Department of Computer Engineering Iterations 2140101 Computer Programming
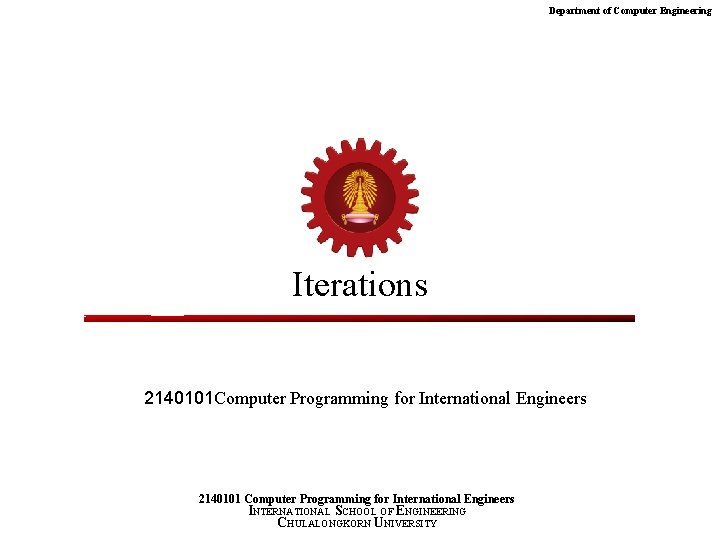
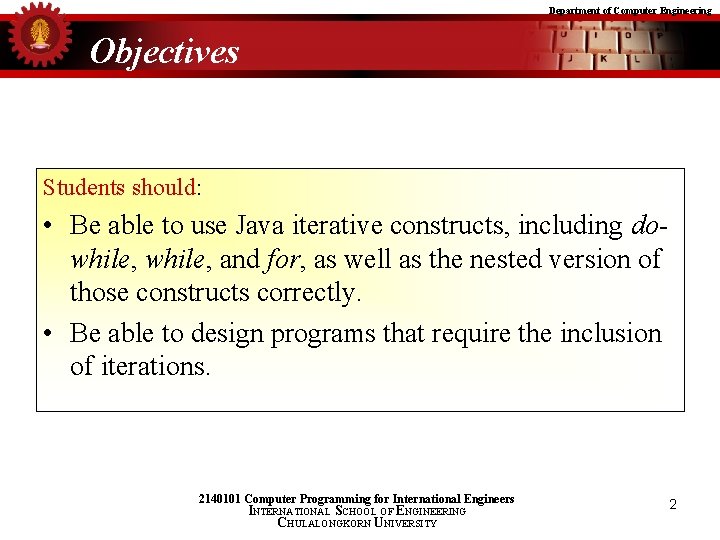
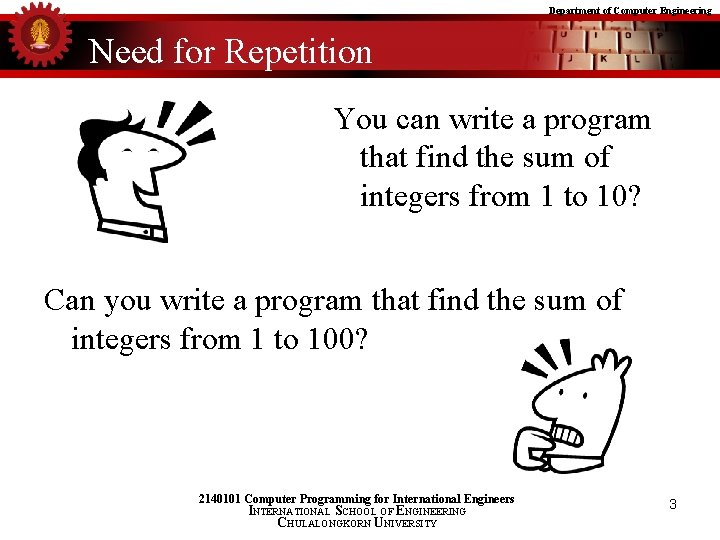
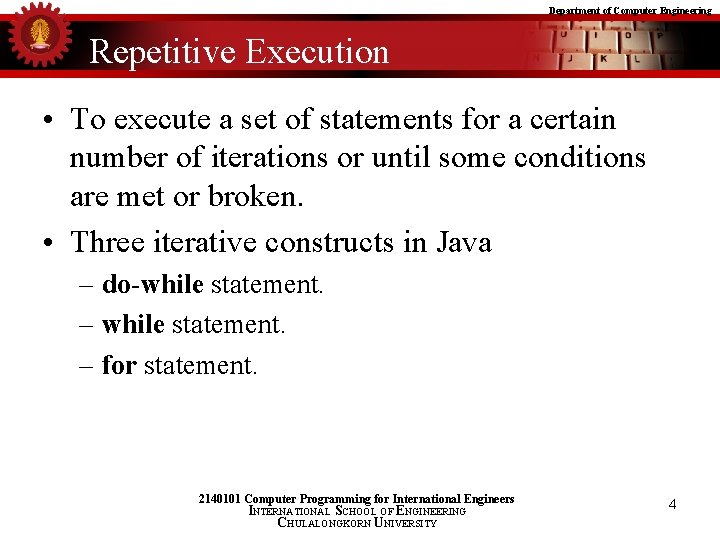
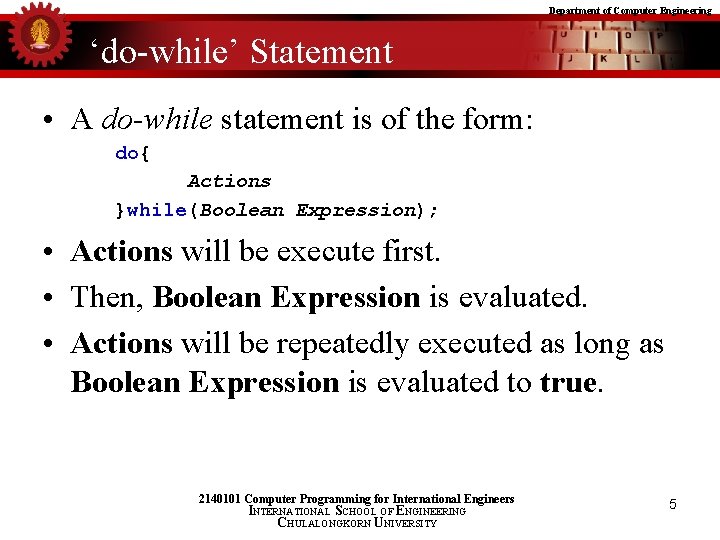
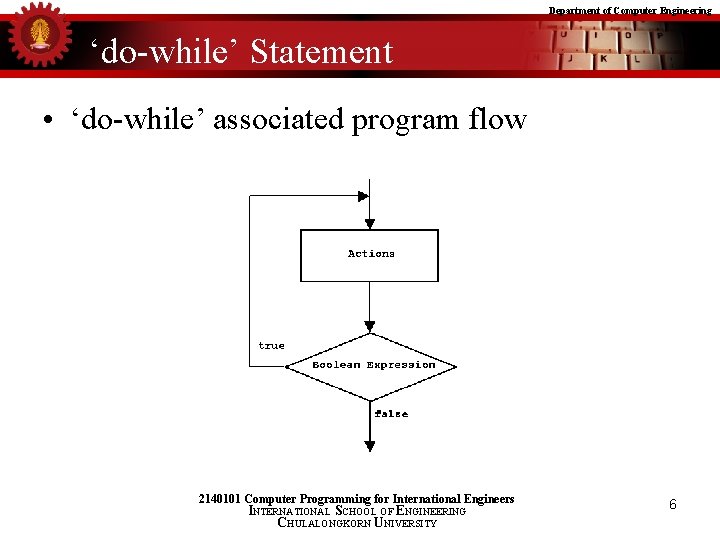
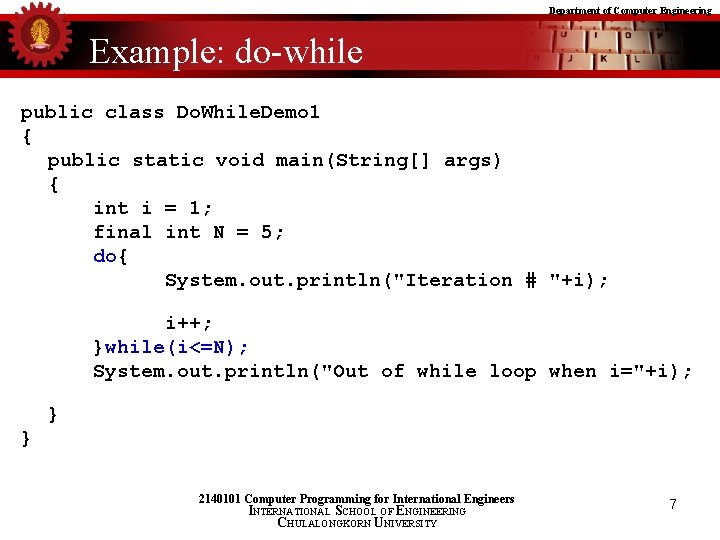
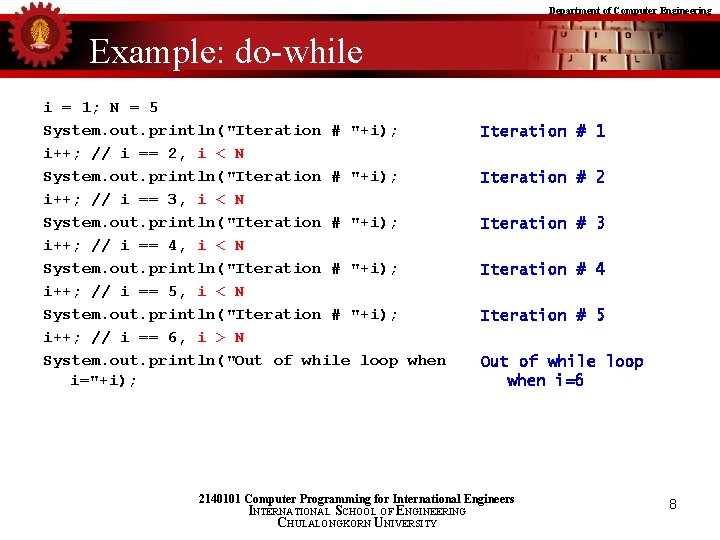
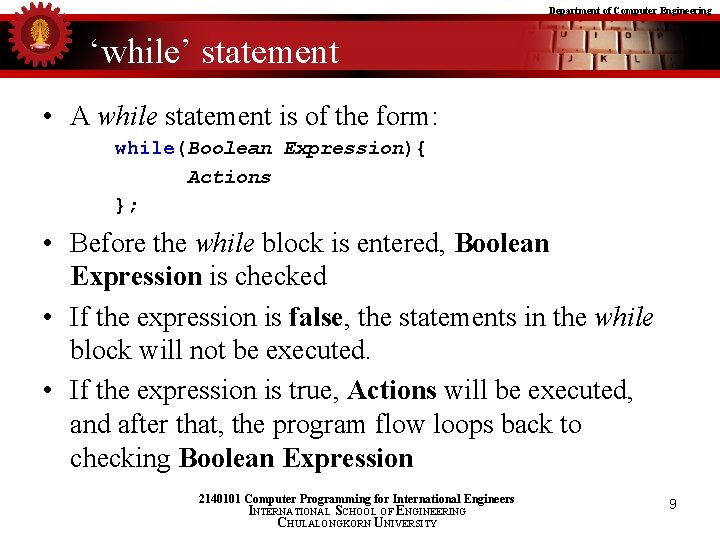
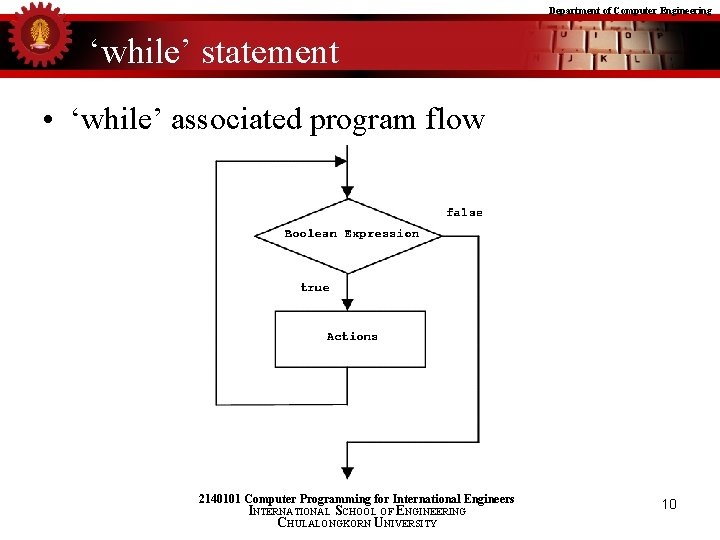
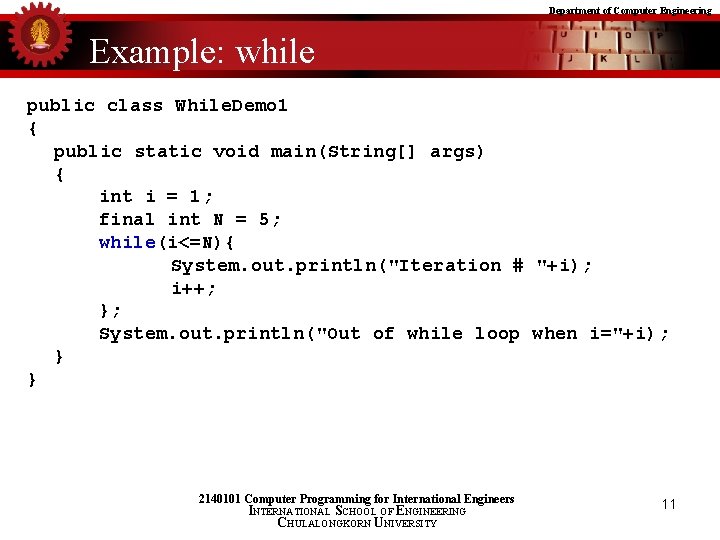
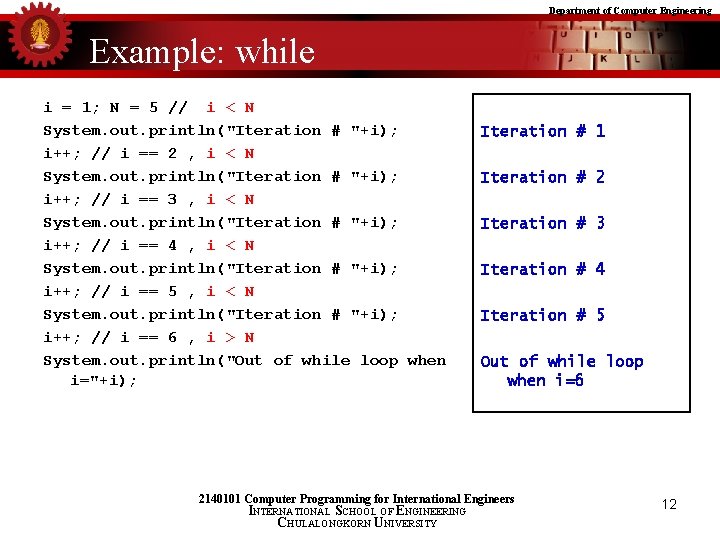
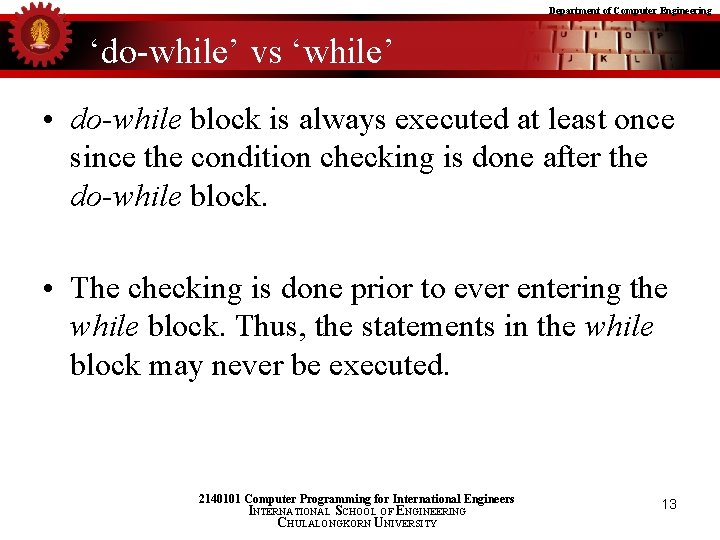
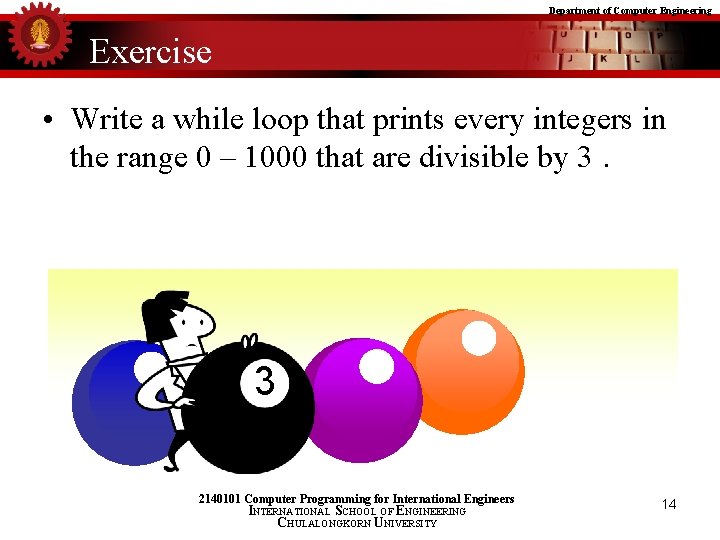
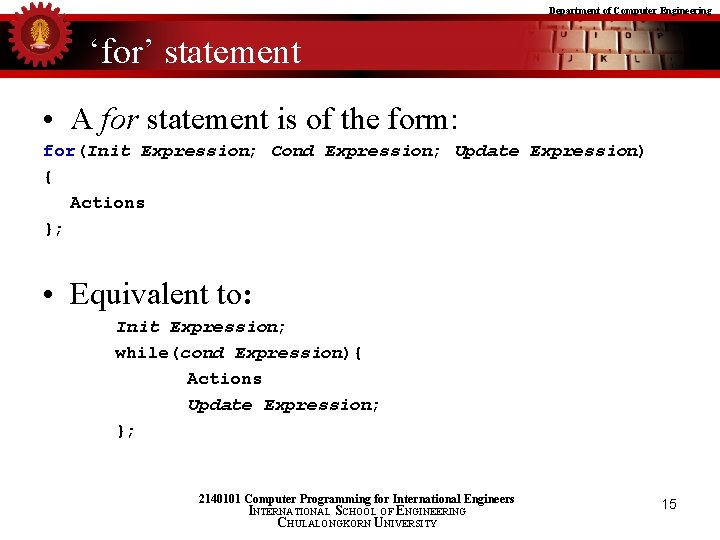
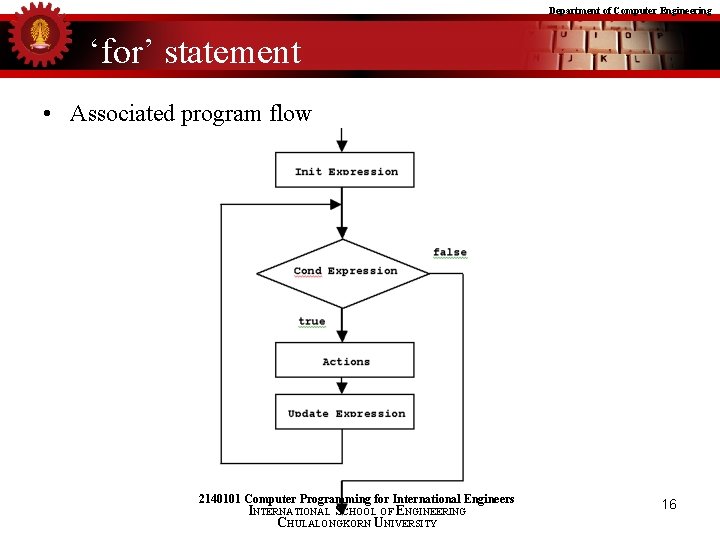
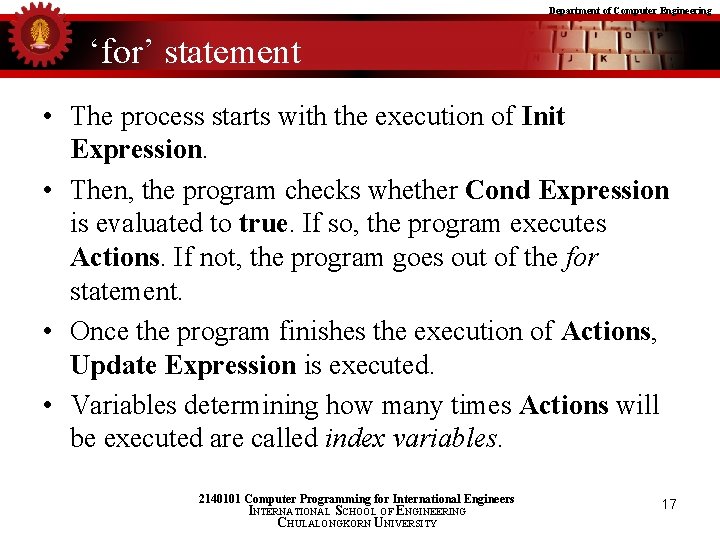
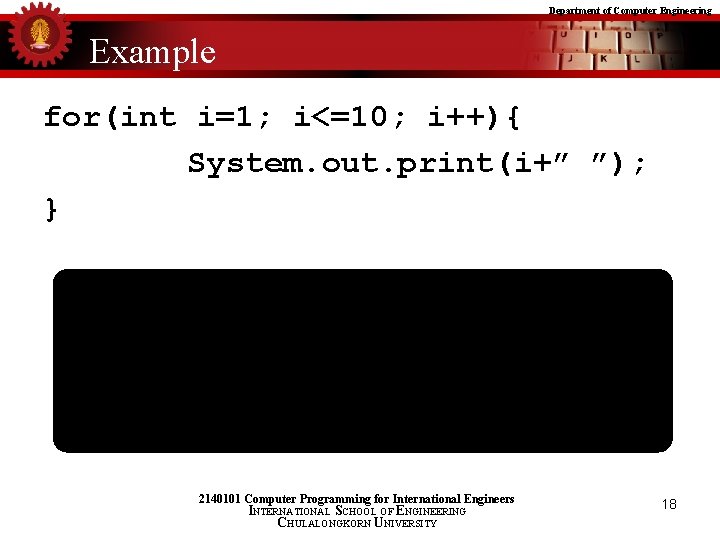
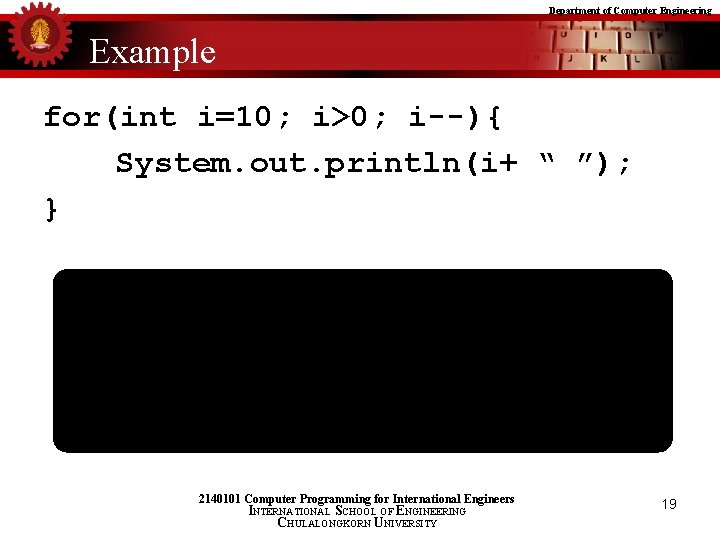
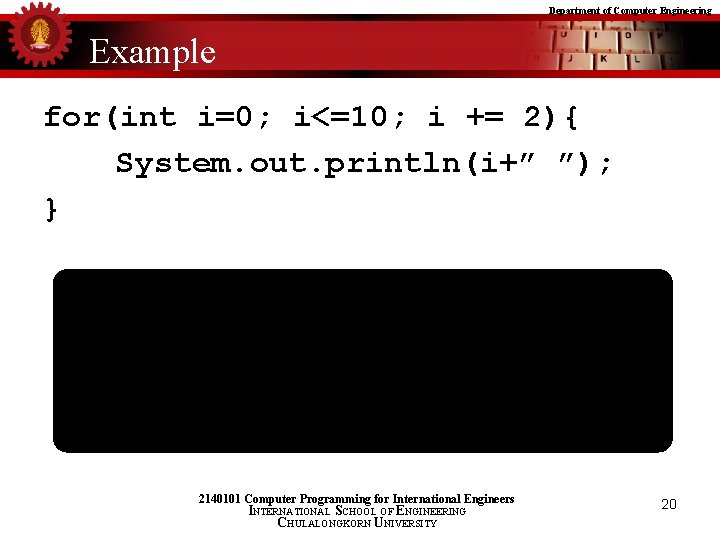
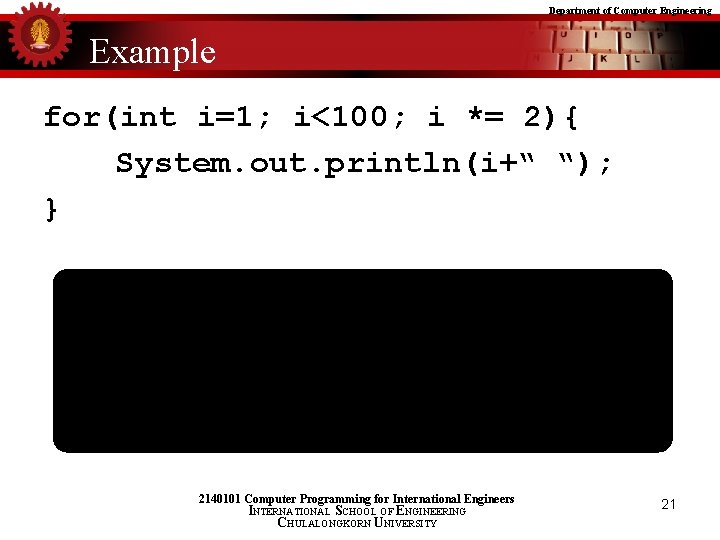
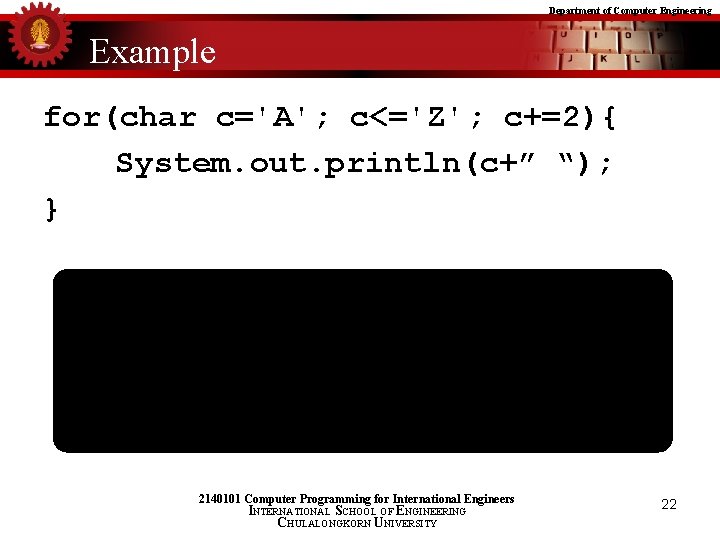
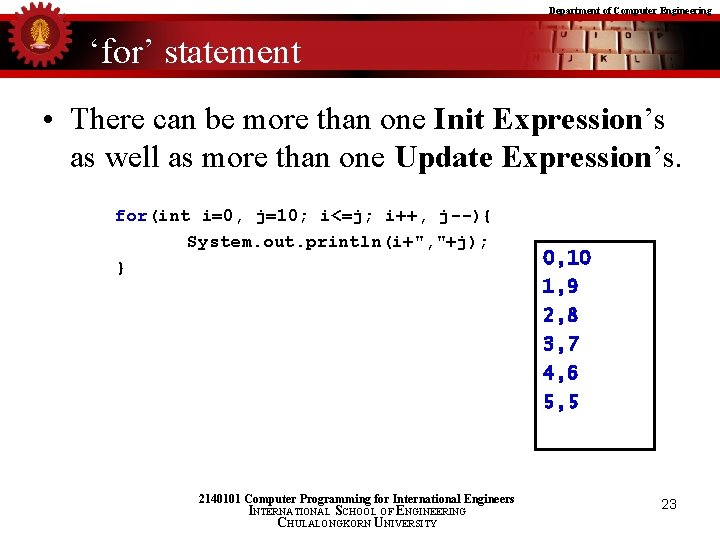
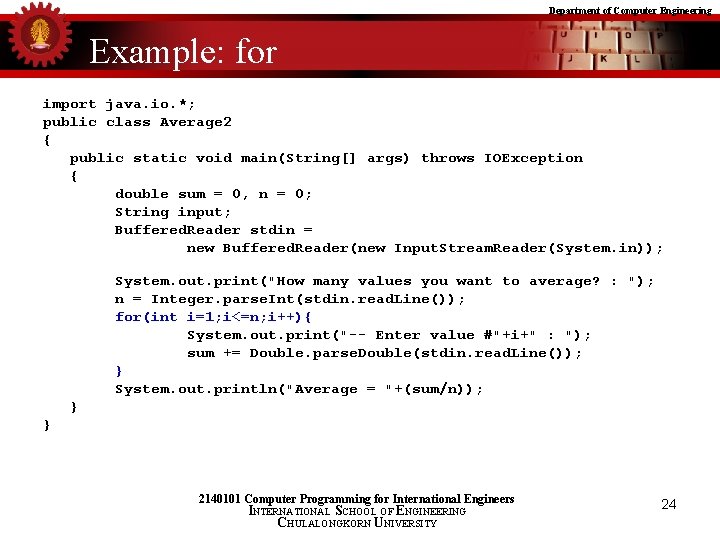
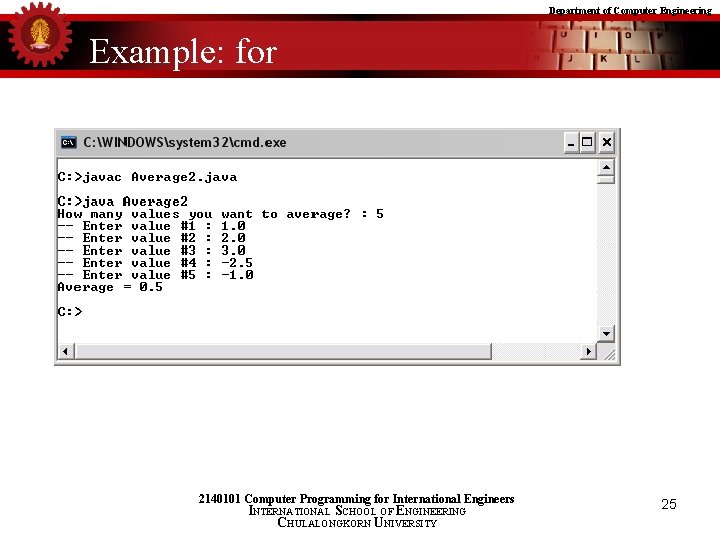
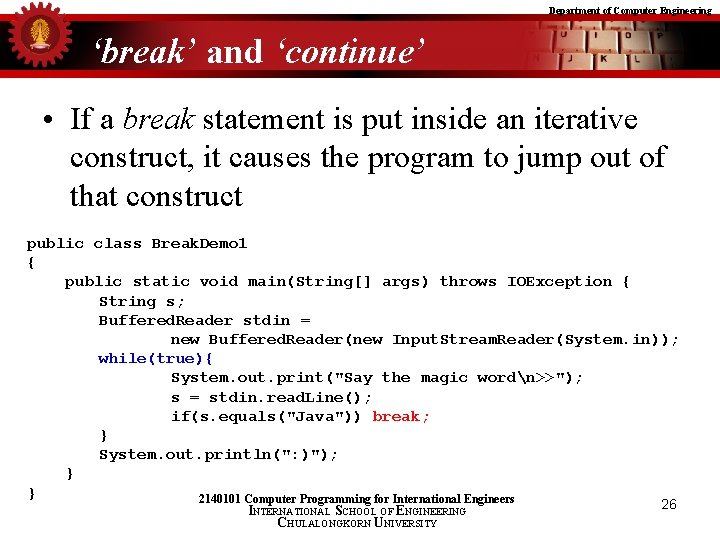
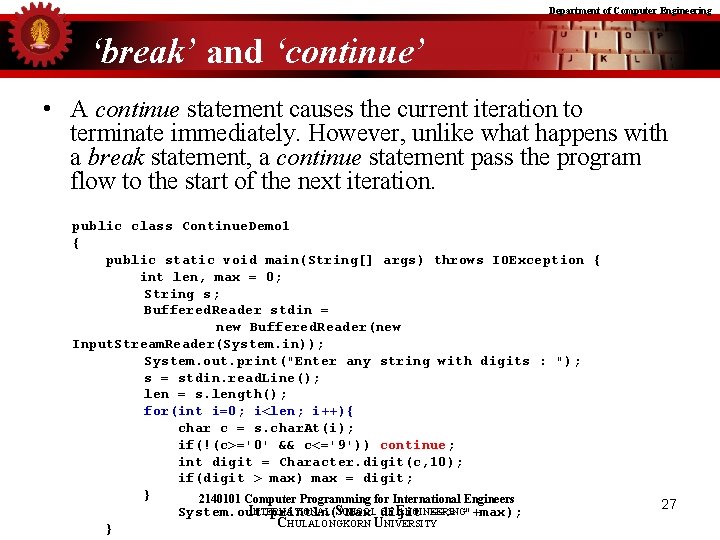
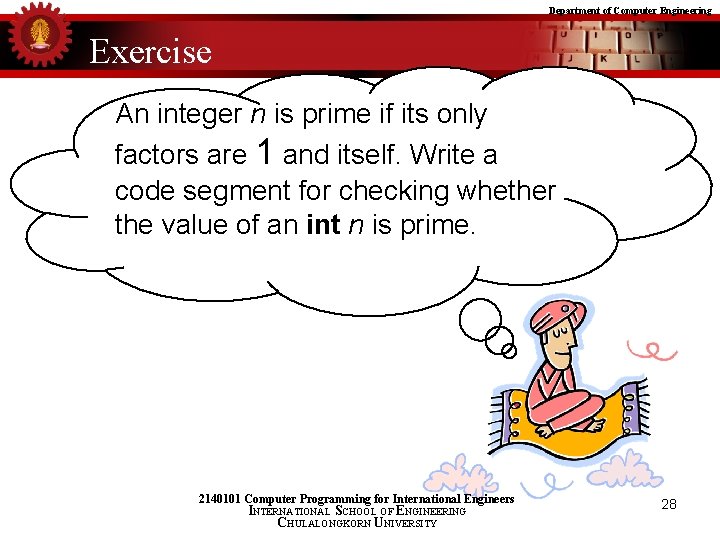
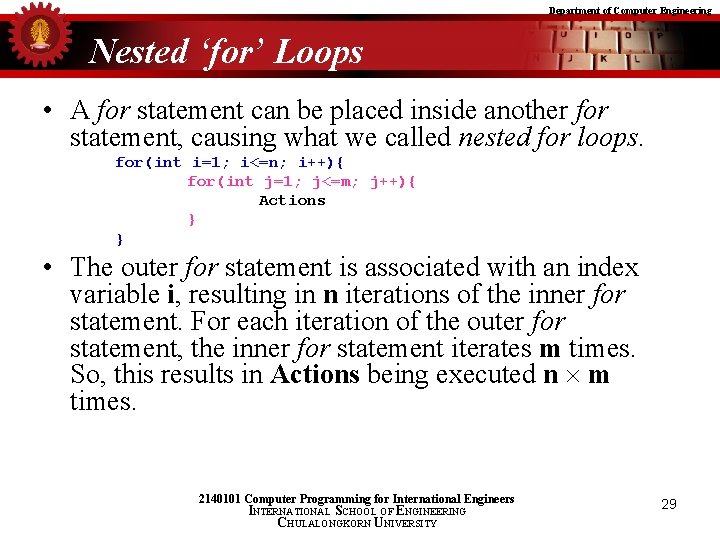
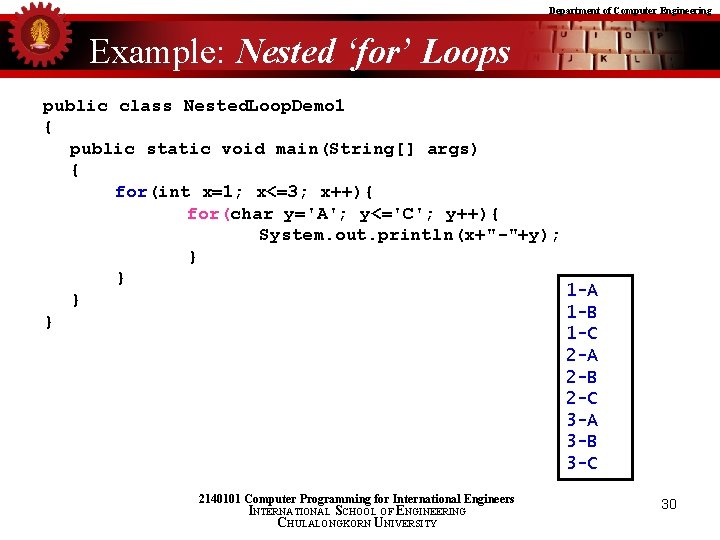
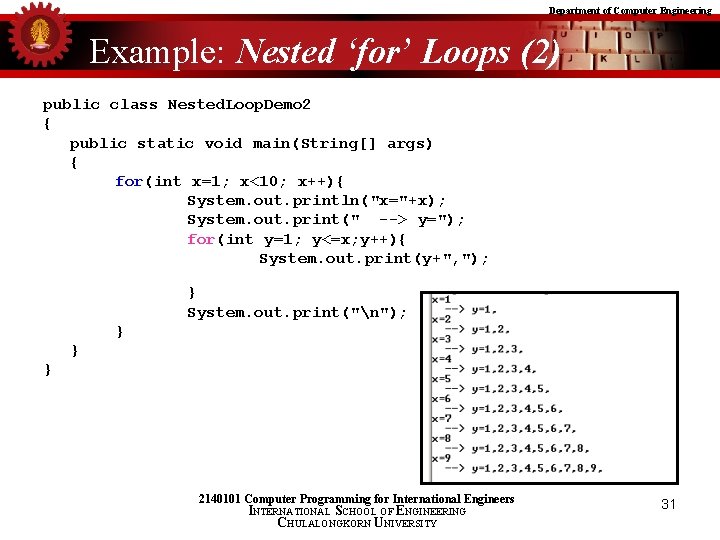
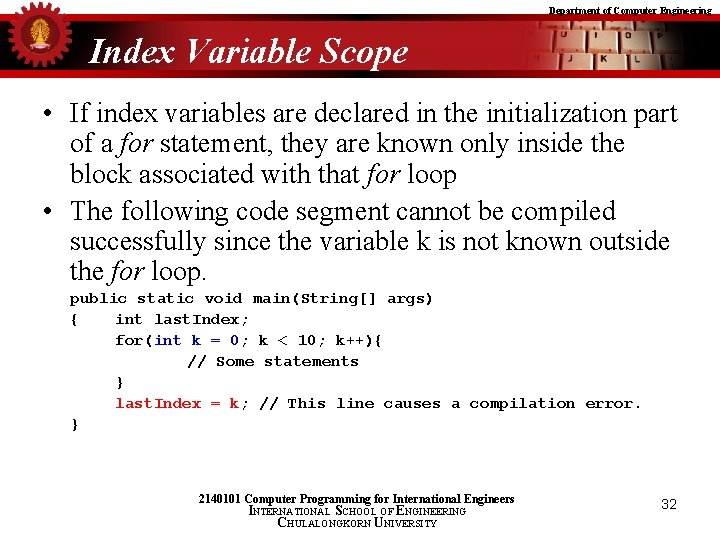
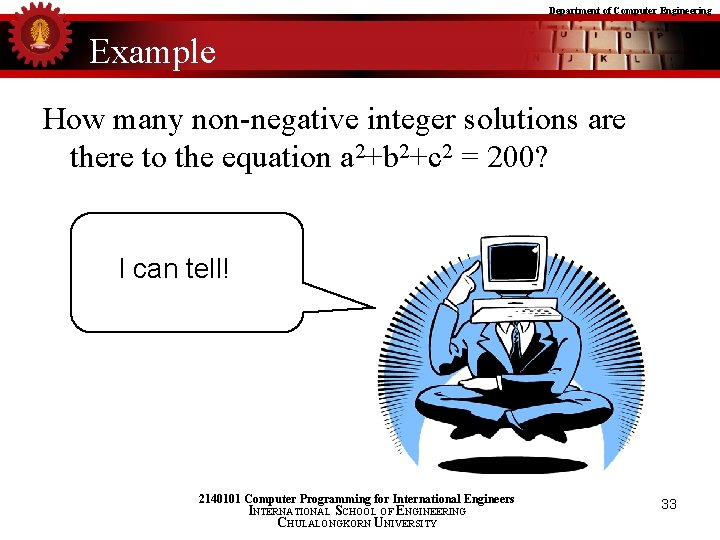
![Department of Computer Engineering Example public class Solutions. Count { public static void main(String[] Department of Computer Engineering Example public class Solutions. Count { public static void main(String[]](https://slidetodoc.com/presentation_image_h/0f36a387e46c6156d869bb9de957da8b/image-34.jpg)
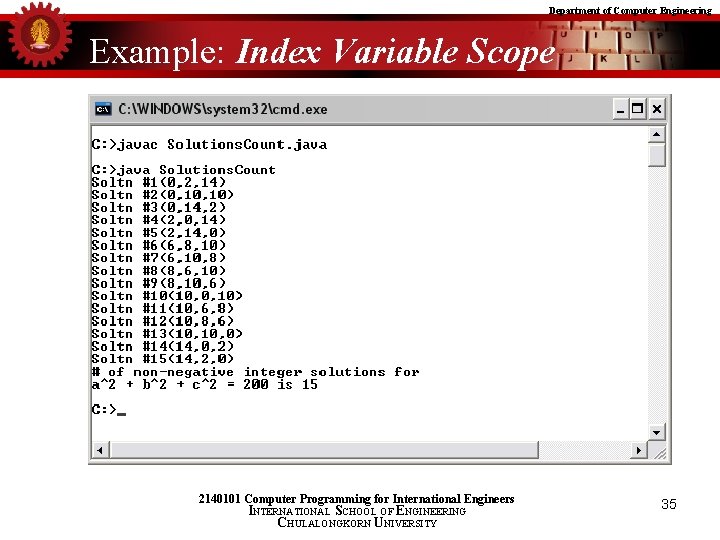
- Slides: 35
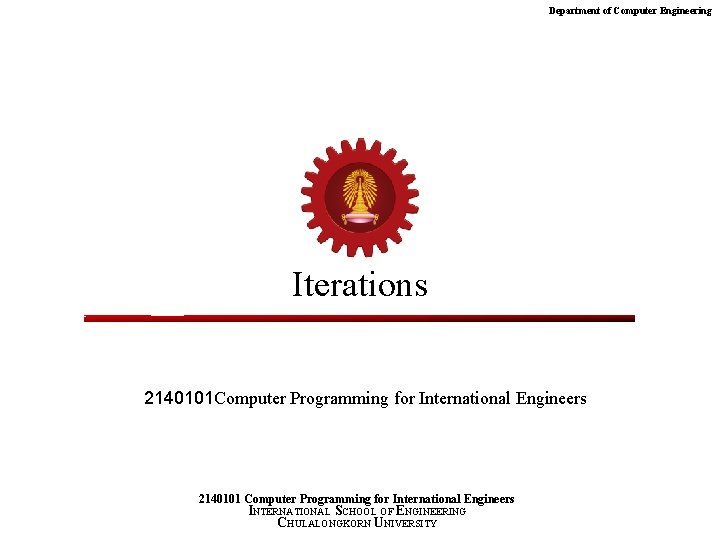
