Java IO L Grewe Overview of java io
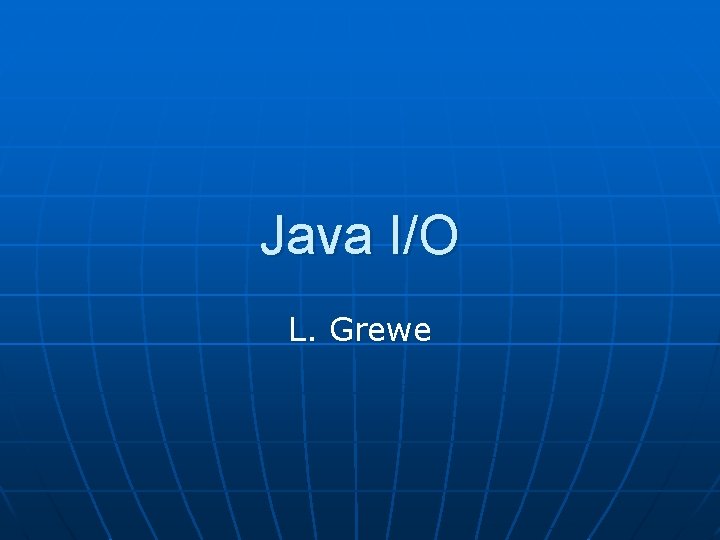
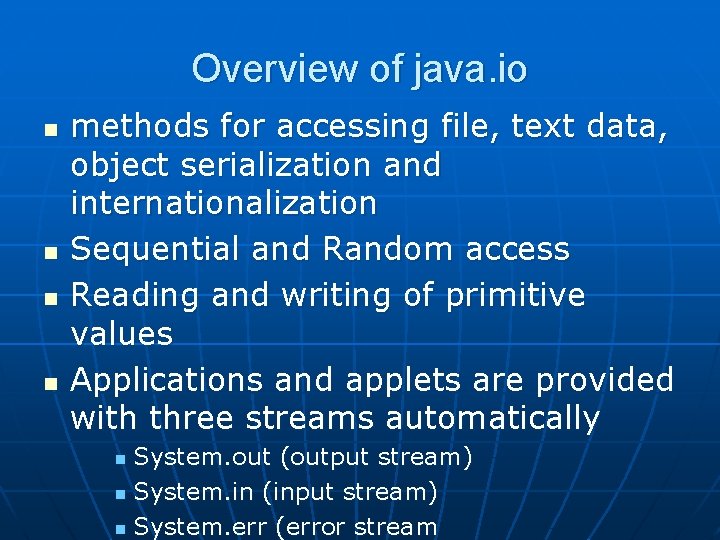
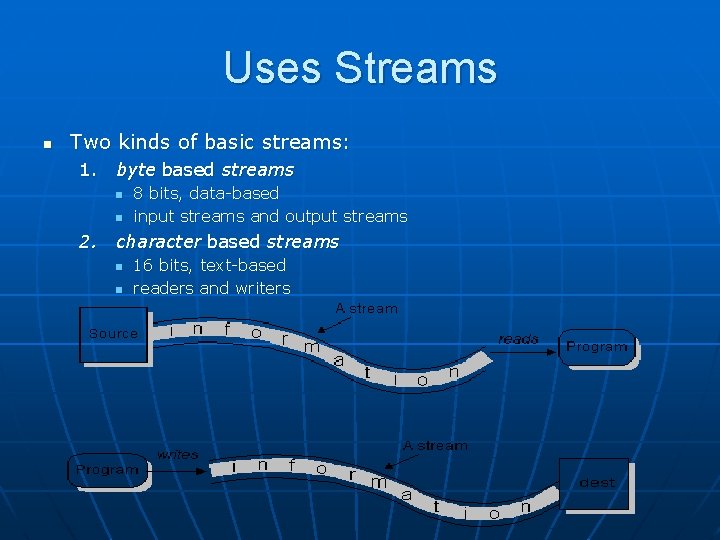
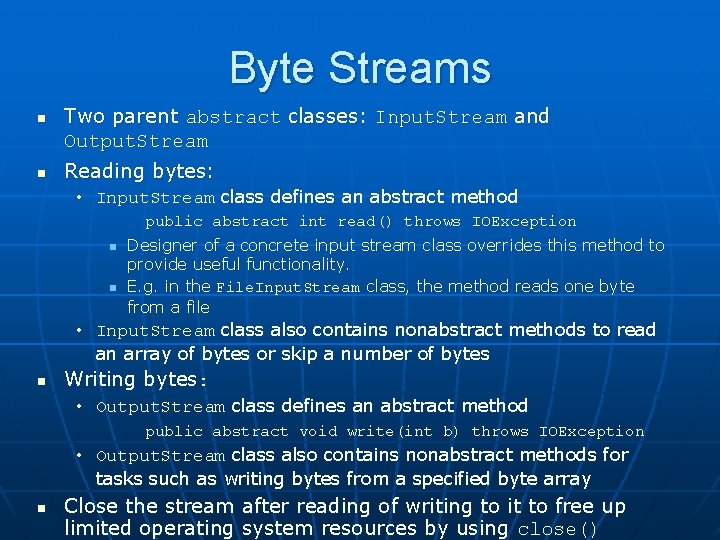
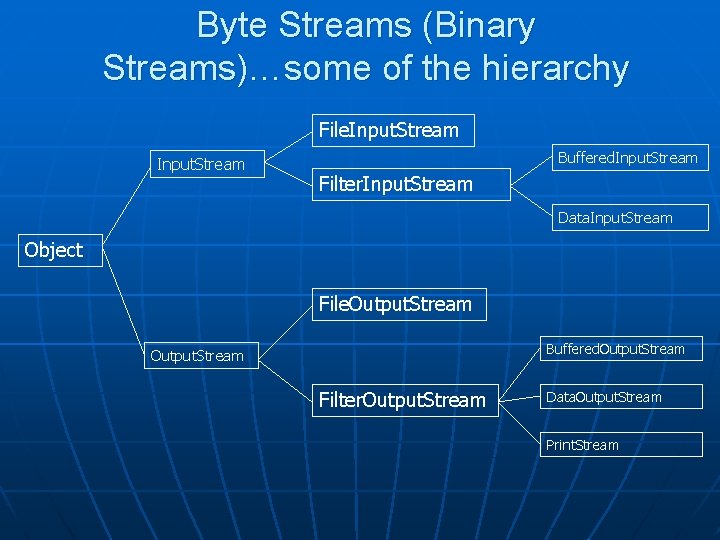
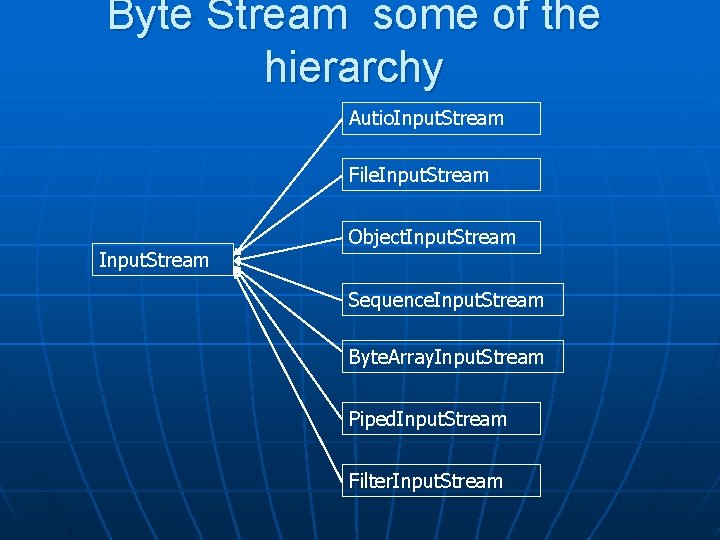
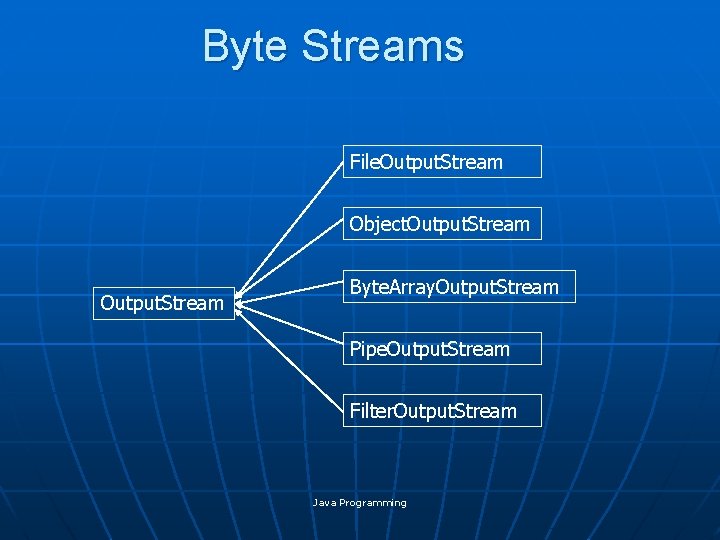
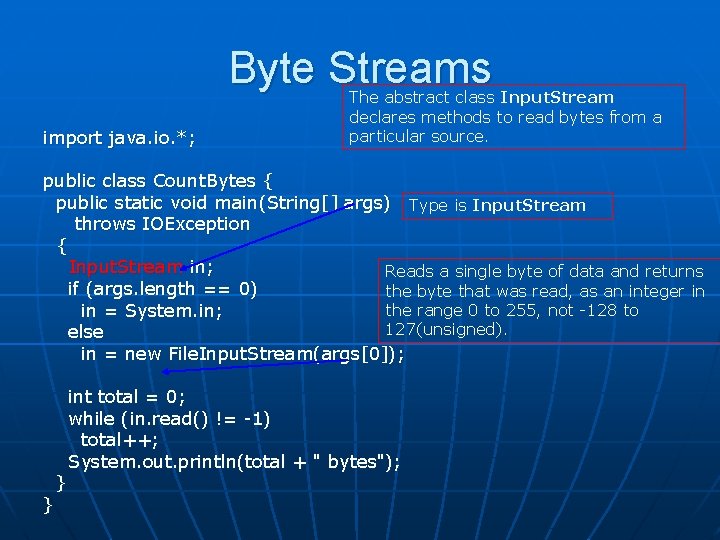
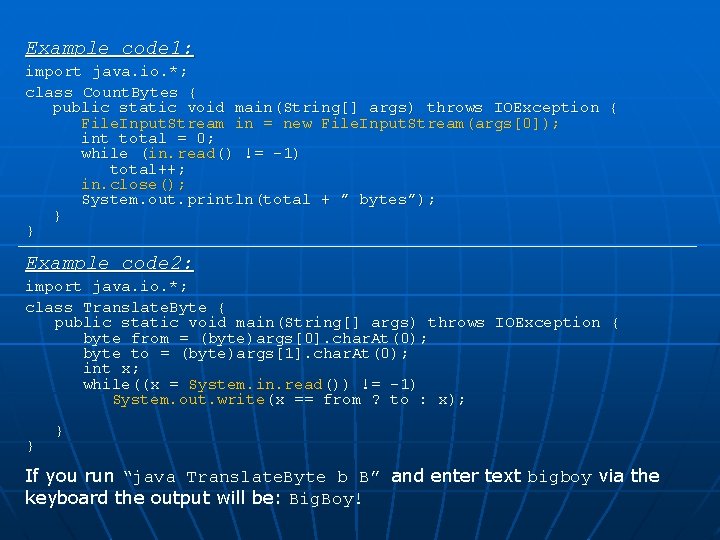
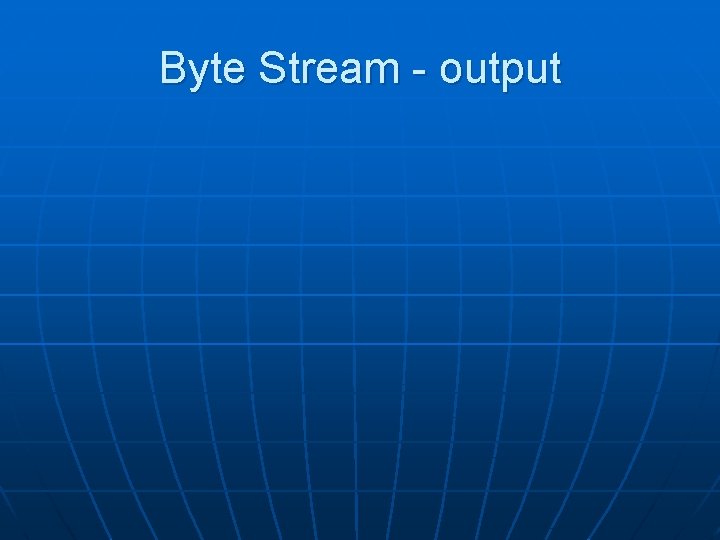
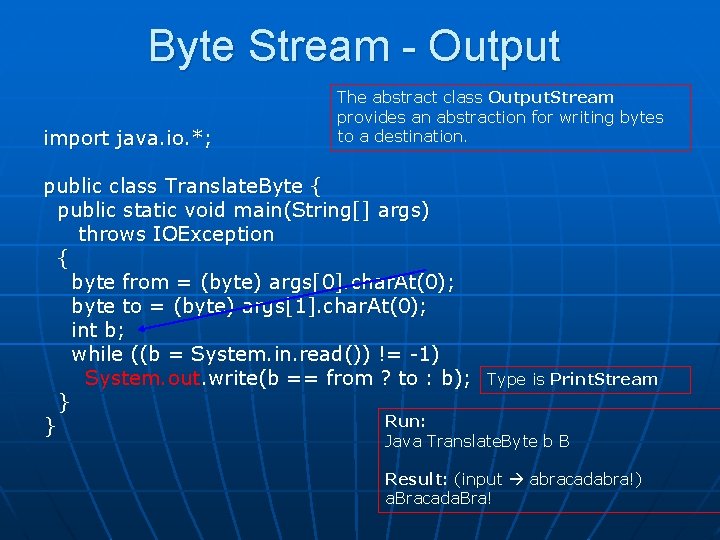
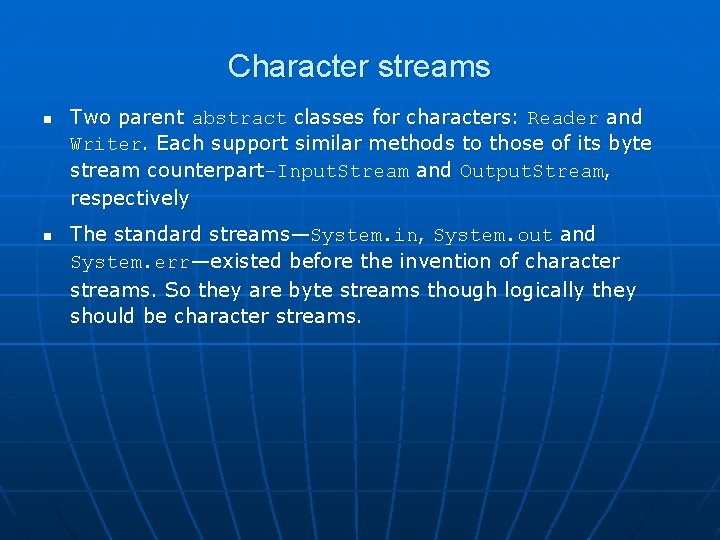
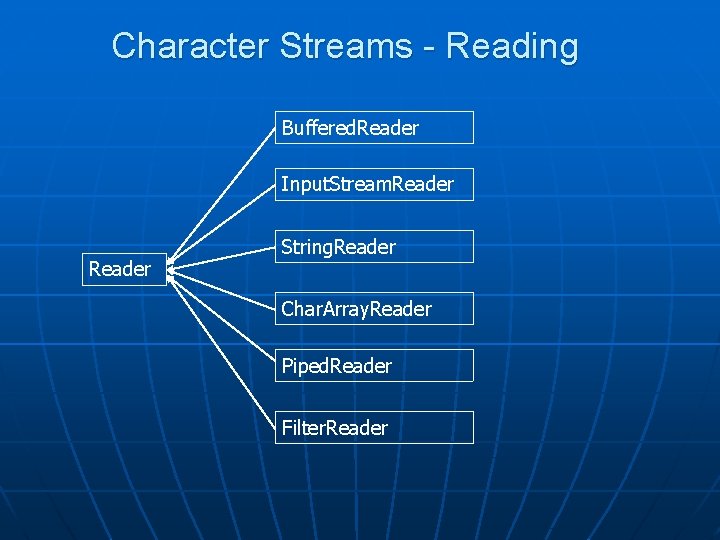
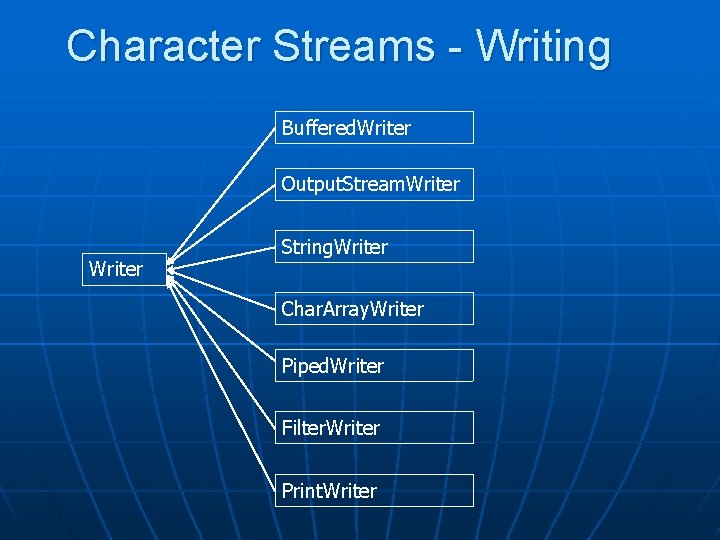
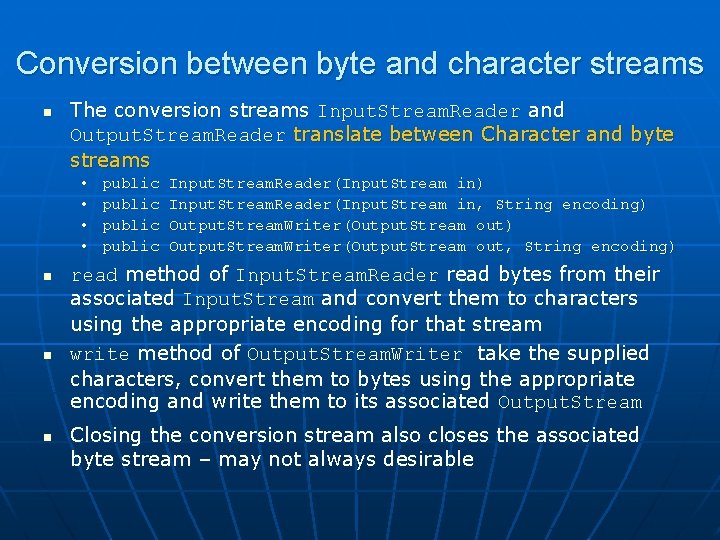
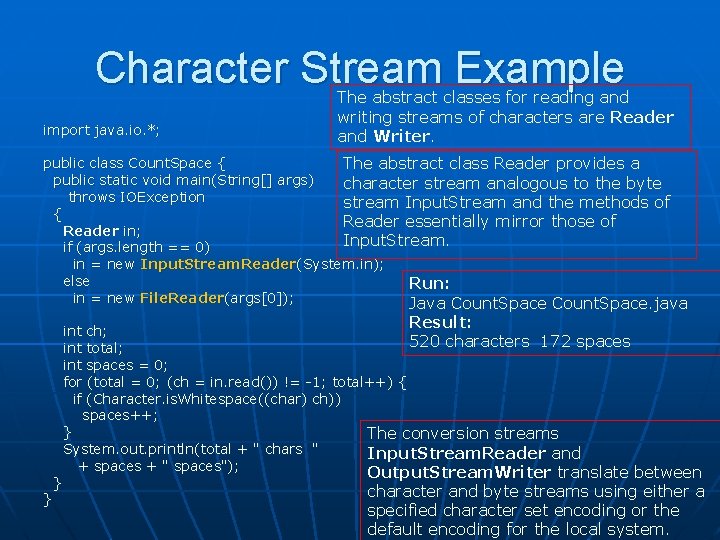
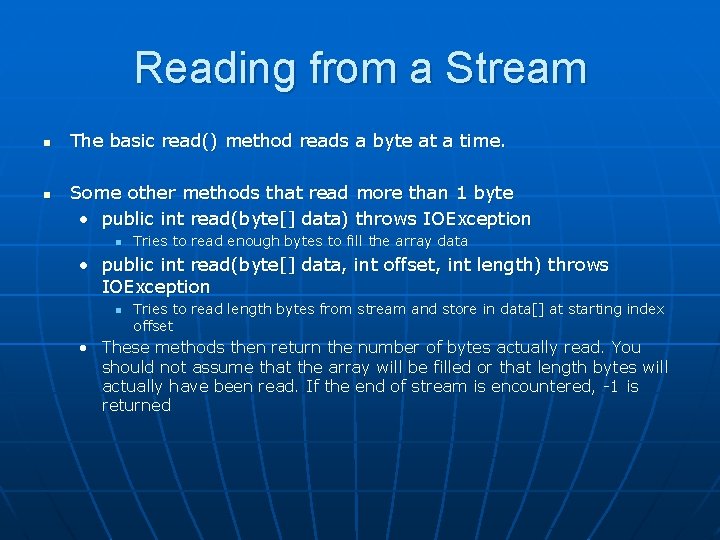
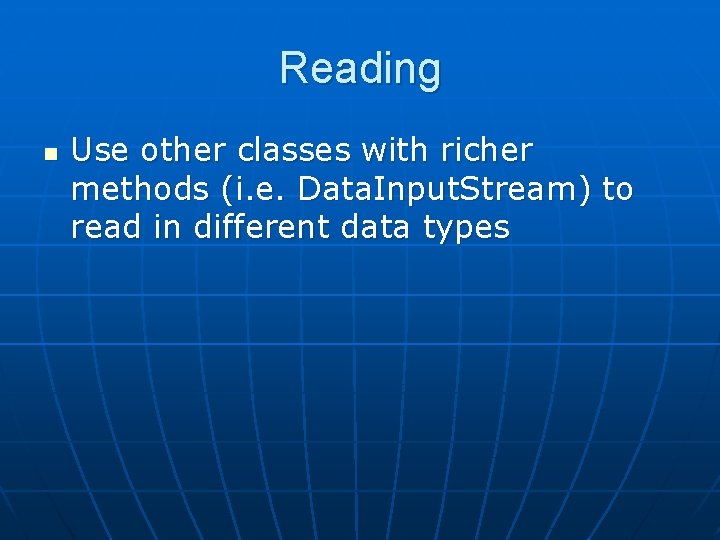
![Writing n n n abstract void write(char[] cbuf, int off, int len) Write a Writing n n n abstract void write(char[] cbuf, int off, int len) Write a](https://slidetodoc.com/presentation_image_h2/0fabfdeb834c28f86d066a85d2cbaede/image-19.jpg)
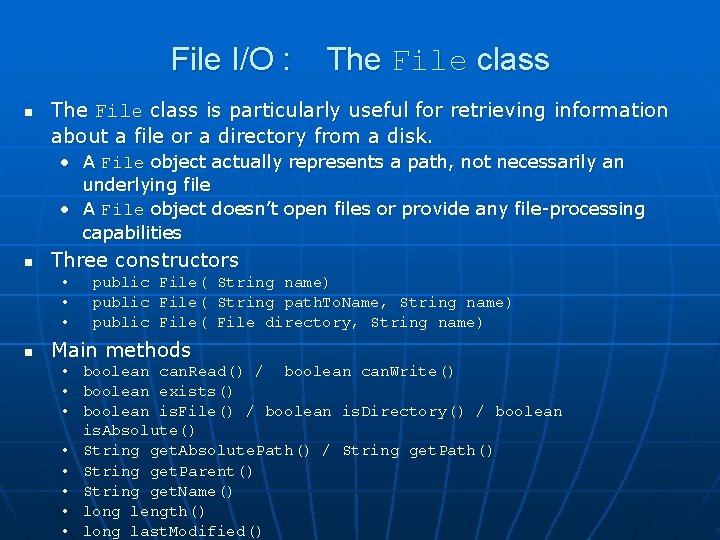
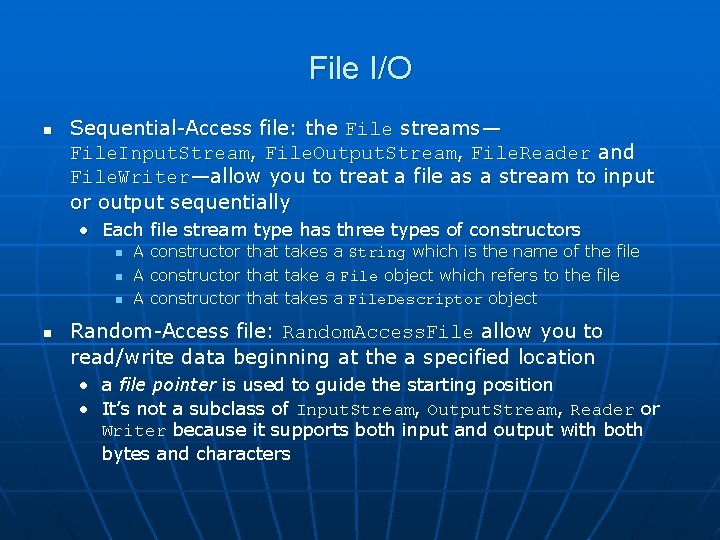
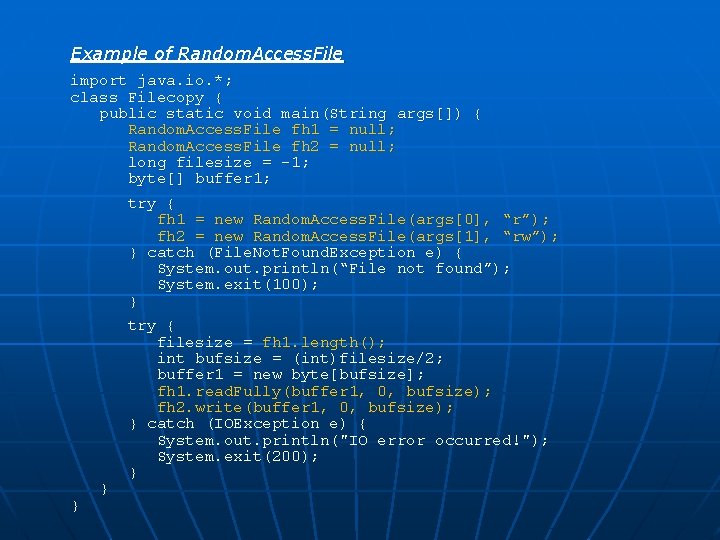
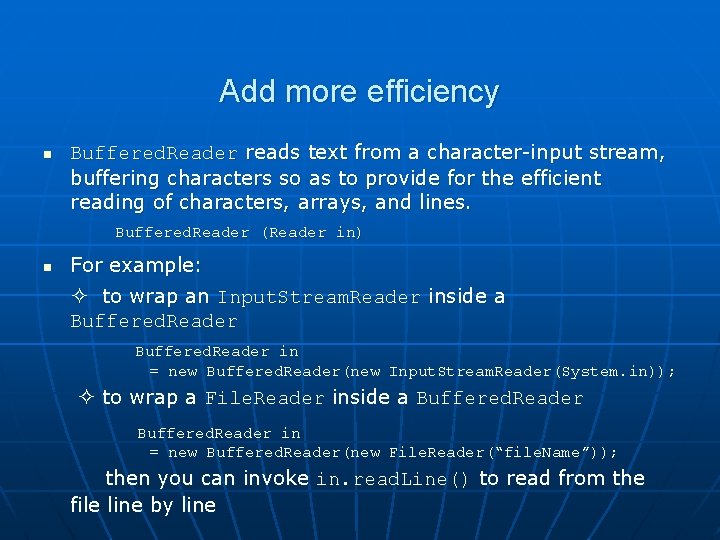
![import java. io. *; public class Efficient. Reader { public static void main (String[] import java. io. *; public class Efficient. Reader { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/0fabfdeb834c28f86d066a85d2cbaede/image-24.jpg)
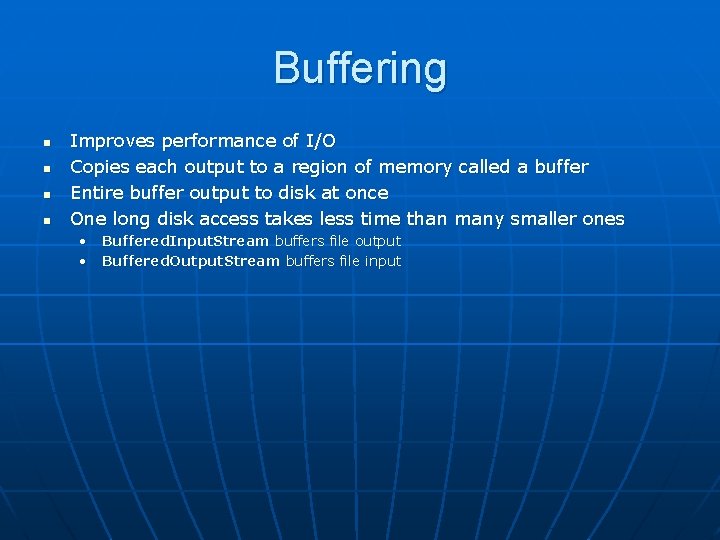
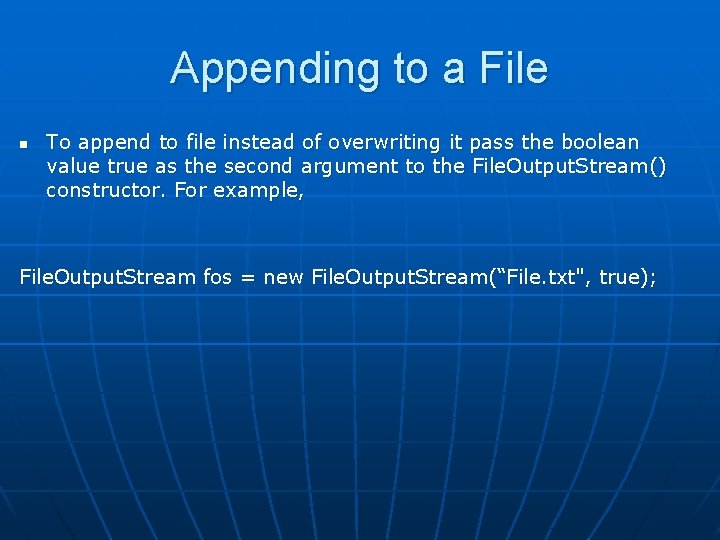
