Java Programming Reference type Contents How Reference type
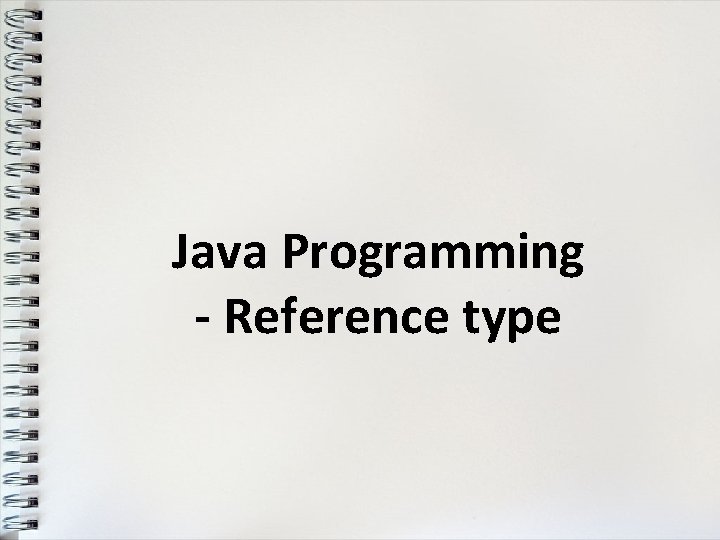
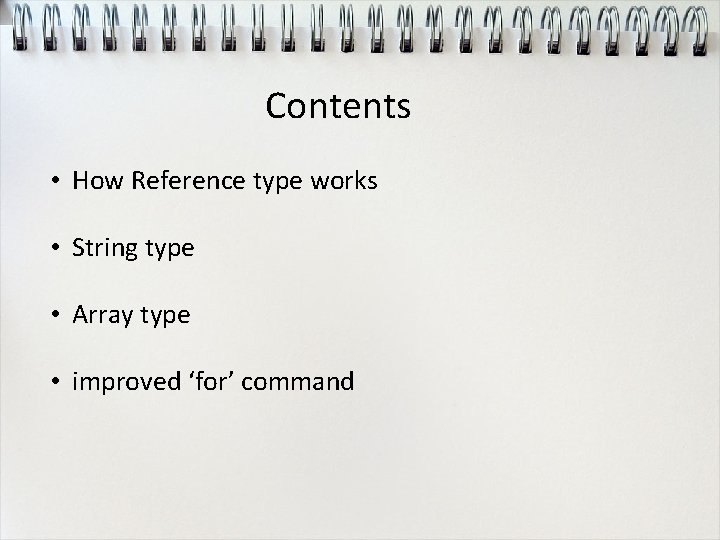
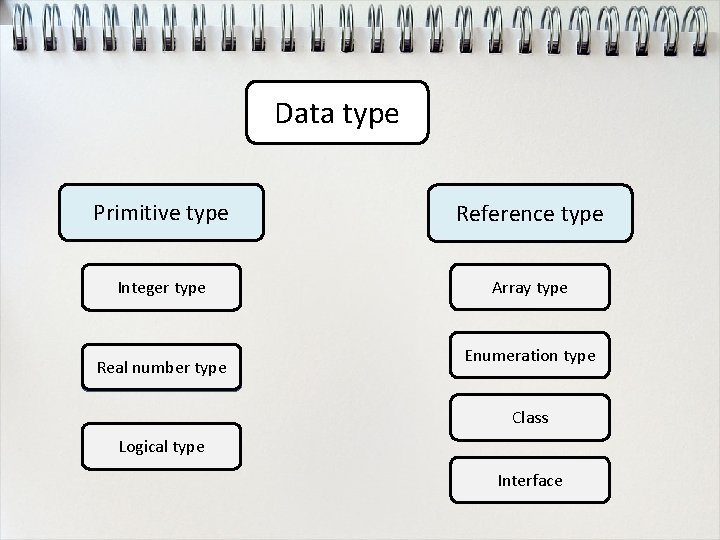
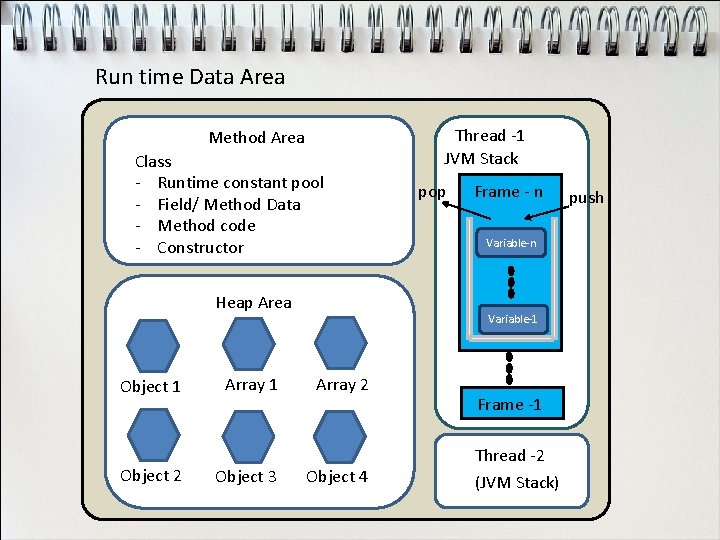
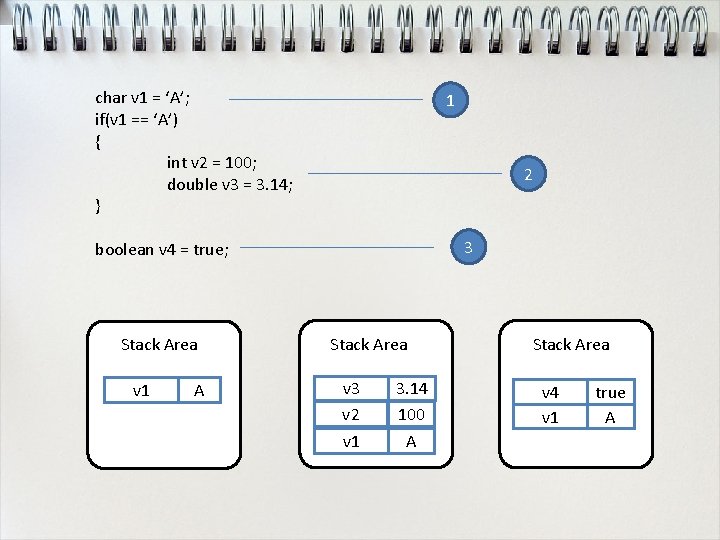
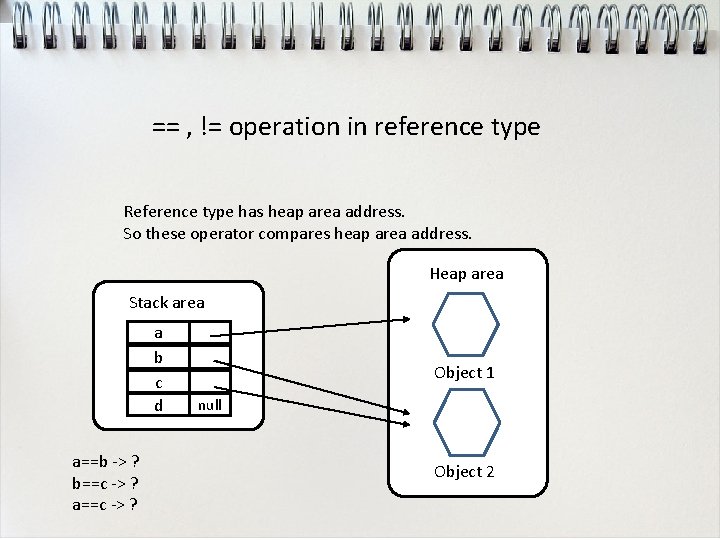
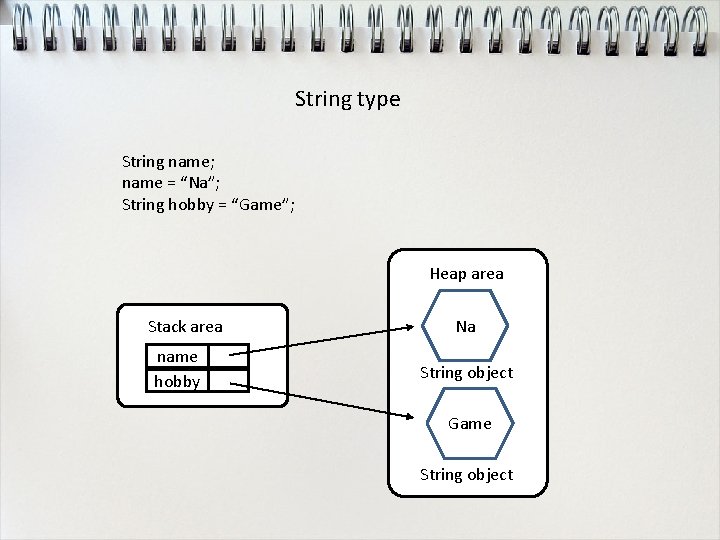
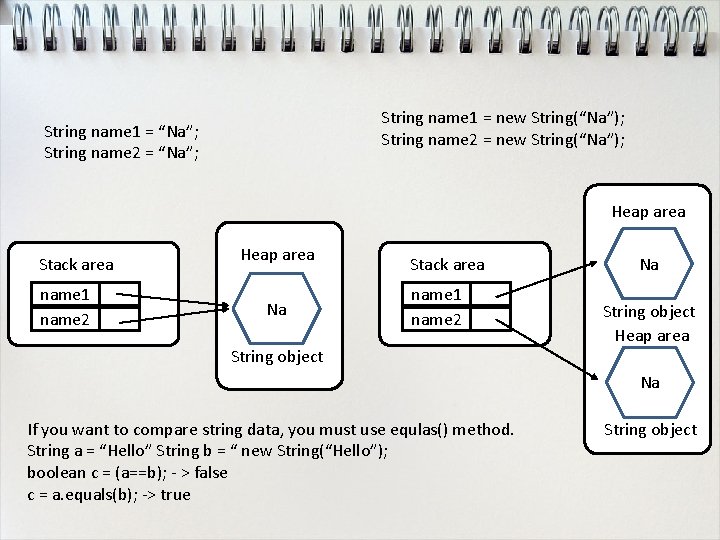
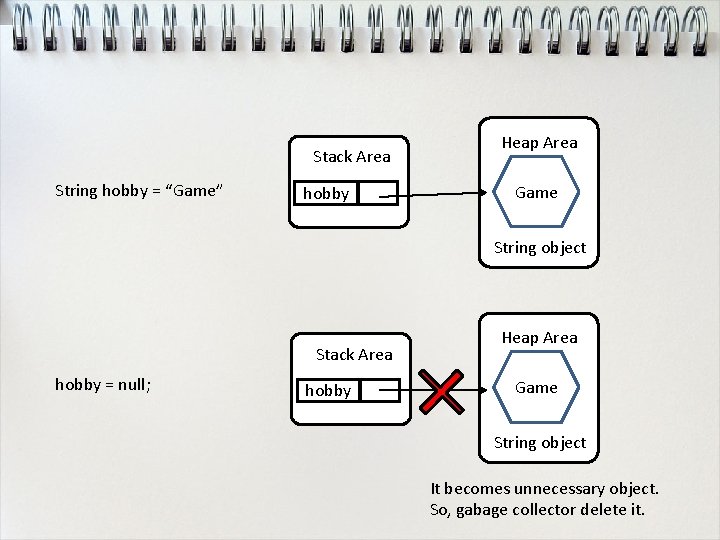
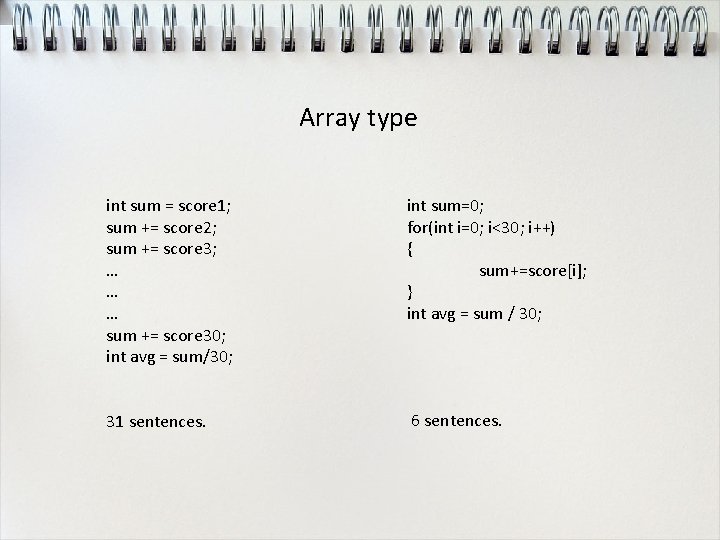
![How to declare Array 1. (type)[] (variable name); ex) int[] int. Array; 2. (type) How to declare Array 1. (type)[] (variable name); ex) int[] int. Array; 2. (type)](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-11.jpg)
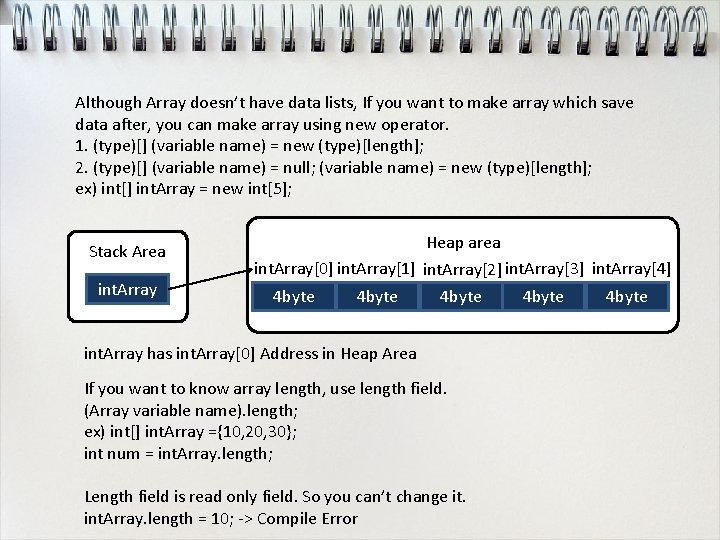