Department of Computer Engineering Iterations 2140101 Computer Programming for International Engineers 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY
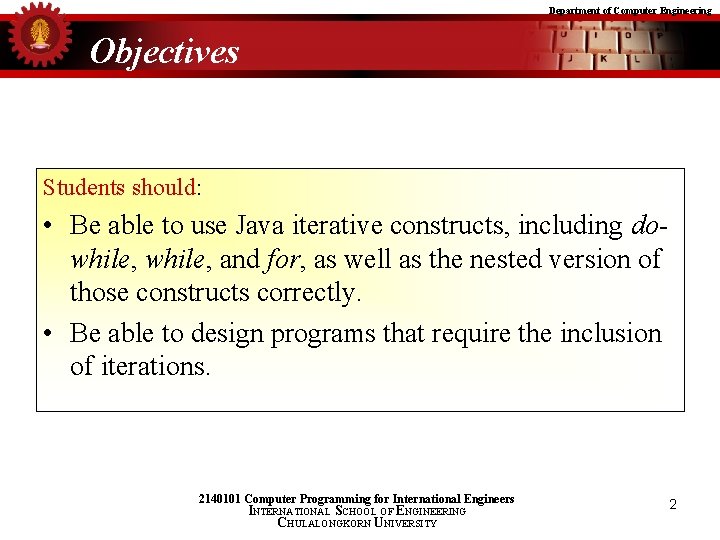
Department of Computer Engineering Objectives Students should: • Be able to use Java iterative constructs, including dowhile, and for, as well as the nested version of those constructs correctly. • Be able to design programs that require the inclusion of iterations. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 2
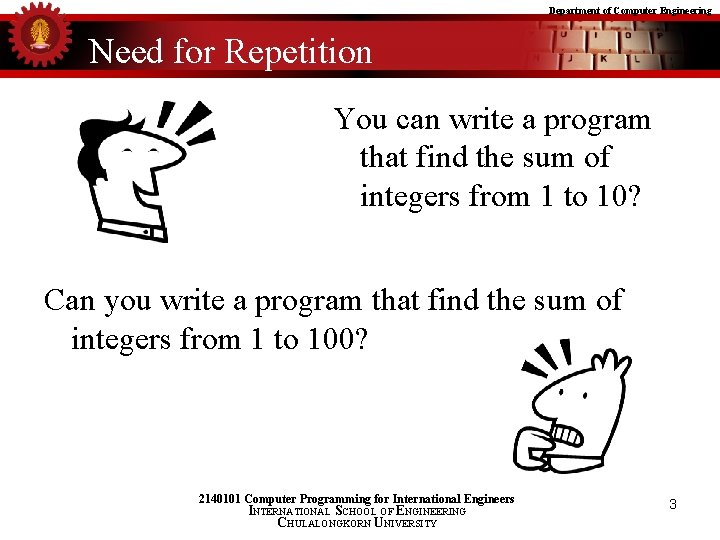
Department of Computer Engineering Need for Repetition You can write a program that find the sum of integers from 1 to 10? Can you write a program that find the sum of integers from 1 to 100? 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 3
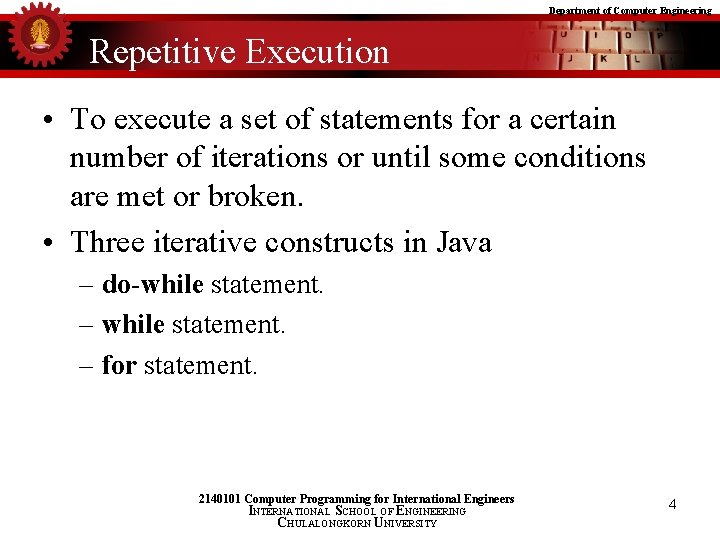
Department of Computer Engineering Repetitive Execution • To execute a set of statements for a certain number of iterations or until some conditions are met or broken. • Three iterative constructs in Java – do-while statement. – for statement. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 4
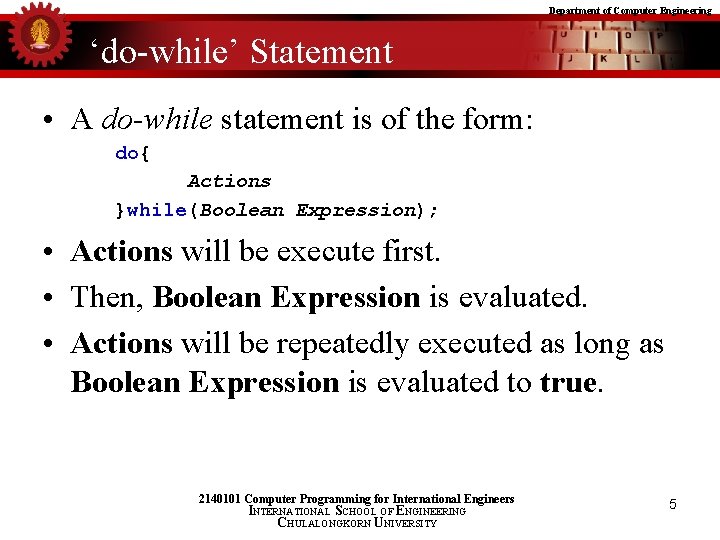
Department of Computer Engineering ‘do-while’ Statement • A do-while statement is of the form: do{ Actions }while(Boolean Expression); • Actions will be execute first. • Then, Boolean Expression is evaluated. • Actions will be repeatedly executed as long as Boolean Expression is evaluated to true. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 5
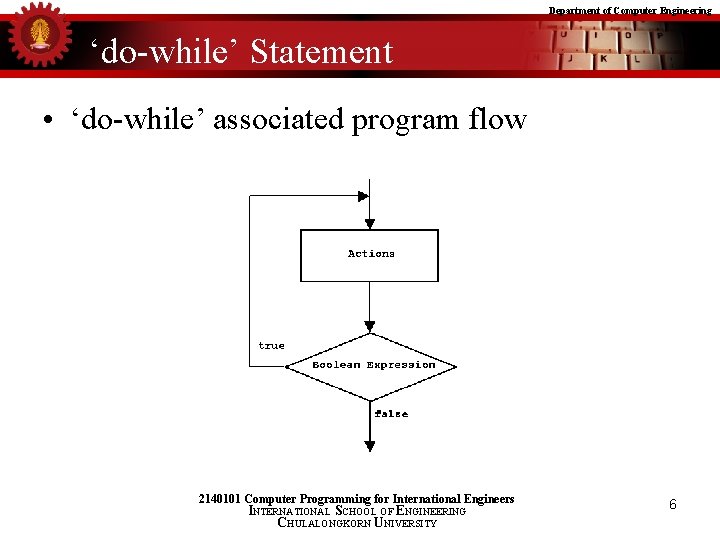
Department of Computer Engineering ‘do-while’ Statement • ‘do-while’ associated program flow 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 6
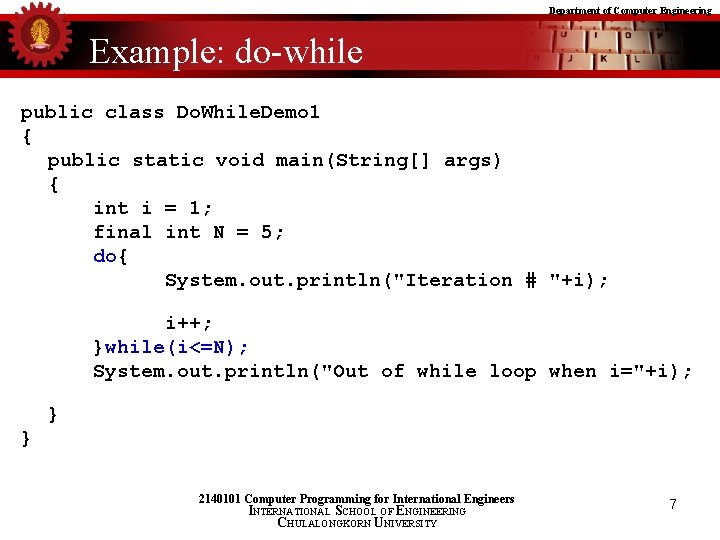
Department of Computer Engineering Example: do-while public class Do. While. Demo 1 { public static void main(String[] args) { int i = 1; final int N = 5; do{ System. out. println("Iteration # "+i); i++; }while(i<=N); System. out. println("Out of while loop when i="+i); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 7
