Memory Management Memory Management Managing the heap Resource
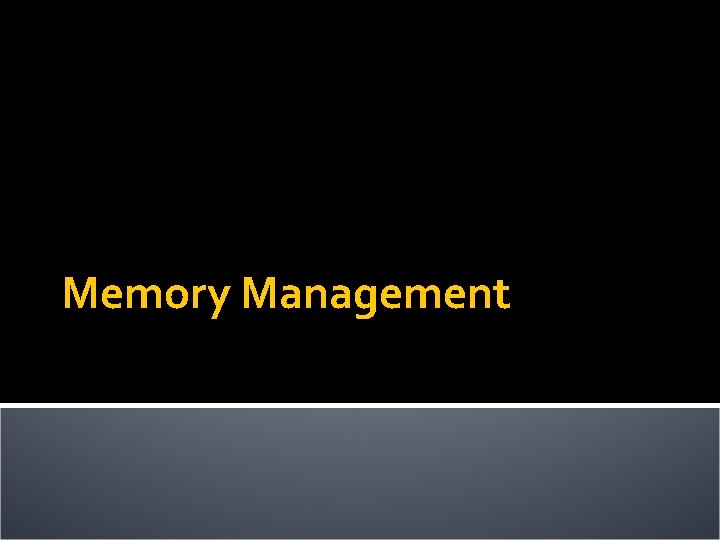
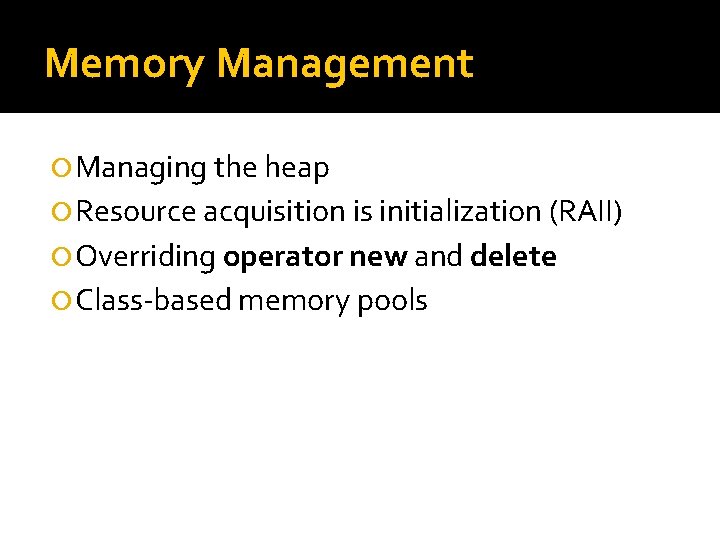
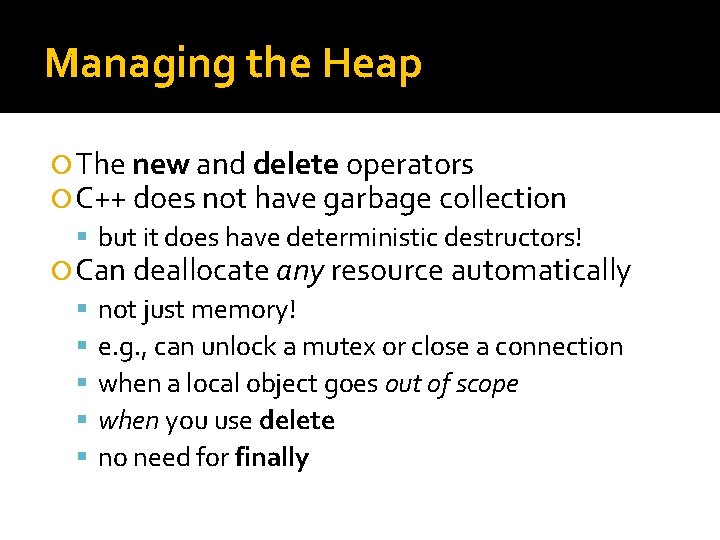
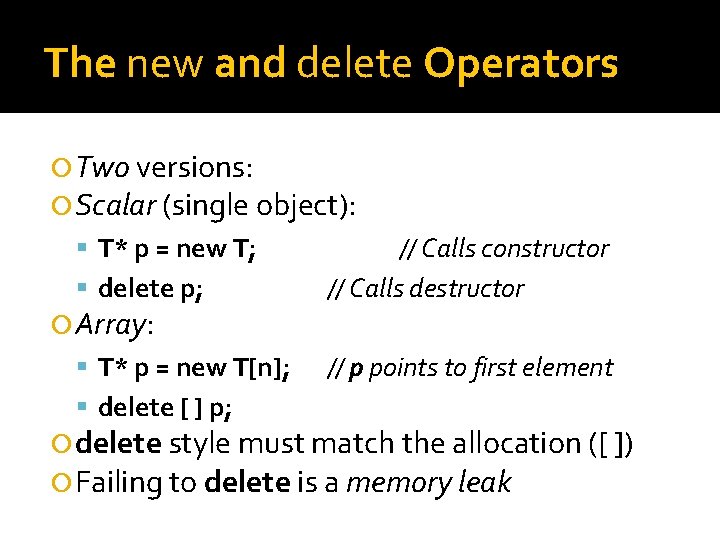
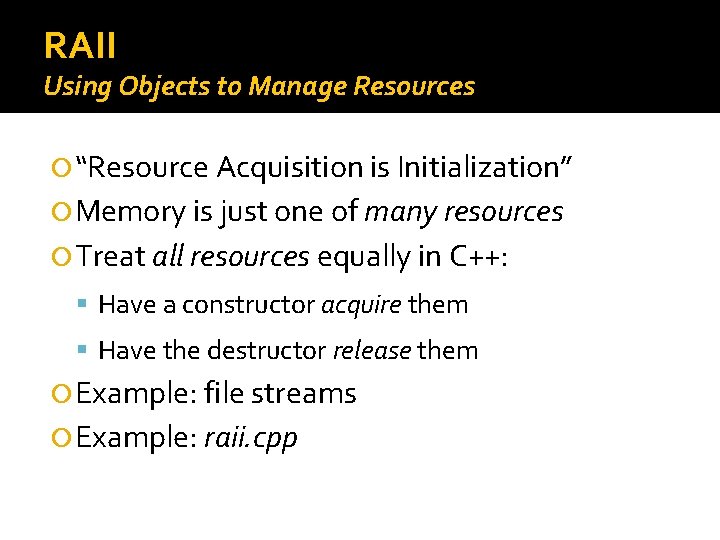
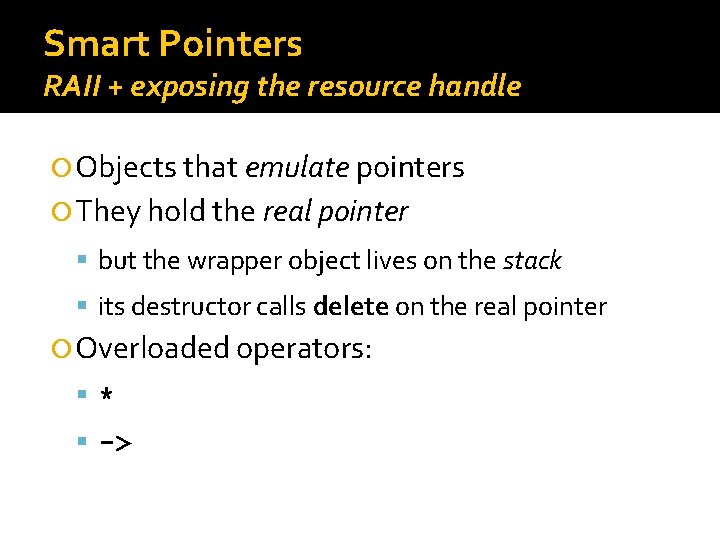
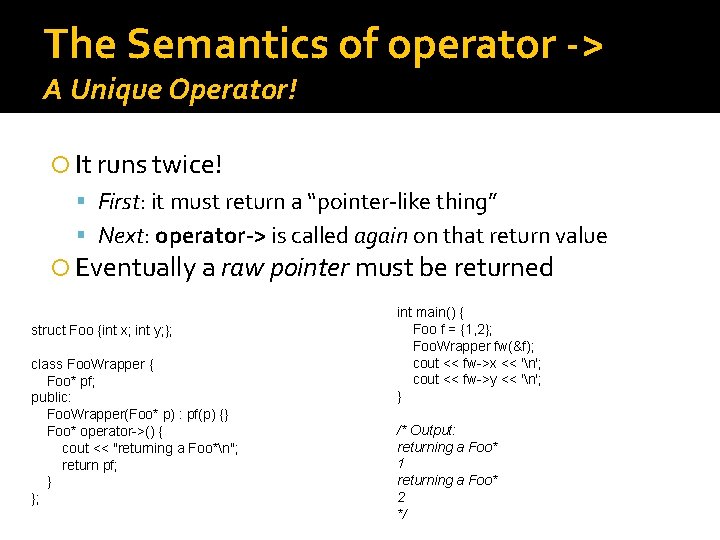
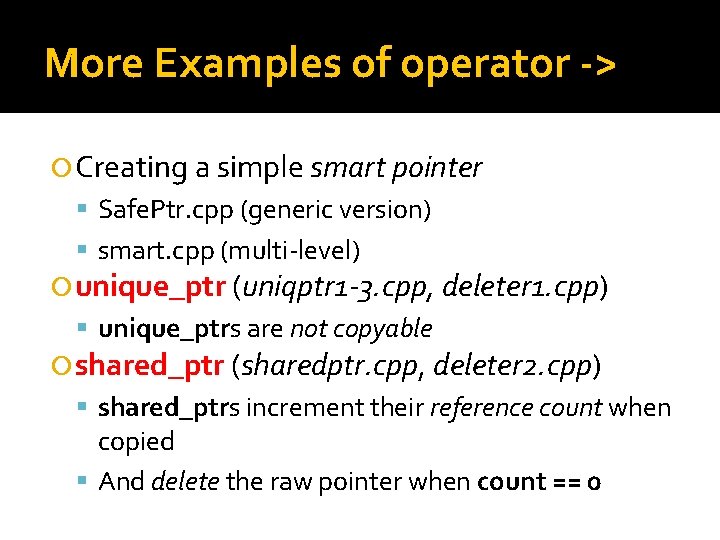
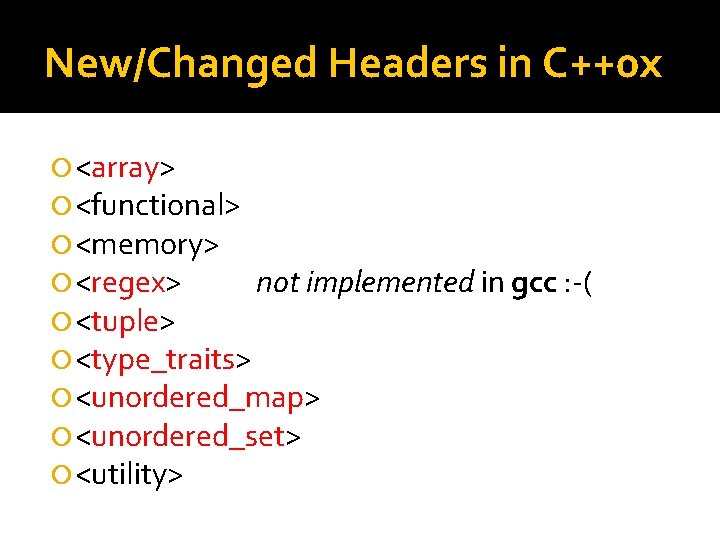
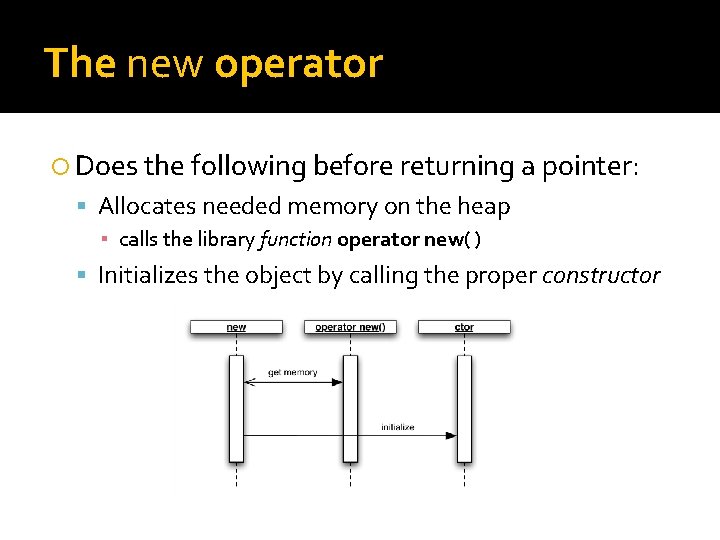
![The new [ ] operator For Arrays Does the following before returning a pointer The new [ ] operator For Arrays Does the following before returning a pointer](https://slidetodoc.com/presentation_image_h2/939a803552cbcf6505dfe011d4491a7e/image-11.jpg)
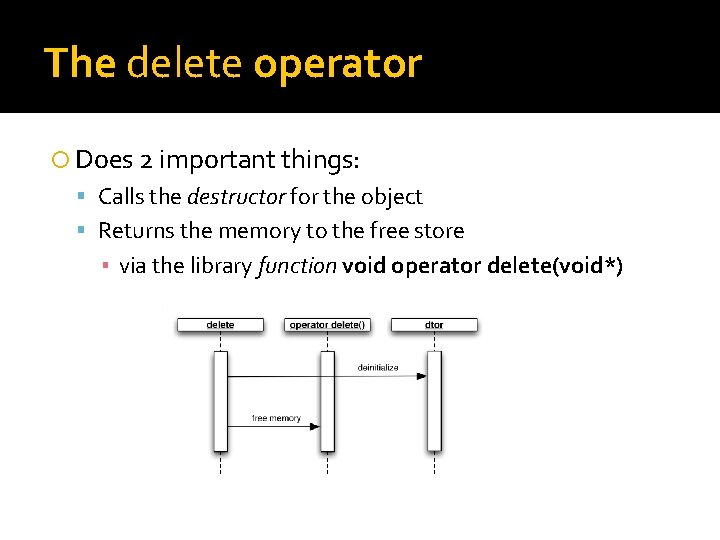
