CSC 211 Data Structures Lecture 8 Dynamic Classes
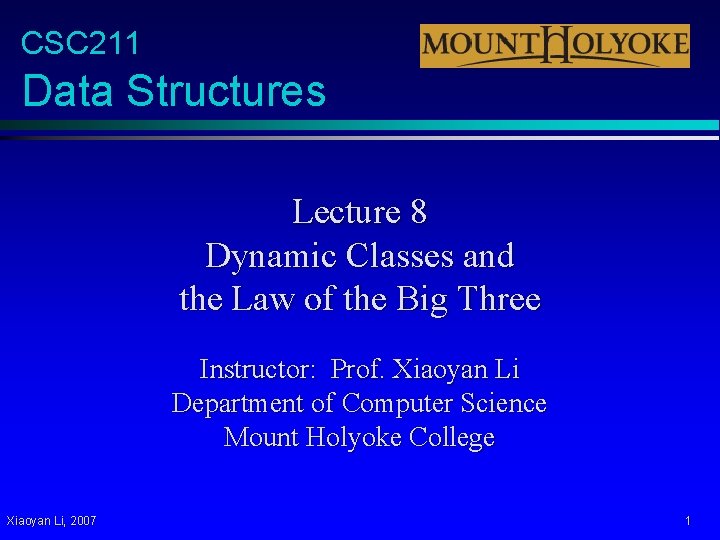
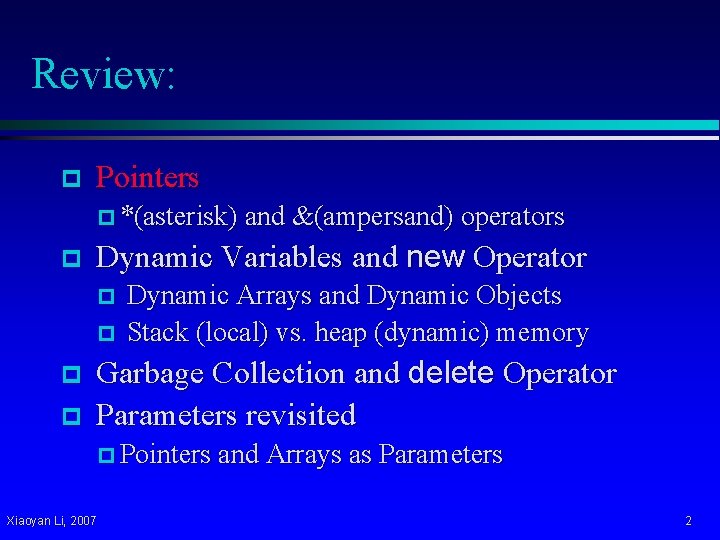
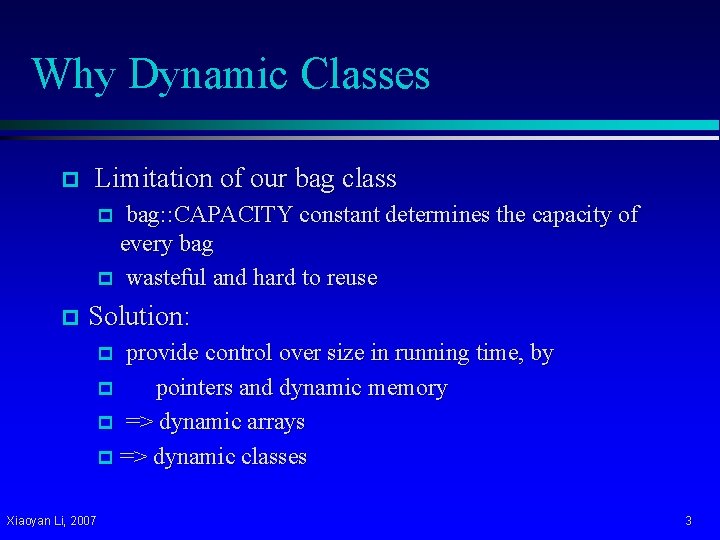
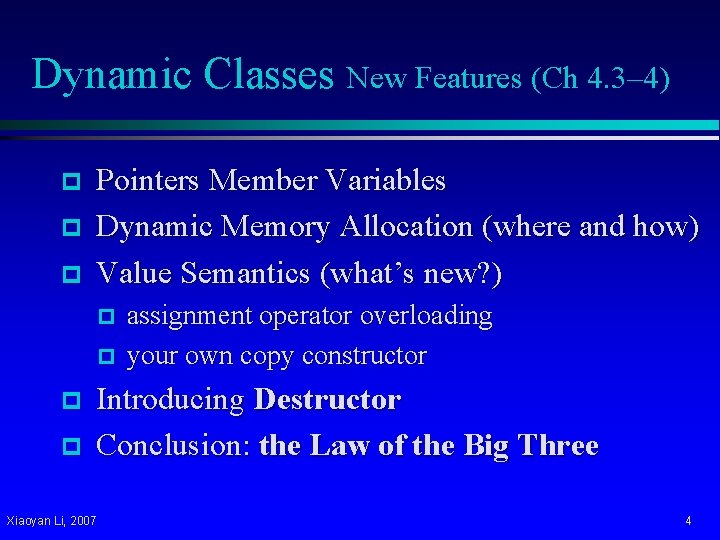
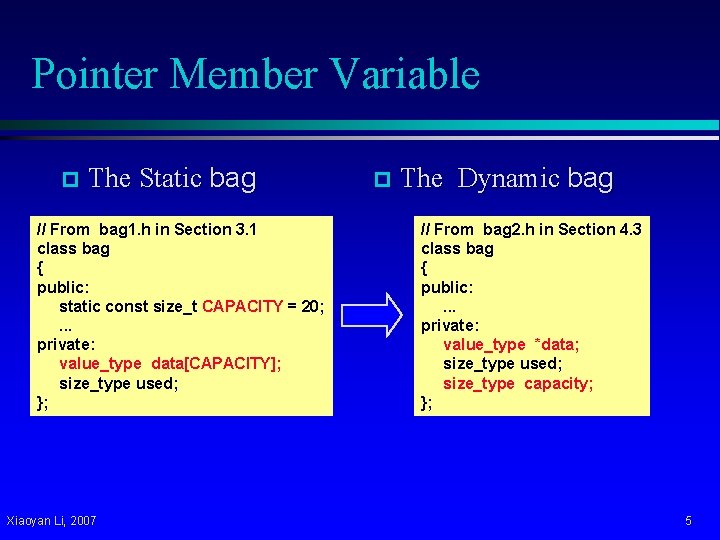
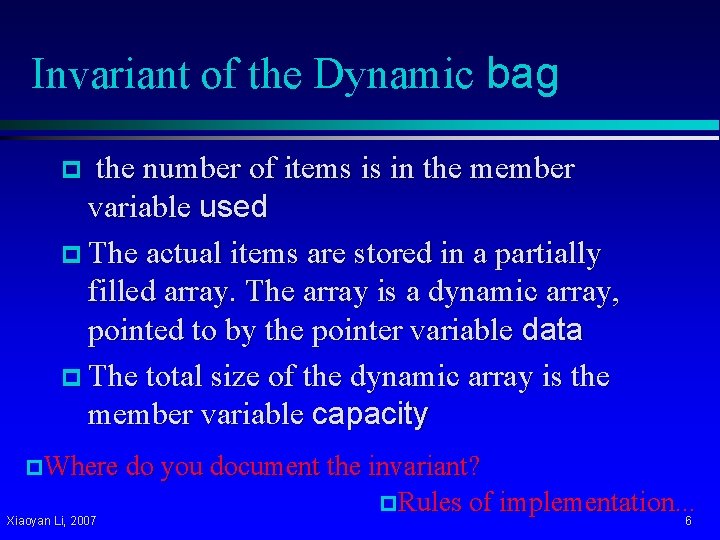
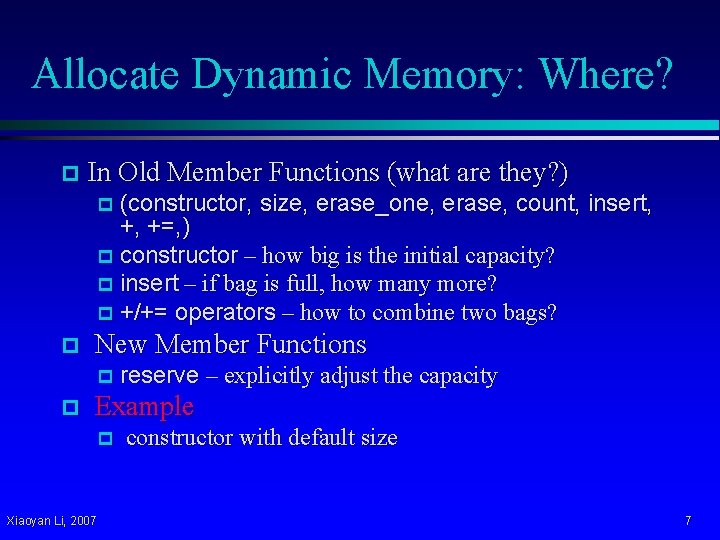
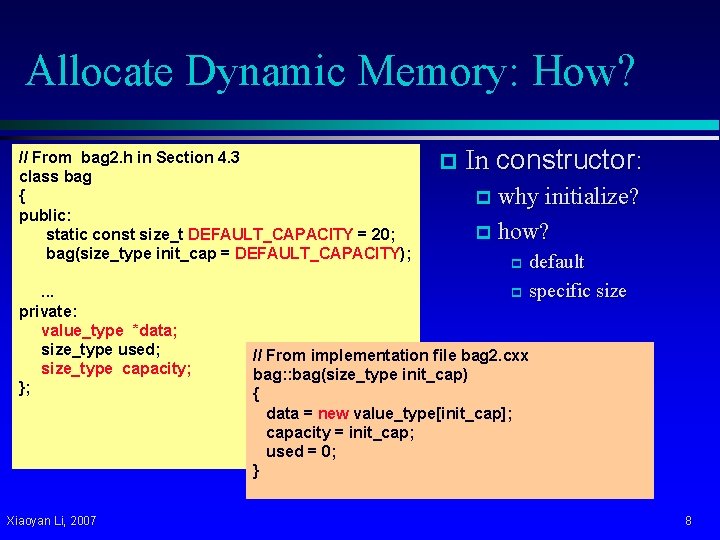
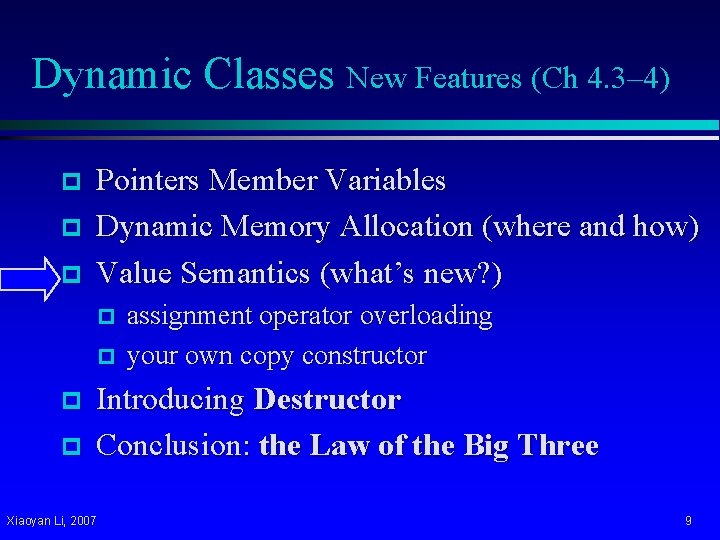
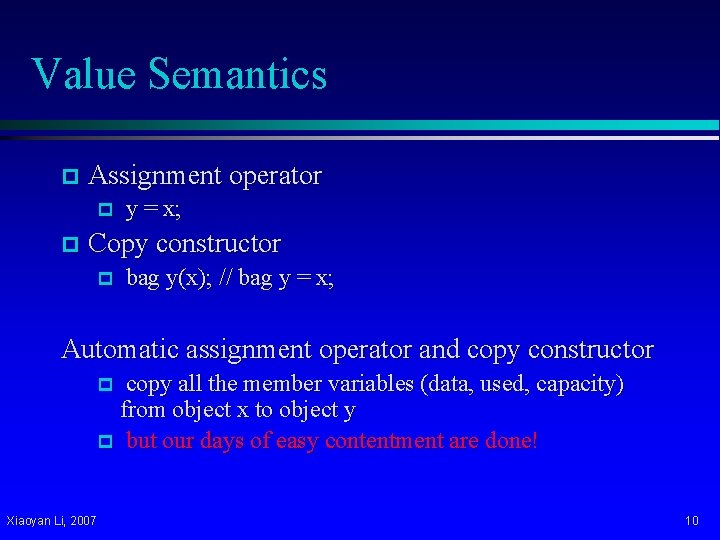
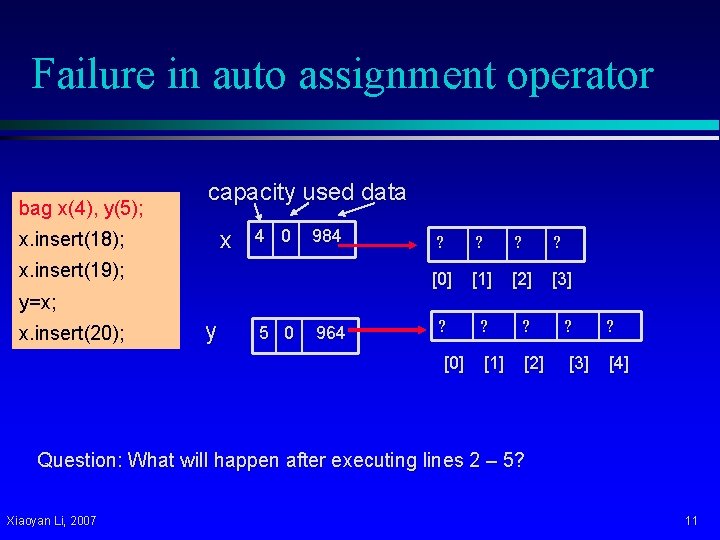
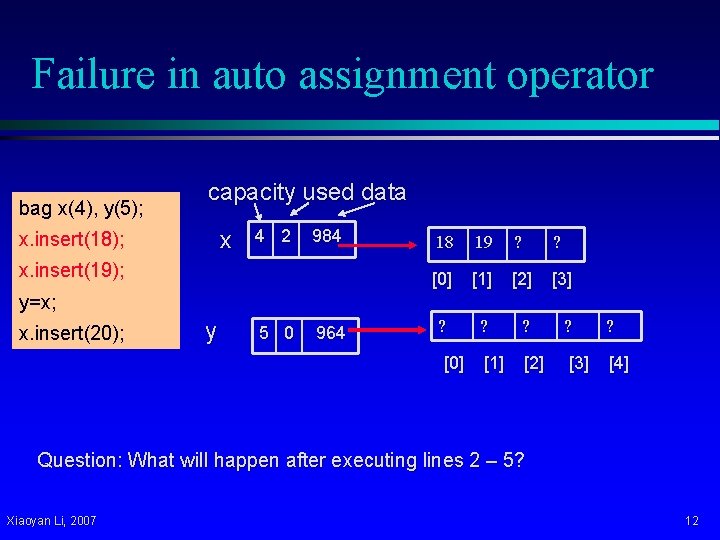
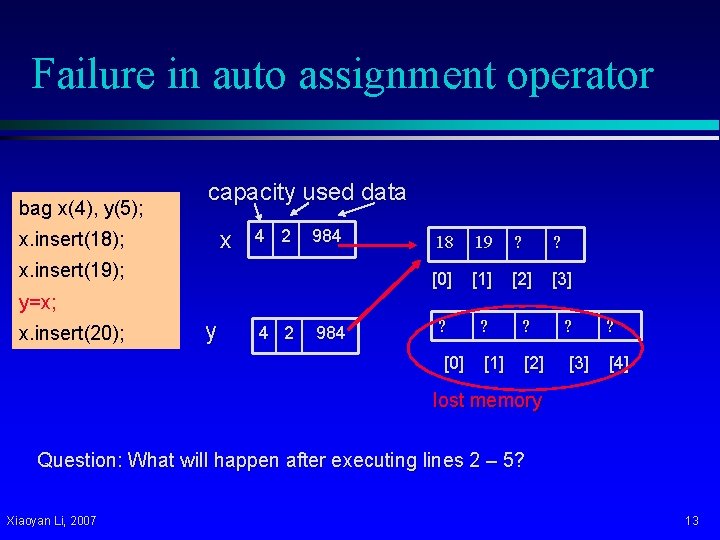
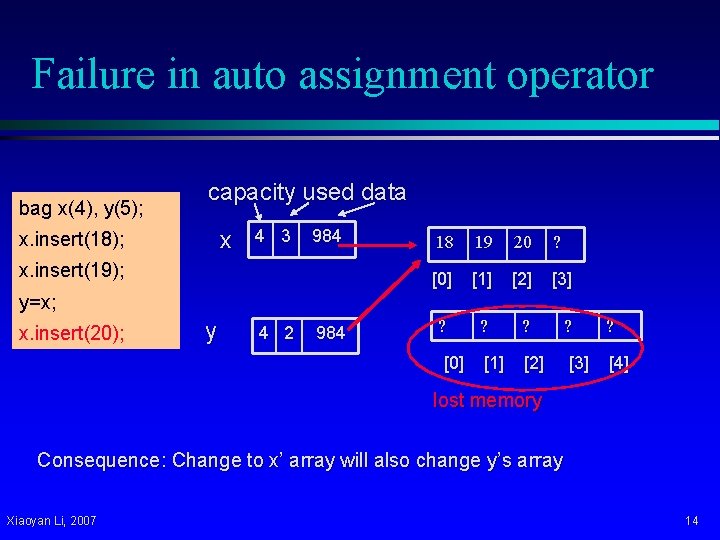
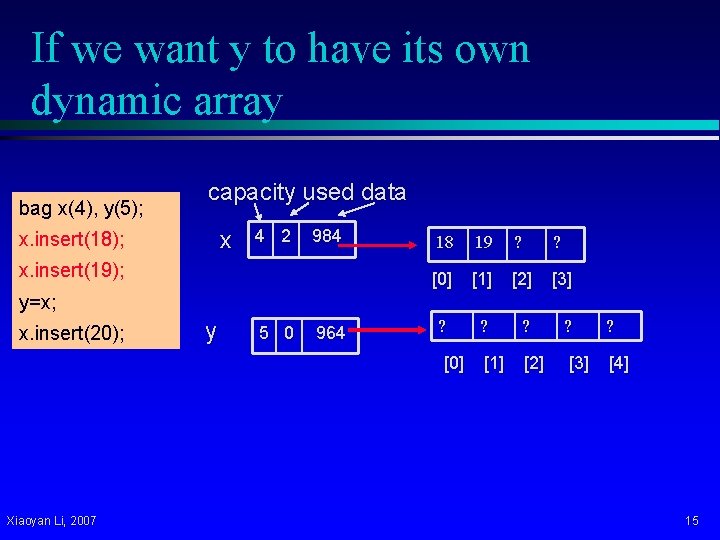
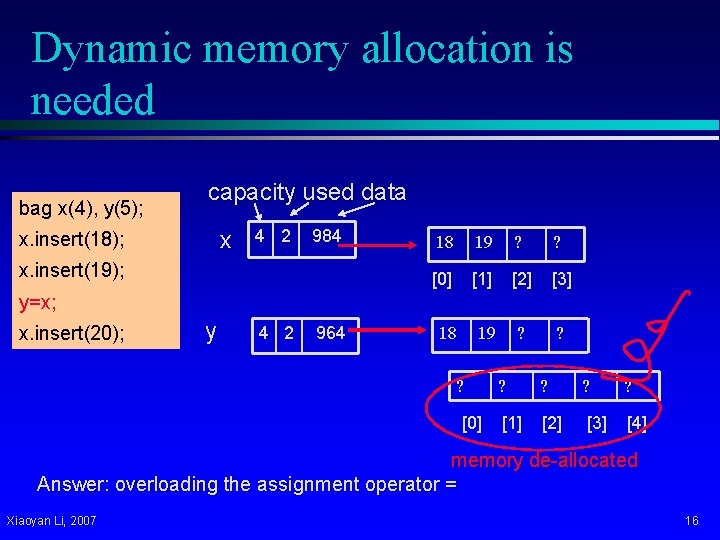
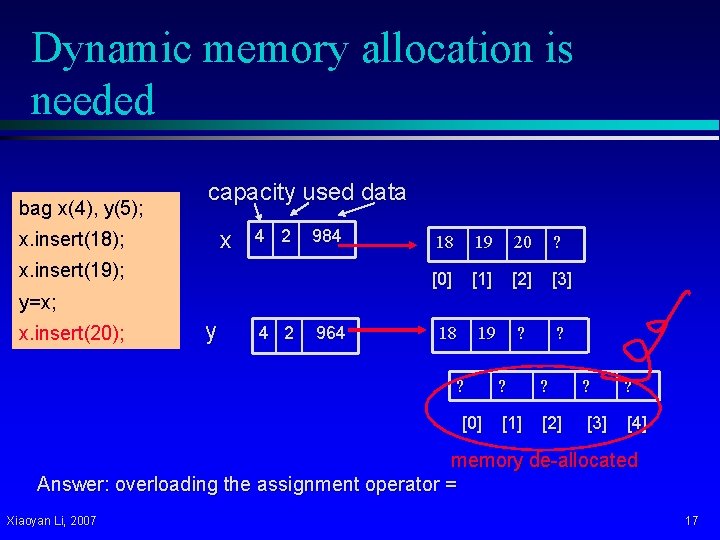
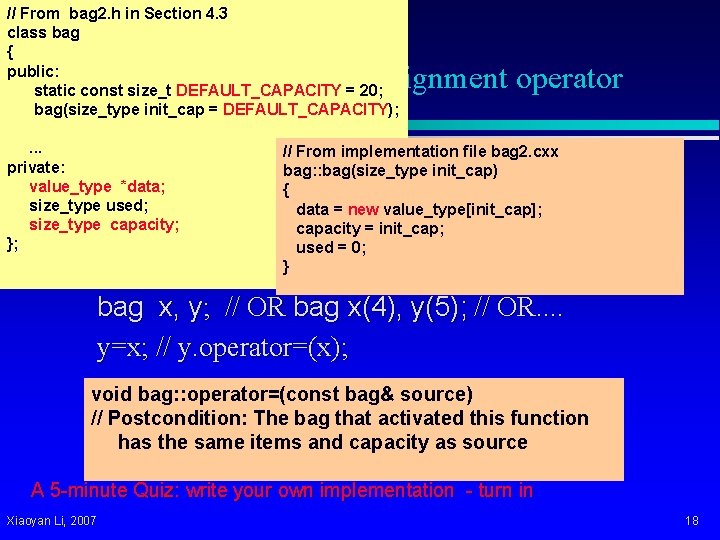
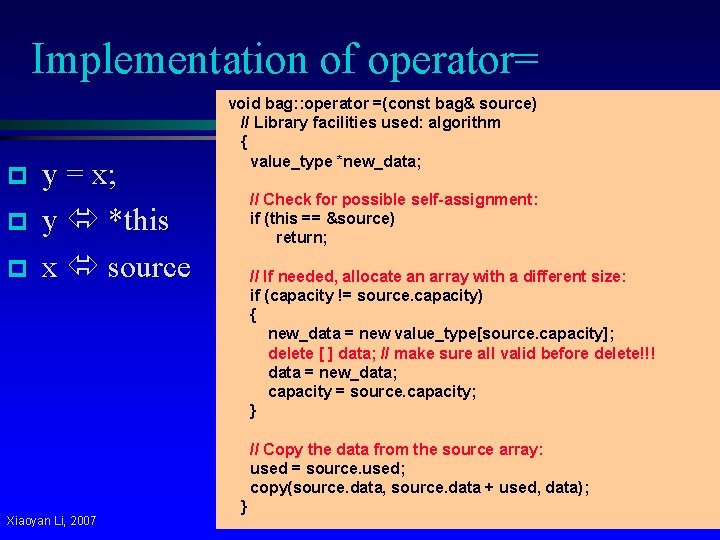
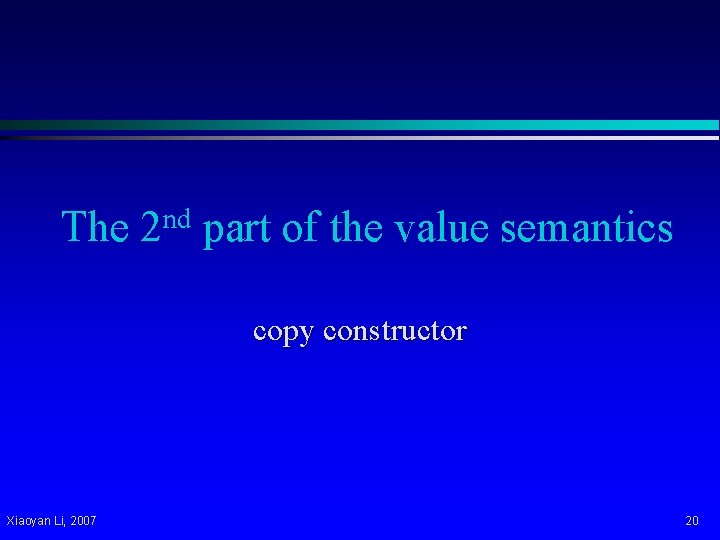
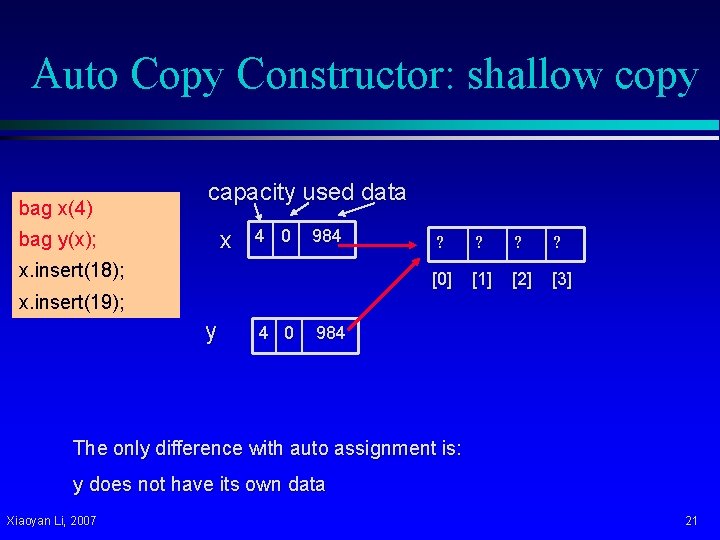
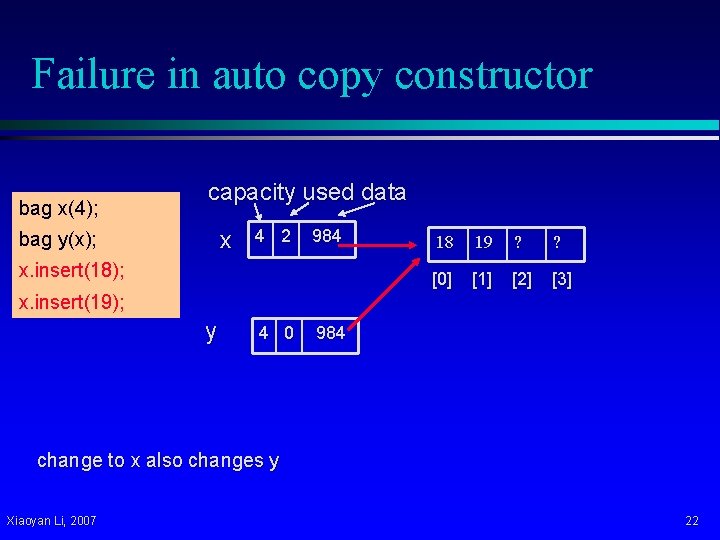
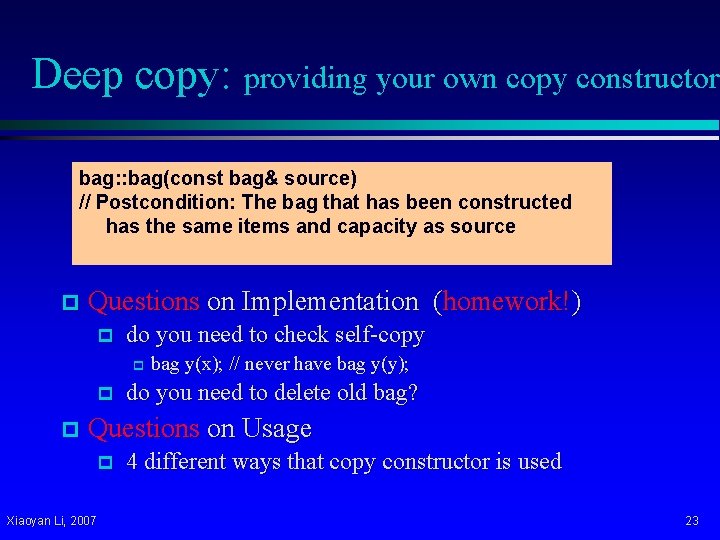
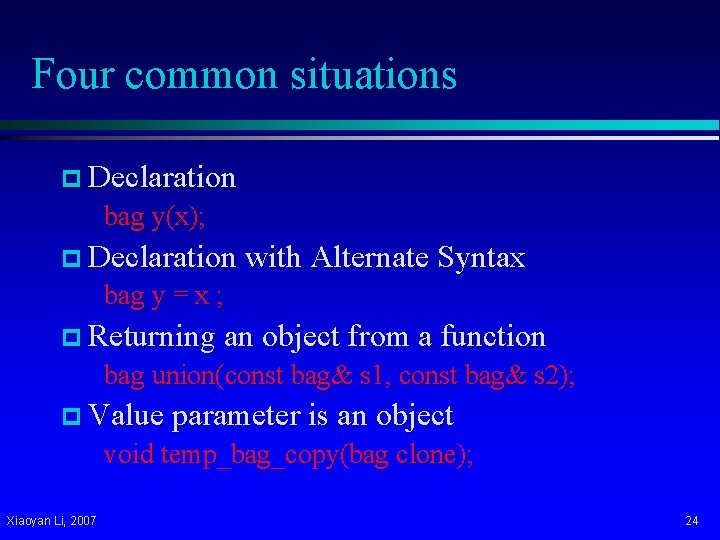
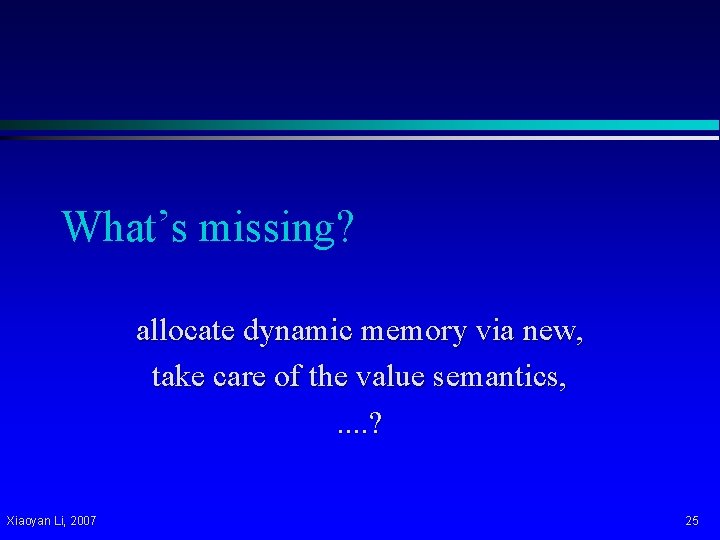
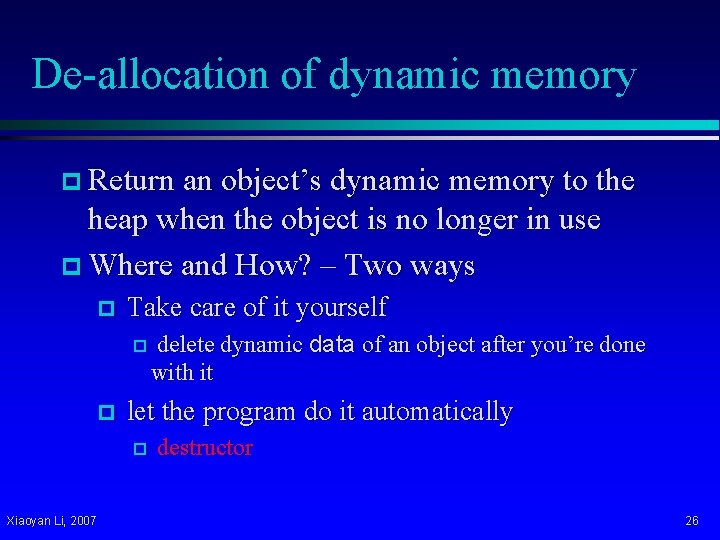
![Destructor bag: : ~bag() { delete [ ] data; } The primary purpose is Destructor bag: : ~bag() { delete [ ] data; } The primary purpose is](https://slidetodoc.com/presentation_image_h2/ff67daee56054198f2ef6555a53c861e/image-27.jpg)
![Destructor p bag: : ~bag() { delete [ ] data; } Some common situations Destructor p bag: : ~bag() { delete [ ] data; } Some common situations](https://slidetodoc.com/presentation_image_h2/ff67daee56054198f2ef6555a53c861e/image-28.jpg)
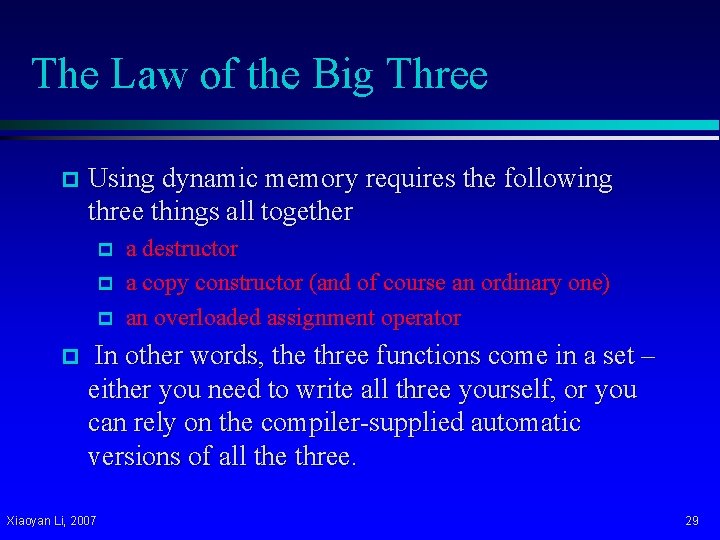
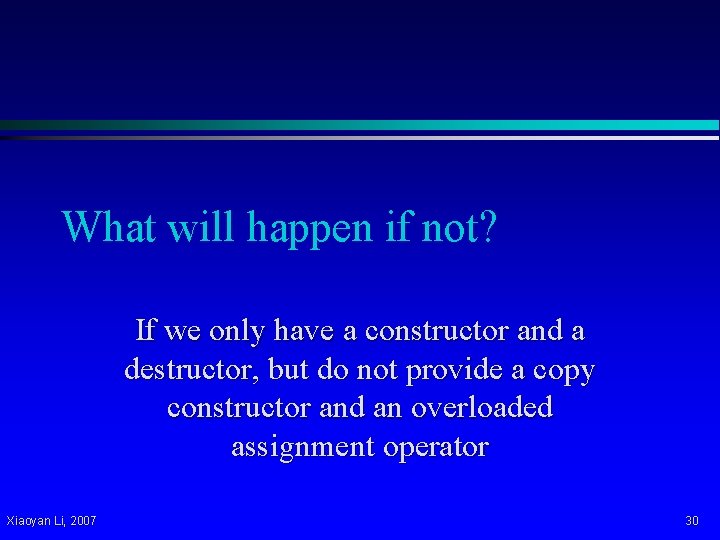
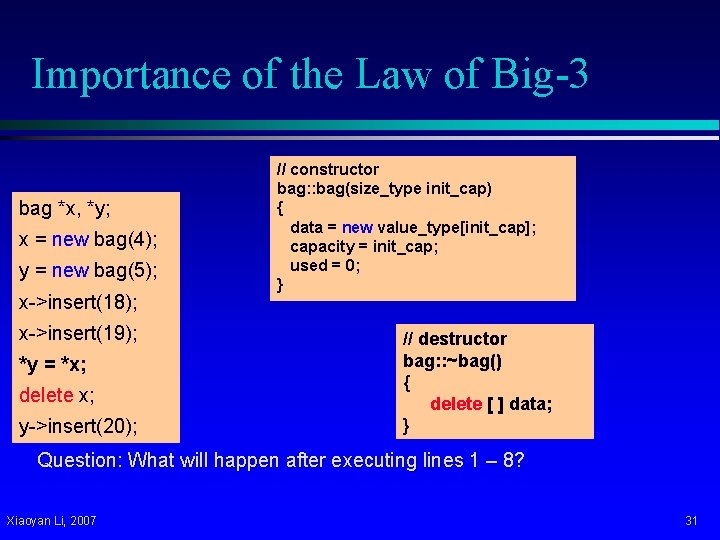
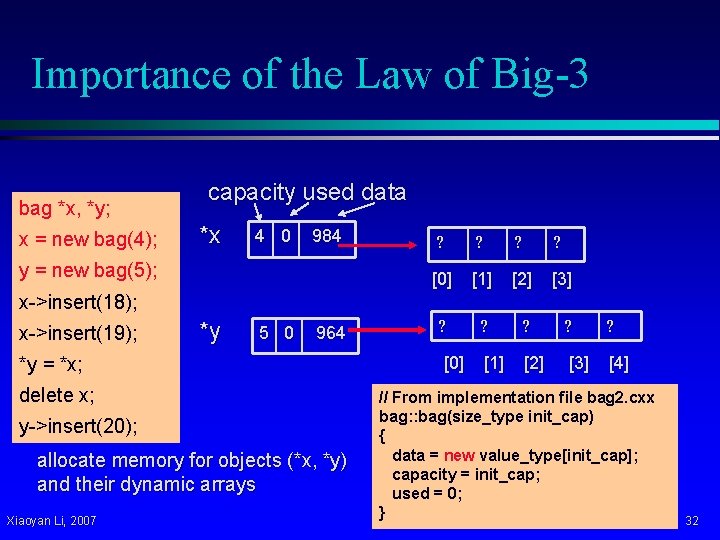
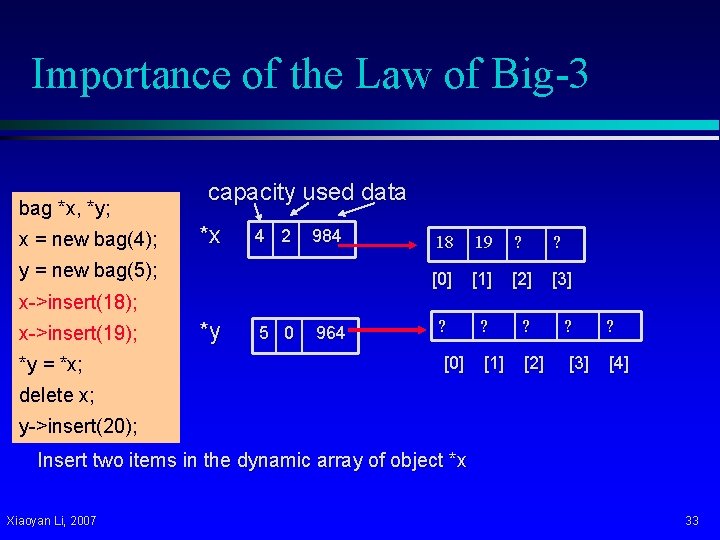
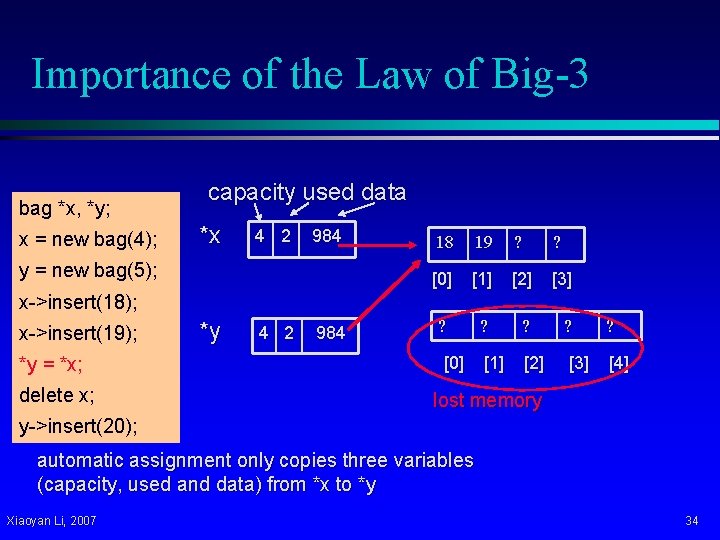
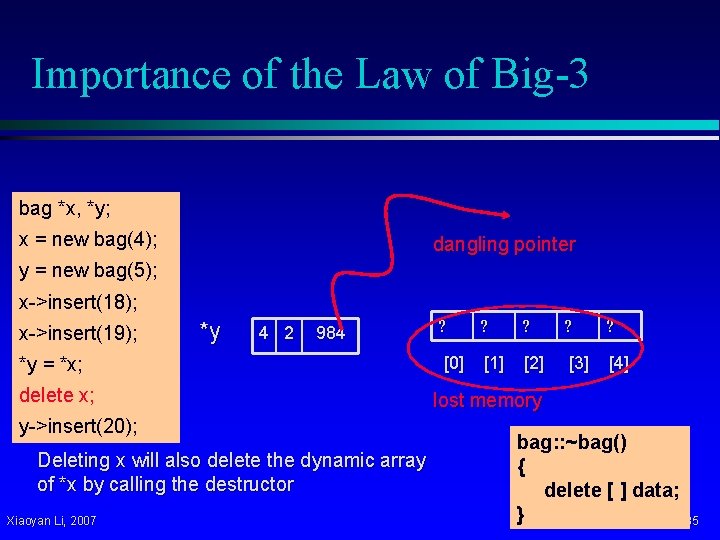
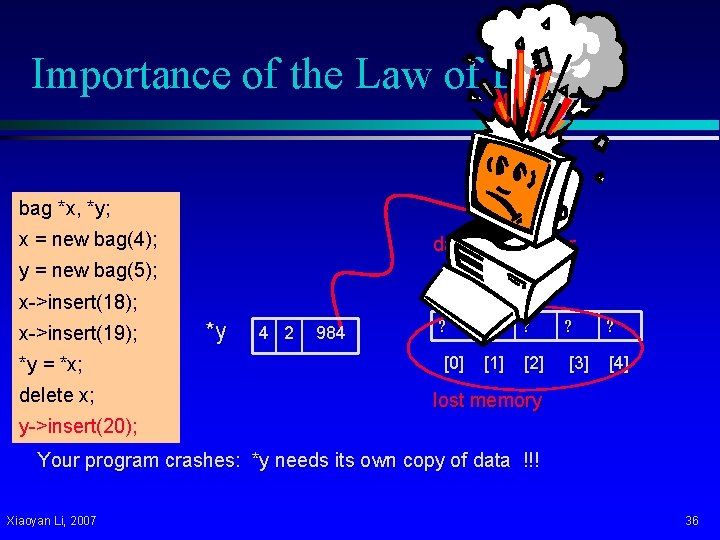
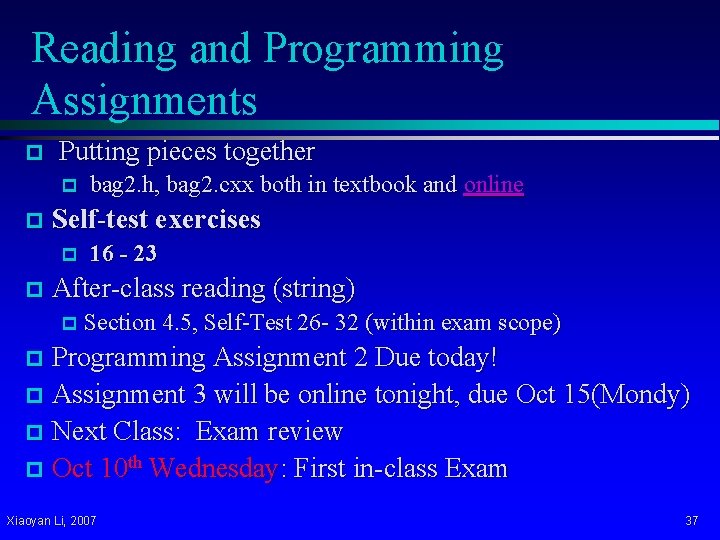
- Slides: 37
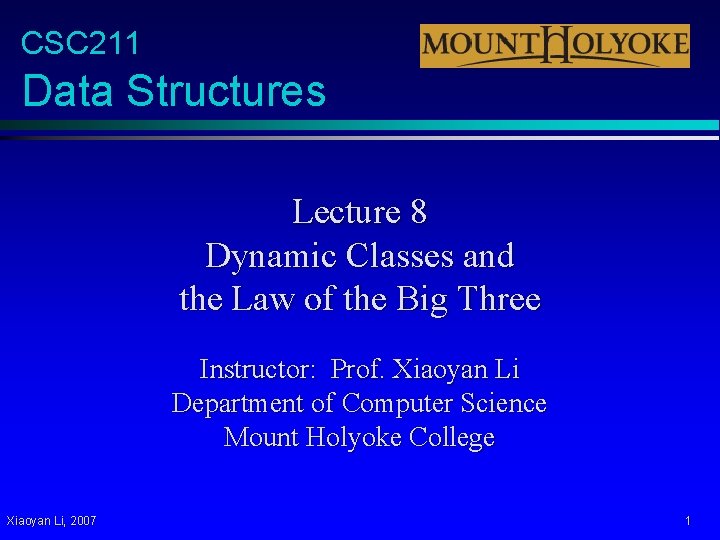
