Chapter 11 Heap Overview The heap is a
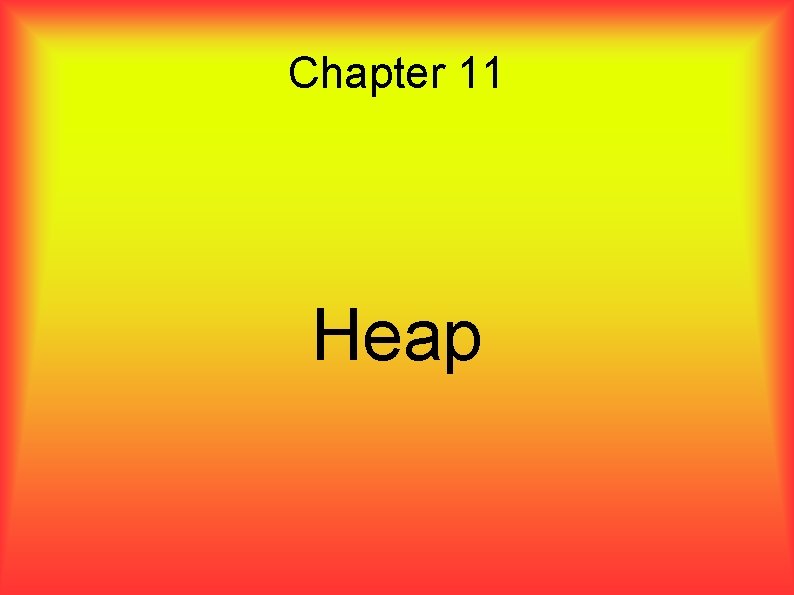
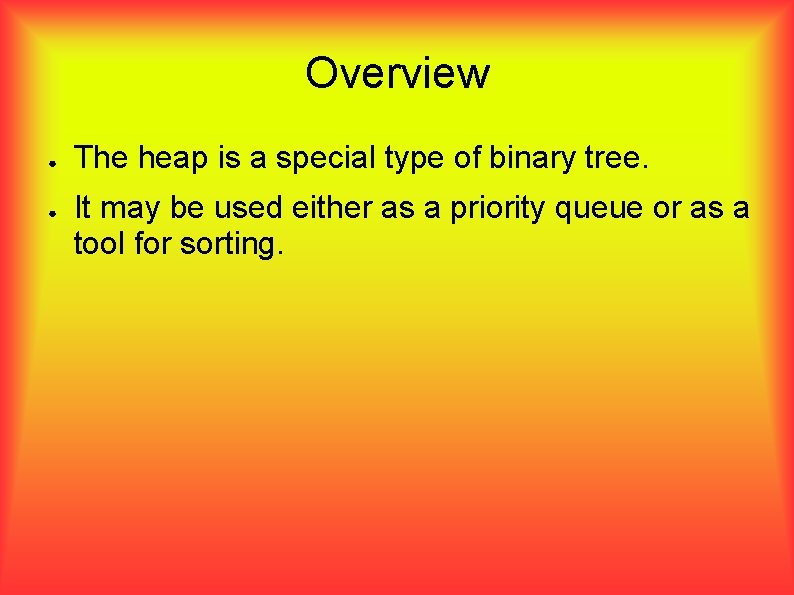
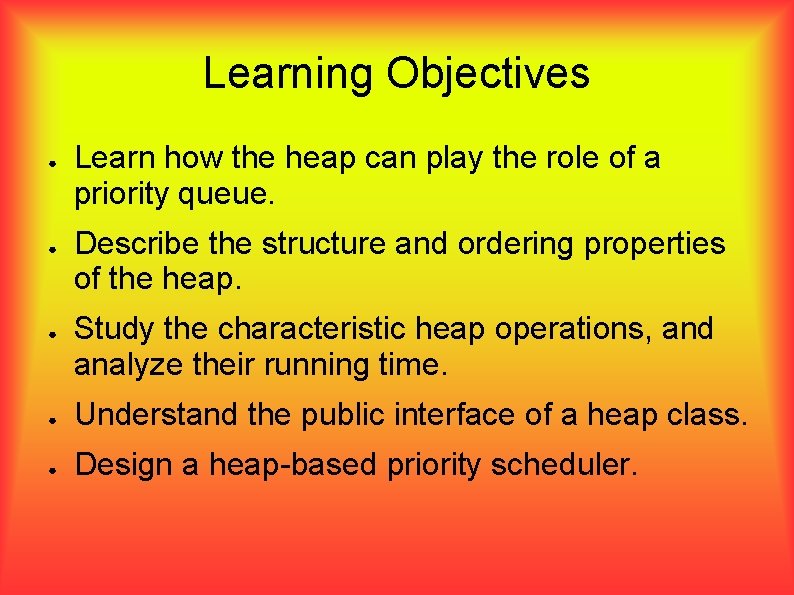
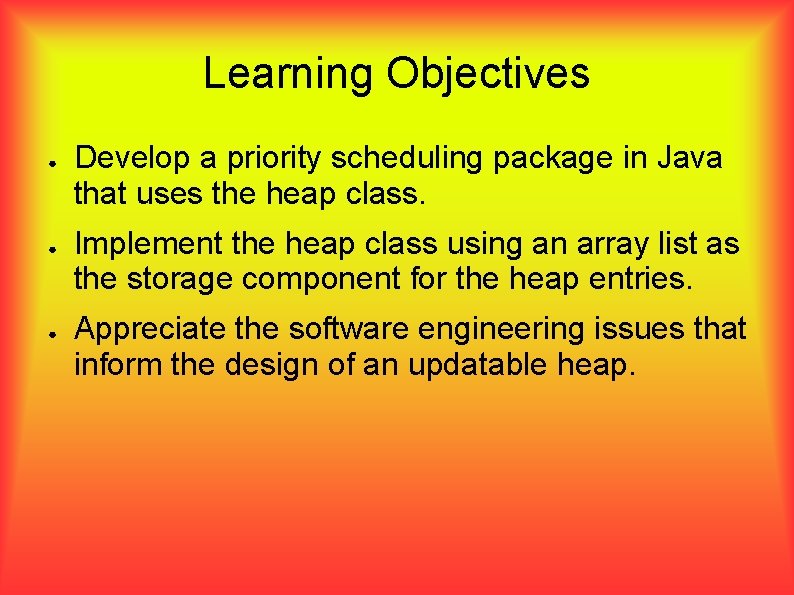
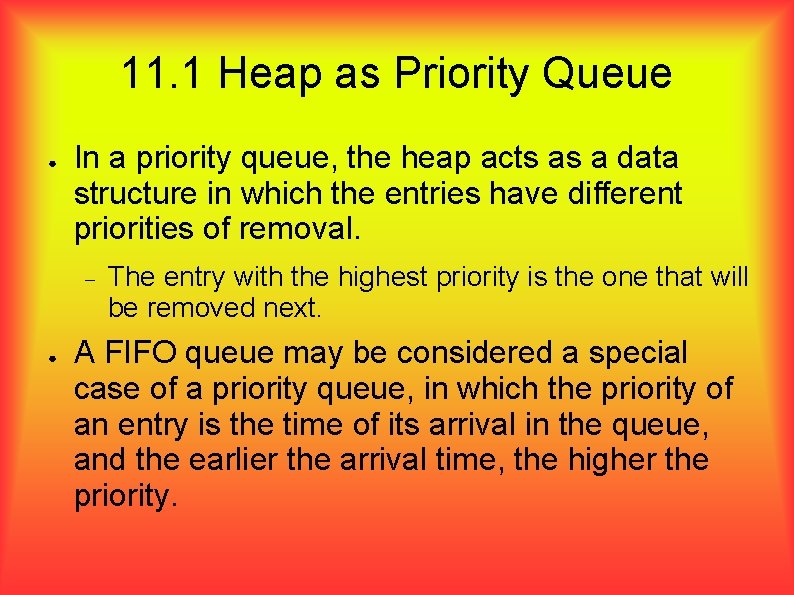
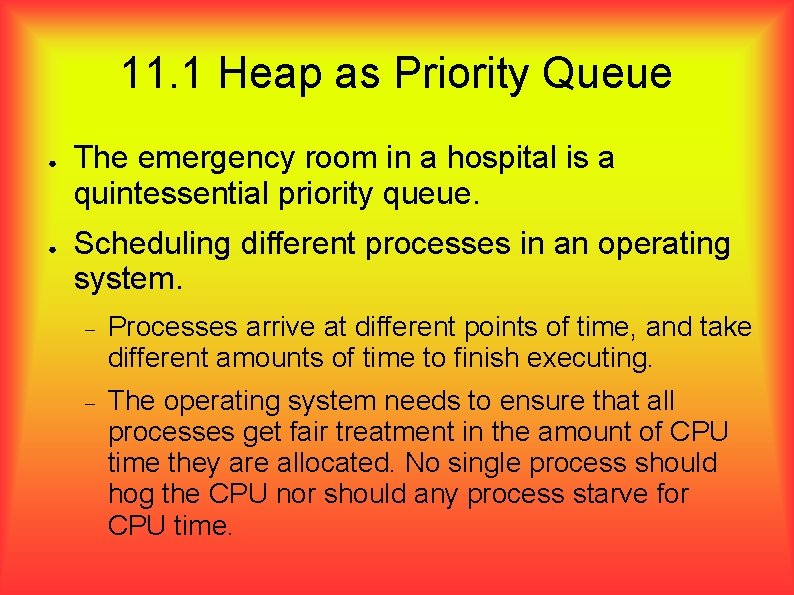
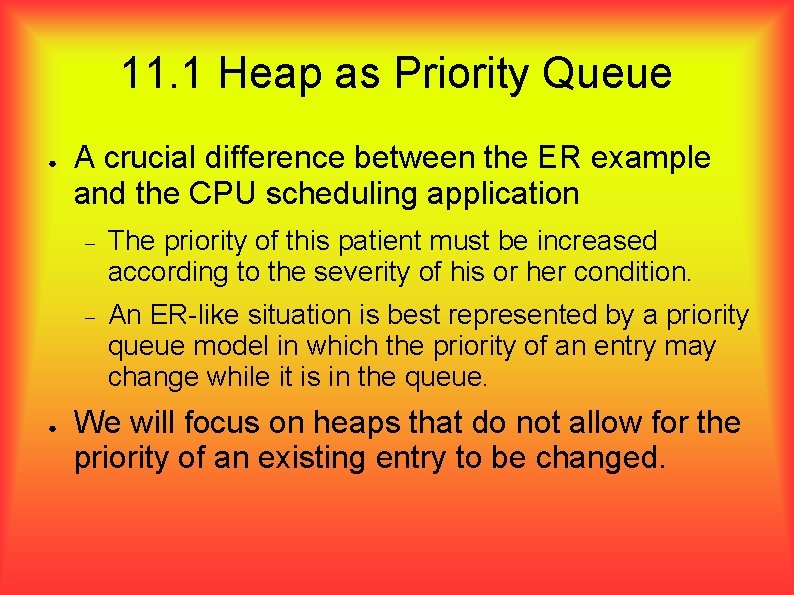
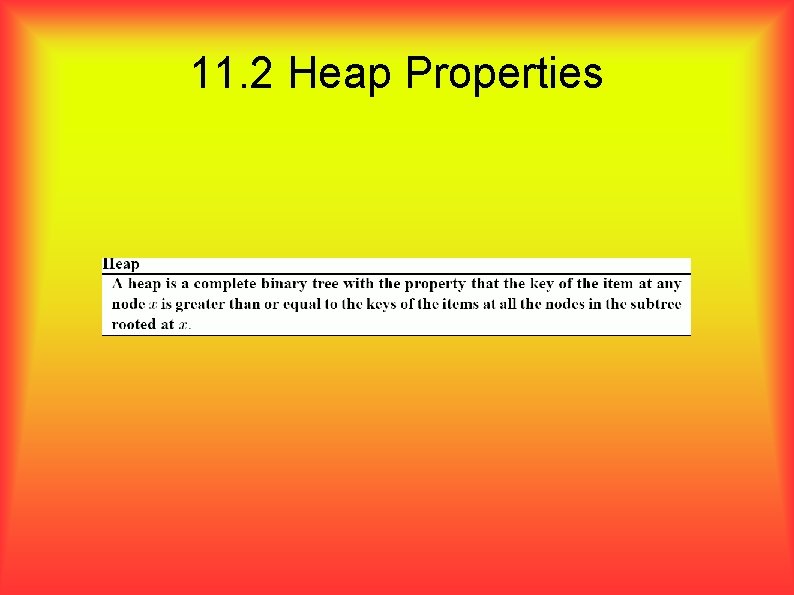
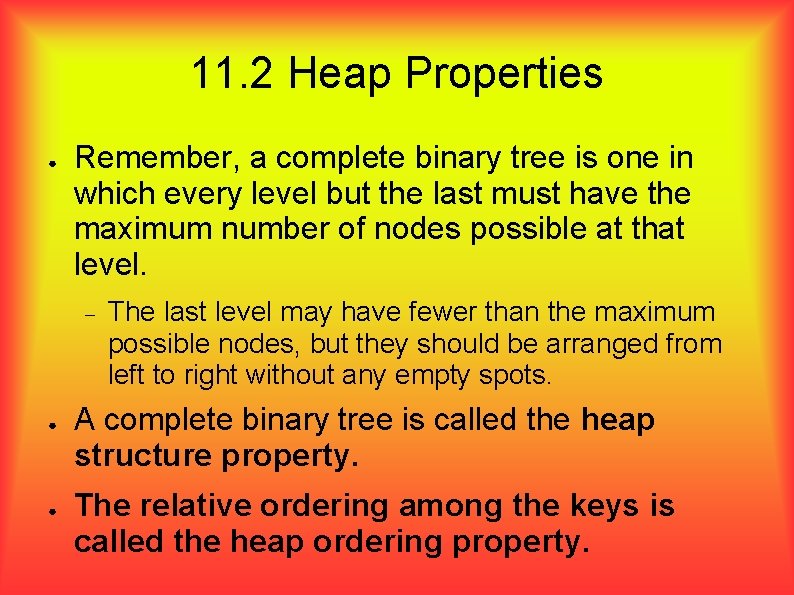
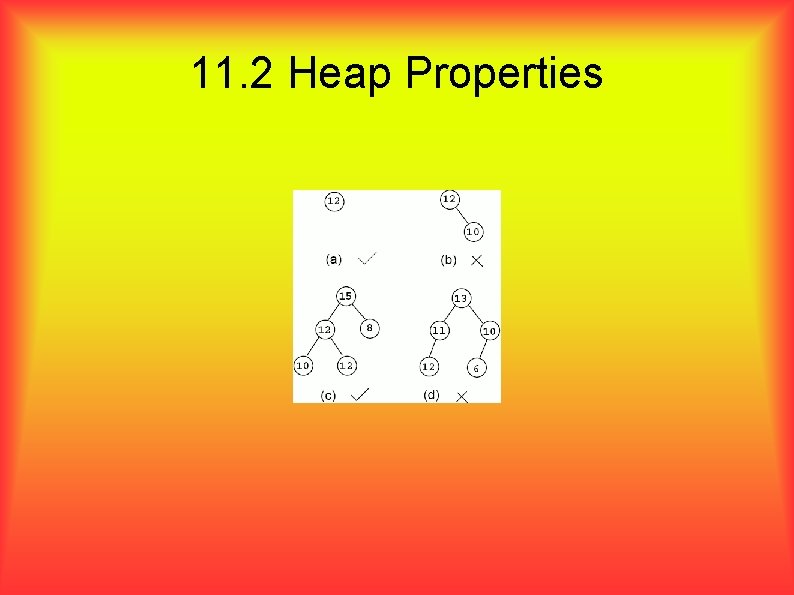
