Fundamentals of Programming Languages II Prof Amol R
![Fundamentals of Programming Languages –II Prof. Amol R. Dhakne [ME-CSE, Ph. D Pursuing] DYPIEMR, Fundamentals of Programming Languages –II Prof. Amol R. Dhakne [ME-CSE, Ph. D Pursuing] DYPIEMR,](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-1.jpg)
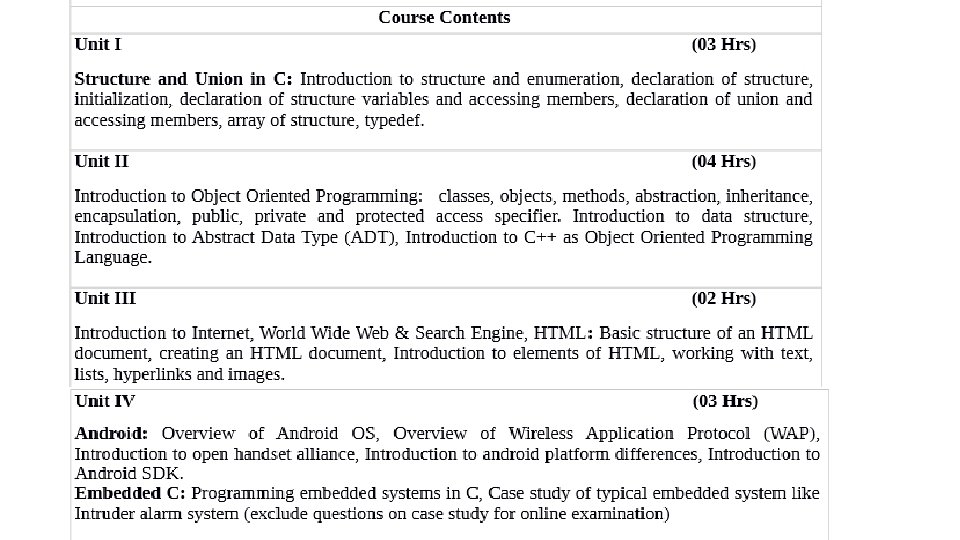
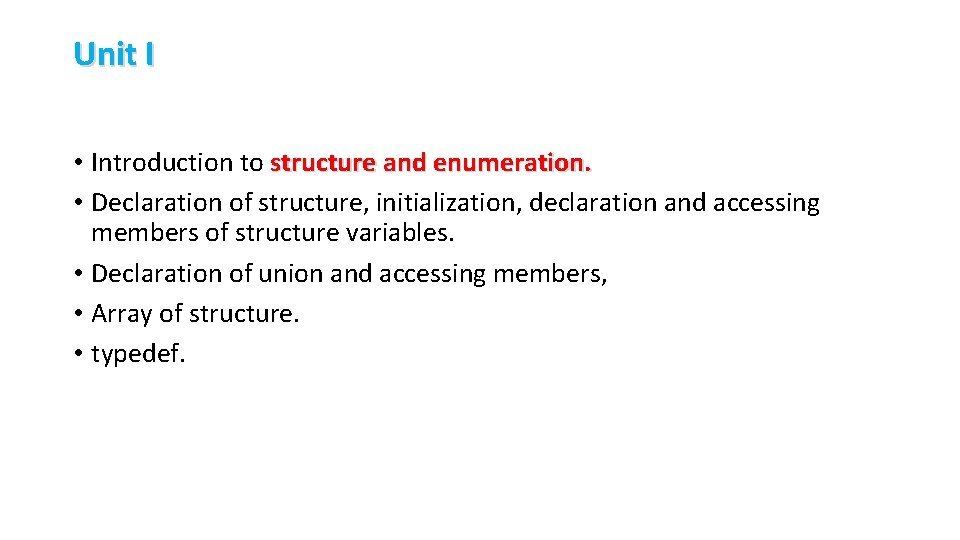
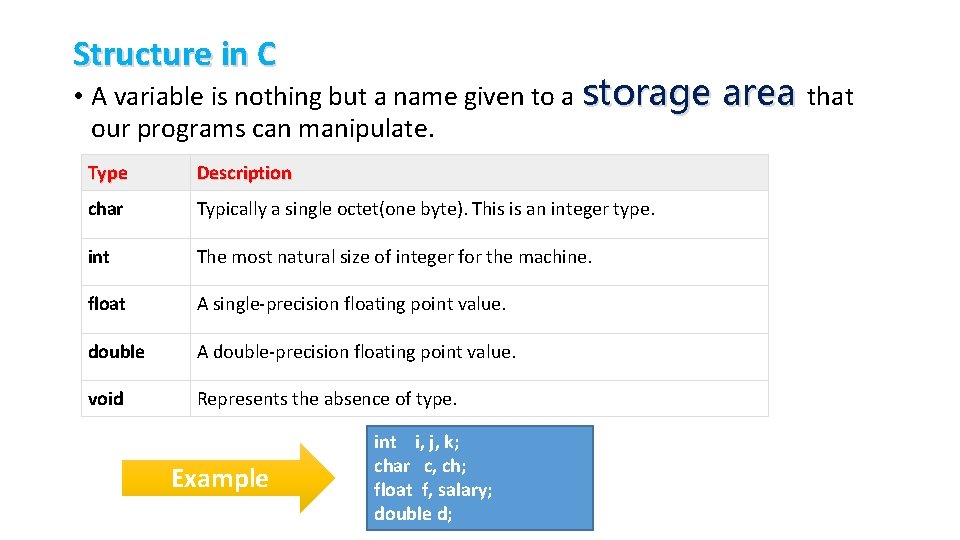
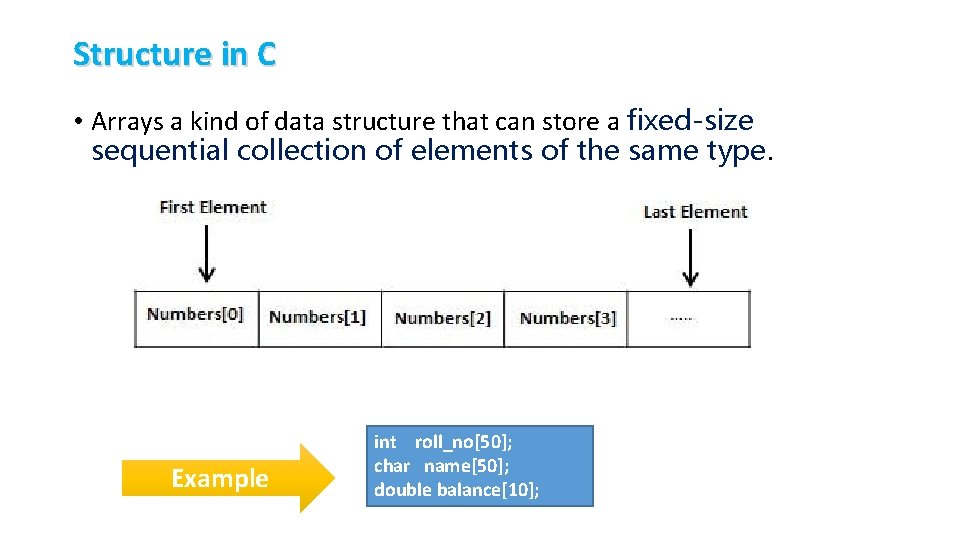
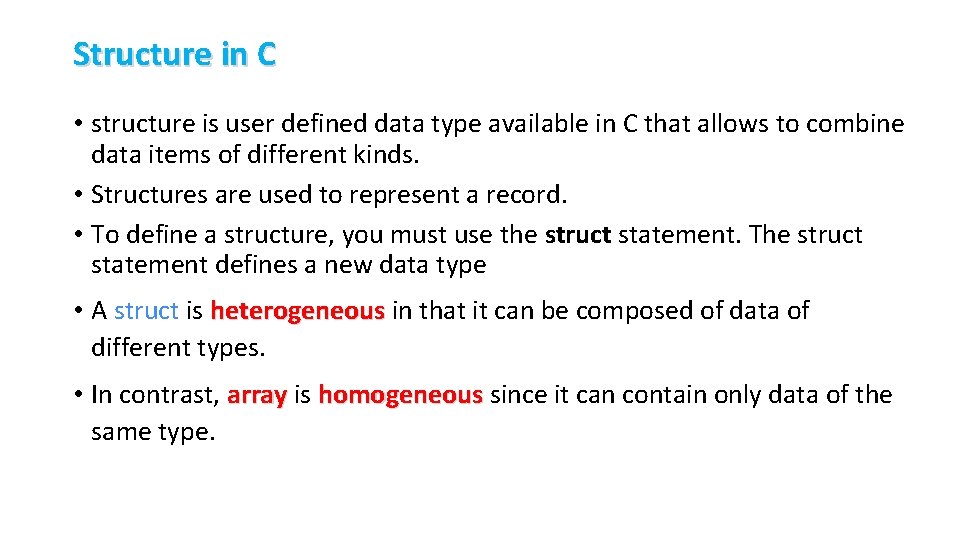
![Structure in C Syntax struct [structure tag] { member definition; . . . member Structure in C Syntax struct [structure tag] { member definition; . . . member](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-7.jpg)
