Chapter 10 User Defined Simple Data Types cstring
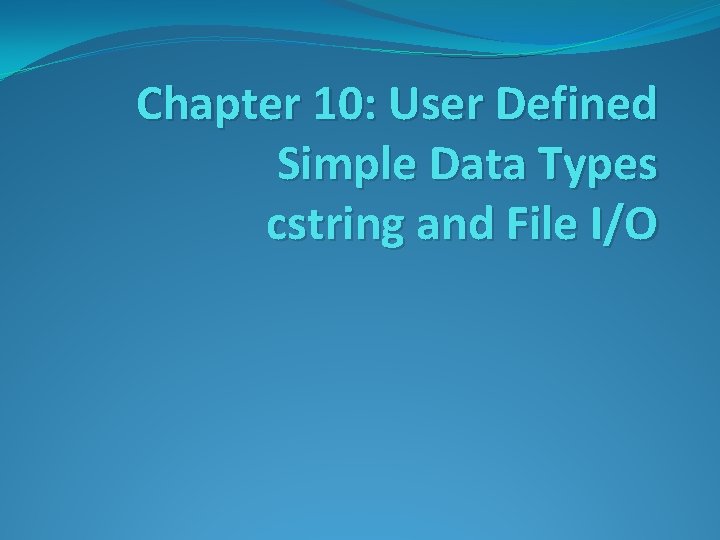
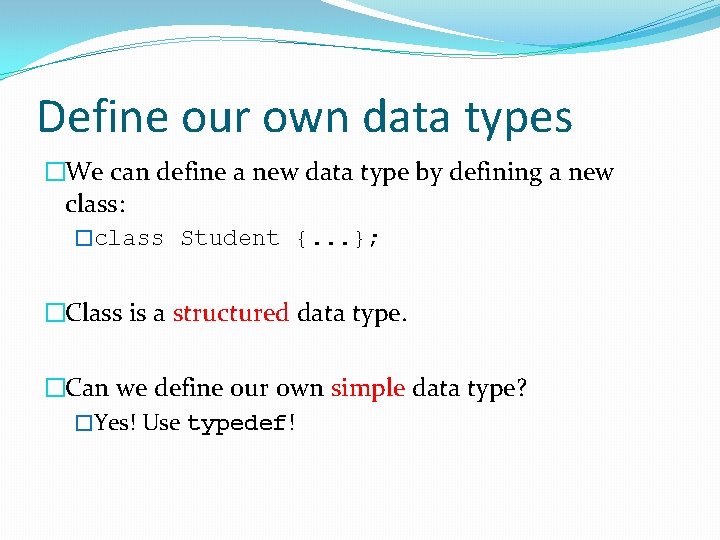
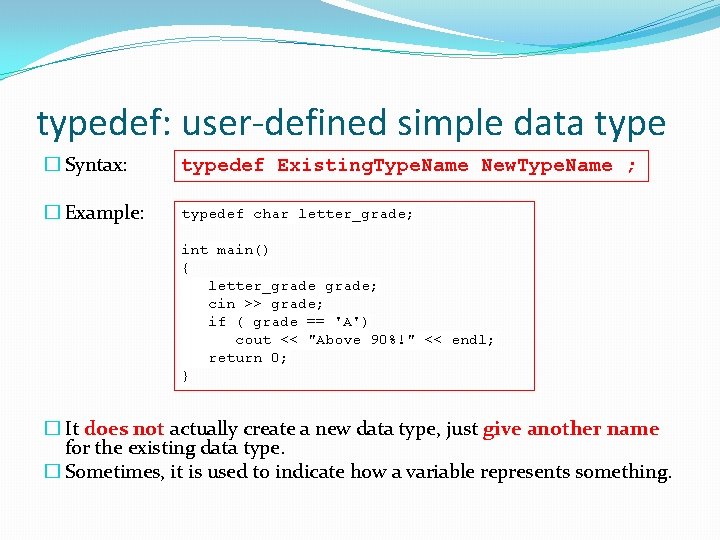
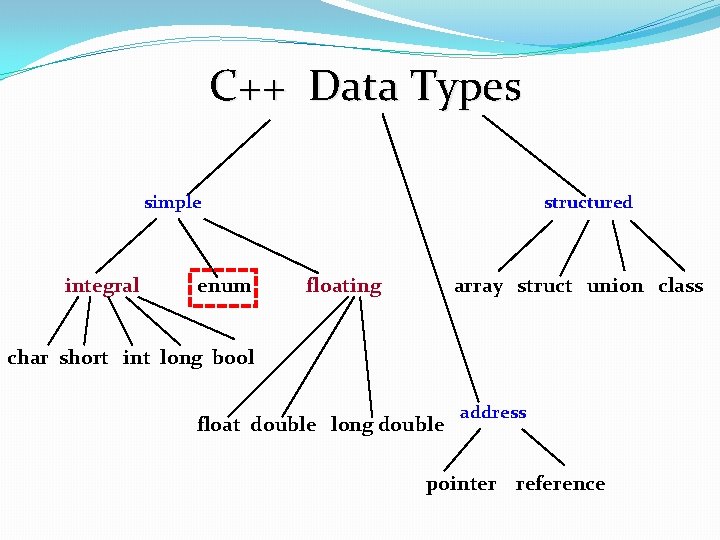
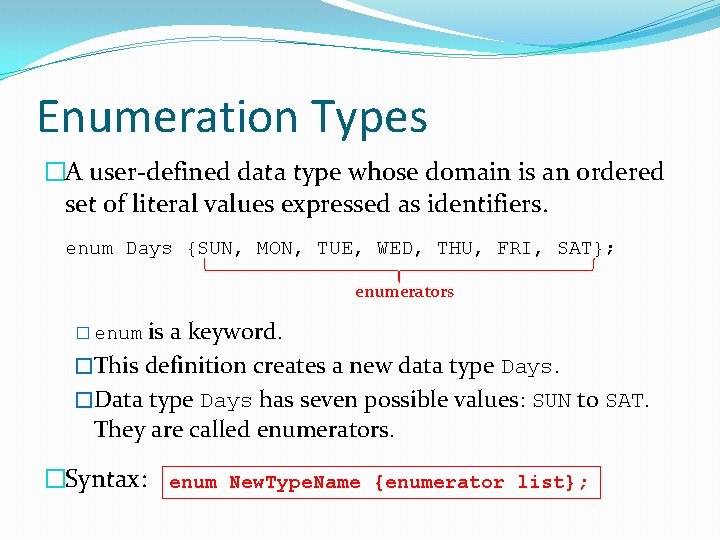
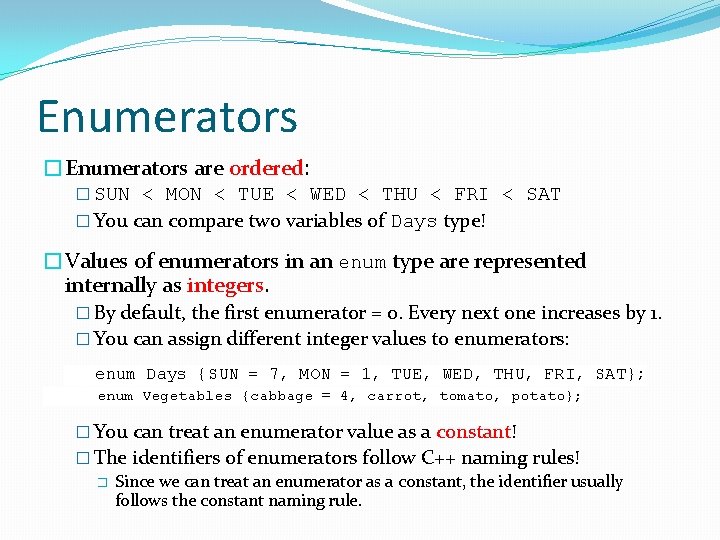
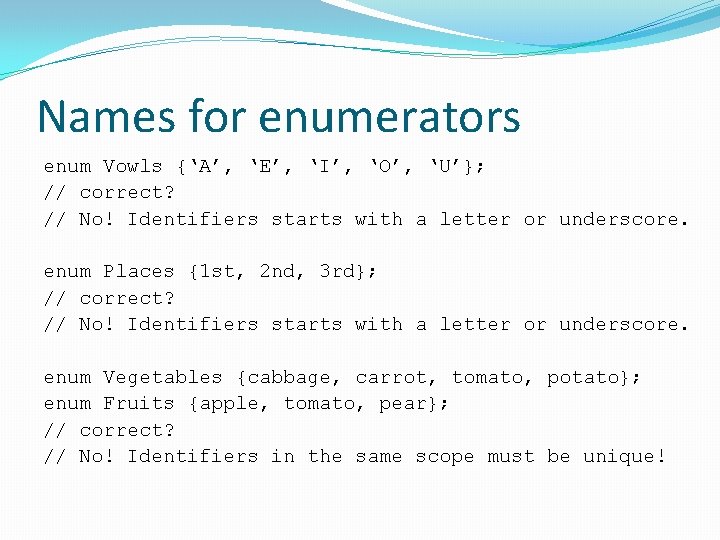
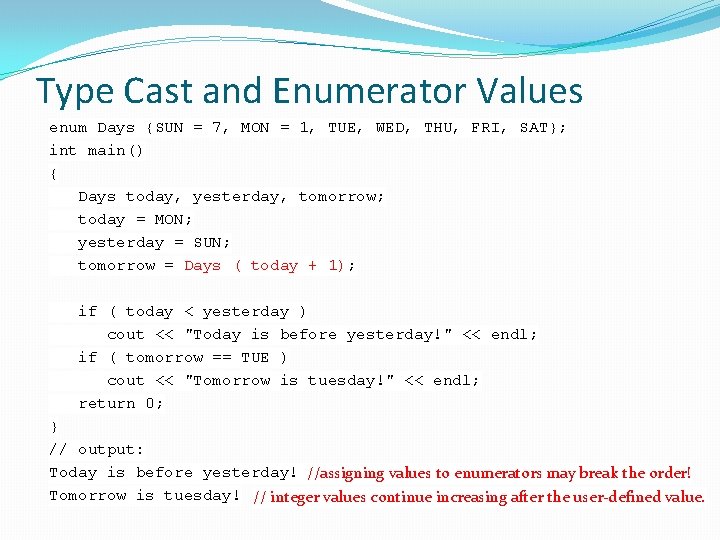
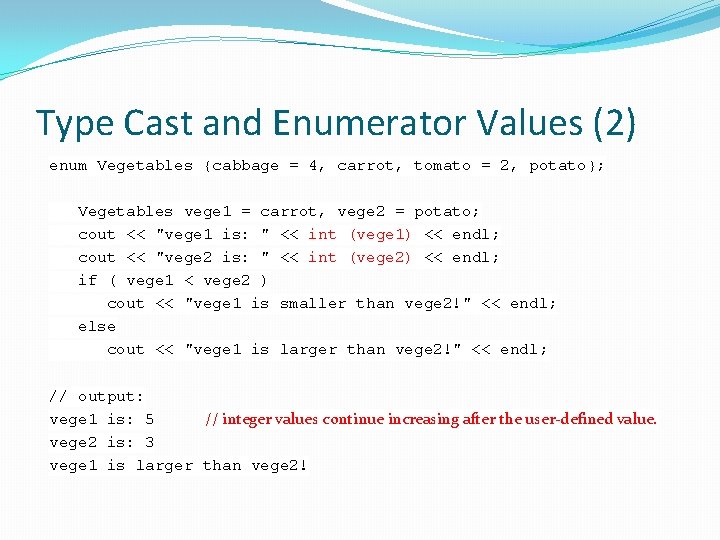
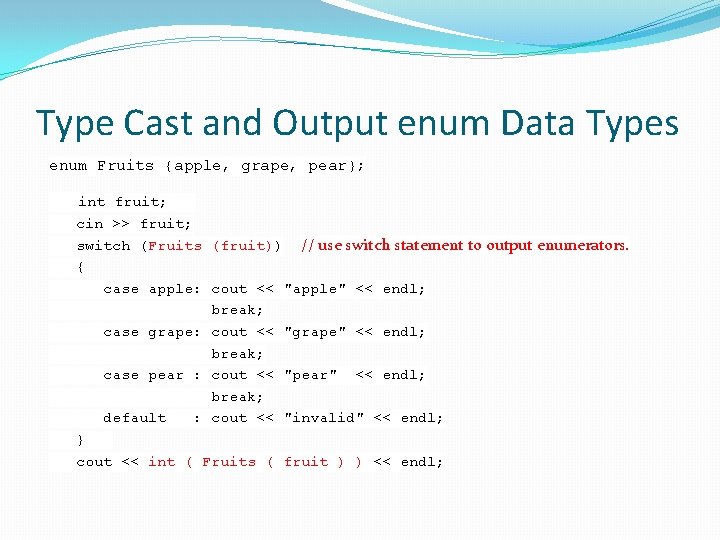
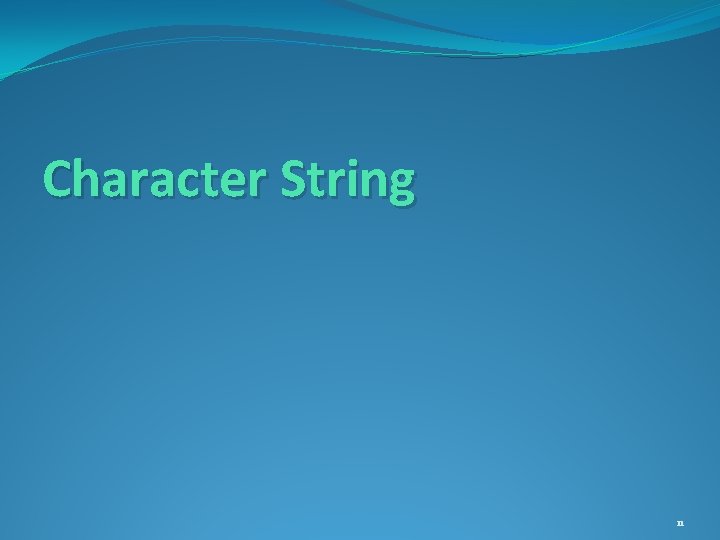
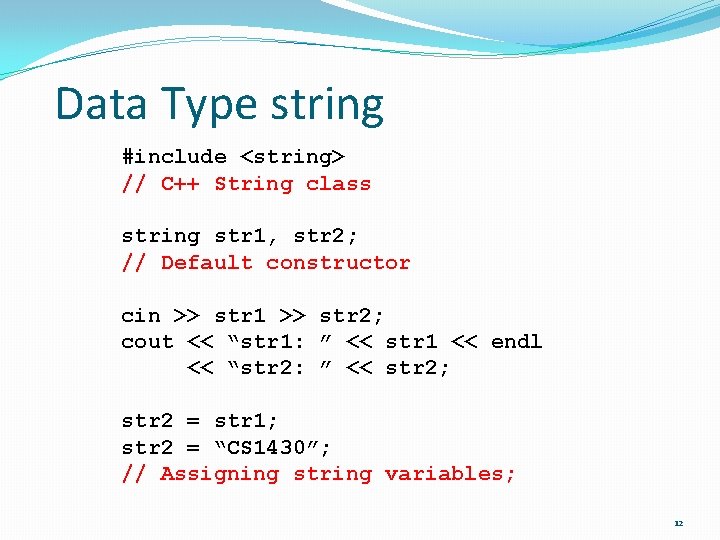
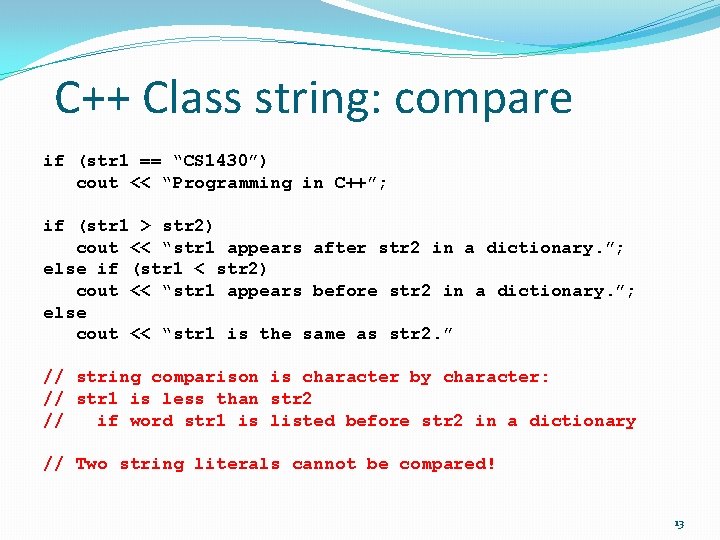
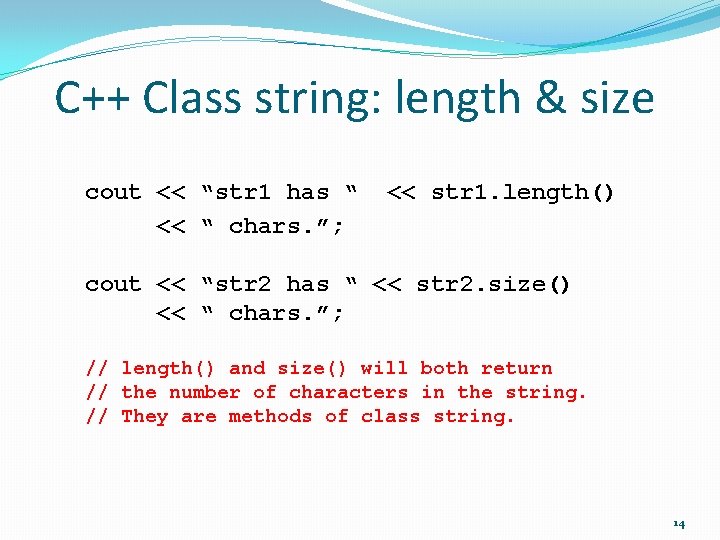
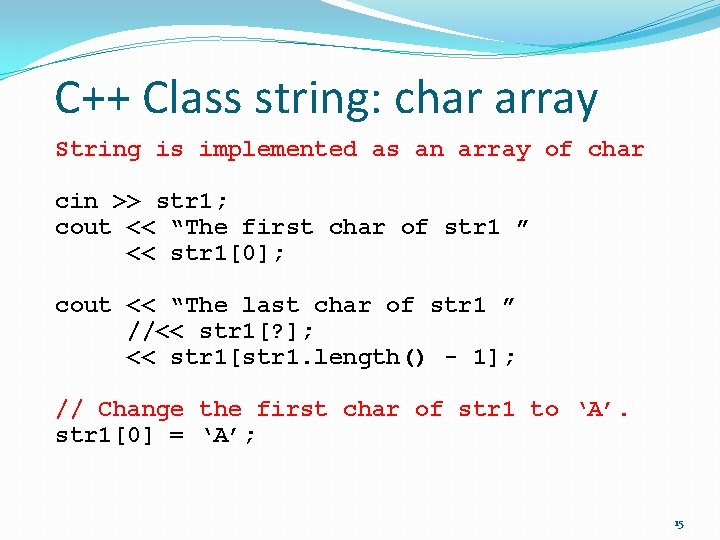
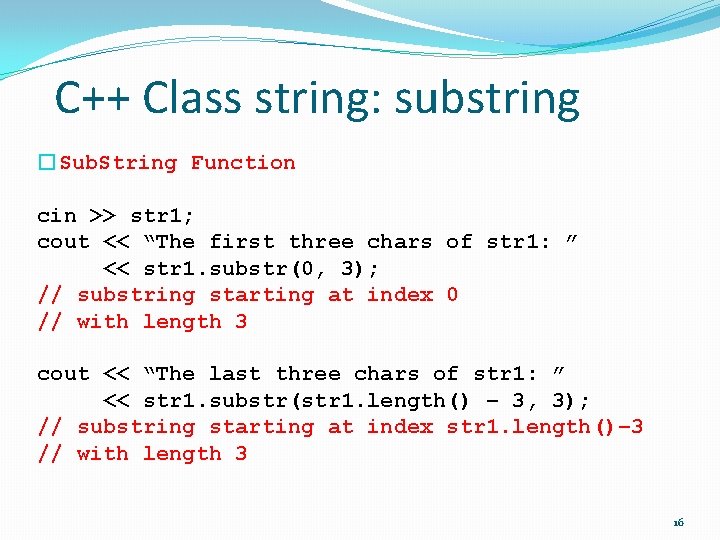
