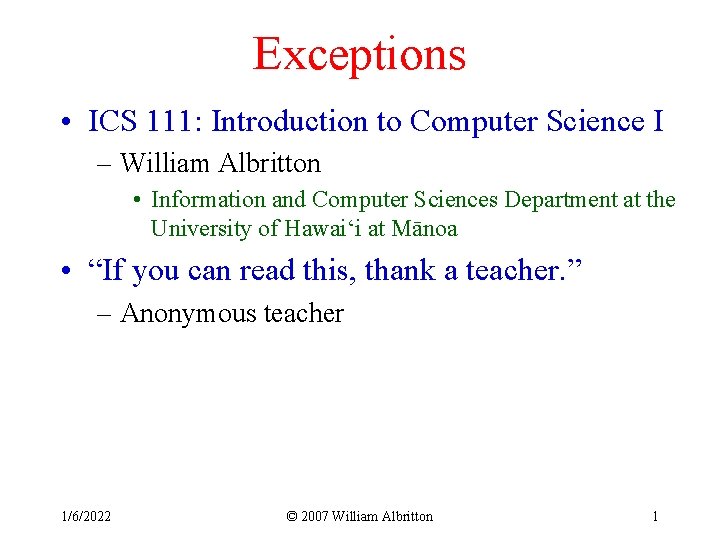
Exceptions • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • “If you can read this, thank a teacher. ” – Anonymous teacher 1/6/2022 © 2007 William Albritton 1
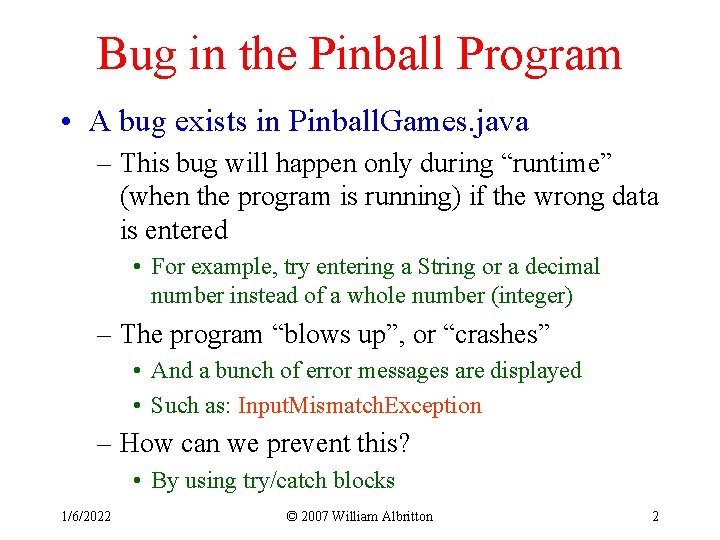
Bug in the Pinball Program • A bug exists in Pinball. Games. java – This bug will happen only during “runtime” (when the program is running) if the wrong data is entered • For example, try entering a String or a decimal number instead of a whole number (integer) – The program “blows up”, or “crashes” • And a bunch of error messages are displayed • Such as: Input. Mismatch. Exception – How can we prevent this? • By using try/catch blocks 1/6/2022 © 2007 William Albritton 2
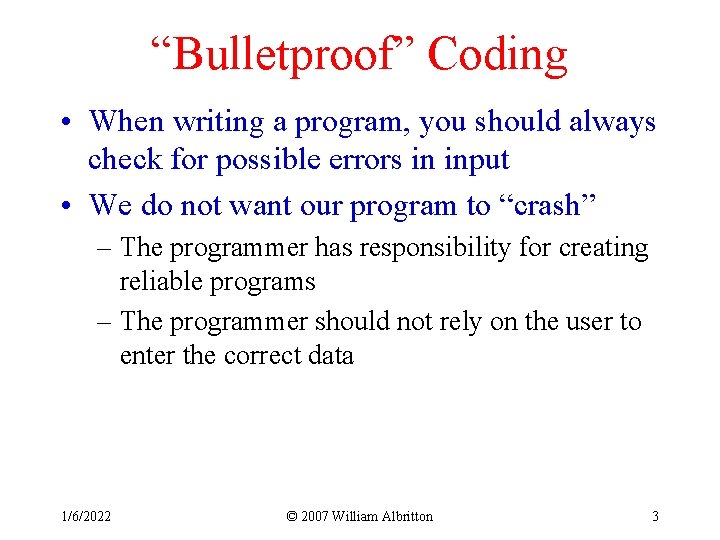
“Bulletproof” Coding • When writing a program, you should always check for possible errors in input • We do not want our program to “crash” – The programmer has responsibility for creating reliable programs – The programmer should not rely on the user to enter the correct data 1/6/2022 © 2007 William Albritton 3
