Chapter 6 Synchronization April 2013 Jongmoo Choi Dept
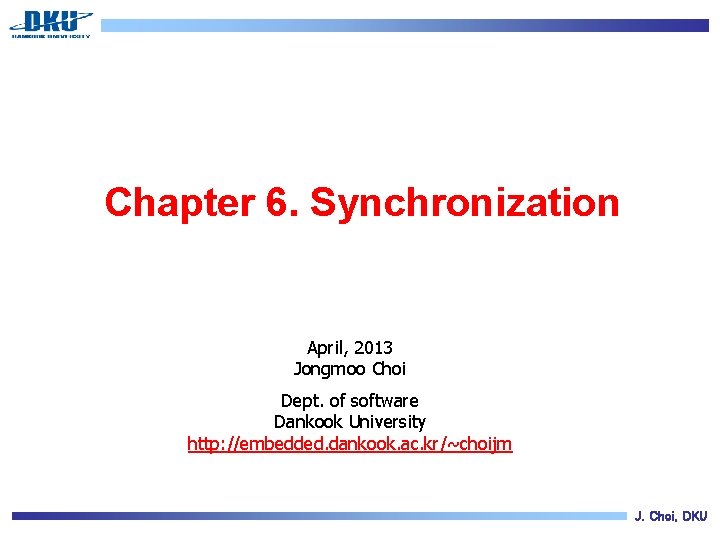
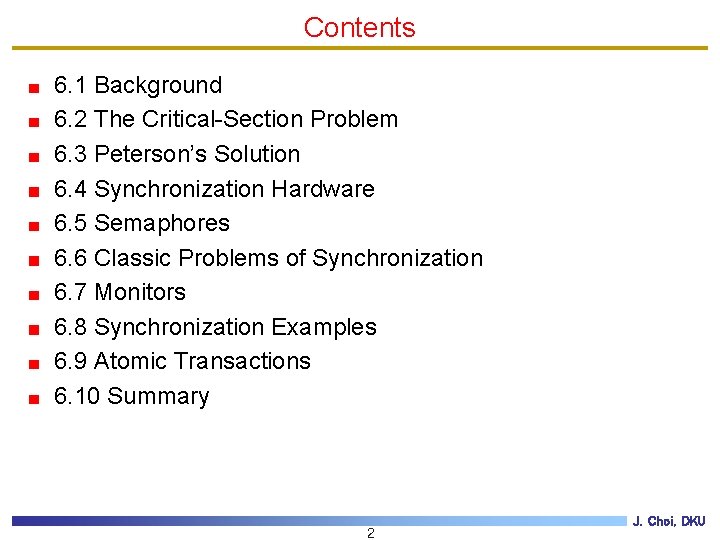
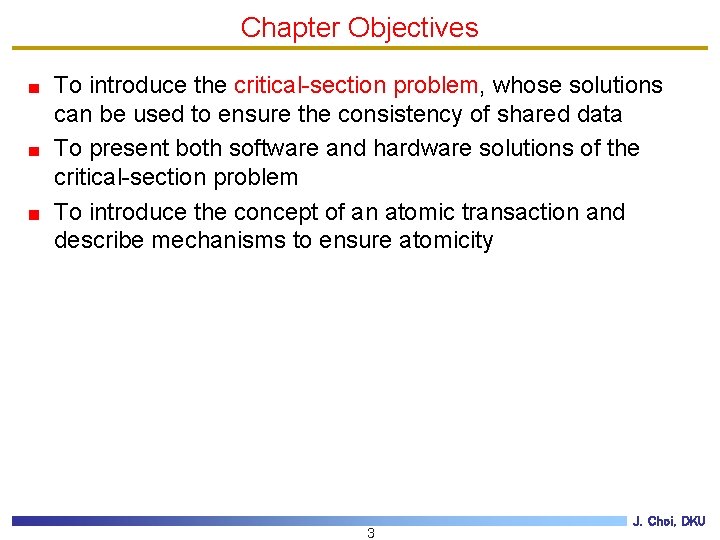
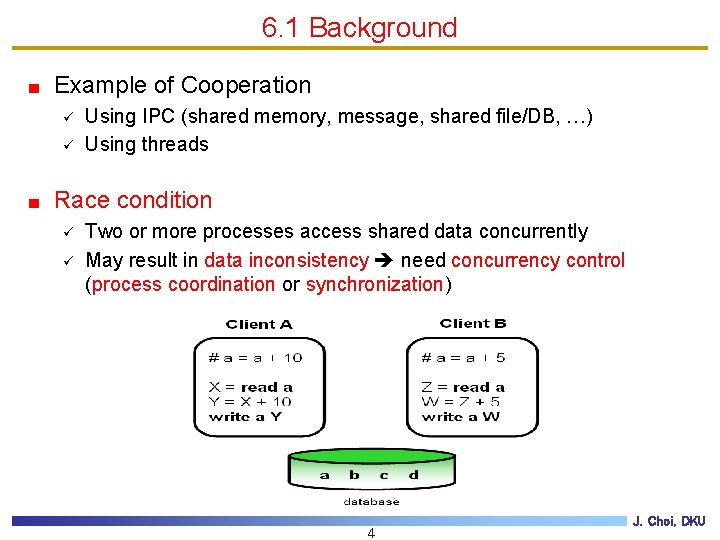
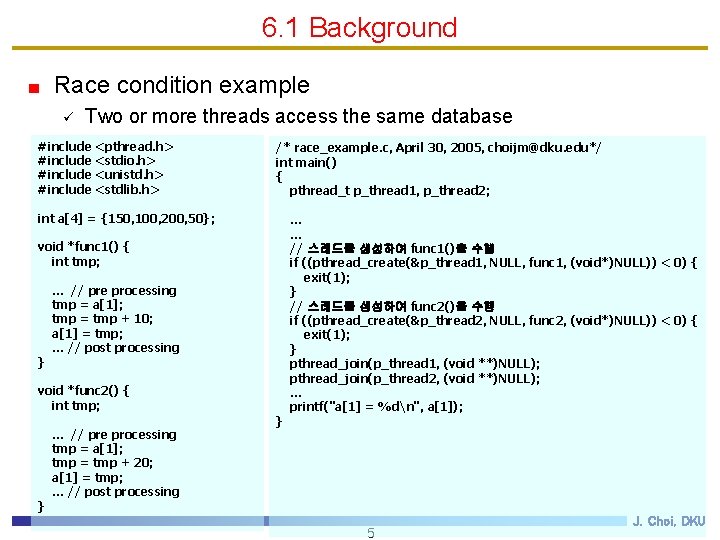
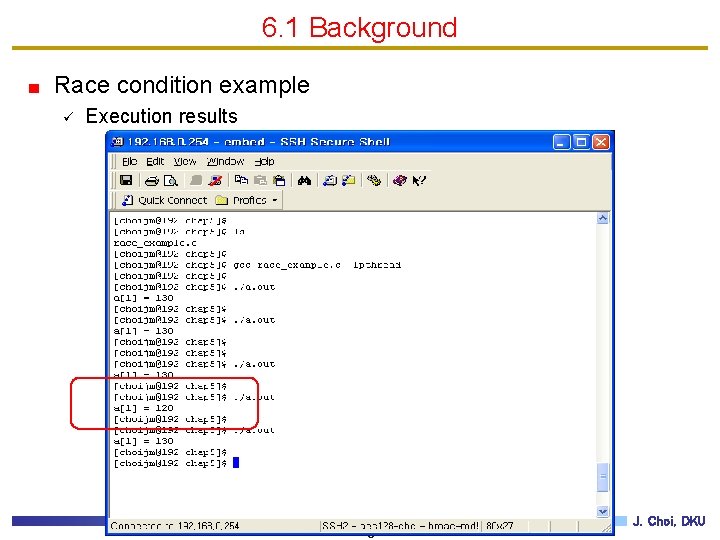
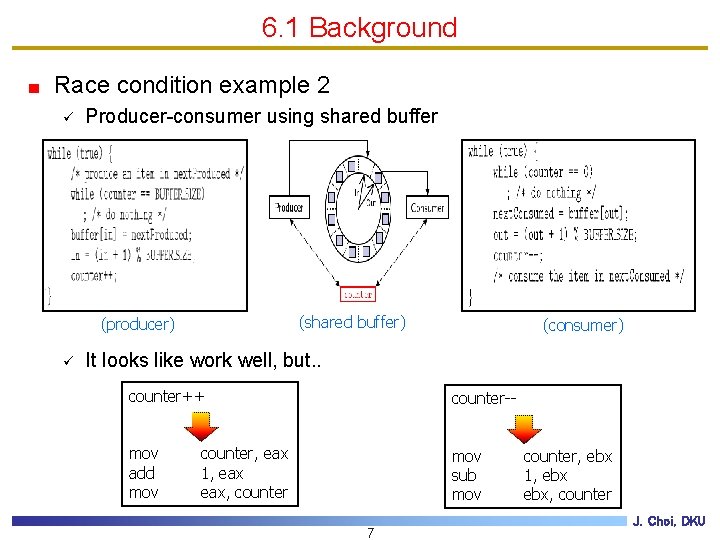
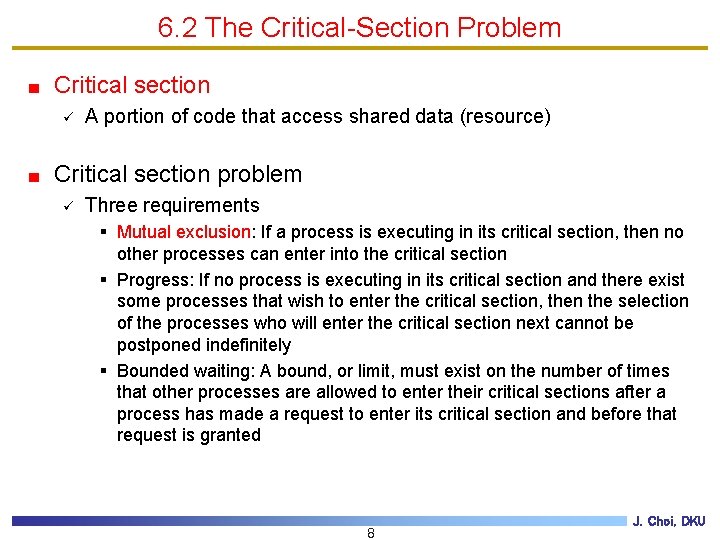
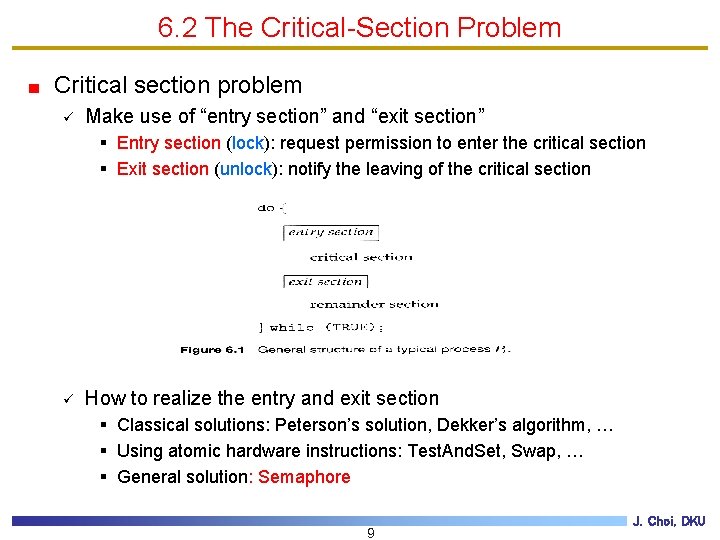
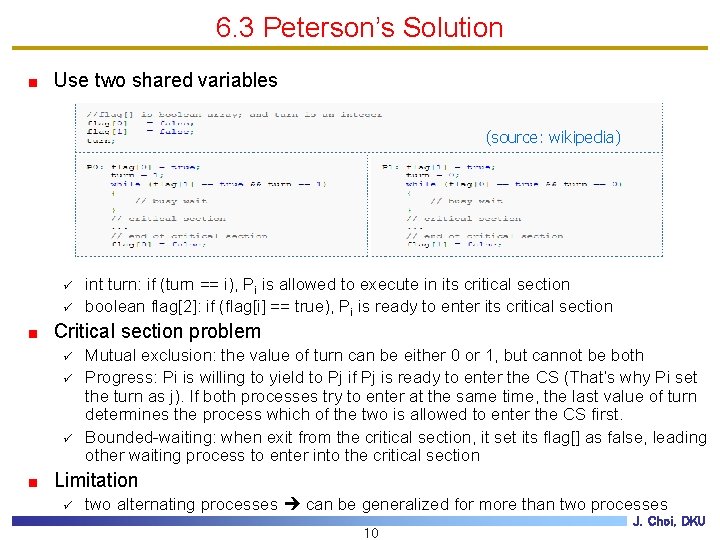
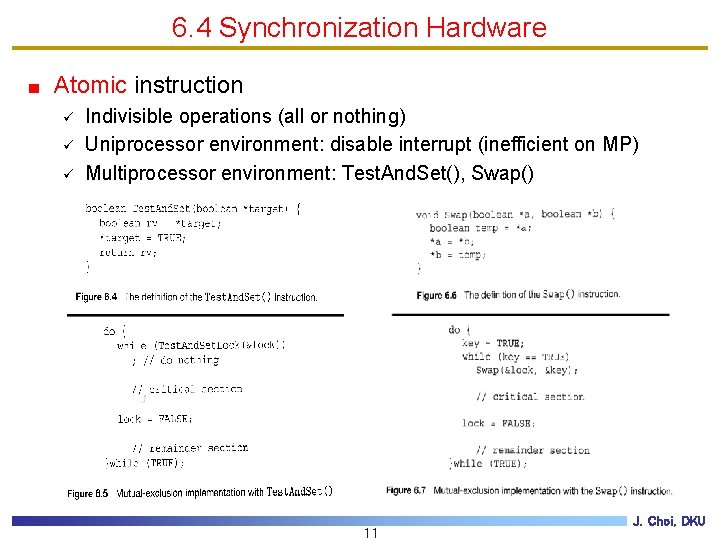
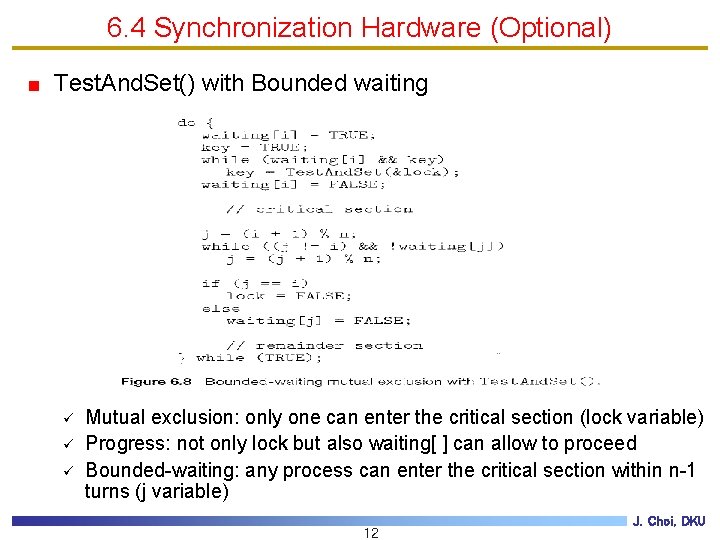
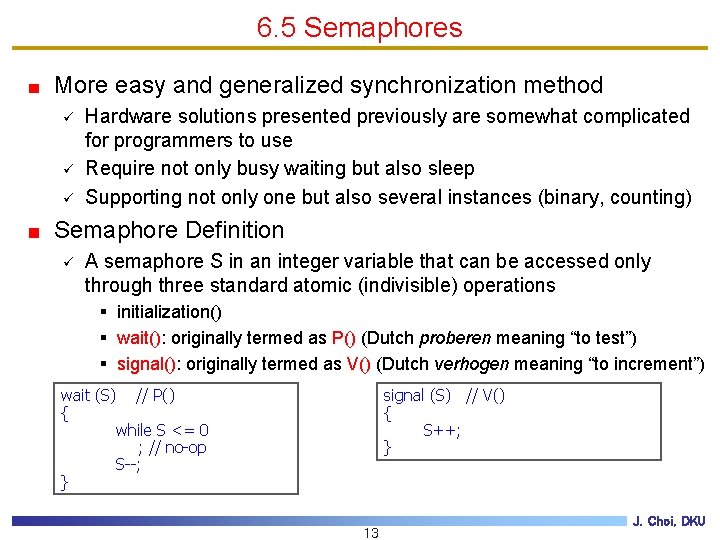
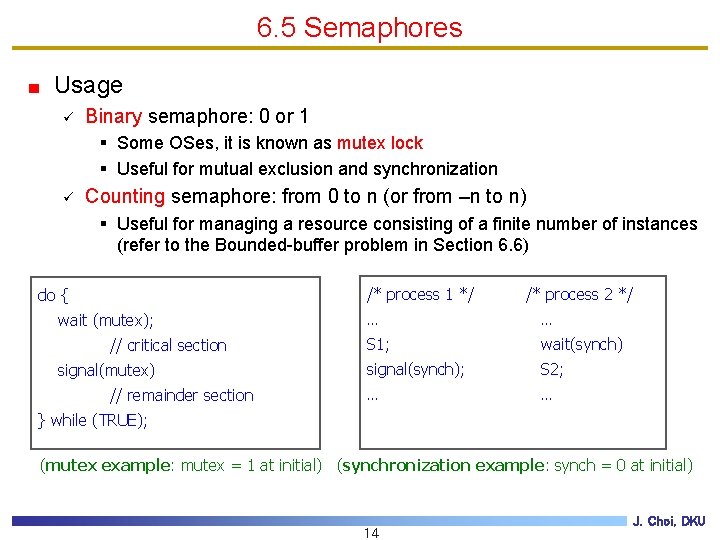
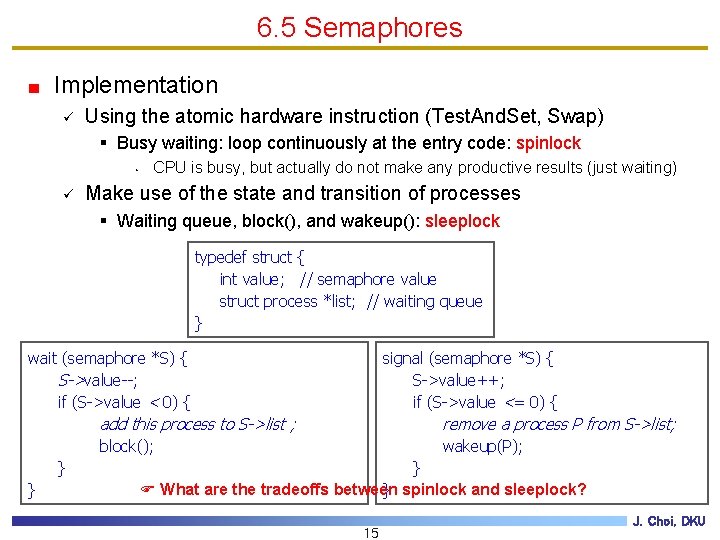
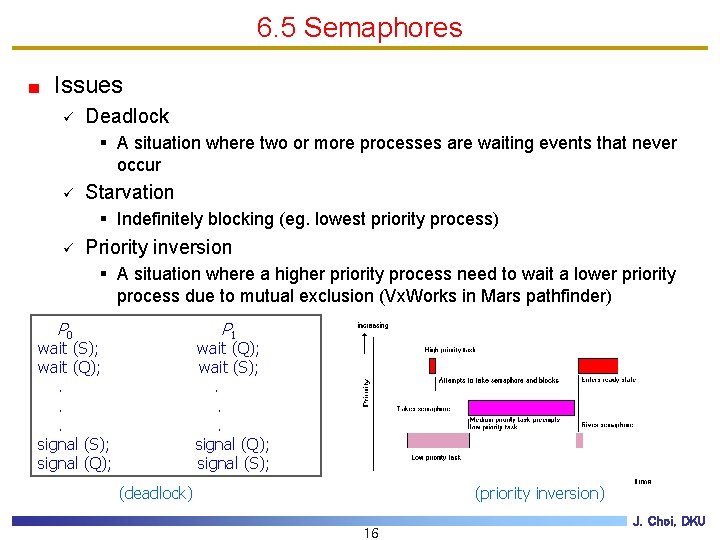
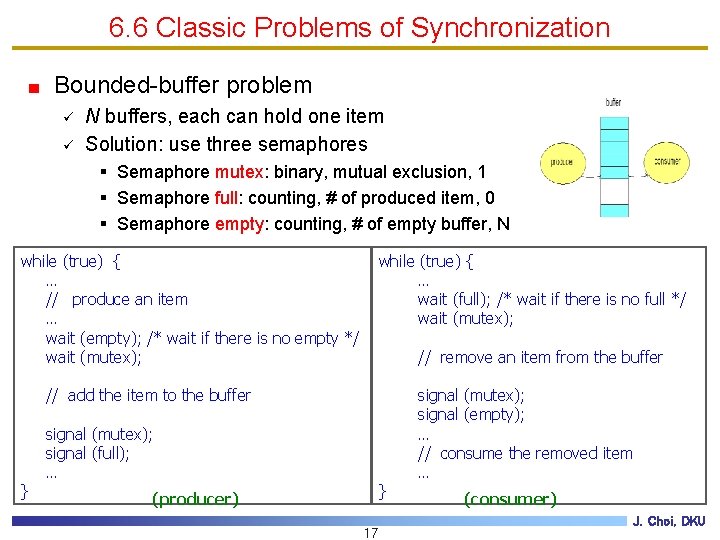
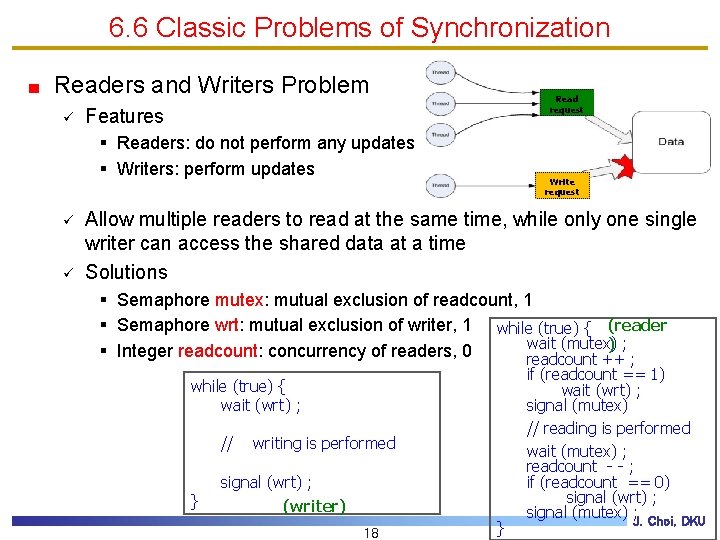
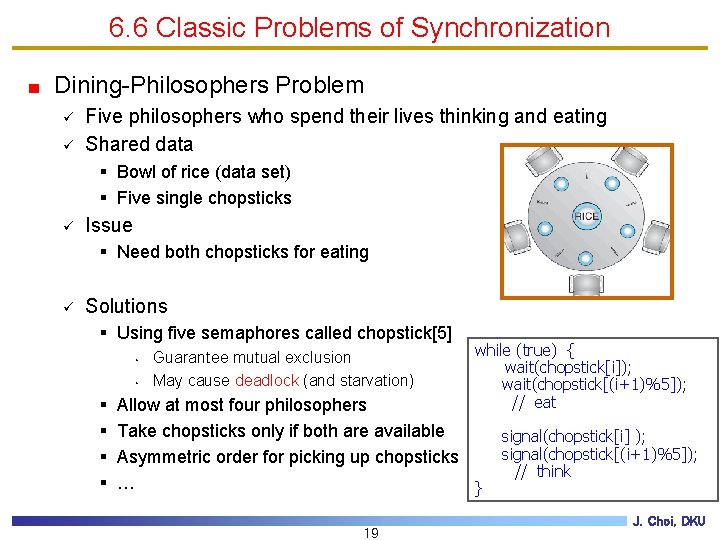
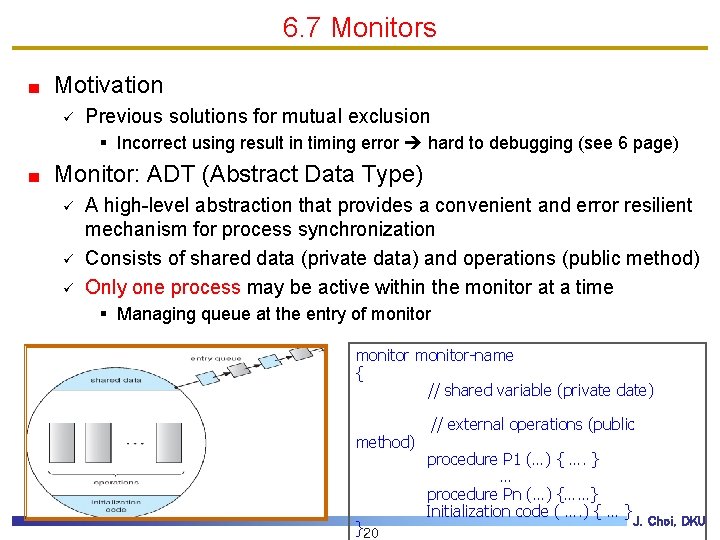
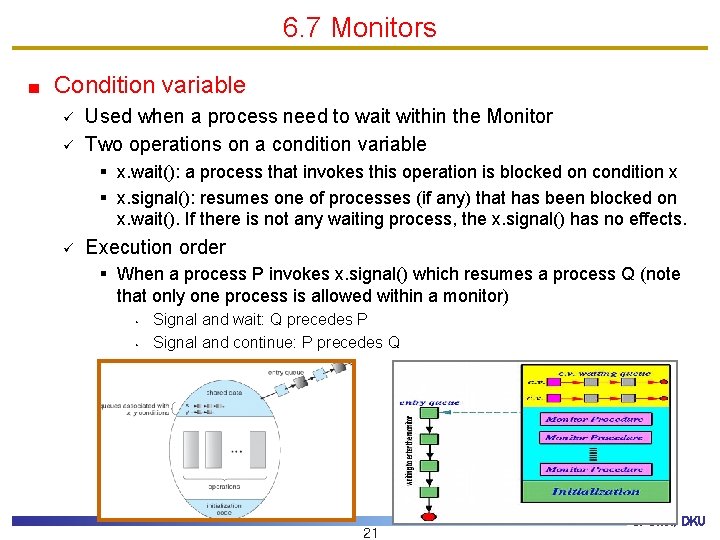
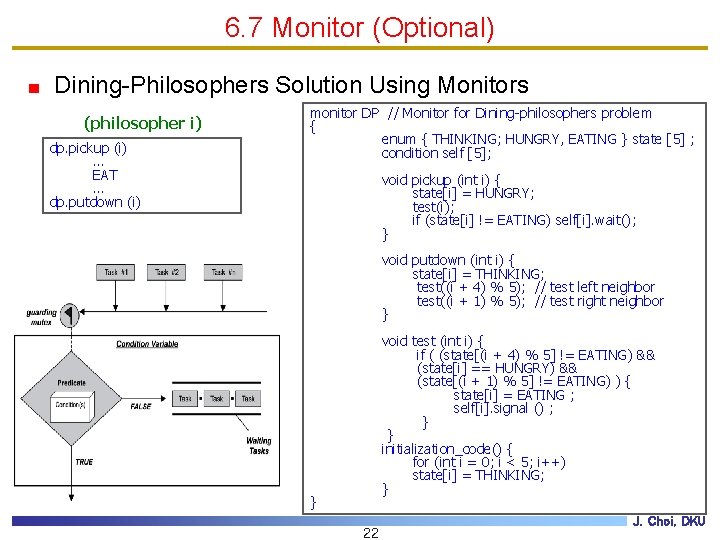
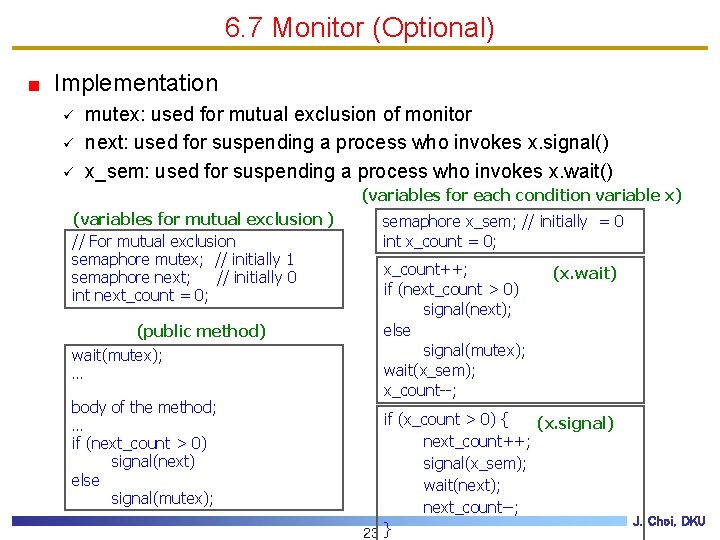
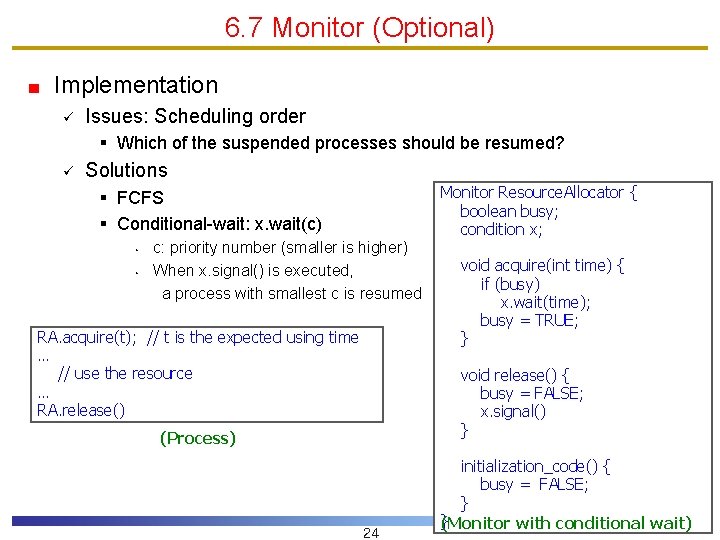
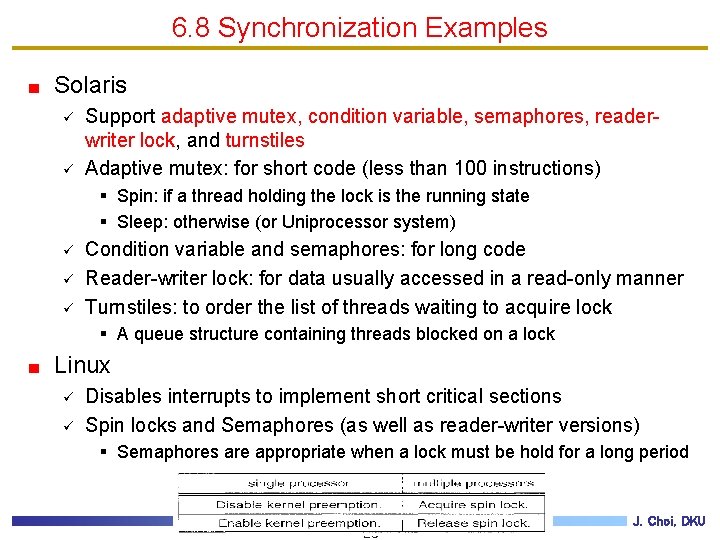
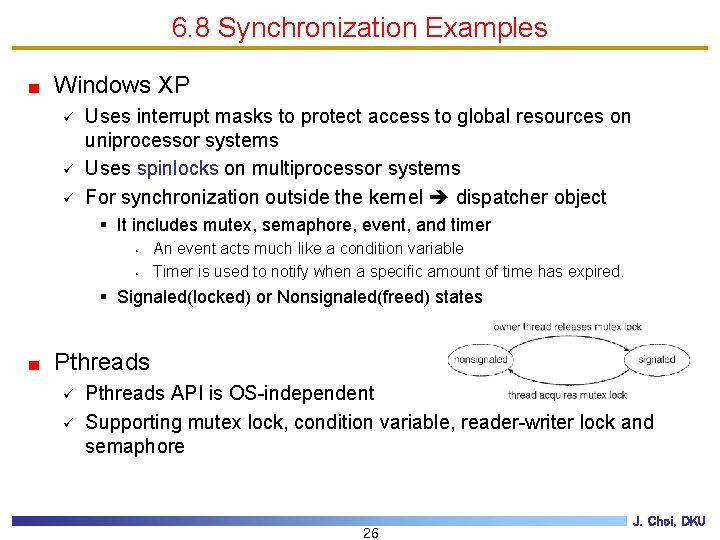
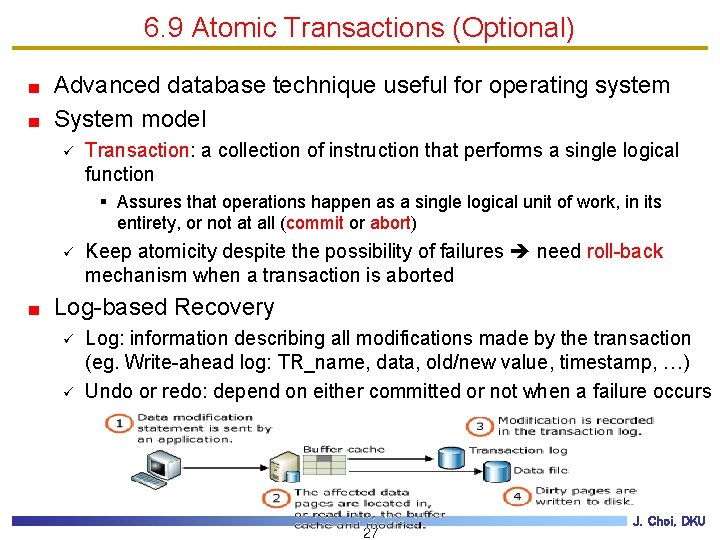
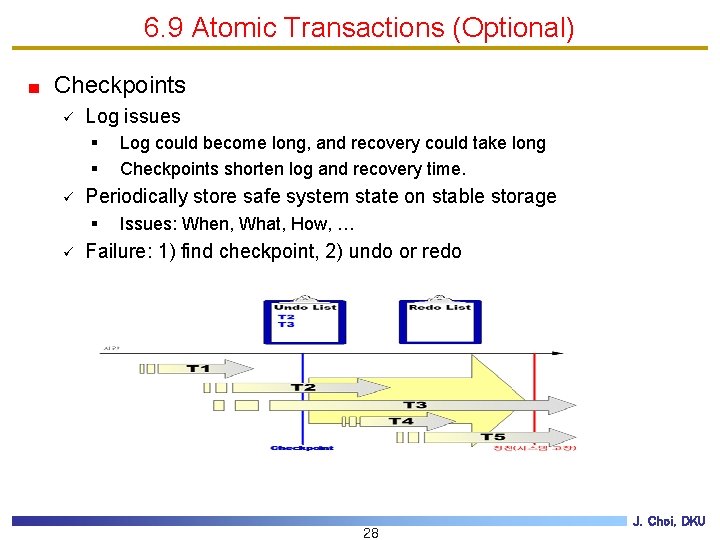
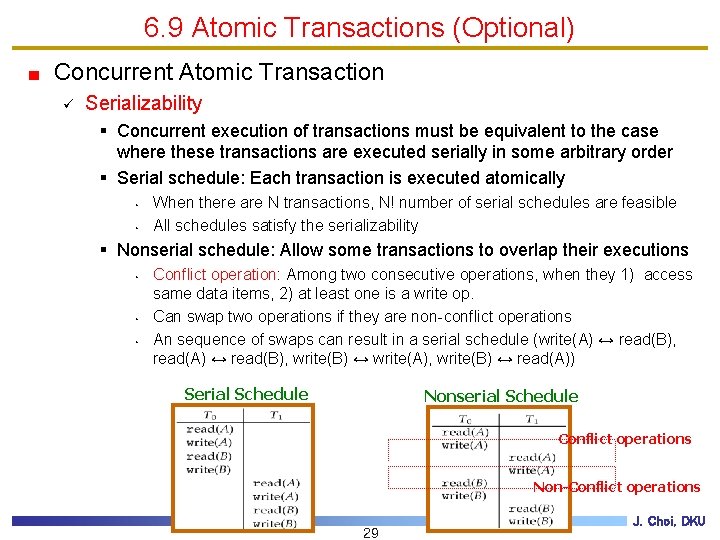
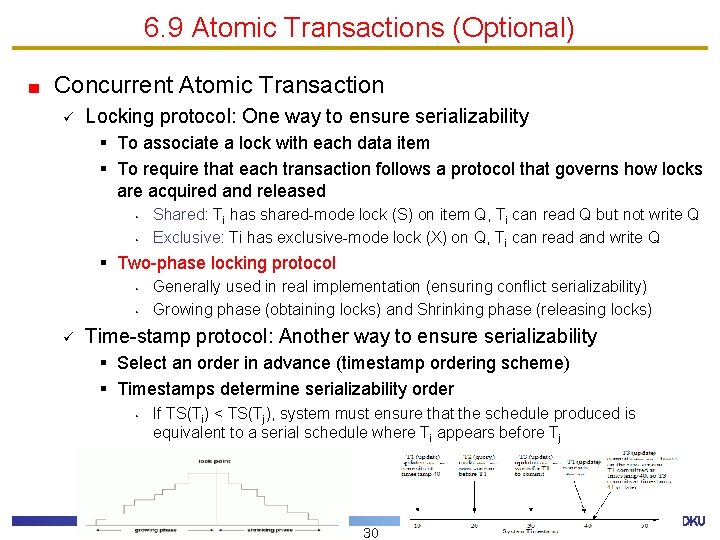
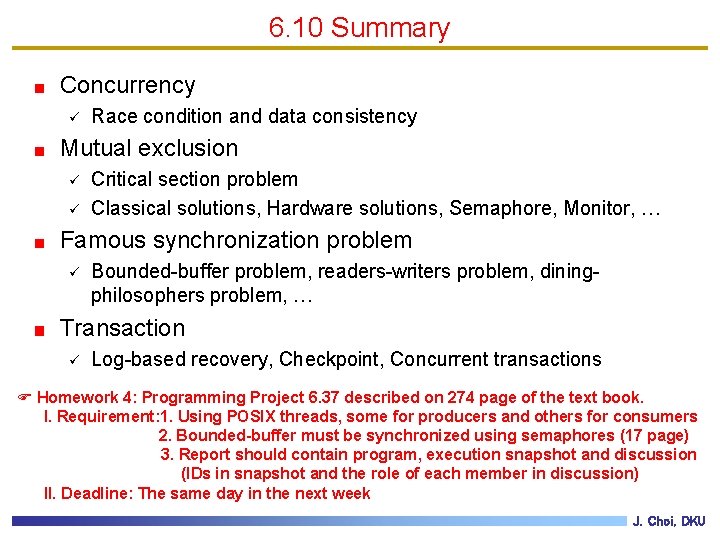
- Slides: 31
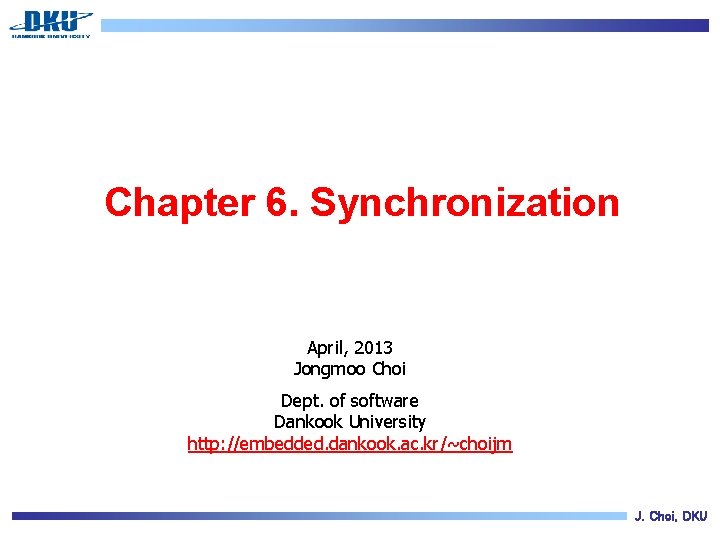
Chapter 6. Synchronization April, 2013 Jongmoo Choi Dept. of software Dankook University http: //embedded. dankook. ac. kr/~choijm J. Choi, DKU
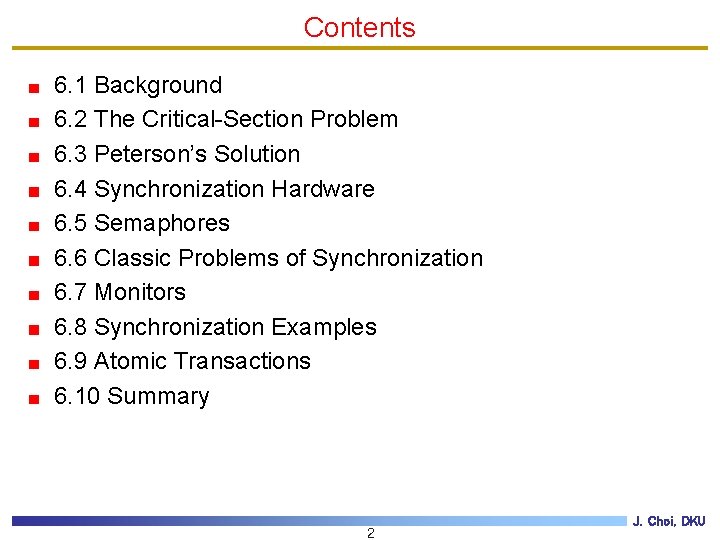
Contents 6. 1 Background 6. 2 The Critical-Section Problem 6. 3 Peterson’s Solution 6. 4 Synchronization Hardware 6. 5 Semaphores 6. 6 Classic Problems of Synchronization 6. 7 Monitors 6. 8 Synchronization Examples 6. 9 Atomic Transactions 6. 10 Summary 2 J. Choi, DKU
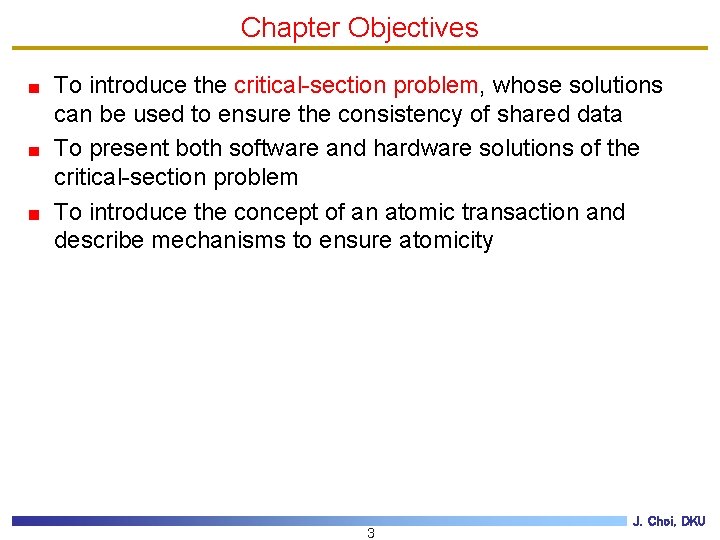
Chapter Objectives To introduce the critical-section problem, whose solutions can be used to ensure the consistency of shared data To present both software and hardware solutions of the critical-section problem To introduce the concept of an atomic transaction and describe mechanisms to ensure atomicity 3 J. Choi, DKU
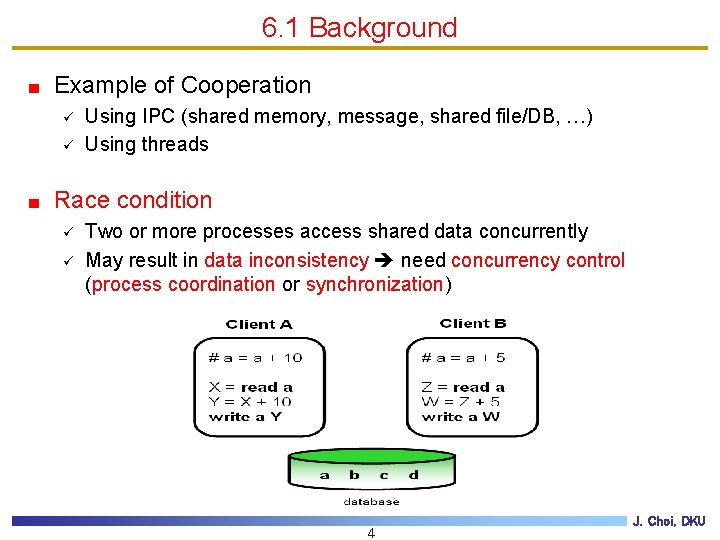
6. 1 Background Example of Cooperation ü ü Using IPC (shared memory, message, shared file/DB, …) Using threads Race condition ü ü Two or more processes access shared data concurrently May result in data inconsistency need concurrency control (process coordination or synchronization) 4 J. Choi, DKU
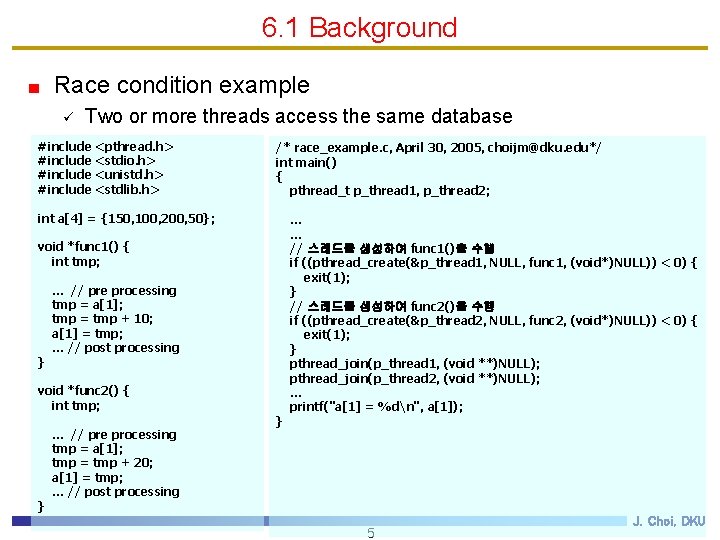
