Chapter 9 Perl continue Advanced Perl Programming Some
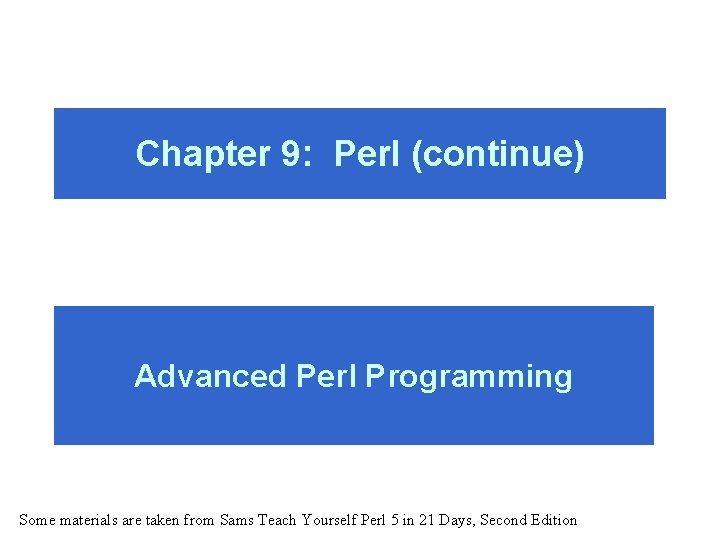
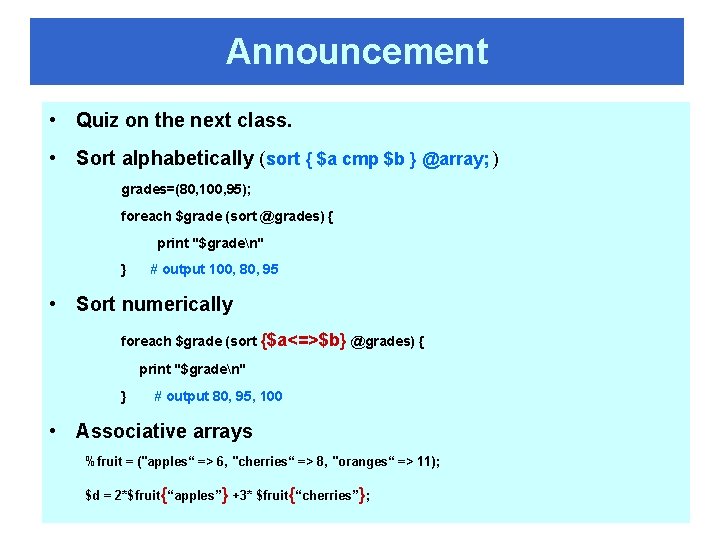
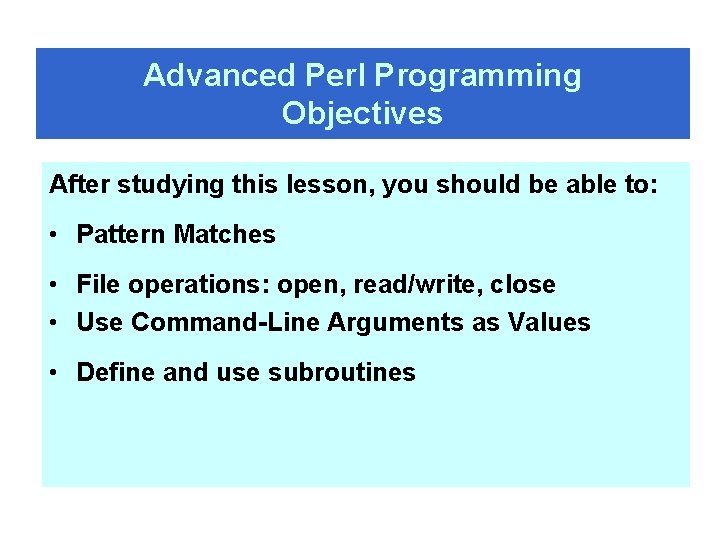
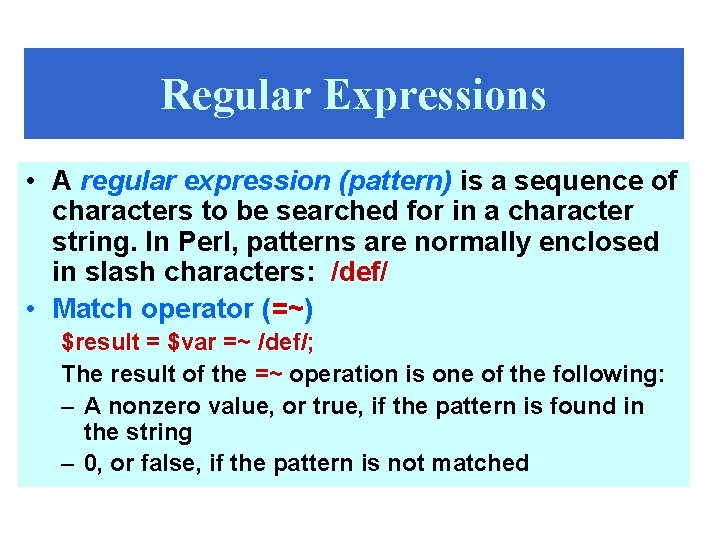
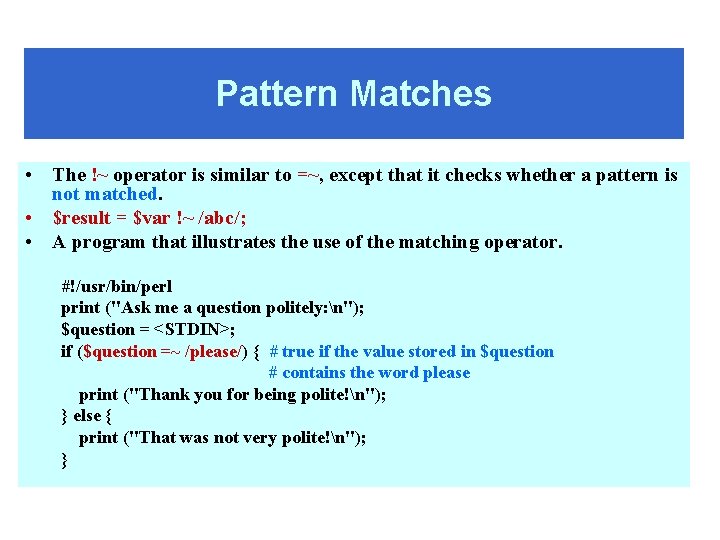
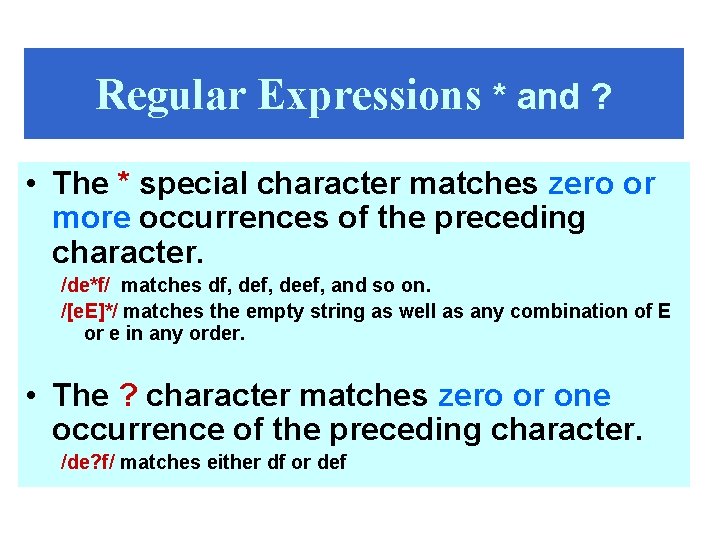
![Regular Expressions [ ] • [ ] special characters enable you to define patterns Regular Expressions [ ] • [ ] special characters enable you to define patterns](https://slidetodoc.com/presentation_image_h2/01766f8650dd9cb5a7ada4ba8e909cf8/image-7.jpg)
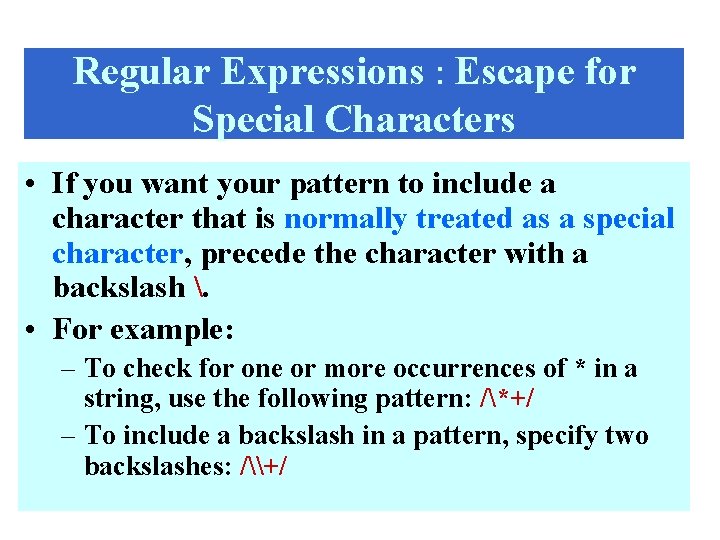
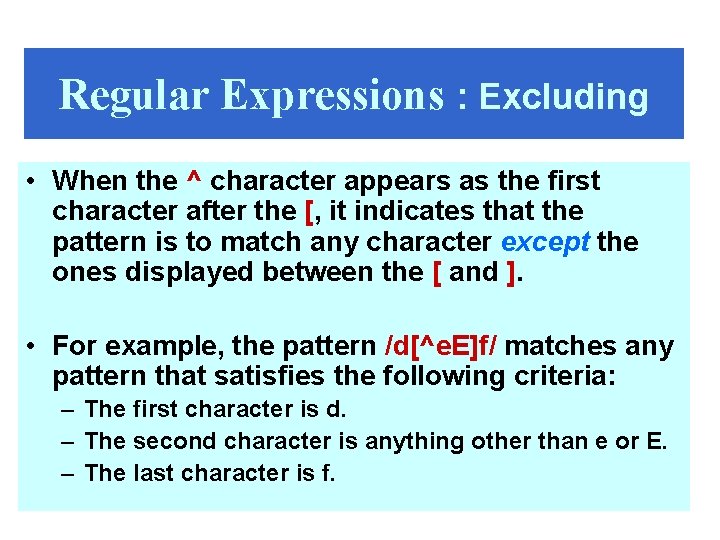
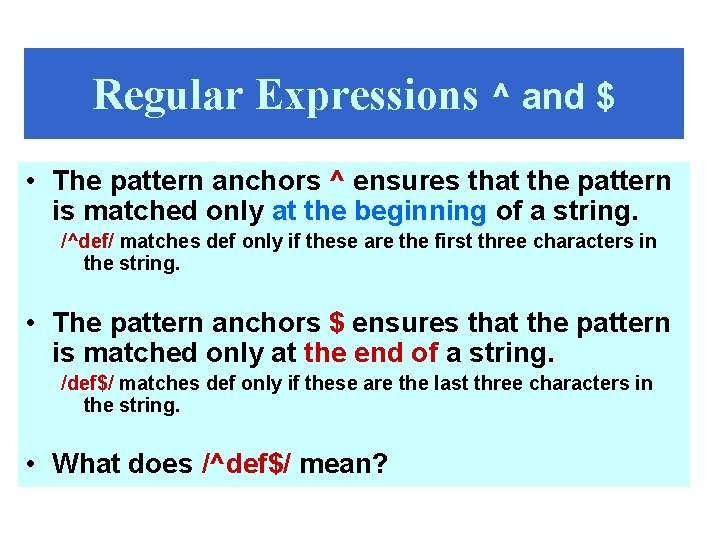
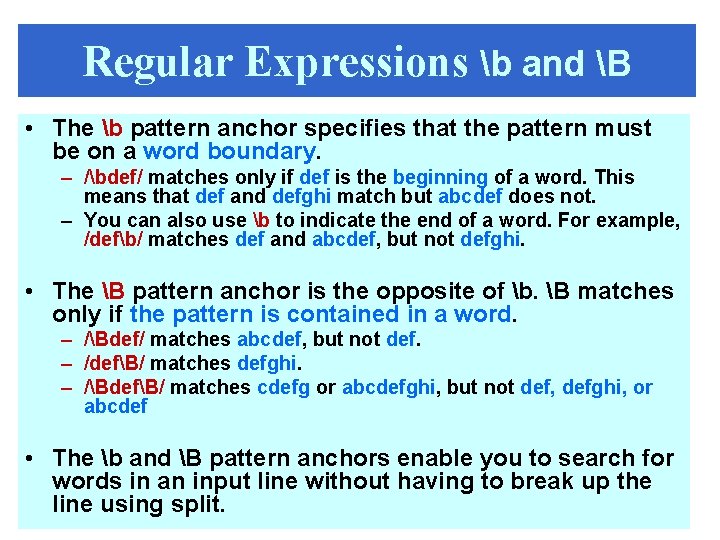
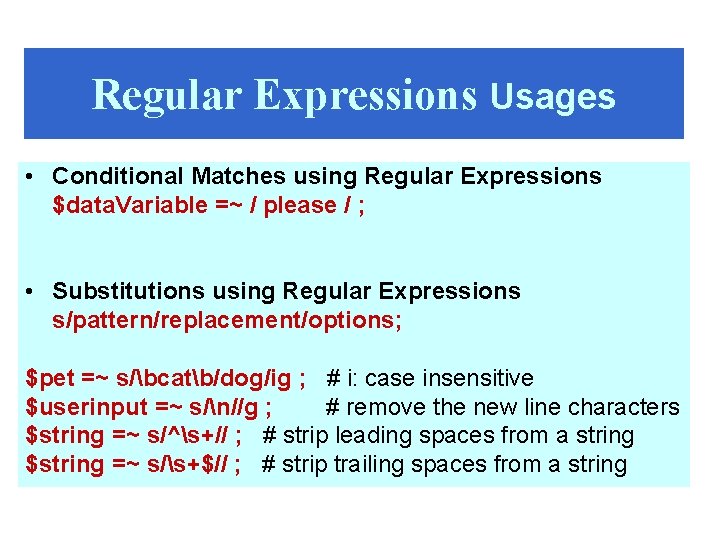
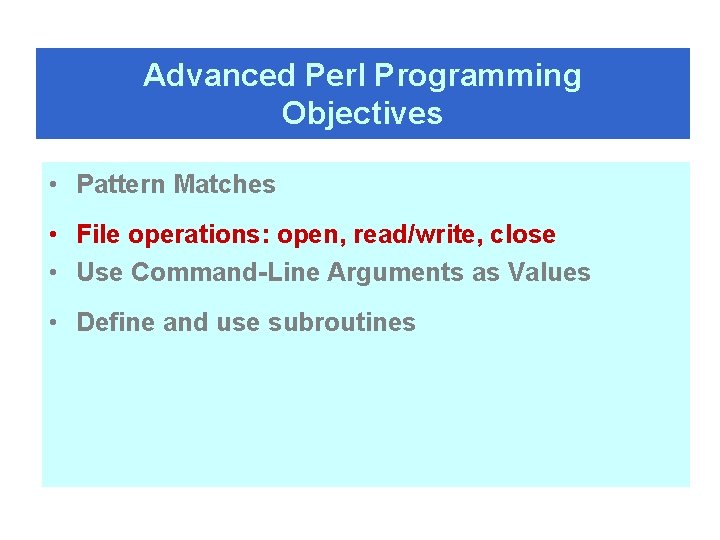
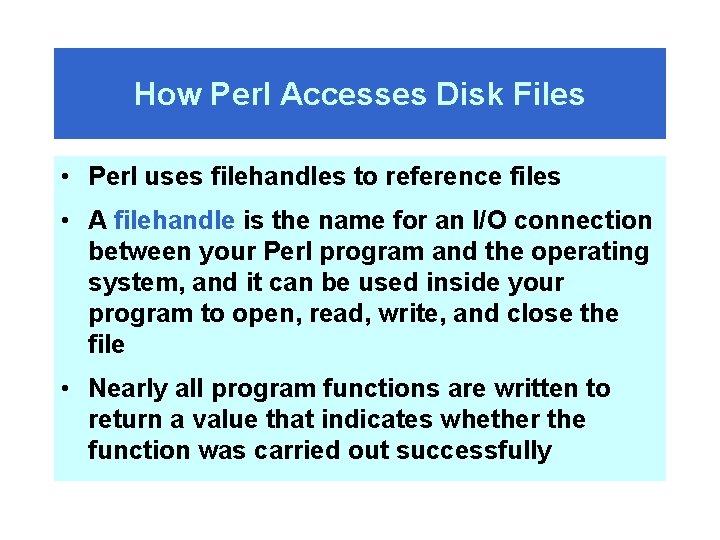
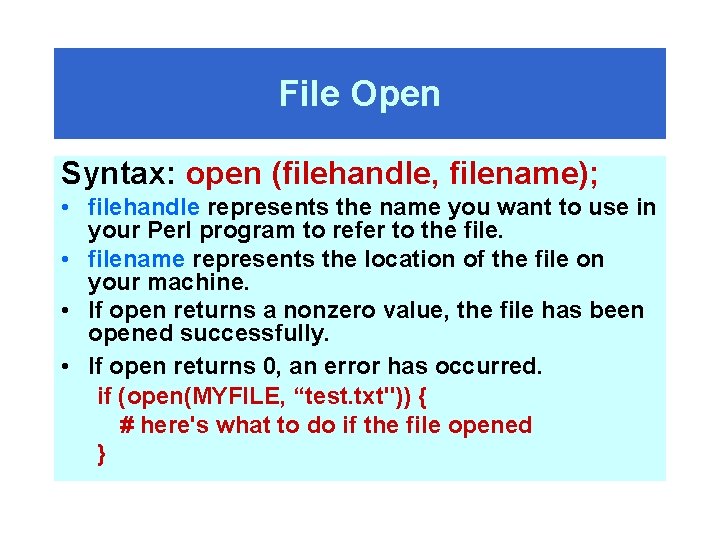
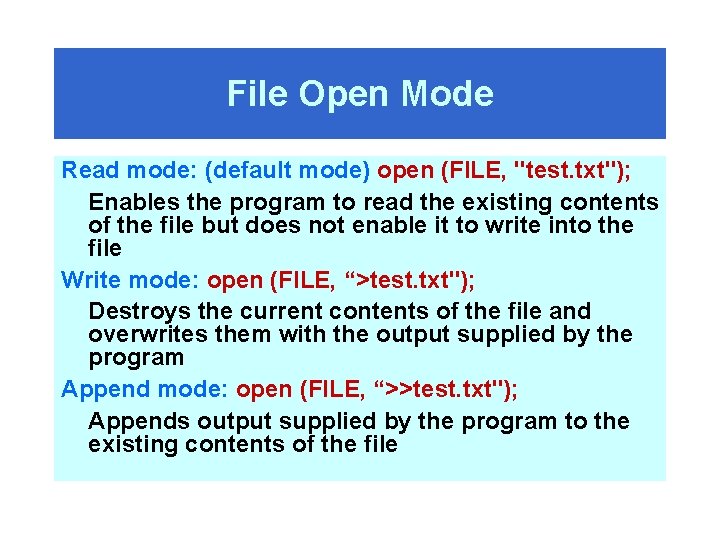
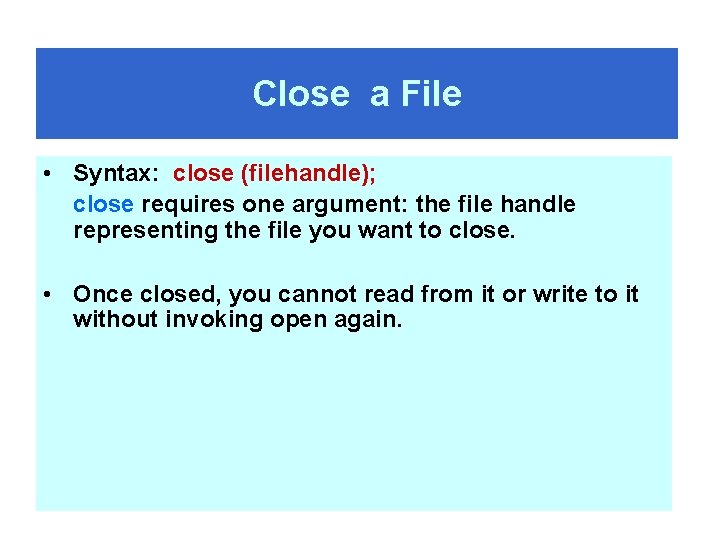
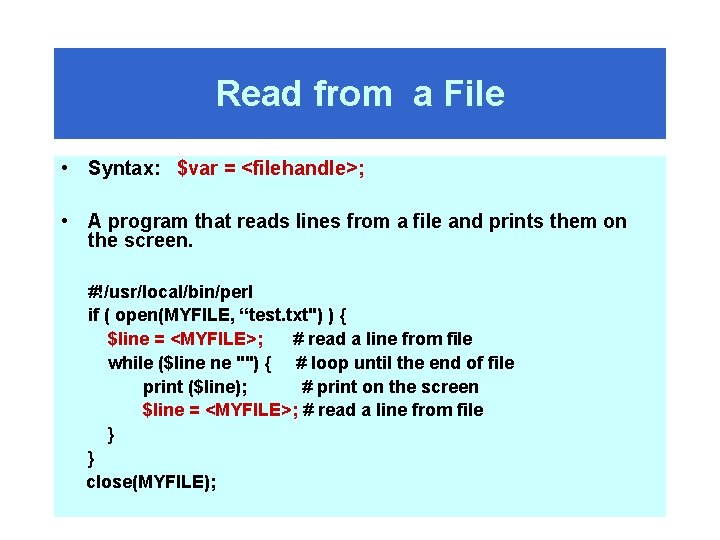
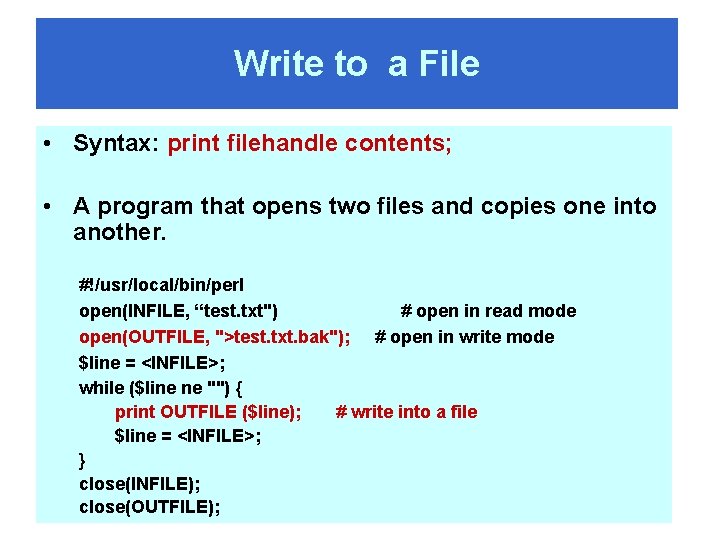