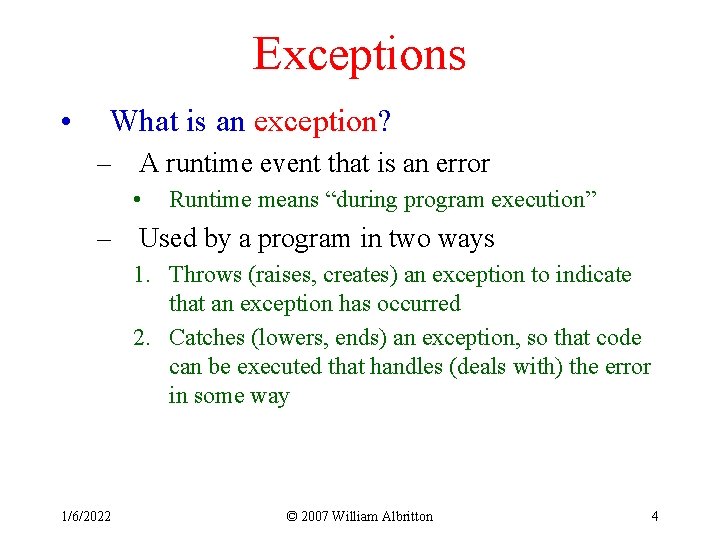
Exceptions • What is an exception? – A runtime event that is an error • Runtime means “during program execution” – Used by a program in two ways 1. Throws (raises, creates) an exception to indicate that an exception has occurred 2. Catches (lowers, ends) an exception, so that code can be executed that handles (deals with) the error in some way 1/6/2022 © 2007 William Albritton 4
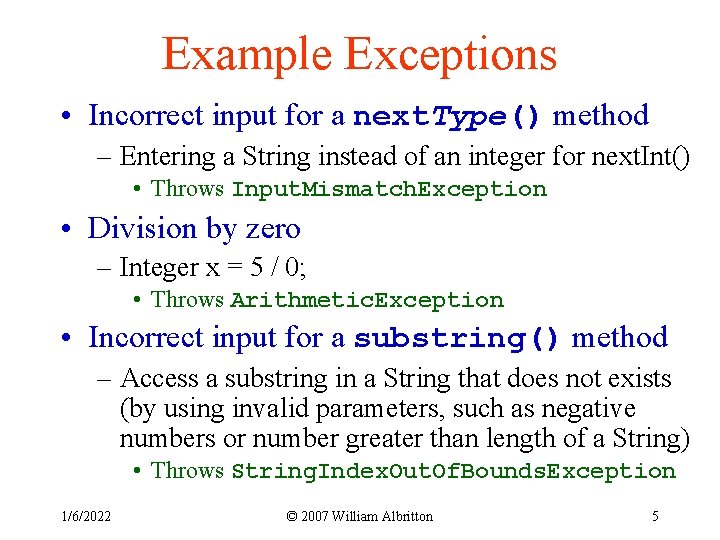
Example Exceptions • Incorrect input for a next. Type() method – Entering a String instead of an integer for next. Int() • Throws Input. Mismatch. Exception • Division by zero – Integer x = 5 / 0; • Throws Arithmetic. Exception • Incorrect input for a substring() method – Access a substring in a String that does not exists (by using invalid parameters, such as negative numbers or number greater than length of a String) • Throws String. Index. Out. Of. Bounds. Exception 1/6/2022 © 2007 William Albritton 5
