Methods Return Values ICS 111 Introduction to Computer
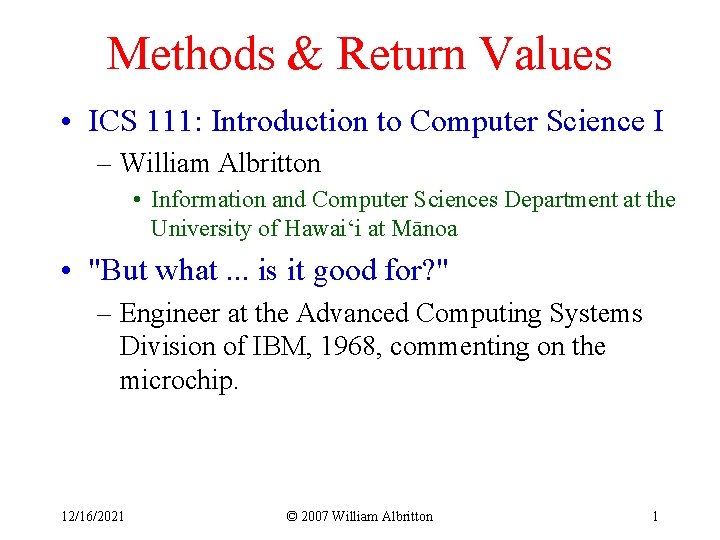
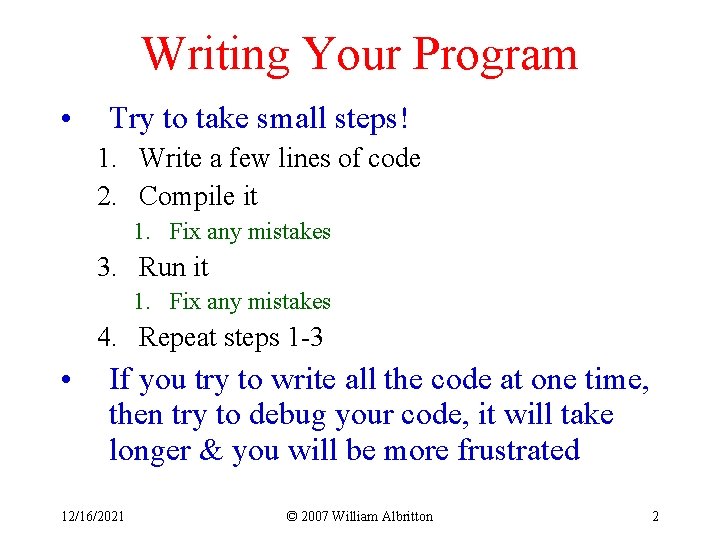
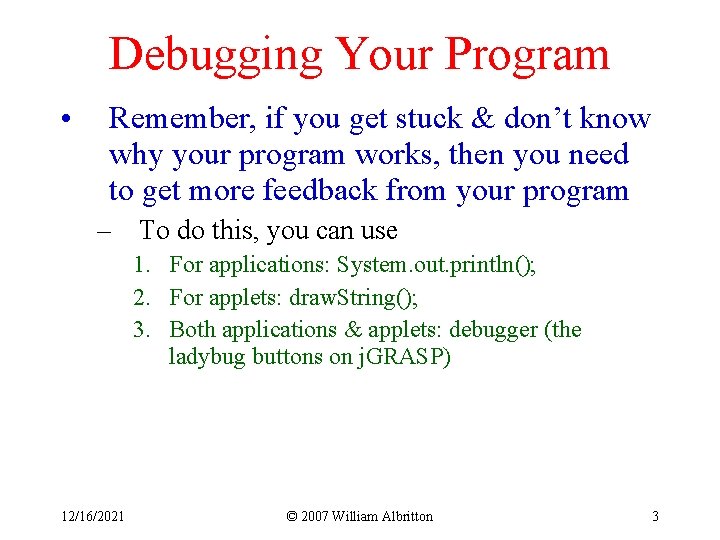
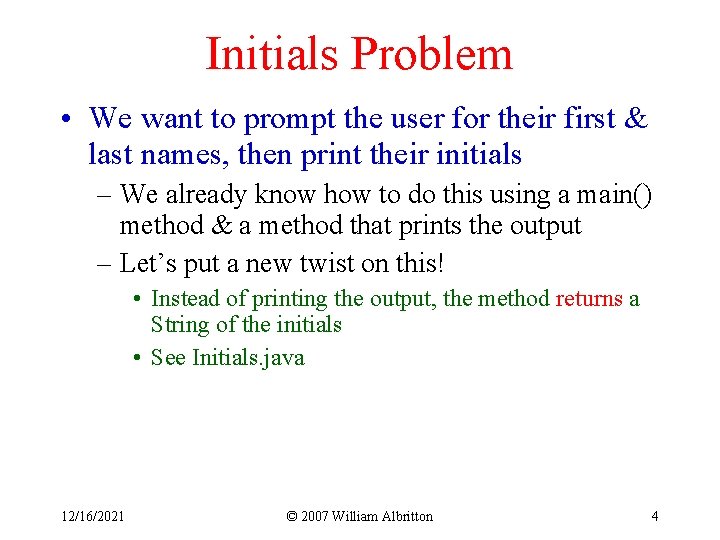
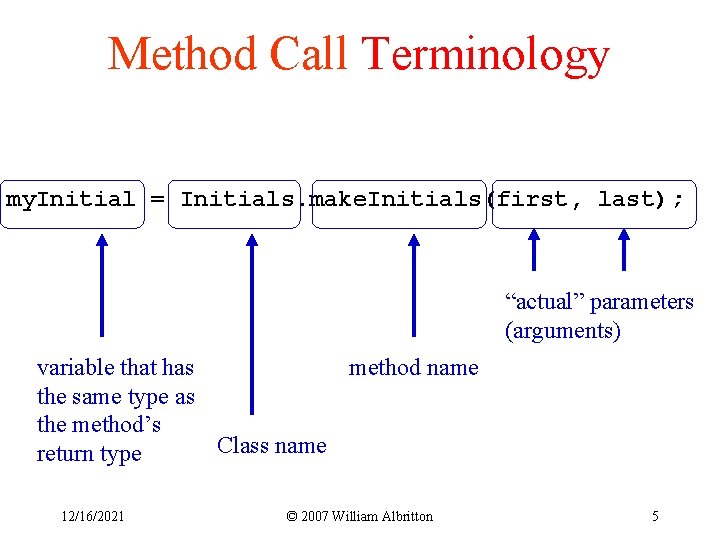
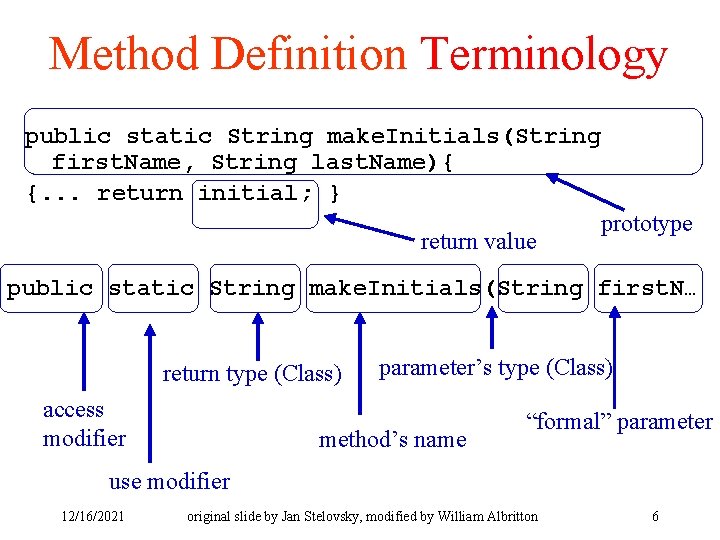
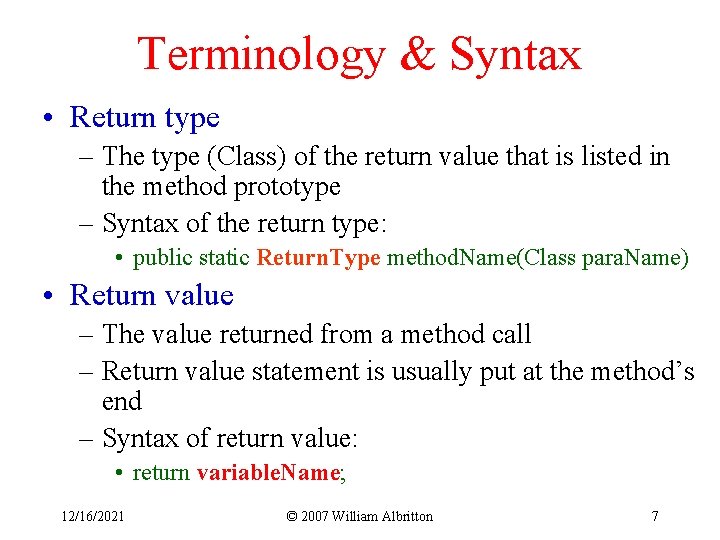
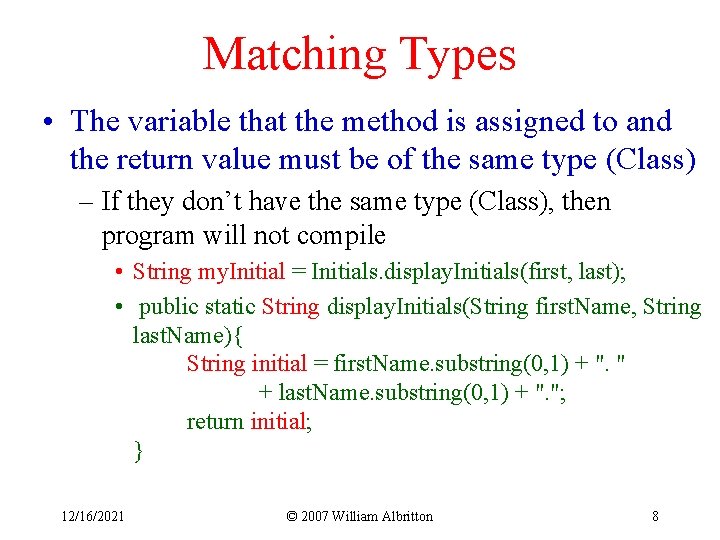
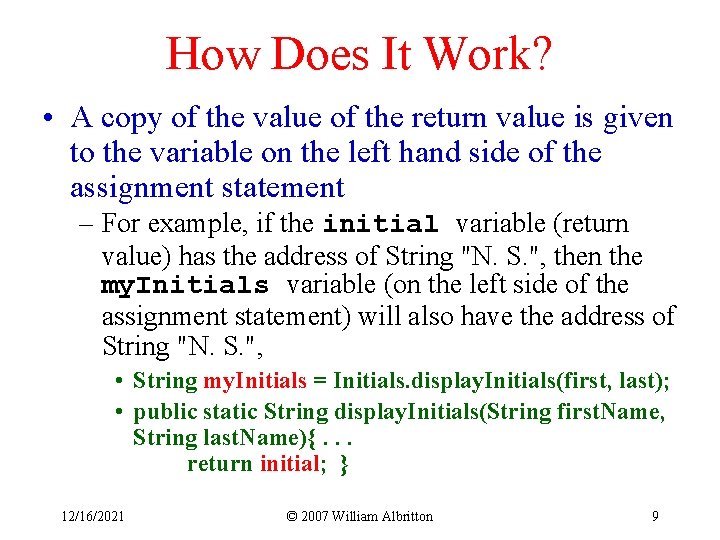
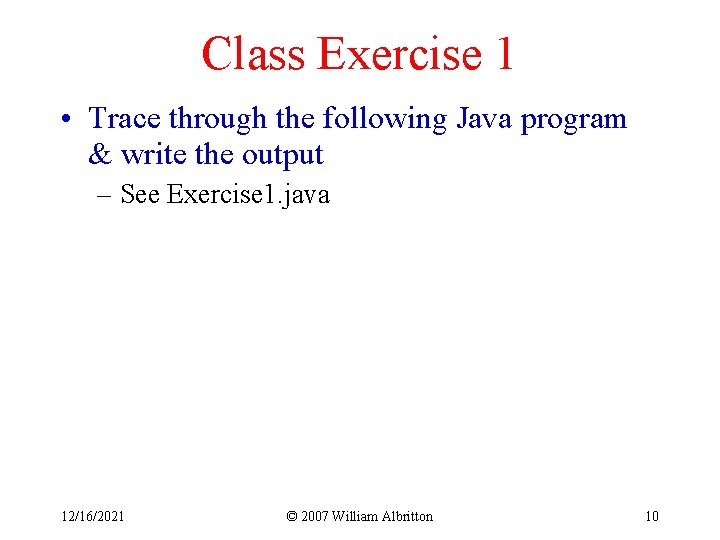
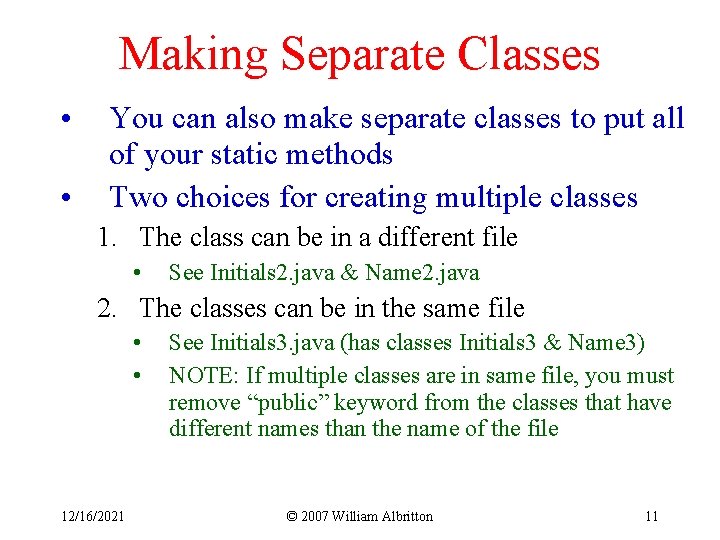
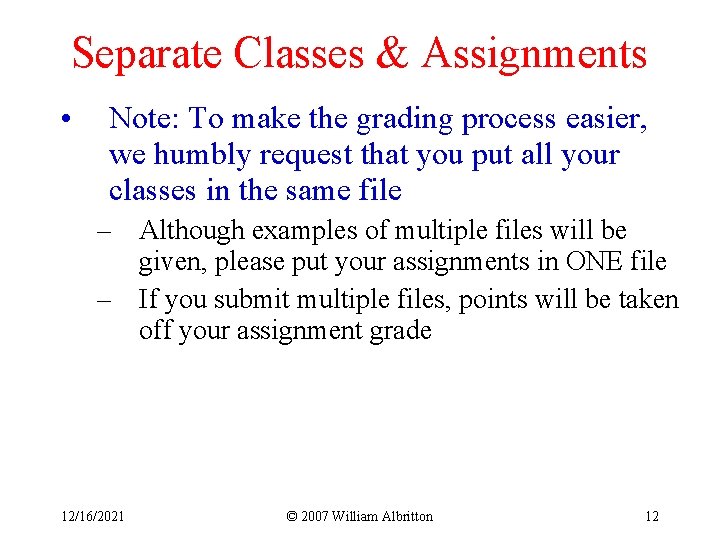
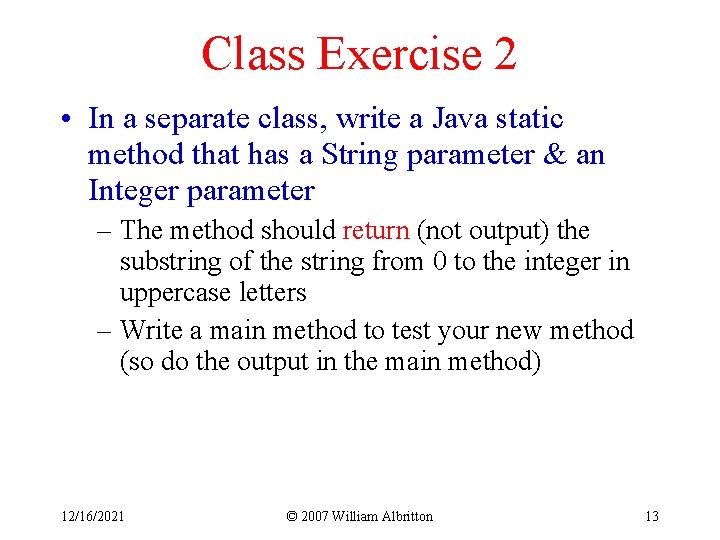
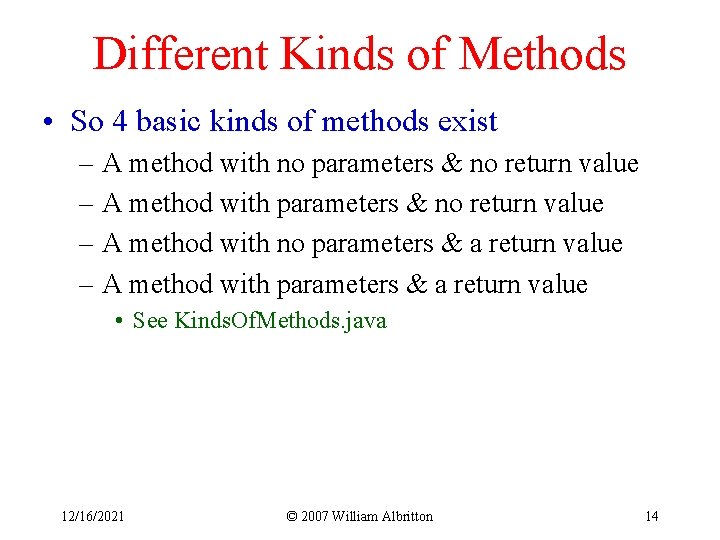
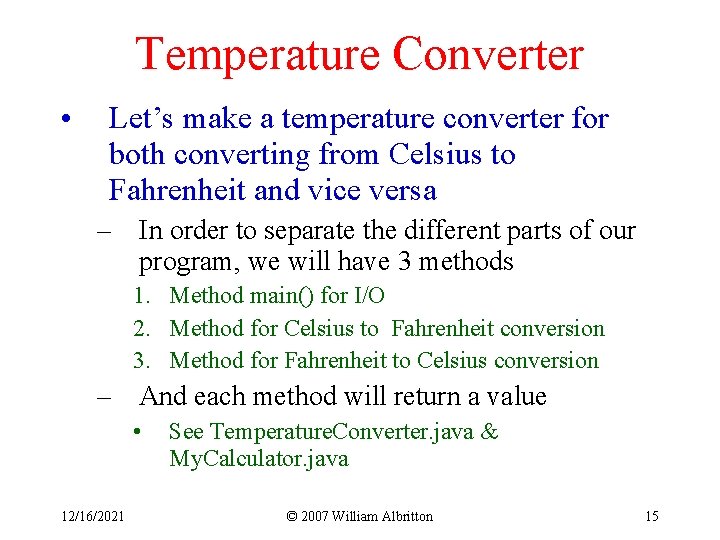
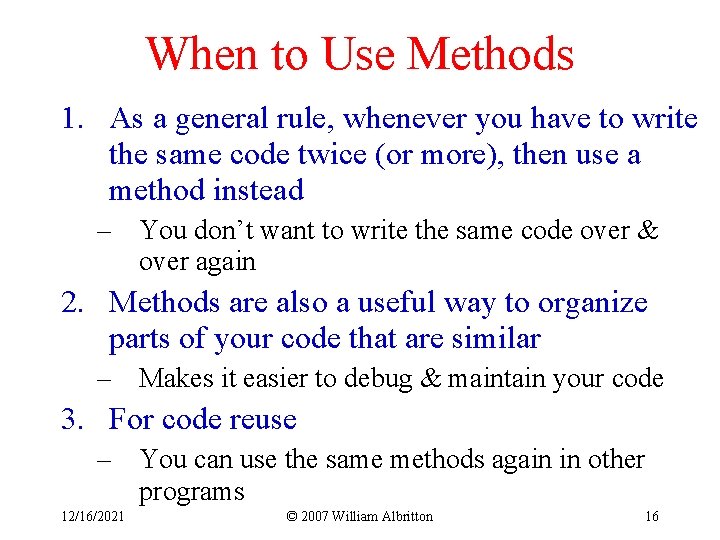
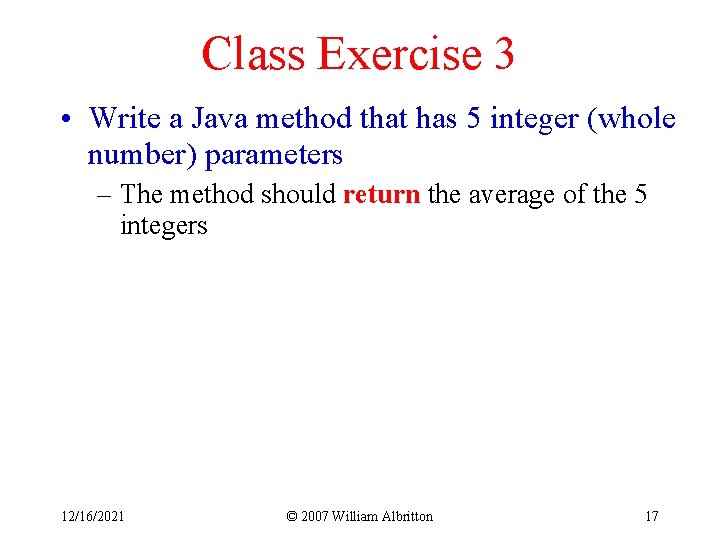
- Slides: 17
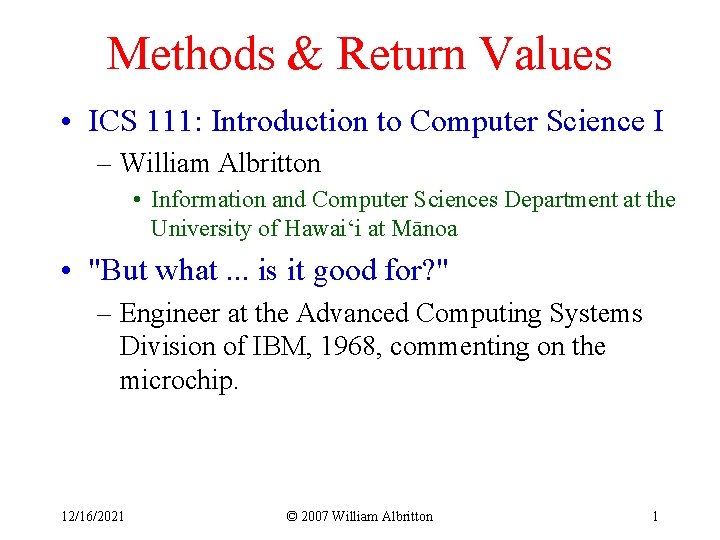
Methods & Return Values • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • "But what. . . is it good for? " – Engineer at the Advanced Computing Systems Division of IBM, 1968, commenting on the microchip. 12/16/2021 © 2007 William Albritton 1