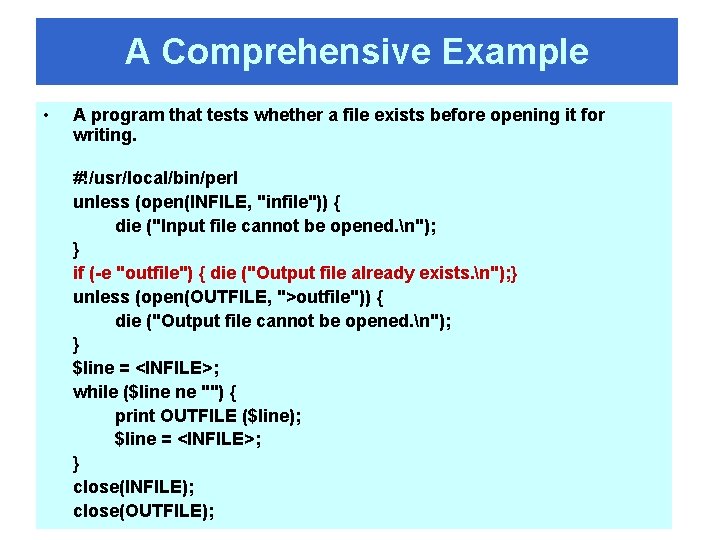
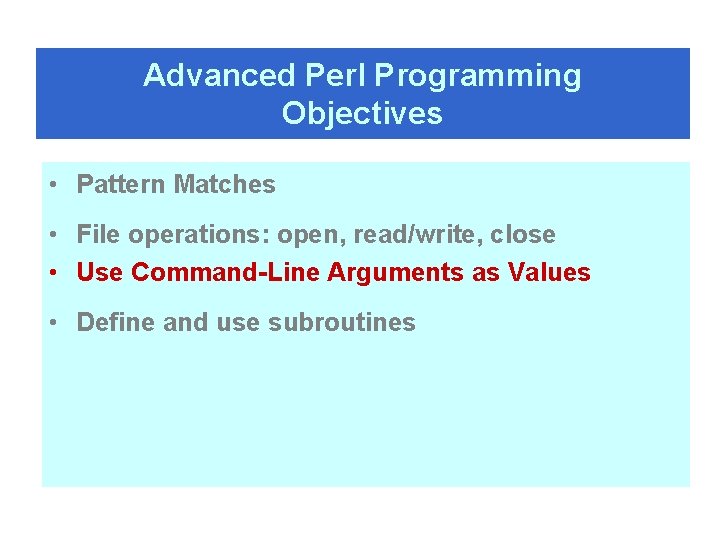
![Command Line Arguments • Perl stores the command-line arguments in @ARGV. • $ARGV[0] contains Command Line Arguments • Perl stores the command-line arguments in @ARGV. • $ARGV[0] contains](https://slidetodoc.com/presentation_image_h2/01766f8650dd9cb5a7ada4ba8e909cf8/image-22.jpg)
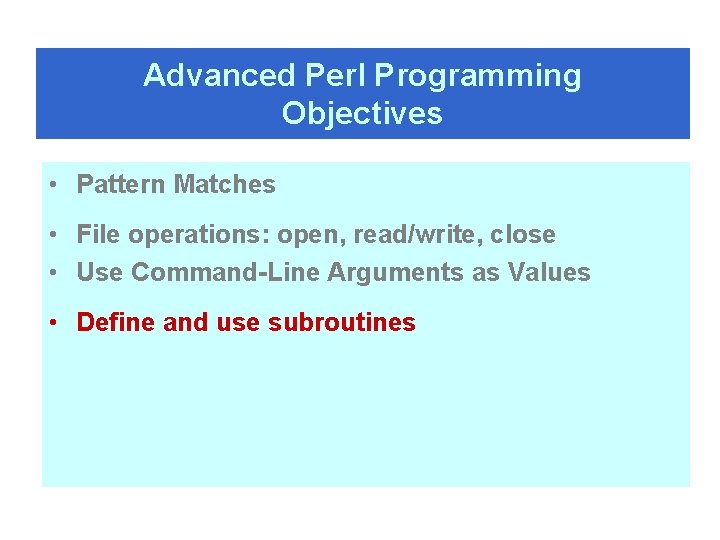
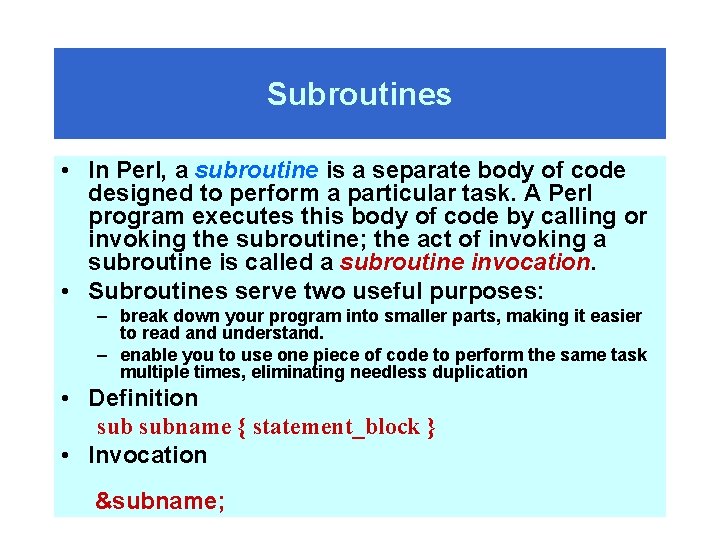
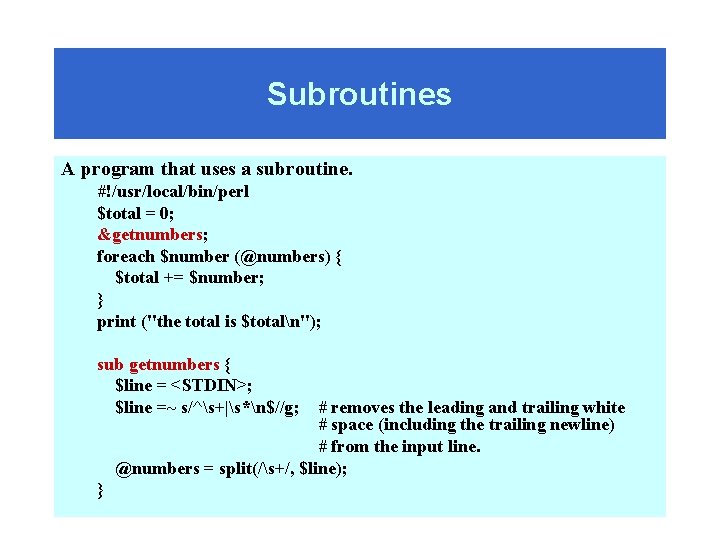
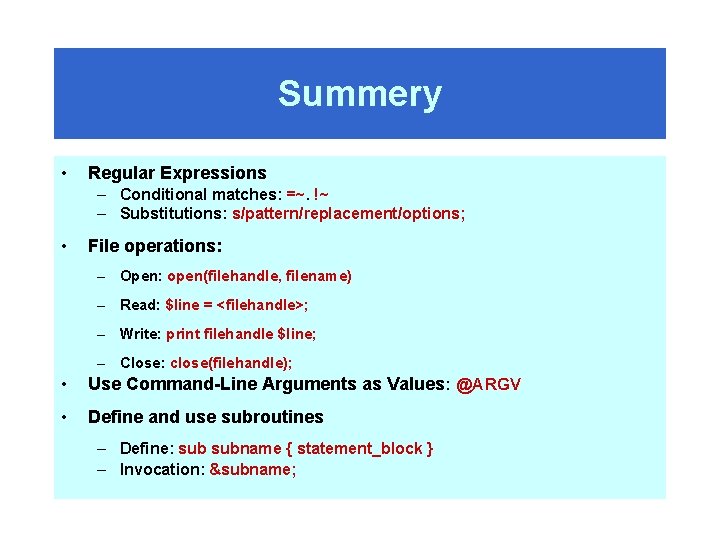
- Slides: 26
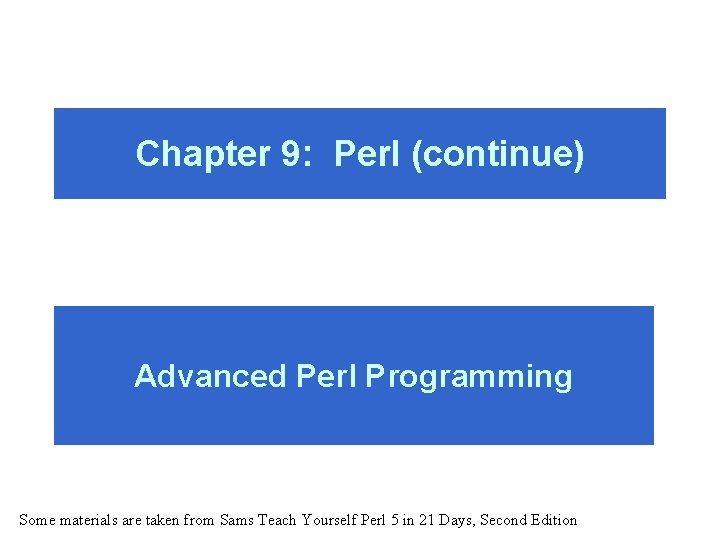
Chapter 9: Perl (continue) Advanced Perl Programming Some materials are taken from Sams Teach Yourself Perl 5 in 21 Days, Second Edition
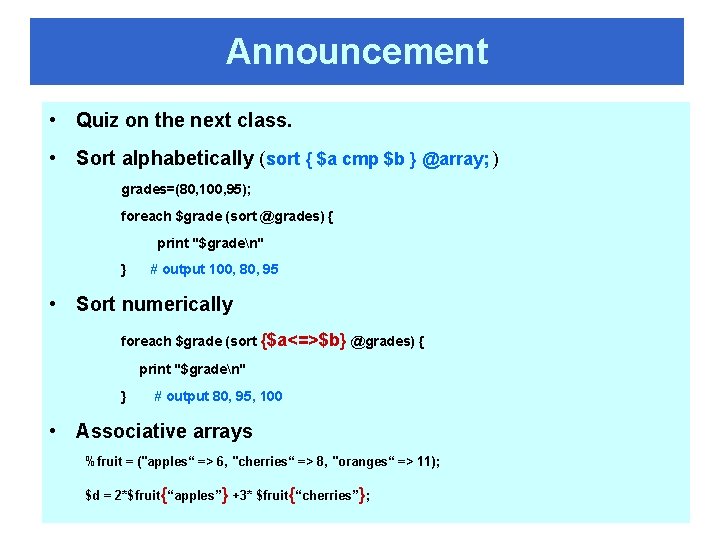
Announcement • Quiz on the next class. • Sort alphabetically (sort { $a cmp $b } @array; ) grades=(80, 100, 95); foreach $grade (sort @grades) { print "$graden" } # output 100, 80, 95 • Sort numerically foreach $grade (sort {$a<=>$b} @grades) { print "$graden" } # output 80, 95, 100 • Associative arrays %fruit = ("apples“ => 6, "cherries“ => 8, "oranges“ => 11); $d = 2*$fruit{“apples”} +3* $fruit{“cherries”};