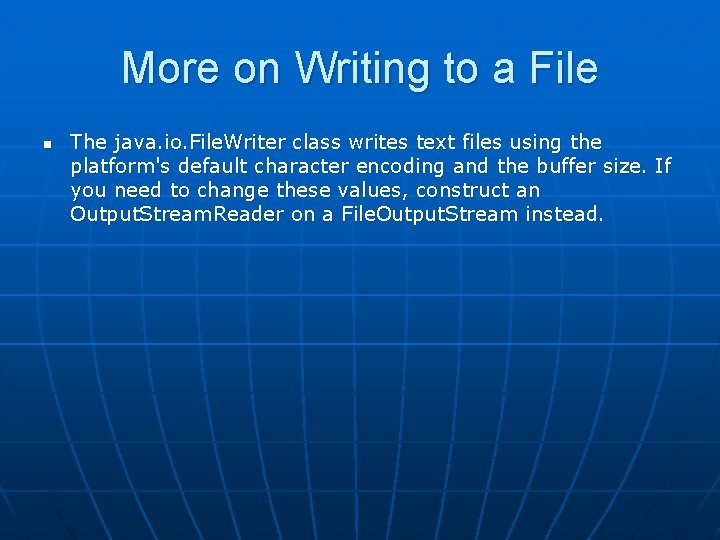
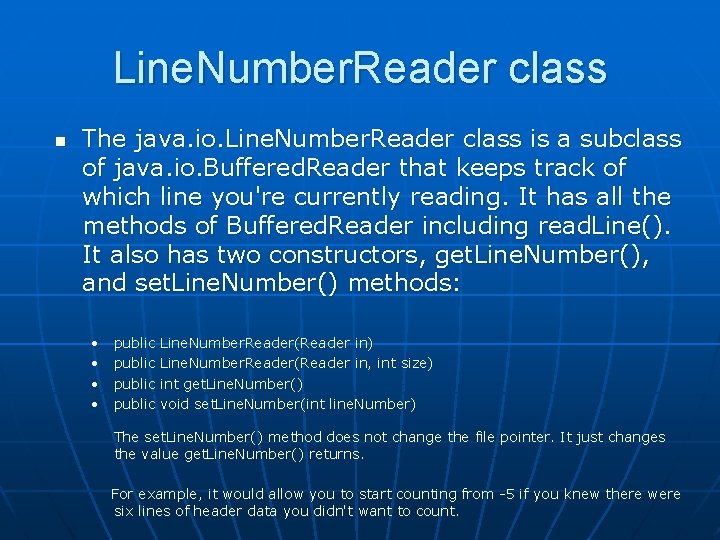
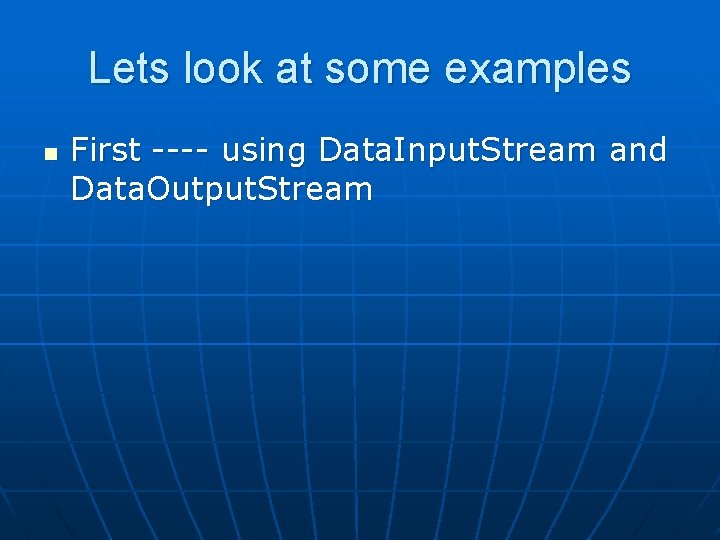
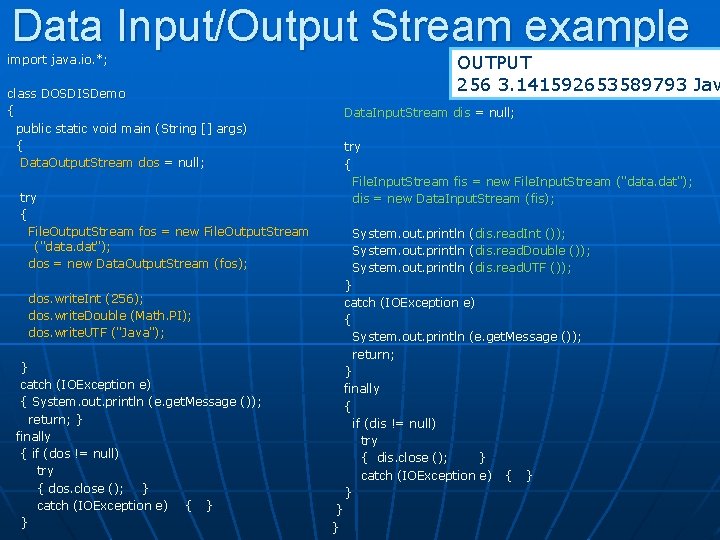
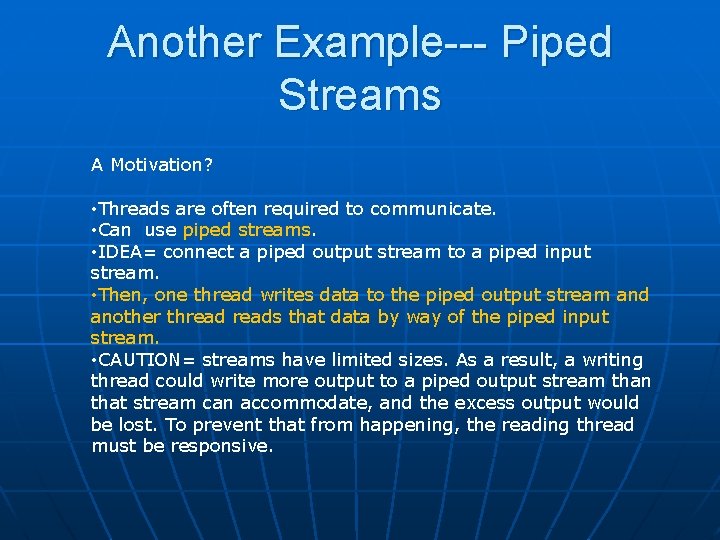
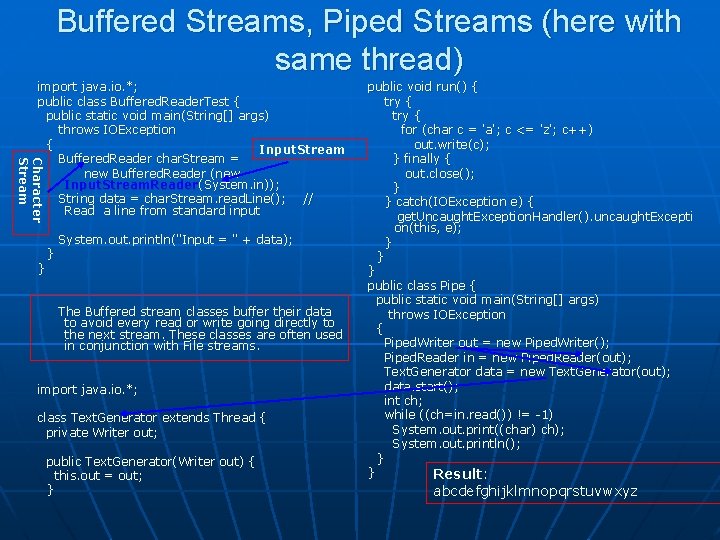
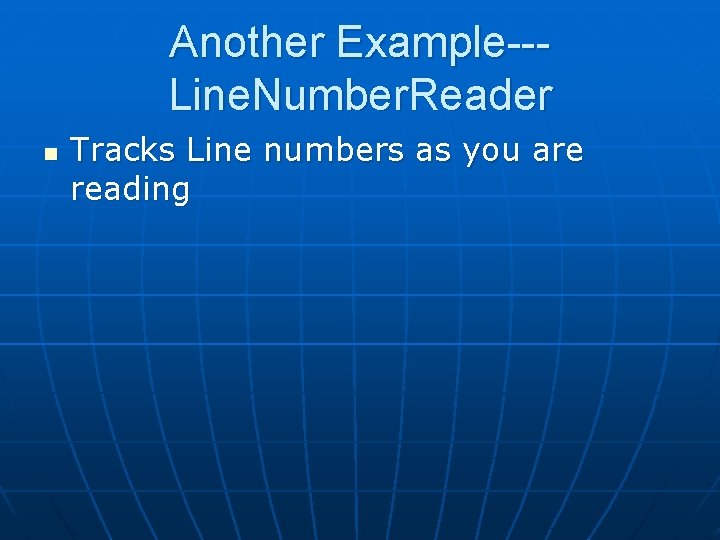
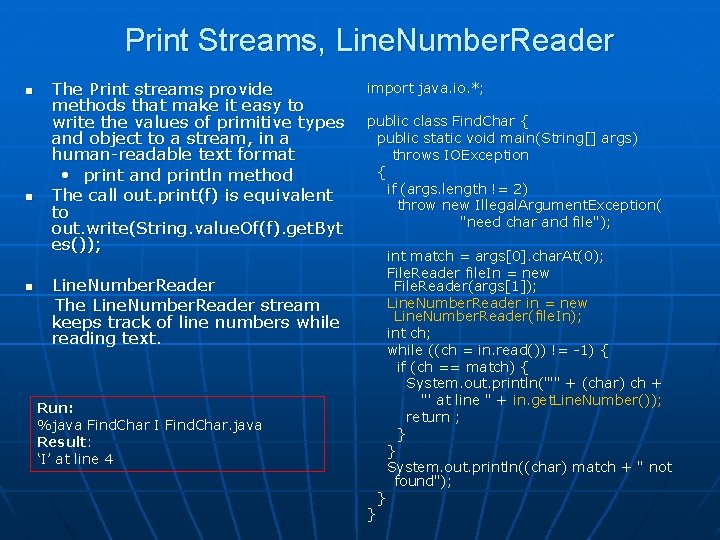
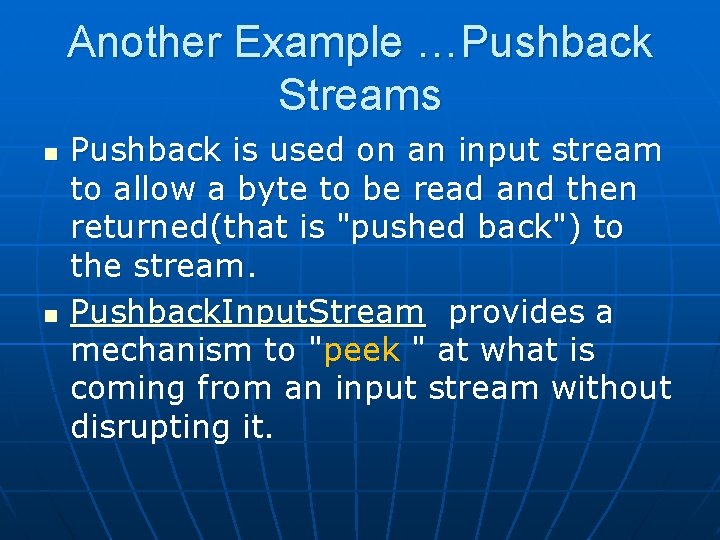
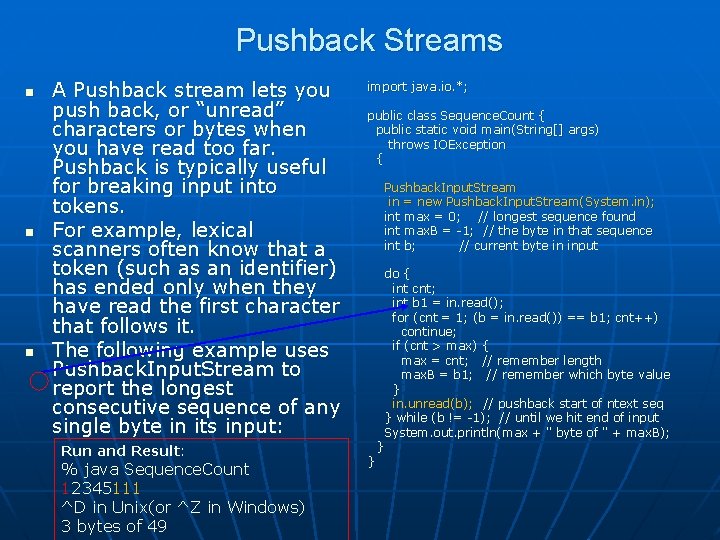
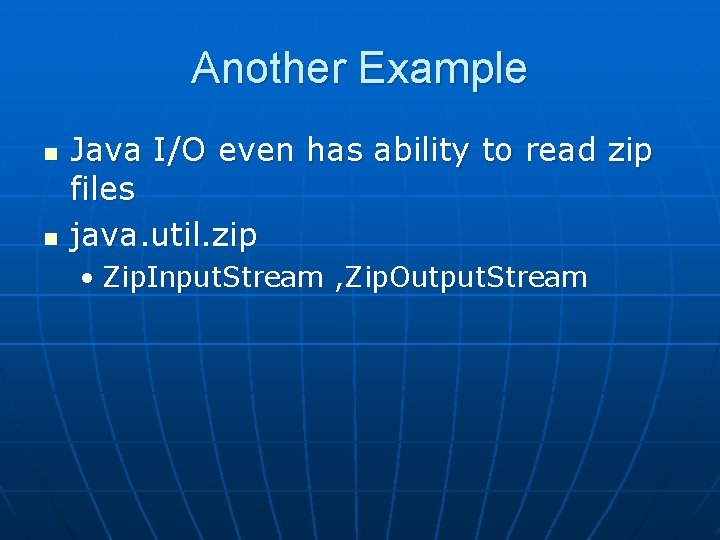
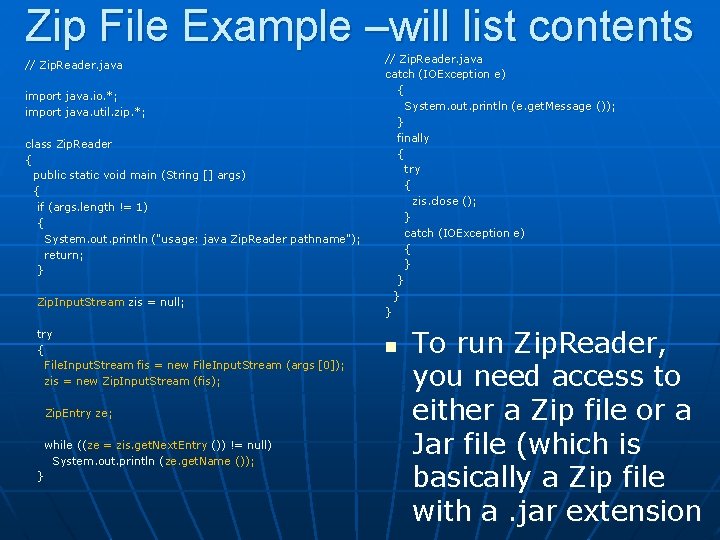
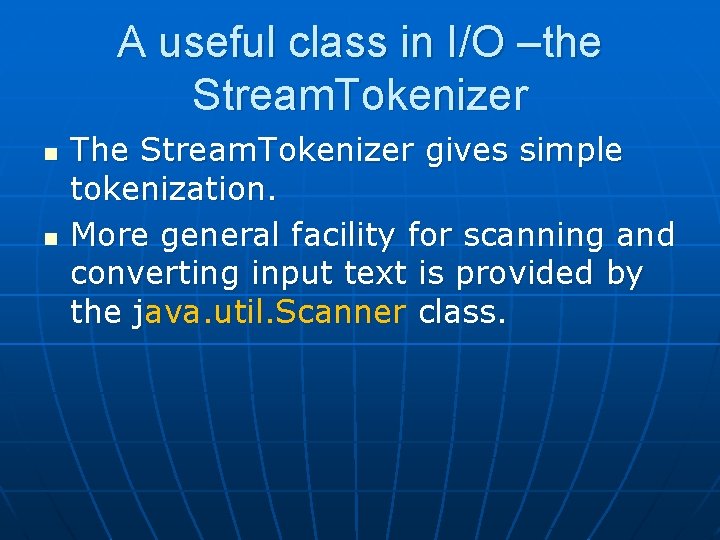
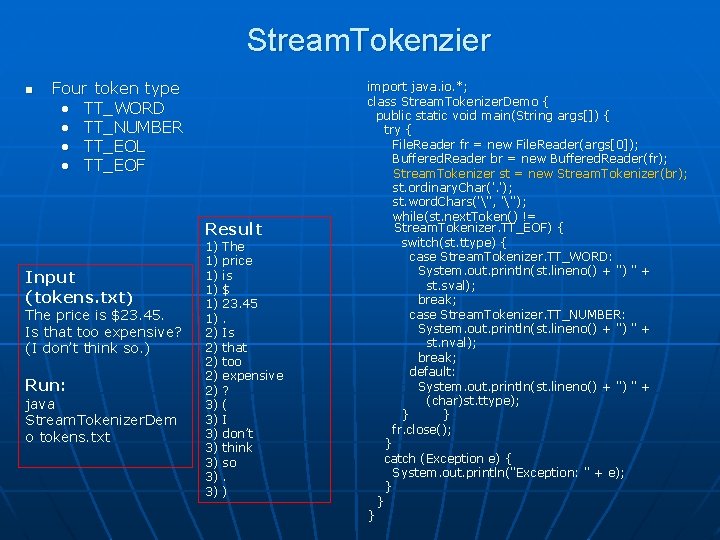
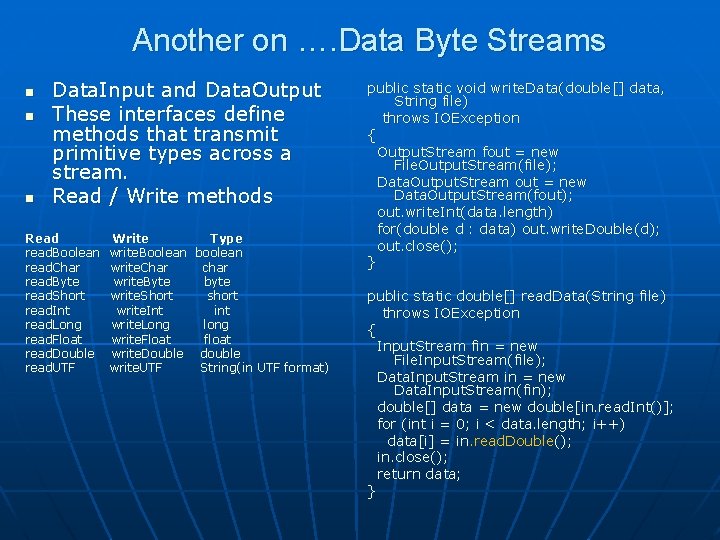
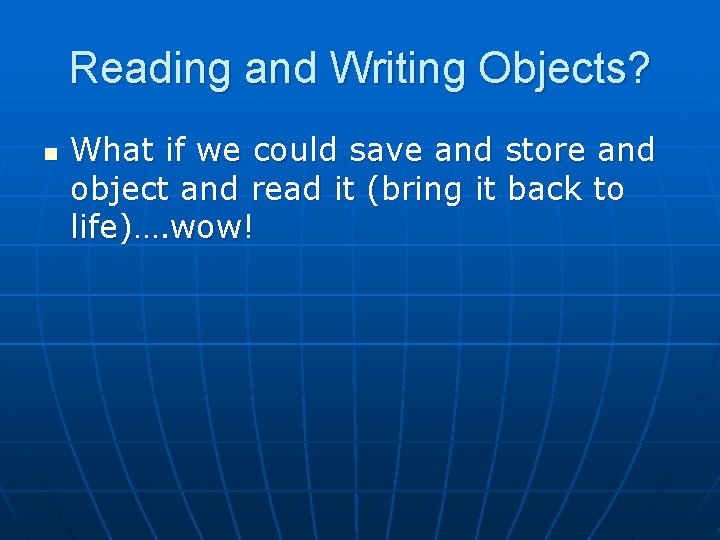
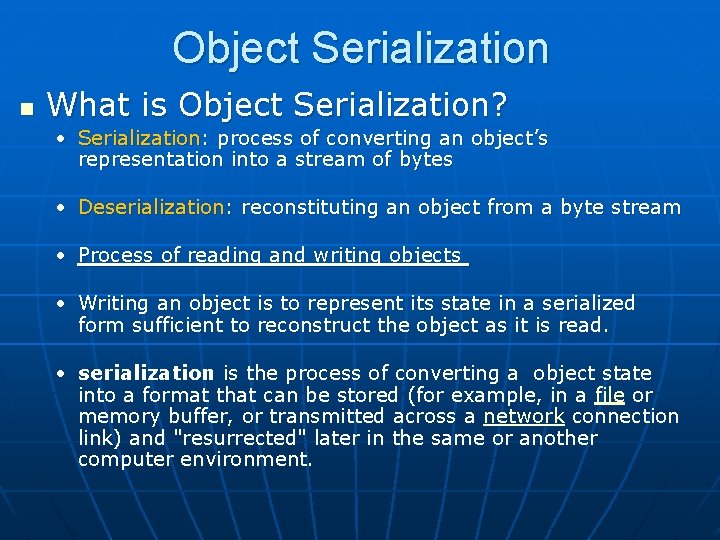
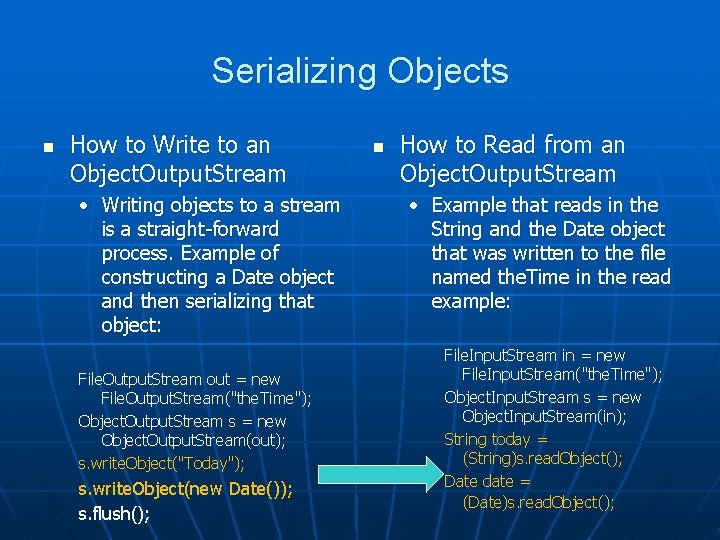
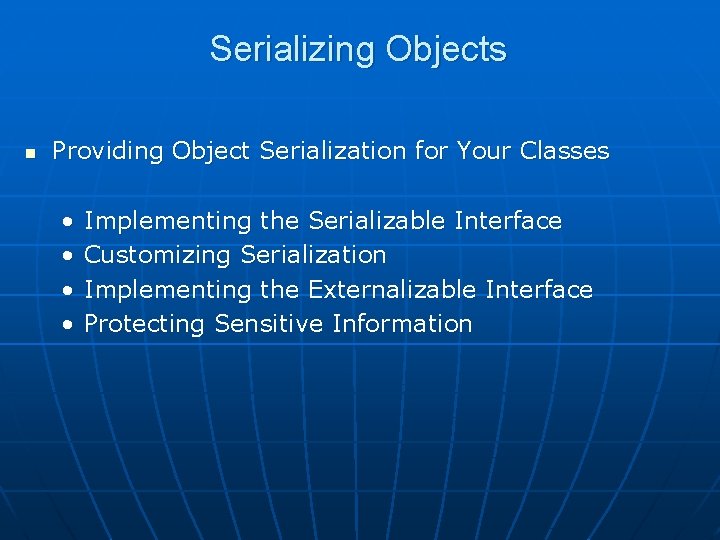
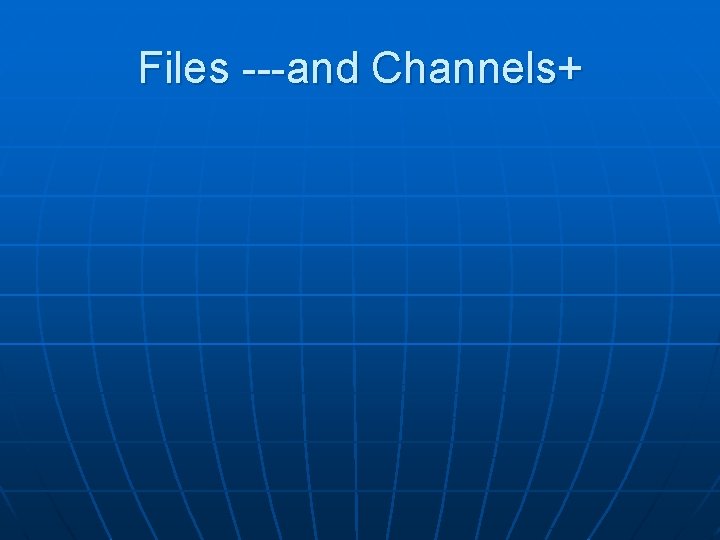
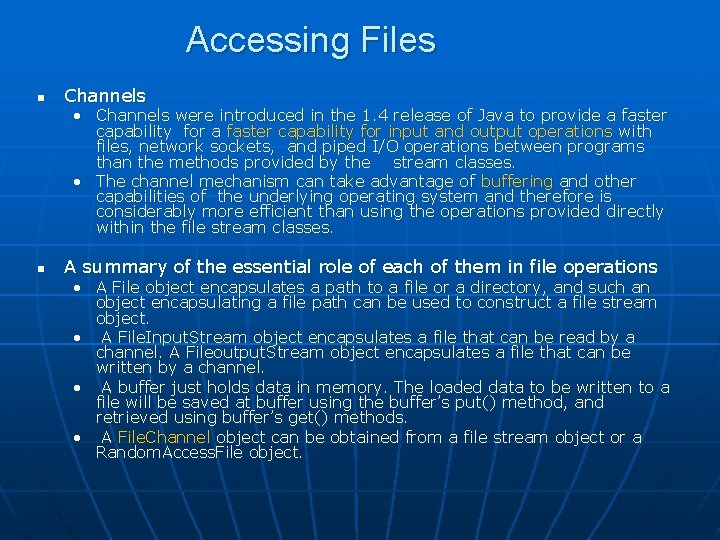
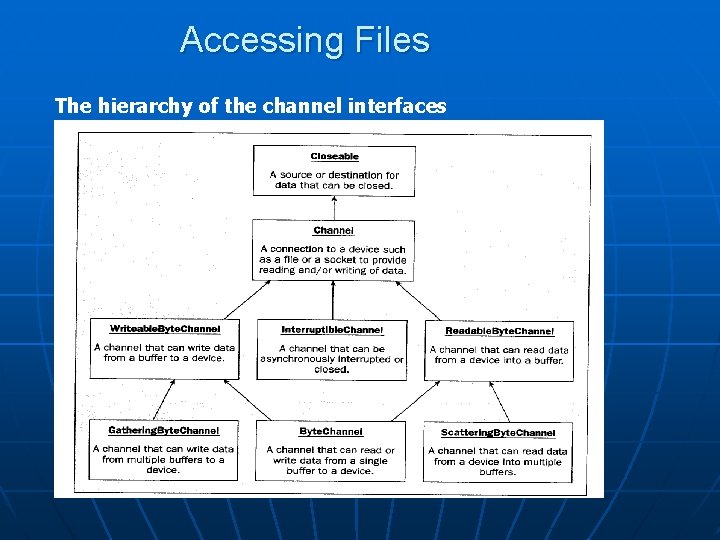
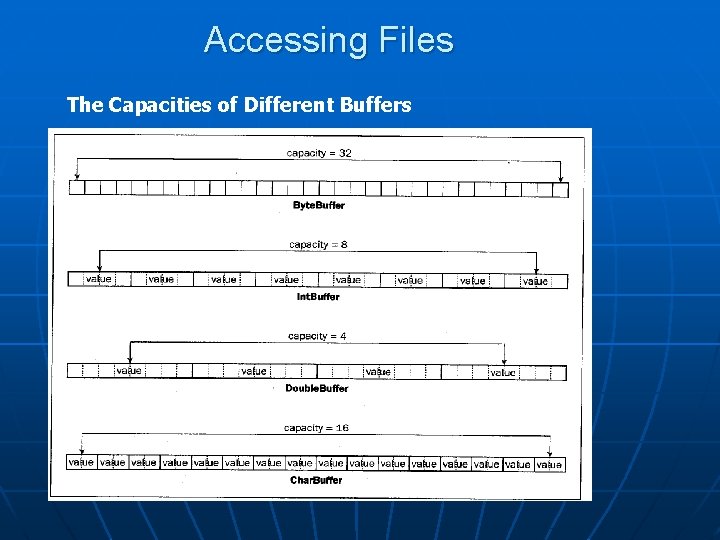
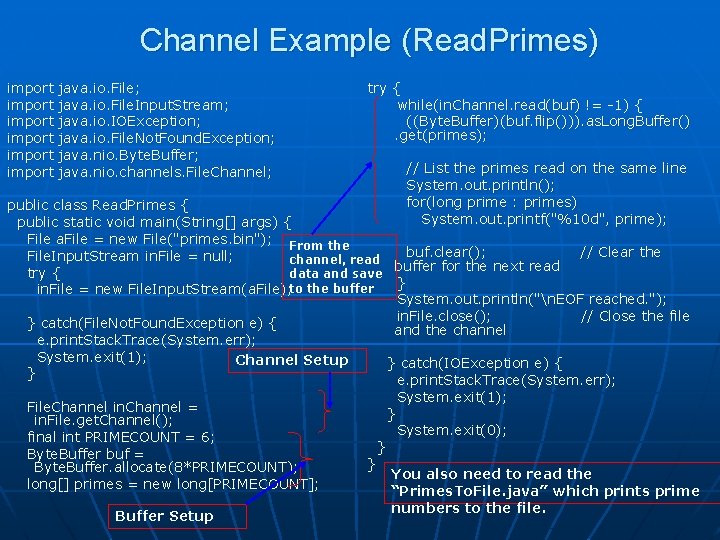
- Slides: 50
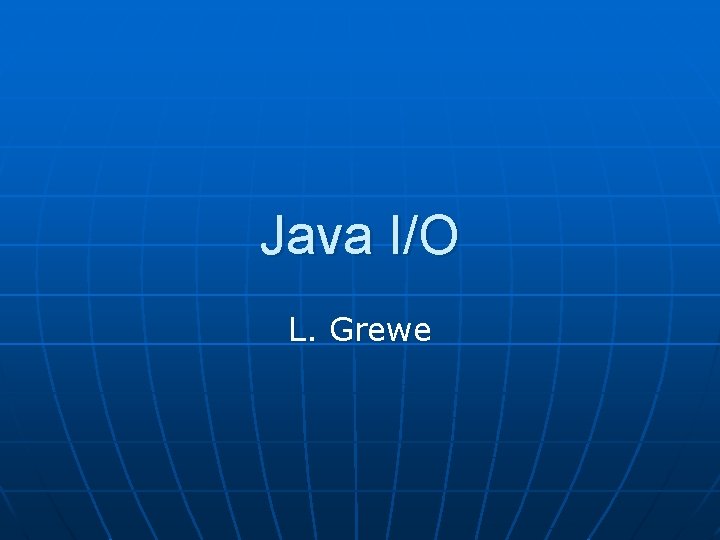
Java I/O L. Grewe
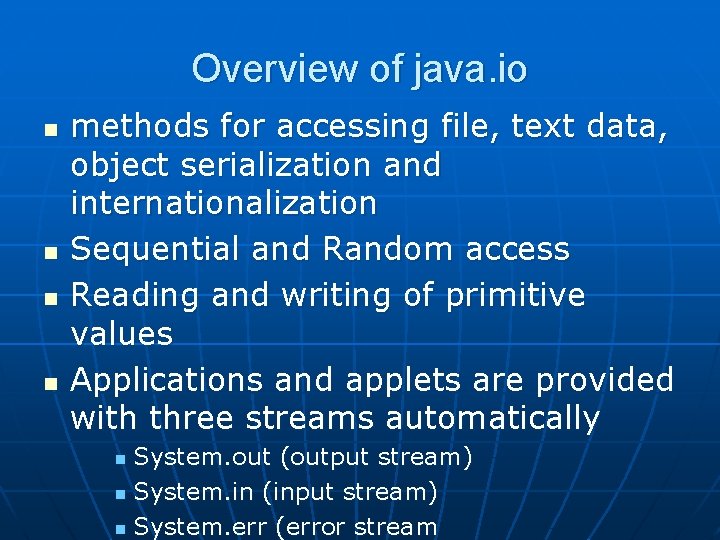
Overview of java. io n n methods for accessing file, text data, object serialization and internationalization Sequential and Random access Reading and writing of primitive values Applications and applets are provided with three streams automatically System. out (output stream) n System. in (input stream) n System. err (error stream n
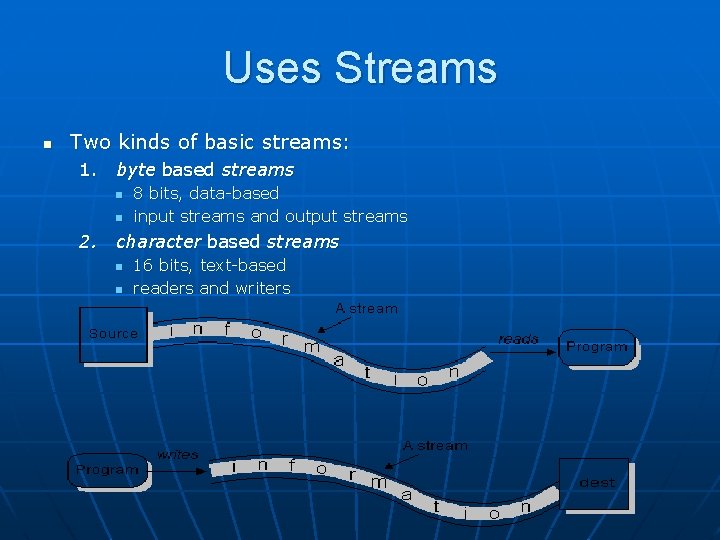
