CSCI 206 Computer Organization Course Summary Major Course
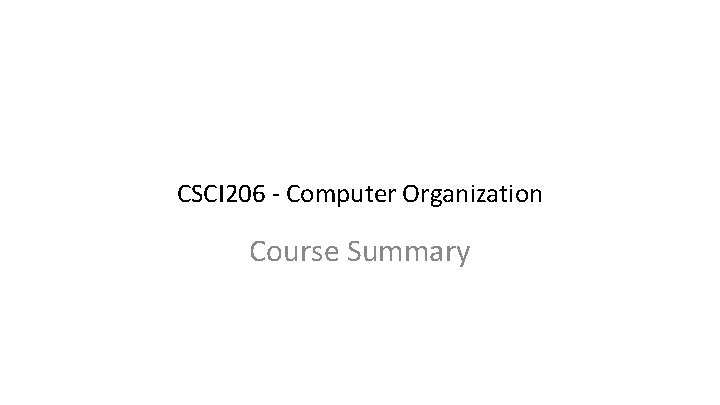
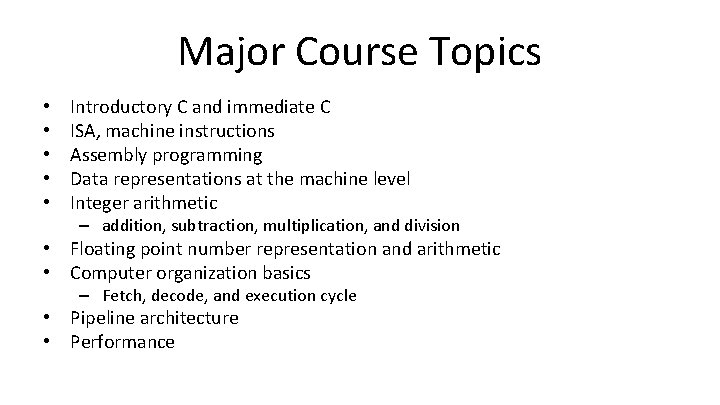
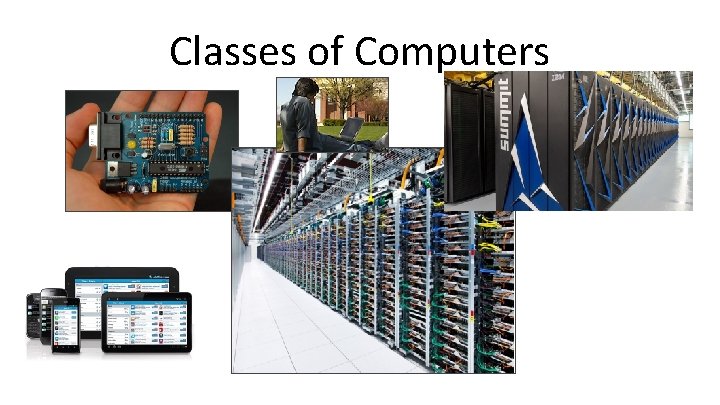
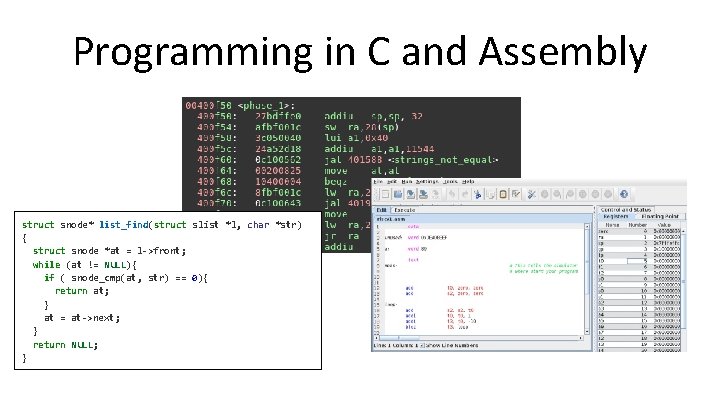
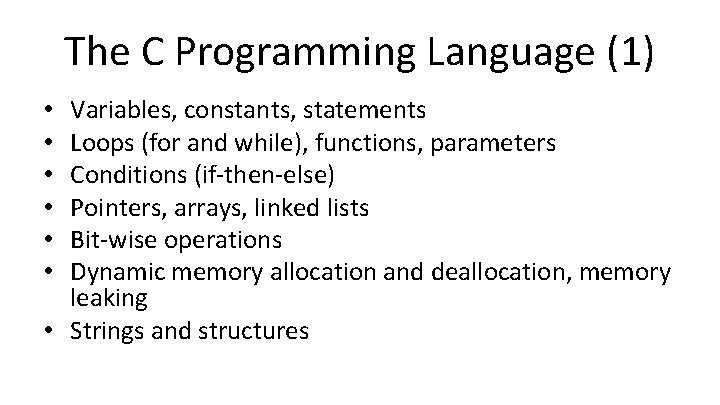
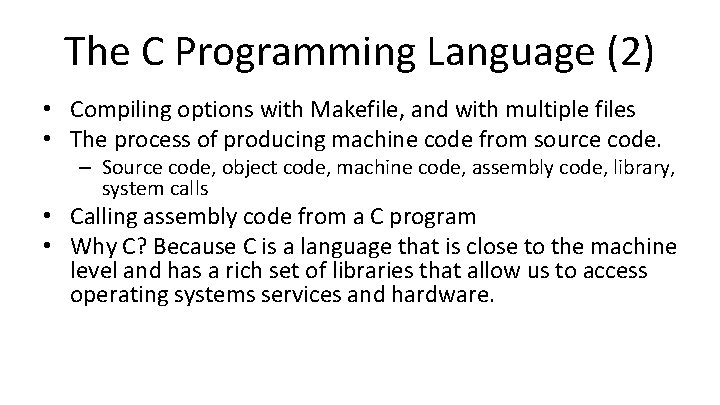
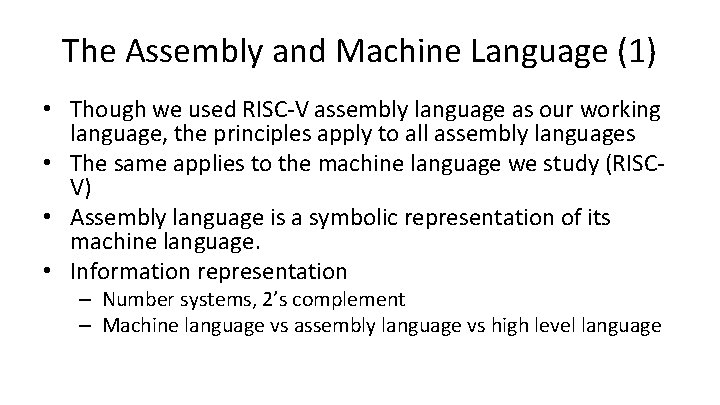
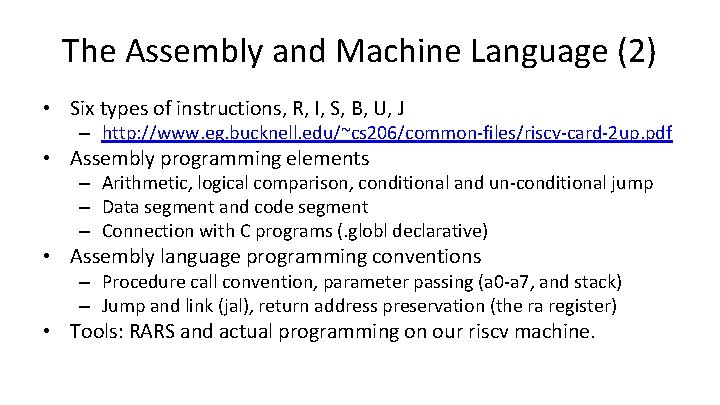
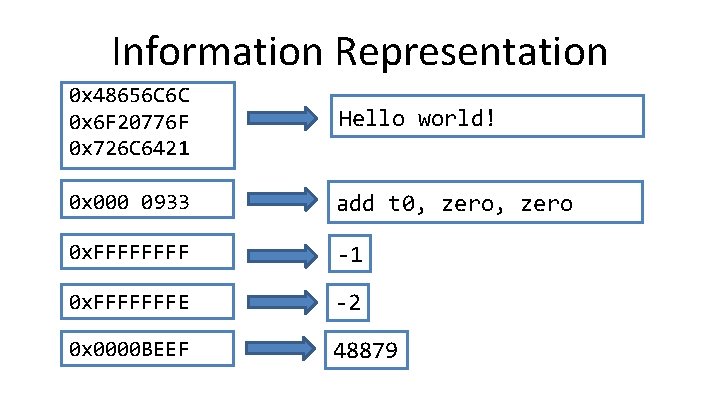
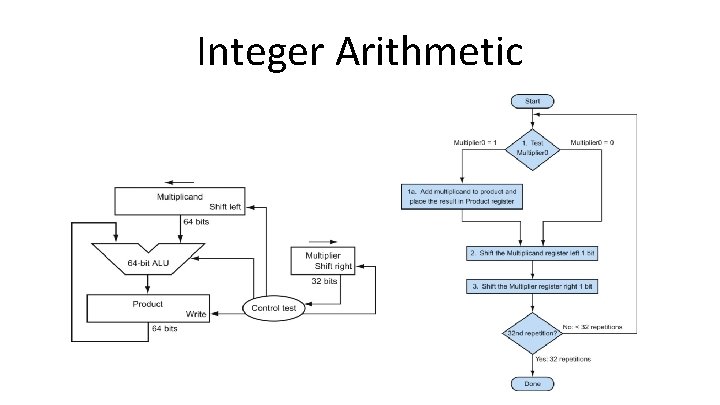
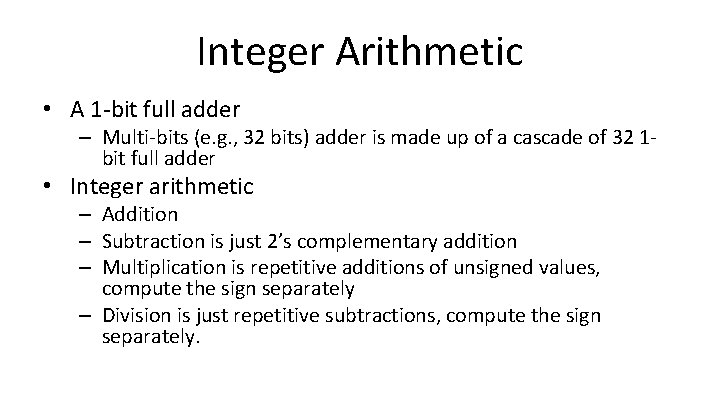
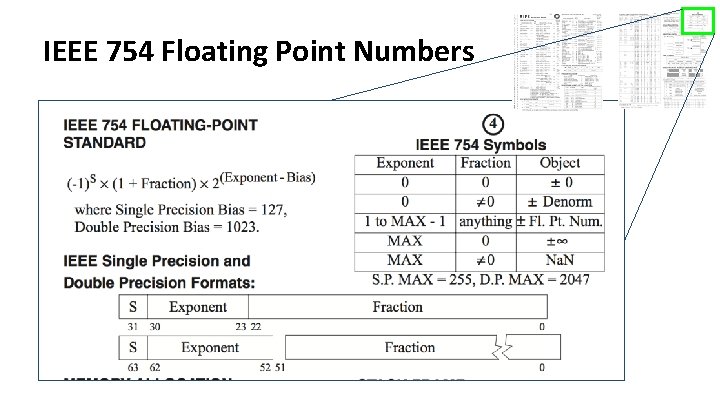
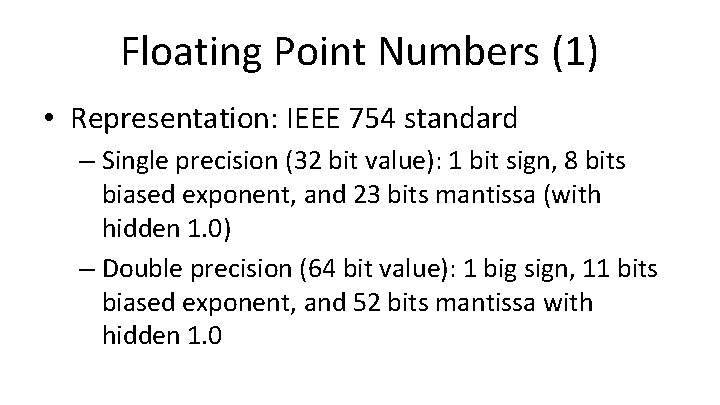
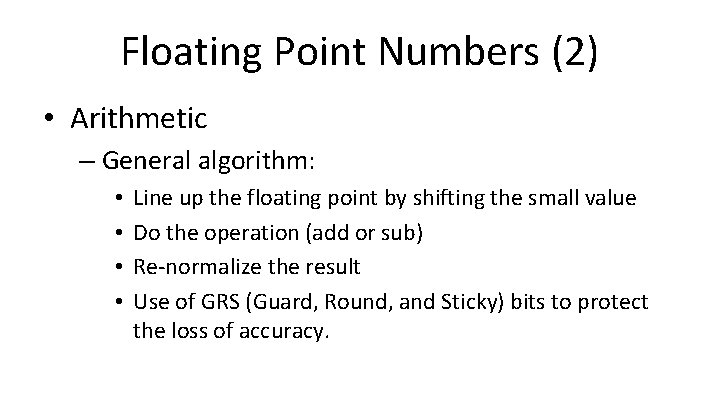
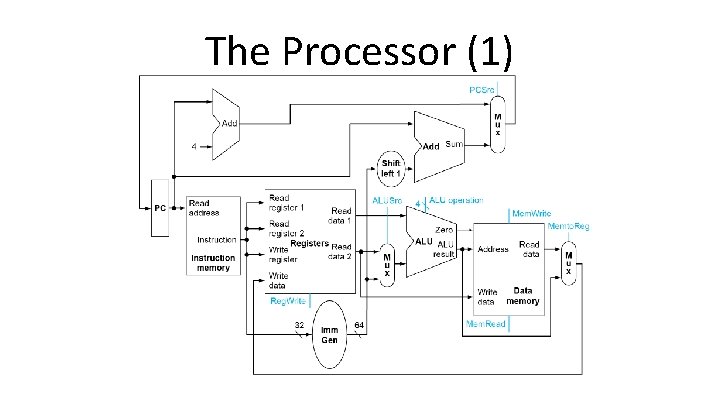
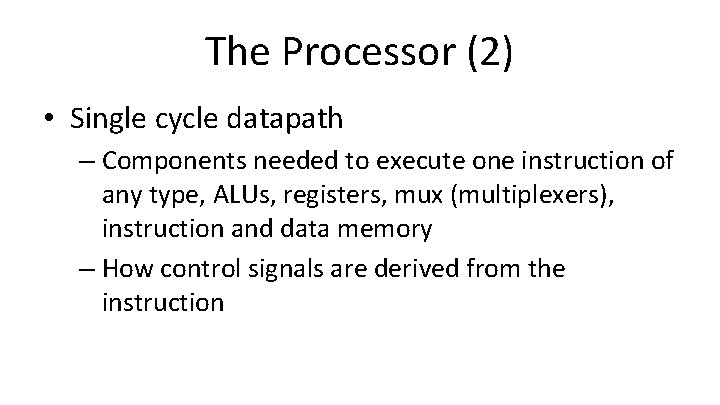
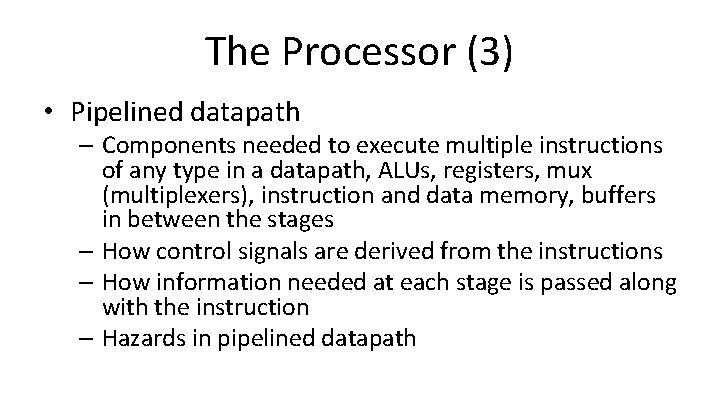