![The delete [ ] operator For Arrays Calls the destructor for each object in The delete [ ] operator For Arrays Calls the destructor for each object in](https://slidetodoc.com/presentation_image_h2/939a803552cbcf6505dfe011d4491a7e/image-13.jpg)
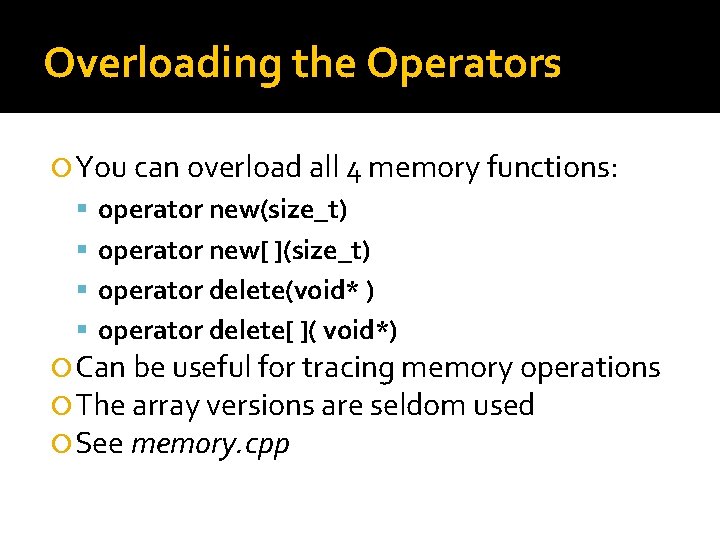
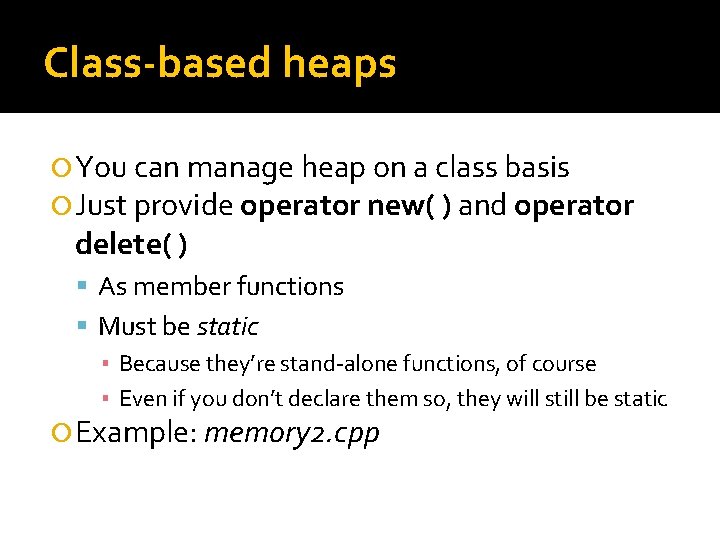
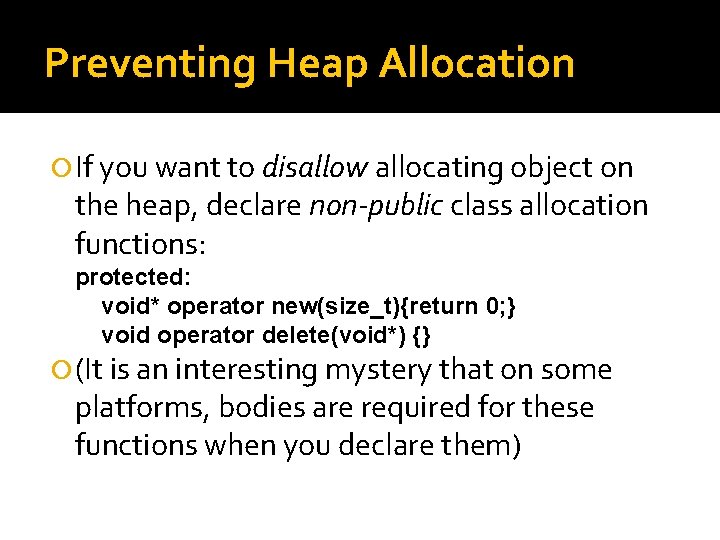
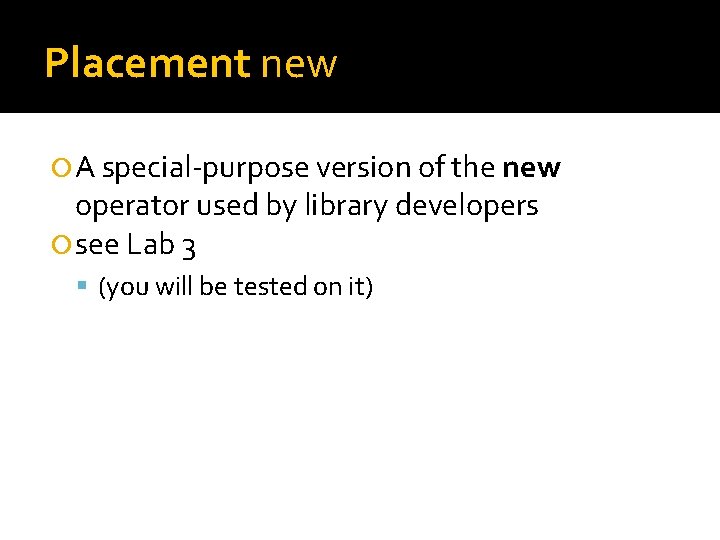
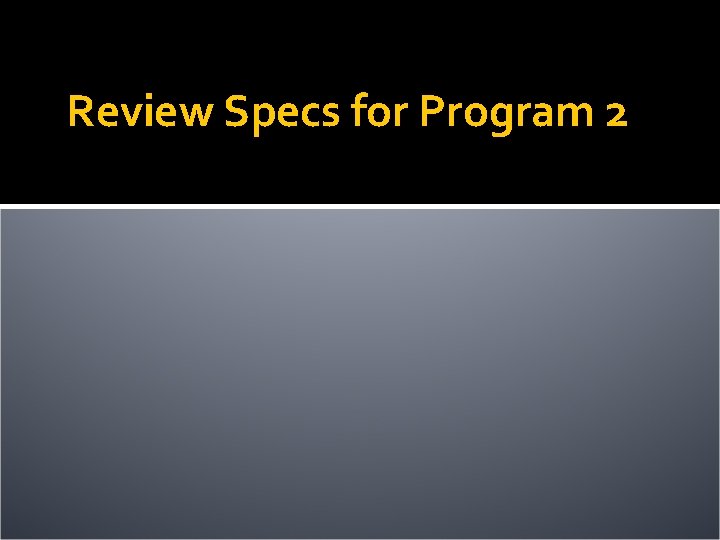
- Slides: 18
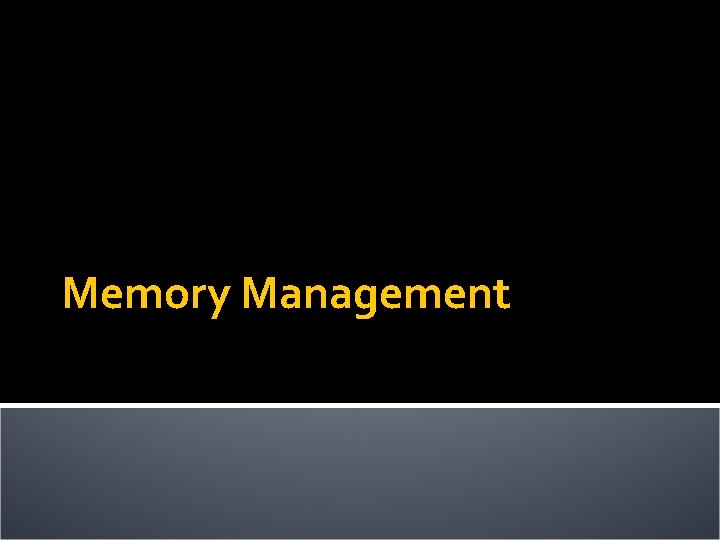
Memory Management
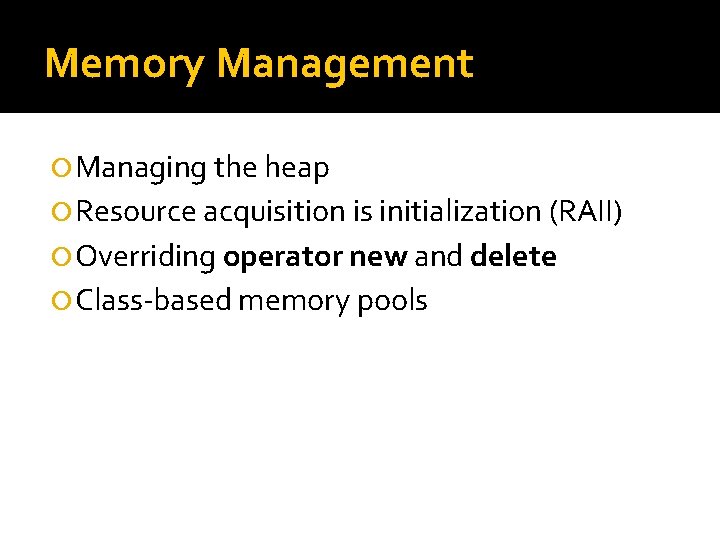
Memory Management Managing the heap Resource acquisition is initialization (RAII) Overriding operator new and delete Class-based memory pools
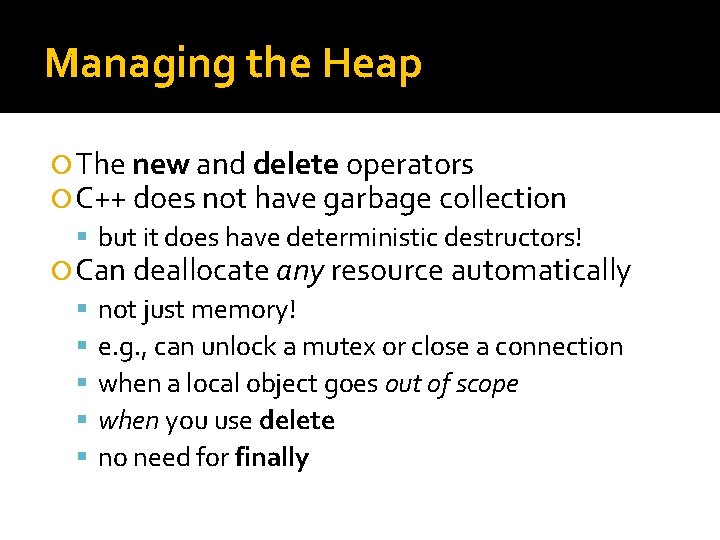
Managing the Heap The new and delete operators C++ does not have garbage collection but it does have deterministic destructors! Can deallocate any resource automatically not just memory! e. g. , can unlock a mutex or close a connection when a local object goes out of scope when you use delete no need for finally