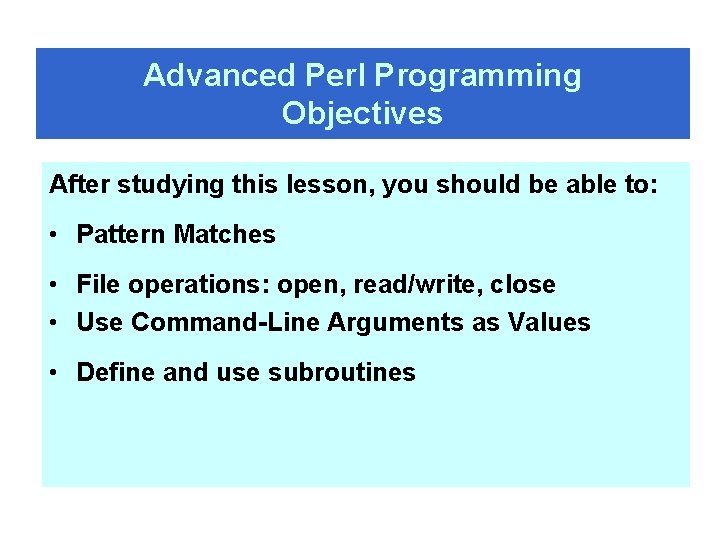
Advanced Perl Programming Objectives After studying this lesson, you should be able to: • Pattern Matches • File operations: open, read/write, close • Use Command-Line Arguments as Values • Define and use subroutines
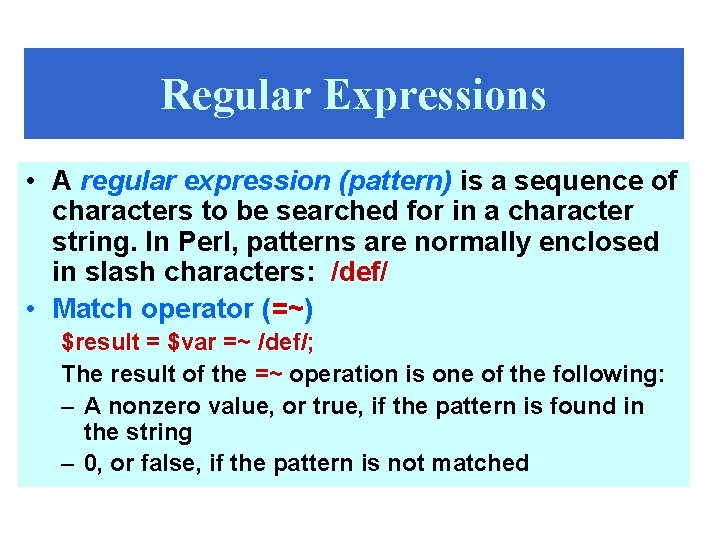
Regular Expressions • A regular expression (pattern) is a sequence of characters to be searched for in a character string. In Perl, patterns are normally enclosed in slash characters: /def/ • Match operator (=~) $result = $var =~ /def/; The result of the =~ operation is one of the following: – A nonzero value, or true, if the pattern is found in the string – 0, or false, if the pattern is not matched
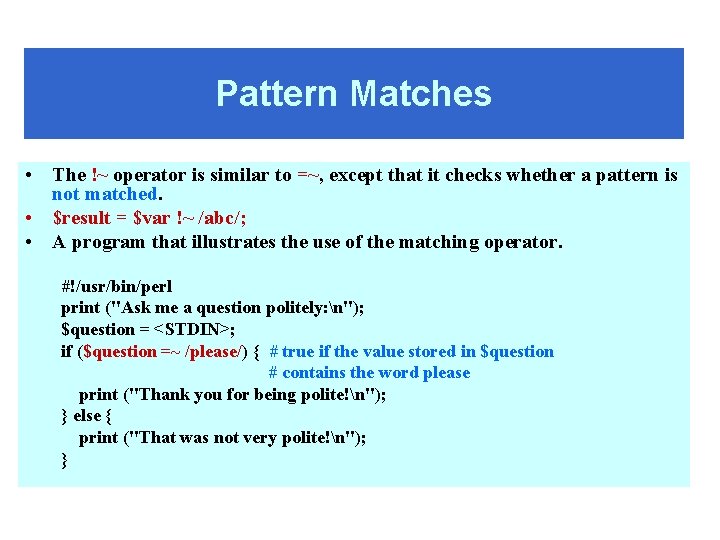
Pattern Matches • The !~ operator is similar to =~, except that it checks whether a pattern is not matched. • $result = $var !~ /abc/; • A program that illustrates the use of the matching operator. #!