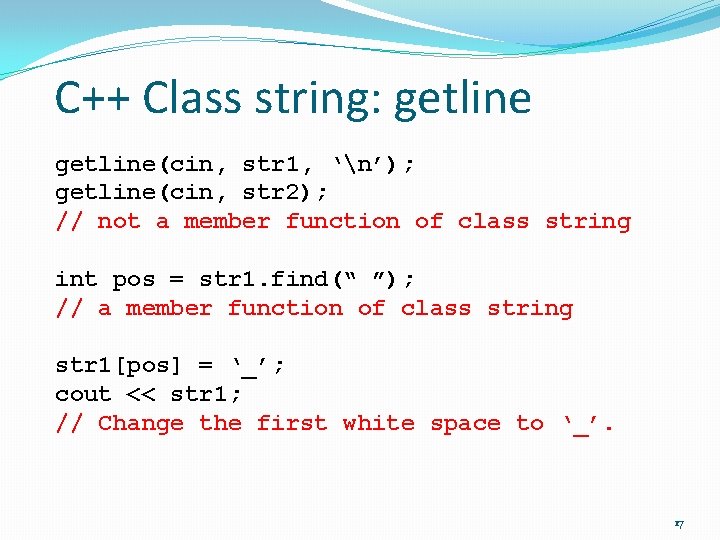
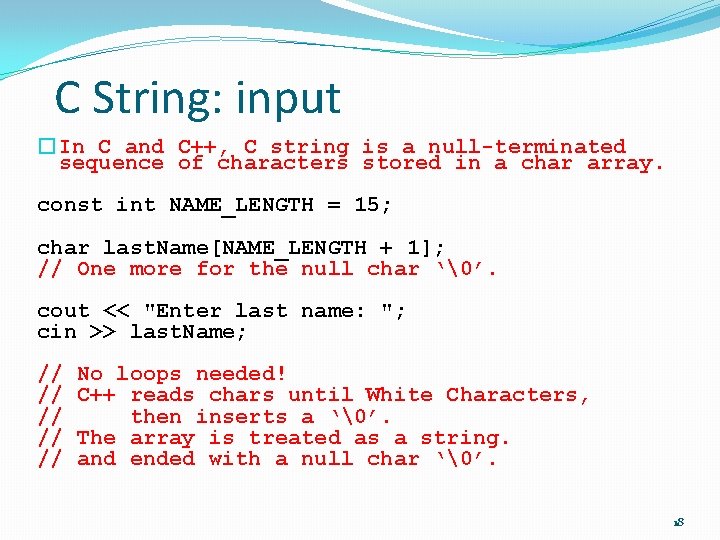
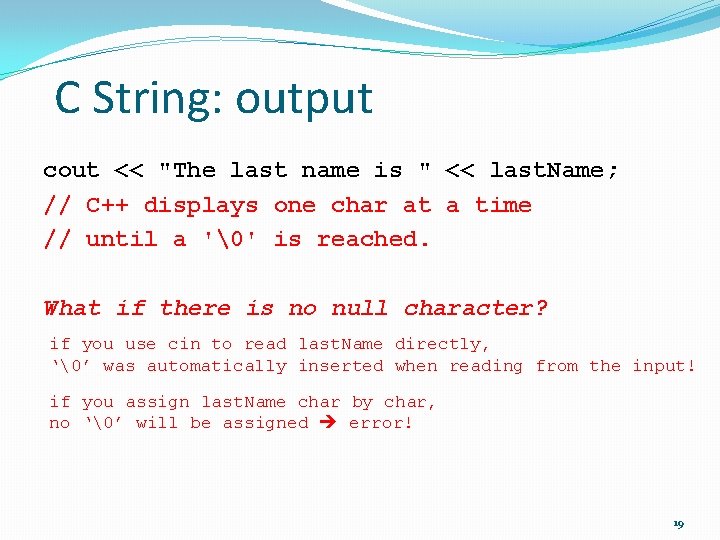
![typedef and C string const int NAME_LENGTH = 15; char last. Name[NAME_LENGTH + 1]; typedef and C string const int NAME_LENGTH = 15; char last. Name[NAME_LENGTH + 1];](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-20.jpg)
![C String: assignment char name 1[16], name 2[16]; // Up to 15 chars! cout C String: assignment char name 1[16], name 2[16]; // Up to 15 chars! cout](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-21.jpg)
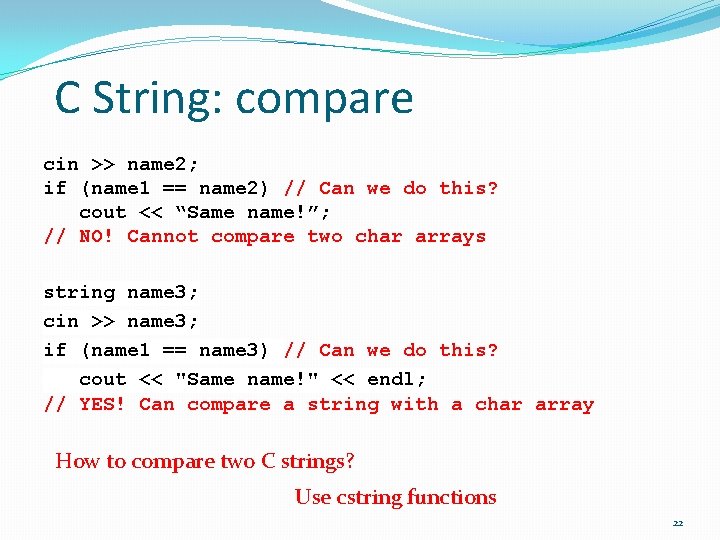
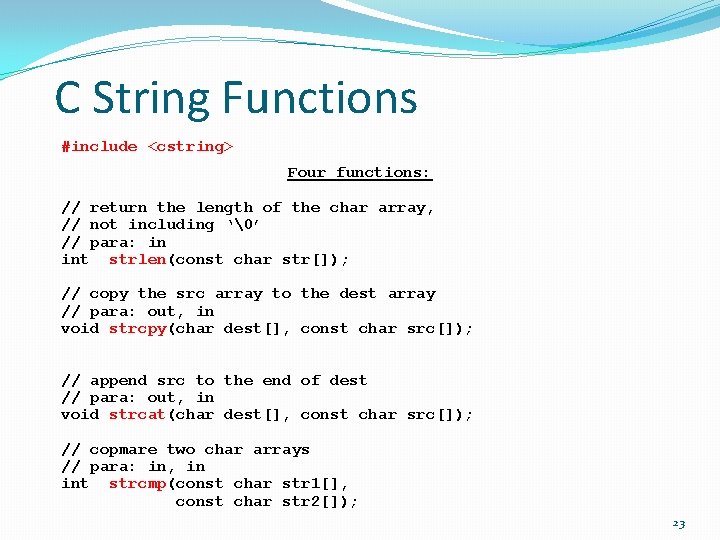