![Category Data type Initialization Primitive type (Integer) byte[] char[] short[] int[] long[] 0 ‘u Category Data type Initialization Primitive type (Integer) byte[] char[] short[] int[] long[] 0 ‘u](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-13.jpg)
![Input Command line public static void main( String[] args ){…} When you run program Input Command line public static void main( String[] args ){…} When you run program](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-14.jpg)
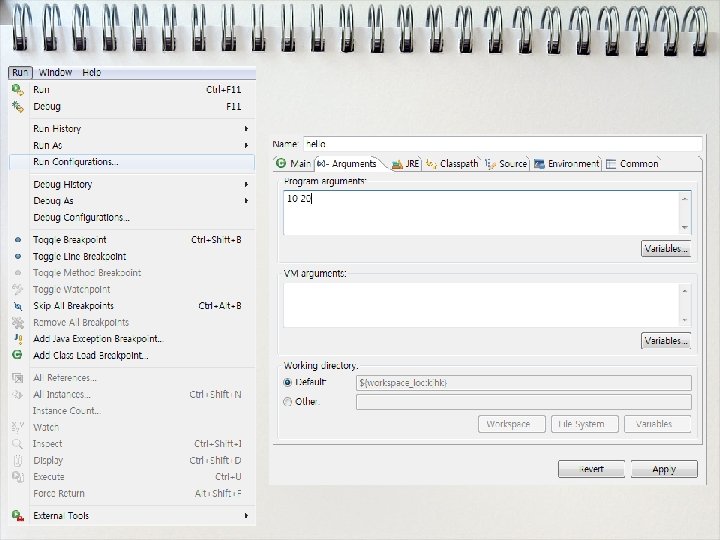
![How to declare multiple dimension array int[][] num ={{0, 1}, {2, 3}}; same with How to declare multiple dimension array int[][] num ={{0, 1}, {2, 3}}; same with](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-16.jpg)