/usr/bin/perl print ("Ask me a question politely: n"); $question = <STDIN>; if ($question =~ /please/) { # true if the value stored in $question # contains the word please print ("Thank you for being polite!n"); } else { print ("That was not very polite!n"); }
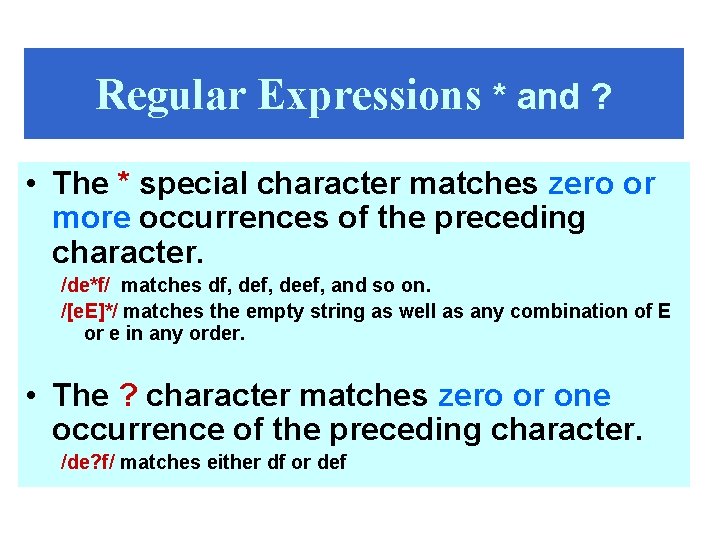
Regular Expressions * and ? • The * special character matches zero or more occurrences of the preceding character. /de*f/ matches df, deef, and so on. /[e. E]*/ matches the empty string as well as any combination of E or e in any order. • The ? character matches zero or one occurrence of the preceding character. /de? f/ matches either df or def
![Regular Expressions special characters enable you to define patterns Regular Expressions [ ] • [ ] special characters enable you to define patterns](https://slidetodoc.com/presentation_image_h2/01766f8650dd9cb5a7ada4ba8e909cf8/image-7.jpg)