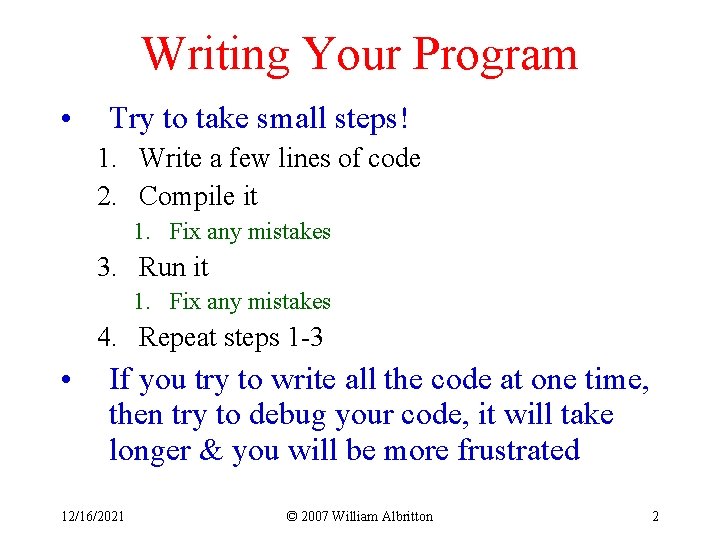
Writing Your Program • Try to take small steps! 1. Write a few lines of code 2. Compile it 1. Fix any mistakes 3. Run it 1. Fix any mistakes 4. Repeat steps 1 -3 • If you try to write all the code at one time, then try to debug your code, it will take longer & you will be more frustrated 12/16/2021 © 2007 William Albritton 2
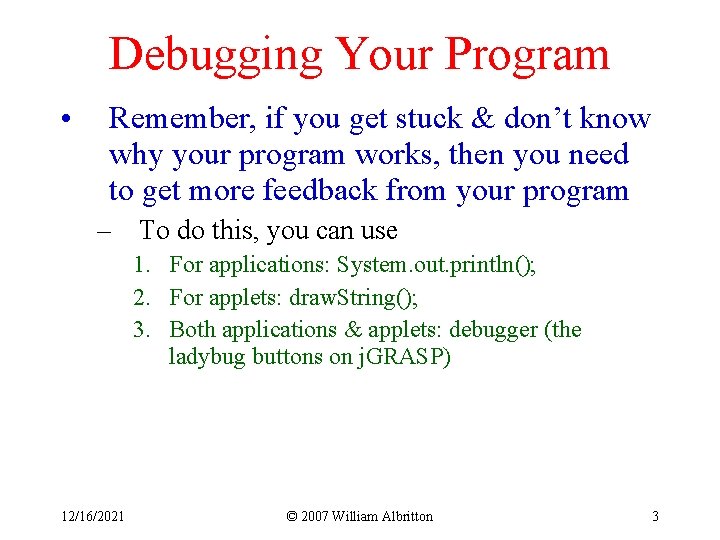
Debugging Your Program • Remember, if you get stuck & don’t know why your program works, then you need to get more feedback from your program – To do this, you can use 1. For applications: System. out. println(); 2. For applets: draw. String(); 3. Both applications & applets: debugger (the ladybug buttons on j. GRASP) 12/16/2021 © 2007 William Albritton 3
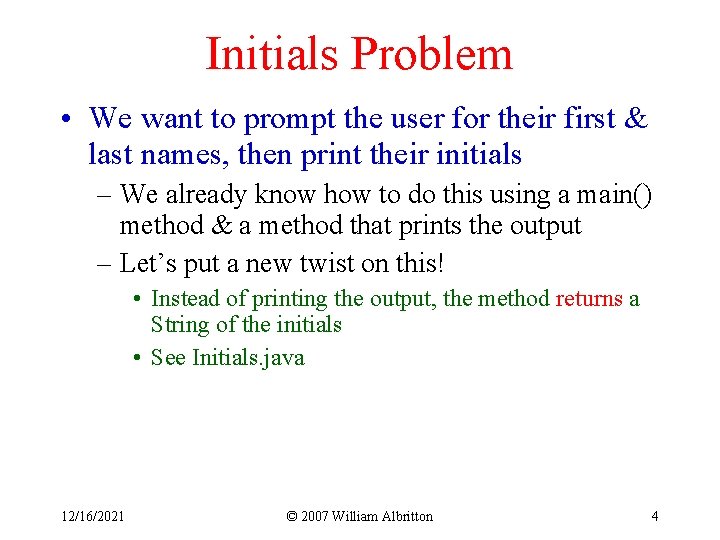
Initials Problem • We want to prompt the user for their first & last names, then print their initials – We already know how to do this using a main() method & a method