![C String Functions #include <cstring> char name 1[16], name 2[16]; cout << "Enter last C String Functions #include <cstring> char name 1[16], name 2[16]; cout << "Enter last](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-24.jpg)
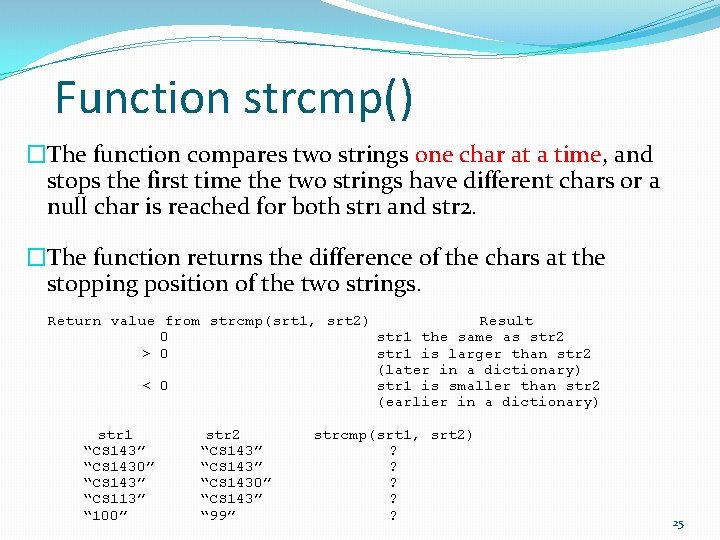
![Function strcmp() #include <cstring> char name 1[16], name 2[16]; cin >> name 1 >> Function strcmp() #include <cstring> char name 1[16], name 2[16]; cin >> name 1 >>](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-26.jpg)
![Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, // Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, //](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-27.jpg)
![Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, // Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, //](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-28.jpg)
![Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, // Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, //](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-29.jpg)
![Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, // Function strcpy() //-----------------------// The function has two parameters: // dest[], array of char, //](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-30.jpg)
![Function strlen() //----------------------// The function has one parameter: // str[], array of char. // Function strlen() //----------------------// The function has one parameter: // str[], array of char. //](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-31.jpg)
![Function strcmp() //---------------------------// The function has two parameters: // str 1[], array of char, Function strcmp() //---------------------------// The function has two parameters: // str 1[], array of char,](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-32.jpg)
![Function strcmp() //---------------------------// The function has two parameters: // str 1[], array of char, Function strcmp() //---------------------------// The function has two parameters: // str 1[], array of char,](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-33.jpg)
![Function strcat() //---------------------------// The function has two parameters: // dest[], array of char, null Function strcat() //---------------------------// The function has two parameters: // dest[], array of char, null](https://slidetodoc.com/presentation_image_h/28ffb4c95f5cabc555bb3bdd1f9b389c/image-34.jpg)
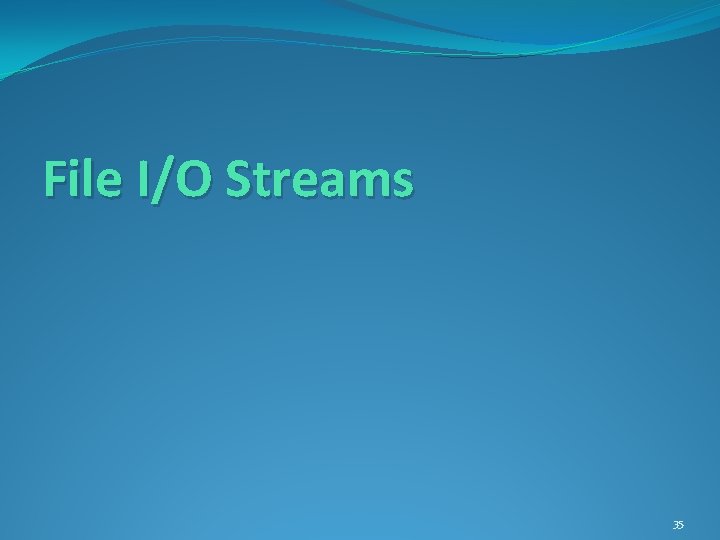
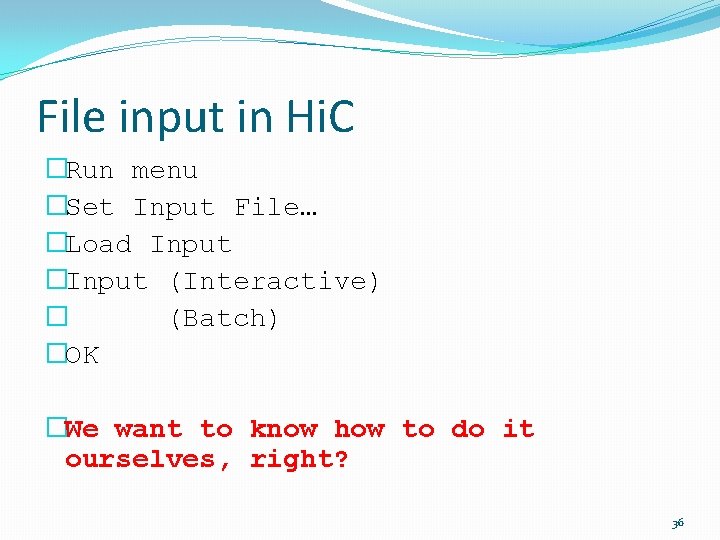
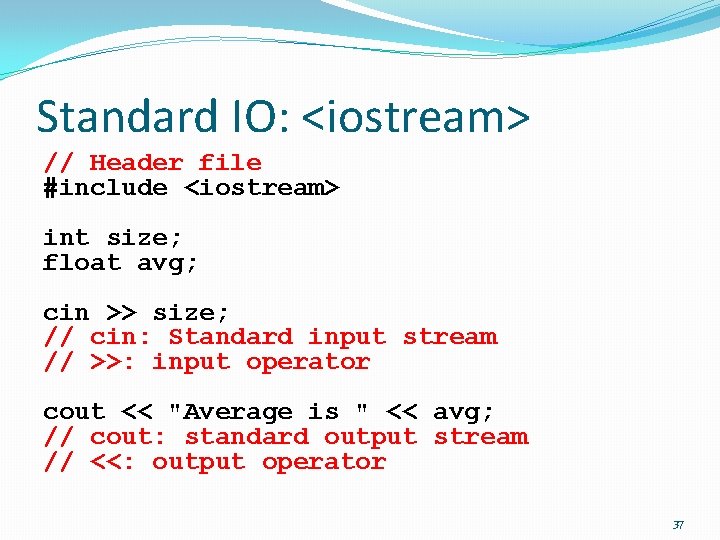
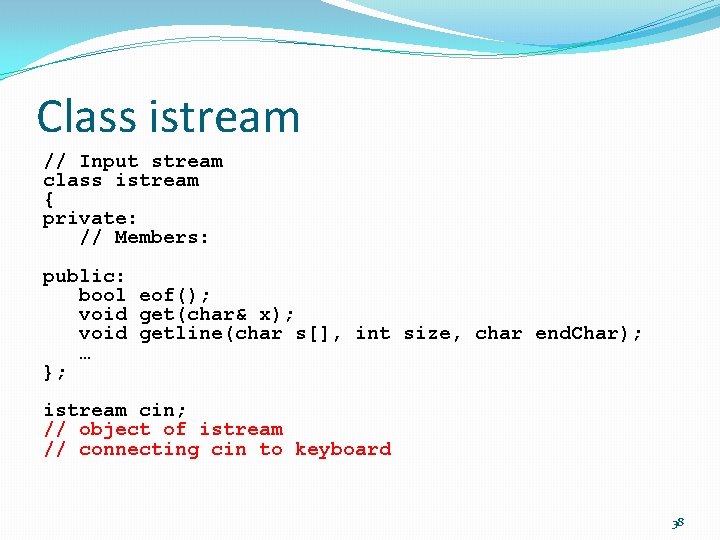
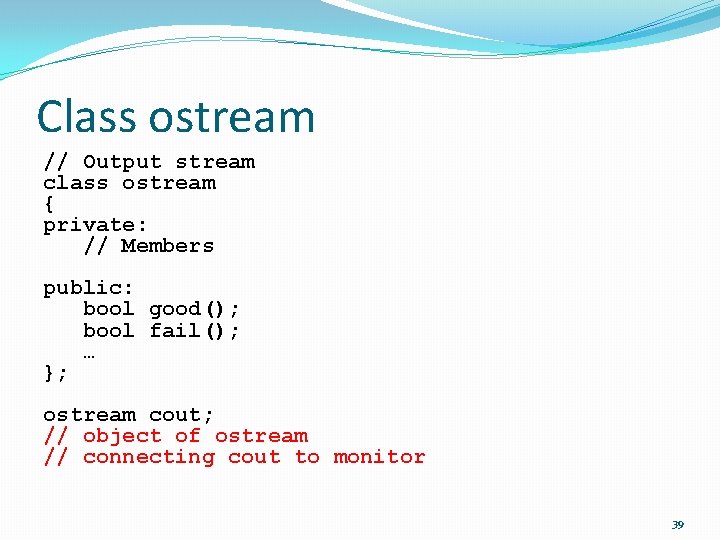
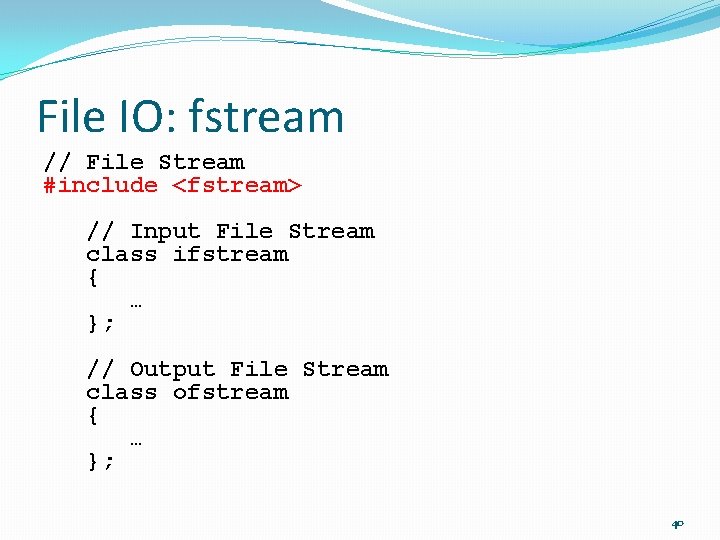
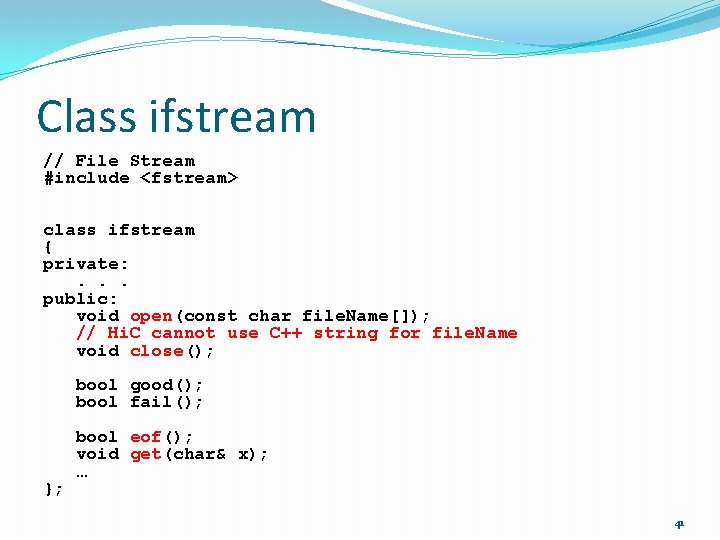
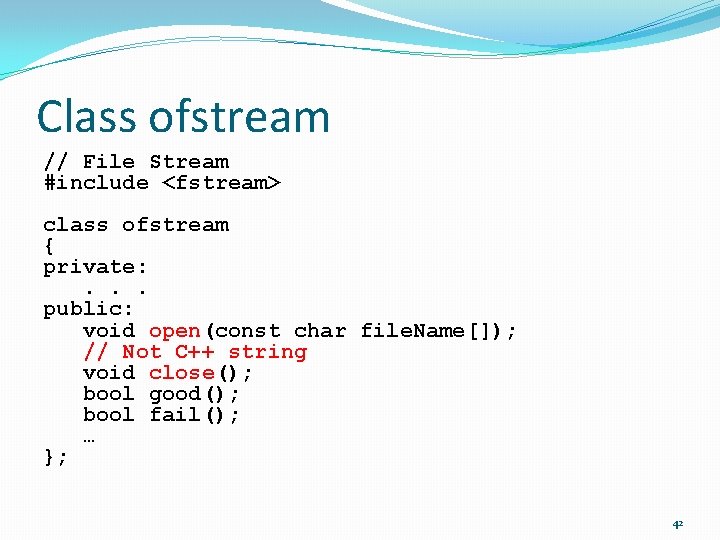
