Java Sockets and Server Sockets Low Level Network
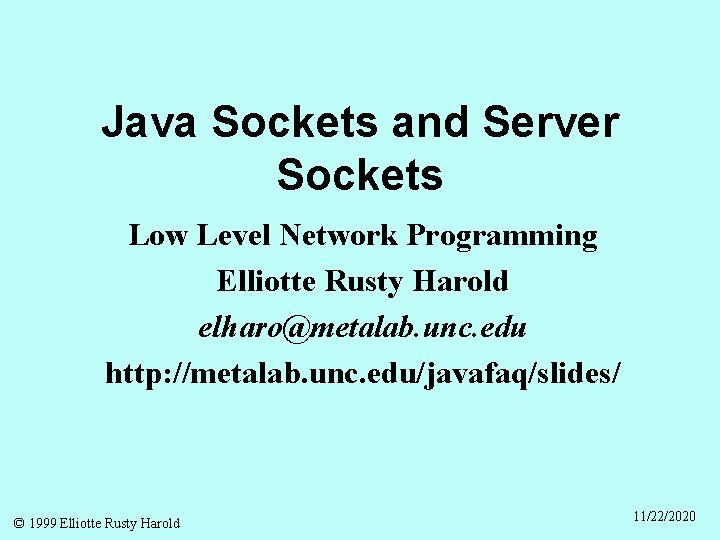
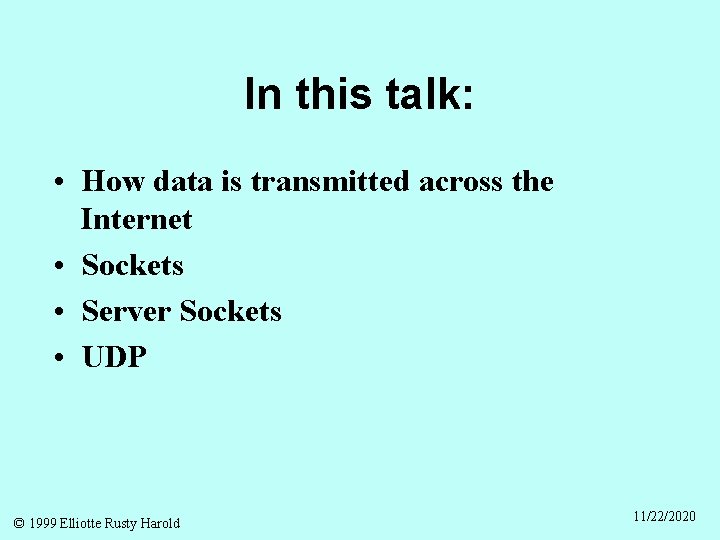
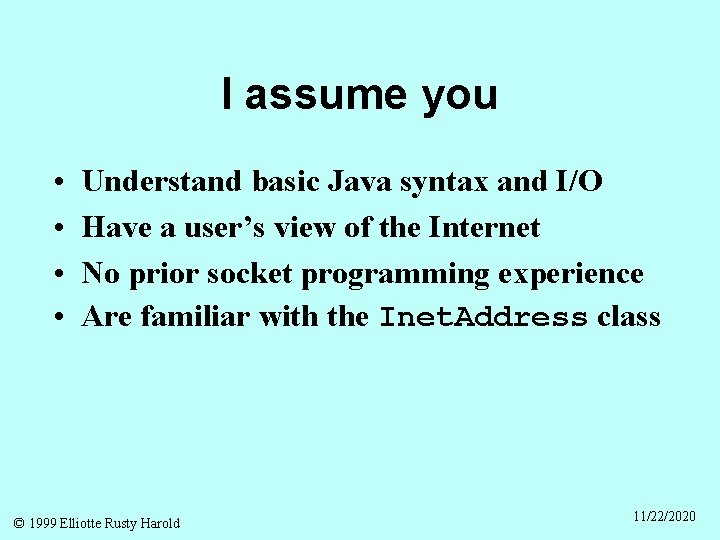
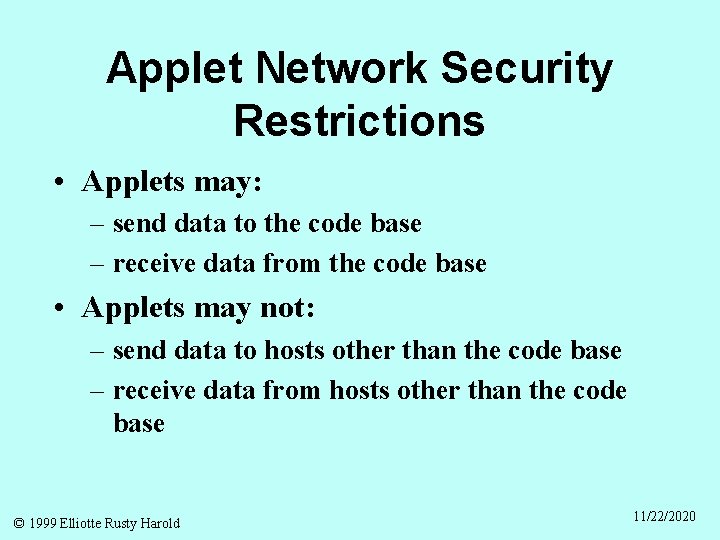
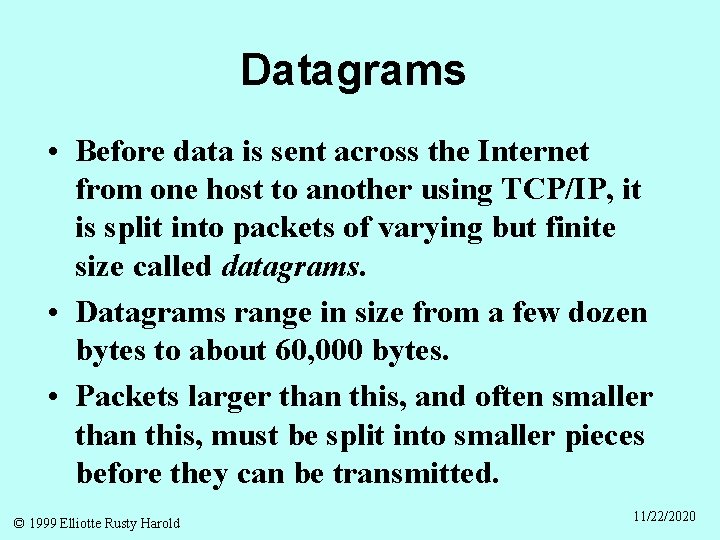
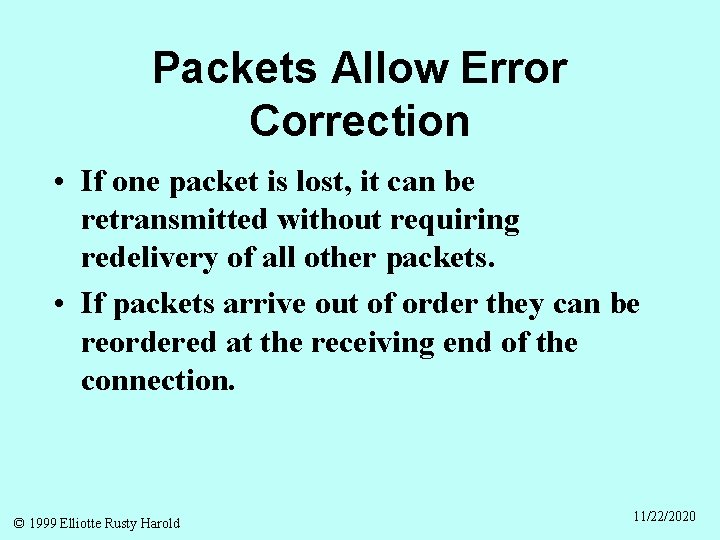
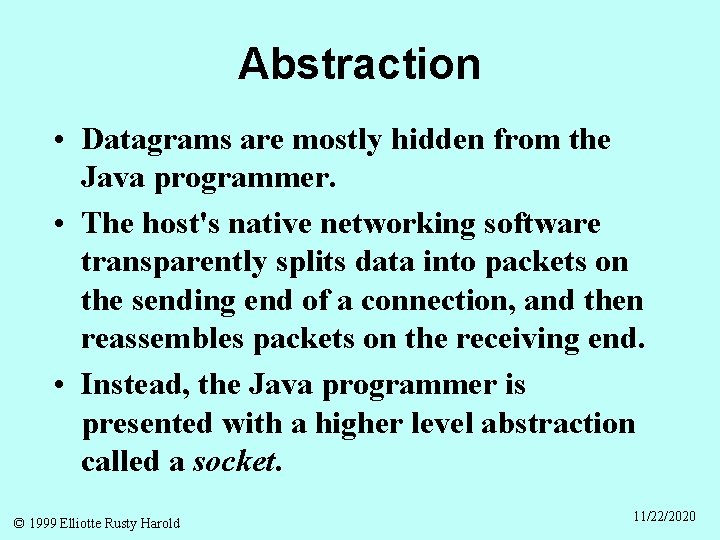
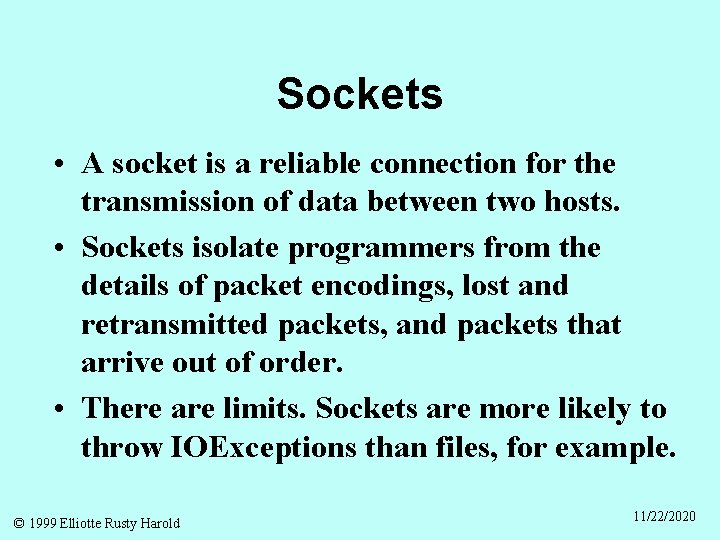
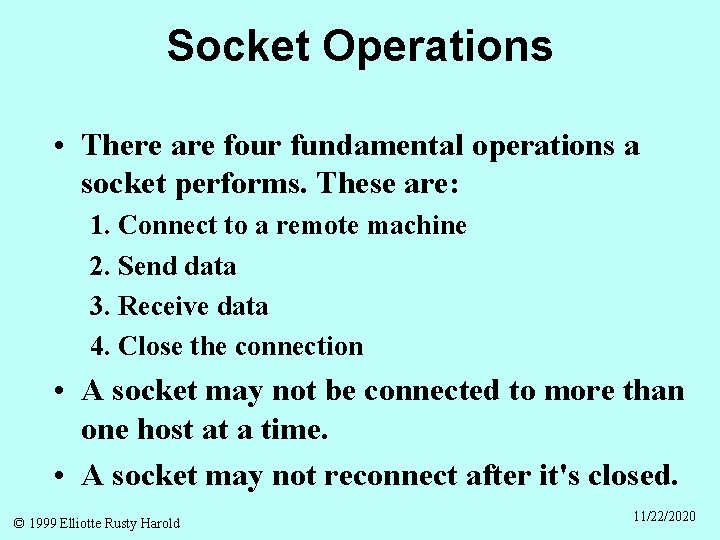
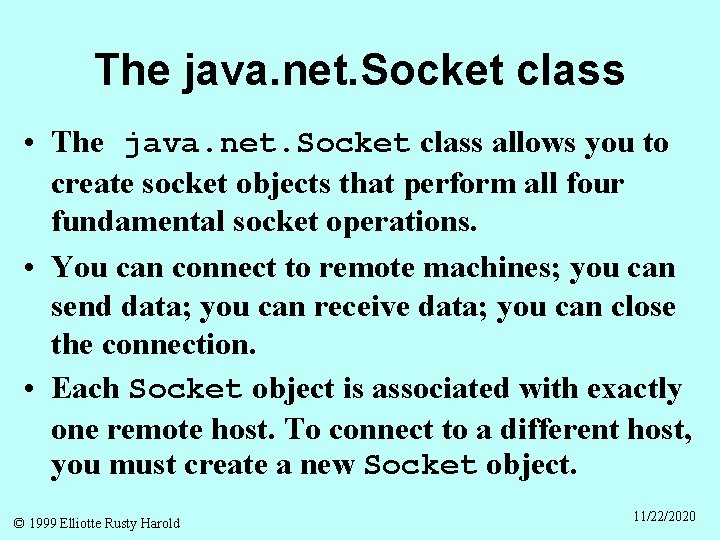
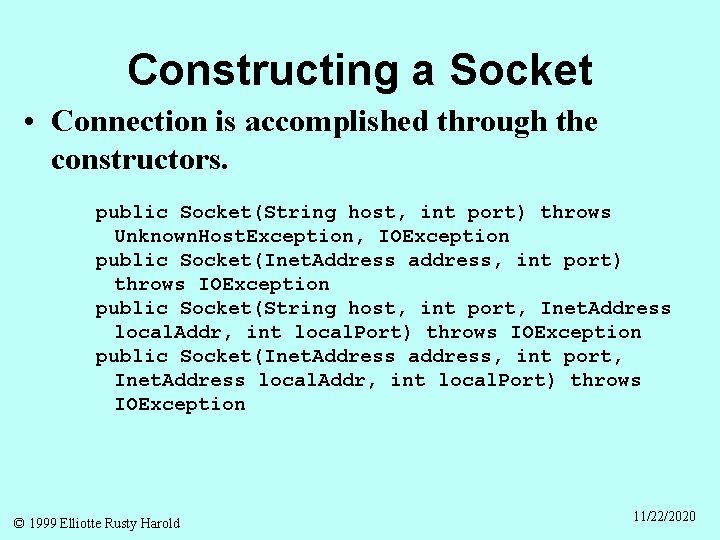
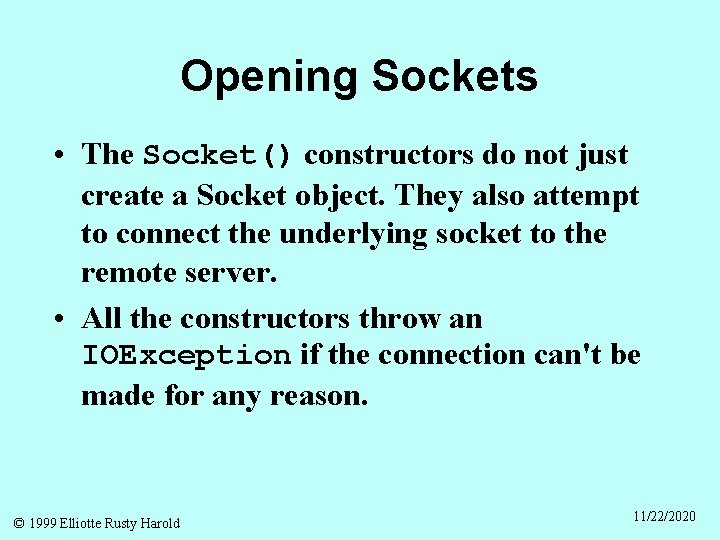
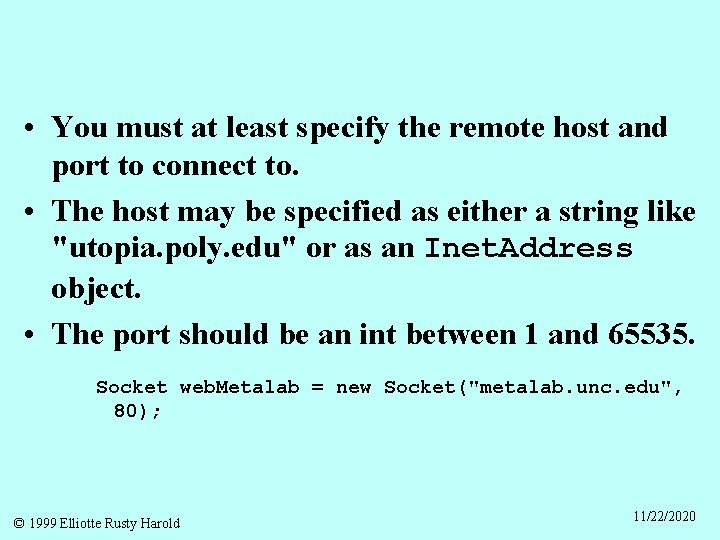
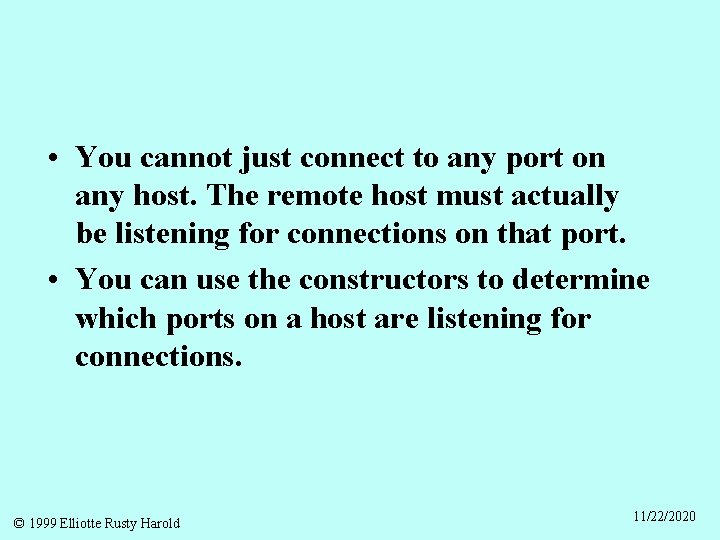
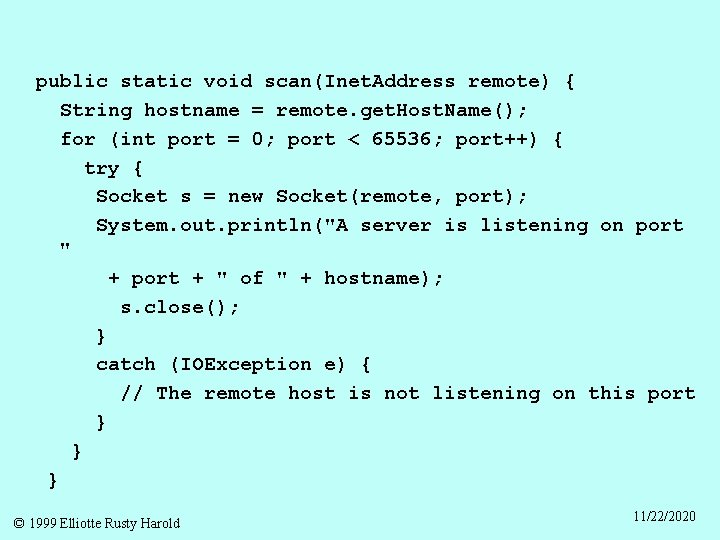
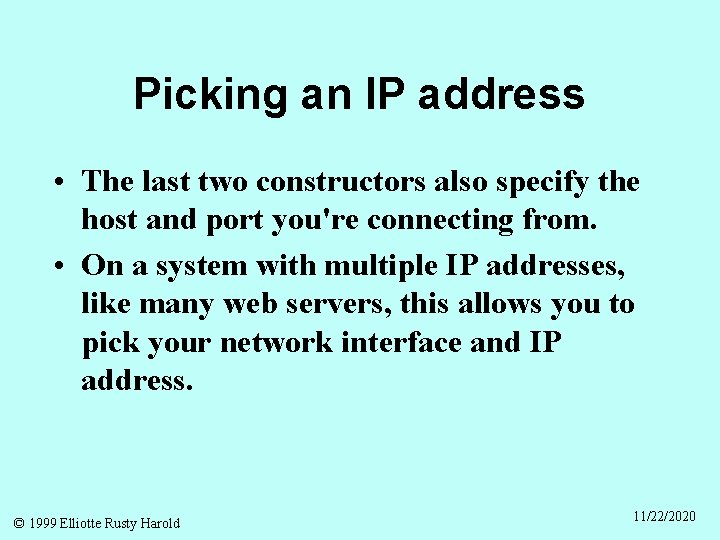
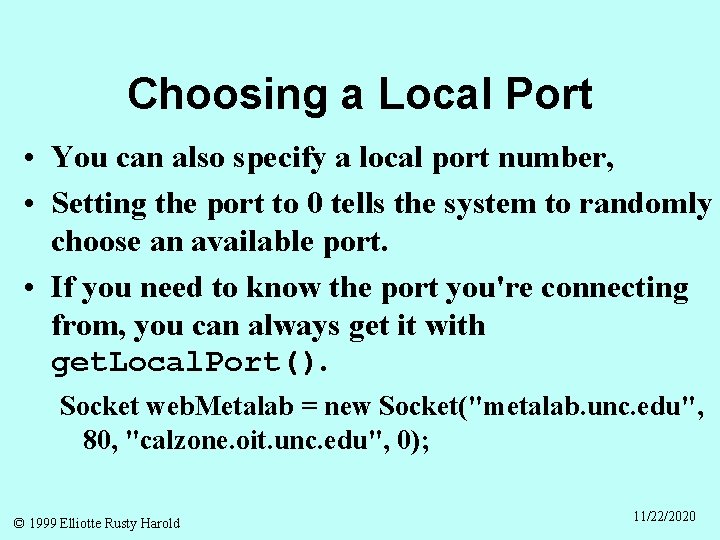
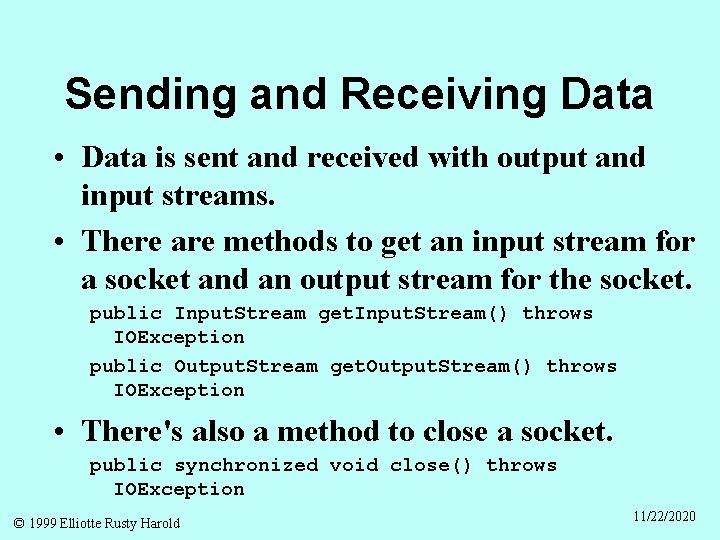
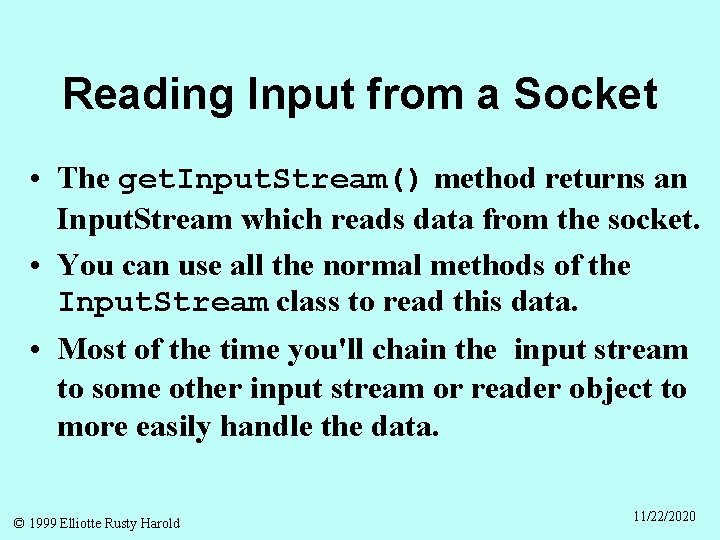
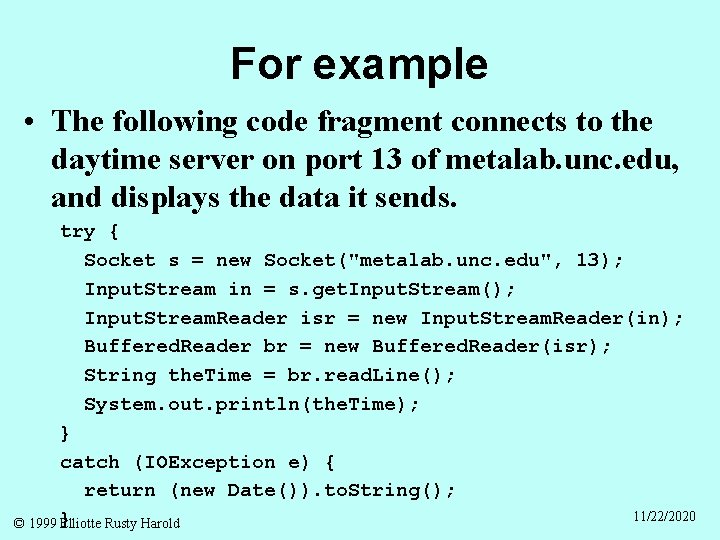
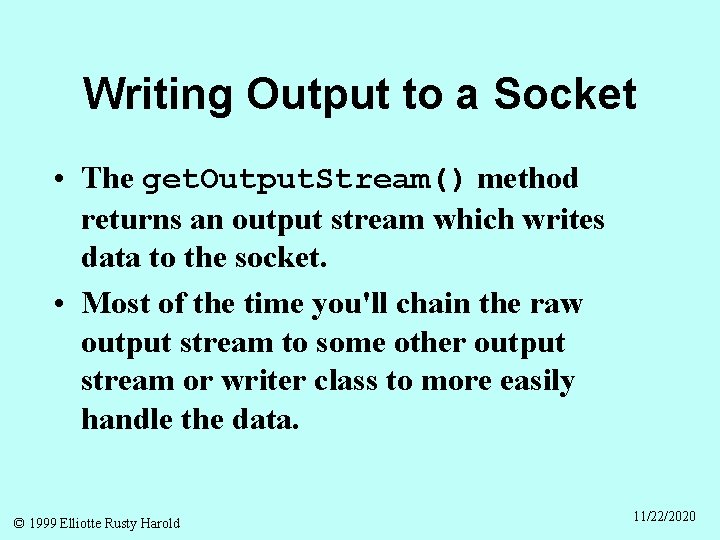
![Discard byte[] b = new byte[128]; try { Socket s = new Socket("metalab. unc. Discard byte[] b = new byte[128]; try { Socket s = new Socket("metalab. unc.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-22.jpg)
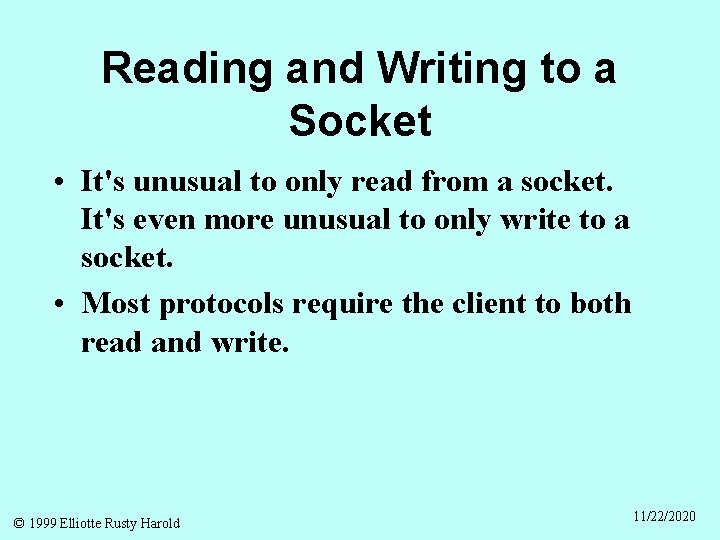
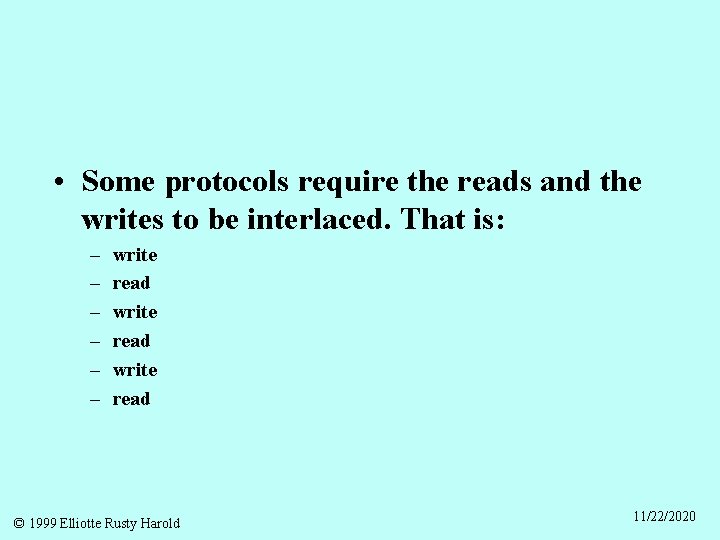
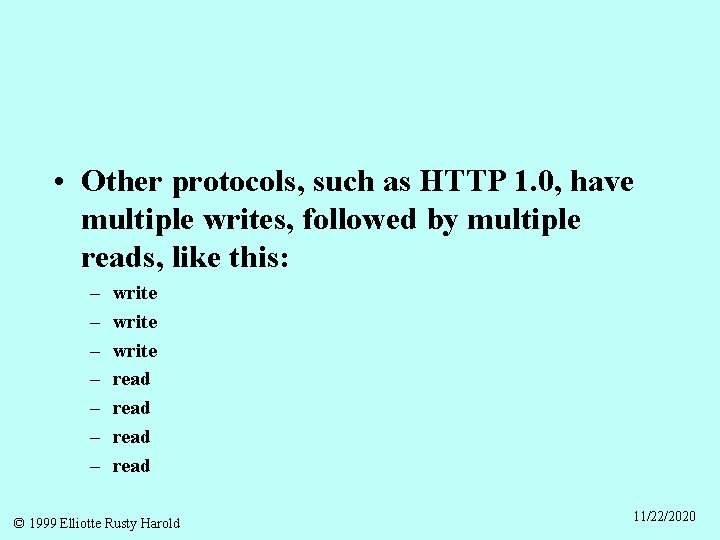
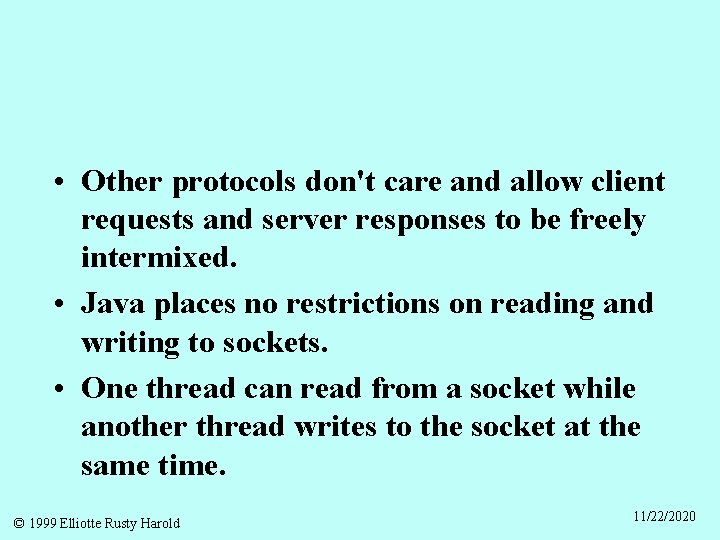
![try { URL u = new URL(args[i]); if (u. get. Port() != -1) port try { URL u = new URL(args[i]); if (u. get. Port() != -1) port](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-27.jpg)
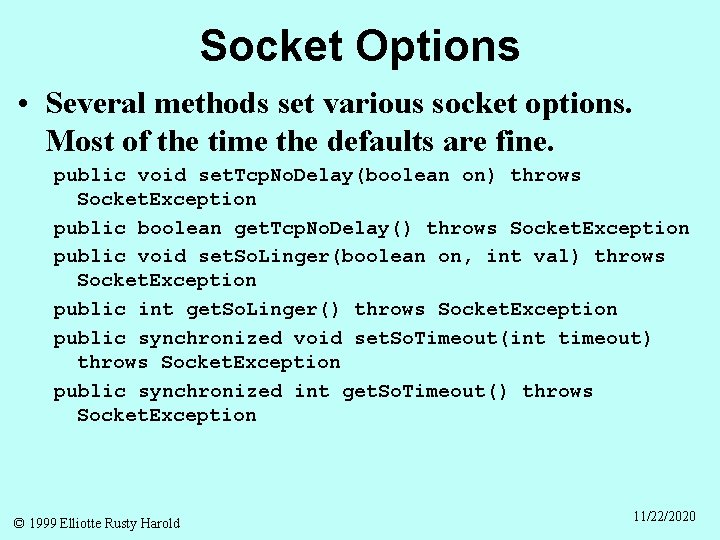
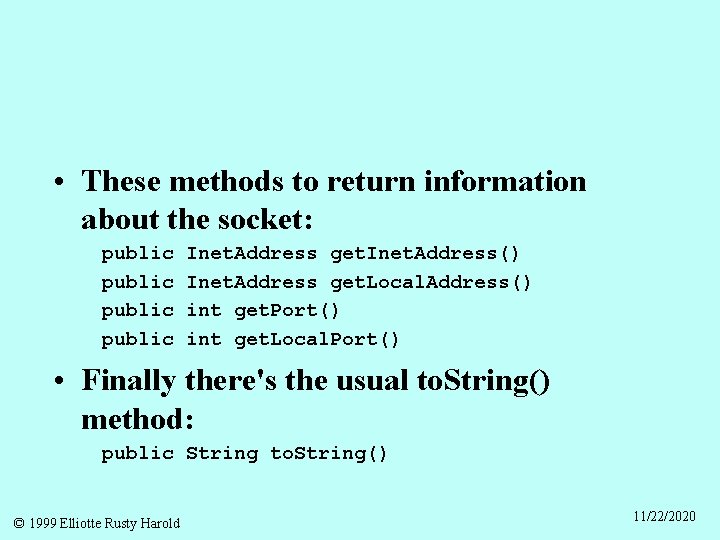
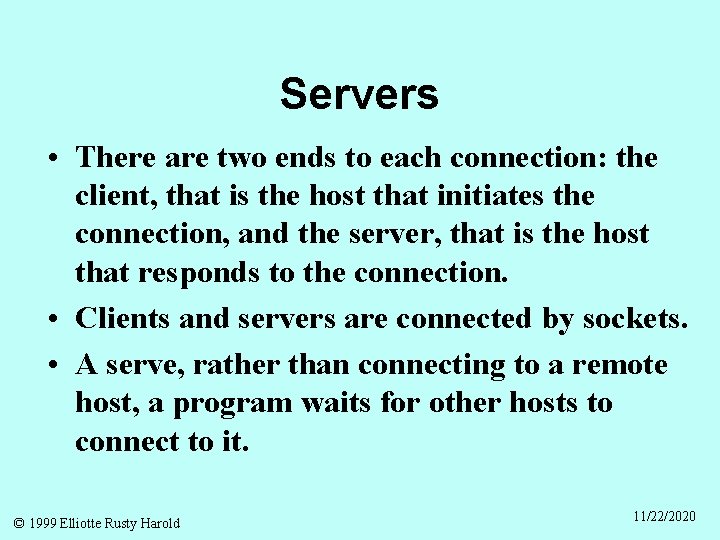
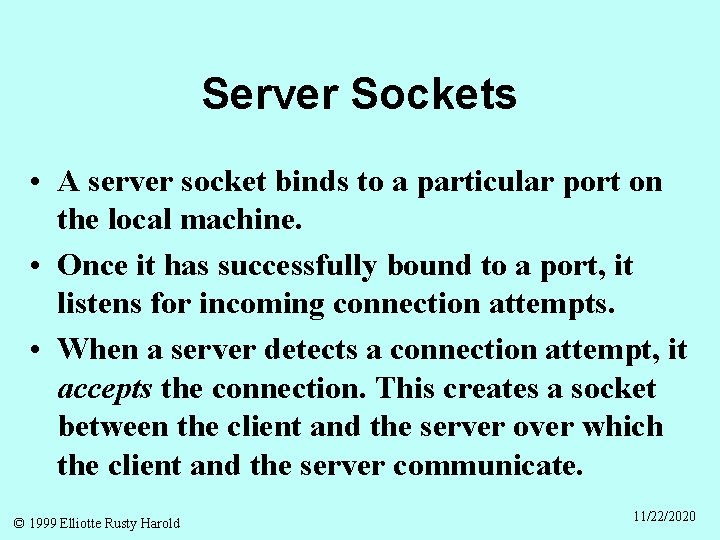
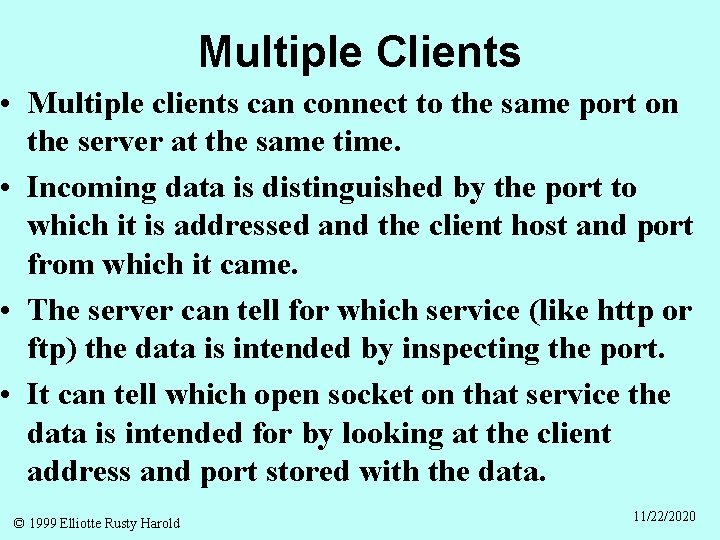
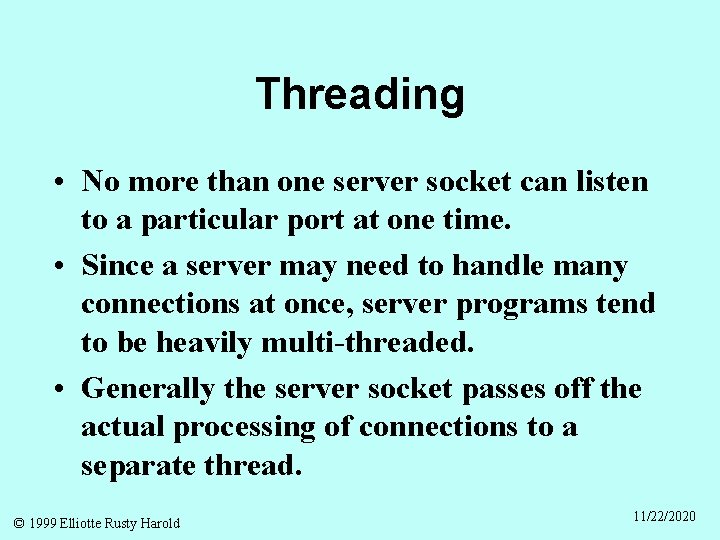
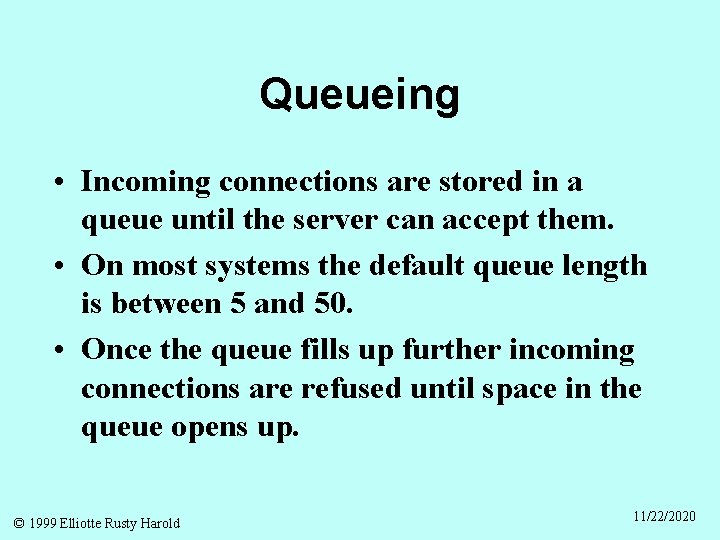
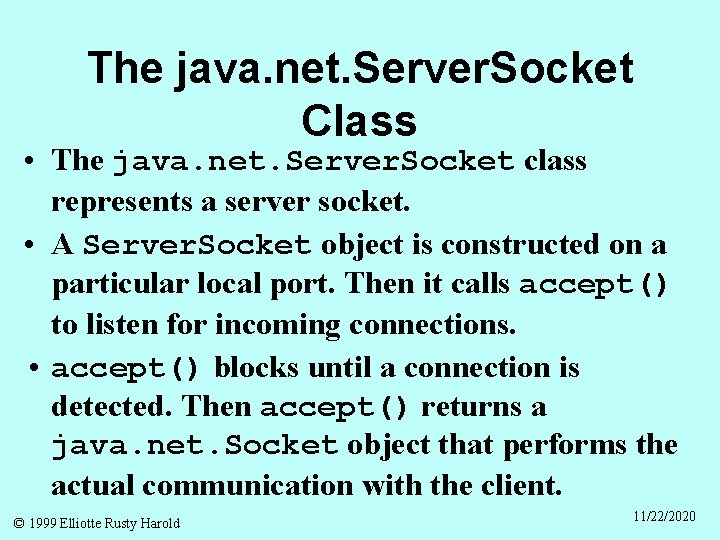
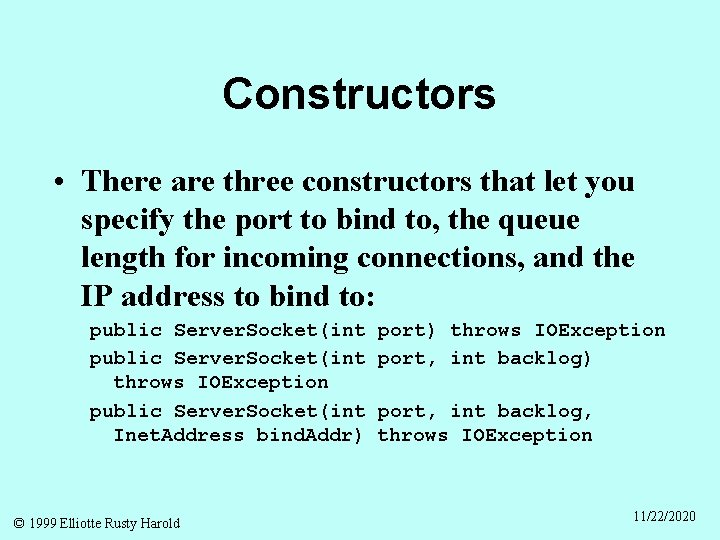
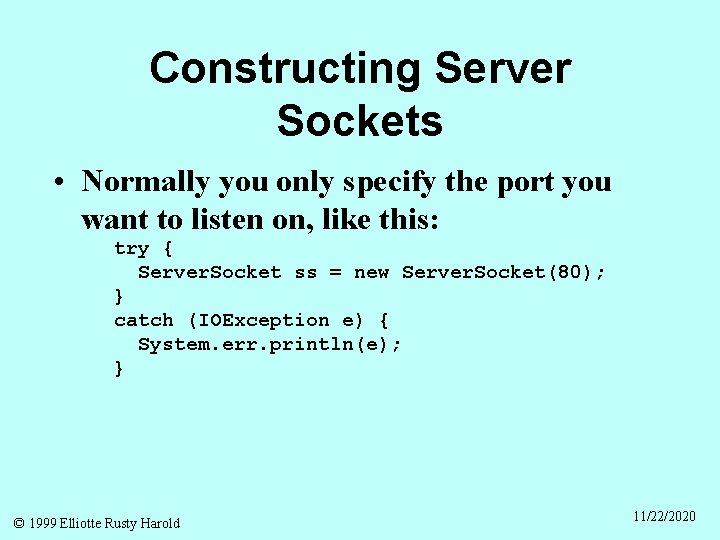
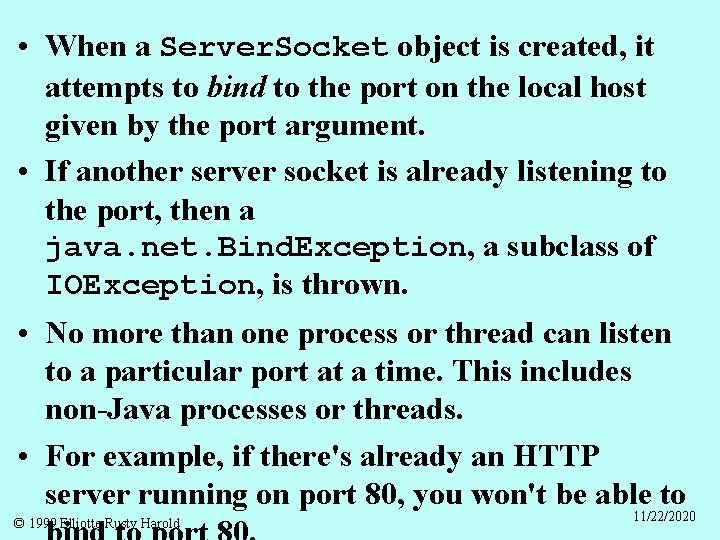
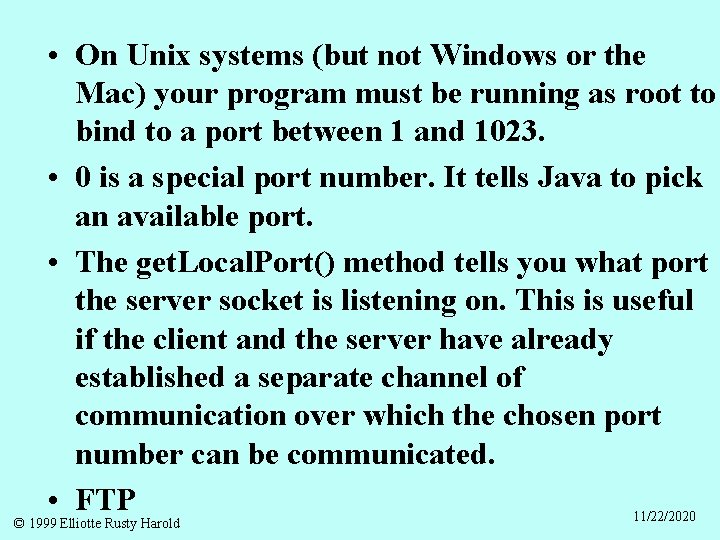
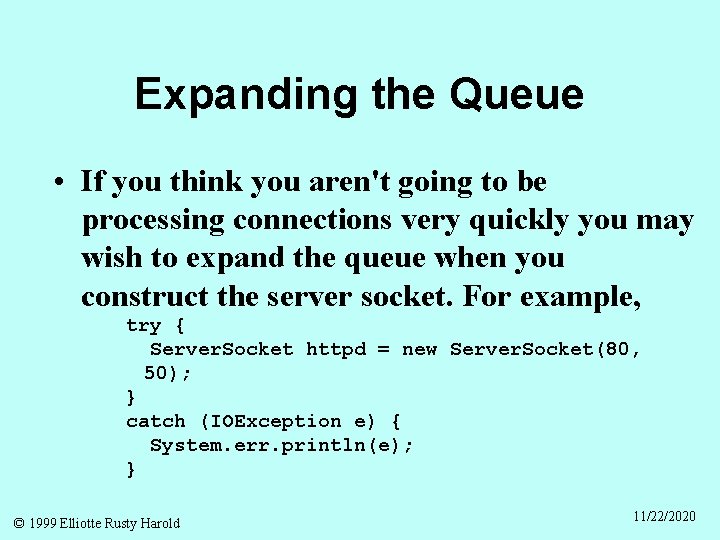
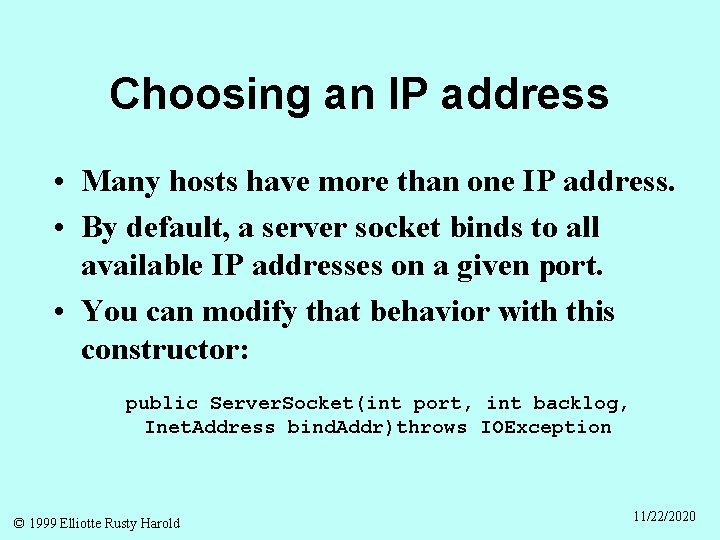
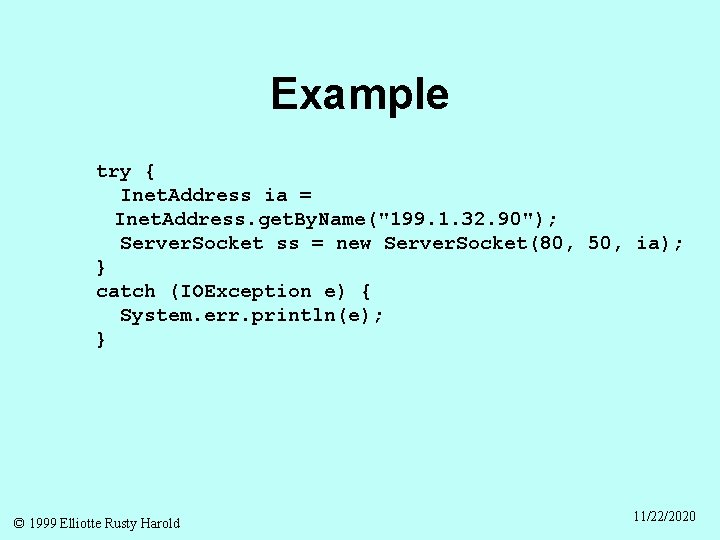
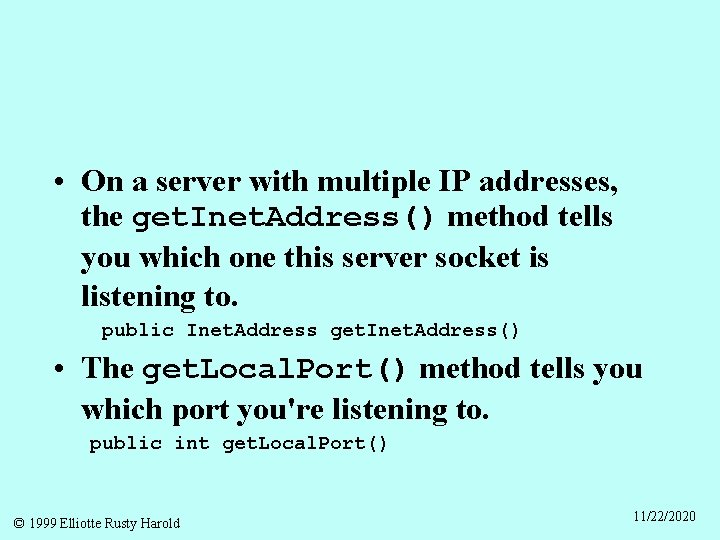
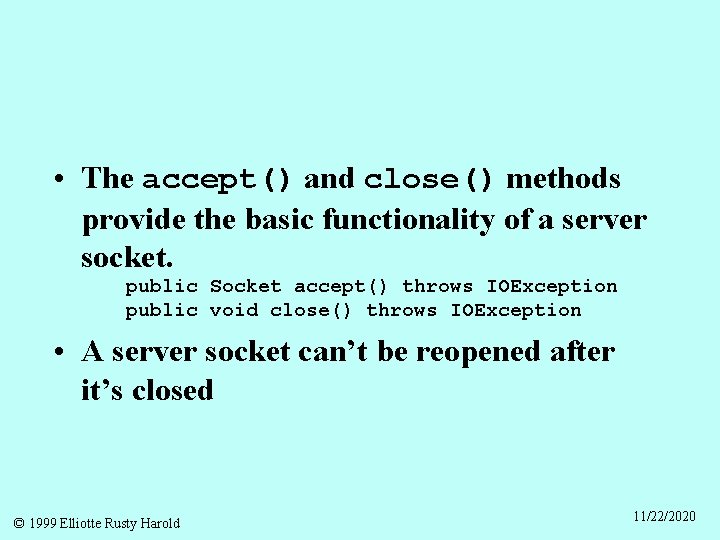
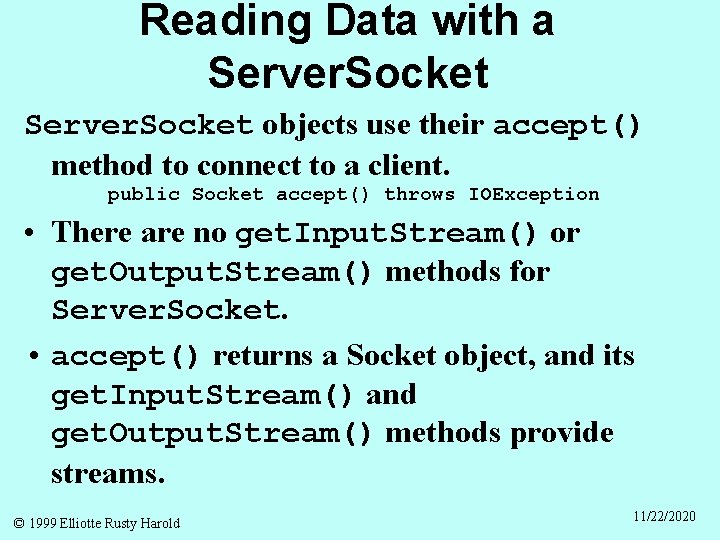
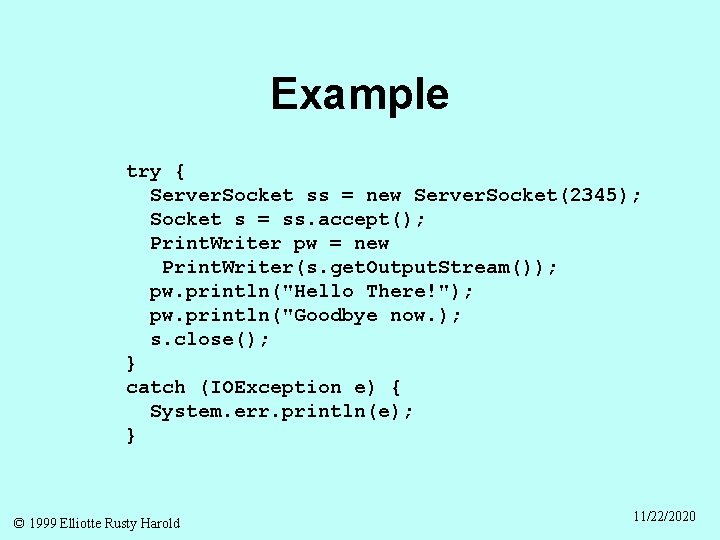
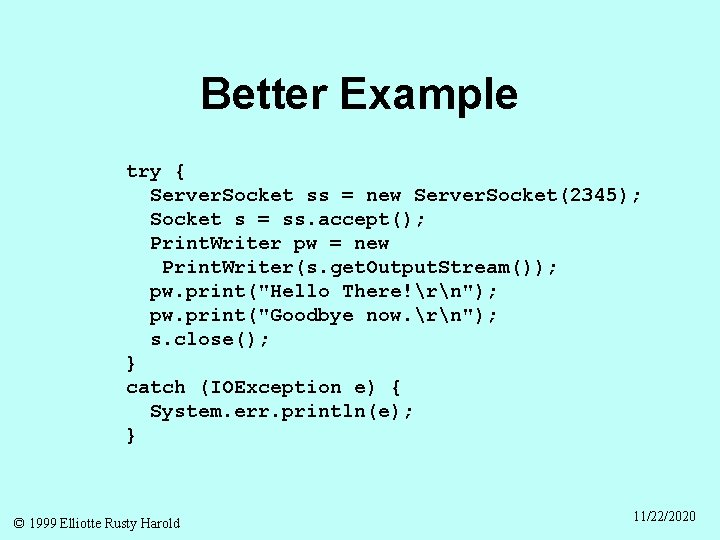
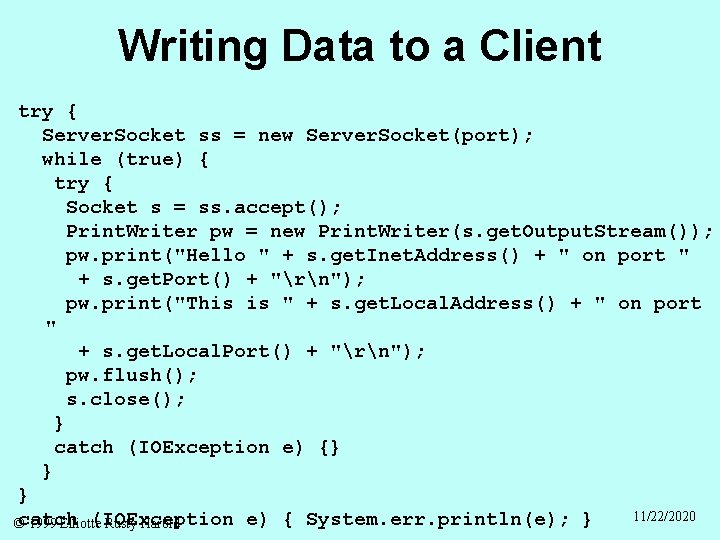
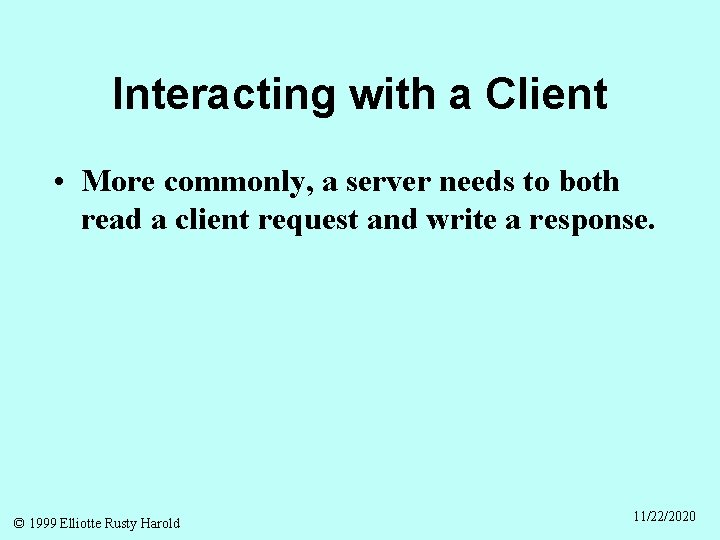
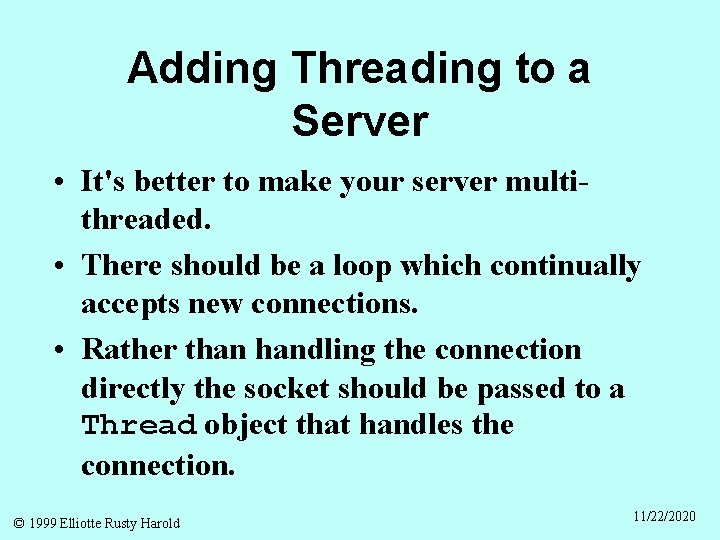
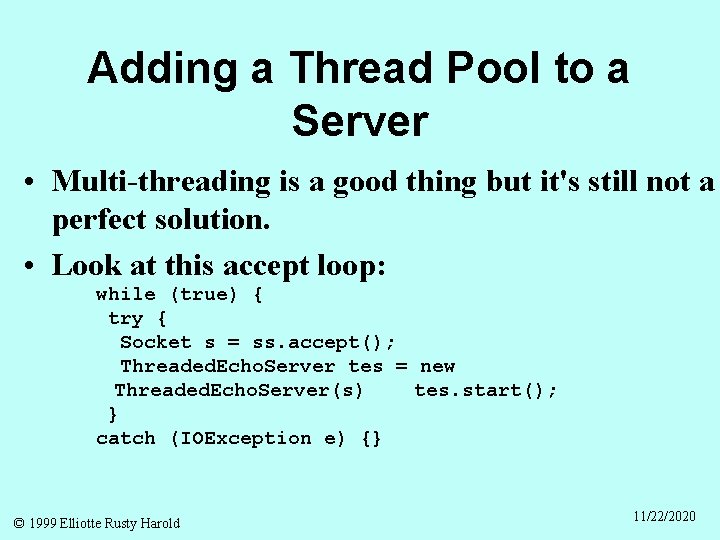
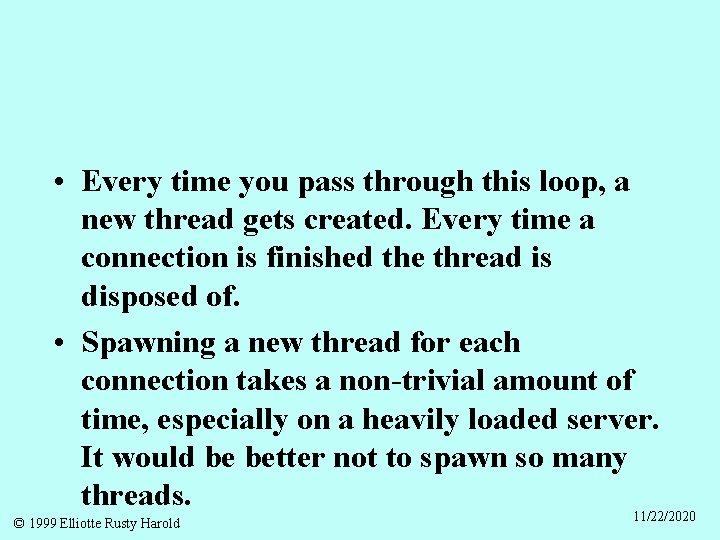
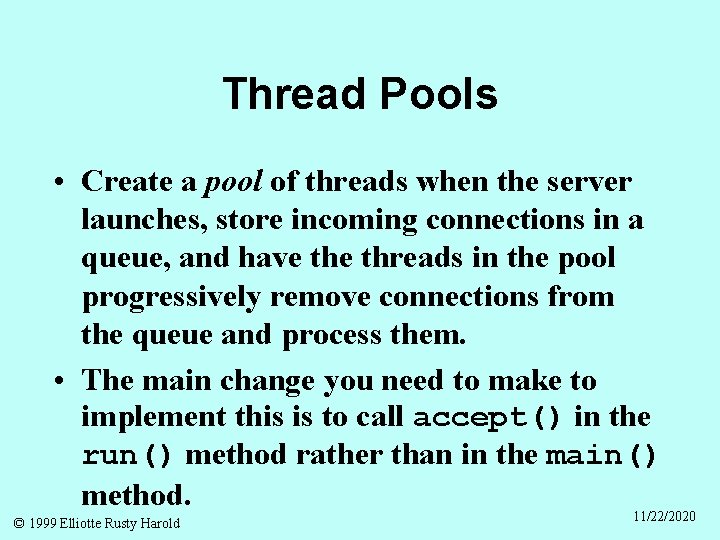
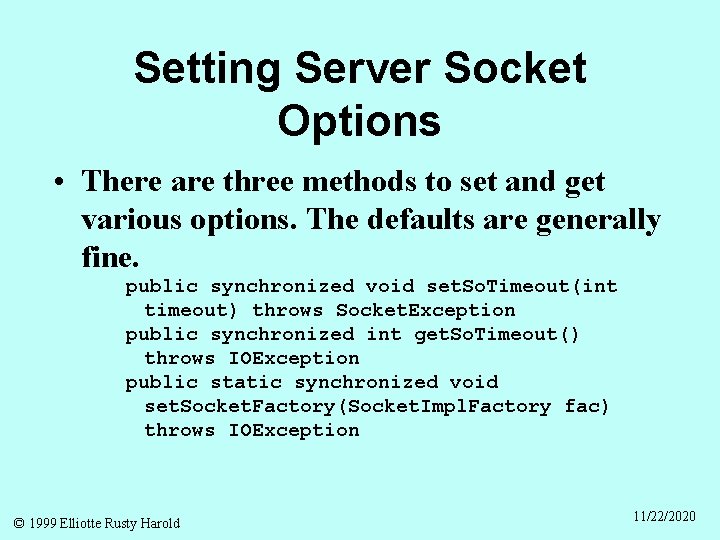
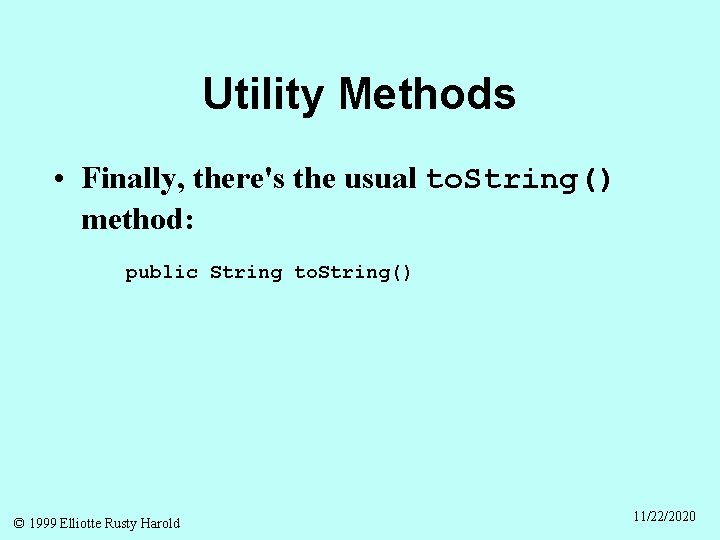
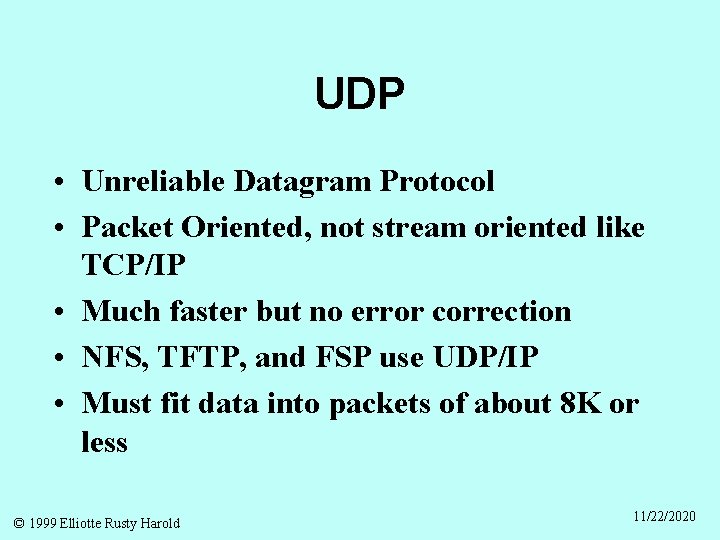
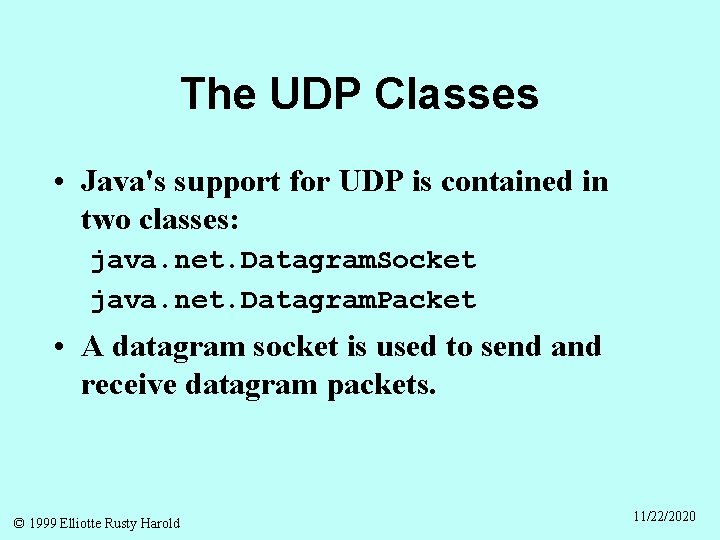
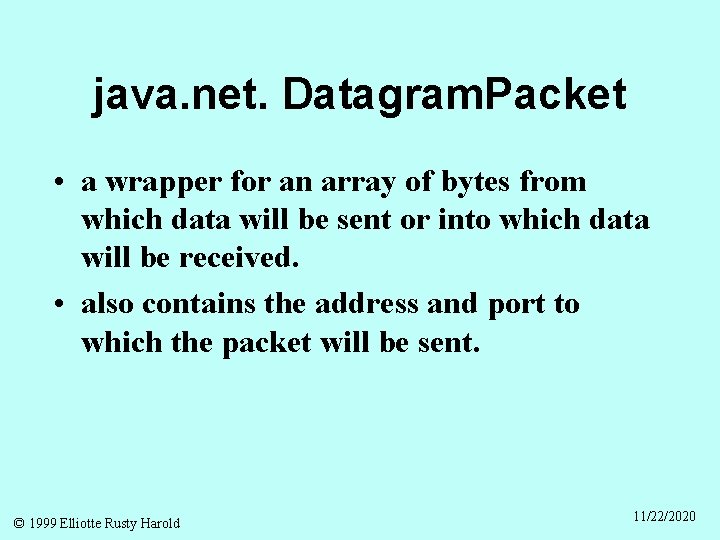
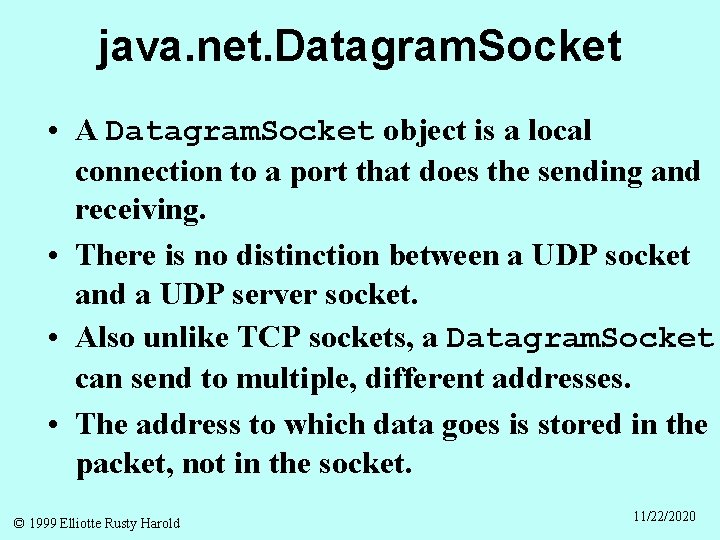
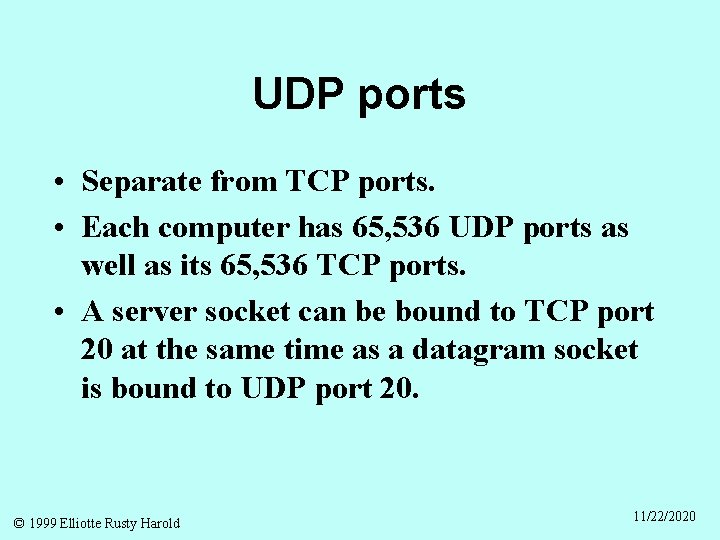
![Two Datagram. Packet Constructors • public Datagram. Packet(byte[] data, int length) • public Datagram. Two Datagram. Packet Constructors • public Datagram. Packet(byte[] data, int length) • public Datagram.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-61.jpg)
![For example, String s = "My first UDP Packet" byte[] b = s. get. For example, String s = "My first UDP Packet" byte[] b = s. get.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-62.jpg)
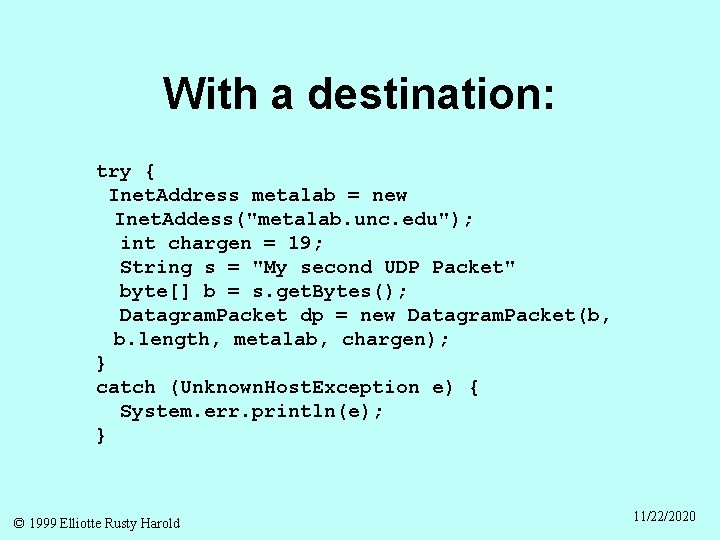
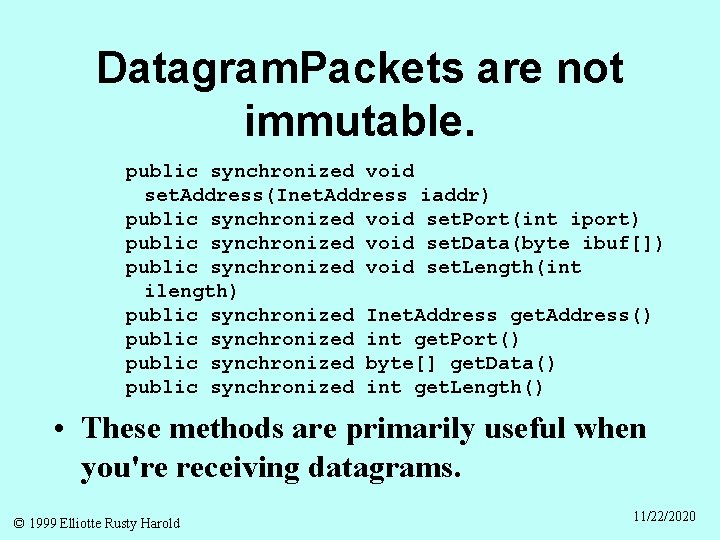
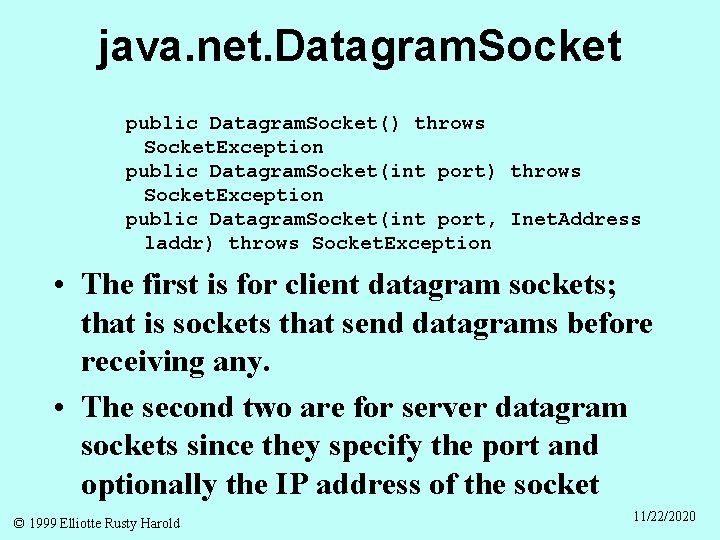
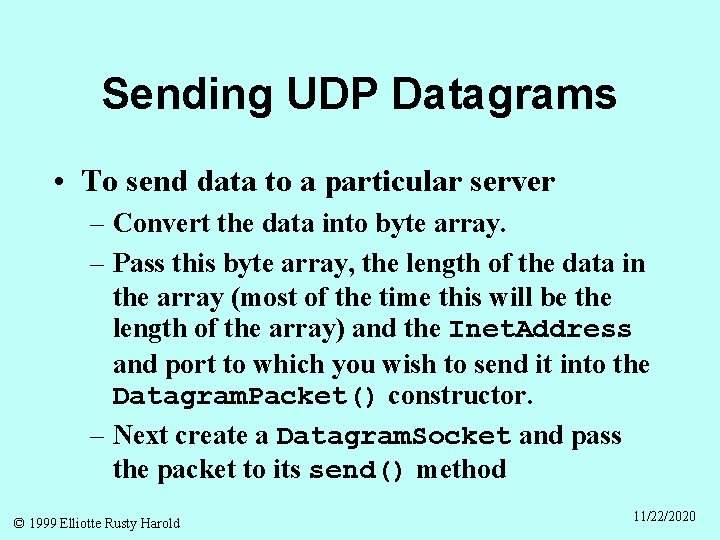
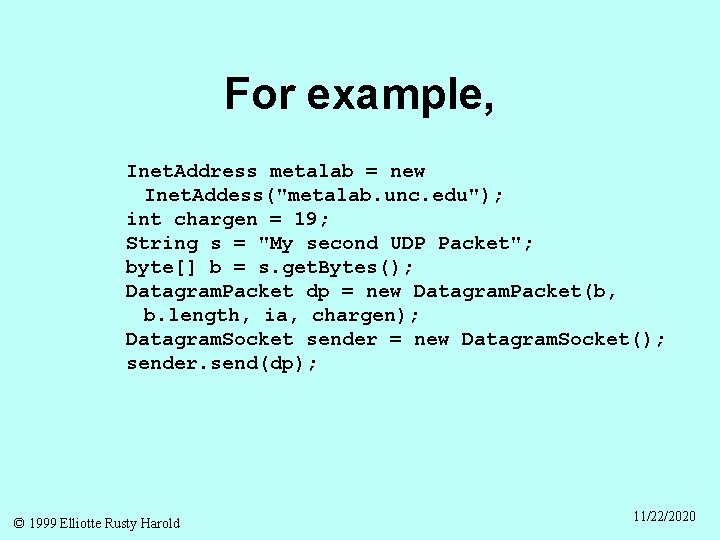
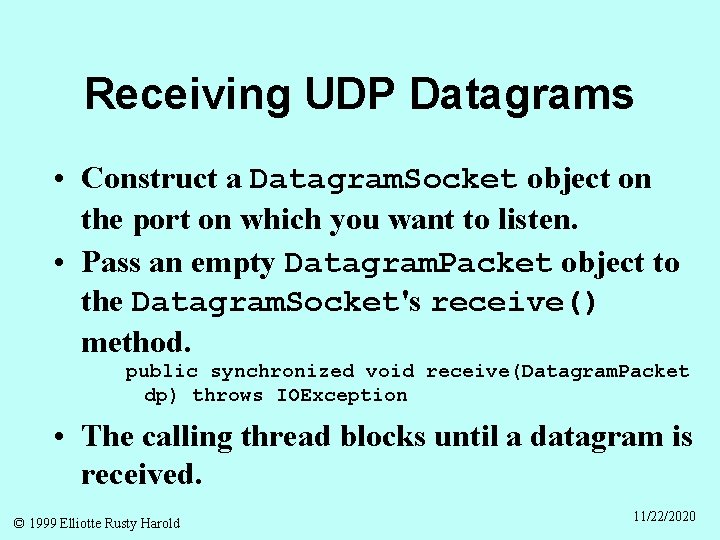
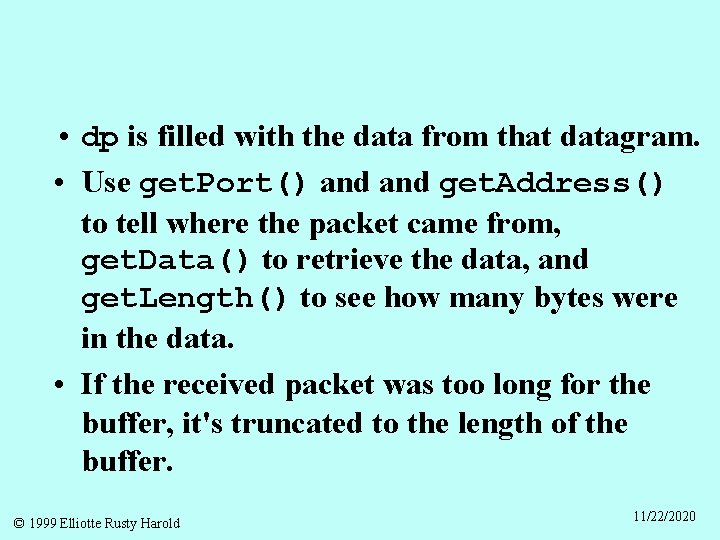
![For example, try { byte buffer = new byte[65536]; Datagram. Packet incoming = new For example, try { byte buffer = new byte[65536]; Datagram. Packet incoming = new](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-70.jpg)
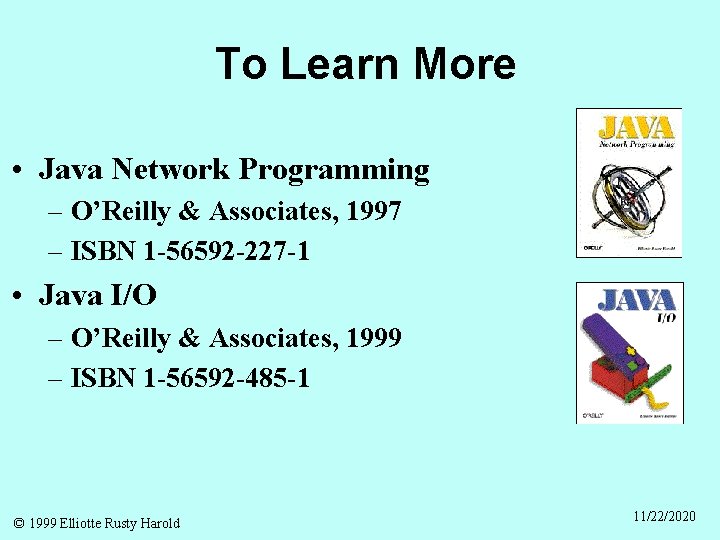
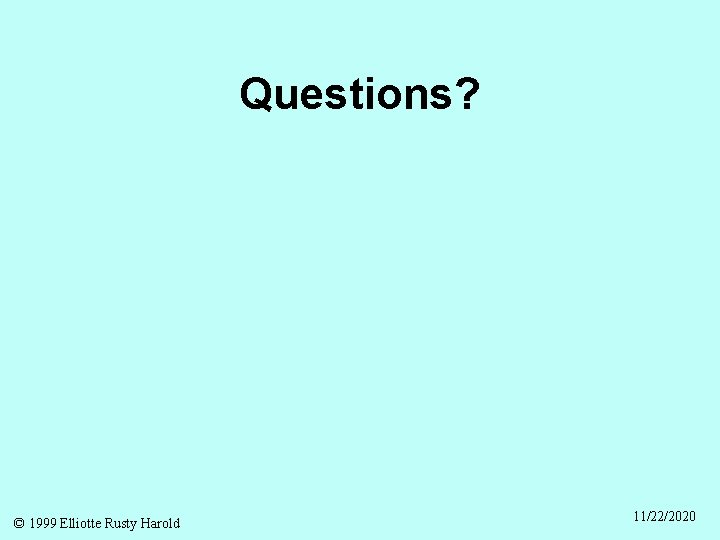
- Slides: 72
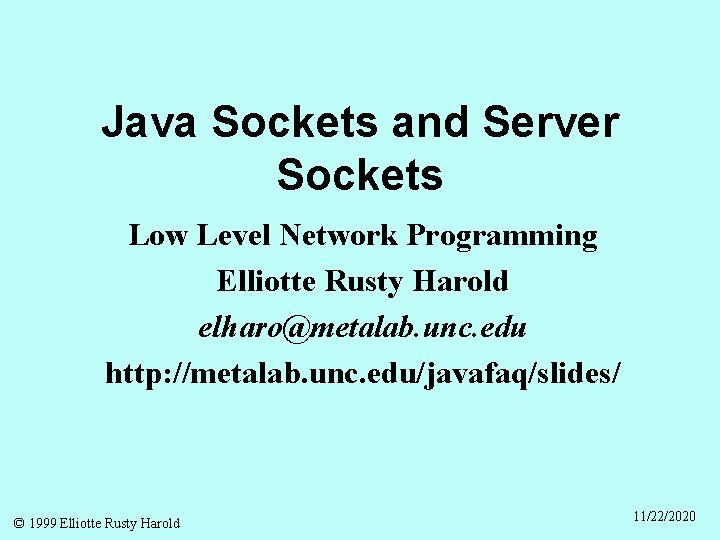
Java Sockets and Server Sockets Low Level Network Programming Elliotte Rusty Harold elharo@metalab. unc. edu http: //metalab. unc. edu/javafaq/slides/ © 1999 Elliotte Rusty Harold 11/22/2020
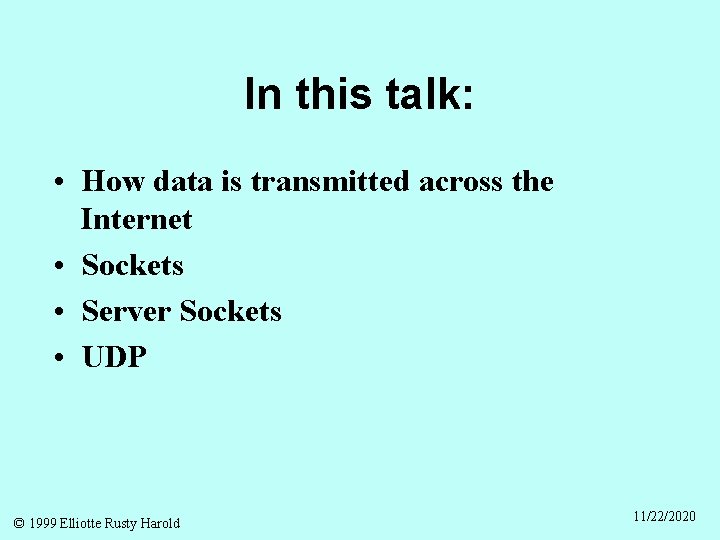