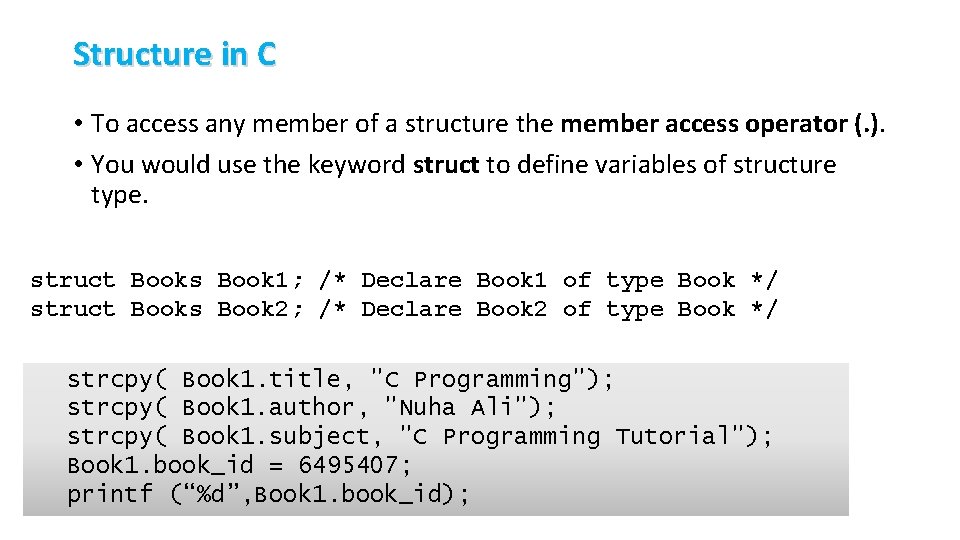
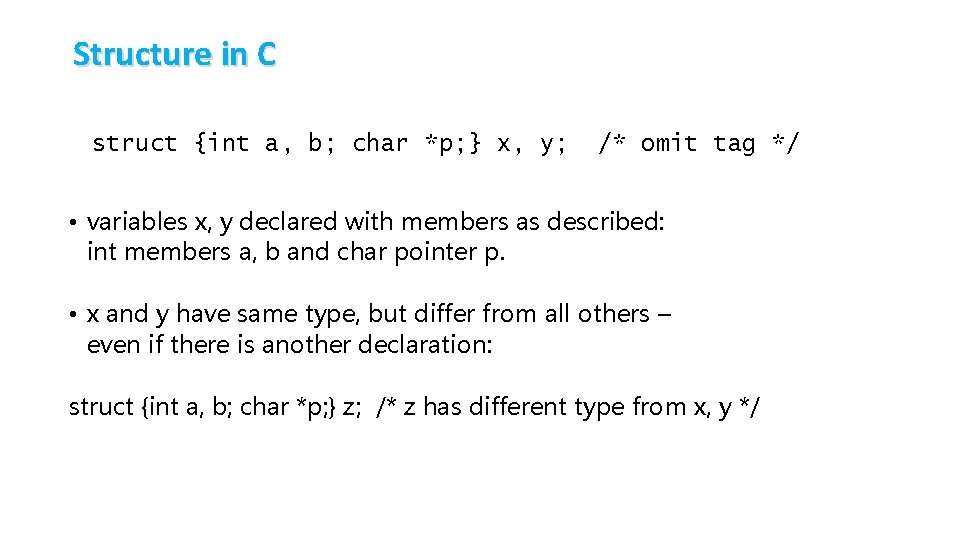
![#include <stdio. h> #include <string. h> struct Books { char title[50]; char author[50]; char #include <stdio. h> #include <string. h> struct Books { char title[50]; char author[50]; char](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-10.jpg)
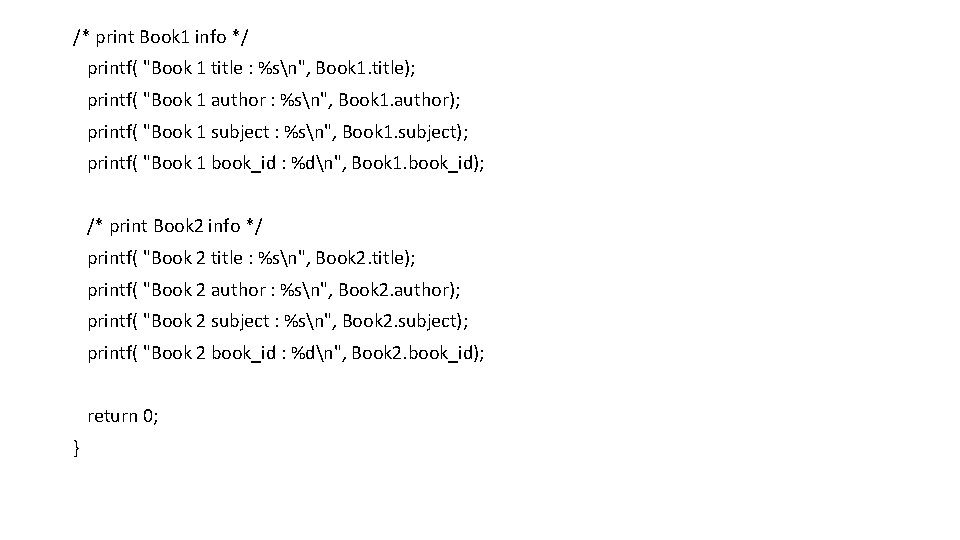
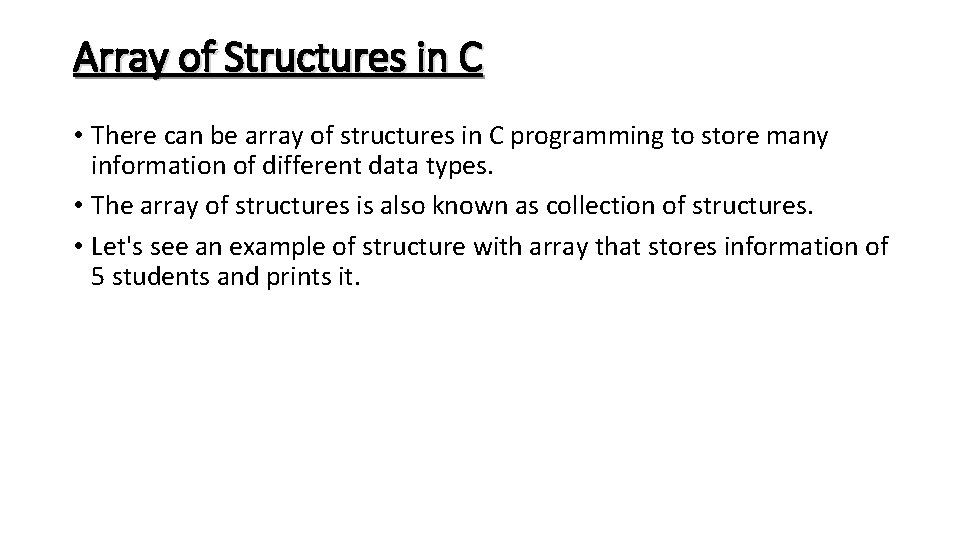
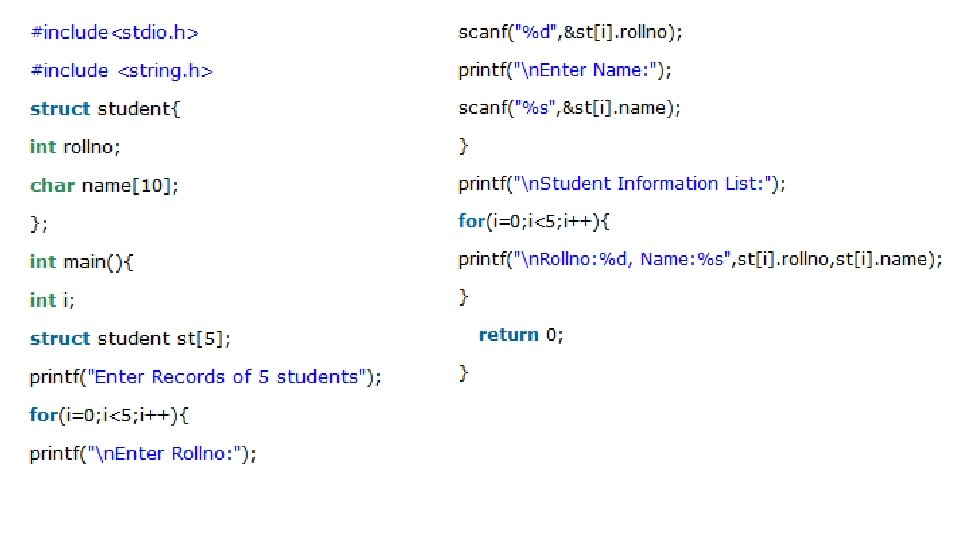
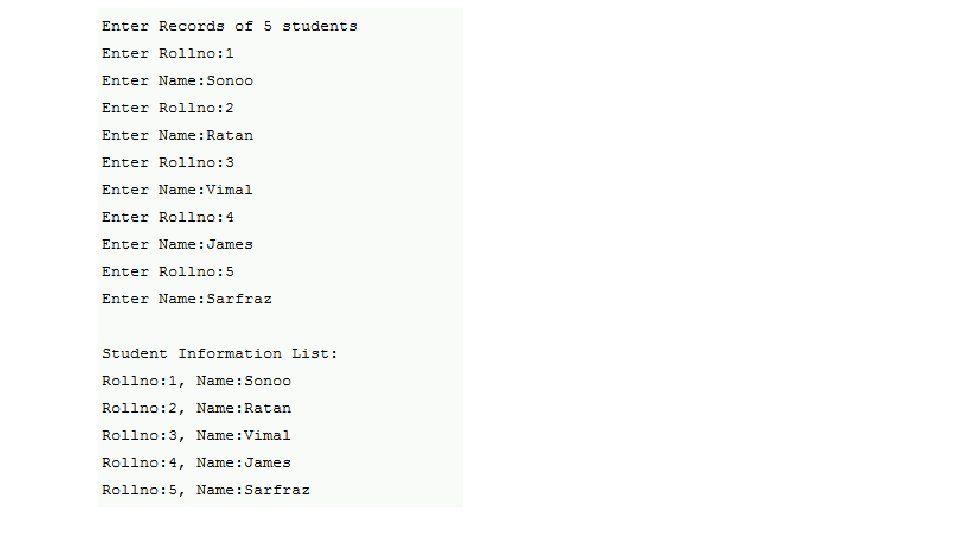
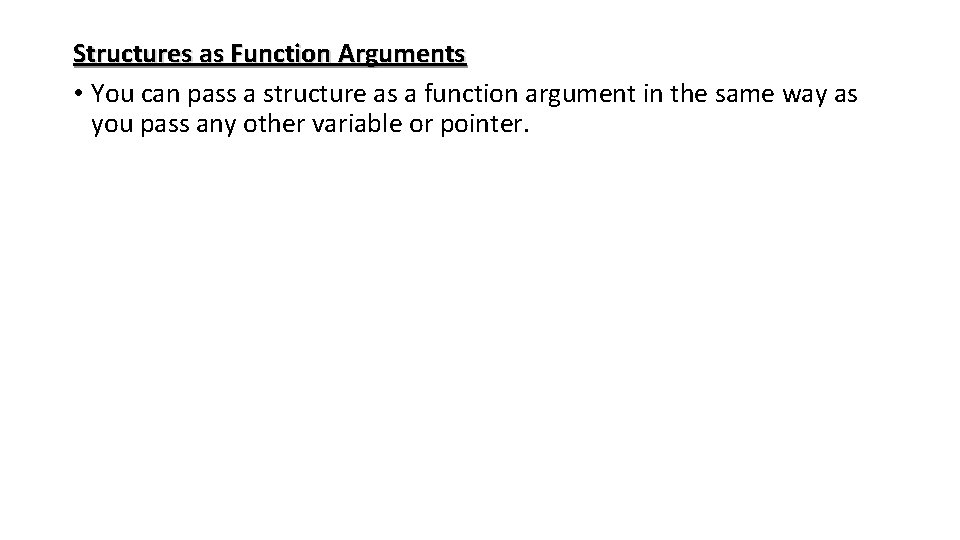
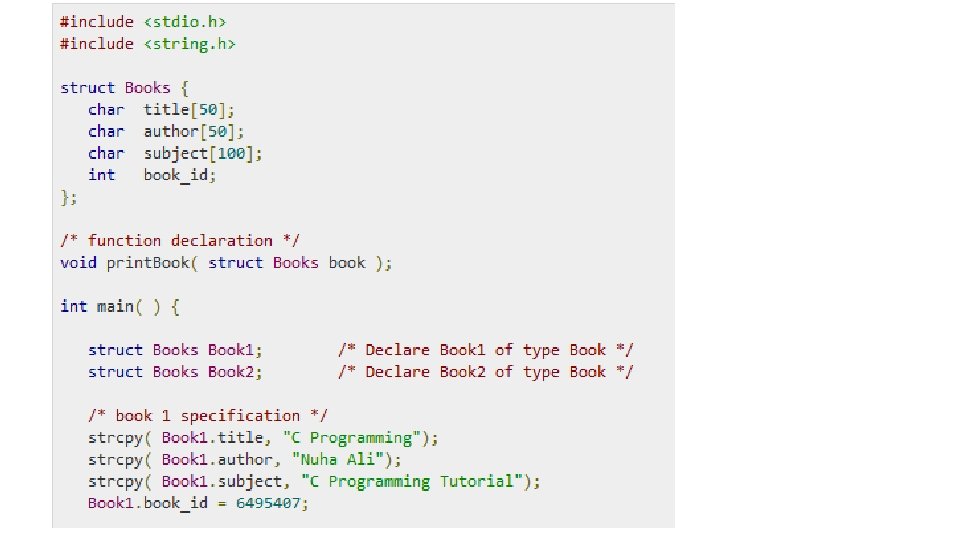
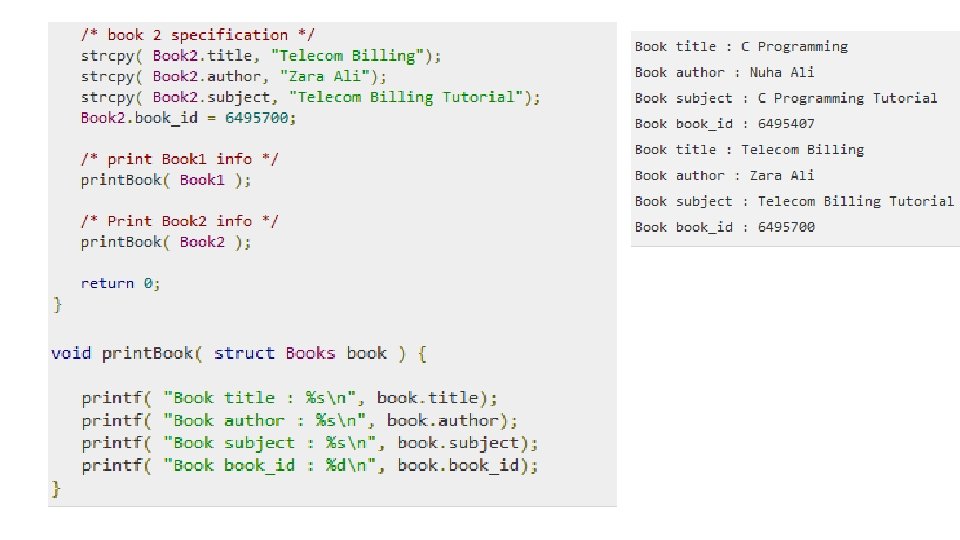
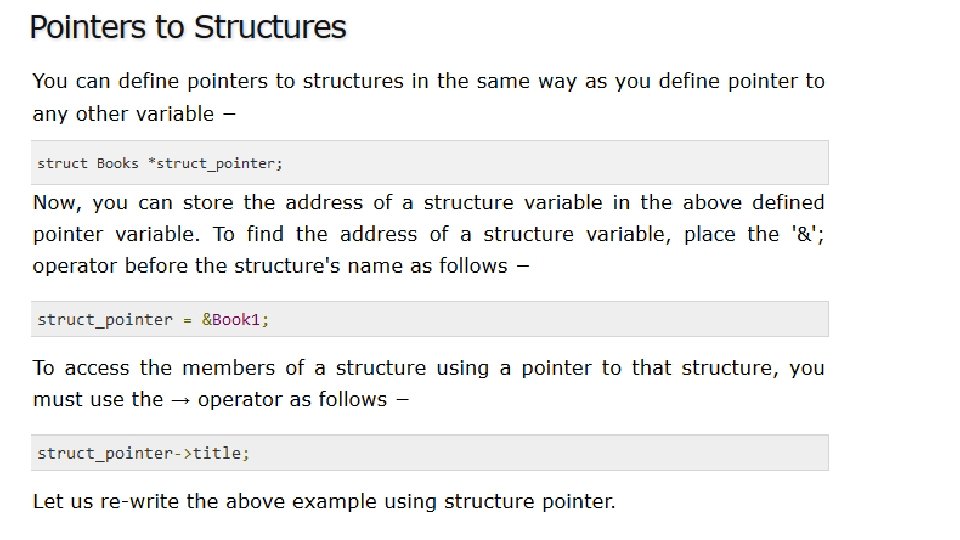