Uses Streams n Two kinds of basic streams: 1. byte based streams n n 2. 8 bits, data-based input streams and output streams character based streams n n 16 bits, text-based readers and writers
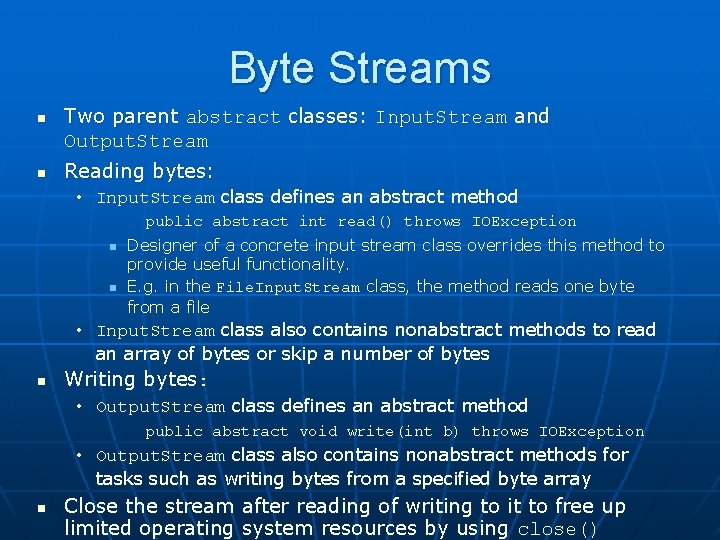
Byte Streams n n Two parent abstract classes: Input. Stream and Output. Stream Reading bytes: • Input. Stream class defines an abstract method public abstract int read() throws IOException n Designer of a concrete input stream class overrides this method to provide useful functionality. E. g. in the File. Input. Stream class, the method reads one byte from a file • Input. Stream class also contains nonabstract methods to read an array of bytes or skip a number of bytes Writing bytes: • Output. Stream class defines an abstract method public abstract void write(int b) throws IOException • Output. Stream class also contains nonabstract methods for tasks such as writing bytes from a specified byte array n Close the stream after reading of writing to it to free up limited operating system resources by using close()
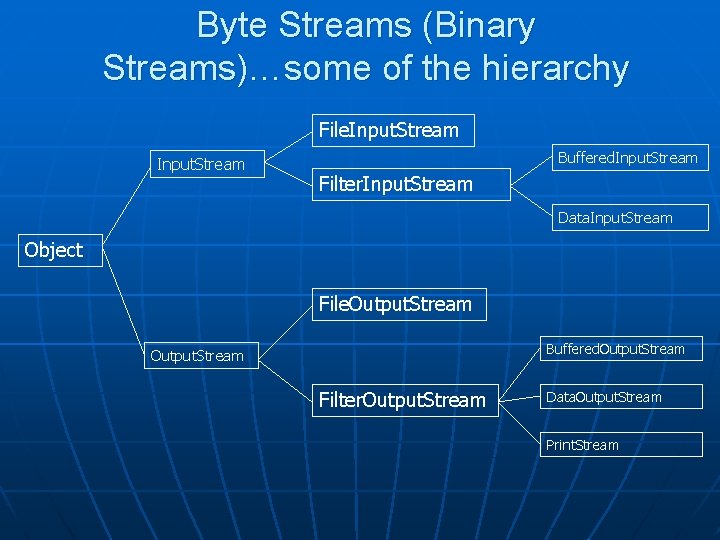
Byte Streams (Binary Streams)…some of the hierarchy File. Input. Stream Buffered. Input. Stream Filter. Input. Stream Data. Input. Stream Object File. Output. Stream Buffered. Output. Stream Filter. Output. Stream Data. Output. Stream Print. Stream
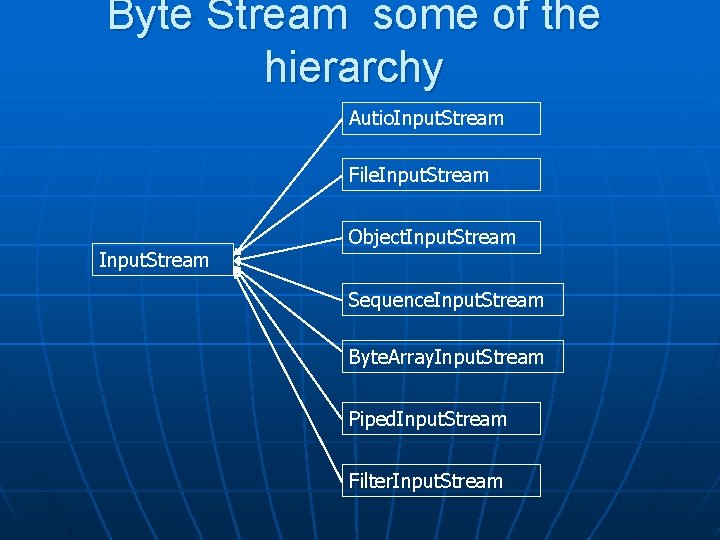
Byte Stream some of the hierarchy Autio. Input. Stream File. Input. Stream Object. Input. Stream Sequence. Input. Stream Byte. Array. Input. Stream Piped. Input. Stream Filter. Input. Stream
