Exceptions Why Exceptions Exceptions are used to signal
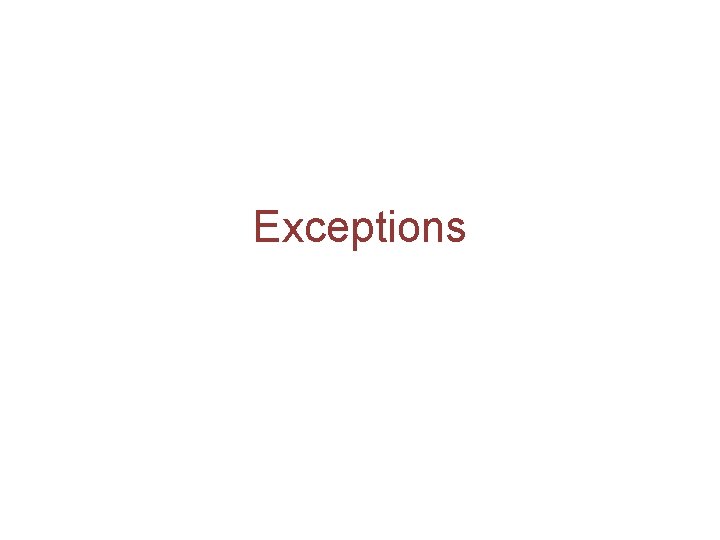
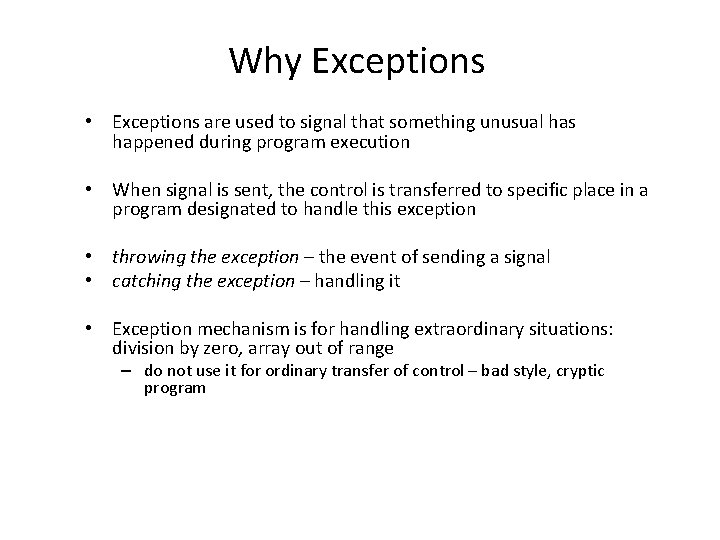
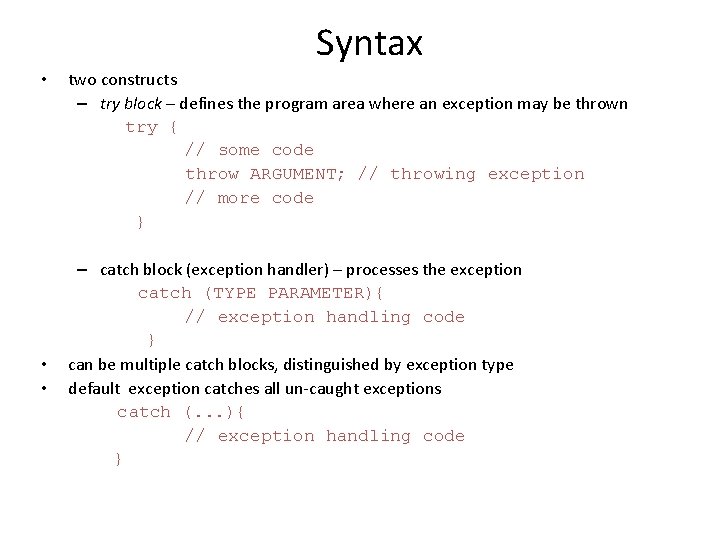
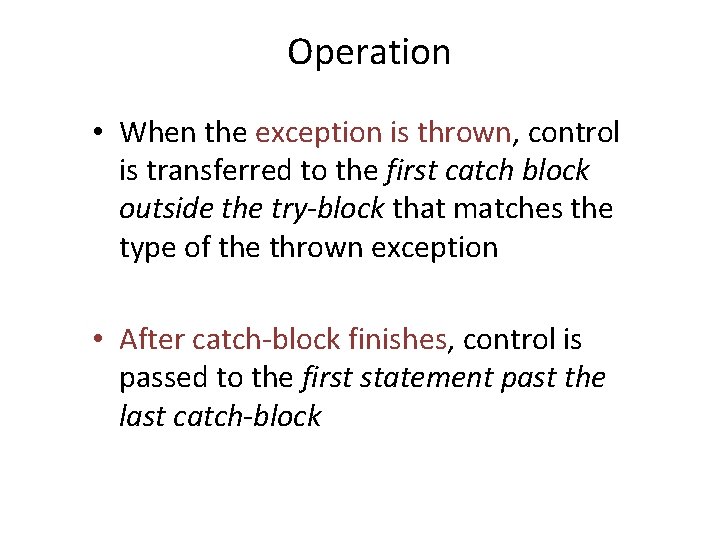
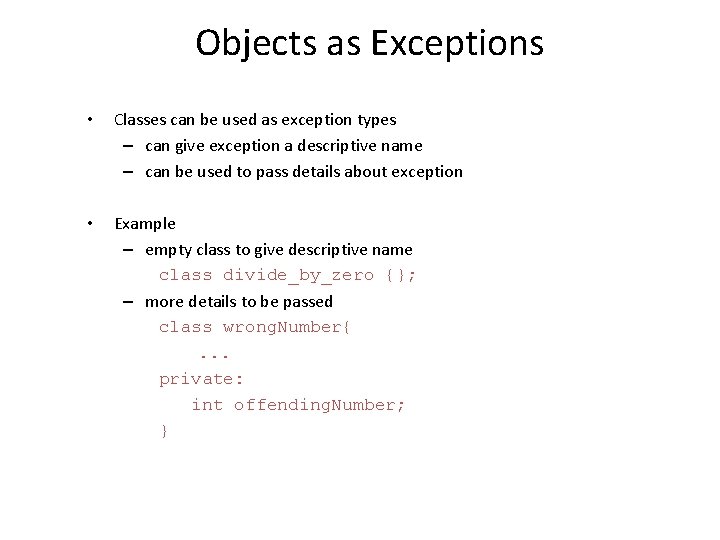
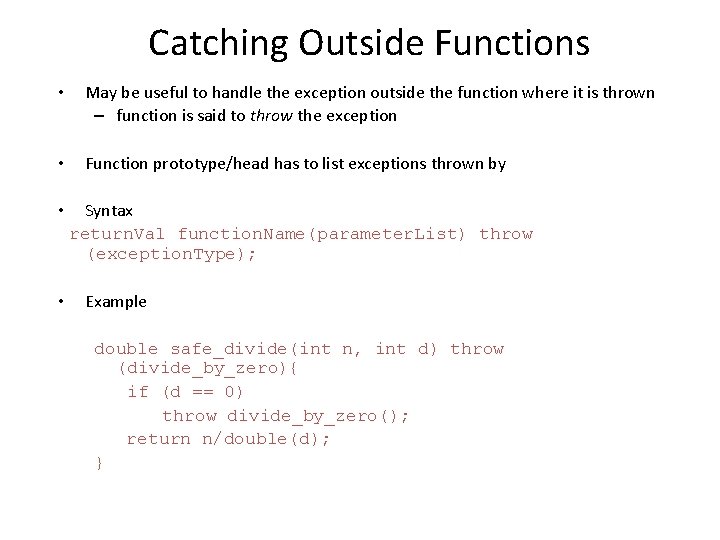
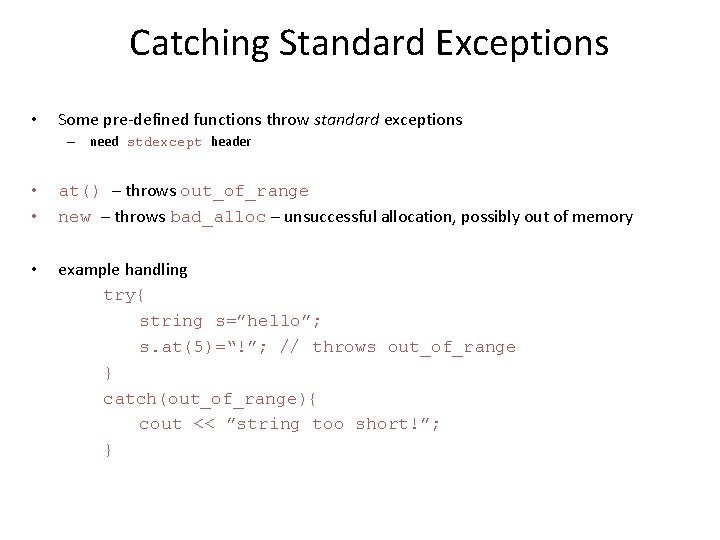
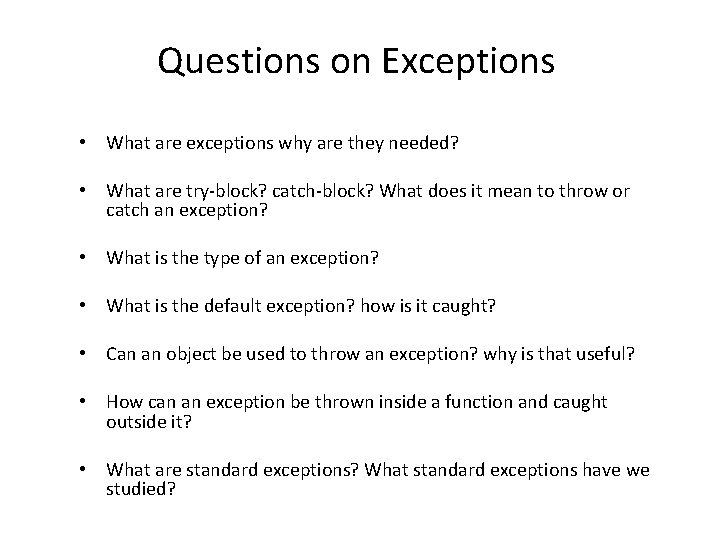
- Slides: 8
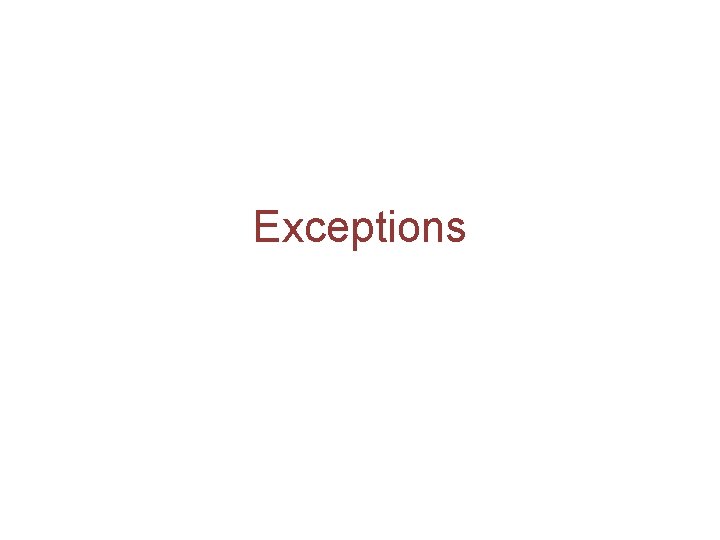
Exceptions
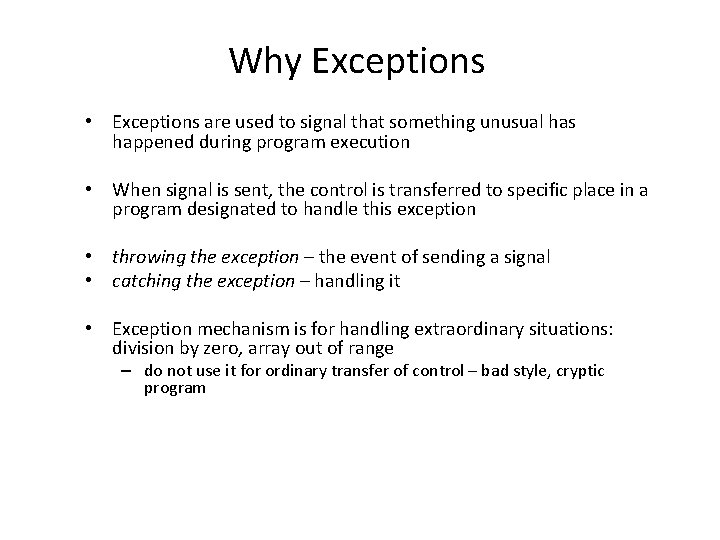
Why Exceptions • Exceptions are used to signal that something unusual has happened