![Java array can have stairs structure like following example int[][] num = new int[2][]; Java array can have stairs structure like following example int[][] num = new int[2][];](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-17.jpg)
![Copy in Array Shallow copy Deep copy String[] old. Int. Array= {"a", "b", "c"}; Copy in Array Shallow copy Deep copy String[] old. Int. Array= {"a", "b", "c"};](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-18.jpg)
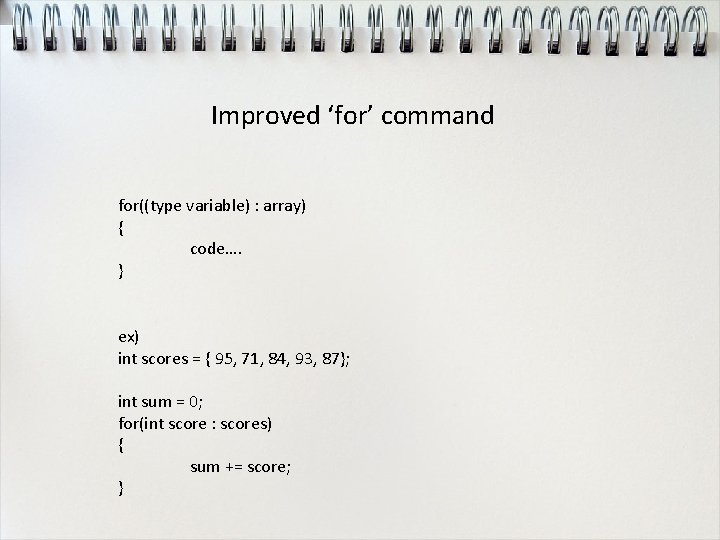
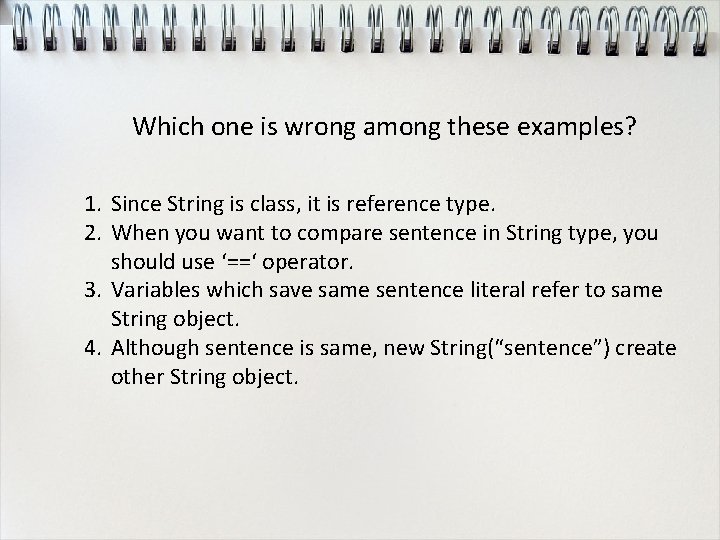
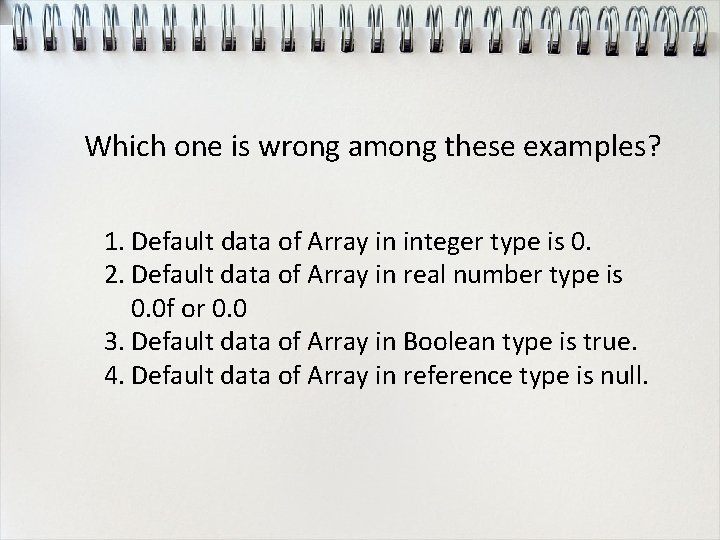
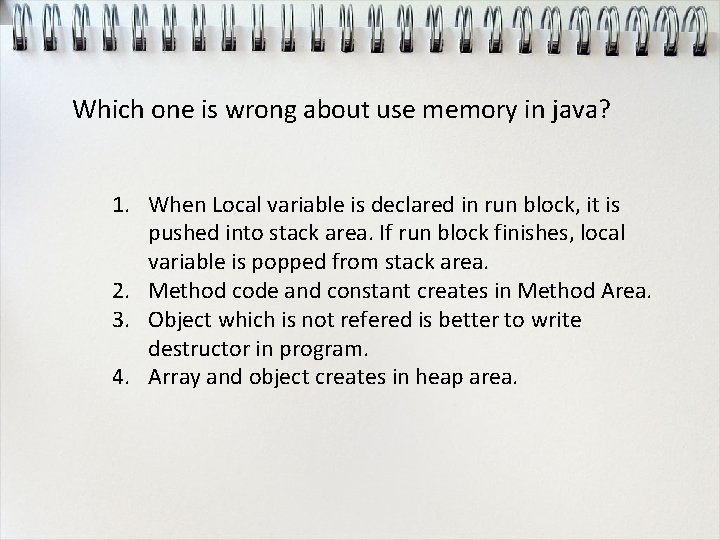
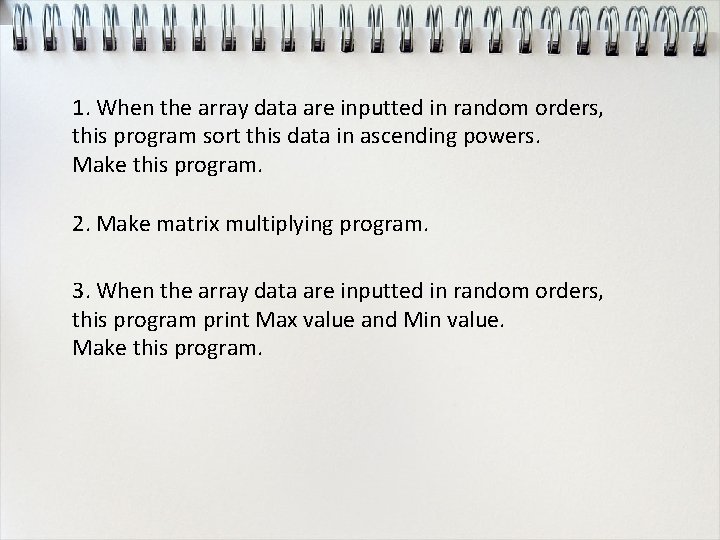
- Slides: 23
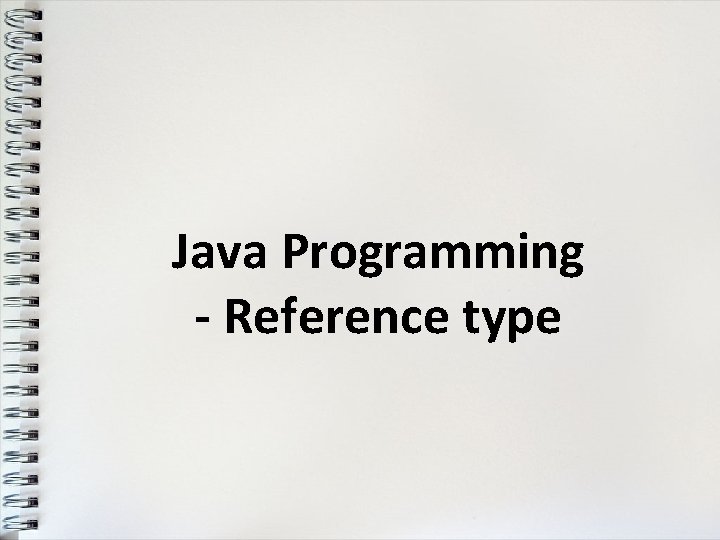
