Maps Dictionaries Hashing Outline and Reading Map ADT
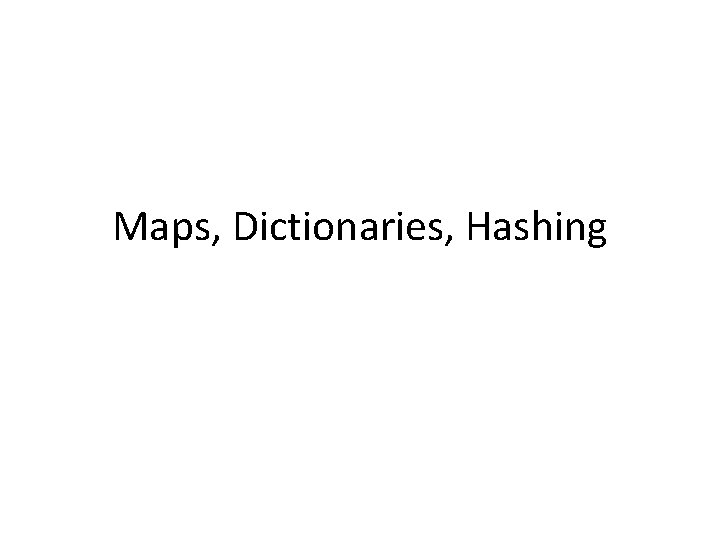
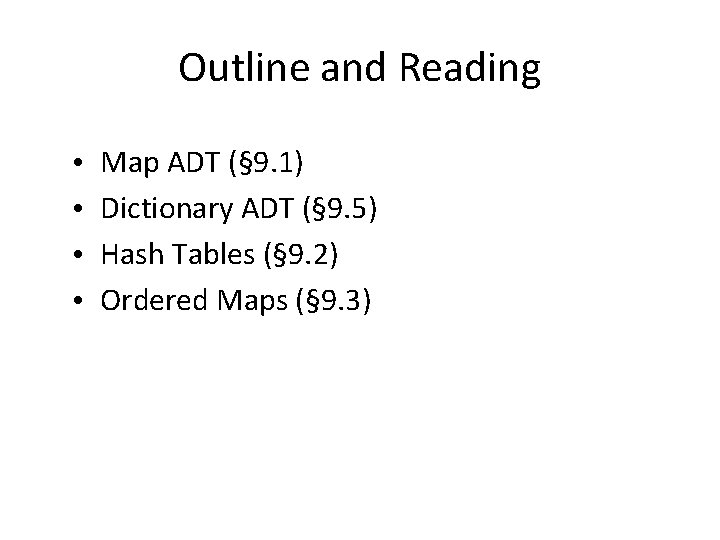
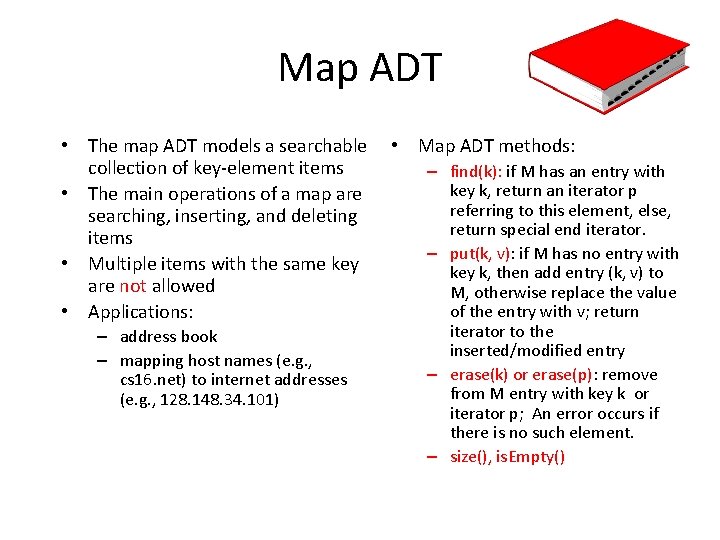
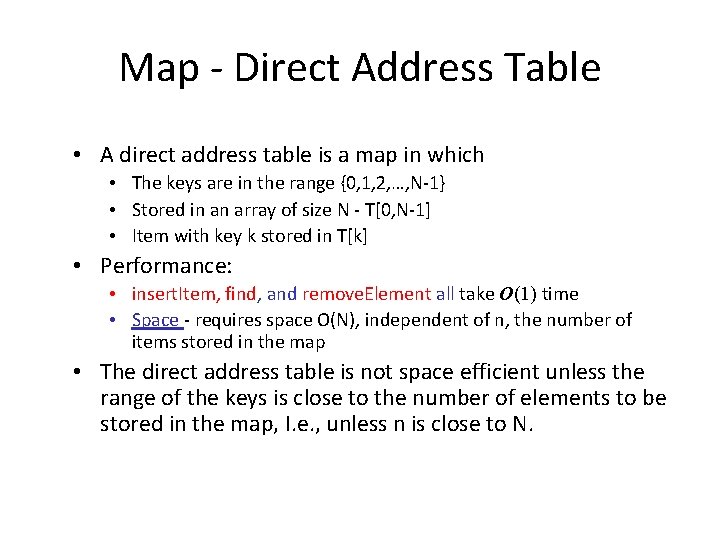
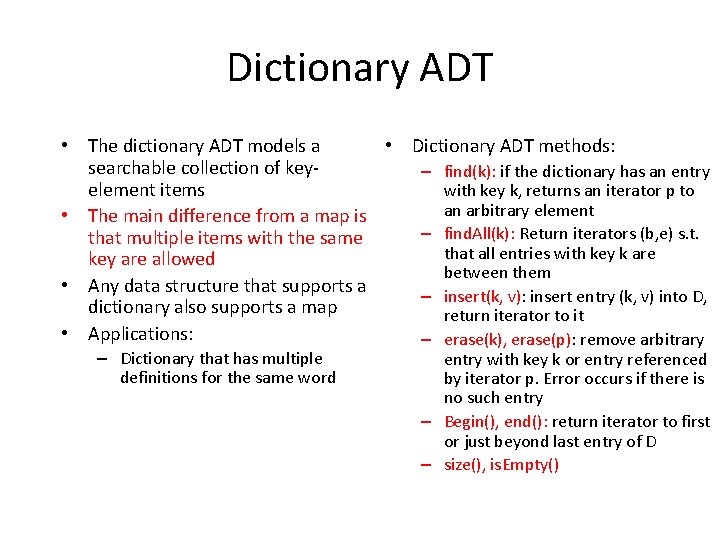
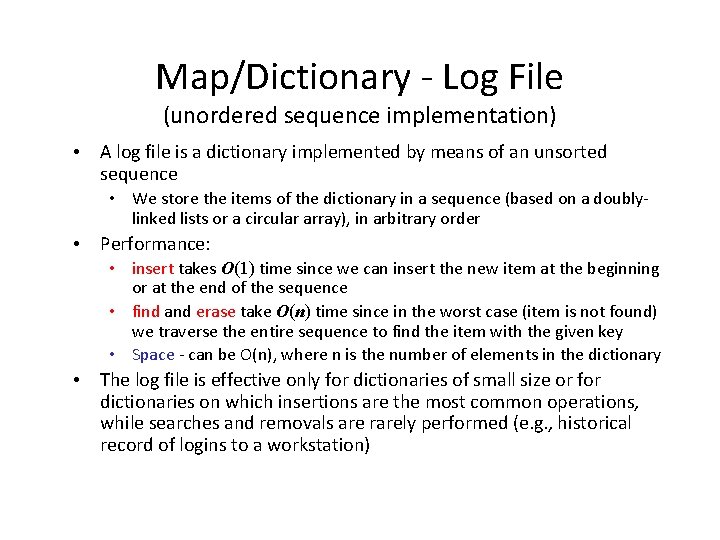
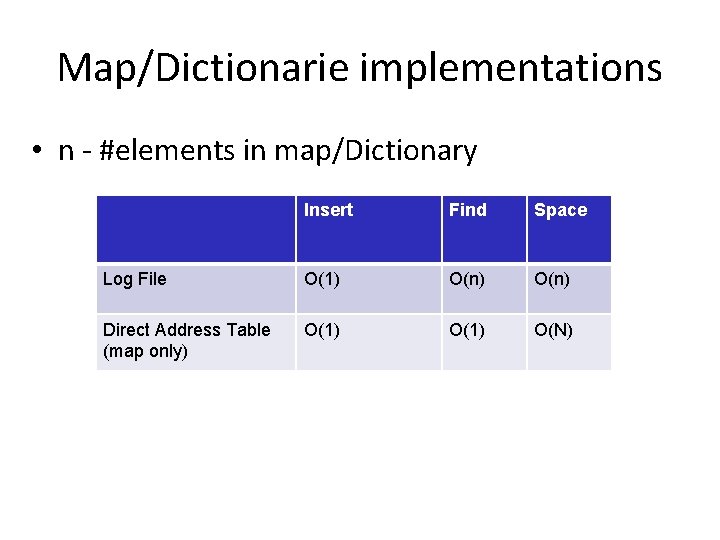
![Hash Tables • Hashing • Hash table (an array) of size N, H[0, N-1] Hash Tables • Hashing • Hash table (an array) of size N, H[0, N-1]](https://slidetodoc.com/presentation_image_h/2bad2aeec47b37b430f06450b8911fcd/image-8.jpg)
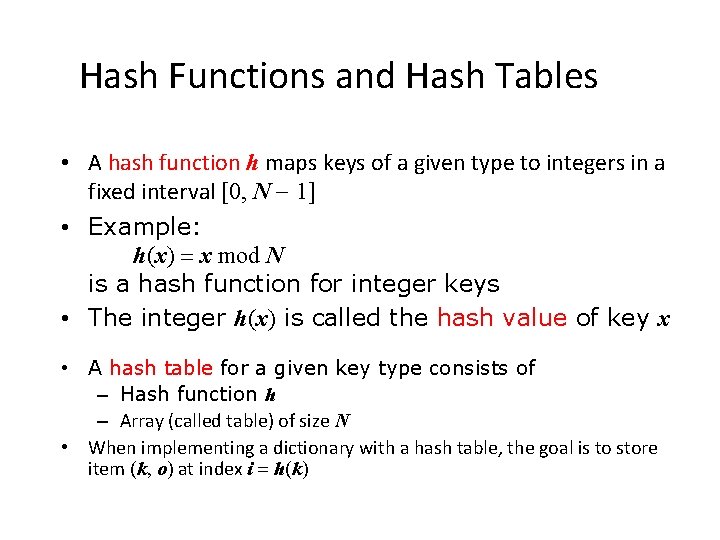
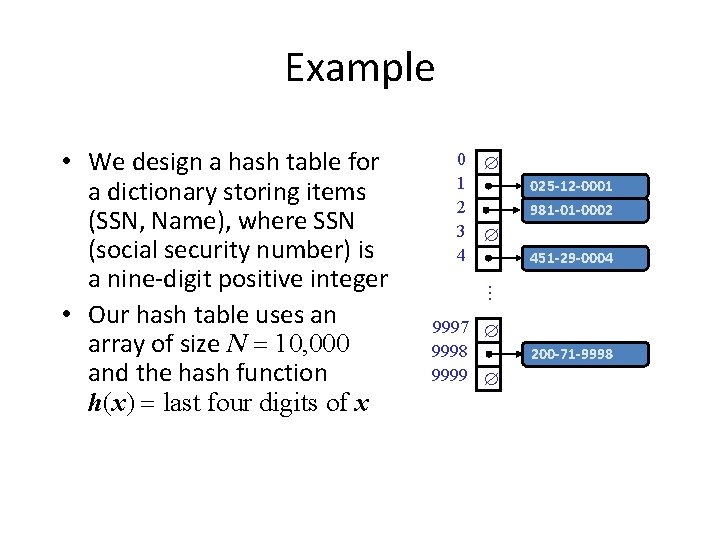
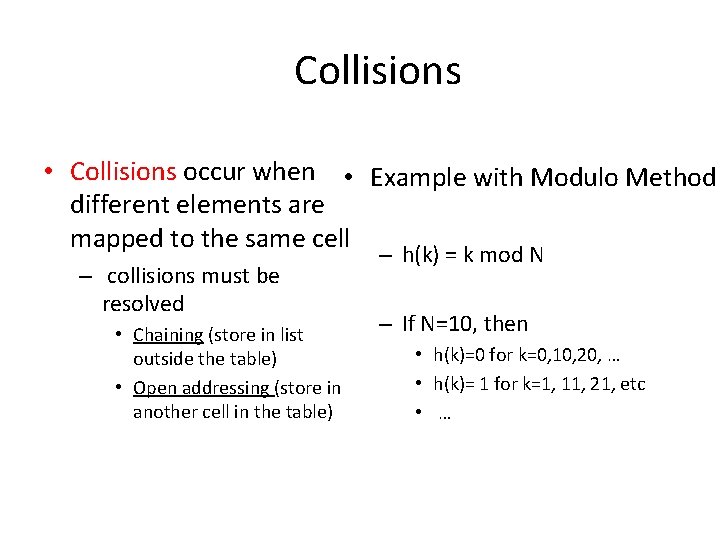
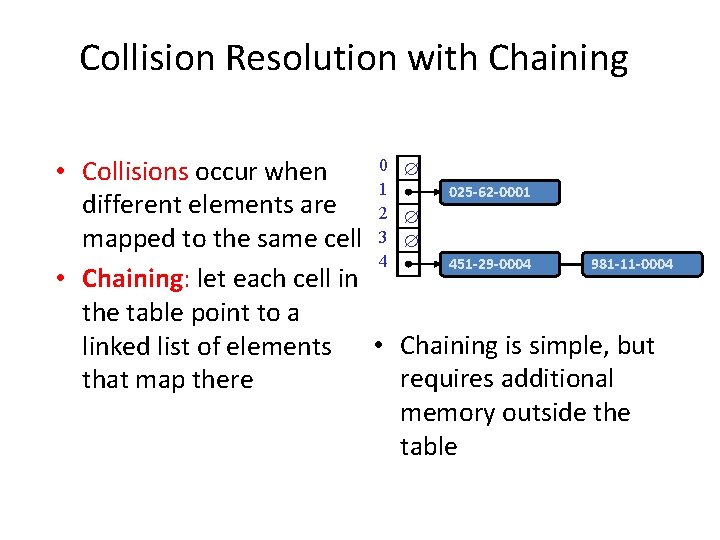
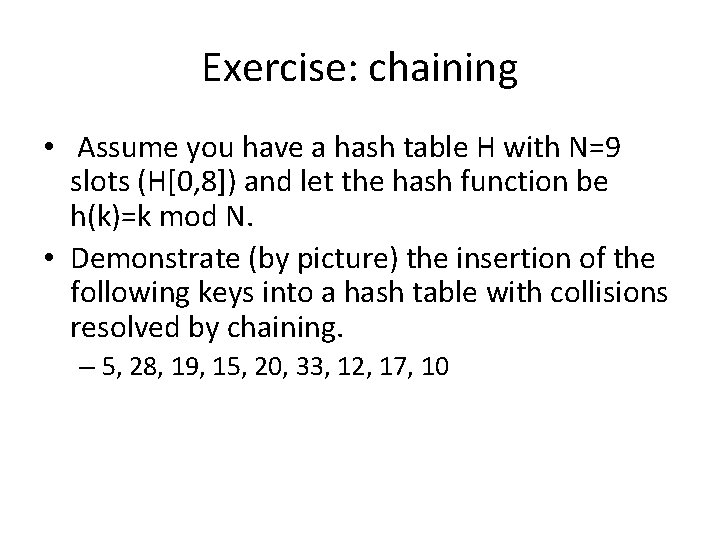
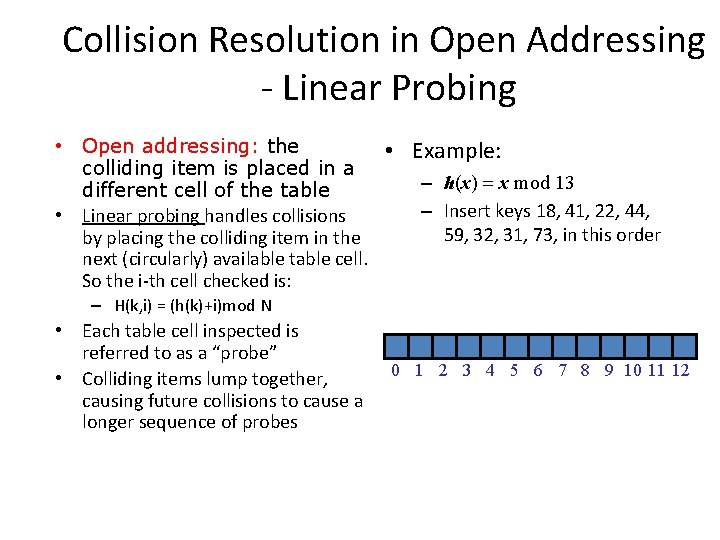
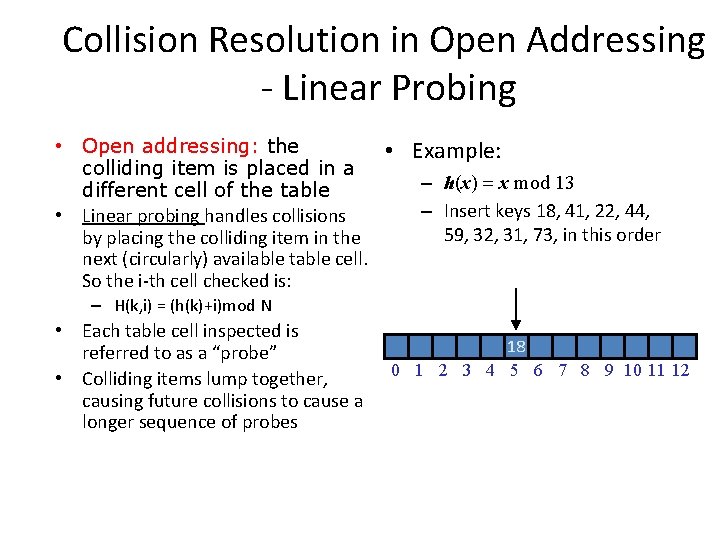
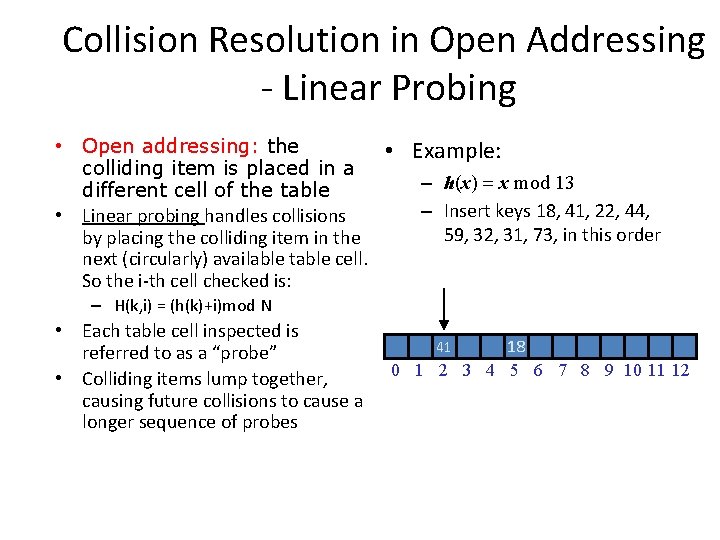
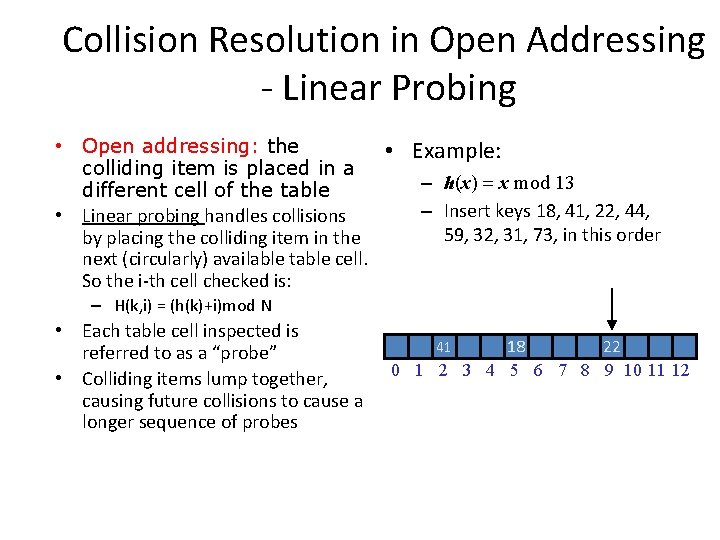
