Department of Computer Engineering Decisions 2140101 Computer Programming
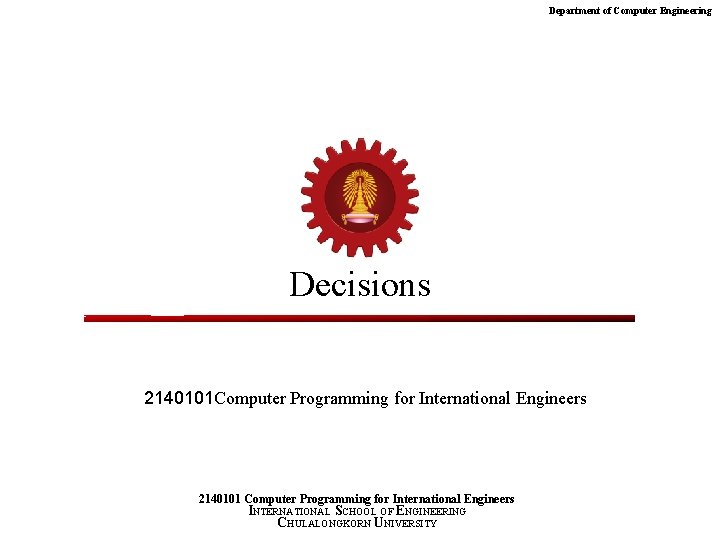
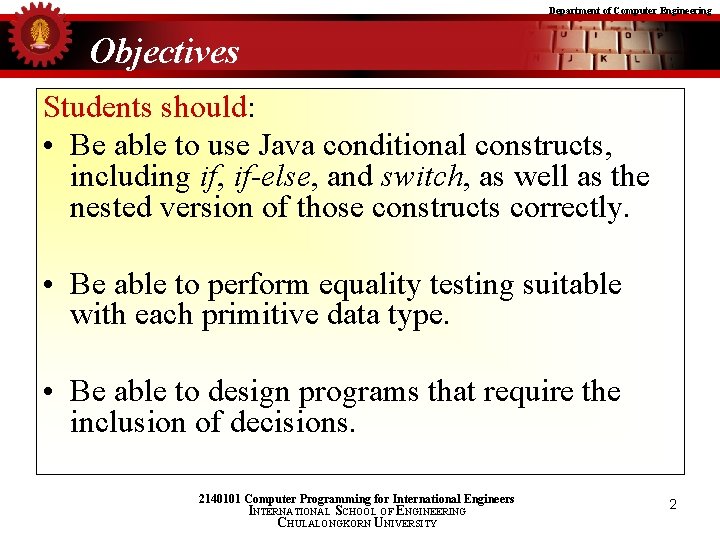
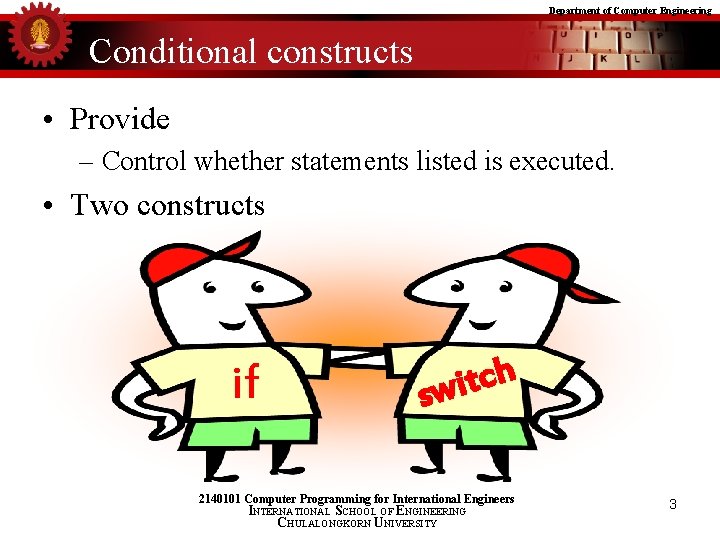
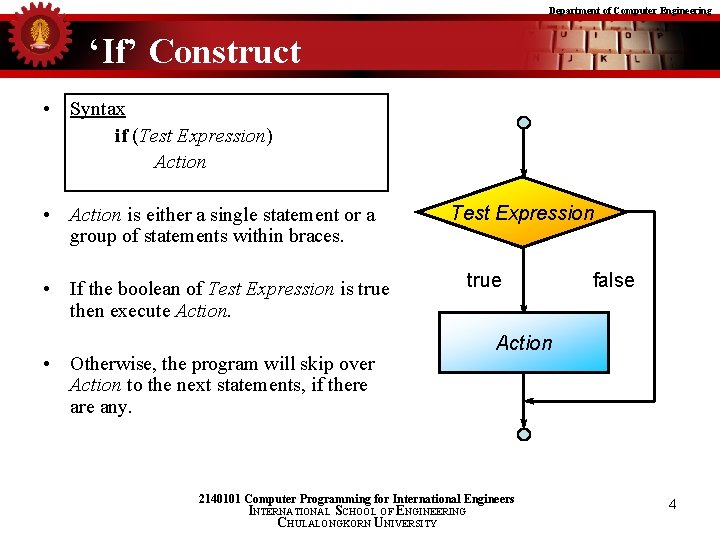
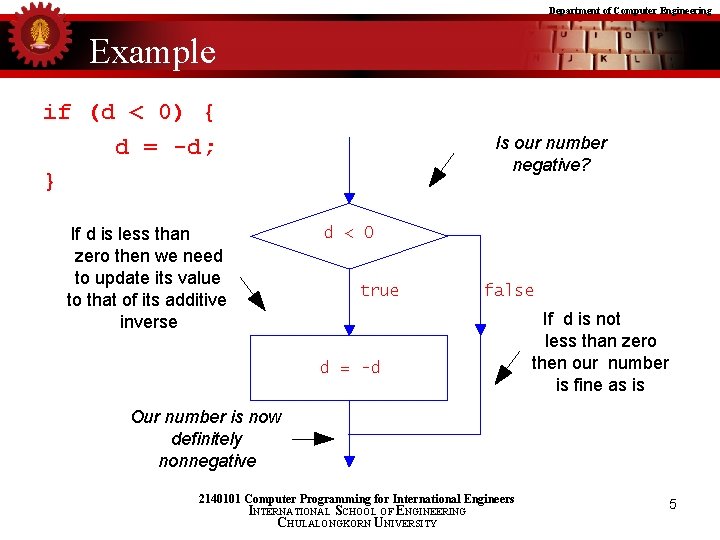
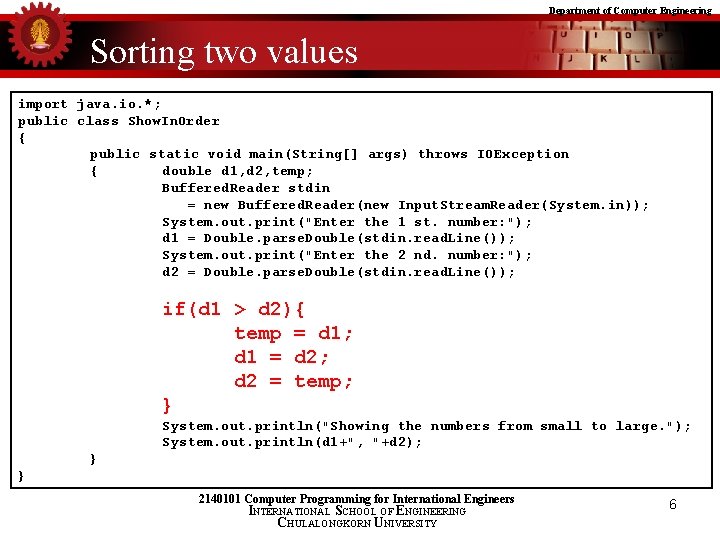
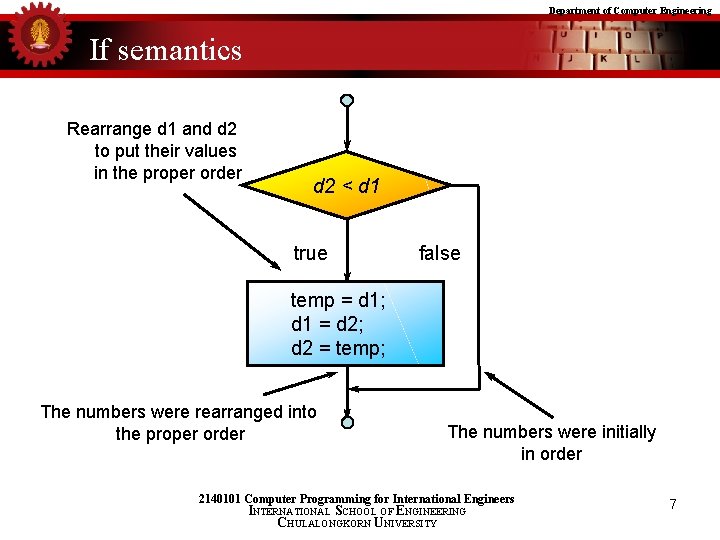
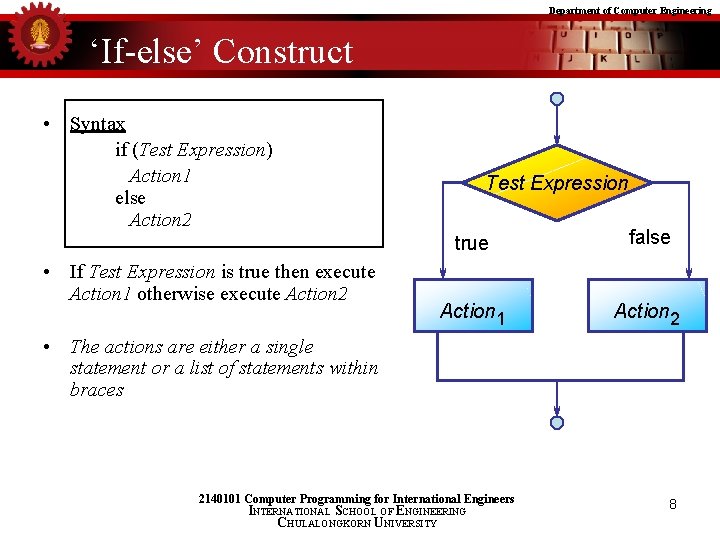
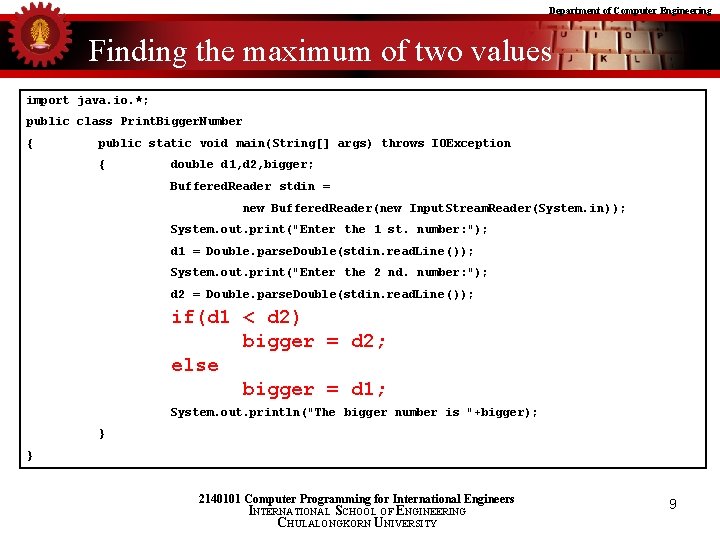
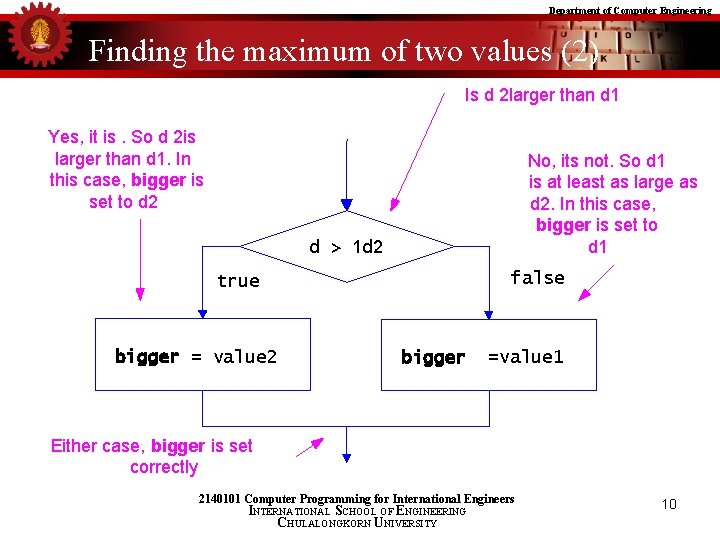
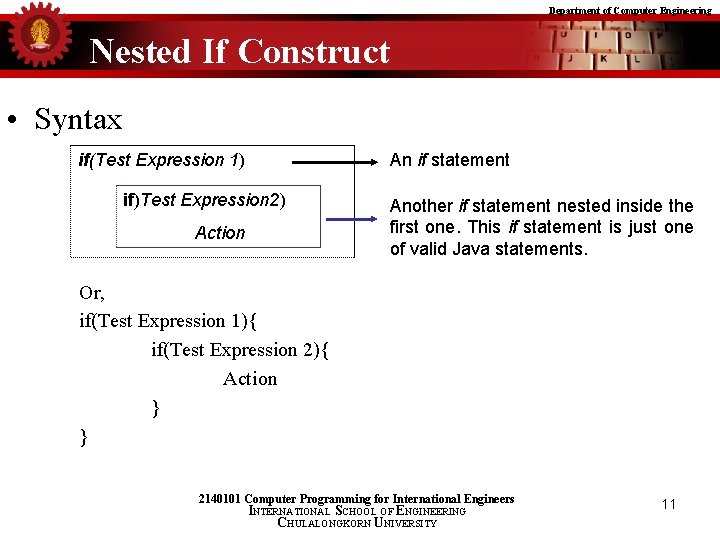
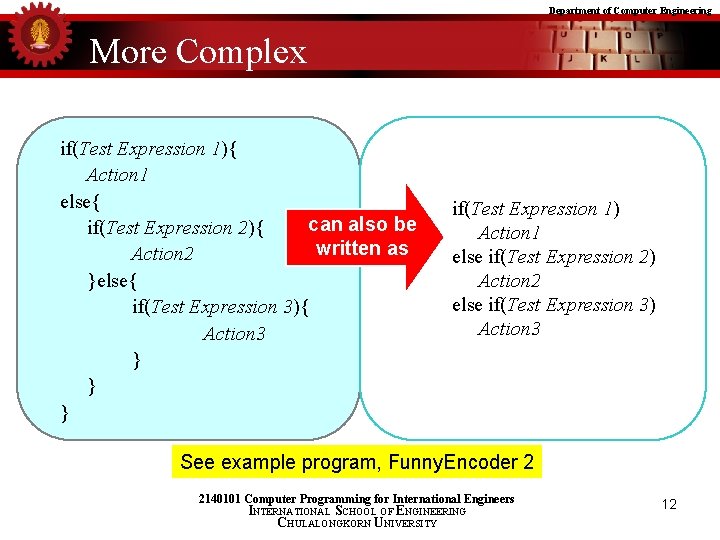
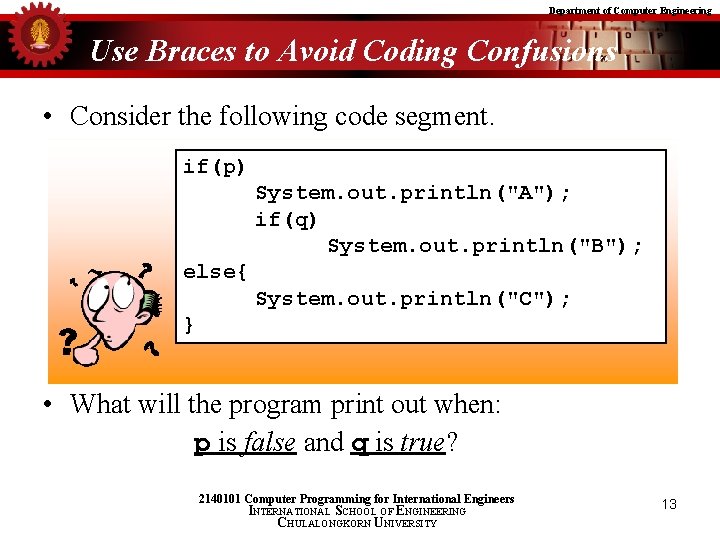
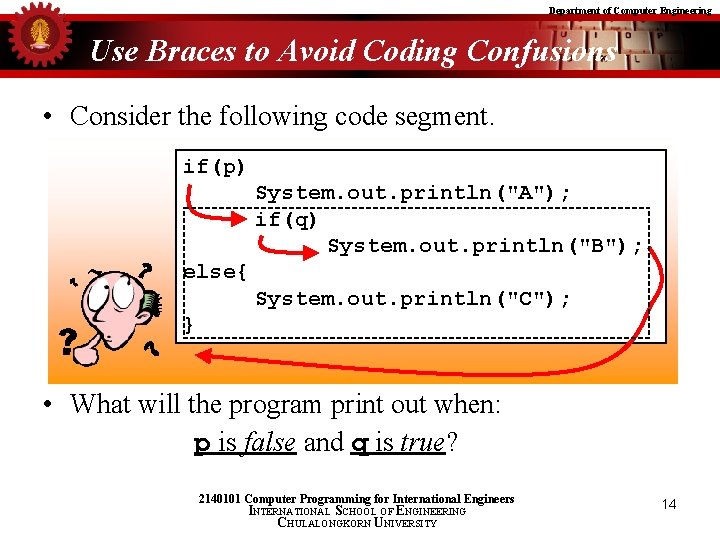
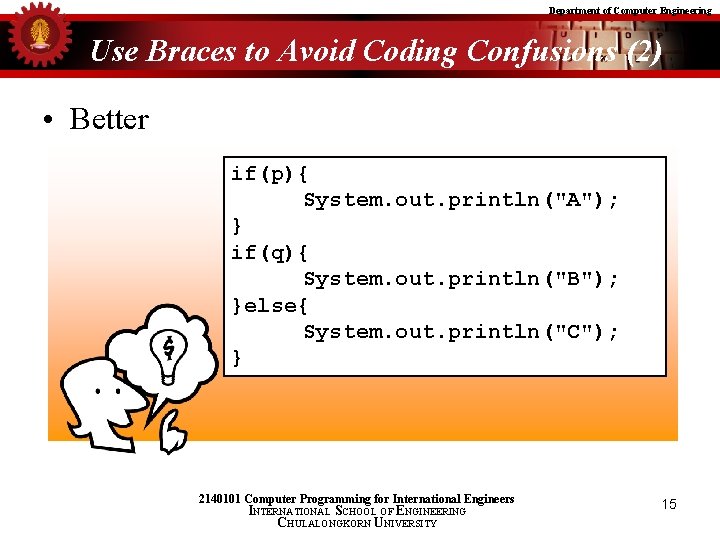
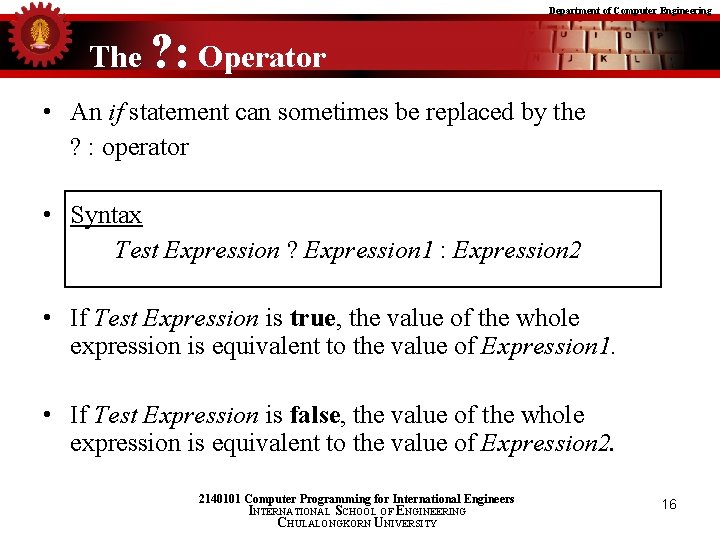
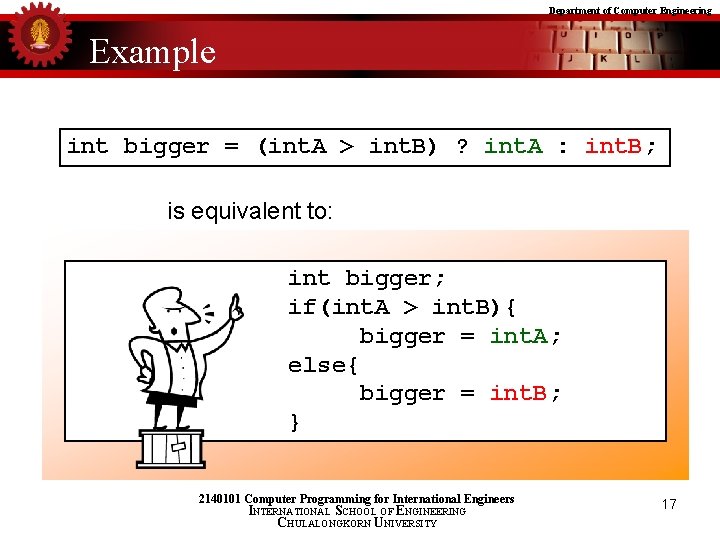
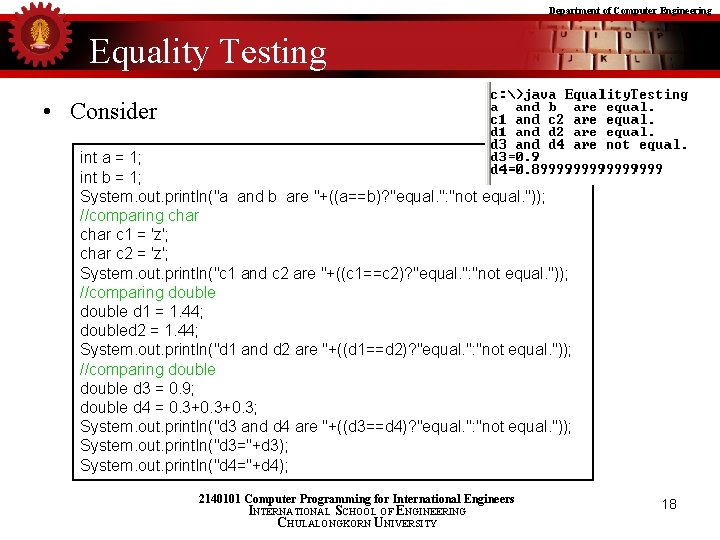
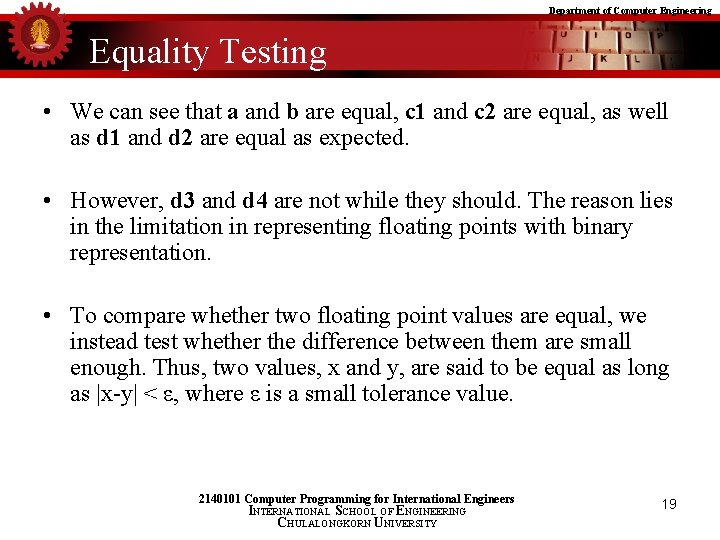
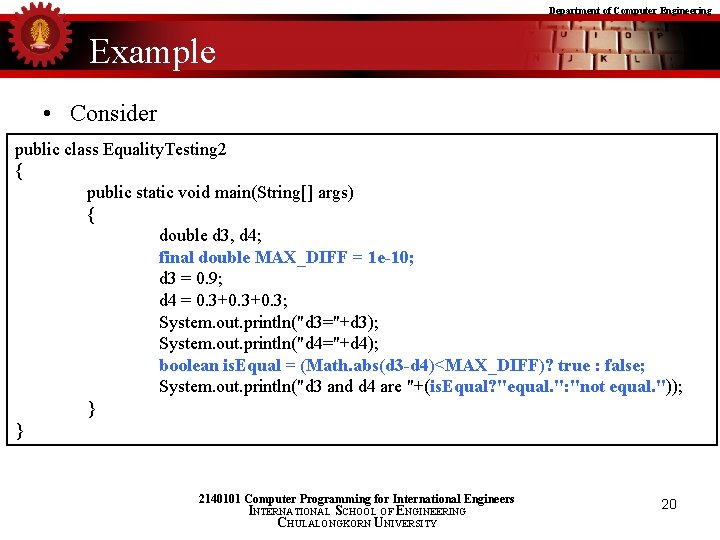
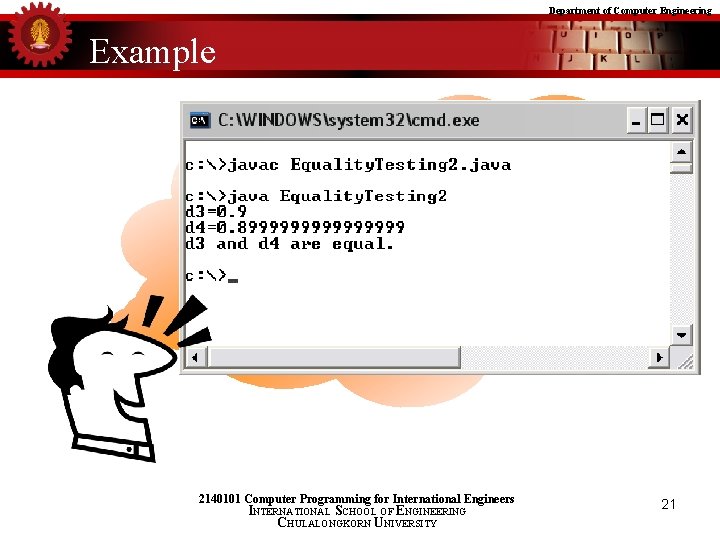
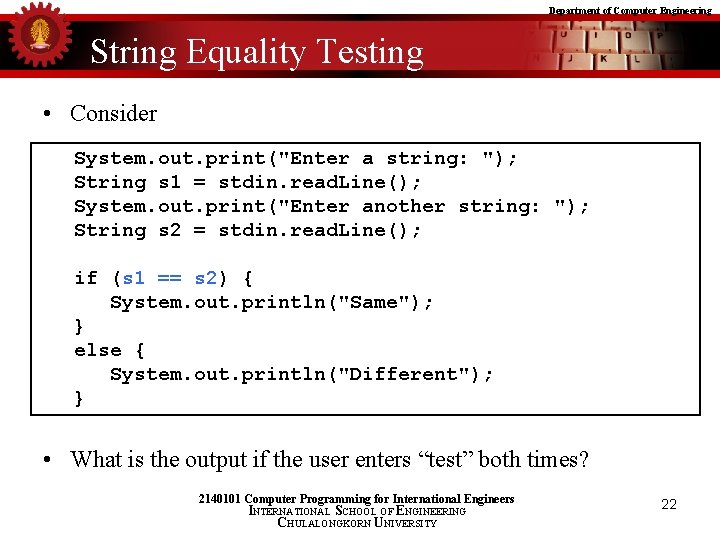
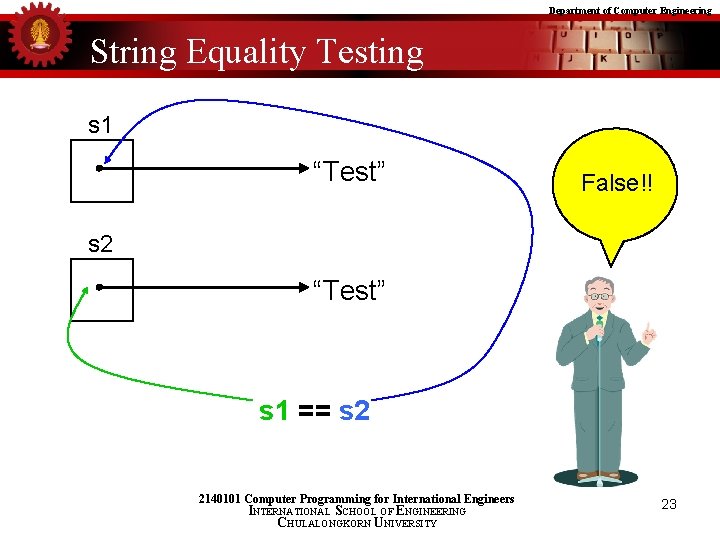
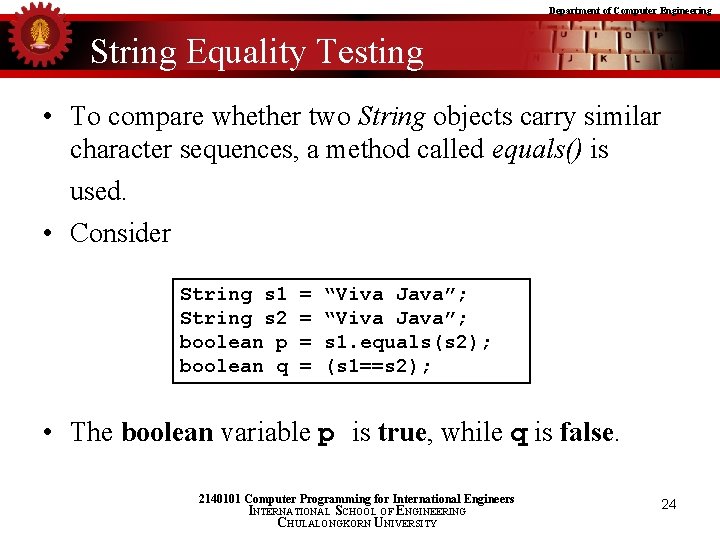
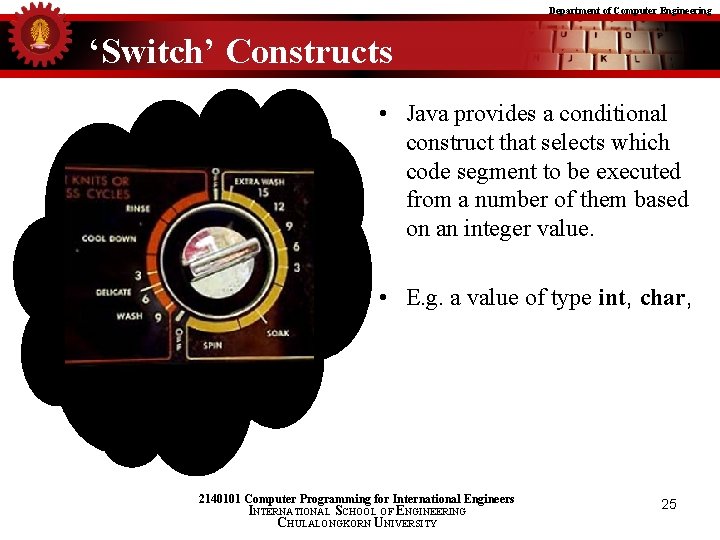
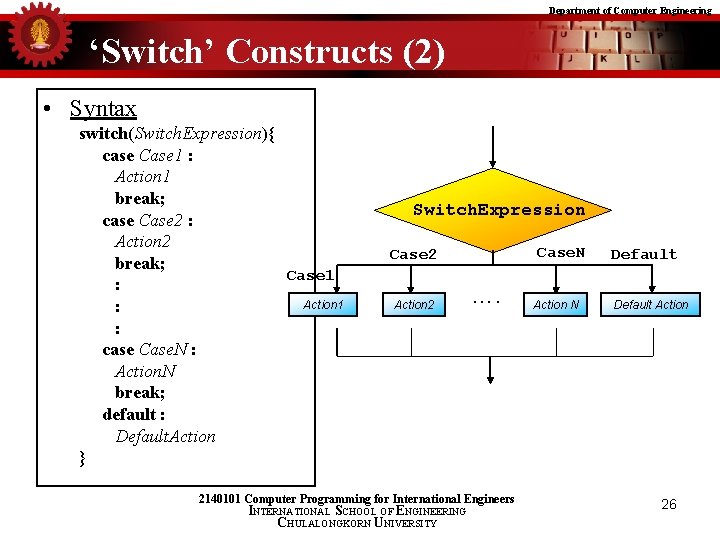
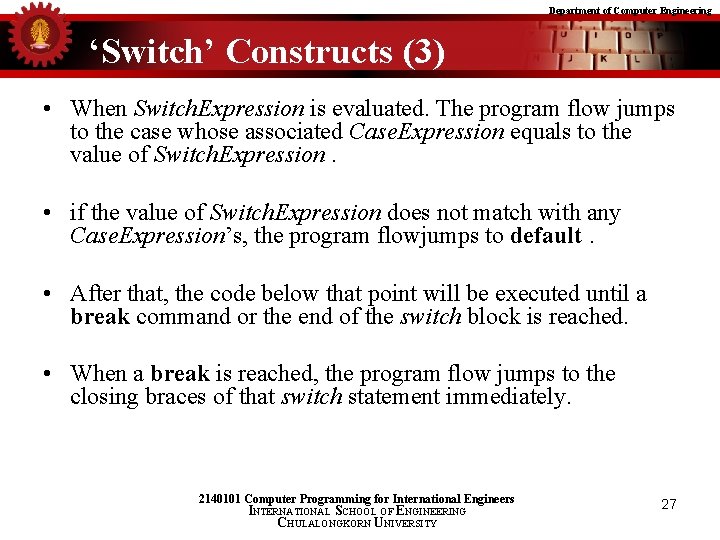
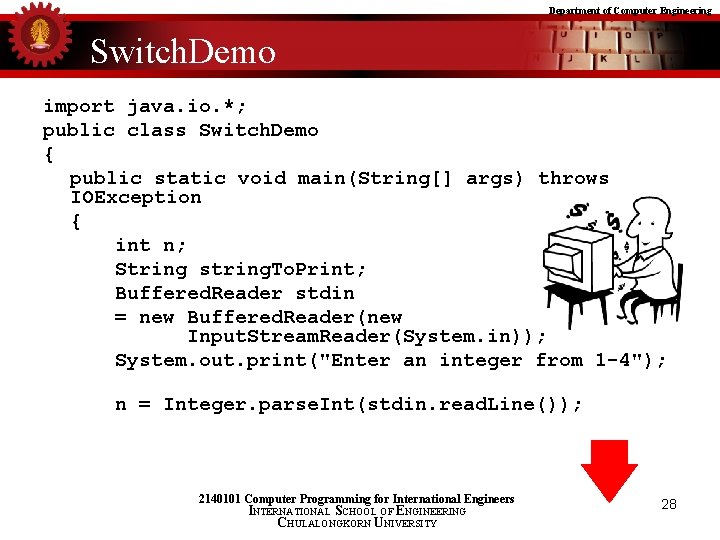
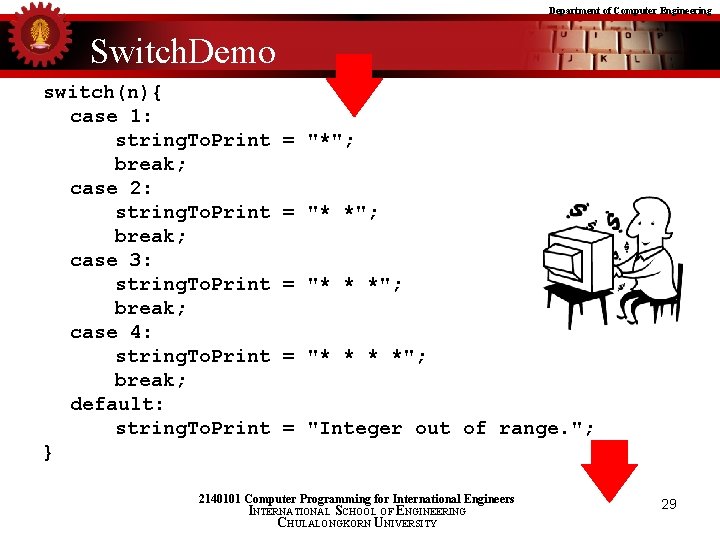
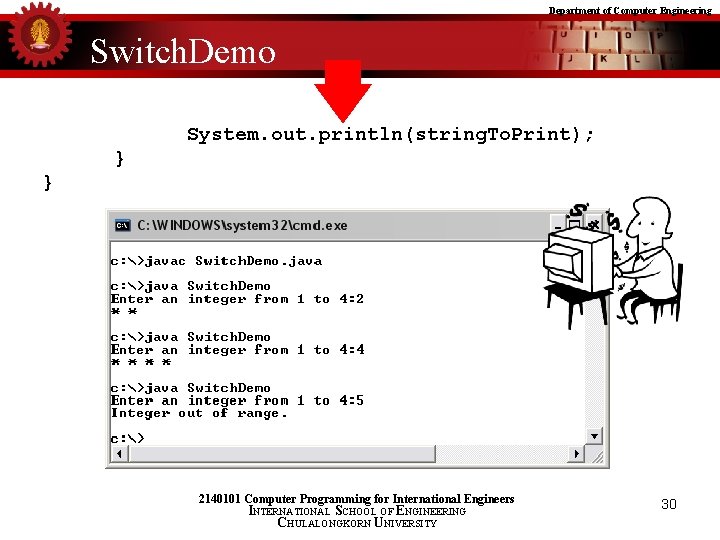
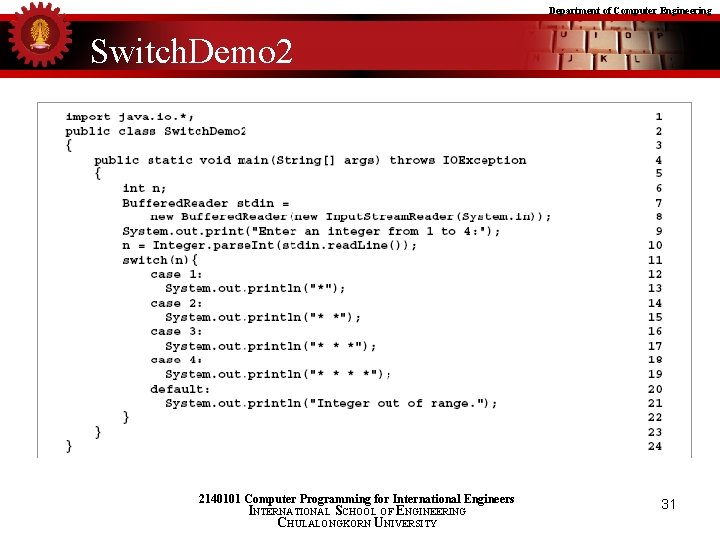
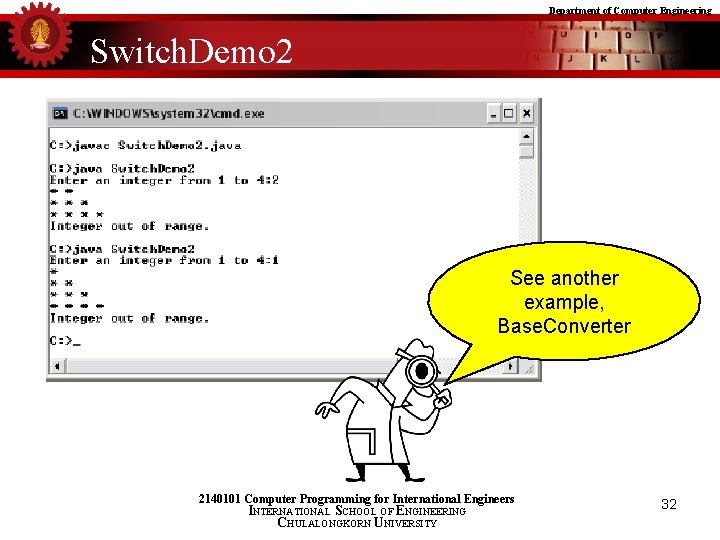
- Slides: 32
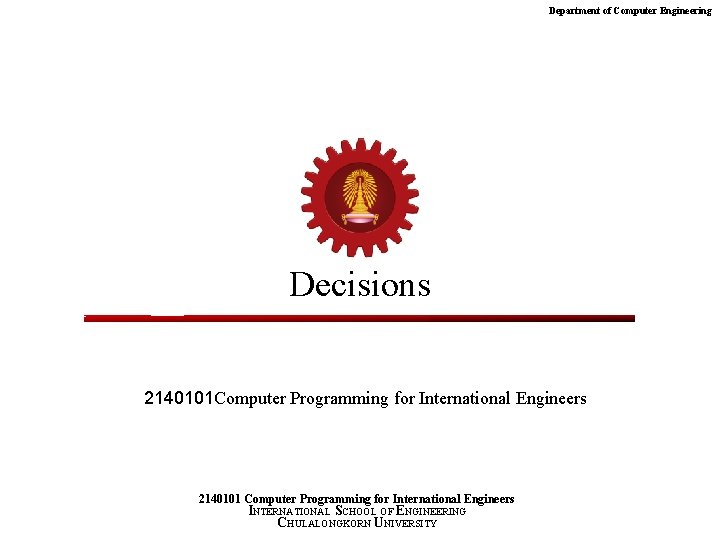
Department of Computer Engineering Decisions 2140101 Computer Programming for International Engineers 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY
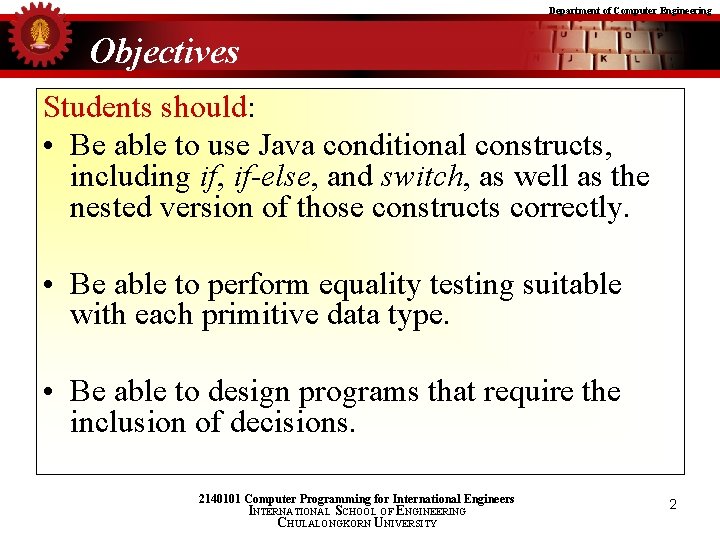
Department of Computer Engineering Objectives Students should: • Be able to use Java conditional constructs, including if, if-else, and switch, as well as the nested version of those constructs correctly. • Be able to perform equality testing suitable with each primitive data type. • Be able to design programs that require the inclusion of decisions. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 2
