COP 3530 Computer Science III Summer 2005 Graphs
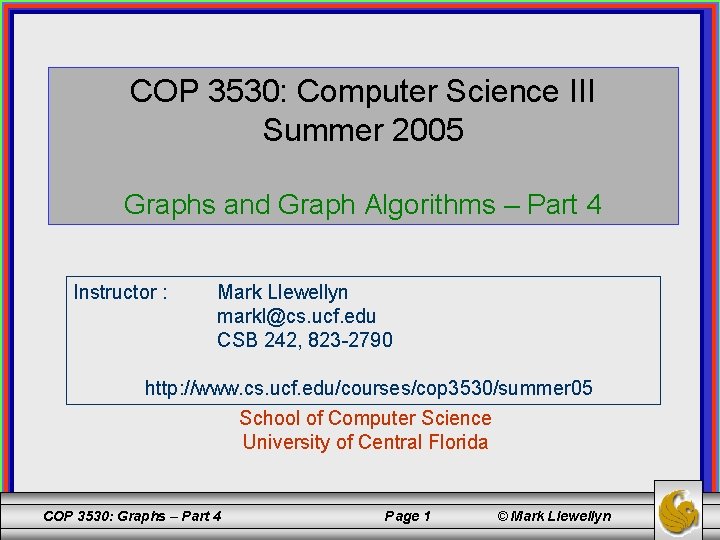
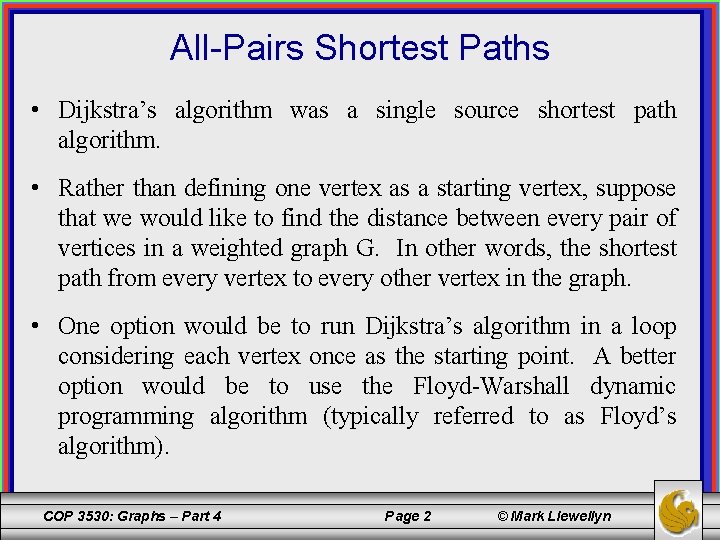
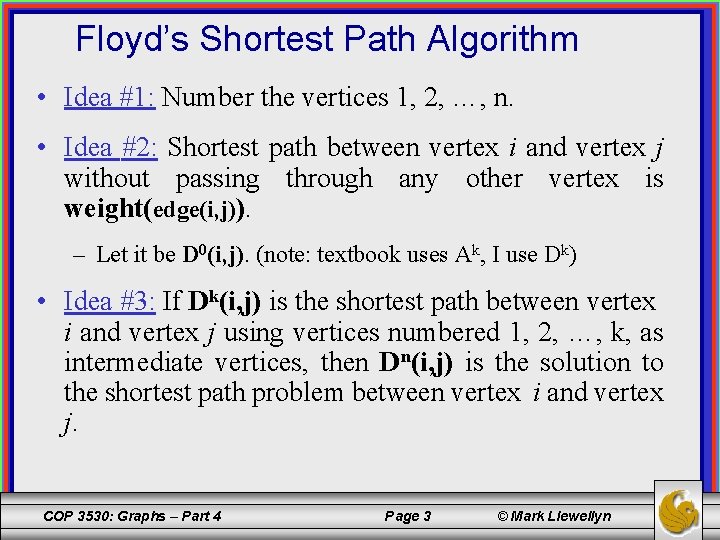
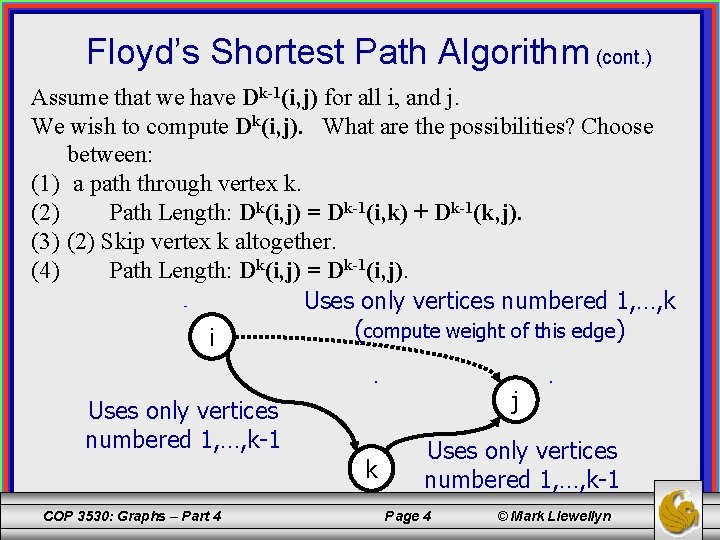
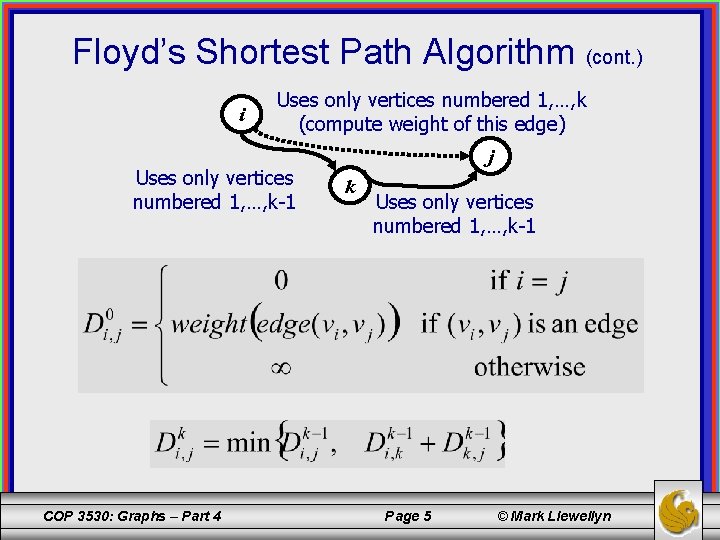
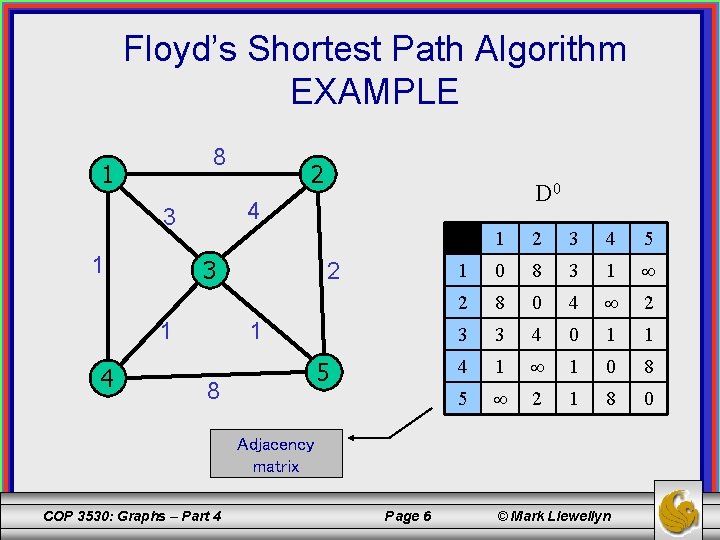
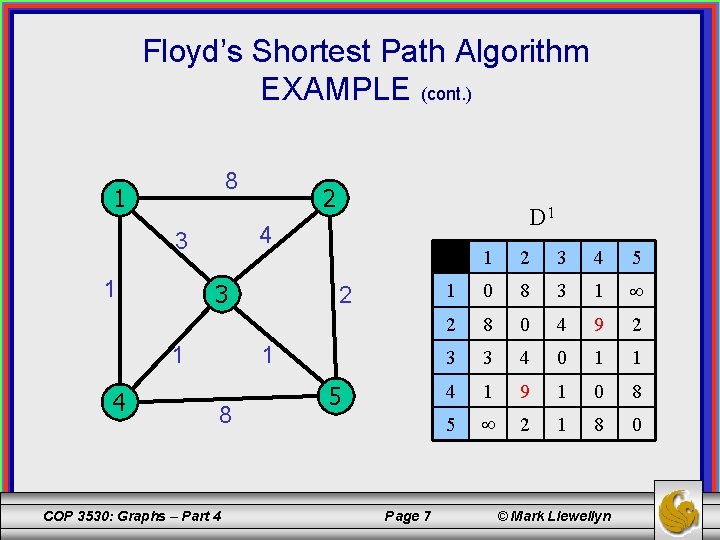
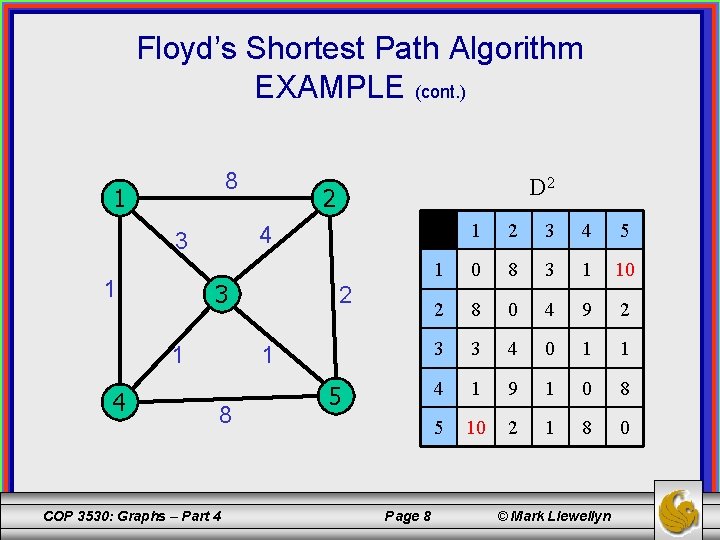
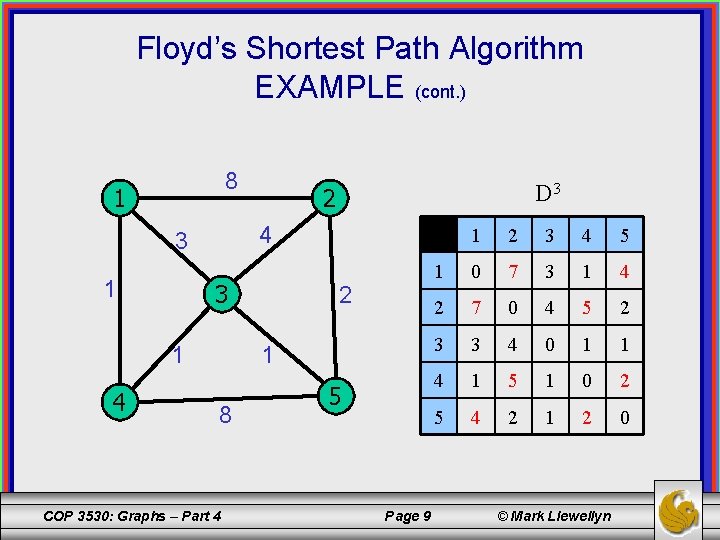
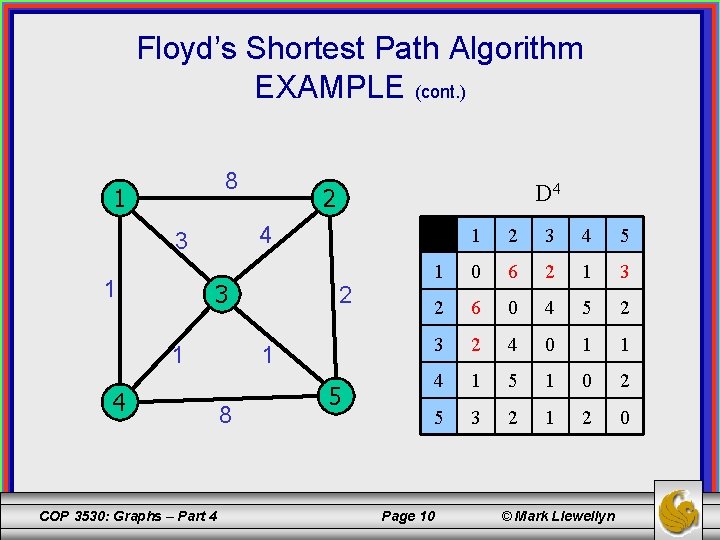
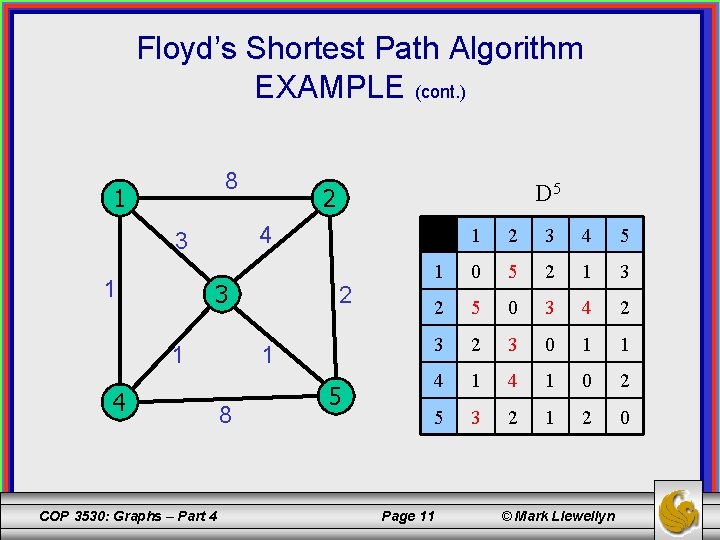
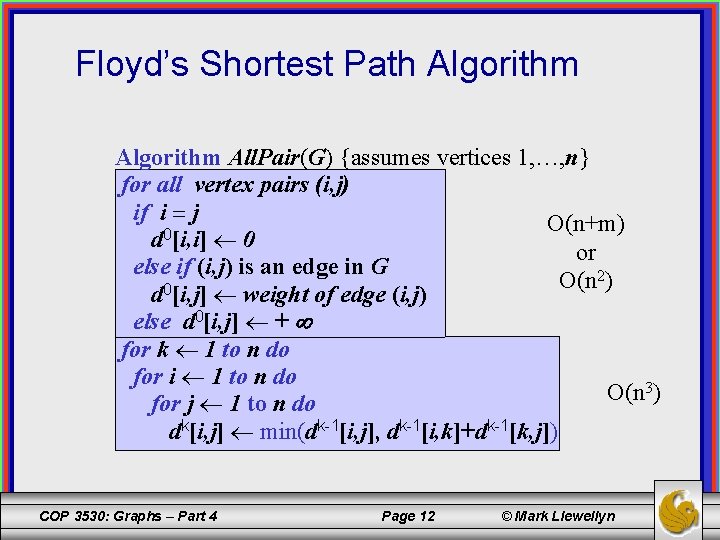
![Floyd’s Shortest Path Algorithm Observation: d k [i, k] = d k-1 [i, k] Floyd’s Shortest Path Algorithm Observation: d k [i, k] = d k-1 [i, k]](https://slidetodoc.com/presentation_image_h2/150633ab07edfaa617183f0dc4b11444/image-13.jpg)
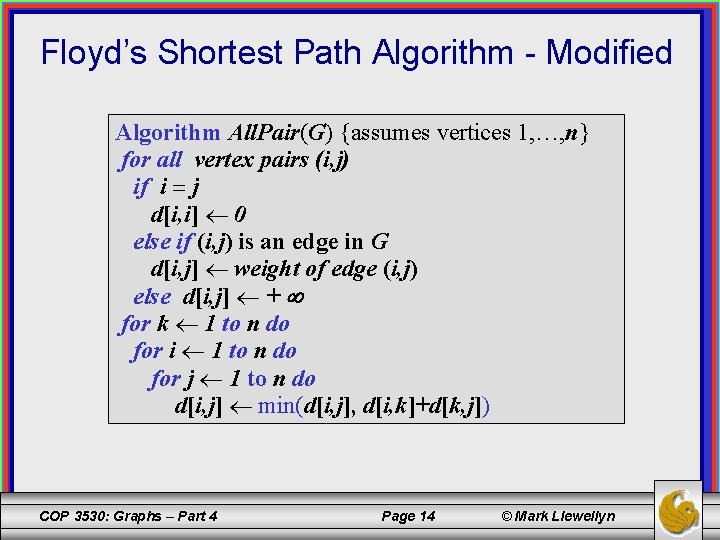
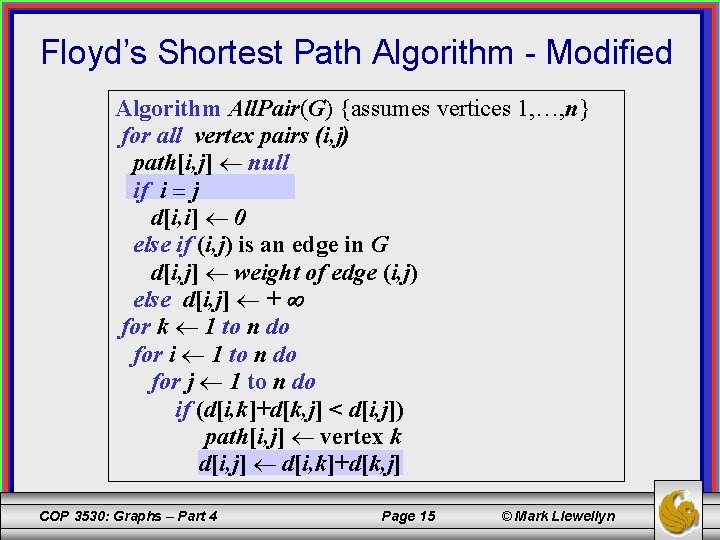
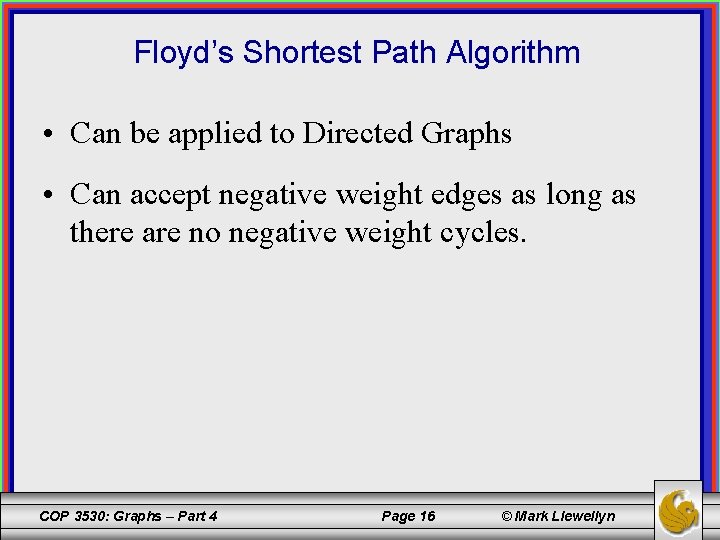
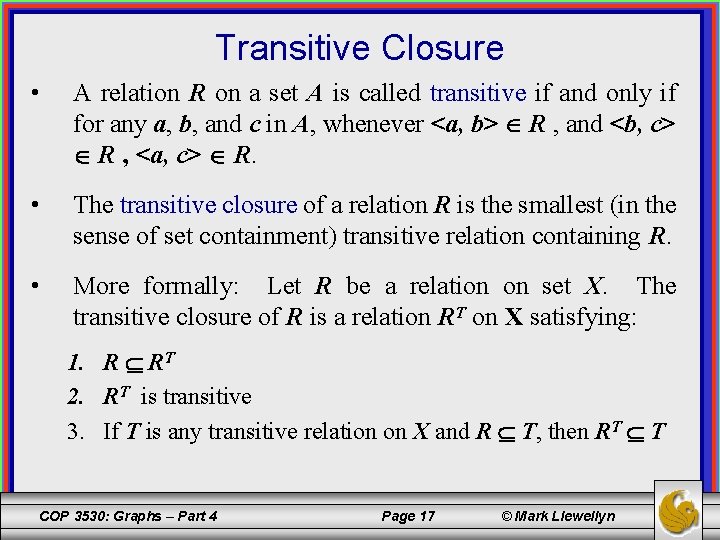
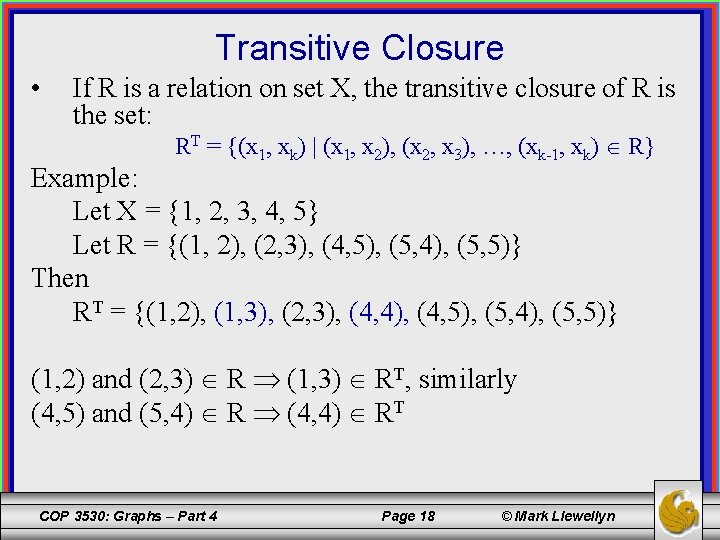
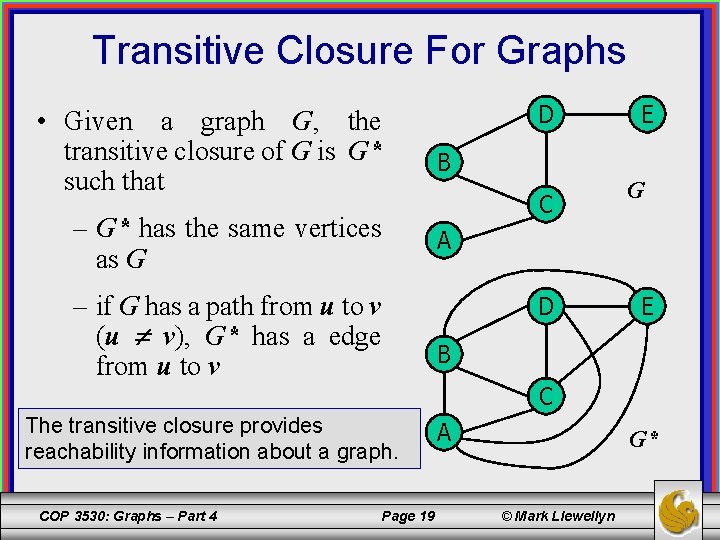
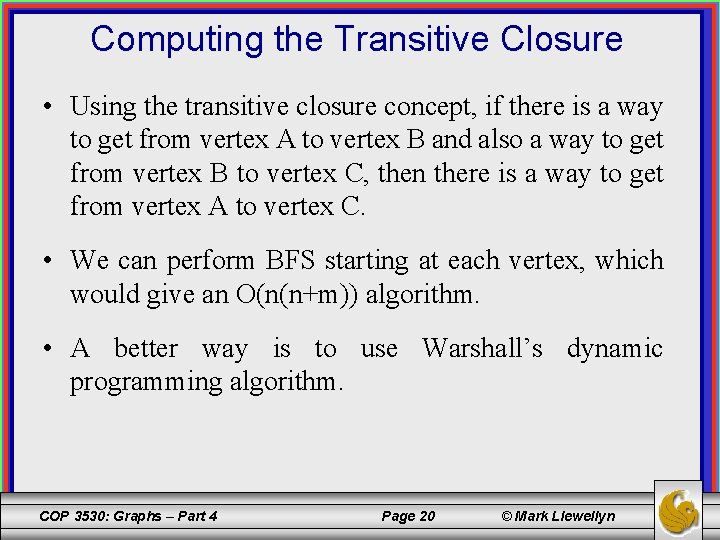
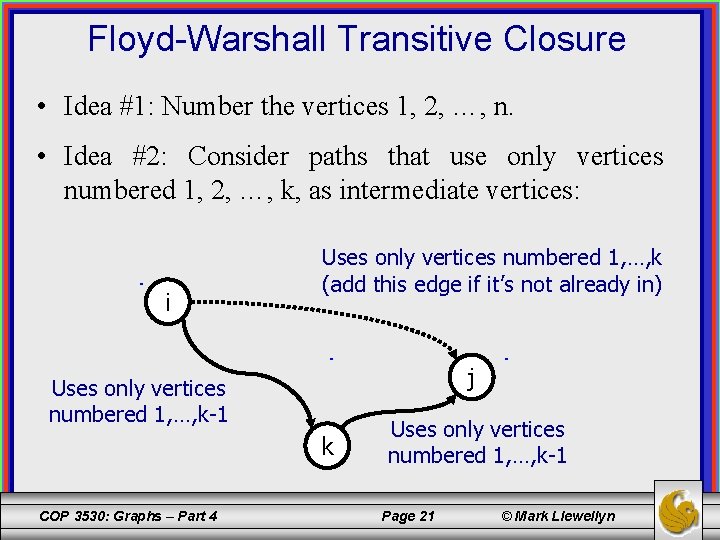
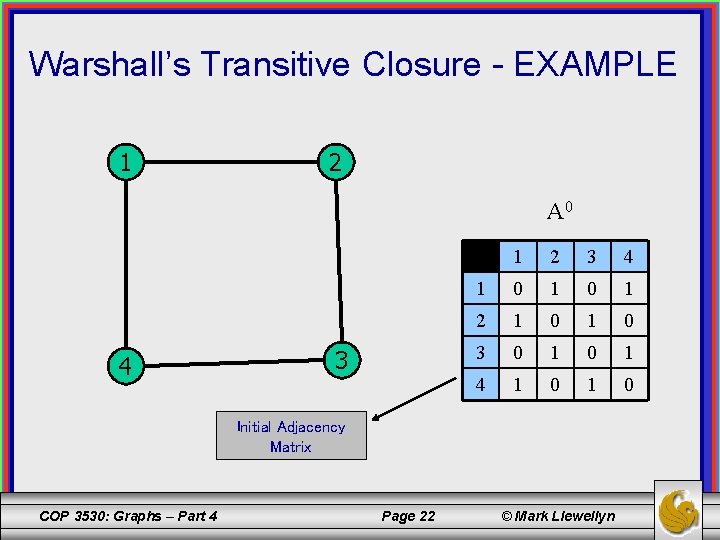
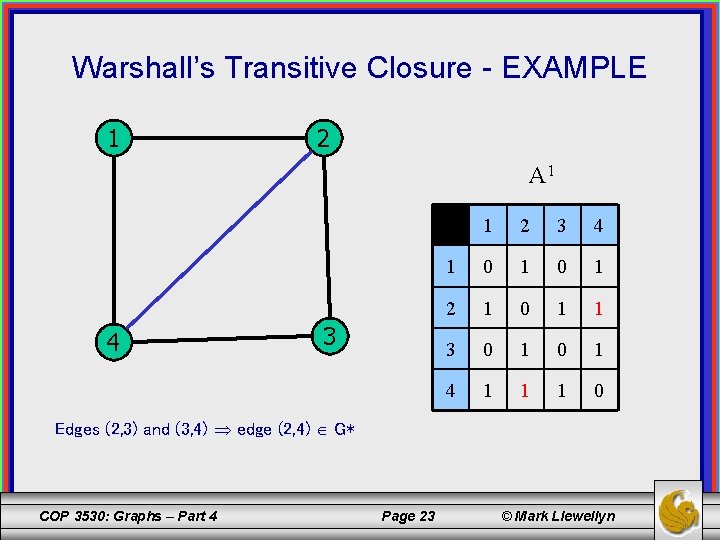
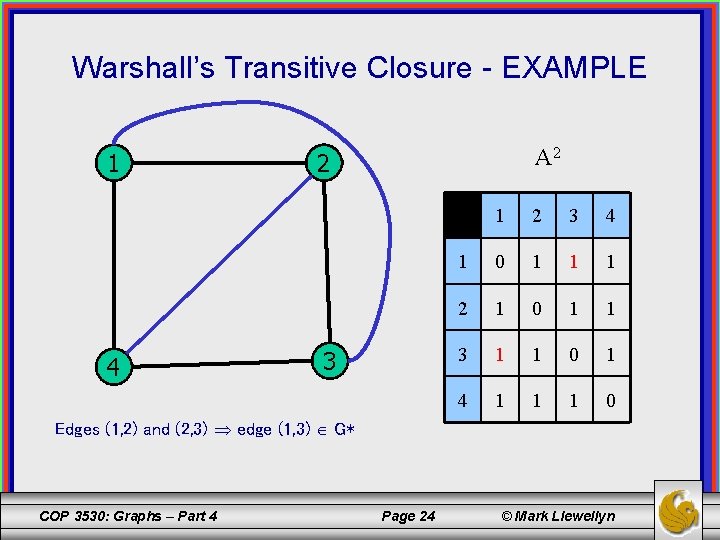
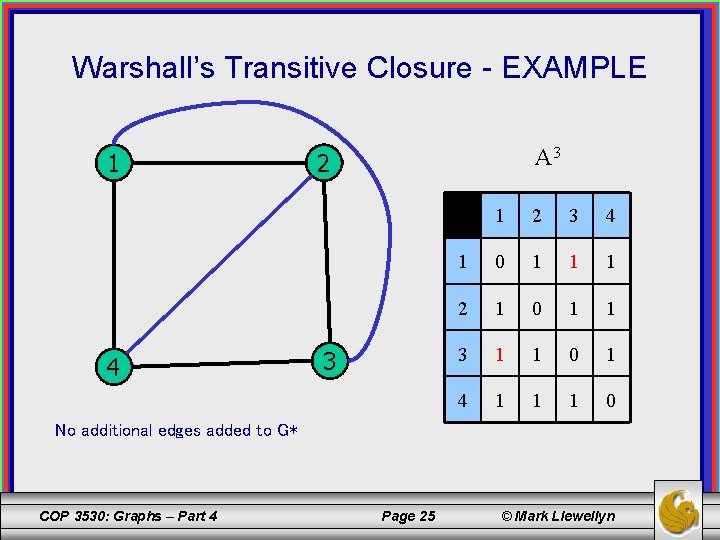
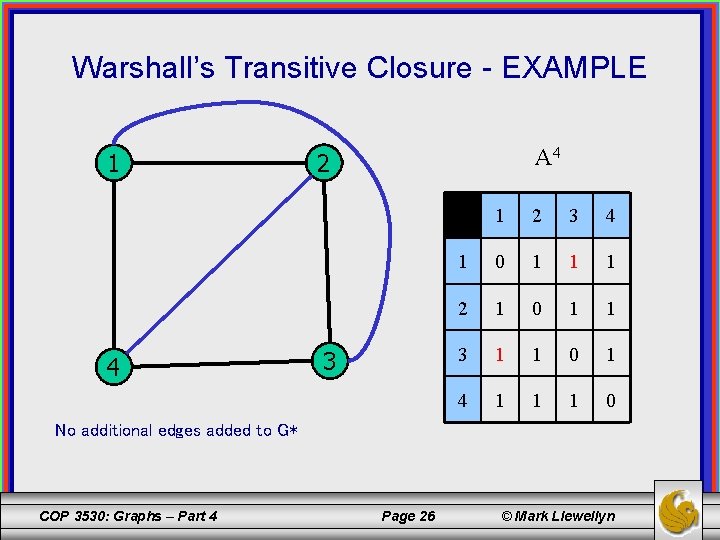
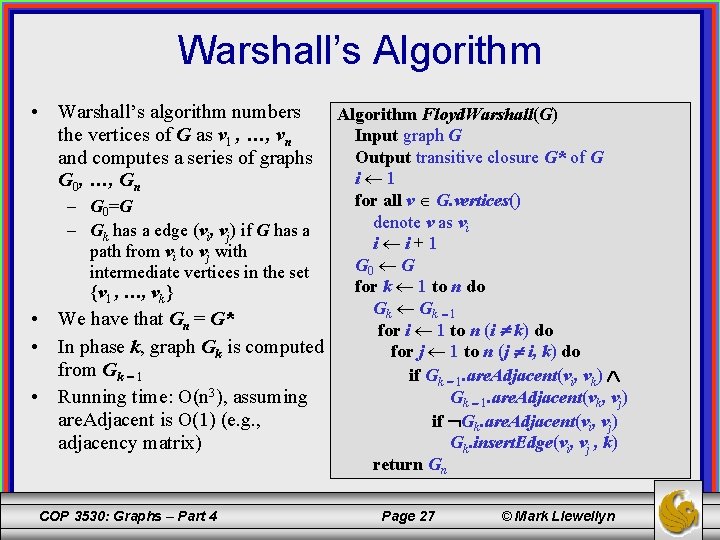
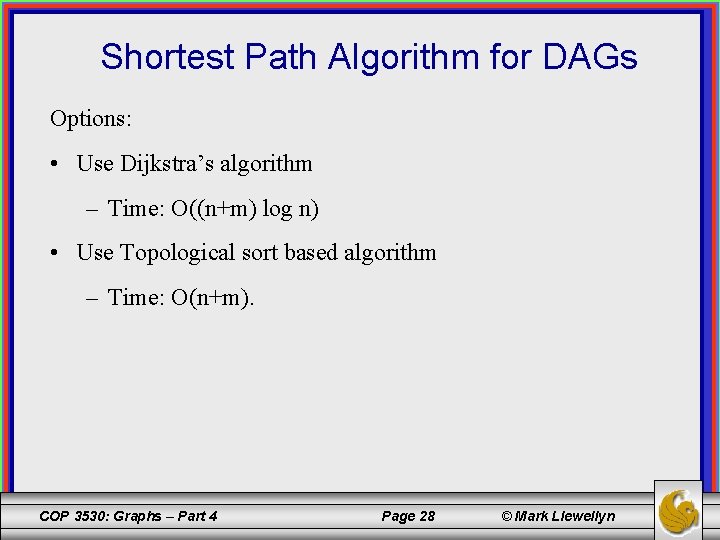
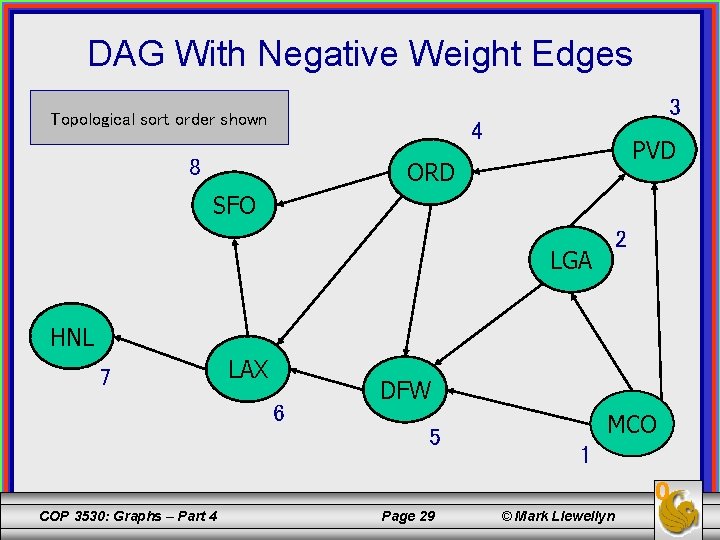
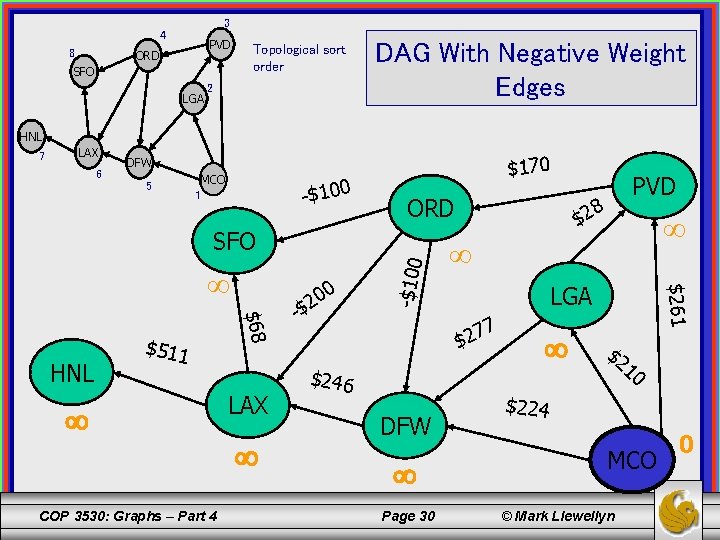
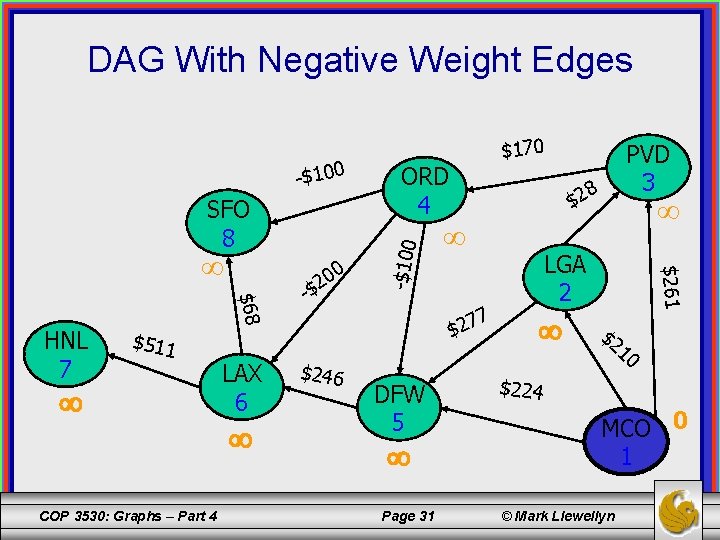
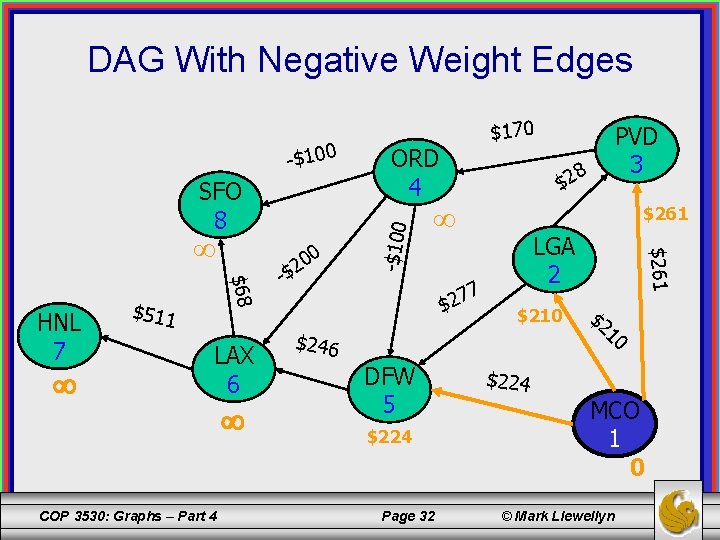
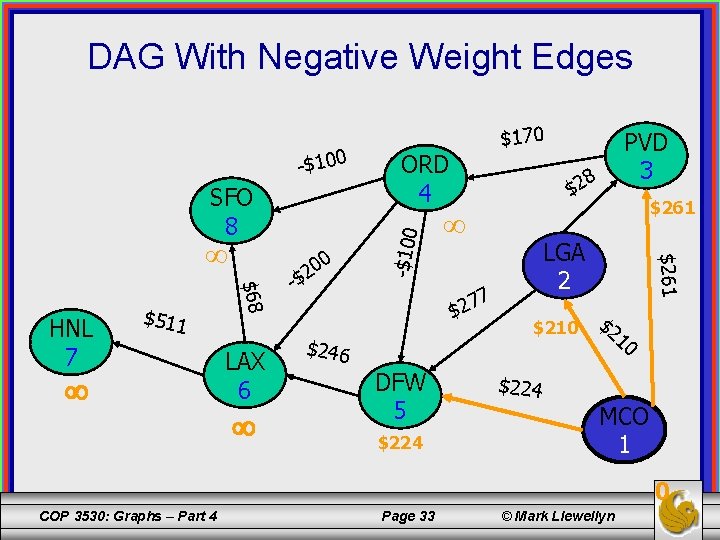
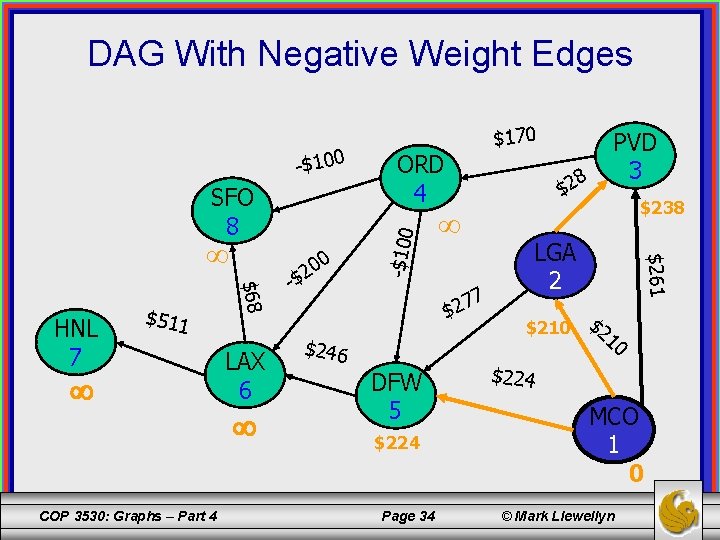
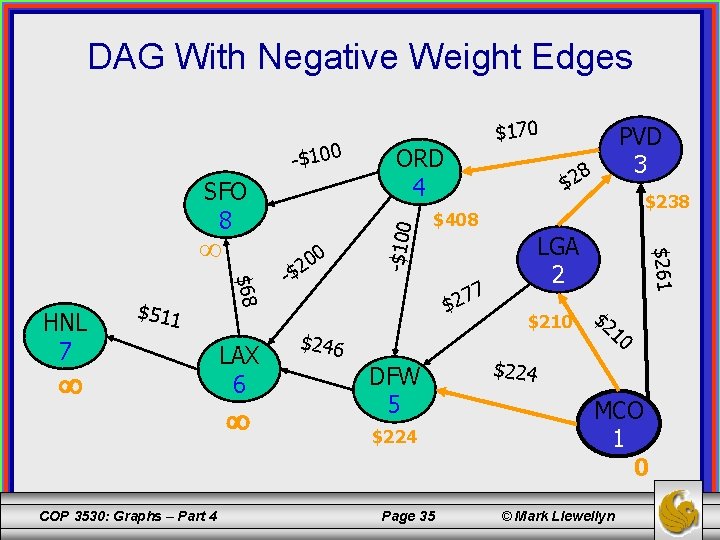
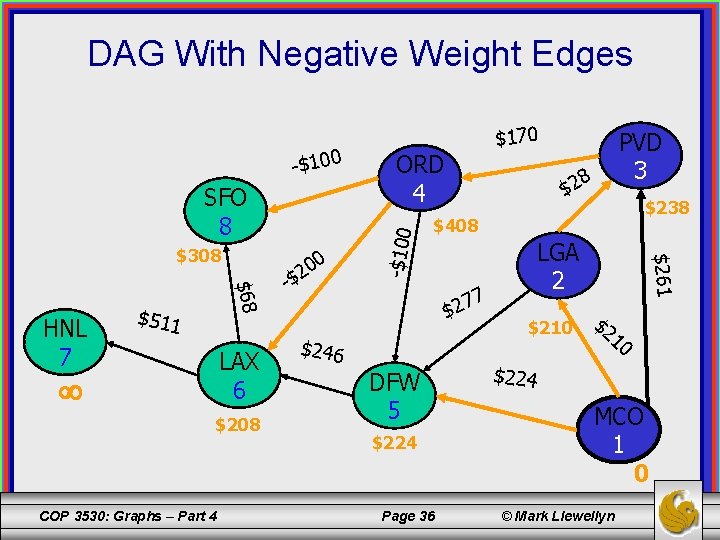
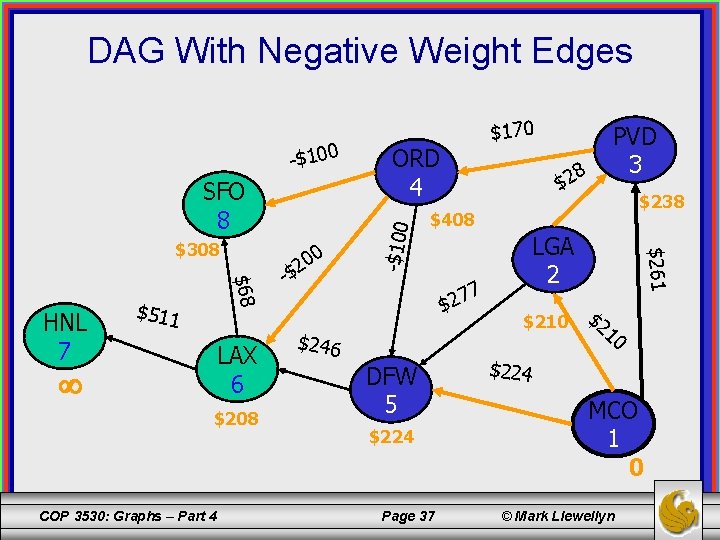
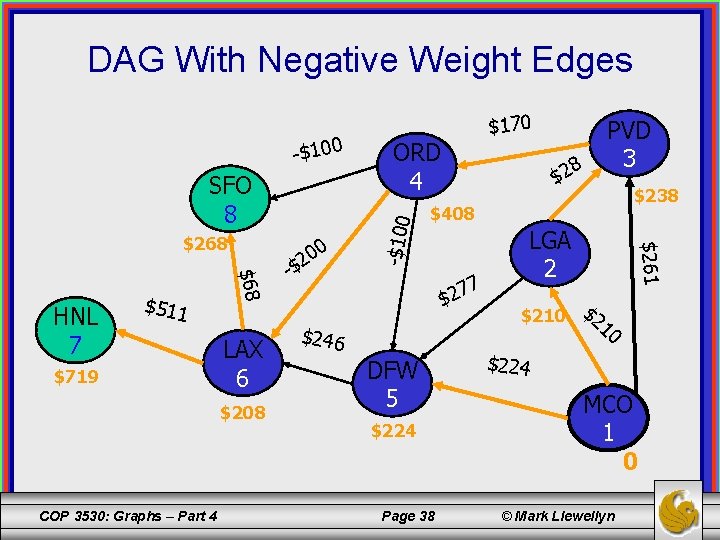
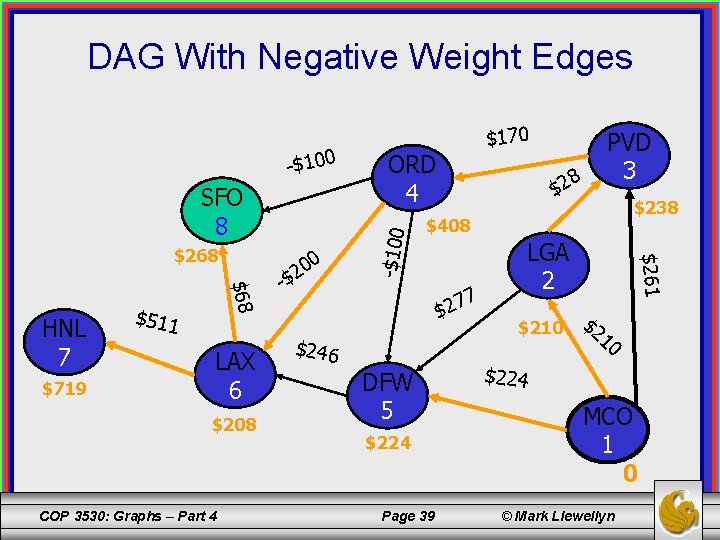
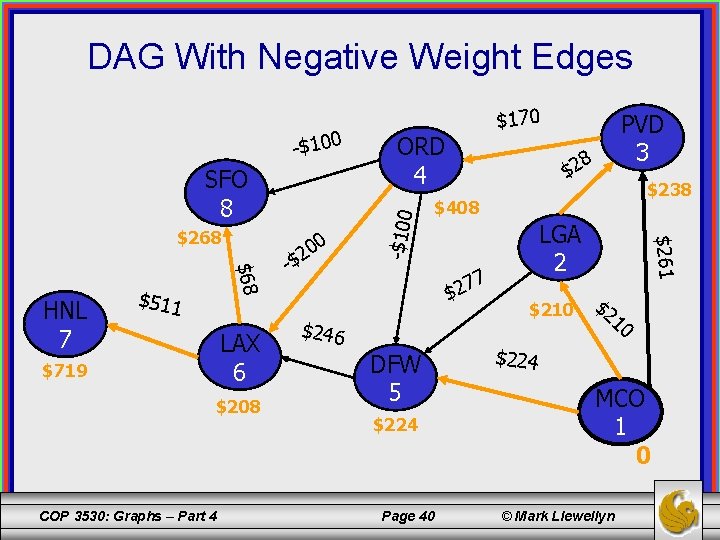
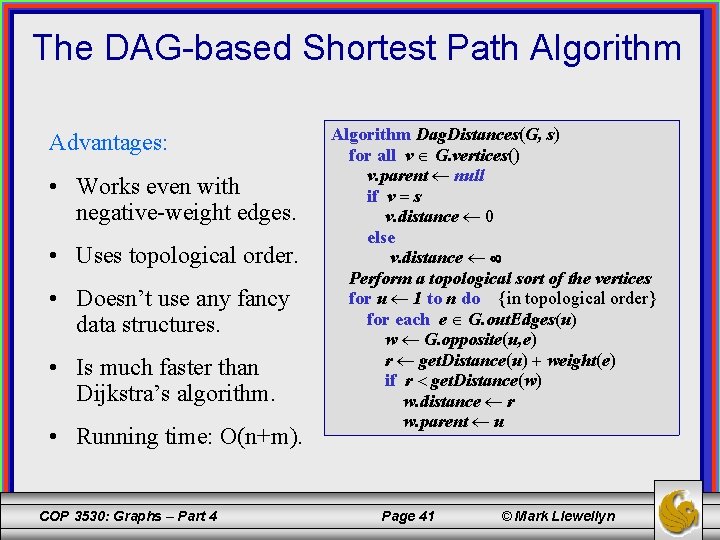
- Slides: 41
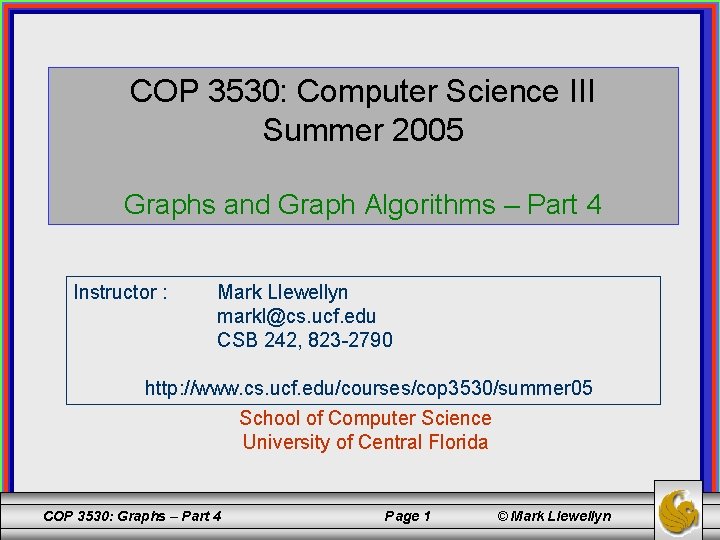