Java Programming - Reference type
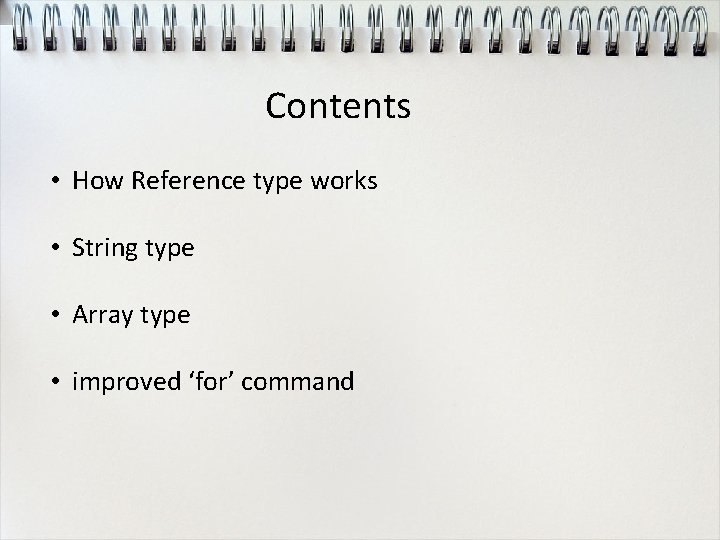
Contents • How Reference type works • String type • Array type • improved ‘for’ command
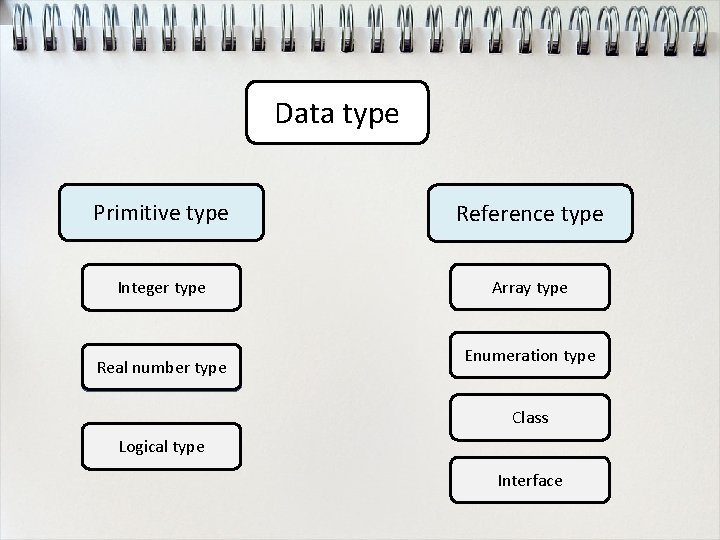
Data type Primitive type Reference type Integer type Array type Real number type Enumeration type Class Logical type Interface
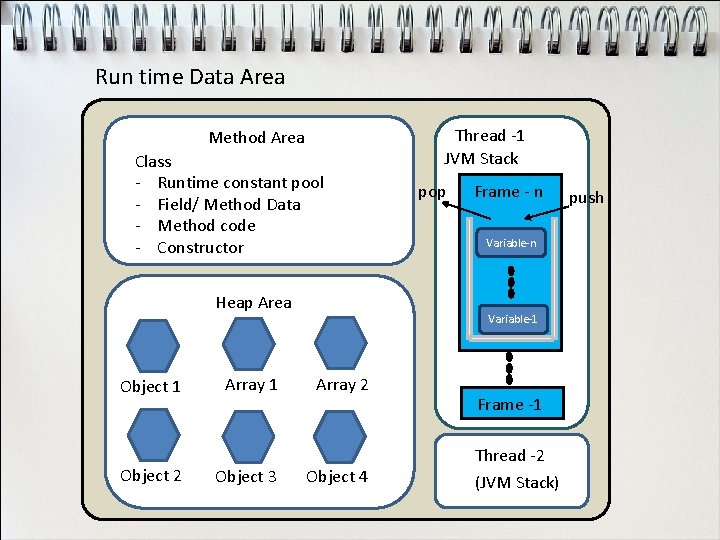
Run time Data Area Method Area Class - Runtime constant pool - Field/ Method Data - Method code - Constructor Heap Area Object 1 Object 2 Array 1 Object 3 Thread -1 JVM Stack pop Frame - n Variable-1 Array 2 Object 4 Frame -1 Thread -2 (JVM Stack) push
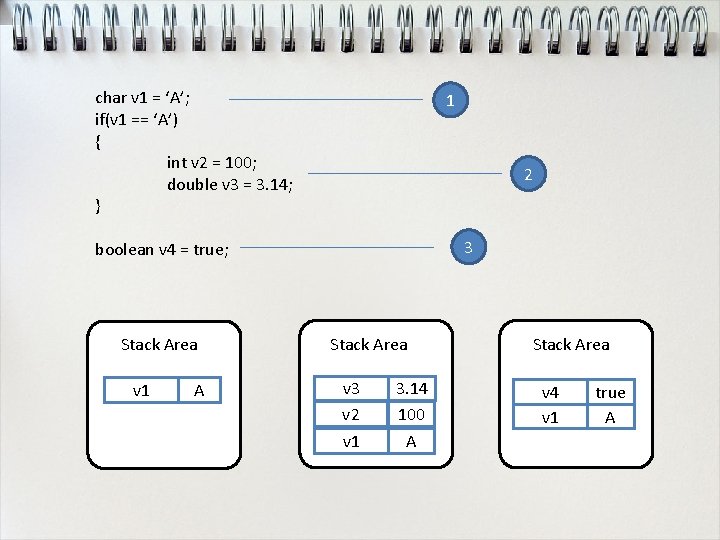