6. 1 Background Race condition example ü Two or more threads access the same database #include <pthread. h> #include <stdio. h> #include <unistd. h> #include <stdlib. h> /* race_example. c, April 30, 2005, choijm@dku. edu*/ int main() { pthread_t p_thread 1, p_thread 2; int a[4] = {150, 100, 200, 50}; void *func 1() { int tmp; } … // pre processing tmp = a[1]; tmp = tmp + 10; a[1] = tmp; … // post processing void *func 2() { int tmp; } … // pre processing tmp = a[1]; tmp = tmp + 20; a[1] = tmp; … // post processing } … … // 스레드를 생성하여 func 1()을 수행 if ((pthread_create(&p_thread 1, NULL, func 1, (void*)NULL)) < 0) { exit(1); } // 스레드를 생성하여 func 2()을 수행 if ((pthread_create(&p_thread 2, NULL, func 2, (void*)NULL)) < 0) { exit(1); } pthread_join(p_thread 1, (void **)NULL); pthread_join(p_thread 2, (void **)NULL); … printf("a[1] = %dn", a[1]); 5 J. Choi, DKU
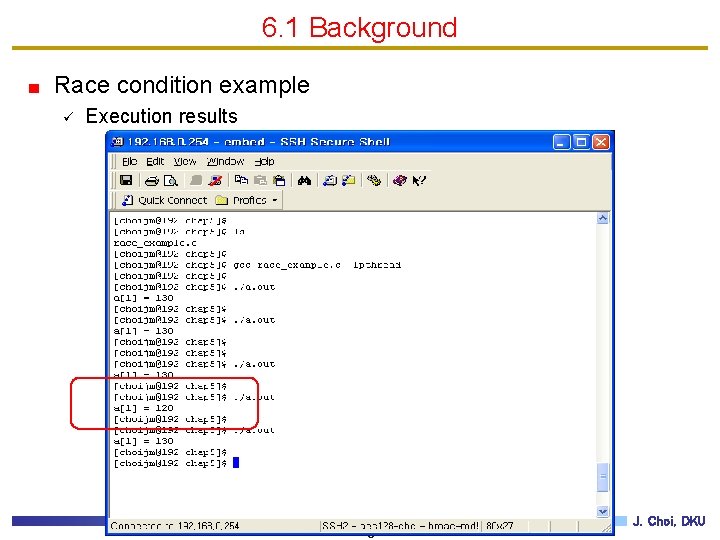
6. 1 Background Race condition example ü Execution results 6 J. Choi, DKU
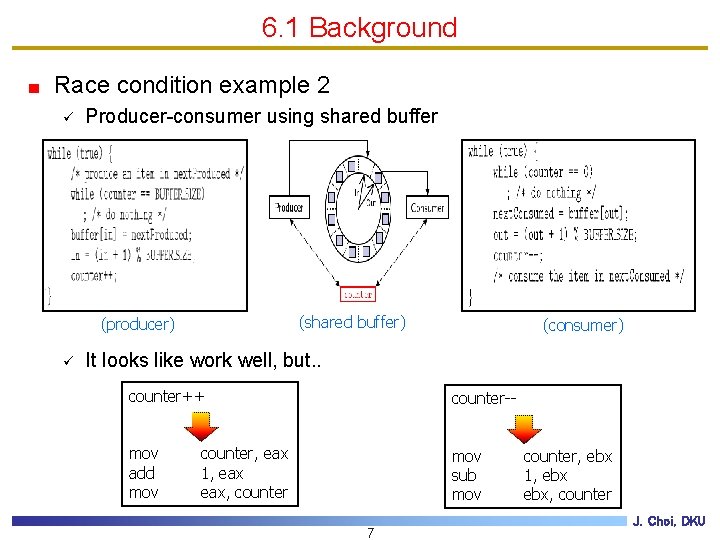
6. 1 Background Race condition example 2 ü Producer-consumer using shared buffer (shared buffer) (producer) ü (consumer) It looks like work well, but. . counter++ counter-- mov add mov sub mov counter, eax 1, eax, counter 7 counter, ebx 1, ebx, counter J. Choi, DKU
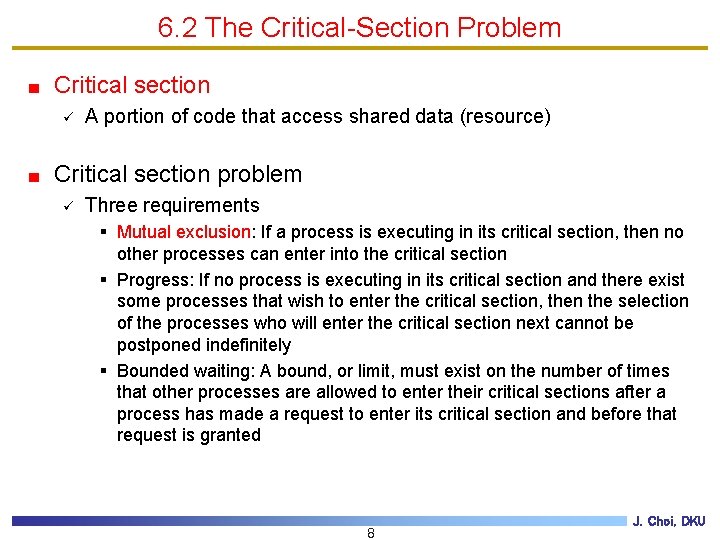
6. 2 The Critical-Section Problem Critical section ü A portion of code that access shared data (resource) Critical section problem ü Three requirements § Mutual exclusion: If a process is executing in its critical section, then no other processes can enter into the critical section § Progress: If no process is executing in its critical section and there exist some processes that wish to enter the critical section, then the selection of the processes who will enter the critical section next cannot be postponed indefinitely § Bounded waiting: A bound, or limit, must exist on the number of times that other processes are allowed to enter their critical sections after a process has made a request to enter its critical section and before that request is granted 8 J. Choi, DKU
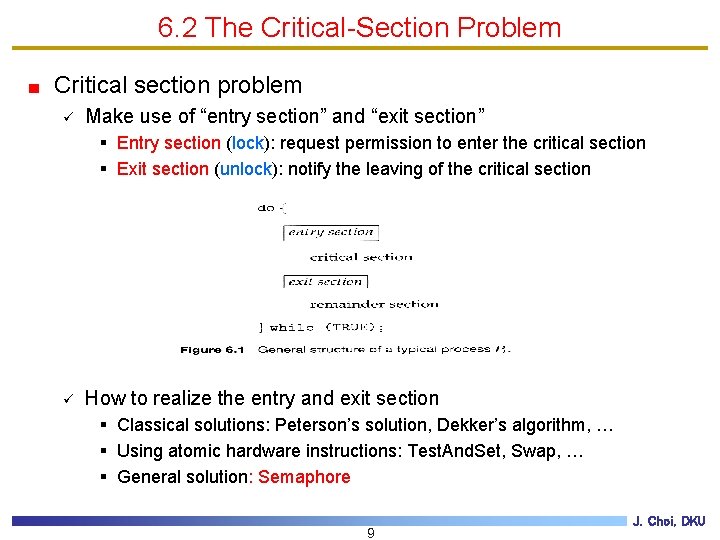
6. 2 The Critical-Section Problem Critical section problem ü Make use of “entry section” and “exit section” § Entry section (lock): request permission to enter the critical section § Exit section (unlock): notify the leaving of the critical section ü How to realize the entry and exit section § Classical solutions: Peterson’s solution, Dekker’s algorithm, … § Using atomic hardware instructions: Test. And. Set, Swap, … § General solution: Semaphore 9 J. Choi, DKU
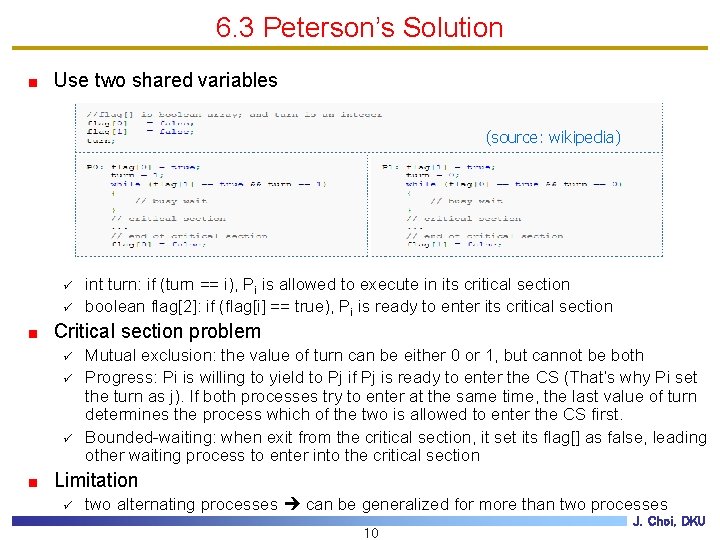
