ECE 408 Fall 2016 Applied Parallel Programming Lecture
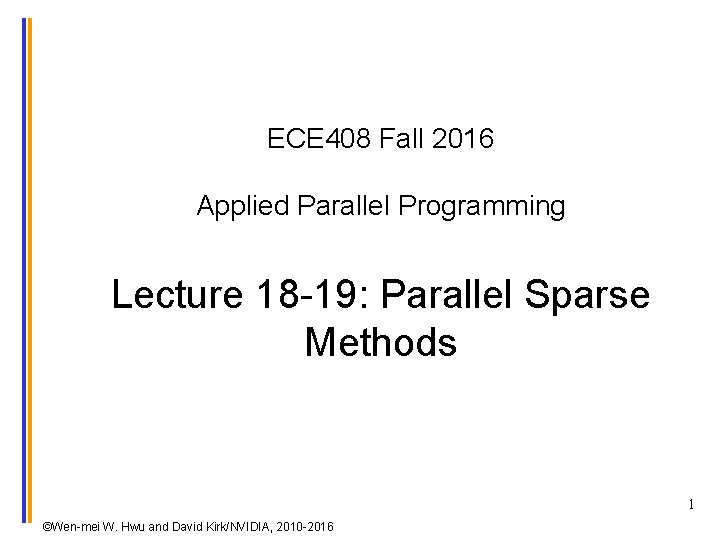
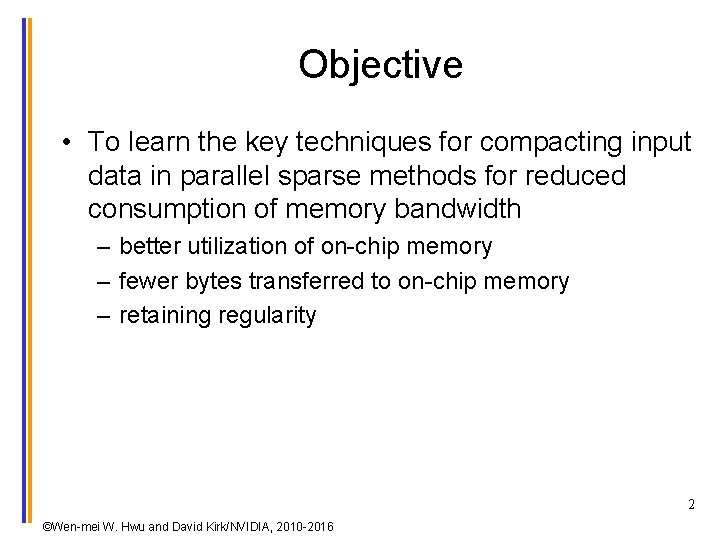
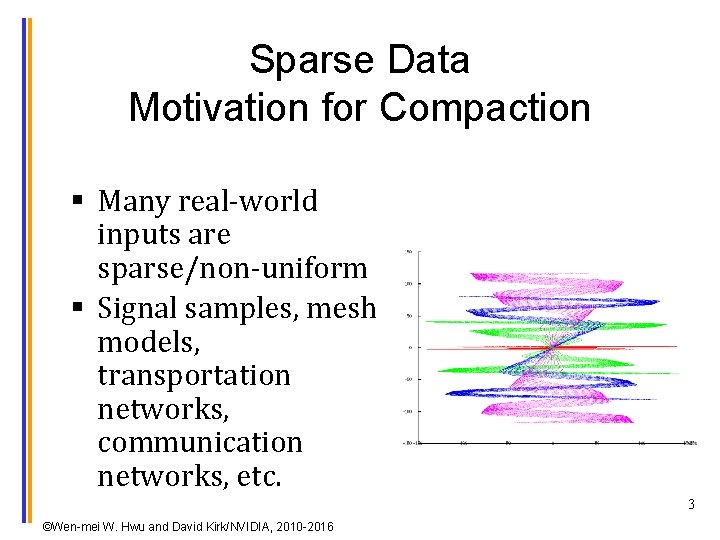
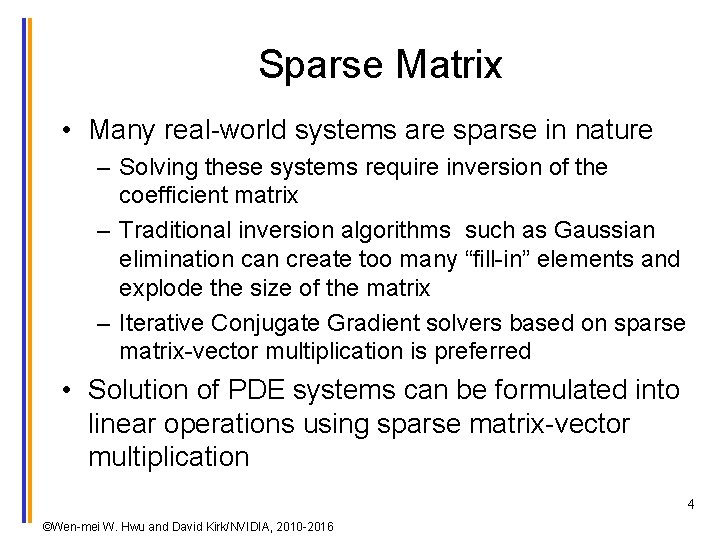
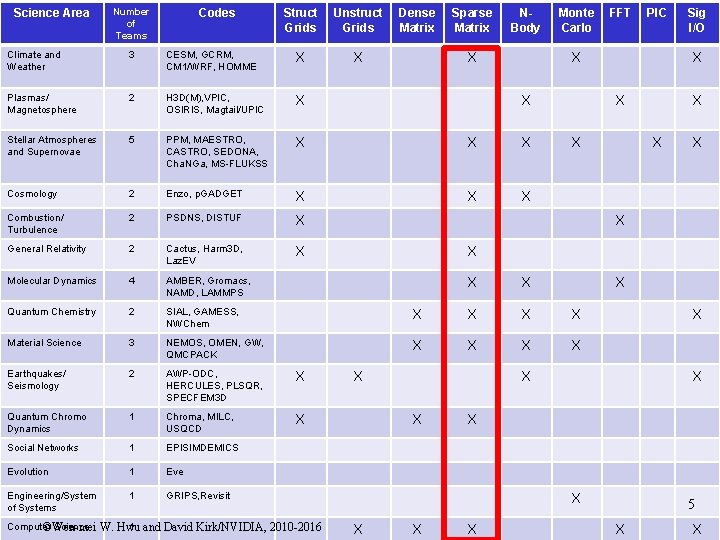
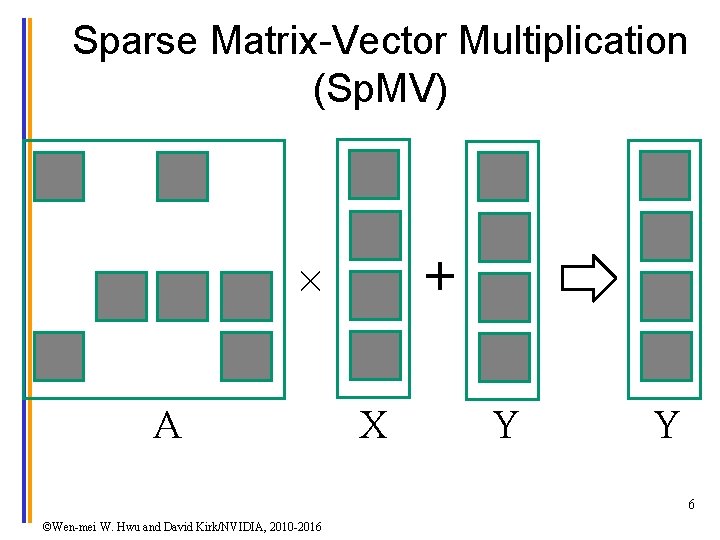
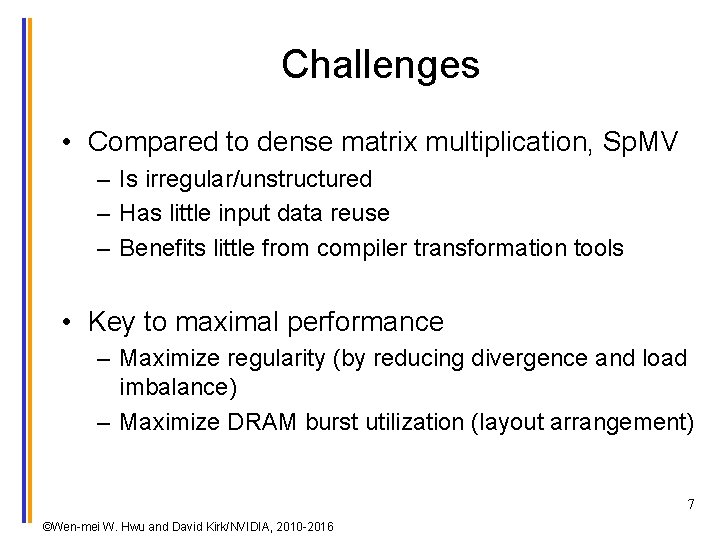
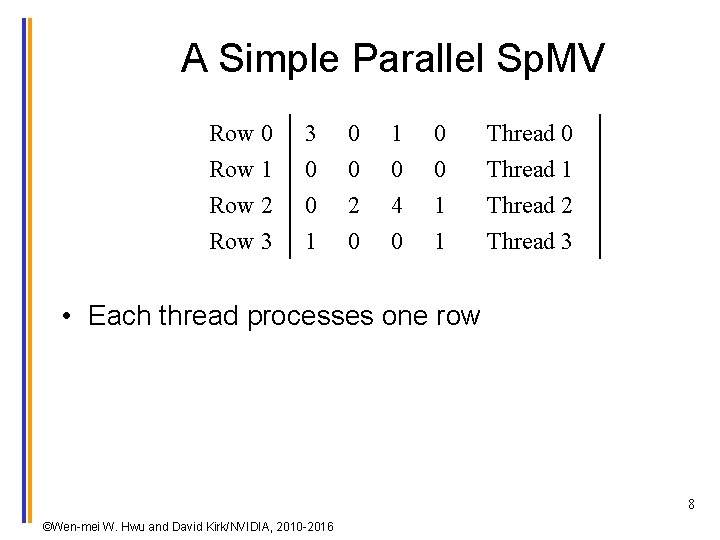
![Compressed Sparse Row (CSR) Format Row 0 Row 2 Nonzero values data[7] { 3, Compressed Sparse Row (CSR) Format Row 0 Row 2 Nonzero values data[7] { 3,](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-9.jpg)
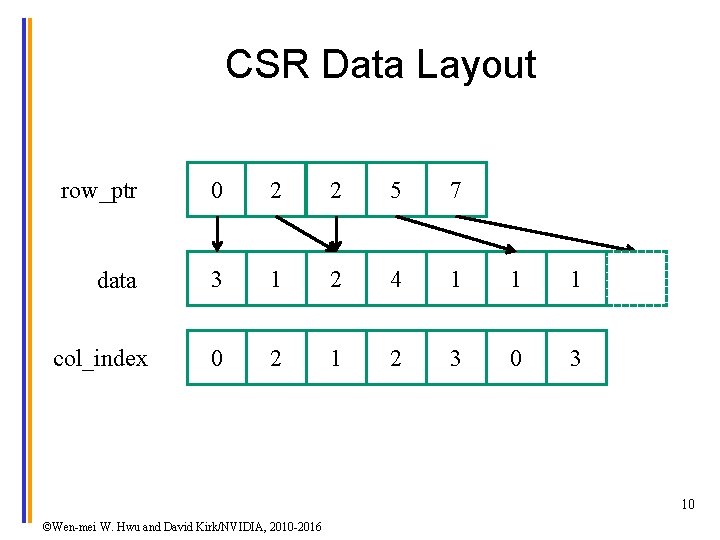
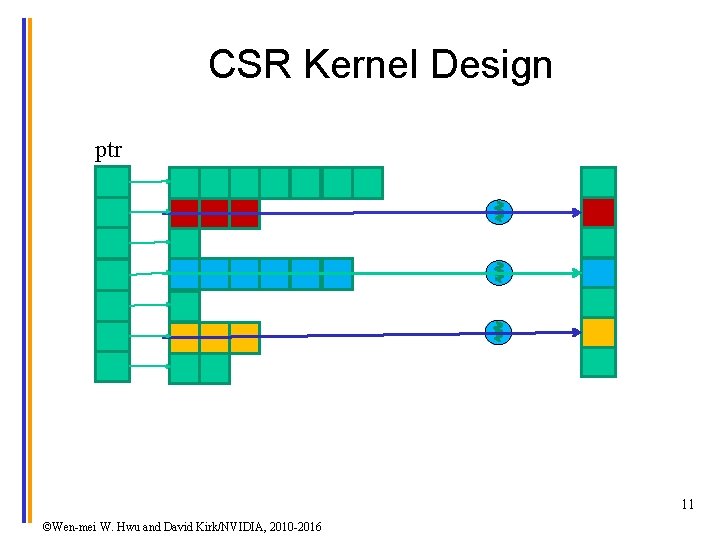
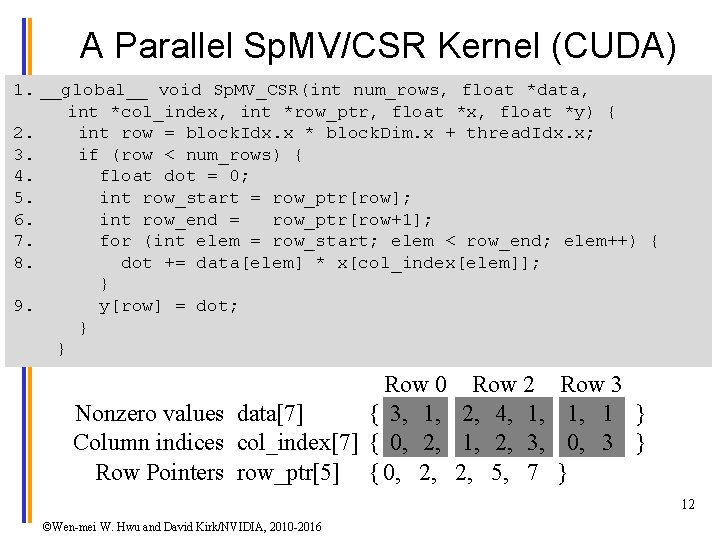
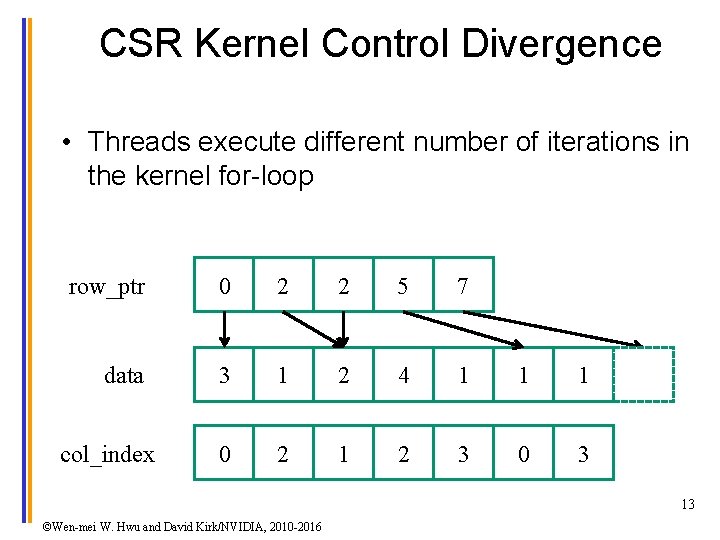
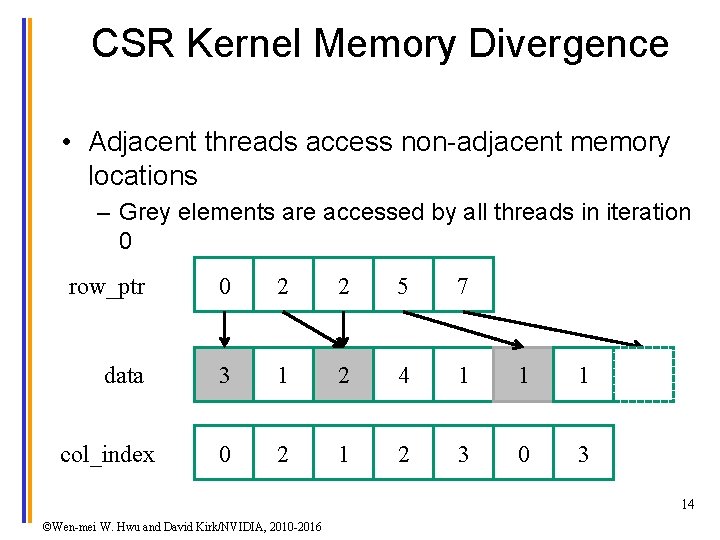
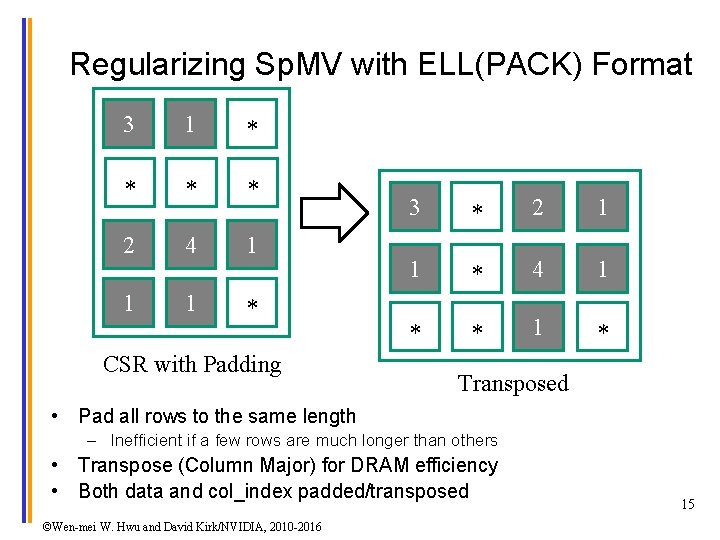
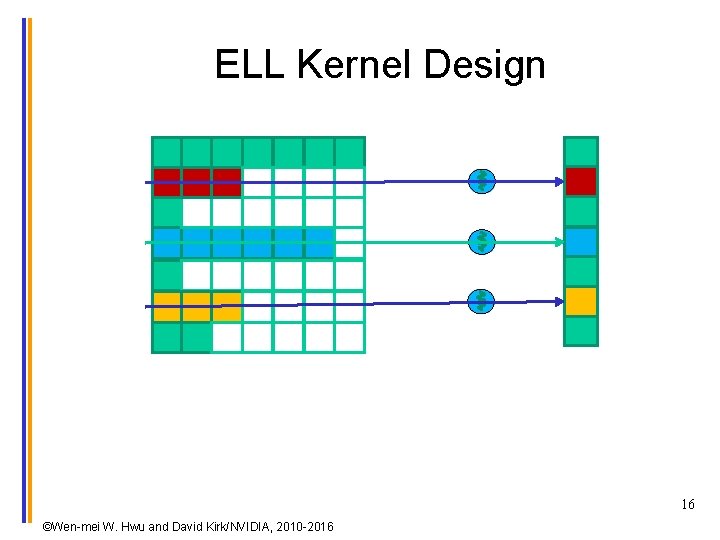
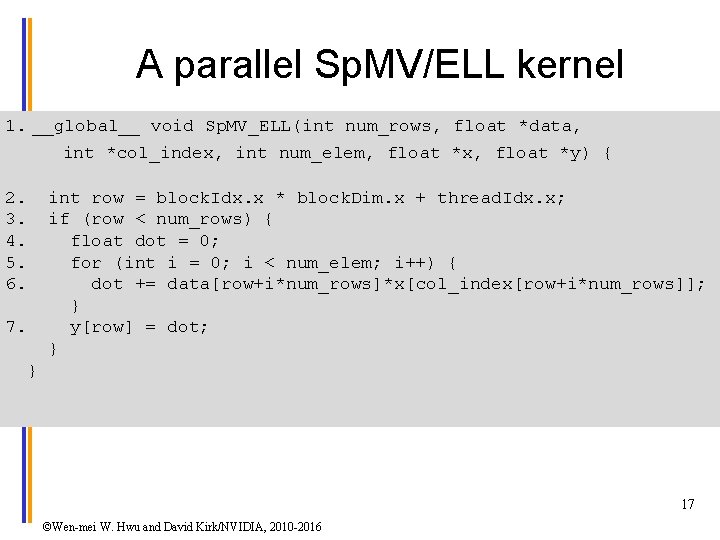
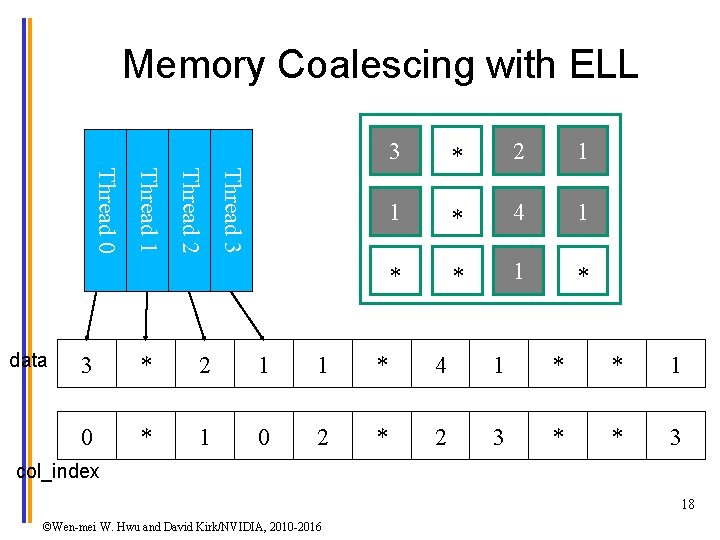
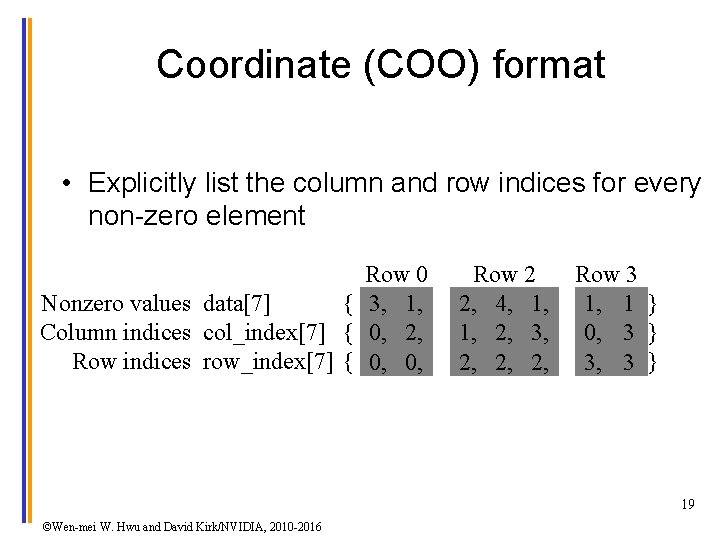
![COO Allows Reordering of Elements Row 0 Nonzero values data[7] { 3, 1, Column COO Allows Reordering of Elements Row 0 Nonzero values data[7] { 3, 1, Column](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-20.jpg)
![1. 2. for (int i = 0; i < num_elem; row++) y[row_index[i]] += data[i] 1. 2. for (int i = 0; i < num_elem; row++) y[row_index[i]] += data[i]](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-21.jpg)
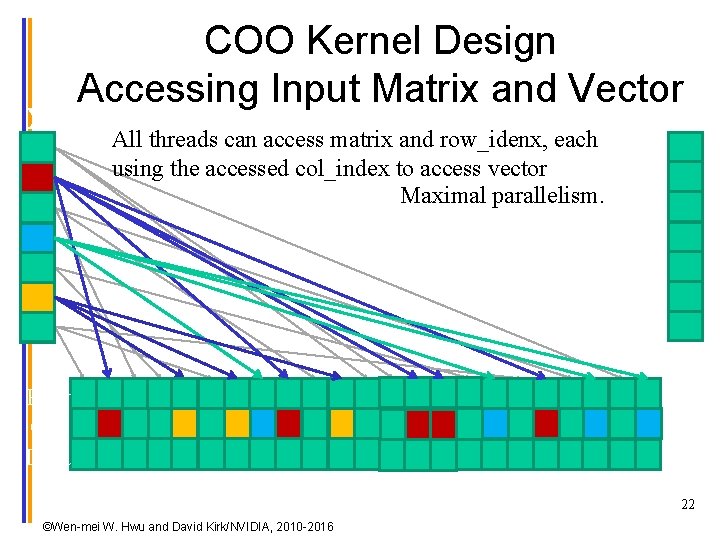
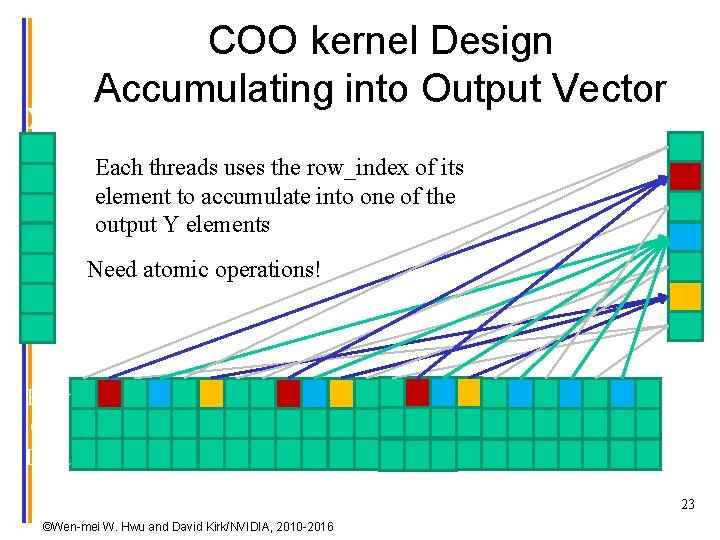
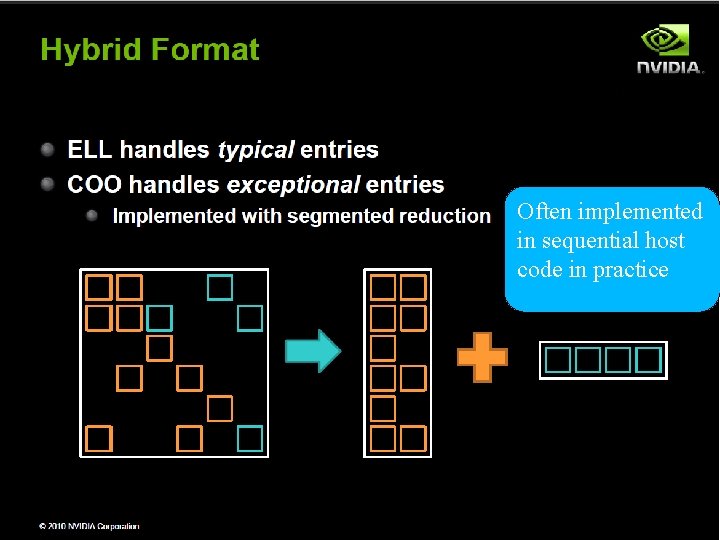
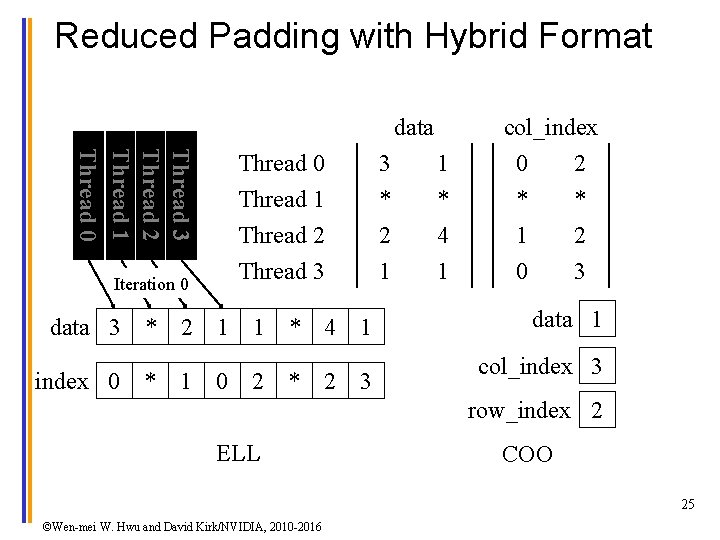
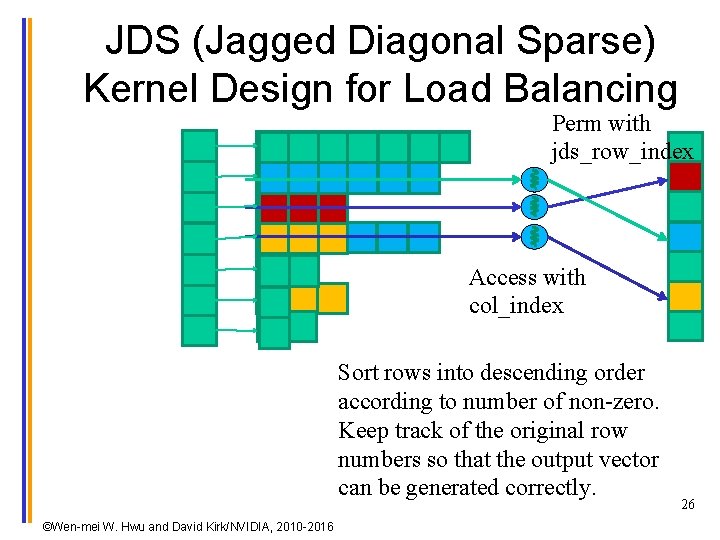
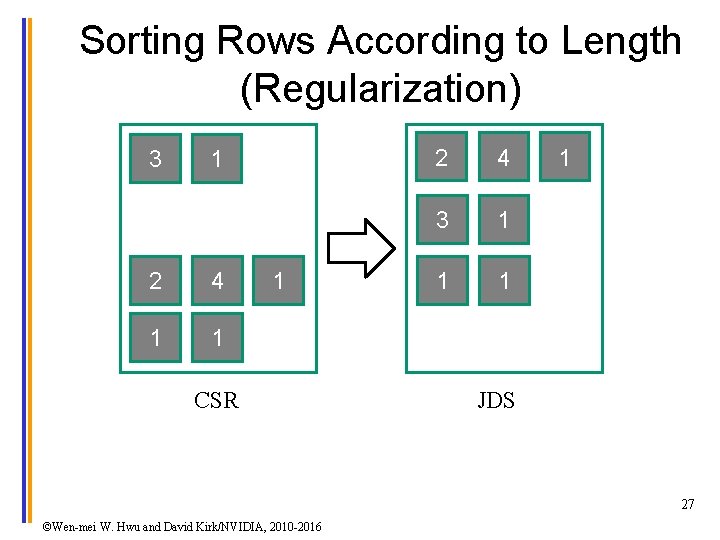
![CSR to JDS Conversion Nonzero values data[7] Column indices col_index[7] Row Pointers row_ptr[5] Row CSR to JDS Conversion Nonzero values data[7] Column indices col_index[7] Row Pointers row_ptr[5] Row](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-28.jpg)
![JDS Summary Nonzero values data[7] { 2, 4, 1, 3, 1, 1, 1 } JDS Summary Nonzero values data[7] { 2, 4, 1, 3, 1, 1, 1 }](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-29.jpg)
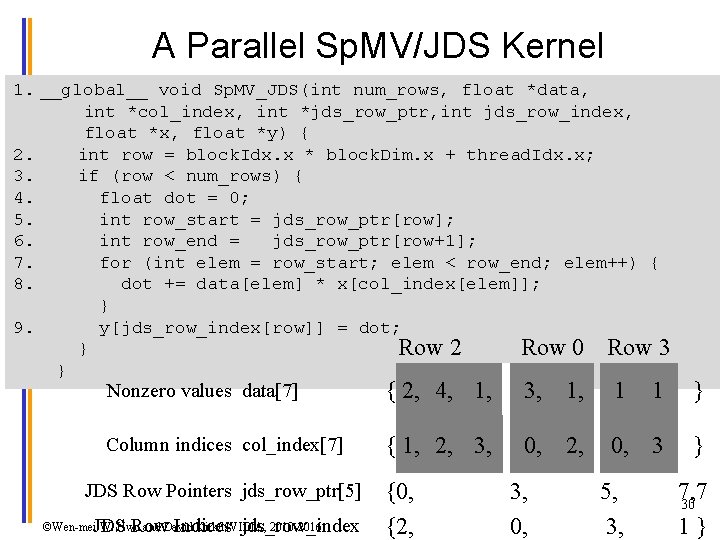
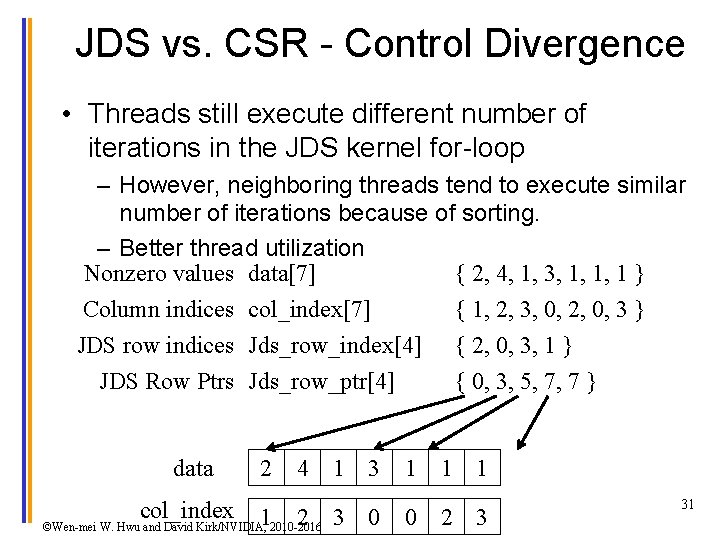
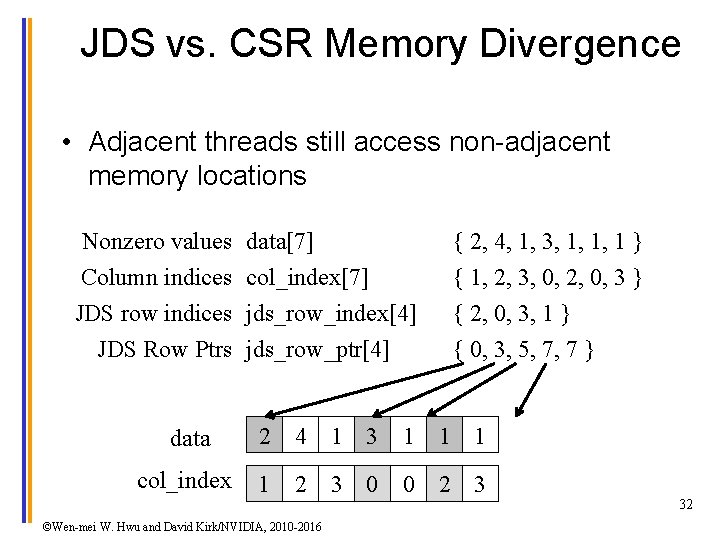