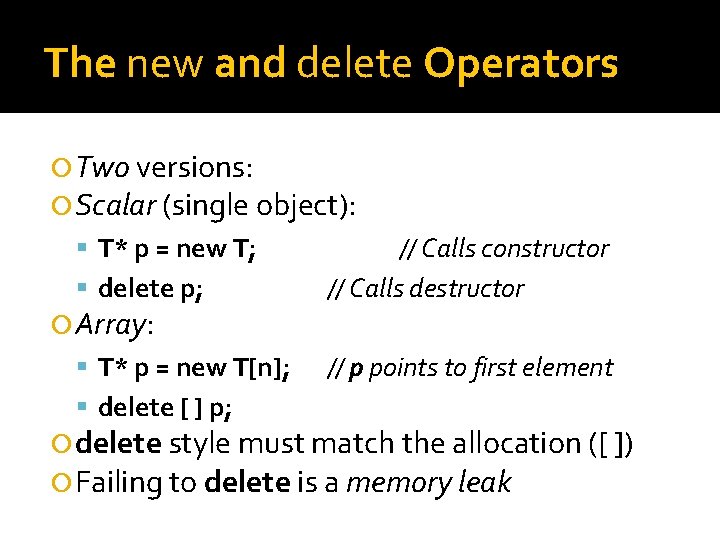
The new and delete Operators Two versions: Scalar (single object): T* p = new T; delete p; // Calls constructor // Calls destructor T* p = new T[n]; // p points to first element Array: delete [ ] p; delete style must match the allocation ([ ]) Failing to delete is a memory leak
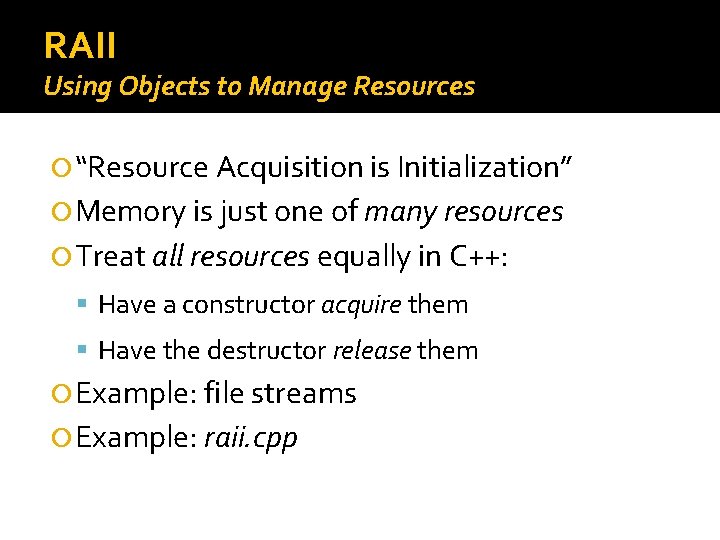
RAII Using Objects to Manage Resources “Resource Acquisition is Initialization” Memory is just one of many resources Treat all resources equally in C++: Have a constructor acquire them Have the destructor release them Example: file streams Example: raii. cpp
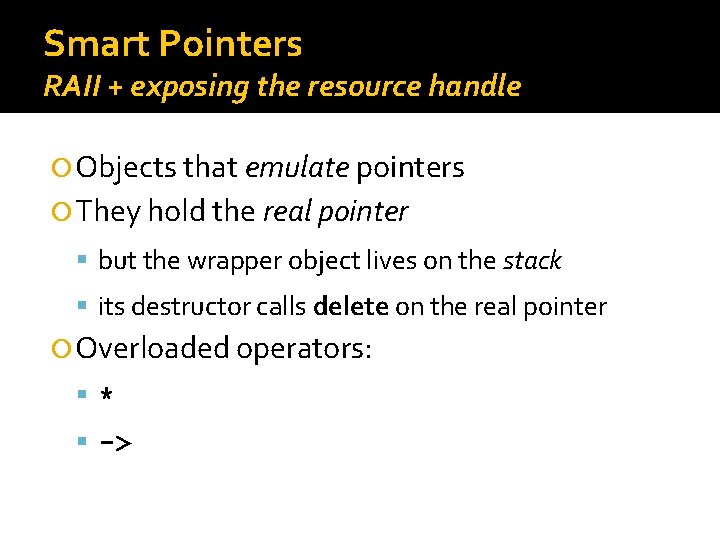
Smart Pointers RAII + exposing the resource handle Objects that emulate pointers They hold the real pointer but the wrapper object lives on the stack its destructor calls delete on the real pointer Overloaded operators: * ->