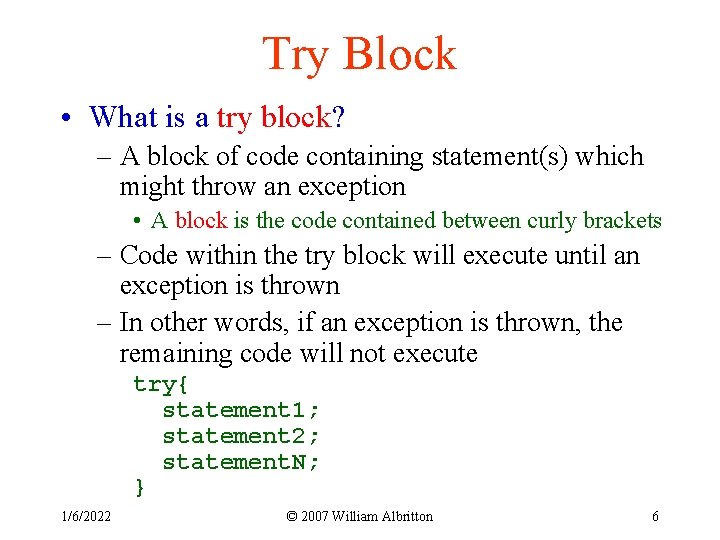
Try Block • What is a try block? – A block of code containing statement(s) which might throw an exception • A block is the code contained between curly brackets – Code within the try block will execute until an exception is thrown – In other words, if an exception is thrown, the remaining code will not execute try{ statement 1; statement 2; statement. N; } 1/6/2022 © 2007 William Albritton 6
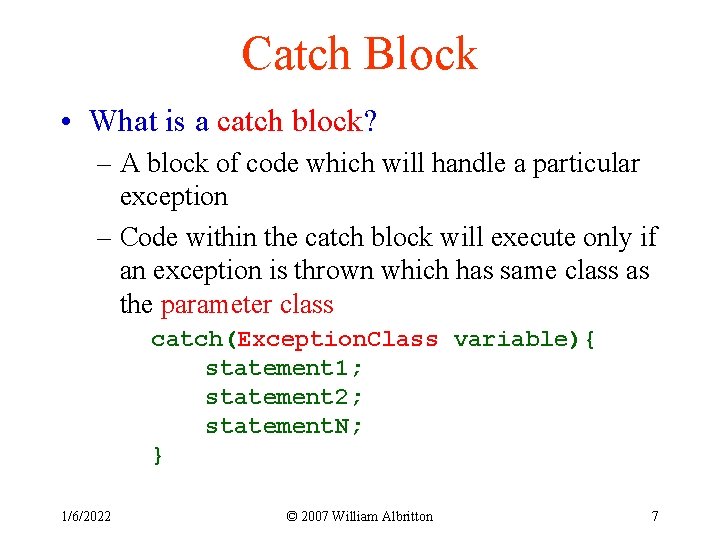
Catch Block • What is a catch block? – A block of code which will handle a particular exception – Code within the catch block will execute only if an exception is thrown which has same class as the parameter class catch(Exception. Class variable){ statement 1; statement 2; statement. N; } 1/6/2022 © 2007 William Albritton 7
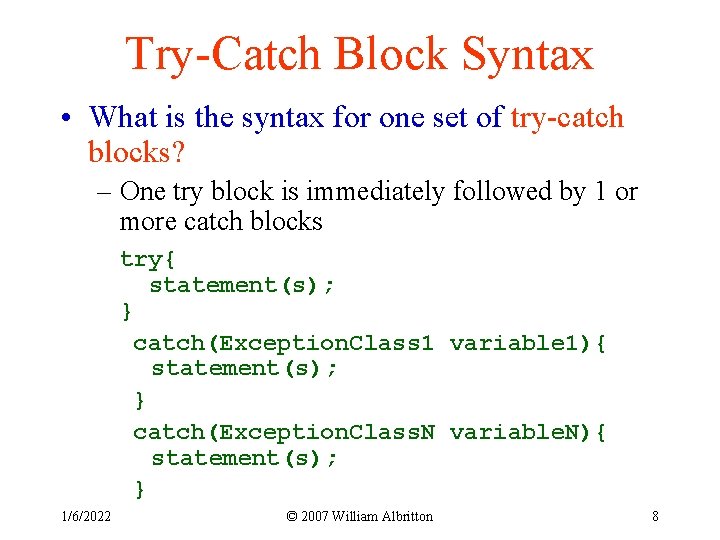