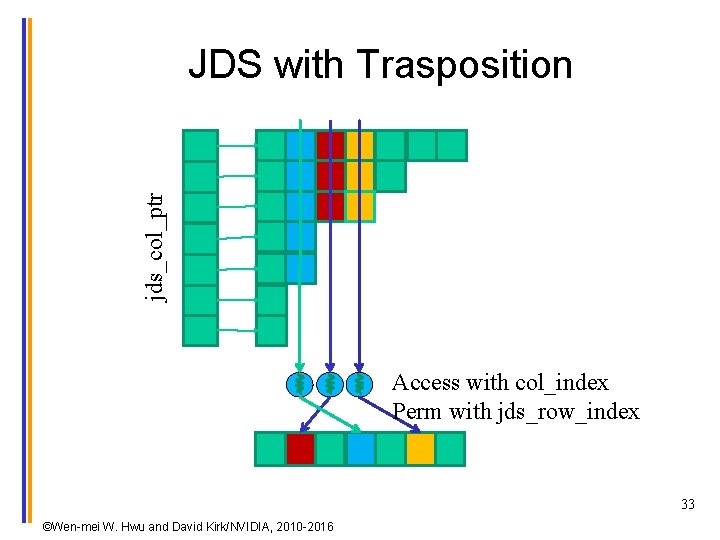
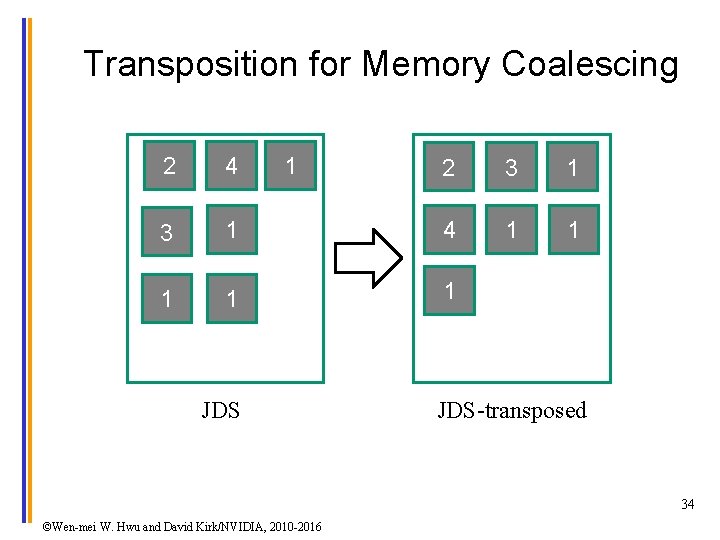
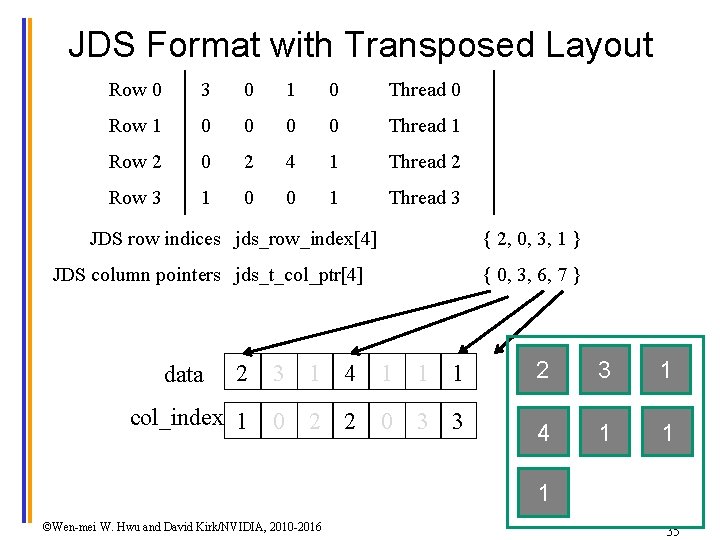
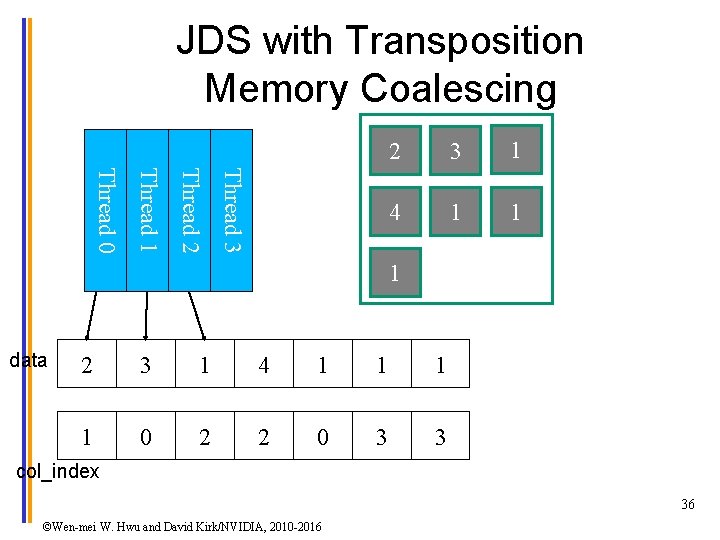
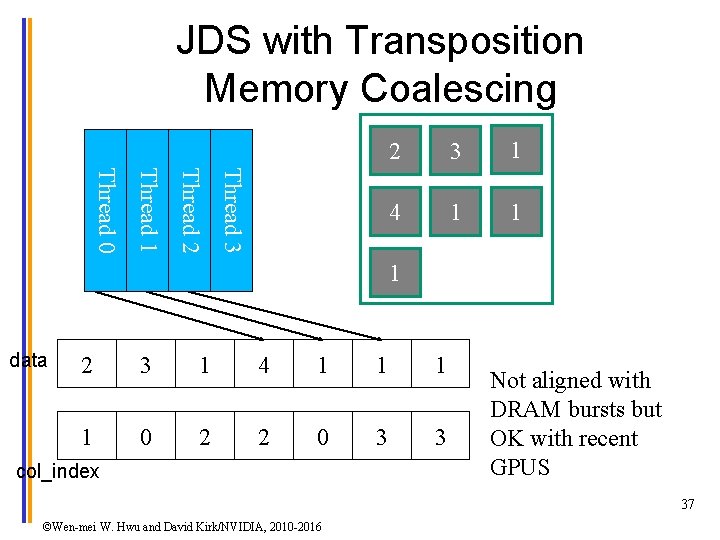
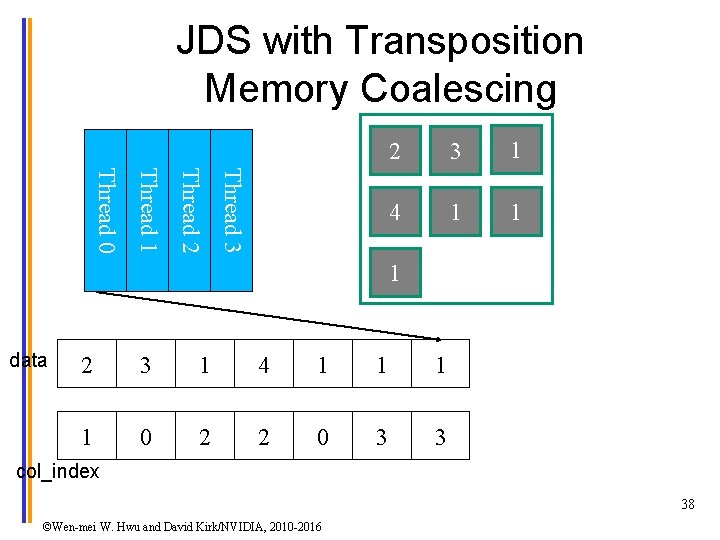
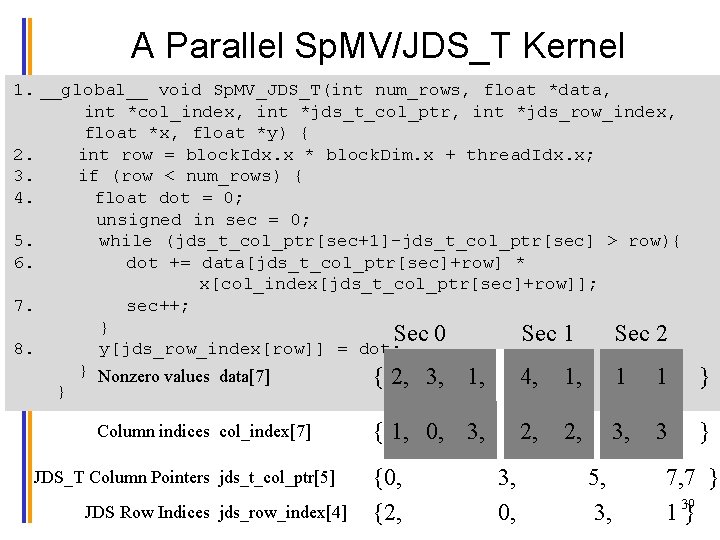
- Slides: 39
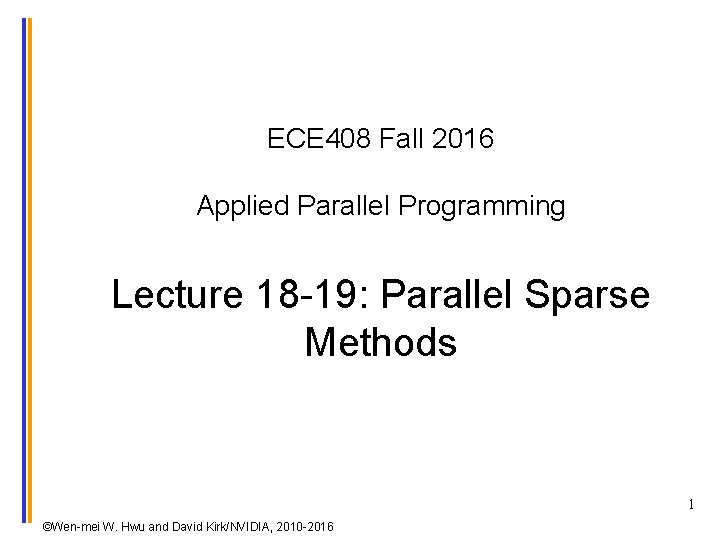
ECE 408 Fall 2016 Applied Parallel Programming Lecture 18 -19: Parallel Sparse Methods 1 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
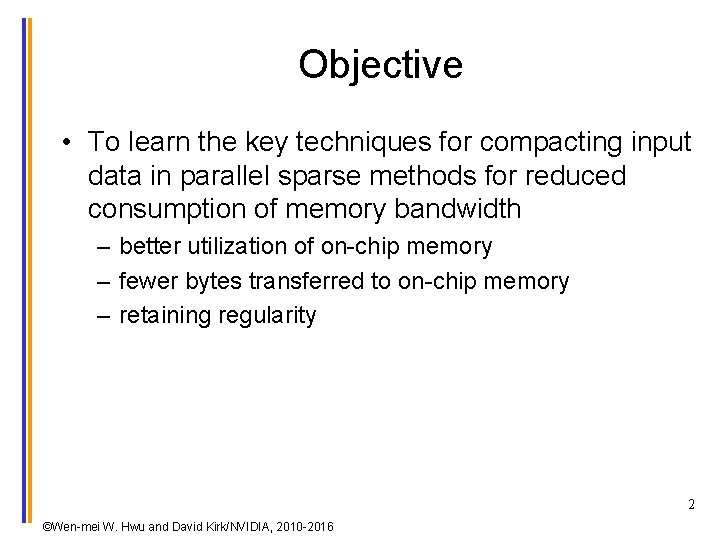
Objective • To learn the key techniques for compacting input data in parallel sparse methods for reduced consumption of memory bandwidth – better utilization of on-chip memory – fewer bytes transferred to on-chip memory – retaining regularity 2 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
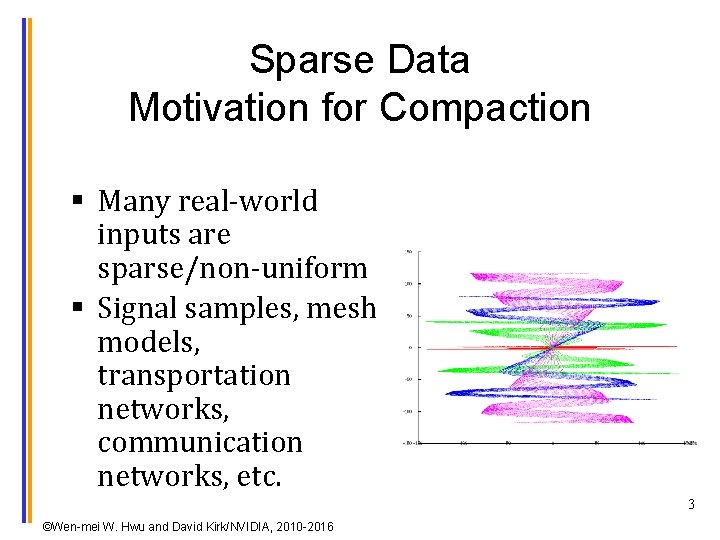
Sparse Data Motivation for Compaction § Many real-world inputs are sparse/non-uniform § Signal samples, mesh models, transportation networks, communication networks, etc. 3 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
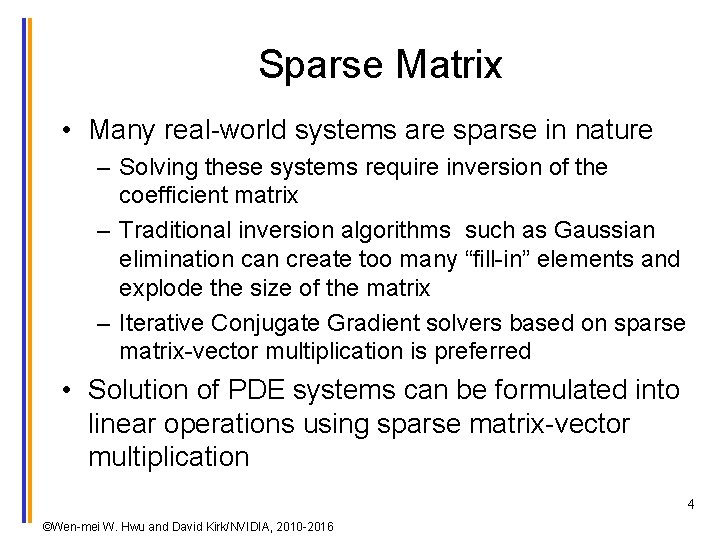
Sparse Matrix • Many real-world systems are sparse in nature – Solving these systems require inversion of the coefficient matrix – Traditional inversion algorithms such as Gaussian elimination can create too many “fill-in” elements and explode the size of the matrix – Iterative Conjugate Gradient solvers based on sparse matrix-vector multiplication is preferred • Solution of PDE systems can be formulated into linear operations using sparse matrix-vector multiplication 4 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
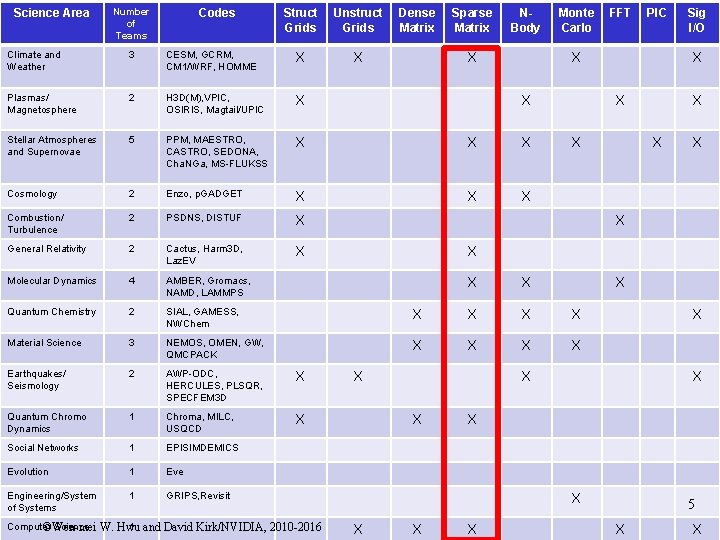
Science Area Number of Teams Codes Struct Grids Unstruct Grids X Dense Matrix Sparse Matrix NBody Climate and Weather 3 CESM, GCRM, CM 1/WRF, HOMME X Plasmas/ Magnetosphere 2 H 3 D(M), VPIC, OSIRIS, Magtail/UPIC X Stellar Atmospheres and Supernovae 5 PPM, MAESTRO, CASTRO, SEDONA, Cha. NGa, MS-FLUKSS X X X Cosmology 2 Enzo, p. GADGET X X X Combustion/ Turbulence 2 PSDNS, DISTUF X General Relativity 2 Cactus, Harm 3 D, Laz. EV X Molecular Dynamics 4 AMBER, Gromacs, NAMD, LAMMPS Quantum Chemistry 2 SIAL, GAMESS, NWChem Material Science 3 NEMOS, OMEN, GW, QMCPACK Earthquakes/ Seismology 2 AWP-ODC, HERCULES, PLSQR, SPECFEM 3 D X Quantum Chromo Dynamics 1 Chroma, MILC, USQCD X Social Networks 1 EPISIMDEMICS Evolution 1 Eve Engineering/System of Systems 1 GRIPS, Revisit Computer Science ©Wen-mei 1 and David Kirk/NVIDIA, 2010 -2016 W. Hwu X Monte Carlo FFT PIC X X Sig I/O X X X X X X X X 5 X X