that prints the output – Let’s put a new twist on this! • Instead of printing the output, the method returns a String of the initials • See Initials. java 12/16/2021 © 2007 William Albritton 4
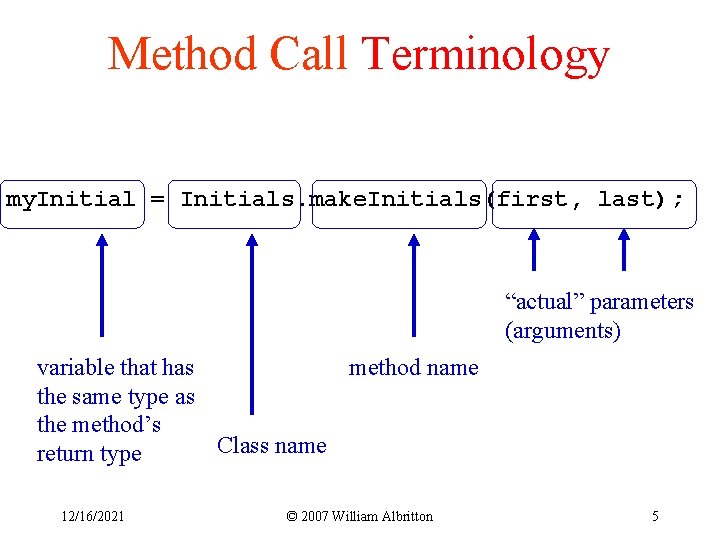
Method Call Terminology my. Initial = Initials. make. Initials(first, last); “actual” parameters (arguments) variable that has method name the same type as the method’s Class name return type 12/16/2021 © 2007 William Albritton 5
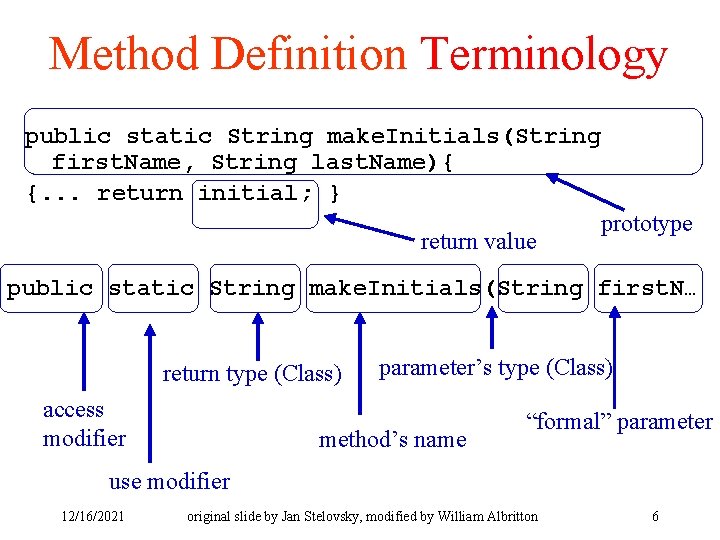
Method Definition Terminology public static String make. Initials(String first. Name, String last. Name){ {. . . return initial; } prototype return value public static String make. Initials(String first. N… return type (Class) access modifier parameter’s type (Class) method’s name “formal” parameter use modifier 12/16/2021 original slide by Jan Stelovsky, modified by William Albritton 6
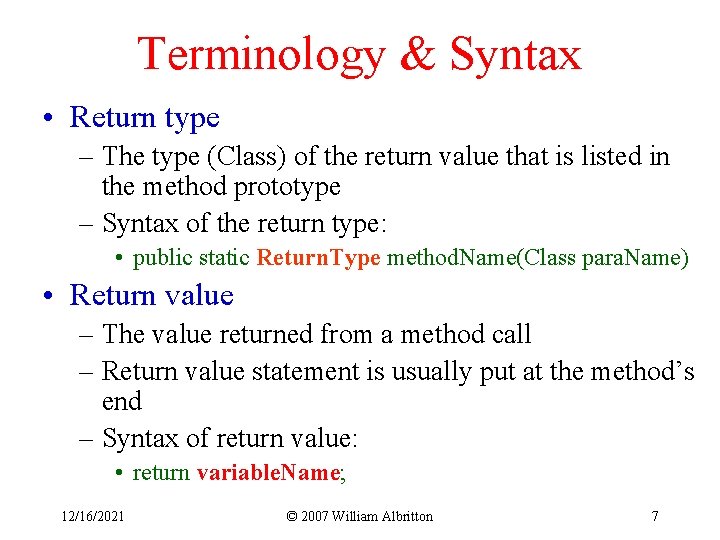