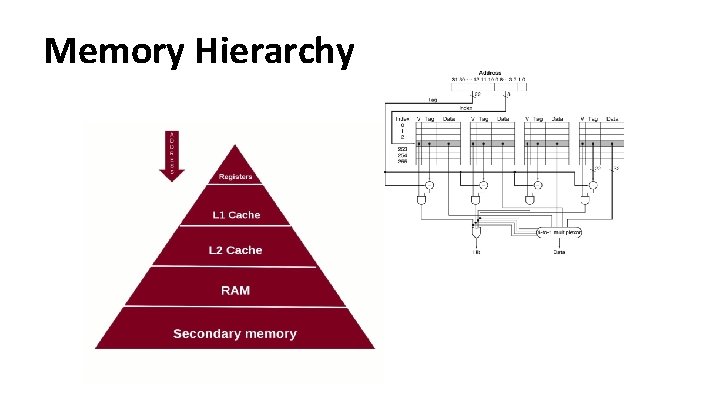
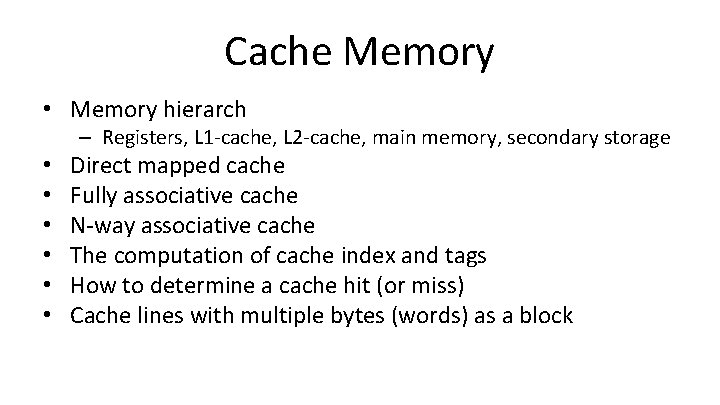
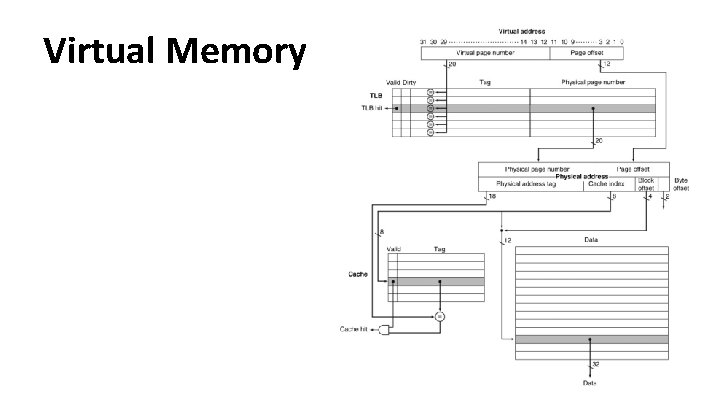
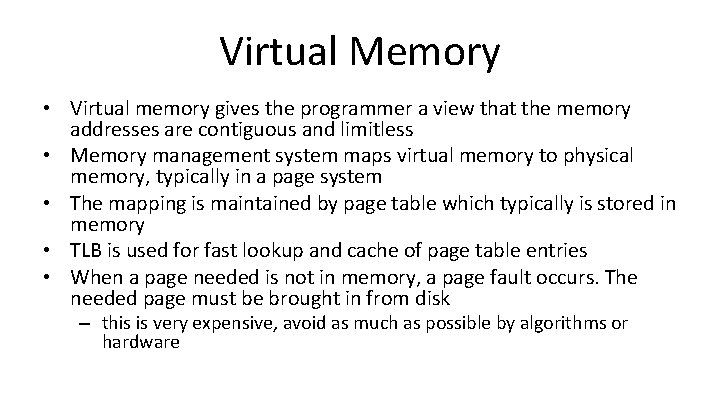
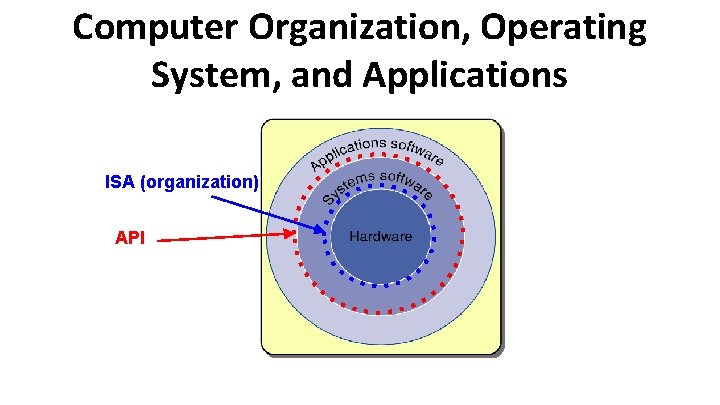
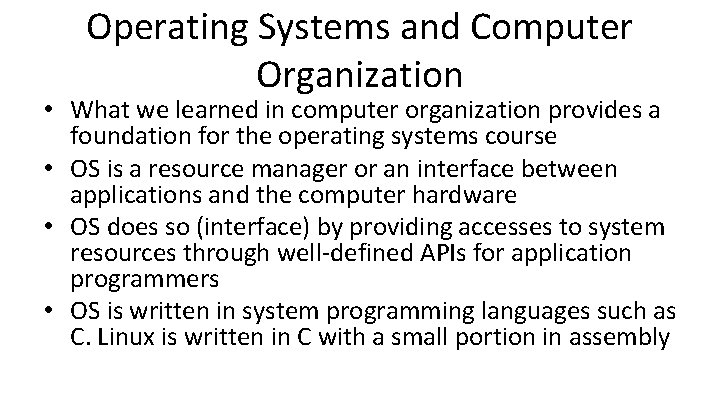
- Slides: 23
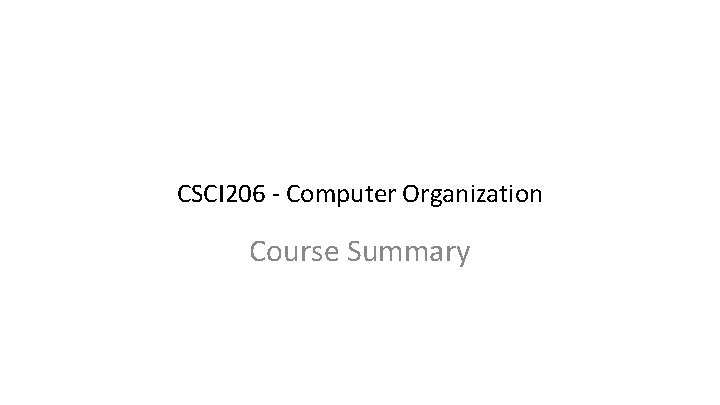
CSCI 206 - Computer Organization Course Summary
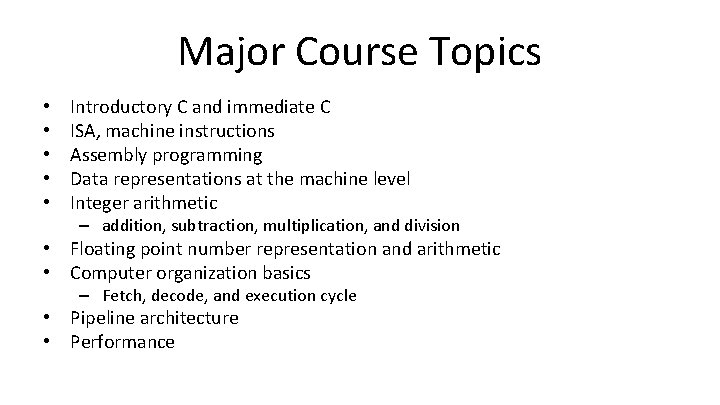
Major Course Topics • • • Introductory C and immediate C ISA, machine instructions Assembly programming Data representations at the machine level Integer arithmetic – addition, subtraction, multiplication, and division • Floating point number representation and arithmetic • Computer organization basics – Fetch, decode, and execution cycle • Pipeline architecture • Performance