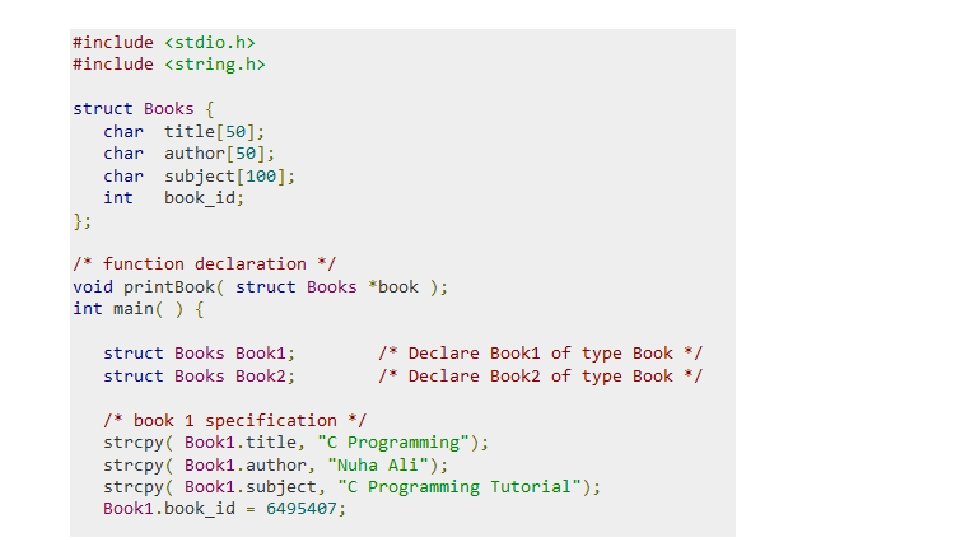
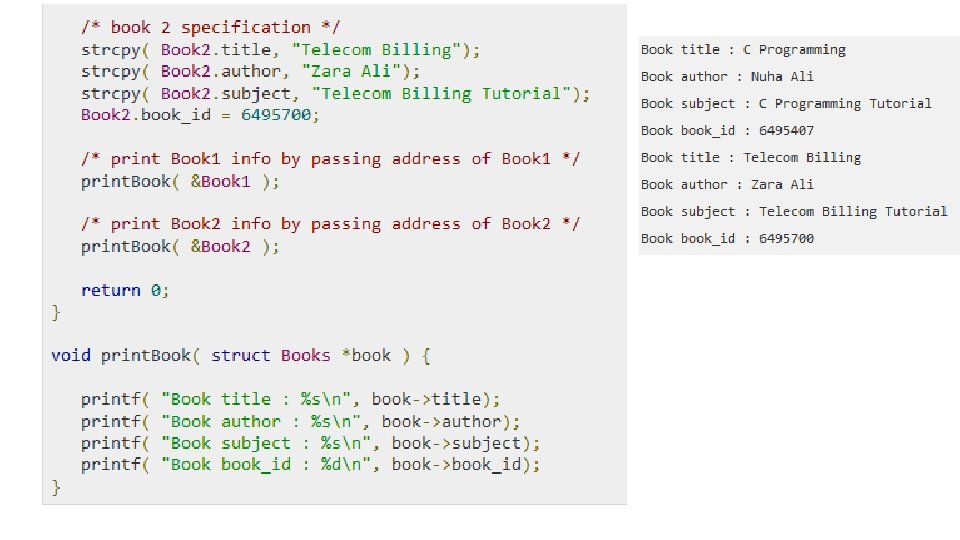
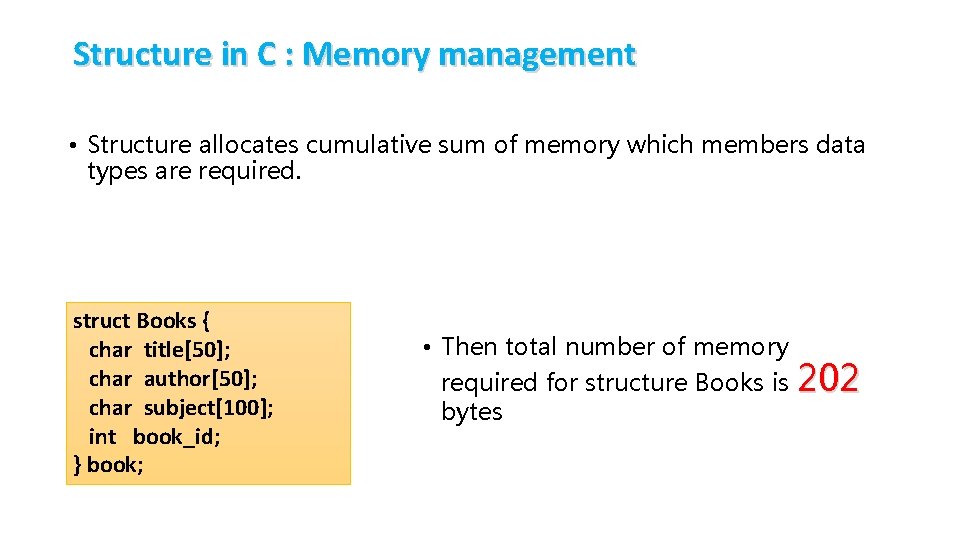
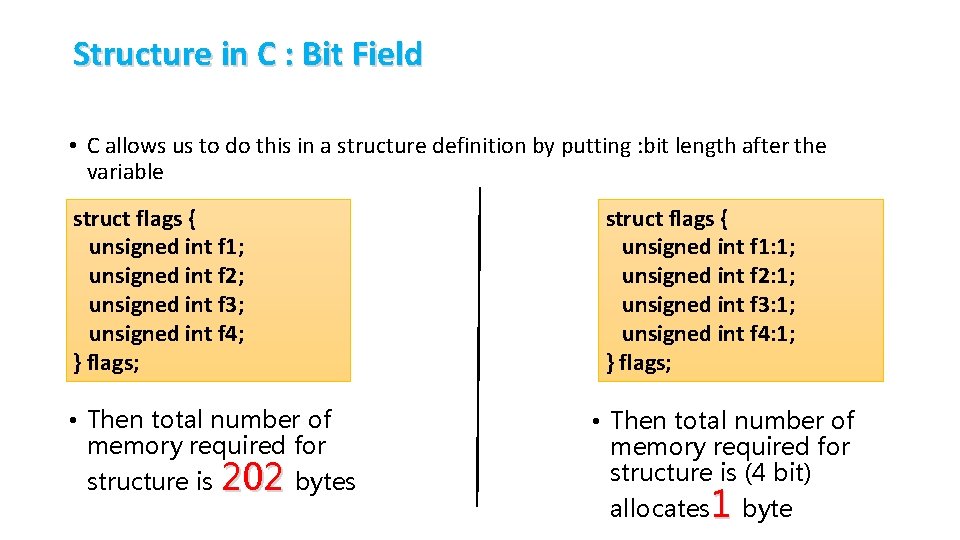
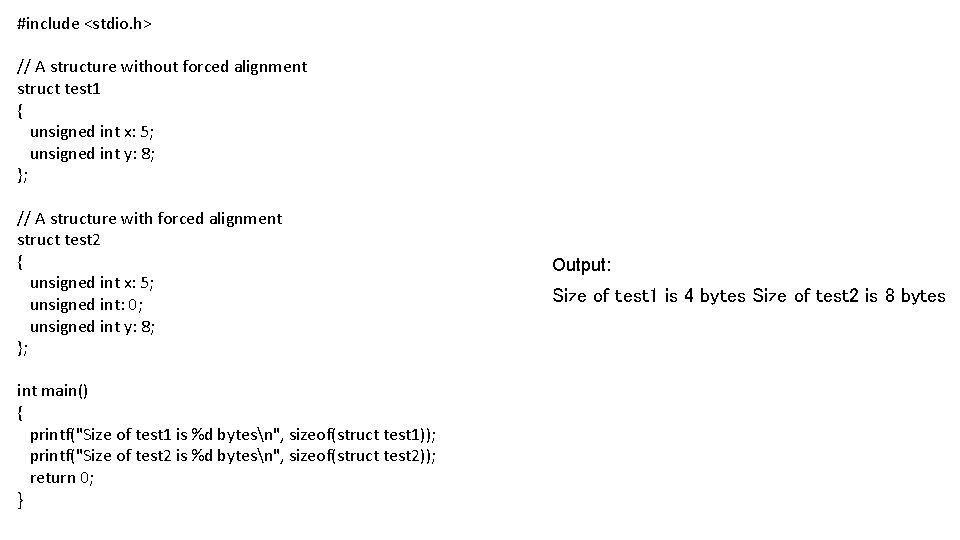
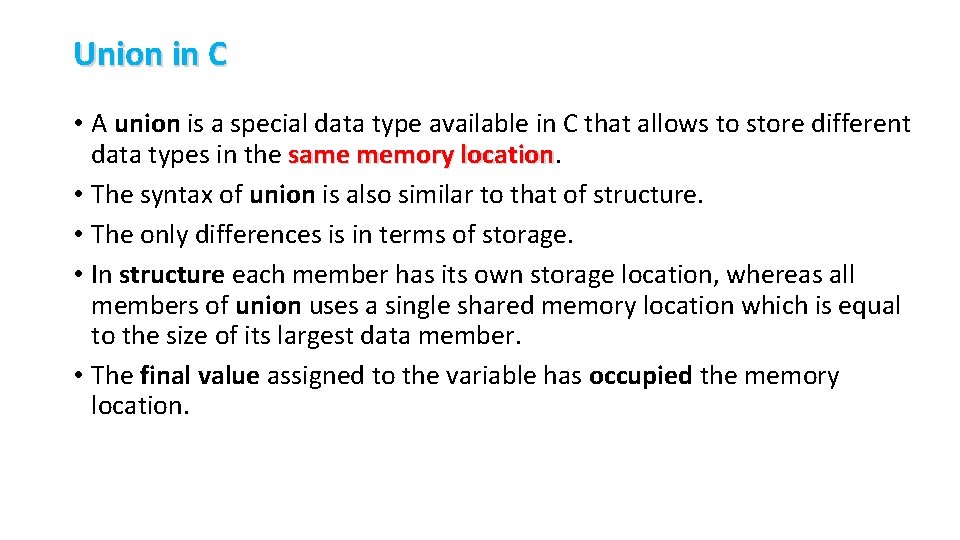
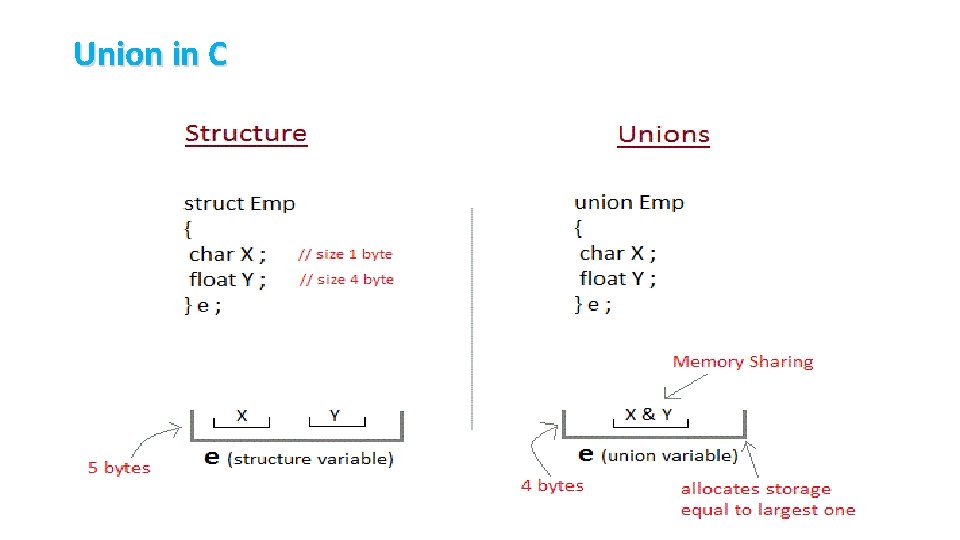
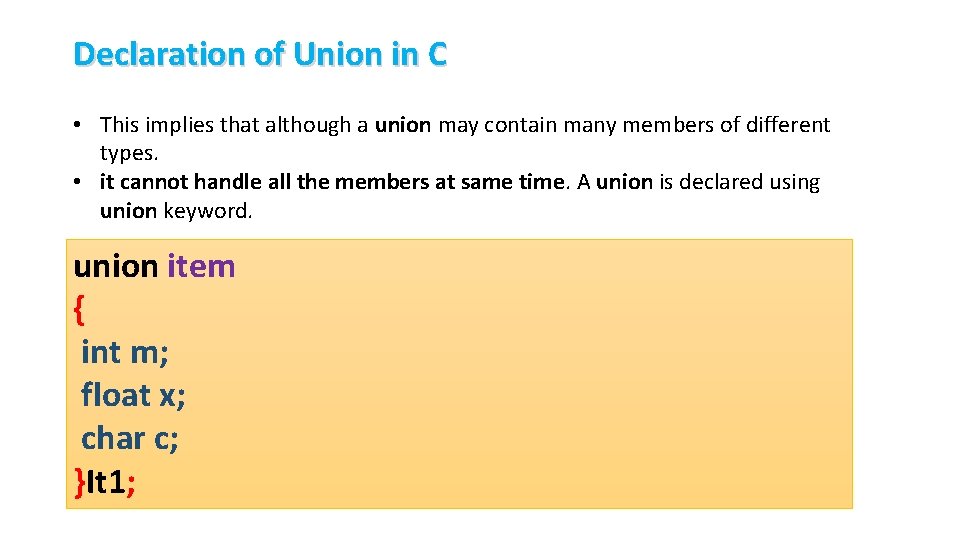
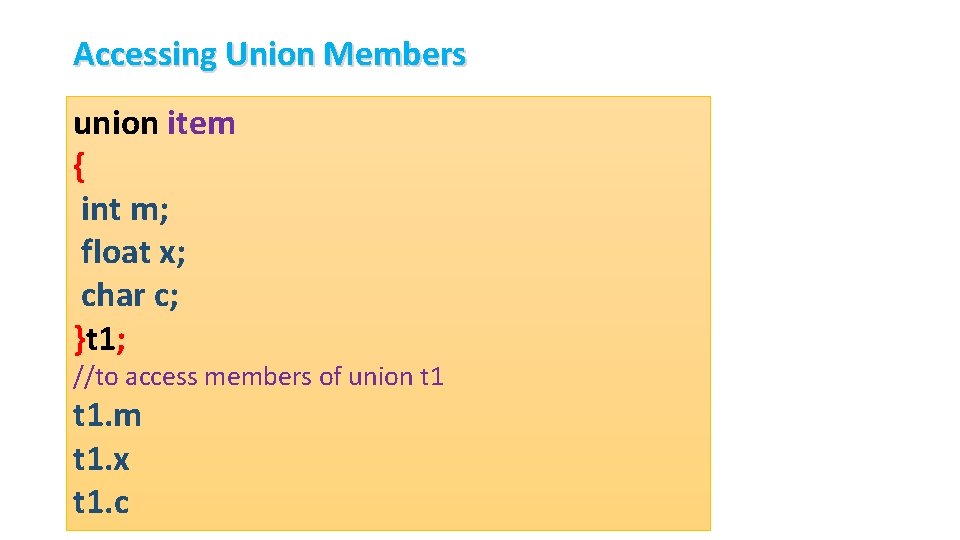
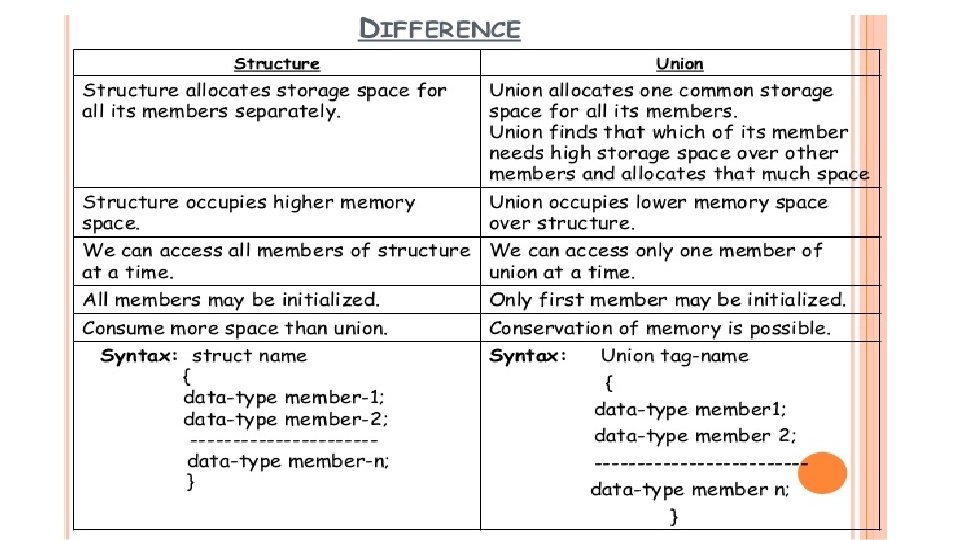
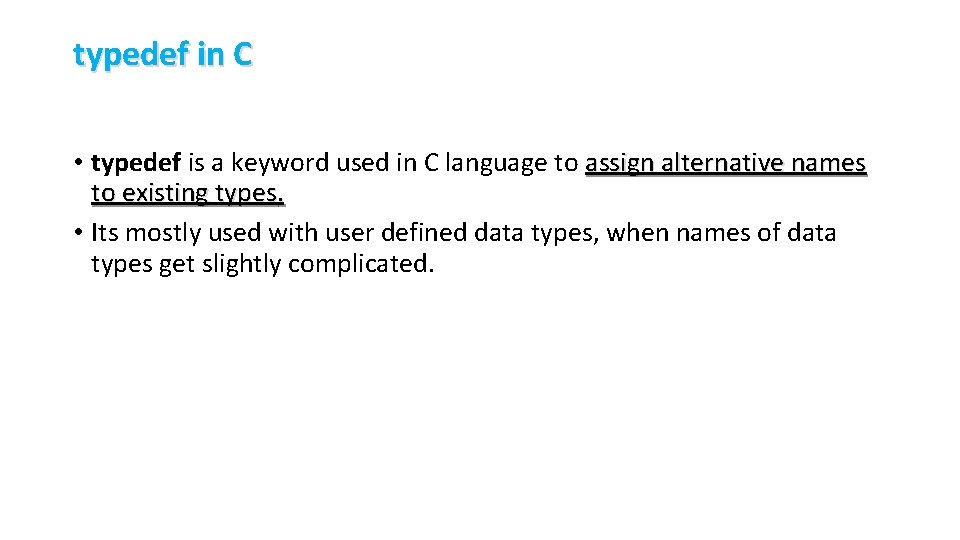
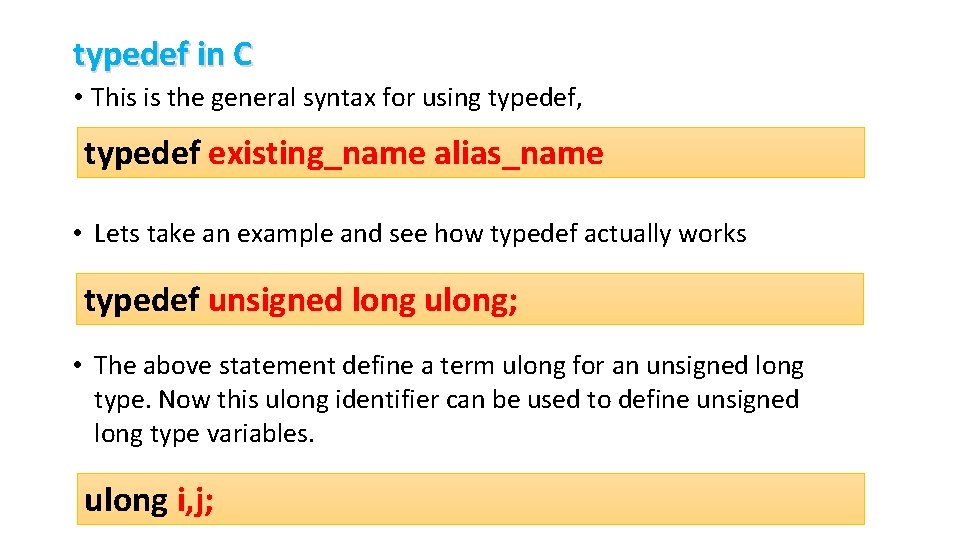
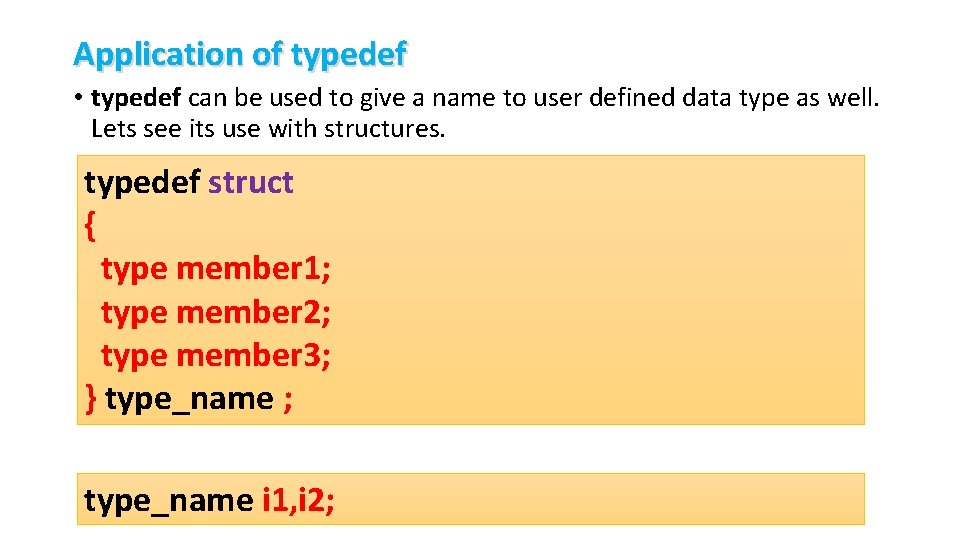
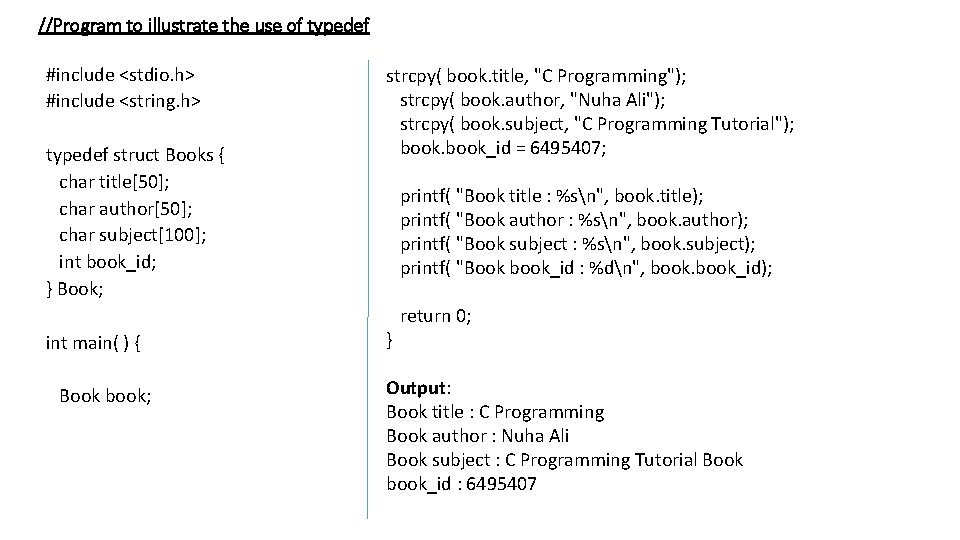
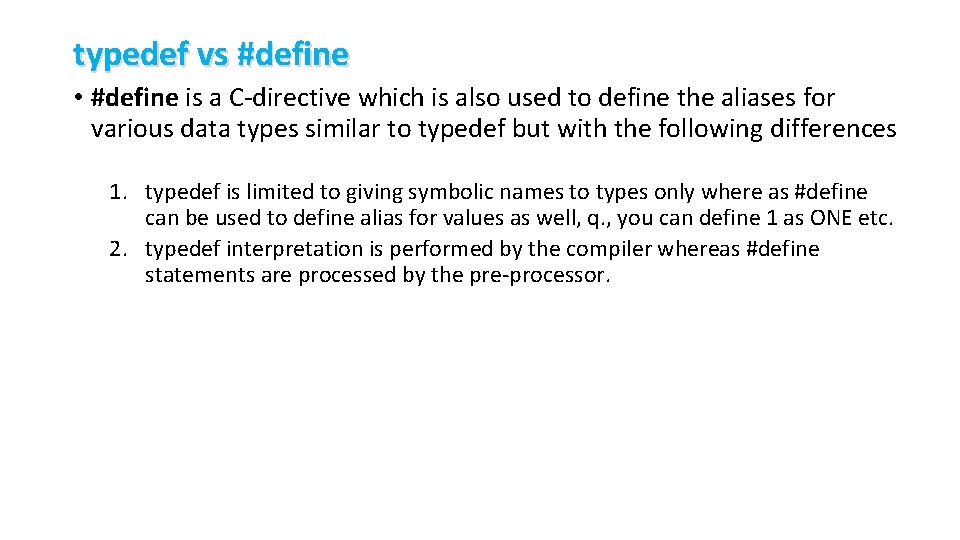
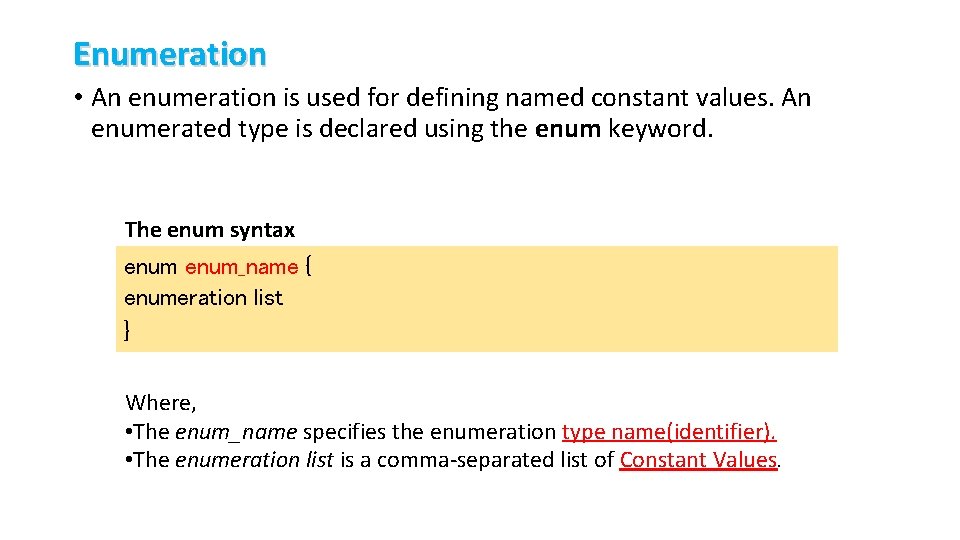
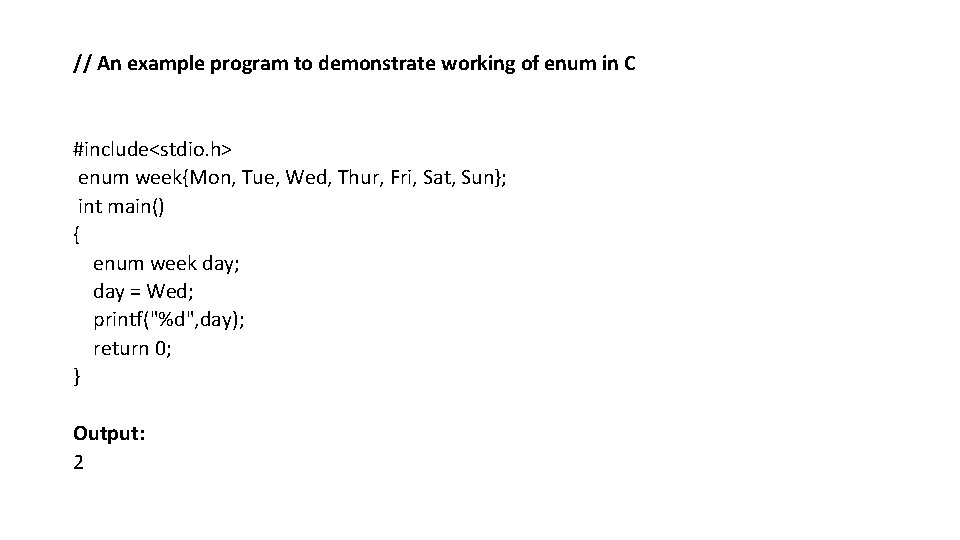
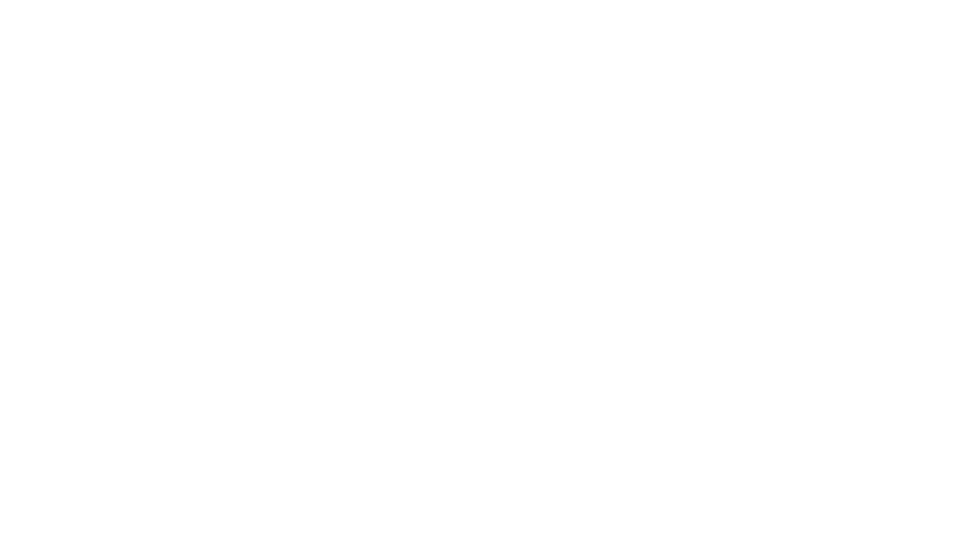