during program execution • When signal is sent, the control is transferred to specific place in a program designated to handle this exception • throwing the exception – the event of sending a signal • catching the exception – handling it • Exception mechanism is for handling extraordinary situations: division by zero, array out of range – do not use it for ordinary transfer of control – bad style, cryptic program
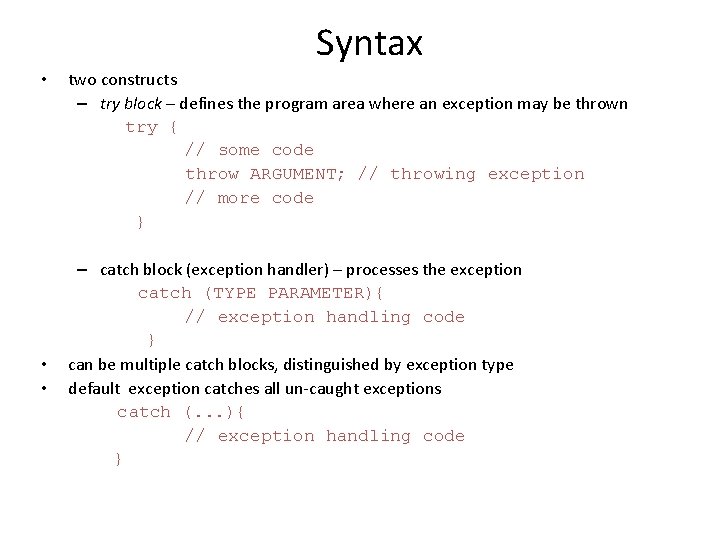
Syntax • • • two constructs – try block – defines the program area where an exception may be thrown try { // some code