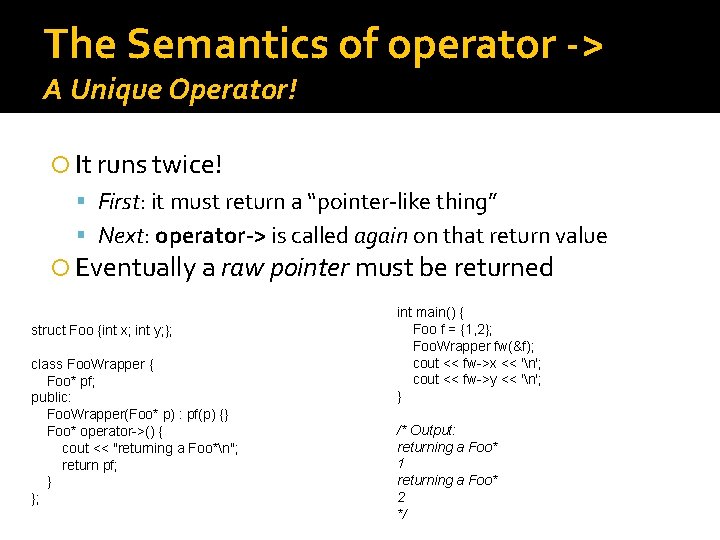
The Semantics of operator -> A Unique Operator! It runs twice! First: it must return a “pointer-like thing” Next: operator-> is called again on that return value Eventually a raw pointer must be returned struct Foo {int x; int y; }; class Foo. Wrapper { Foo* pf; public: Foo. Wrapper(Foo* p) : pf(p) {} Foo* operator->() { cout << "returning a Foo*n"; return pf; } }; int main() { Foo f = {1, 2}; Foo. Wrapper fw(&f); cout << fw->x << 'n'; cout << fw->y << 'n'; } /* Output: returning a Foo* 1 returning a Foo* 2 */
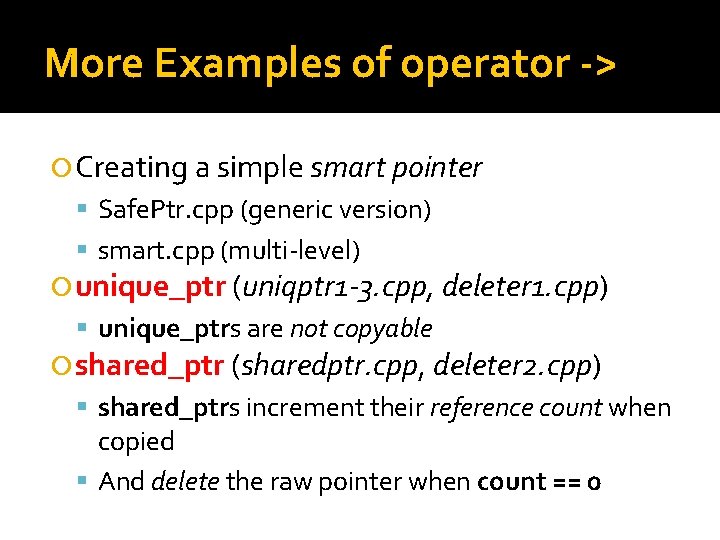
More Examples of operator -> Creating a simple smart pointer Safe. Ptr. cpp (generic version) smart. cpp (multi-level) unique_ptr (uniqptr 1 -3. cpp, deleter 