- Slides: 36
![Fundamentals of Programming Languages II Prof Amol R Dhakne MECSE Ph D Pursuing DYPIEMR Fundamentals of Programming Languages –II Prof. Amol R. Dhakne [ME-CSE, Ph. D Pursuing] DYPIEMR,](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-1.jpg)
Fundamentals of Programming Languages –II Prof. Amol R. Dhakne [ME-CSE, Ph. D Pursuing] DYPIEMR, Akurdi, Pune
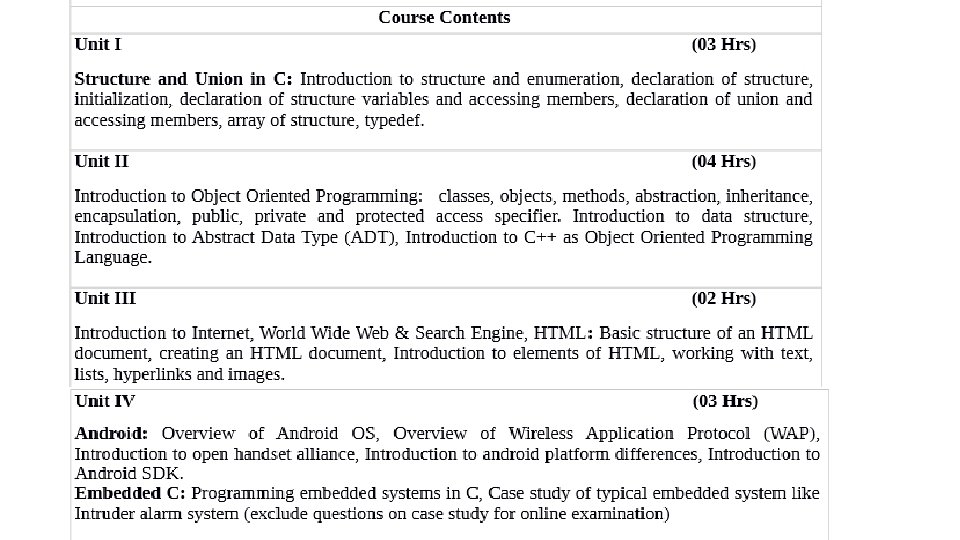
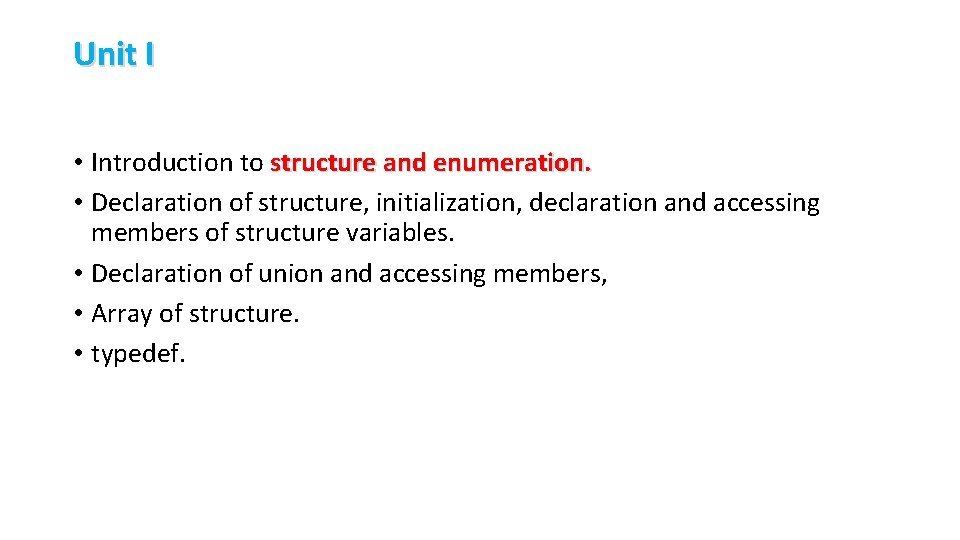
Unit I • Introduction to structure and enumeration. • Declaration of structure, initialization, declaration and accessing members of structure variables. • Declaration of union and accessing members, • Array of structure. • typedef.