COP 3530: Computer Science III Summer 2005 Graphs and Graph Algorithms – Part 4 Instructor : Mark Llewellyn markl@cs. ucf. edu CSB 242, 823 -2790 http: //www. cs. ucf. edu/courses/cop 3530/summer 05 School of Computer Science University of Central Florida COP 3530: Graphs – Part 4 Page 1 © Mark Llewellyn
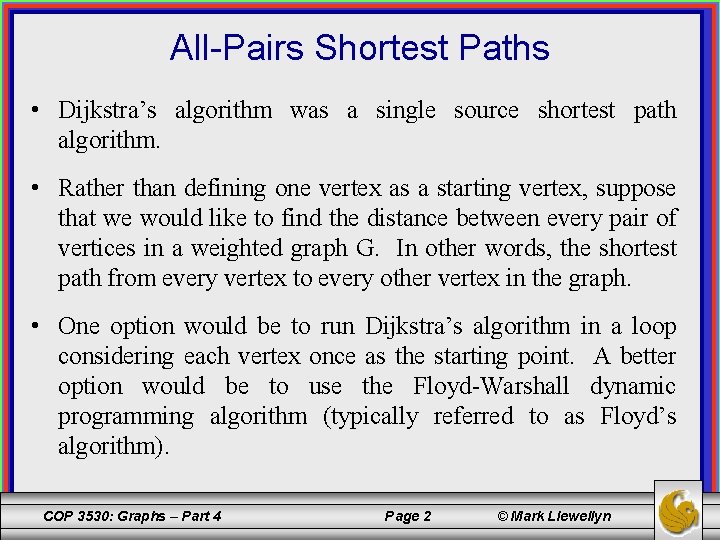
All-Pairs Shortest Paths • Dijkstra’s algorithm was a single source shortest path algorithm. • Rather than defining one vertex as a starting vertex, suppose that we would like to find the distance between every pair of vertices in a weighted graph G. In other words, the shortest path from every vertex to every other vertex in the graph. • One option would be to run Dijkstra’s algorithm in a loop considering each vertex once as the starting point. A better option would be to use the Floyd-Warshall dynamic programming algorithm (typically referred to as Floyd’s algorithm). COP 3530: Graphs – Part 4 Page 2 © Mark Llewellyn
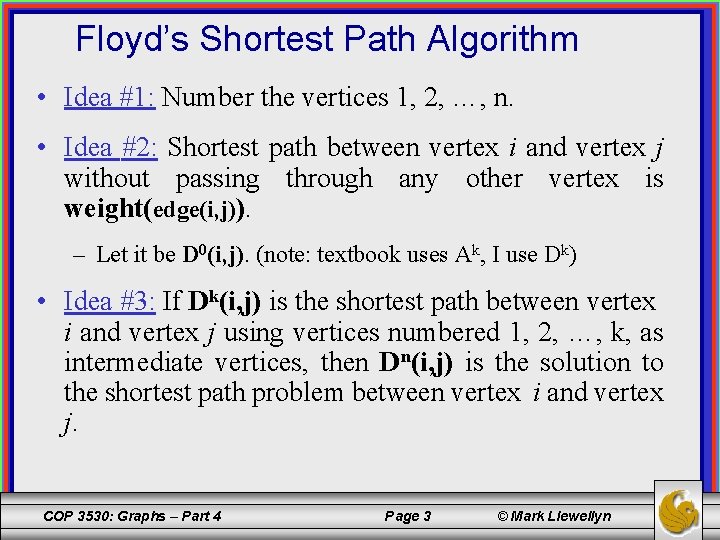
Floyd’s Shortest Path Algorithm • Idea #1: Number the vertices 1, 2, …, n. • Idea #2: Shortest path between vertex i and vertex j without passing through any other vertex is weight(edge(i, j)). – Let it be D 0(i, j). (note: textbook uses Ak, I use Dk) • Idea #3: If Dk(i, j) is the shortest path between vertex i and vertex j using vertices numbered 1, 2, …, k, as intermediate vertices, then Dn(i, j) is the solution to the shortest path problem between vertex i and vertex j. COP 3530: Graphs – Part 4 Page 3 © Mark Llewellyn
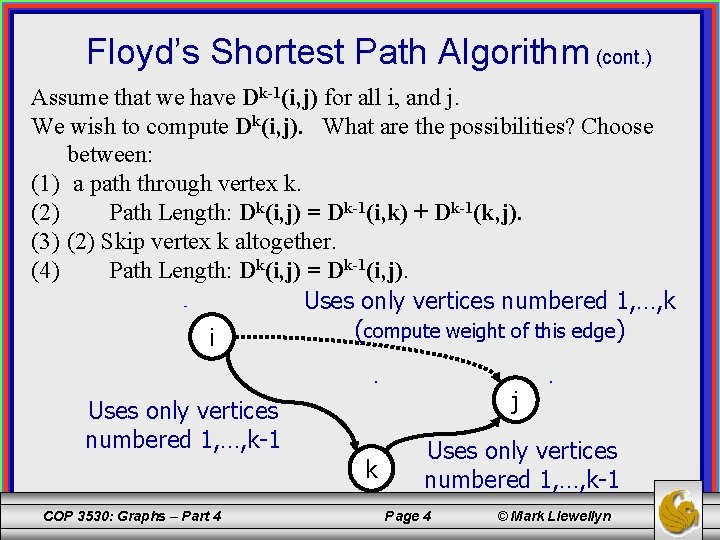
Floyd’s Shortest Path Algorithm (cont. ) Assume that we have Dk-1(i, j) for all i, and j. We wish to compute Dk(i, j). What are the possibilities? Choose between: (1) a path through vertex k. (2) Path Length: Dk(i, j) = Dk-1(i, k) + Dk-1(k, j). (3) (2) Skip vertex k altogether. (4) Path Length: Dk(i, j) = Dk-1(i, j). Uses only vertices numbered 1, …, k (compute weight of this edge) i j Uses only vertices numbered 1, …, k-1 k COP 3530: Graphs – Part 4 Uses only vertices numbered 1, …, k-1 Page 4 © Mark Llewellyn
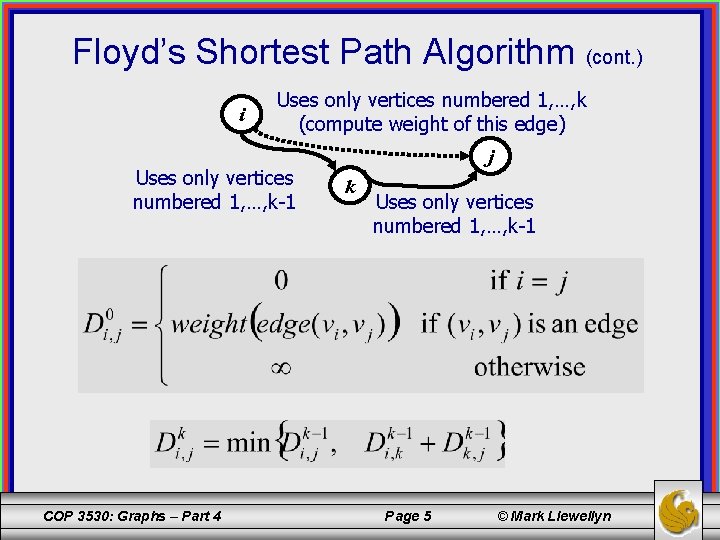