1. cpp) unique_ptrs are not copyable shared_ptr (sharedptr. cpp, deleter 2. cpp) shared_ptrs increment their reference count when copied And delete the raw pointer when count == 0
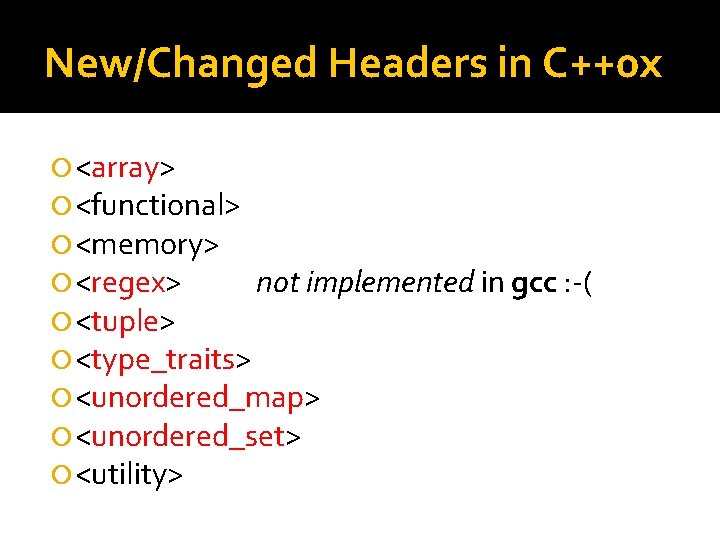
New/Changed Headers in C++0 x <array> <functional> <memory> <regex> not implemented in gcc : -( <tuple> <type_traits> <unordered_map> <unordered_set> <utility>
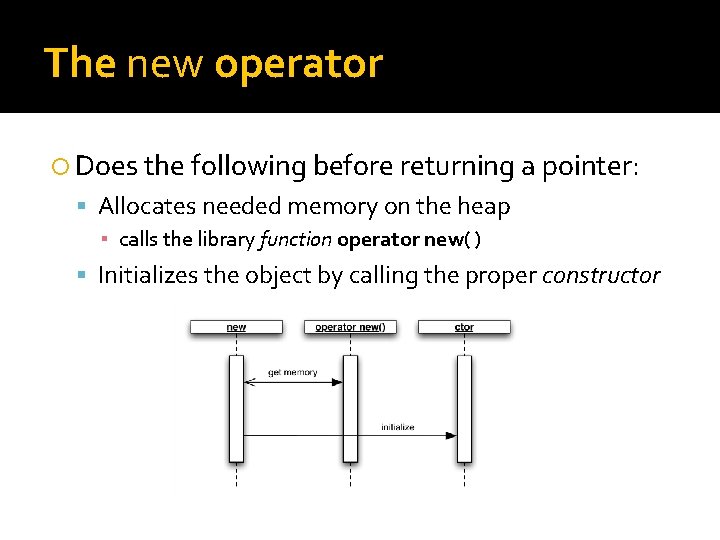
The new operator Does the following before returning a pointer: Allocates needed memory on the heap ▪ calls the library function operator new( ) Initializes the object by calling the proper constructor
![The new operator For Arrays Does the following before returning a pointer The new [ ] operator For Arrays Does the following before returning a pointer](https://slidetodoc.com/presentation_image_h2/939a803552cbcf6505dfe011d4491a7e/image-11.jpg)