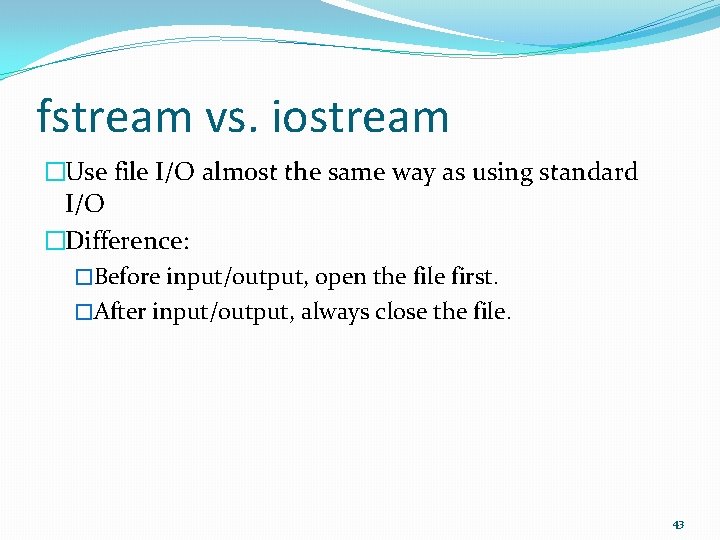
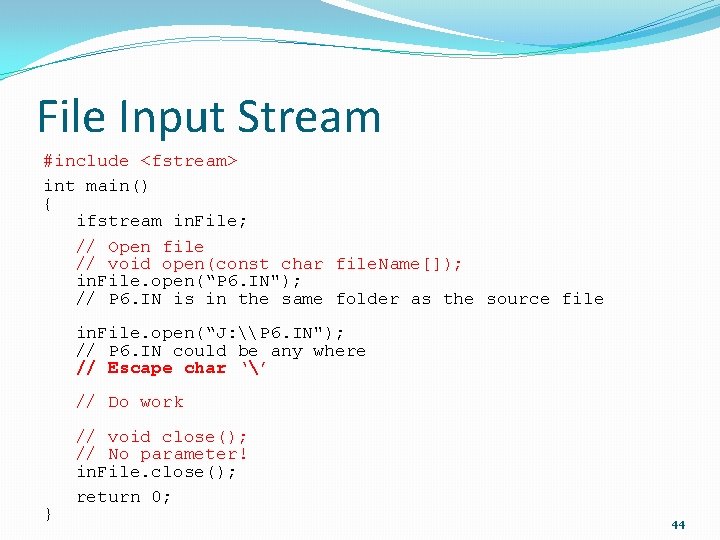
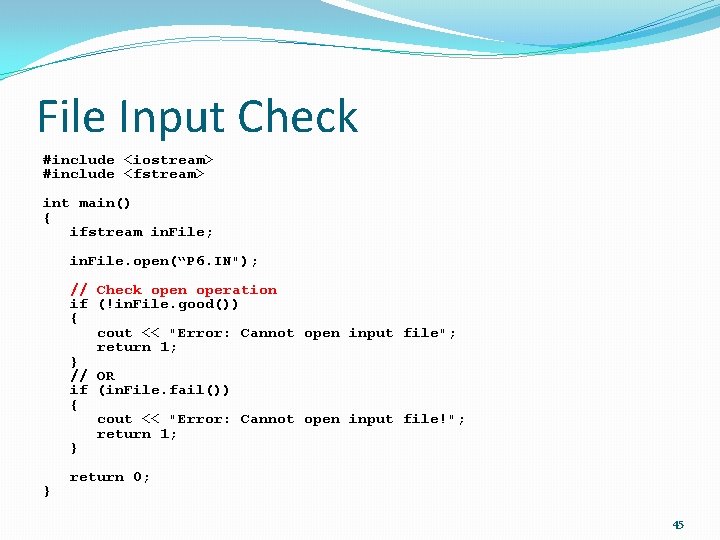
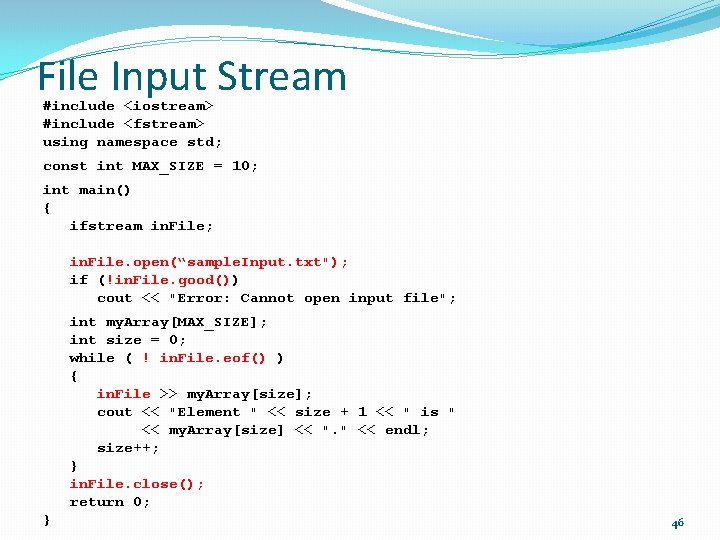
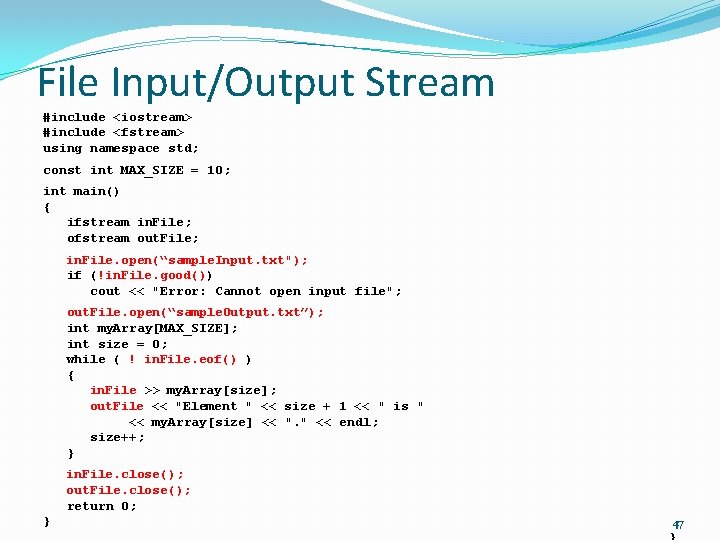
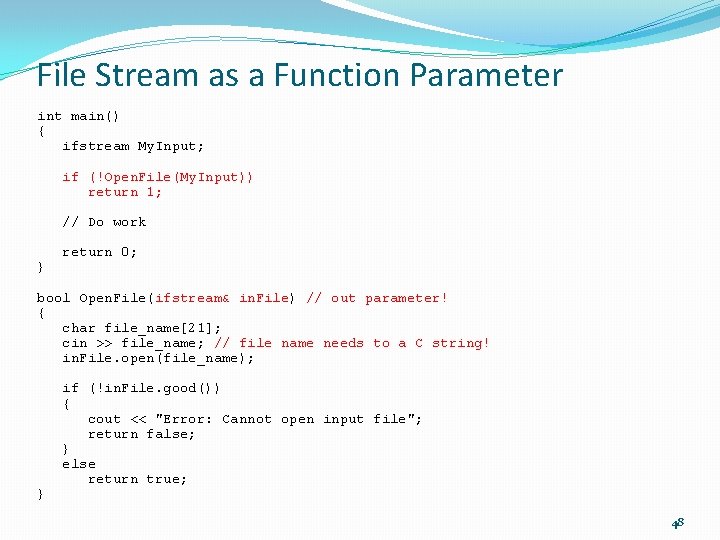
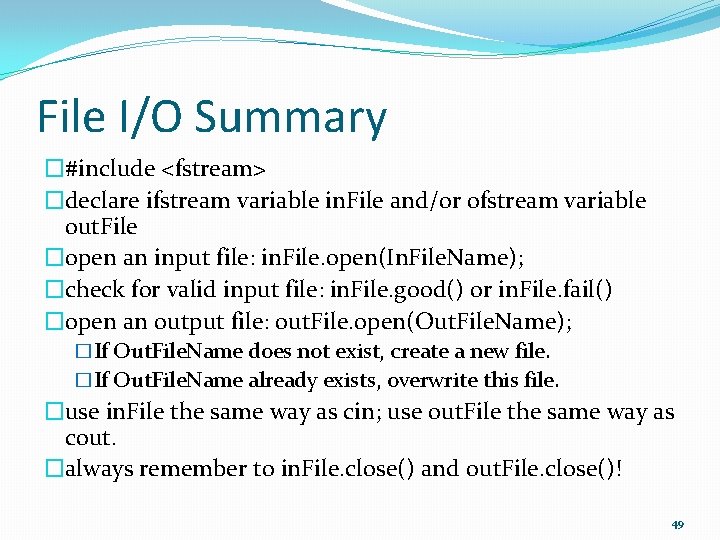
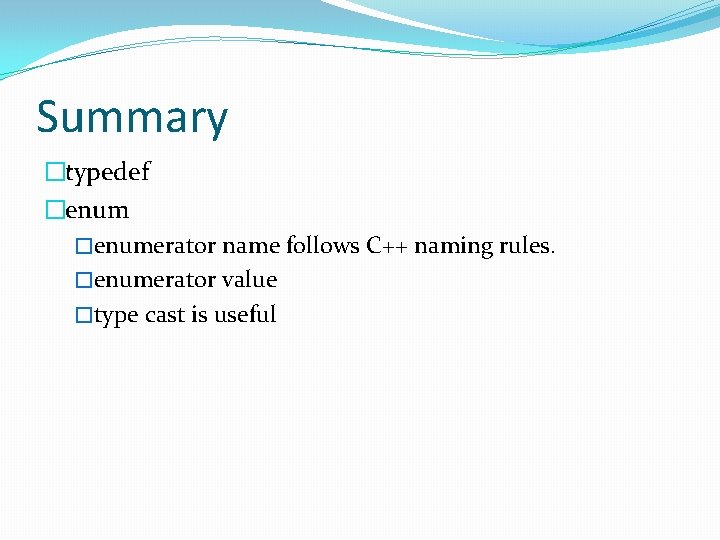
- Slides: 50
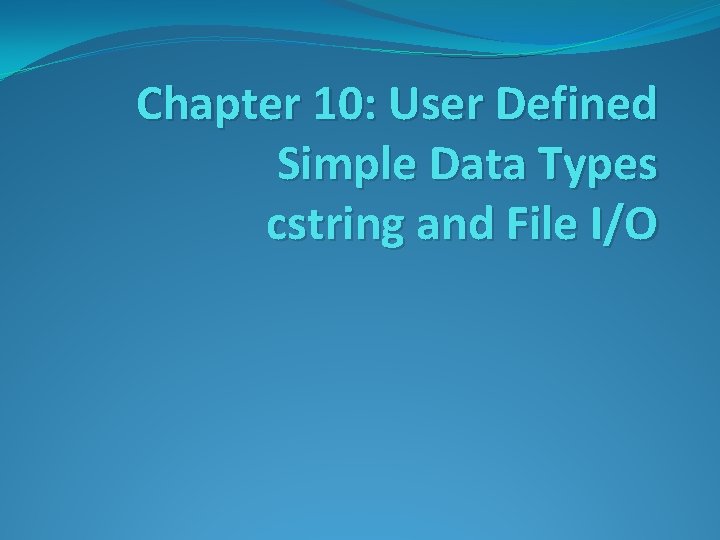
Chapter 10: User Defined Simple Data Types cstring and File I/O
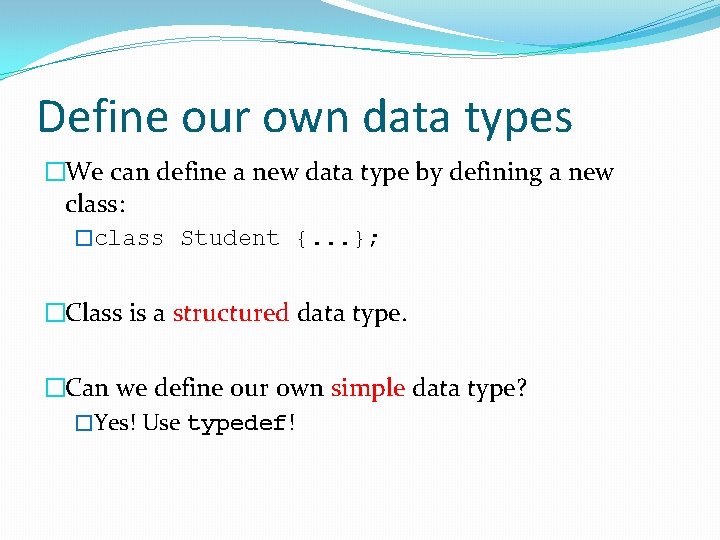
Define our own data types �We can define a new data type by defining a new class: �class Student {. . . }; �Class is a structured data type. �Can we define our own simple data type? �Yes! Use typedef!