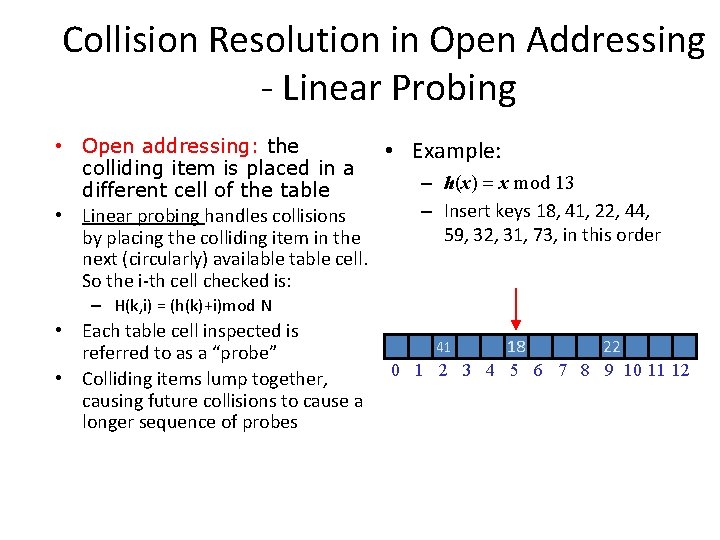
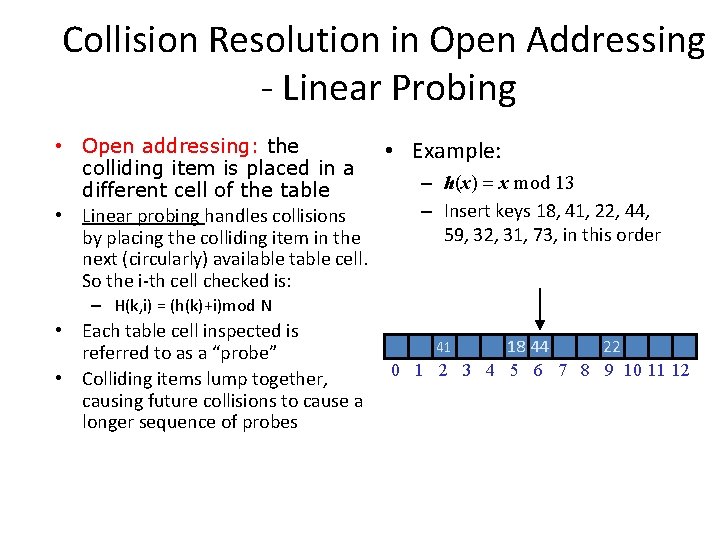
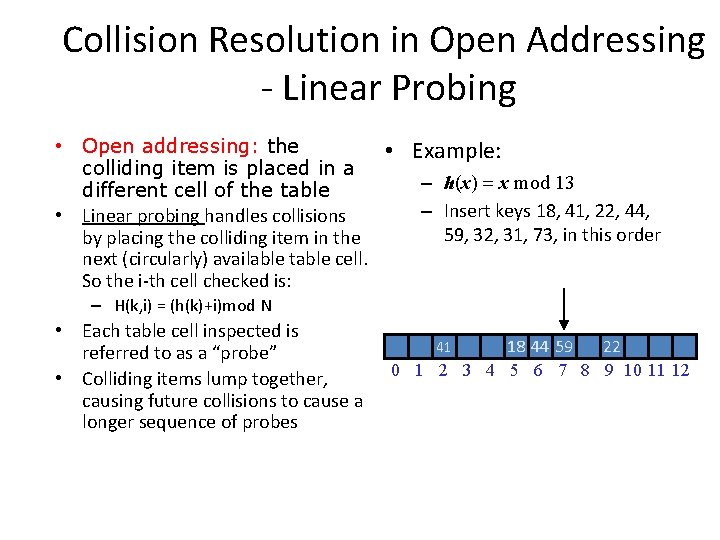
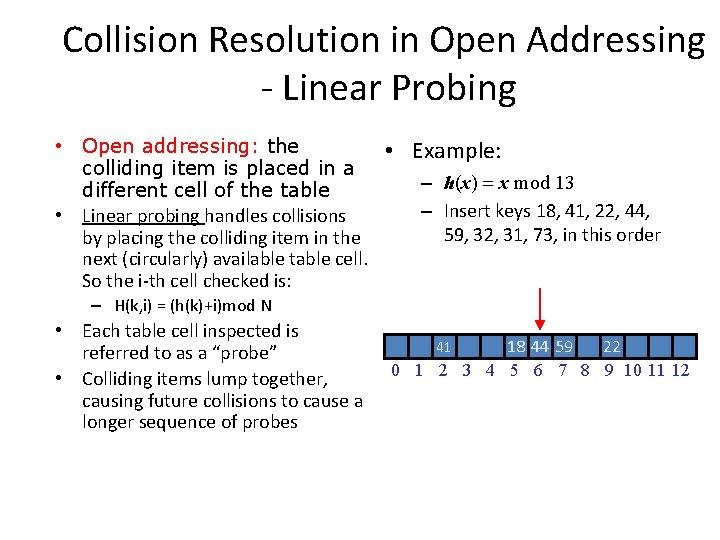
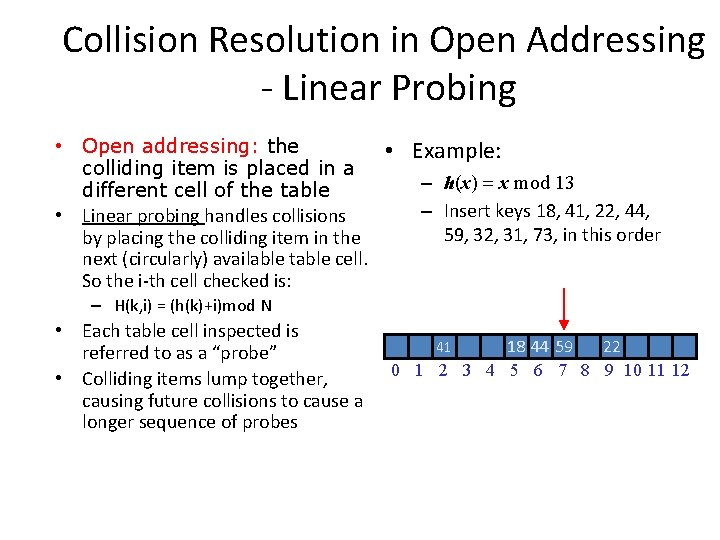
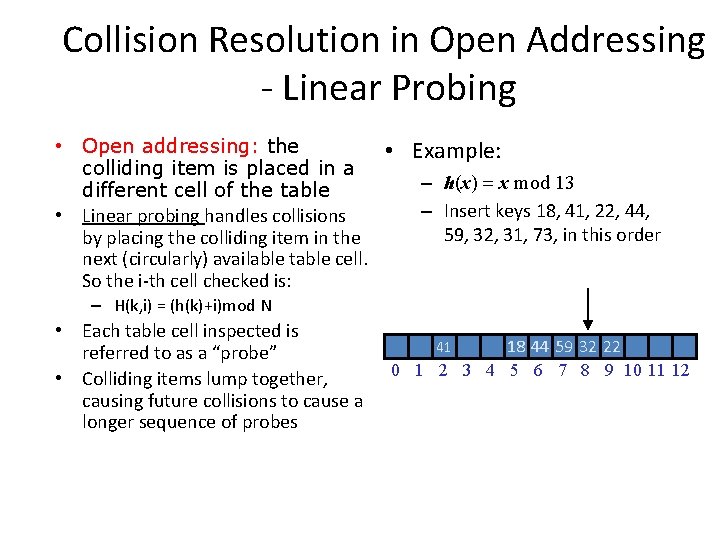
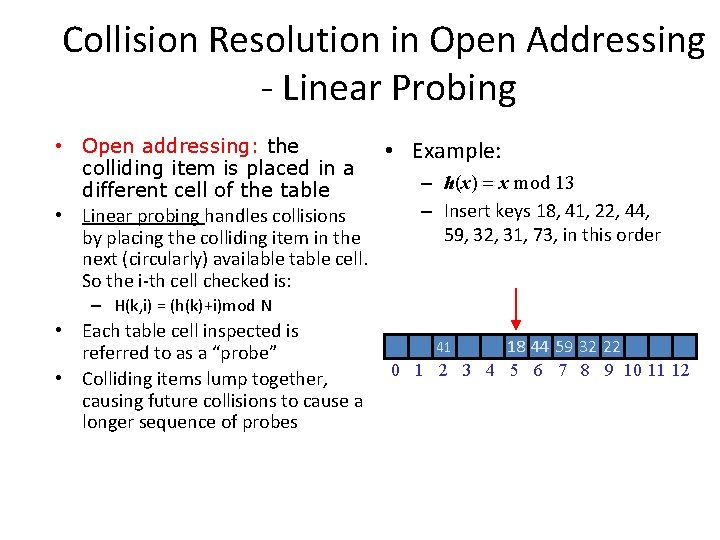
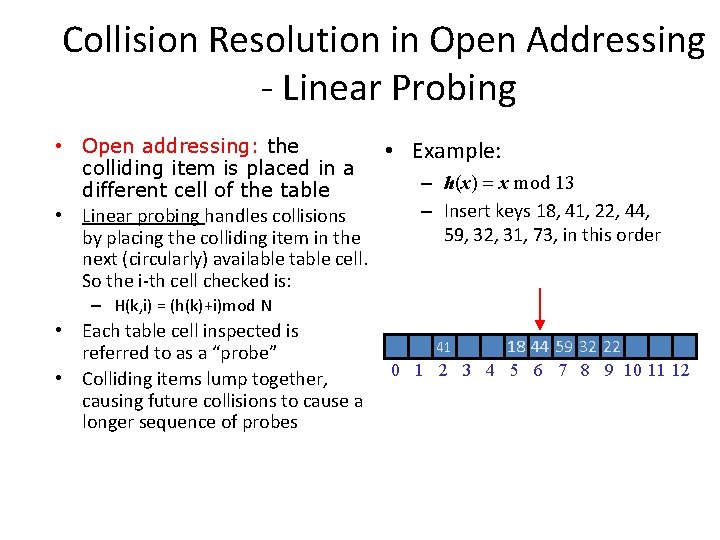
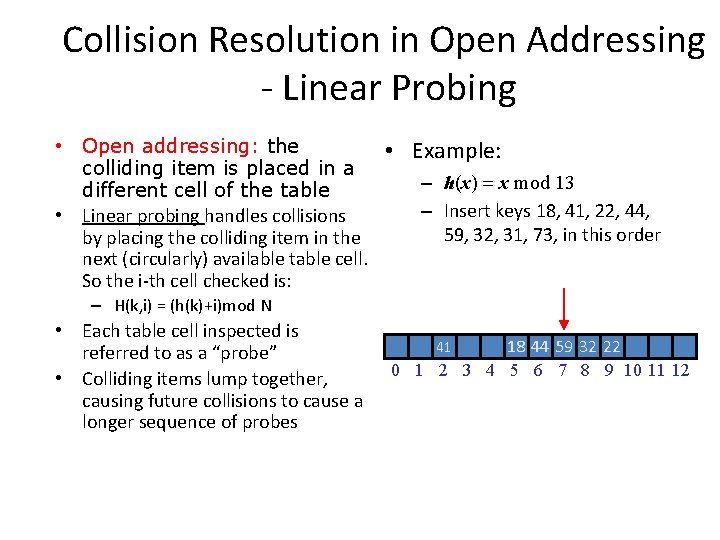
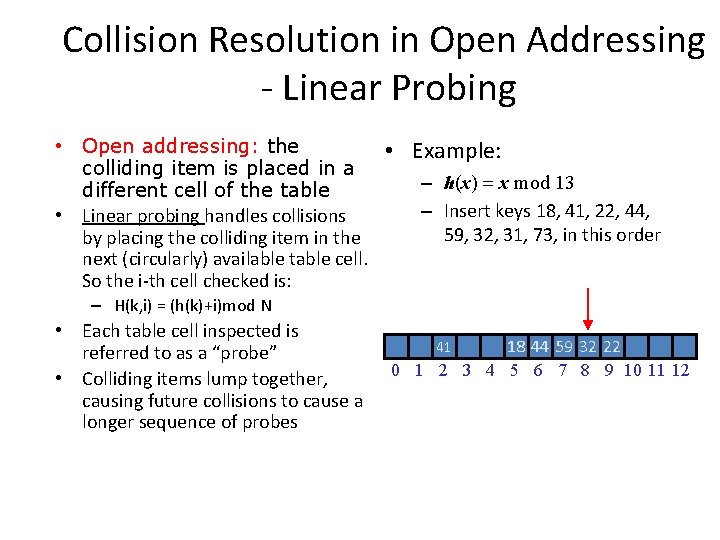
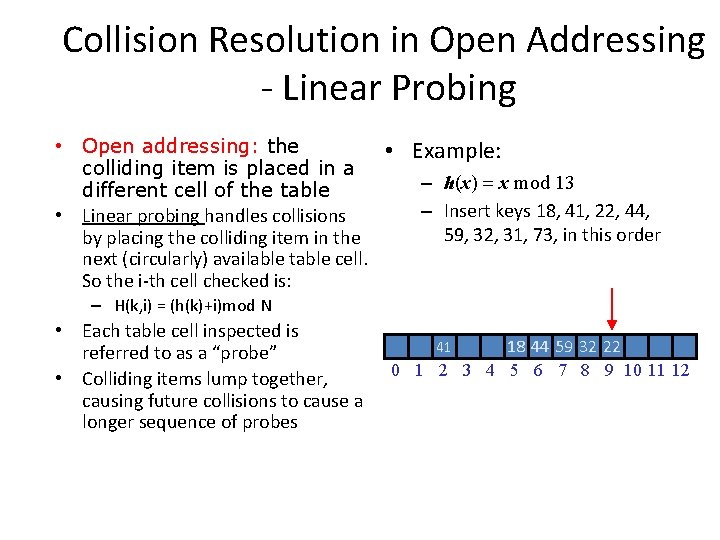
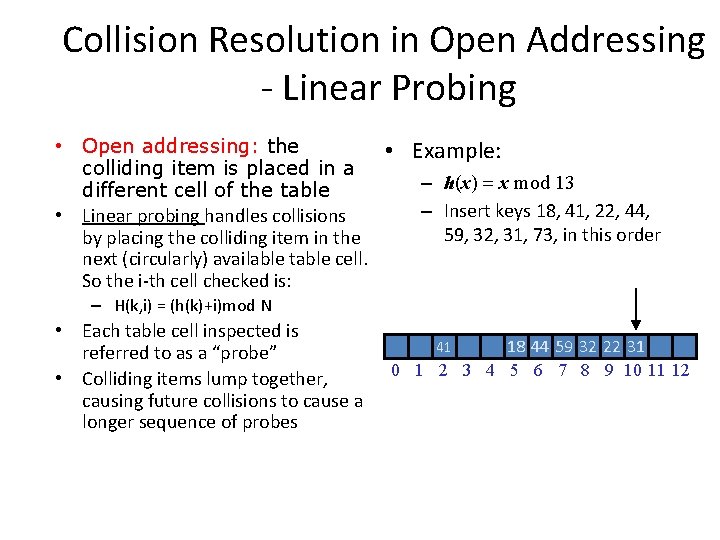
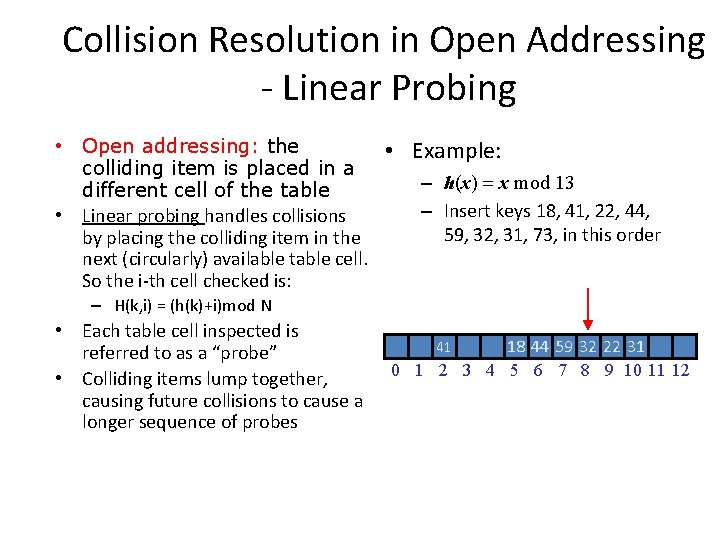
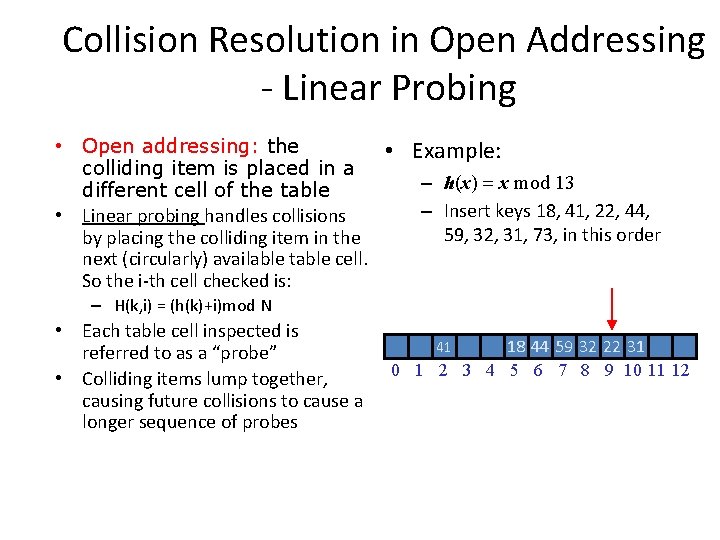
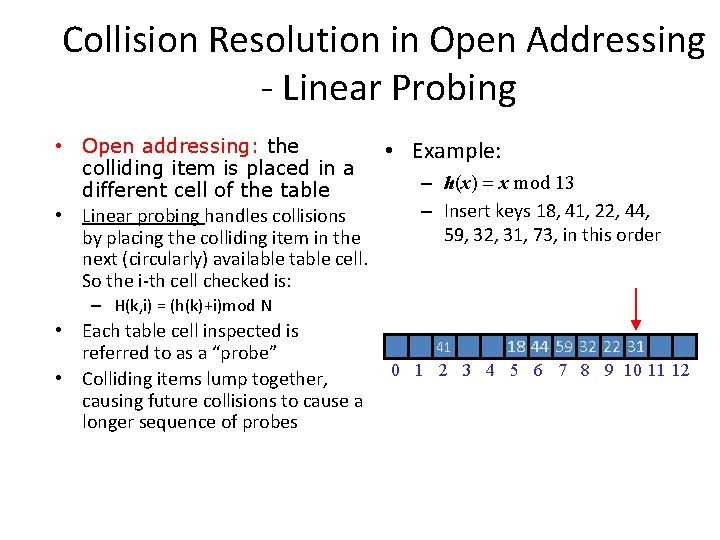
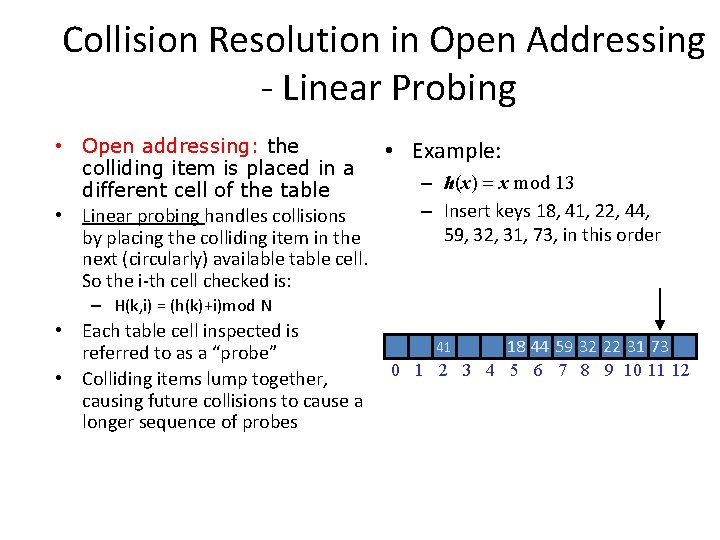
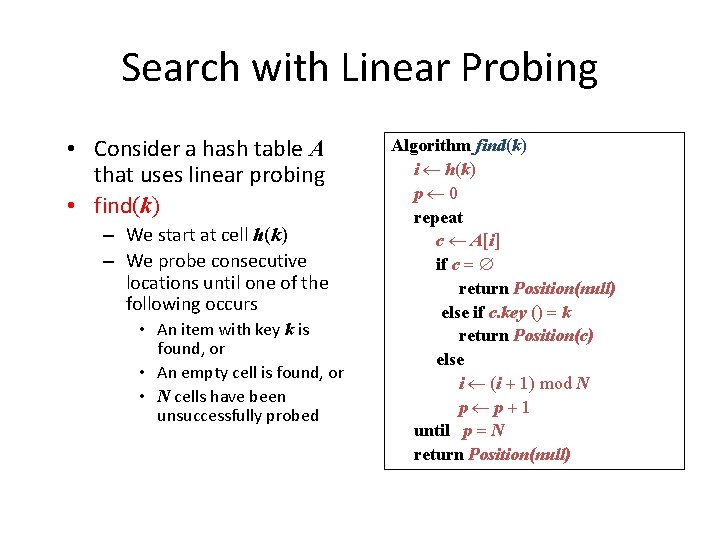
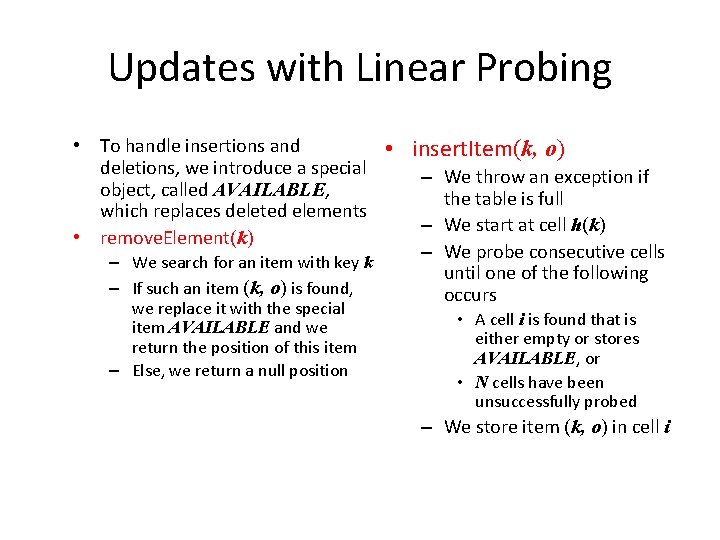
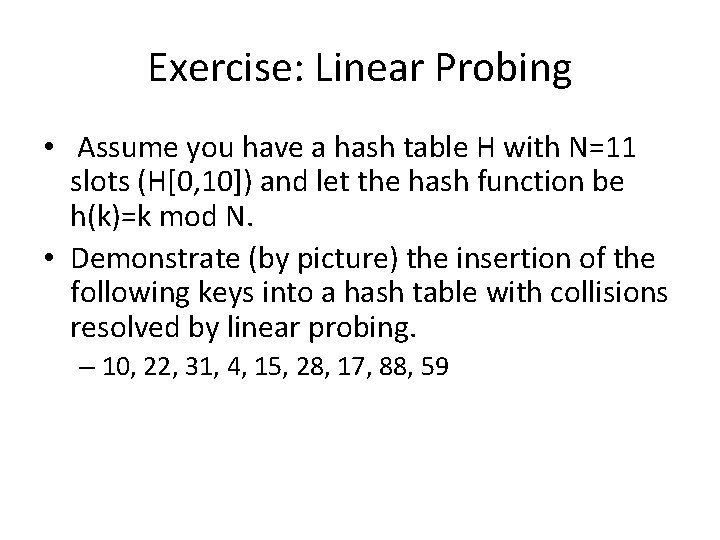
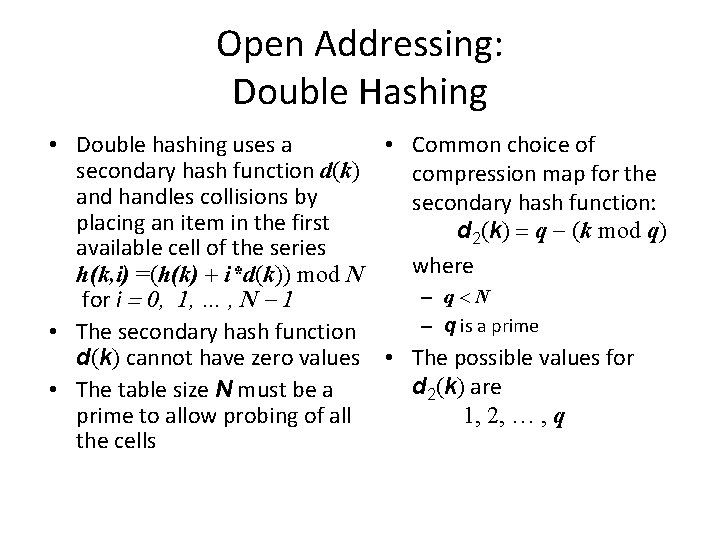
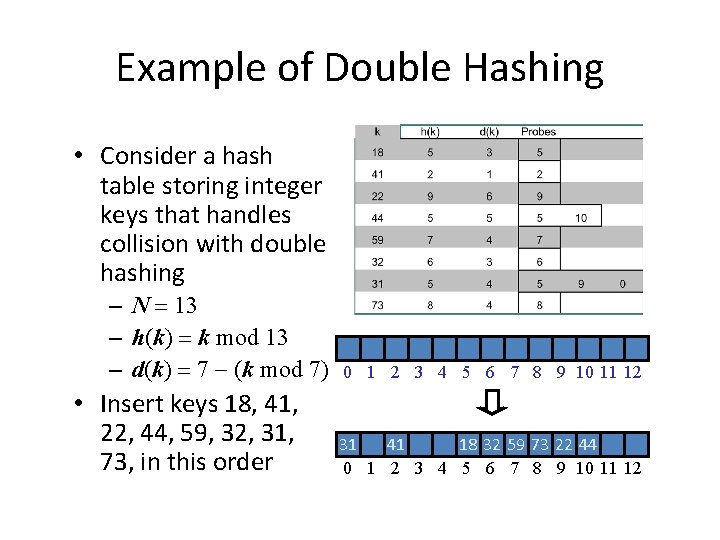
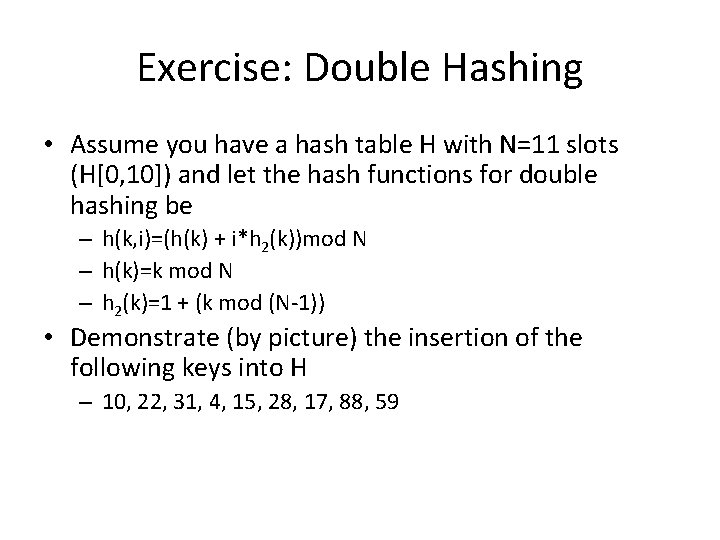
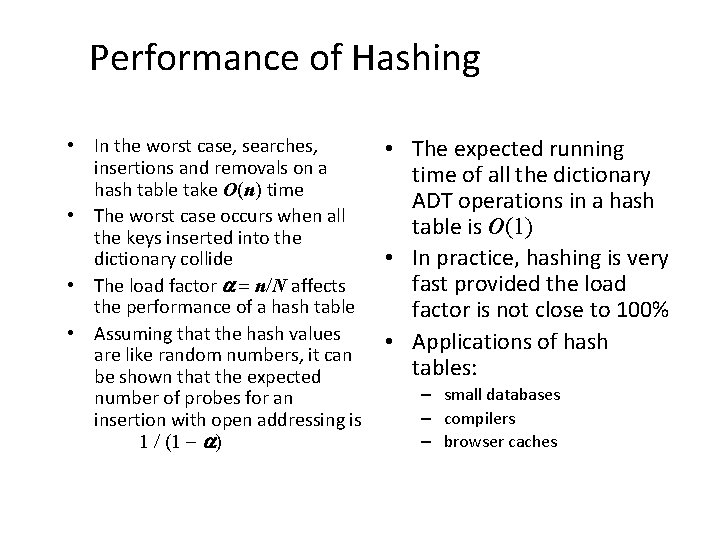
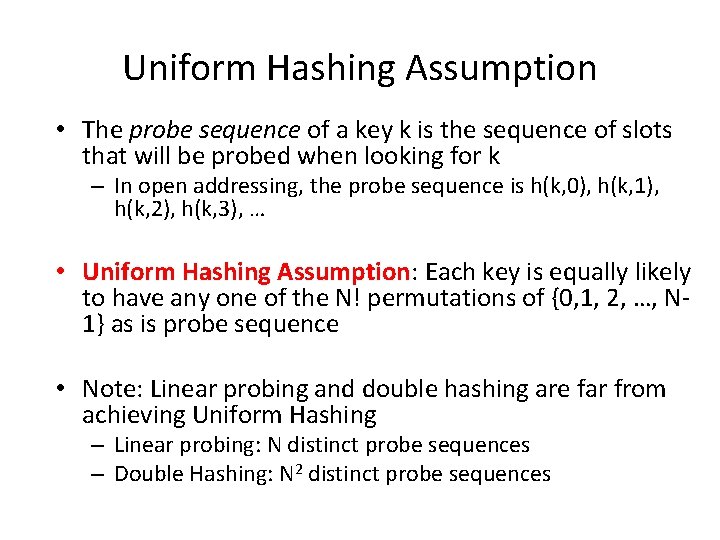