6. 3 Peterson’s Solution Use two shared variables (source: wikipedia) ü ü int turn: if (turn == i), Pi is allowed to execute in its critical section boolean flag[2]: if (flag[i] == true), Pi is ready to enter its critical section Critical section problem ü ü ü Mutual exclusion: the value of turn can be either 0 or 1, but cannot be both Progress: Pi is willing to yield to Pj if Pj is ready to enter the CS (That’s why Pi set the turn as j). If both processes try to enter at the same time, the last value of turn determines the process which of the two is allowed to enter the CS first. Bounded-waiting: when exit from the critical section, it set its flag[] as false, leading other waiting process to enter into the critical section Limitation ü two alternating processes can be generalized for more than two processes 10 J. Choi, DKU
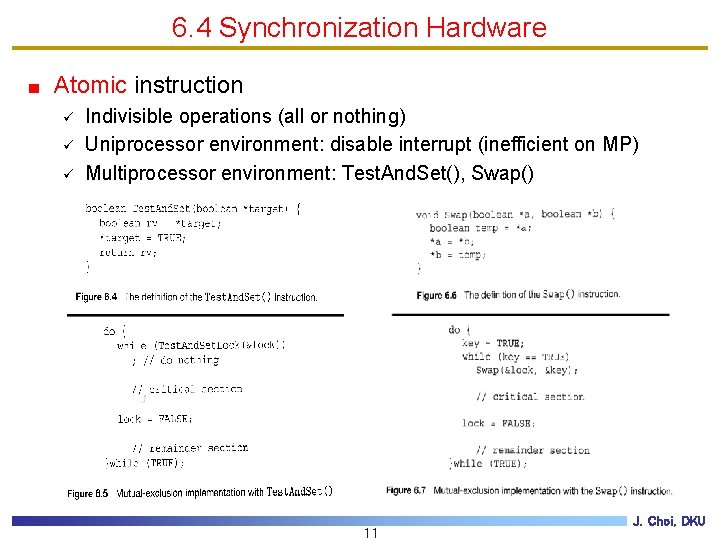
6. 4 Synchronization Hardware Atomic instruction ü ü ü Indivisible operations (all or nothing) Uniprocessor environment: disable interrupt (inefficient on MP) Multiprocessor environment: Test. And. Set(), Swap() 11 J. Choi, DKU
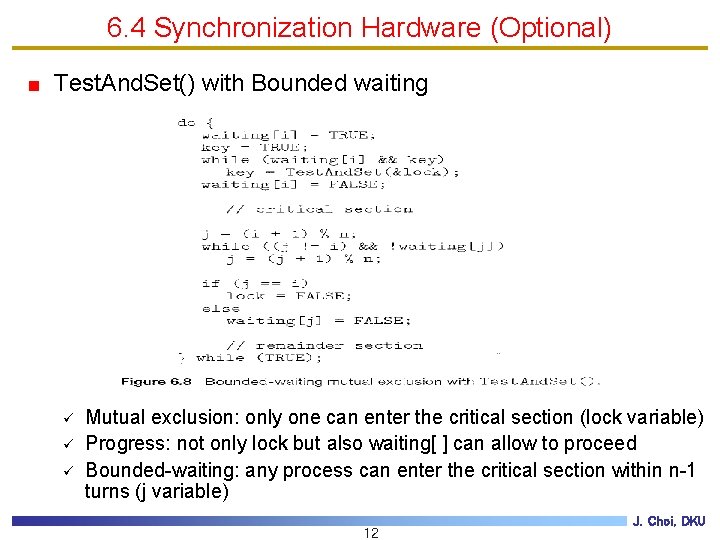
6. 4 Synchronization Hardware (Optional) Test. And. Set() with Bounded waiting ü ü ü Mutual exclusion: only one can enter the critical section (lock variable) Progress: not only lock but also waiting[ ] can allow to proceed Bounded-waiting: any process can enter the critical section within n-1 turns (j variable) 12 J. Choi, DKU
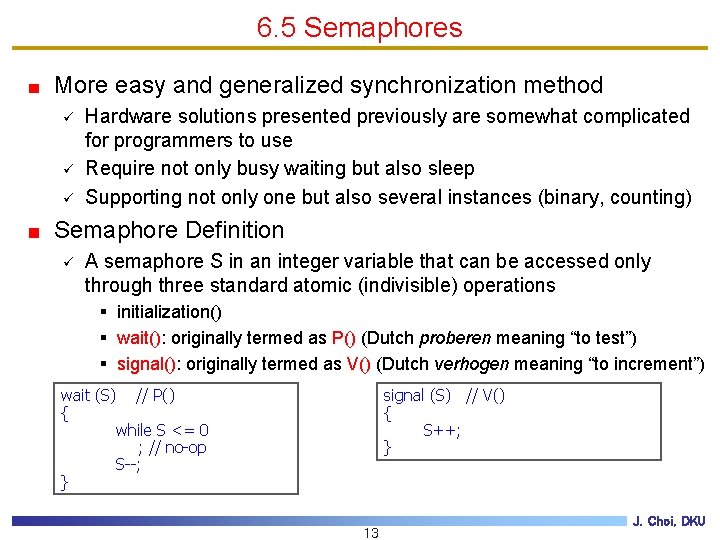
6. 5 Semaphores More easy and generalized synchronization method ü ü ü Hardware solutions presented previously are somewhat complicated for programmers to use Require not only busy waiting but also sleep Supporting not only one but also several instances (binary, counting) Semaphore Definition ü A semaphore S in an integer variable that can be accessed only through three standard atomic (indivisible) operations § initialization() § wait(): originally termed as P() (Dutch proberen meaning “to test”) § signal(): originally termed as V() (Dutch verhogen meaning “to increment”) signal (S) // V() { S++; } wait (S) // P() { while S <= 0 ; // no-op S--; } 13 J. Choi, DKU
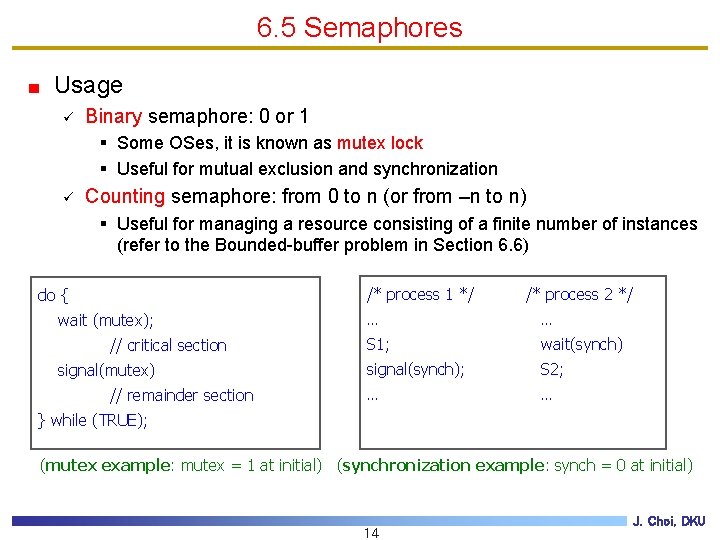
6. 5 Semaphores Usage ü Binary semaphore: 0 or 1 § Some OSes, it is known as mutex lock § Useful for mutual exclusion and synchronization ü Counting semaphore: from 0 to n (or from –n to n) § Useful for managing a resource consisting of a finite number of instances (refer to the Bounded-buffer problem in Section 6. 6) /* process 1 */ do { wait (mutex); // critical section signal(mutex) // remainder section /* process 2 */ … … S 1; wait(synch) signal(synch); S 2; … … } while (TRUE); (mutex example: mutex = 1 at initial) (synchronization example: synch = 0 at initial) 14 J. Choi, DKU