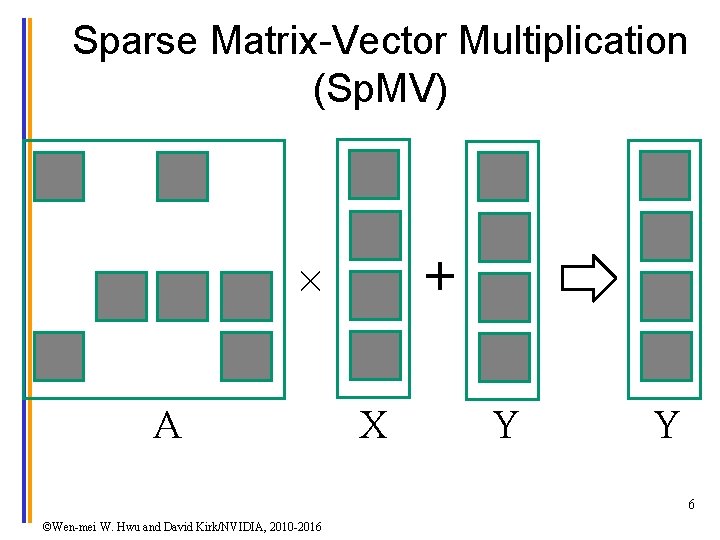
Sparse Matrix-Vector Multiplication (Sp. MV) + × A X Y Y 6 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
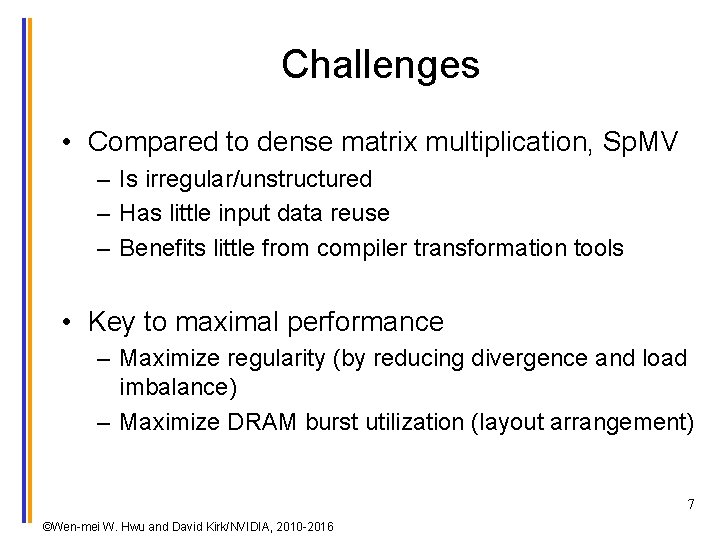
Challenges • Compared to dense matrix multiplication, Sp. MV – Is irregular/unstructured – Has little input data reuse – Benefits little from compiler transformation tools • Key to maximal performance – Maximize regularity (by reducing divergence and load imbalance) – Maximize DRAM burst utilization (layout arrangement) 7 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
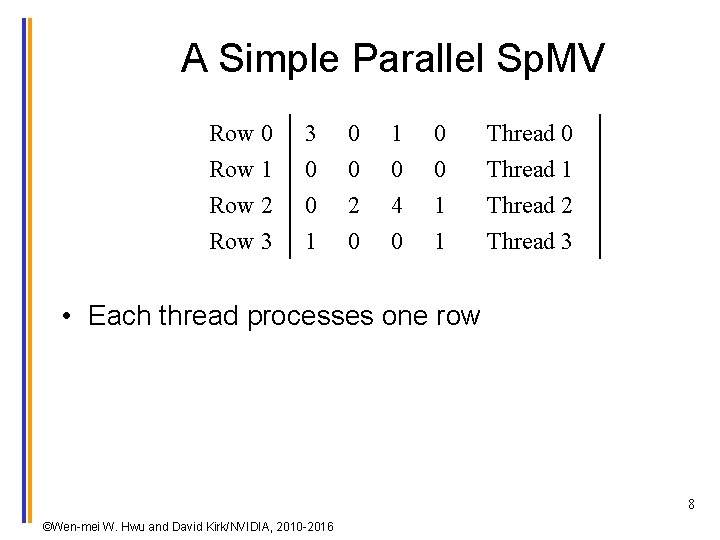
A Simple Parallel Sp. MV Row 0 Row 1 Row 2 Row 3 3 0 0 1 0 0 2 0 1 0 4 0 0 0 1 1 Thread 0 Thread 1 Thread 2 Thread 3 • Each thread processes one row 8 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
![Compressed Sparse Row CSR Format Row 0 Row 2 Nonzero values data7 3 Compressed Sparse Row (CSR) Format Row 0 Row 2 Nonzero values data[7] { 3,](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-9.jpg)
Compressed Sparse Row (CSR) Format Row 0 Row 2 Nonzero values data[7] { 3, 1, 2, 4, 1, Column indices col_index[7] { 0, 2, 1, 2, 3, Row Pointers ptr[5] { 0, 2, 2, 5, 7 Row 3 1, 1 } 0, 3 } } 9 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
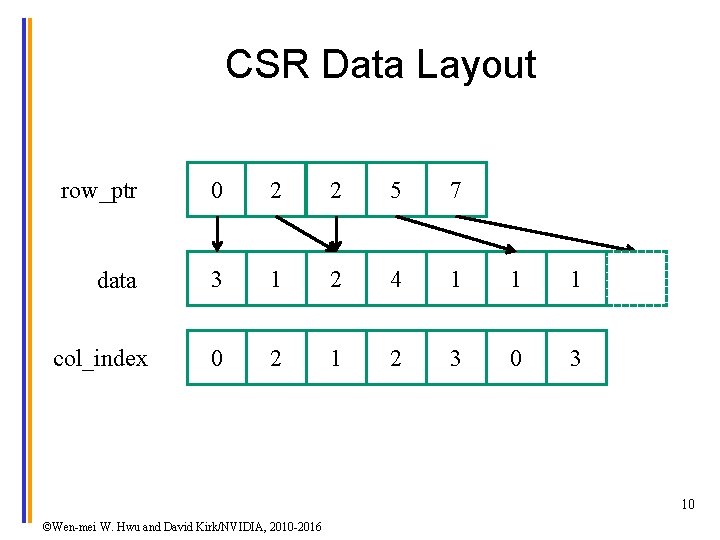
CSR Data Layout row_ptr 0 2 2 5 7 data 3 1 2 4 1 1 1 0 2 1 2 3 0 3 col_index 10 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
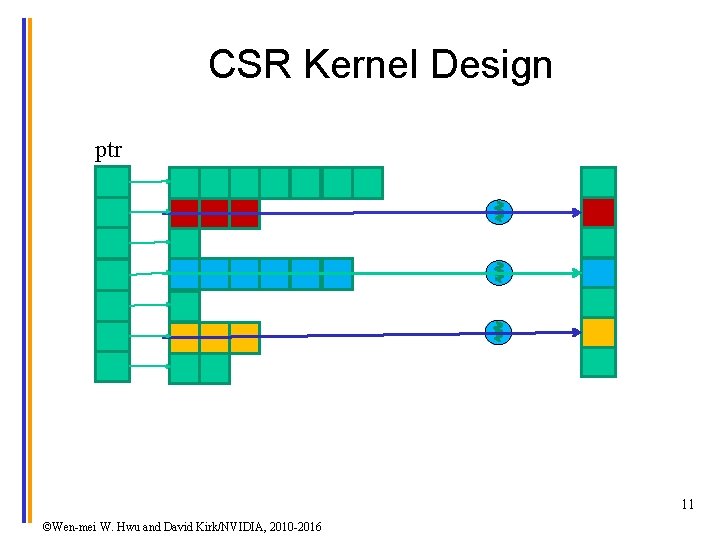
CSR Kernel Design ptr CSR Format ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 11
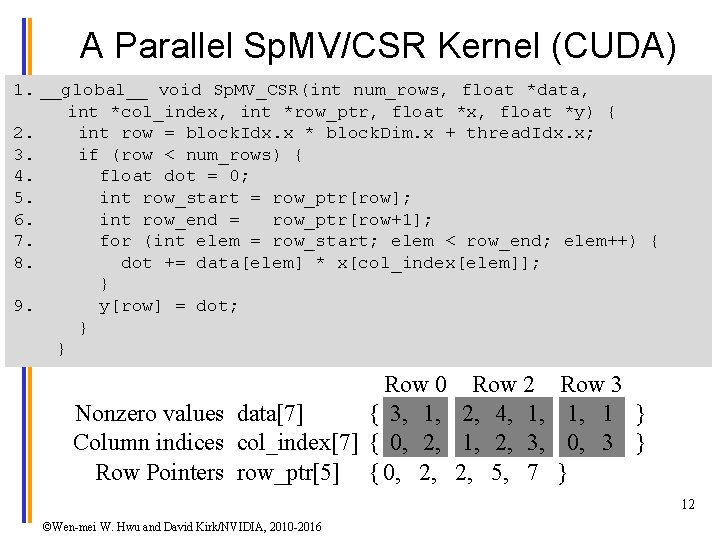
A Parallel Sp. MV/CSR Kernel (CUDA) 1. __global__ void Sp. MV_CSR(int num_rows, float *data, int *col_index, int *row_ptr, float *x, float *y) { 2. int row = block. Idx. x * block. Dim. x + thread. Idx. x; 3. if (row < num_rows) { 4. float dot = 0; 5. int row_start = row_ptr[row]; 6. int row_end = row_ptr[row+1]; 7. for (int elem = row_start; elem < row_end; elem++) { 8. dot += data[elem] * x[col_index[elem]]; } 9. y[row] = dot; } } Row 0 Row 2 Nonzero values data[7] { 3, 1, 2, 4, 1, Column indices col_index[7] { 0, 2, 1, 2, 3, Row Pointers row_ptr[5] { 0, 2, 2, 5, 7 Row 3 1, 1 } 0, 3 } } 12 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