throw ARGUMENT; // throwing exception // more code } – catch block (exception handler) – processes the exception catch (TYPE PARAMETER){ // exception handling code } can be multiple catch blocks, distinguished by exception type default exception catches all un-caught exceptions catch (. . . ){ // exception handling code }
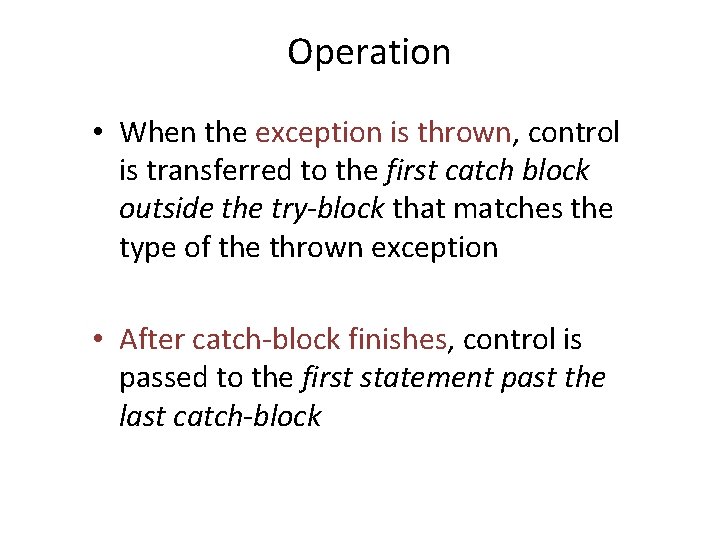
Operation • When the exception is thrown, control is transferred to the first catch block outside the try-block that matches the type of the thrown exception • After catch-block finishes, control is passed to the first statement past the last catch-block