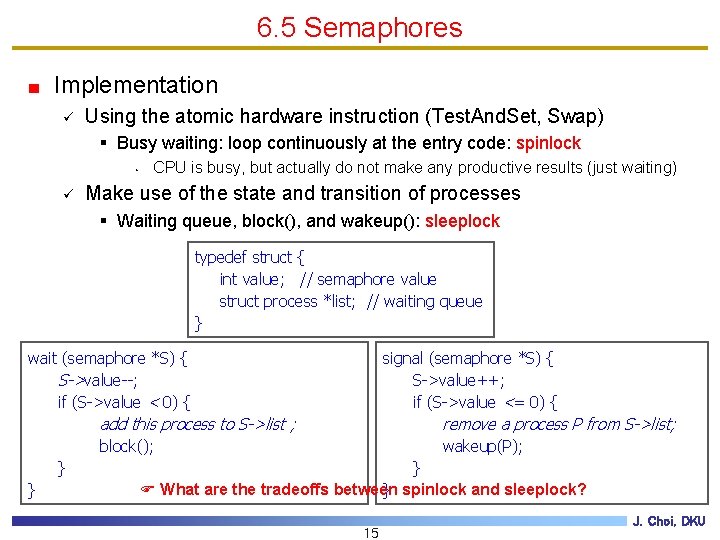
6. 5 Semaphores Implementation ü Using the atomic hardware instruction (Test. And. Set, Swap) § Busy waiting: loop continuously at the entry code: spinlock • ü CPU is busy, but actually do not make any productive results (just waiting) Make use of the state and transition of processes § Waiting queue, block(), and wakeup(): sleeplock typedef struct { int value; // semaphore value struct process *list; // waiting queue } wait (semaphore *S) { S->value--; if (S->value < 0) { } } signal (semaphore *S) { S->value++; if (S->value <= 0) { add this process to S->list ; remove a process P from S->list; block(); wakeup(P); } F What are the tradeoffs between } spinlock and sleeplock? 15 J. Choi, DKU
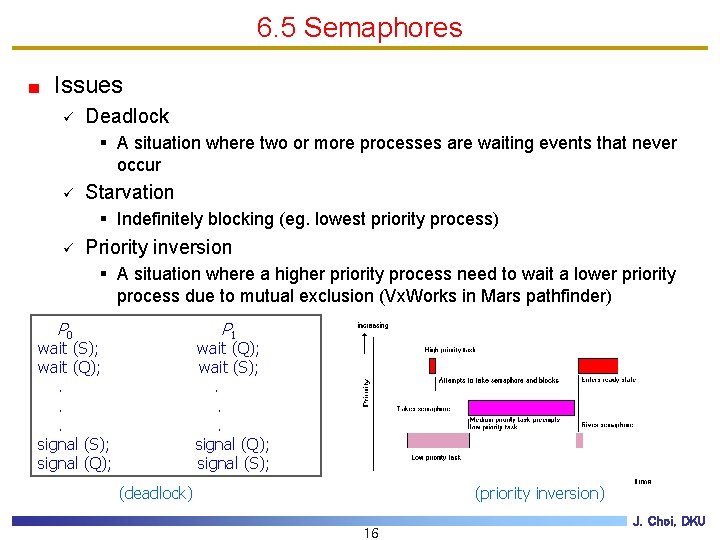
6. 5 Semaphores Issues ü Deadlock § A situation where two or more processes are waiting events that never occur ü Starvation § Indefinitely blocking (eg. lowest priority process) ü Priority inversion § A situation where a higher priority process need to wait a lower priority process due to mutual exclusion (Vx. Works in Mars pathfinder) P 0 P 1 wait (S); wait (Q); . . . signal (S); signal (Q); wait (Q); wait (S); . . . signal (Q); signal (S); (priority inversion) (deadlock) 16 J. Choi, DKU
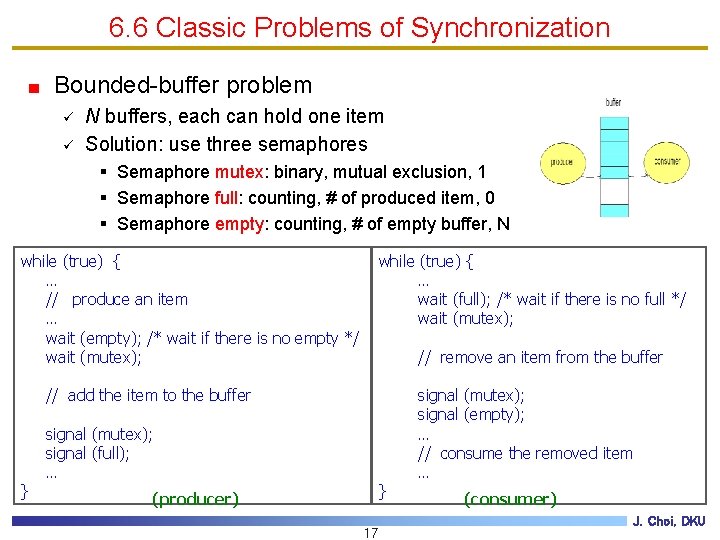
6. 6 Classic Problems of Synchronization Bounded-buffer problem ü ü N buffers, each can hold one item Solution: use three semaphores § Semaphore mutex: binary, mutual exclusion, 1 § Semaphore full: counting, # of produced item, 0 § Semaphore empty: counting, # of empty buffer, N while (true) { … // produce an item … wait (empty); /* wait if there is no empty */ wait (mutex); while (true) { … wait (full); /* wait if there is no full */ wait (mutex); // remove an item from the buffer // add the item to the buffer } signal (mutex); signal (full); … (producer) } 17 signal (mutex); signal (empty); … // consume the removed item … (consumer) J. Choi, DKU
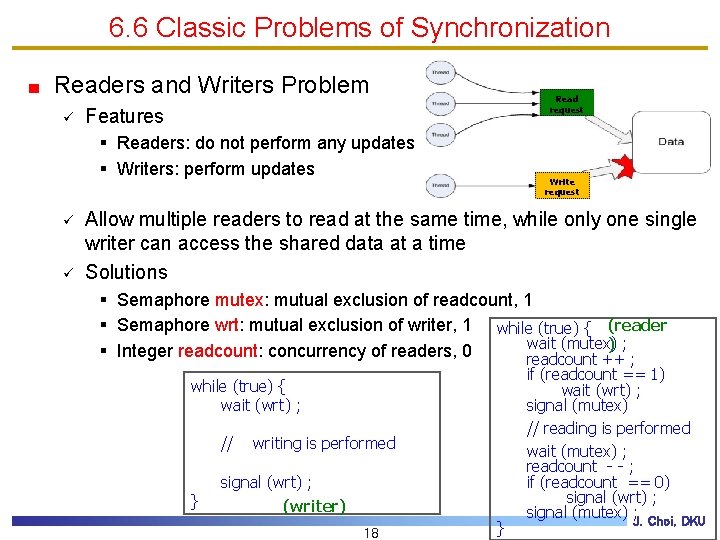
6. 6 Classic Problems of Synchronization Readers and Writers Problem ü Read request Features § Readers: do not perform any updates § Writers: perform updates ü ü Write request Allow multiple readers to read at the same time, while only one single writer can access the shared data at a time Solutions § Semaphore mutex: mutual exclusion of readcount, 1 § Semaphore wrt: mutual exclusion of writer, 1 while (true) { (reader wait (mutex) ) ; § Integer readcount: concurrency of readers, 0 readcount ++ ; while (true) { wait (wrt) ; // } writing is performed signal (wrt) ; (writer) 18 } if (readcount == 1) wait (wrt) ; signal (mutex) // reading is performed wait (mutex) ; readcount - - ; if (readcount == 0) signal (wrt) ; signal (mutex) ; J. Choi, DKU