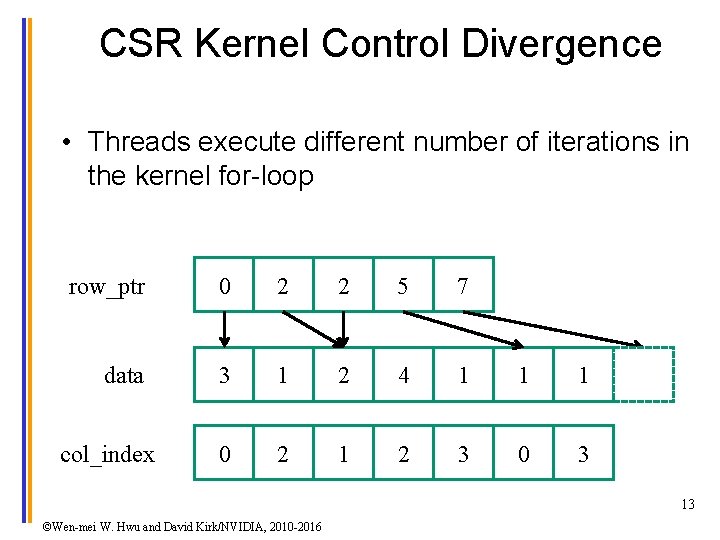
CSR Kernel Control Divergence • Threads execute different number of iterations in the kernel for-loop row_ptr 0 2 2 5 7 data 3 1 2 4 1 1 1 0 2 1 2 3 0 3 col_index 13 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
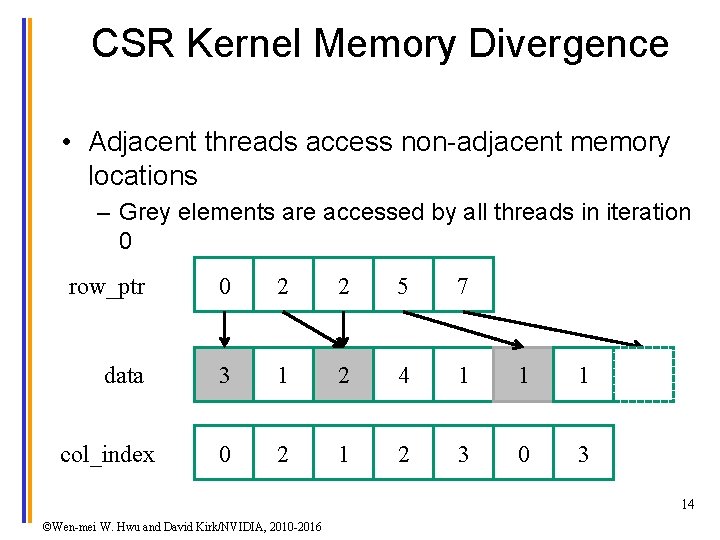
CSR Kernel Memory Divergence • Adjacent threads access non-adjacent memory locations – Grey elements are accessed by all threads in iteration 0 row_ptr 0 2 2 5 7 data 3 1 2 4 1 1 1 0 2 1 2 3 0 3 col_index 14 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
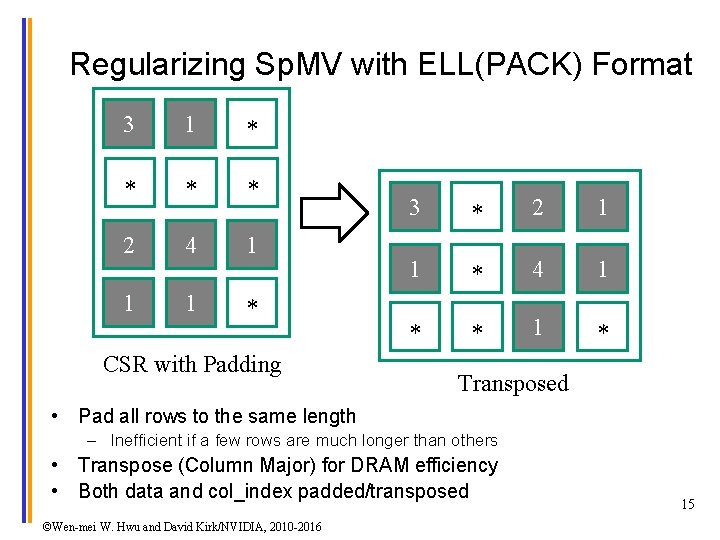
Regularizing Sp. MV with ELL(PACK) Format * * 2 4 1 1 1 * CSR with Padding Thread 3 * Thread 2 * Thread 1 1 Thread 0 3 3 * 2 1 1 * 4 1 * * 1 * Transposed • Pad all rows to the same length – Inefficient if a few rows are much longer than others • Transpose (Column Major) for DRAM efficiency • Both data and col_index padded/transposed ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 15
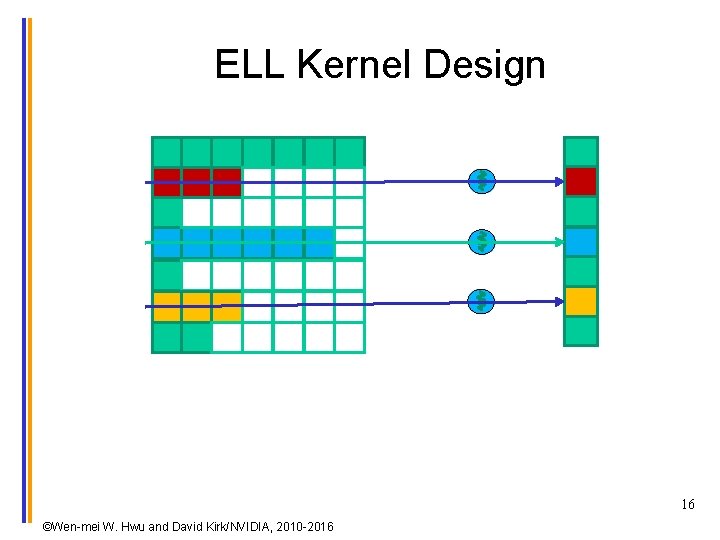
ELL Kernel Design ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 A ELL Format 16
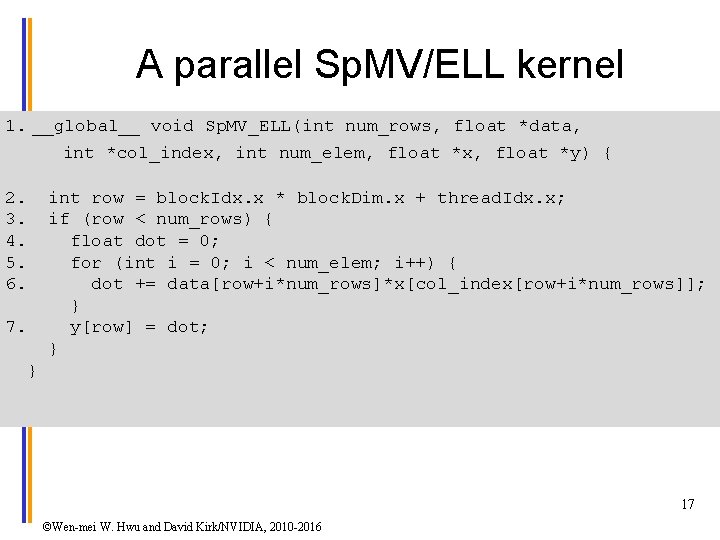
A parallel Sp. MV/ELL kernel 1. __global__ void Sp. MV_ELL(int num_rows, float *data, int *col_index, int num_elem, float *x, float *y) { 2. 3. 4. 5. 6. int row = block. Idx. x * block. Dim. x + thread. Idx. x; if (row < num_rows) { float dot = 0; for (int i = 0; i < num_elem; i++) { dot += data[row+i*num_rows]*x[col_index[row+i*num_rows]]; } y[row] = dot; } 7. } 17 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
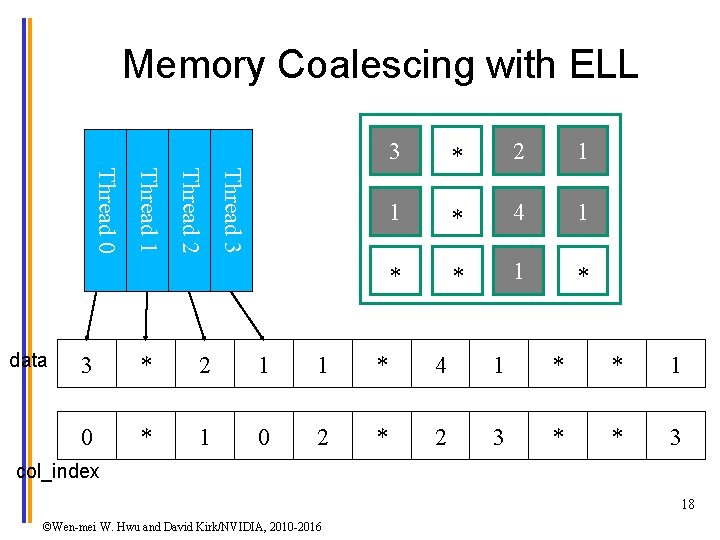
Memory Coalescing with ELL Thread 3 Thread 2 Thread 1 Thread 0 data 3 * 2 1 1 * 4 1 * * 1 * 3 * 2 1 1 * 4 1 * * 1 0 2 * 2 3 * * 3 col_index 18 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
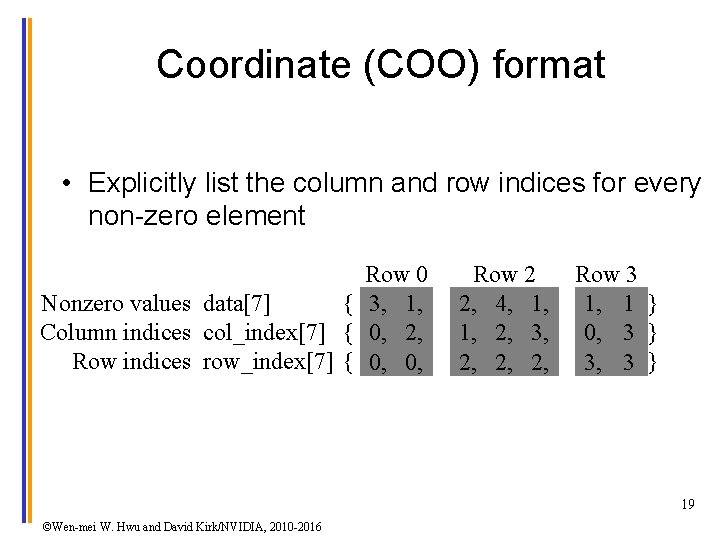