Try-Catch Block Syntax • What is the syntax for one set of try-catch blocks? – One try block is immediately followed by 1 or more catch blocks try{ statement(s); } catch(Exception. Class 1 variable 1){ statement(s); } catch(Exception. Class. N variable. N){ statement(s); } 1/6/2022 © 2007 William Albritton 8
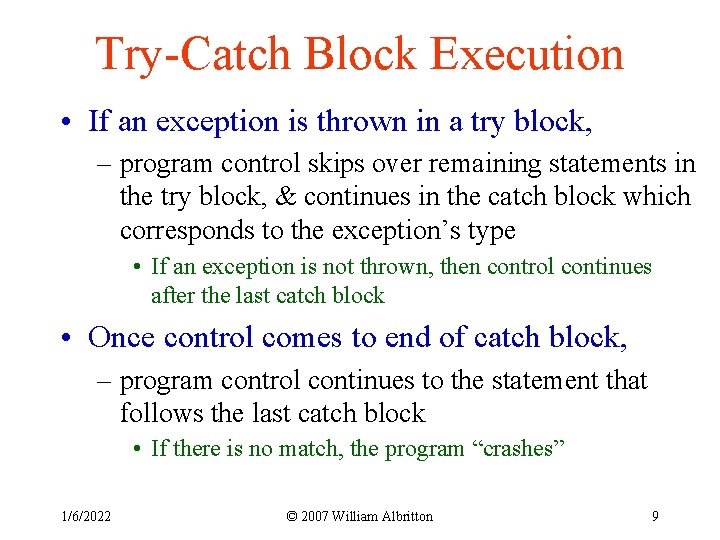
Try-Catch Block Execution • If an exception is thrown in a try block, – program control skips over remaining statements in the try block, & continues in the catch block which corresponds to the exception’s type • If an exception is not thrown, then control continues after the last catch block • Once control comes to end of catch block, – program control continues to the statement that follows the last catch block • If there is no match, the program “crashes” 1/6/2022 © 2007 William Albritton 9
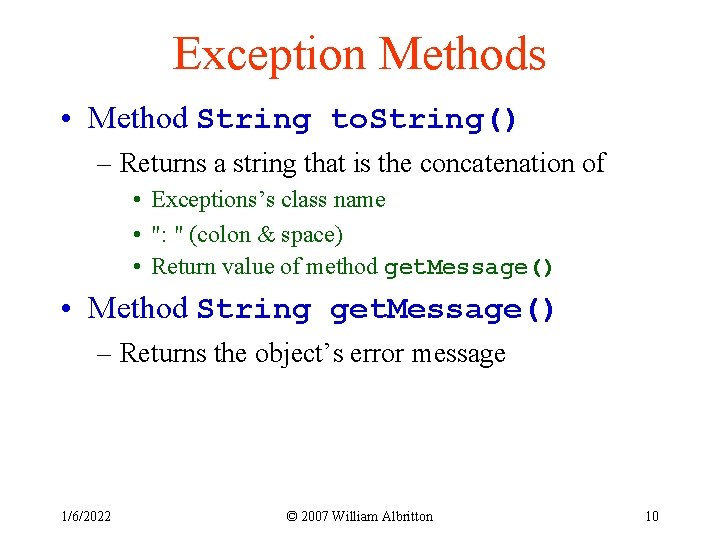