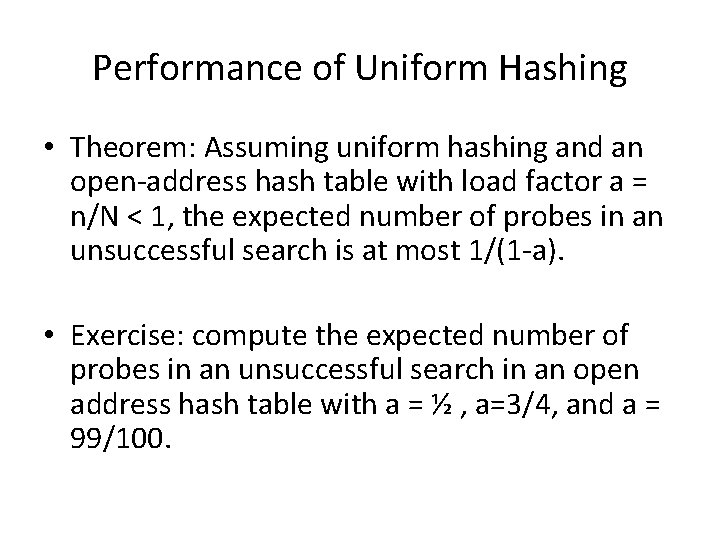
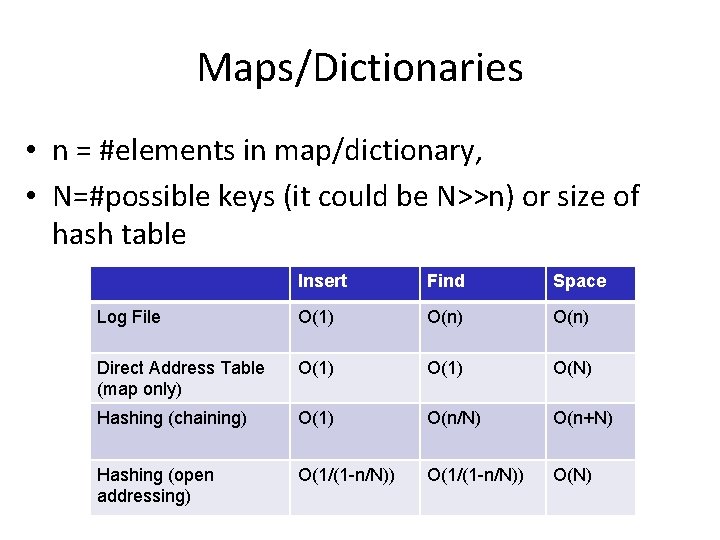
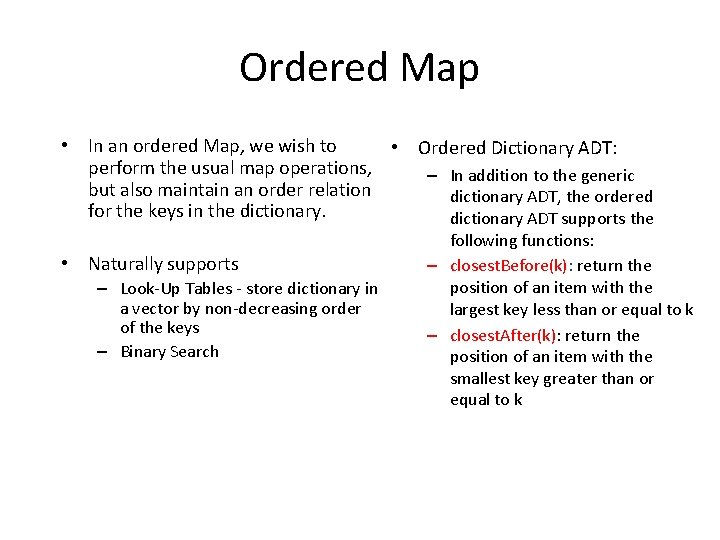
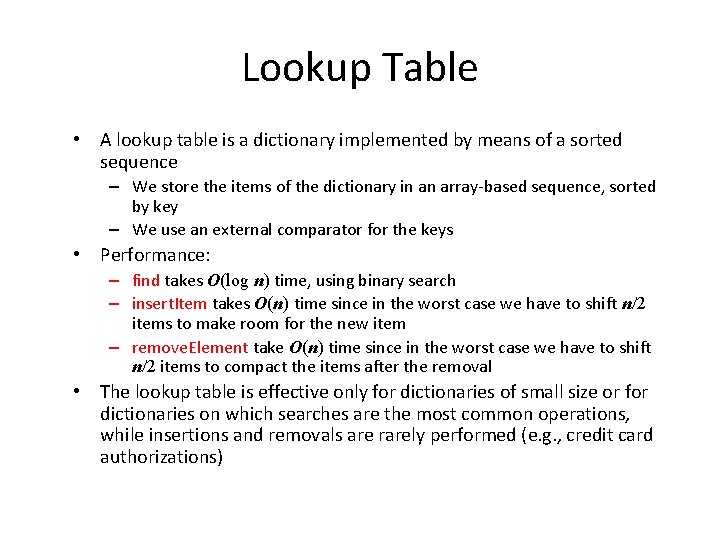
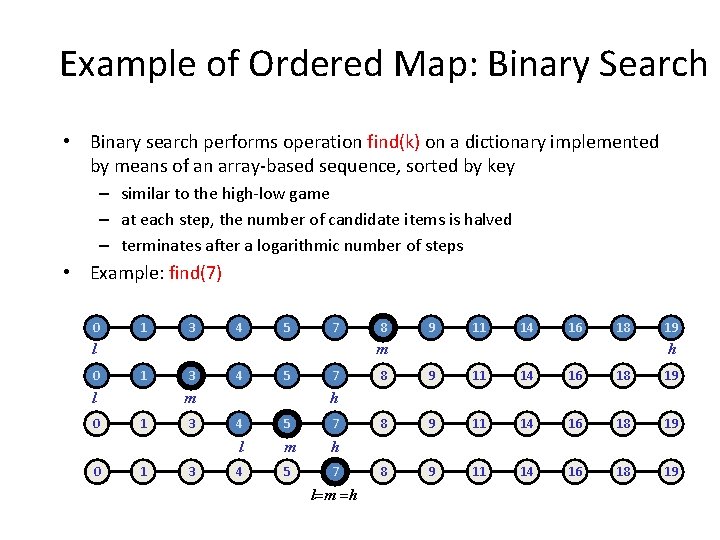
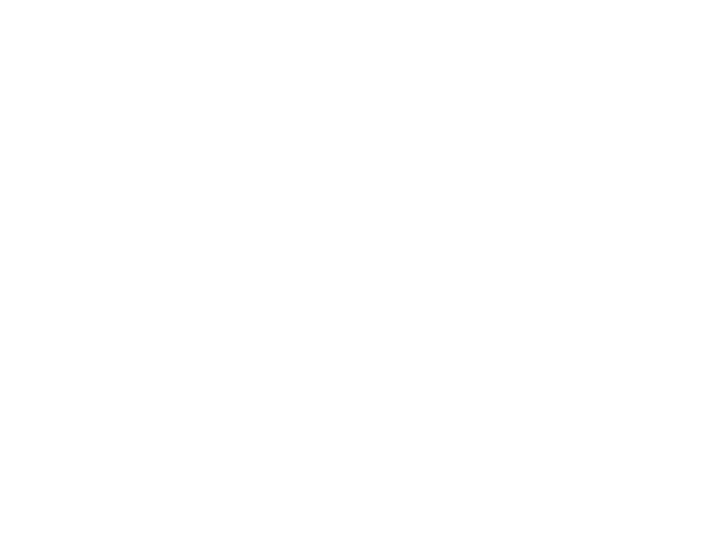
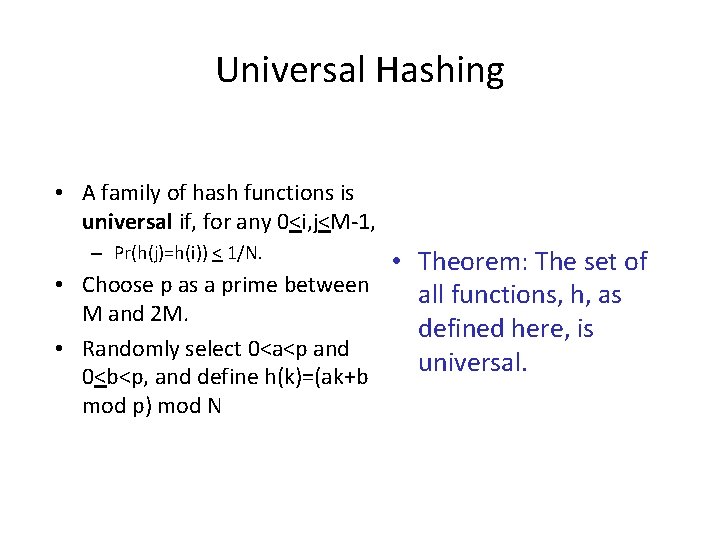
- Slides: 48
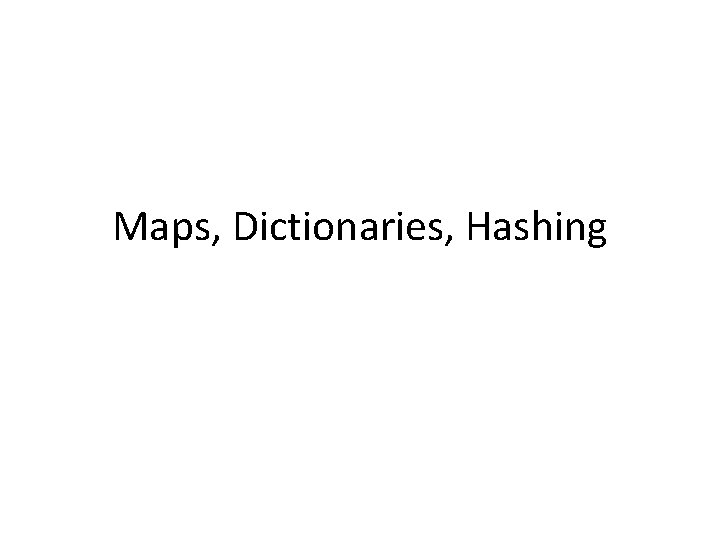
Maps, Dictionaries, Hashing
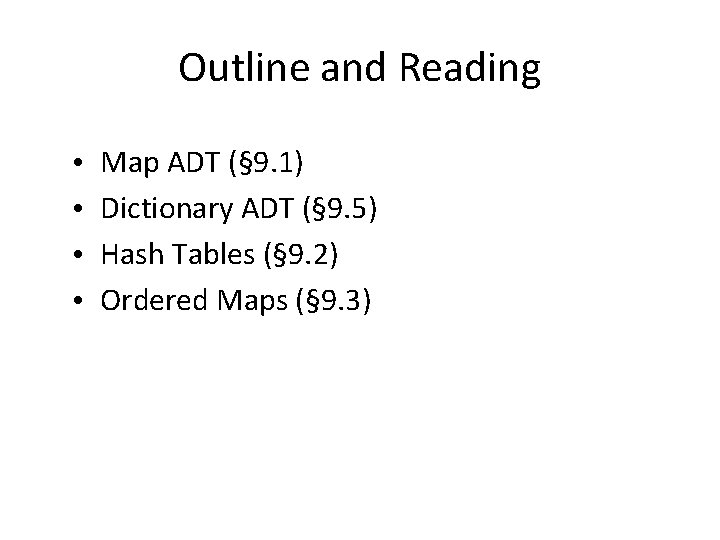
Outline and Reading • • Map ADT (§ 9. 1) Dictionary ADT (§ 9. 5) Hash Tables (§ 9. 2) Ordered Maps (§ 9. 3)
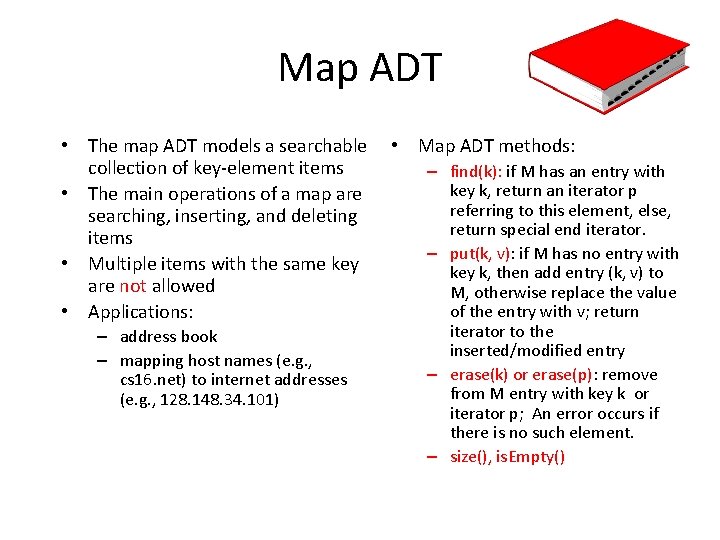
Map ADT • The map ADT models a searchable collection of key-element items • The main operations of a map are searching, inserting, and deleting items • Multiple items with the same key are not allowed • Applications: – address book – mapping host names (e. g. , cs 16. net) to internet addresses (e. g. , 128. 148. 34. 