Coordinate (COO) format • Explicitly list the column and row indices for every non-zero element Row 0 Nonzero values data[7] { 3, 1, Column indices col_index[7] { 0, 2, Row indices row_index[7] { 0, 0, Row 2 2, 4, 1, 1, 2, 3, 2, 2, 2, Row 3 1, 1 } 0, 3 } 3, 3 } 19 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
![COO Allows Reordering of Elements Row 0 Nonzero values data7 3 1 Column COO Allows Reordering of Elements Row 0 Nonzero values data[7] { 3, 1, Column](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-20.jpg)
COO Allows Reordering of Elements Row 0 Nonzero values data[7] { 3, 1, Column indices col_index[7] { 0, 2, Row indices row_index[7] { 0, 0, Row 2 2, 4, 1, 1, 2, 3, 2, 2, 2, Row 3 1, 1 } 0, 3 } 3, 3 } Nonzero values data[7] { 1 1, 2, 4, 3, 1 1 } Column indices col_index[7] { 0 2, 1, 2, 0, 3, 3 } Row indices row_index[7] { 3 0, 2, 2, 0, 2, 3 } 20 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
![1 2 for int i 0 i numelem row yrowindexi datai 1. 2. for (int i = 0; i < num_elem; row++) y[row_index[i]] += data[i]](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-21.jpg)
1. 2. for (int i = 0; i < num_elem; row++) y[row_index[i]] += data[i] * x[col_index[i]]; a sequential loop that implements Sp. MV/COO 21 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
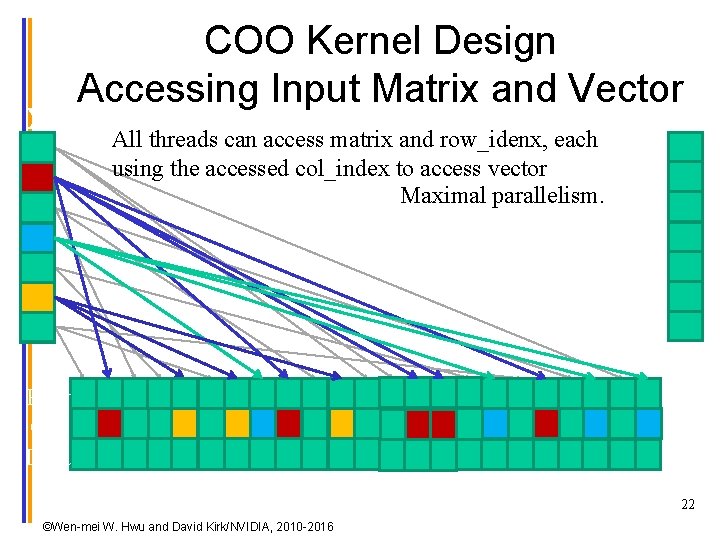
x COO Kernel Design Accessing Input Matrix and Vector Ax = v All threads can access matrix and row_idenx, each using the accessed col_index to access vector Maximal parallelism. . Row Col Data ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 A COO Storage Format v 22
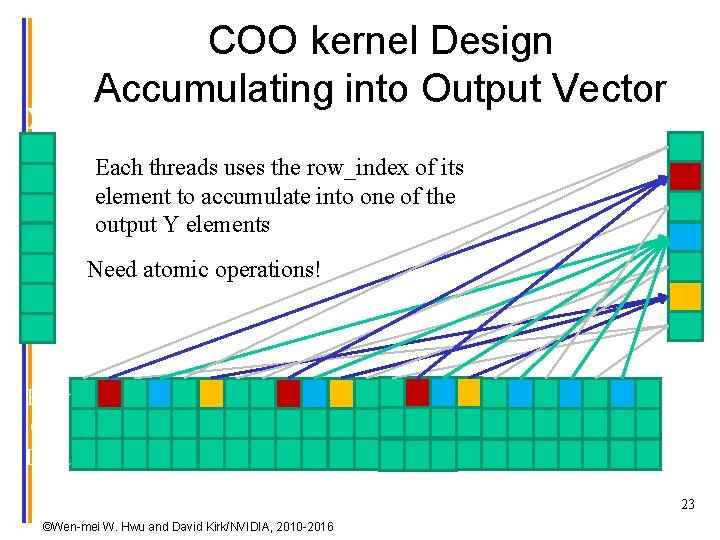
x COO kernel Design Accumulating into Output Vector Ax = v Each threads uses the row_index of its v element to accumulate into one of the output Y elements Need atomic operations! Row Col Data ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 A 23
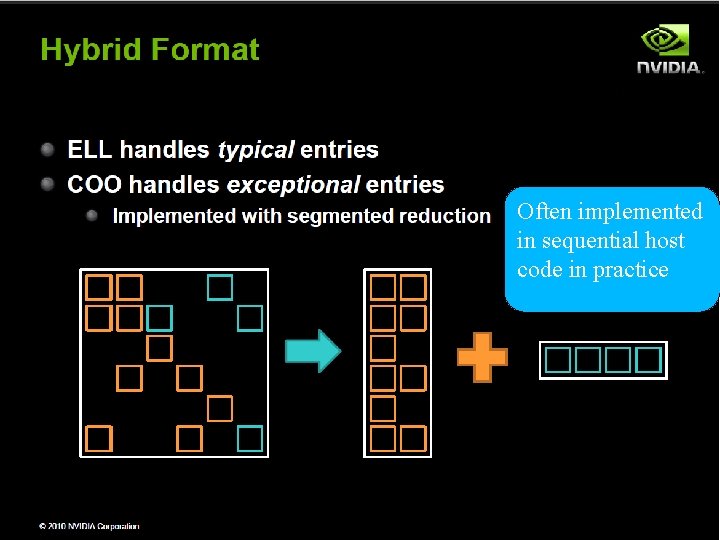
Often implemented in sequential host code in practice 24 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
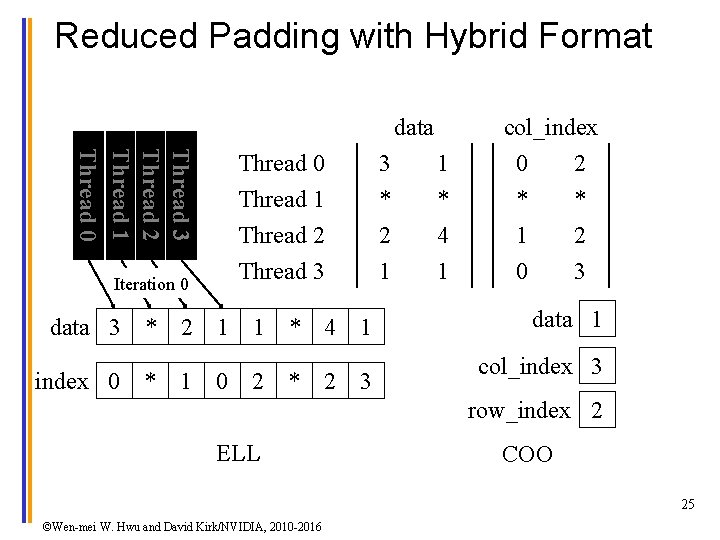
Reduced Padding with Hybrid Format data Thread 3 Thread 2 Thread 1 Thread 0 Iteration 0 Thread 1 Thread 2 3 * 2 1 * 4 Thread 3 1 1 data 3 * 2 1 1 * 4 1 index 0 * 1 0 2 * 2 3 col_index 0 2 * * 1 2 0 3 data 1 col_index 3 row_index 2 ELL COO 25 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