Exception Methods • Method String to. String() – Returns a string that is the concatenation of • Exceptions’s class name • ": " (colon & space) • Return value of method get. Message() • Method String get. Message() – Returns the object’s error message 1/6/2022 © 2007 William Albritton 10
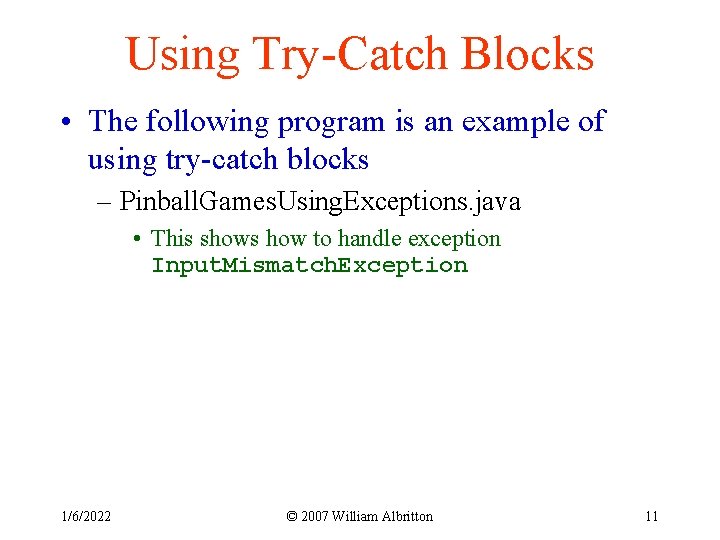
Using Try-Catch Blocks • The following program is an example of using try-catch blocks – Pinball. Games. Using. Exceptions. java • This shows how to handle exception Input. Mismatch. Exception 1/6/2022 © 2007 William Albritton 11
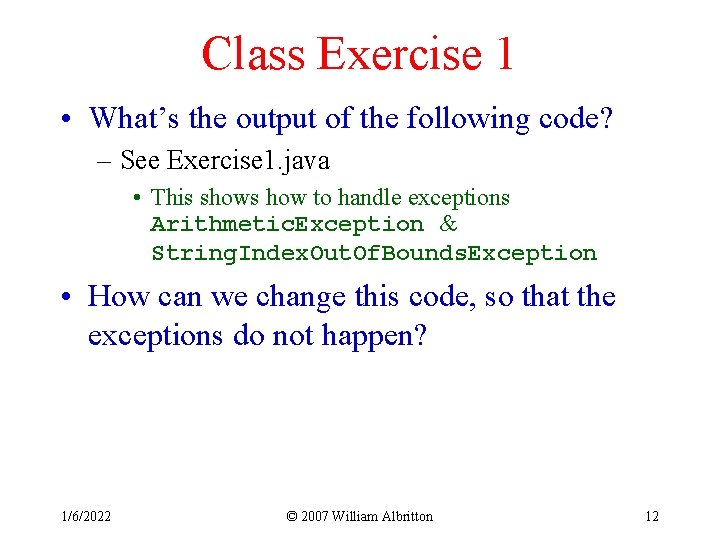
Class Exercise 1 • What’s the output of the following code? – See Exercise 1. java • This shows how to handle exceptions Arithmetic. Exception & String. Index. Out. Of. Bounds. Exception • How can we change this code, so that the exceptions do not happen? 1/6/2022 © 2007 William Albritton 12
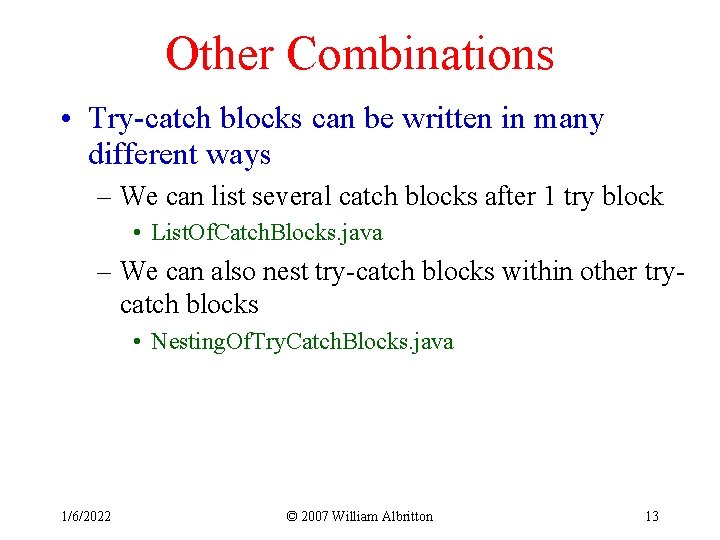
Other Combinations • Try-catch blocks can be written in many different ways – We can list several catch blocks after 1 try block • List. Of. Catch. Blocks. java – We can also nest try-catch blocks within other trycatch blocks • Nesting. Of. Try. Catch. Blocks. java 1/6/2022 © 2007 William Albritton 13