In this talk: • How data is transmitted across the Internet • Sockets • Server Sockets • UDP © 1999 Elliotte Rusty Harold 11/22/2020
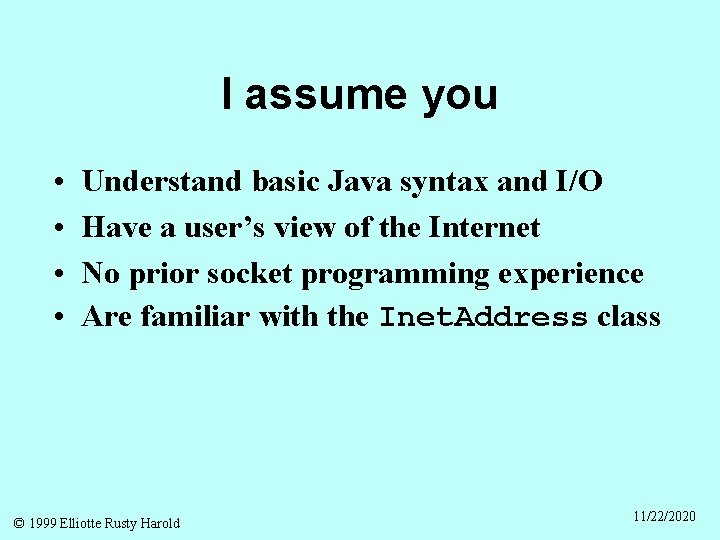
I assume you • • Understand basic Java syntax and I/O Have a user’s view of the Internet No prior socket programming experience Are familiar with the Inet. Address class © 1999 Elliotte Rusty Harold 11/22/2020
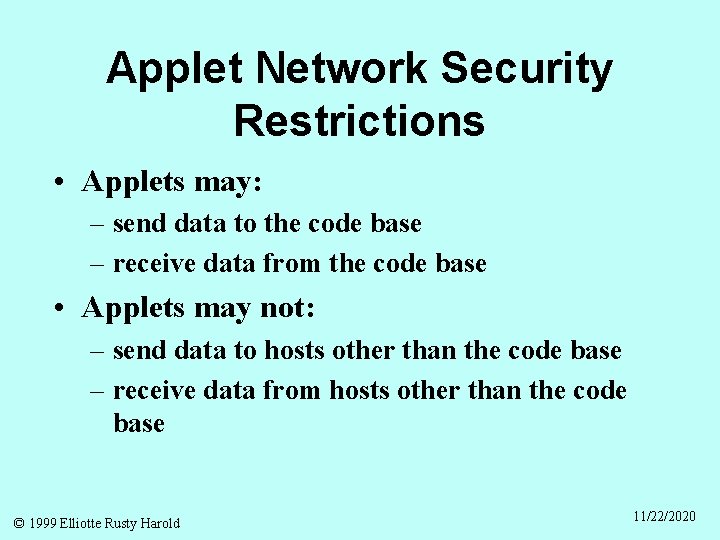
Applet Network Security Restrictions • Applets may: – send data to the code base – receive data from the code base • Applets may not: – send data to hosts other than the code base – receive data from hosts other than the code base © 1999 Elliotte Rusty Harold 11/22/2020
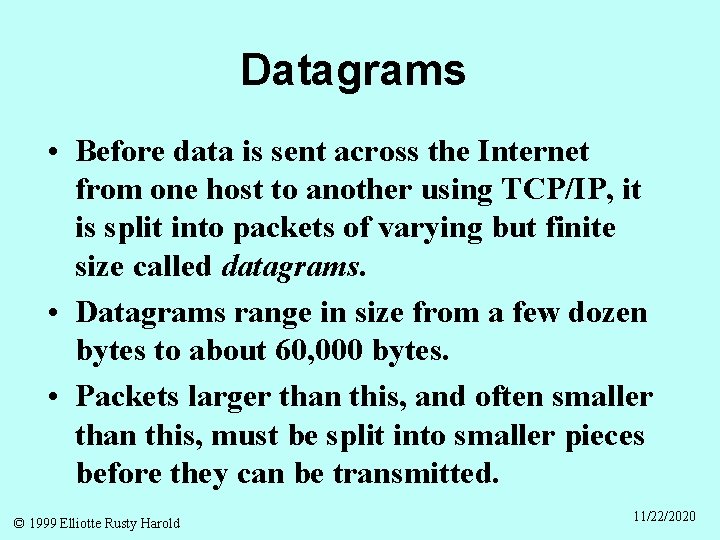
Datagrams • Before data is sent across the Internet from one host to another using TCP/IP, it is split into packets of varying but finite size called datagrams. • Datagrams range in size from a few dozen bytes to about 60, 000 bytes. • Packets larger than this, and often smaller than this, must be split into smaller pieces before they can be transmitted. © 1999 Elliotte Rusty Harold 11/22/2020
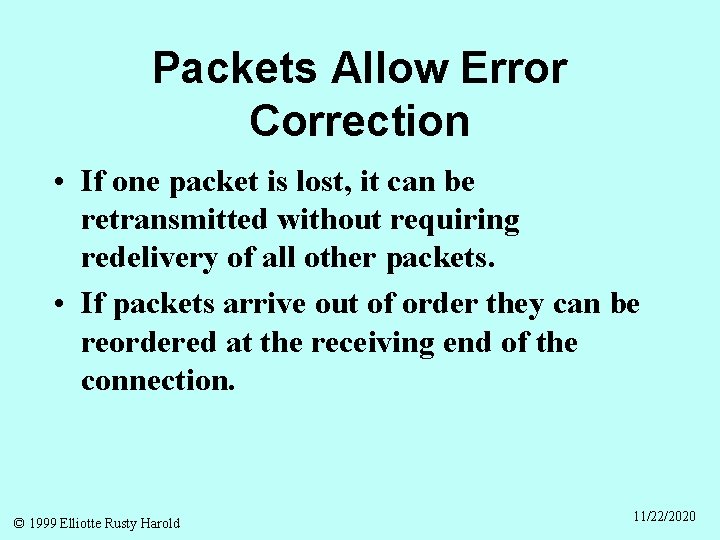
Packets Allow Error Correction • If one packet is lost, it can be retransmitted without requiring redelivery of all other packets. • If packets arrive out of order they can be reordered at the receiving end of the connection. © 1999 Elliotte Rusty Harold 11/22/2020
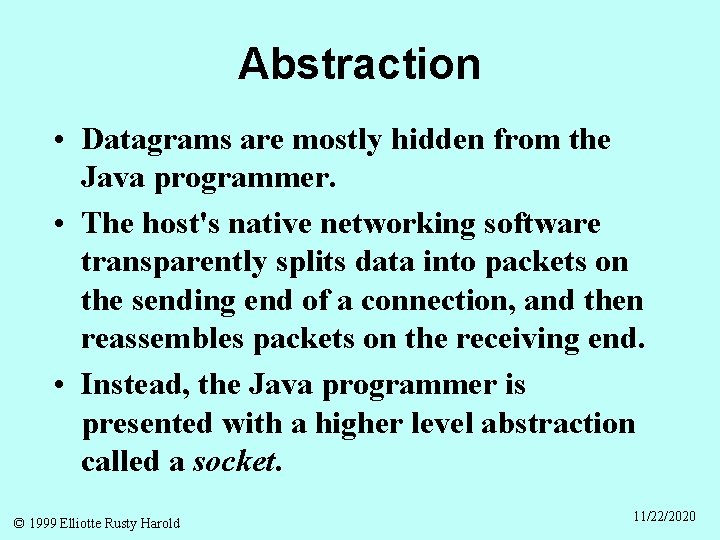
Abstraction • Datagrams are mostly hidden from the Java programmer. • The host's native networking software transparently splits data into packets on the sending end of a connection, and then reassembles packets on the receiving end. • Instead, the Java programmer is presented with a higher level abstraction called a socket. © 1999 Elliotte Rusty Harold 11/22/2020
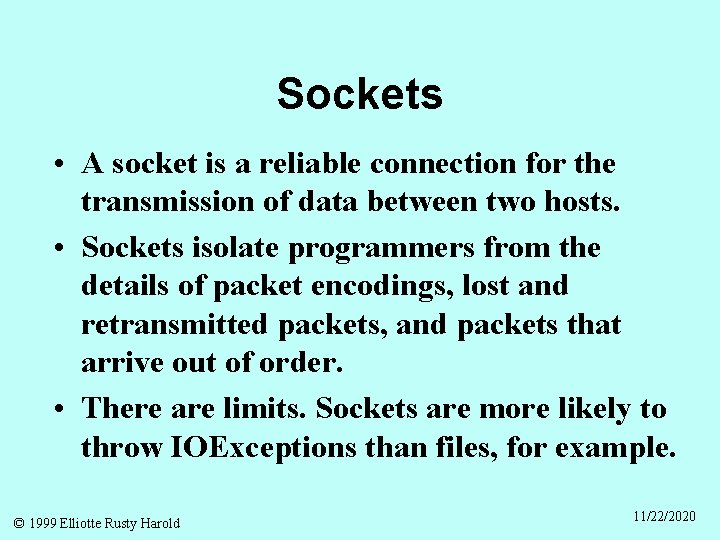
Sockets • A socket is a reliable connection for the transmission of data between two hosts. • Sockets isolate programmers from the details of packet encodings, lost and retransmitted packets, and packets that arrive out of order. • There are limits. Sockets are more likely to throw IOExceptions than files, for example. © 1999 Elliotte Rusty Harold 11/22/2020
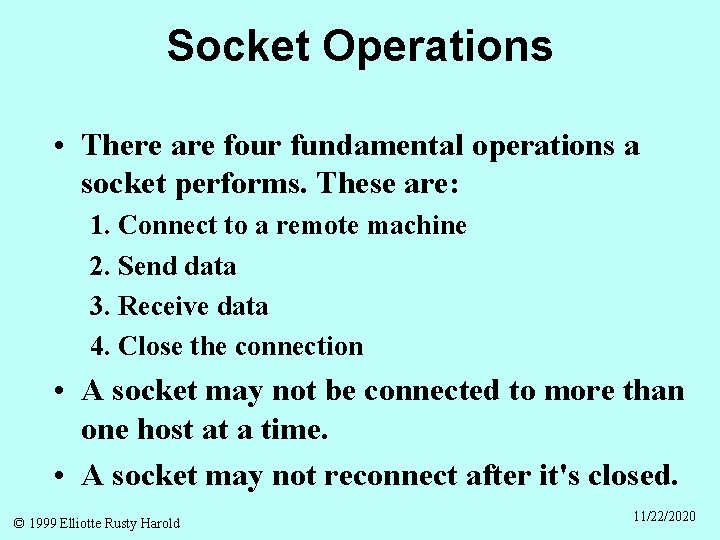
Socket Operations • There are four fundamental operations a socket performs. These are: 1. Connect to a remote machine 2. Send data 3. Receive data 4. Close the connection • A socket may not be connected to more than one host at a time. • A socket may not reconnect after it's closed. © 1999 Elliotte Rusty Harold 11/22/2020
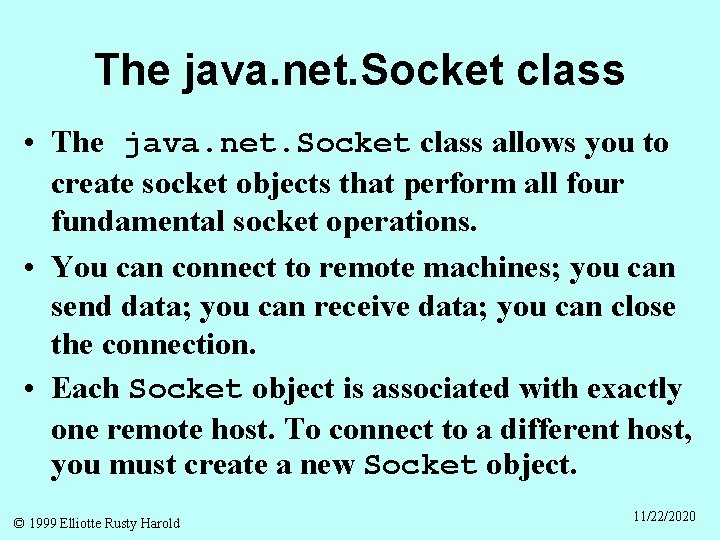
The java. net. Socket class • The java. net. Socket class allows you to create socket objects that perform all four fundamental socket operations. • You can connect to remote machines; you can send data; you can receive data; you can close the connection. • Each Socket object is associated with exactly one remote host. To connect to a different host, you must create a new Socket object. © 1999 Elliotte Rusty Harold 11/22/2020
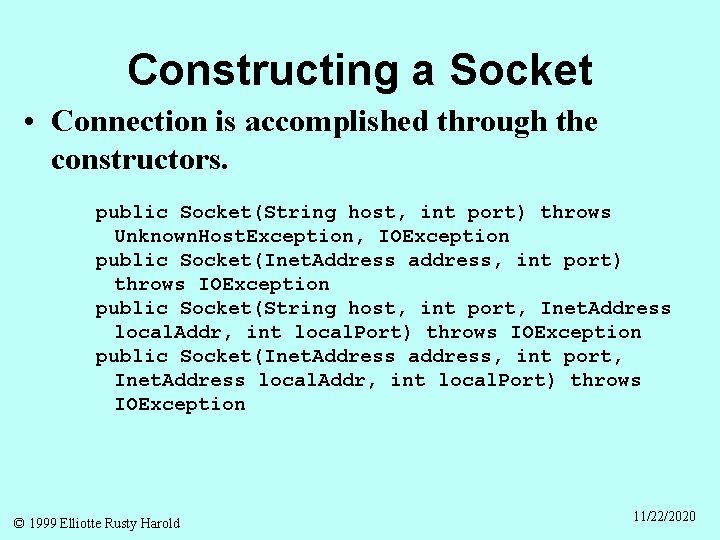
Constructing a Socket • Connection is accomplished through the constructors. public Socket(String host, int port) throws Unknown. Host. Exception, IOException public Socket(Inet. Address address, int port) throws IOException public Socket(String host, int port, Inet. Address local. Addr, int local. Port) throws IOException public Socket(Inet. Address address, int port, Inet. Address local. Addr, int local. Port) throws IOException © 1999 Elliotte Rusty Harold 11/22/2020
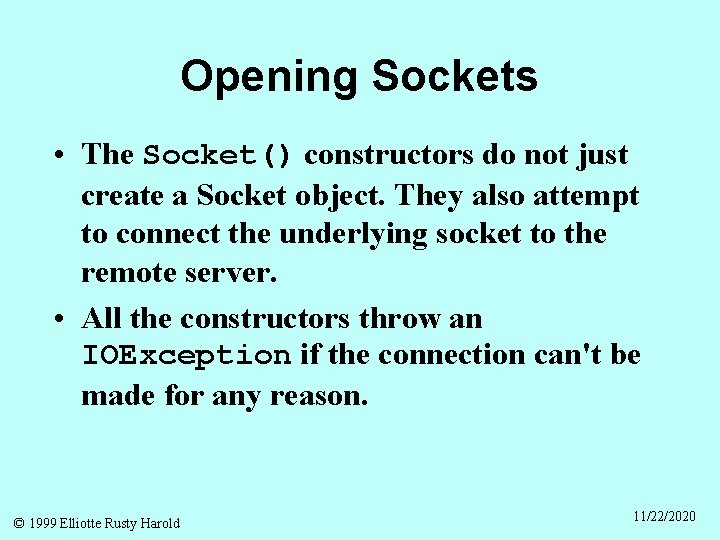
Opening Sockets • The Socket() constructors do not just create a Socket object. They also attempt to connect the underlying socket to the remote server. • All the constructors throw an IOException if the connection can't be made for any reason. © 1999 Elliotte Rusty Harold 11/22/2020
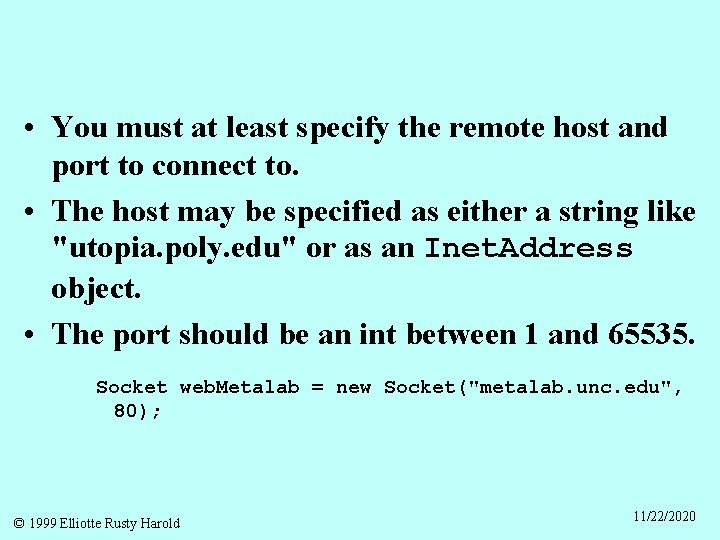
• You must at least specify the remote host and port to connect to. • The host may be specified as either a string like "utopia. poly. edu" or as an Inet. Address object. • The port should be an int between 1 and 65535. Socket web. Metalab = new Socket("metalab. unc. edu", 80); © 1999 Elliotte Rusty Harold 11/22/2020