The new [ ] operator For Arrays Does the following before returning a pointer to the first element: Allocates needed memory on the heap ▪ calls the library function operator new[ ]( ) Initializes each object by calling the proper constructor
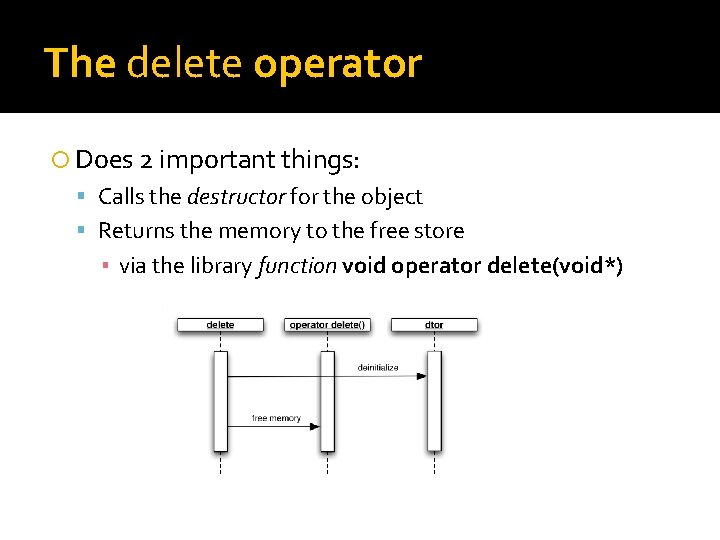
The delete operator Does 2 important things: Calls the destructor for the object Returns the memory to the free store ▪ via the library function void operator delete(void*)
![The delete operator For Arrays Calls the destructor for each object in The delete [ ] operator For Arrays Calls the destructor for each object in](https://slidetodoc.com/presentation_image_h2/939a803552cbcf6505dfe011d4491a7e/image-13.jpg)
The delete [ ] operator For Arrays Calls the destructor for each object in the array Returns the memory to the free store via void operator delete(void*); You must use delete [ ] for arrays