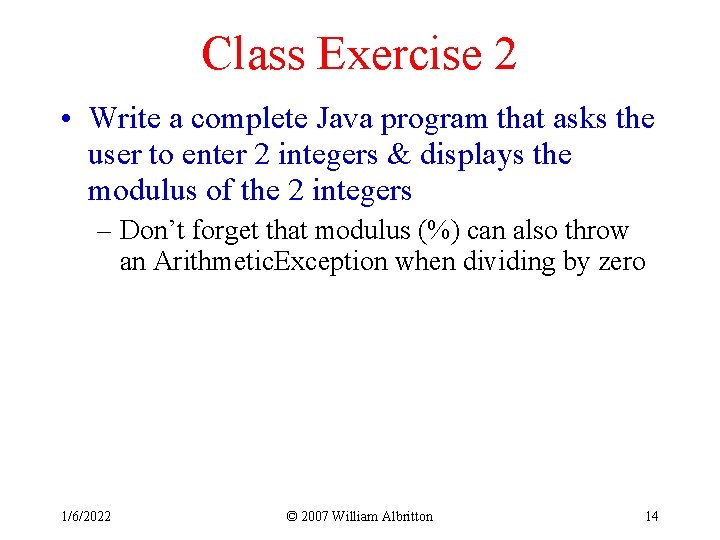
Class Exercise 2 • Write a complete Java program that asks the user to enter 2 integers & displays the modulus of the 2 integers – Don’t forget that modulus (%) can also throw an Arithmetic. Exception when dividing by zero 1/6/2022 © 2007 William Albritton 14
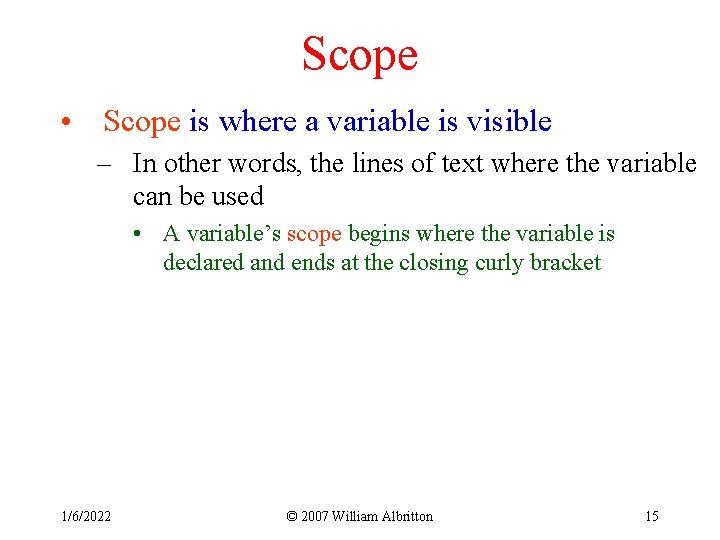
Scope • Scope is where a variable is visible – In other words, the lines of text where the variable can be used • A variable’s scope begins where the variable is declared and ends at the closing curly bracket 1/6/2022 © 2007 William Albritton 15
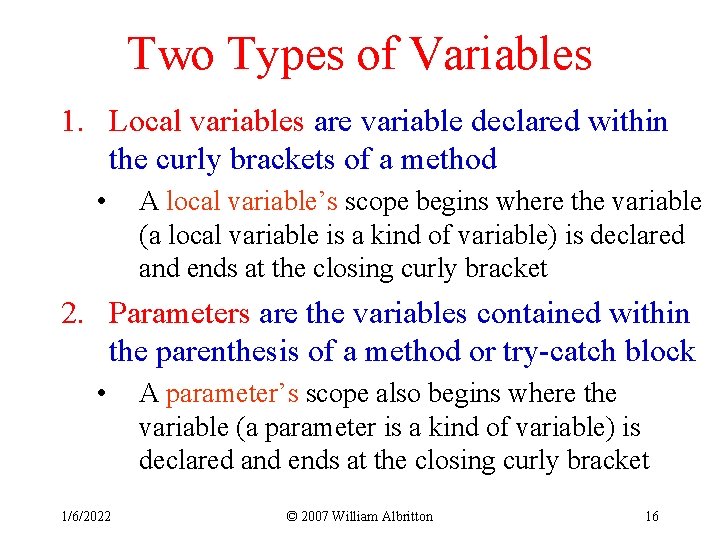
Two Types of Variables 1. Local variables are variable declared within the curly brackets of a method • A local variable’s scope begins where the variable (a local variable is a kind of variable) is declared