101) • Map ADT methods: – find(k): if M has an entry with key k, return an iterator p referring to this element, else, return special end iterator. – put(k, v): if M has no entry with key k, then add entry (k, v) to M, otherwise replace the value of the entry with v; return iterator to the inserted/modified entry – erase(k) or erase(p): remove from M entry with key k or iterator p; An error occurs if there is no such element. – size(), is. Empty()
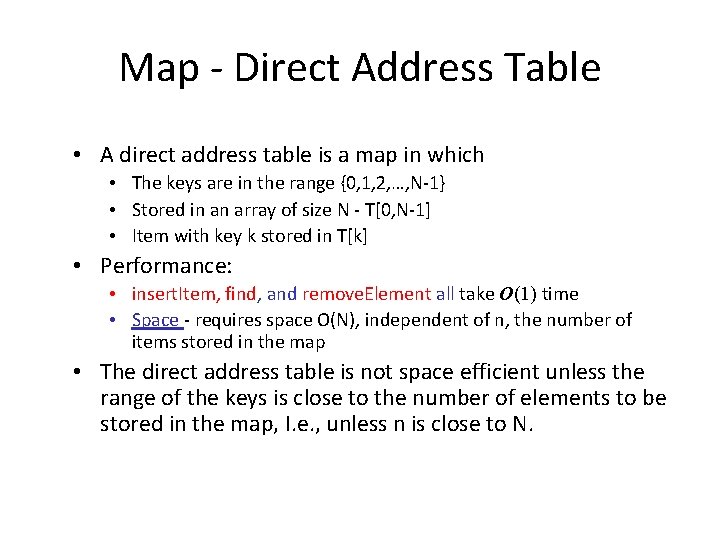
Map - Direct Address Table • A direct address table is a map in which • The keys are in the range {0, 1, 2, …, N-1} • Stored in an array of size N - T[0, N-1] • Item with key k stored in T[k] • Performance: • insert. Item, find, and remove. Element all take O(1) time • Space - requires space O(N), independent of n, the number of items stored in the map • The direct address table is not space efficient unless the range of the keys is close to the number of elements to be stored in the map, I. e. , unless n is close to N.