Terminology & Syntax • Return type – The type (Class) of the return value that is listed in the method prototype – Syntax of the return type: • public static Return. Type method. Name(Class para. Name) • Return value – The value returned from a method call – Return value statement is usually put at the method’s end – Syntax of return value: • return variable. Name; 12/16/2021 © 2007 William Albritton 7
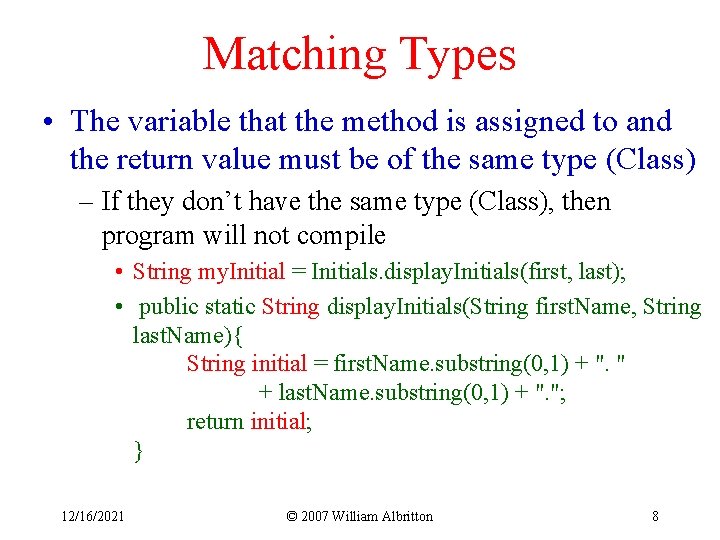
Matching Types • The variable that the method is assigned to and the return value must be of the same type (Class) – If they don’t have the same type (Class), then program will not compile • String my. Initial = Initials. display. Initials(first, last); • public static String display. Initials(String first. Name, String last. Name){ String initial = first. Name. substring(0, 1) + ". " + last. Name. substring(0, 1) + ". "; return initial; } 12/16/2021 © 2007 William Albritton 8
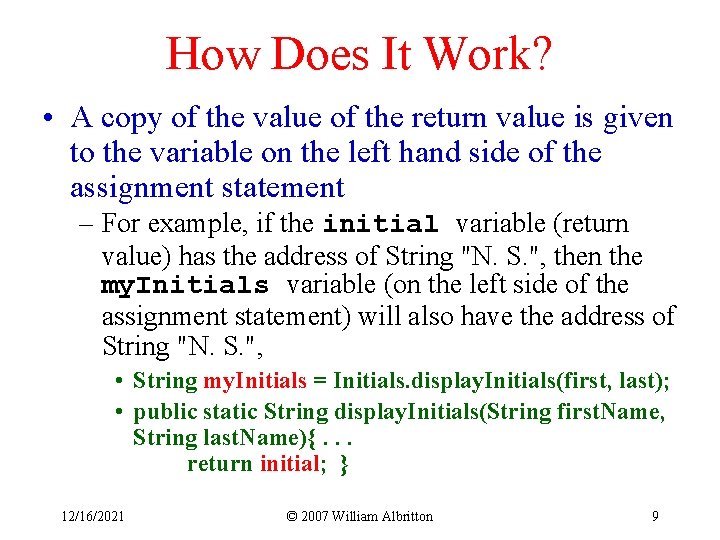