Floyd’s Shortest Path Algorithm (cont. ) i Uses only vertices numbered 1, …, k (compute weight of this edge) Uses only vertices numbered 1, …, k-1 COP 3530: Graphs – Part 4 j k Uses only vertices numbered 1, …, k-1 Page 5 © Mark Llewellyn
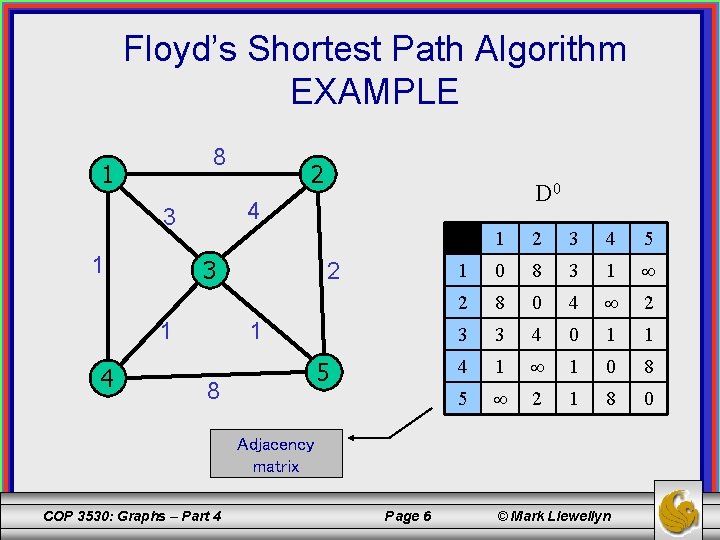
Floyd’s Shortest Path Algorithm EXAMPLE 8 1 3 1 4 D 0 4 3 1 2 2 1 5 8 1 2 3 4 5 1 0 8 3 1 2 8 0 4 2 3 3 4 0 1 1 4 1 1 0 8 5 2 1 8 0 Adjacency matrix COP 3530: Graphs – Part 4 Page 6 © Mark Llewellyn
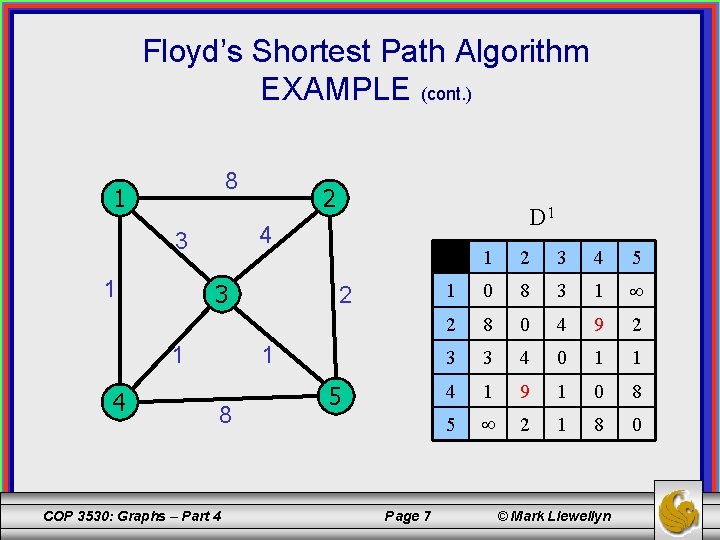
Floyd’s Shortest Path Algorithm EXAMPLE (cont. ) 8 1 3 1 4 D 1 4 3 1 2 2 1 8 COP 3530: Graphs – Part 4 5 Page 7 1 2 3 4 5 1 0 8 3 1 2 8 0 4 9 2 3 3 4 0 1 1 4 1 9 1 0 8 5 2 1 8 0 © Mark Llewellyn
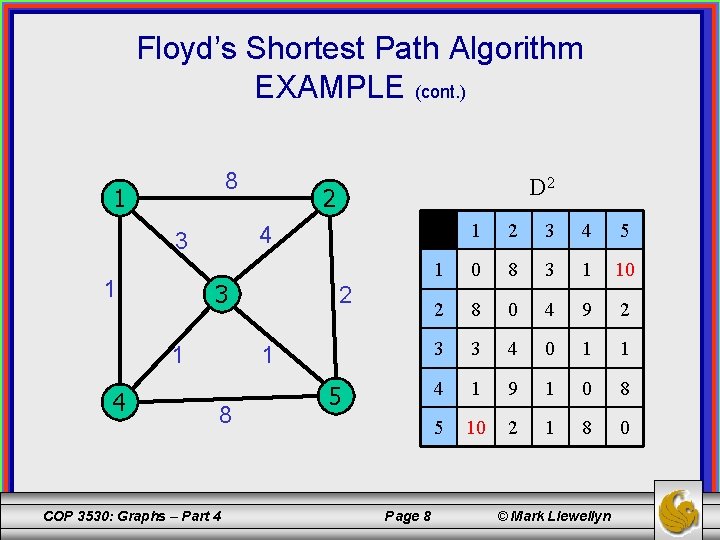
Floyd’s Shortest Path Algorithm EXAMPLE (cont. ) 8 1 3 1 4 2 1 2 3 4 5 1 0 8 3 1 10 2 8 0 4 9 2 3 3 4 0 1 1 4 1 9 1 0 8 5 10 2 1 8 0 4 3 1 D 2 2 1 8 COP 3530: Graphs – Part 4 5 Page 8 © Mark Llewellyn
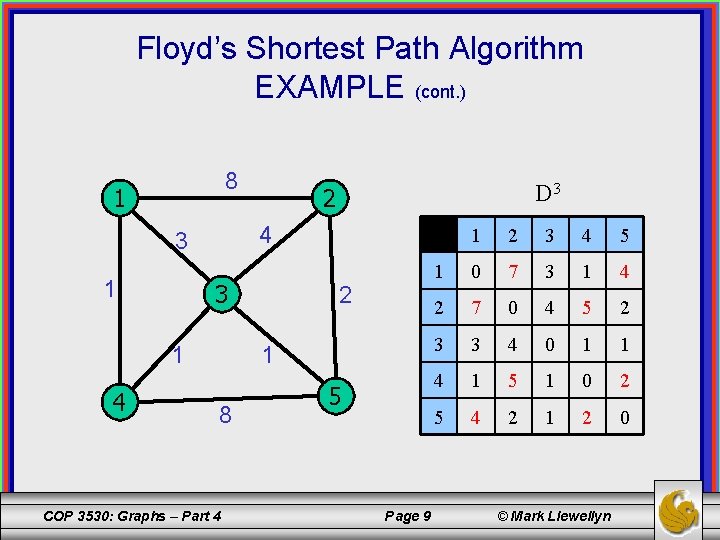
Floyd’s Shortest Path Algorithm EXAMPLE (cont. ) 8 1 4 3 1 4 D 3 2 2 1 8 COP 3530: Graphs – Part 4 5 Page 9 1 2 3 4 5 1 0 7 3 1 4 2 7 0 4 5 2 3 3 4 0 1 1 4 1 5 1 0 2 5 4 2 1 2 0 © Mark Llewellyn
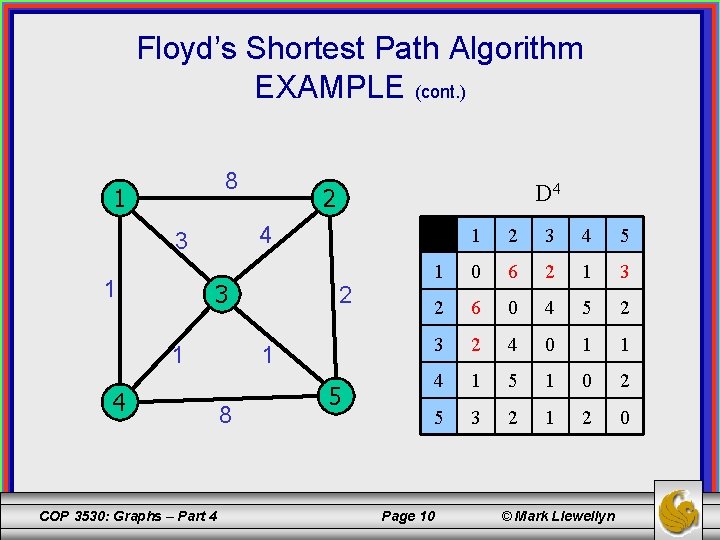
Floyd’s Shortest Path Algorithm EXAMPLE (cont. ) 8 1 4 3 1 D 4 2 3 1 4 COP 3530: Graphs – Part 4 2 1 8 5 1 2 3 4 5 1 0 6 2 1 3 2 6 0 4 5 2 3 2 4 0 1 1 4 1 5 1 0 2 5 3 2 1 2 0 Page 10 © Mark Llewellyn
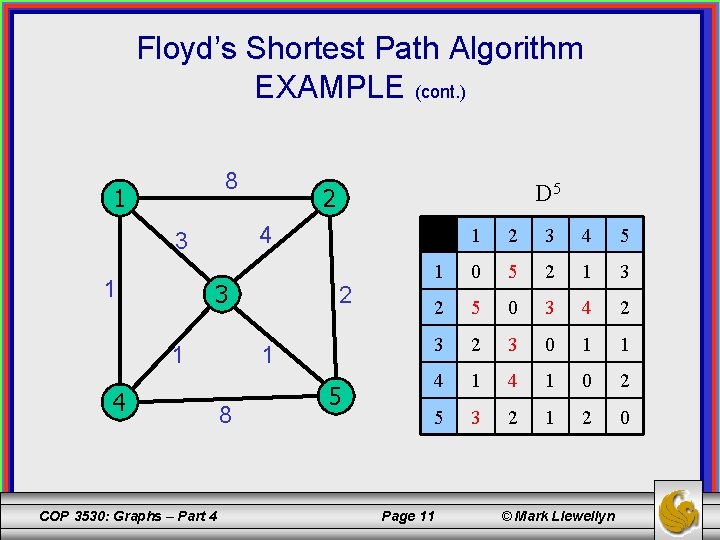
Floyd’s Shortest Path Algorithm EXAMPLE (cont. ) 8 1 4 3 1 D 5 2 3 1 4 COP 3530: Graphs – Part 4 2 1 8 5 1 2 3 4 5 1 0 5 2 1 3 2 5 0 3 4 2 3 0 1 1 4 1 0 2 5 3 2 1 2 0 Page 11 © Mark Llewellyn
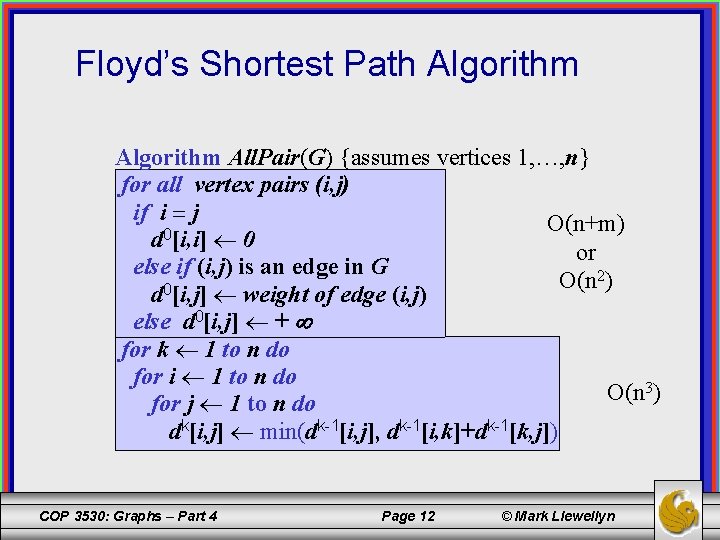
Floyd’s Shortest Path Algorithm All. Pair(G) {assumes vertices 1, …, n} for all vertex pairs (i, j) if i = j O(n+m) 0 d [i, i] 0 or else if (i, j) is an edge in G O(n 2) 0 d [i, j] weight of edge (i, j) else d 0[i, j] + for k 1 to n do for i 1 to n do 3) O(n for j 1 to n do dk[i, j] min(dk-1[i, j], dk-1[i, k]+dk-1[k, j]) COP 3530: Graphs – Part 4 Page 12 © Mark Llewellyn