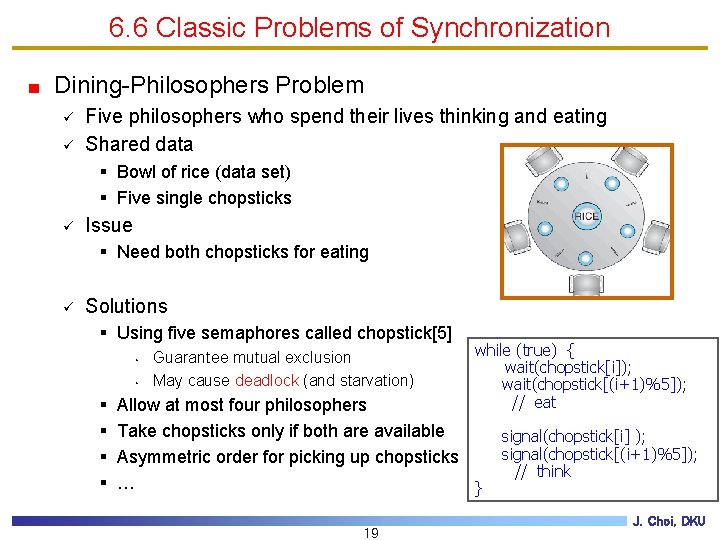
6. 6 Classic Problems of Synchronization Dining-Philosophers Problem ü ü Five philosophers who spend their lives thinking and eating Shared data § Bowl of rice (data set) § Five single chopsticks ü Issue § Need both chopsticks for eating ü Solutions § Using five semaphores called chopstick[5] • • § § Guarantee mutual exclusion May cause deadlock (and starvation) while (true) { wait(chopstick[i]); wait(chopstick[(i+1)%5]); // eat Allow at most four philosophers Take chopsticks only if both are available Asymmetric order for picking up chopsticks … } 19 signal(chopstick[i] ); signal(chopstick[(i+1)%5]); // think J. Choi, DKU
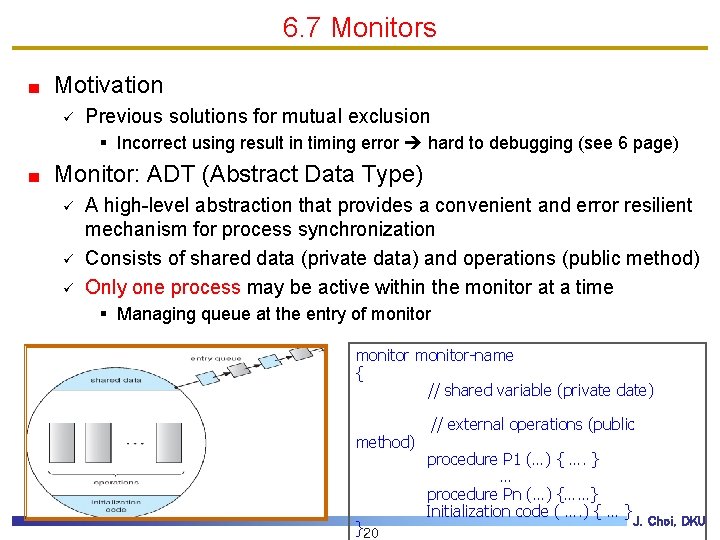
6. 7 Monitors Motivation ü Previous solutions for mutual exclusion § Incorrect using result in timing error hard to debugging (see 6 page) Monitor: ADT (Abstract Data Type) ü ü ü A high-level abstraction that provides a convenient and error resilient mechanism for process synchronization Consists of shared data (private data) and operations (public method) Only one process may be active within the monitor at a time § Managing queue at the entry of monitor-name { // shared variable (private date) method) }20 // external operations (public procedure P 1 (…) { …. } … procedure Pn (…) {……} Initialization code ( …. ) { … } J. Choi, DKU
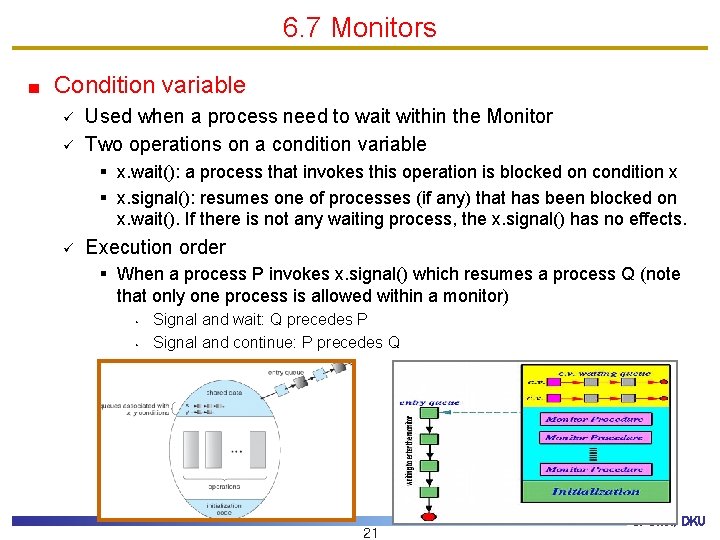
6. 7 Monitors Condition variable ü ü Used when a process need to wait within the Monitor Two operations on a condition variable § x. wait(): a process that invokes this operation is blocked on condition x § x. signal(): resumes one of processes (if any) that has been blocked on x. wait(). If there is not any waiting process, the x. signal() has no effects. ü Execution order § When a process P invokes x. signal() which resumes a process Q (note that only one process is allowed within a monitor) • • Signal and wait: Q precedes P Signal and continue: P precedes Q 21 J. Choi, DKU
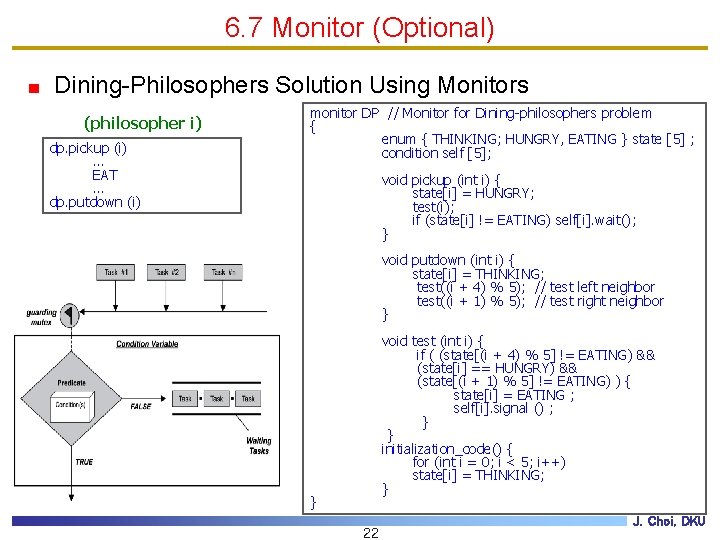