char v 1 = ‘A’; if(v 1 == ‘A’) { int v 2 = 100; double v 3 = 3. 14; } 1 2 3 boolean v 4 = true; Stack Area v 1 A Stack Area v 3 v 2 v 1 3. 14 100 A Stack Area v 4 v 1 true A
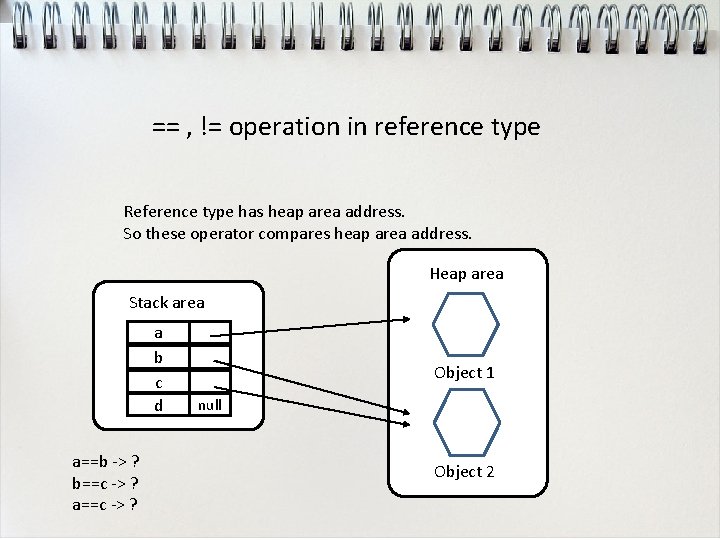
== , != operation in reference type Reference type has heap area address. So these operator compares heap area address. Heap area Stack area a b c d a==b -> ? b==c -> ? a==c -> ? Object 1 null Object 2
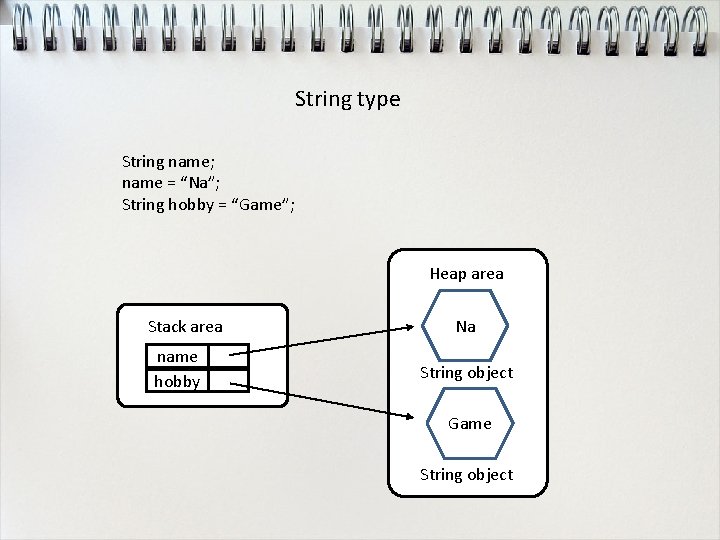
String type String name; name = “Na”; String hobby = “Game”; Heap area Stack area name hobby Na String object Game String object
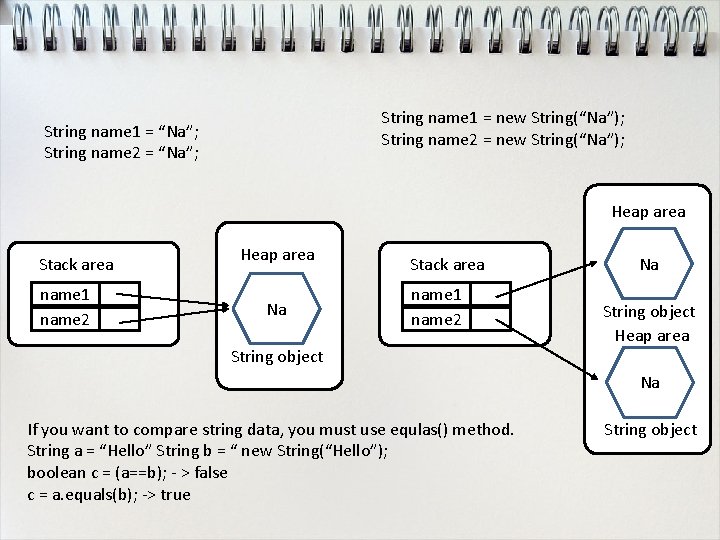
String name 1 = new String(“Na”); String name 2 = new String(“Na”); String name 1 = “Na”; String name 2 = “Na”; Heap area Stack area name 1 name 2 Heap area Na