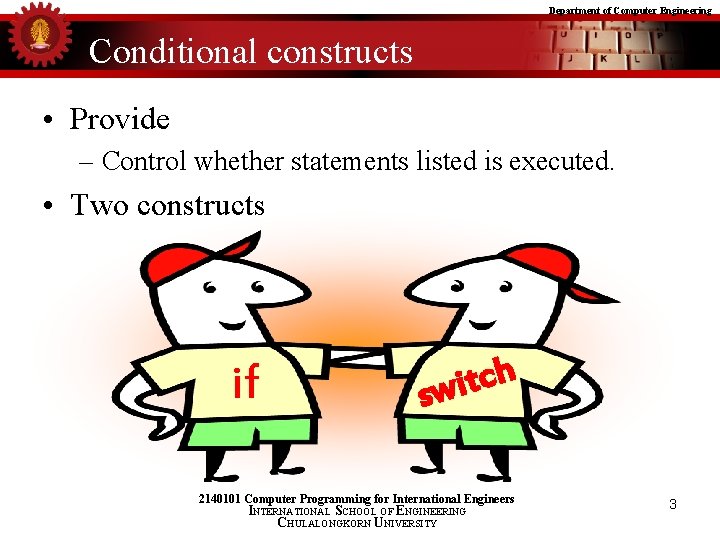
Department of Computer Engineering Conditional constructs • Provide – Control whether statements listed is executed. • Two constructs if h c t i sw 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 3
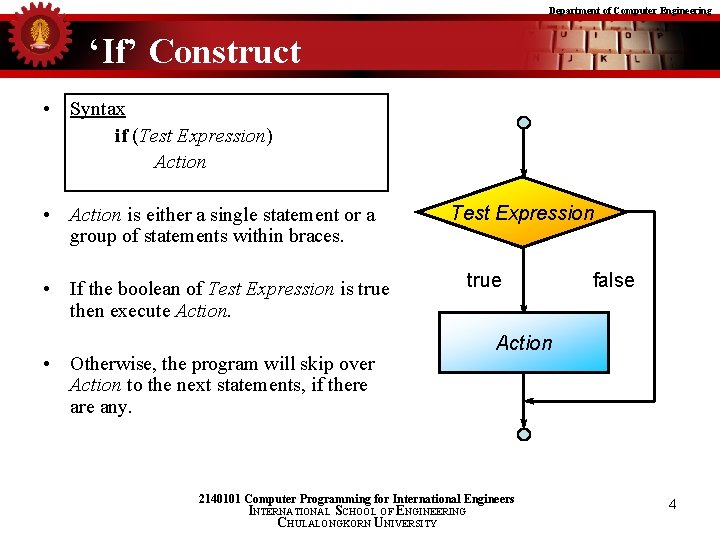
Department of Computer Engineering ‘If’ Construct • Syntax if (Test Expression) Action • Action is either a single statement or a group of statements within braces. Test Expression • If the boolean of Test Expression is true then execute Action. • Otherwise, the program will skip over Action to the next statements, if there any. true Action 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY false 4
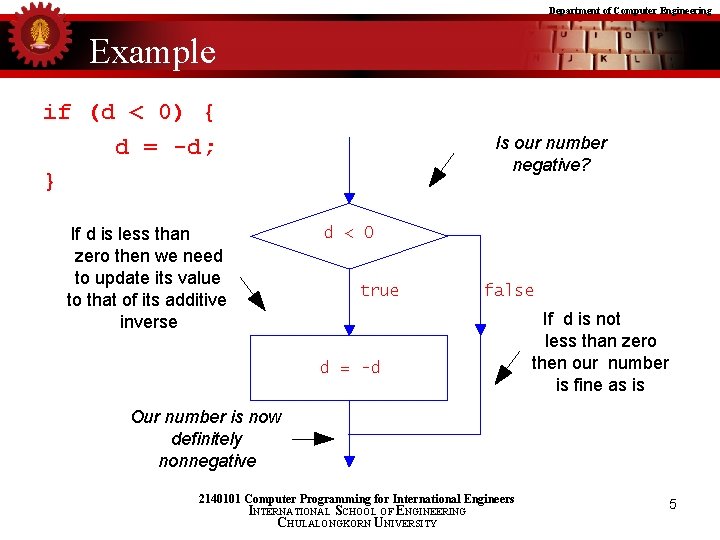
Department of Computer Engineering Example if (d < 0) { d = -d; } Is our number negative? If d is less than zero then we need to update its value to that of its additive inverse d < 0 true false d = -d If d is not less than zero then our number is fine as is Our number is now definitely nonnegative 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 5
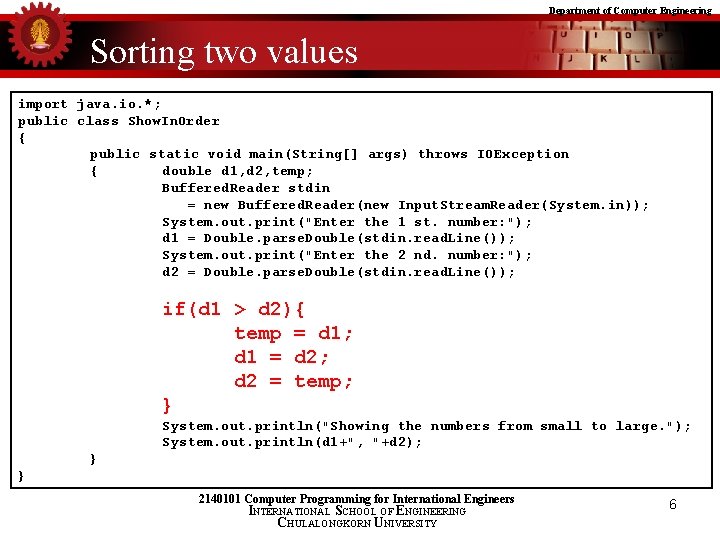
Department of Computer Engineering Sorting two values import java. io. *; public class Show. In. Order { public static void main(String[] args) throws IOException { double d 1, d 2, temp; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in)); System. out. print("Enter the 1 st. number: "); d 1 = Double. parse. Double(stdin. read. Line()); System. out. print("Enter the 2 nd. number: "); d 2 = Double. parse. Double(stdin. read. Line()); if(d 1 > d 2){ temp = d 1; d 1 = d 2; d 2 = temp; } System. out. println("Showing the numbers from small to large. "); System. out. println(d 1+", "+d 2); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 6