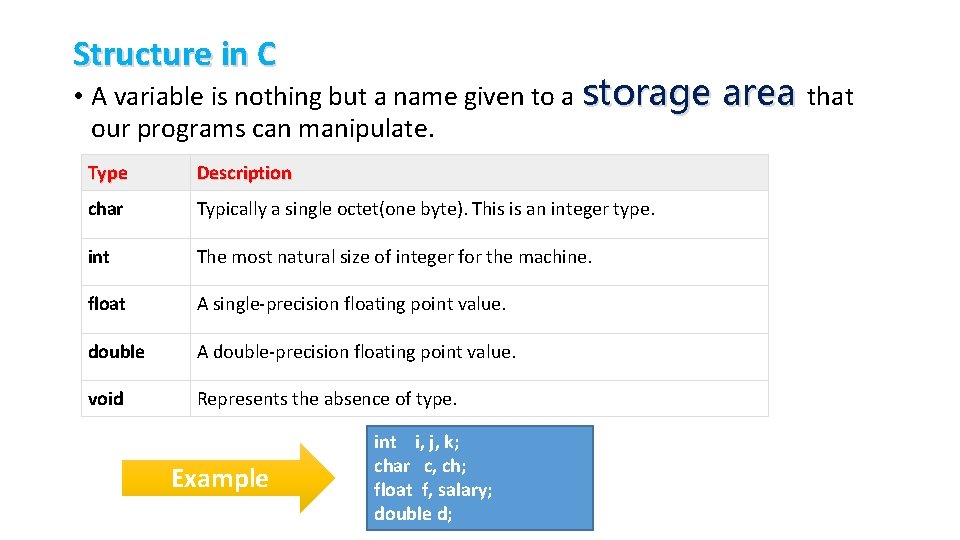
Structure in C • A variable is nothing but a name given to a storage our programs can manipulate. Type Description char Typically a single octet(one byte). This is an integer type. int The most natural size of integer for the machine. float A single-precision floating point value. double A double-precision floating point value. void Represents the absence of type. Example int i, j, k; char c, ch; float f, salary; double d; area that
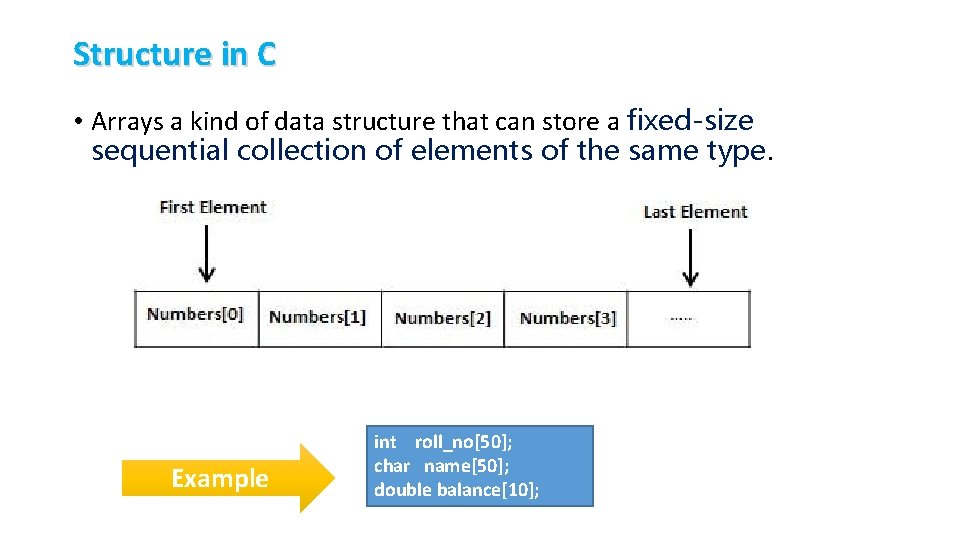
Structure in C • Arrays a kind of data structure that can store a fixed-size sequential collection of elements of the same type. Example int roll_no[50]; char name[50]; double balance[10];
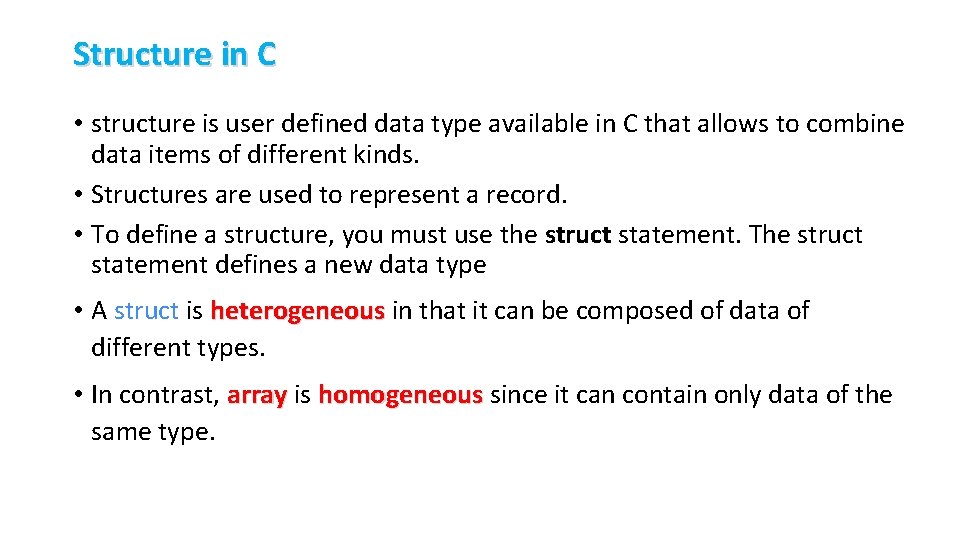
Structure in C • structure is user defined data type available in C that allows to combine data items of different kinds. • Structures are used to represent a record. • To define a structure, you must use the struct statement. The struct statement defines a new data type • A struct is heterogeneous in that it can be composed of data of heterogeneous different types. • In contrast, array is array homogeneous since it can contain only data of the homogeneous same type.
![Structure in C Syntax struct structure tag member definition member Structure in C Syntax struct [structure tag] { member definition; . . . member](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-7.jpg)
Structure in C Syntax struct [structure tag] { member definition; . . . member definition; } [one or more structure variables]; Example struct Books { char title[50]; char author[50]; char subject[100]; int book_id; } book;
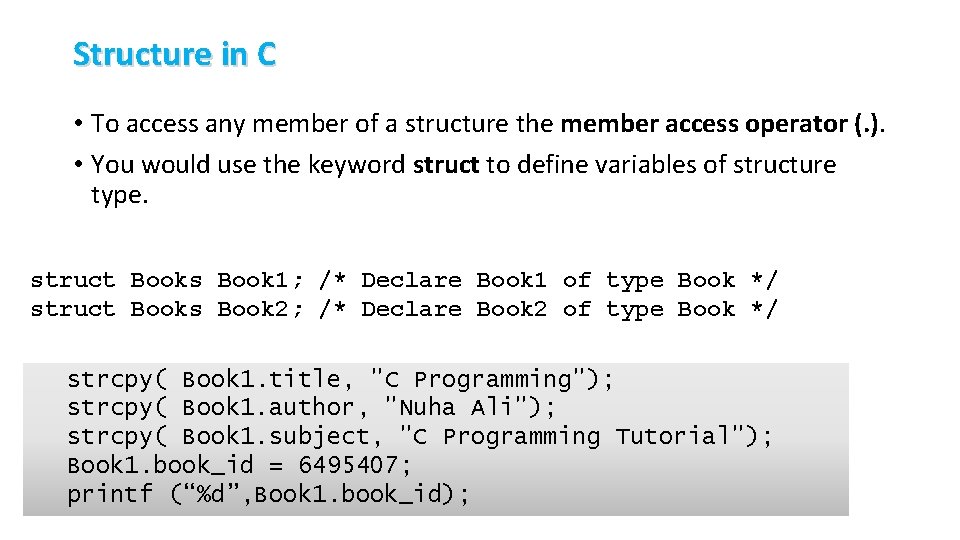
Structure in C • To access any member of a structure the member access operator (. ). • You would use the keyword struct to define variables of structure type. struct Books Book 1; /* Declare Book 1 of type Book */ struct Books Book 2; /* Declare Book 2 of type Book */ strcpy( Book 1. title, "C Programming"); strcpy( Book 1. author, "Nuha Ali"); strcpy( Book 1. subject, "C Programming Tutorial"); Book 1. book_id = 6495407; printf (“%d”, Book 1. book_id);
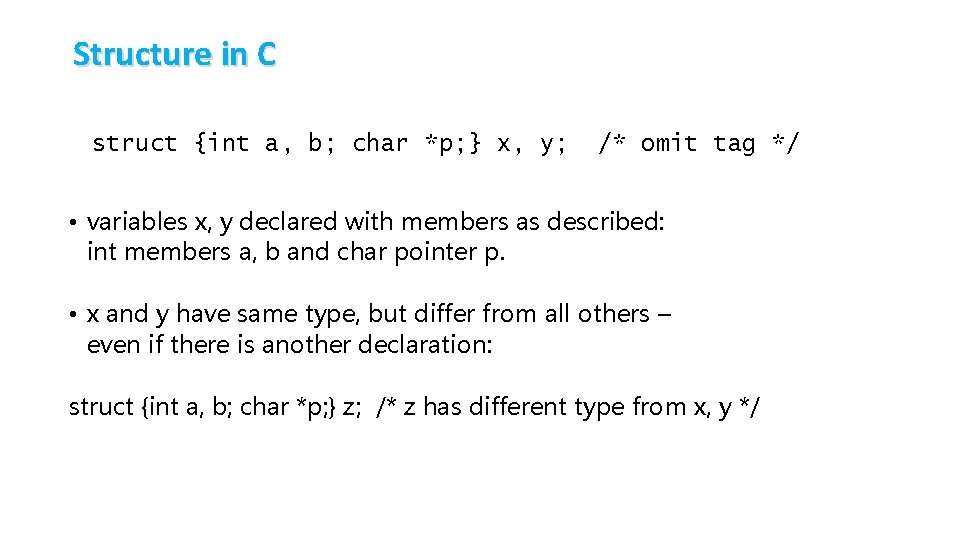
Structure in C struct {int a, b; char *p; } x, y; /* omit tag */ • variables x, y declared with members as described: int members a, b and char pointer p. • x and y have same type, but differ from all others – even if there is another declaration: struct {int a, b; char *p; } z; /* z has different type from x, y */
![include stdio h include string h struct Books char title50 char author50 char #include <stdio. h> #include <string. h> struct Books { char title[50]; char author[50]; char](https://slidetodoc.com/presentation_image/376f2a0e8c92c592b5764fe2179e07bd/image-10.jpg)
#include <stdio. h> #include <string. h> struct Books { char title[50]; char author[50]; char subject[100]; int book_id; }; int main( ) { struct Books Book 1; /* Declare Book 1 of type Book */ struct Books Book 2; /* Declare Book 2 of type Book */ /* book 1 specification */ strcpy( Book 1. title, "C Programming"); strcpy( Book 1. author, "Nuha Ali"); strcpy( Book 1. subject, "C Programming Tutorial"); Book 1. book_id = 6495407; /* book 2 specification */ strcpy( Book 2. title, "Telecom Billing"); strcpy( Book 2. author, "Zara Ali"); strcpy( Book 2. subject, "Telecom Billing Tutorial"); Book 2. book_id = 6495700;
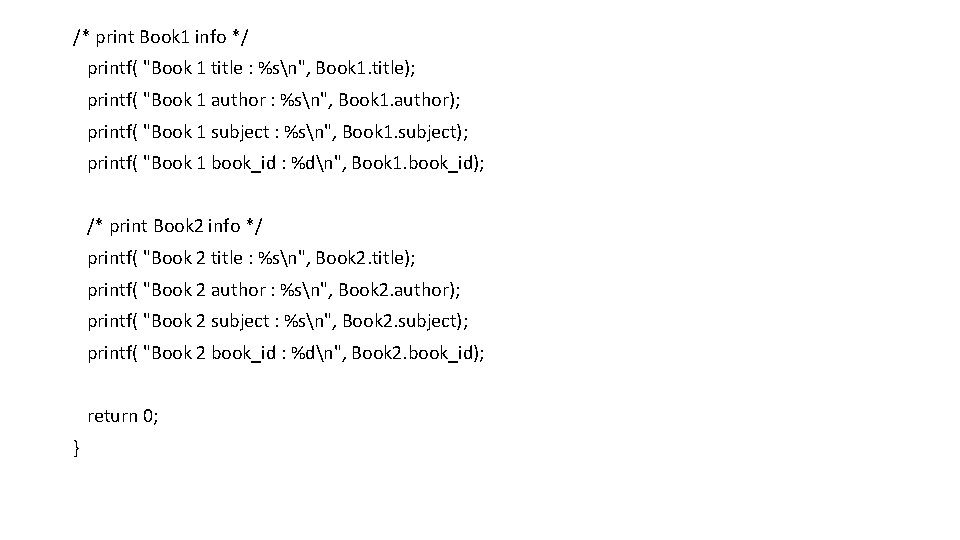
/* print Book 1 info */ printf( "Book 1 title : %sn", Book 1. title); printf( "Book 1 author : %sn", Book 1. author); printf( "Book 1 subject : %sn", Book 1. subject); printf( "Book 1 book_id : %dn", Book 1. book_id); /* print Book 2 info */ printf( "Book 2 title : %sn", Book 2. title); printf( "Book 2 author : %sn", Book 2. author); printf( "Book 2 subject : %sn", Book 2. subject); printf( "Book 2 book_id : %dn", Book 2. book_id); return 0; }
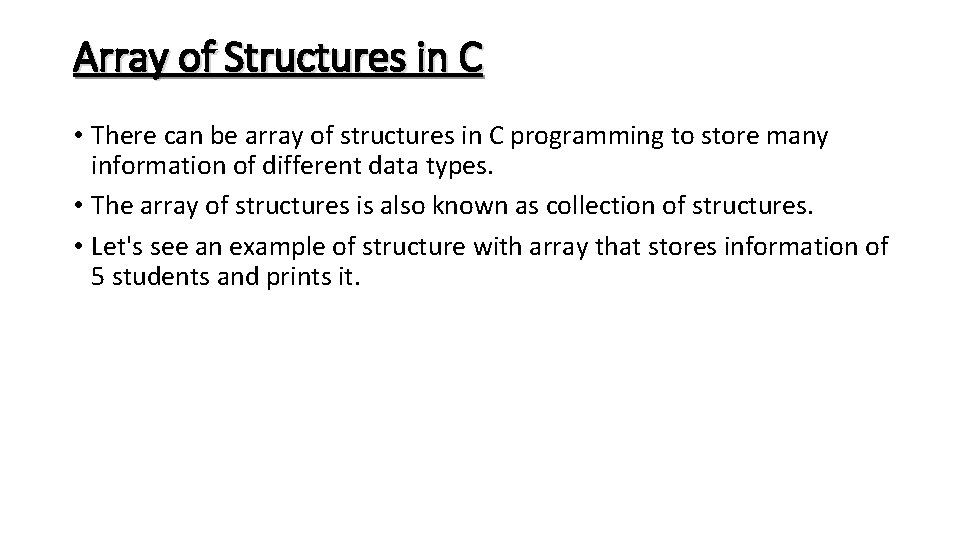
Array of Structures in C • There can be array of structures in C programming to store many information of different data types. • The array of structures is also known as collection of structures. • Let's see an example of structure with array that stores information of 5 students and prints it.