Stack area name 1 name 2 String object Na String object Heap area Na If you want to compare string data, you must use equlas() method. String a = “Hello” String b = “ new String(“Hello”); boolean c = (a==b); - > false c = a. equals(b); -> true String object
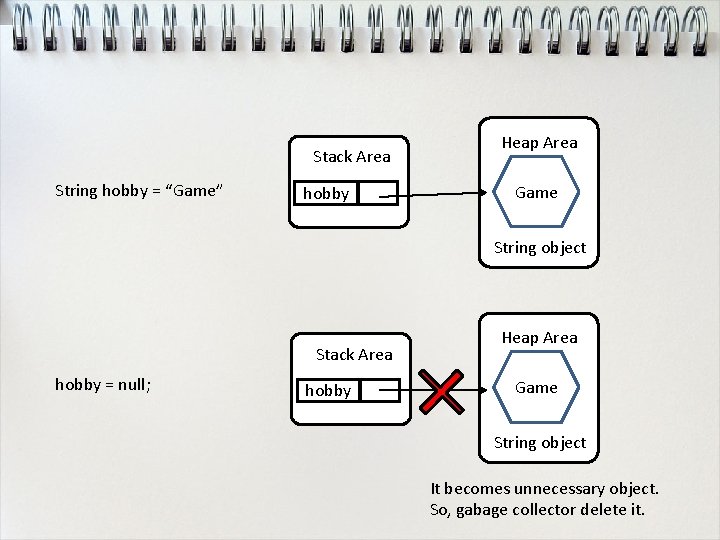
Stack Area String hobby = “Game” hobby Heap Area Game String object Stack Area hobby = null; hobby Heap Area Game String object It becomes unnecessary object. So, gabage collector delete it.
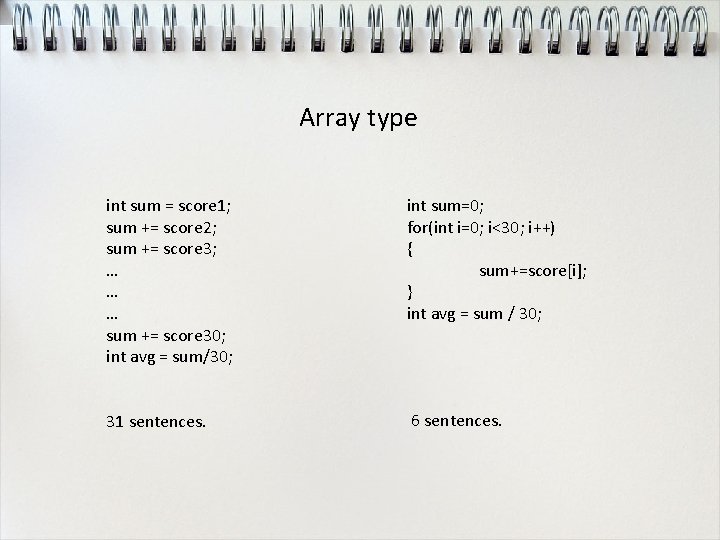
Array type int sum = score 1; sum += score 2; sum += score 3; … … … sum += score 30; int avg = sum/30; int sum=0; for(int i=0; i<30; i++) { sum+=score[i]; } int avg = sum / 30; 31 sentences. 6 sentences.