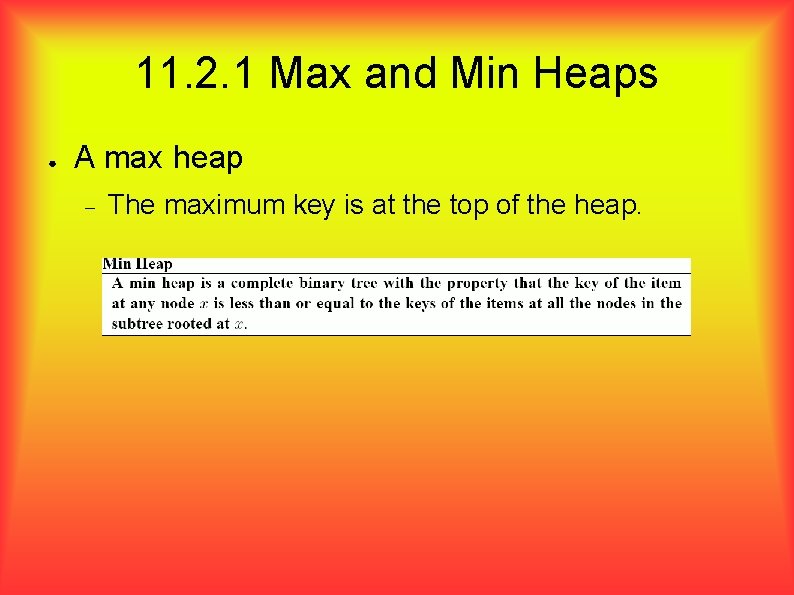
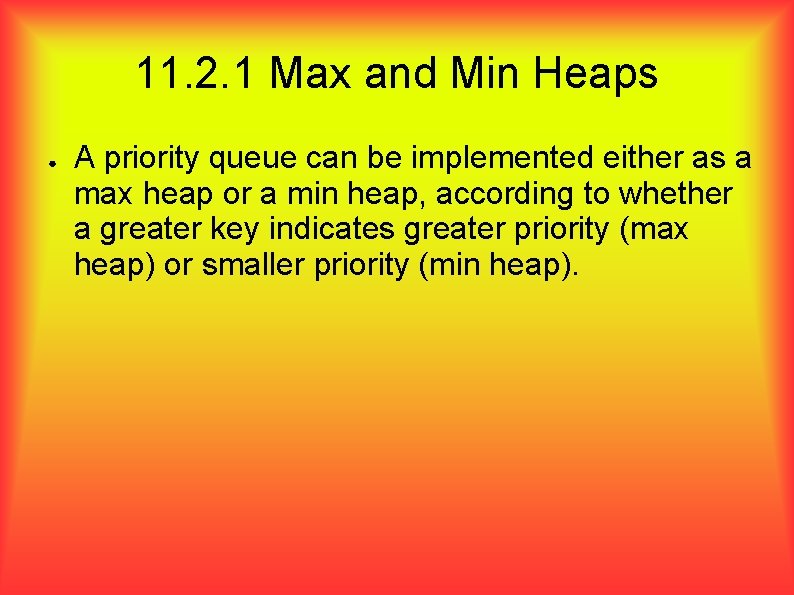
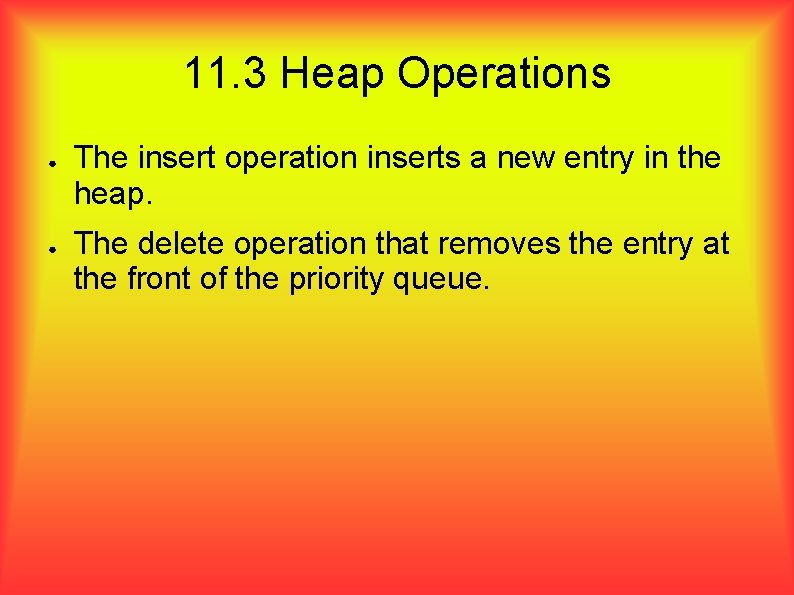
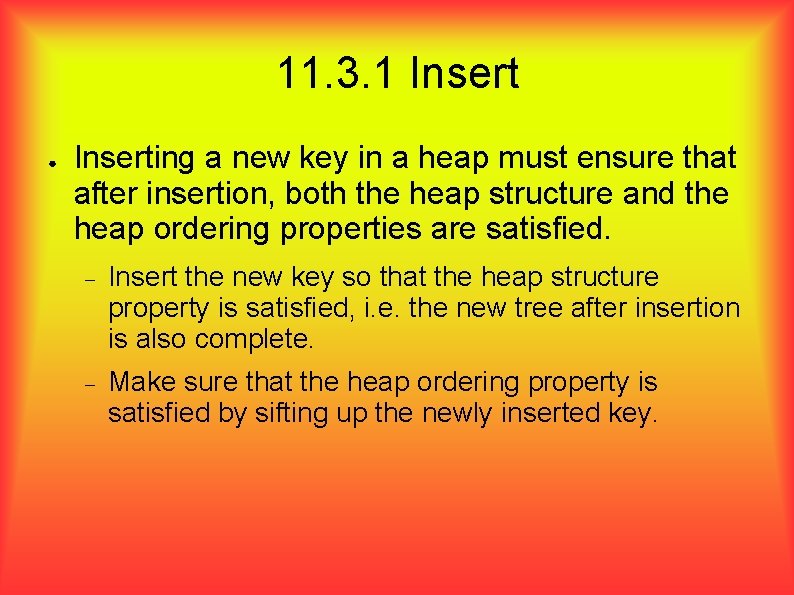
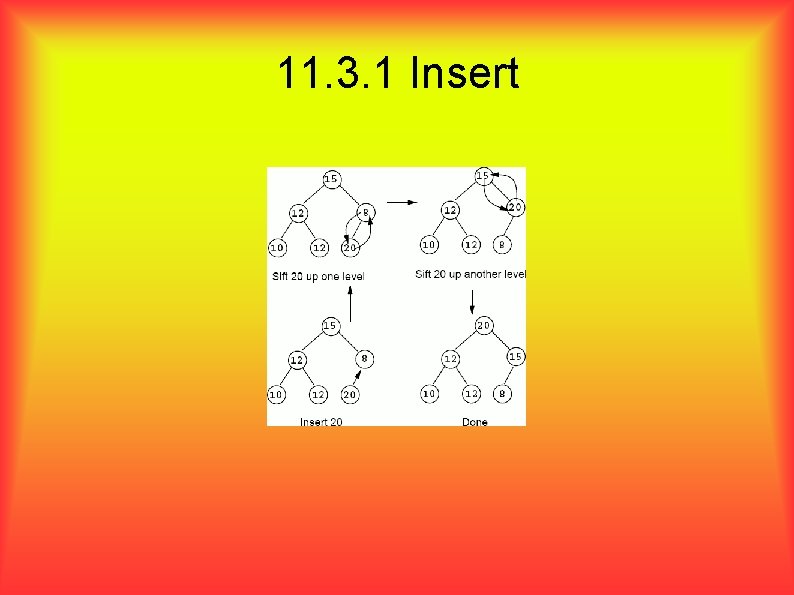
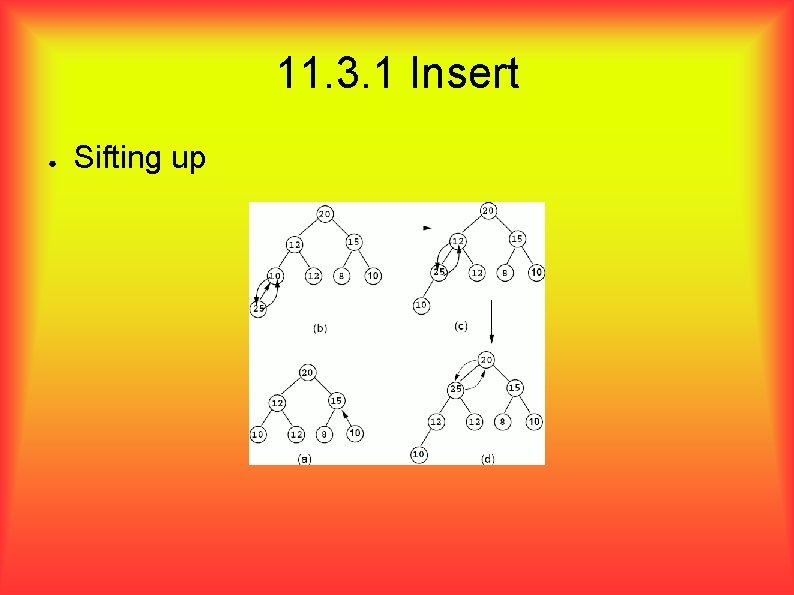
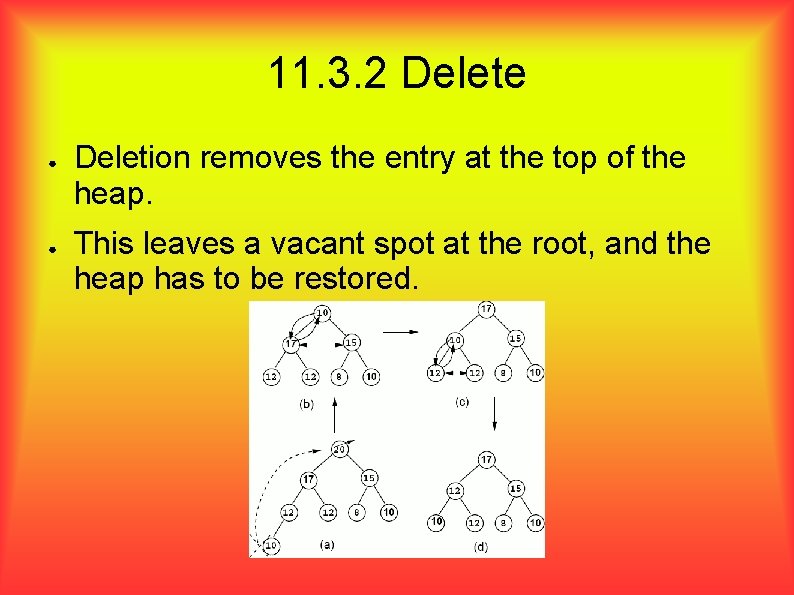
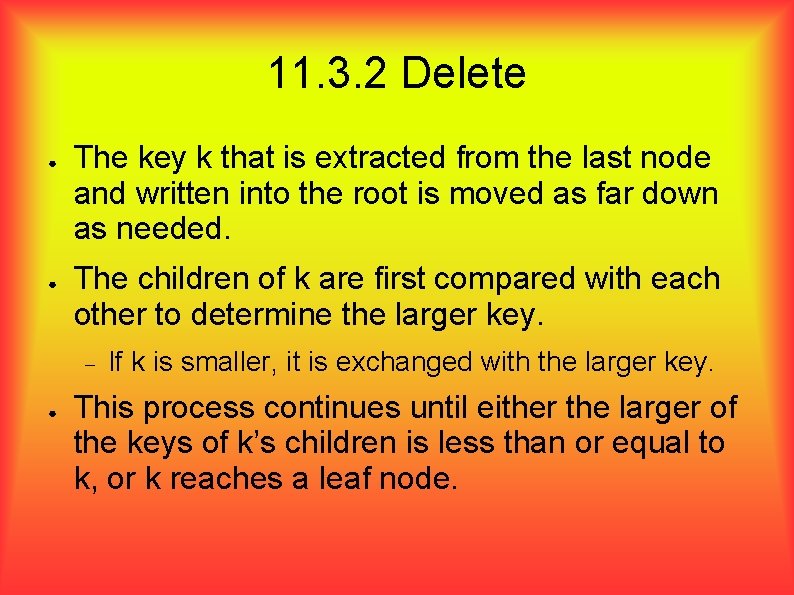
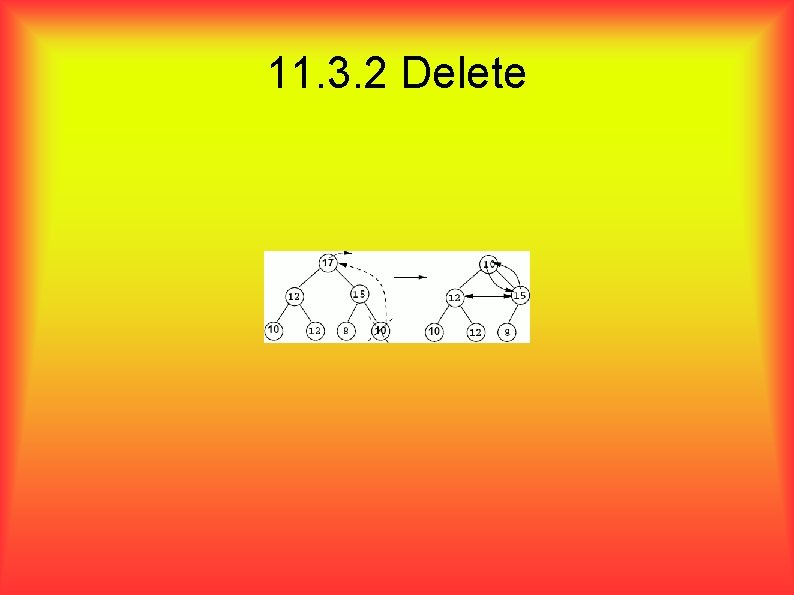
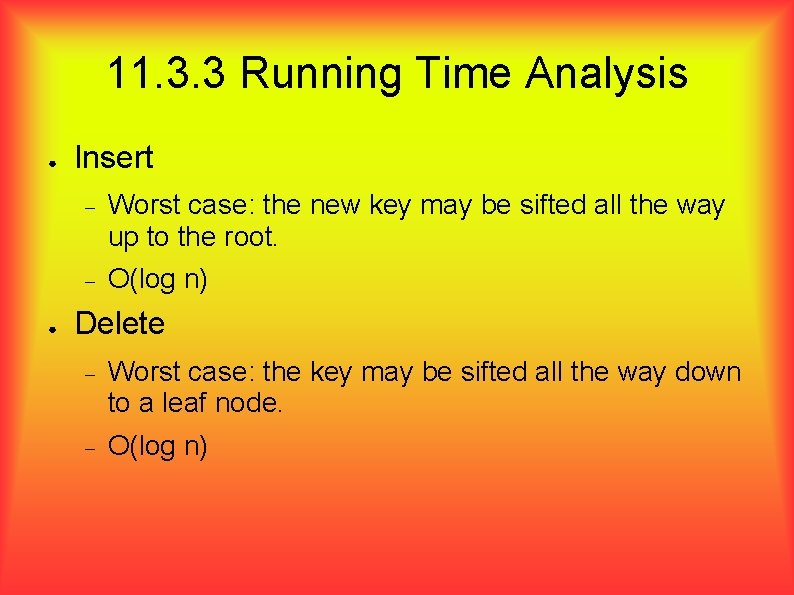