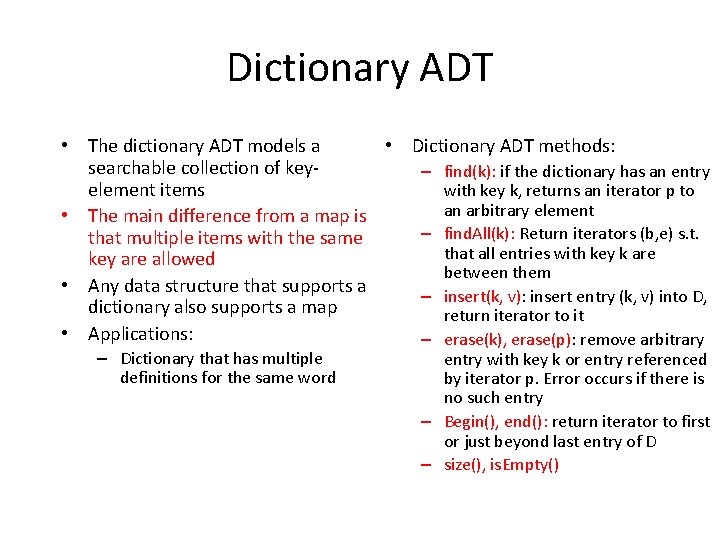
Dictionary ADT • The dictionary ADT models a • Dictionary ADT methods: searchable collection of key– find(k): if the dictionary has an entry element items with key k, returns an iterator p to an arbitrary element • The main difference from a map is – find. All(k): Return iterators (b, e) s. t. that multiple items with the same that all entries with key k are key are allowed between them • Any data structure that supports a – insert(k, v): insert entry (k, v) into D, dictionary also supports a map return iterator to it • Applications: – erase(k), erase(p): remove arbitrary – Dictionary that has multiple definitions for the same word entry with key k or entry referenced by iterator p. Error occurs if there is no such entry – Begin(), end(): return iterator to first or just beyond last entry of D – size(), is. Empty()
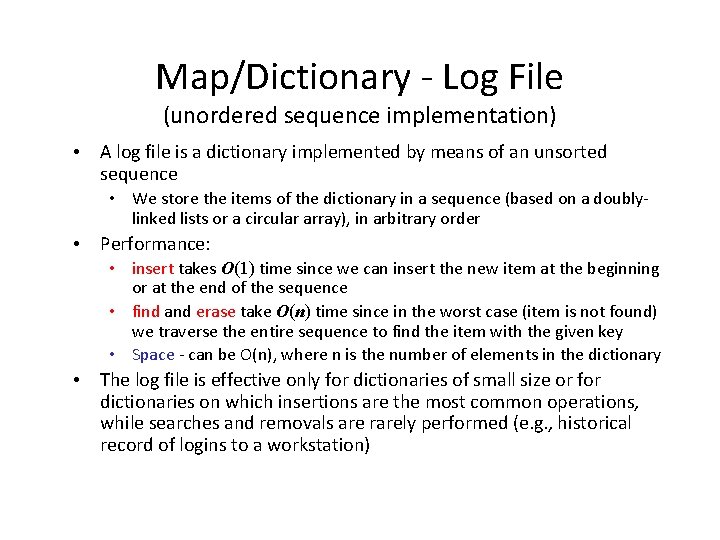
Map/Dictionary - Log File (unordered sequence implementation) • A log file is a dictionary implemented by means of an unsorted sequence • We store the items of the dictionary in a sequence (based on a doublylinked lists or a circular array), in arbitrary order • Performance: • insert takes O(1) time since we can insert the new item at the beginning or at the end of the sequence • find and erase take O(n) time since in the worst case (item is not found) we traverse the entire sequence to find the item with the given key • Space - can be O(n), where n is the number of elements in the dictionary • The log file is effective only for dictionaries of small size or for dictionaries on which insertions are the most common operations, while searches and removals are rarely performed (e. g. , historical record of logins to a workstation)
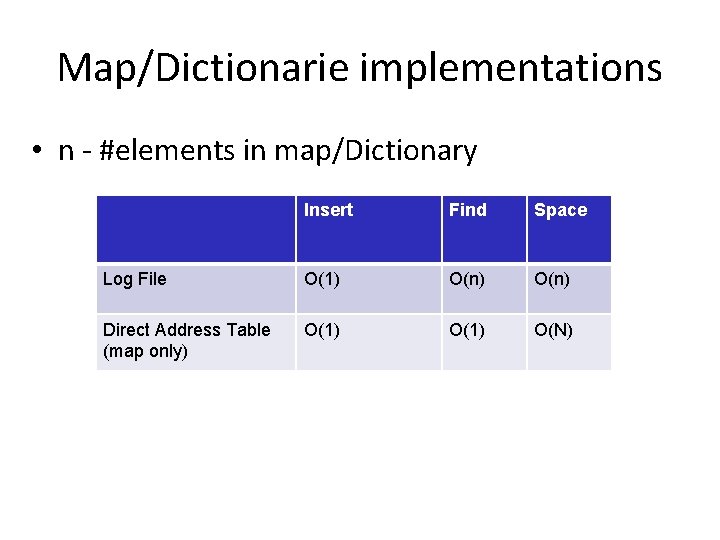
Map/Dictionarie implementations • n - #elements in map/Dictionary Insert Find Space Log File O(1) O(n) Direct Address Table (map only) O(1) O(N)
![Hash Tables Hashing Hash table an array of size N H0 N1 Hash Tables • Hashing • Hash table (an array) of size N, H[0, N-1]](https://slidetodoc.com/presentation_image_h/2bad2aeec47b37b430f06450b8911fcd/image-8.jpg)
Hash Tables • Hashing • Hash table (an array) of size N, H[0, N-1] • Hash function h that maps keys to indices in H • Issues • Hash functions - need method to transform key to an index in H that will have nice properties. • Collisions - some keys will map to the same index of H (otherwise we have a Direct Address Table). Several methods to resolve the collisions • Chaining - put elements that hash to same location in a linked list • Open addressing - if a collision occurs, have a method to select another location in the table
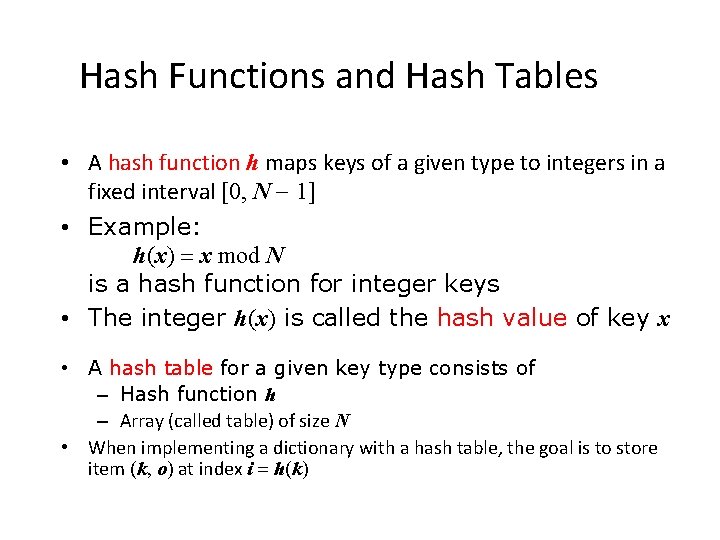
Hash Functions and Hash Tables • A hash function h maps keys of a given type to integers in a fixed interval [0, N - 1] • Example: h(x) = x mod N is a hash function for integer keys • The integer h(x) is called the hash value of key x • A hash table for a given key type consists of – Hash function h – Array (called table) of size N • When implementing a dictionary with a hash table, the goal is to store item (k, o) at index i = h(k)
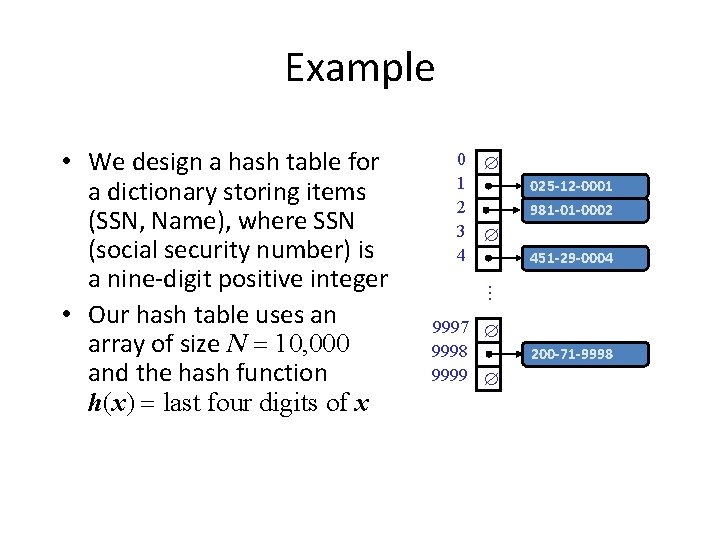
Example 0 1 2 3 4 025 -12 -0001 981 -01 -0002 451 -29 -0004 … • We design a hash table for a dictionary storing items (SSN, Name), where SSN (social security number) is a nine-digit positive integer • Our hash table uses an array of size N = 10, 000 and the hash function h(x) = last four digits of x 9997 9998 9999 200 -71 -9998
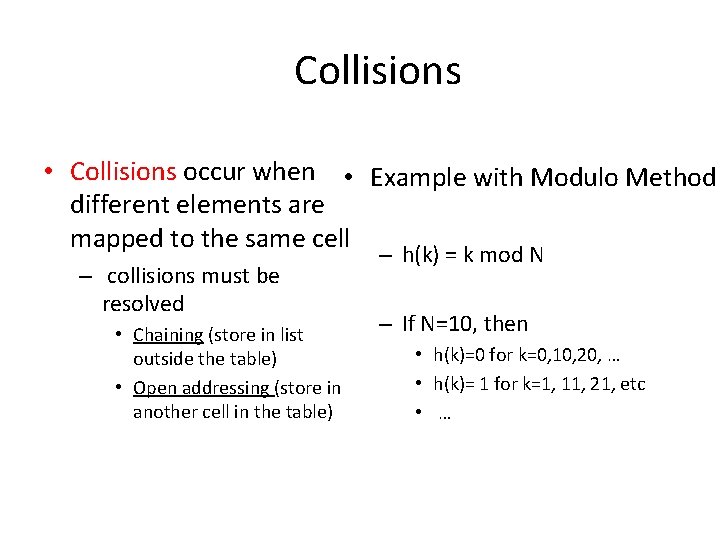
Collisions • Collisions occur when • Example with Modulo Method different elements are mapped to the same cell – collisions must be resolved • Chaining (store in list outside the table) • Open addressing (store in another cell in the table) – h(k) = k mod N – If N=10, then • h(k)=0 for k=0, 10, 20, … • h(k)= 1 for k=1, 11, 21, etc • …