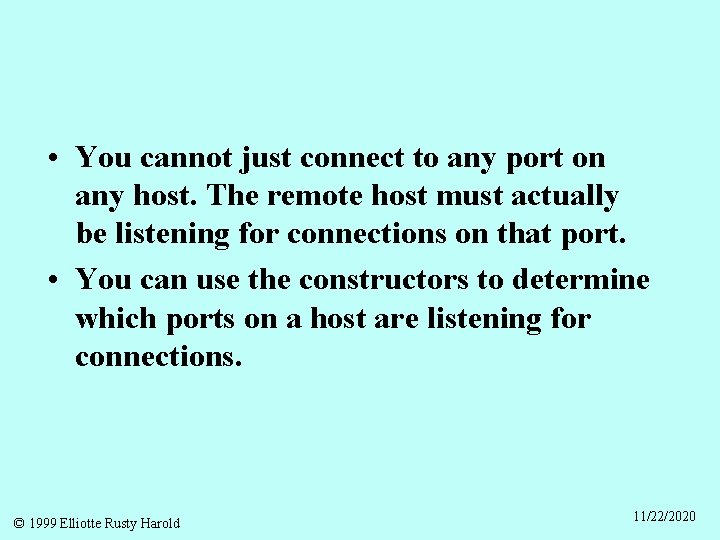
• You cannot just connect to any port on any host. The remote host must actually be listening for connections on that port. • You can use the constructors to determine which ports on a host are listening for connections. © 1999 Elliotte Rusty Harold 11/22/2020
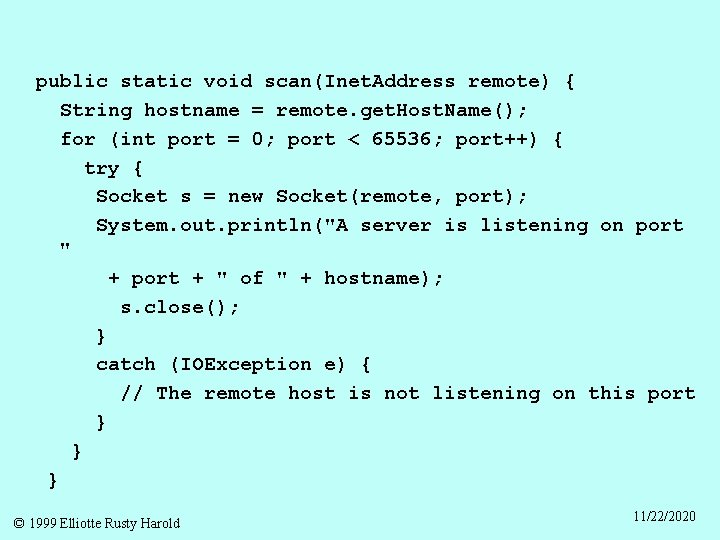
public static void scan(Inet. Address remote) { String hostname = remote. get. Host. Name(); for (int port = 0; port < 65536; port++) { try { Socket s = new Socket(remote, port); System. out. println("A server is listening on port " + port + " of " + hostname); s. close(); } catch (IOException e) { // The remote host is not listening on this port } } } © 1999 Elliotte Rusty Harold 11/22/2020
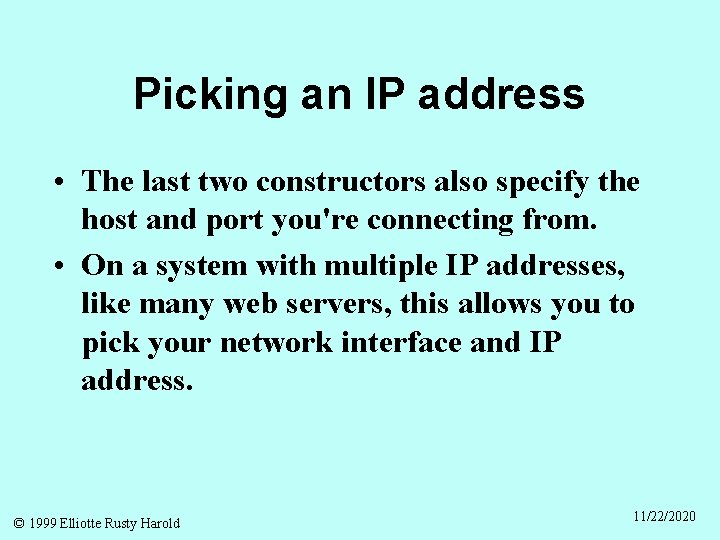
Picking an IP address • The last two constructors also specify the host and port you're connecting from. • On a system with multiple IP addresses, like many web servers, this allows you to pick your network interface and IP address. © 1999 Elliotte Rusty Harold 11/22/2020
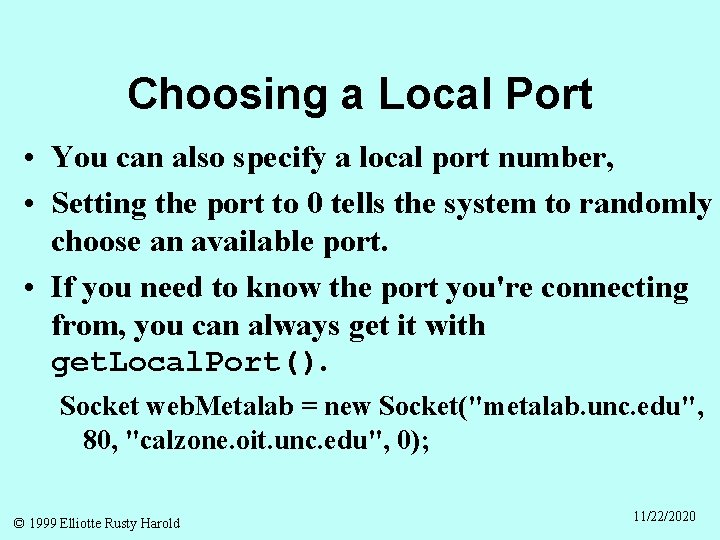
Choosing a Local Port • You can also specify a local port number, • Setting the port to 0 tells the system to randomly choose an available port. • If you need to know the port you're connecting from, you can always get it with get. Local. Port(). Socket web. Metalab = new Socket("metalab. unc. edu", 80, "calzone. oit. unc. edu", 0); © 1999 Elliotte Rusty Harold 11/22/2020
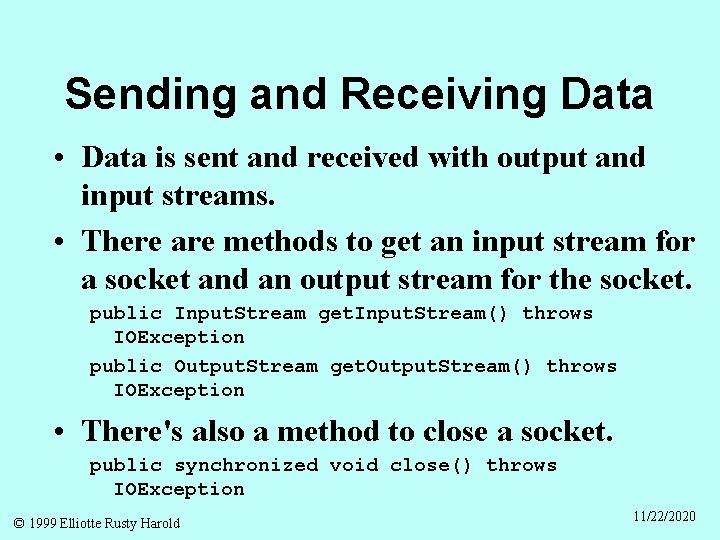
Sending and Receiving Data • Data is sent and received with output and input streams. • There are methods to get an input stream for a socket and an output stream for the socket. public Input. Stream get. Input. Stream() throws IOException public Output. Stream get. Output. Stream() throws IOException • There's also a method to close a socket. public synchronized void close() throws IOException © 1999 Elliotte Rusty Harold 11/22/2020
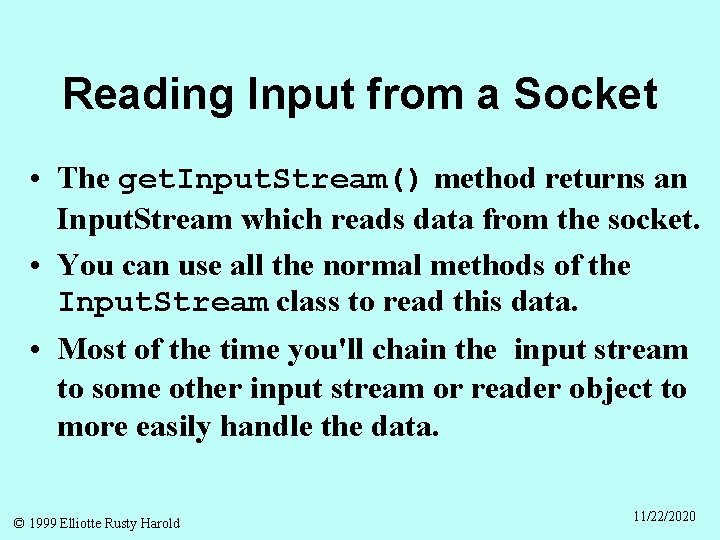
Reading Input from a Socket • The get. Input. Stream() method returns an Input. Stream which reads data from the socket. • You can use all the normal methods of the Input. Stream class to read this data. • Most of the time you'll chain the input stream to some other input stream or reader object to more easily handle the data. © 1999 Elliotte Rusty Harold 11/22/2020
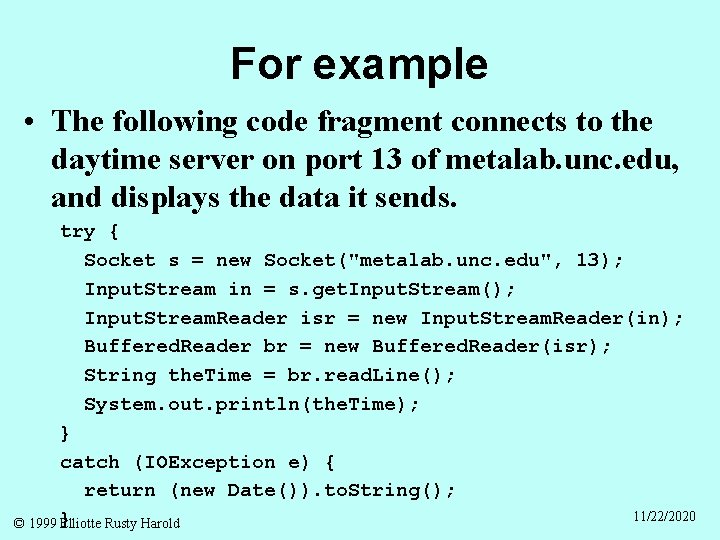
For example • The following code fragment connects to the daytime server on port 13 of metalab. unc. edu, and displays the data it sends. try { Socket s = new Socket("metalab. unc. edu", 13); Input. Stream in = s. get. Input. Stream(); Input. Stream. Reader isr = new Input. Stream. Reader(in); Buffered. Reader br = new Buffered. Reader(isr); String the. Time = br. read. Line(); System. out. println(the. Time); } catch (IOException e) { return (new Date()). to. String(); 11/22/2020 © 1999 } Elliotte Rusty Harold
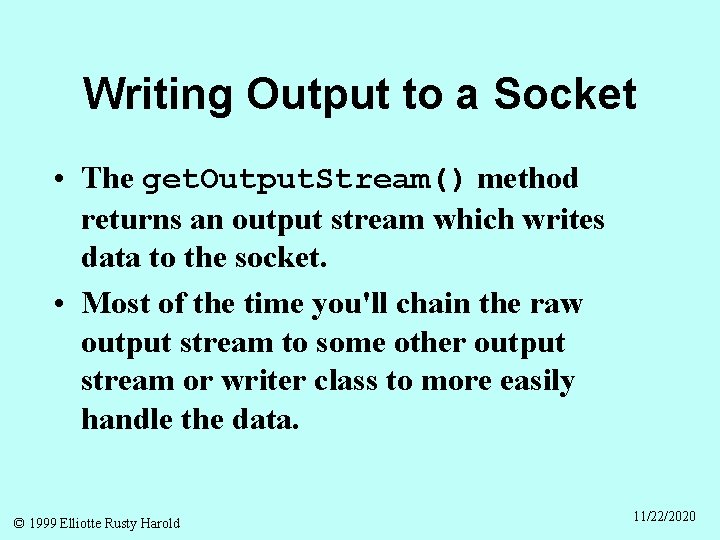
Writing Output to a Socket • The get. Output. Stream() method returns an output stream which writes data to the socket. • Most of the time you'll chain the raw output stream to some other output stream or writer class to more easily handle the data. © 1999 Elliotte Rusty Harold 11/22/2020
![Discard byte b new byte128 try Socket s new Socketmetalab unc Discard byte[] b = new byte[128]; try { Socket s = new Socket("metalab. unc.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-22.jpg)
Discard byte[] b = new byte[128]; try { Socket s = new Socket("metalab. unc. edu", 9); Output. Stream the. Output = s. get. Output. Stream(); while (true) { int n = the. Input. available(); if (n > b. length) n = b. length; int m = the. Input. read(b, 0, n); if (m == -1) break; the. Output. write(b, 0, n); } s. close(); } catch (IOException e) {} © 1999 Elliotte Rusty Harold 11/22/2020
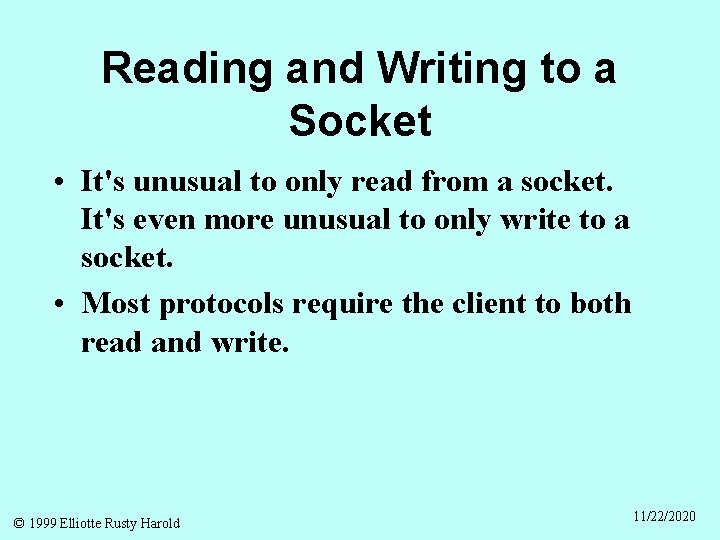
Reading and Writing to a Socket • It's unusual to only read from a socket. It's even more unusual to only write to a socket. • Most protocols require the client to both read and write. © 1999 Elliotte Rusty Harold 11/22/2020