How Does It Work? • A copy of the value of the return value is given to the variable on the left hand side of the assignment statement – For example, if the initial variable (return value) has the address of String "N. S. ", then the my. Initials variable (on the left side of the assignment statement) will also have the address of String "N. S. ", • String my. Initials = Initials. display. Initials(first, last); • public static String display. Initials(String first. Name, String last. Name){. . . return initial; } 12/16/2021 © 2007 William Albritton 9
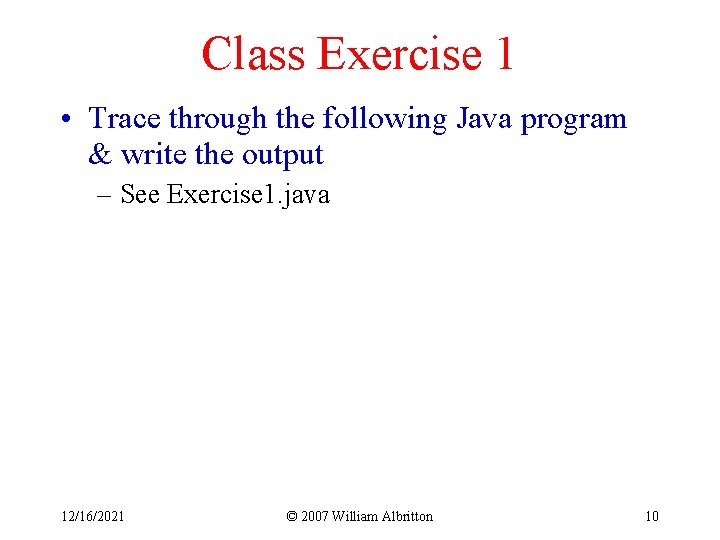
Class Exercise 1 • Trace through the following Java program & write the output – See Exercise 1. java 12/16/2021 © 2007 William Albritton 10
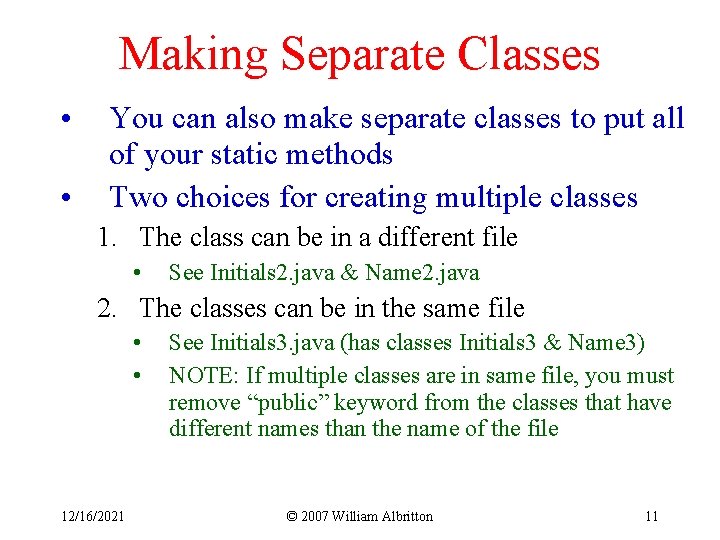
Making Separate Classes • • You can also make separate classes to put all of your static methods Two choices for creating multiple classes 1. The class can be in a different file • See Initials 2. java & Name 2. java 2. The classes can be in the same file • • 12/16/2021 See Initials 3. java (has classes Initials 3 & Name 3) NOTE: If multiple classes are in same file, you must remove “public” keyword from the classes that have different names than the name of the file © 2007 William Albritton 11