![Floyds Shortest Path Algorithm Observation d k i k d k1 i k Floyd’s Shortest Path Algorithm Observation: d k [i, k] = d k-1 [i, k]](https://slidetodoc.com/presentation_image_h2/150633ab07edfaa617183f0dc4b11444/image-13.jpg)
Floyd’s Shortest Path Algorithm Observation: d k [i, k] = d k-1 [i, k] d k [k, j] = d k-1 [k, j] This observation leads to the following simplification of the algorithm: COP 3530: Graphs – Part 4 Page 13 © Mark Llewellyn
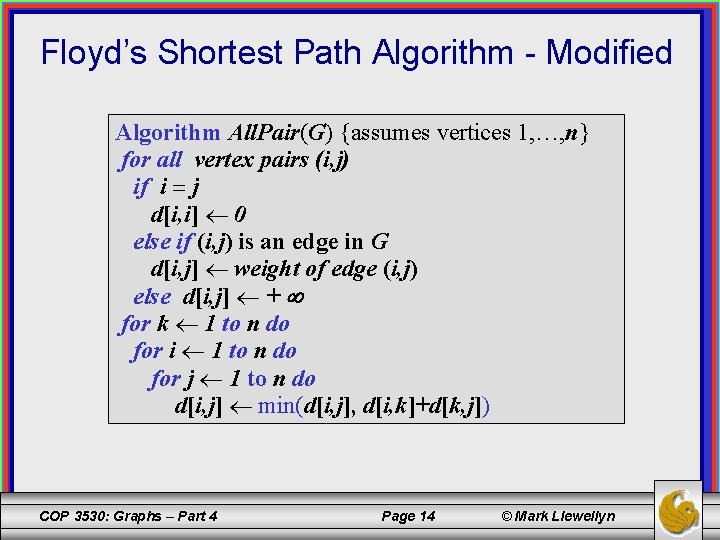
Floyd’s Shortest Path Algorithm - Modified Algorithm All. Pair(G) {assumes vertices 1, …, n} for all vertex pairs (i, j) if i = j d[i, i] 0 else if (i, j) is an edge in G d[i, j] weight of edge (i, j) else d[i, j] + for k 1 to n do for i 1 to n do for j 1 to n do d[i, j] min(d[i, j], d[i, k]+d[k, j]) COP 3530: Graphs – Part 4 Page 14 © Mark Llewellyn
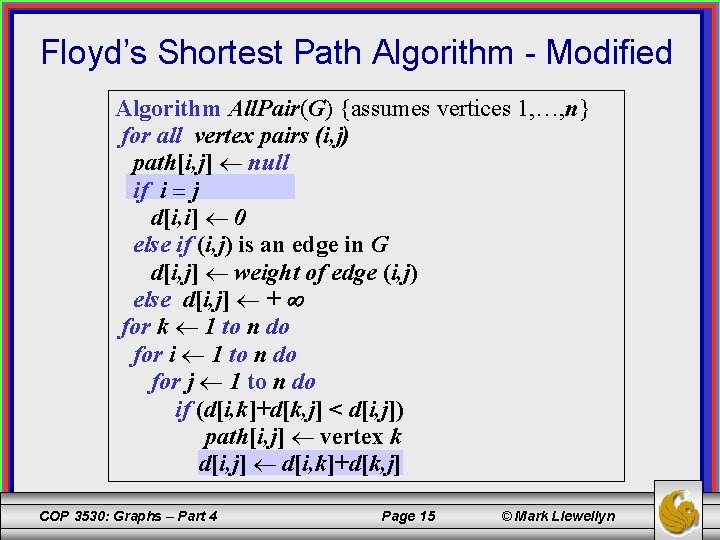
Floyd’s Shortest Path Algorithm - Modified Algorithm All. Pair(G) {assumes vertices 1, …, n} for all vertex pairs (i, j) path[i, j] null if i = j d[i, i] 0 else if (i, j) is an edge in G d[i, j] weight of edge (i, j) else d[i, j] + for k 1 to n do for i 1 to n do for j 1 to n do if (d[i, k]+d[k, j] < d[i, j]) path[i, j] vertex k d[i, j] d[i, k]+d[k, j] COP 3530: Graphs – Part 4 Page 15 © Mark Llewellyn
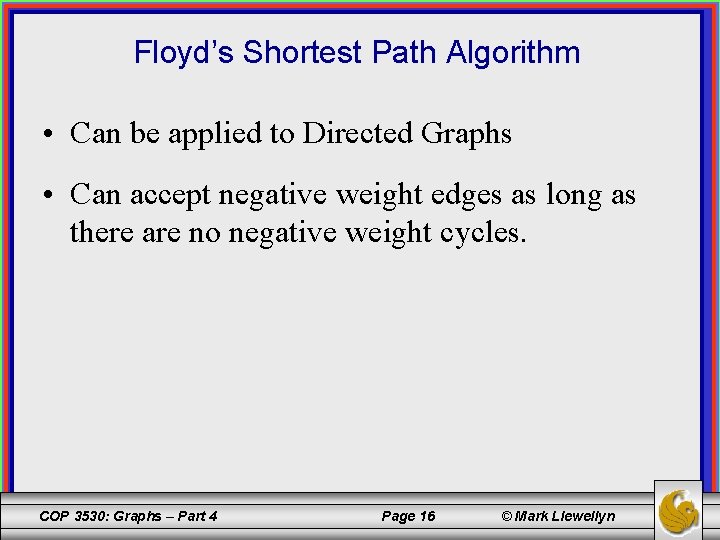
Floyd’s Shortest Path Algorithm • Can be applied to Directed Graphs • Can accept negative weight edges as long as there are no negative weight cycles. COP 3530: Graphs – Part 4 Page 16 © Mark Llewellyn
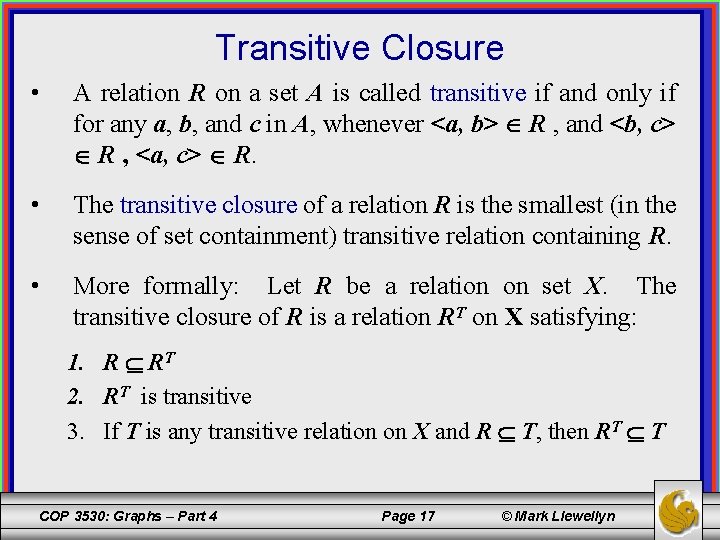
Transitive Closure • A relation R on a set A is called transitive if and only if for any a, b, and c in A, whenever <a, b> R , and <b, c> R , <a, c> R. • The transitive closure of a relation R is the smallest (in the sense of set containment) transitive relation containing R. • More formally: Let R be a relation on set X. The transitive closure of R is a relation RT on X satisfying: 1. R RT 2. RT is transitive 3. If T is any transitive relation on X and R T, then RT T COP 3530: Graphs – Part 4 Page 17 © Mark Llewellyn
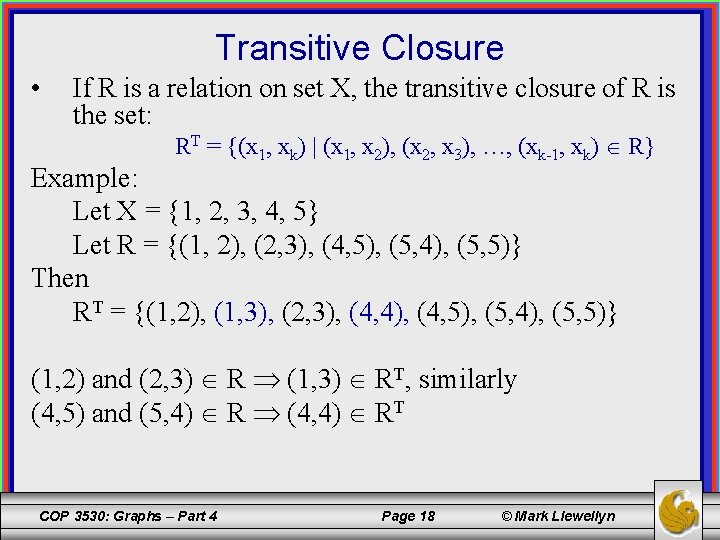