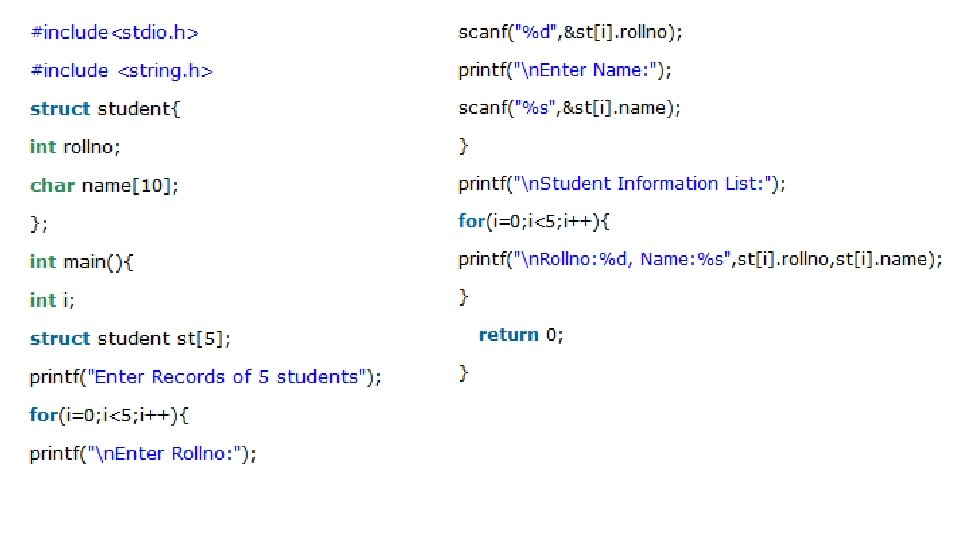
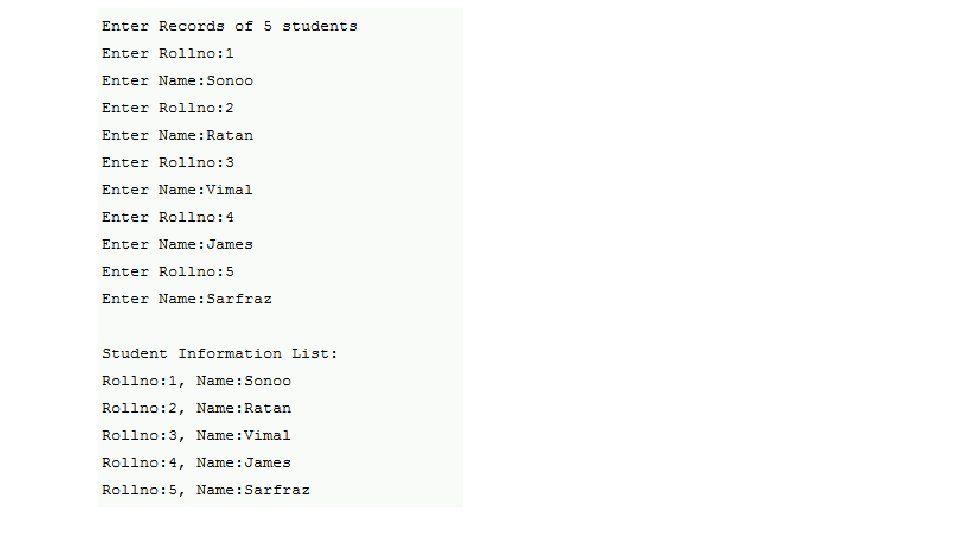
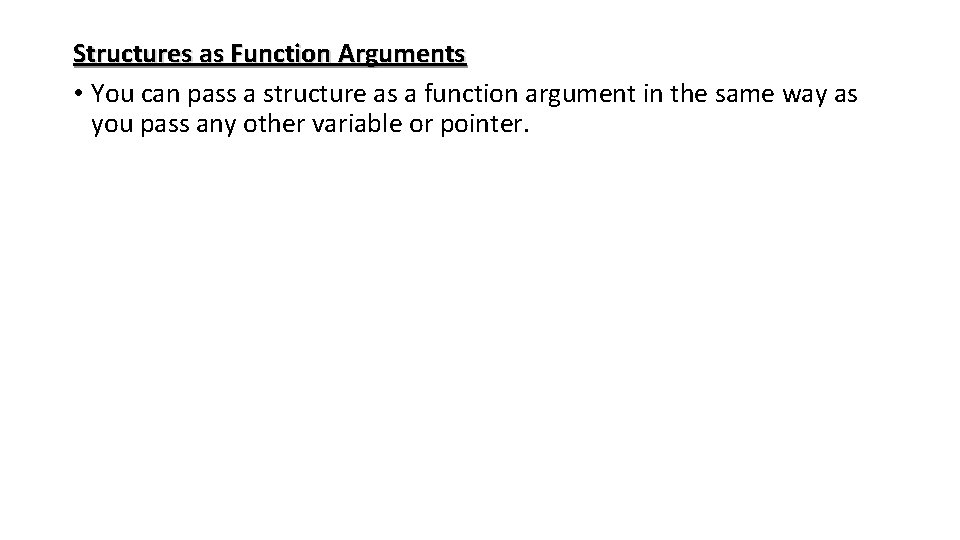
Structures as Function Arguments • You can pass a structure as a function argument in the same way as you pass any other variable or pointer.
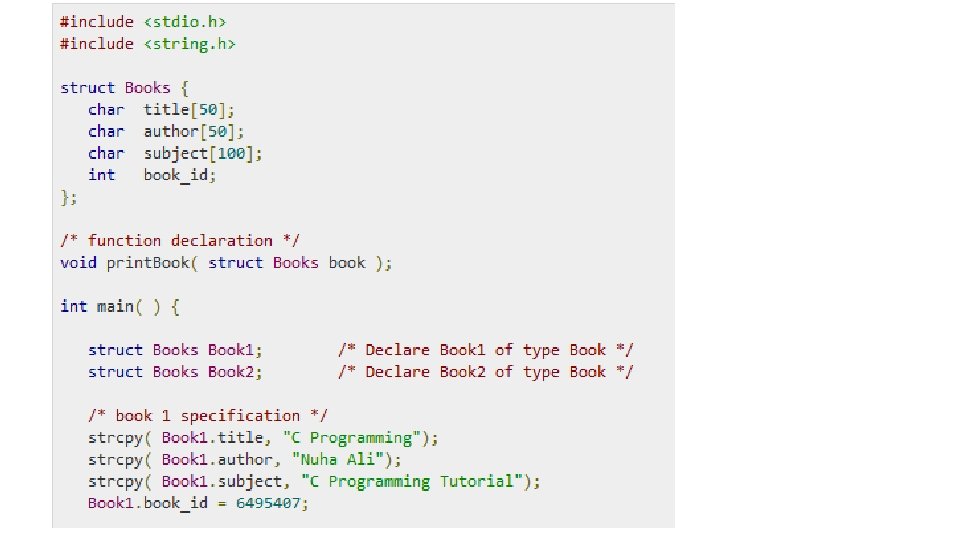
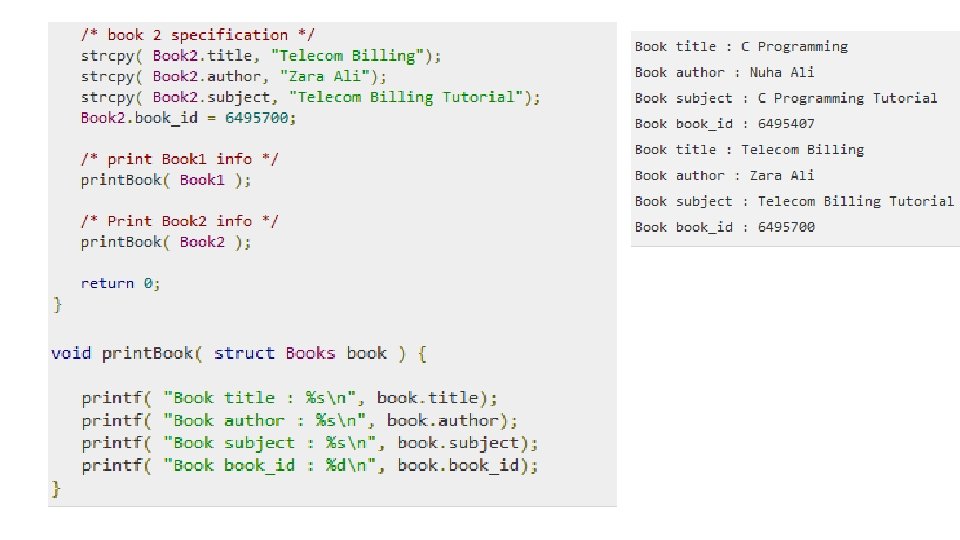
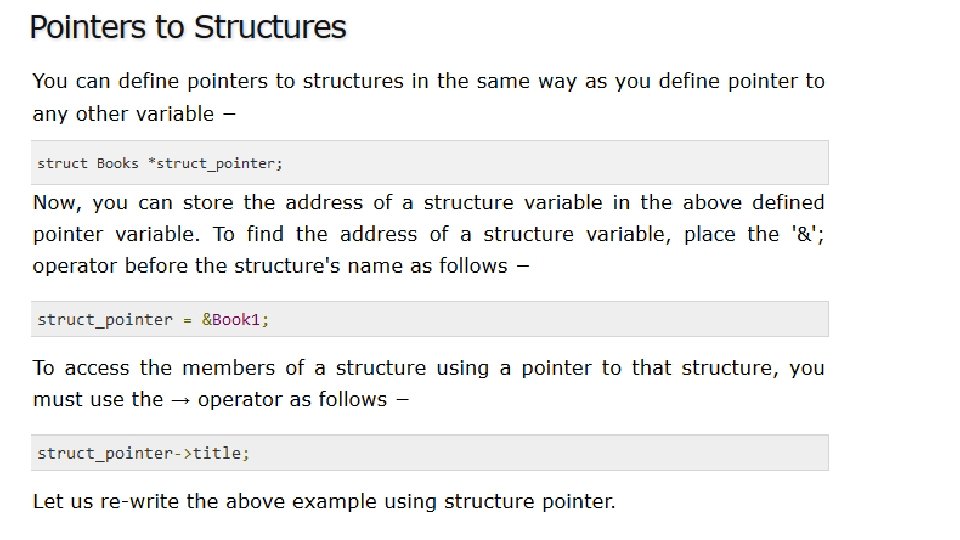
8 6 rdt
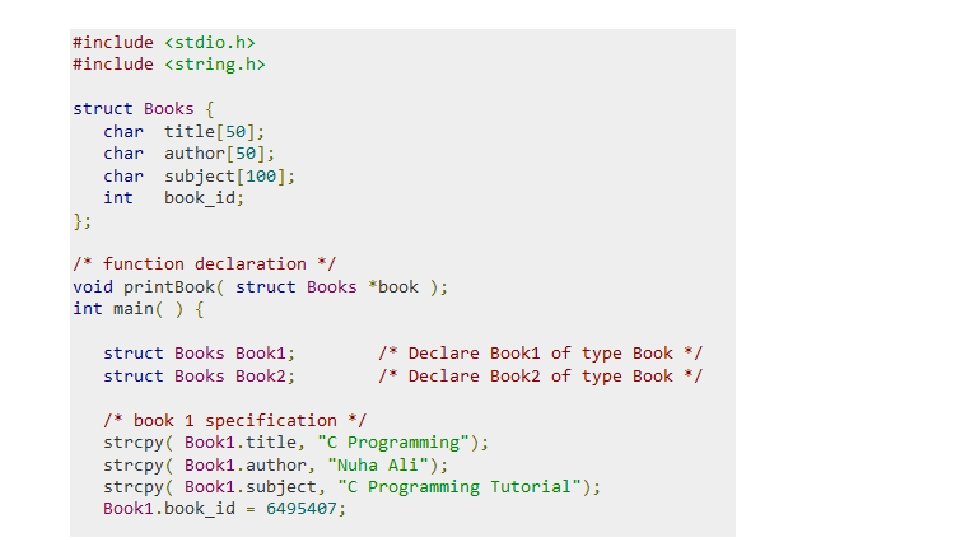
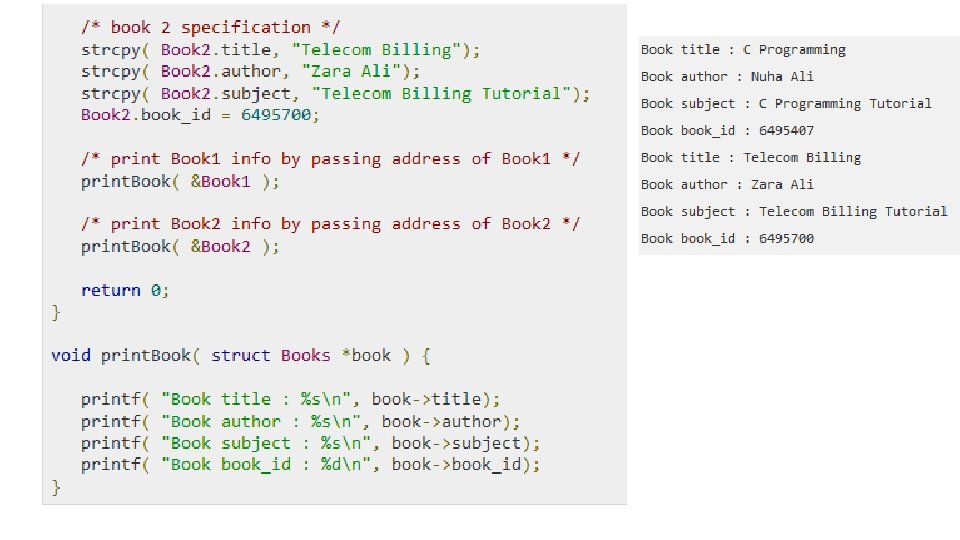
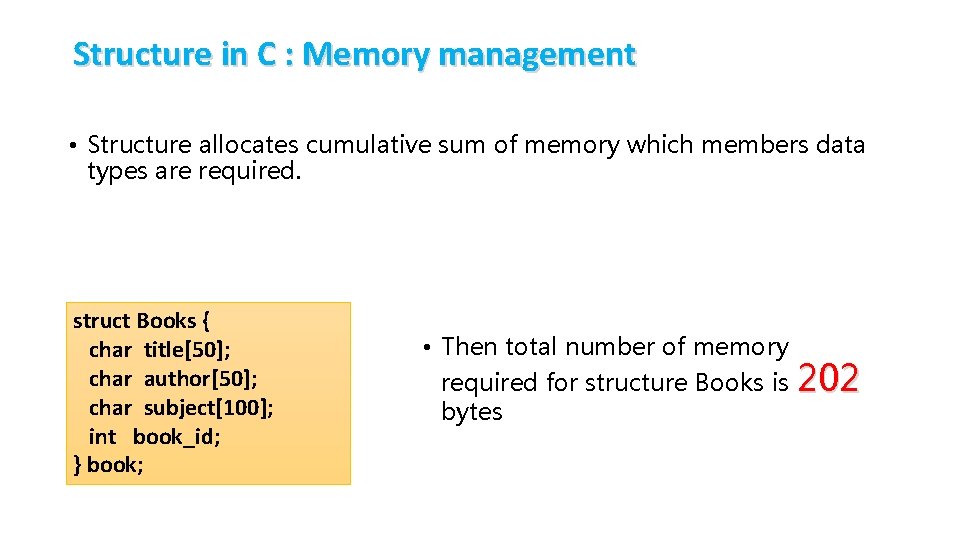
Structure in C : Memory management • Structure allocates cumulative sum of memory which members data types are required. struct Books { char title[50]; char author[50]; char subject[100]; int book_id; } book; • Then total number of memory required for structure Books is bytes 202
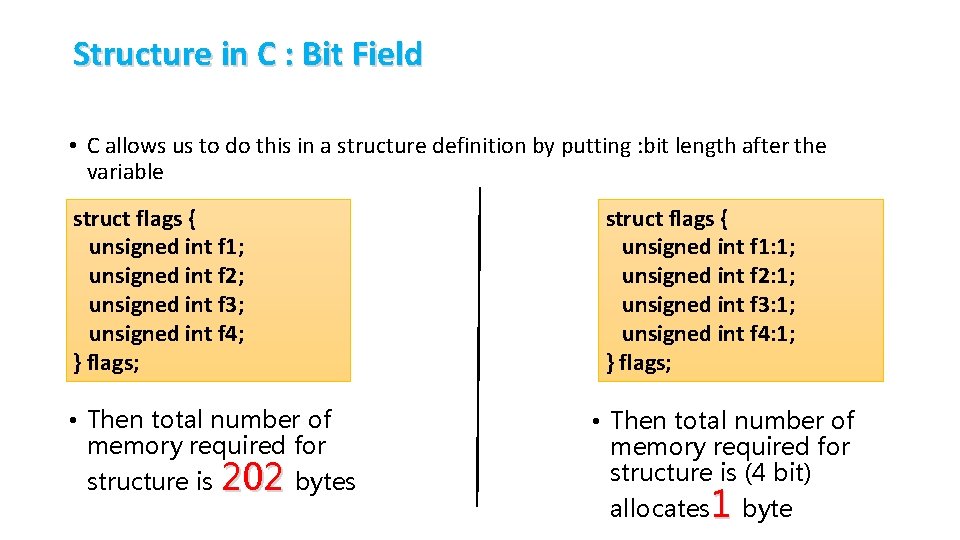
Structure in C : Bit Field • C allows us to do this in a structure definition by putting : bit length after the variable struct flags { unsigned int f 1; unsigned int f 2; unsigned int f 3; unsigned int f 4; } flags; • Then total number of memory required for structure is 202 bytes struct flags { unsigned int f 1: 1; unsigned int f 2: 1; unsigned int f 3: 1; unsigned int f 4: 1; } flags; • Then total number of memory required for structure is (4 bit) allocates 1 byte
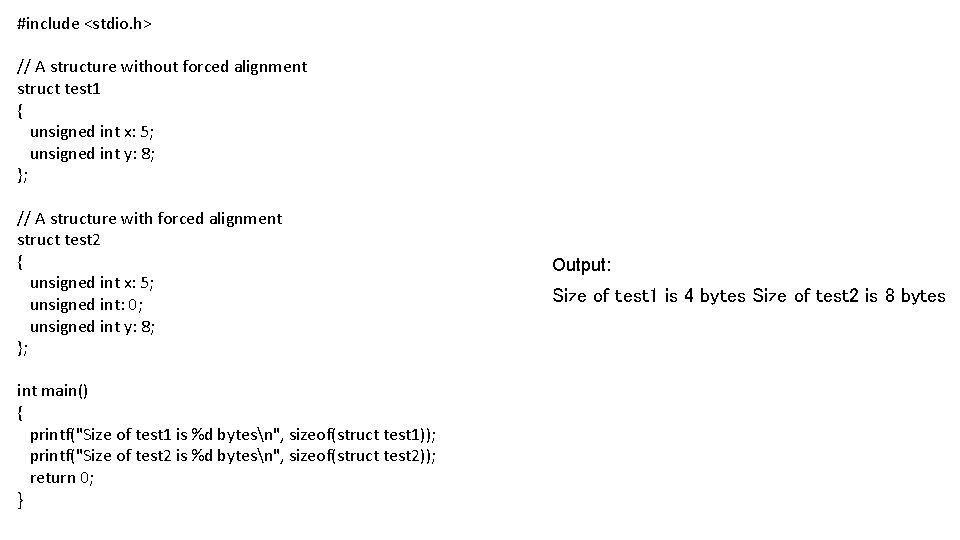