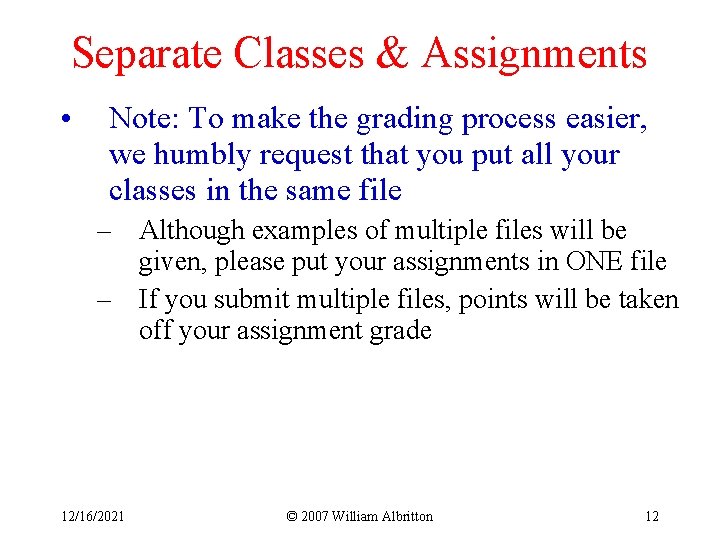
Separate Classes & Assignments • Note: To make the grading process easier, we humbly request that you put all your classes in the same file – Although examples of multiple files will be given, please put your assignments in ONE file – If you submit multiple files, points will be taken off your assignment grade 12/16/2021 © 2007 William Albritton 12
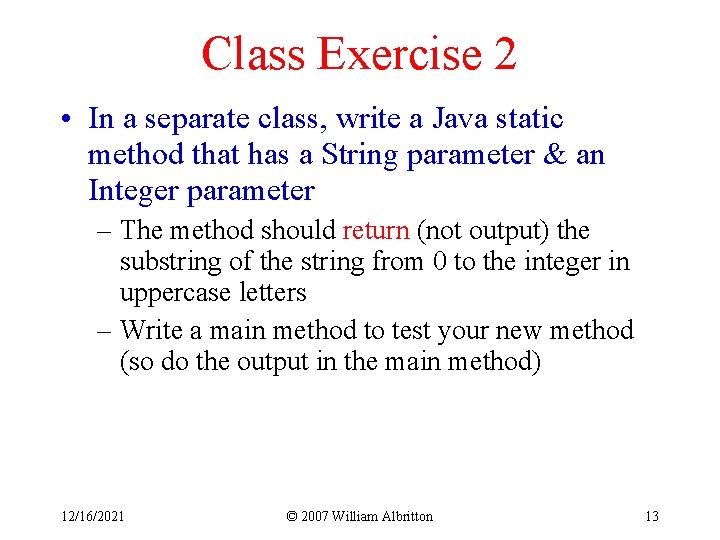
Class Exercise 2 • In a separate class, write a Java static method that has a String parameter & an Integer parameter – The method should return (not output) the substring of the string from 0 to the integer in uppercase letters – Write a main method to test your new method (so do the output in the main method) 12/16/2021 © 2007 William Albritton 13
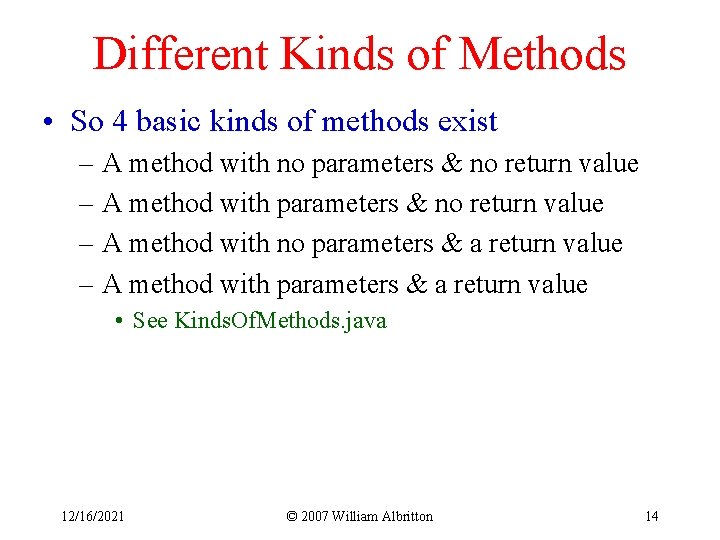