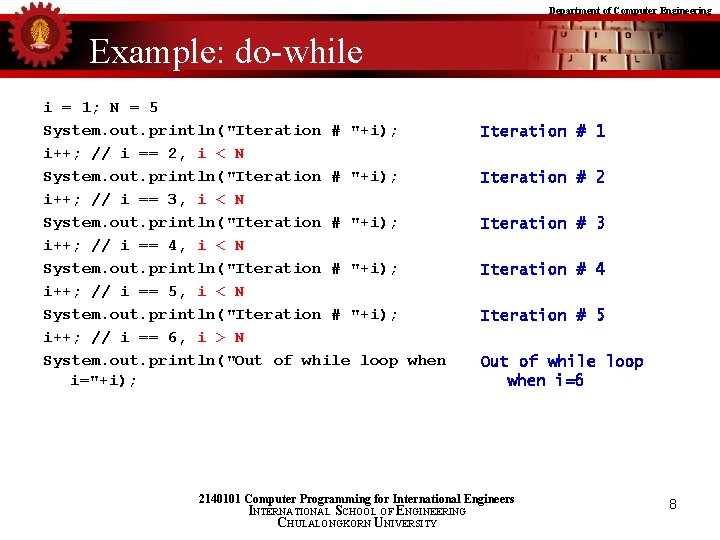
Department of Computer Engineering Example: do-while i = 1; N = 5 System. out. println("Iteration # "+i); i++; // i == 2, i < N System. out. println("Iteration # "+i); i++; // i == 3, i < N System. out. println("Iteration # "+i); i++; // i == 4, i < N System. out. println("Iteration # "+i); i++; // i == 5, i < N System. out. println("Iteration # "+i); i++; // i == 6, i > N System. out. println("Out of while loop when i="+i); Iteration # 1 Iteration # 2 Iteration # 3 Iteration # 4 Iteration # 5 Out of while loop when i=6 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 8
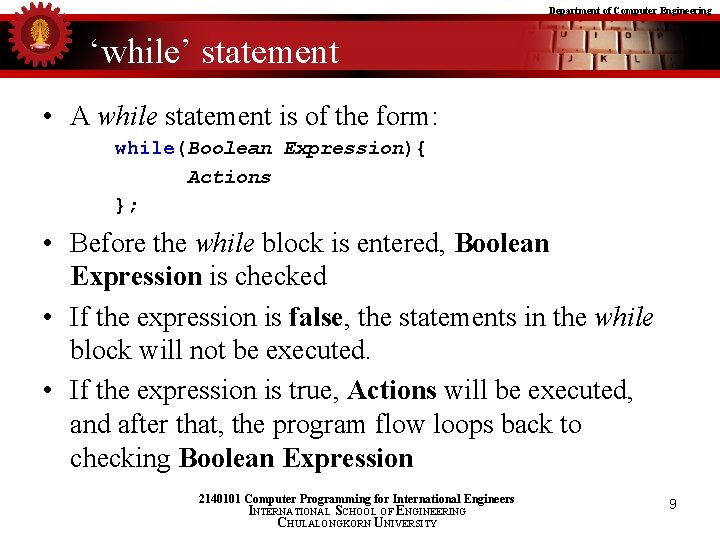
Department of Computer Engineering ‘while’ statement • A while statement is of the form: while(Boolean Expression){ Actions }; • Before the while block is entered, Boolean Expression is checked • If the expression is false, the statements in the while block will not be executed. • If the expression is true, Actions will be executed, and after that, the program flow loops back to checking Boolean Expression 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 9
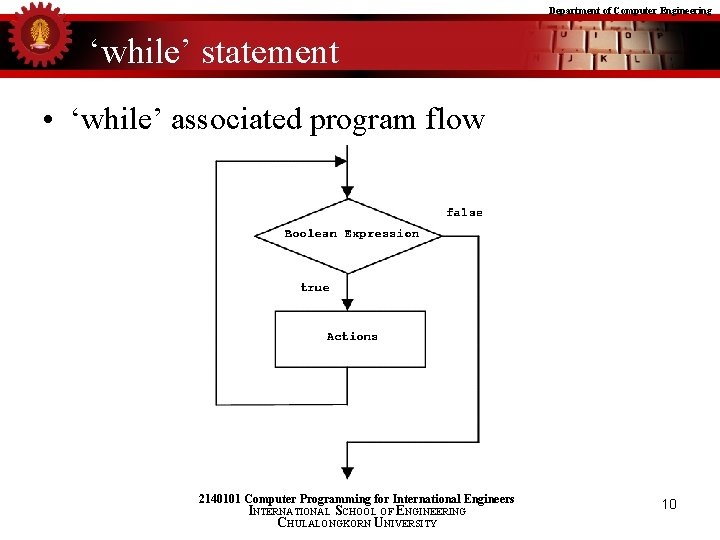
Department of Computer Engineering ‘while’ statement • ‘while’ associated program flow 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 10
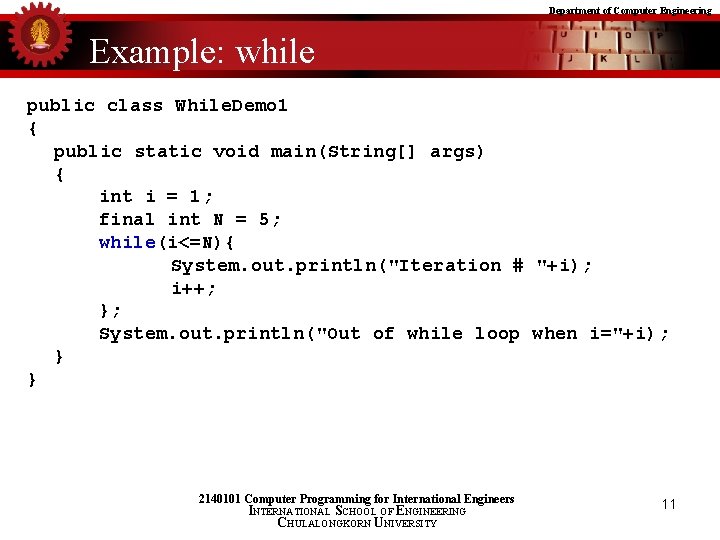
Department of Computer Engineering Example: while public class While. Demo 1 { public static void main(String[] args) { int i = 1; final int N = 5; while(i<=N){ System. out. println("Iteration # "+i); i++; }; System. out. println("Out of while loop when i="+i); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 11
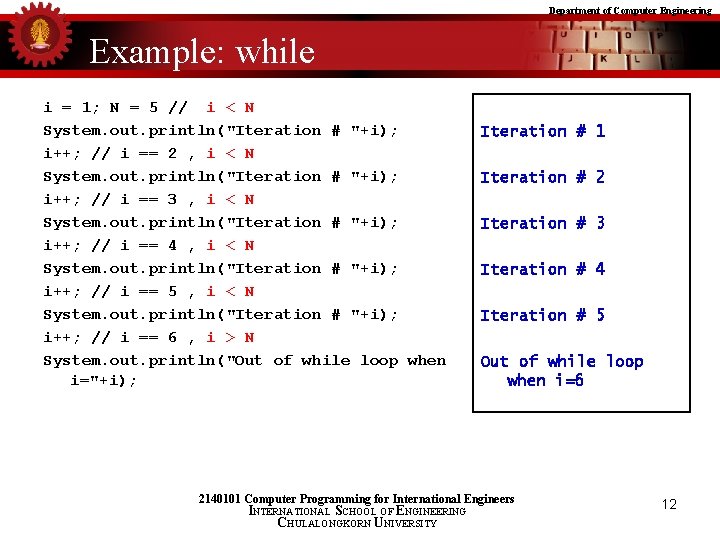