6. 7 Monitor (Optional) Dining-Philosophers Solution Using Monitors (philosopher i) dp. pickup (i) … EAT … dp. putdown (i) monitor DP // Monitor for Dining-philosophers problem { enum { THINKING; HUNGRY, EATING } state [5] ; condition self [5]; void pickup (int i) { state[i] = HUNGRY; test(i); if (state[i] != EATING) self[i]. wait(); } void putdown (int i) { state[i] = THINKING; test((i + 4) % 5); // test left neighbor test((i + 1) % 5); // test right neighbor } void test (int i) { if ( (state[(i + 4) % 5] != EATING) && (state[i] == HUNGRY) && (state[(i + 1) % 5] != EATING) ) { state[i] = EATING ; self[i]. signal () ; } } initialization_code() { for (int i = 0; i < 5; i++) state[i] = THINKING; } } 22 J. Choi, DKU
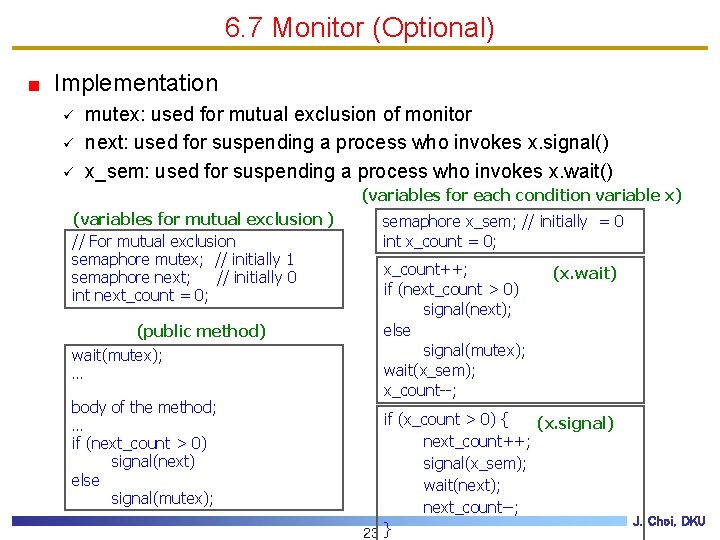
6. 7 Monitor (Optional) Implementation ü ü ü mutex: used for mutual exclusion of monitor next: used for suspending a process who invokes x. signal() x_sem: used for suspending a process who invokes x. wait() (variables for each condition variable x) (variables for mutual exclusion ) // For mutual exclusion semaphore mutex; // initially 1 semaphore next; // initially 0 int next_count = 0; (public method) wait(mutex); … body of the method; … if (next_count > 0) signal(next) else signal(mutex); semaphore x_sem; // initially = 0 int x_count = 0; x_count++; if (next_count > 0) signal(next); else signal(mutex); wait(x_sem); x_count--; (x. wait) if (x_count > 0) { (x. signal) next_count++; signal(x_sem); wait(next); next_count--; 23 } J. Choi, DKU
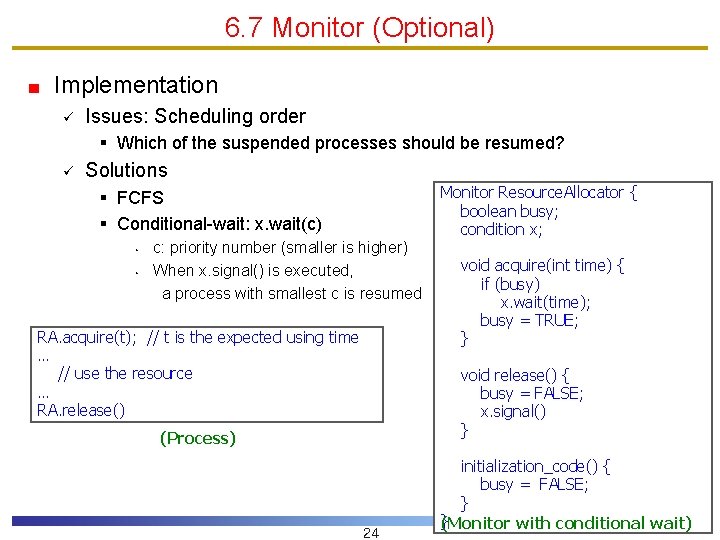
6. 7 Monitor (Optional) Implementation ü Issues: Scheduling order § Which of the suspended processes should be resumed? ü Solutions Monitor Resource. Allocator { boolean busy; condition x; § FCFS § Conditional-wait: x. wait(c) • • c: priority number (smaller is higher) When x. signal() is executed, a process with smallest c is resumed RA. acquire(t); // t is the expected using time … // use the resource … RA. release() void acquire(int time) { if (busy) x. wait(time); busy = TRUE; } void release() { busy = FALSE; x. signal() } (Process) 24 initialization_code() { busy = FALSE; } J. Choi, DKU }(Monitor with conditional wait)
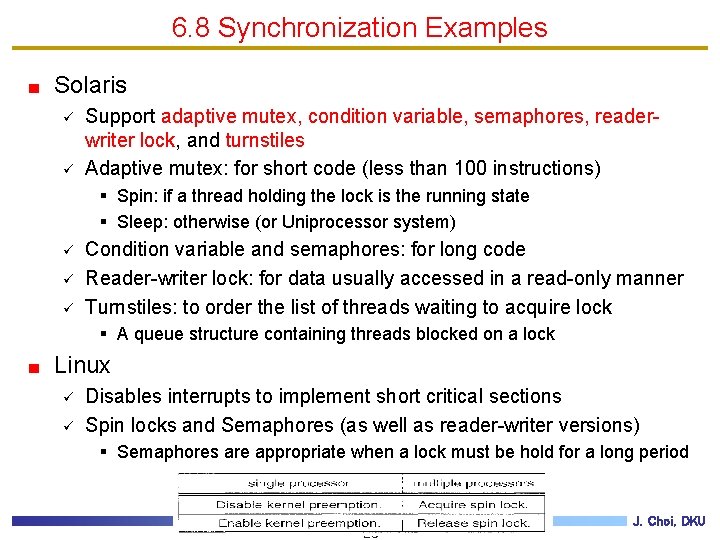
6. 8 Synchronization Examples Solaris ü ü Support adaptive mutex, condition variable, semaphores, readerwriter lock, and turnstiles Adaptive mutex: for short code (less than 100 instructions) § Spin: if a thread holding the lock is the running state § Sleep: otherwise (or Uniprocessor system) ü ü ü Condition variable and semaphores: for long code Reader-writer lock: for data usually accessed in a read-only manner Turnstiles: to order the list of threads waiting to acquire lock § A queue structure containing threads blocked on a lock Linux ü ü Disables interrupts to implement short critical sections Spin locks and Semaphores (as well as reader-writer versions) § Semaphores are appropriate when a lock must be hold for a long period 25 J. Choi, DKU