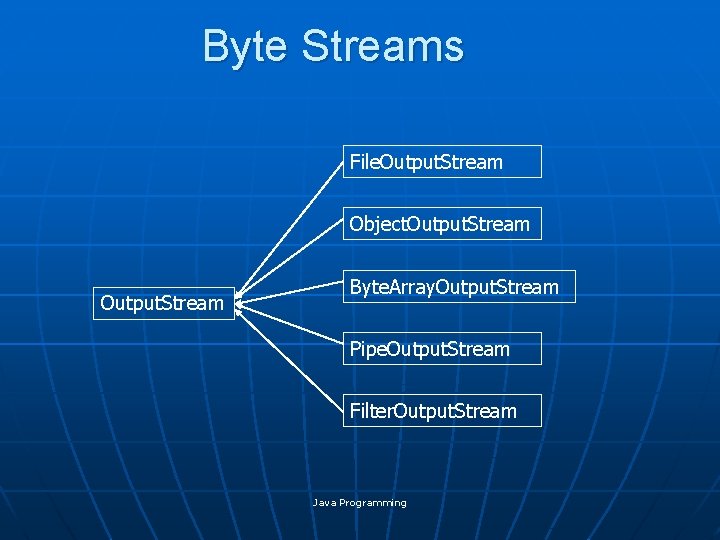
Byte Streams File. Output. Stream Object. Output. Stream Byte. Array. Output. Stream Pipe. Output. Stream Filter. Output. Stream Java Programming
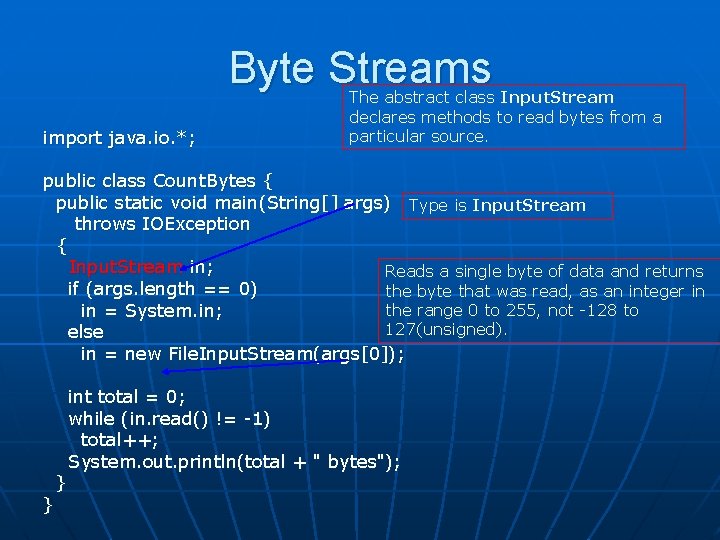
Byte Streams import java. io. *; The abstract class Input. Stream declares methods to read bytes from a particular source. public class Count. Bytes { public static void main(String[] args) Type is Input. Stream throws IOException { Input. Stream in; Reads a single byte of data and returns if (args. length == 0) the byte that was read, as an integer in the range 0 to 255, not -128 to in = System. in; 127(unsigned). else in = new File. Input. Stream(args[0]); } } int total = 0; while (in. read() != -1) total++; System. out. println(total + " bytes");
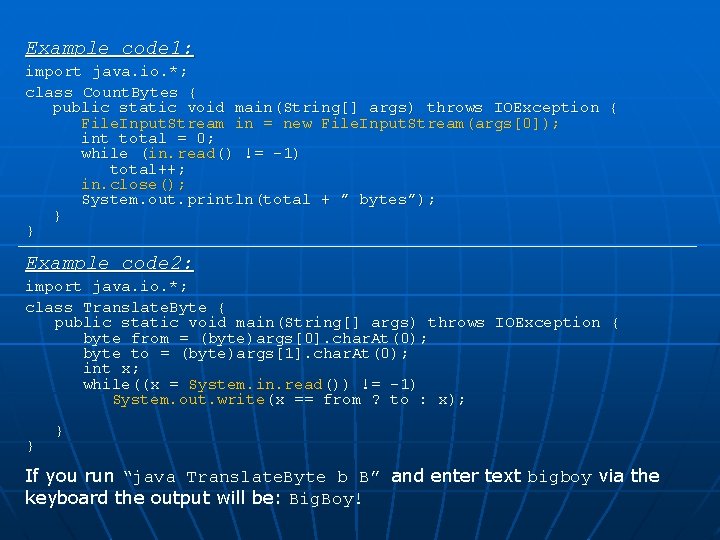
Example code 1: import java. io. *; class Count. Bytes { public static void main(String[] args) throws IOException { File. Input. Stream in = new File. Input. Stream(args[0]); int total = 0; while (in. read() != -1) total++; in. close(); System. out. println(total + ” bytes”); } } Example code 2: import java. io. *; class Translate. Byte { public static void main(String[] args) throws IOException { byte from = (byte)args[0]. char. At(0); byte to = (byte)args[1]. char. At(0); int x; while((x = System. in. read()) != -1) System. out. write(x == from ? to : x); } } If you run “java Translate. Byte b B” and enter text bigboy via the keyboard the output will be: Big. Boy!