CSC 211 Data Structures Lecture 8 Dynamic Classes and the Law of the Big Three Instructor: Prof. Xiaoyan Li Department of Computer Science Mount Holyoke College Xiaoyan Li, 2007 1
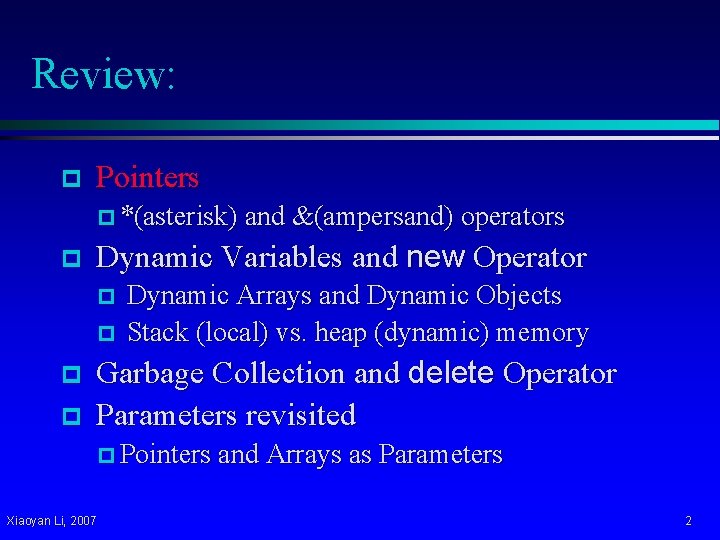
Review: p Pointers p *(asterisk) and &(ampersand) operators p Dynamic Variables and new Operator p p Dynamic Arrays and Dynamic Objects Stack (local) vs. heap (dynamic) memory Garbage Collection and delete Operator Parameters revisited p Pointers and Arrays as Parameters Xiaoyan Li, 2007 2
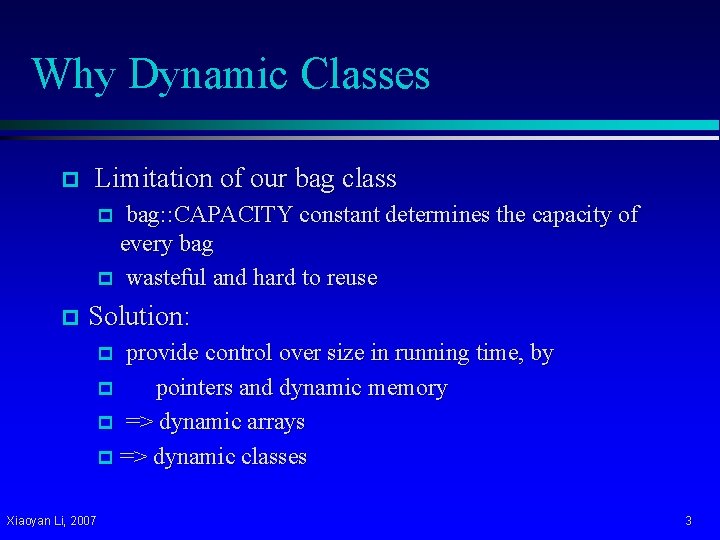
Why Dynamic Classes p Limitation of our bag class bag: : CAPACITY constant determines the capacity of every bag p wasteful and hard to reuse p p Solution: provide control over size in running time, by p pointers and dynamic memory p => dynamic arrays p => dynamic classes p Xiaoyan Li, 2007 3
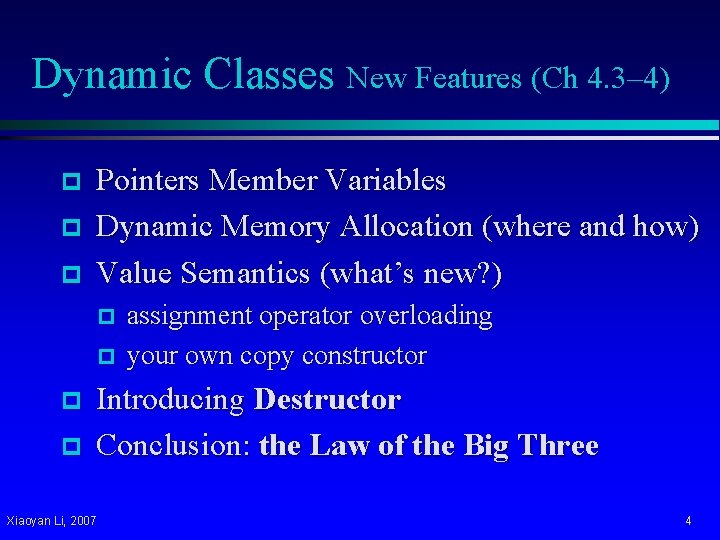
Dynamic Classes New Features (Ch 4. 3– 4) p p p Pointers Member Variables Dynamic Memory Allocation (where and how) Value Semantics (what’s new? ) p p assignment operator overloading your own copy constructor Introducing Destructor Conclusion: the Law of the Big Three Xiaoyan Li, 2007 4
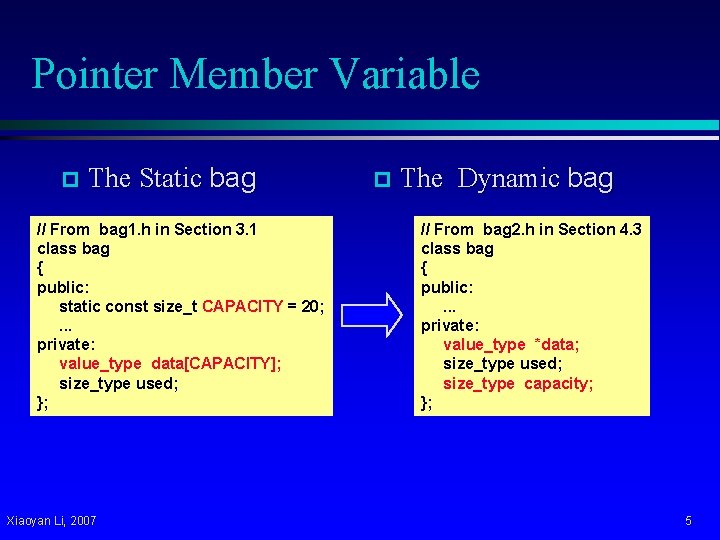
Pointer Member Variable p The Static bag // From bag 1. h in Section 3. 1 class bag { public: static const size_t CAPACITY = 20; . . . private: value_type data[CAPACITY]; size_type used; }; Xiaoyan Li, 2007 p The Dynamic bag // From bag 2. h in Section 4. 3 class bag { public: . . . private: value_type *data; size_type used; size_type capacity; }; 5
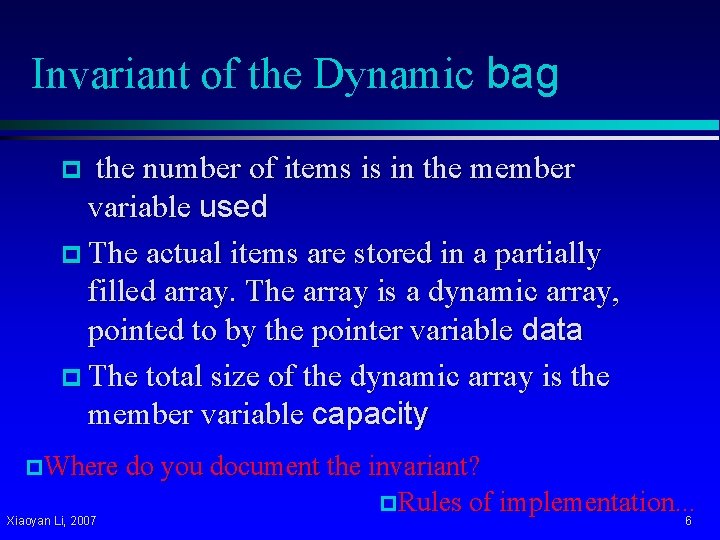
Invariant of the Dynamic bag the number of items is in the member variable used p The actual items are stored in a partially filled array. The array is a dynamic array, pointed to by the pointer variable data p The total size of the dynamic array is the member variable capacity p p. Where do you document the invariant? Xiaoyan Li, 2007 p. Rules of implementation. . . 6
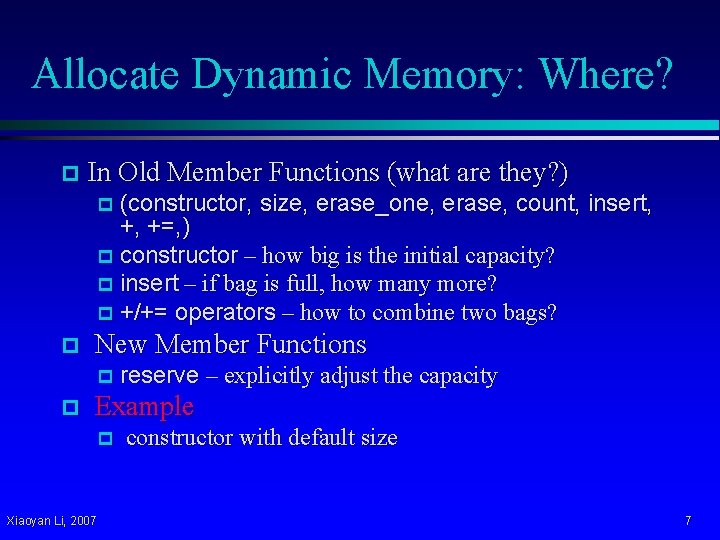