Transitive Closure • If R is a relation on set X, the transitive closure of R is the set: RT = {(x 1, xk) | (x 1, x 2), (x 2, x 3), …, (xk-1, xk) R} Example: Let X = {1, 2, 3, 4, 5} Let R = {(1, 2), (2, 3), (4, 5), (5, 4), (5, 5)} Then RT = {(1, 2), (1, 3), (2, 3), (4, 4), (4, 5), (5, 4), (5, 5)} (1, 2) and (2, 3) R (1, 3) RT, similarly (4, 5) and (5, 4) R (4, 4) RT COP 3530: Graphs – Part 4 Page 18 © Mark Llewellyn
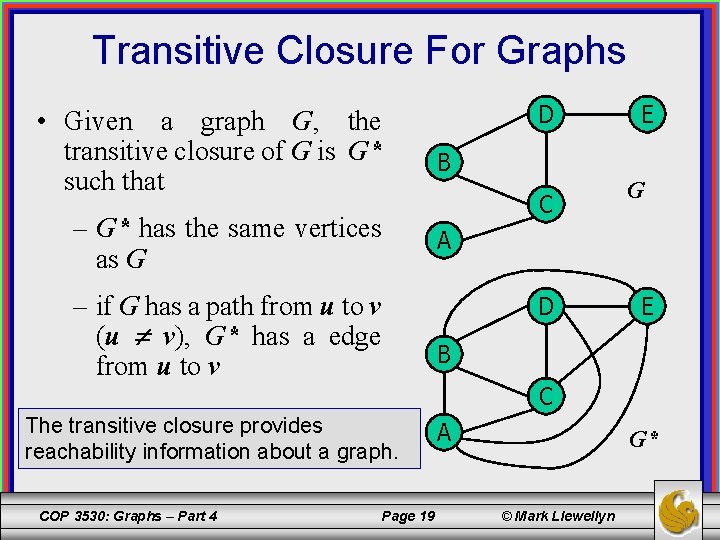
Transitive Closure For Graphs D • Given a graph G, the transitive closure of G is G* such that B – G* has the same vertices as G A – if G has a path from u to v (u v), G* has a edge from u to v C D E G E B C The transitive closure provides reachability information about a graph. COP 3530: Graphs – Part 4 Page 19 A G* © Mark Llewellyn
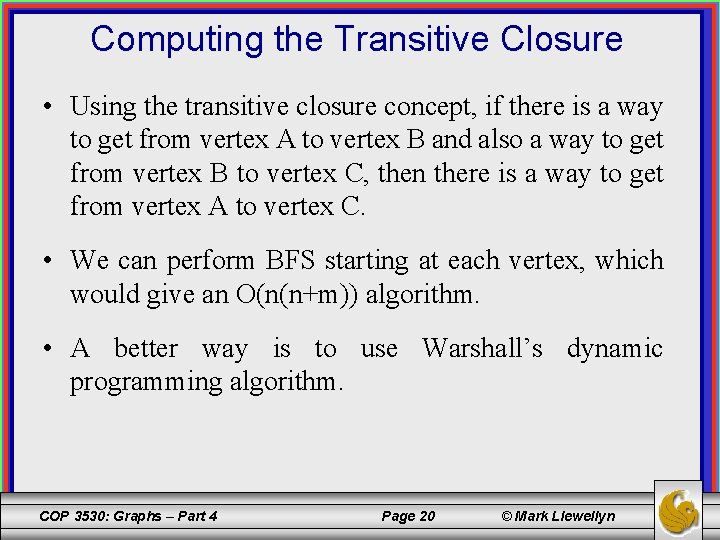
Computing the Transitive Closure • Using the transitive closure concept, if there is a way to get from vertex A to vertex B and also a way to get from vertex B to vertex C, then there is a way to get from vertex A to vertex C. • We can perform BFS starting at each vertex, which would give an O(n(n+m)) algorithm. • A better way is to use Warshall’s dynamic programming algorithm. COP 3530: Graphs – Part 4 Page 20 © Mark Llewellyn
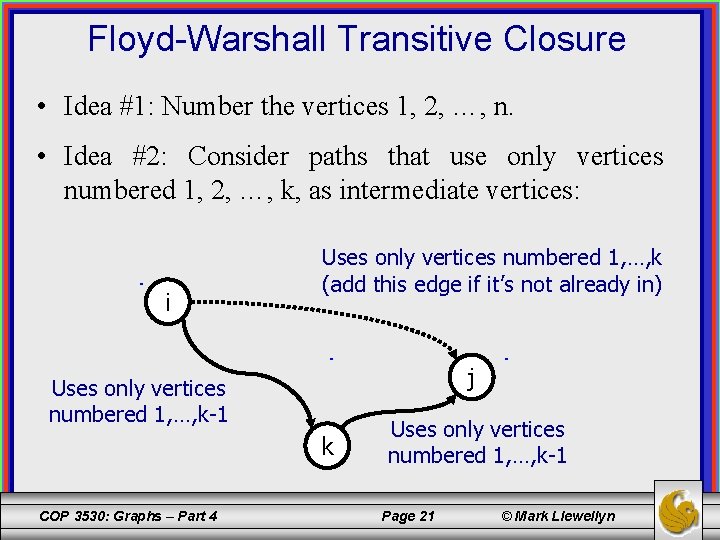
Floyd-Warshall Transitive Closure • Idea #1: Number the vertices 1, 2, …, n. • Idea #2: Consider paths that use only vertices numbered 1, 2, …, k, as intermediate vertices: i Uses only vertices numbered 1, …, k (add this edge if it’s not already in) j Uses only vertices numbered 1, …, k-1 k COP 3530: Graphs – Part 4 Uses only vertices numbered 1, …, k-1 Page 21 © Mark Llewellyn
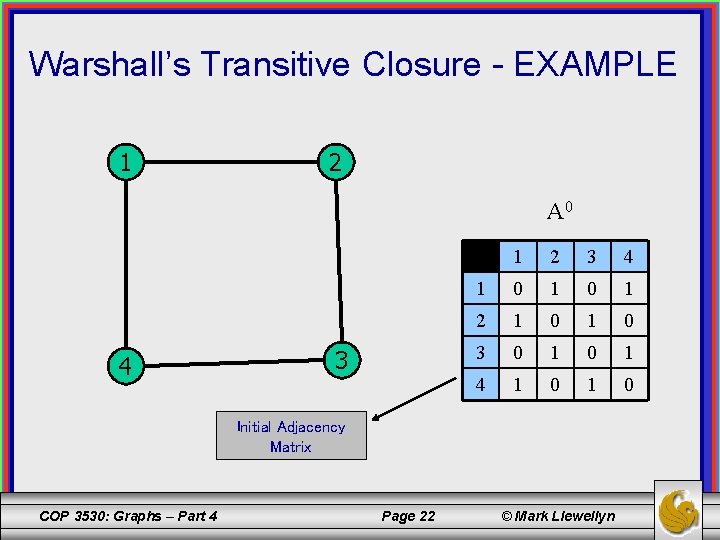
Warshall’s Transitive Closure - EXAMPLE 1 2 A 0 4 3 1 2 3 4 1 0 1 2 1 0 3 0 1 4 1 0 Initial Adjacency Matrix COP 3530: Graphs – Part 4 Page 22 © Mark Llewellyn
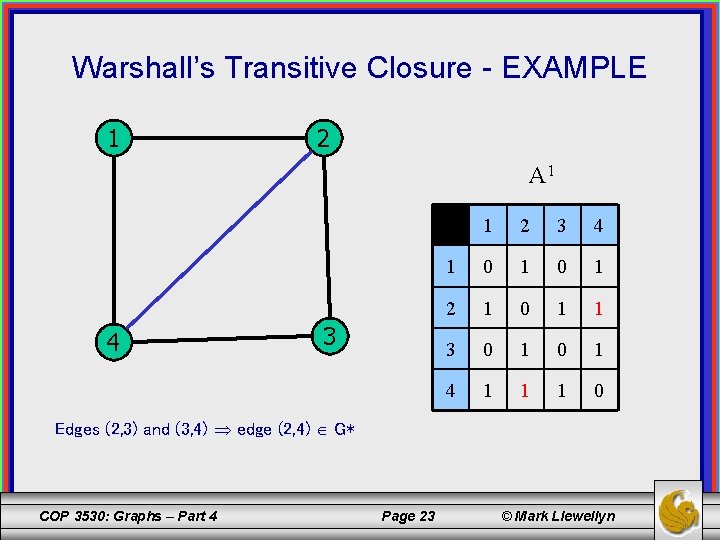
Warshall’s Transitive Closure - EXAMPLE 1 2 A 1 4 3 1 2 3 4 1 0 1 2 1 0 1 1 3 0 1 4 1 1 1 0 Edges (2, 3) and (3, 4) edge (2, 4) G* COP 3530: Graphs – Part 4 Page 23 © Mark Llewellyn
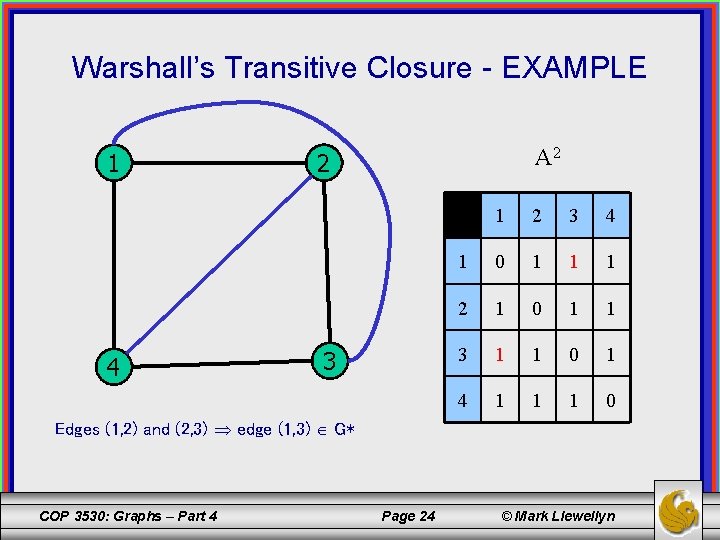