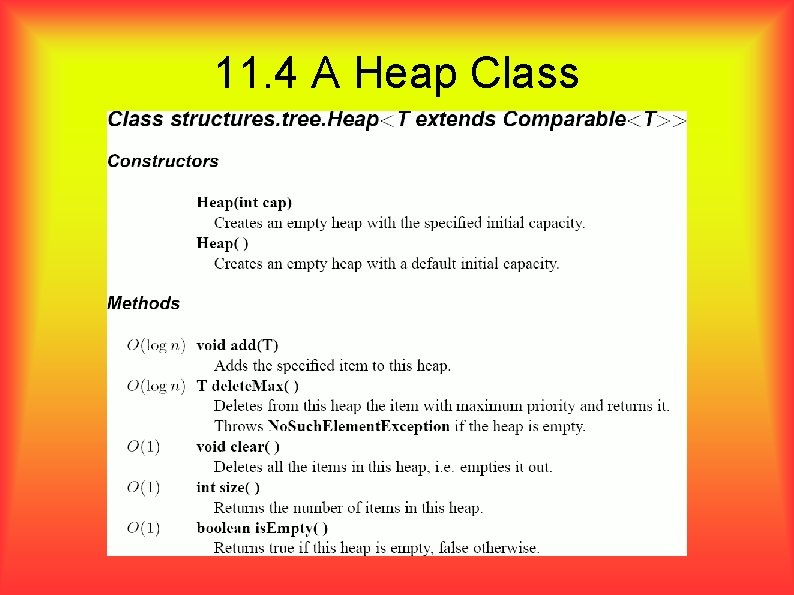
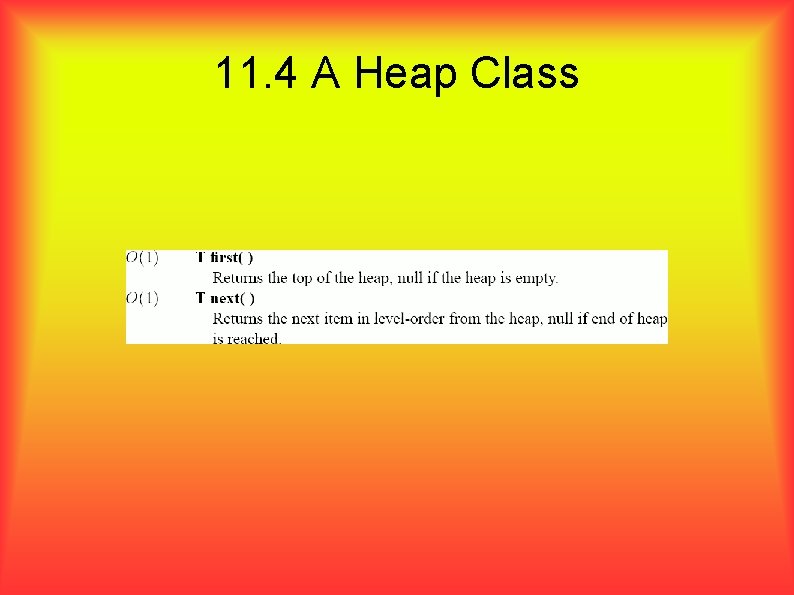
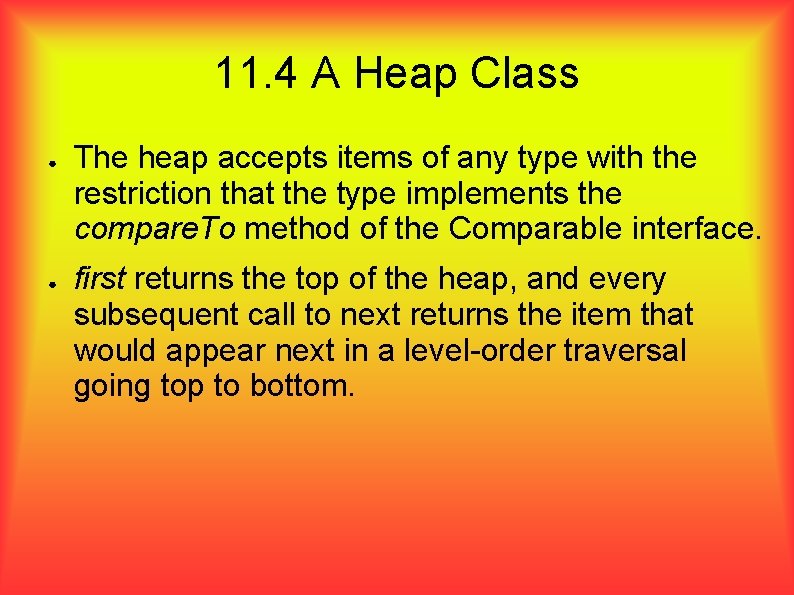
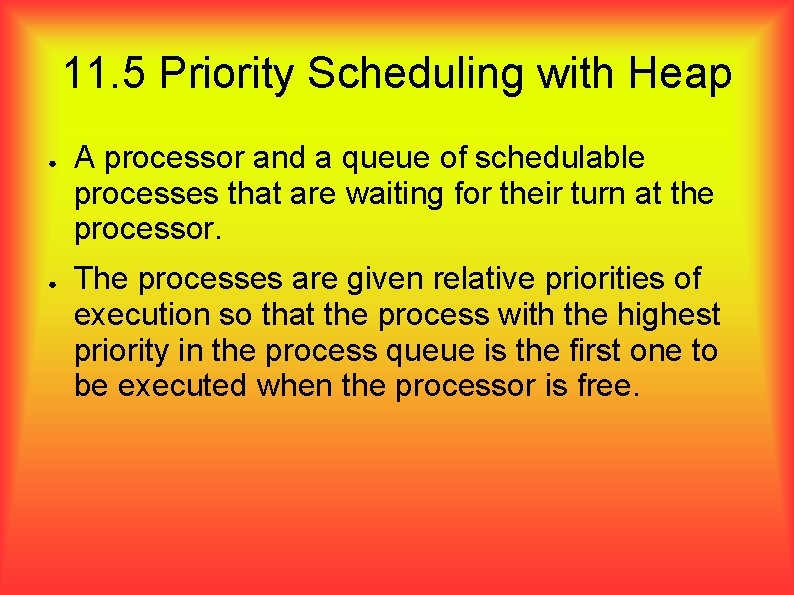
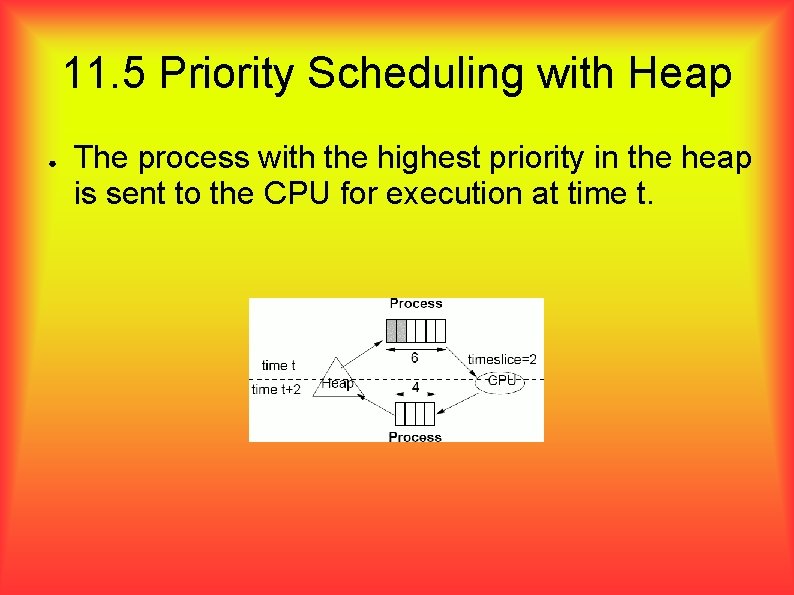
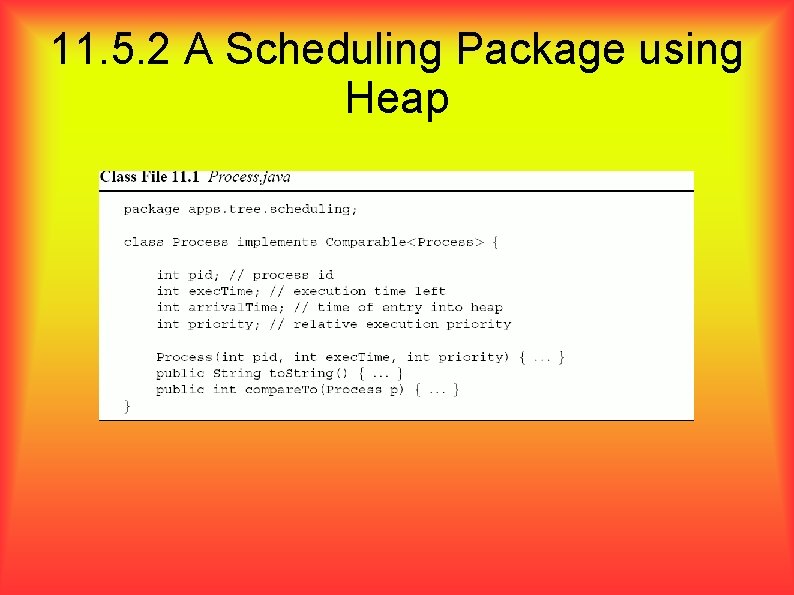
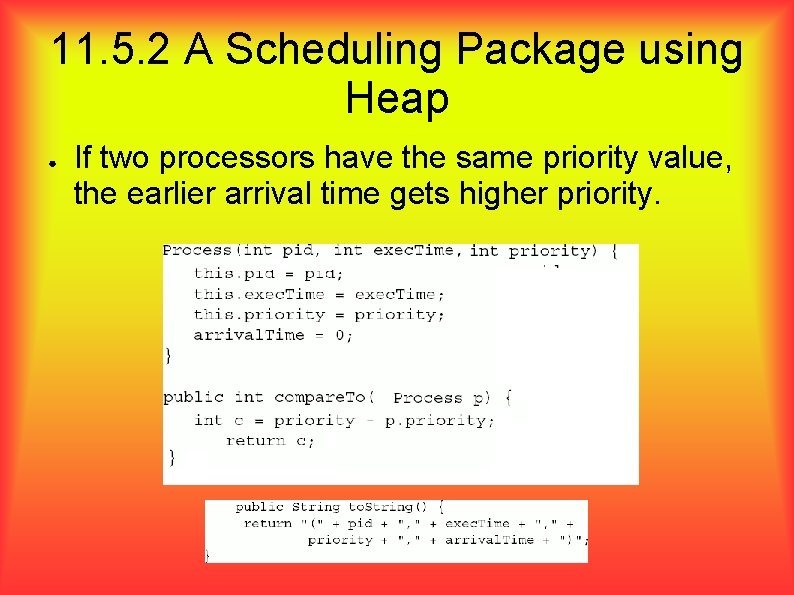
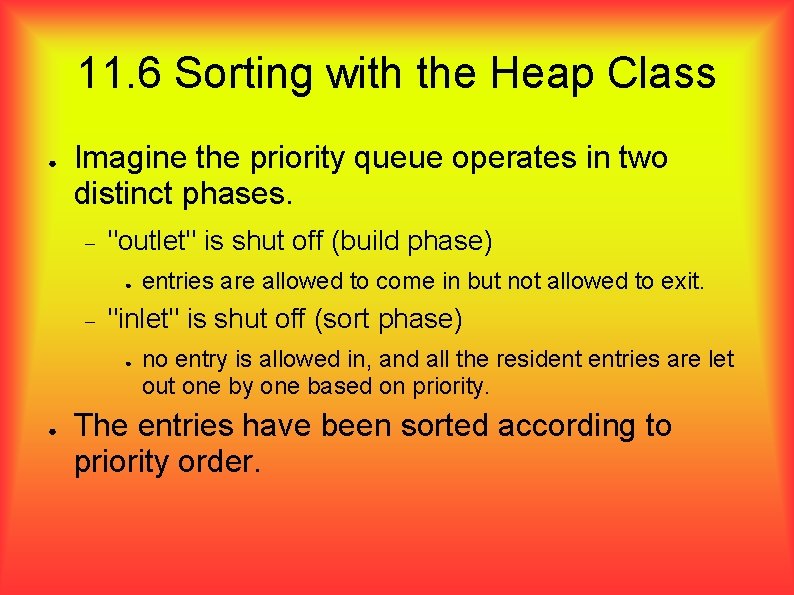
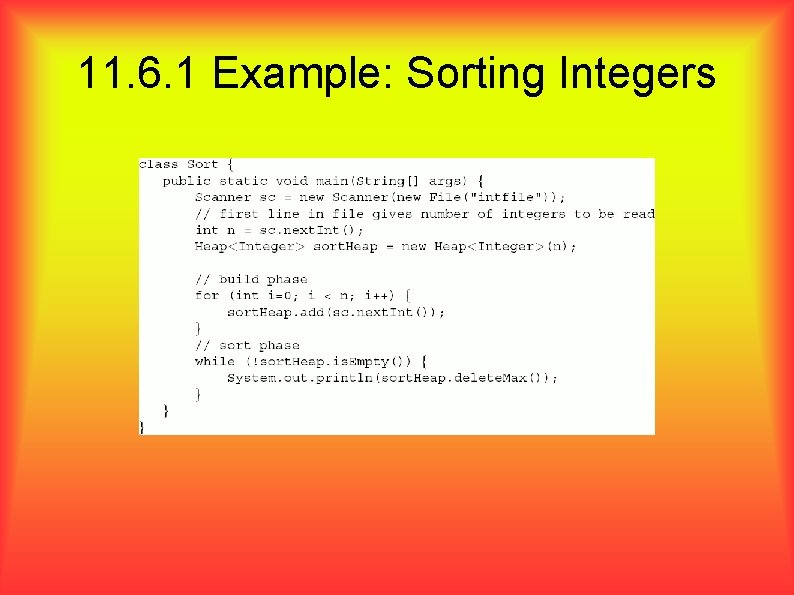
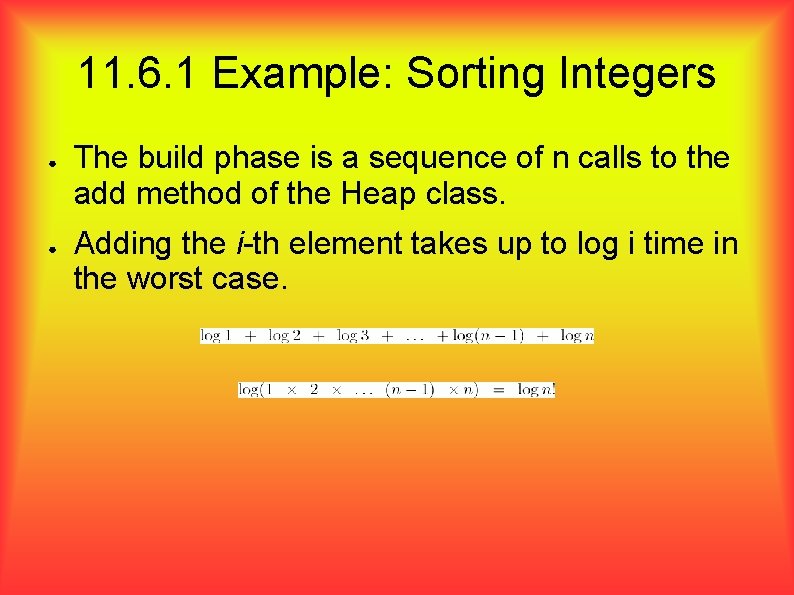
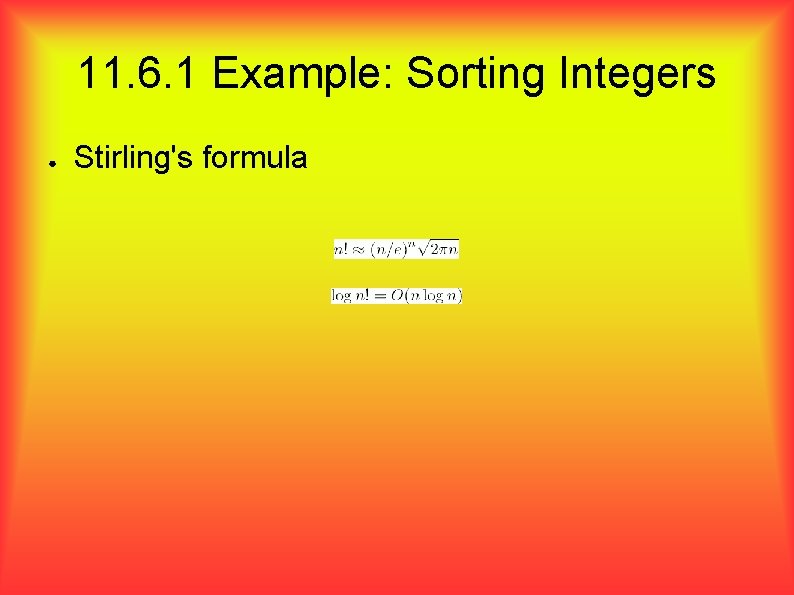