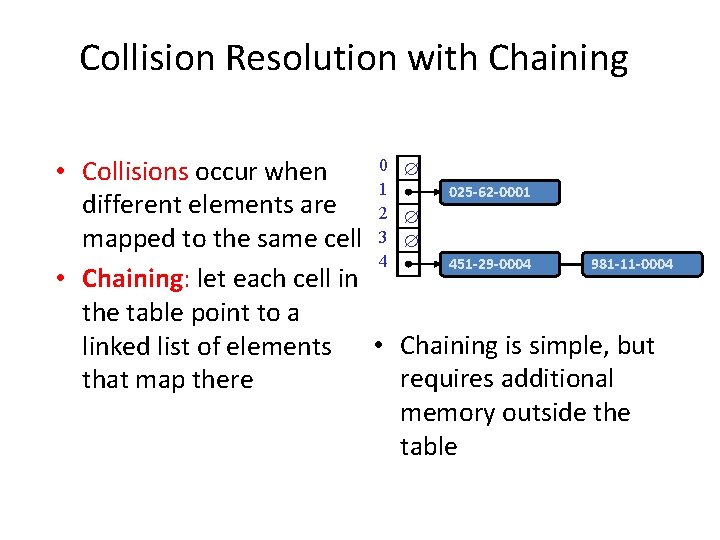
Collision Resolution with Chaining 0 • Collisions occur when 1 025 -62 -0001 different elements are 2 mapped to the same cell 3 4 451 -29 -0004 981 -11 -0004 • Chaining: let each cell in the table point to a linked list of elements • Chaining is simple, but requires additional that map there memory outside the table
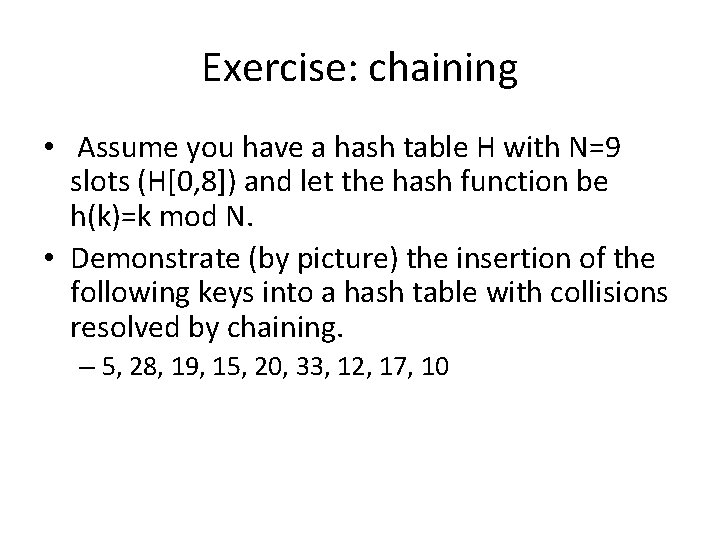
Exercise: chaining • Assume you have a hash table H with N=9 slots (H[0, 8]) and let the hash function be h(k)=k mod N. • Demonstrate (by picture) the insertion of the following keys into a hash table with collisions resolved by chaining. – 5, 28, 19, 15, 20, 33, 12, 17, 10
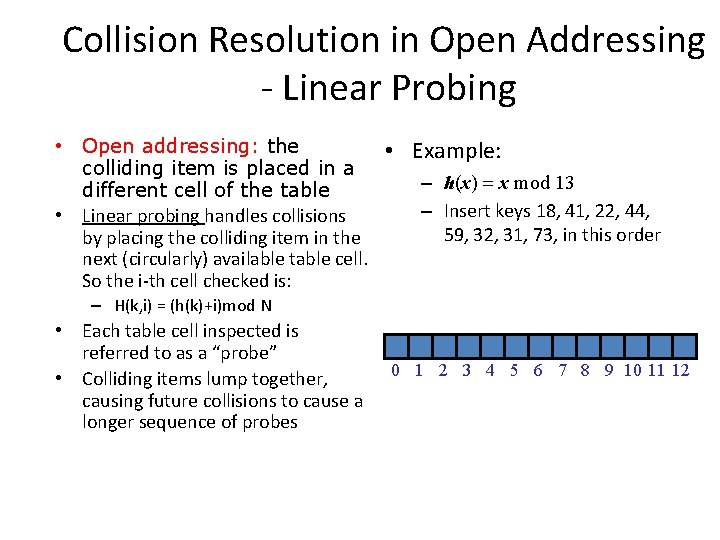
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 0 1 2 3 4 5 6 7 8 9 10 11 12
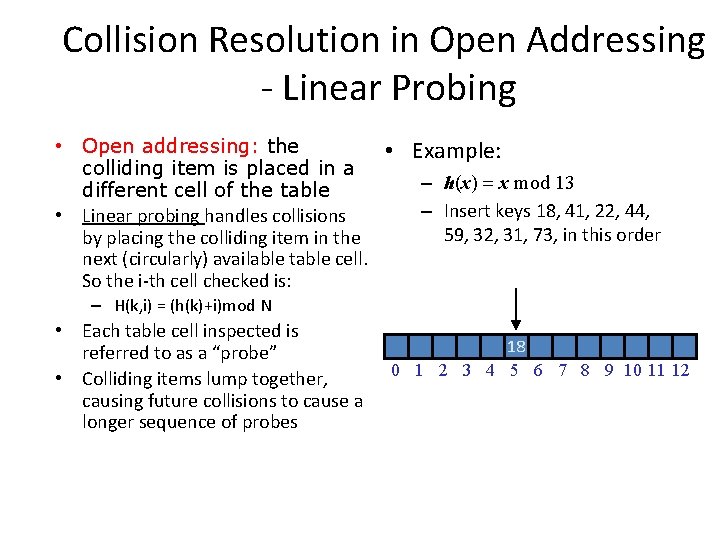
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 0 1 2 3 4 5 6 7 8 9 10 11 12
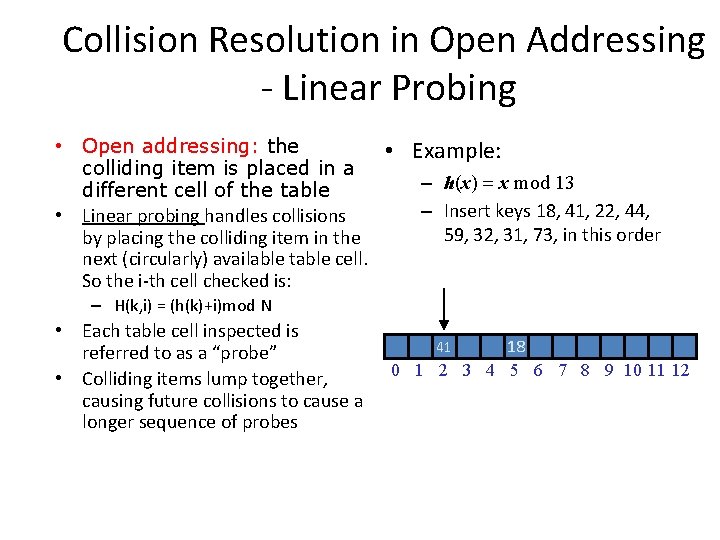
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 0 1 2 3 4 5 6 7 8 9 10 11 12 41
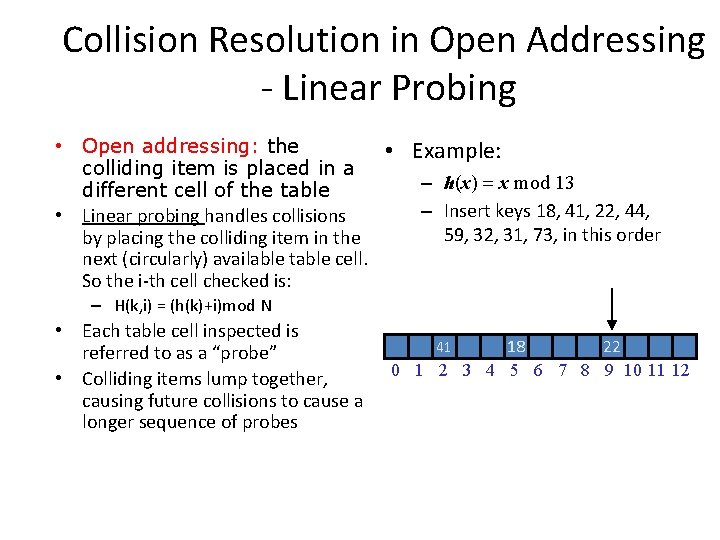
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
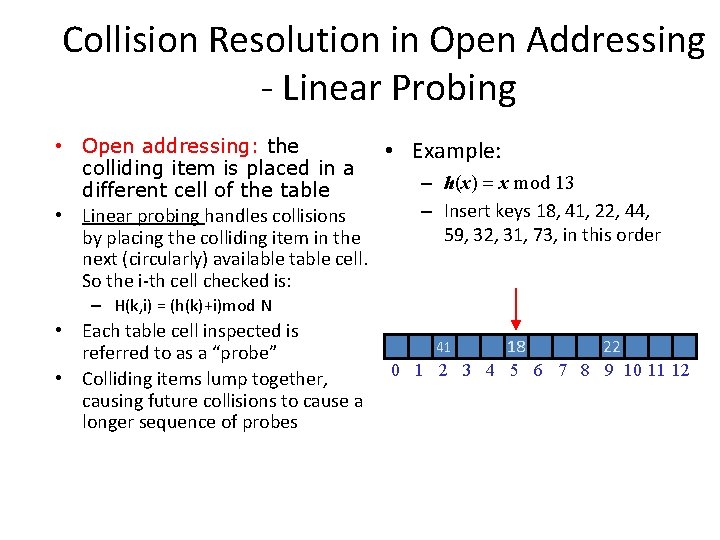
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
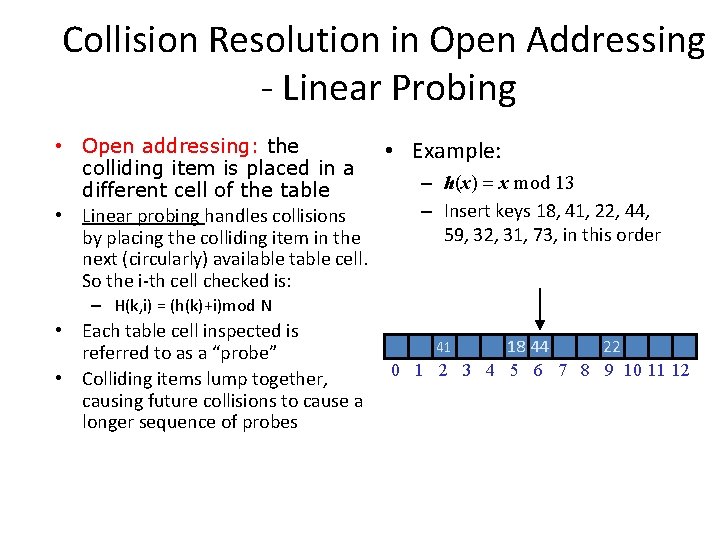
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
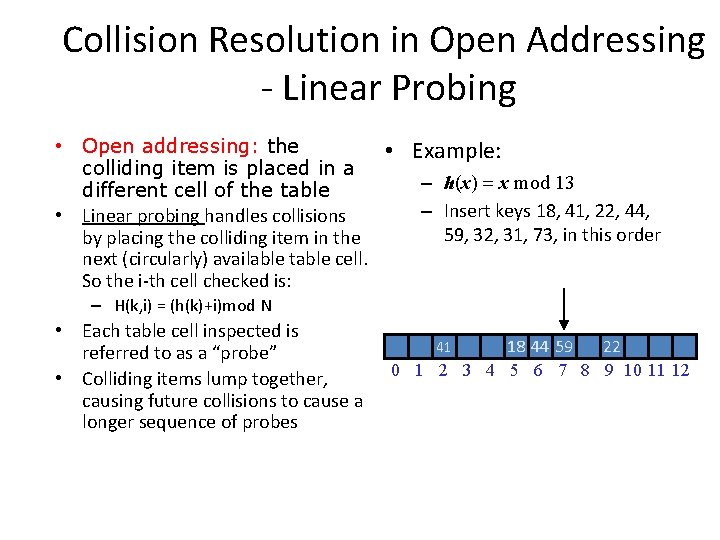
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
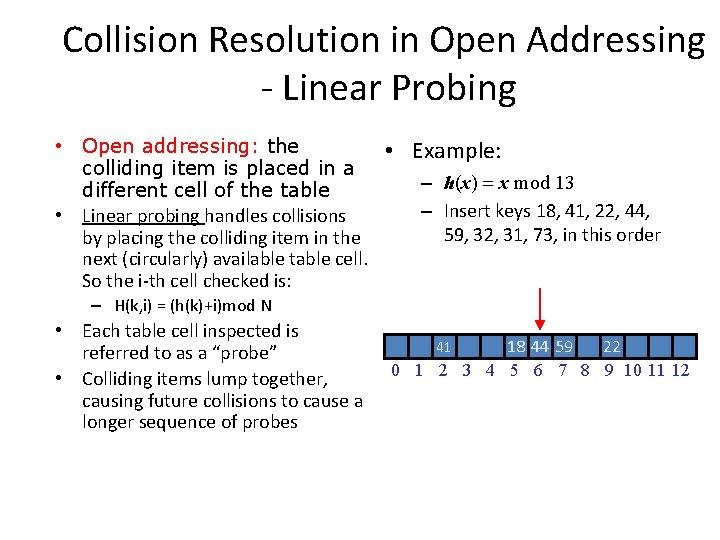
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
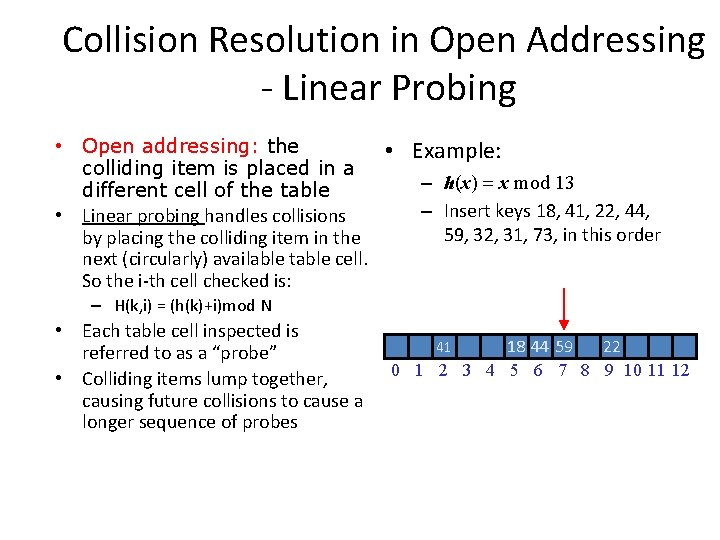
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
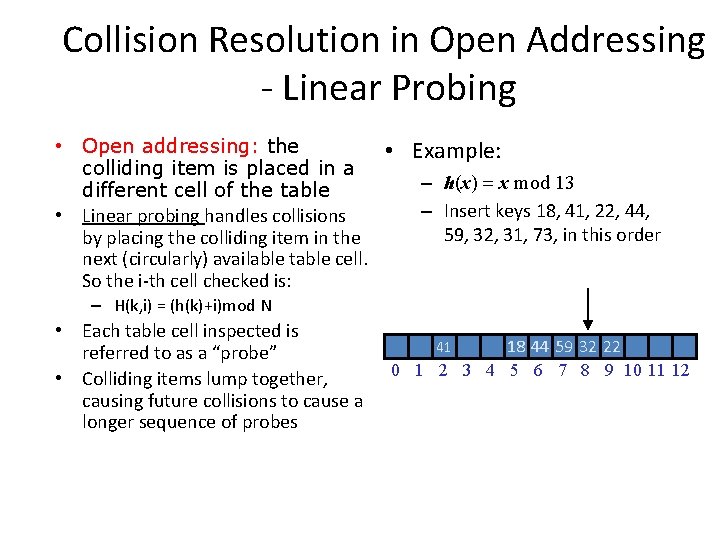
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
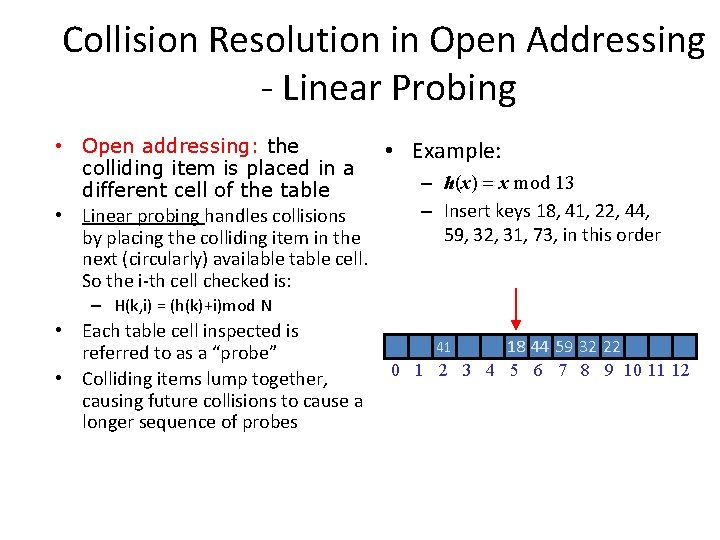
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
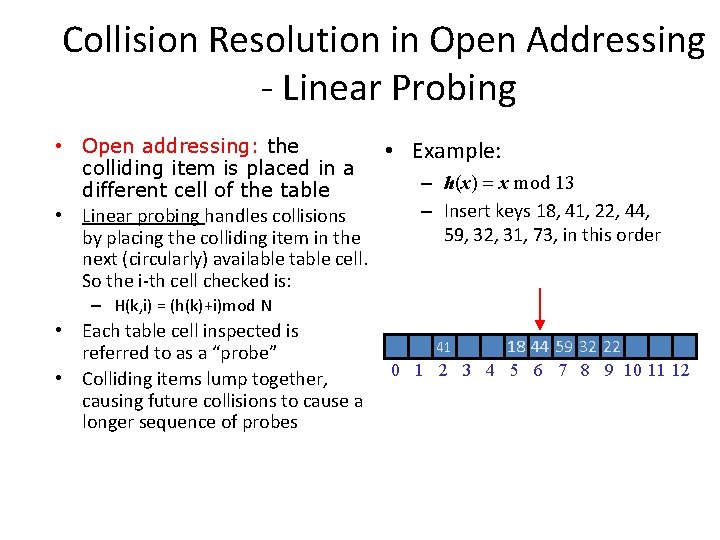
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
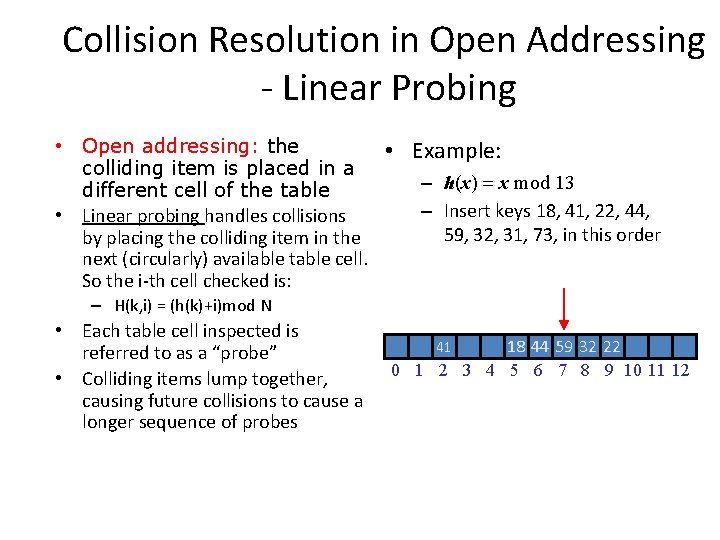
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
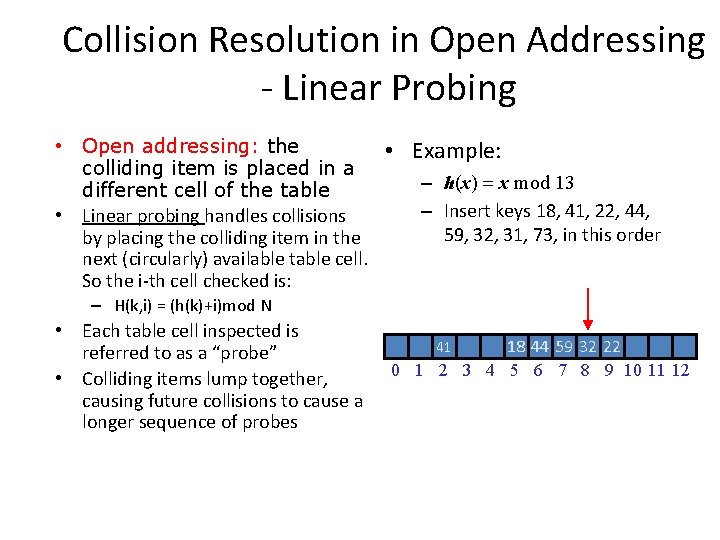
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
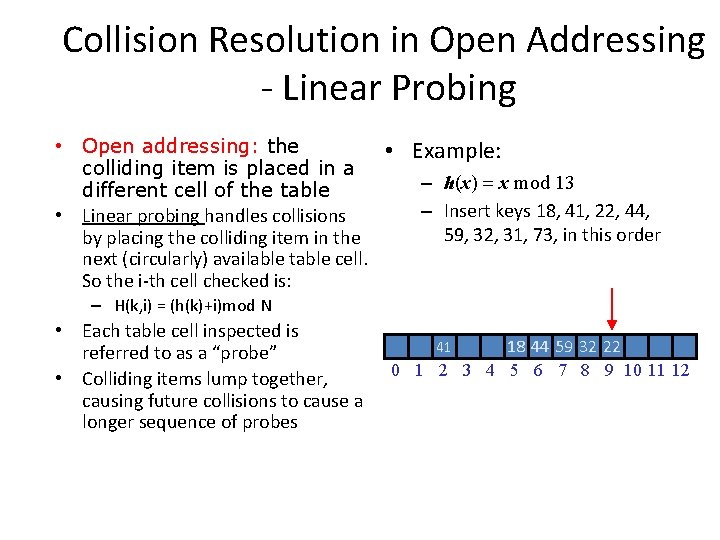
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 0 1 2 3 4 5 6 7 8 9 10 11 12 41
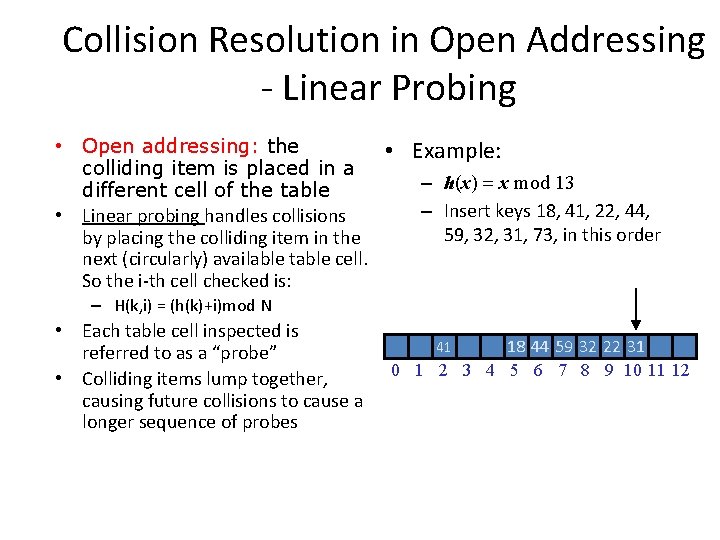
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 31 0 1 2 3 4 5 6 7 8 9 10 11 12 41
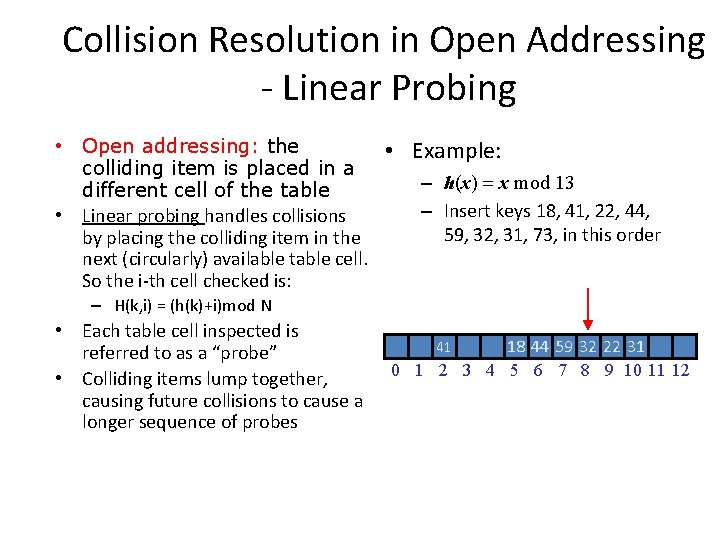
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 31 0 1 2 3 4 5 6 7 8 9 10 11 12 41
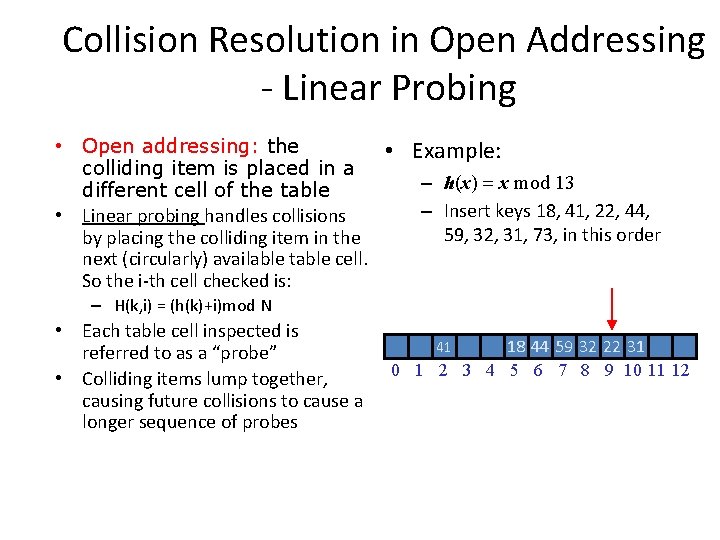
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 31 0 1 2 3 4 5 6 7 8 9 10 11 12 41
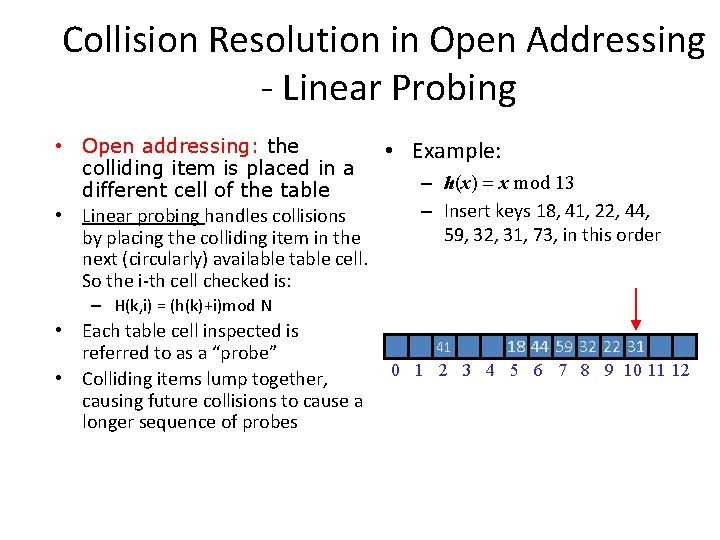
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 31 0 1 2 3 4 5 6 7 8 9 10 11 12 41
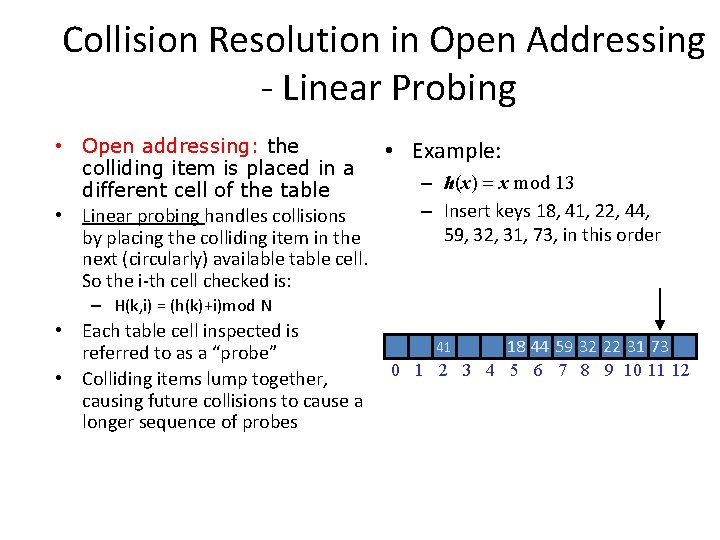
Collision Resolution in Open Addressing - Linear Probing • Open addressing: the • Example: colliding item is placed in a – h(x) = x mod 13 different cell of the table – Insert keys 18, 41, 22, 44, • Linear probing handles collisions 59, 32, 31, 73, in this order by placing the colliding item in the next (circularly) available table cell. So the i-th cell checked is: – H(k, i) = (h(k)+i)mod N • Each table cell inspected is referred to as a “probe” • Colliding items lump together, causing future collisions to cause a longer sequence of probes 18 44 59 32 22 31 73 0 1 2 3 4 5 6 7 8 9 10 11 12 41
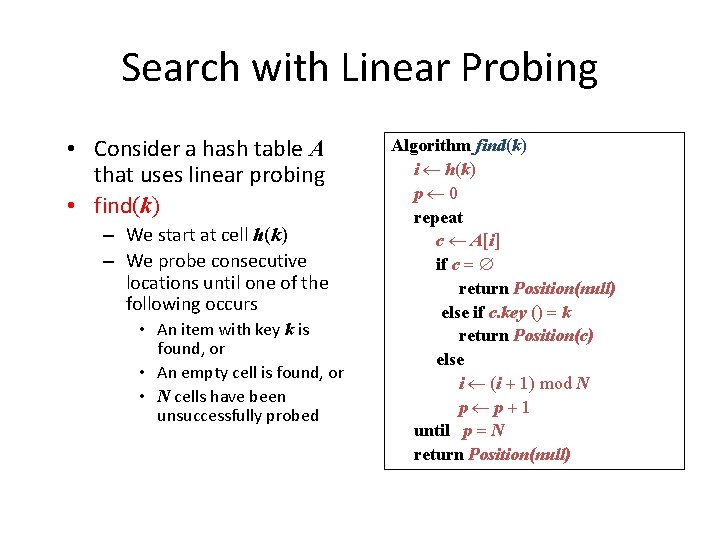
Search with Linear Probing • Consider a hash table A that uses linear probing • find(k) – We start at cell h(k) – We probe consecutive locations until one of the following occurs • An item with key k is found, or • An empty cell is found, or • N cells have been unsuccessfully probed Algorithm find(k) i h(k) p 0 repeat c A[i] if c = return Position(null) else if c. key () = k return Position(c) else i (i + 1) mod N p p+1 until p = N return Position(null)