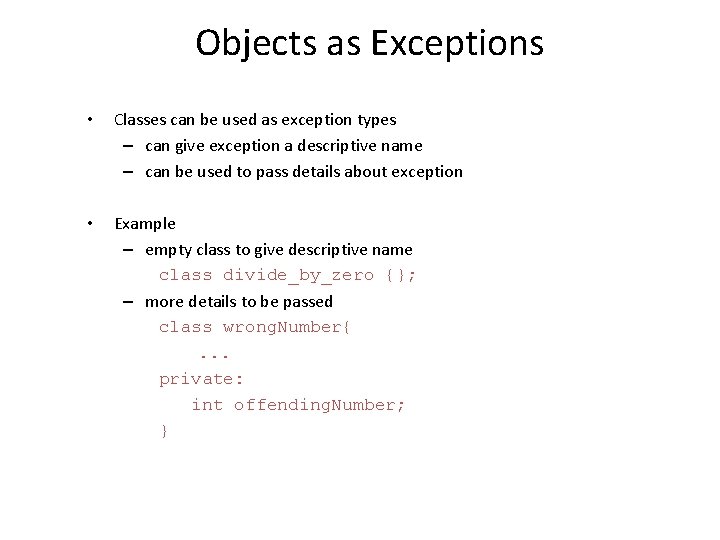
Objects as Exceptions • Classes can be used as exception types – can give exception a descriptive name – can be used to pass details about exception • Example – empty class to give descriptive name class divide_by_zero {}; – more details to be passed class wrong. Number{. . . private: int offending. Number; }
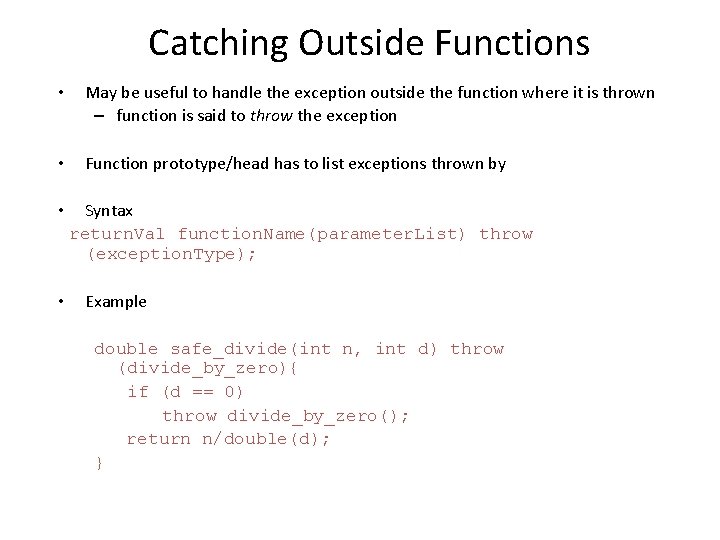
Catching Outside Functions • May be useful to handle the exception outside the function where it is thrown – function is said to throw the