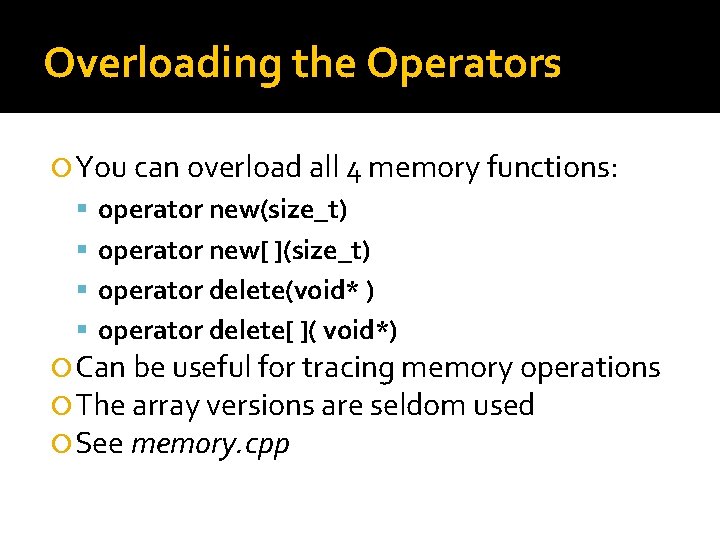
Overloading the Operators You can overload all 4 memory functions: operator new(size_t) operator new[ ](size_t) operator delete(void* ) operator delete[ ]( void*) Can be useful for tracing memory operations The array versions are seldom used See memory. cpp
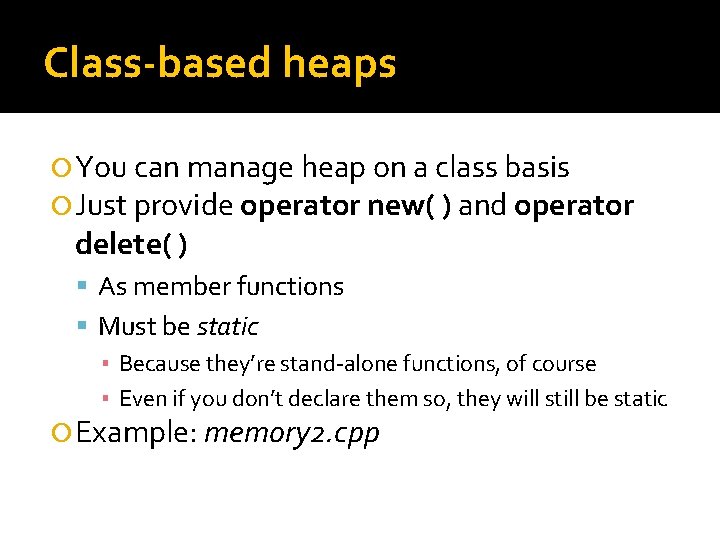
Class-based heaps You can manage heap on a class basis Just provide operator new( ) and operator delete( ) As member functions Must be static ▪ Because they’re stand-alone functions, of course ▪ Even if you don’t declare them so, they will still be static Example: memory 2. cpp
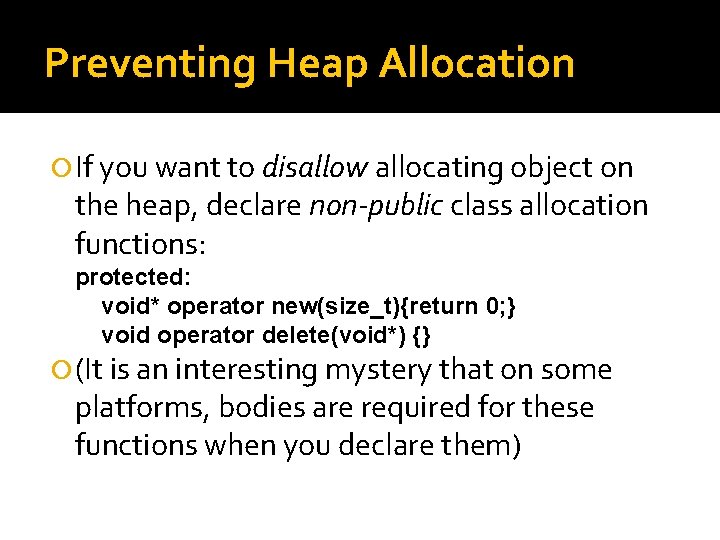
Preventing Heap Allocation If you want to disallow allocating object on the heap, declare non-public class allocation functions: protected: void* operator new(size_t){return 0; } void operator