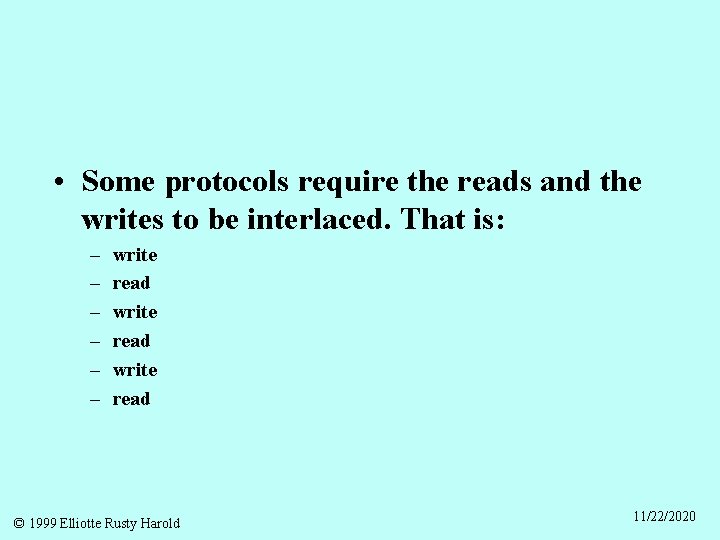
• Some protocols require the reads and the writes to be interlaced. That is: – – – write read © 1999 Elliotte Rusty Harold 11/22/2020
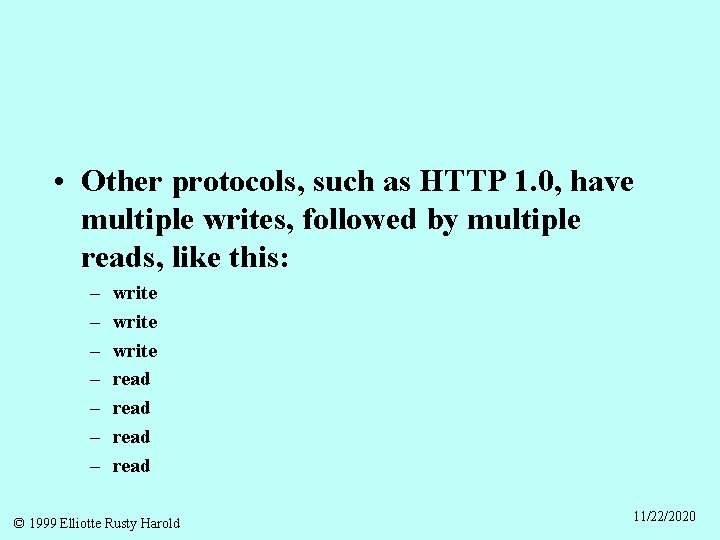
• Other protocols, such as HTTP 1. 0, have multiple writes, followed by multiple reads, like this: – – – – write read © 1999 Elliotte Rusty Harold 11/22/2020
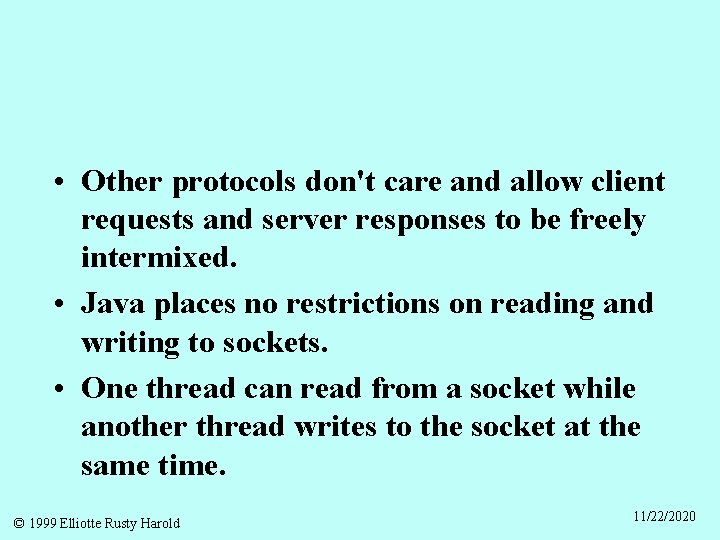
• Other protocols don't care and allow client requests and server responses to be freely intermixed. • Java places no restrictions on reading and writing to sockets. • One thread can read from a socket while another thread writes to the socket at the same time. © 1999 Elliotte Rusty Harold 11/22/2020
![try URL u new URLargsi if u get Port 1 port try { URL u = new URL(args[i]); if (u. get. Port() != -1) port](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-27.jpg)
try { URL u = new URL(args[i]); if (u. get. Port() != -1) port = u. get. Port(); if (!(u. get. Protocol(). equals. Ignore. Case("http"))) { System. err. println("I only understand http. "); } Socket s = new Socket(u. get. Host(), u. get. Port()); Output. Stream the. Output = s. get. Output. Stream(); Print. Writer pw = new Print. Writer(the. Output, false); pw. print("GET " + u. get. File() + " HTTP/1. 0rn"); pw. print("Accept: text/plain, text/html, text/*rn"); pw. print("rn"); pw. flush(); Input. Stream in = s. get. Input. Stream(); Input. Stream. Reader isr = new Input. Stream. Reader(in); Buffered. Reader br = new Buffered. Reader(isr); String the. Line; while ((the. Line = br. read. Line()) != null) { System. out. println(the. Line); } } 11/22/2020 © 1999 Elliotte Rusty Harold catch (Malformed. URLException e) {
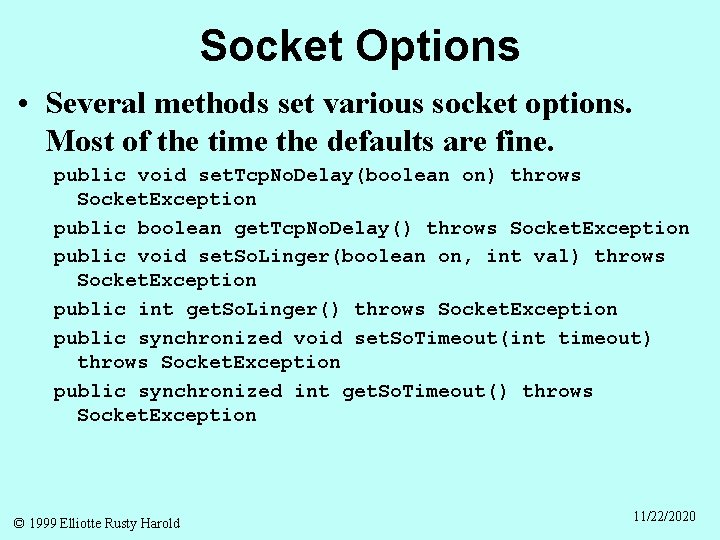
Socket Options • Several methods set various socket options. Most of the time the defaults are fine. public void set. Tcp. No. Delay(boolean on) throws Socket. Exception public boolean get. Tcp. No. Delay() throws Socket. Exception public void set. So. Linger(boolean on, int val) throws Socket. Exception public int get. So. Linger() throws Socket. Exception public synchronized void set. So. Timeout(int timeout) throws Socket. Exception public synchronized int get. So. Timeout() throws Socket. Exception © 1999 Elliotte Rusty Harold 11/22/2020
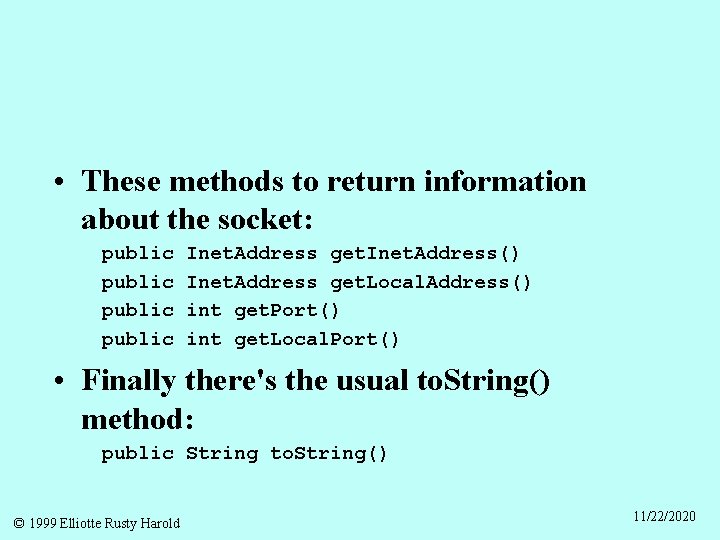
• These methods to return information about the socket: public Inet. Address get. Inet. Address() Inet. Address get. Local. Address() int get. Port() int get. Local. Port() • Finally there's the usual to. String() method: public String to. String() © 1999 Elliotte Rusty Harold 11/22/2020
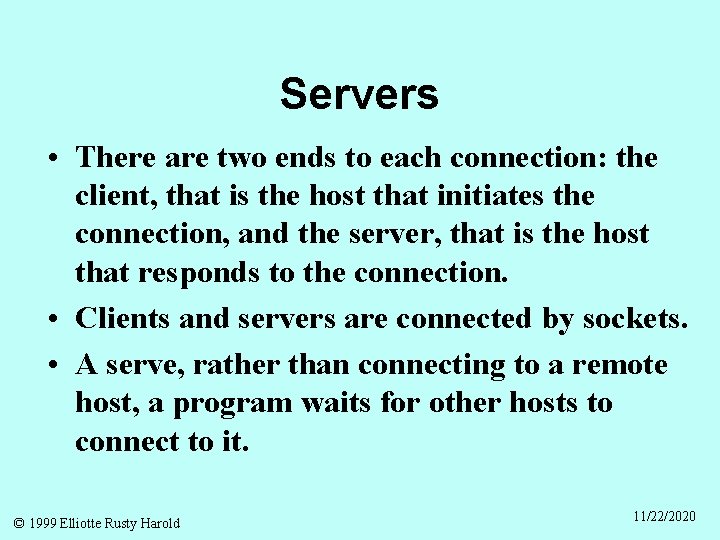
Servers • There are two ends to each connection: the client, that is the host that initiates the connection, and the server, that is the host that responds to the connection. • Clients and servers are connected by sockets. • A serve, rather than connecting to a remote host, a program waits for other hosts to connect to it. © 1999 Elliotte Rusty Harold 11/22/2020
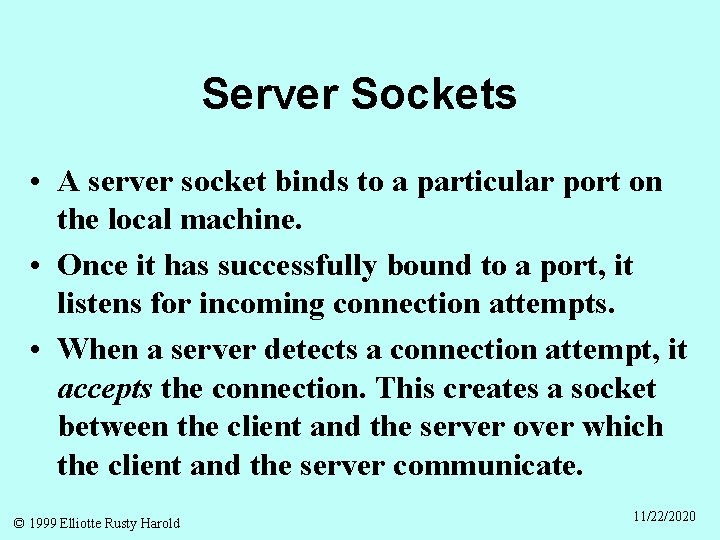
Server Sockets • A server socket binds to a particular port on the local machine. • Once it has successfully bound to a port, it listens for incoming connection attempts. • When a server detects a connection attempt, it accepts the connection. This creates a socket between the client and the server over which the client and the server communicate. © 1999 Elliotte Rusty Harold 11/22/2020
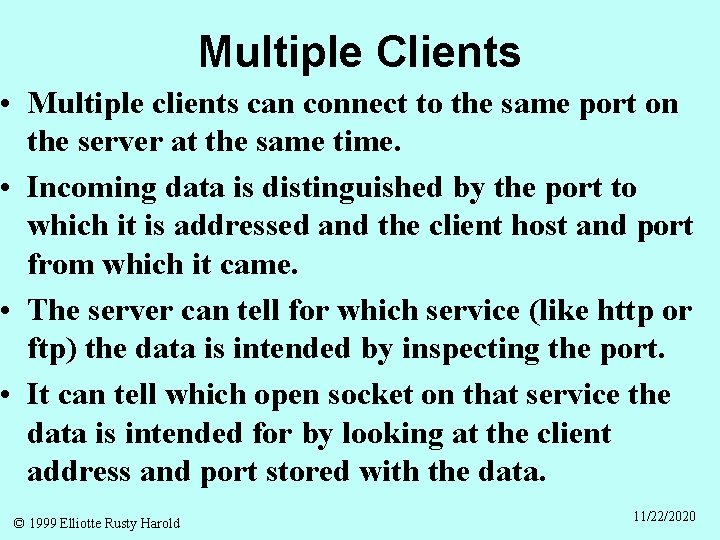
Multiple Clients • Multiple clients can connect to the same port on the server at the same time. • Incoming data is distinguished by the port to which it is addressed and the client host and port from which it came. • The server can tell for which service (like http or ftp) the data is intended by inspecting the port. • It can tell which open socket on that service the data is intended for by looking at the client address and port stored with the data. © 1999 Elliotte Rusty Harold 11/22/2020
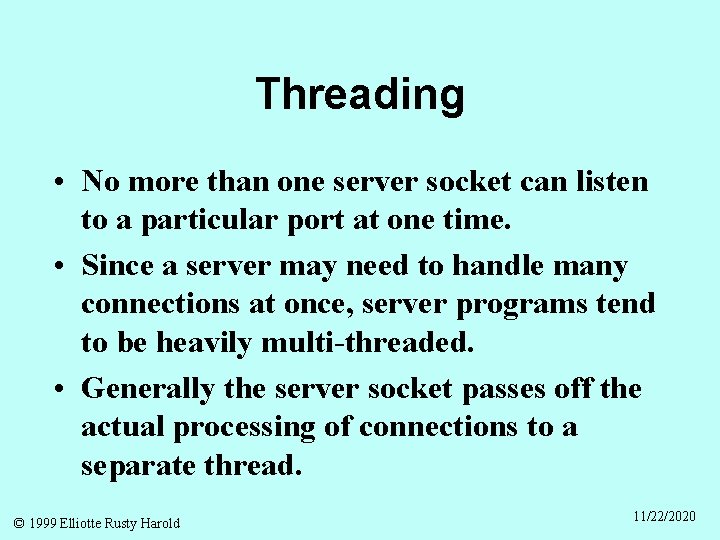