exception • Function prototype/head has to list exceptions thrown by • • Syntax return. Val function. Name(parameter. List) throw (exception. Type); Example double safe_divide(int n, int d) throw (divide_by_zero){ if (d == 0) throw divide_by_zero(); return n/double(d); }
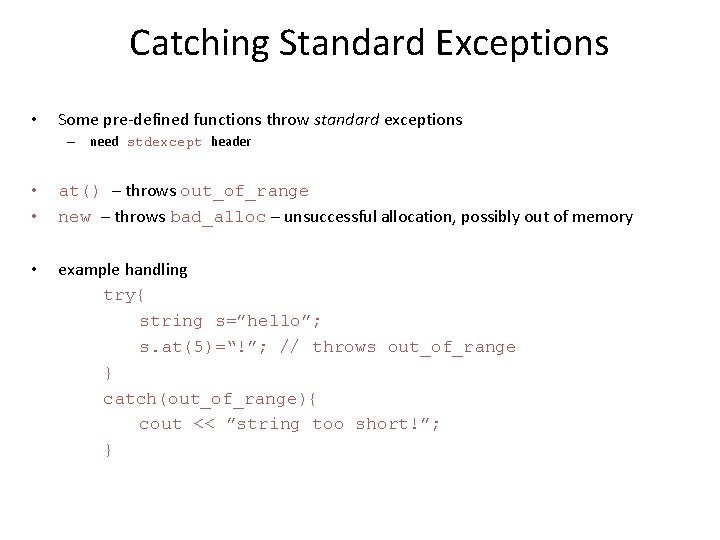
Catching Standard Exceptions • Some pre-defined functions throw standard exceptions – need stdexcept header • • at() – throws out_of_range new – throws bad_alloc – unsuccessful allocation, possibly out of memory • example handling try{ string s=”hello”; s. at(5)=“!”; // throws out_of_range } catch(out_of_range){ cout << ”string too short!”; }