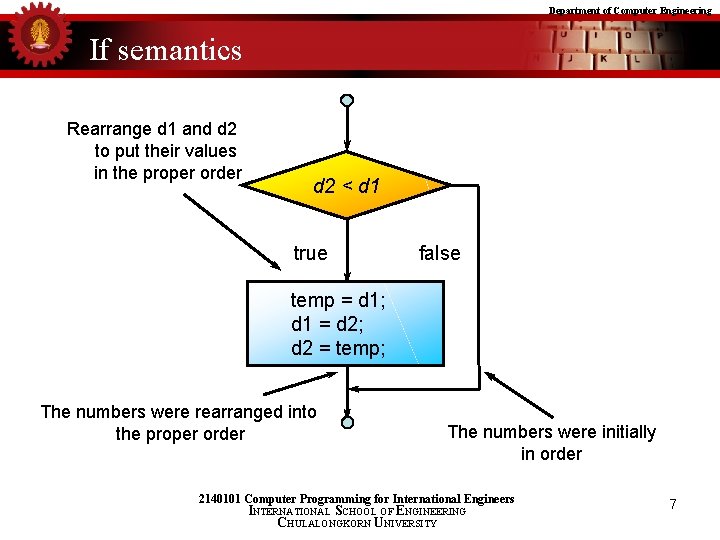
Department of Computer Engineering If semantics Rearrange d 1 and d 2 to put their values in the proper order d 2 < d 1 true false temp = d 1; d 1 = d 2; d 2 = temp; The numbers were rearranged into the proper order The numbers were initially in order 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 7
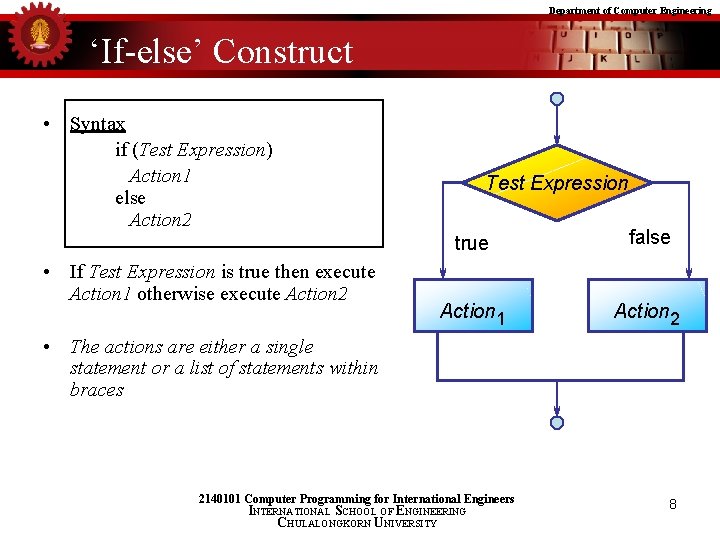
Department of Computer Engineering ‘If-else’ Construct • Syntax if (Test Expression) Action 1 else Action 2 • If Test Expression is true then execute Action 1 otherwise execute Action 2 Test Expression true false Action 1 Action 2 • The actions are either a single statement or a list of statements within braces 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 8
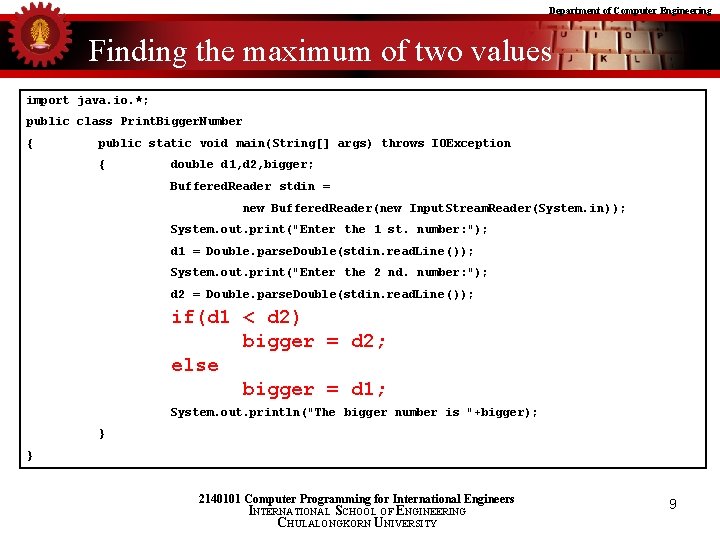
Department of Computer Engineering Finding the maximum of two values import java. io. *; public class Print. Bigger. Number { public static void main(String[] args) throws IOException { double d 1, d 2, bigger; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in )); System. out. print("Enter the 1 st. number: "); d 1 = Double. parse. Double(stdin. read. Line ()); System. out. print("Enter the 2 nd. number: "); d 2 = Double. parse. Double(stdin. read. Line ()); if(d 1 < d 2) bigger = d 2; else bigger = d 1; System. out. println("The bigger number is "+bigger); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 9
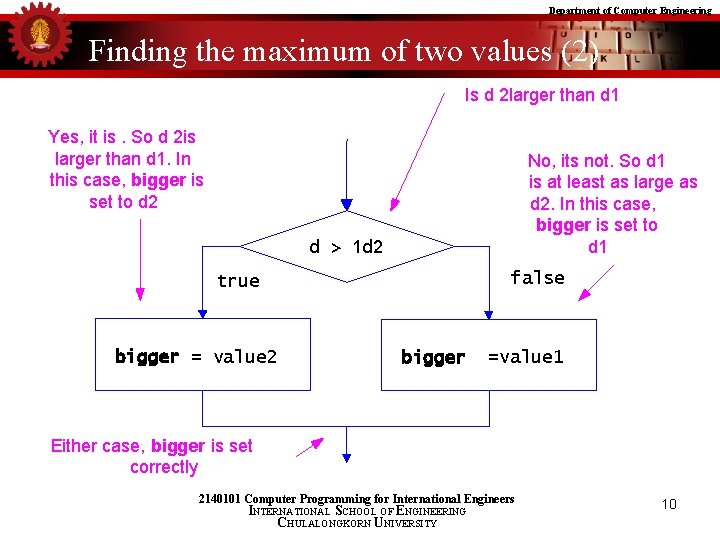
Department of Computer Engineering Finding the maximum of two values (2) Is d 2 larger than d 1 Yes, it is. So d 2 is larger than d 1. In this case, bigger is set to d 2 No, its not. So d 1 is at least as large as d 2. In this case, bigger is set to d 1 d > 1 d 2 false true bigger = value 2 bigger =value 1 Either case, bigger is set correctly 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 10