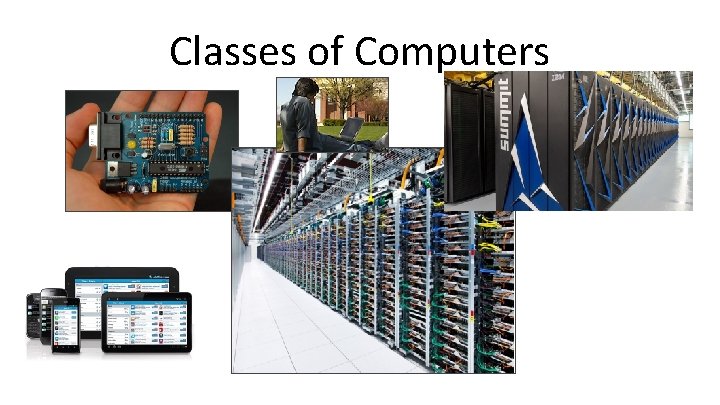
Classes of Computers
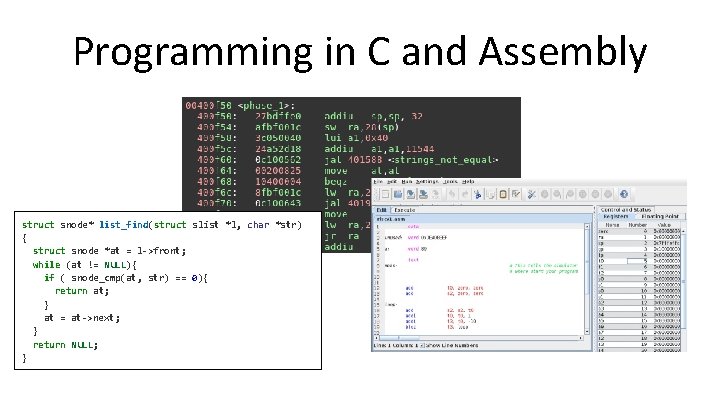
Programming in C and Assembly struct snode* list_find(struct slist *l, char *str) { struct snode *at = l->front; while (at != NULL){ if ( snode_cmp(at, str) == 0){ return at; } at = at->next; } return NULL; }
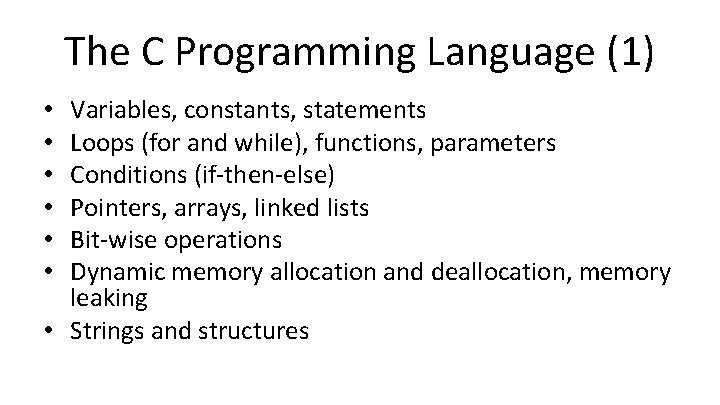
The C Programming Language (1) Variables, constants, statements Loops (for and while), functions, parameters Conditions (if-then-else) Pointers, arrays, linked lists Bit-wise operations Dynamic memory allocation and deallocation, memory leaking • Strings and structures • • •
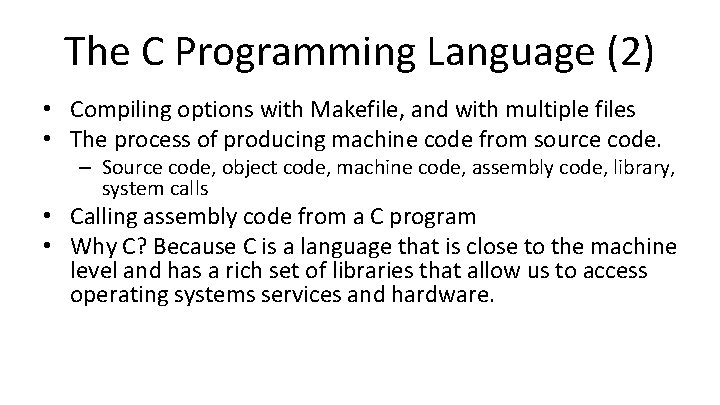