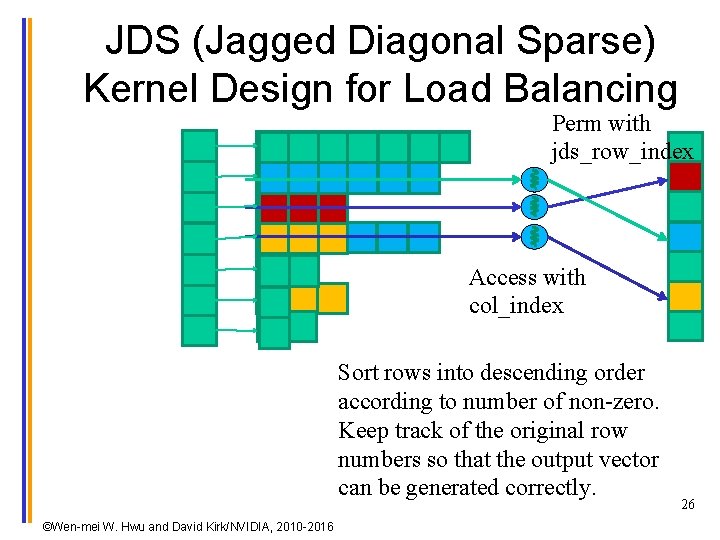
JDS (Jagged Diagonal Sparse) Kernel Design for Load Balancing Perm with jds_row_index Access with col_index Sort rows into descending order according to number of non-zero. Keep track of the original row numbers so that the output vector JDS Format can be generated correctly. ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 26
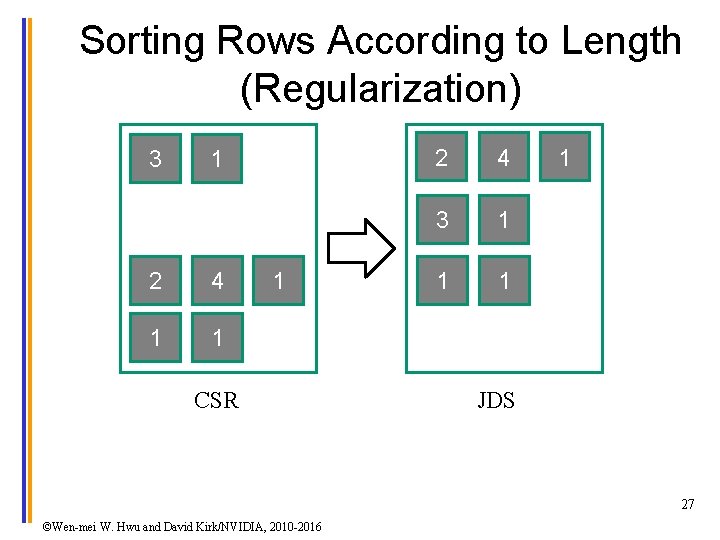
Sorting Rows According to Length (Regularization) 3 1 2 4 1 1 1 CSR 2 4 1 3 1 Row 0 1 1 Row 3 Row 2 Row 1 JDS 27 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
![CSR to JDS Conversion Nonzero values data7 Column indices colindex7 Row Pointers rowptr5 Row CSR to JDS Conversion Nonzero values data[7] Column indices col_index[7] Row Pointers row_ptr[5] Row](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-28.jpg)
CSR to JDS Conversion Nonzero values data[7] Column indices col_index[7] Row Pointers row_ptr[5] Row 0 Row 2 { 3, 1, 2, 4, 1, { 0, 2, 1, 2, 3, { 0, 2, 2, Row 2 Row 3 1, 1 } 0, 3 } 5, 7 } Row 0 Row 3 Nonzero values data[7] { 2, 4, 1, 3, 1, 1 1 } Column indices col_index[7] { 1, 2, 3, 0, 2, 0, 3 } JDS Row Pointers jds_row_ptr[5] JDS Row Indices jds_row_index ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 {0, {2, 3, 0, 5, 3, 7, 7 } 1} 28
![JDS Summary Nonzero values data7 2 4 1 3 1 1 1 JDS Summary Nonzero values data[7] { 2, 4, 1, 3, 1, 1, 1 }](https://slidetodoc.com/presentation_image_h2/1a43cb432548a01d76f8c71cfca052e8/image-29.jpg)
JDS Summary Nonzero values data[7] { 2, 4, 1, 3, 1, 1, 1 } Column indices col_index[7] JDS row indices Jds_row_index[4] JDS Row Ptrs Jds_row_ptr[4] { 1, 2, 3, 0, 2, 0, 3 } { 2, 0, 3, 1 } { 0, 3, 5, 7, 7 } 2 4 1 3 1 1 2 3 0 0 2 3 29 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
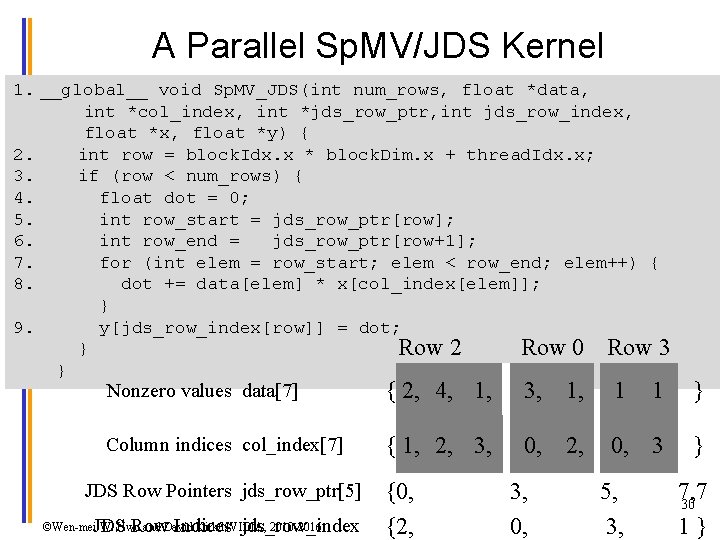
A Parallel Sp. MV/JDS Kernel 1. __global__ void Sp. MV_JDS(int num_rows, float *data, int *col_index, int *jds_row_ptr, int jds_row_index, float *y) { 2. int row = block. Idx. x * block. Dim. x + thread. Idx. x; 3. if (row < num_rows) { 4. float dot = 0; 5. int row_start = jds_row_ptr[row]; 6. int row_end = jds_row_ptr[row+1]; 7. for (int elem = row_start; elem < row_end; elem++) { 8. dot += data[elem] * x[col_index[elem]]; } 9. y[jds_row_index[row]] = dot; } Row 2 Row 0 Row 3 } Nonzero values data[7] { 2, 4, 1, 3, 1, 1 1 } Column indices col_index[7] { 1, 2, 3, 0, 2, 0, 3 } JDS Row Pointers jds_row_ptr[5] JDS Row Indices jds_row_index ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 {0, {2, 3, 0, 5, 3, 7, 7 30 1}