Allocate Dynamic Memory: Where? p In Old Member Functions (what are they? ) p (constructor, size, erase_one, erase, count, insert, +, +=, ) p constructor – how big is the initial capacity? p insert – if bag is full, how many more? p +/+= operators – how to combine two bags? p New Member Functions p reserve – explicitly adjust the capacity p Example p Xiaoyan Li, 2007 constructor with default size 7
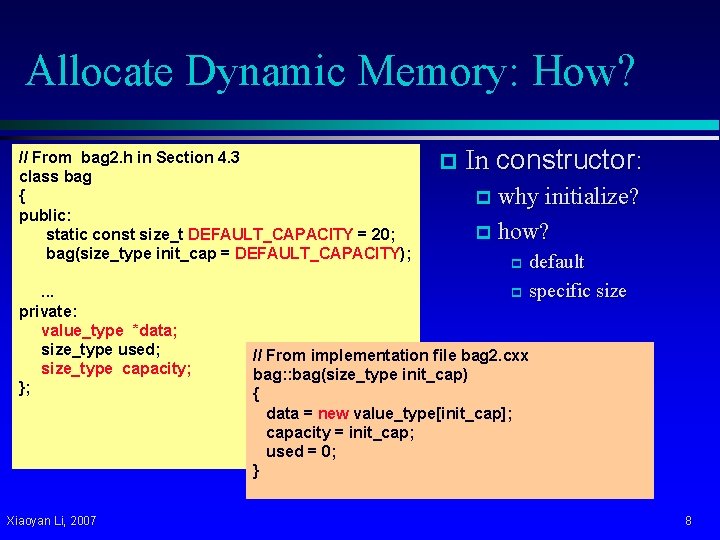
Allocate Dynamic Memory: How? // From bag 2. h in Section 4. 3 class bag { public: static const size_t DEFAULT_CAPACITY = 20; bag(size_type init_cap = DEFAULT_CAPACITY); . . . private: value_type *data; size_type used; size_type capacity; }; Xiaoyan Li, 2007 p In constructor: p why initialize? p how? default p specific size p // From implementation file bag 2. cxx bag: : bag(size_type init_cap) { data = new value_type[init_cap]; capacity = init_cap; used = 0; } 8
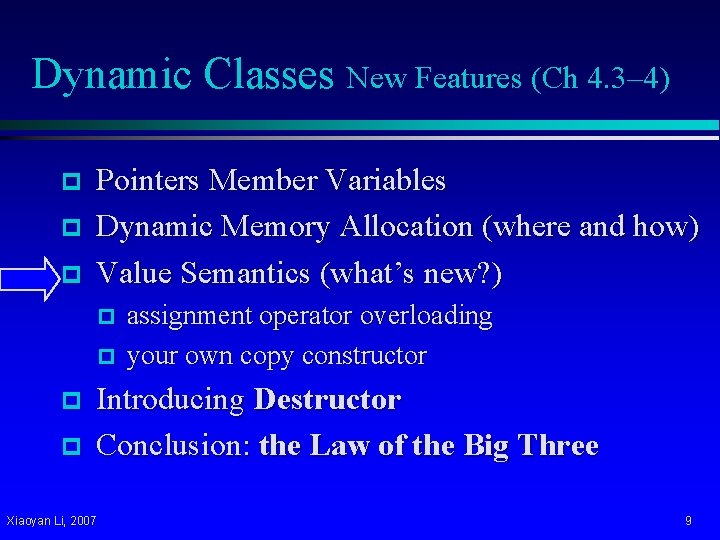
Dynamic Classes New Features (Ch 4. 3– 4) p p p Pointers Member Variables Dynamic Memory Allocation (where and how) Value Semantics (what’s new? ) p p assignment operator overloading your own copy constructor Introducing Destructor Conclusion: the Law of the Big Three Xiaoyan Li, 2007 9
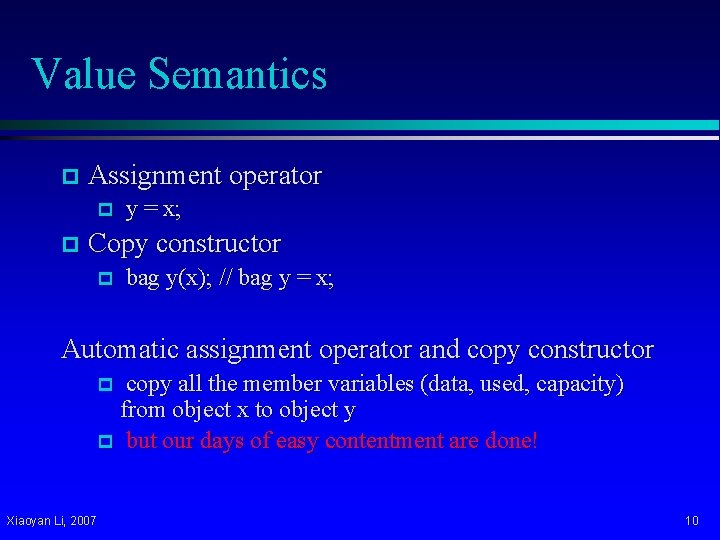
Value Semantics p Assignment operator p p y = x; Copy constructor p bag y(x); // bag y = x; Automatic assignment operator and copy constructor copy all the member variables (data, used, capacity) from object x to object y p but our days of easy contentment are done! p Xiaoyan Li, 2007 10
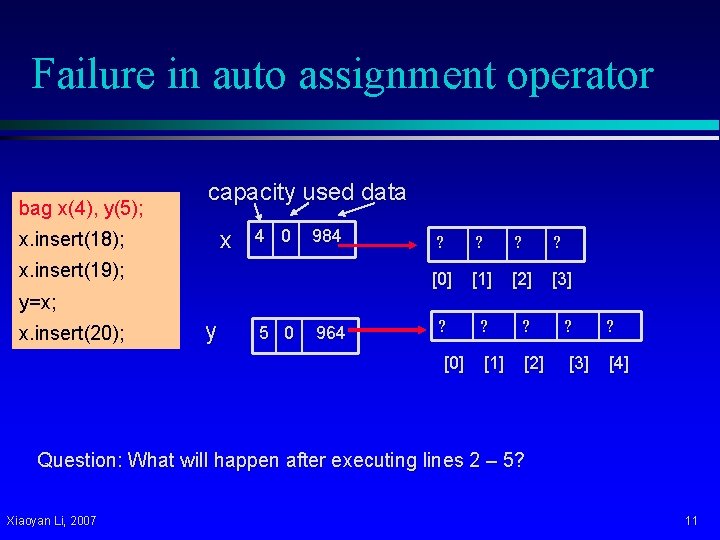
Failure in auto assignment operator bag x(4), y(5); capacity used data x x. insert(18); 4 0 984 x. insert(19); ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] y=x; x. insert(20); y 5 0 964 [0] Question: What will happen after executing lines 2 – 5? Xiaoyan Li, 2007 11
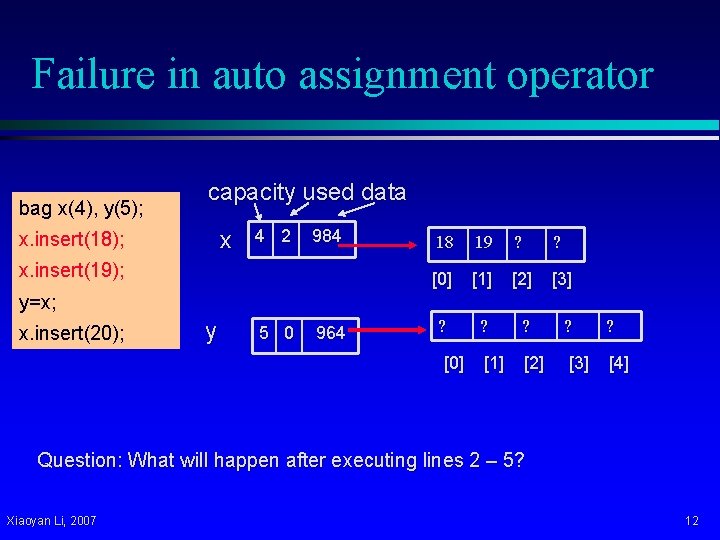
Failure in auto assignment operator bag x(4), y(5); capacity used data x x. insert(18); 4 2 984 x. insert(19); 18 19 ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] y=x; x. insert(20); y 5 0 964 [0] Question: What will happen after executing lines 2 – 5? Xiaoyan Li, 2007 12