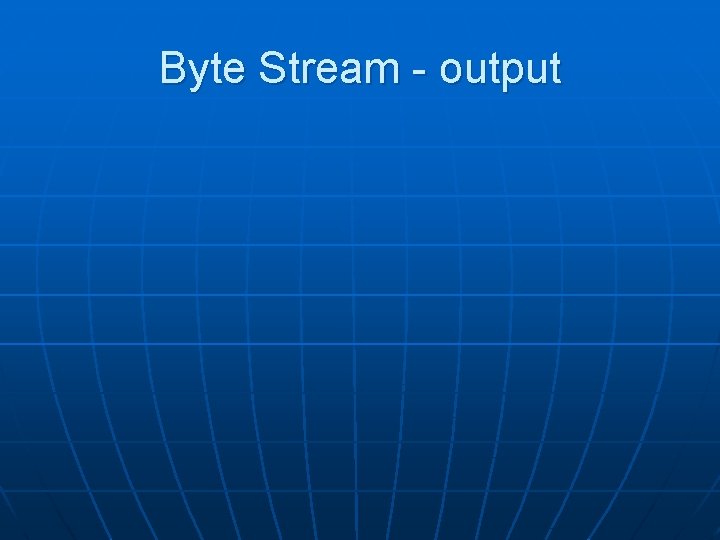
Byte Stream - output
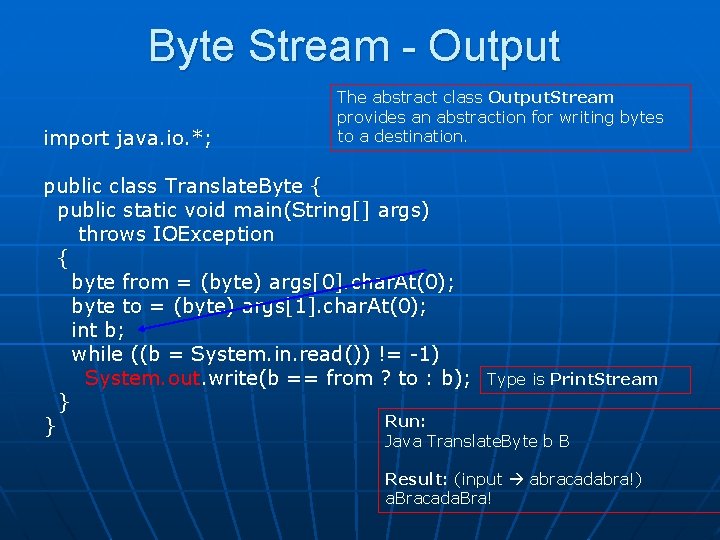
Byte Stream - Output import java. io. *; The abstract class Output. Stream provides an abstraction for writing bytes to a destination. public class Translate. Byte { public static void main(String[] args) throws IOException { byte from = (byte) args[0]. char. At(0); byte to = (byte) args[1]. char. At(0); int b; while ((b = System. in. read()) != -1) System. out. write(b == from ? to : b); } Run: } Type is Print. Stream Java Translate. Byte b B Result: (input abracadabra!) a. Bracada. Bra!
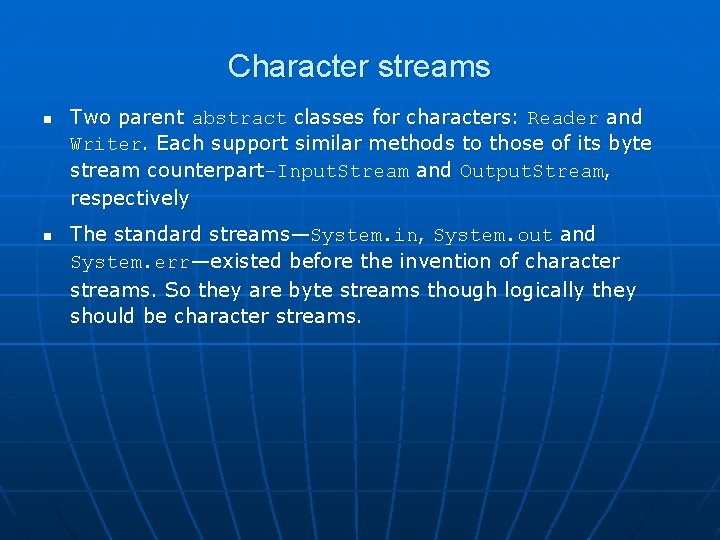
Character streams n n Two parent abstract classes for characters: Reader and Writer. Each support similar methods to those of its byte stream counterpart–Input. Stream and Output. Stream, respectively The standard streams—System. in, System. out and System. err—existed before the invention of character streams. So they are byte streams though logically they should be character streams.
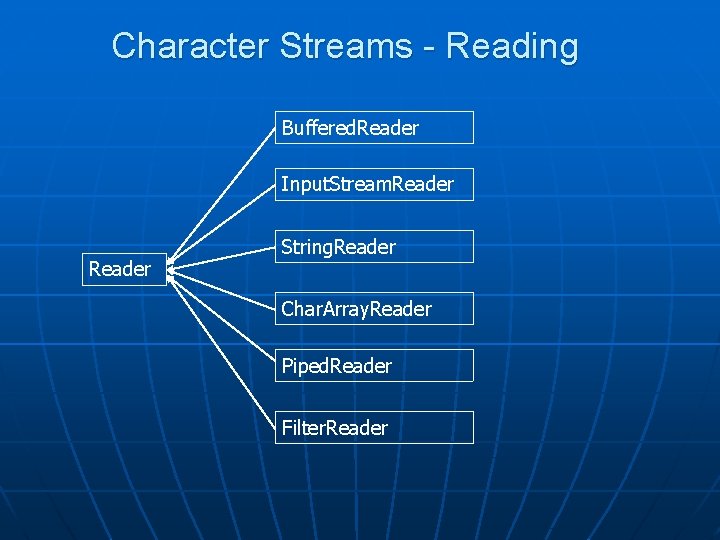
Character Streams - Reading Buffered. Reader Input. Stream. Reader String. Reader Char. Array. Reader Piped. Reader Filter. Reader
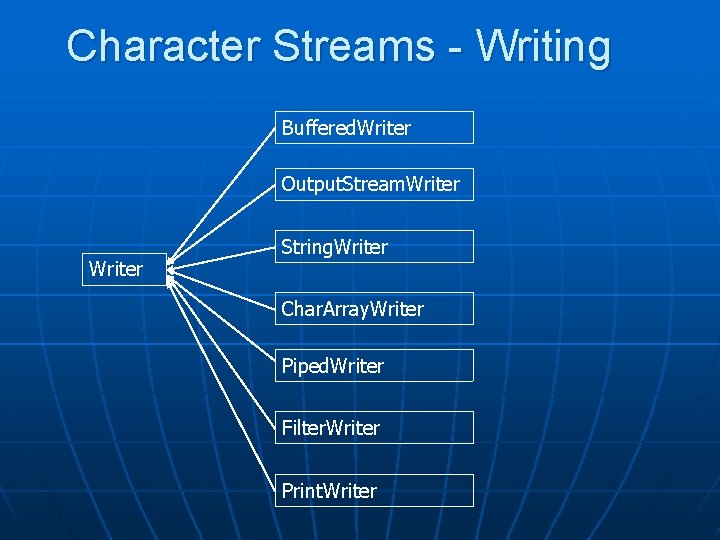
Character Streams - Writing Buffered. Writer Output. Stream. Writer String. Writer Char. Array. Writer Piped. Writer Filter. Writer Print. Writer
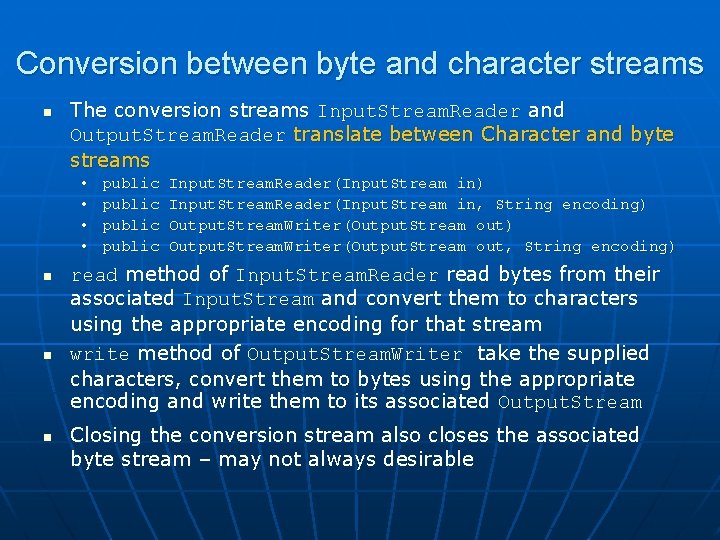
Conversion between byte and character streams n The conversion streams Input. Stream. Reader and Output. Stream. Reader translate between Character and byte streams • • n n n public Input. Stream. Reader(Input. Stream in) Input. Stream. Reader(Input. Stream in, String encoding) Output. Stream. Writer(Output. Stream out, String encoding) read method of Input. Stream. Reader read bytes from their associated Input. Stream and convert them to characters using the appropriate encoding for that stream write method of Output. Stream. Writer take the supplied characters, convert them to bytes using the appropriate encoding and write them to its associated Output. Stream Closing the conversion stream also closes the associated byte stream – may not always desirable
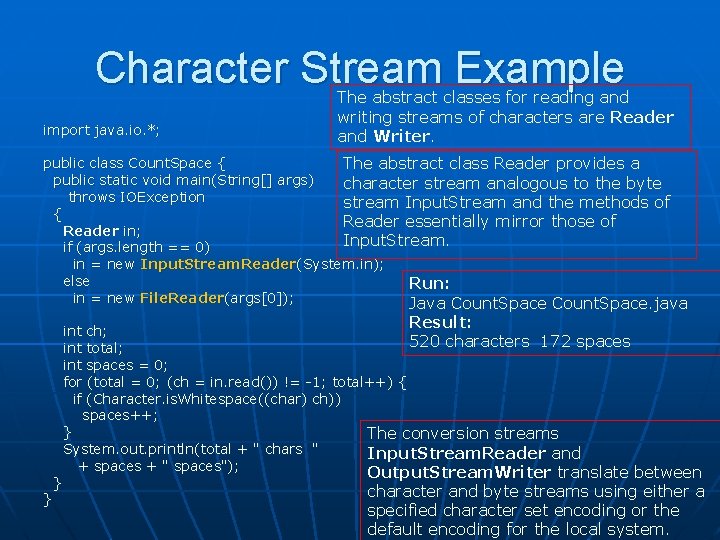
Character Stream Example import java. io. *; The abstract classes for reading and writing streams of characters are Reader and Writer. public class Count. Space { The abstract class Reader provides a public static void main(String[] args) character stream analogous to the byte throws IOException stream Input. Stream and the methods of { Reader essentially mirror those of Reader in; Input. Stream. if (args. length == 0) in = new Input. Stream. Reader(System. in); else Run: in = new File. Reader(args[0]); Java Count. Space. java Result: } } int ch; 520 characters 172 spaces int total; int spaces = 0; for (total = 0; (ch = in. read()) != -1; total++) { if (Character. is. Whitespace((char) ch)) spaces++; } The conversion streams System. out. println(total + " chars " Input. Stream. Reader and + spaces + " spaces"); Output. Stream. Writer translate between character and byte streams using either a specified character set encoding or the default encoding for the local system.
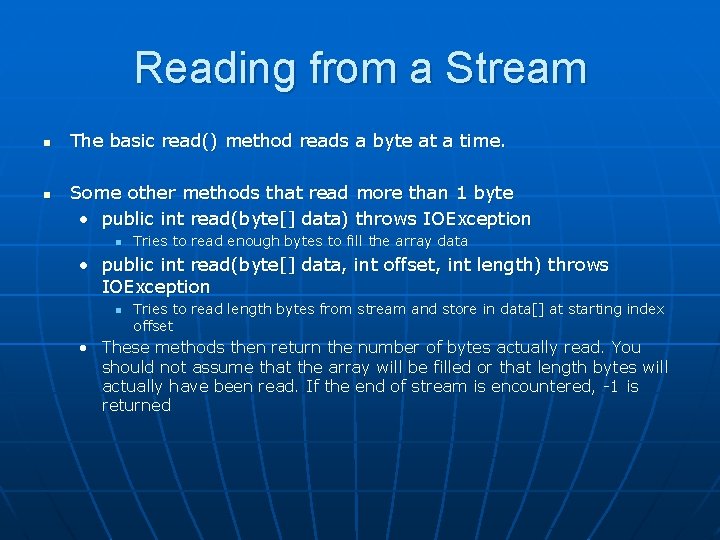
Reading from a Stream n n The basic read() method reads a byte at a time. Some other methods that read more than 1 byte • public int read(byte[] data) throws IOException n Tries to read enough bytes to fill the array data • public int read(byte[] data, int offset, int length) throws IOException n Tries to read length bytes from stream and store in data[] at starting index offset • These methods then return the number of bytes actually read. You should not assume that the array will be filled or that length bytes will actually have been read. If the end of stream is encountered, -1 is returned
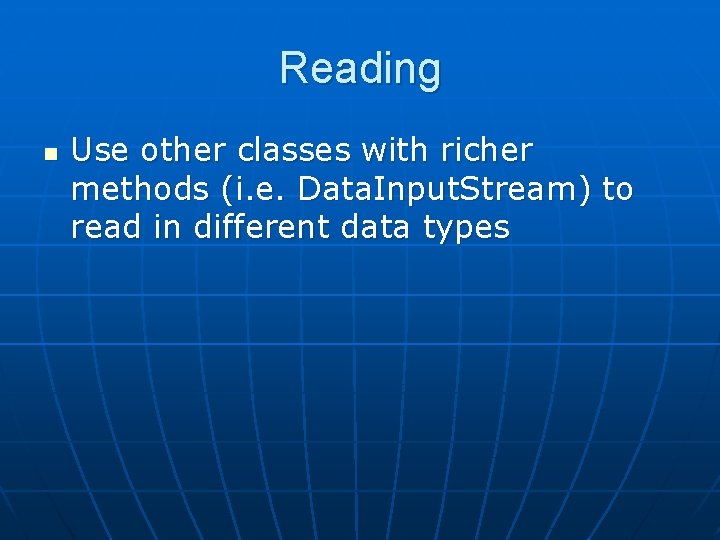
Reading n Use other classes with richer methods (i. e. Data. Input. Stream) to read in different data types
![Writing n n n abstract void writechar cbuf int off int len Write a Writing n n n abstract void write(char[] cbuf, int off, int len) Write a](https://slidetodoc.com/presentation_image_h2/0fabfdeb834c28f86d066a85d2cbaede/image-19.jpg)
Writing n n n abstract void write(char[] cbuf, int off, int len) Write a portion of an array of characters. void write(int c) Write a single character. voidwrite(String str) Write a string. voidwrite(String str, int off, int len) Write a portion of a string. Other methods – for byte based – print*(*) abstract void close() Close the stream, flushing it abstract void flush() Flush the stream. voidwrite(char[] cbuf) Write an array of characters.