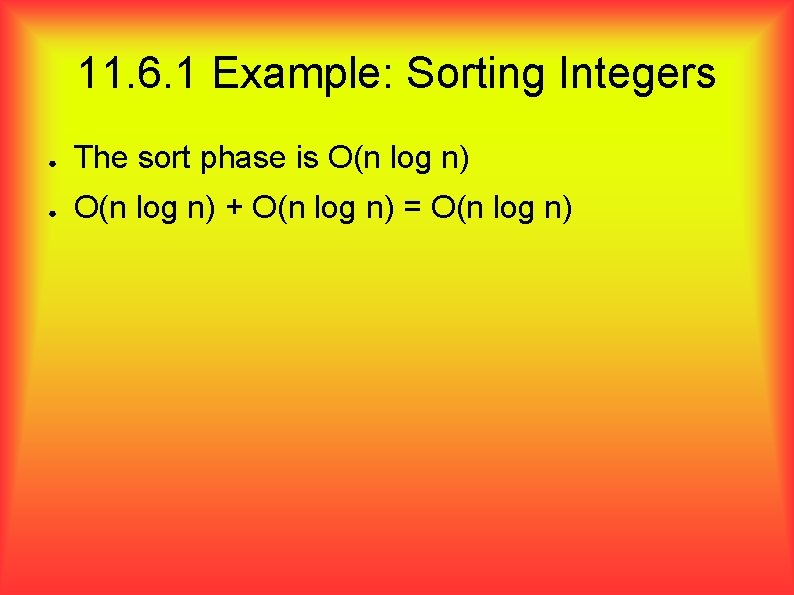
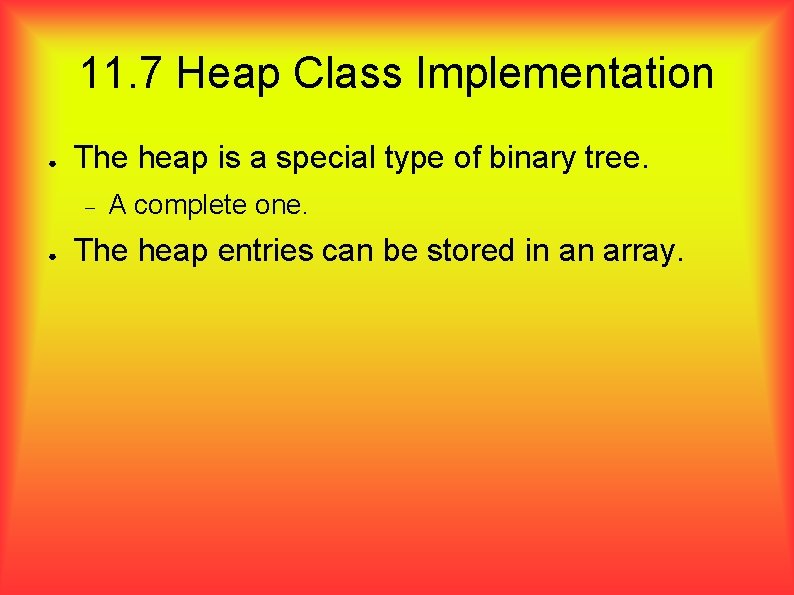
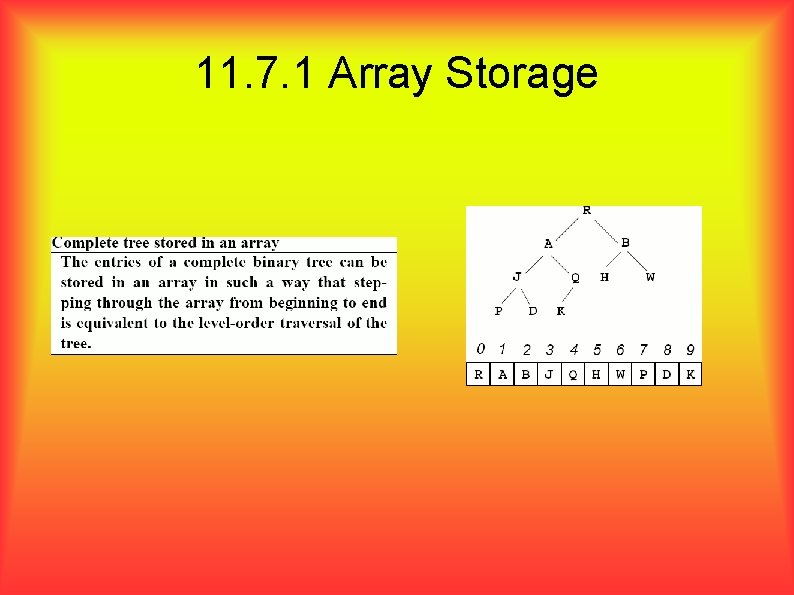
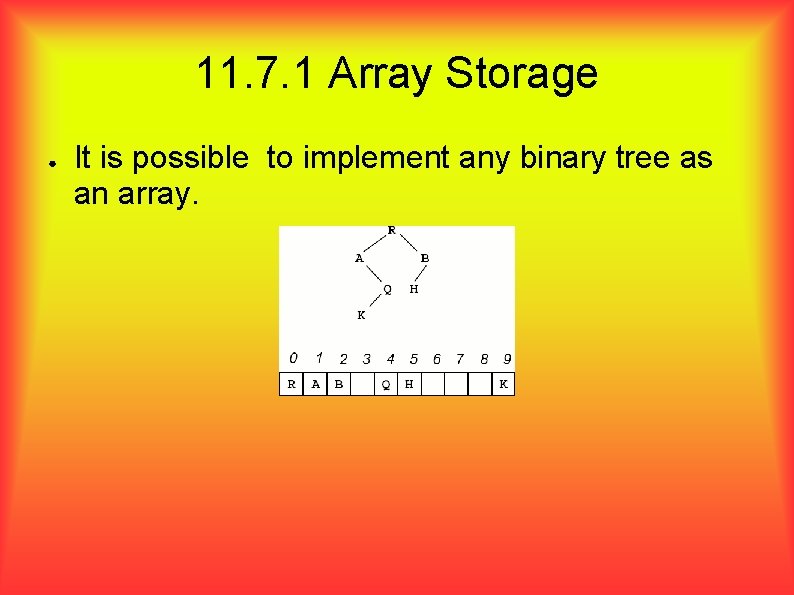
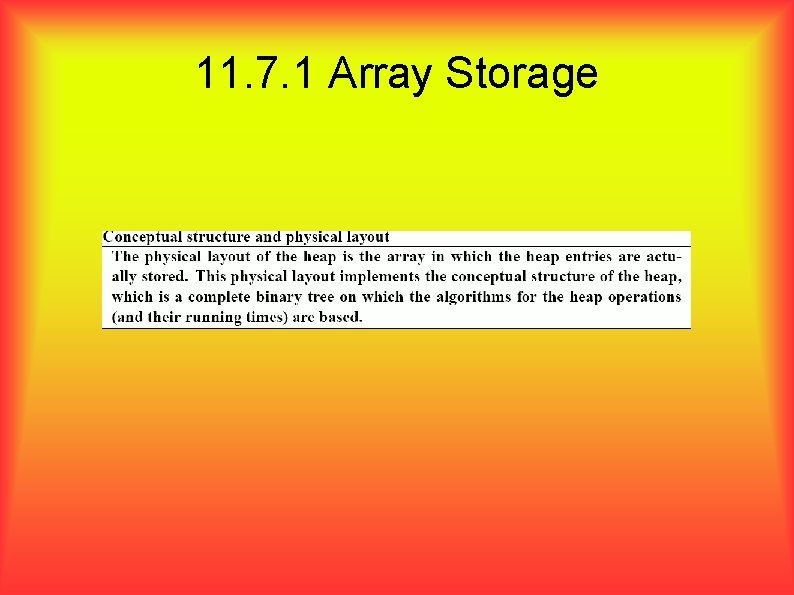
- Slides: 36
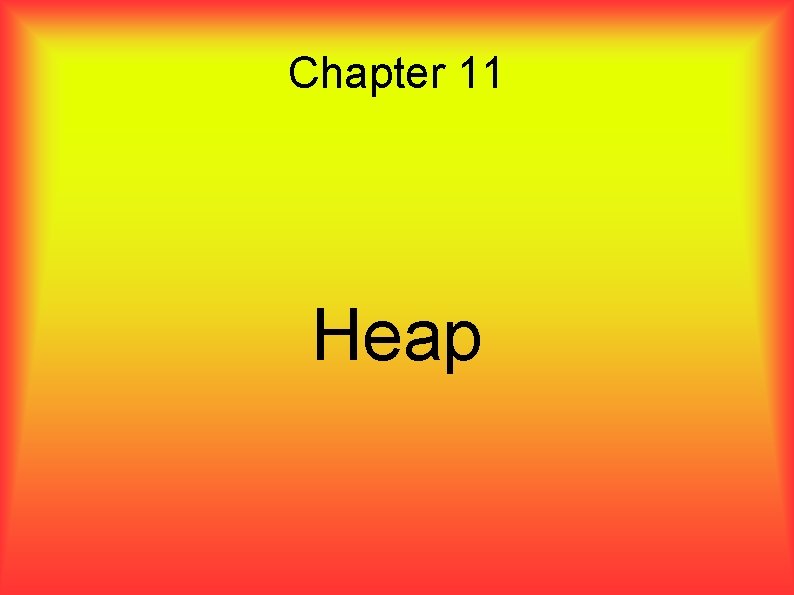
Chapter 11 Heap
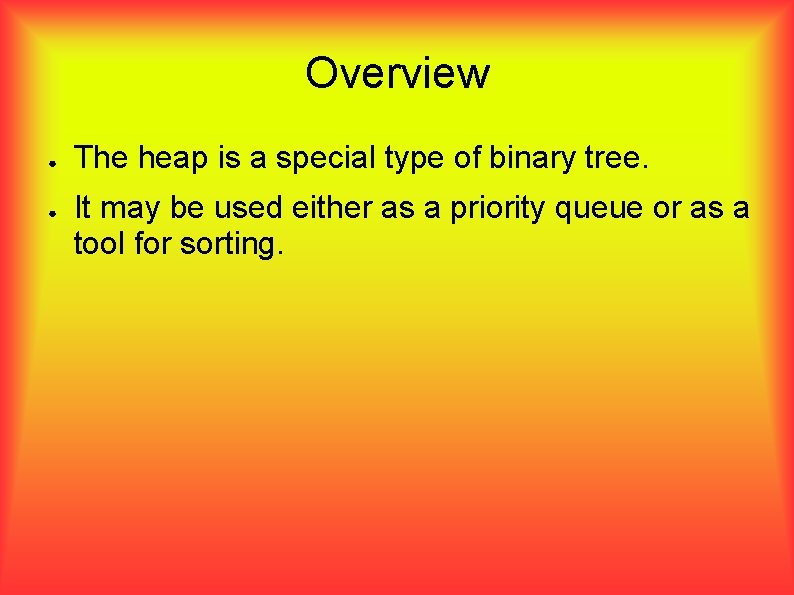
Overview ● ● The heap is a special type of binary tree. It may be used either as a priority queue or as a tool for sorting.