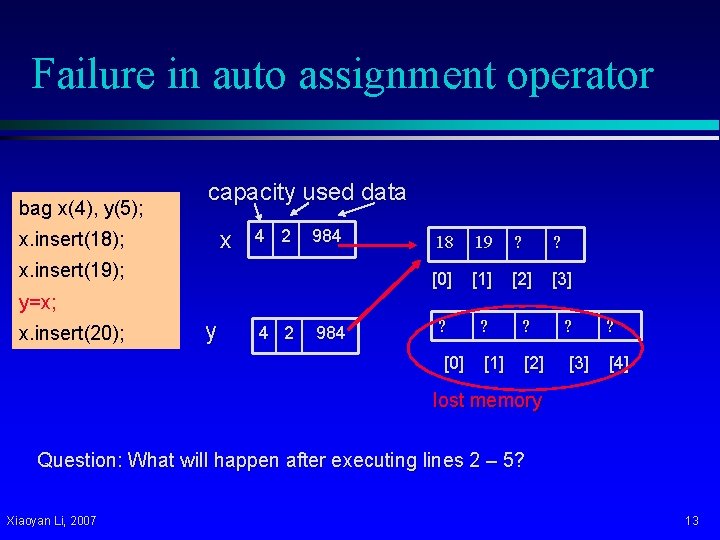
Failure in auto assignment operator bag x(4), y(5); capacity used data x x. insert(18); 4 2 984 x. insert(19); 18 19 ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] y=x; x. insert(20); y 4 2 984 [0] lost memory Question: What will happen after executing lines 2 – 5? Xiaoyan Li, 2007 13
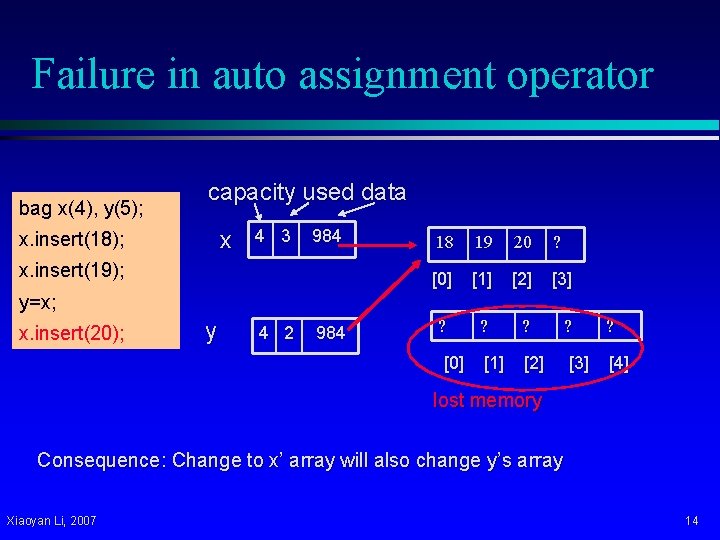
Failure in auto assignment operator bag x(4), y(5); capacity used data x x. insert(18); 4 3 984 x. insert(19); 18 19 20 ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] y=x; x. insert(20); y 4 2 984 [0] lost memory Consequence: Change to x’ array will also change y’s array Xiaoyan Li, 2007 14
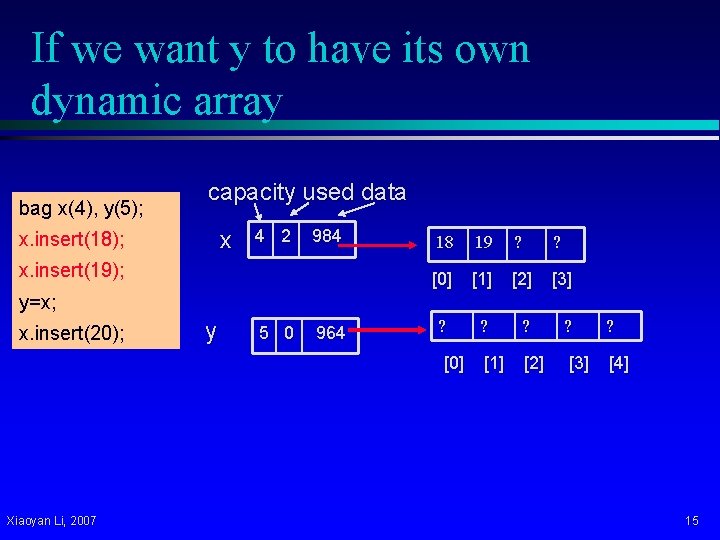
If we want y to have its own dynamic array bag x(4), y(5); capacity used data x x. insert(18); 4 2 984 x. insert(19); 18 19 ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] y=x; x. insert(20); y 5 0 964 [0] Xiaoyan Li, 2007 15
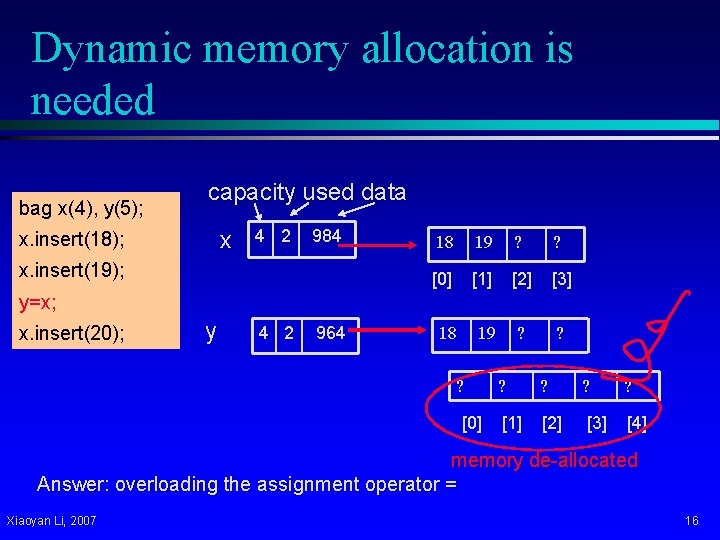
Dynamic memory allocation is needed bag x(4), y(5); capacity used data x x. insert(18); 4 2 984 x. insert(19); 18 19 ? ? [0] [1] [2] [3] 18 19 ? ? y=x; x. insert(20); y 4 2 964 ? [0] ? ? [1] [2] [3] [4] memory de-allocated Answer: overloading the assignment operator = Xiaoyan Li, 2007 16
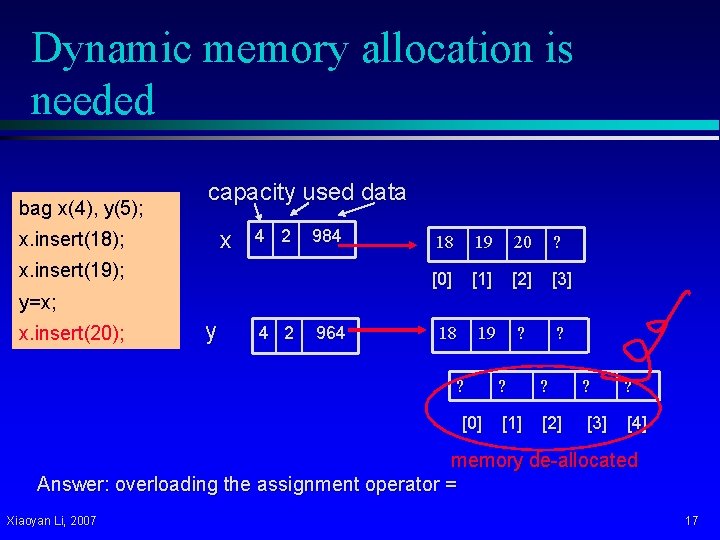
Dynamic memory allocation is needed bag x(4), y(5); capacity used data x x. insert(18); 4 2 984 x. insert(19); 18 19 20 ? [0] [1] [2] [3] 18 19 ? ? y=x; x. insert(20); y 4 2 964 ? [0] ? ? [1] [2] [3] [4] memory de-allocated Answer: overloading the assignment operator = Xiaoyan Li, 2007 17
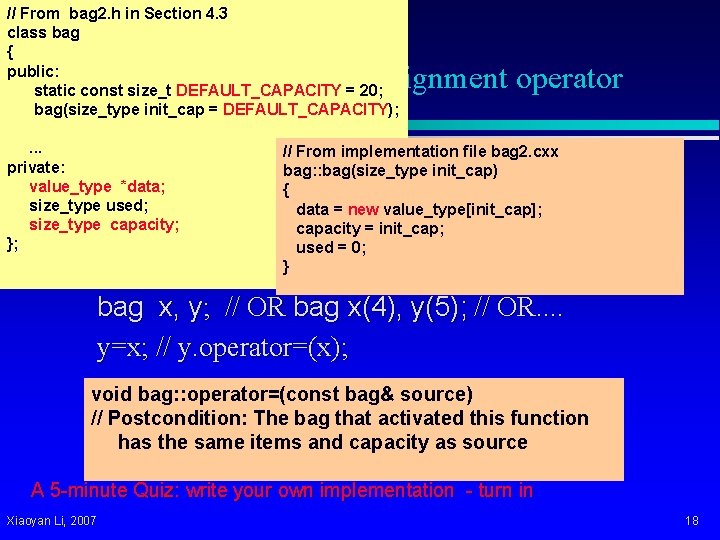
// From bag 2. h in Section 4. 3 class bag { public: static const size_t DEFAULT_CAPACITY = 20; bag(size_type init_cap = DEFAULT_CAPACITY); Solution: overloading assignment operator . . . private: p value_type *data; size_type used; size_type p capacity; }; // From implementation file bag 2. cxx bag: : bag(size_type init_cap) { data = new value_type[init_cap]; capacity = init_cap; used = 0; } Your