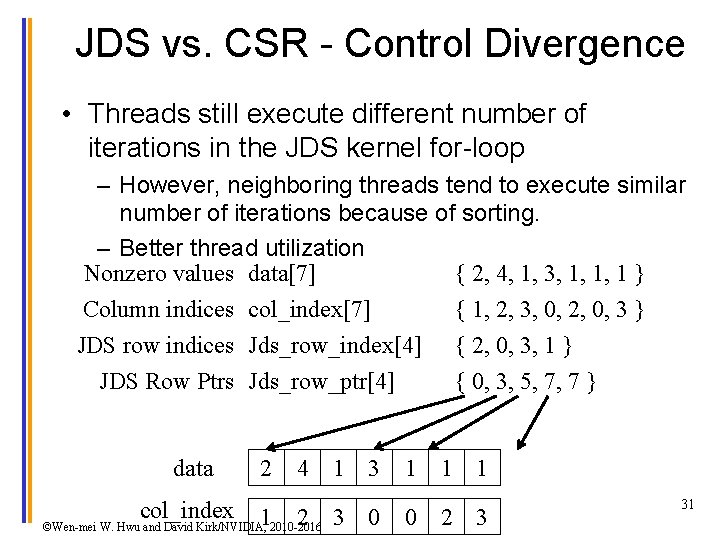
JDS vs. CSR - Control Divergence • Threads still execute different number of iterations in the JDS kernel for-loop – However, neighboring threads tend to execute similar number of iterations because of sorting. – Better thread utilization Nonzero values data[7] { 2, 4, 1, 3, 1, 1, 1 } Column indices col_index[7] { 1, 2, 3, 0, 2, 0, 3 } JDS row indices Jds_row_index[4] { 2, 0, 3, 1 } JDS Row Ptrs Jds_row_ptr[4] { 0, 3, 5, 7, 7 } data col_index 2 1 4 2 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 1 3 3 0 1 1 2 3 31
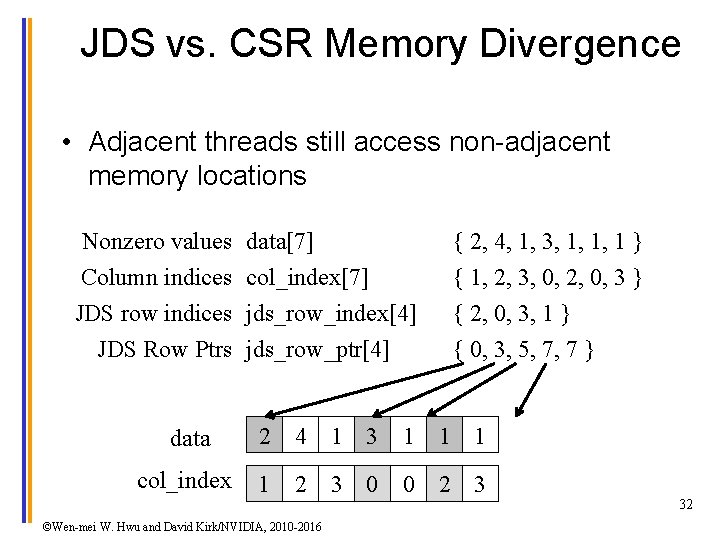
JDS vs. CSR Memory Divergence • Adjacent threads still access non-adjacent memory locations Nonzero values Column indices JDS row indices JDS Row Ptrs data[7] col_index[7] jds_row_index[4] jds_row_ptr[4] { 2, 4, 1, 3, 1, 1, 1 } { 1, 2, 3, 0, 2, 0, 3 } { 2, 0, 3, 1 } { 0, 3, 5, 7, 7 } data 2 4 1 3 1 1 1 col_index 1 2 3 0 0 2 3 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 32
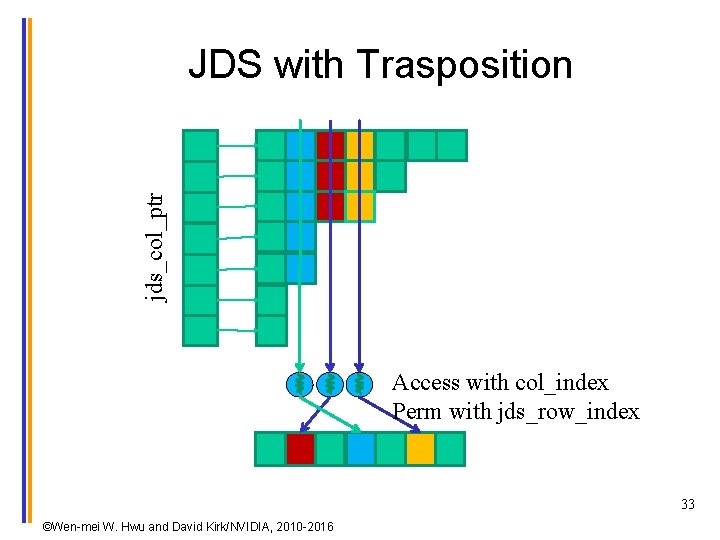
jds_col_ptr JDS with Trasposition Access with col_index Perm with jds_row_index 33 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
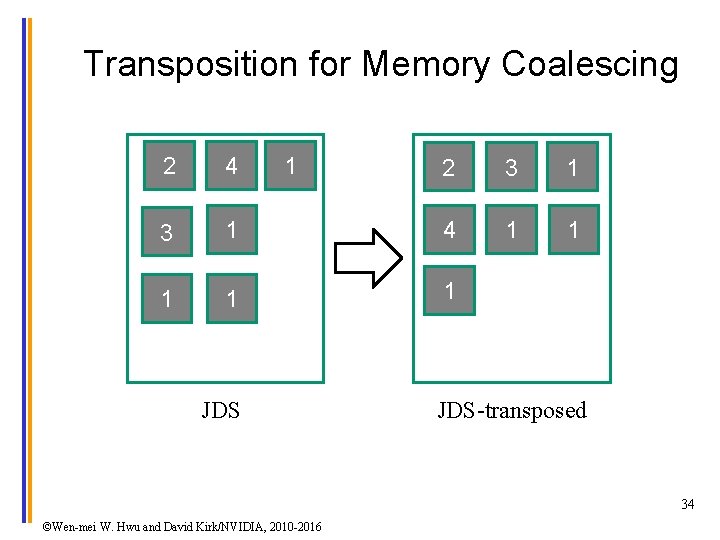
Transposition for Memory Coalescing 2 3 1 Row 2 1 4 1 1 Row 0 1 1 2 4 3 1 1 Row 3 Row 1 JDS-transposed 34 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
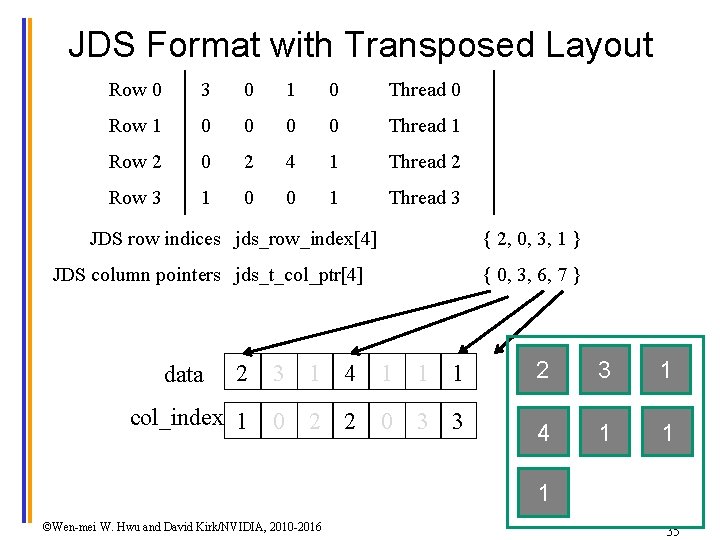
JDS Format with Transposed Layout Row 0 3 0 1 0 Thread 0 Row 1 0 0 Thread 1 Row 2 0 2 4 1 Thread 2 Row 3 1 0 0 1 Thread 3 JDS row indices jds_row_index[4] { 2, 0, 3, 1 } JDS column pointers jds_t_col_ptr[4] { 0, 3, 6, 7 } 2 3 1 4 1 1 1 2 3 1 col_index 1 0 2 2 0 3 3 4 1 1 data 1 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016 35
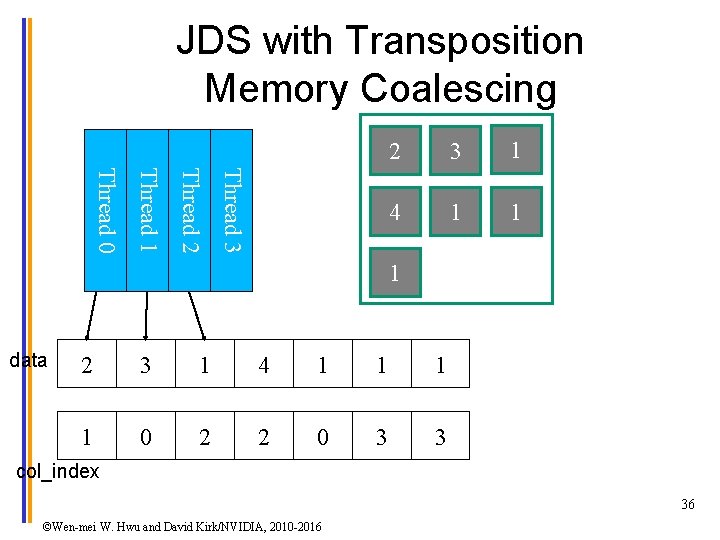
JDS with Transposition Memory Coalescing Thread 3 Thread 2 Thread 1 Thread 0 2 3 1 4 1 1 1 data 2 3 1 4 1 1 0 2 2 0 3 3 col_index 36 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