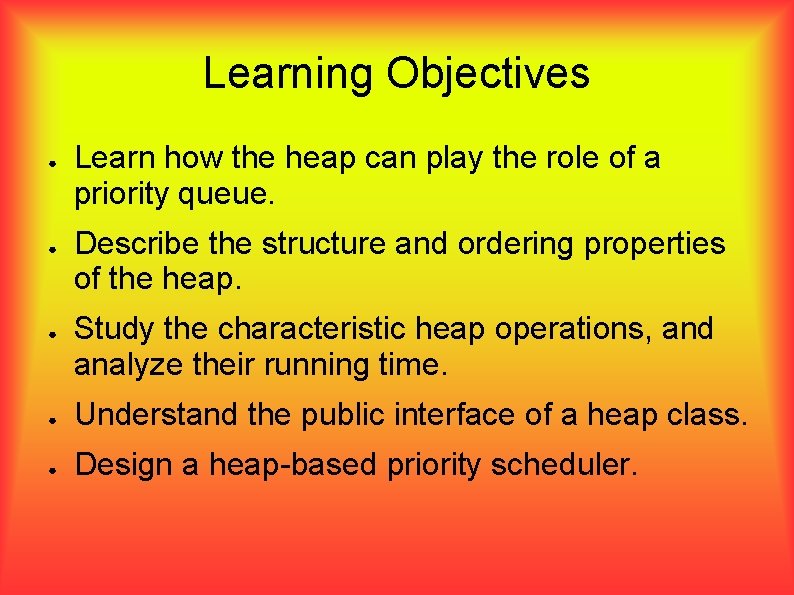
Learning Objectives ● ● ● Learn how the heap can play the role of a priority queue. Describe the structure and ordering properties of the heap. Study the characteristic heap operations, and analyze their running time. ● Understand the public interface of a heap class. ● Design a heap-based priority scheduler.
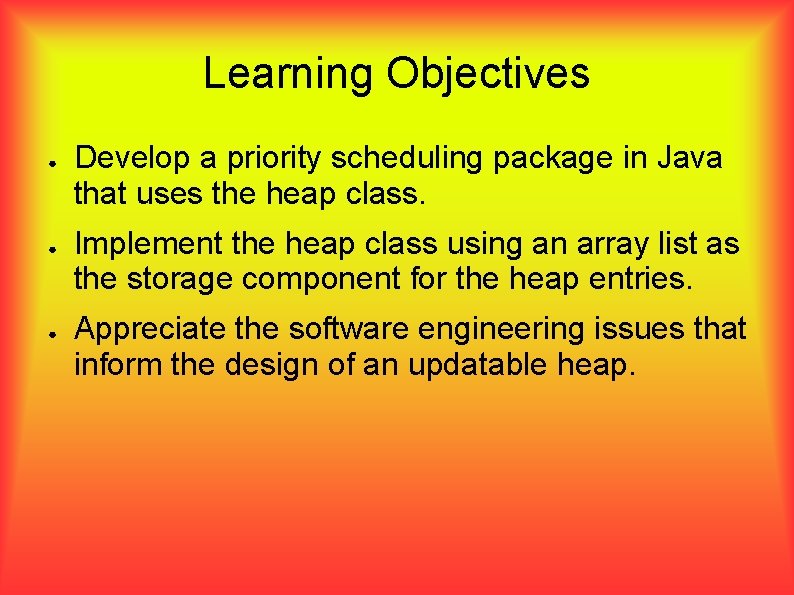
Learning Objectives ● ● ● Develop a priority scheduling package in Java that uses the heap class. Implement the heap class using an array list as the storage component for the heap entries. Appreciate the software engineering issues that inform the design of an updatable heap.
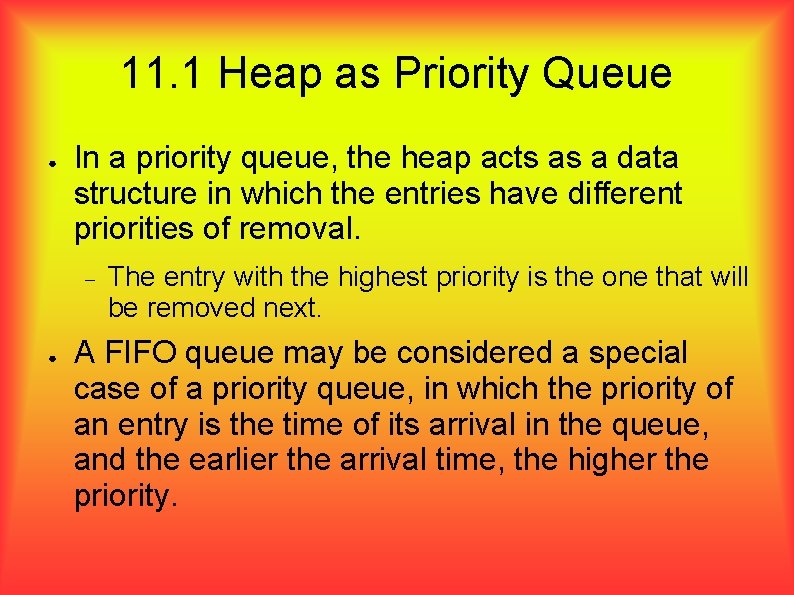
11. 1 Heap as Priority Queue ● In a priority queue, the heap acts as a data structure in which the entries have different priorities of removal. ● The entry with the highest priority is the one that will be removed next. A FIFO queue may be considered a special case of a priority queue, in which the priority of an entry is the time of its arrival in the queue, and the earlier the arrival time, the higher the priority.
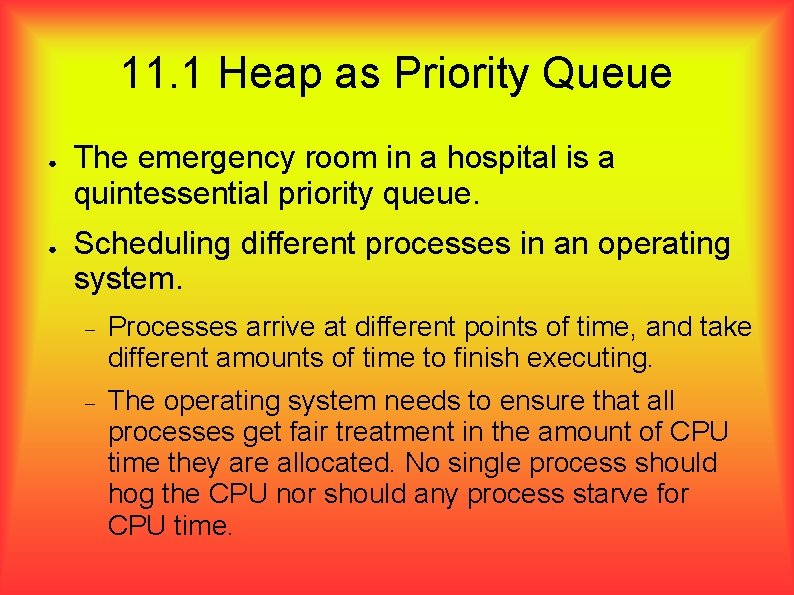
11. 1 Heap as Priority Queue ● ● The emergency room in a hospital is a quintessential priority queue. Scheduling different processes in an operating system. Processes arrive at different points of time, and take different amounts of time to finish executing. The operating system needs to ensure that all processes get fair treatment in the amount of CPU time they are allocated. No single process should hog the CPU nor should any process starve for CPU time.
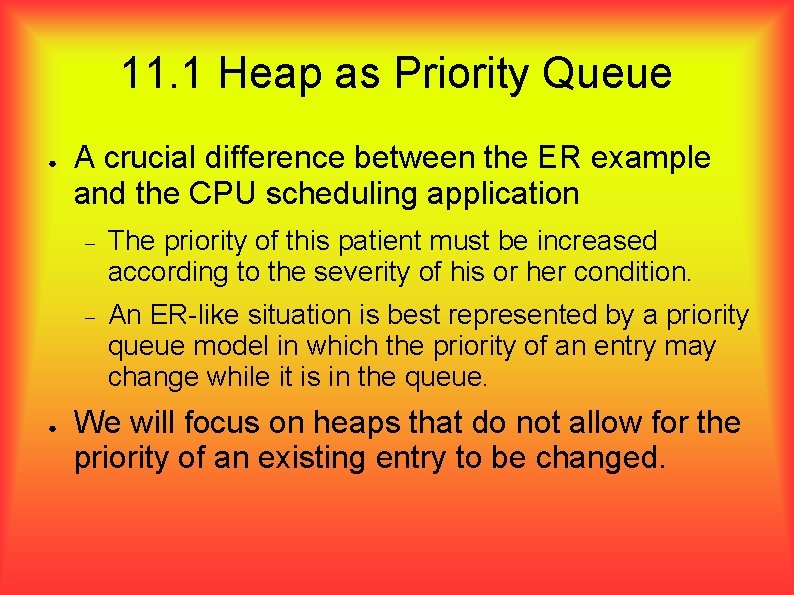
11. 1 Heap as Priority Queue ● ● A crucial difference between the ER example and the CPU scheduling application The priority of this patient must be increased according to the severity of his or her condition. An ER-like situation is best represented by a priority queue model in which the priority of an entry may change while it is in the queue. We will focus on heaps that do not allow for the priority of an existing entry to be changed.
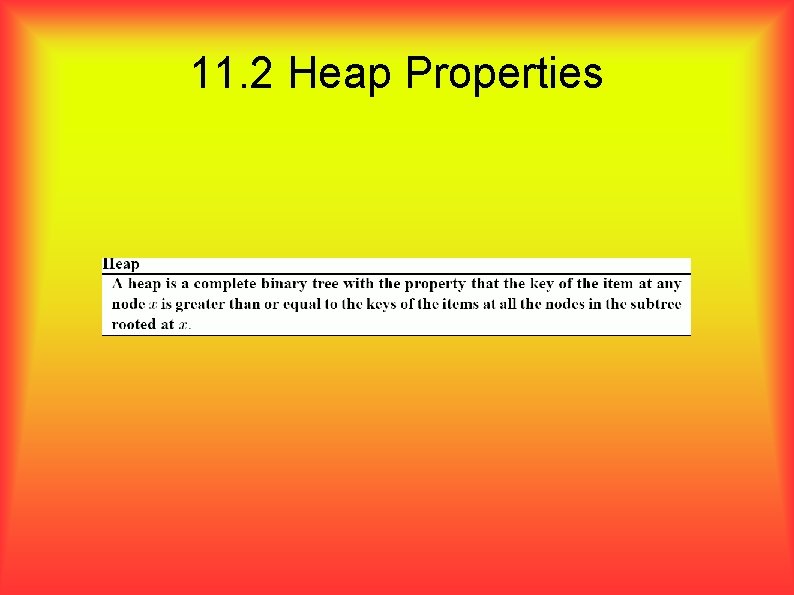
11. 2 Heap Properties
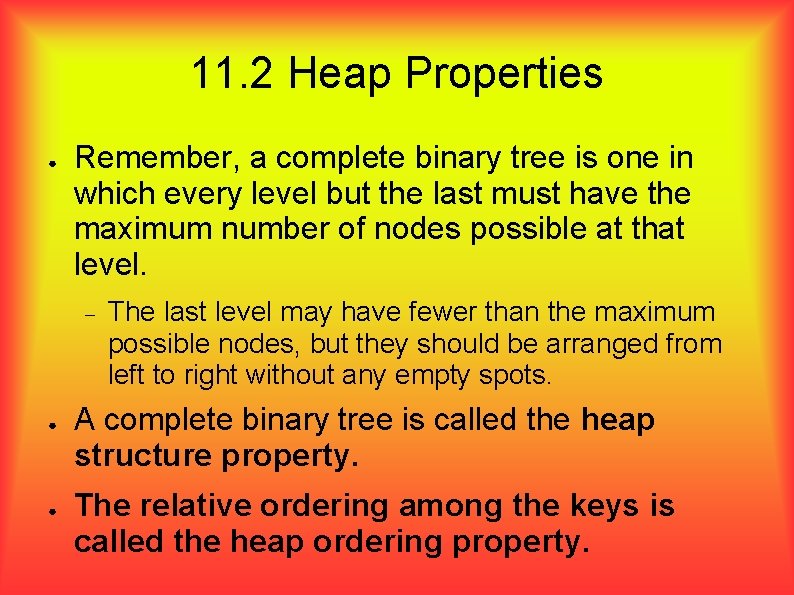
11. 2 Heap Properties ● Remember, a complete binary tree is one in which every level but the last must have the maximum number of nodes possible at that level. ● ● The last level may have fewer than the maximum possible nodes, but they should be arranged from left to right without any empty spots. A complete binary tree is called the heap structure property. The relative ordering among the keys is called the heap ordering property.