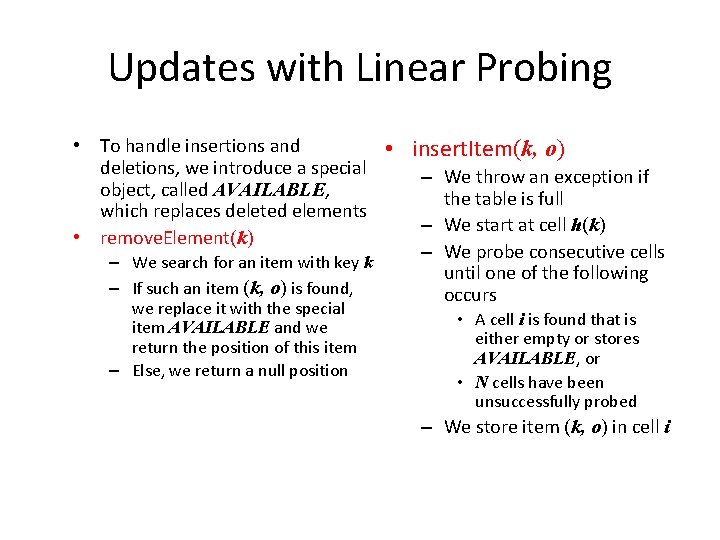
Updates with Linear Probing • To handle insertions and • insert. Item(k, o) deletions, we introduce a special – We throw an exception if object, called AVAILABLE, the table is full which replaces deleted elements – We start at cell h(k) • remove. Element(k) – We probe consecutive cells – We search for an item with key k until one of the following – If such an item (k, o) is found, occurs we replace it with the special item AVAILABLE and we return the position of this item – Else, we return a null position • A cell i is found that is either empty or stores AVAILABLE, or • N cells have been unsuccessfully probed – We store item (k, o) in cell i
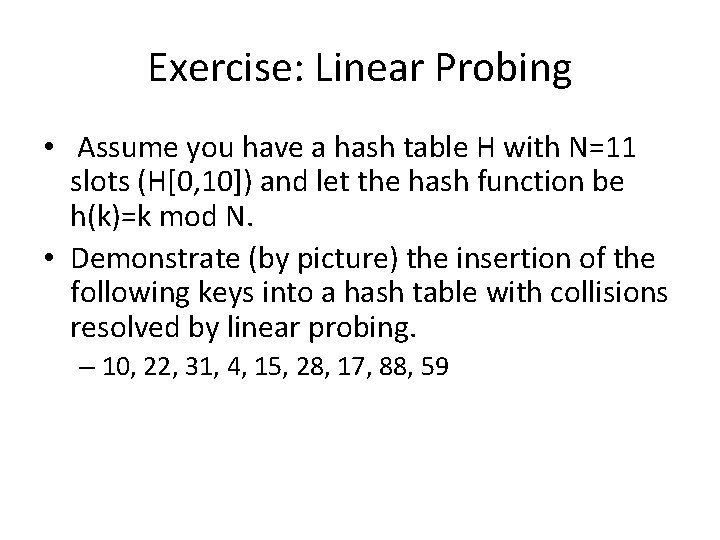
Exercise: Linear Probing • Assume you have a hash table H with N=11 slots (H[0, 10]) and let the hash function be h(k)=k mod N. • Demonstrate (by picture) the insertion of the following keys into a hash table with collisions resolved by linear probing. – 10, 22, 31, 4, 15, 28, 17, 88, 59
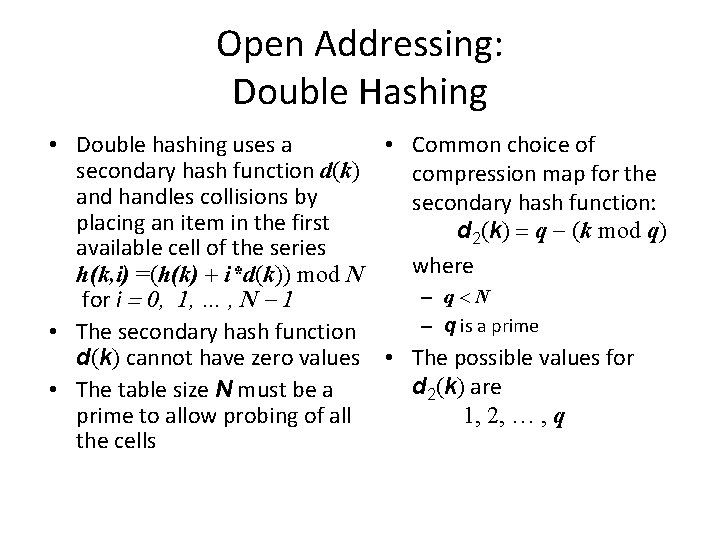
Open Addressing: Double Hashing • Double hashing uses a • Common choice of secondary hash function d(k) compression map for the and handles collisions by secondary hash function: placing an item in the first d 2(k) = q - (k mod q) available cell of the series where h(k, i) =(h(k) + i*d(k)) mod N – q<N for i = 0, 1, … , N - 1 – q is a prime • The secondary hash function d(k) cannot have zero values • The possible values for d 2(k) are • The table size N must be a prime to allow probing of all 1, 2, … , q the cells
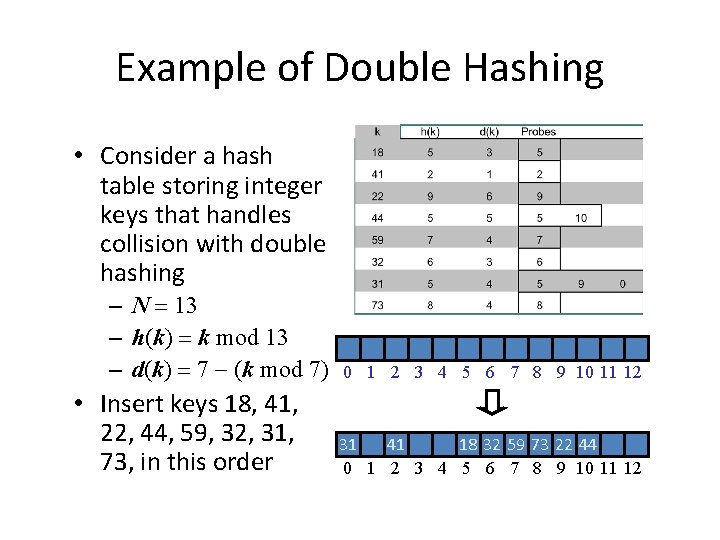
Example of Double Hashing • Consider a hash table storing integer keys that handles collision with double hashing – N = 13 – h(k) = k mod 13 – d(k) = 7 - (k mod 7) • Insert keys 18, 41, 22, 44, 59, 32, 31, 73, in this order 0 1 2 3 4 5 6 7 8 9 10 11 12 31 41 18 32 59 73 22 44 0 1 2 3 4 5 6 7 8 9 10 11 12
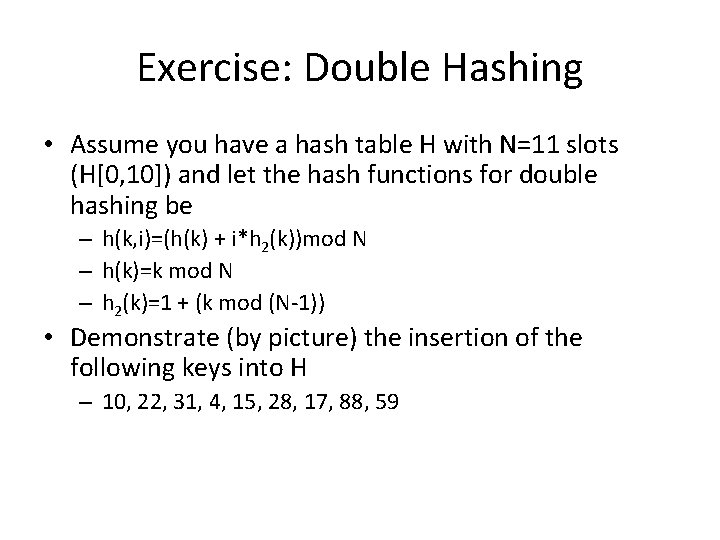
Exercise: Double Hashing • Assume you have a hash table H with N=11 slots (H[0, 10]) and let the hash functions for double hashing be – h(k, i)=(h(k) + i*h 2(k))mod N – h(k)=k mod N – h 2(k)=1 + (k mod (N-1)) • Demonstrate (by picture) the insertion of the following keys into H – 10, 22, 31, 4, 15, 28, 17, 88, 59
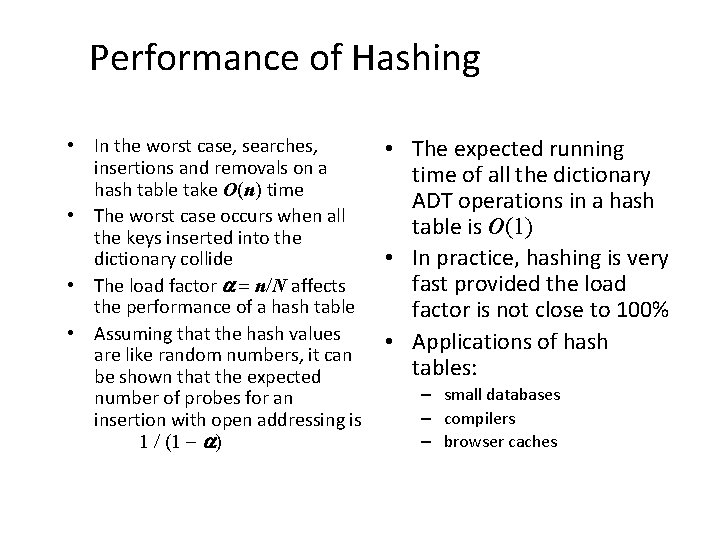
Performance of Hashing • In the worst case, searches, insertions and removals on a hash table take O(n) time • The worst case occurs when all the keys inserted into the dictionary collide • The load factor a = n/N affects the performance of a hash table • Assuming that the hash values are like random numbers, it can be shown that the expected number of probes for an insertion with open addressing is 1 / (1 - a) • The expected running time of all the dictionary ADT operations in a hash table is O(1) • In practice, hashing is very fast provided the load factor is not close to 100% • Applications of hash tables: – small databases – compilers – browser caches
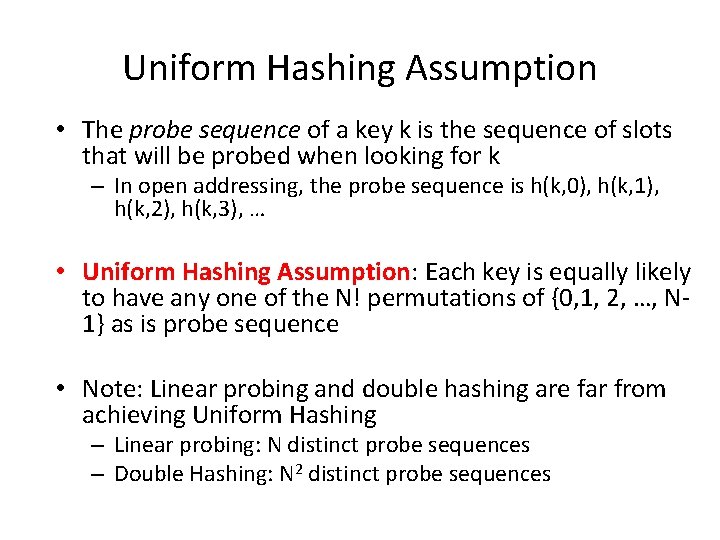
Uniform Hashing Assumption • The probe sequence of a key k is the sequence of slots that will be probed when looking for k – In open addressing, the probe sequence is h(k, 0), h(k, 1), h(k, 2), h(k, 3), … • Uniform Hashing Assumption: Each key is equally likely to have any one of the N! permutations of {0, 1, 2, …, N 1} as is probe sequence • Note: Linear probing and double hashing are far from achieving Uniform Hashing – Linear probing: N distinct probe sequences – Double Hashing: N 2 distinct probe sequences
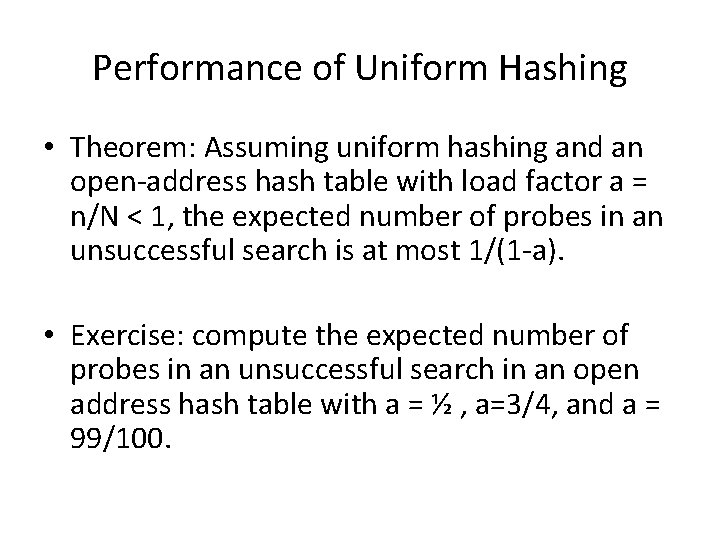
Performance of Uniform Hashing • Theorem: Assuming uniform hashing and an open-address hash table with load factor a = n/N < 1, the expected number of probes in an unsuccessful search is at most 1/(1 -a). • Exercise: compute the expected number of probes in an unsuccessful search in an open address hash table with a = ½ , a=3/4, and a = 99/100.