![How to declare Array 1 type variable name ex int int Array 2 type How to declare Array 1. (type)[] (variable name); ex) int[] int. Array; 2. (type)](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-11.jpg)
How to declare Array 1. (type)[] (variable name); ex) int[] int. Array; 2. (type) (variable name)[]; ex) double. Array[]; When you declare array variable, you can’t declare using brace in other codes ex)int[] int. Array; int. Array={data 1, data 2, data 3…. }; -> Compile Error First, declare the array variable. Then if the data lists are decided later, you can designate the data lists by using ‘new’ operator like the following example. Put “type[]” which used in declaring the array variables right after the ‘new’ operators, and list the result in the braces. ex)int[] int. Array; int. Array = new int[] {0, 1, 2, 3}; The processes are identical even when the parameter of the method is in array. ex)int add(int[] scores){…. . } int] result = add({95, 90, 85}); -> Compile Error int result = add(new int[]{95, 90, 85});
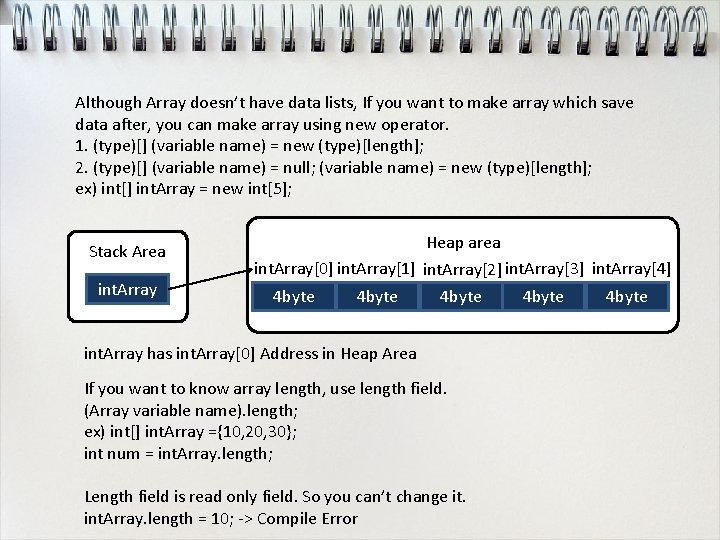
Although Array doesn’t have data lists, If you want to make array which save data after, you can make array using new operator. 1. (type)[] (variable name) = new (type)[length]; 2. (type)[] (variable name) = null; (variable name) = new (type)[length]; ex) int[] int. Array = new int[5]; Stack Area int. Array Heap area int. Array[0] int. Array[1] int. Array[2] int. Array[3] int. Array[4] 4 byte int. Array has int. Array[0] Address in Heap Area If you want to know array length, use length field. (Array variable name). length; ex) int[] int. Array ={10, 20, 30}; int num = int. Array. length; Length field is read only field. So you can’t change it. int. Array. length = 10; -> Compile Error 4 byte
![Category Data type Initialization Primitive type Integer byte char short int long 0 u Category Data type Initialization Primitive type (Integer) byte[] char[] short[] int[] long[] 0 ‘u](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-13.jpg)
Category Data type Initialization Primitive type (Integer) byte[] char[] short[] int[] long[] 0 ‘u 0000’ 0 0 0 L Primitive type (Real Number) float[] double[] 0. 0 F 0. 0 Primitive type (Logical) boolean[] false Reference type class[] null Interface[] null
![Input Command line public static void main String args When you run program Input Command line public static void main( String[] args ){…} When you run program](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-14.jpg)
Input Command line public static void main( String[] args ){…} When you run program by java class, JVM creates String Array which length is 0. String Array is provided as a parameter to main method. If you provide string lists like following example java class string 0 string 1 string 2 …. string n-1 String[] args = {string 0, string 1, string 2, …. , string n-1}; public static void main(String[] args){…. } Computer create string array which have string list. In addition, When main method is called, it is provided as a parameter.
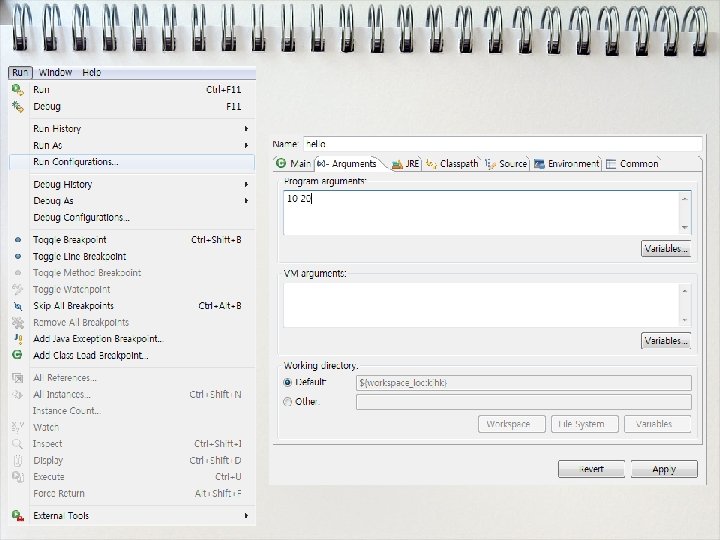
![How to declare multiple dimension array int num 0 1 2 3 same with How to declare multiple dimension array int[][] num ={{0, 1}, {2, 3}}; same with](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-16.jpg)
How to declare multiple dimension array int[][] num ={{0, 1}, {2, 3}}; same with int[][] num; num = int[][]{{0, 1}, {2, 3}}; Stack Area num Heap Area length: 2 int Array A length: 2 int Array B int Array C
![Java array can have stairs structure like following example int num new int2 Java array can have stairs structure like following example int[][] num = new int[2][];](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-17.jpg)
Java array can have stairs structure like following example int[][] num = new int[2][]; num[0] = new int[2]; num[1] = new int[3]; Stack Area num. length // 2 Array A length num[0]. length //2 Array B length num[1]. length //3 Array C length Heap Area length: 2 int Array A length: 2 int Array B int Array C
![Copy in Array Shallow copy Deep copy String old Int Array a b c Copy in Array Shallow copy Deep copy String[] old. Int. Array= {"a", "b", "c"};](https://slidetodoc.com/presentation_image/2acdf59e3228c08eb5535a7947682262/image-18.jpg)
Copy in Array Shallow copy Deep copy String[] old. Int. Array= {"a", "b", "c"}; String[] new. Int. Array= new String[5]; for(int i=0; i<old. Int. Array. length; i++) new. Int. Array[i] = old. Int. Array[i]; for(int i=0; i<old. Int. Array. length; i++) new. Int. Array[i] = new String(old. Int. Array[i]);
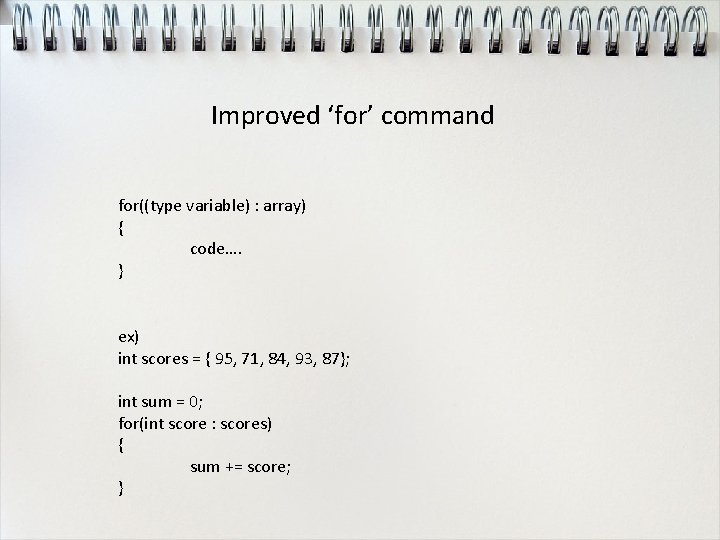
Improved ‘for’ command for((type variable) : array) { code…. } ex) int scores = { 95, 71, 84, 93, 87}; int sum = 0; for(int score : scores) { sum += score; }
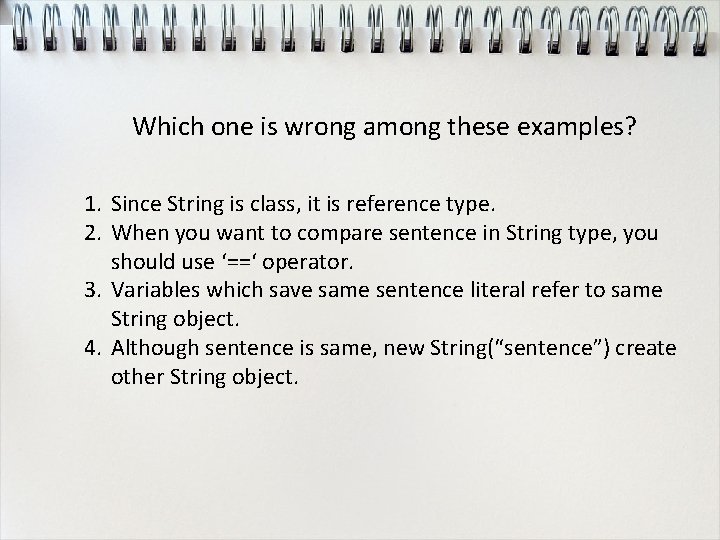
Which one is wrong among these examples? 1. Since String is class, it is reference type. 2. When you want to compare sentence in String type, you should use ‘==‘ operator. 3. Variables which save same sentence literal refer to same String object. 4. Although sentence is same, new String(“sentence”) create other String object.