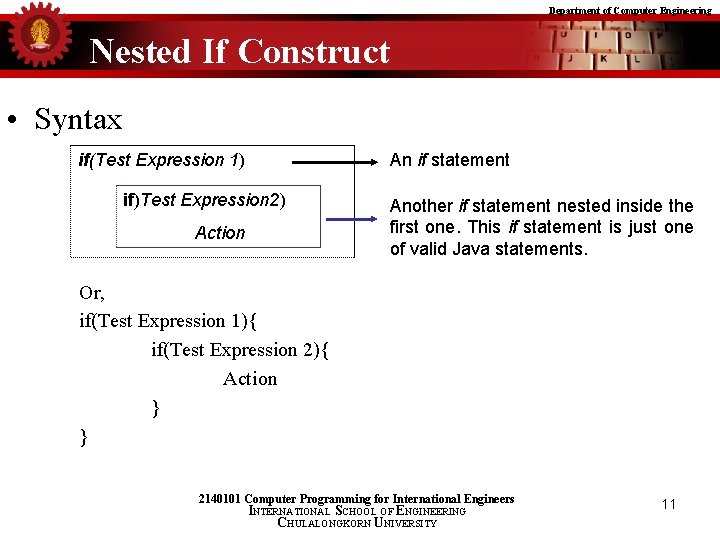
Department of Computer Engineering Nested If Construct • Syntax if(Test Expression 1) An if statement if)Test Expression 2) Action Another if statement nested inside the first one. This if statement is just one of valid Java statements. Or, if(Test Expression 1){ if(Test Expression 2){ Action } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 11
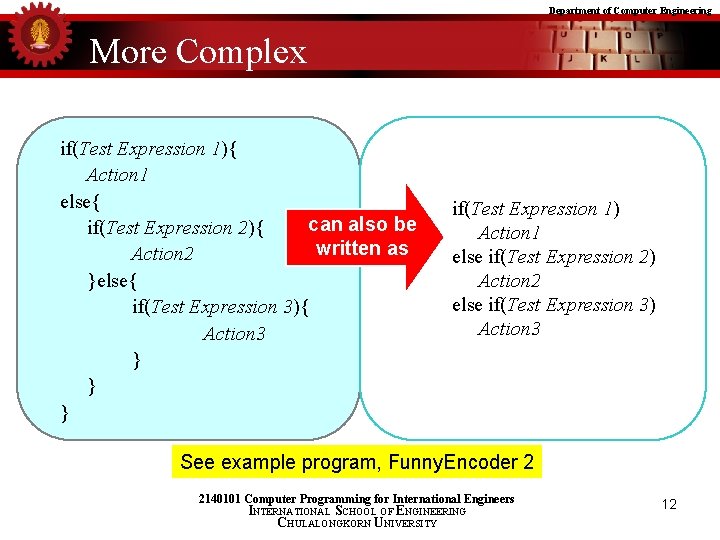
Department of Computer Engineering More Complex if(Test Expression 1){ Action 1 else{ can also be if(Test Expression 2){ written as Action 2 }else{ if(Test Expression 3){ Action 3 } } } if(Test Expression 1) Action 1 else if(Test Expression 2) Action 2 else if(Test Expression 3) Action 3 See example program, Funny. Encoder 2 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 12
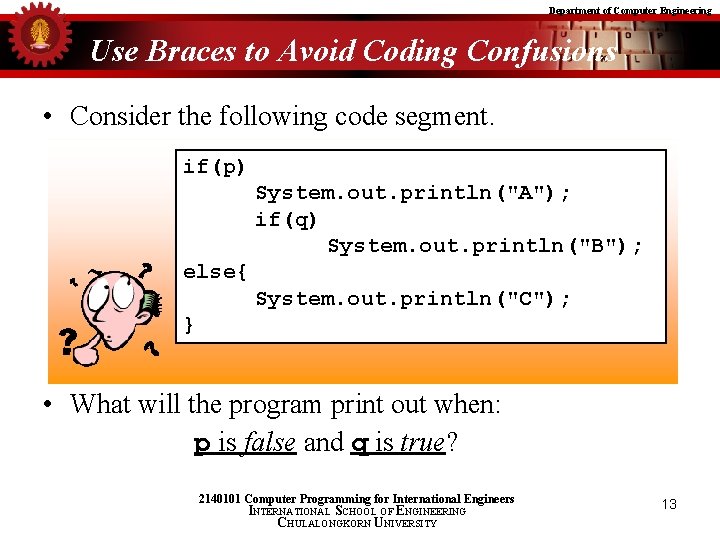
Department of Computer Engineering Use Braces to Avoid Coding Confusions • Consider the following code segment. if(p) System. out. println("A"); if(q) System. out. println("B"); else{ System. out. println("C"); } • What will the program print out when: p is false and q is true? 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 13
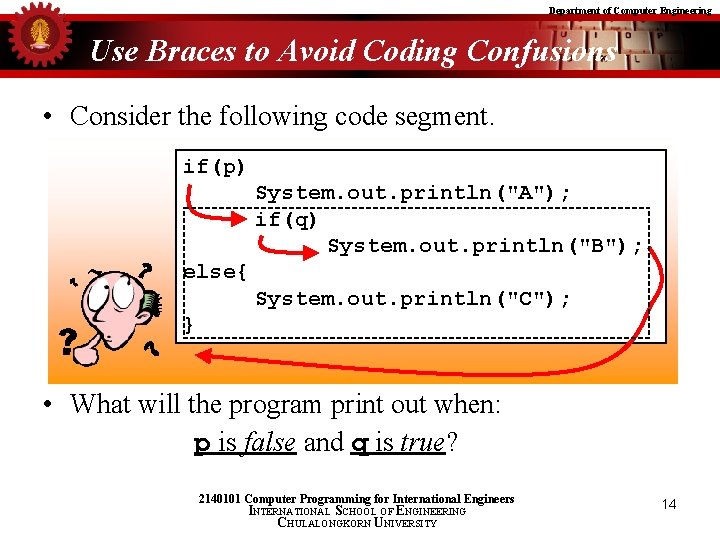
Department of Computer Engineering Use Braces to Avoid Coding Confusions • Consider the following code segment. if(p) System. out. println("A"); if(q) System. out. println("B"); else{ System. out. println("C"); } • What will the program print out when: p is false and q is true? 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 14
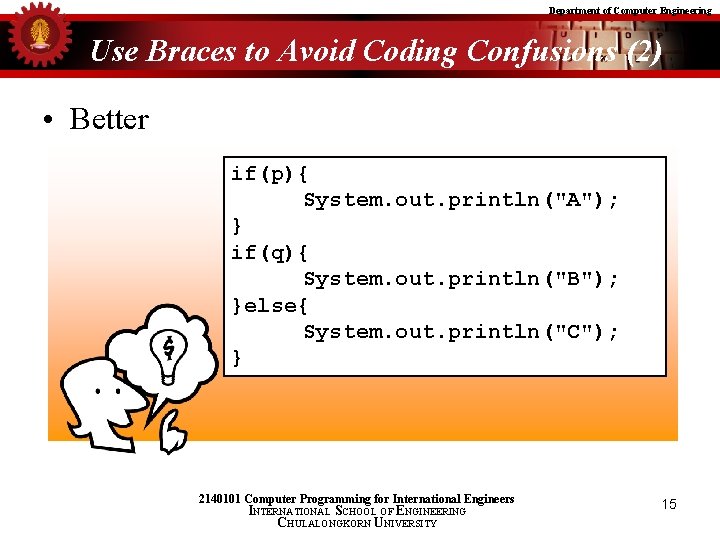
Department of Computer Engineering Use Braces to Avoid Coding Confusions (2) • Better if(p){ System. out. println("A"); } if(q){ System. out. println("B"); }else{ System. out. println("C"); } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 15
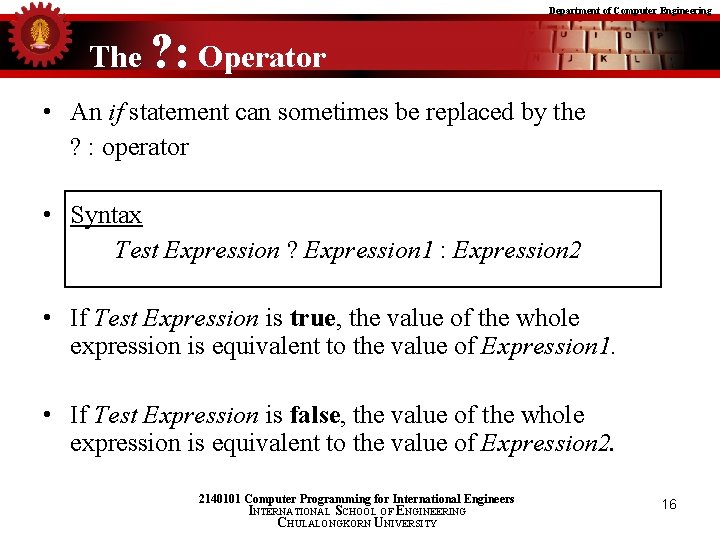