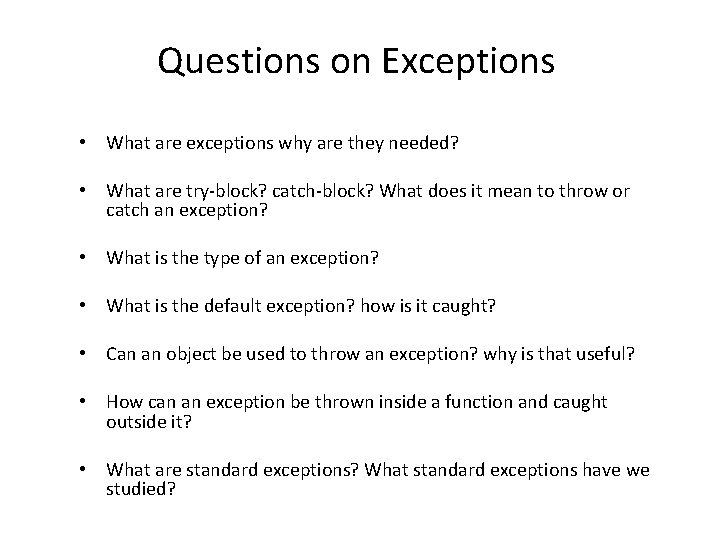
Questions on Exceptions • What are exceptions why are they needed? • What are try-block? catch-block? What does it mean to throw or catch an exception? • What is the type of an exception? • What is the default exception? how is it caught? • Can an object be used to throw an exception? why is that useful? • How can an exception be thrown inside a function and caught outside it? • What are standard exceptions? What standard exceptions have we studied?