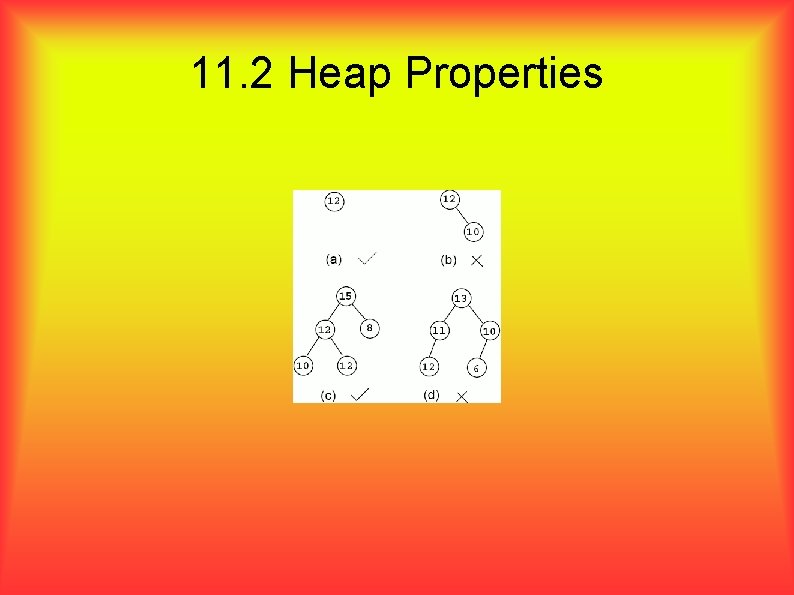
11. 2 Heap Properties
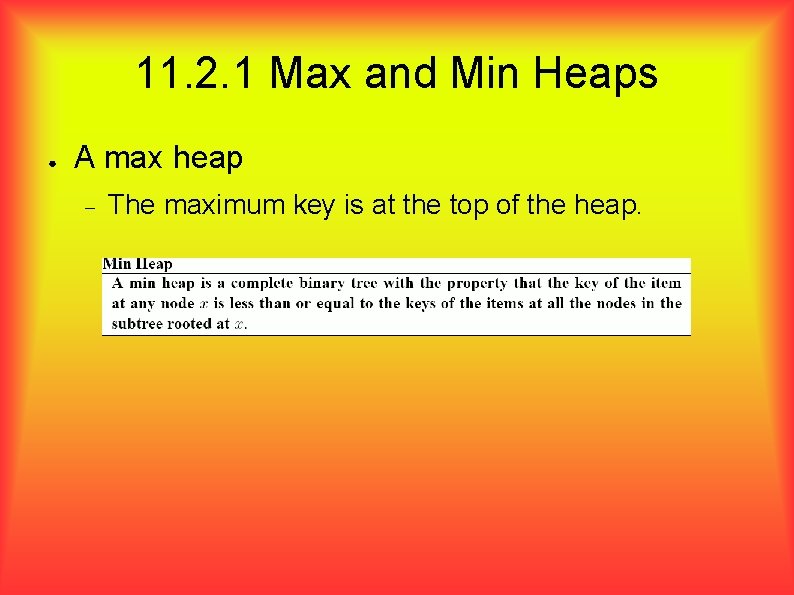
11. 2. 1 Max and Min Heaps ● A max heap The maximum key is at the top of the heap.
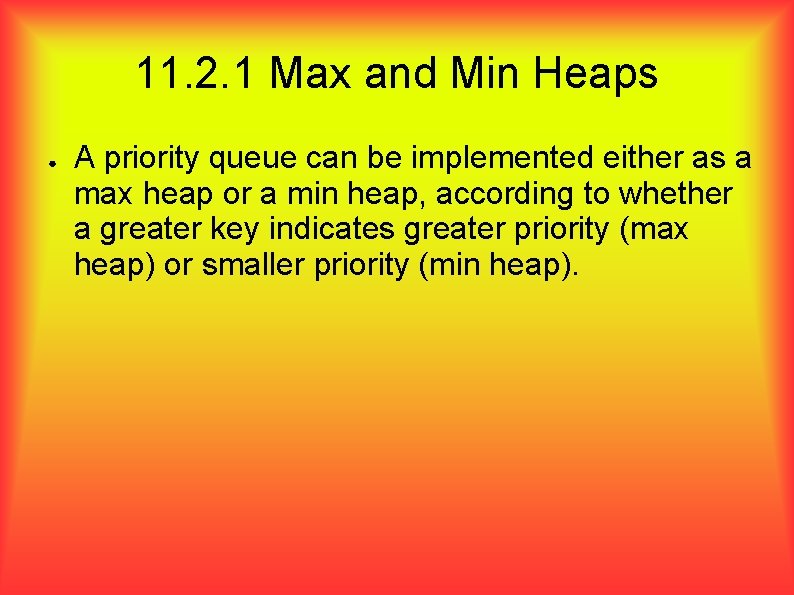
11. 2. 1 Max and Min Heaps ● A priority queue can be implemented either as a max heap or a min heap, according to whether a greater key indicates greater priority (max heap) or smaller priority (min heap).
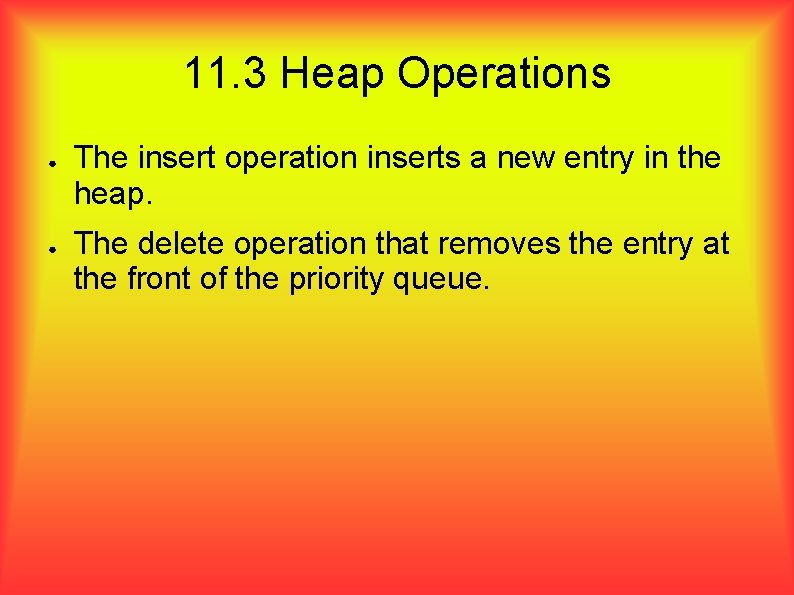
11. 3 Heap Operations ● ● The insert operation inserts a new entry in the heap. The delete operation that removes the entry at the front of the priority queue.
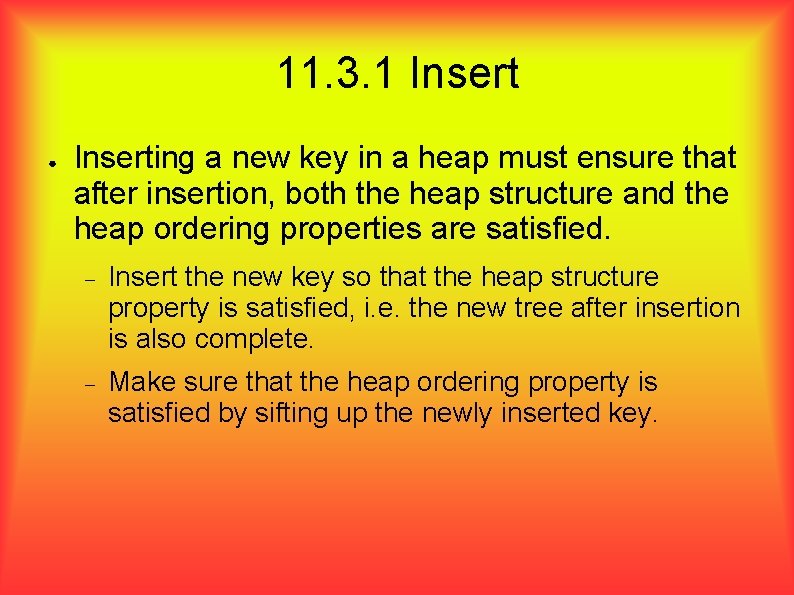
11. 3. 1 Insert ● Inserting a new key in a heap must ensure that after insertion, both the heap structure and the heap ordering properties are satisfied. Insert the new key so that the heap structure property is satisfied, i. e. the new tree after insertion is also complete. Make sure that the heap ordering property is satisfied by sifting up the newly inserted key.
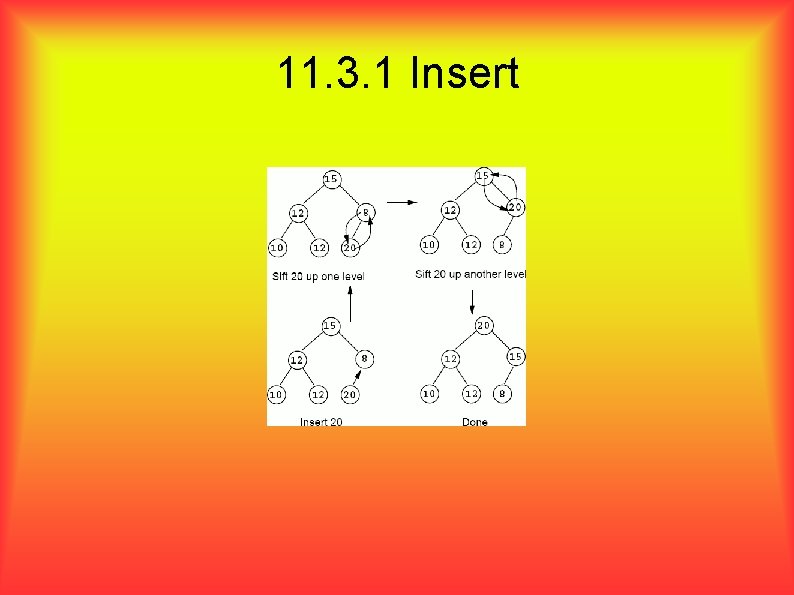
11. 3. 1 Insert
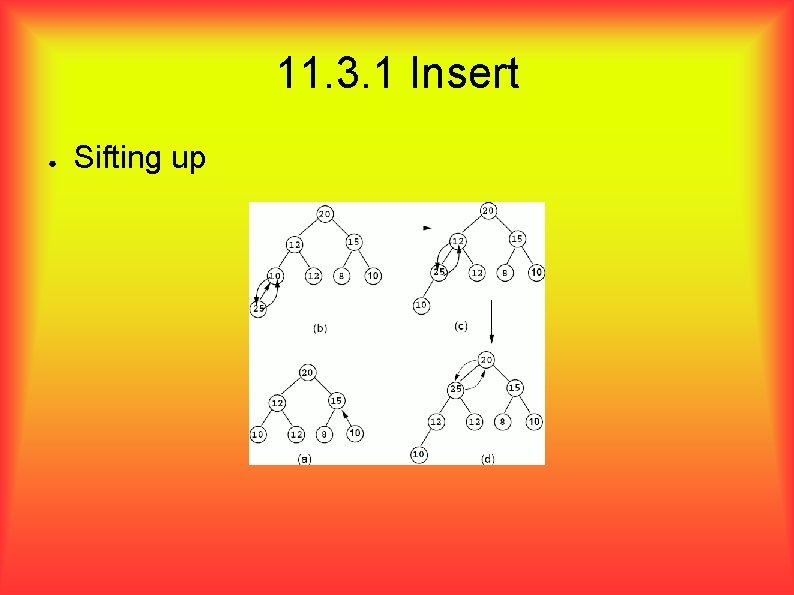
11. 3. 1 Insert ● Sifting up
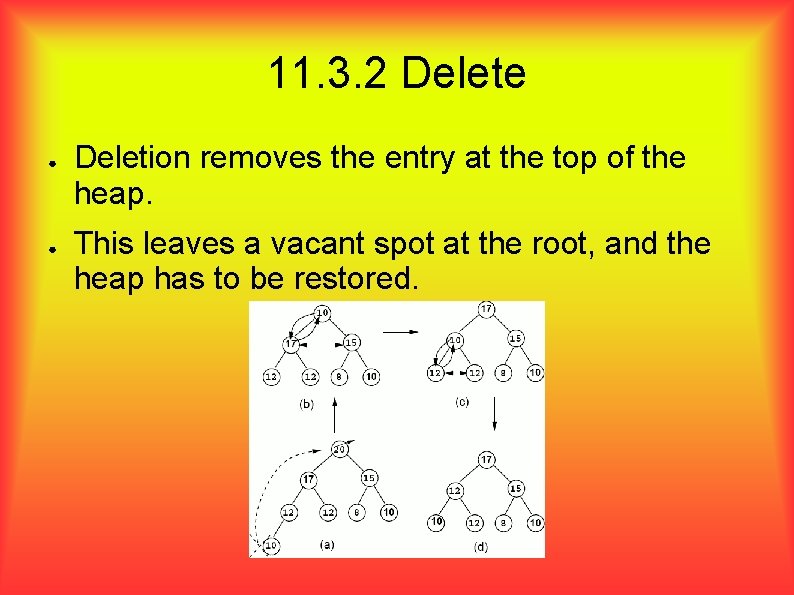
11. 3. 2 Delete ● ● Deletion removes the entry at the top of the heap. This leaves a vacant spot at the root, and the heap has to be restored.
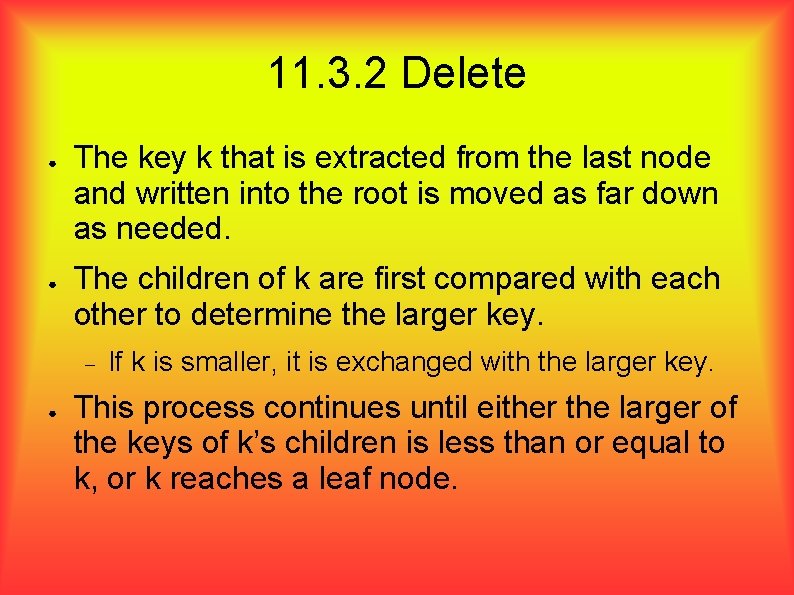
11. 3. 2 Delete ● ● The key k that is extracted from the last node and written into the root is moved as far down as needed. The children of k are first compared with each other to determine the larger key. ● If k is smaller, it is exchanged with the larger key. This process continues until either the larger of the keys of k’s children is less than or equal to k, or k reaches a leaf node.