Threading • No more than one server socket can listen to a particular port at one time. • Since a server may need to handle many connections at once, server programs tend to be heavily multi-threaded. • Generally the server socket passes off the actual processing of connections to a separate thread. © 1999 Elliotte Rusty Harold 11/22/2020
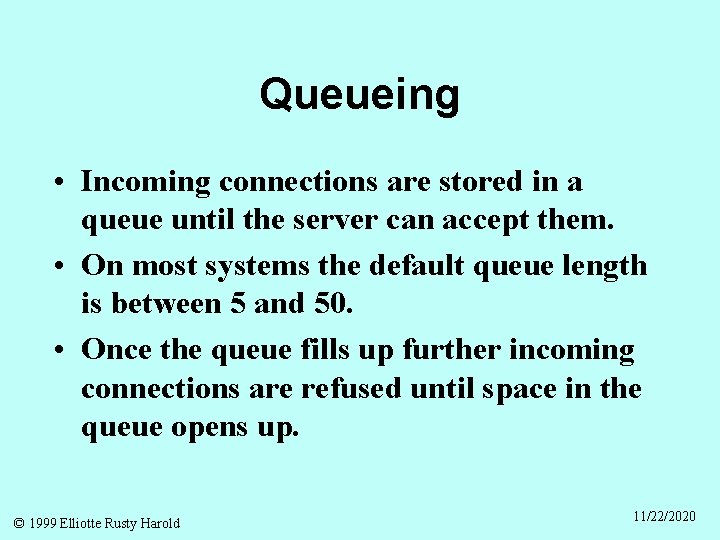
Queueing • Incoming connections are stored in a queue until the server can accept them. • On most systems the default queue length is between 5 and 50. • Once the queue fills up further incoming connections are refused until space in the queue opens up. © 1999 Elliotte Rusty Harold 11/22/2020
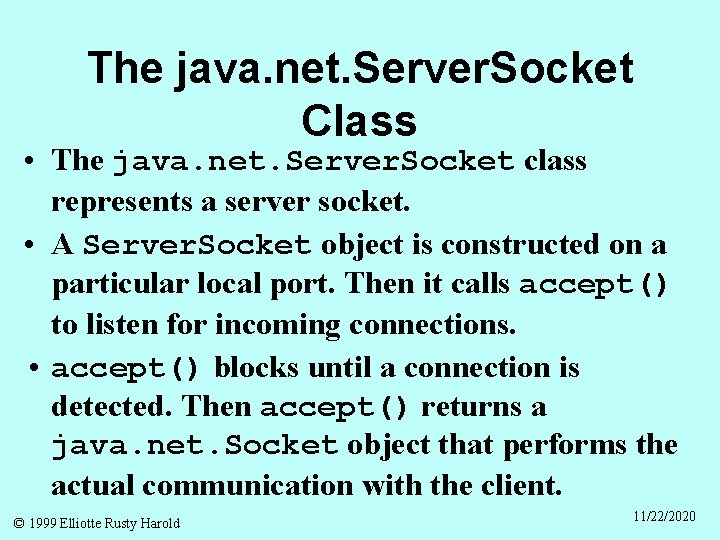
The java. net. Server. Socket Class • The java. net. Server. Socket class represents a server socket. • A Server. Socket object is constructed on a particular local port. Then it calls accept() to listen for incoming connections. • accept() blocks until a connection is detected. Then accept() returns a java. net. Socket object that performs the actual communication with the client. © 1999 Elliotte Rusty Harold 11/22/2020
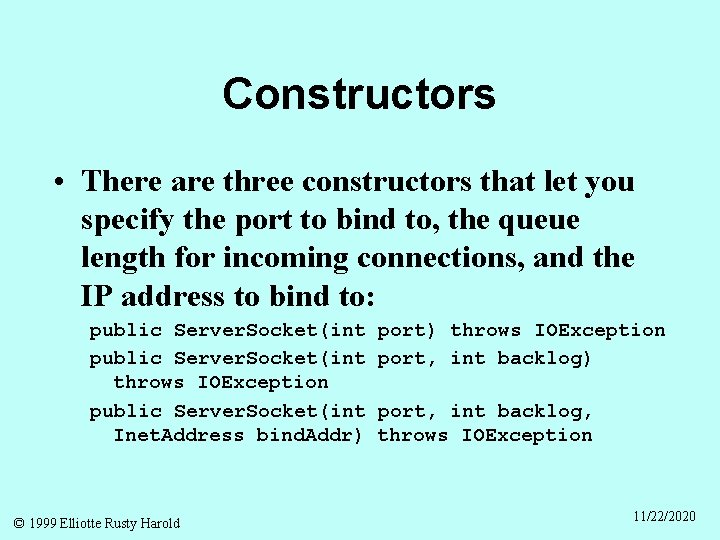
Constructors • There are three constructors that let you specify the port to bind to, the queue length for incoming connections, and the IP address to bind to: public Server. Socket(int throws IOException public Server. Socket(int Inet. Address bind. Addr) © 1999 Elliotte Rusty Harold port) throws IOException port, int backlog) port, int backlog, throws IOException 11/22/2020
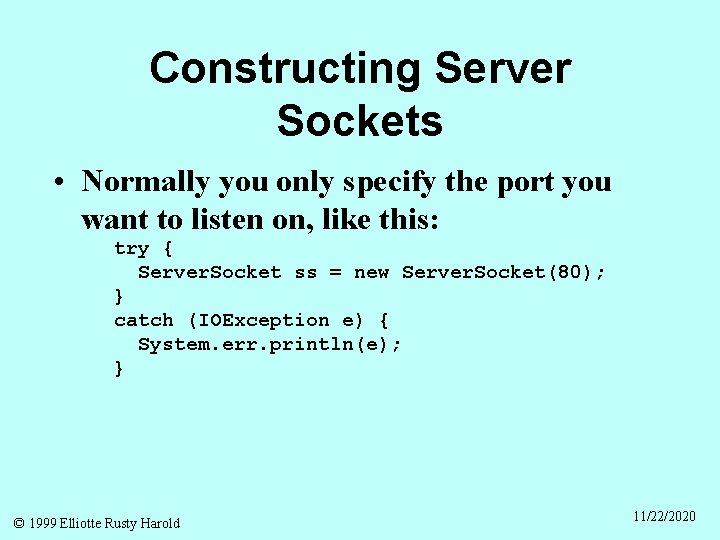
Constructing Server Sockets • Normally you only specify the port you want to listen on, like this: try { Server. Socket ss = new Server. Socket(80); } catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
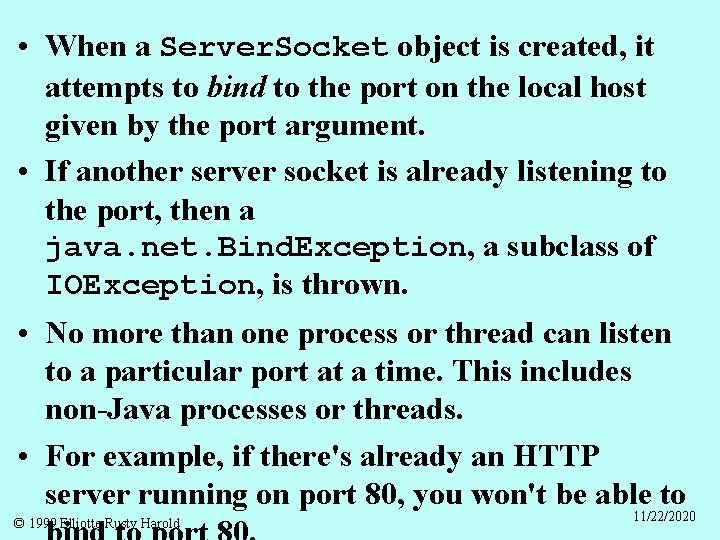
• When a Server. Socket object is created, it attempts to bind to the port on the local host given by the port argument. • If another server socket is already listening to the port, then a java. net. Bind. Exception, a subclass of IOException, is thrown. • No more than one process or thread can listen to a particular port at a time. This includes non-Java processes or threads. • For example, if there's already an HTTP server running on port 80, you won't be able to © 1999 Elliotte Rusty Harold 11/22/2020
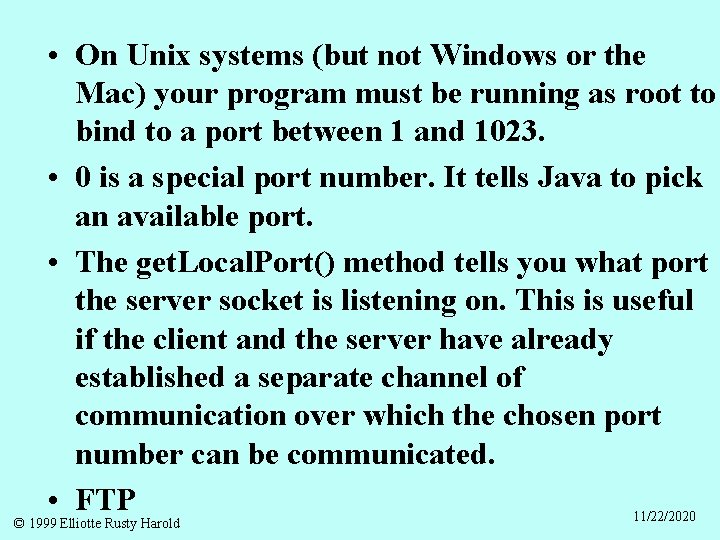
• On Unix systems (but not Windows or the Mac) your program must be running as root to bind to a port between 1 and 1023. • 0 is a special port number. It tells Java to pick an available port. • The get. Local. Port() method tells you what port the server socket is listening on. This is useful if the client and the server have already established a separate channel of communication over which the chosen port number can be communicated. • FTP 11/22/2020 © 1999 Elliotte Rusty Harold
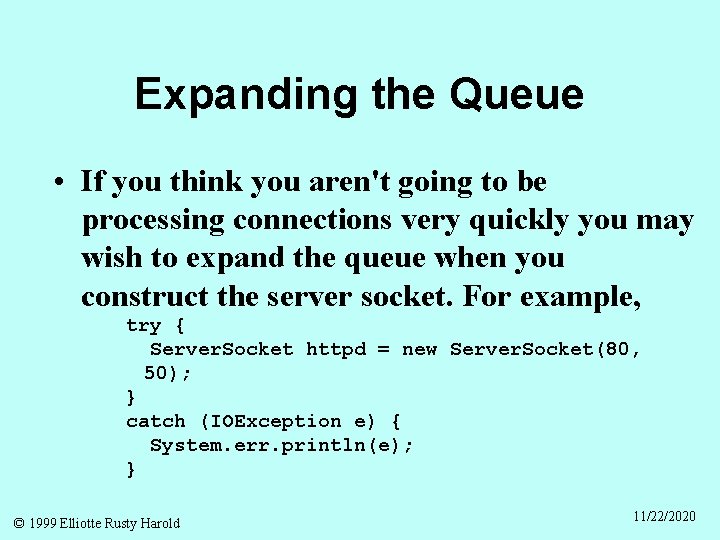
Expanding the Queue • If you think you aren't going to be processing connections very quickly you may wish to expand the queue when you construct the server socket. For example, try { Server. Socket httpd = new Server. Socket(80, 50); } catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
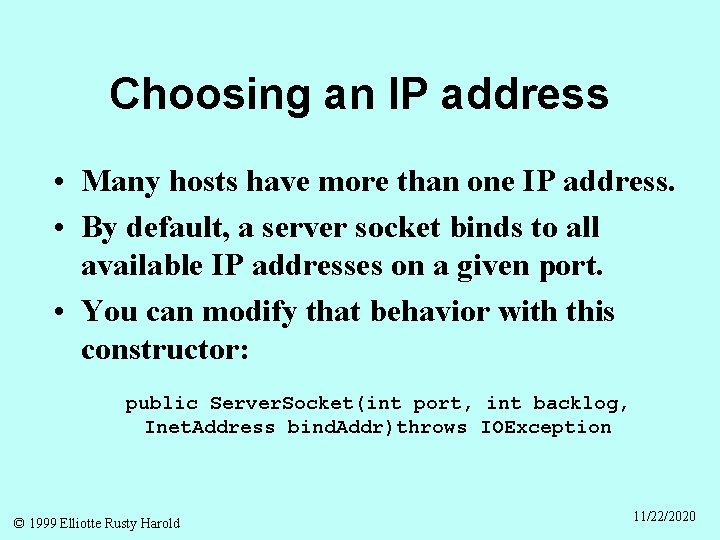
Choosing an IP address • Many hosts have more than one IP address. • By default, a server socket binds to all available IP addresses on a given port. • You can modify that behavior with this constructor: public Server. Socket(int port, int backlog, Inet. Address bind. Addr)throws IOException © 1999 Elliotte Rusty Harold 11/22/2020
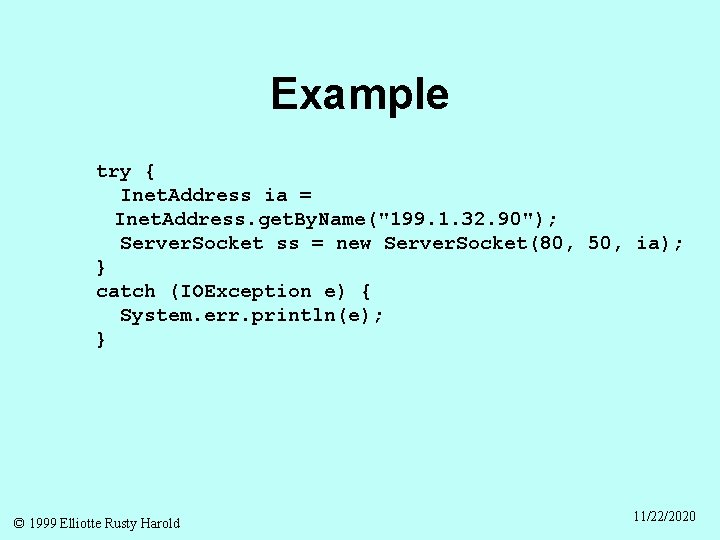
Example try { Inet. Address ia = Inet. Address. get. By. Name("199. 1. 32. 90"); Server. Socket ss = new Server. Socket(80, 50, ia); } catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
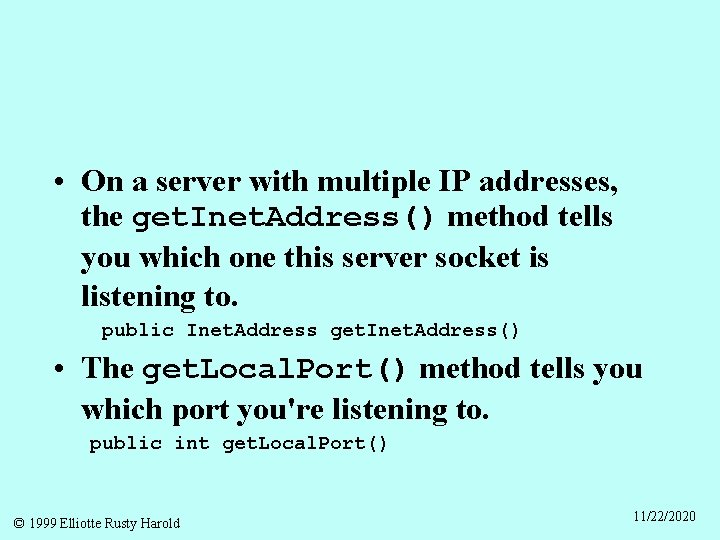
• On a server with multiple IP addresses, the get. Inet. Address() method tells you which one this server socket is listening to. public Inet. Address get. Inet. Address() • The get. Local. Port() method tells you which port you're listening to. public int get. Local. Port() © 1999 Elliotte Rusty Harold 11/22/2020
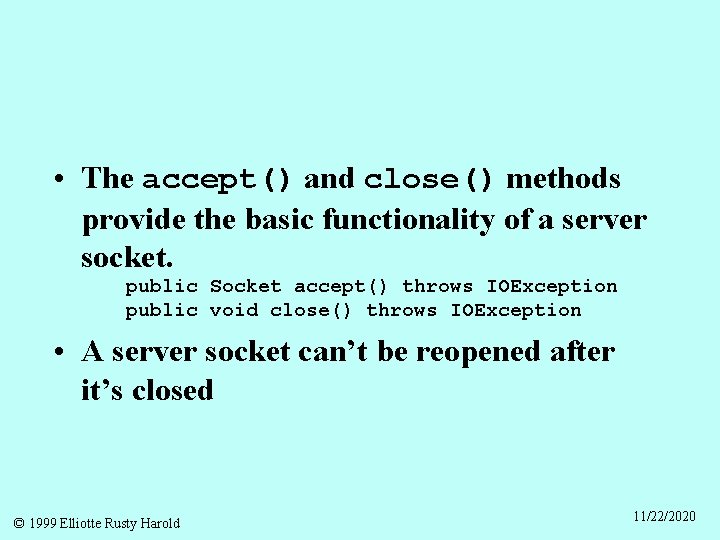