Department of Computer Engineering Example: while i = 1; N = 5 // i < N System. out. println("Iteration # "+i); i++; // i == 2 , i < N System. out. println("Iteration # "+i); i++; // i == 3 , i < N System. out. println("Iteration # "+i); i++; // i == 4 , i < N System. out. println("Iteration # "+i); i++; // i == 5 , i < N System. out. println("Iteration # "+i); i++; // i == 6 , i > N System. out. println("Out of while loop when i="+i); Iteration # 1 Iteration # 2 Iteration # 3 Iteration # 4 Iteration # 5 Out of while loop when i=6 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 12
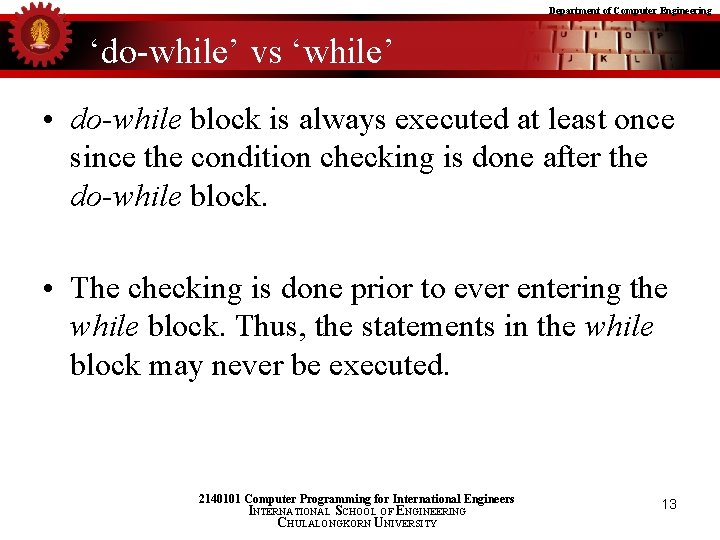
Department of Computer Engineering ‘do-while’ vs ‘while’ • do-while block is always executed at least once since the condition checking is done after the do-while block. • The checking is done prior to ever entering the while block. Thus, the statements in the while block may never be executed. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 13
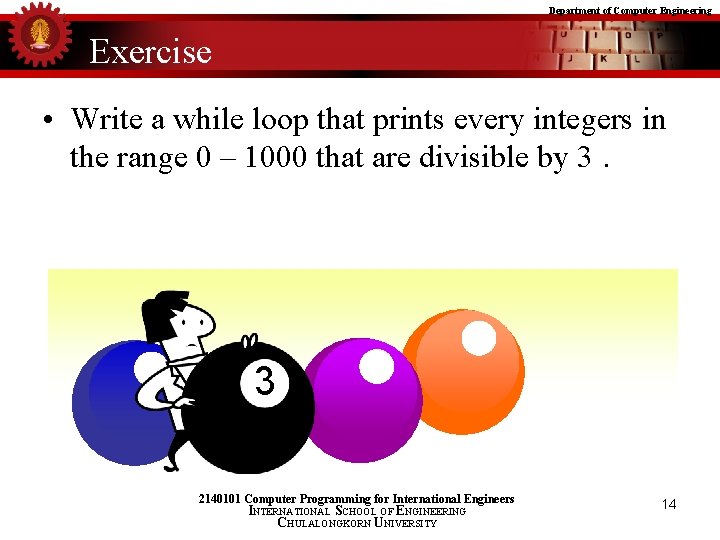
Department of Computer Engineering Exercise • Write a while loop that prints every integers in the range 0 – 1000 that are divisible by 3. 3 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 14
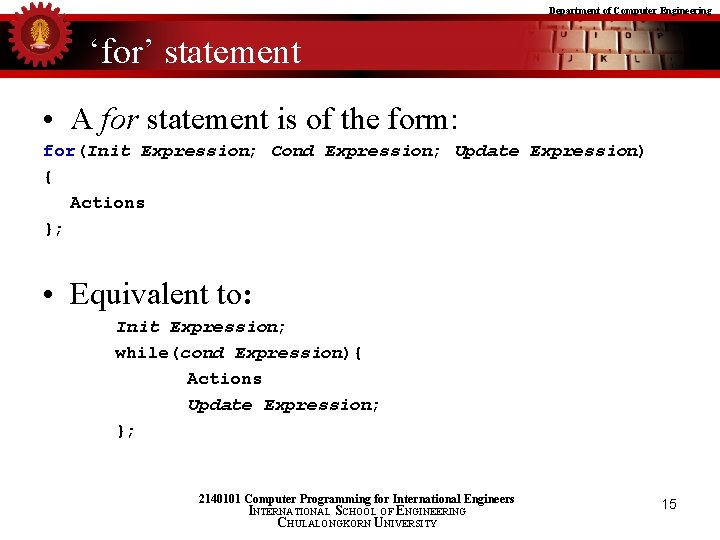
Department of Computer Engineering ‘for’ statement • A for statement is of the form: for(Init Expression; Cond Expression; Update Expression) { Actions }; • Equivalent to: Init Expression; while(cond Expression){ Actions Update Expression; }; 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 15
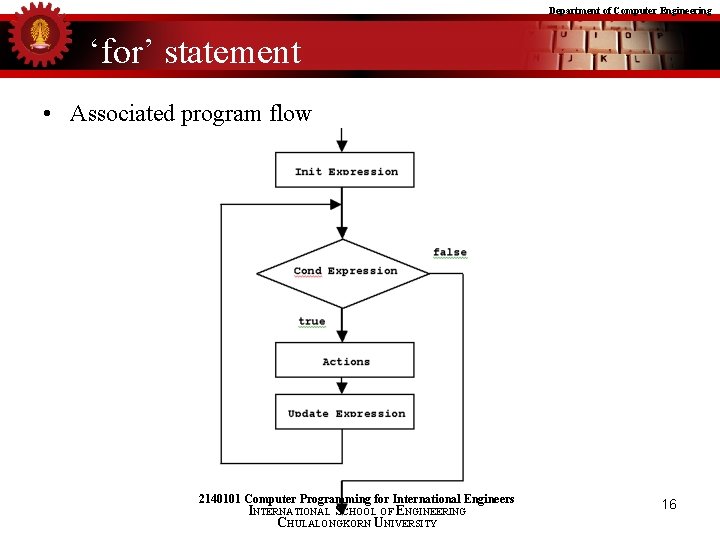
Department of Computer Engineering ‘for’ statement • Associated program flow 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 16
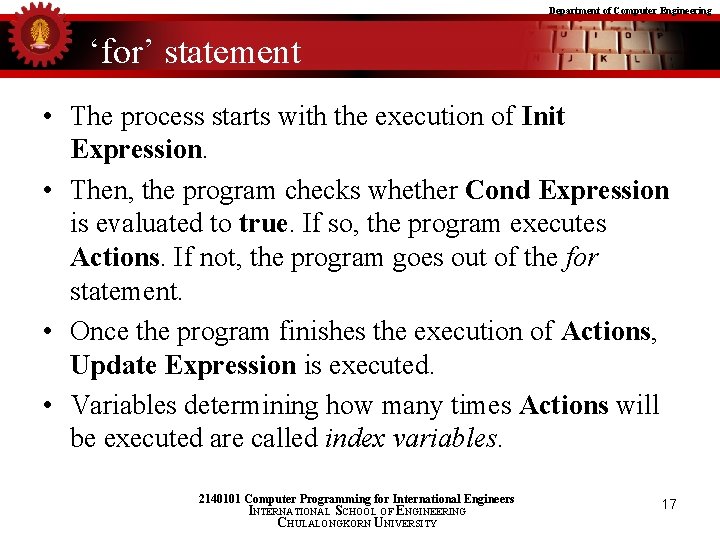