own assignment operator C++ Requires the overloaded assignment operator to be a member function bag x, y; // OR bag x(4), y(5); // OR. . y=x; // y. operator=(x); void bag: : operator=(const bag& source) // Postcondition: The bag that activated this function has the same items and capacity as source A 5 -minute Quiz: write your own implementation - turn in Xiaoyan Li, 2007 18
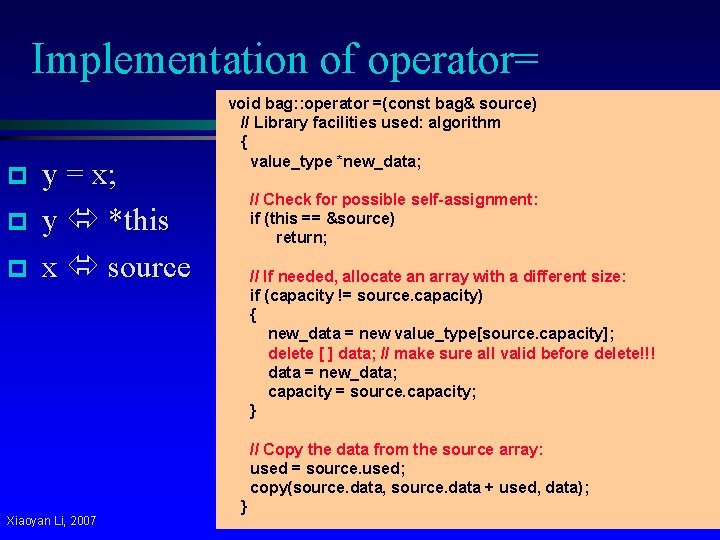
Implementation of operator= p p p y = x; y *this x source void bag: : operator =(const bag& source) // Library facilities used: algorithm { value_type *new_data; // Check for possible self-assignment: if (this == &source) return; // If needed, allocate an array with a different size: if (capacity != source. capacity) { new_data = new value_type[source. capacity]; delete [ ] data; // make sure all valid before delete!!! data = new_data; capacity = source. capacity; } // Copy the data from the source array: used = source. used; copy(source. data, source. data + used, data); Xiaoyan Li, 2007 } 19
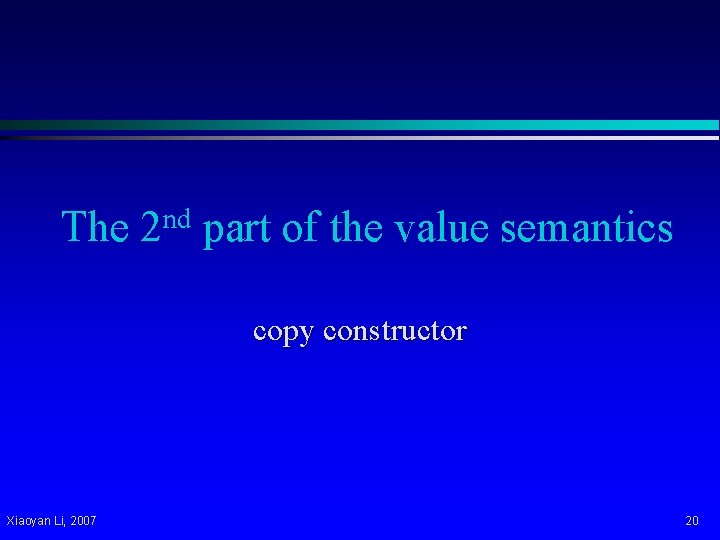
The nd 2 part of the value semantics copy constructor Xiaoyan Li, 2007 20
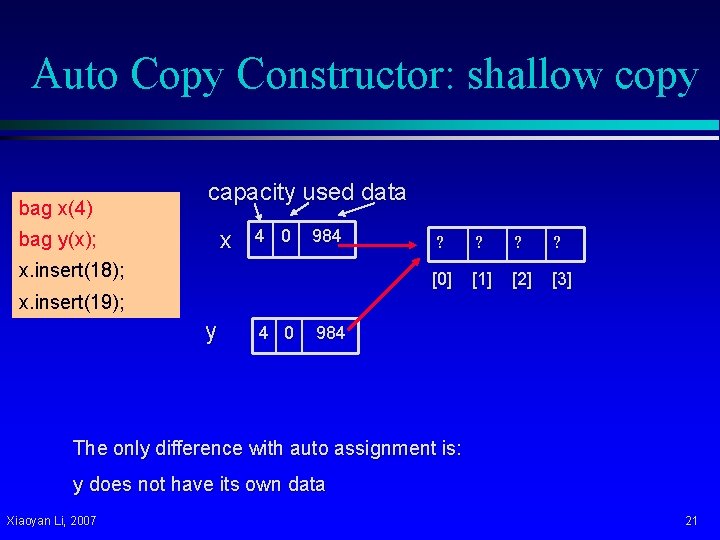
Auto Copy Constructor: shallow copy bag x(4) capacity used data x bag y(x); 4 0 984 x. insert(18); ? ? [0] [1] [2] [3] x. insert(19); y 4 0 984 The only difference with auto assignment is: y does not have its own data Xiaoyan Li, 2007 21
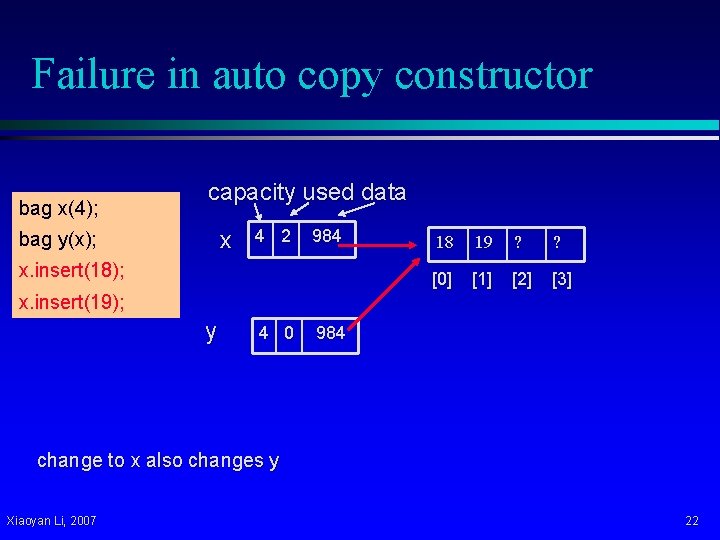
Failure in auto copy constructor bag x(4); capacity used data x bag y(x); 4 2 984 x. insert(18); 18 19 ? ? [0] [1] [2] [3] x. insert(19); y 4 0 984 change to x also changes y Xiaoyan Li, 2007 22
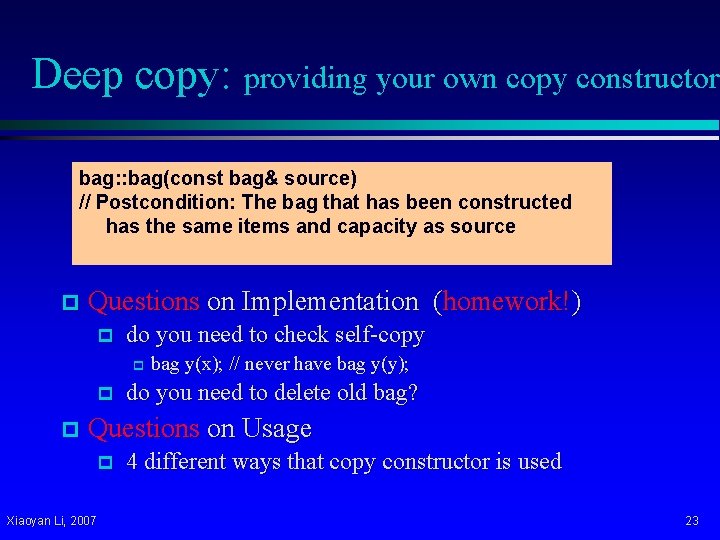
Deep copy: providing your own copy constructor bag: : bag(const bag& source) // Postcondition: The bag that has been constructed has the same items and capacity as source p Questions on Implementation (homework!) p do you need to check self-copy p p p bag y(x); // never have bag y(y); do you need to delete old bag? Questions on Usage p Xiaoyan Li, 2007 4 different ways that copy constructor is used 23