and ends at the closing curly bracket 2. Parameters are the variables contained within the parenthesis of a method or try-catch block • 1/6/2022 A parameter’s scope also begins where the variable (a parameter is a kind of variable) is declared and ends at the closing curly bracket © 2007 William Albritton 16
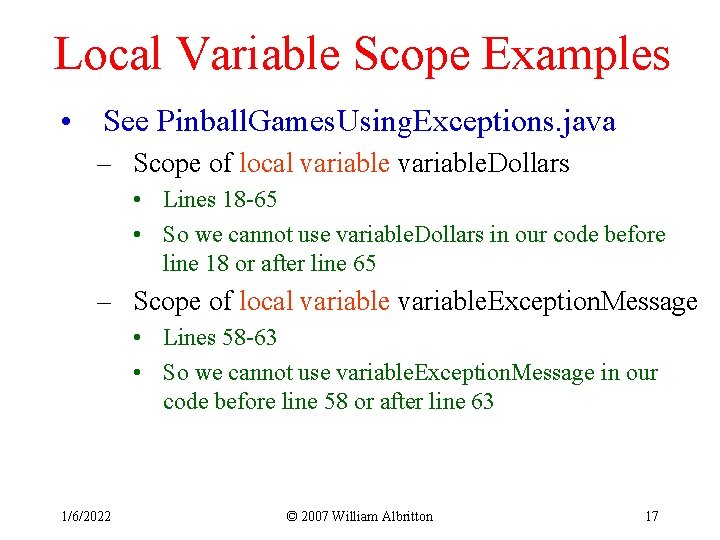
Local Variable Scope Examples • See Pinball. Games. Using. Exceptions. java – Scope of local variable. Dollars • Lines 18 -65 • So we cannot use variable. Dollars in our code before line 18 or after line 65 – Scope of local variable. Exception. Message • Lines 58 -63 • So we cannot use variable. Exception. Message in our code before line 58 or after line 63 1/6/2022 © 2007 William Albritton 17
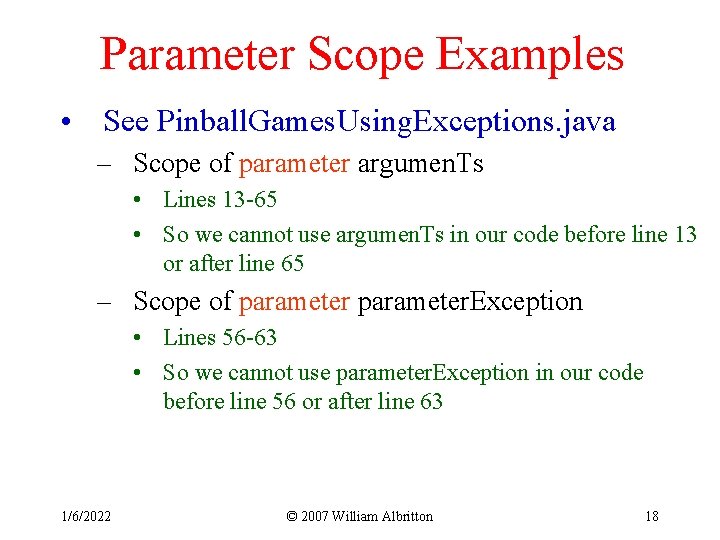
Parameter Scope Examples • See Pinball. Games. Using. Exceptions. java – Scope of parameter argumen. Ts • Lines 13 -65 • So we cannot use argumen. Ts in our code before line 13 or after