Different Kinds of Methods • So 4 basic kinds of methods exist – A method with no parameters & no return value – A method with no parameters & a return value – A method with parameters & a return value • See Kinds. Of. Methods. java 12/16/2021 © 2007 William Albritton 14
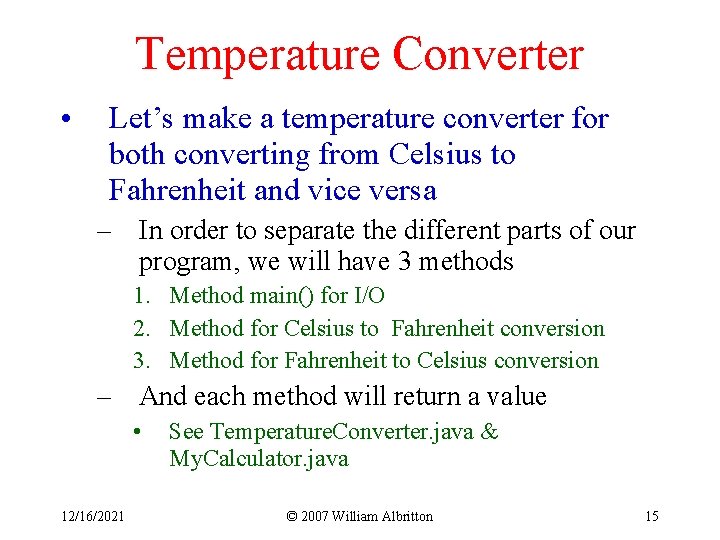
Temperature Converter • Let’s make a temperature converter for both converting from Celsius to Fahrenheit and vice versa – In order to separate the different parts of our program, we will have 3 methods 1. Method main() for I/O 2. Method for Celsius to Fahrenheit conversion 3. Method for Fahrenheit to Celsius conversion – And each method will return a value • 12/16/2021 See Temperature. Converter. java & My. Calculator. java © 2007 William Albritton 15
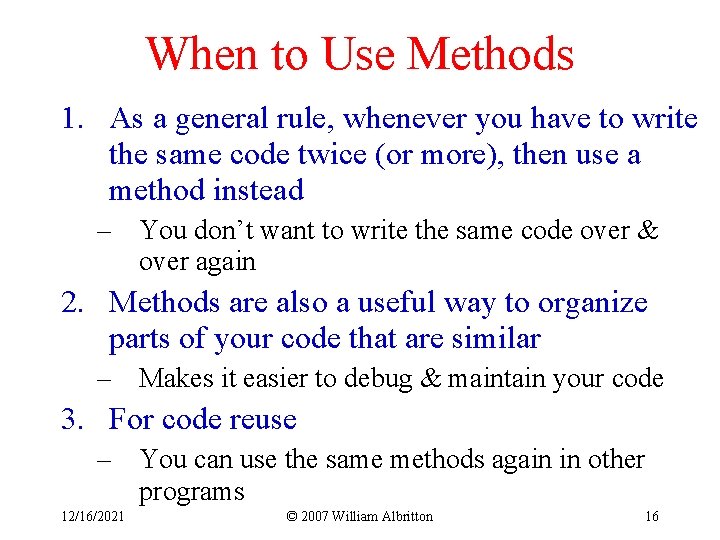
When to Use Methods 1. As a general rule, whenever you have to write the same code twice (or more), then use a method instead – You don’t want to write the same code over & over again 2. Methods are also a useful way to organize parts of your code that