Department of Computer Engineering The ? : Operator • An if statement can sometimes be replaced by the ? : operator • Syntax Test Expression ? Expression 1 : Expression 2 • If Test Expression is true, the value of the whole expression is equivalent to the value of Expression 1. • If Test Expression is false, the value of the whole expression is equivalent to the value of Expression 2. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 16
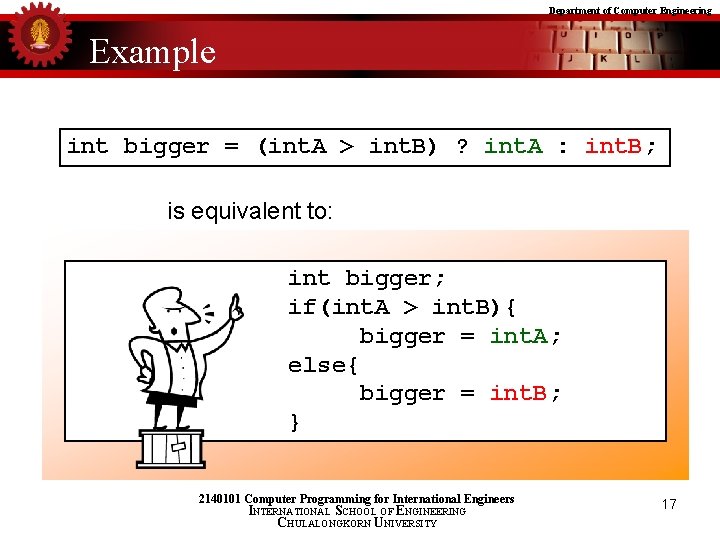
Department of Computer Engineering Example int bigger = (int. A > int. B) ? int. A : int. B; is equivalent to: int bigger; if(int. A > int. B){ bigger = int. A; else{ bigger = int. B; } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 17
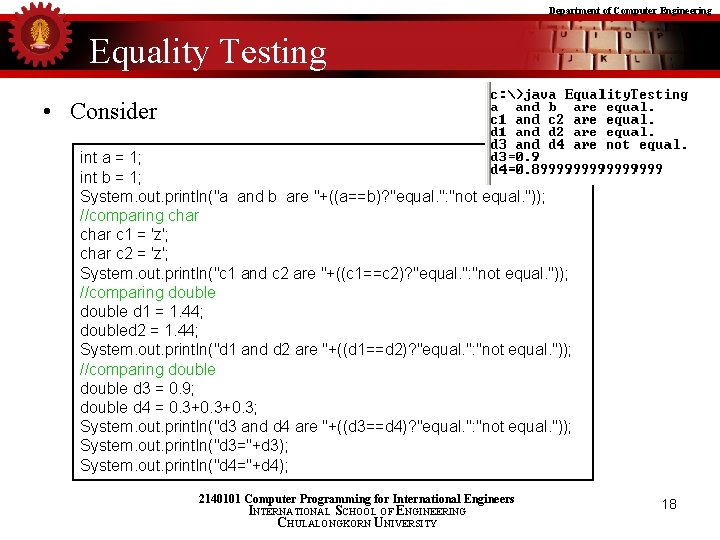
Department of Computer Engineering Equality Testing • Consider int a = 1; int b = 1; System. out. println("a and b are "+((a==b)? "equal. ": "not equal. ")); //comparing char c 1 = 'z'; char c 2 = 'z'; System. out. println("c 1 and c 2 are "+((c 1==c 2)? "equal. ": "not equal. ")); //comparing double d 1 = 1. 44; doubled 2 = 1. 44; System. out. println("d 1 and d 2 are "+((d 1==d 2)? "equal. ": "not equal. ")); //comparing double d 3 = 0. 9; double d 4 = 0. 3+0. 3; System. out. println("d 3 and d 4 are "+((d 3==d 4)? "equal. ": "not equal. ")); System. out. println("d 3="+d 3); System. out. println("d 4="+d 4); 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 18
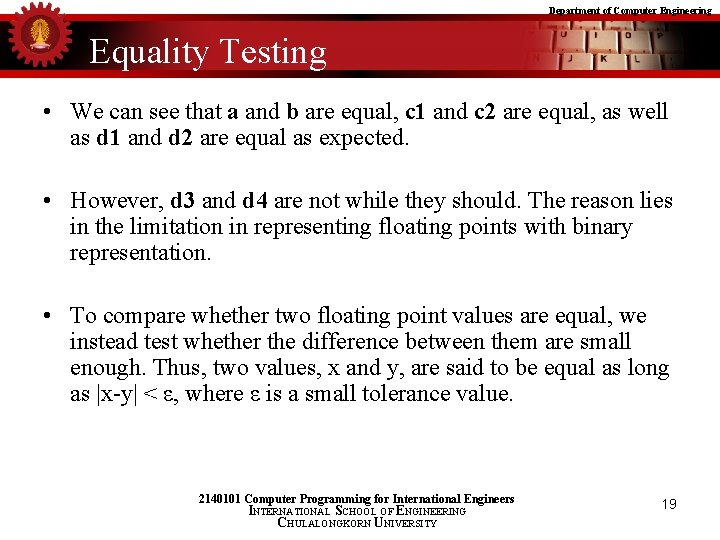
Department of Computer Engineering Equality Testing • We can see that a and b are equal, c 1 and c 2 are equal, as well as d 1 and d 2 are equal as expected. • However, d 3 and d 4 are not while they should. The reason lies in the limitation in representing floating points with binary representation. • To compare whether two floating point values are equal, we instead test whether the difference between them are small enough. Thus, two values, x and y, are said to be equal as long as |x-y| < ε, where ε is a small tolerance value. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 19