• The accept() and close() methods provide the basic functionality of a server socket. public Socket accept() throws IOException public void close() throws IOException • A server socket can’t be reopened after it’s closed © 1999 Elliotte Rusty Harold 11/22/2020
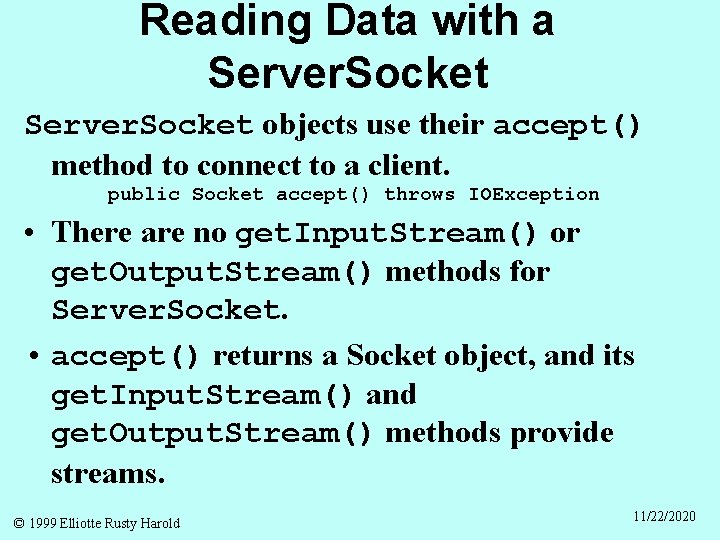
Reading Data with a Server. Socket objects use their accept() method to connect to a client. public Socket accept() throws IOException • There are no get. Input. Stream() or get. Output. Stream() methods for Server. Socket. • accept() returns a Socket object, and its get. Input. Stream() and get. Output. Stream() methods provide streams. © 1999 Elliotte Rusty Harold 11/22/2020
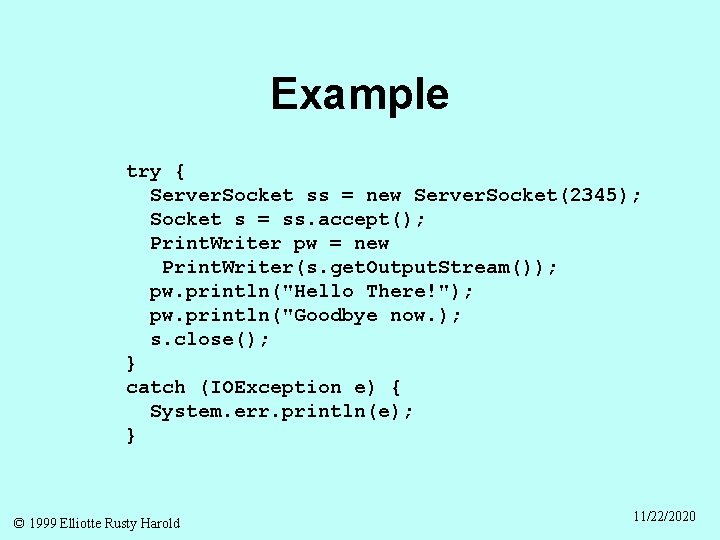
Example try { Server. Socket ss = new Server. Socket(2345); Socket s = ss. accept(); Print. Writer pw = new Print. Writer(s. get. Output. Stream()); pw. println("Hello There!"); pw. println("Goodbye now. ); s. close(); } catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
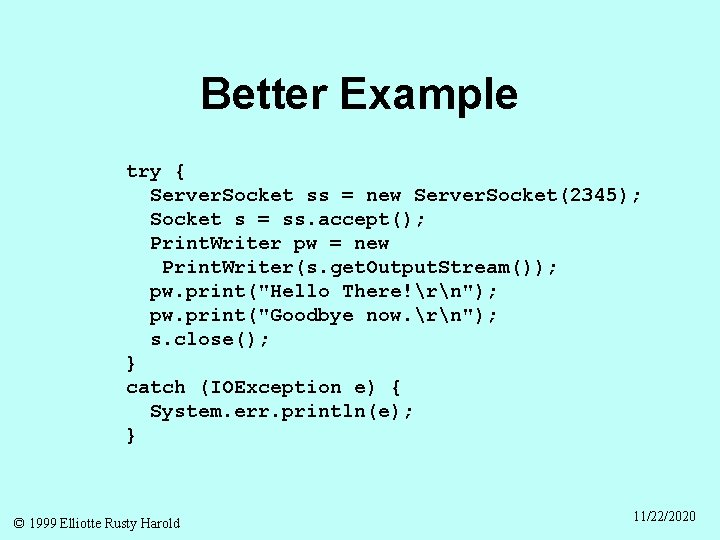
Better Example try { Server. Socket ss = new Server. Socket(2345); Socket s = ss. accept(); Print. Writer pw = new Print. Writer(s. get. Output. Stream()); pw. print("Hello There!rn"); pw. print("Goodbye now. rn"); s. close(); } catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
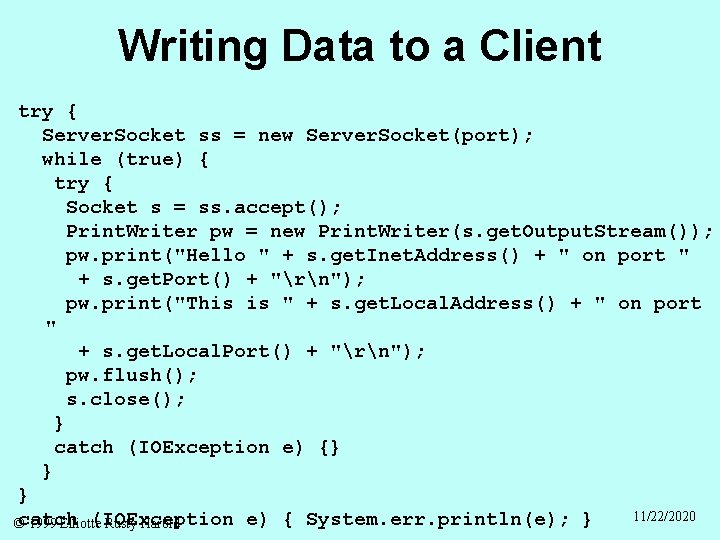
Writing Data to a Client try { Server. Socket ss = new Server. Socket(port); while (true) { try { Socket s = ss. accept(); Print. Writer pw = new Print. Writer(s. get. Output. Stream()); pw. print("Hello " + s. get. Inet. Address() + " on port " + s. get. Port() + "rn"); pw. print("This is " + s. get. Local. Address() + " on port " + s. get. Local. Port() + "rn"); pw. flush(); s. close(); } catch (IOException e) {} } } 11/22/2020 catch (IOException e) { System. err. println(e); } © 1999 Elliotte Rusty Harold
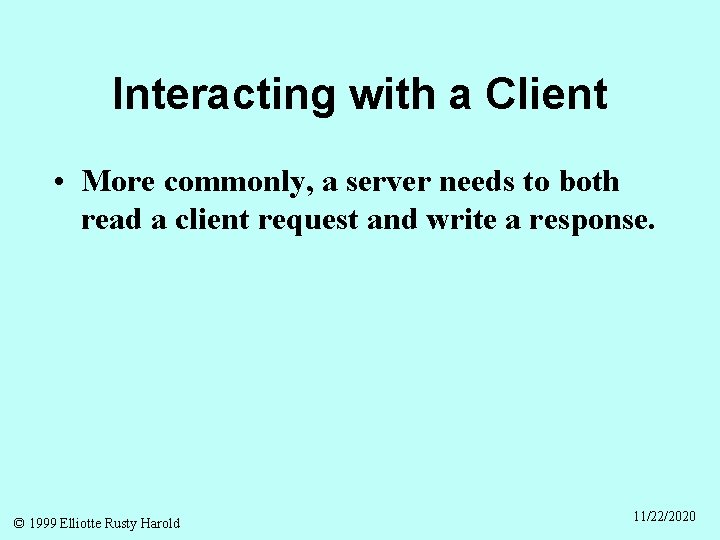
Interacting with a Client • More commonly, a server needs to both read a client request and write a response. © 1999 Elliotte Rusty Harold 11/22/2020
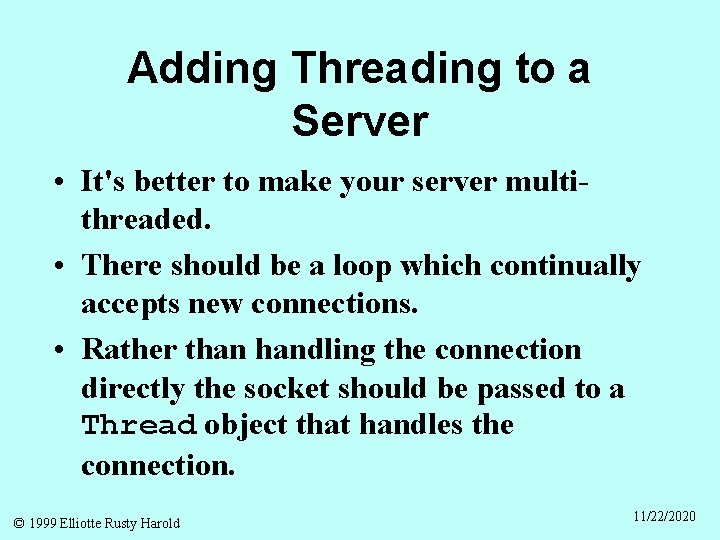
Adding Threading to a Server • It's better to make your server multithreaded. • There should be a loop which continually accepts new connections. • Rather than handling the connection directly the socket should be passed to a Thread object that handles the connection. © 1999 Elliotte Rusty Harold 11/22/2020
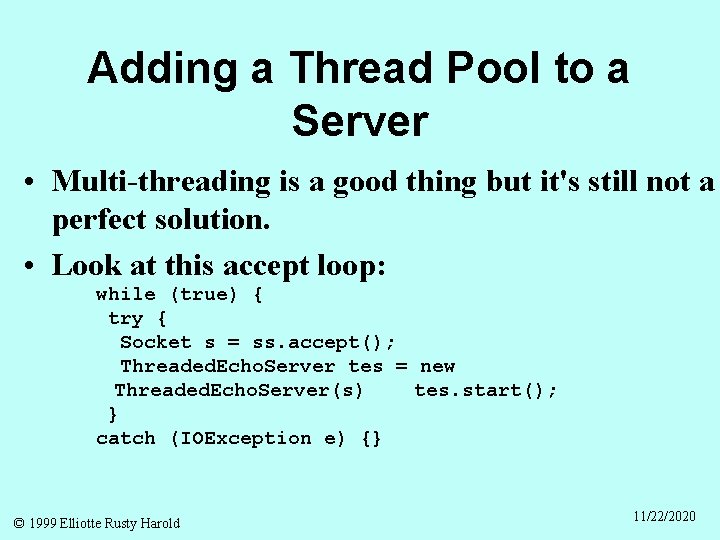
Adding a Thread Pool to a Server • Multi-threading is a good thing but it's still not a perfect solution. • Look at this accept loop: while (true) { try { Socket s = ss. accept(); Threaded. Echo. Server tes = new Threaded. Echo. Server(s) tes. start(); } catch (IOException e) {} © 1999 Elliotte Rusty Harold 11/22/2020
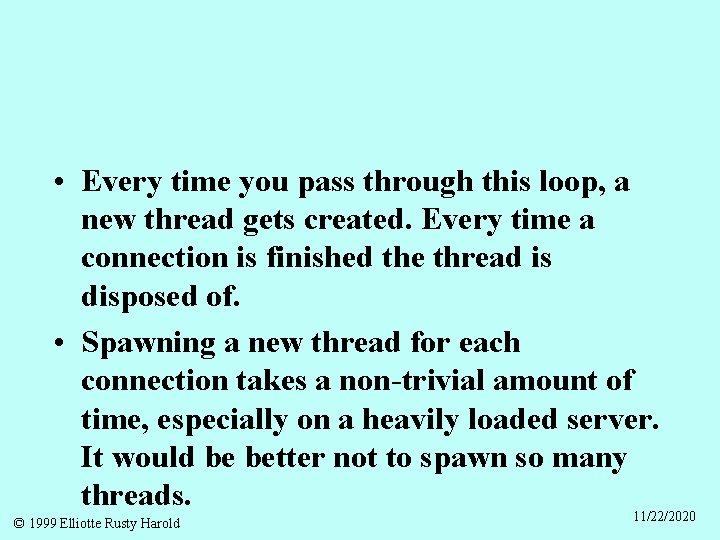
• Every time you pass through this loop, a new thread gets created. Every time a connection is finished the thread is disposed of. • Spawning a new thread for each connection takes a non-trivial amount of time, especially on a heavily loaded server. It would be better not to spawn so many threads. 11/22/2020 © 1999 Elliotte Rusty Harold
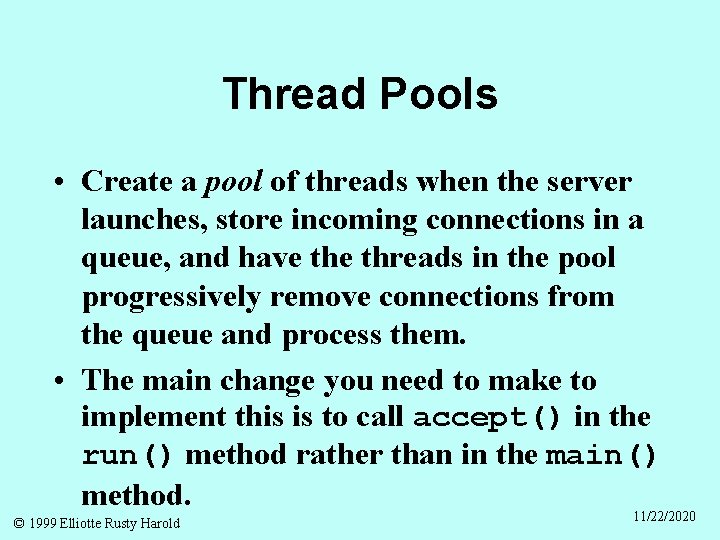
Thread Pools • Create a pool of threads when the server launches, store incoming connections in a queue, and have threads in the pool progressively remove connections from the queue and process them. • The main change you need to make to implement this is to call accept() in the run() method rather than in the main() method. 11/22/2020 © 1999 Elliotte Rusty Harold
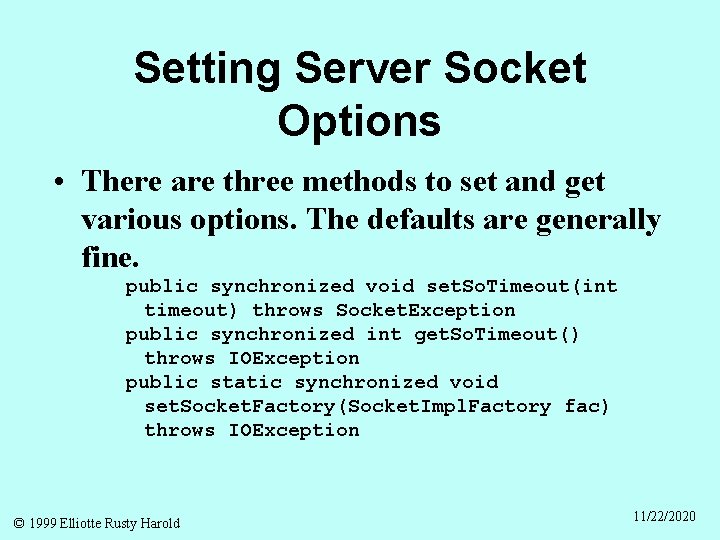
Setting Server Socket Options • There are three methods to set and get various options. The defaults are generally fine. public synchronized void set. So. Timeout(int timeout) throws Socket. Exception public synchronized int get. So. Timeout() throws IOException public static synchronized void set. Socket. Factory(Socket. Impl. Factory fac) throws IOException © 1999 Elliotte Rusty Harold 11/22/2020
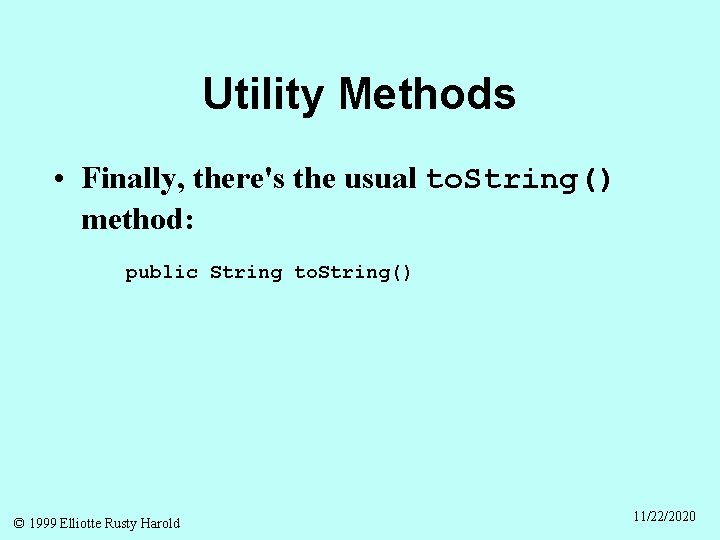
Utility Methods • Finally, there's the usual to. String() method: public String to. String() © 1999 Elliotte Rusty Harold 11/22/2020
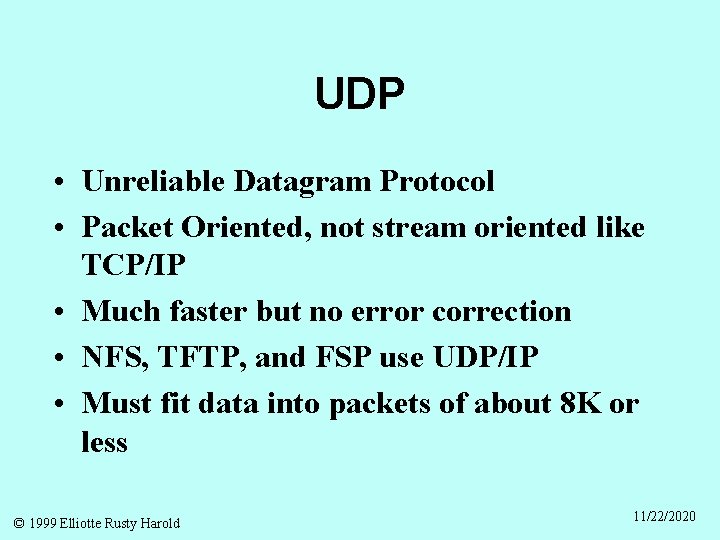