line 65 – Scope of parameter. Exception • Lines 56 -63 • So we cannot use parameter. Exception in our code before line 56 or after line 63 1/6/2022 © 2007 William Albritton 18
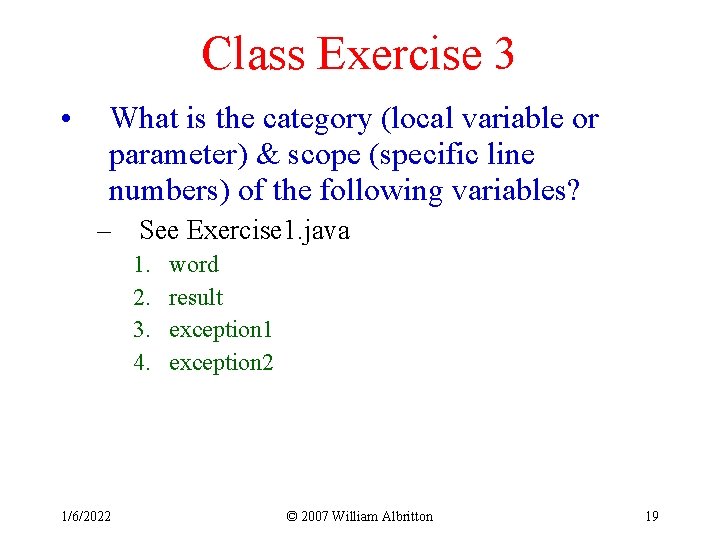
Class Exercise 3 • What is the category (local variable or parameter) & scope (specific line numbers) of the following variables? – See Exercise 1. java 1. 2. 3. 4. 1/6/2022 word result exception 1 exception 2 © 2007 William Albritton 19
110 000 110 & 111 000 111
Ics 111
Ics 111
Ics 111
Advanced incident reporting system
My favourite subject is
It 111 introduction to computing
Wwwk-6.thinkcentral
Introduction to computer science midterm exam
Introduction to computer science midterm exam test
Introduction to computer science quiz
Python programming an introduction to computer science
Spl exceptions
Robust programs
Stark law exceptions
Present continuous questions
Php exception handler
Exception of koch postulates
Koch's postulates exceptions
Pasteurized