Regular Expressions [ ] • [ ] special characters enable you to define patterns that match one of a group of alternatives. – For example: /d[e. E]f/ matches def or d. Ef • Combine [ ] with + to match a sequence of characters of any length. – For example: d[e. E]+f/ matches all of the following: def, d. Ef, deef, d. EEEeee. Eef
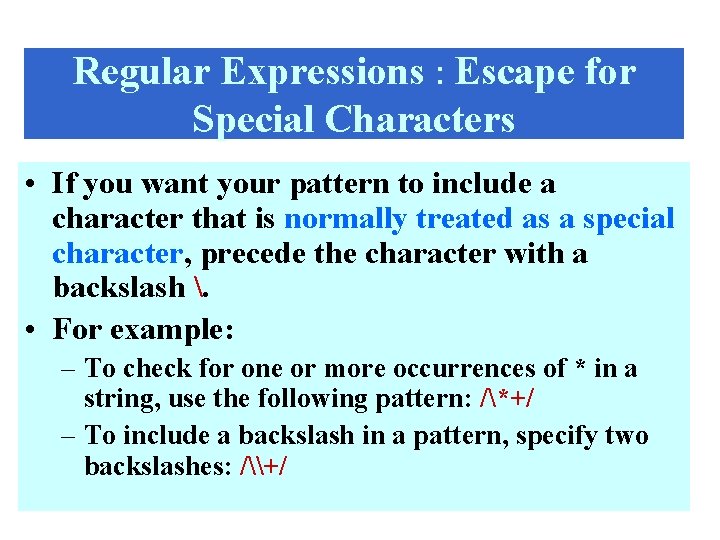
Regular Expressions : Escape for Special Characters • If you want your pattern to include a character that is normally treated as a special character, precede the character with a backslash . • For example: – To check for one or more occurrences of * in a string, use the following pattern: /*+/ – To include a backslash in a pattern, specify two backslashes: /\+/
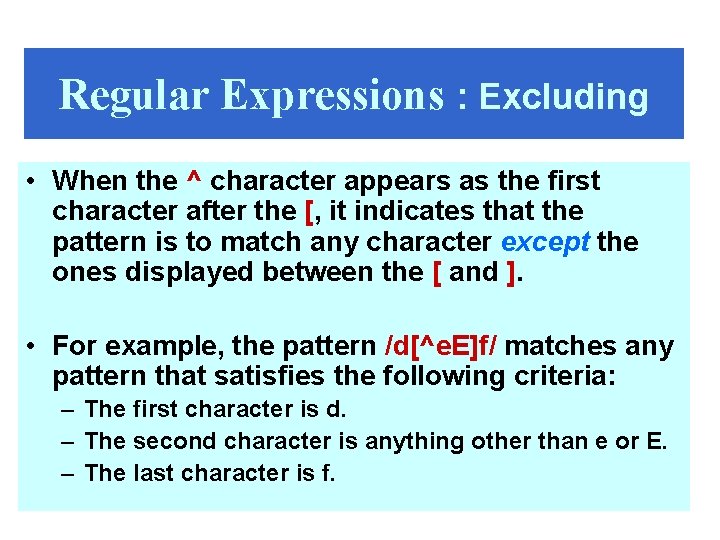
Regular Expressions : Excluding • When the ^ character appears as the first character after the [, it indicates that the pattern is to match any character except the ones displayed between the [ and ]. • For example, the pattern /d[^e. E]f/ matches any pattern that satisfies the following criteria: – The first character is d. – The second character is anything other than e or E. – The last character is f.