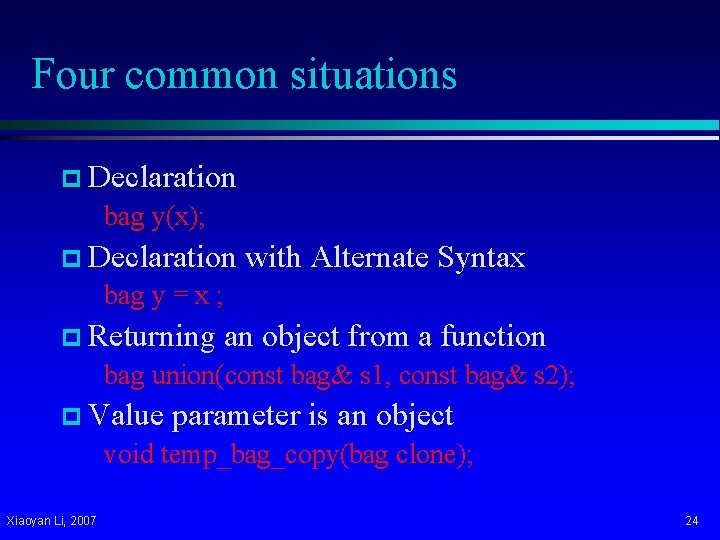
Four common situations p Declaration bag y(x); p Declaration with Alternate Syntax bag y = x ; p Returning an object from a function bag union(const bag& s 1, const bag& s 2); p Value parameter is an object void temp_bag_copy(bag clone); Xiaoyan Li, 2007 24
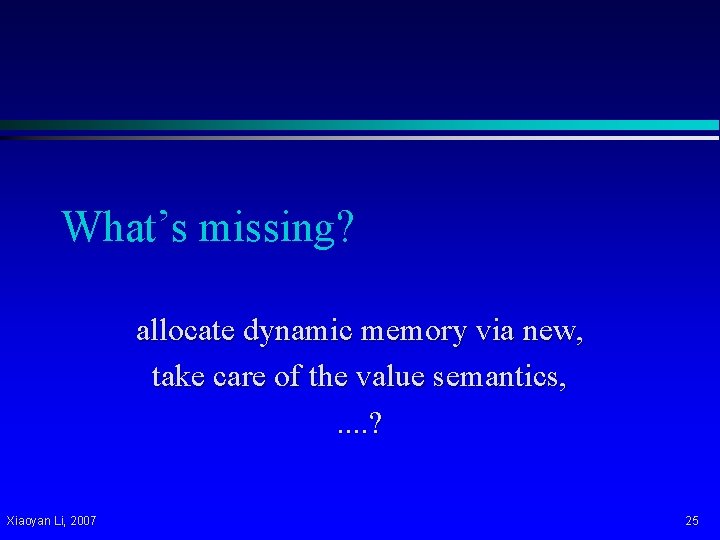
What’s missing? allocate dynamic memory via new, take care of the value semantics, . . ? Xiaoyan Li, 2007 25
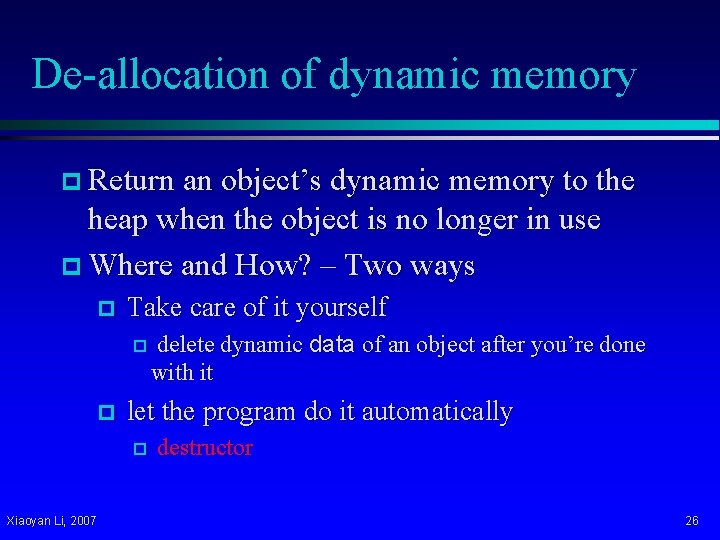
De-allocation of dynamic memory p Return an object’s dynamic memory to the heap when the object is no longer in use p Where and How? – Two ways p Take care of it yourself p p let the program do it automatically p Xiaoyan Li, 2007 delete dynamic data of an object after you’re done with it destructor 26
![Destructor bag bag delete data The primary purpose is Destructor bag: : ~bag() { delete [ ] data; } The primary purpose is](https://slidetodoc.com/presentation_image_h2/ff67daee56054198f2ef6555a53c861e/image-27.jpg)
Destructor bag: : ~bag() { delete [ ] data; } The primary purpose is to return an object’s dynamic memory to the heap, and to do other “cleanup” p Three unique features of the destructor p The name of the destructor is always ~ followed by the class name; p No parameters, no return values; p Activated automatically whenever an object becomes inaccessible p Question: when this happens? Xiaoyan Li, 2007 27
![Destructor p bag bag delete data Some common situations Destructor p bag: : ~bag() { delete [ ] data; } Some common situations](https://slidetodoc.com/presentation_image_h2/ff67daee56054198f2ef6555a53c861e/image-28.jpg)
Destructor p bag: : ~bag() { delete [ ] data; } Some common situations causing automatic destructor activation Upon function return, objects as local variables destroyed; p Upon function return, objects as value parameters destroyed; p when an object is explicitly deleted p Question: shall we put destructor in how-to-use-abag documentation? Xiaoyan Li, 2007 28
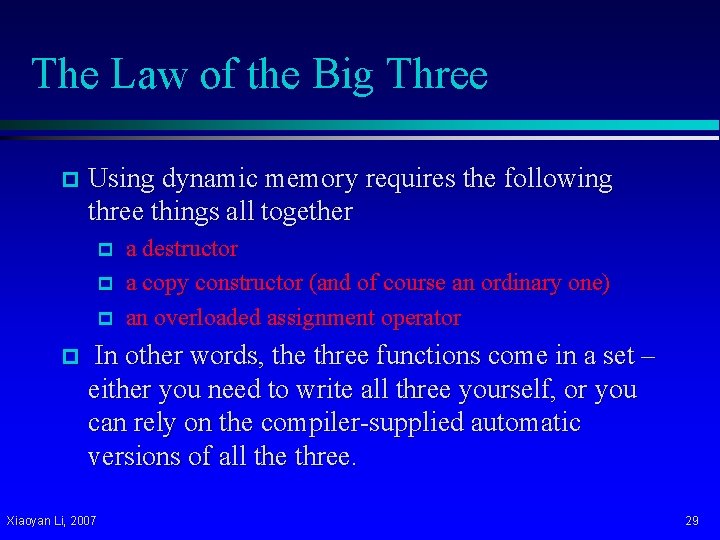