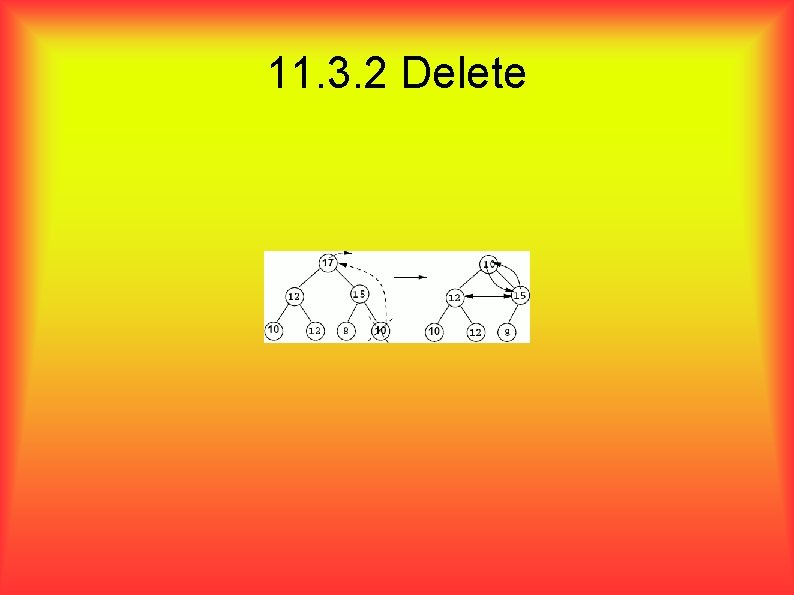
11. 3. 2 Delete
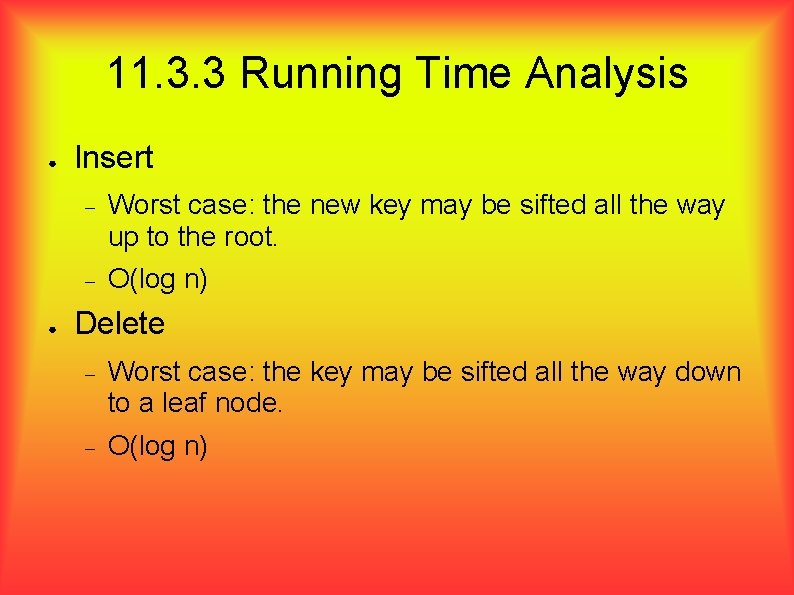
11. 3. 3 Running Time Analysis ● ● Insert Worst case: the new key may be sifted all the way up to the root. O(log n) Delete Worst case: the key may be sifted all the way down to a leaf node. O(log n)
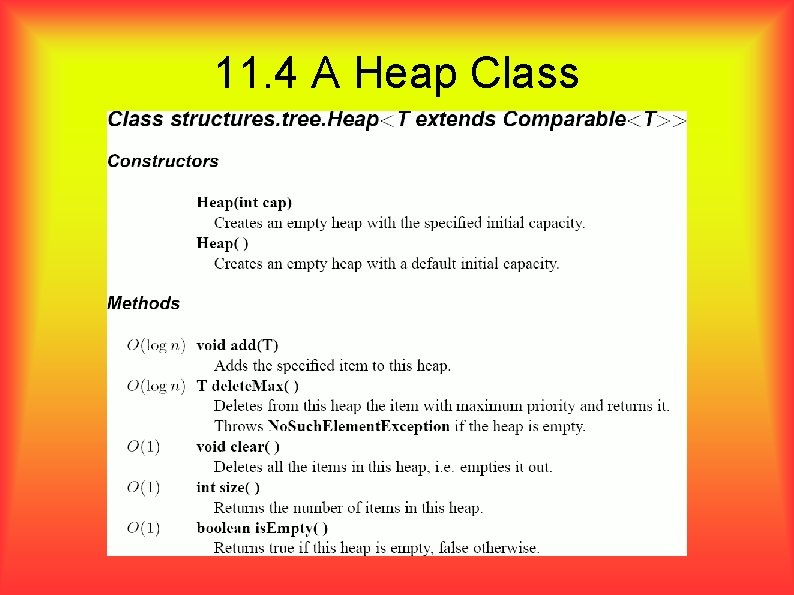
11. 4 A Heap Class
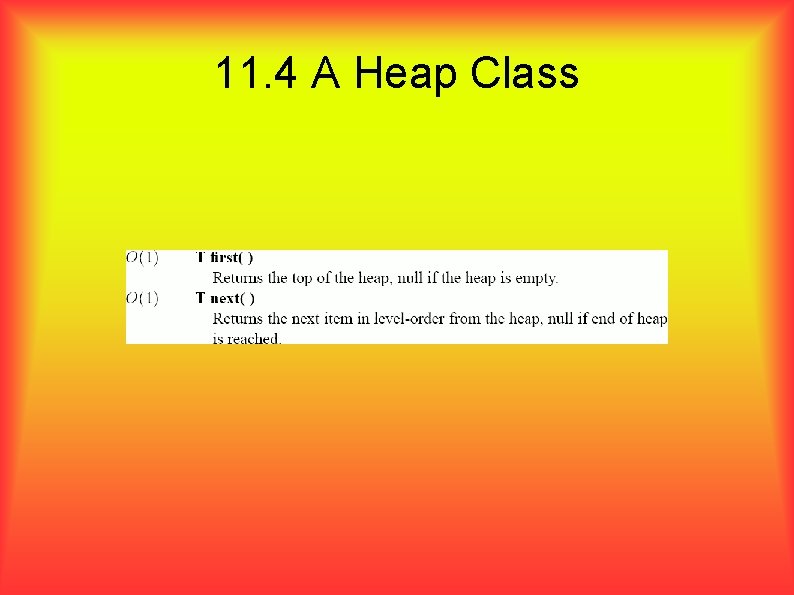
11. 4 A Heap Class
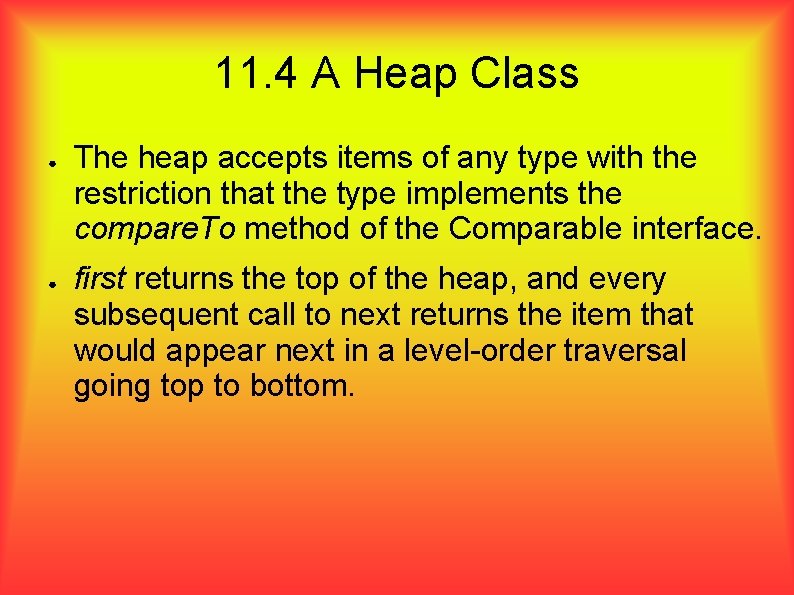
11. 4 A Heap Class ● ● The heap accepts items of any type with the restriction that the type implements the compare. To method of the Comparable interface. first returns the top of the heap, and every subsequent call to next returns the item that would appear next in a level-order traversal going top to bottom.
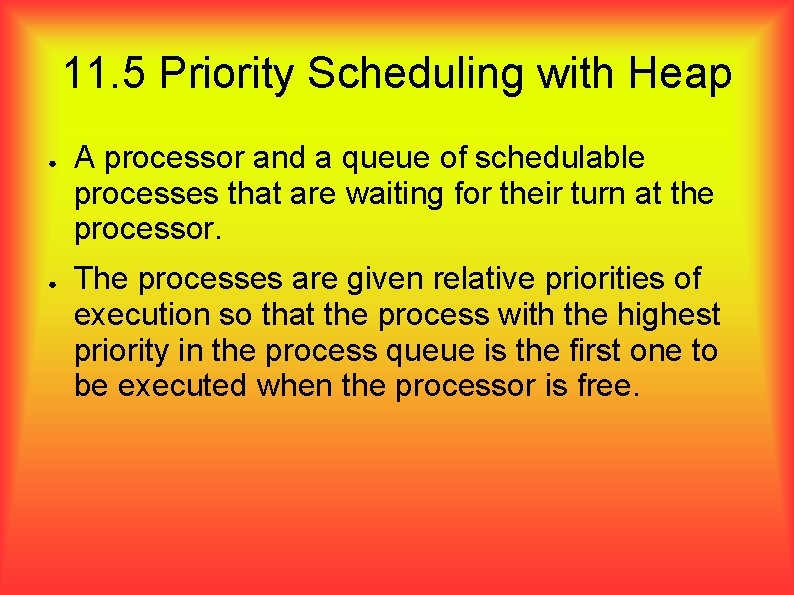
11. 5 Priority Scheduling with Heap ● ● A processor and a queue of schedulable processes that are waiting for their turn at the processor. The processes are given relative priorities of execution so that the process with the highest priority in the process queue is the first one to be executed when the processor is free.
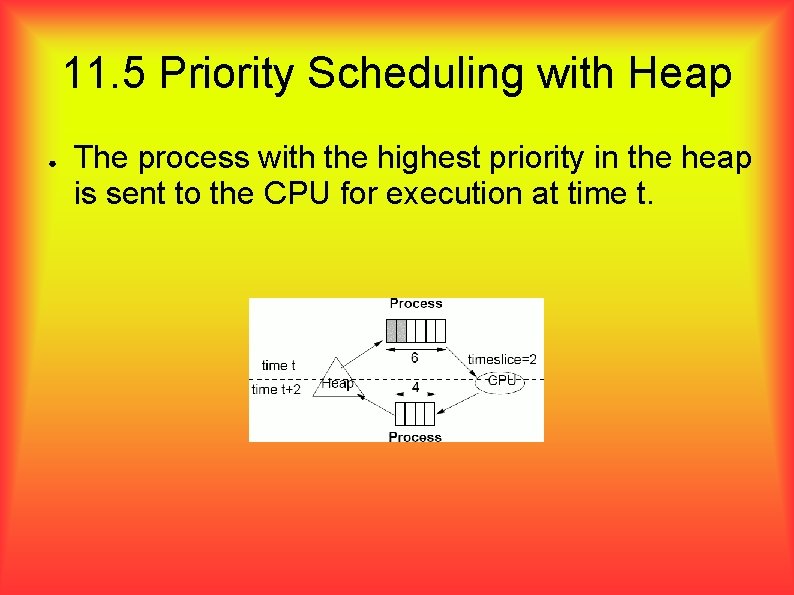
11. 5 Priority Scheduling with Heap ● The process with the highest priority in the heap is sent to the CPU for execution at time t.
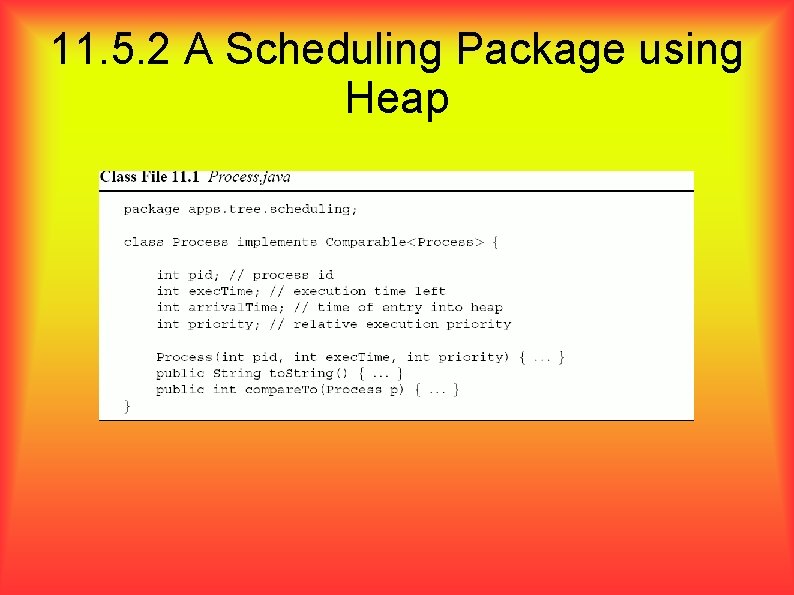
11. 5. 2 A Scheduling Package using Heap
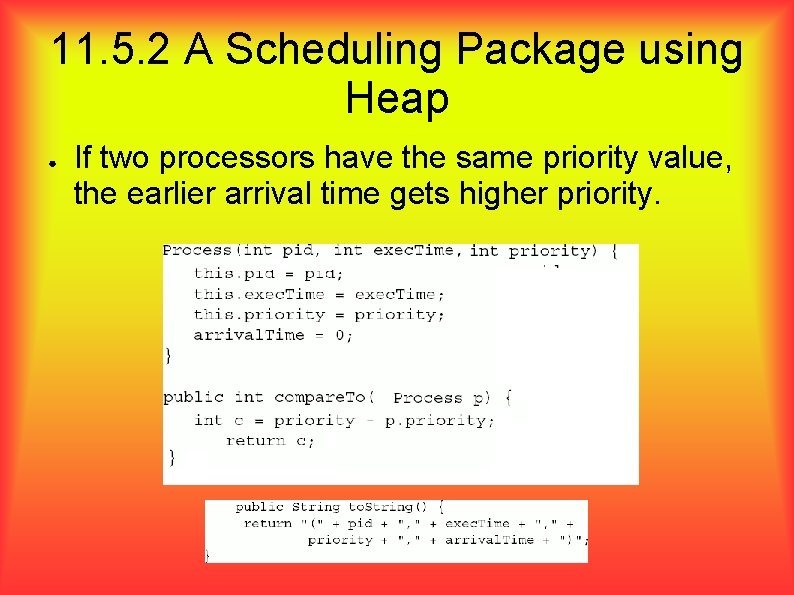
11. 5. 2 A Scheduling Package using Heap ● If two processors have the same priority value, the earlier arrival time gets higher priority.