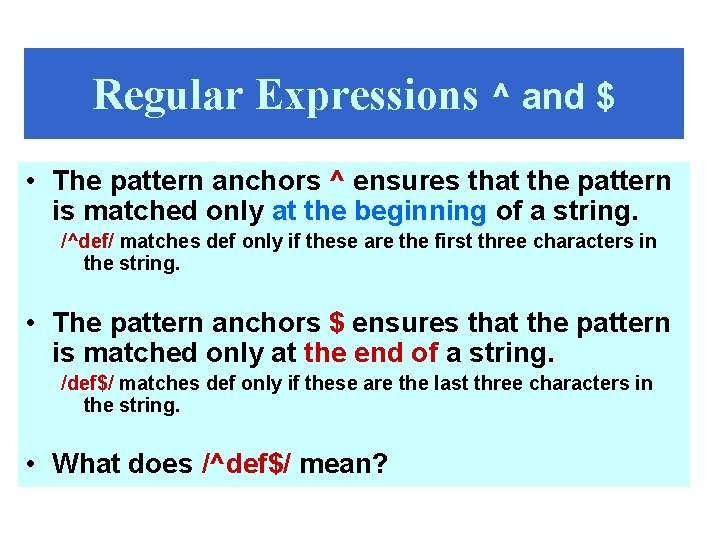
Regular Expressions ^ and $ • The pattern anchors ^ ensures that the pattern is matched only at the beginning of a string. /^def/ matches def only if these are the first three characters in the string. • The pattern anchors $ ensures that the pattern is matched only at the end of a string. /def$/ matches def only if these are the last three characters in the string. • What does /^def$/ mean?
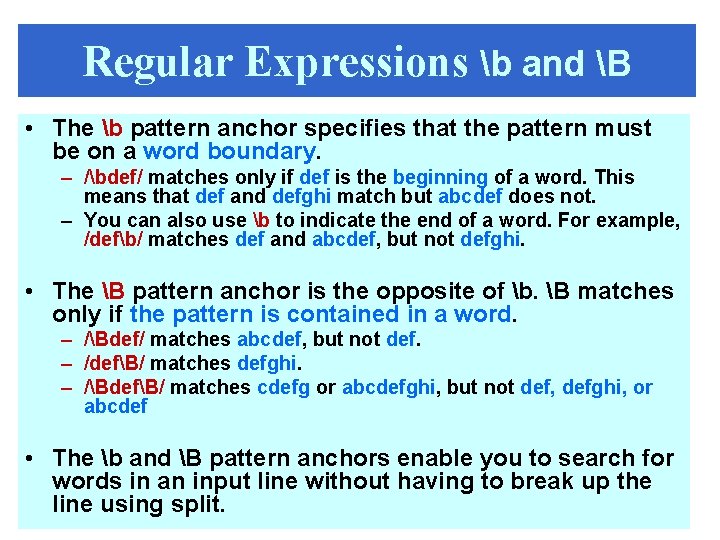
Regular Expressions b and B • The b pattern anchor specifies that the pattern must be on a word boundary. – /bdef/ matches only if def is the beginning of a word. This means that def and defghi match but abcdef does not. – You can also use b to indicate the end of a word. For example, /defb/ matches def and abcdef, but not defghi. • The B pattern anchor is the opposite of b. B matches only if the pattern is contained in a word. – /Bdef/ matches abcdef, but not def. – /defB/ matches defghi. – /BdefB/ matches cdefg or abcdefghi, but not def, defghi, or abcdef • The b and B pattern anchors enable you to search for words in an input line without having to break up the line using split.
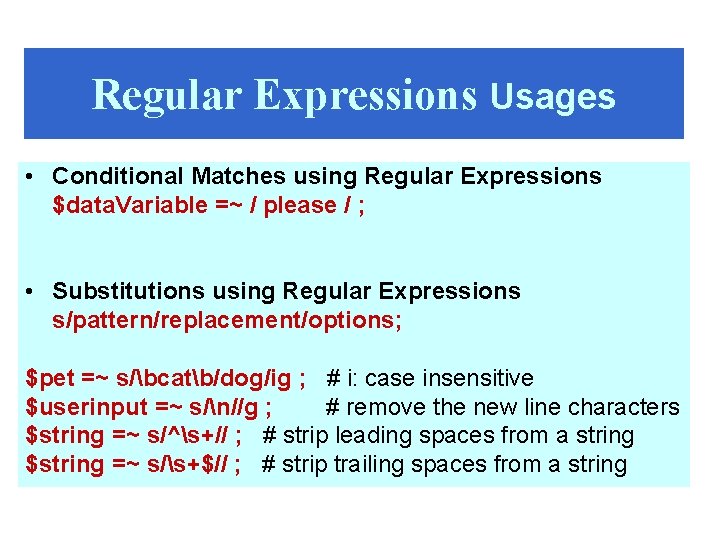
Regular Expressions Usages • Conditional Matches using Regular Expressions $data. Variable =~ / please / ; • Substitutions using Regular Expressions s/pattern/replacement/options; $pet =~ s/bcatb/dog/ig ; # i: case insensitive $userinput =~ s/n//g ; # remove the new line characters $string =~ s/^s+// ; # strip leading spaces from a string $string =~ s/s+$// ; # strip trailing spaces from a string
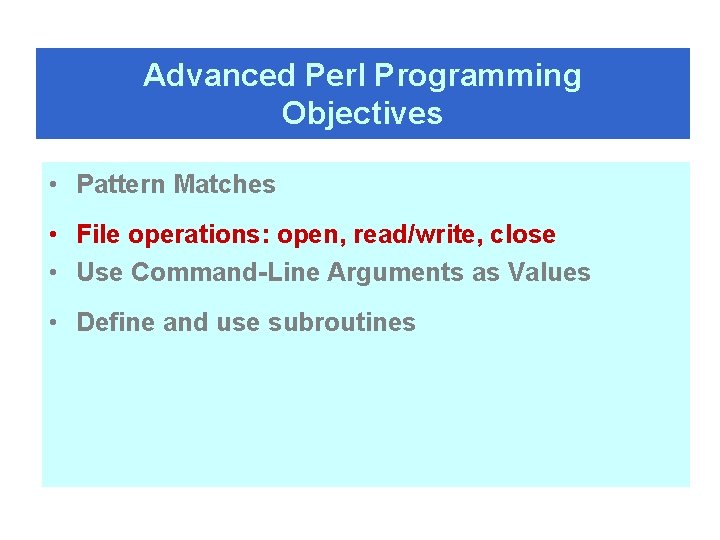
Advanced Perl Programming Objectives • Pattern Matches • File operations: open, read/write, close • Use Command-Line Arguments as Values • Define and use subroutines
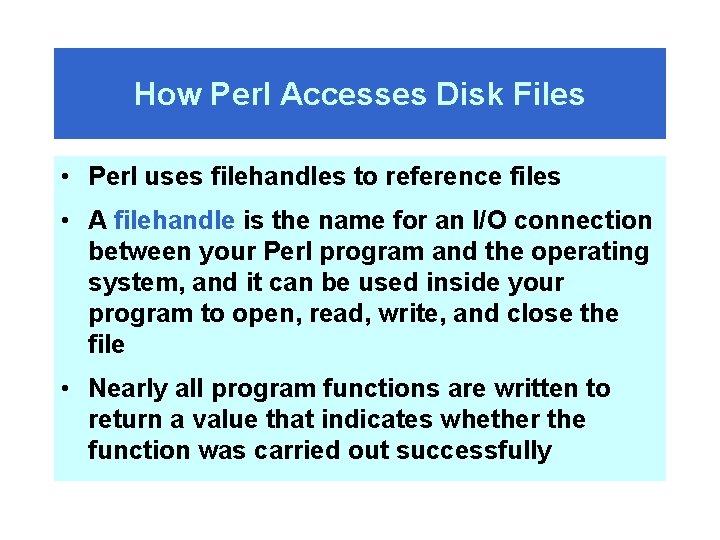
How Perl Accesses Disk Files • Perl uses filehandles to reference files • A filehandle is the name for an I/O connection between your Perl program and the operating system, and it can be used inside your program to open, read, write, and close the file • Nearly all program functions are written to return a value that indicates whether the function was carried out successfully
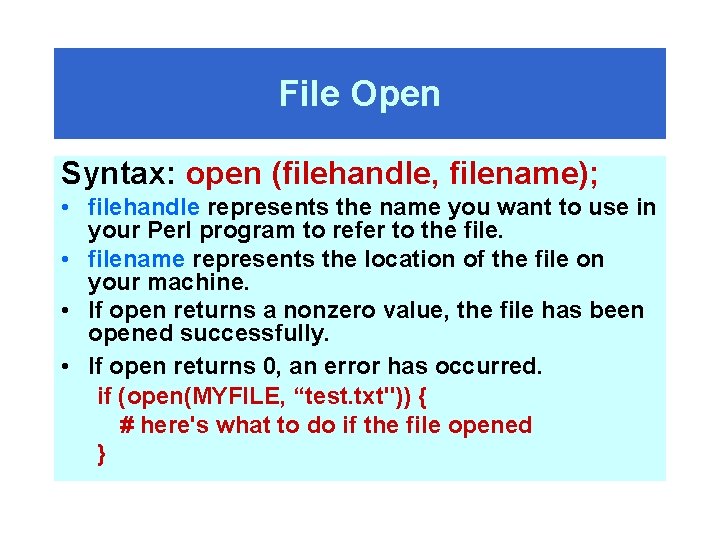
File Open Syntax: open (filehandle, filename); • filehandle represents the name you want to use in your Perl program to refer to the file. • filename represents the location of the file on your machine. • If open returns a nonzero value, the file has been opened successfully. • If open returns 0, an error has occurred. if (open(MYFILE, “test. txt")) { # here's what to do if the file opened }
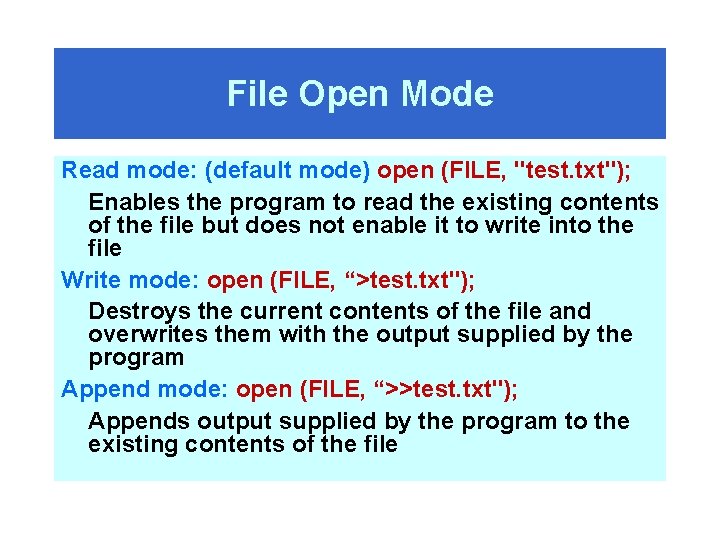