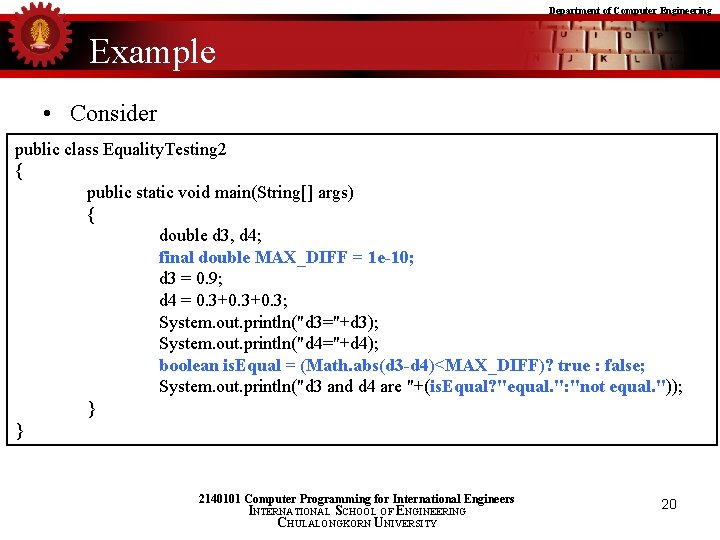
Department of Computer Engineering Example • Consider public class Equality. Testing 2 { public static void main(String[] args) { double d 3, d 4; final double MAX_DIFF = 1 e-10; d 3 = 0. 9; d 4 = 0. 3+0. 3; System. out. println("d 3="+d 3); System. out. println("d 4="+d 4); boolean is. Equal = (Math. abs(d 3 -d 4)<MAX_DIFF)? true : false; System. out. println("d 3 and d 4 are "+(is. Equal? "equal. ": "not equal. ")); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 20
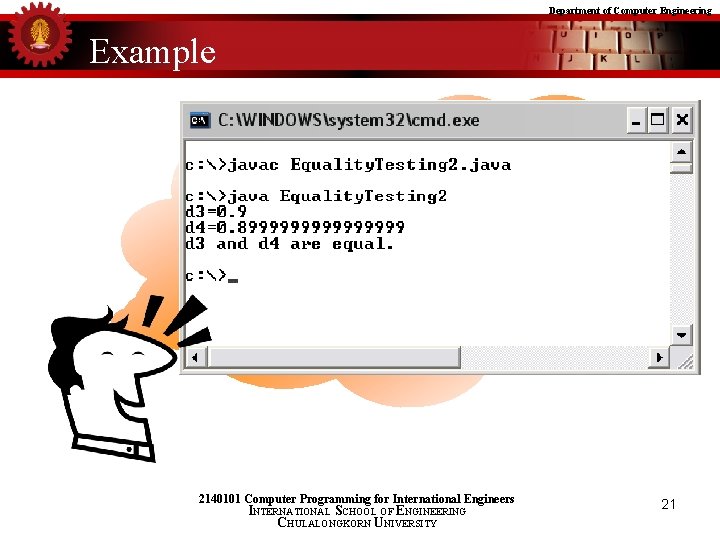
Department of Computer Engineering Example 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 21
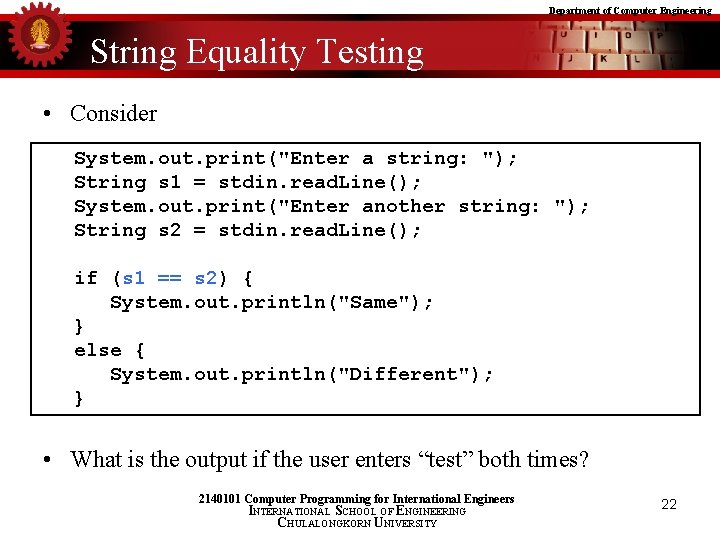
Department of Computer Engineering String Equality Testing • Consider System. out. print("Enter a string: "); String s 1 = stdin. read. Line(); System. out. print("Enter another string: "); String s 2 = stdin. read. Line(); if (s 1 == s 2) { System. out. println("Same"); } else { System. out. println("Different"); } • What is the output if the user enters “test” both times? 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 22
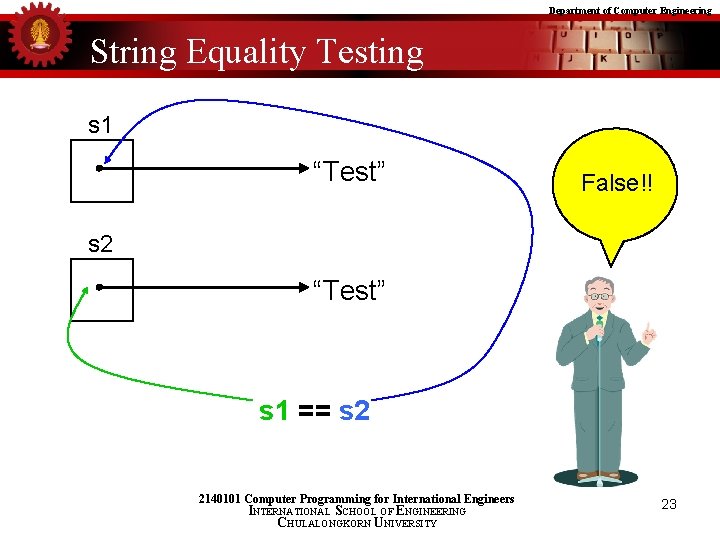
Department of Computer Engineering String Equality Testing s 1 “Test” False!! s 2 “Test” s 1 == s 2 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 23
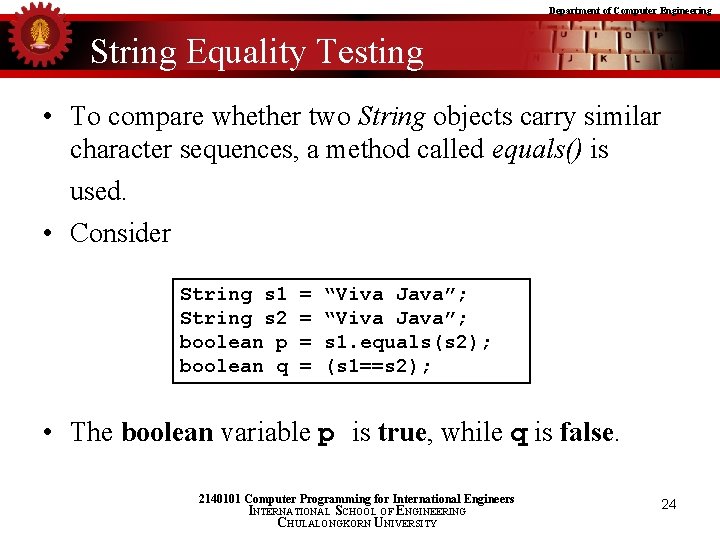
Department of Computer Engineering String Equality Testing • To compare whether two String objects carry similar character sequences, a method called equals() is used. • Consider String s 1 String s 2 boolean p boolean q = = “Viva Java”; s 1. equals(s 2); (s 1==s 2); • The boolean variable p is true, while q is false. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 24