UDP • Unreliable Datagram Protocol • Packet Oriented, not stream oriented like TCP/IP • Much faster but no error correction • NFS, TFTP, and FSP use UDP/IP • Must fit data into packets of about 8 K or less © 1999 Elliotte Rusty Harold 11/22/2020
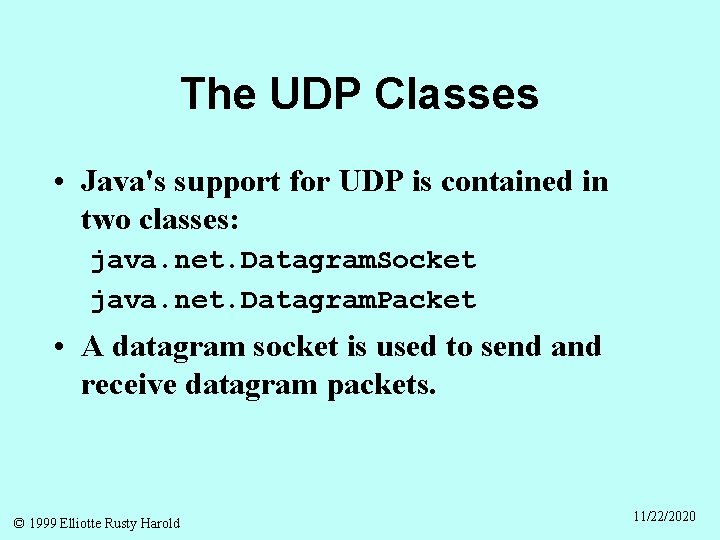
The UDP Classes • Java's support for UDP is contained in two classes: java. net. Datagram. Socket java. net. Datagram. Packet • A datagram socket is used to send and receive datagram packets. © 1999 Elliotte Rusty Harold 11/22/2020
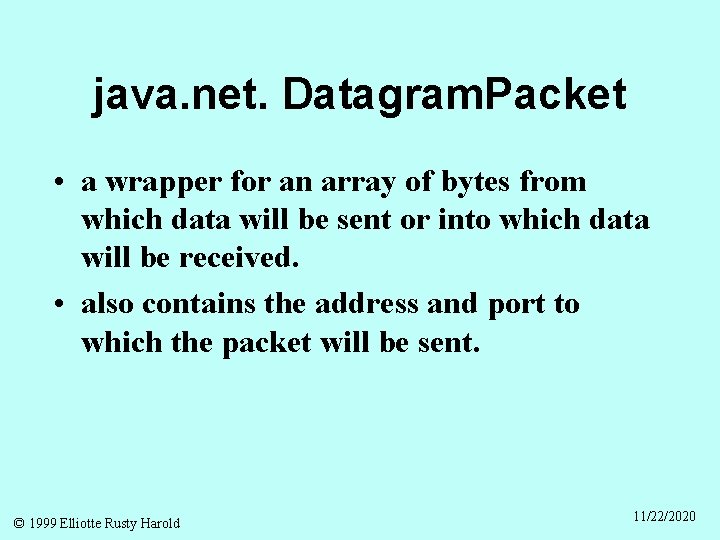
java. net. Datagram. Packet • a wrapper for an array of bytes from which data will be sent or into which data will be received. • also contains the address and port to which the packet will be sent. © 1999 Elliotte Rusty Harold 11/22/2020
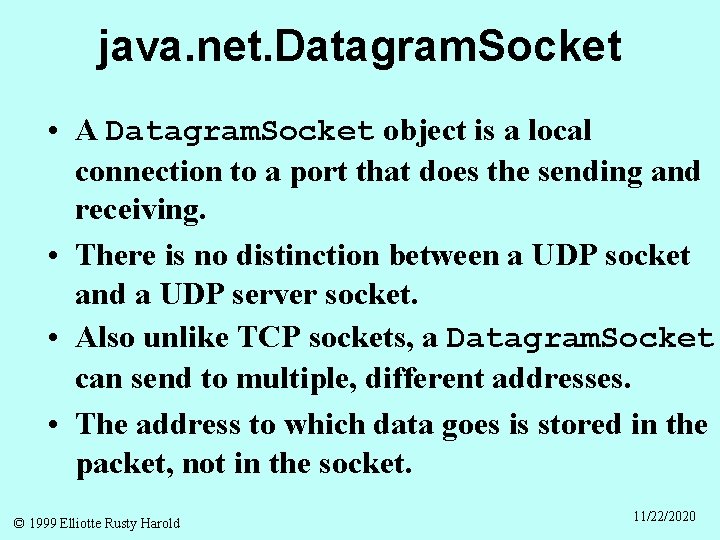
java. net. Datagram. Socket • A Datagram. Socket object is a local connection to a port that does the sending and receiving. • There is no distinction between a UDP socket and a UDP server socket. • Also unlike TCP sockets, a Datagram. Socket can send to multiple, different addresses. • The address to which data goes is stored in the packet, not in the socket. © 1999 Elliotte Rusty Harold 11/22/2020
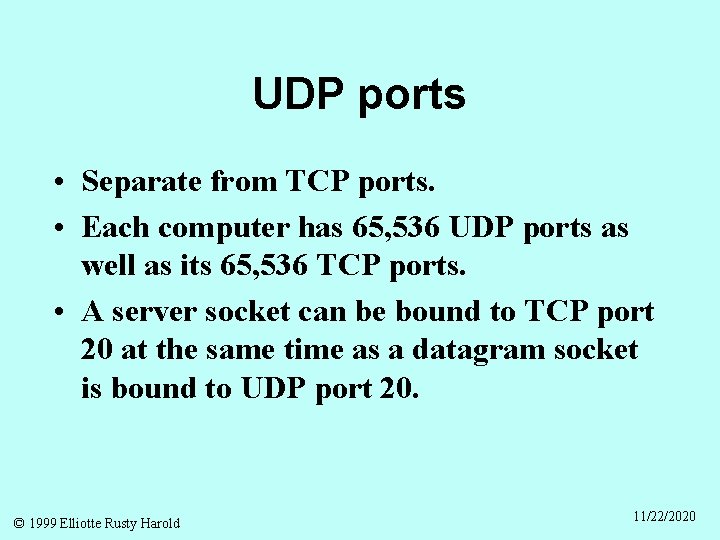
UDP ports • Separate from TCP ports. • Each computer has 65, 536 UDP ports as well as its 65, 536 TCP ports. • A server socket can be bound to TCP port 20 at the same time as a datagram socket is bound to UDP port 20. © 1999 Elliotte Rusty Harold 11/22/2020
![Two Datagram Packet Constructors public Datagram Packetbyte data int length public Datagram Two Datagram. Packet Constructors • public Datagram. Packet(byte[] data, int length) • public Datagram.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-61.jpg)
Two Datagram. Packet Constructors • public Datagram. Packet(byte[] data, int length) • public Datagram. Packet(byte[] data, int length, Inet. Address iaddr, int iport) • First is for receiving, second is for sending © 1999 Elliotte Rusty Harold 11/22/2020
![For example String s My first UDP Packet byte b s get For example, String s = "My first UDP Packet" byte[] b = s. get.](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-62.jpg)
For example, String s = "My first UDP Packet" byte[] b = s. get. Bytes(); Datagram. Packet dp = new Datagram. Packet(b, b. length); © 1999 Elliotte Rusty Harold 11/22/2020
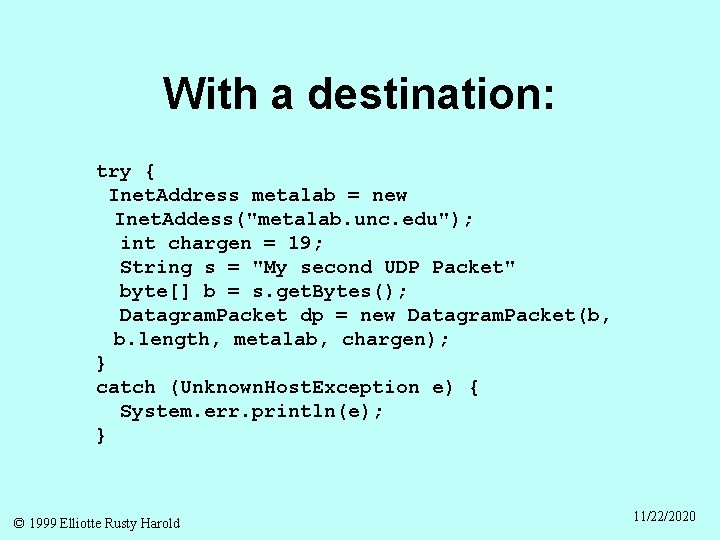
With a destination: try { Inet. Address metalab = new Inet. Addess("metalab. unc. edu"); int chargen = 19; String s = "My second UDP Packet" byte[] b = s. get. Bytes(); Datagram. Packet dp = new Datagram. Packet(b, b. length, metalab, chargen); } catch (Unknown. Host. Exception e) { System. err. println(e); } © 1999 Elliotte Rusty Harold 11/22/2020
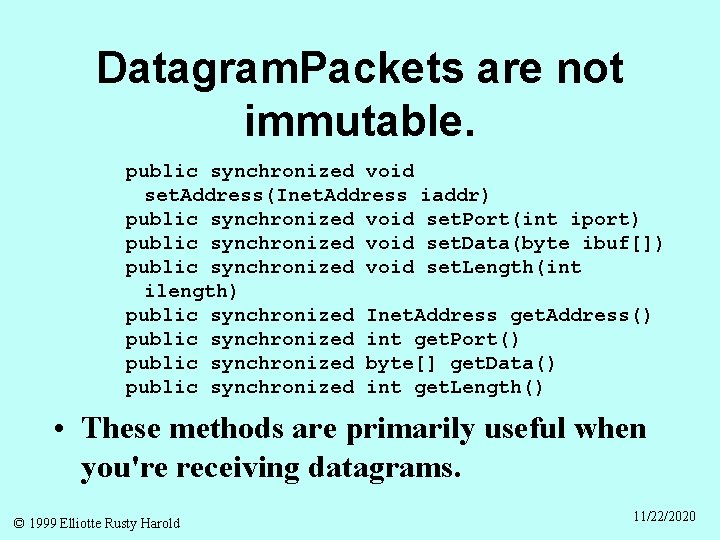
Datagram. Packets are not immutable. public synchronized void set. Address(Inet. Address iaddr) public synchronized void set. Port(int iport) public synchronized void set. Data(byte ibuf[]) public synchronized void set. Length(int ilength) public synchronized Inet. Address get. Address() public synchronized int get. Port() public synchronized byte[] get. Data() public synchronized int get. Length() • These methods are primarily useful when you're receiving datagrams. © 1999 Elliotte Rusty Harold 11/22/2020
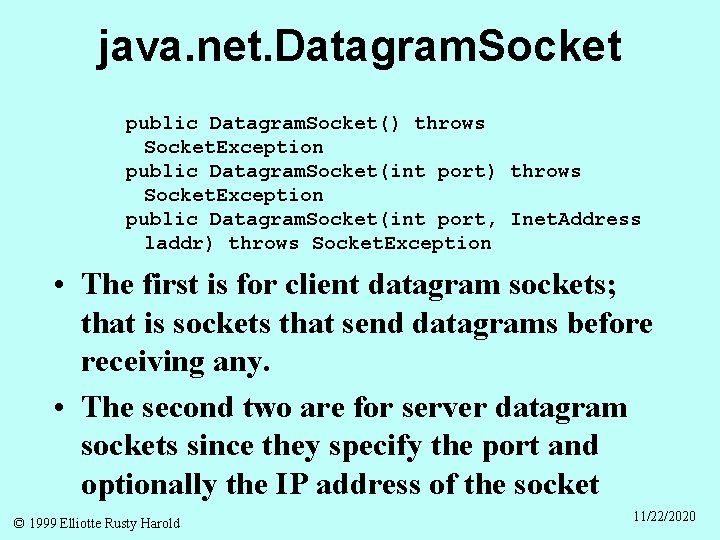
java. net. Datagram. Socket public Datagram. Socket() throws Socket. Exception public Datagram. Socket(int port, Inet. Address laddr) throws Socket. Exception • The first is for client datagram sockets; that is sockets that send datagrams before receiving any. • The second two are for server datagram sockets since they specify the port and optionally the IP address of the socket © 1999 Elliotte Rusty Harold 11/22/2020
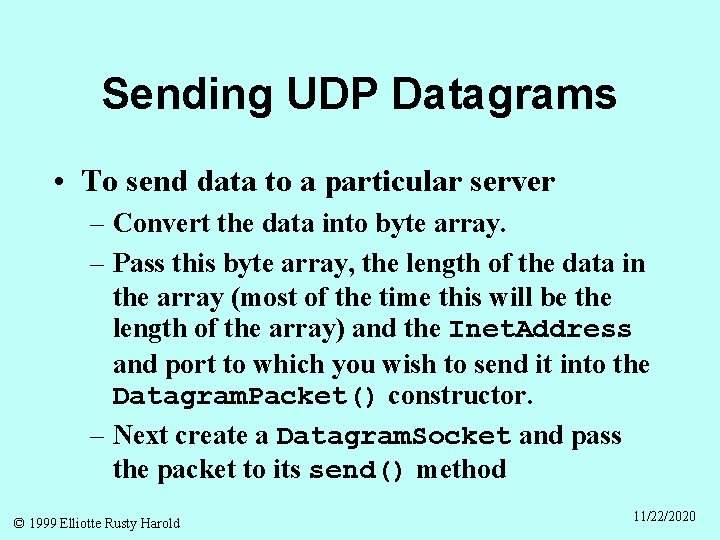
Sending UDP Datagrams • To send data to a particular server – Convert the data into byte array. – Pass this byte array, the length of the data in the array (most of the time this will be the length of the array) and the Inet. Address and port to which you wish to send it into the Datagram. Packet() constructor. – Next create a Datagram. Socket and pass the packet to its send() method © 1999 Elliotte Rusty Harold 11/22/2020