#include <stdio. h> // A structure without forced alignment struct test 1 { unsigned int x: 5; unsigned int y: 8; }; // A structure with forced alignment struct test 2 { unsigned int x: 5; unsigned int: 0; unsigned int y: 8; }; int main() { printf("Size of test 1 is %d bytesn", sizeof(struct test 1)); printf("Size of test 2 is %d bytesn", sizeof(struct test 2)); return 0; } Output: Size of test 1 is 4 bytes Size of test 2 is 8 bytes
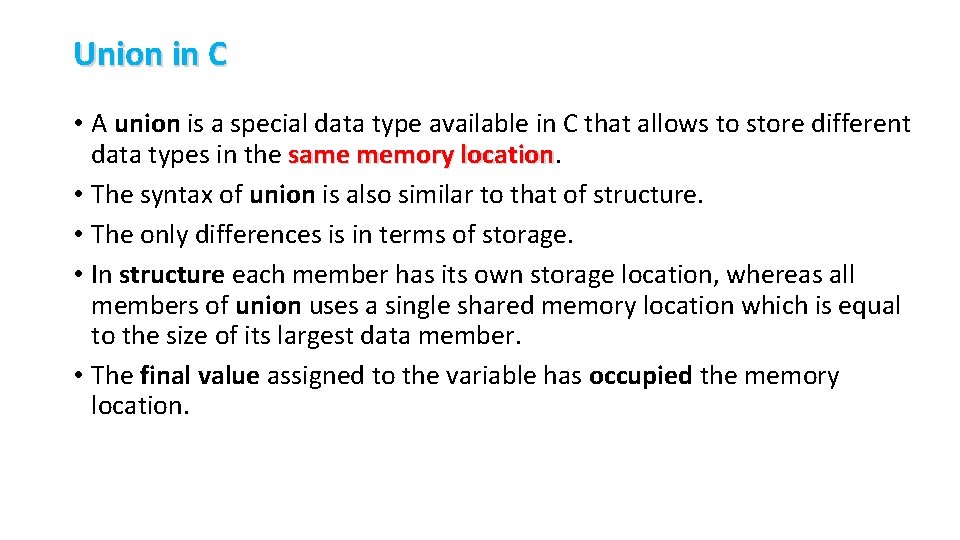
Union in C • A union is a special data type available in C that allows to store different data types in the same memory location • The syntax of union is also similar to that of structure. • The only differences is in terms of storage. • In structure each member has its own storage location, whereas all members of union uses a single shared memory location which is equal to the size of its largest data member. • The final value assigned to the variable has occupied the memory location.
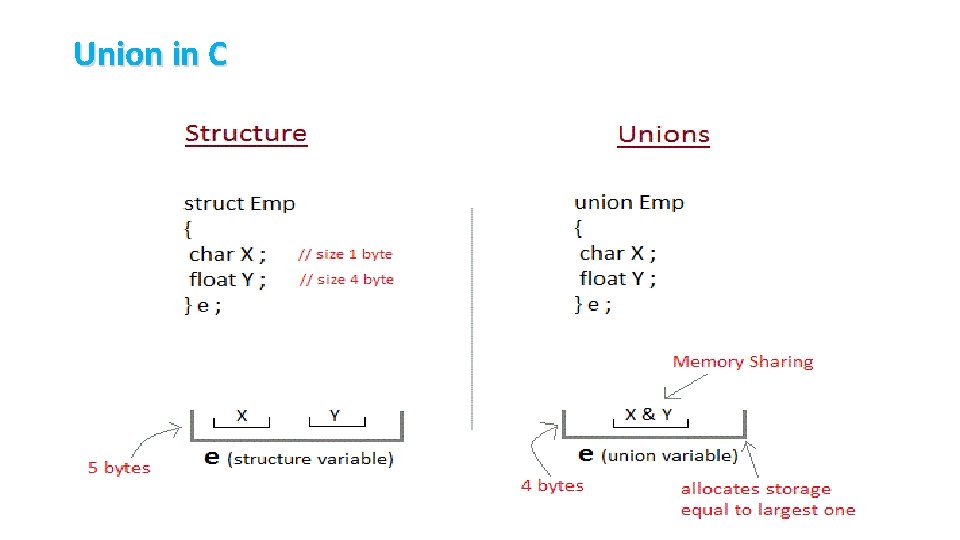
Union in C
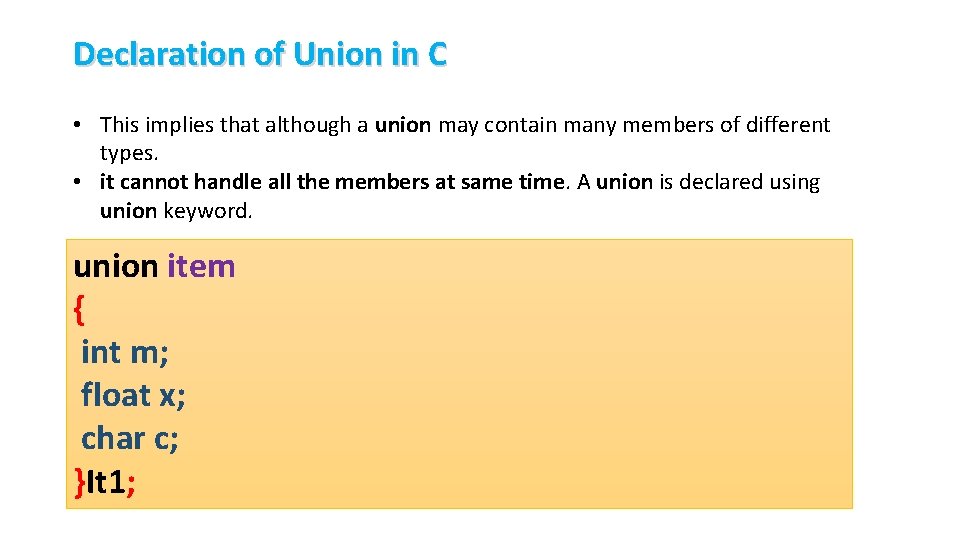
Declaration of Union in C • This implies that although a union may contain many members of different types. • it cannot handle all the members at same time. A union is declared using union keyword. union item { int m; float x; char c; }It 1;
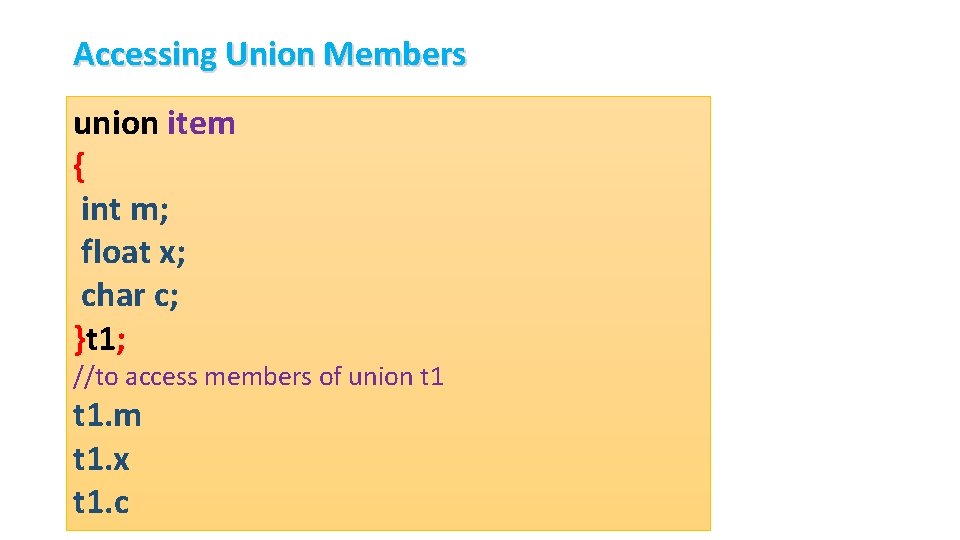
Accessing Union Members union item { int m; float x; char c; }t 1; //to access members of union t 1. m t 1. x t 1. c
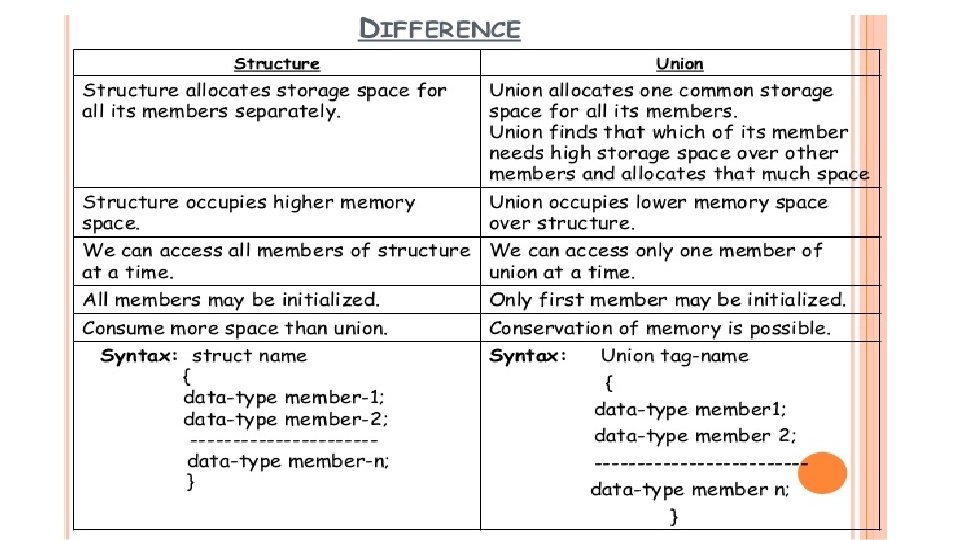
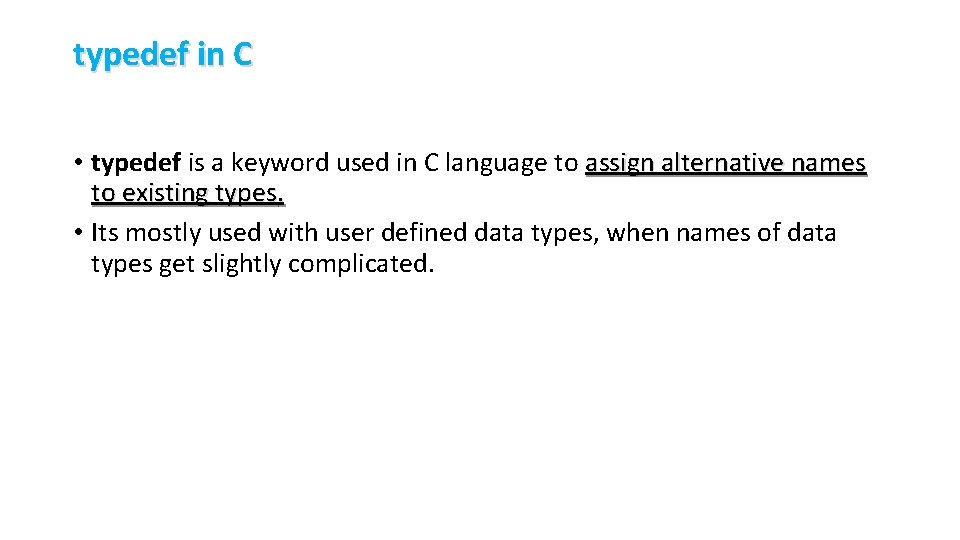
typedef in C • typedef is a keyword used in C language to assign alternative names to existing types. • Its mostly used with user defined data types, when names of data types get slightly complicated.