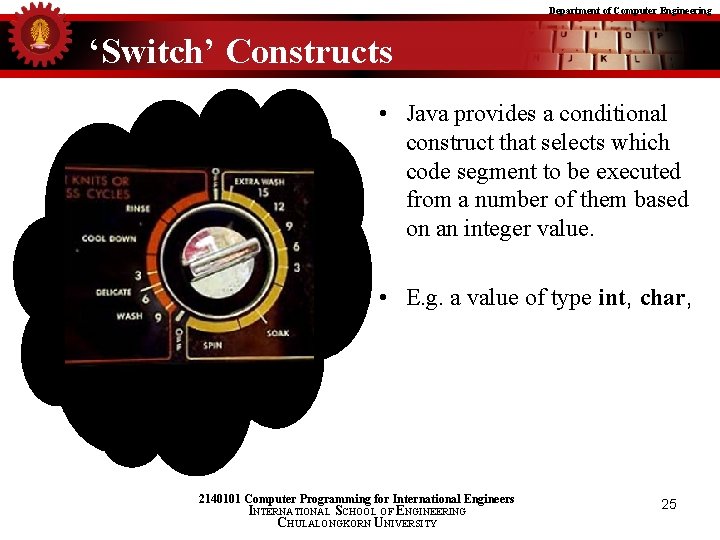
Department of Computer Engineering ‘Switch’ Constructs • Java provides a conditional construct that selects which code segment to be executed from a number of them based on an integer value. • E. g. a value of type int, char, 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 25
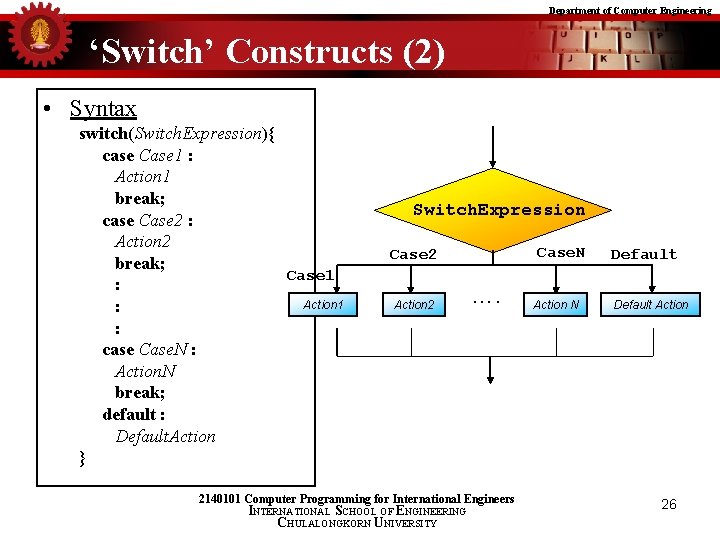
Department of Computer Engineering ‘Switch’ Constructs (2) • Syntax switch(Switch. Expression){ case Case 1 : Action 1 break; case Case 2 : Action 2 break; Case 1 : Action 1 : : case Case. N : Action. N break; default : Default. Action } Switch. Expression Case. N Case 2 Action 2 …. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY Action N Default Action 26
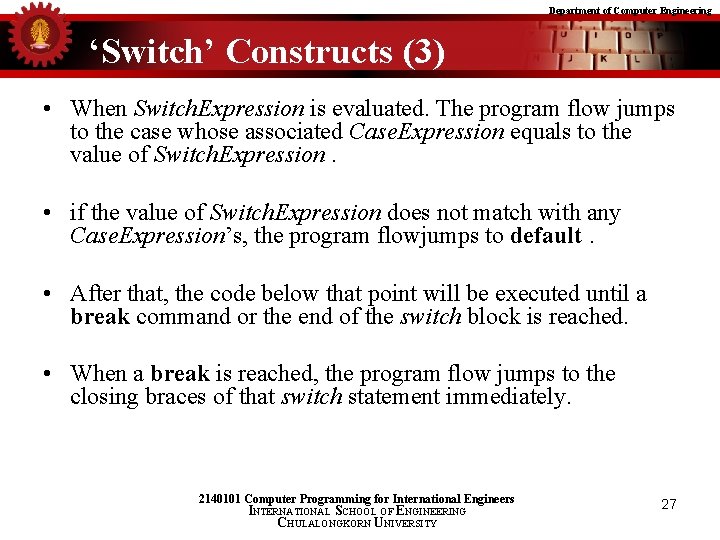
Department of Computer Engineering ‘Switch’ Constructs (3) • When Switch. Expression is evaluated. The program flow jumps to the case whose associated Case. Expression equals to the value of Switch. Expression. • if the value of Switch. Expression does not match with any Case. Expression’s, the program flowjumps to default. • After that, the code below that point will be executed until a break command or the end of the switch block is reached. • When a break is reached, the program flow jumps to the closing braces of that switch statement immediately. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 27
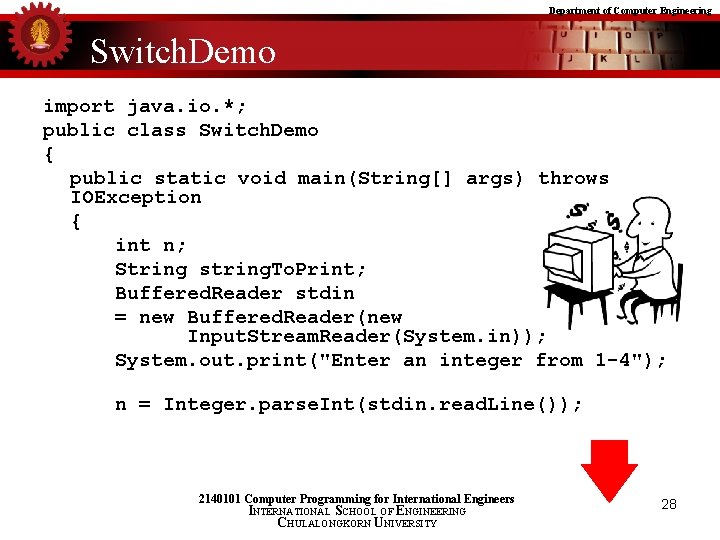
Department of Computer Engineering Switch. Demo import java. io. *; public class Switch. Demo { public static void main(String[] args) throws IOException { int n; String string. To. Print; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in)); System. out. print("Enter an integer from 1 -4"); n = Integer. parse. Int(stdin. read. Line()); 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 28
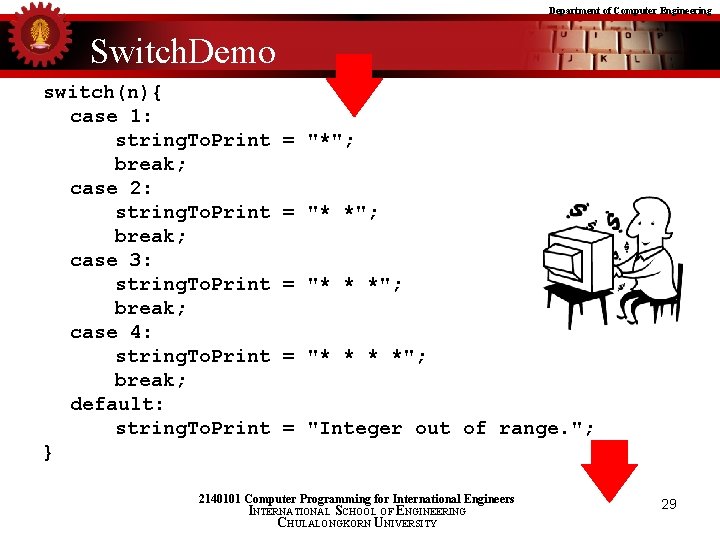