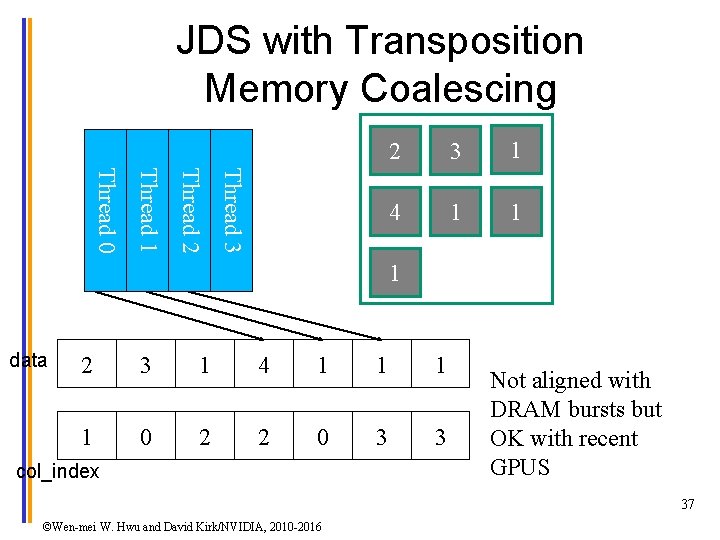
JDS with Transposition Memory Coalescing Thread 3 Thread 2 Thread 1 Thread 0 2 3 1 4 1 1 1 data 2 3 1 4 1 1 0 2 2 0 3 3 col_index Not aligned with DRAM bursts but OK with recent GPUS 37 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
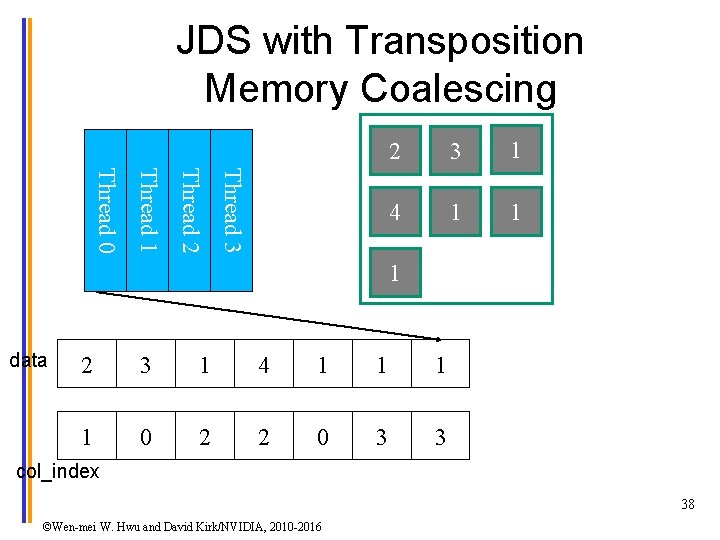
JDS with Transposition Memory Coalescing Thread 3 Thread 2 Thread 1 Thread 0 2 3 1 4 1 1 1 data 2 3 1 4 1 1 0 2 2 0 3 3 col_index 38 ©Wen-mei W. Hwu and David Kirk/NVIDIA, 2010 -2016
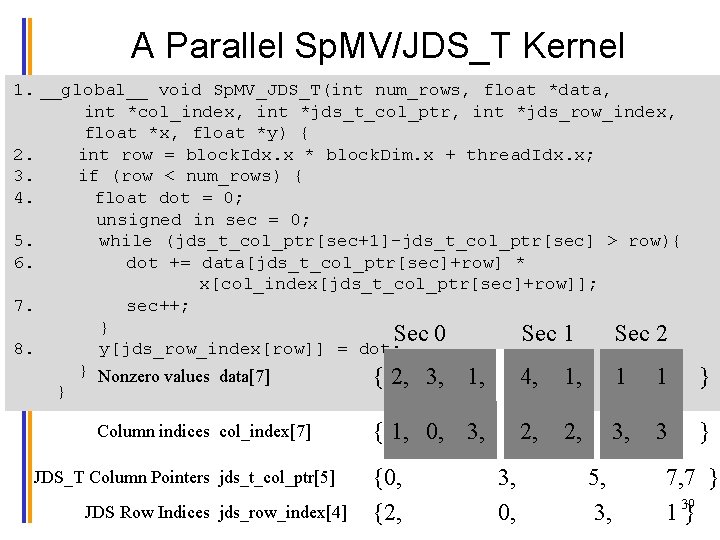
A Parallel Sp. MV/JDS_T Kernel 1. __global__ void Sp. MV_JDS_T(int num_rows, float *data, int *col_index, int *jds_t_col_ptr, int *jds_row_index, float *y) { 2. int row = block. Idx. x * block. Dim. x + thread. Idx. x; 3. if (row < num_rows) { 4. float dot = 0; unsigned in sec = 0; 5. while (jds_t_col_ptr[sec+1]-jds_t_col_ptr[sec] > row){ 6. dot += data[jds_t_col_ptr[sec]+row] * x[col_index[jds_t_col_ptr[sec]+row]]; 7. sec++; } Sec 0 Sec 1 Sec 2 8. y[jds_row_index[row]] = dot; } Nonzero values data[7] { 2, 3, 1, 4, 1, 1 1 } Column indices col_index[7] JDS_T Column Pointers jds_t_col_ptr[5] JDS Row Indices jds_row_index[4] { 1, 0, 3, {0, {2, 2, 3, 0, 2, 3, 5, 3, 3 } } 7, 7 } 1 39}
Uiuc ece 408
Ece 408
Ece 408
Ece 408
Ece 408
01:640:244 lecture notes - lecture 15: plat, idah, farad
Cse408
408b2 form
Usace section 408
Nyc doe chapter 408 forms
Cs 408 sabancı
Pub 408
Amministratore di sostegno lite tra fratelli
Ce 408
Free fall 2016
C data types with examples
Inertia moment
The parallel axis theorem for an area is applied between
Cloud computing lecture
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Components of system programming
Linear vs integer programming
Definisi linear
Programming massively parallel processors
Scala parallel map
What is parallel programming in java
An introduction to parallel programming peter pacheco
Counting sort mpi
Mpi parallel programming in c
Programming massively parallel processors
Programming massively parallel processors
Parallel programming platforms
F# parallel programming
Parallel programming
Programming massively parallel processors, kirk et al.
Define like and unlike forces
Parts of fingerprint
Parallelism
Parallel struc