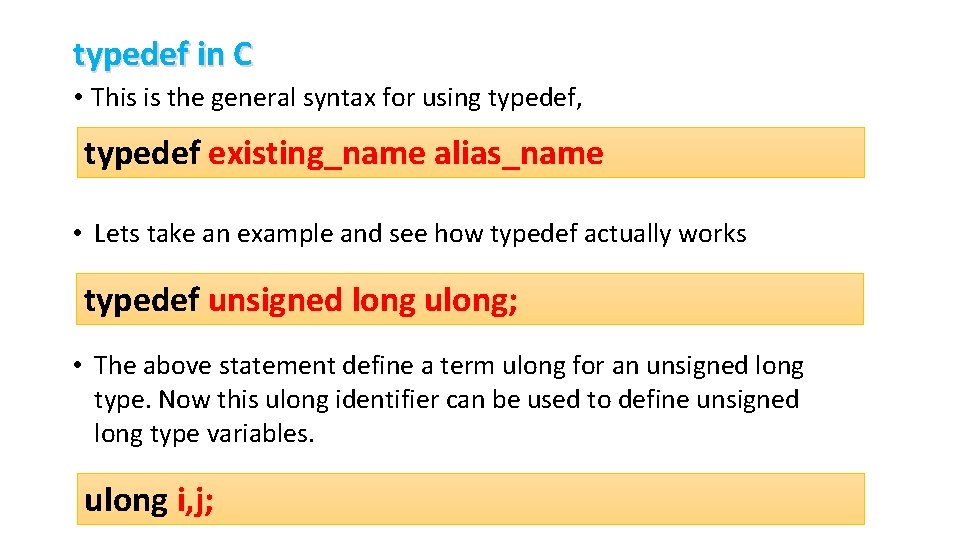
typedef in C • This is the general syntax for using typedef, typedef existing_name alias_name • Lets take an example and see how typedef actually works typedef unsigned long ulong; • The above statement define a term ulong for an unsigned long type. Now this ulong identifier can be used to define unsigned long type variables. ulong i, j;
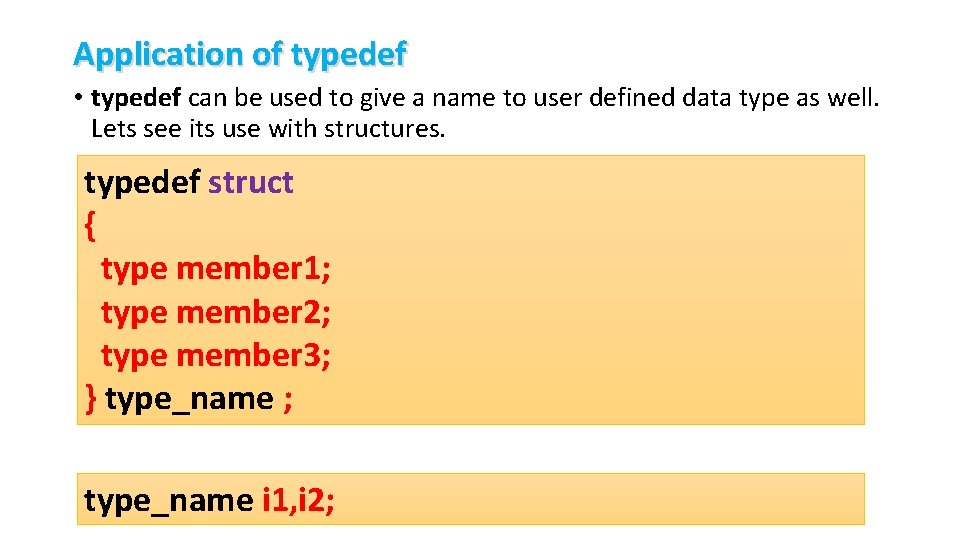
Application of typedef • typedef can be used to give a name to user defined data type as well. Lets see its use with structures. typedef struct { type member 1; type member 2; type member 3; } type_name ; type_name i 1, i 2;
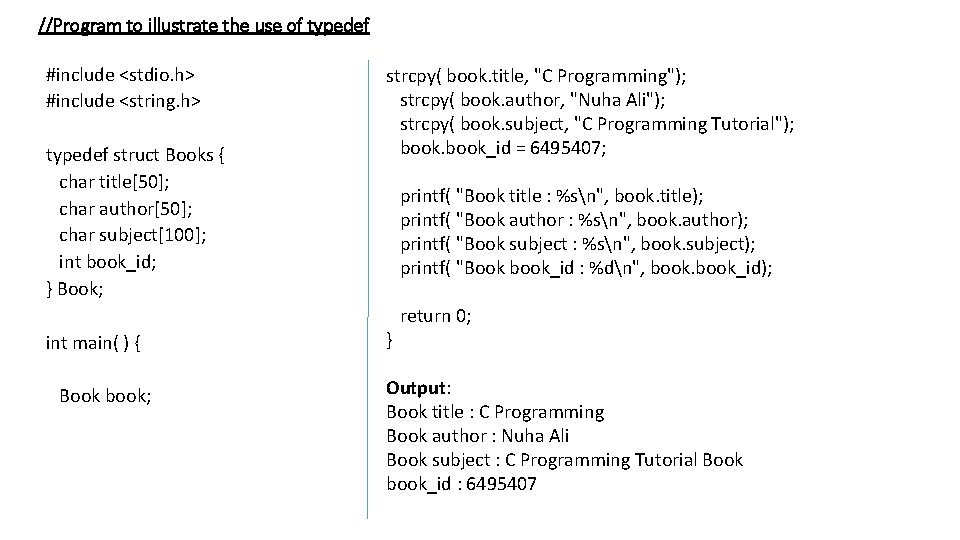
//Program to illustrate the use of typedef #include <stdio. h> #include <string. h> typedef struct Books { char title[50]; char author[50]; char subject[100]; int book_id; } Book; int main( ) { strcpy( book. title, "C Programming"); strcpy( book. author, "Nuha Ali"); strcpy( book. subject, "C Programming Tutorial"); book_id = 6495407; printf( "Book title : %sn", book. title); printf( "Book author : %sn", book. author); printf( "Book subject : %sn", book. subject); printf( "Book book_id : %dn", book_id); Book book; Output: Book title : C Programming Book author : Nuha Ali Book subject : C Programming Tutorial Book book_id : 6495407 return 0; }
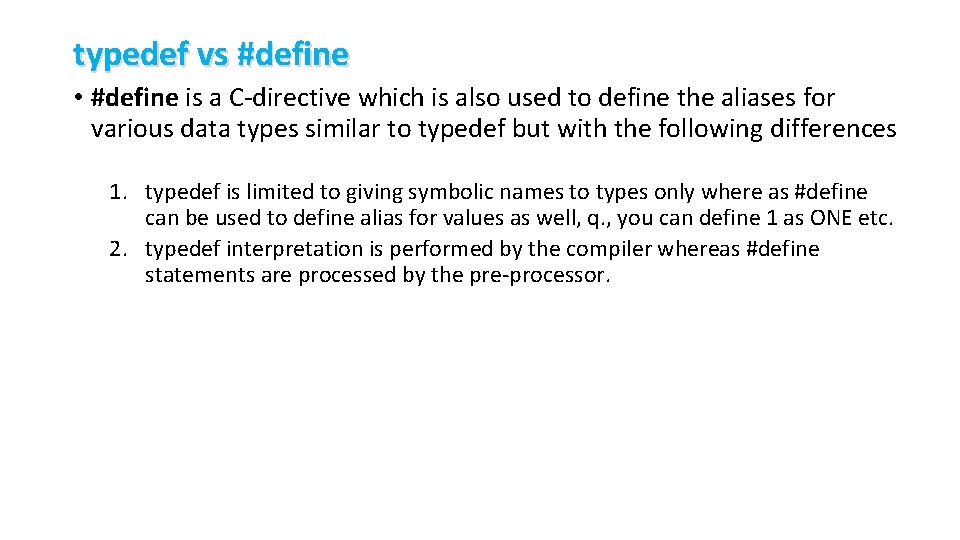
typedef vs #define • #define is a C-directive which is also used to define the aliases for various data types similar to typedef but with the following differences 1. typedef is limited to giving symbolic names to types only where as #define can be used to define alias for values as well, q. , you can define 1 as ONE etc. 2. typedef interpretation is performed by the compiler whereas #define statements are processed by the pre-processor.
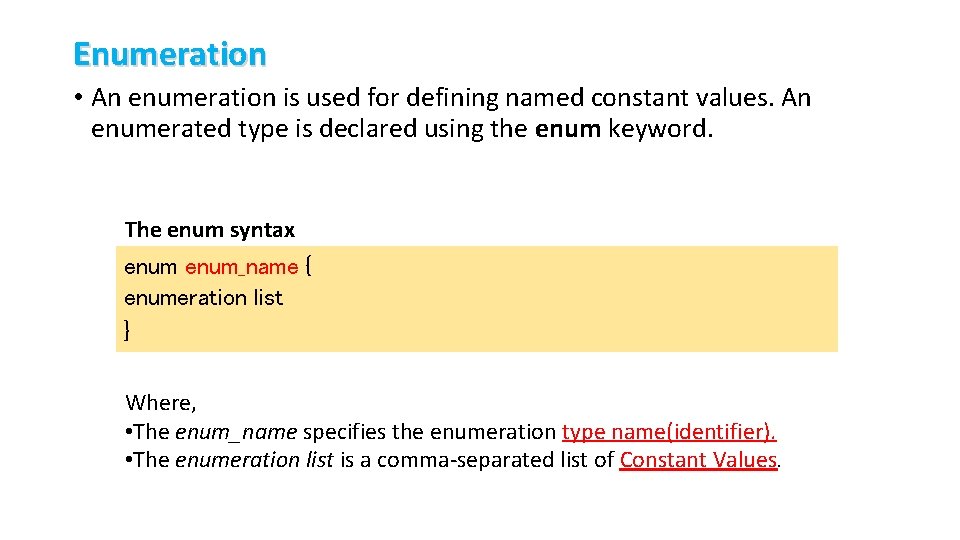
Enumeration • An enumeration is used for defining named constant values. An enumerated type is declared using the enum keyword. The enum syntax enum_name { enumeration list } Where, • The enum_name specifies the enumeration type name(identifier). • The enumeration list is a comma-separated list of Constant Values.
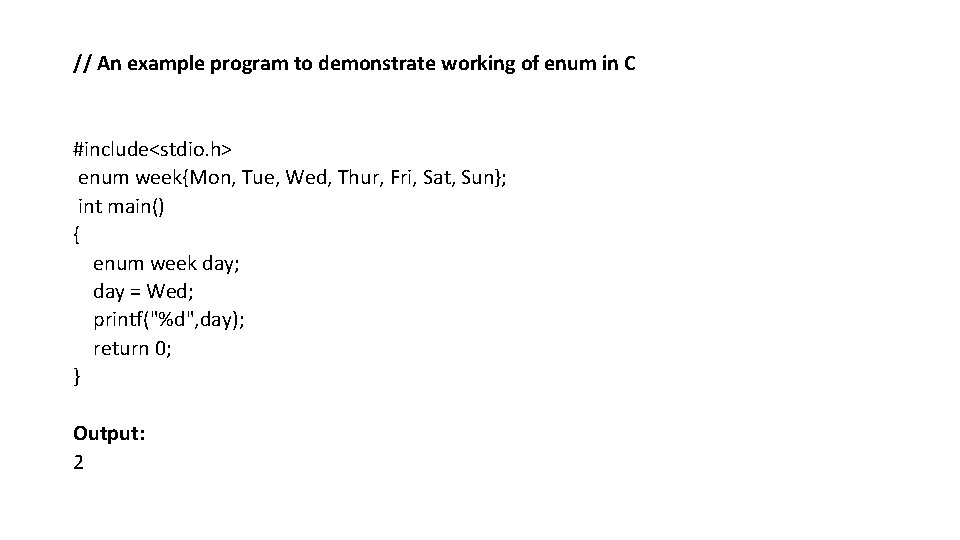
// An example program to demonstrate working of enum in C #include<stdio. h> enum week{Mon, Tue, Wed, Thur, Fri, Sat, Sun}; int main() { enum week day; day = Wed; printf("%d", day); return 0; } Output: 2
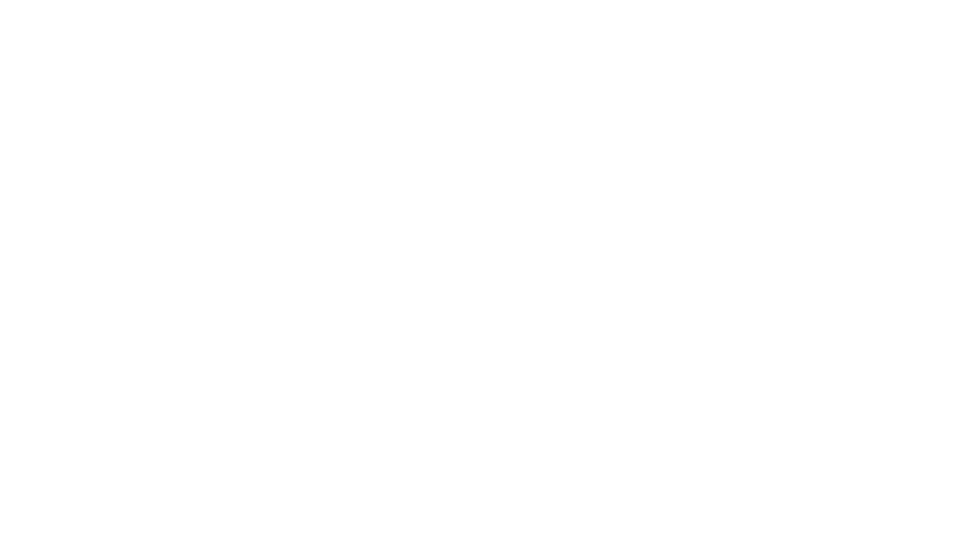
Amol shukla
Cse 340 principles of programming languages
Iat 265
Introduction to programming languages
Lisp_q
Vineeth kashyap
Reasons for studying concepts of programming languages
Alternative programming languages
Middle level programming languages
Programming languages
Elsa gunter uiuc
Types of programming languages
Storage management in programming languages
Real time programming language
Plc coding language
Programming languages
Cornell programming languages
Strongly typed vs weakly typed
Programming languages flowchart
Attribute grammar in principles of programming languages
Real time example of multithreading in java
Advantages of high level language
Xenia programming languages
Xkcd programming
Procedural programming languages
Programming languages
Cs 421 uiuc
Transmission programming languages
Cxc it
Brief history of programming languages
Mainstream programming languages
If programming languages were cars
Comparative programming languages
Low level linux programming
Programming languages
Real-time systems and programming languages
Fundamentals of functional programming language