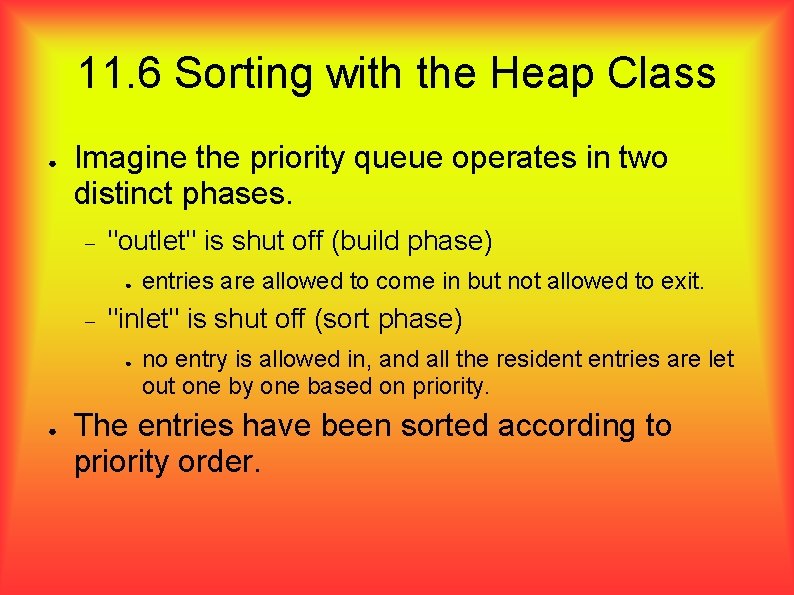
11. 6 Sorting with the Heap Class ● Imagine the priority queue operates in two distinct phases. "outlet" is shut off (build phase) ● "inlet" is shut off (sort phase) ● ● entries are allowed to come in but not allowed to exit. no entry is allowed in, and all the resident entries are let out one by one based on priority. The entries have been sorted according to priority order.
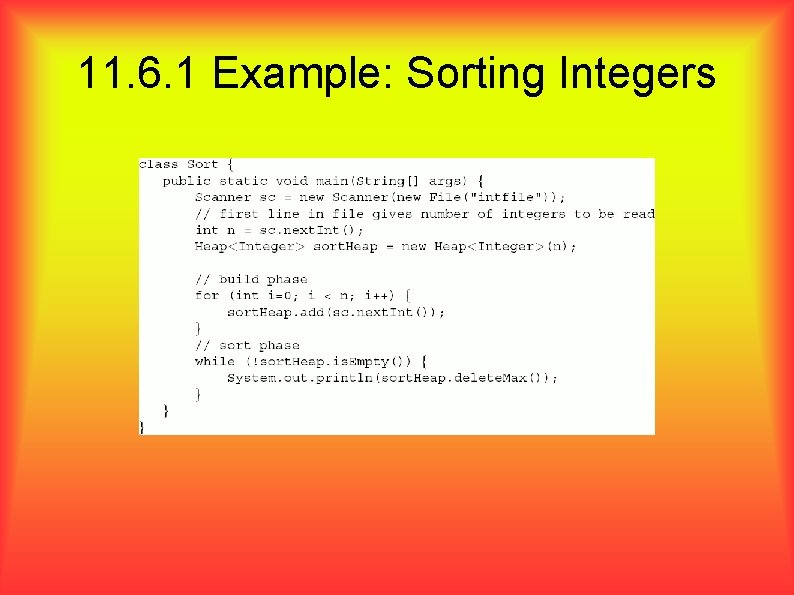
11. 6. 1 Example: Sorting Integers
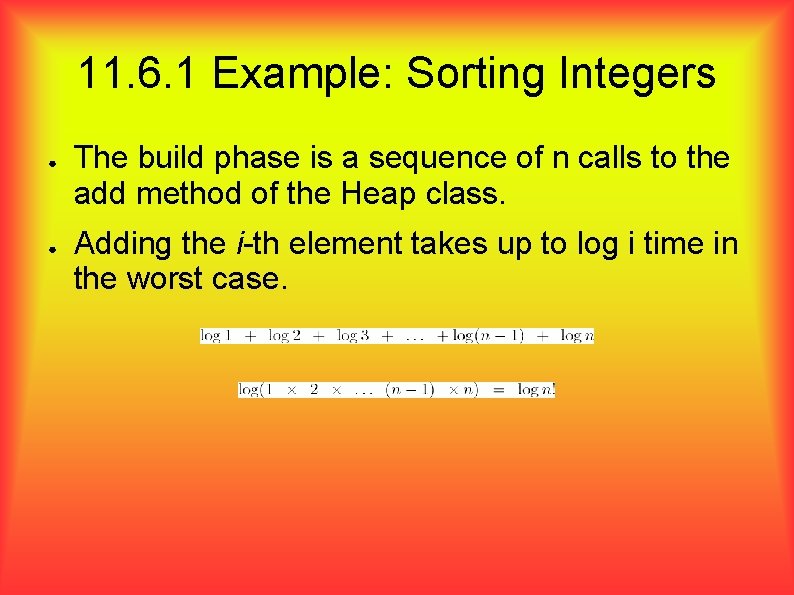
11. 6. 1 Example: Sorting Integers ● ● The build phase is a sequence of n calls to the add method of the Heap class. Adding the i-th element takes up to log i time in the worst case.
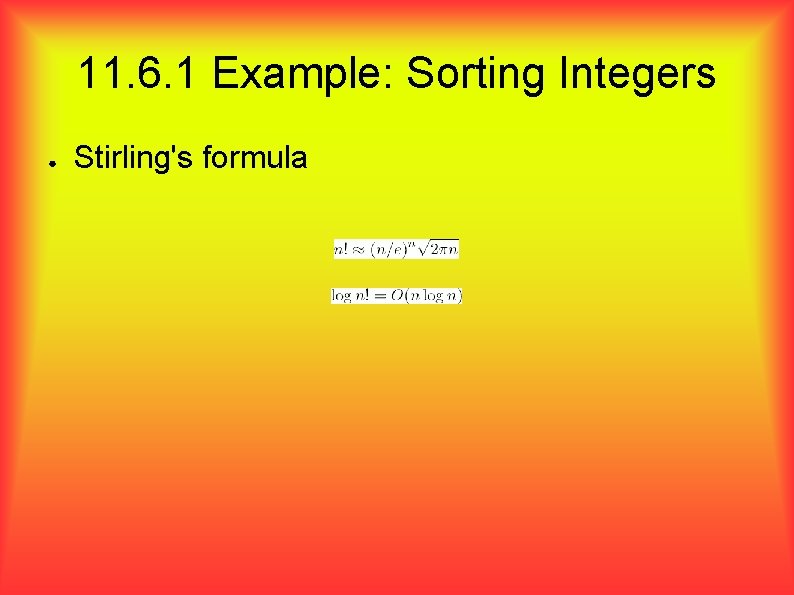
11. 6. 1 Example: Sorting Integers ● Stirling's formula
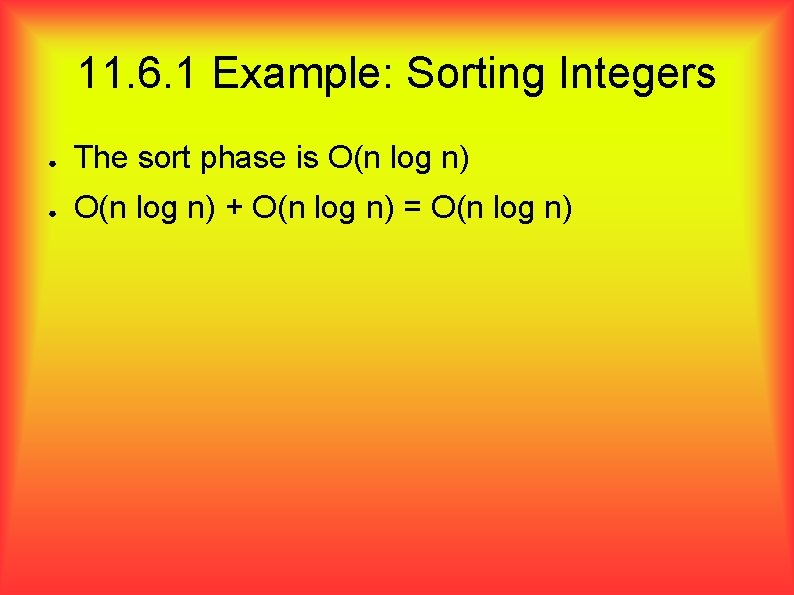
11. 6. 1 Example: Sorting Integers ● The sort phase is O(n log n) ● O(n log n) + O(n log n) = O(n log n)
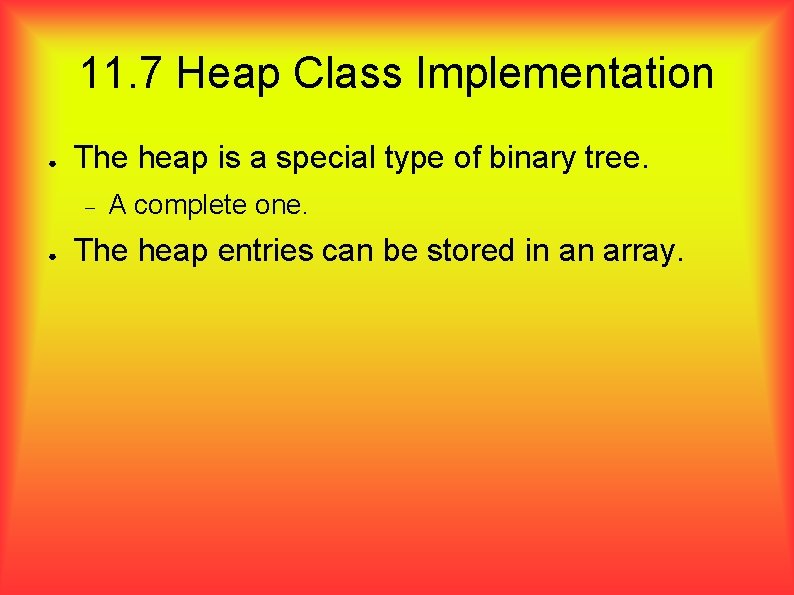
11. 7 Heap Class Implementation ● The heap is a special type of binary tree. ● A complete one. The heap entries can be stored in an array.
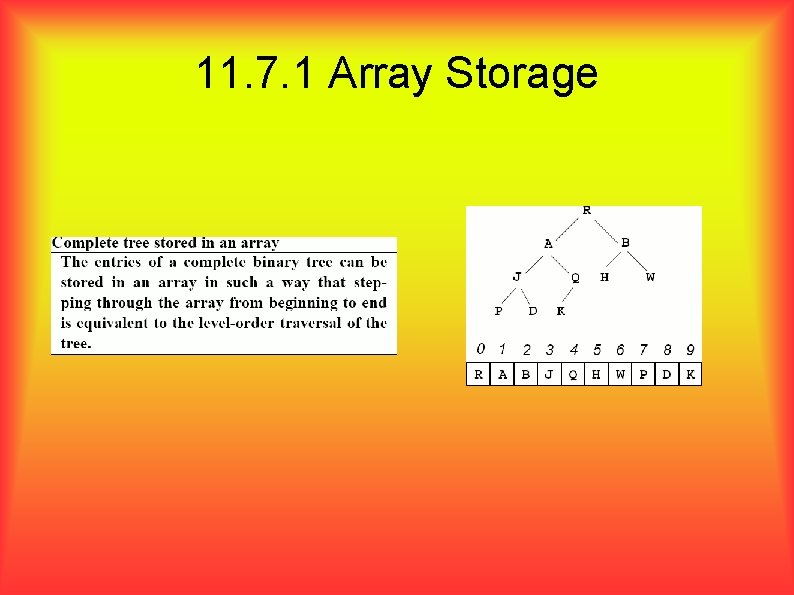
11. 7. 1 Array Storage
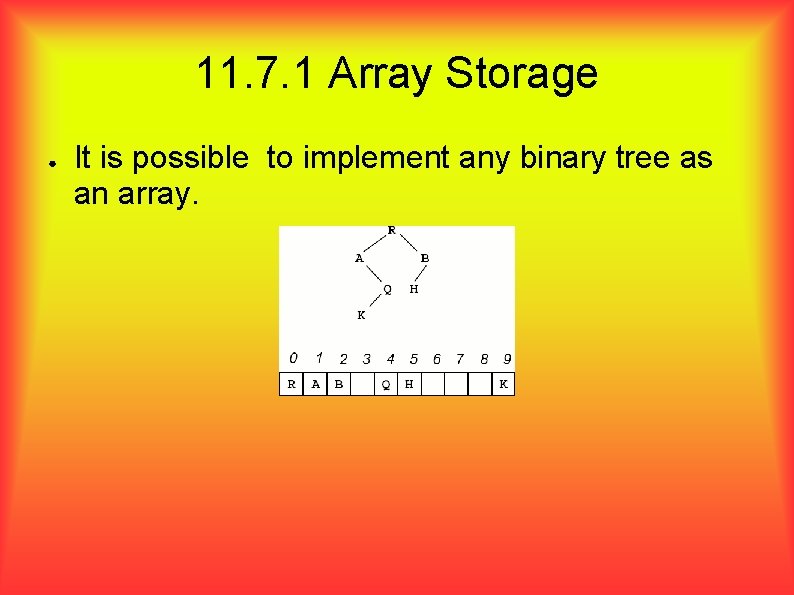
11. 7. 1 Array Storage ● It is possible to implement any binary tree as an array.
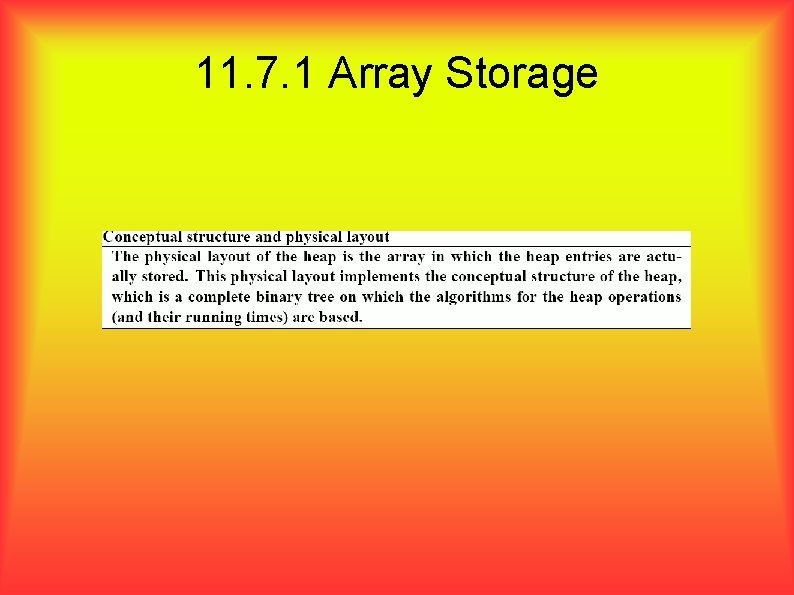
11. 7. 1 Array Storage