are similar – Makes it easier to debug & maintain your code 3. For code reuse – You can use the same methods again in other programs 12/16/2021 © 2007 William Albritton 16
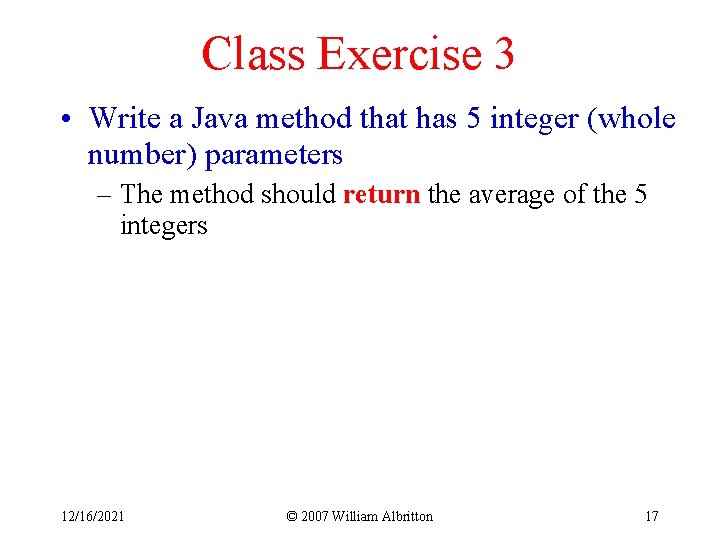
Class Exercise 3 • Write a Java method that has 5 integer (whole number) parameters – The method should return the average of the 5 integers 12/16/2021 © 2007 William Albritton 17
110 000 110 & 111 000 111
Ics 111
Ics 33 midterm
Ics 111
Ics 400: advanced ics for complex incidents-aberdeen
It 111 introduction to computing
Western values vs eastern values
Machavillian
Terminal values and instrumental values
What are the two possible values of bit
Explain the concept of human values.
Indirect methods of contoring uses how many methods
Introduction to risk and return
Advantages and disadvantages of boundary fill algorithm
Interactive picture construction techniques
Computer architecture performance evaluation methods
Scientific methods in computer science
Flair furniture company linear programming