Department of Computer Engineering Switch. Demo switch(n){ case 1: string. To. Print break; case 2: string. To. Print break; case 3: string. To. Print break; case 4: string. To. Print break; default: string. To. Print } = "*"; = "* * * *"; = "Integer out of range. "; 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 29
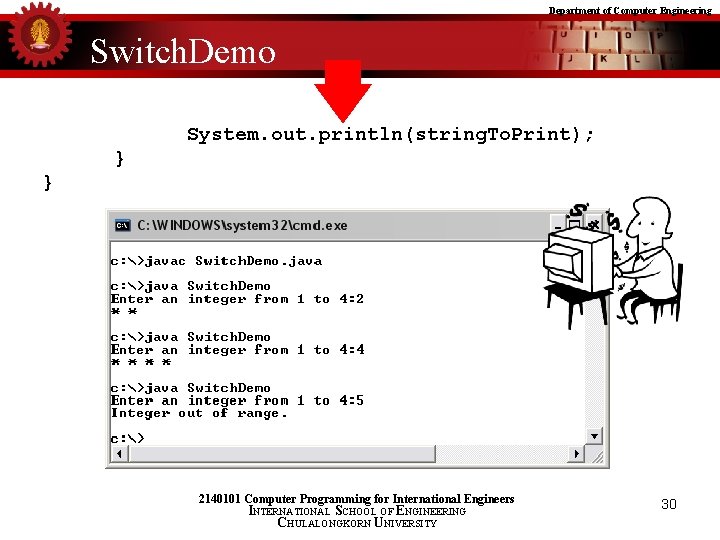
Department of Computer Engineering Switch. Demo System. out. println(string. To. Print); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 30
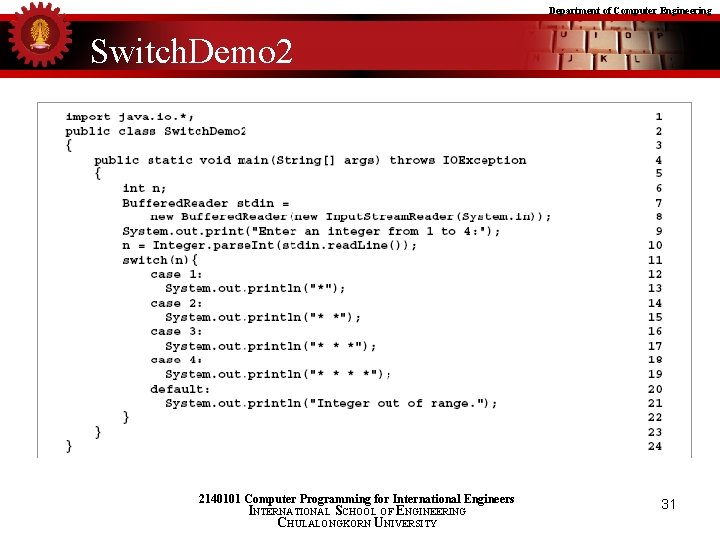
Department of Computer Engineering Switch. Demo 2 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 31
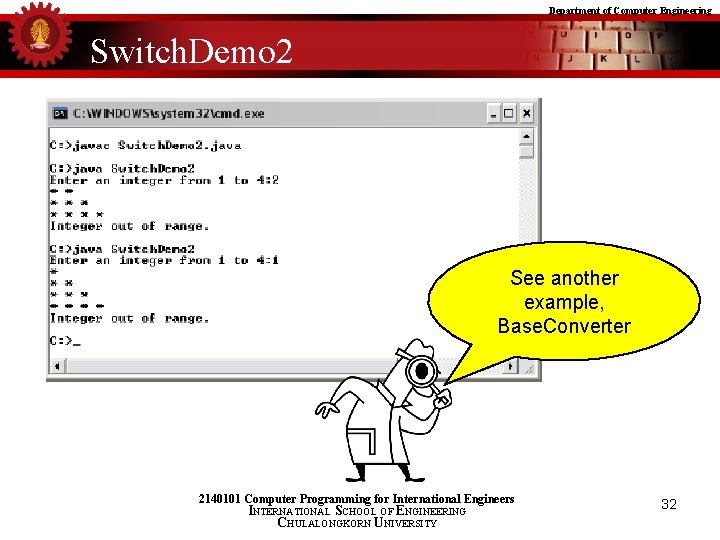
Department of Computer Engineering Switch. Demo 2 See another example, Base. Converter 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 32
Meaning of poster making
Screening decisions and preference decisions
Tum
Computer engineering department
Engineering economic decisions
What is system in software engineering
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Components of system programming
Integer programming vs linear programming
Programing adalah
Electrical engineering department
Engineering department hotel
City of houston design manual
Kpi for engineering department
Department of information engineering university of padova
Information engineering padova
Iit delhi material science
University of bridgeport computer science faculty
University of bridgeport computer science
Ucla electrical engineering department
University of sargodha engineering department
Extreme programming in software engineering
Mechanical engineering programming
Ucl computer science bsc
Northwestern computer science department
Computer science department rutgers
Meredith hutchin stanford
Fsu cs faculty
Trimentoring
Department of computer science christ
Webnis
Forward engineering and reverse engineering