Warshall’s Transitive Closure - EXAMPLE 1 4 A 2 2 3 1 2 3 4 1 0 1 1 1 2 1 0 1 1 3 1 1 0 1 4 1 1 1 0 Edges (1, 2) and (2, 3) edge (1, 3) G* COP 3530: Graphs – Part 4 Page 24 © Mark Llewellyn
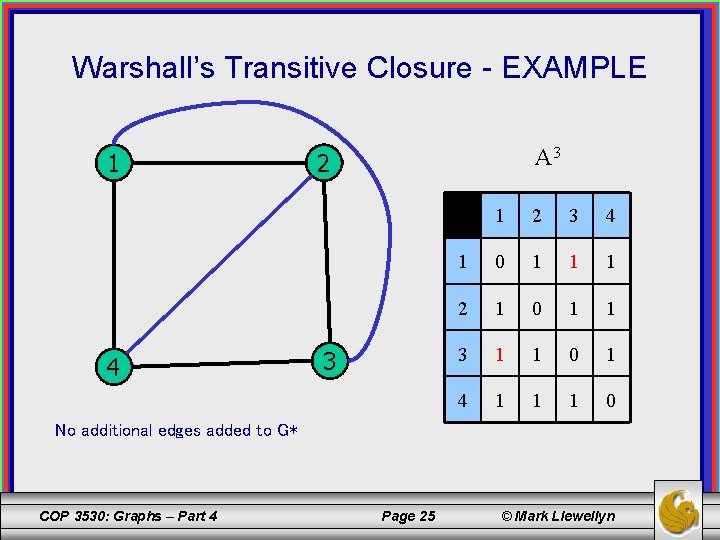
Warshall’s Transitive Closure - EXAMPLE 1 4 A 3 2 3 1 2 3 4 1 0 1 1 1 2 1 0 1 1 3 1 1 0 1 4 1 1 1 0 No additional edges added to G* COP 3530: Graphs – Part 4 Page 25 © Mark Llewellyn
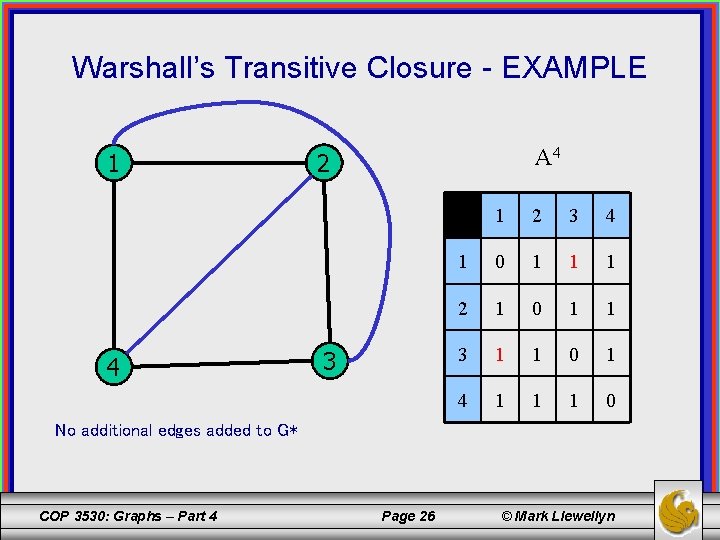
Warshall’s Transitive Closure - EXAMPLE 1 4 A 4 2 3 1 2 3 4 1 0 1 1 1 2 1 0 1 1 3 1 1 0 1 4 1 1 1 0 No additional edges added to G* COP 3530: Graphs – Part 4 Page 26 © Mark Llewellyn
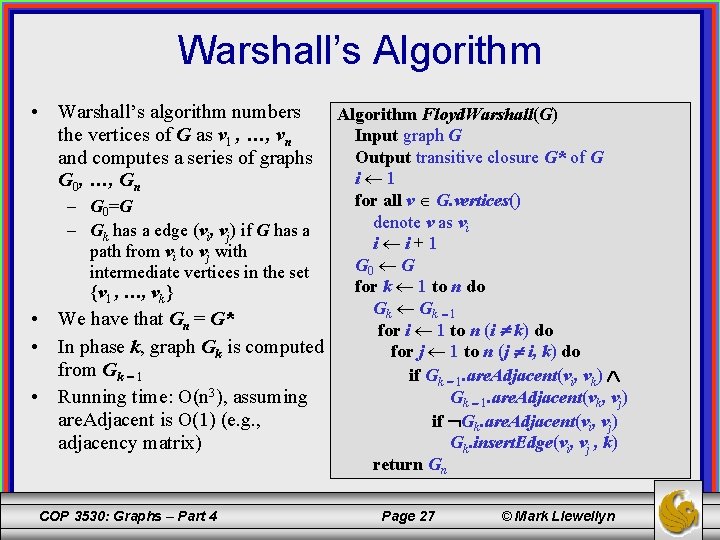
Warshall’s Algorithm • Warshall’s algorithm numbers the vertices of G as v 1 , …, vn and computes a series of graphs G 0, …, Gn • • • Algorithm Floyd. Warshall(G) Input graph G Output transitive closure G* of G i 1 for all v G. vertices() – G 0=G denote v as vi – Gk has a edge (vi, vj) if G has a i i+1 path from vi to vj with G 0 G intermediate vertices in the set for k 1 to n do {v 1 , …, vk} Gk - 1 We have that Gn = G* for i 1 to n (i k) do In phase k, graph Gk is computed for j 1 to n (j i, k) do from Gk - 1 if Gk - 1. are. Adjacent(vi, vk) Gk - 1. are. Adjacent(vk, vj) Running time: O(n 3), assuming are. Adjacent is O(1) (e. g. , if Gk. are. Adjacent(vi, vj) Gk. insert. Edge(vi, vj , k) adjacency matrix) return Gn COP 3530: Graphs – Part 4 Page 27 © Mark Llewellyn
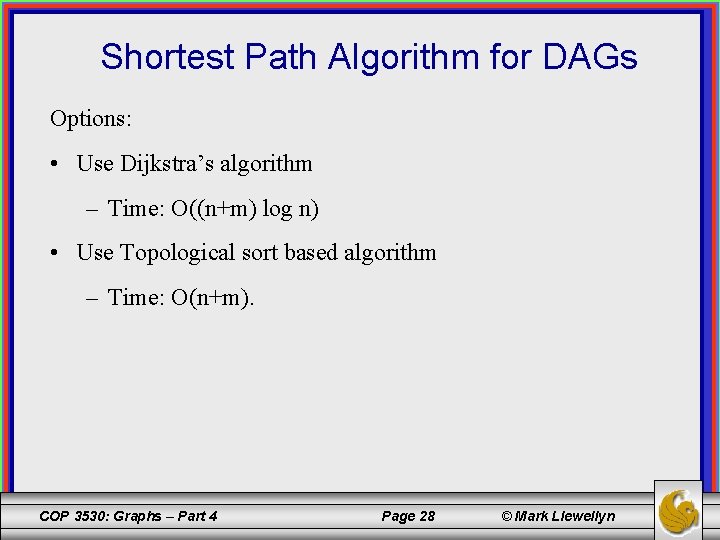
Shortest Path Algorithm for DAGs Options: • Use Dijkstra’s algorithm – Time: O((n+m) log n) • Use Topological sort based algorithm – Time: O(n+m). COP 3530: Graphs – Part 4 Page 28 © Mark Llewellyn
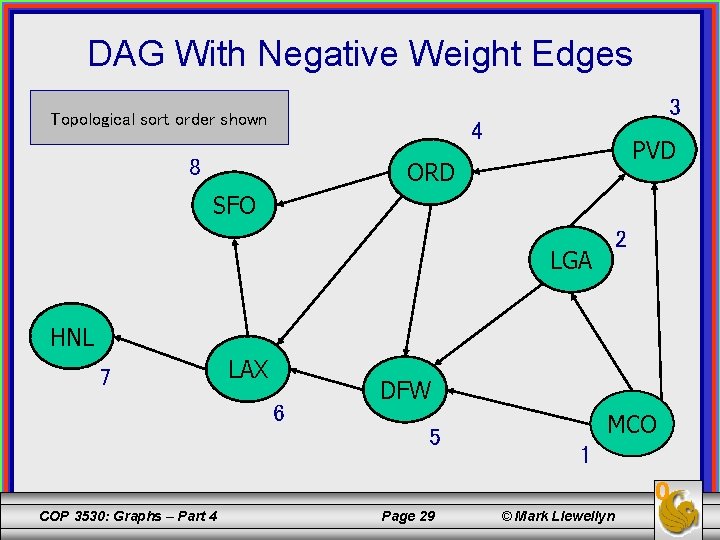
DAG With Negative Weight Edges 3 Topological sort order shown 4 8 PVD ORD SFO 2 LGA HNL 7 LAX 6 DFW 5 MCO 1 0 COP 3530: Graphs – Part 4 Page 29 © Mark Llewellyn
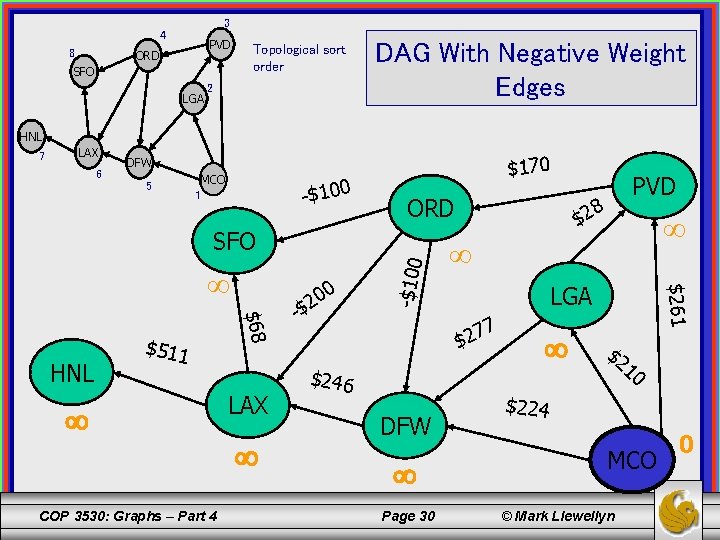
3 4 8 PVD ORD SFO LGA Topological sort order 2 DAG With Negative Weight Edges HNL 7 LAX 6 $170 DFW 5 MCO 1 -$100 ORD COP 3530: Graphs – Part 4 $68 HNL $511 LAX 0 - 0 2 $ 8 $2 LGA 7 7 2 $ $246 DFW Page 30 $261 -$100 SFO PVD $2 10 $224 MCO © Mark Llewellyn 0