Department of Computer Engineering ‘for’ statement • The process starts with the execution of Init Expression. • Then, the program checks whether Cond Expression is evaluated to true. If so, the program executes Actions. If not, the program goes out of the for statement. • Once the program finishes the execution of Actions, Update Expression is executed. • Variables determining how many times Actions will be executed are called index variables. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 17
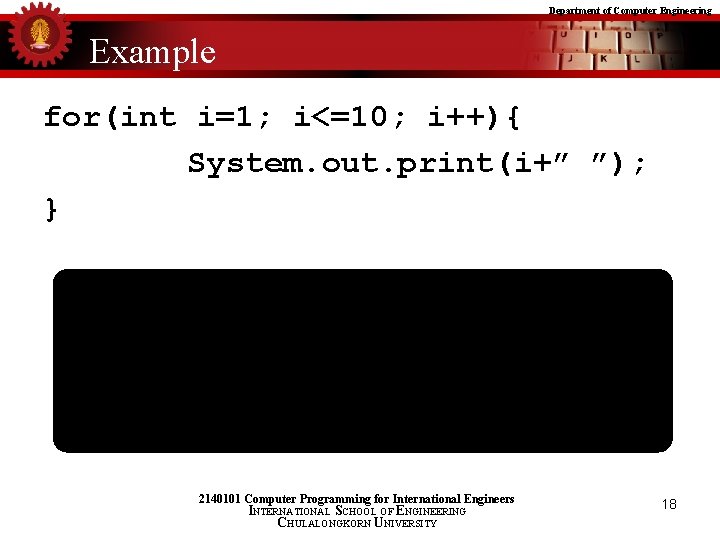
Department of Computer Engineering Example for(int i=1; i<=10; i++){ System. out. print(i+” ”); } 1 2 3 4 5 6 7 8 9 10 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 18
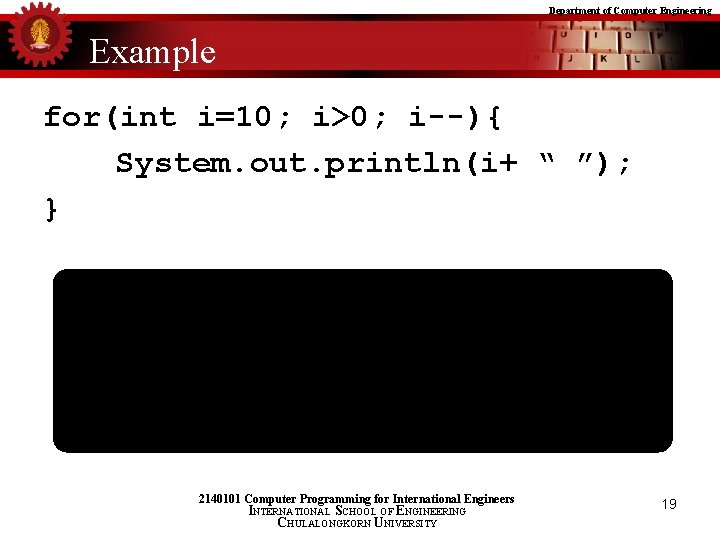
Department of Computer Engineering Example for(int i=10; i>0; i--){ System. out. println(i+ “ ”); } 10 9 8 7 6 5 4 3 2 1 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 19
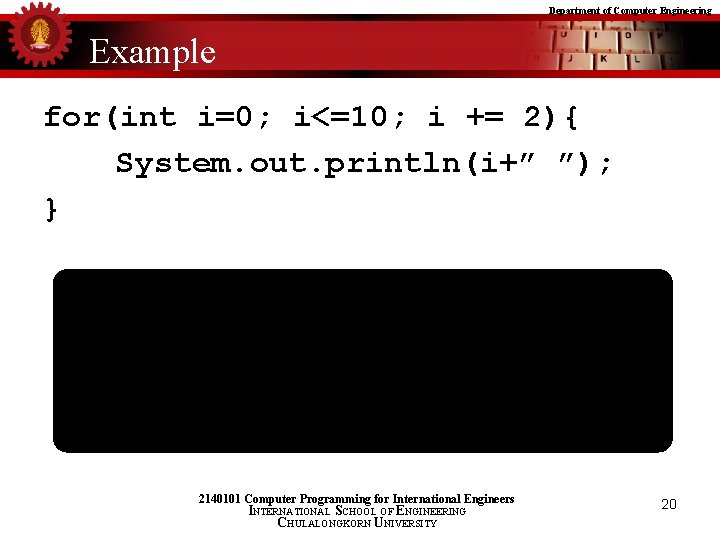
Department of Computer Engineering Example for(int i=0; i<=10; i += 2){ System. out. println(i+” ”); } 0 2 4 6 8 10 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 20
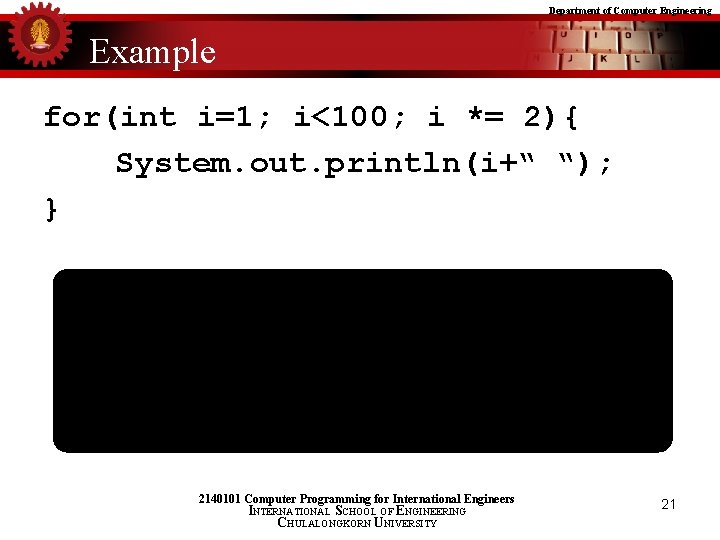
Department of Computer Engineering Example for(int i=1; i<100; i *= 2){ System. out. println(i+“ “); } 1 2 4 8 16 32 64 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 21
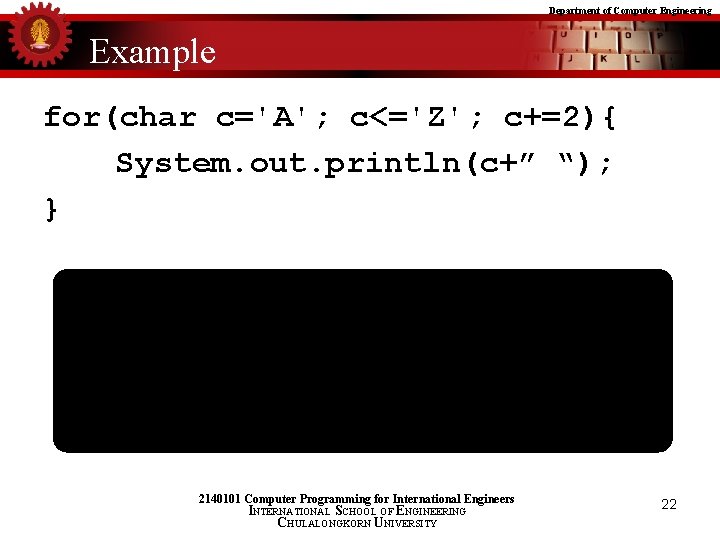
Department of Computer Engineering Example for(char c='A'; c<='Z'; c+=2){ System. out. println(c+” “); } ACEGIKMOQSUWY 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 22
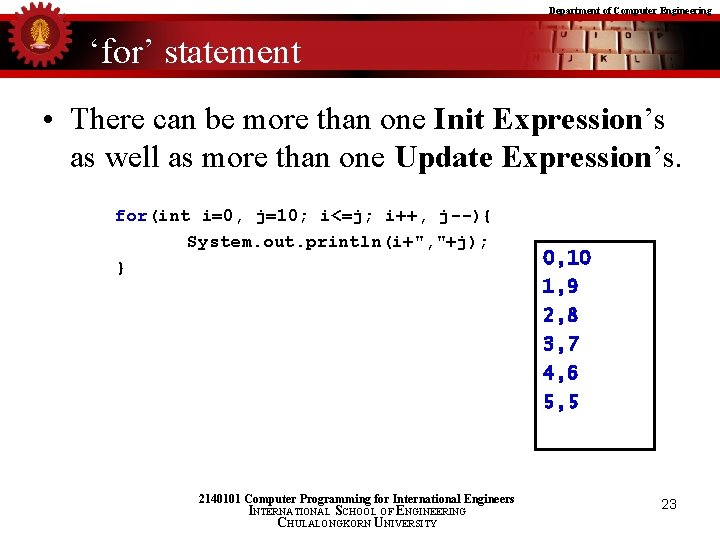
Department of Computer Engineering ‘for’ statement • There can be more than one Init Expression’s as well as more than one Update Expression’s. for(int i=0, j=10; i<=j; i++, j--){ System. out. println(i+", "+j); } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 0, 10 1, 9 2, 8 3, 7 4, 6 5, 5 23
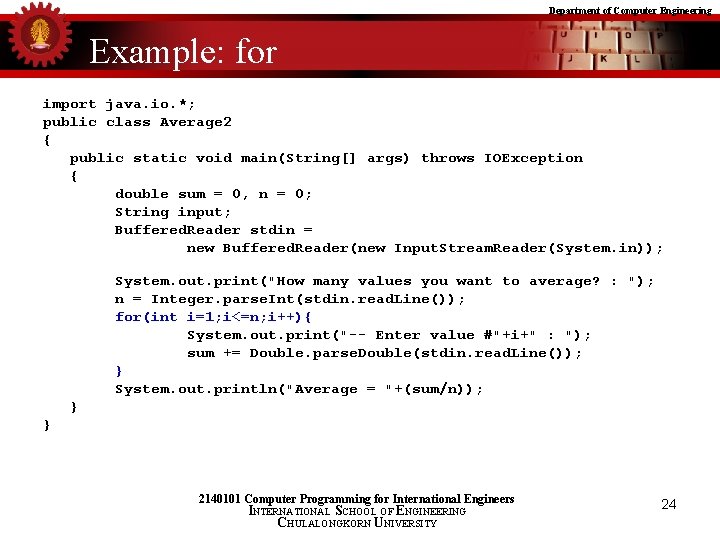