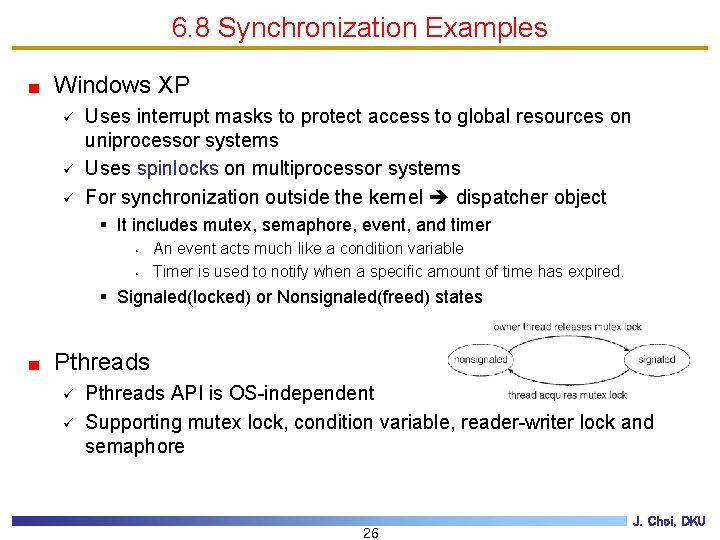
6. 8 Synchronization Examples Windows XP ü ü ü Uses interrupt masks to protect access to global resources on uniprocessor systems Uses spinlocks on multiprocessor systems For synchronization outside the kernel dispatcher object § It includes mutex, semaphore, event, and timer • • An event acts much like a condition variable Timer is used to notify when a specific amount of time has expired. § Signaled(locked) or Nonsignaled(freed) states Pthreads ü ü Pthreads API is OS-independent Supporting mutex lock, condition variable, reader-writer lock and semaphore 26 J. Choi, DKU
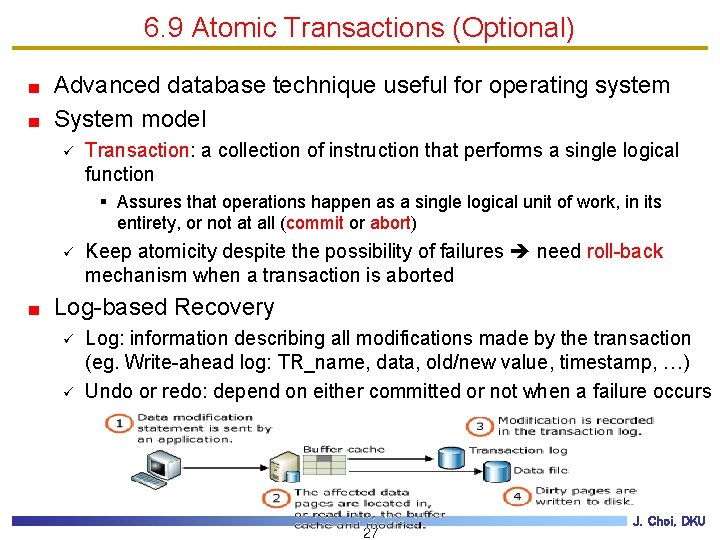
6. 9 Atomic Transactions (Optional) Advanced database technique useful for operating system System model ü Transaction: a collection of instruction that performs a single logical function § Assures that operations happen as a single logical unit of work, in its entirety, or not at all (commit or abort) ü Keep atomicity despite the possibility of failures need roll-back mechanism when a transaction is aborted Log-based Recovery ü ü Log: information describing all modifications made by the transaction (eg. Write-ahead log: TR_name, data, old/new value, timestamp, …) Undo or redo: depend on either committed or not when a failure occurs 27 J. Choi, DKU
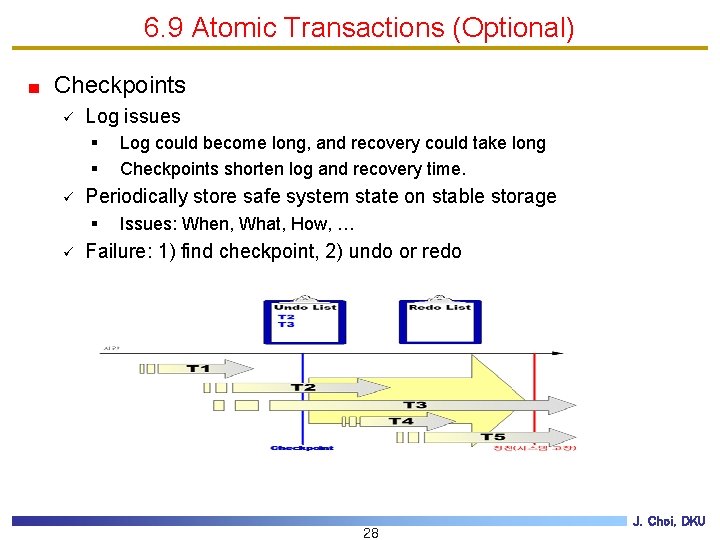
6. 9 Atomic Transactions (Optional) Checkpoints ü Log issues § § ü Periodically store safe system state on stable storage § ü Log could become long, and recovery could take long Checkpoints shorten log and recovery time. Issues: When, What, How, … Failure: 1) find checkpoint, 2) undo or redo 28 J. Choi, DKU
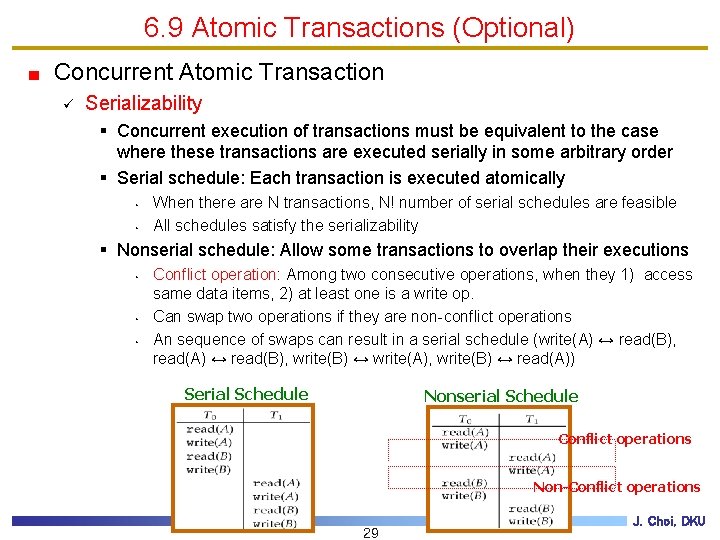
6. 9 Atomic Transactions (Optional) Concurrent Atomic Transaction ü Serializability § Concurrent execution of transactions must be equivalent to the case where these transactions are executed serially in some arbitrary order § Serial schedule: Each transaction is executed atomically • • When there are N transactions, N! number of serial schedules are feasible All schedules satisfy the serializability § Nonserial schedule: Allow some transactions to overlap their executions • • • Conflict operation: Among two consecutive operations, when they 1) access same data items, 2) at least one is a write op. Can swap two operations if they are non-conflict operations An sequence of swaps can result in a serial schedule (write(A) ↔ read(B), read(A) ↔ read(B), write(B) ↔ write(A), write(B) ↔ read(A)) Serial Schedule Nonserial Schedule Conflict operations Non-Conflict operations 29 J. Choi, DKU