File Open Mode Read mode: (default mode) open (FILE, "test. txt"); Enables the program to read the existing contents of the file but does not enable it to write into the file Write mode: open (FILE, “>test. txt"); Destroys the current contents of the file and overwrites them with the output supplied by the program Append mode: open (FILE, “>>test. txt"); Appends output supplied by the program to the existing contents of the file
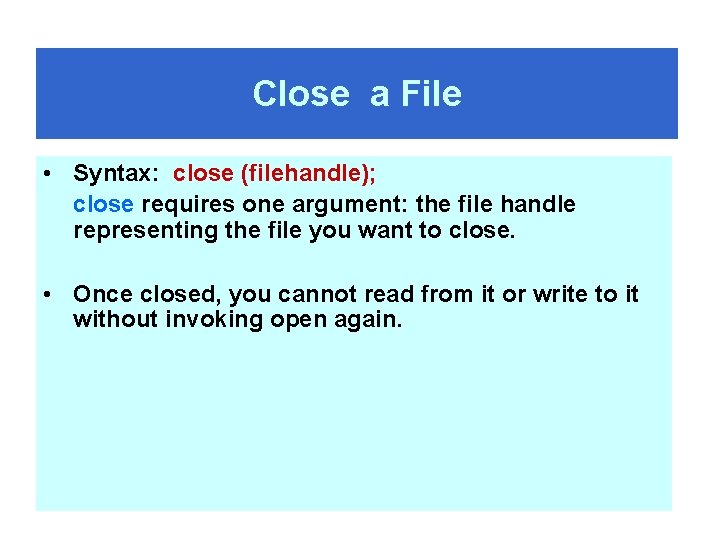
Close a File • Syntax: close (filehandle); close requires one argument: the file handle representing the file you want to close. • Once closed, you cannot read from it or write to it without invoking open again.
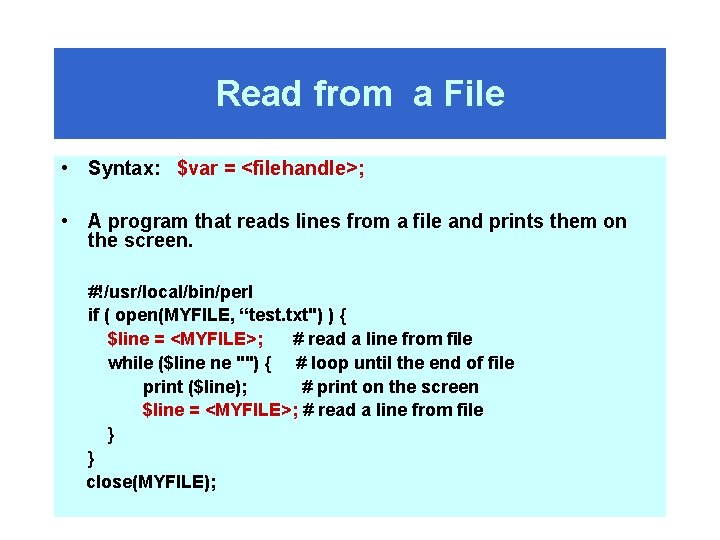
Read from a File • Syntax: $var = <filehandle>; • A program that reads lines from a file and prints them on the screen. #!/usr/local/bin/perl if ( open(MYFILE, “test. txt") ) { $line = <MYFILE>; # read a line from file while ($line ne "") { # loop until the end of file print ($line); # print on the screen $line = <MYFILE>; # read a line from file } } close(MYFILE);
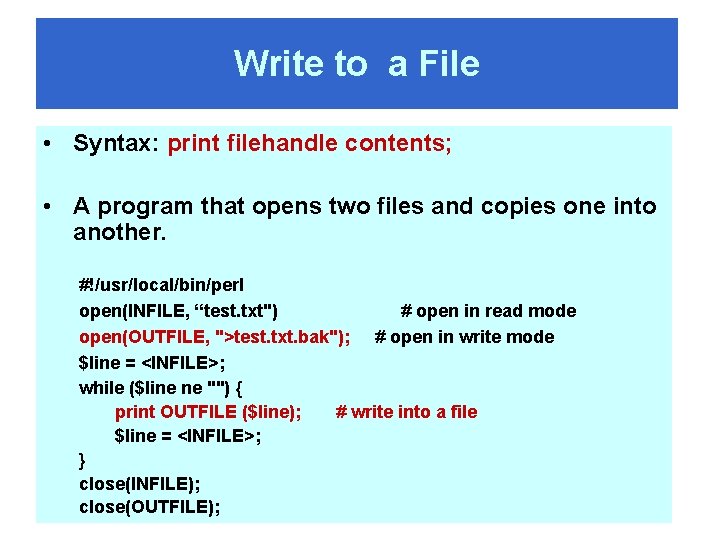
Write to a File • Syntax: print filehandle contents; • A program that opens two files and copies one into another. #!/usr/local/bin/perl open(INFILE, “test. txt") # open in read mode open(OUTFILE, ">test. txt. bak"); # open in write mode $line = <INFILE>; while ($line ne "") { print OUTFILE ($line); # write into a file $line = <INFILE>; } close(INFILE); close(OUTFILE);
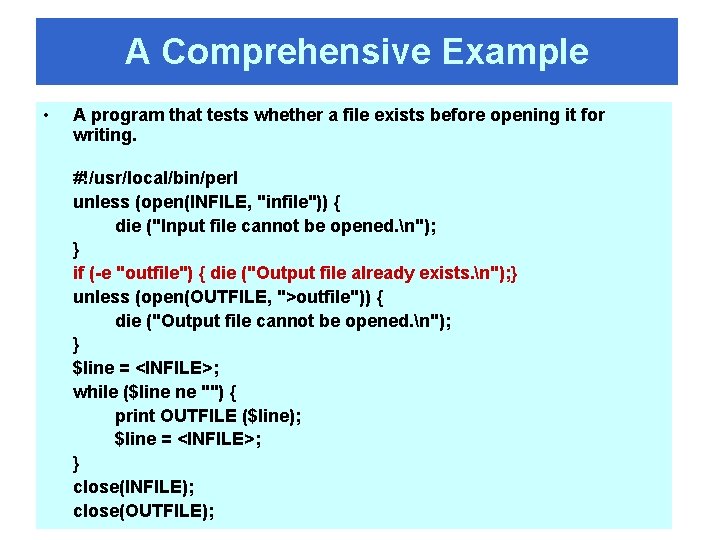
A Comprehensive Example • A program that tests whether a file exists before opening it for writing. #!/usr/local/bin/perl unless (open(INFILE, "infile")) { die ("Input file cannot be opened. n"); } if (-e "outfile") { die ("Output file already exists. n"); } unless (open(OUTFILE, ">outfile")) { die ("Output file cannot be opened. n"); } $line = <INFILE>; while ($line ne "") { print OUTFILE ($line); $line = <INFILE>; } close(INFILE); close(OUTFILE);
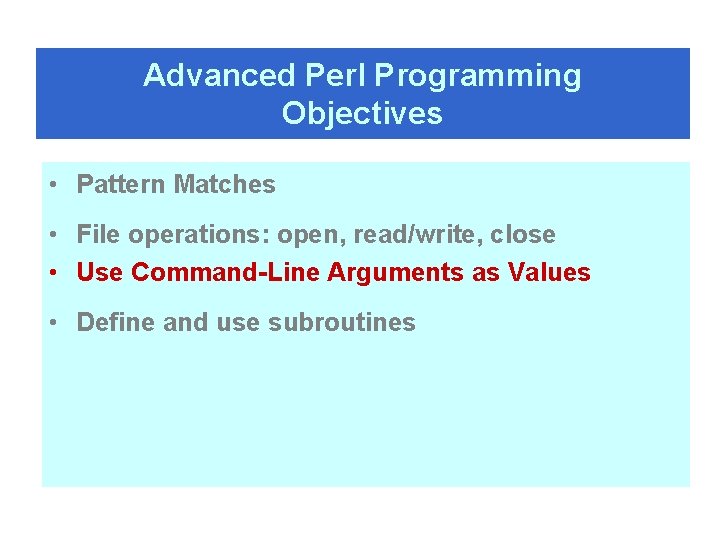
Advanced Perl Programming Objectives • Pattern Matches • File operations: open, read/write, close • Use Command-Line Arguments as Values • Define and use subroutines
![Command Line Arguments Perl stores the commandline arguments in ARGV ARGV0 contains Command Line Arguments • Perl stores the command-line arguments in @ARGV. • $ARGV[0] contains](https://slidetodoc.com/presentation_image_h2/01766f8650dd9cb5a7ada4ba8e909cf8/image-22.jpg)
Command Line Arguments • Perl stores the command-line arguments in @ARGV. • $ARGV[0] contains the first argument, $ARGV[1] contains the second argument, etc • An Example, input. pl: #!/usr/bin/perl $num. Args = $#ARGV + 1; print “You gave $num. Args command-line arguments. n"; foreach $argnum (0. . $#ARGV) { print "$ARGV[$argnum], "; } Running results: $ Input. pl 1 2 3 4 You gave me 4 command-line arguments. 1, 2, 3, 4