The Law of the Big Three p Using dynamic memory requires the following three things all together p p a destructor a copy constructor (and of course an ordinary one) an overloaded assignment operator In other words, the three functions come in a set – either you need to write all three yourself, or you can rely on the compiler-supplied automatic versions of all the three. Xiaoyan Li, 2007 29
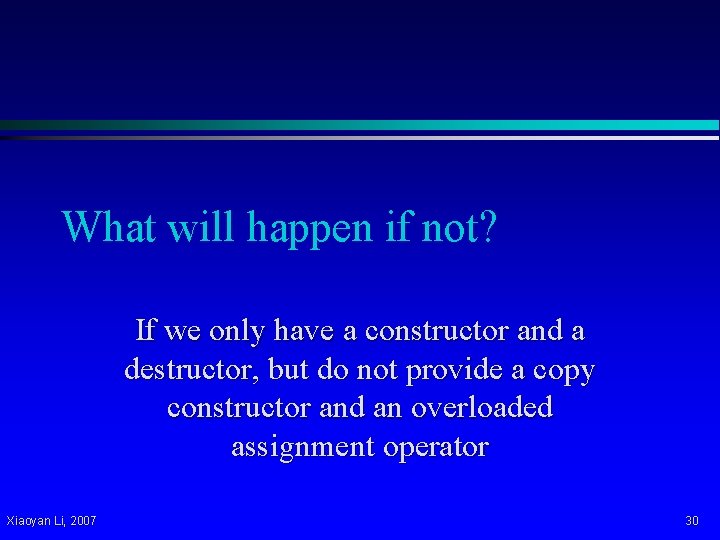
What will happen if not? If we only have a constructor and a destructor, but do not provide a copy constructor and an overloaded assignment operator Xiaoyan Li, 2007 30
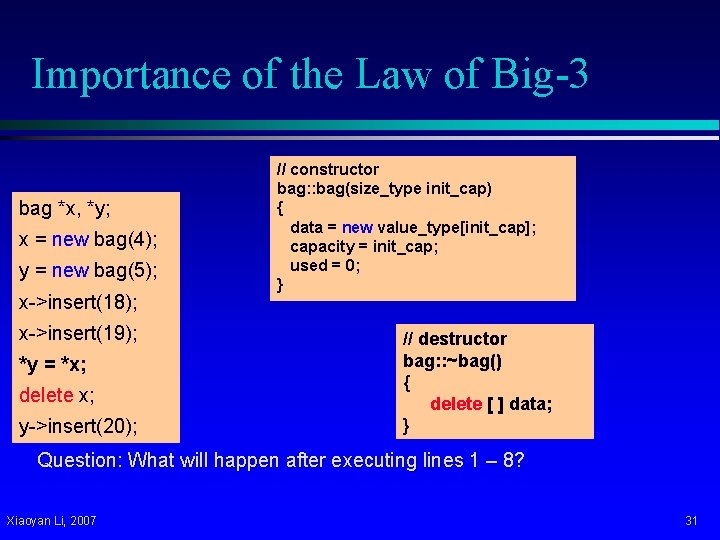
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); y = new bag(5); x->insert(18); x->insert(19); *y = *x; delete x; y->insert(20); // constructor bag: : bag(size_type init_cap) { data = new value_type[init_cap]; capacity = init_cap; used = 0; } // destructor bag: : ~bag() { delete [ ] data; } Question: What will happen after executing lines 1 – 8? Xiaoyan Li, 2007 31
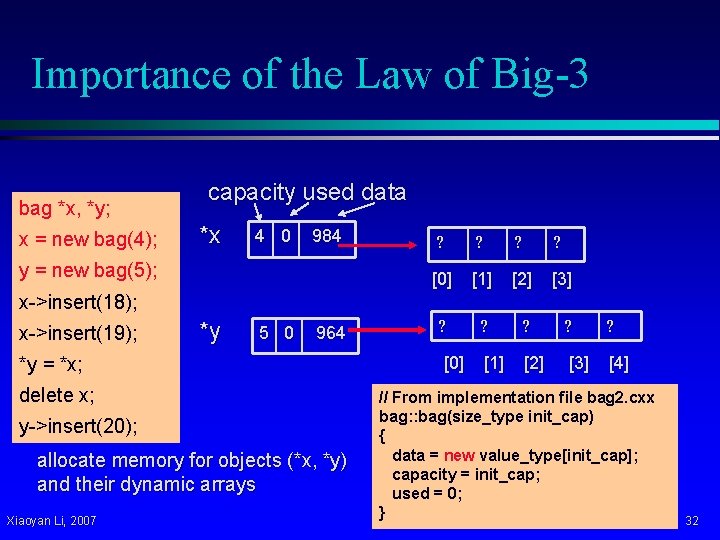
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); capacity used data *x 4 0 984 y = new bag(5); ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] x->insert(18); x->insert(19); *y 5 0 964 *y = *x; delete x; y->insert(20); allocate memory for objects (*x, *y) and their dynamic arrays Xiaoyan Li, 2007 [0] // From implementation file bag 2. cxx bag: : bag(size_type init_cap) { data = new value_type[init_cap]; capacity = init_cap; used = 0; } 32
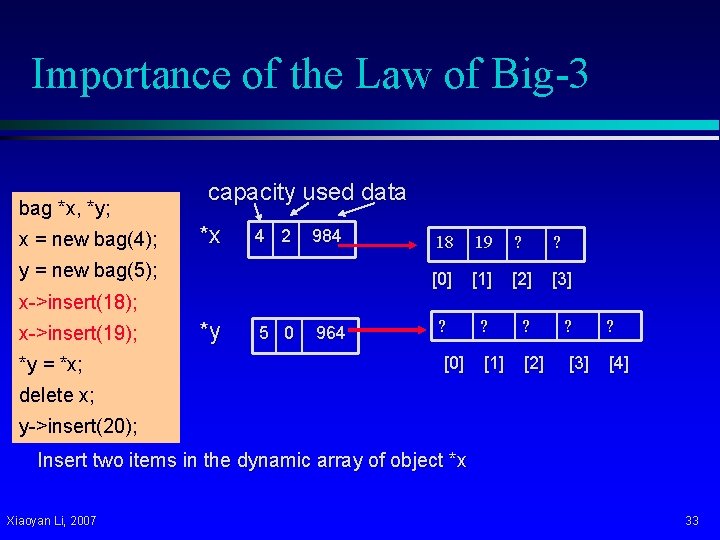
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); capacity used data *x 4 2 984 y = new bag(5); 18 19 ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] x->insert(18); x->insert(19); *y = *x; *y 5 0 964 [0] delete x; y->insert(20); Insert two items in the dynamic array of object *x Xiaoyan Li, 2007 33
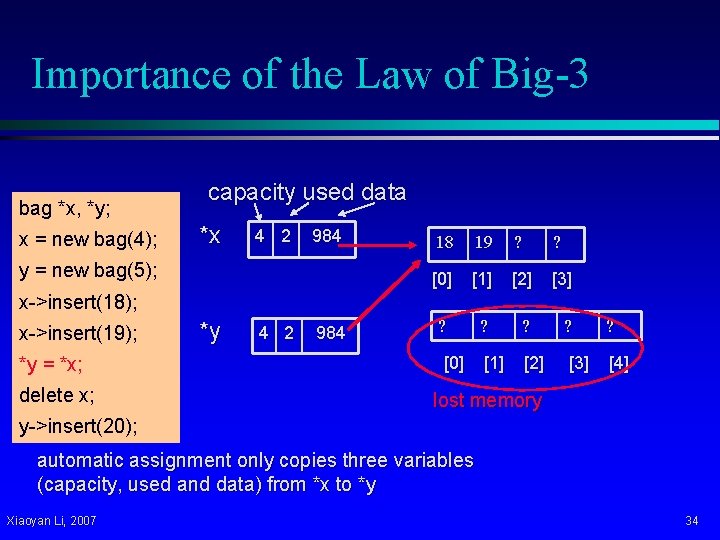