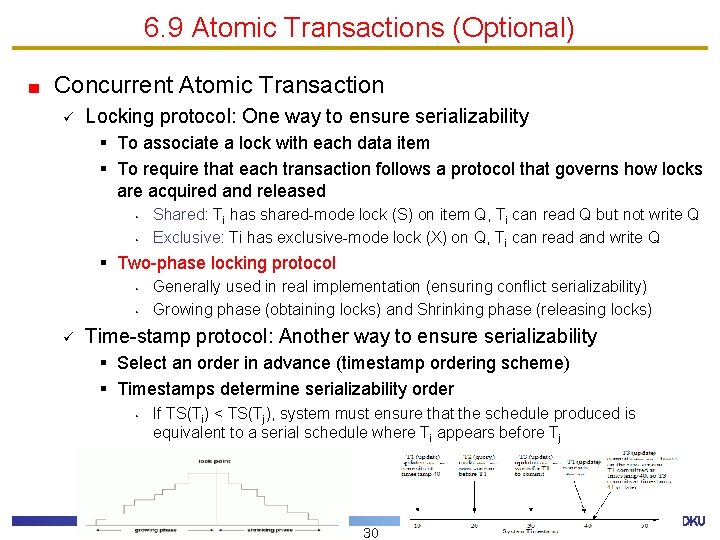
6. 9 Atomic Transactions (Optional) Concurrent Atomic Transaction ü Locking protocol: One way to ensure serializability § To associate a lock with each data item § To require that each transaction follows a protocol that governs how locks are acquired and released • • Shared: Ti has shared-mode lock (S) on item Q, Ti can read Q but not write Q Exclusive: Ti has exclusive-mode lock (X) on Q, Ti can read and write Q § Two-phase locking protocol • • ü Generally used in real implementation (ensuring conflict serializability) Growing phase (obtaining locks) and Shrinking phase (releasing locks) Time-stamp protocol: Another way to ensure serializability § Select an order in advance (timestamp ordering scheme) § Timestamps determine serializability order • If TS(Ti) < TS(Tj), system must ensure that the schedule produced is equivalent to a serial schedule where Ti appears before Tj 30 J. Choi, DKU
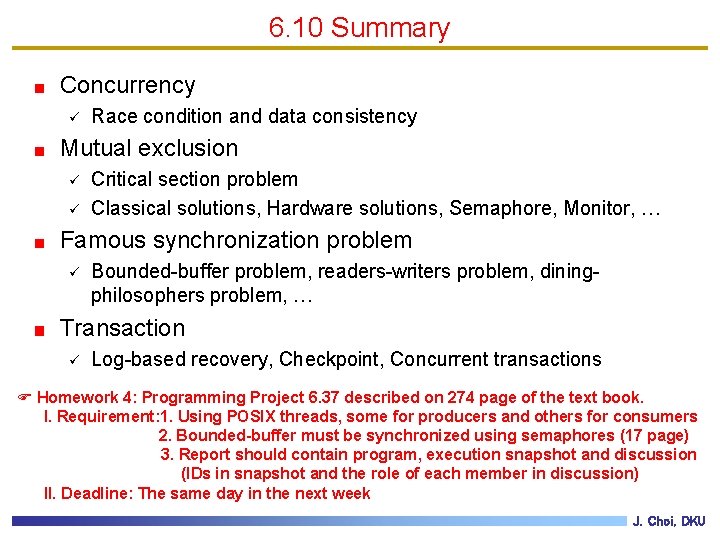
6. 10 Summary Concurrency ü Race condition and data consistency Mutual exclusion ü ü Critical section problem Classical solutions, Hardware solutions, Semaphore, Monitor, … Famous synchronization problem ü Bounded-buffer problem, readers-writers problem, diningphilosophers problem, … Transaction ü Log-based recovery, Checkpoint, Concurrent transactions F Homework 4: Programming Project 6. 37 described on 274 page of the text book. I. Requirement: 1. Using POSIX threads, some for producers and others for consumers 2. Bounded-buffer must be synchronized using semaphores (17 page) 3. Report should contain program, execution snapshot and discussion (IDs in snapshot and the role of each member in discussion) II. Deadline: The same day in the next week J. Choi, DKU
Jongmoo choi
Dept nmr spectroscopy
Department of agriculture consumer services
Finance departments
Worcester public health department
Dept. name of organization
Mn dept of education
Department of finance and administration
Dept. name of organization (of affiliation)
Ohio dept of dd
Hjdkdkd
Vaginal dept
Gome dept
Gome dept
Nyttofunktion
Gome dept
Hoe dept
Fire dept interview questions
Maine dept of agriculture
Dept of education
Florida dept of agriculture and consumer services
Florida dept of agriculture and consumer services
Dept a
Central islip fire dept
Micah ennis
Dept of education
Dept c13 nmr
Pt dept logistik
Nys dept of homeland security
Affiliate disclodures
La geaux biz
Oxford dept of continuing education