The C Programming Language (2) • Compiling options with Makefile, and with multiple files • The process of producing machine code from source code. – Source code, object code, machine code, assembly code, library, system calls • Calling assembly code from a C program • Why C? Because C is a language that is close to the machine level and has a rich set of libraries that allow us to access operating systems services and hardware.
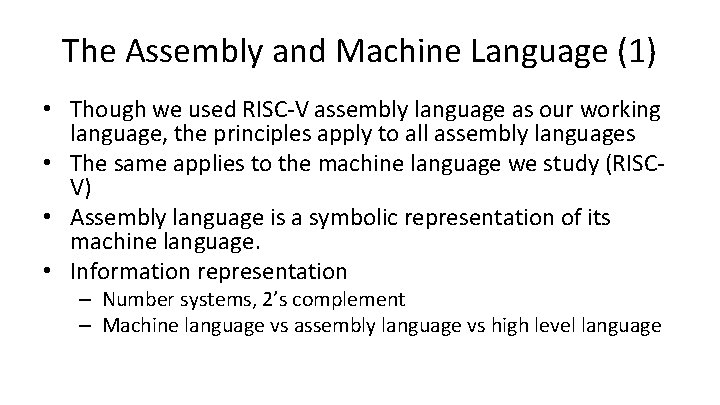
The Assembly and Machine Language (1) • Though we used RISC-V assembly language as our working language, the principles apply to all assembly languages • The same applies to the machine language we study (RISCV) • Assembly language is a symbolic representation of its machine language. • Information