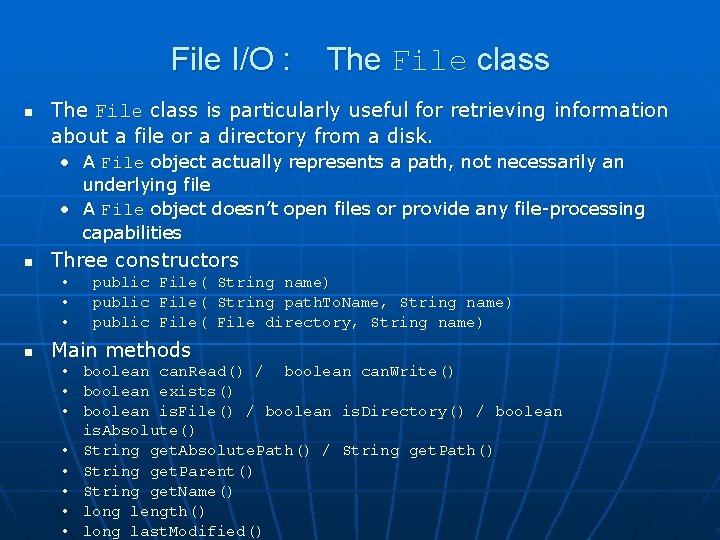
File I/O : n The File class is particularly useful for retrieving information about a file or a directory from a disk. • A File object actually represents a path, not necessarily an underlying file • A File object doesn’t open files or provide any file-processing capabilities n Three constructors • • • n public File( String name) public File( String path. To. Name, String name) public File( File directory, String name) Main methods • • boolean can. Read() / boolean can. Write() boolean exists() boolean is. File() / boolean is. Directory() / boolean is. Absolute() String get. Absolute. Path() / String get. Path() String get. Parent() String get. Name() long length() long last. Modified()
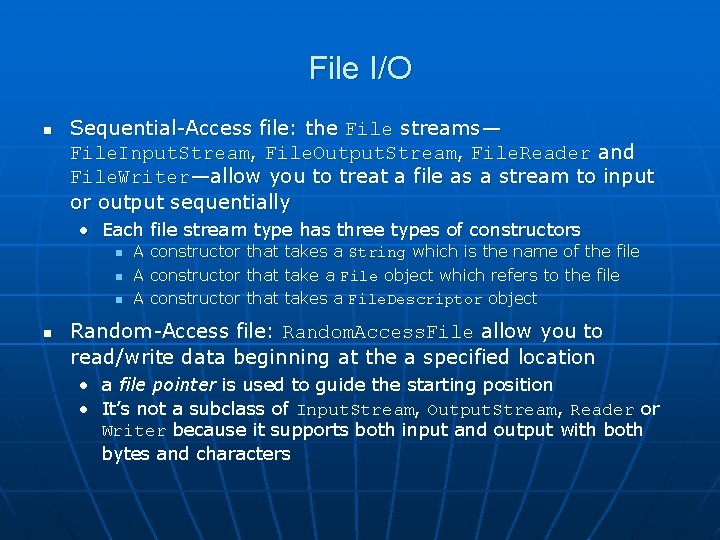
File I/O n Sequential-Access file: the File streams— File. Input. Stream, File. Output. Stream, File. Reader and File. Writer—allow you to treat a file as a stream to input or output sequentially • Each file stream type has three types of constructors n n A A A constructor that takes a String which is the name of the file take a File object which refers to the file takes a File. Descriptor object Random-Access file: Random. Access. File allow you to read/write data beginning at the a specified location • a file pointer is used to guide the starting position • It’s not a subclass of Input. Stream, Output. Stream, Reader or Writer because it supports both input and output with both bytes and characters
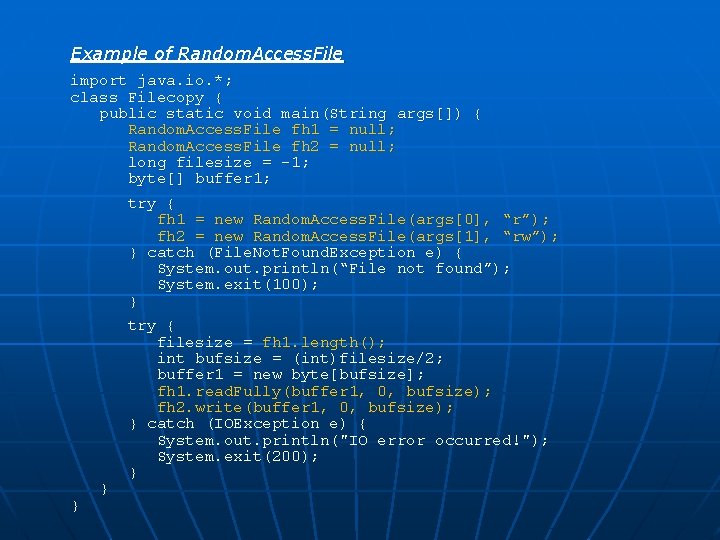
Example of Random. Access. File import java. io. *; class Filecopy { public static void main(String args[]) { Random. Access. File fh 1 = null; Random. Access. File fh 2 = null; long filesize = -1; byte[] buffer 1; try { fh 1 = new Random. Access. File(args[0], “r”); fh 2 = new Random. Access. File(args[1], “rw”); } catch (File. Not. Found. Exception e) { System. out. println(“File not found”); System. exit(100); } try { filesize = fh 1. length(); int bufsize = (int)filesize/2; buffer 1 = new byte[bufsize]; fh 1. read. Fully(buffer 1, 0, bufsize); fh 2. write(buffer 1, 0, bufsize); } catch (IOException e) { System. out. println("IO error occurred!"); System. exit(200); } } }
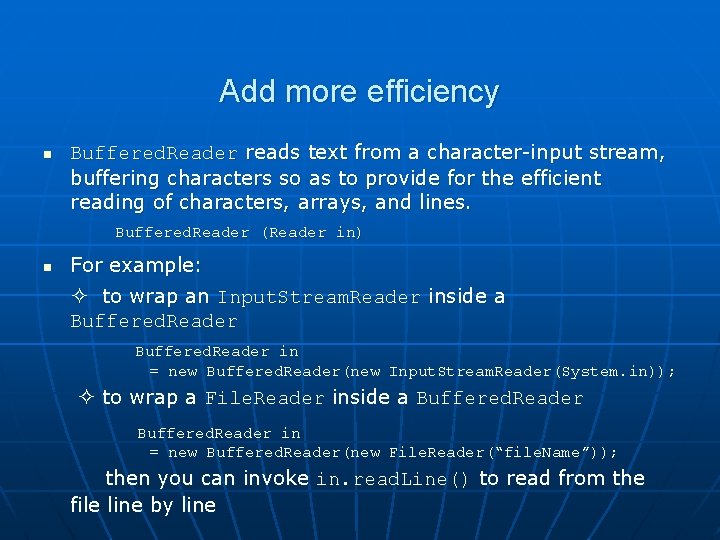
Add more efficiency n Buffered. Reader reads text from a character-input stream, buffering characters so as to provide for the efficient reading of characters, arrays, and lines. Buffered. Reader (Reader in) n For example: to wrap an Input. Stream. Reader inside a Buffered. Reader in = new Buffered. Reader(new Input. Stream. Reader(System. in)); to wrap a File. Reader inside a Buffered. Reader in = new Buffered. Reader(new File. Reader(“file. Name”)); then you can invoke in. read. Line() to read from the file line by line
![import java io public class Efficient Reader public static void main String import java. io. *; public class Efficient. Reader { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/0fabfdeb834c28f86d066a85d2cbaede/image-24.jpg)
import java. io. *; public class Efficient. Reader { public static void main (String[] args) { try { Buffered. Reader br = new Buffered. Reader(new File. Reader(args[0])); // get line String line = br. read. Line(); } } // while not end of file… keep reading and displaying lines while (line != null) { System. out. println("Read a line: "); System. out. println(line); line = br. read. Line(); } // close stream br. close(); } catch(File. Not. Found. Exception fe) { System. out. println("File not found: “+ args[0]"); } catch(IOException ioe) { System. out. println("Can’t read from file: “+args[0]); }
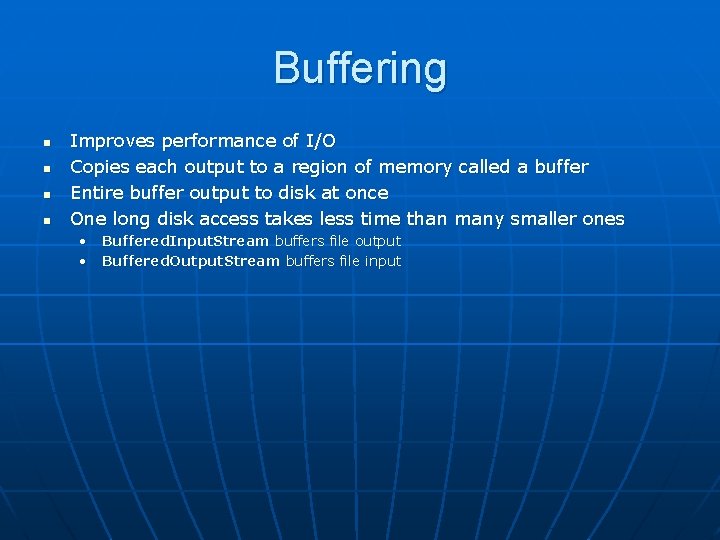
Buffering n n Improves performance of I/O Copies each output to a region of memory called a buffer Entire buffer output to disk at once One long disk access takes less time than many smaller ones • Buffered. Input. Stream buffers file output • Buffered. Output. Stream buffers file input
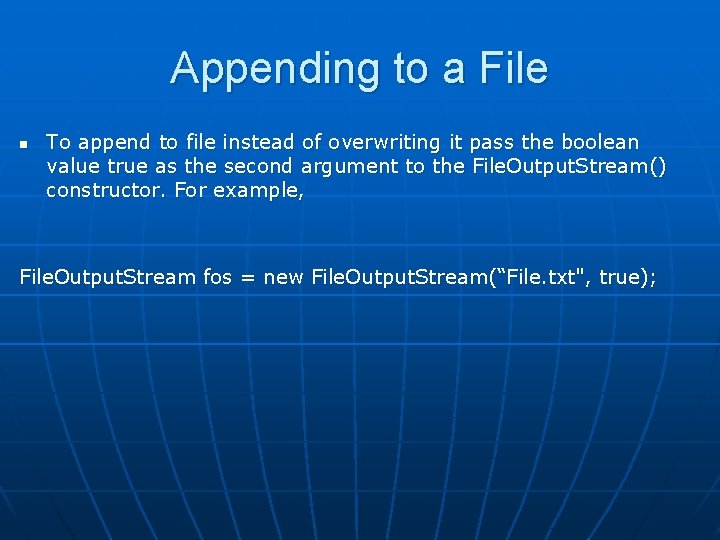
Appending to a File n To append to file instead of overwriting it pass the boolean value true as the second argument to the File. Output. Stream() constructor. For example, File. Output. Stream fos = new File. Output. Stream(“File. txt", true);
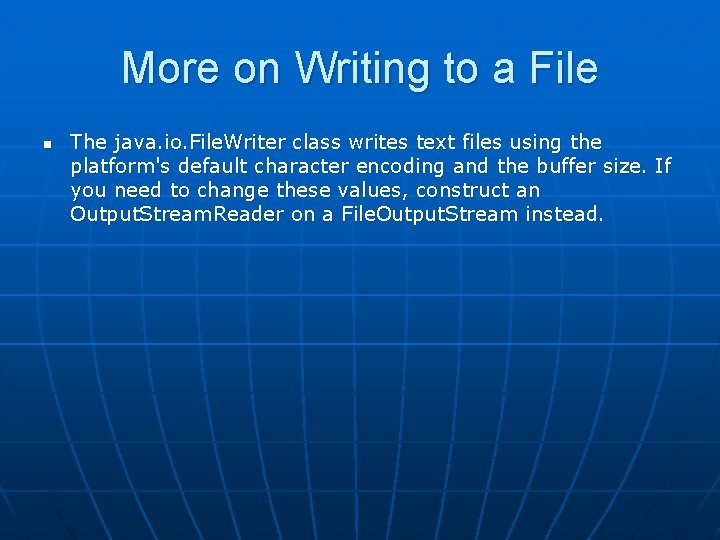
More on Writing to a File n The java. io. File. Writer class writes text files using the platform's default character encoding and the buffer size. If you need to change these values, construct an Output. Stream. Reader on a File. Output. Stream instead.
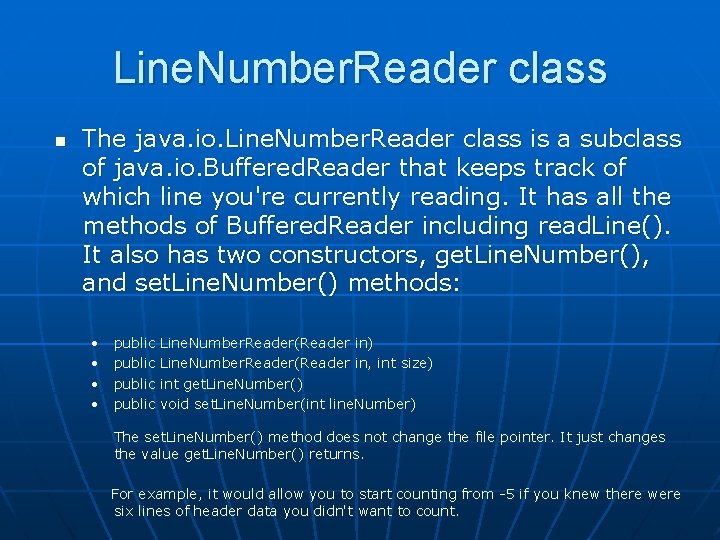
Line. Number. Reader class n The java. io. Line. Number. Reader class is a subclass of java. io. Buffered. Reader that keeps track of which line you're currently reading. It has all the methods of Buffered. Reader including read. Line(). It also has two constructors, get. Line. Number(), and set. Line. Number() methods: • • public Line. Number. Reader(Reader in) Line. Number. Reader(Reader in, int size) int get. Line. Number() void set. Line. Number(int line. Number) The set. Line. Number() method does not change the file pointer. It just changes the value get. Line. Number() returns. For example, it would allow you to start counting from -5 if you knew there were six lines of header data you didn't want to count.
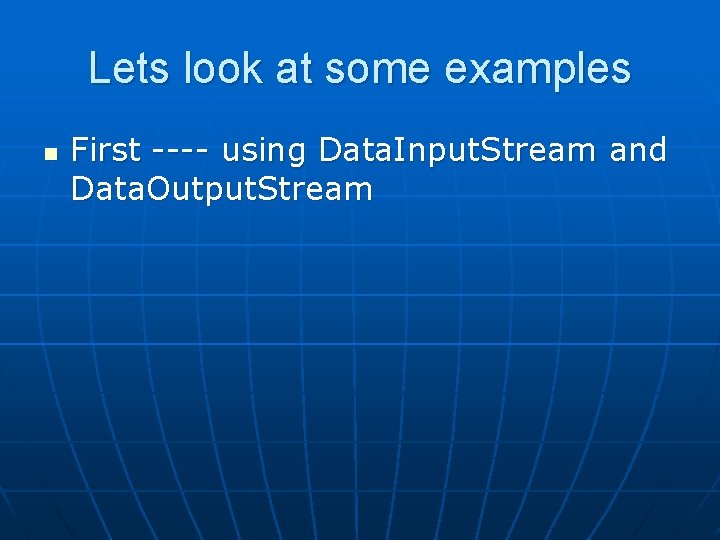
Lets look at some examples n First ---- using Data. Input. Stream and Data. Output. Stream
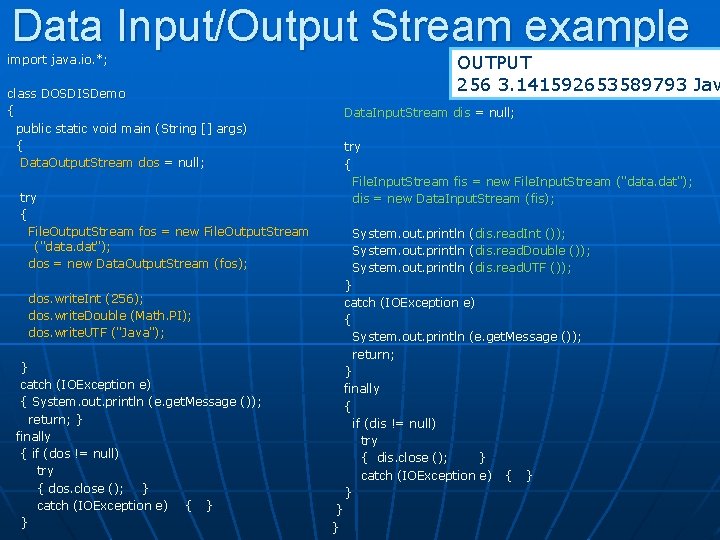
Data Input/Output Stream example OUTPUT 256 3. 141592653589793 Jav import java. io. *; class DOSDISDemo { public static void main (String [] args) { Data. Output. Stream dos = null; Data. Input. Stream dis = null; try { File. Input. Stream fis = new File. Input. Stream ("data. dat"); dis = new Data. Input. Stream (fis); try { File. Output. Stream fos = new File. Output. Stream ("data. dat"); dos = new Data. Output. Stream (fos); System. out. println ( dis. read. Int ()); System. out. println ( dis. read. Double ()); System. out. println ( dis. read. UTF ()); } catch (IOException e) { System. out. println (e. get. Message ()); return; } finally { if (dis != null) try { dis. close (); } catch (IOException e) { } } dos. write. Int (256); dos. write. Double (Math. PI); dos. write. UTF ("Java"); } catch (IOException e) { System. out. println (e. get. Message ()); return; } finally { if (dos != null) try { dos. close (); } catch (IOException e) { } }
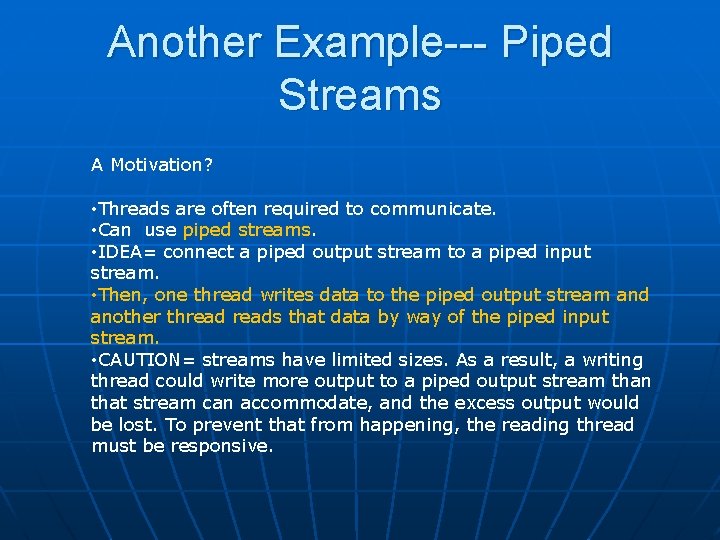
Another Example--- Piped Streams A Motivation? • Threads are often required to communicate. • Can use piped streams. • IDEA= connect a piped output stream to a piped input stream. • Then, one thread writes data to the piped output stream and another threads that data by way of the piped input stream. • CAUTION= streams have limited sizes. As a result, a writing thread could write more output to a piped output stream than that stream can accommodate, and the excess output would be lost. To prevent that from happening, the reading thread must be responsive.