Department of Computer Engineering Example: for import java. io. *; public class Average 2 { public static void main(String[] args) throws IOException { double sum = 0, n = 0; String input; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in)); System. out. print("How many values you want to average? : "); n = Integer. parse. Int(stdin. read. Line()); for(int i=1; i<=n; i++){ System. out. print("-- Enter value #"+i+" : "); sum += Double. parse. Double(stdin. read. Line()); } System. out. println("Average = "+(sum/n)); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 24
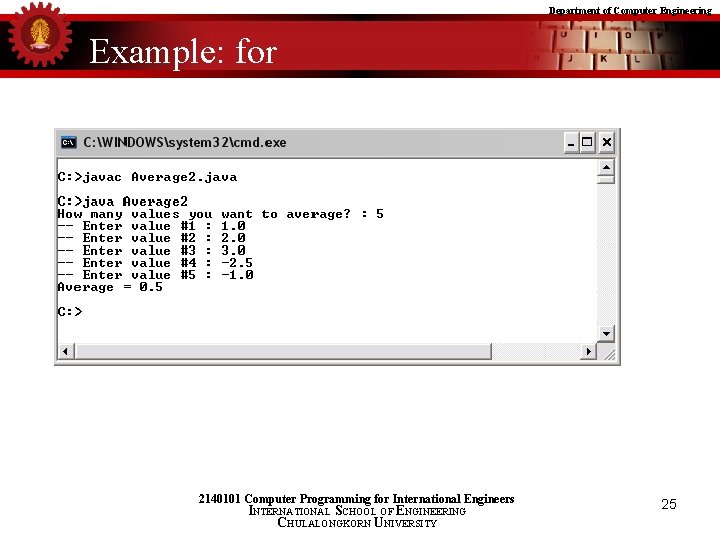
Department of Computer Engineering Example: for 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 25
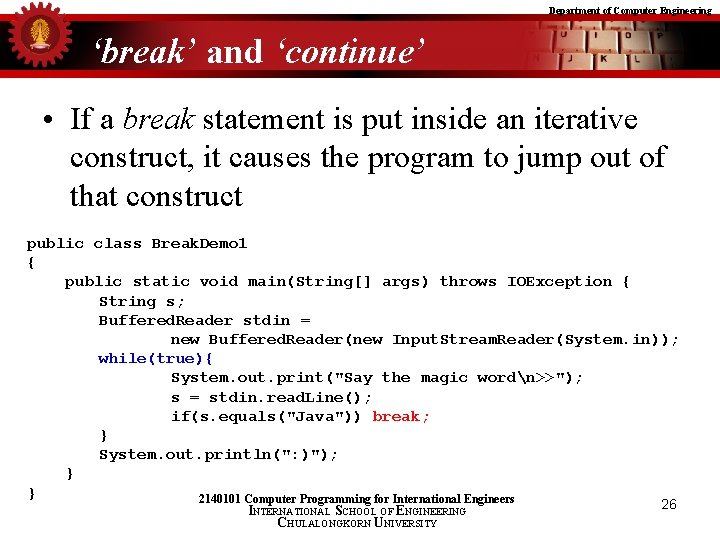
Department of Computer Engineering ‘break’ and ‘continue’ • If a break statement is put inside an iterative construct, it causes the program to jump out of that construct public class Break. Demo 1 { public static void main(String[] args) throws IOException { String s; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in)); while(true){ System. out. print("Say the magic wordn>>"); s = stdin. read. Line(); if(s. equals("Java")) break; } System. out. println(": )"); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 26
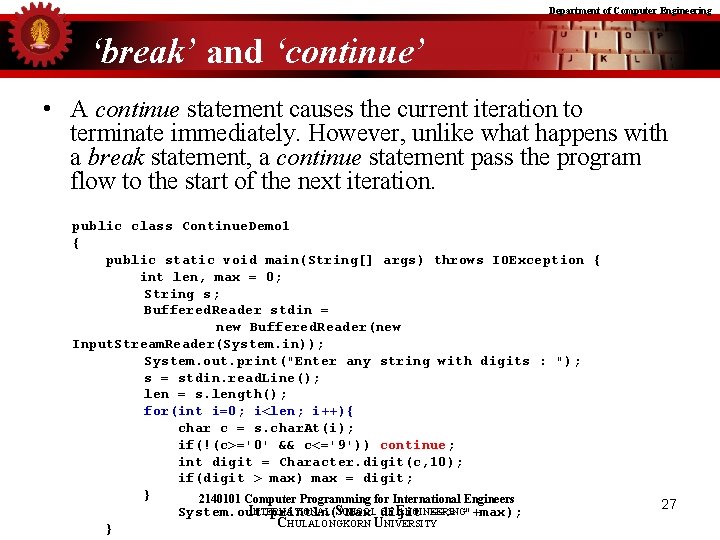
Department of Computer Engineering ‘break’ and ‘continue’ • A continue statement causes the current iteration to terminate immediately. However, unlike what happens with a break statement, a continue statement pass the program flow to the start of the next iteration. public class Continue. Demo 1 { public static void main(String[] args) throws IOException { int len, max = 0; String s; Buffered. Reader stdin = new Buffered. Reader(new Input. Stream. Reader(System. in)); System. out. print("Enter any string with digits : "); s = stdin. read. Line(); len = s. length(); for(int i=0; i<len; i++){ char c = s. char. At(i); if(!(c>='0' && c<='9')) continue; int digit = Character. digit(c, 10); if(digit > max) max = digit; } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL digit OF ENGINEERING System. out. println("Max --> "+max); C HULALONGKORN UNIVERSITY } 27