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); capacity used data *x 4 2 984 y = new bag(5); 18 19 ? ? [0] [1] [2] [3] ? ? ? [1] [2] [3] [4] x->insert(18); x->insert(19); *y = *x; delete x; *y 4 2 984 [0] lost memory y->insert(20); automatic assignment only copies three variables (capacity, used and data) from *x to *y Xiaoyan Li, 2007 34
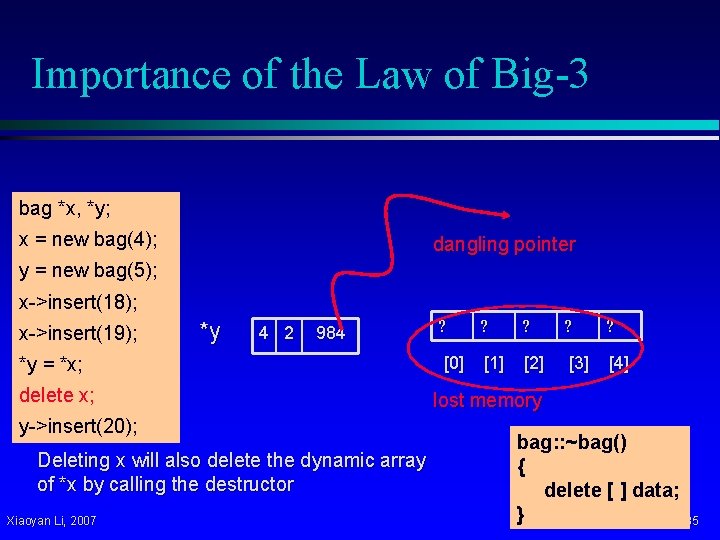
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); dangling pointer y = new bag(5); x->insert(18); x->insert(19); *y 4 2 984 *y = *x; delete x; y->insert(20); Deleting x will also delete the dynamic array of *x by calling the destructor Xiaoyan Li, 2007 ? [0] ? ? [1] [2] [3] [4] lost memory bag: : ~bag() { delete [ ] data; } 35
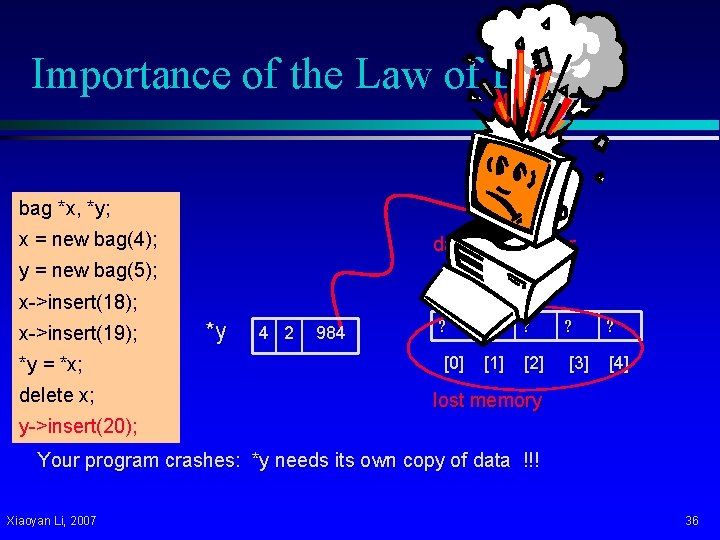
Importance of the Law of Big-3 bag *x, *y; x = new bag(4); dangling pointer y = new bag(5); x->insert(18); x->insert(19); *y = *x; delete x; *y 4 2 984 ? [0] ? ? [1] [2] [3] [4] lost memory y->insert(20); Your program crashes: *y needs its own copy of data !!! Xiaoyan Li, 2007 36
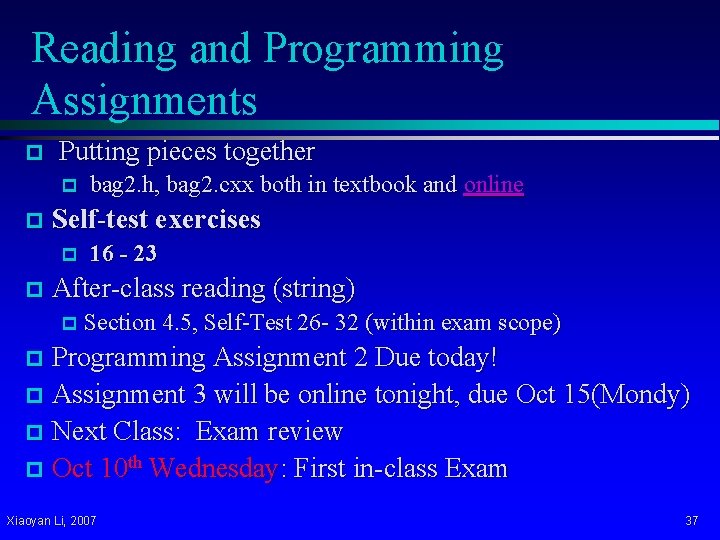
Reading and Programming Assignments p Putting pieces together p p Self-test exercises p p bag 2. h, bag 2. cxx both in textbook and online 16 - 23 After-class reading (string) p Section 4. 5, Self-Test 26 - 32 (within exam scope) Programming Assignment 2 Due today! p Assignment 3 will be online tonight, due Oct 15(Mondy) p Next Class: Exam review p Oct 10 th Wednesday: First in-class Exam p Xiaoyan Li, 2007 37
Dynamic data structure in java
Dynamic equivalence problem
Dynamic data structures
Qualquer classe e subclasse
Pre ap classes vs regular classes
01:640:244 lecture notes - lecture 15: plat, idah, farad
Structures vs classes
Dp class 2
Examples of homologous
Dynamic of structures
Dynamic of structures
Interval data structure
What is static and dynamic data structure
Transferered
Csc data entry
Opwekking 211
Miller indices 210
Snohomish county coordinated entry
Poli 211
Physics 211 exam 1
Legea 211/2011
Luyana211
Mgt 211
Is 211 nationwide
Csce 211
211 la taxonomy
Trigo buck sy 211 fecha de siembra
Csce 211
Comp 211
Comp 211
Comp 211
211 org md
매트랩 subplot
Nur 211 final exam
Supportability analysis
Log 211
Jus 211
211 orange county