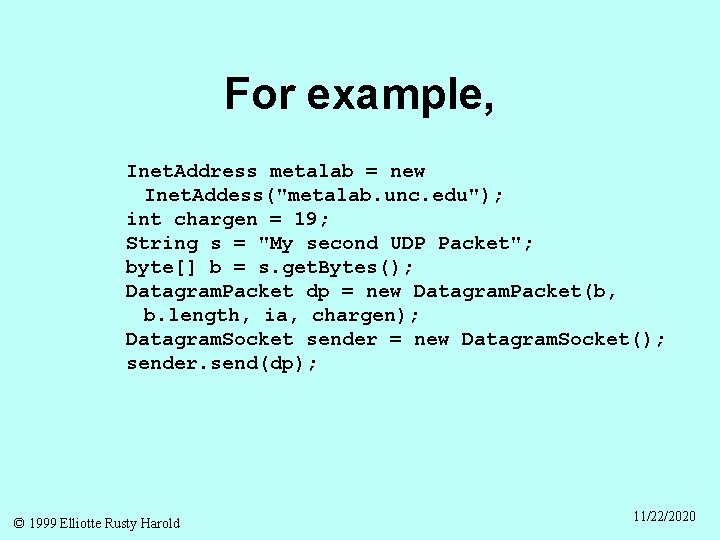
For example, Inet. Address metalab = new Inet. Addess("metalab. unc. edu"); int chargen = 19; String s = "My second UDP Packet"; byte[] b = s. get. Bytes(); Datagram. Packet dp = new Datagram. Packet(b, b. length, ia, chargen); Datagram. Socket sender = new Datagram. Socket(); sender. send(dp); © 1999 Elliotte Rusty Harold 11/22/2020
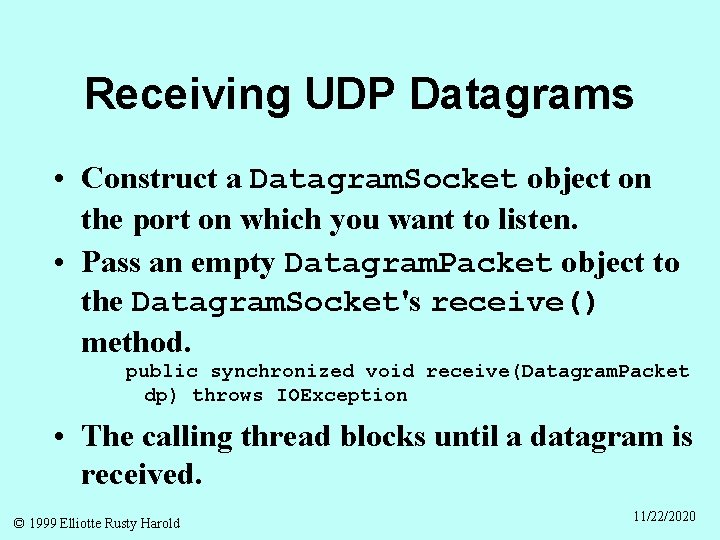
Receiving UDP Datagrams • Construct a Datagram. Socket object on the port on which you want to listen. • Pass an empty Datagram. Packet object to the Datagram. Socket's receive() method. public synchronized void receive(Datagram. Packet dp) throws IOException • The calling thread blocks until a datagram is received. © 1999 Elliotte Rusty Harold 11/22/2020
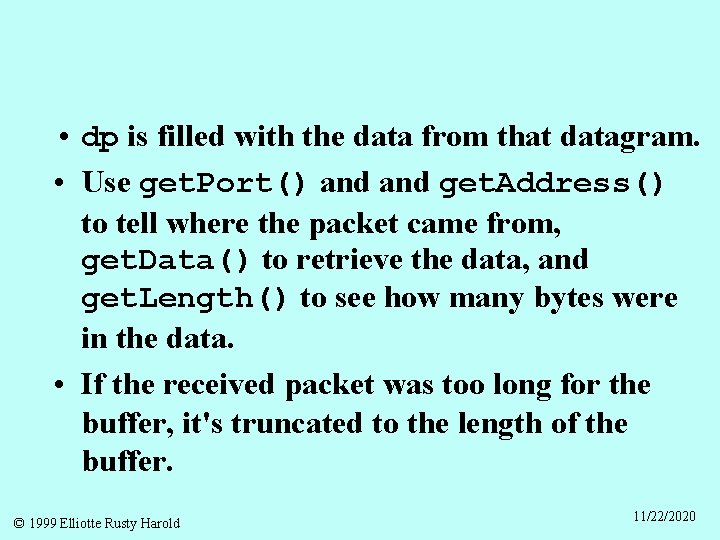
• dp is filled with the data from that datagram. • Use get. Port() and get. Address() to tell where the packet came from, get. Data() to retrieve the data, and get. Length() to see how many bytes were in the data. • If the received packet was too long for the buffer, it's truncated to the length of the buffer. © 1999 Elliotte Rusty Harold 11/22/2020
![For example try byte buffer new byte65536 Datagram Packet incoming new For example, try { byte buffer = new byte[65536]; Datagram. Packet incoming = new](https://slidetodoc.com/presentation_image_h/867bb86b8eb6460fb827992900fb34d6/image-70.jpg)
For example, try { byte buffer = new byte[65536]; Datagram. Packet incoming = new Datagram. Packet(buffer, buffer. length); Datagram. Socket ds = new Datagram. Socket(2134); ds. receive(incoming); byte[] data = incoming. get. Data(); String s = new String(data, 0, data. get. Length()); System. out. println("Port " + incoming. get. Port() + " on " + incoming. get. Address() + " sent this message: "); System. out. println(s); } catch (IOException e) { System. err. println(e); © 1999 Elliotte Rusty Harold } 11/22/2020
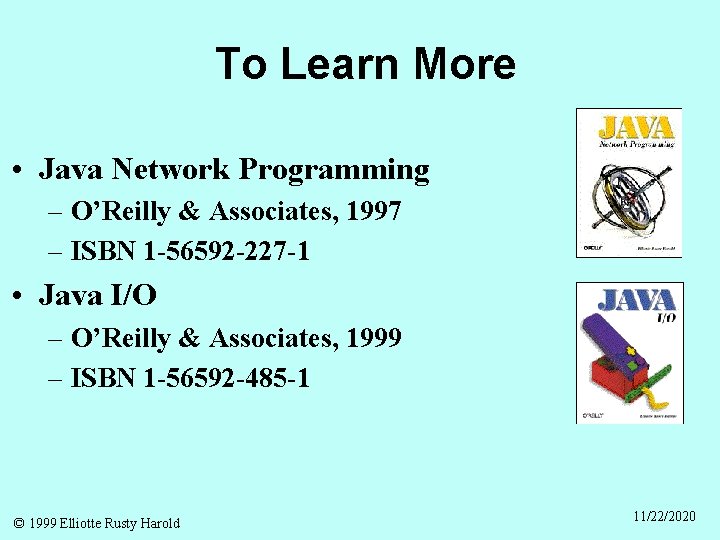
To Learn More • Java Network Programming – O’Reilly & Associates, 1997 – ISBN 1 -56592 -227 -1 • Java I/O – O’Reilly & Associates, 1999 – ISBN 1 -56592 -485 -1 © 1999 Elliotte Rusty Harold 11/22/2020
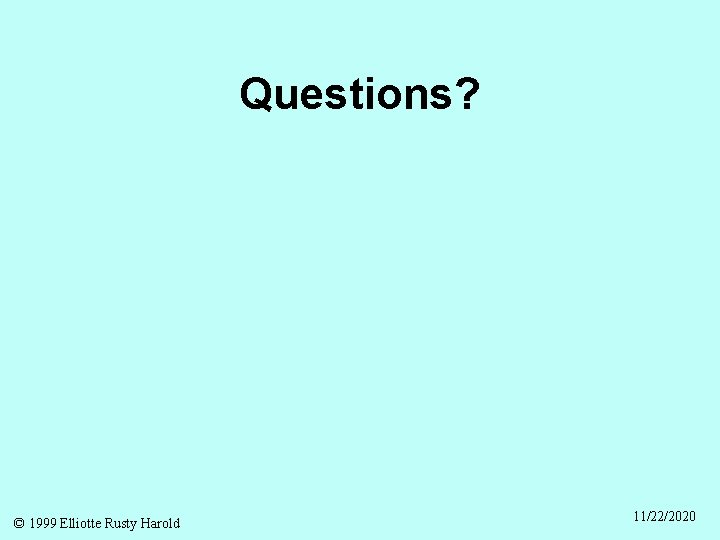
Questions? © 1999 Elliotte Rusty Harold 11/22/2020
Features of peer to peer network and client server network
Tcp/ip sockets in java
Low-level thinking in high-level shading languages
Unix network programming stevens
Sociability continuum
Mid = low + (high - low) / 2
Significant figures
Low voltage hazards
Sockets and threads
Low latency microservices in java
Tcp echo server
Nocturnal low level jet
Which diagram describes "who can do what in a system".
Low level programming languages
Low level jet
Machine language is a low level language
What are radiant points for
Designing and implementing brand architecture strategies
Low level disinfectant
Maritime front
Prognostic chart symbols
Materi sistem informasi manajemen
Hubel and wiesel experiment
Stratocumulus clouds
Low level anime
Low level protocol
Writing device drivers for embedded systems
Alexis farah
Low level analysis
Pure mobile web apps are written only in
Echelle fitzpatrick
Xkcd programming languages comparison
Debugging history
What is jsp file
Pircbot
Java irc server
Application
Java.rmi.server.codebase
Lập trình socket giao tiếp tcp client/server java
Knock knock server java
Java server pages life cycle
Java server pages
Java server pages
Jsp o que é
Censer
Tcp ip sockets in c
Car steering components
Sockets direct protocol
Socket primitives
Reliable datagram sockets
Raw socket
D3azeg_clwi -site:youtube.com
Cleanroom 380v sockets
Socket r
Tanenbaum linux
Secure sockets
도나도나 tcp
Reliable datagram sockets
Berkeley sockets
Components of client server architecture
Server client architecture
Windows server 2003 network infrastructure
Datagram switching
Network centric computing
Significance level and confidence level
Confidence level and significance level
Confidence level and significance level
Dfd level 0 and level 1
Import java util scanner
Java import java.util.*
Import java.applet.applet
Java import java.io.*
Import java.io.file;