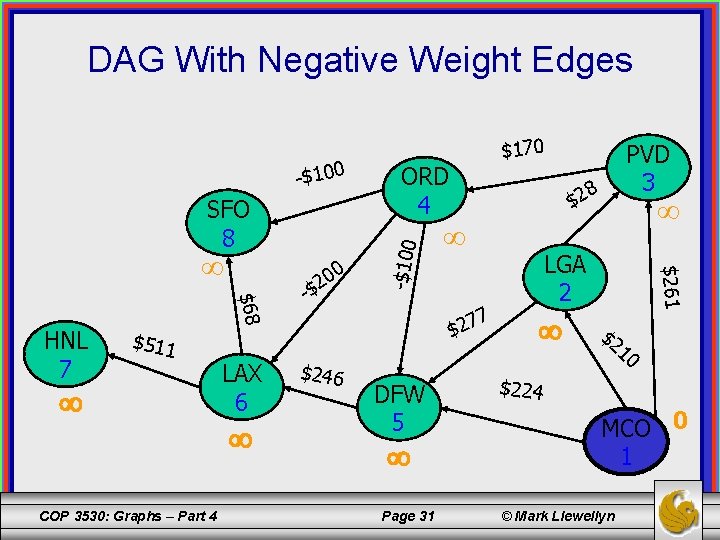
DAG With Negative Weight Edges SFO 8 $68 HNL 7 0 20 $511 COP 3530: Graphs – Part 4 LAX 6 -$ $246 8 $2 7 7 2 $ DFW 5 Page 31 PVD 3 LGA 2 $261 ORD 4 -$100 $170 $2 10 $224 MCO 0 1 © Mark Llewellyn
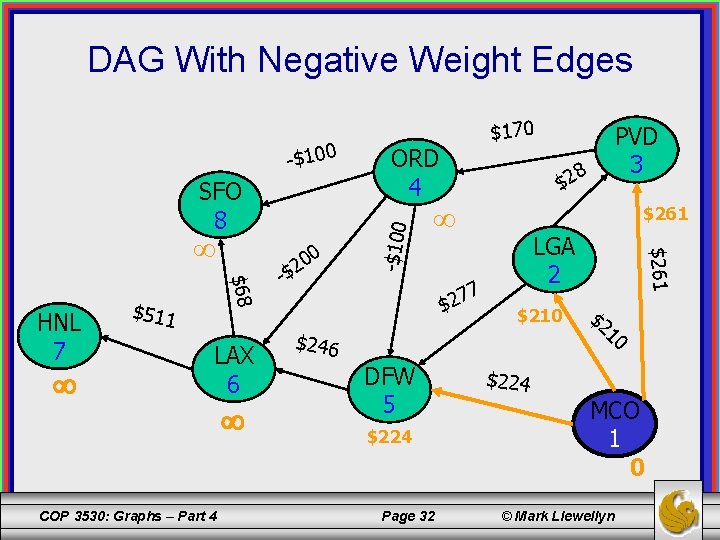
DAG With Negative Weight Edges SFO 8 $68 HNL 7 $511 LAX 6 COP 3530: Graphs – Part 4 0 20 -$ 8 $2 7 7 2 $ $261 LGA 2 $210 $246 DFW 5 $224 Page 32 PVD 3 $224 $261 ORD 4 -$100 $170 $2 10 MCO 1 0 © Mark Llewellyn
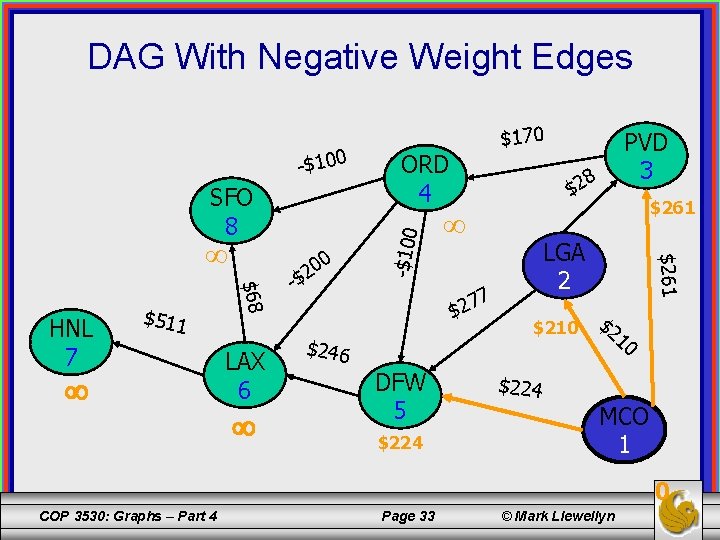
DAG With Negative Weight Edges SFO 8 $511 $68 HNL 7 LAX 6 0 20 -$ 8 $2 DFW 5 $224 $261 LGA 2 7 $246 PVD 3 $210 $224 $261 ORD 4 -$100 $170 $2 10 MCO 1 0 COP 3530: Graphs – Part 4 Page 33 © Mark Llewellyn
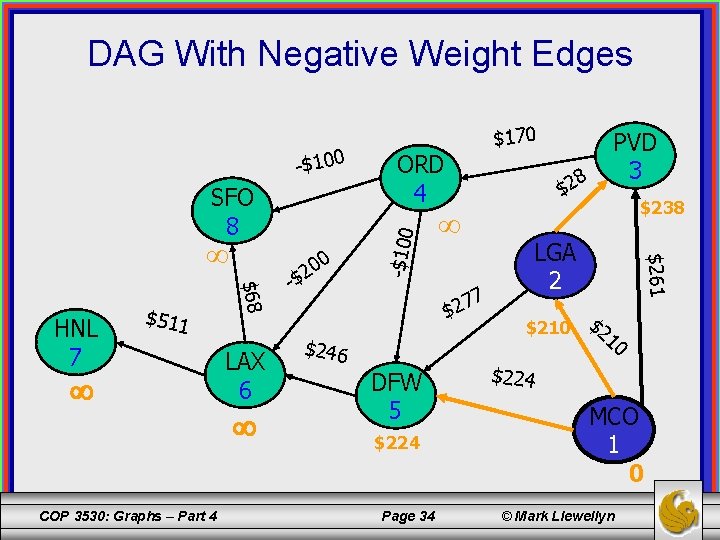
DAG With Negative Weight Edges SFO 8 $511 COP 3530: Graphs – Part 4 $68 HNL 7 LAX 6 00 2 $ - 8 $2 7 $246 DFW 5 $224 Page 34 PVD 3 $238 LGA 2 $261 ORD 4 -$100 $170 $210 $224 MCO 1 0 © Mark Llewellyn
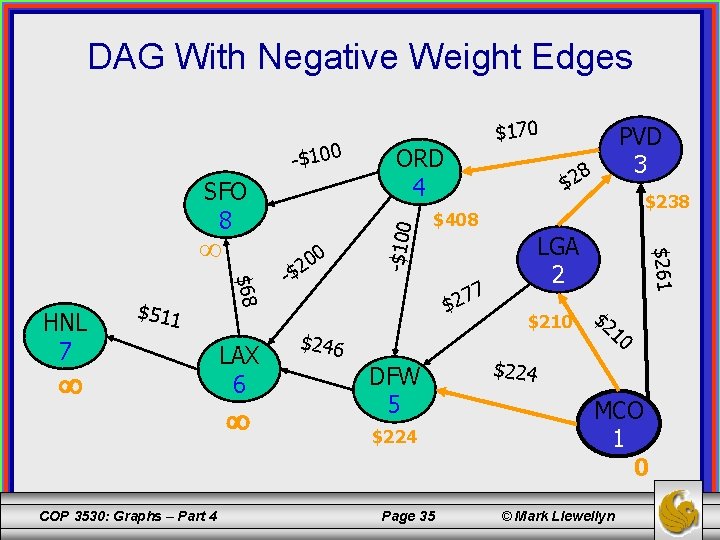
DAG With Negative Weight Edges SFO 8 $511 COP 3530: Graphs – Part 4 $68 HNL 7 LAX 6 0 20 -$ $2 PVD 3 8 $238 $408 7 7 2 $ $246 DFW 5 $224 Page 35 LGA 2 $210 $261 ORD 4 -$100 $170 $2 10 $224 MCO 1 0 © Mark Llewellyn
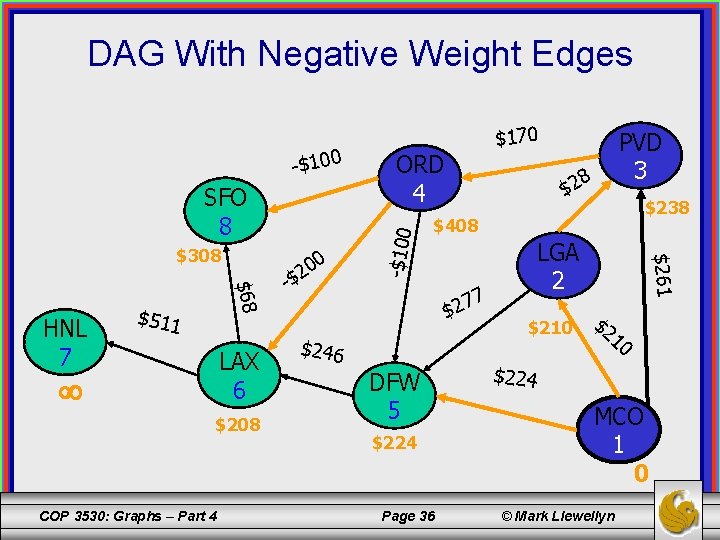
DAG With Negative Weight Edges SFO 8 $68 HNL 7 $511 LAX 6 $208 COP 3530: Graphs – Part 4 0 20 -$ $2 PVD 3 8 $238 $408 LGA 2 7 $246 DFW 5 $224 Page 36 $210 $261 $308 ORD 4 -$100 $170 $2 10 $224 MCO 1 0 © Mark Llewellyn
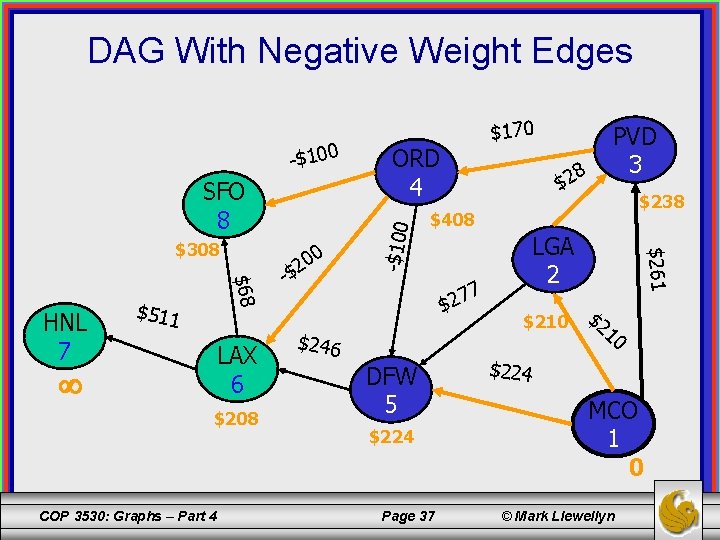
DAG With Negative Weight Edges SFO 8 $68 HNL 7 $511 LAX 6 $208 COP 3530: Graphs – Part 4 0 20 -$ $2 PVD 3 8 $238 $408 7 7 2 $ $246 DFW 5 $224 Page 37 LGA 2 $210 $261 $308 ORD 4 -$100 $170 $2 10 $224 MCO 1 0 © Mark Llewellyn
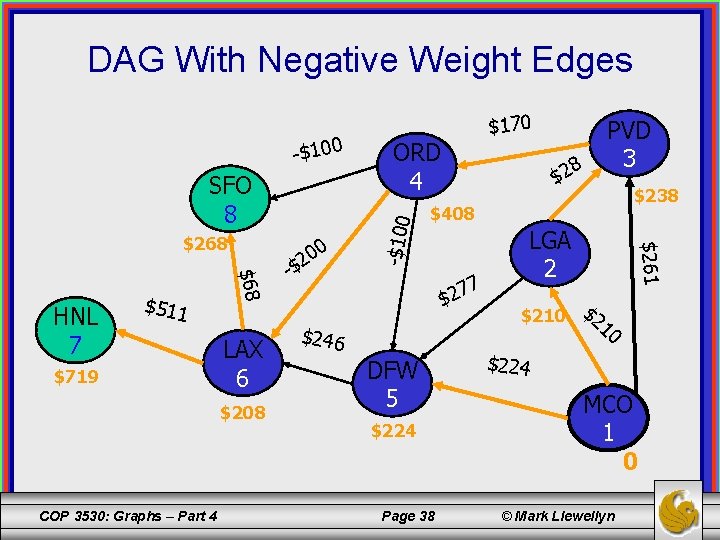
DAG With Negative Weight Edges SFO 8 $511 $719 $68 HNL 7 LAX 6 $208 COP 3530: Graphs – Part 4 0 20 -$ $2 8 PVD 3 $238 $408 7 $246 DFW 5 $224 Page 38 LGA 2 $261 $268 ORD 4 -$100 $170 $210 $224 MCO 1 0 © Mark Llewellyn