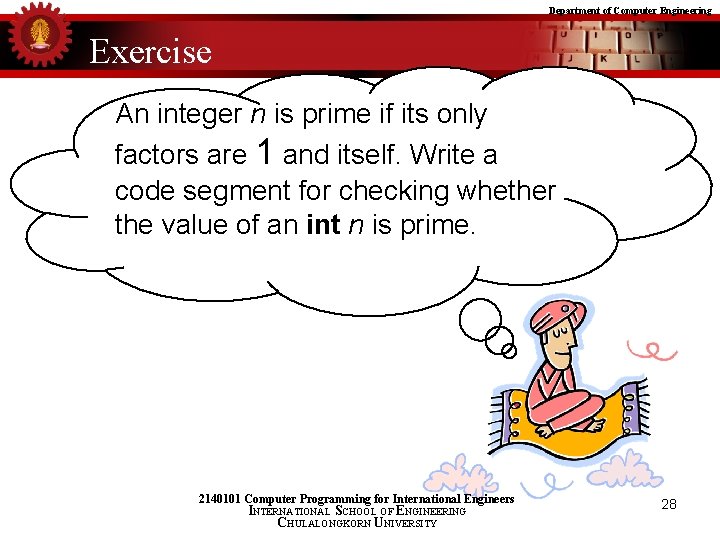
Department of Computer Engineering Exercise An integer n is prime if its only factors are 1 and itself. Write a code segment for checking whether the value of an int n is prime. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 28
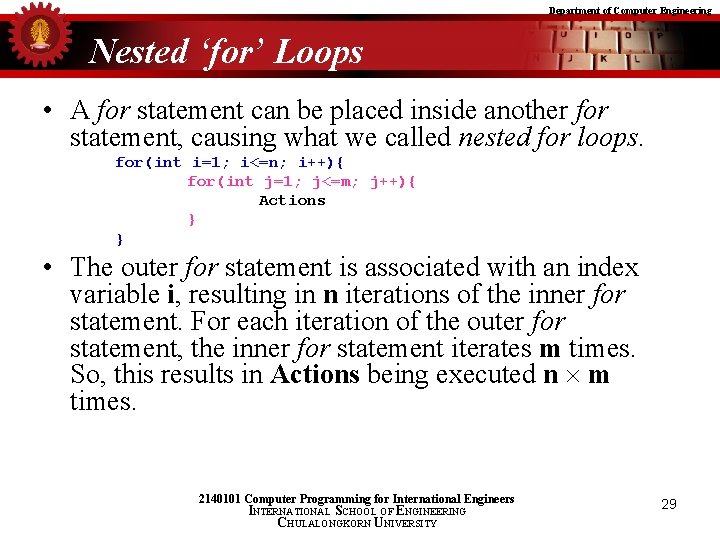
Department of Computer Engineering Nested ‘for’ Loops • A for statement can be placed inside another for statement, causing what we called nested for loops. for(int i=1; i<=n; i++){ for(int j=1; j<=m; j++){ Actions } } • The outer for statement is associated with an index variable i, resulting in n iterations of the inner for statement. For each iteration of the outer for statement, the inner for statement iterates m times. So, this results in Actions being executed n m times. 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 29
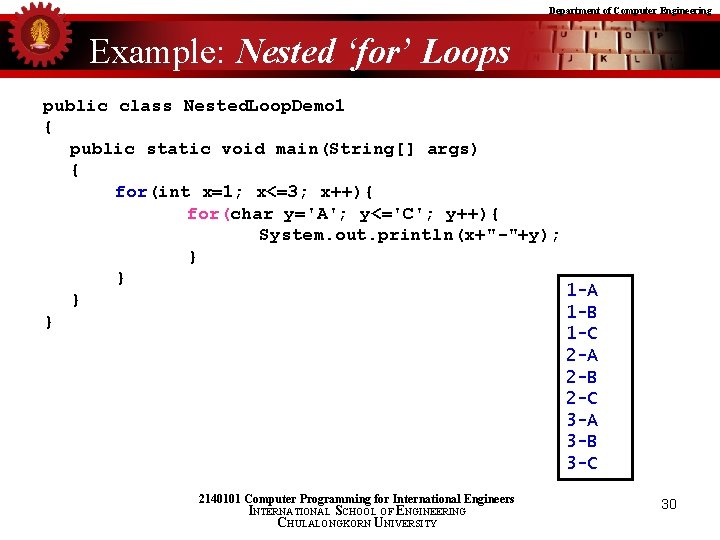
Department of Computer Engineering Example: Nested ‘for’ Loops public class Nested. Loop. Demo 1 { public static void main(String[] args) { for(int x=1; x<=3; x++){ for(char y='A'; y<='C'; y++){ System. out. println(x+"-"+y); } } 1 -A } 1 -B } 1 -C 2 -A 2 -B 2 -C 3 -A 3 -B 3 -C 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 30
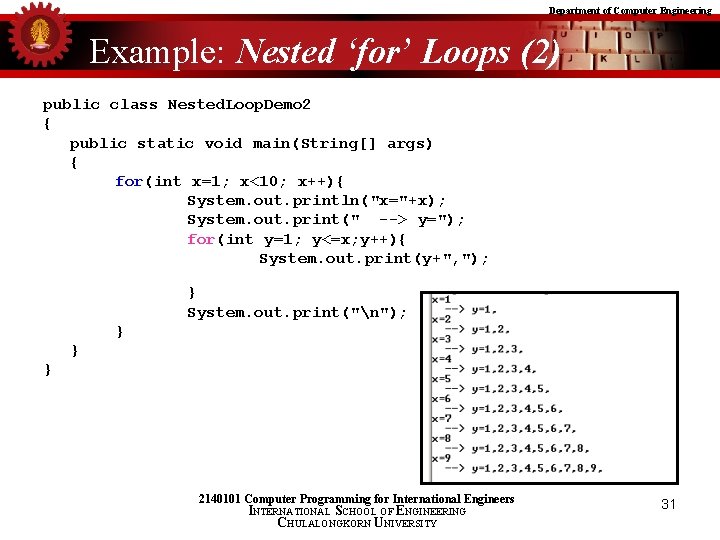
Department of Computer Engineering Example: Nested ‘for’ Loops (2) public class Nested. Loop. Demo 2 { public static void main(String[] args) { for(int x=1; x<10; x++){ System. out. println("x="+x); System. out. print(" --> y="); for(int y=1; y<=x; y++){ System. out. print(y+", "); } System. out. print("n"); } } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 31
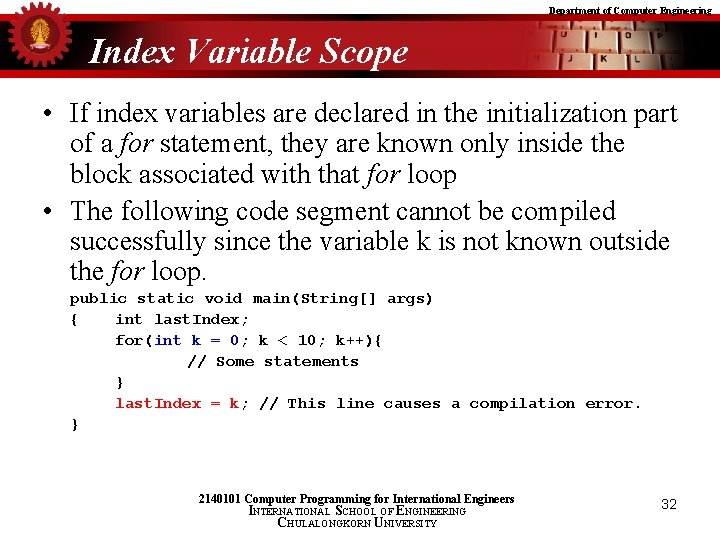