representation – Number systems, 2’s complement – Machine language vs assembly language vs high level language
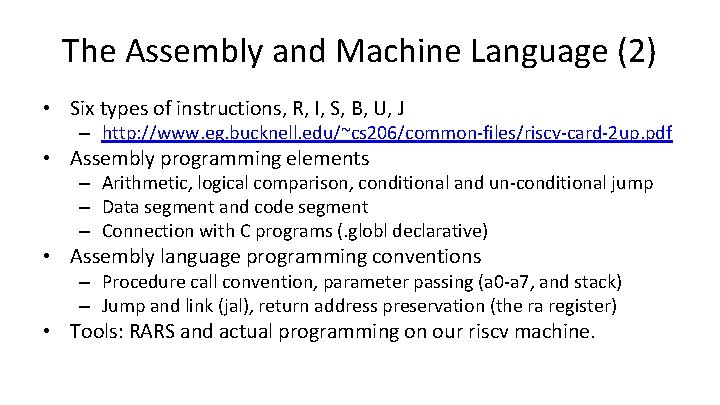
The Assembly and Machine Language (2) • Six types of instructions, R, I, S, B, U, J – http: //www. eg. bucknell. edu/~cs 206/common-files/riscv-card-2 up. pdf • Assembly programming elements – Arithmetic, logical comparison, conditional and un-conditional jump – Data segment and code segment – Connection with C programs (. globl declarative) • Assembly language programming conventions – Procedure call convention, parameter passing (a 0 -a 7, and stack) – Jump and link (jal), return address preservation (the ra register) • Tools: RARS and actual programming on our riscv machine.
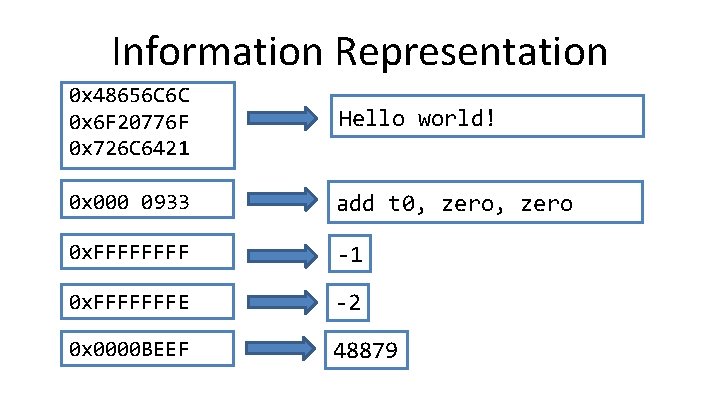
Information Representation 0 x 48656 C 6 C 0 x 6 F 20776 F 0 x 726 C 6421 Hello world! 0 x 000 0933 add t 0, zero 0 x. FFFF -1 0 x. FFFFFFFE -2 0 x 0000 BEEF 48879
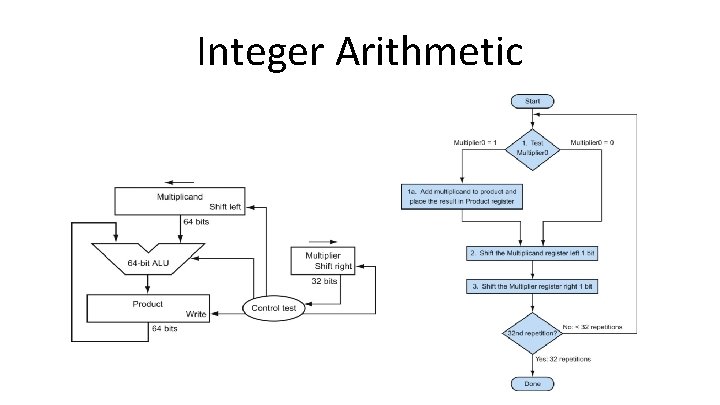
Integer Arithmetic
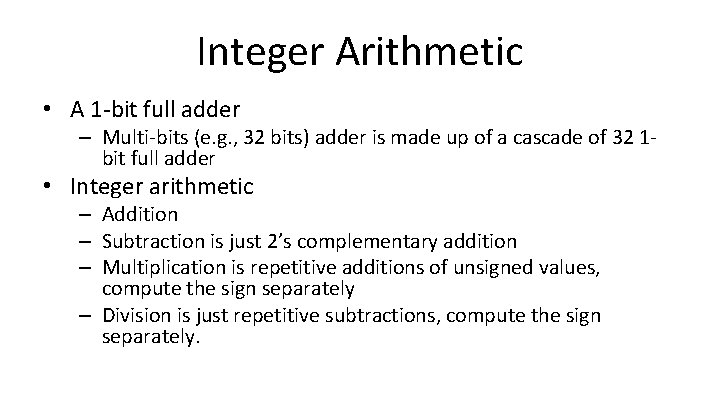
Integer Arithmetic • A 1 -bit full adder – Multi-bits (e. g. , 32 bits) adder is made up of a cascade of 32 1 bit full adder • Integer arithmetic – Addition – Subtraction is just 2’s complementary addition – Multiplication is repetitive additions of unsigned values, compute the sign separately – Division is just repetitive subtractions, compute the sign separately.
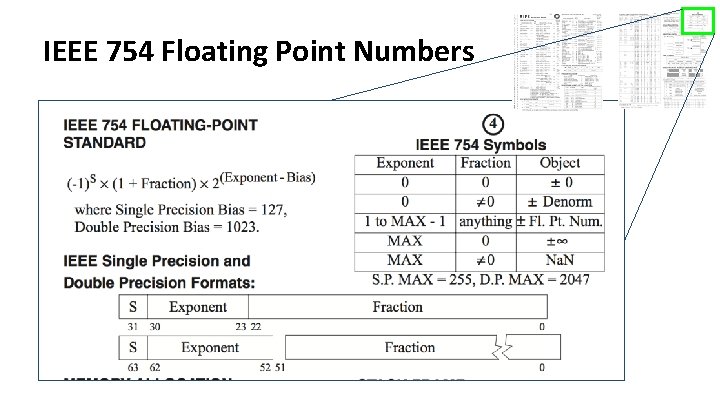
IEEE 754 Floating Point Numbers
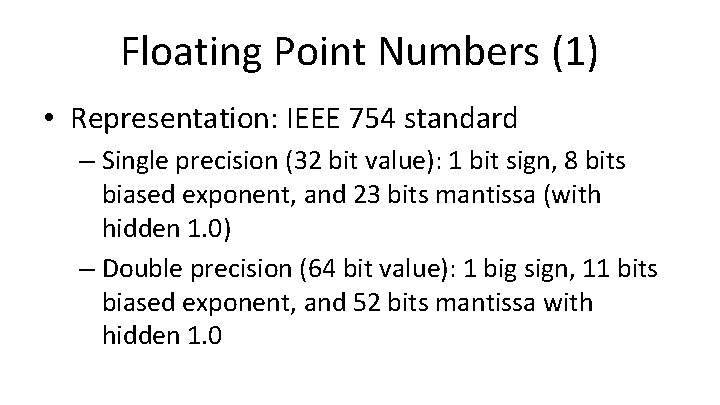
Floating Point Numbers (1) • Representation: IEEE 754 standard – Single precision (32 bit value): 1 bit sign, 8 bits biased exponent, and 23 bits mantissa (with hidden 1. 0) – Double precision (64 bit value): 1 big sign, 11 bits biased exponent, and 52 bits mantissa with hidden 1. 0
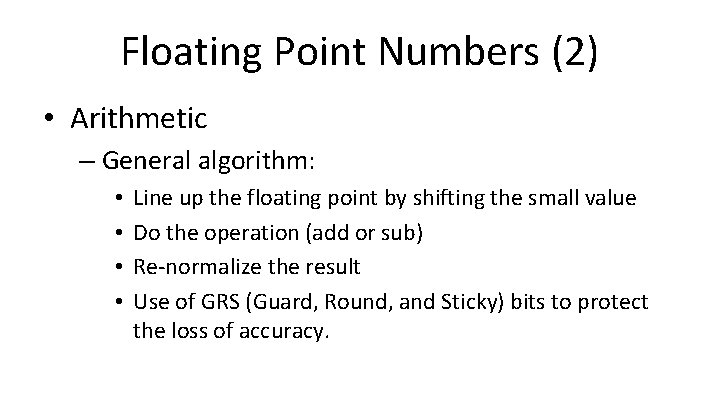
Floating Point Numbers (2) • Arithmetic – General algorithm: • • Line up the floating point by shifting the small value Do the operation (add or sub) Re-normalize the result Use of GRS (Guard, Round, and Sticky) bits to protect the loss of accuracy.
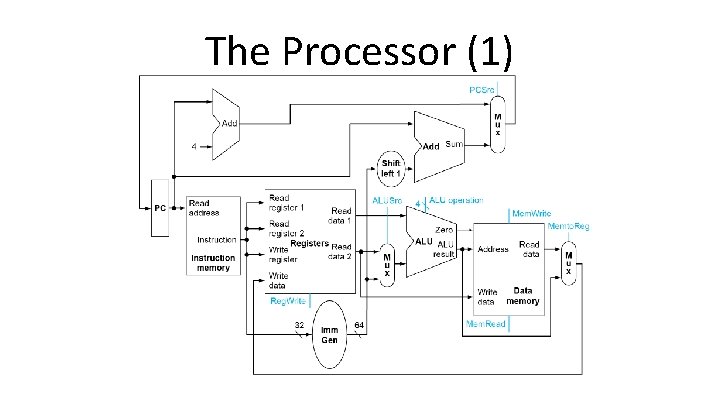
The Processor (1)
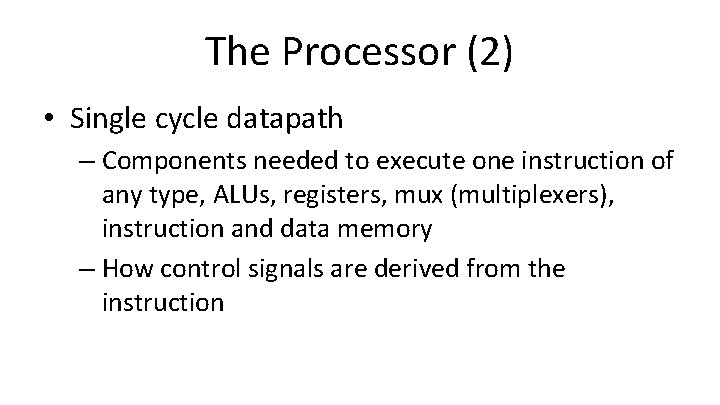
The Processor (2) • Single cycle datapath – Components needed to execute one instruction of any type, ALUs, registers, mux (multiplexers), instruction and data memory – How control signals are derived from the instruction
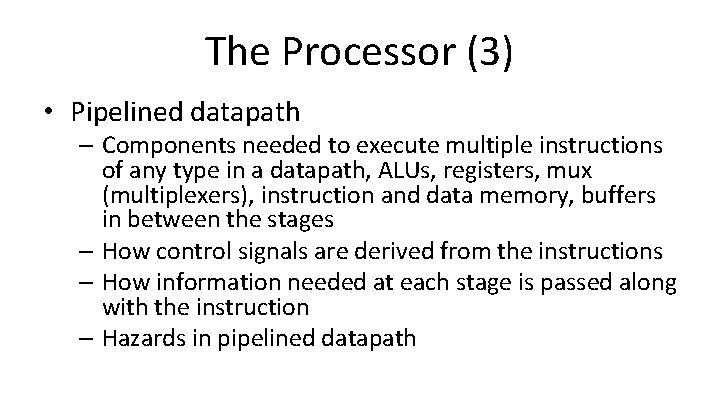
The Processor (3) • Pipelined datapath – Components needed to execute multiple instructions of any type in a datapath, ALUs, registers, mux (multiplexers), instruction and data memory, buffers in between the stages – How control signals are derived from the instructions – How information needed at each stage is passed along with the instruction – Hazards in pipelined datapath
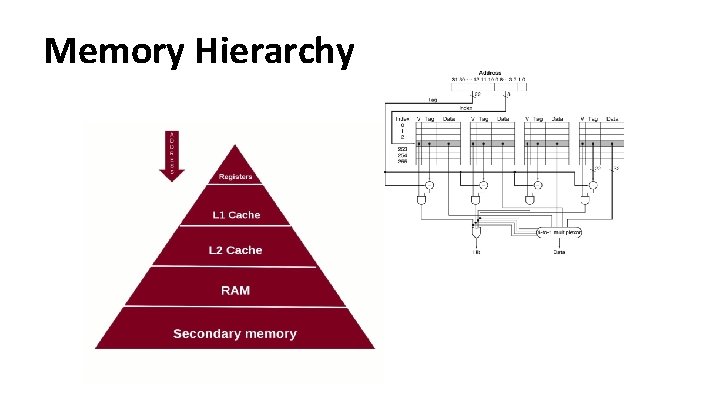
Memory Hierarchy
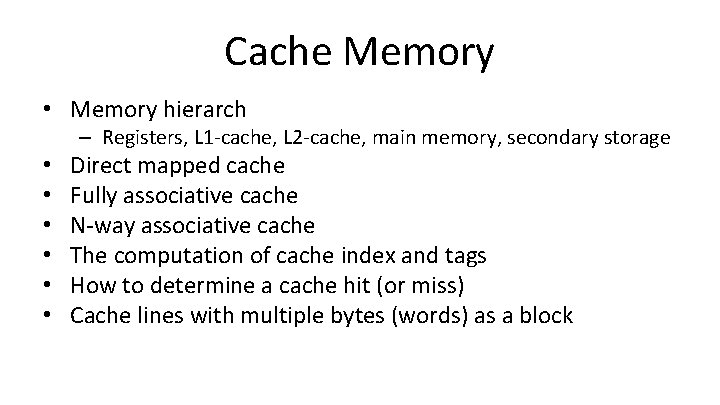
Cache Memory • Memory hierarch – Registers, L 1 -cache, L 2 -cache, main memory, secondary storage • • • Direct mapped cache Fully associative cache N-way associative cache The computation of cache index and tags How to determine a cache hit (or miss) Cache lines with multiple bytes (words) as a block
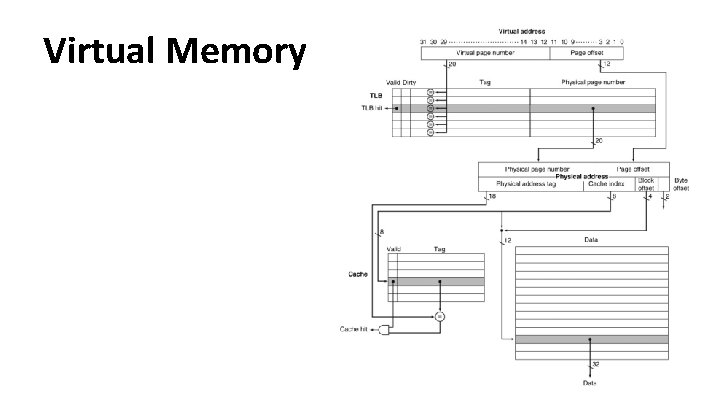
Virtual Memory
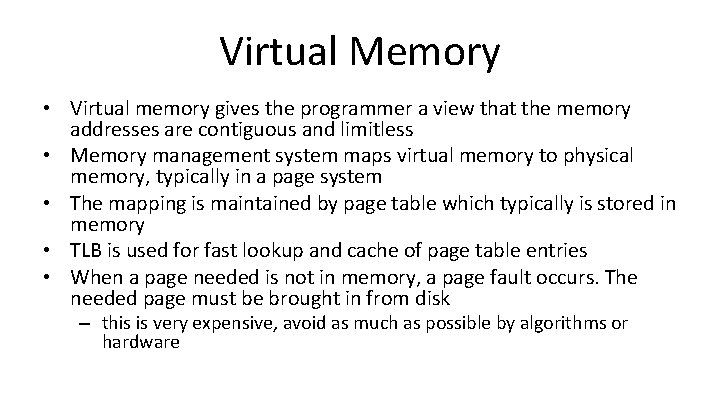
Virtual Memory • Virtual memory gives the programmer a view that the memory addresses are contiguous and limitless • Memory management system maps virtual memory to physical memory, typically in a page system • The mapping is maintained by page table which typically is stored in memory • TLB is used for fast lookup and cache of page table entries • When a page needed is not in memory, a page fault occurs. The needed page must be brought in from disk – this is very expensive, avoid as much as possible by algorithms or hardware
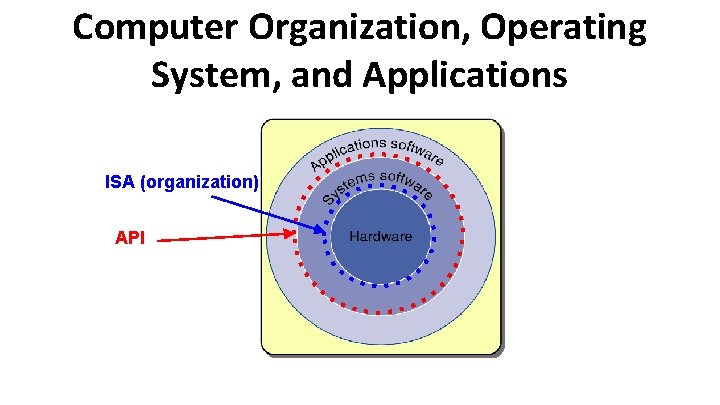
Computer Organization, Operating System, and Applications ISA (organization) API
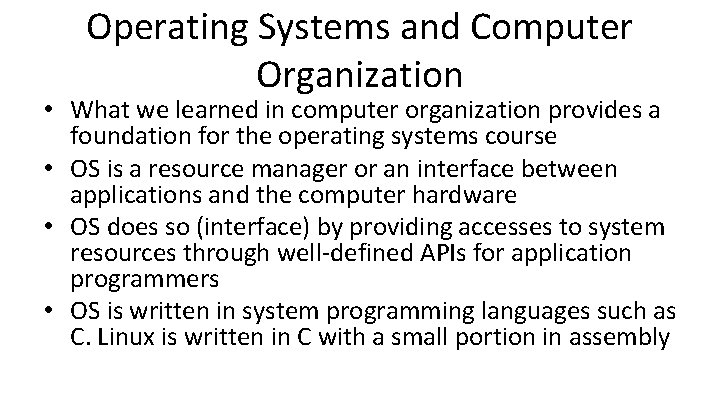
Operating Systems and Computer Organization • What we learned in computer organization provides a foundation for the operating systems course • OS is a resource manager or an interface between applications and the computer hardware • OS does so (interface) by providing accesses to system resources through well-defined APIs for application programmers • OS is written in system programming languages such as C. Linux is written in C with a small portion in assembly
Process organization in computer organization
Computer organization course
Basic structure of computer system
Diff between computer architecture and organization
Design of basic computer with flowchart
Flowchart for memory reference instructions
Ellos _________ al golf.
Ee 206
Comp 206
221 bce
Arizona fair wages and healthy families act
Csce 206 tamu
Round 787 206 to the nearest ten
206 tl
Fct beton
206 ossa
206 to binary
La pelvis es un hueso largo corto o plano
Econ 206
Comp 206
Make the common case fast
En 206-1
En 206
Difference between catabolism and anabolism