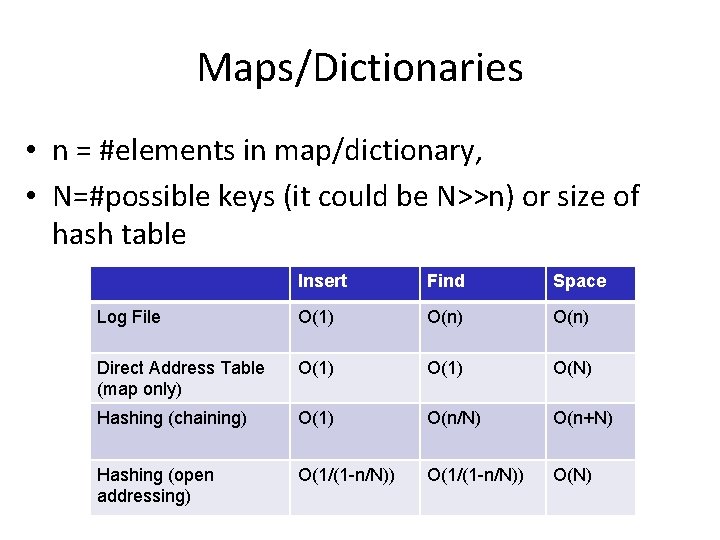
Maps/Dictionaries • n = #elements in map/dictionary, • N=#possible keys (it could be N>>n) or size of hash table Insert Find Space Log File O(1) O(n) Direct Address Table (map only) O(1) O(N) Hashing (chaining) O(1) O(n/N) O(n+N) Hashing (open addressing) O(1/(1 -n/N)) O(N)
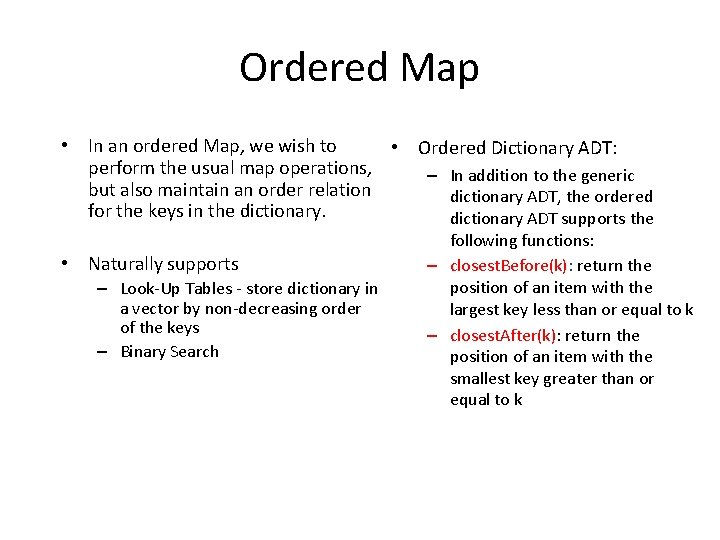
Ordered Map • In an ordered Map, we wish to • Ordered Dictionary ADT: perform the usual map operations, – In addition to the generic but also maintain an order relation dictionary ADT, the ordered for the keys in the dictionary ADT supports the • Naturally supports – Look-Up Tables - store dictionary in a vector by non-decreasing order of the keys – Binary Search following functions: – closest. Before(k): return the position of an item with the largest key less than or equal to k – closest. After(k): return the position of an item with the smallest key greater than or equal to k
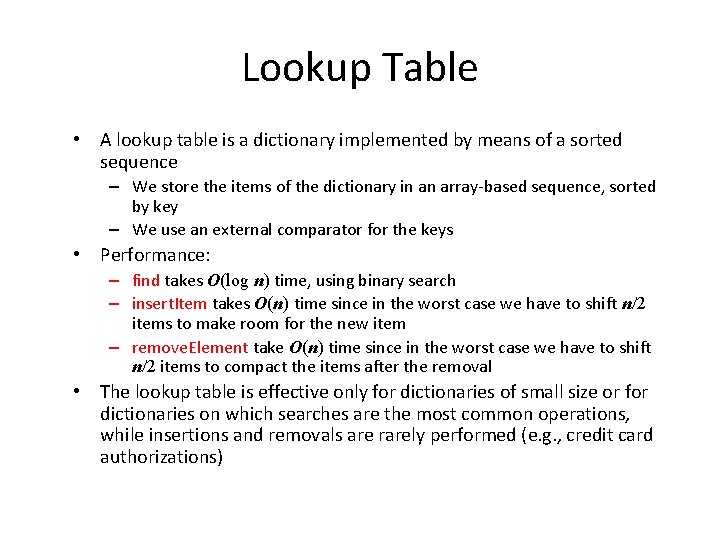
Lookup Table • A lookup table is a dictionary implemented by means of a sorted sequence – We store the items of the dictionary in an array-based sequence, sorted by key – We use an external comparator for the keys • Performance: – find takes O(log n) time, using binary search – insert. Item takes O(n) time since in the worst case we have to shift n/2 items to make room for the new item – remove. Element take O(n) time since in the worst case we have to shift n/2 items to compact the items after the removal • The lookup table is effective only for dictionaries of small size or for dictionaries on which searches are the most common operations, while insertions and removals are rarely performed (e. g. , credit card authorizations)
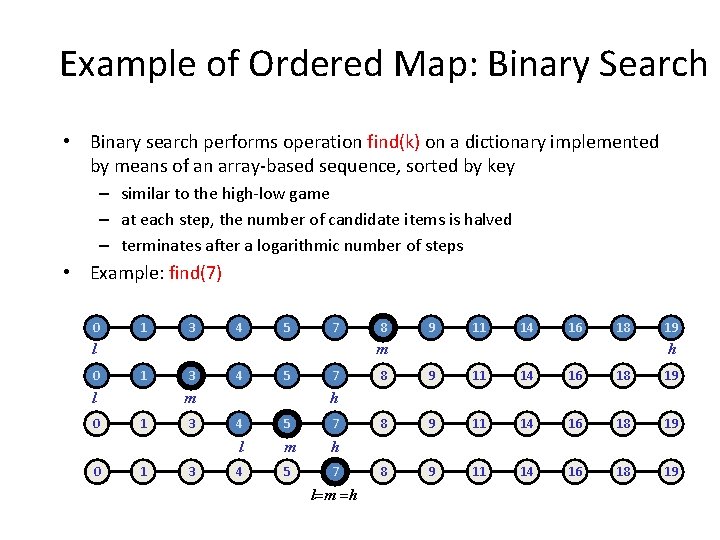
Example of Ordered Map: Binary Search • Binary search performs operation find(k) on a dictionary implemented by means of an array-based sequence, sorted by key – similar to the high-low game – at each step, the number of candidate items is halved – terminates after a logarithmic number of steps • Example: find(7) 0 1 3 4 5 7 1 0 3 4 5 m l 0 9 11 14 16 18 m l 0 8 1 1 3 3 7 19 h 8 9 11 14 16 18 19 h 4 5 7 l m h 4 5 7 l=m =h
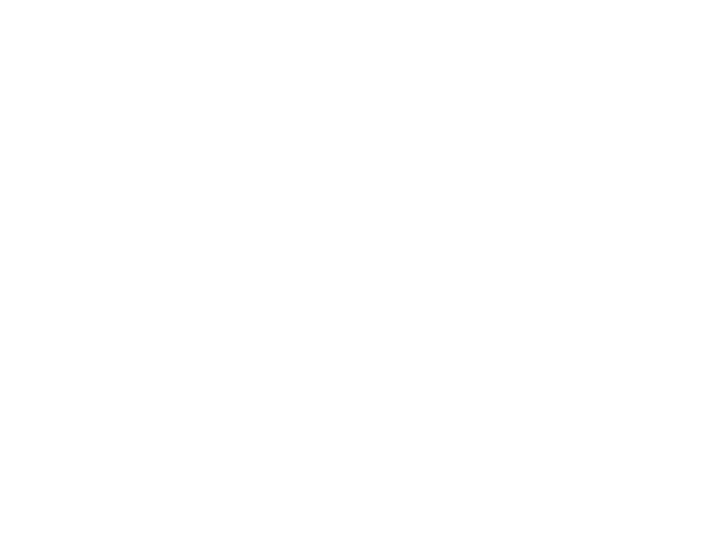
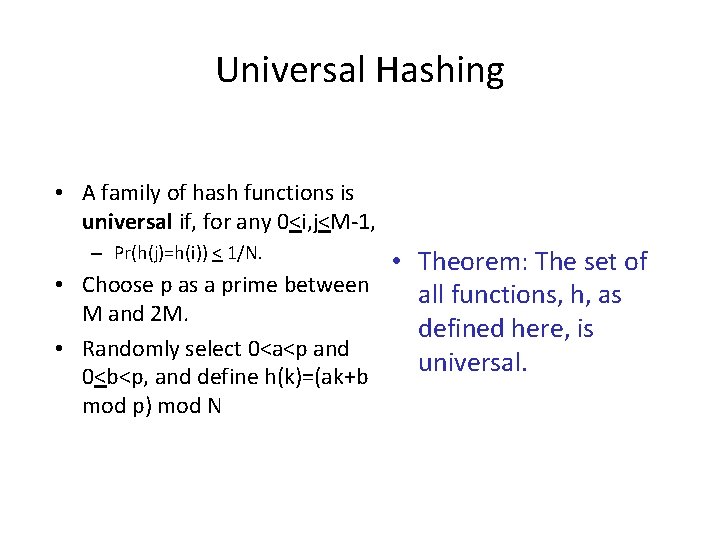
Universal Hashing • A family of hash functions is universal if, for any 0<i, j<M-1, – Pr(h(j)=h(i)) < 1/N. • Theorem: The set of • Choose p as a prime between all functions, h, as M and 2 M. defined here, is • Randomly select 0<a<p and universal. 0<b<p, and define h(k)=(ak+b mod p) mod N
What is open hashing and closed hashing
Dynamic hashing
Static hashing and dynamic hashing
Linear hashing
Pre reading while reading and post reading activities
Dictionaries are mutable
Analyzing systems using data dictionaries
Types of dictionary
Types of dictionaries
Types of dictionaries
Database of latin dictionaries
Maps google maps reittihaku
Map adt java
Map adt
It in a sentence
Story maps for reading comprehension
Reading weather maps
Hachure lines topographic map
Shear strain symbol
Encoding and encryption
Probability theory in hashing and load balancing
Difference between hashing and skip list
Hash goals
Password hashing and preprocessing
Password hashing and preprocessing
Tree map thinking maps
Sequence thinking map
Reading aims
Types of reading skill
Intensive reading and extensive reading
Extensive reading
How to develop reading skills in students
Reading outline example
Antarctica outline map
Bagaimana mencari data hashing dengan kunci modulus n?
Static hashing
Spectral hashing
Double hashing
Linear probing
Extendible hashing deletion
Hash multiplication method
Mid-square hash function
Quadratic hashing
Separate chaining rehash
Hashing exercises
Hashing collision resolution
Alexandra stefan
What is hashing in database management system
Tables de 14