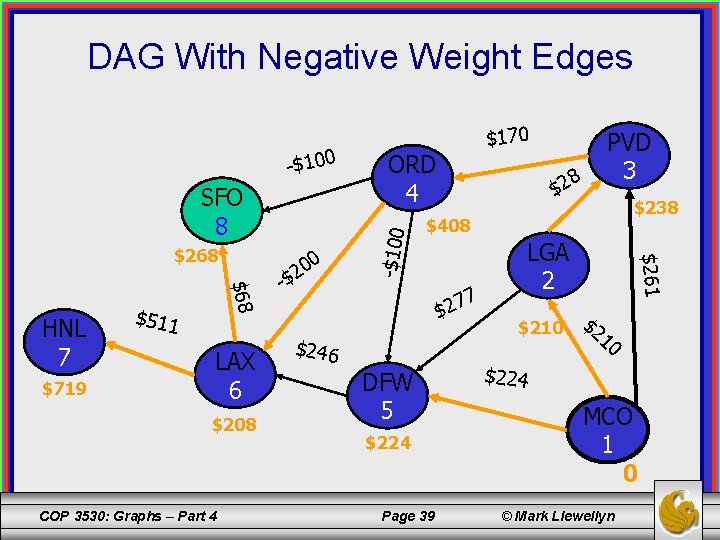
DAG With Negative Weight Edges SFO 8 $719 $68 HNL 7 $511 LAX 6 $208 COP 3530: Graphs – Part 4 00 2 -$ $2 8 PVD 3 $238 $408 7 $246 DFW 5 $224 Page 39 LGA 2 $261 $268 ORD 4 -$100 $170 $210 $224 MCO 1 0 © Mark Llewellyn
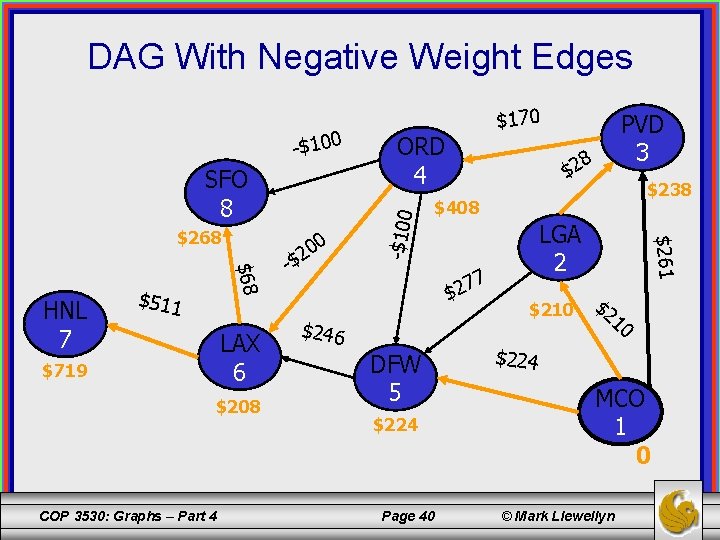
DAG With Negative Weight Edges SFO 8 $68 HNL 7 $511 LAX 6 $719 $208 COP 3530: Graphs – Part 4 00 2 -$ $2 PVD 3 8 $238 $408 7 7 2 $ $246 DFW 5 $224 Page 40 LGA 2 $210 $261 $268 ORD 4 -$100 $170 $2 10 $224 MCO 1 0 © Mark Llewellyn
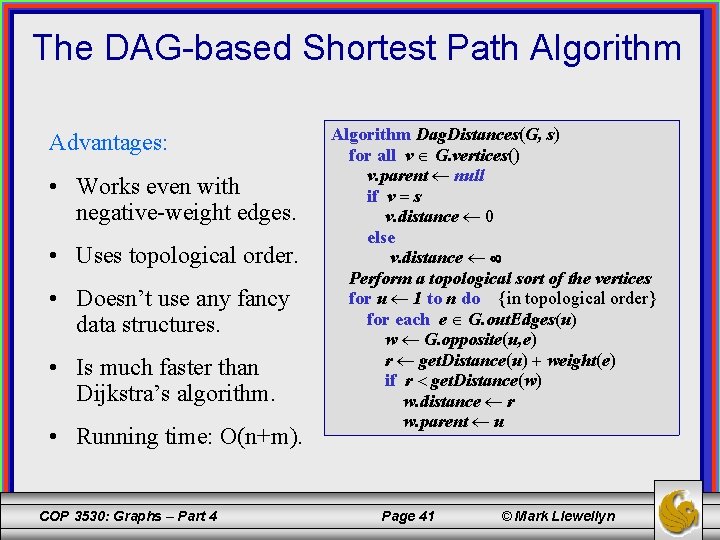
The DAG-based Shortest Path Algorithm Advantages: • Works even with negative-weight edges. • Uses topological order. • Doesn’t use any fancy data structures. • Is much faster than Dijkstra’s algorithm. • Running time: O(n+m). COP 3530: Graphs – Part 4 Algorithm Dag. Distances(G, s) for all v G. vertices() v. parent null if v = s v. distance 0 else v. distance Perform a topological sort of the vertices for u 1 to n do {in topological order} for each e G. out. Edges(u) w G. opposite(u, e) r get. Distance(u) + weight(e) if r < get. Distance(w) w. distance r w. parent u Page 41 © Mark Llewellyn
Cop 3530
Good cop bad cop interrogation
Cop 1 cop 2
Gtr 3530
Cpa prep alberta
Hamlet act iii scene ii
Good state graphs and bad state graphs
Graphs that compare distance and time are called
Graphs that enlighten and graphs that deceive
Degree and leading coefficient
My favourite subject english
Making science graphs and interpreting data
Manipulated variable and responding variable graph
Why are line graphs powerful tools in science?
The nature of science communicating with graphs
So cal science olympiad
Summer science program
Elizabeth bowman vanderbilt
Science olympiad summer institute
Science olympiad summer institute
Multipurpose refrigeration systems with a single compressor
Air cycle process
Pem pal
Cop of refrigerator
Evde küpe benzeyen eşyalar
Les polly cop
Cop 4910
Cop 4710
Cop 4710 ucf
Cop 3402
Cop2500
Cop 2500
Anima assoclima
Cop2500
Brooklyn cop
Audit cop
Cop 16
Snow cop
Cop 5725
Cop 3502
Cop 3330 ucf
Cop 3330 fsu