Department of Computer Engineering Index Variable Scope • If index variables are declared in the initialization part of a for statement, they are known only inside the block associated with that for loop • The following code segment cannot be compiled successfully since the variable k is not known outside the for loop. public static void main(String[] args) { int last. Index; for(int k = 0; k < 10; k++){ // Some statements } last. Index = k; // This line causes a compilation error. } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 32
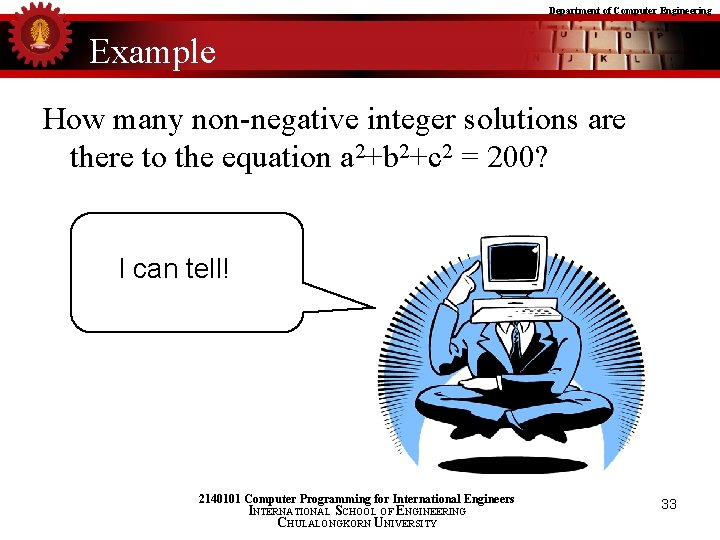
Department of Computer Engineering Example How many non-negative integer solutions are there to the equation a 2+b 2+c 2 = 200? I can tell! 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 33
![Department of Computer Engineering Example public class Solutions Count public static void mainString Department of Computer Engineering Example public class Solutions. Count { public static void main(String[]](https://slidetodoc.com/presentation_image_h/0f36a387e46c6156d869bb9de957da8b/image-34.jpg)
Department of Computer Engineering Example public class Solutions. Count { public static void main(String[] args) { int a, b, c, n. Solutions=0; int max. Possible = (int)Math. floor(200); for(a = 0; a < max. Possible; a++){ for(b = 0; b < max. Possible; b++){ for(c = 0; c < max. Possible; c++){ if(a*a+b*b+c*c == 200){ n. Solutions++; System. out. print("Soltn #"+n. Solutions); System. out. println("("+a+", "+b+", "+c+")"); } } System. out. println("# of non-negative integer solutions for "); System. out. println("a^2 + b^2 + c^2 = 200 is "+n. Solutions); } } 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 34
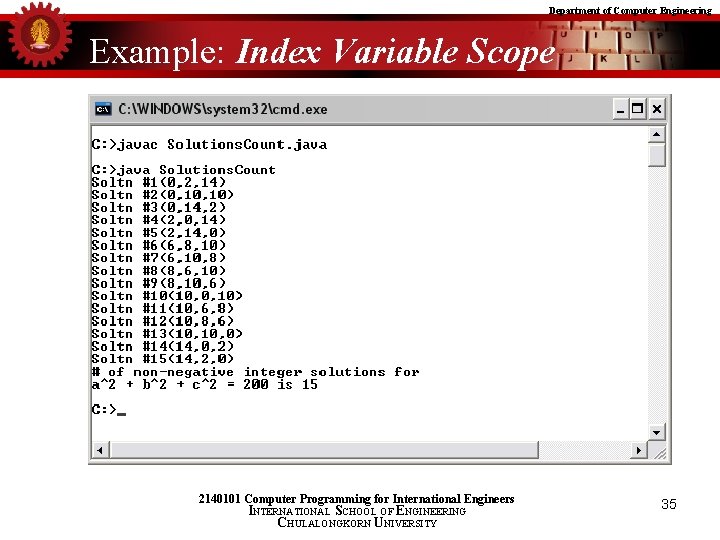
Department of Computer Engineering Example: Index Variable Scope 2140101 Computer Programming for International Engineers INTERNATIONAL SCHOOL OF ENGINEERING CHULALONGKORN UNIVERSITY 35