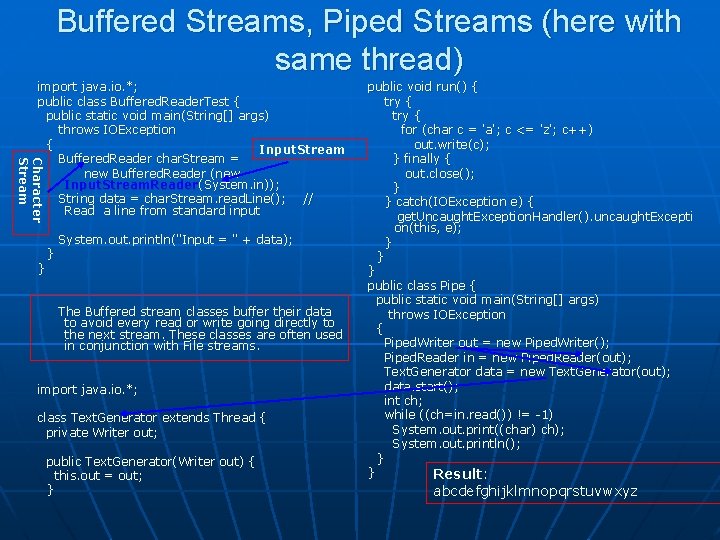
Buffered Streams, Piped Streams (here with same thread) Character Stream import java. io. *; public class Buffered. Reader. Test { public static void main(String[] args) throws IOException { Input. Stream Buffered. Reader char. Stream = new Buffered. Reader (new Input. Stream. Reader(System. in)); String data = char. Stream. read. Line(); // Read a line from standard input } } System. out. println("Input = " + data); The Buffered stream classes buffer their data to avoid every read or write going directly to the next stream. These classes are often used in conjunction with File streams. import java. io. *; class Text. Generator extends Thread { private Writer out; public Text. Generator(Writer out) { this. out = out; } public void run() { try { for (char c = 'a'; c <= 'z'; c++) out. write(c); } finally { out. close(); } } catch(IOException e) { get. Uncaught. Exception. Handler(). uncaught. Excepti on(this, e); } } } public class Pipe { public static void main(String[] args) throws IOException { Piped. Writer out = new Piped. Writer(); Piped. Reader in = new Piped. Reader(out); Text. Generator data = new Text. Generator(out); data. start(); int ch; while ((ch=in. read()) != -1) System. out. print((char) ch); System. out. println(); } } Result: abcdefghijklmnopqrstuvwxyz
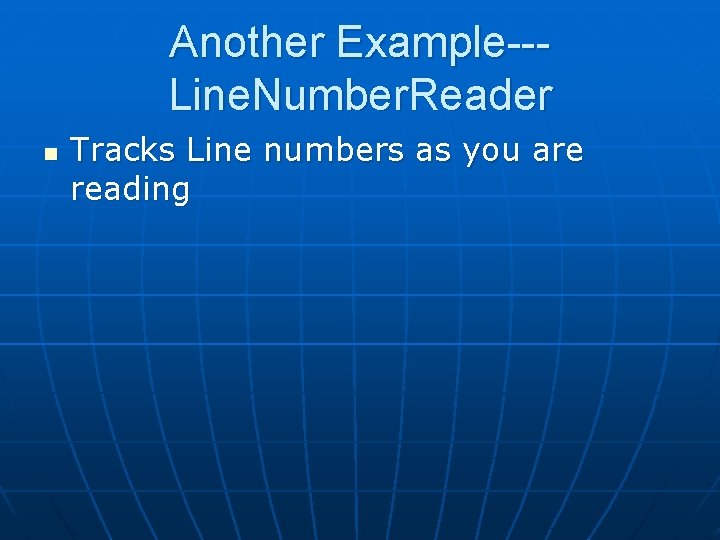
Another Example--Line. Number. Reader n Tracks Line numbers as you are reading
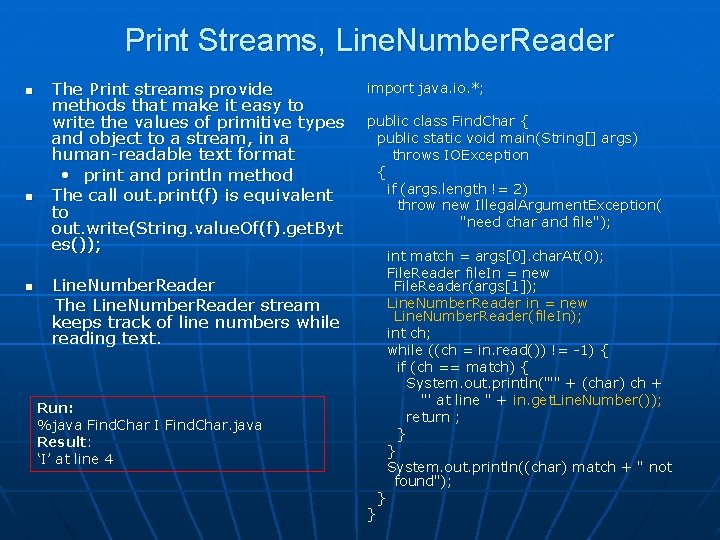
Print Streams, Line. Number. Reader n n n The Print streams provide methods that make it easy to write the values of primitive types and object to a stream, in a human-readable text format • print and println method The call out. print(f) is equivalent to out. write(String. value. Of(f). get. Byt es()); import java. io. *; public class Find. Char { public static void main(String[] args) throws IOException { if (args. length != 2) throw new Illegal. Argument. Exception( "need char and file"); Line. Number. Reader The Line. Number. Reader stream keeps track of line numbers while reading text. Run: %java Find. Char I Find. Char. java Result: ‘I’ at line 4 } } int match = args[0]. char. At(0); File. Reader file. In = new File. Reader(args[1]); Line. Number. Reader in = new Line. Number. Reader(file. In); int ch; while ((ch = in. read()) != -1) { if (ch == match) { System. out. println("'" + (char) ch + "' at line " + in. get. Line. Number()); return ; } } System. out. println((char) match + " not found");
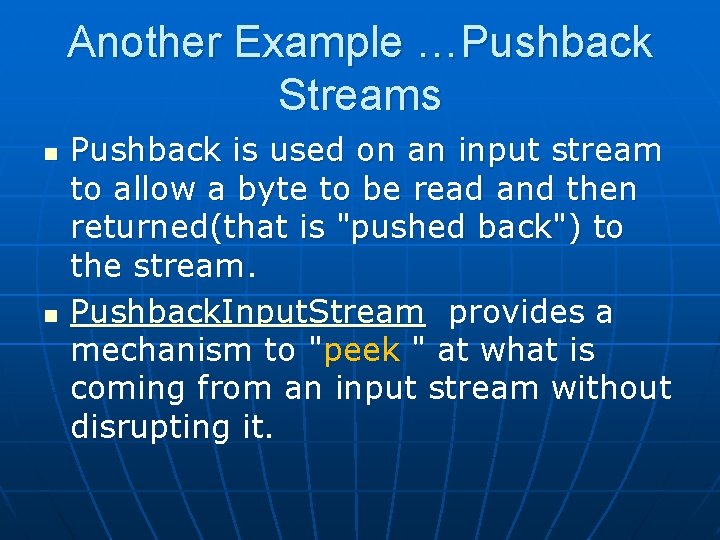
Another Example …Pushback Streams n n Pushback is used on an input stream to allow a byte to be read and then returned(that is "pushed back") to the stream. Pushback. Input. Stream provides a mechanism to "peek " at what is coming from an input stream without disrupting it.
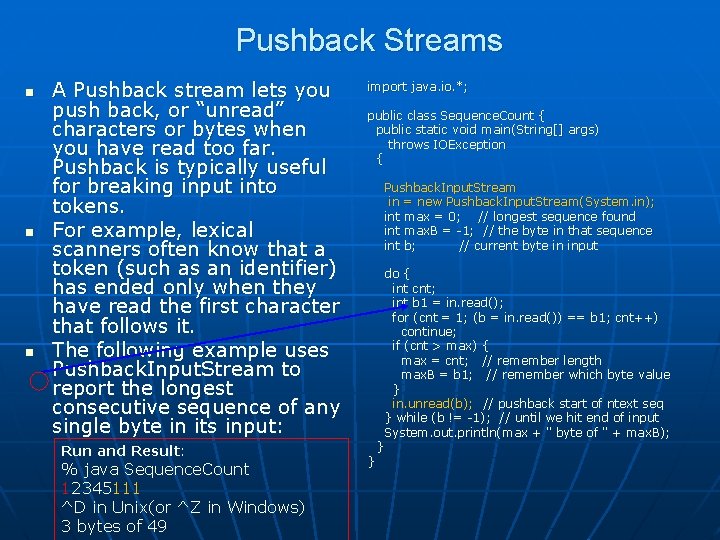
Pushback Streams n n n A Pushback stream lets you push back, or “unread” characters or bytes when you have read too far. Pushback is typically useful for breaking input into tokens. For example, lexical scanners often know that a token (such as an identifier) has ended only when they have read the first character that follows it. The following example uses Pushback. Input. Stream to report the longest consecutive sequence of any single byte in its input: Run and Result: % java Sequence. Count 12345111 ^D in Unix(or ^Z in Windows) 3 bytes of 49 import java. io. *; public class Sequence. Count { public static void main(String[] args) throws IOException { Pushback. Input. Stream in = new Pushback. Input. Stream(System. in); int max = 0; // longest sequence found int max. B = -1; // the byte in that sequence int b; // current byte in input } } do { int cnt; int b 1 = in. read(); for (cnt = 1; (b = in. read()) == b 1; cnt++) continue; if (cnt > max) { max = cnt; // remember length max. B = b 1; // remember which byte value } in. unread(b); // pushback start of ntext seq } while (b != -1); // until we hit end of input System. out. println(max + " byte of " + max. B);
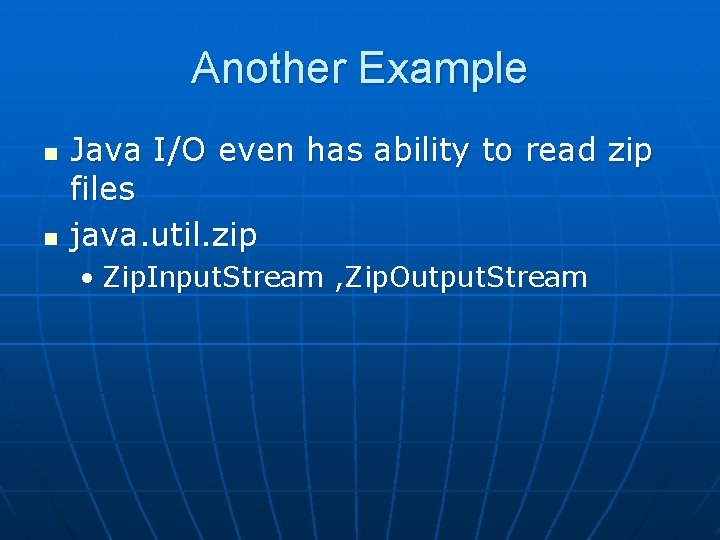
Another Example n n Java I/O even has ability to read zip files java. util. zip • Zip. Input. Stream , Zip. Output. Stream
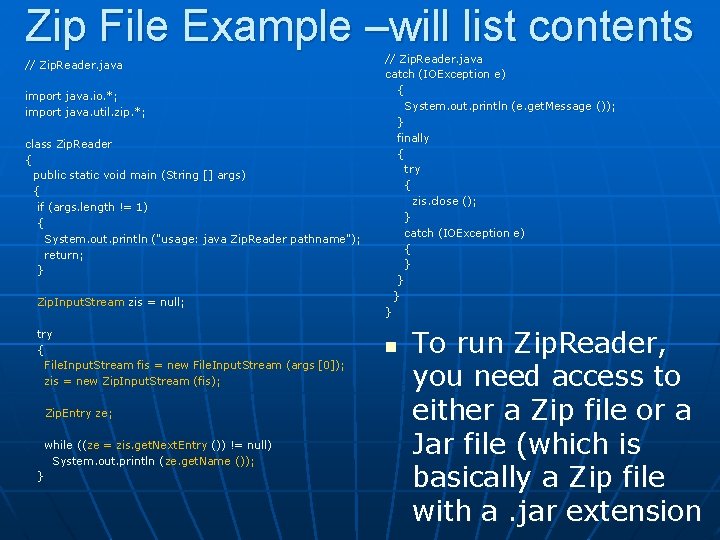
Zip File Example –will list contents // Zip. Reader. java import java. io. *; import java. util. zip. *; class Zip. Reader { public static void main (String [] args) { if (args. length != 1) { System. out. println ("usage: java Zip. Reader pathname"); return; } Zip. Input. Stream zis = null; try { File. Input. Stream fis = new File. Input. Stream (args [0]); zis = new Zip. Input. Stream (fis); Zip. Entry ze; while ((ze = zis. get. Next. Entry ()) != null) System. out. println (ze. get. Name ()); } // Zip. Reader. java catch (IOException e) { System. out. println (e. get. Message ()); } finally { try { zis. close (); } catch (IOException e) { } } n To run Zip. Reader, you need access to either a Zip file or a Jar file (which is basically a Zip file with a. jar extension
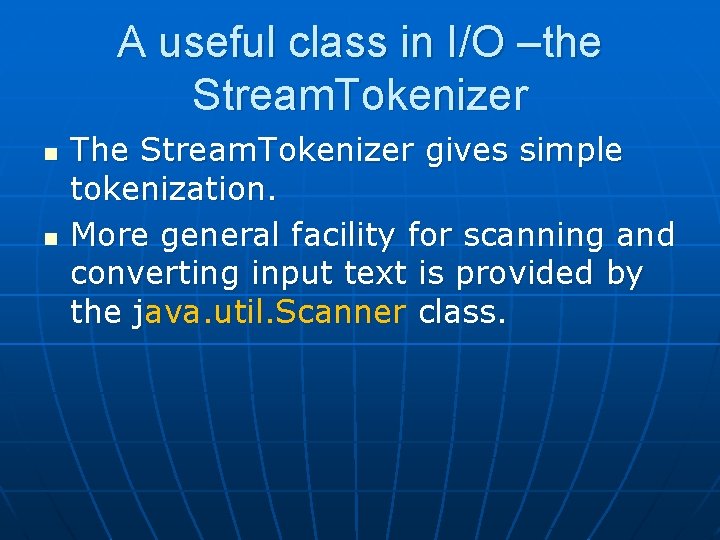
A useful class in I/O –the Stream. Tokenizer n n The Stream. Tokenizer gives simple tokenization. More general facility for scanning and converting input text is provided by the java. util. Scanner class.