delete(void*) {} (It is an interesting mystery that on some platforms, bodies are required for these functions when you declare them)
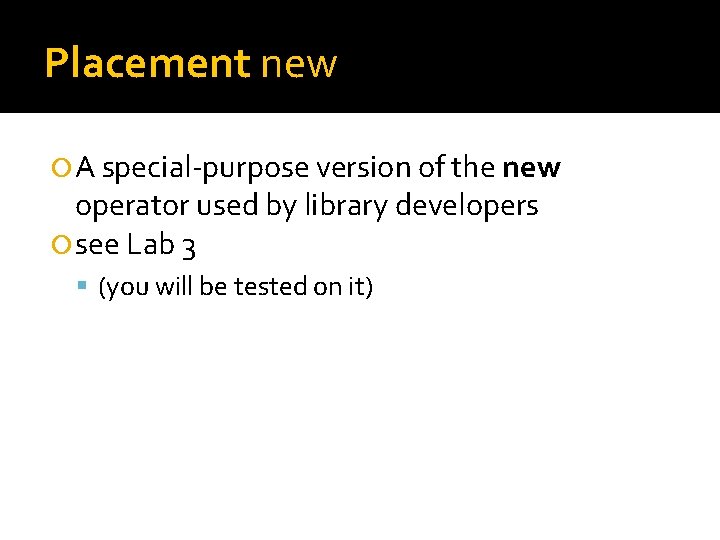
Placement new A special-purpose version of the new operator used by library developers see Lab 3 (you will be tested on it)
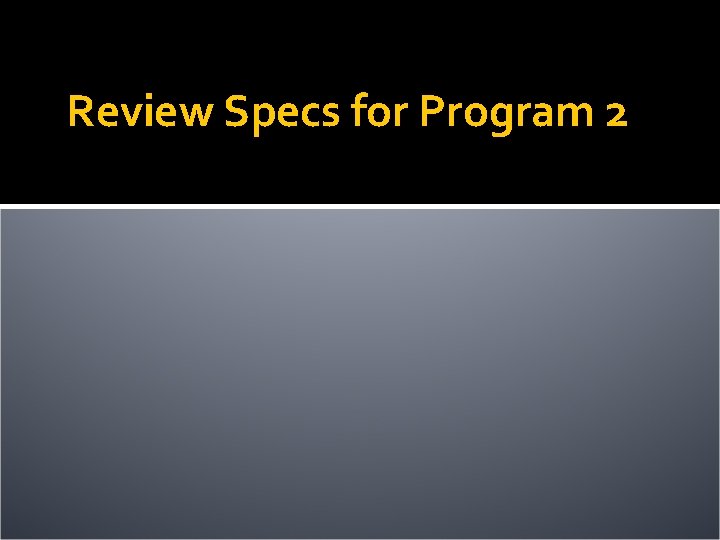
Review Specs for Program 2
Heap vs binary heap
Stack and heap memory
Vmware resource management
Memory resource management in vmware esx server
Resource leveling is the approach to even out the peaks of
Contoh resource loading
Time management human resources
Retail organization structure
Literal sense in hrm
Hát kết hợp bộ gõ cơ thể
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Gấu đi như thế nào
Glasgow thang điểm
Alleluia hat len nguoi oi
Kể tên các môn thể thao
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới