CSCI 206 Computer Organization Department of Computer Science
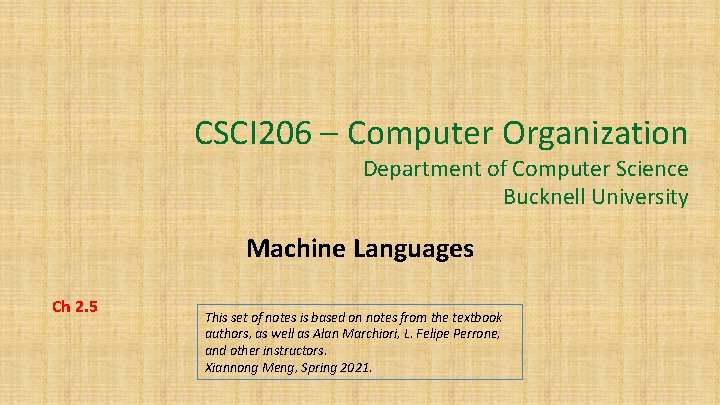
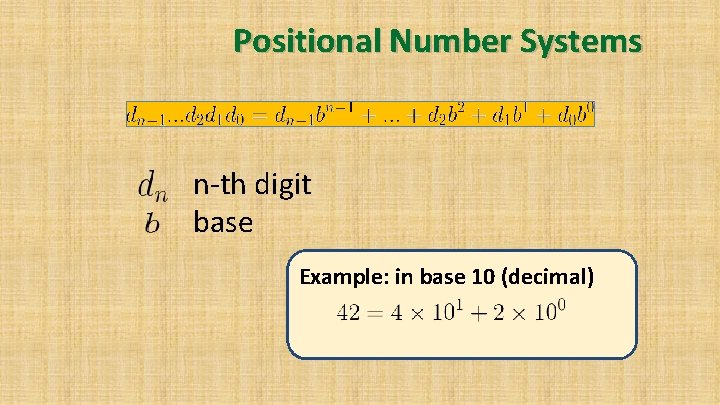
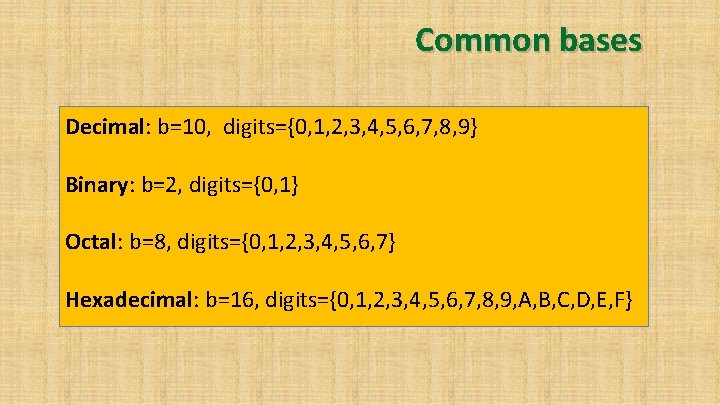
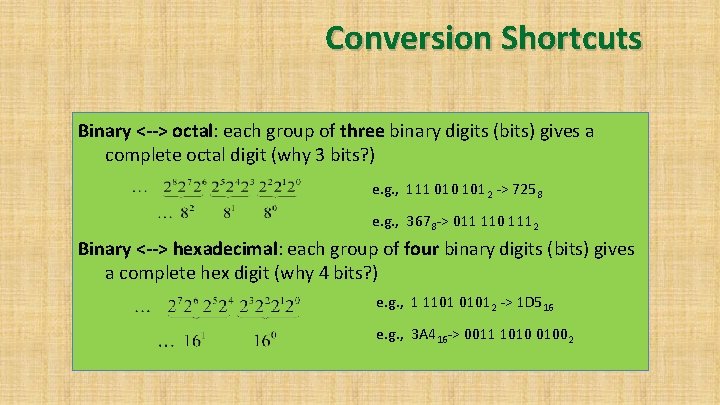
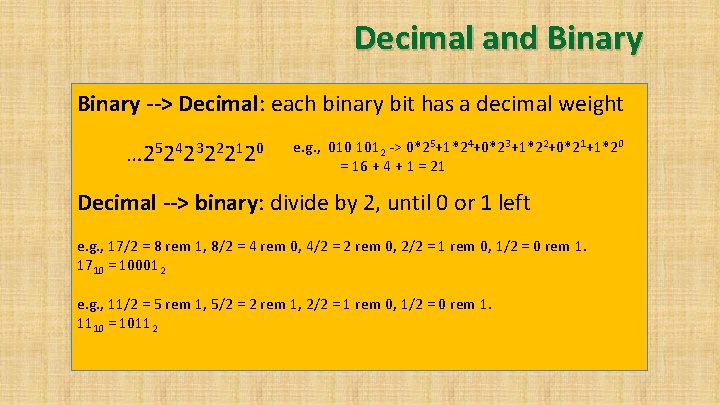
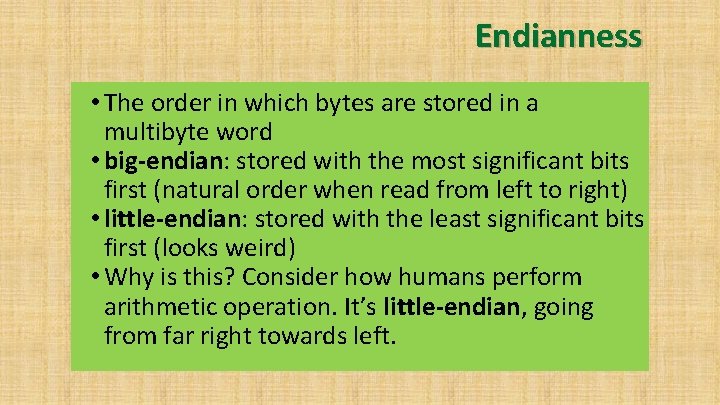
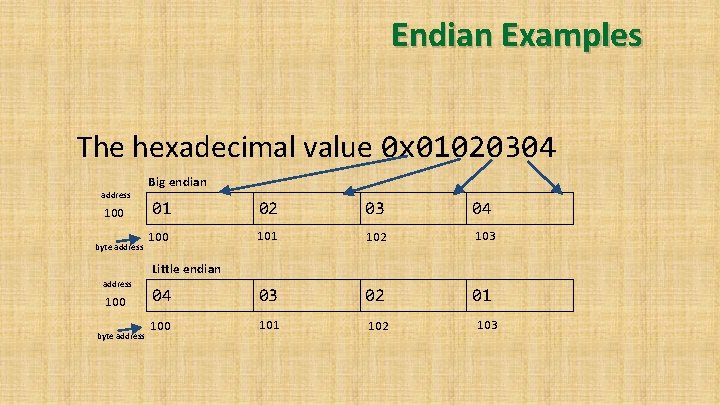
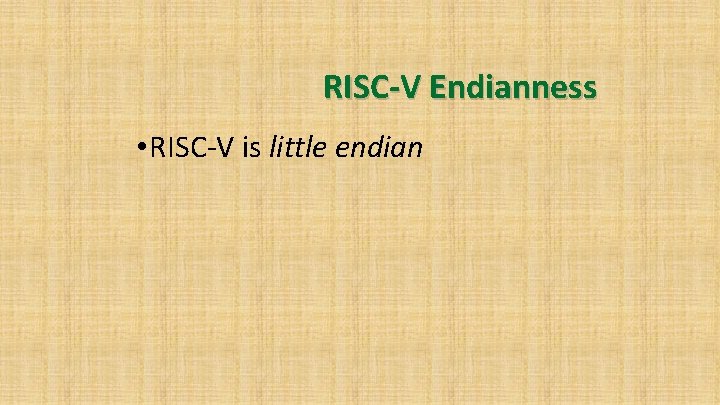
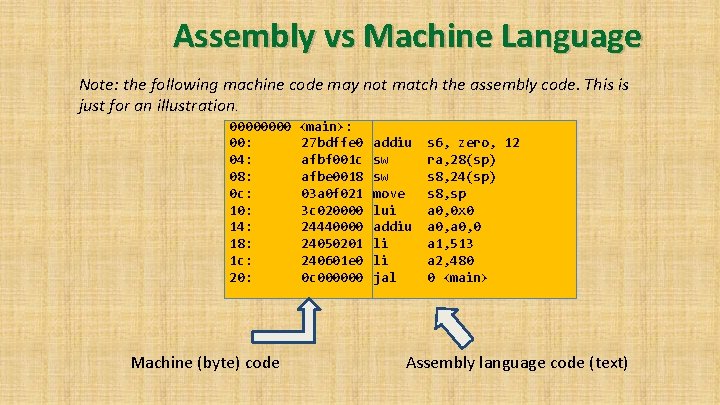
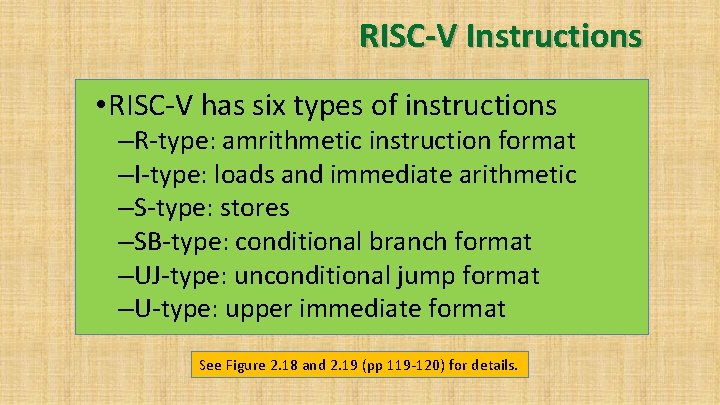
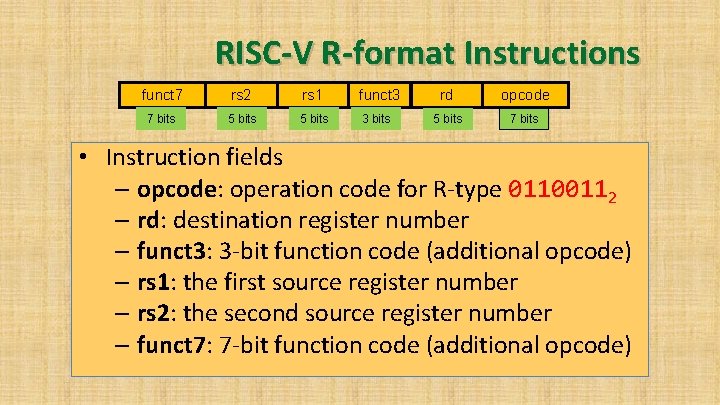
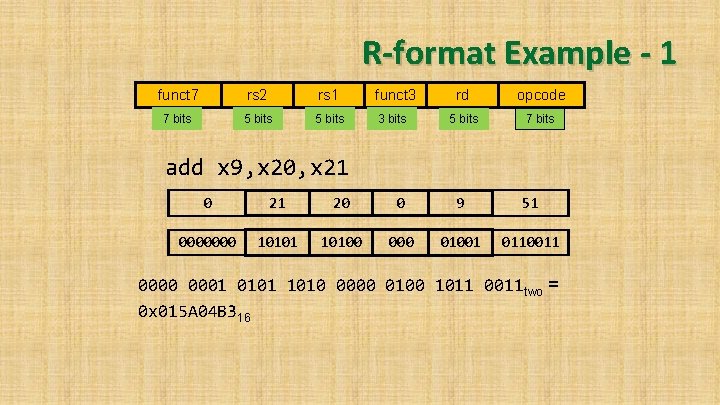
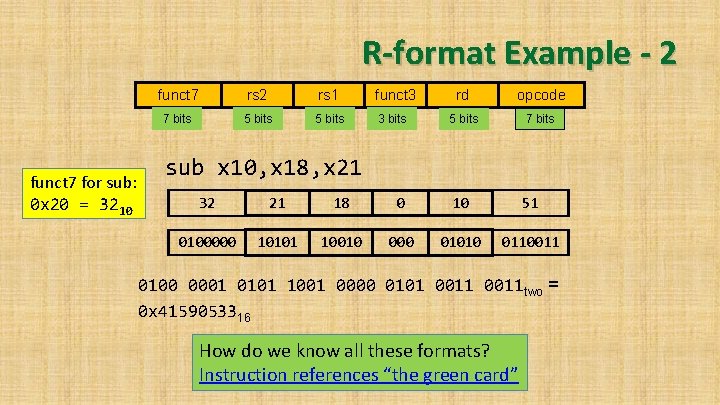
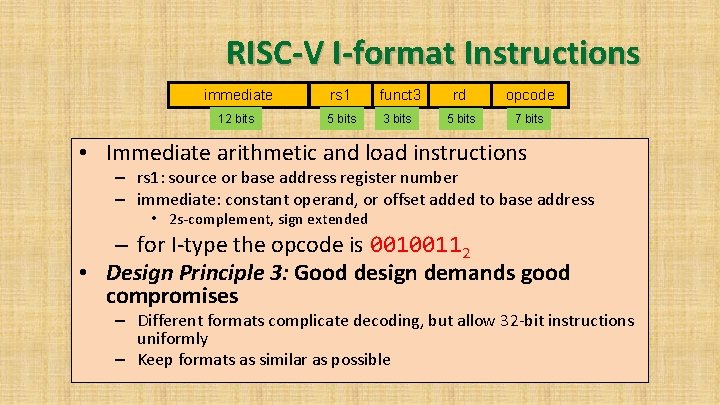
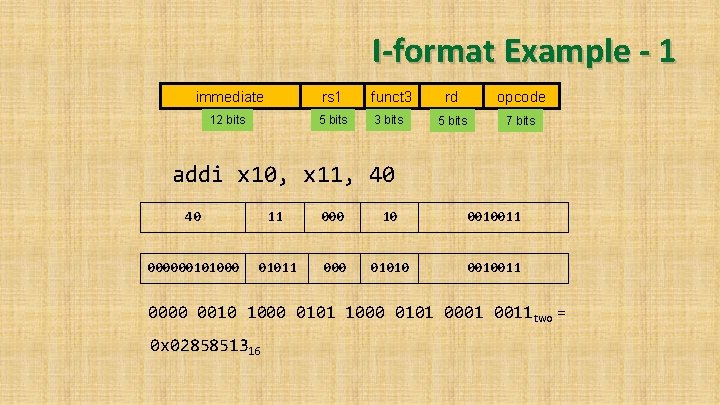
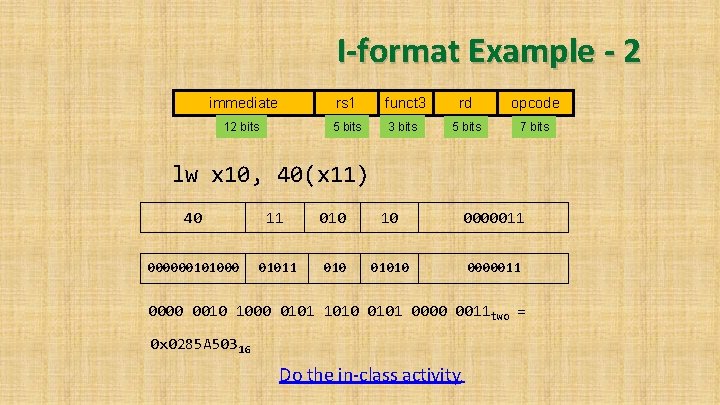
- Slides: 16
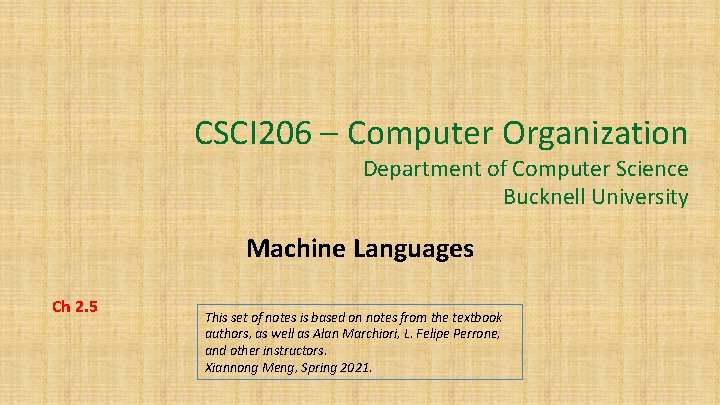
CSCI 206 – Computer Organization Department of Computer Science Bucknell University Machine Languages Ch 2. 5 This set of notes is based on notes from the textbook authors, as well as Alan Marchiori, L. Felipe Perrone, and other instructors. Xiannong Meng, Spring 2021.
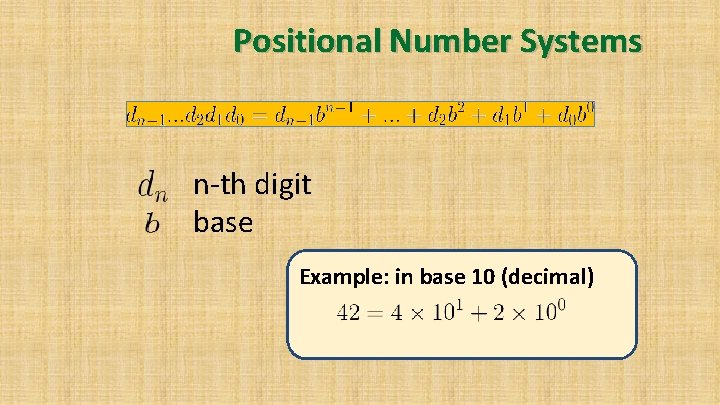
Positional Number Systems n-th digit base Example: in base 10 (decimal)
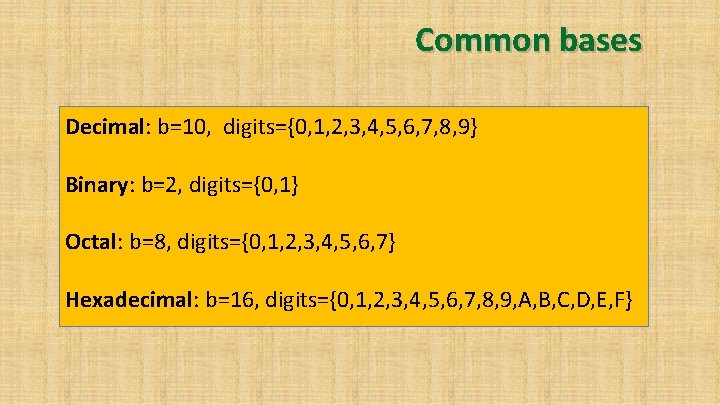
Common bases Decimal: b=10, digits={0, 1, 2, 3, 4, 5, 6, 7, 8, 9} Binary: b=2, digits={0, 1} Octal: b=8, digits={0, 1, 2, 3, 4, 5, 6, 7} Hexadecimal: b=16, digits={0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F}
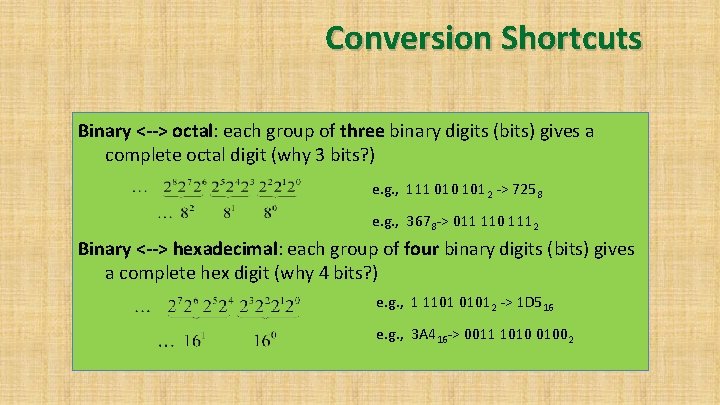
Conversion Shortcuts Binary <--> octal: each group of three binary digits (bits) gives a complete octal digit (why 3 bits? ) e. g. , 111 010 1012 -> 7258 e. g. , 3678 -> 011 110 1112 Binary <--> hexadecimal: each group of four binary digits (bits) gives a complete hex digit (why 4 bits? ) e. g. , 1 1101 01012 -> 1 D 516 e. g. , 3 A 416 -> 0011 1010 01002
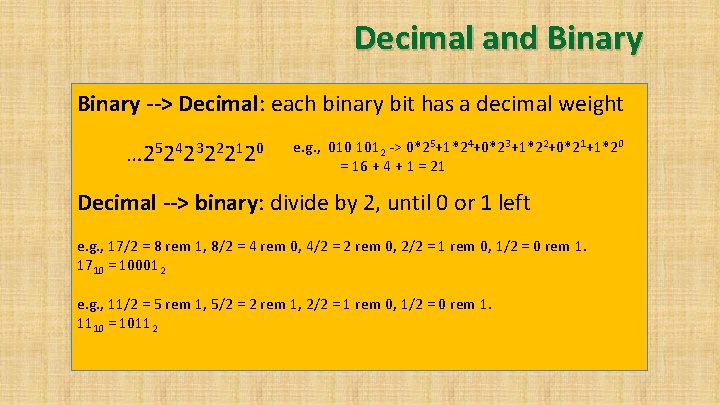
Decimal and Binary --> Decimal: each binary bit has a decimal weight … 252423222120 e. g. , 010 1012 -> 0*25+1*24+0*23+1*22+0*21+1*20 = 16 + 4 + 1 = 21 Decimal --> binary: divide by 2, until 0 or 1 left e. g. , 17/2 = 8 rem 1, 8/2 = 4 rem 0, 4/2 = 2 rem 0, 2/2 = 1 rem 0, 1/2 = 0 rem 1. 1710 = 100012 e. g. , 11/2 = 5 rem 1, 5/2 = 2 rem 1, 2/2 = 1 rem 0, 1/2 = 0 rem 1. 1110 = 10112
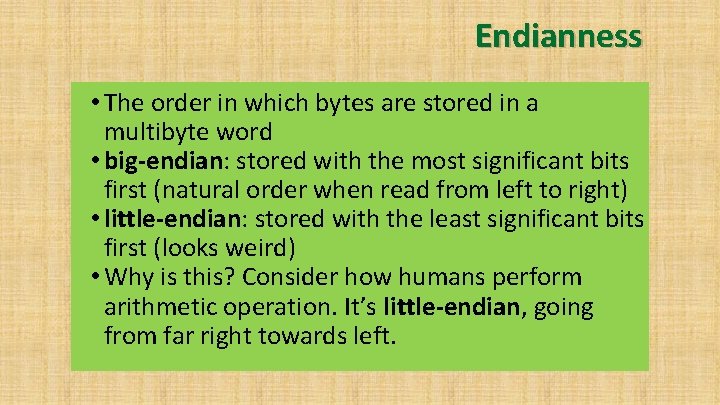
Endianness • The order in which bytes are stored in a multibyte word • big-endian: stored with the most significant bits first (natural order when read from left to right) • little-endian: stored with the least significant bits first (looks weird) • Why is this? Consider how humans perform arithmetic operation. It’s little-endian, going from far right towards left.
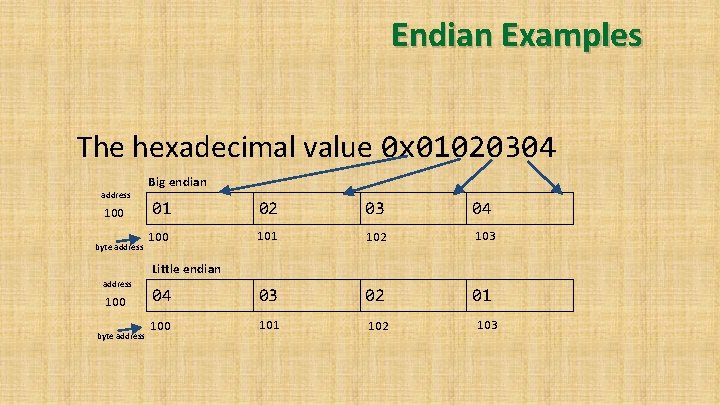
Endian Examples The hexadecimal value 0 x 01020304 address 100 byte address Big endian 01 02 03 04 100 101 102 103 04 03 02 01 100 101 102 Little endian address 100 byte address 103
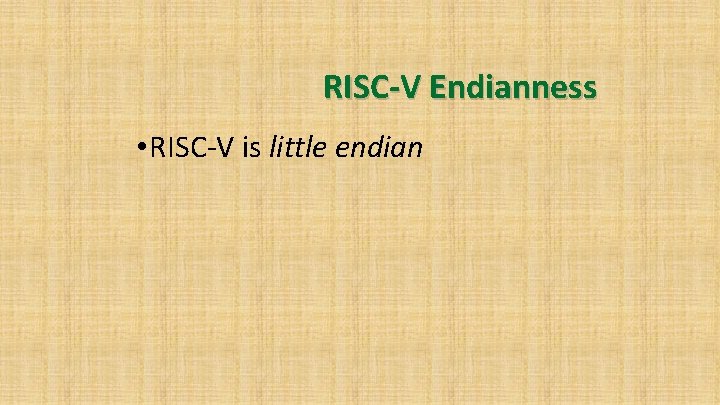
RISC-V Endianness • RISC-V is little endian
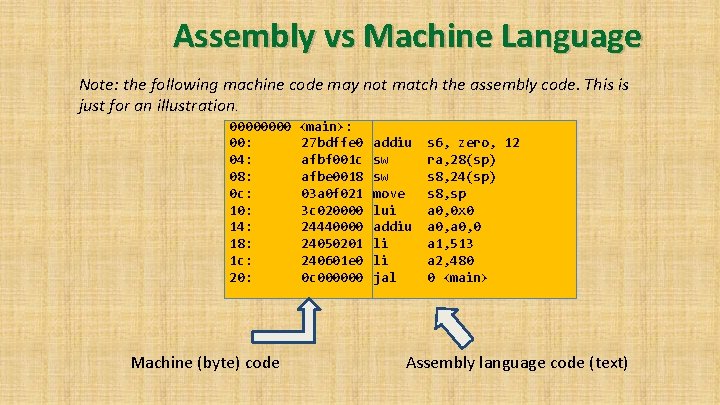
Assembly vs Machine Language Note: the following machine code may not match the assembly code. This is just for an illustration. 0000 00: 04: 08: 0 c: 10: 14: 18: 1 c: 20: Machine (byte) code <main>: 27 bdffe 0 afbf 001 c afbe 0018 03 a 0 f 021 3 c 020000 24440000 24050201 240601 e 0 0 c 000000 addiu sw sw move lui addiu li li jal s 6, zero, 12 ra, 28(sp) s 8, 24(sp) s 8, sp a 0, 0 x 0 a 0, 0 a 1, 513 a 2, 480 0 <main> Assembly language code (text)
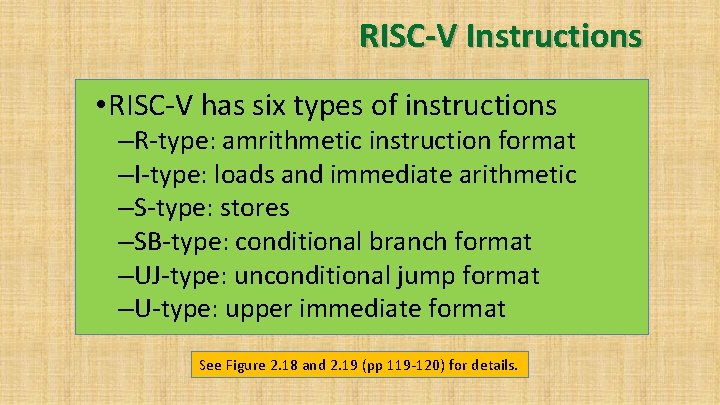
RISC-V Instructions • RISC-V has six types of instructions –R-type: amrithmetic instruction format –I-type: loads and immediate arithmetic –S-type: stores –SB-type: conditional branch format –UJ-type: unconditional jump format –U-type: upper immediate format See Figure 2. 18 and 2. 19 (pp 119 -120) for details.
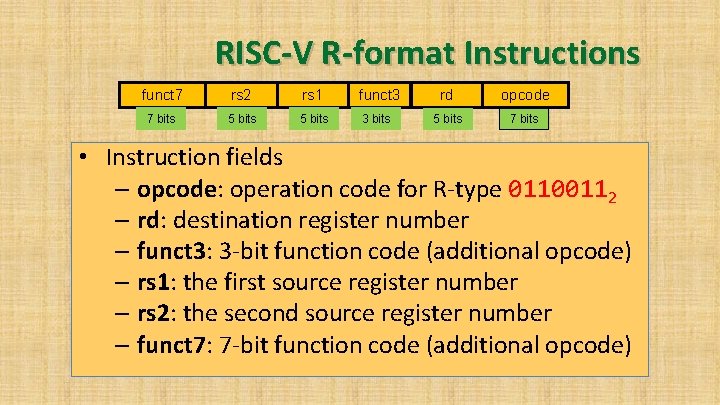
RISC-V R-format Instructions funct 7 rs 2 rs 1 funct 3 7 bits 5 bits 3 bits rd opcode 5 bits 7 bits • Instruction fields – opcode: operation code for R-type 01100112 – rd: destination register number – funct 3: 3 -bit function code (additional opcode) – rs 1: the first source register number – rs 2: the second source register number – funct 7: 7 -bit function code (additional opcode)
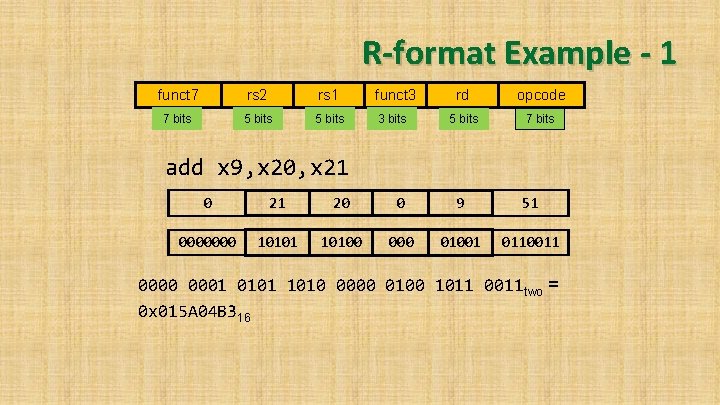
R-format Example - 1 funct 7 rs 2 rs 1 funct 3 7 bits 5 bits 3 bits rd opcode 5 bits 7 bits add x 9, x 20, x 21 0 21 20 0 9 51 0000000 10101 101001 0110011 0000 0001 0101 1010 0000 0100 1011 0011 two = 0 x 015 A 04 B 316
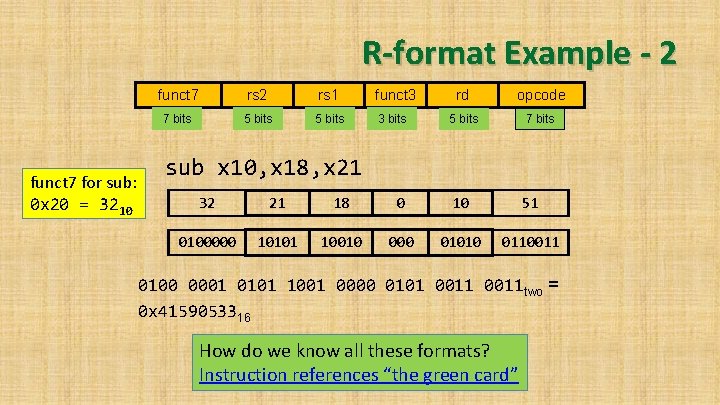
R-format Example - 2 funct 7 for sub: 0 x 20 = 3210 funct 7 rs 2 rs 1 funct 3 7 bits 5 bits 3 bits rd opcode 5 bits 7 bits sub x 10, x 18, x 21 32 21 18 0 10 51 0100000 10101 10010 000 01010 0110011 0100 0001 0101 1001 0000 0101 0011 two = 0 x 4159053316 How do we know all these formats? Instruction references “the green card”
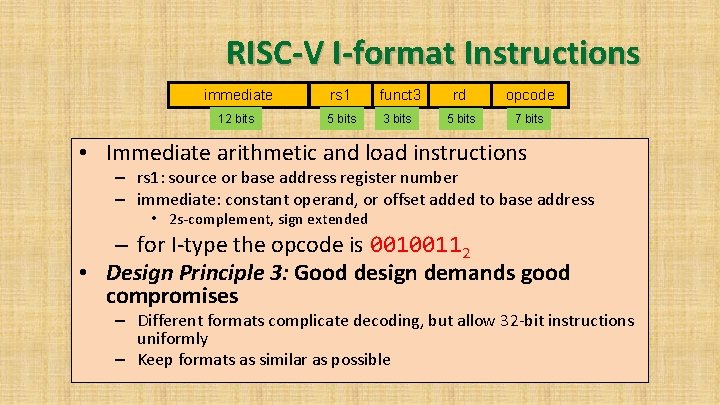
RISC-V I-format Instructions immediate rs 1 funct 3 rd opcode 12 bits 5 bits 3 bits 5 bits 7 bits • Immediate arithmetic and load instructions – rs 1: source or base address register number – immediate: constant operand, or offset added to base address • 2 s-complement, sign extended – for I-type the opcode is 00100112 • Design Principle 3: Good design demands good compromises – Different formats complicate decoding, but allow 32 -bit instructions uniformly – Keep formats as similar as possible
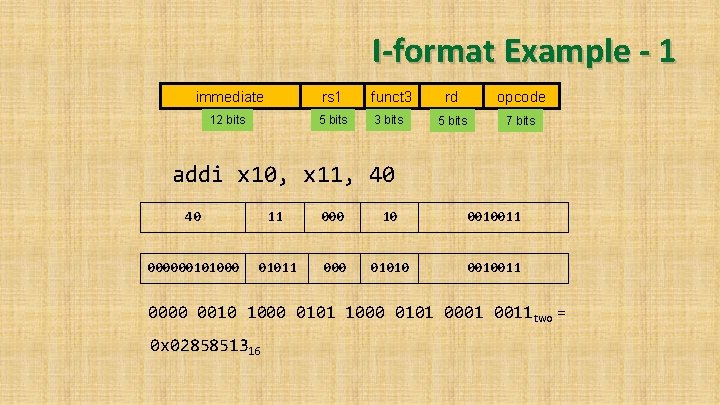
I-format Example - 1 immediate rs 1 funct 3 rd opcode 12 bits 5 bits 3 bits 5 bits 7 bits addi x 10, x 11, 40 40 11 000 10 0010011 000000101000 01011 000 01010 0010011 0000 0010 1000 0101 0001 0011 two = 0 x 0285851316
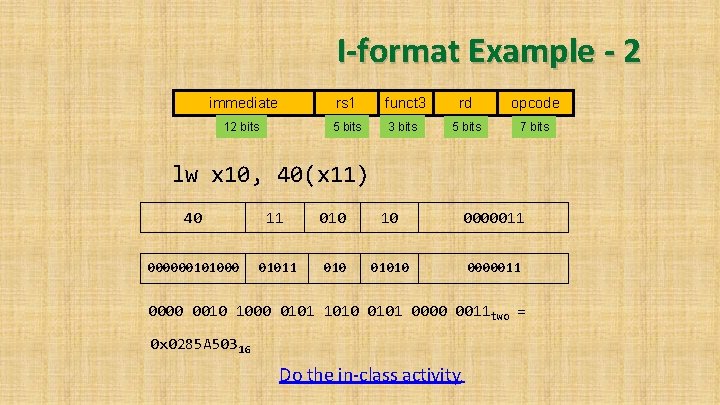
I-format Example - 2 immediate rs 1 funct 3 rd opcode 12 bits 5 bits 3 bits 5 bits 7 bits lw x 10, 40(x 11) 40 11 010 10 0000011 000000101000 01011 01010 0000011 0000 0010 1000 0101 1010 0101 0000 0011 two = 0 x 0285 A 50316 Do the in-class activity.
Process organization in computer organization
Brad karp ucl
Northwestern computer science department
Computer science department rutgers
Computer science department stanford
Florida state university computer science faculty
Trimentoring
Department of computer science christ
Mice.cs.columbia
My favourite subject is english because
Structure of computers
Computer architecture and organisation
Basic computer organization and design
Basic computer organization
Ir + a + infinitive (p. 206) answers
Ee 206
Comp 206