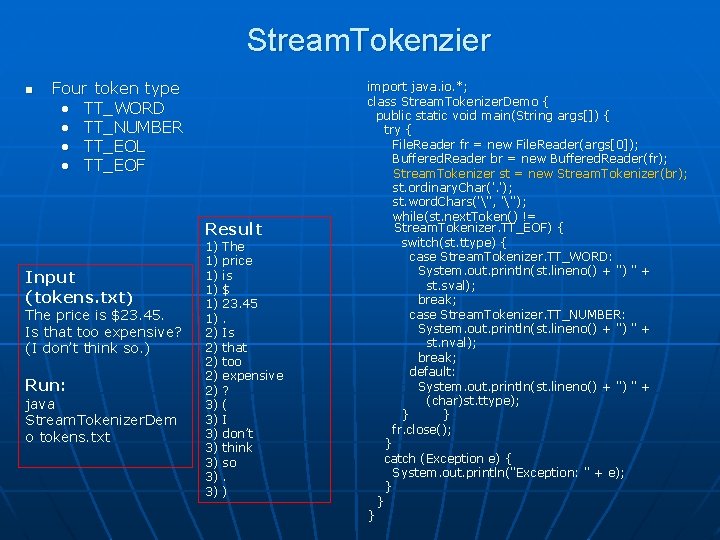
Stream. Tokenzier n Four token type • TT_WORD • TT_NUMBER • TT_EOL • TT_EOF Result Input (tokens. txt) The price is $23. 45. Is that too expensive? (I don’t think so. ) Run: java Stream. Tokenizer. Dem o tokens. txt 1) 1) 1) 2) 2) 2) 3) 3) The price is $ 23. 45. Is that too expensive ? ( I don’t think so. ) import java. io. *; class Stream. Tokenizer. Demo { public static void main(String args[]) { try { File. Reader fr = new File. Reader(args[0]); Buffered. Reader br = new Buffered. Reader(fr); Stream. Tokenizer st = new Stream. Tokenizer(br); st. ordinary. Char('. '); st. word. Chars(''', '''); while(st. next. Token() != Stream. Tokenizer. TT_EOF) { switch(st. ttype) { case Stream. Tokenizer. TT_WORD: System. out. println(st. lineno() + ") " + st. sval); break; case Stream. Tokenizer. TT_NUMBER: System. out. println(st. lineno() + ") " + st. nval); break; default: System. out. println(st. lineno() + ") " + (char)st. ttype); } } fr. close(); } catch (Exception e) { System. out. println("Exception: " + e); } } }
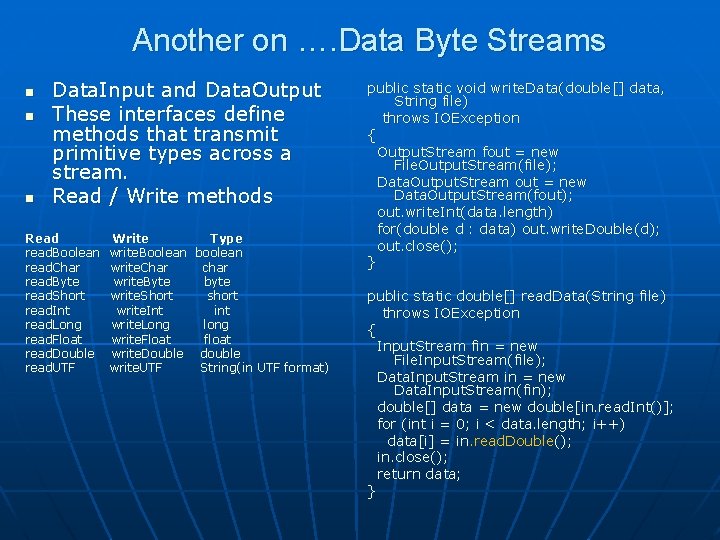
Another on …. Data Byte Streams n n n Data. Input and Data. Output These interfaces define methods that transmit primitive types across a stream. Read / Write methods Read read. Boolean read. Char read. Byte read. Short read. Int read. Long read. Float read. Double read. UTF Write Type write. Boolean boolean write. Char char write. Byte byte write. Short short write. Int int write. Long long write. Float float write. Double double write. UTF String(in UTF format) public static void write. Data(double[] data, String file) throws IOException { Output. Stream fout = new File. Output. Stream(file); Data. Output. Stream out = new Data. Output. Stream(fout); out. write. Int(data. length) for(double d : data) out. write. Double(d); out. close(); } public static double[] read. Data(String file) throws IOException { Input. Stream fin = new File. Input. Stream(file); Data. Input. Stream in = new Data. Input. Stream(fin); double[] data = new double[in. read. Int()]; for (int i = 0; i < data. length; i++) data[i] = in. read. Double(); in. close(); return data; }
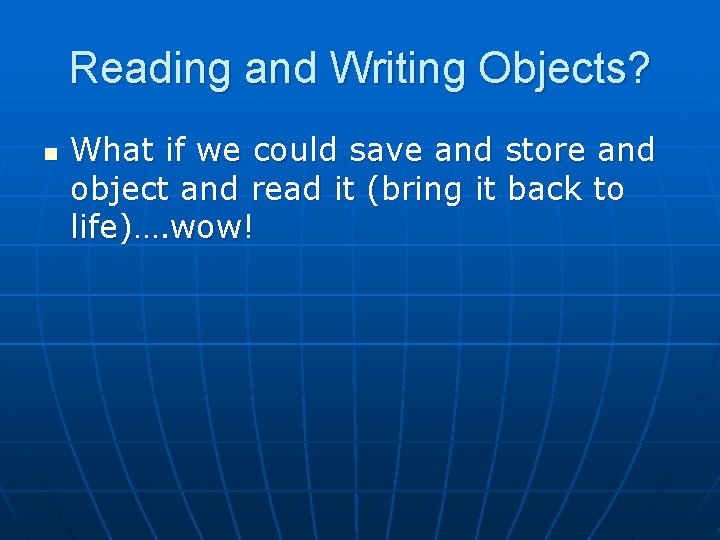
Reading and Writing Objects? n What if we could save and store and object and read it (bring it back to life)…. wow!
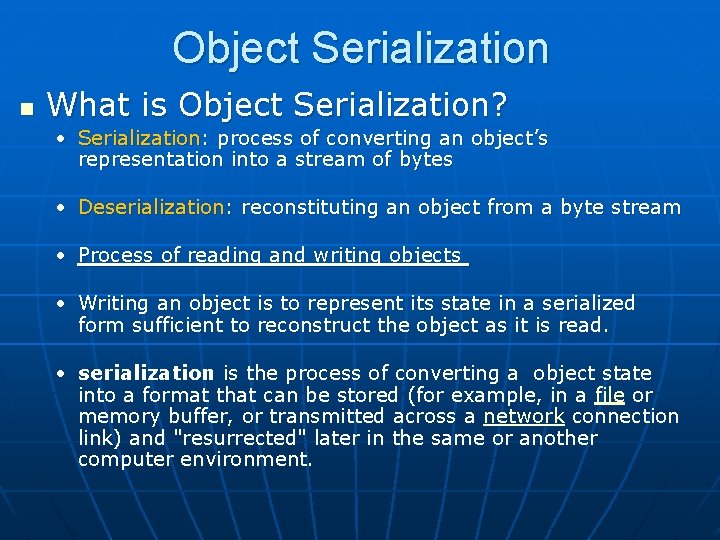
Object Serialization n What is Object Serialization? • Serialization: process of converting an object’s representation into a stream of bytes • Deserialization: reconstituting an object from a byte stream • Process of reading and writing objects • Writing an object is to represent its state in a serialized form sufficient to reconstruct the object as it is read. • serialization is the process of converting a object state into a format that can be stored (for example, in a file or memory buffer, or transmitted across a network connection link) and "resurrected" later in the same or another computer environment.
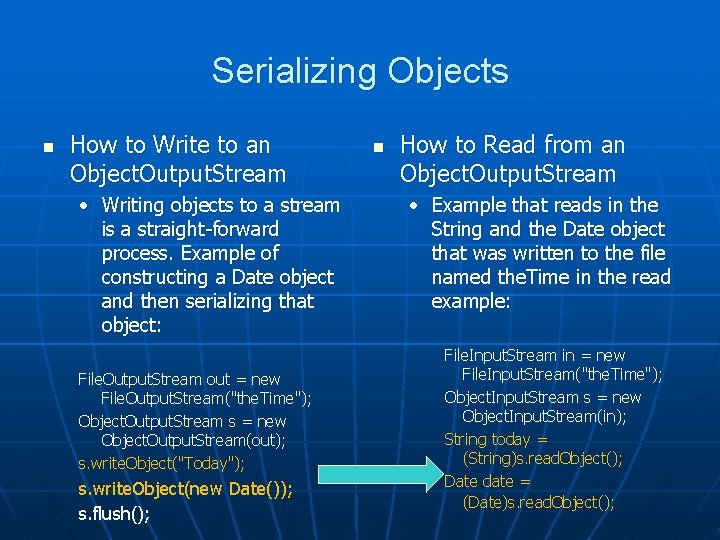
Serializing Objects n How to Write to an Object. Output. Stream • Writing objects to a stream is a straight-forward process. Example of constructing a Date object and then serializing that object: File. Output. Stream out = new File. Output. Stream("the. Time"); Object. Output. Stream s = new Object. Output. Stream(out); s. write. Object("Today"); s. write. Object(new Date()); s. flush(); n How to Read from an Object. Output. Stream • Example that reads in the String and the Date object that was written to the file named the. Time in the read example: File. Input. Stream in = new File. Input. Stream("the. Time"); Object. Input. Stream s = new Object. Input. Stream(in); String today = (String)s. read. Object(); Date date = (Date)s. read. Object();
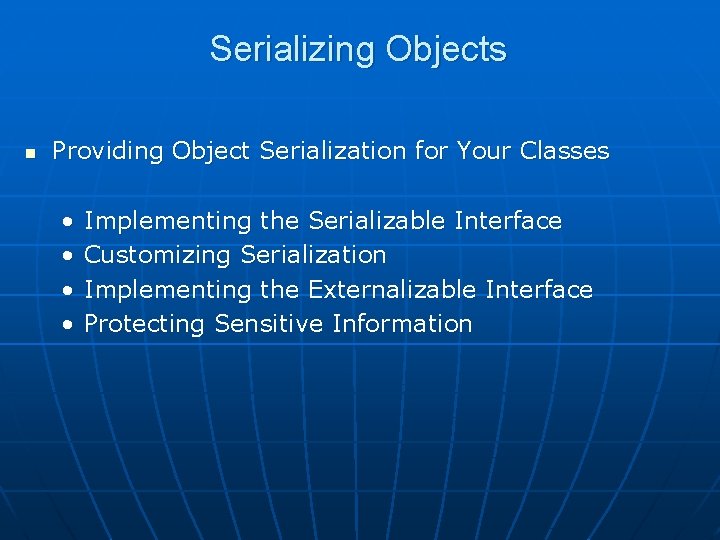
Serializing Objects n Providing Object Serialization for Your Classes • • Implementing the Serializable Interface Customizing Serialization Implementing the Externalizable Interface Protecting Sensitive Information
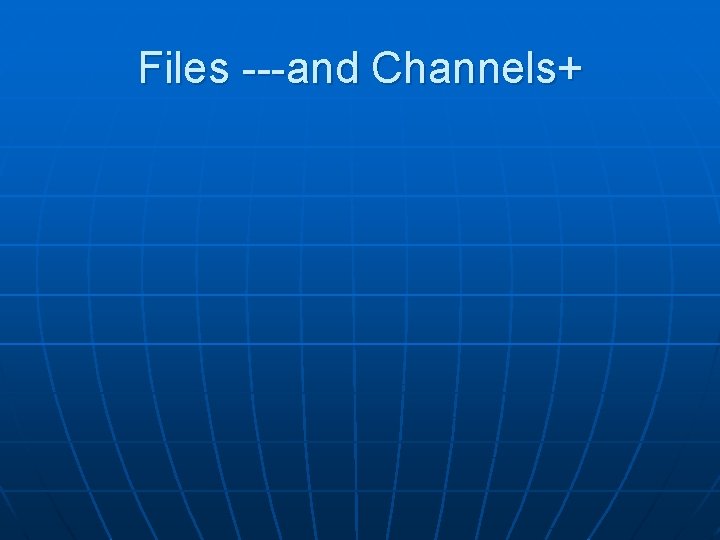
Files ---and Channels+
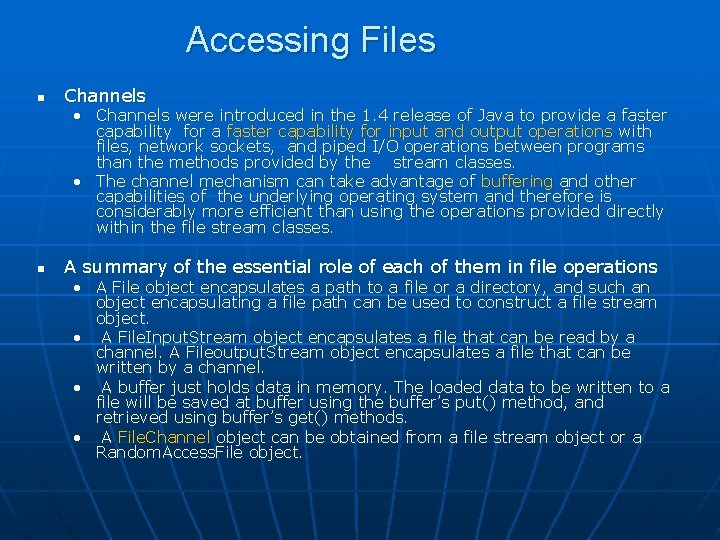
Accessing Files n Channels • Channels were introduced in the 1. 4 release of Java to provide a faster capability for input and output operations with files, network sockets, and piped I/O operations between programs than the methods provided by the stream classes. • The channel mechanism can take advantage of buffering and other capabilities of the underlying operating system and therefore is considerably more efficient than using the operations provided directly within the file stream classes. n A summary of the essential role of each of them in file operations • A File object encapsulates a path to a file or a directory, and such an object encapsulating a file path can be used to construct a file stream object. • A File. Input. Stream object encapsulates a file that can be read by a channel. A Fileoutput. Stream object encapsulates a file that can be written by a channel. • A buffer just holds data in memory. The loaded data to be written to a file will be saved at buffer using the buffer’s put() method, and retrieved using buffer’s get() methods. • A File. Channel object can be obtained from a file stream object or a Random. Access. File object.
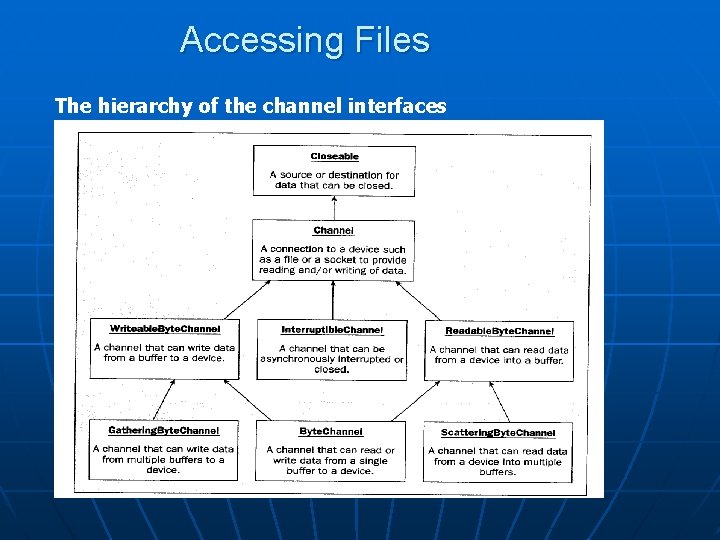
Accessing Files The hierarchy of the channel interfaces
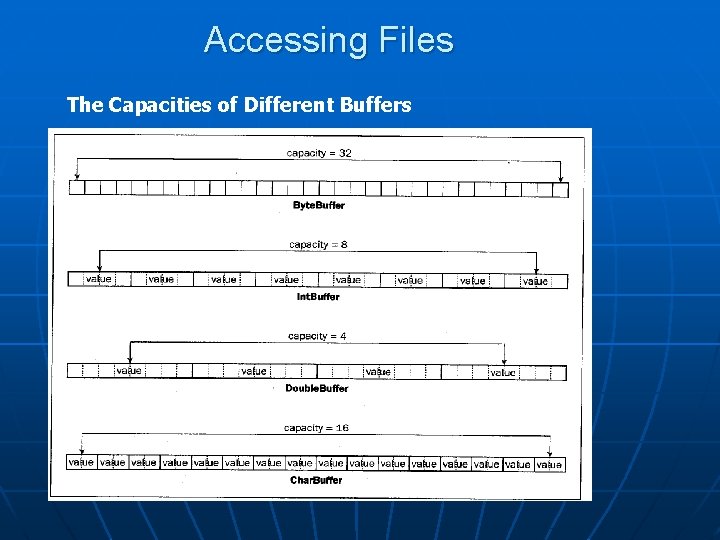
Accessing Files The Capacities of Different Buffers
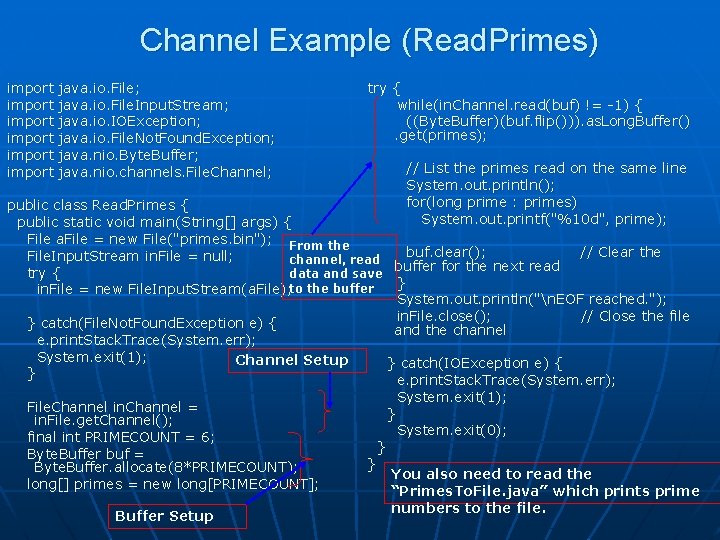
Channel Example (Read. Primes) import import java. io. File; java. io. File. Input. Stream; java. io. IOException; java. io. File. Not. Found. Exception; java. nio. Byte. Buffer; java. nio. channels. File. Channel; try { while(in. Channel. read(buf) != -1) { ((Byte. Buffer)(buf. flip())). as. Long. Buffer(). get(primes); // List the primes read on the same line System. out. println(); for(long prime : primes) System. out. printf("%10 d", prime); public class Read. Primes { public static void main(String[] args) { File a. File = new File("primes. bin"); From the buf. clear(); // Clear the File. Input. Stream in. File = null; channel, read buffer for the next read try { data and save } in. File = new File. Input. Stream(a. File); to the buffer System. out. println("n. EOF reached. "); in. File. close(); // Close the file } catch(File. Not. Found. Exception e) { and the channel e. print. Stack. Trace(System. err); System. exit(1); Channel Setup } catch(IOException e) { } e. print. Stack. Trace(System. err); System. exit(1); File. Channel in. Channel = } in. File. get. Channel(); System. exit(0); final int PRIMECOUNT = 6; } Byte. Buffer buf = } Byte. Buffer. allocate(8*PRIMECOUNT); You also need to read the long[] primes = new long[PRIMECOUNT]; “Primes. To. File. java” which prints prime Buffer Setup numbers to the file.
Virtuozzo docker
Collections overview in java
Import java.util
Import java.util.*
Language
Import java.util.*
Java thread import
Import javax.swing.*
Java util random
Perbedaan awt dan swing
Java util import
Import java.io.*
Java swing form example
Ejb remote vs local
Import java.awt.* import java.awt.event.*
Java
The overall heat transfer coefficient
System analysis
Real time interaction management forrester
Moritz zimmermann sap
Overview of oracle architecture
Sap easy cost planning
Sap sd overview
Texas public school finance overview
Vocabulary overview guide
Overview of business environment
Department overview template
Basha high school counselors
Psalms overview
Amway business overview
Sap mobile platform overview
Www overview
Business intelligence overview
Ajax overview
Overview of cellular respiration
Exchange online protection overview
Apple inc introduction
Corporate finance overview
Overview funding programmes
Fisma overview
Overview of personal finance chapter 1
Set associative mapping in cache memory
What happens in scene 3 of oedipus rex
Slidetodoc.com
Arteries of abdomen
Motivation to learn an overview of contemporary theories
Subsystem of gsm architecture
Virusmax
Mainframe education
Data quality and data cleaning an overview
Informatica powercenter overview