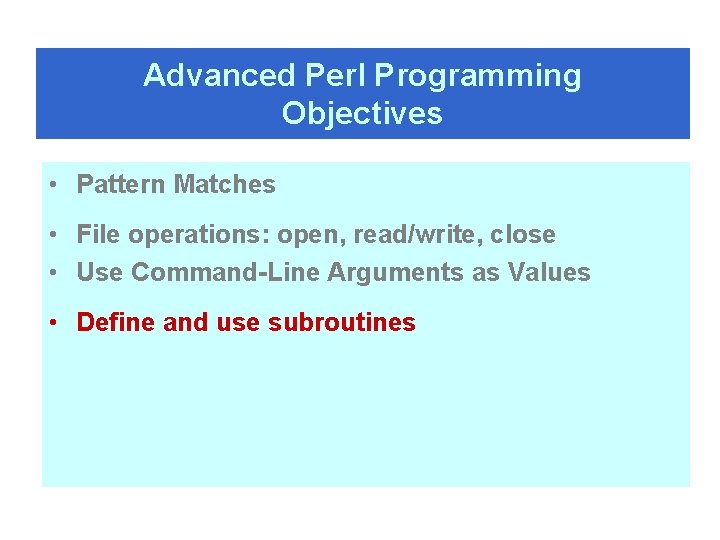
Advanced Perl Programming Objectives • Pattern Matches • File operations: open, read/write, close • Use Command-Line Arguments as Values • Define and use subroutines
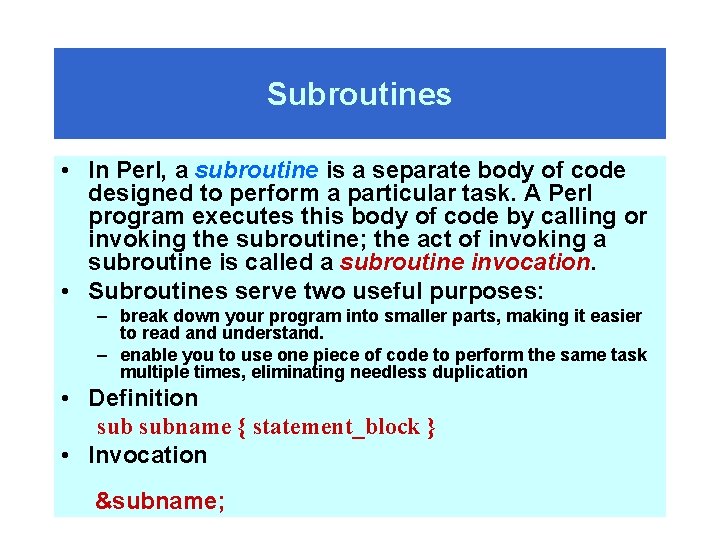
Subroutines • In Perl, a subroutine is a separate body of code designed to perform a particular task. A Perl program executes this body of code by calling or invoking the subroutine; the act of invoking a subroutine is called a subroutine invocation. • Subroutines serve two useful purposes: – break down your program into smaller parts, making it easier to read and understand. – enable you to use one piece of code to perform the same task multiple times, eliminating needless duplication • Definition subname { statement_block } • Invocation &subname;
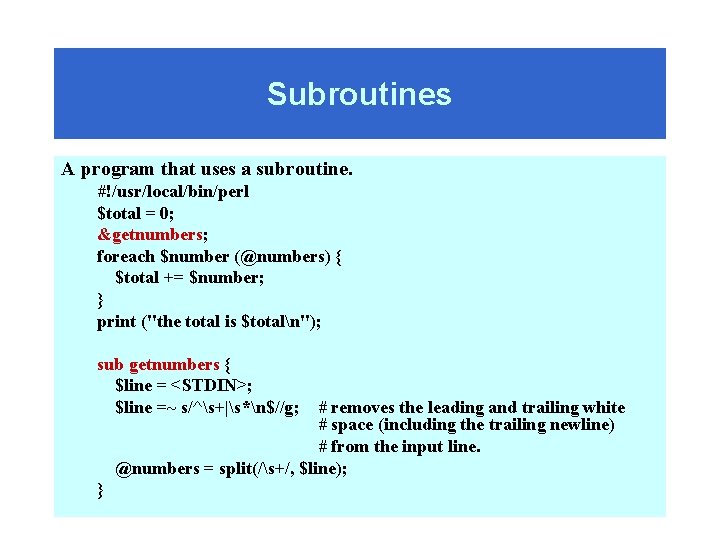
Subroutines A program that uses a subroutine. #!/usr/local/bin/perl $total = 0; &getnumbers; foreach $number (@numbers) { $total += $number; } print ("the total is $totaln"); sub getnumbers { $line = <STDIN>; $line =~ s/^s+|s*n$//g; # removes the leading and trailing white # space (including the trailing newline) # from the input line. @numbers = split(/s+/, $line); }
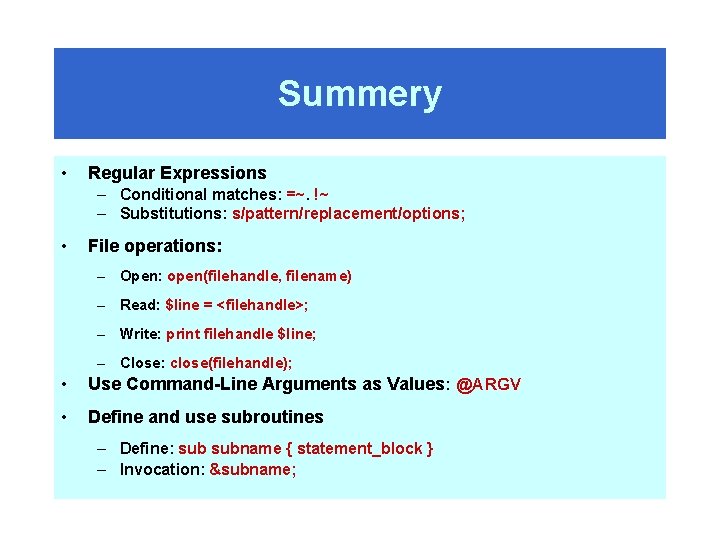
Summery • Regular Expressions – Conditional matches: =~. !~ – Substitutions: s/pattern/replacement/options; • File operations: – Open: open(filehandle, filename) – Read: $line = <filehandle>; – Write: print filehandle $line; – Close: close(filehandle); • Use Command-Line Arguments as Values: @ARGV • Define and use subroutines – Define: subname { statement_block } – Invocation: &subname;
Perl cgi programming
Cgi linkage in perl
Chromosome example in real life
Advanced dynamic programming
Advanced internet programming
What is imperative statement in assembly language
Language processor
Advanced programming in java
Sometimes you win some sometimes you lose some
Sometimes you win some sometimes you lose some
Cake countable or uncountable noun
What are some contact forces and some noncontact forces
Some say the world will end in fire some say in ice
Some say the world will end in fire some say in ice
Some may trust in horses
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Windows 10 system programming, part 1
Linear vs integer programming
Definisi integer
Verb patterns: object + infinitive
Traction extension
Click anywhere to continue
Beschrijvende observatie
Fondazioni discontinue
Strisce longitudinali
Ligne d'identification soudure