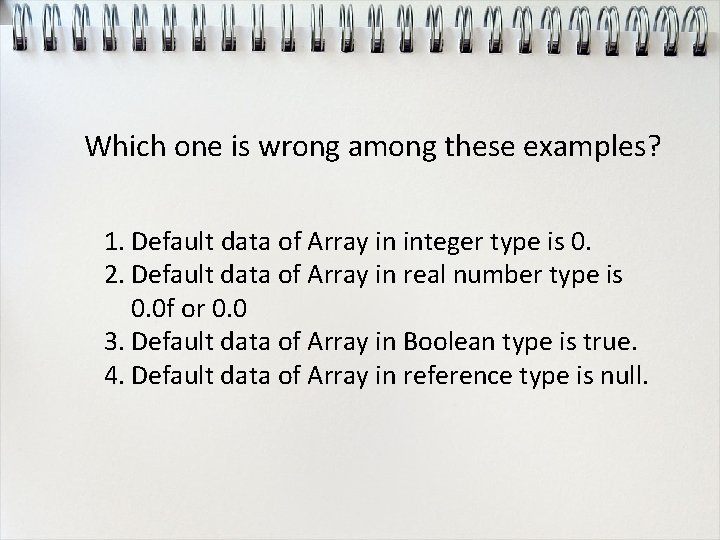
Which one is wrong among these examples? 1. Default data of Array in integer type is 0. 2. Default data of Array in real number type is 0. 0 f or 0. 0 3. Default data of Array in Boolean type is true. 4. Default data of Array in reference type is null.
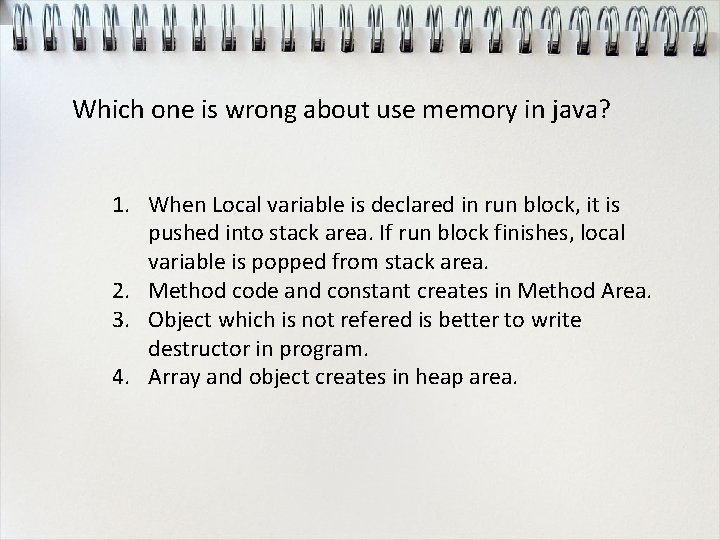
Which one is wrong about use memory in java? 1. When Local variable is declared in run block, it is pushed into stack area. If run block finishes, local variable is popped from stack area. 2. Method code and constant creates in Method Area. 3. Object which is not refered is better to write destructor in program. 4. Array and object creates in heap area.
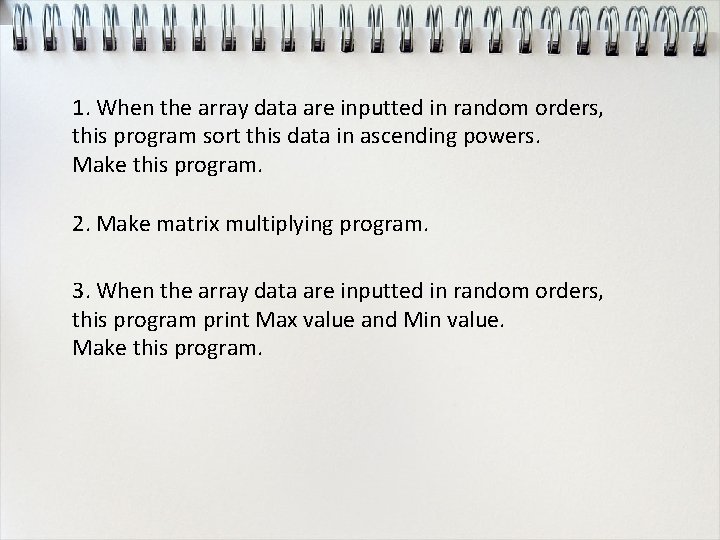
1. When the array data are inputted in random orders, this program sort this data in ascending powers. Make this program. 2. Make matrix multiplying program. 3. When the array data are inputted in random orders, this program print Max value and Min value. Make this program.
Reference type in java
Reference type and value type
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Windows 10 system programming, part 1
Integer programming vs linear programming
Definisi linear
Application
Parallel programming java
Object oriented programming exercises java
Problem solving
Event driven programming in java
Elementary programming in java
Java asynchronous programming
Java structured programming
Importance of java programming
Khan academy java
Event driven programming in java
Defensive programming java
Java programming refresher
Java games programming
Java symbols
Java an introduction to problem solving and programming
Elementary programming in java