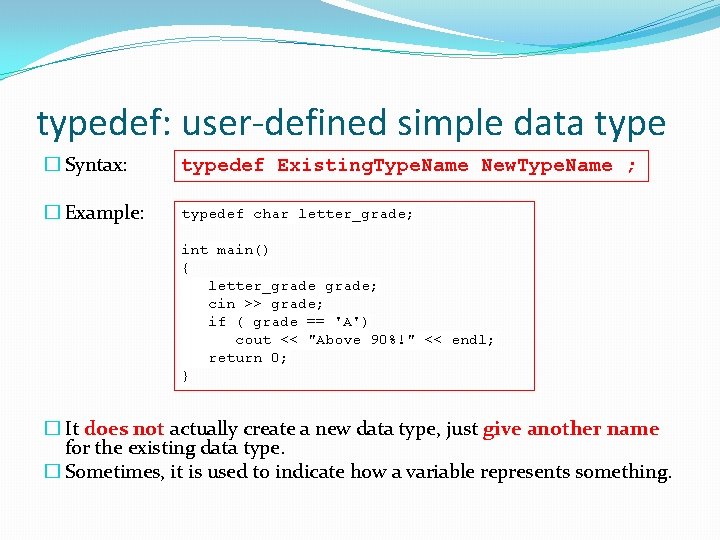
typedef: user-defined simple data type � Syntax: typedef Existing. Type. Name New. Type. Name ; � Example: typedef char letter_grade; int main() { letter_grade; cin >> grade; if ( grade == 'A') cout << "Above 90%!" << endl; return 0; } � It does not actually create a new data type, just give another name for the existing data type. � Sometimes, it is used to indicate how a variable represents something.
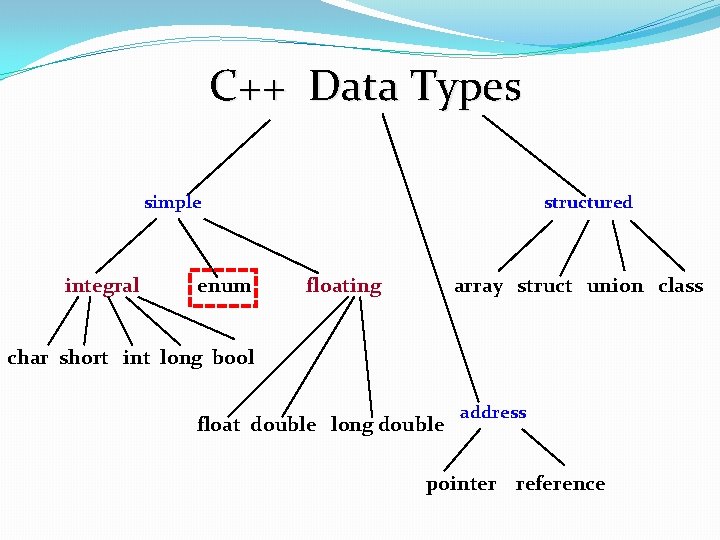
C++ Data Types simple integral enum structured floating array struct union class char short int long bool float double long double address pointer reference
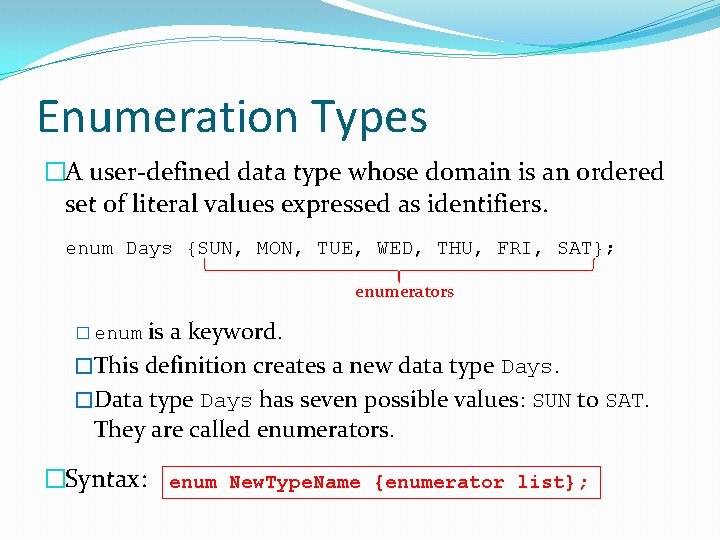
Enumeration Types �A user-defined data type whose domain is an ordered set of literal values expressed as identifiers. enum Days {SUN, MON, TUE, WED, THU, FRI, SAT}; enumerators is a keyword. �This definition creates a new data type Days. �Data type Days has seven possible values: SUN to SAT. They are called enumerators. � enum �Syntax: enum New. Type. Name {enumerator list};
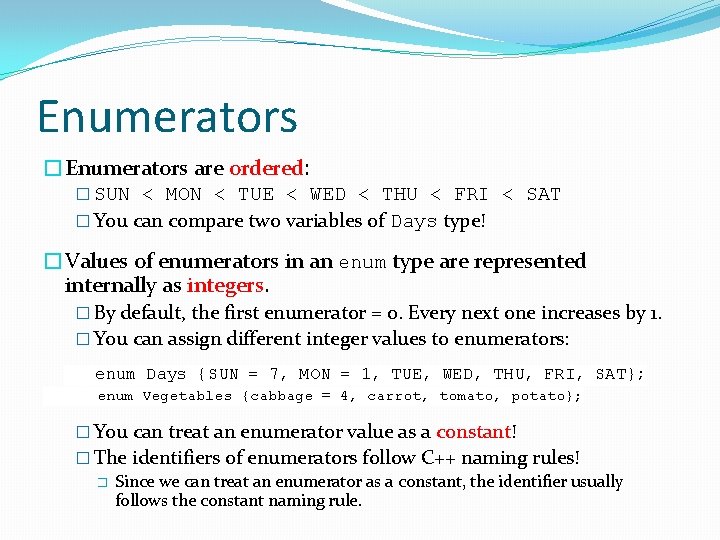
Enumerators �Enumerators are ordered: � SUN < MON < TUE < WED < THU < FRI < SAT � You can compare two variables of Days type! �Values of enumerators in an enum type are represented internally as integers. � By default, the first enumerator = 0. Every next one increases by 1. � You can assign different integer values to enumerators: enum Days {SUN = 7, MON = 1, TUE, WED, THU, FRI, SAT}; enum Vegetables {cabbage = 4, carrot, tomato, potato}; � You can treat an enumerator value as a constant! � The identifiers of enumerators follow C++ naming rules! � Since we can treat an enumerator as a constant, the identifier usually follows the constant naming rule.
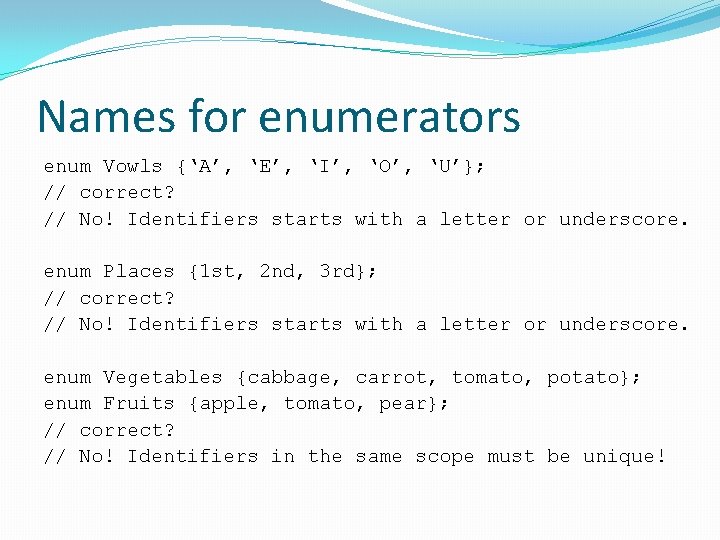
Names for enumerators enum Vowls {‘A’, ‘E’, ‘I’, ‘O’, ‘U’}; // correct? // No! Identifiers starts with a letter or underscore. enum Places {1 st, 2 nd, 3 rd}; // correct? // No! Identifiers starts with a letter or underscore. enum Vegetables {cabbage, carrot, tomato, potato}; enum Fruits {apple, tomato, pear}; // correct? // No! Identifiers in the same scope must be unique!
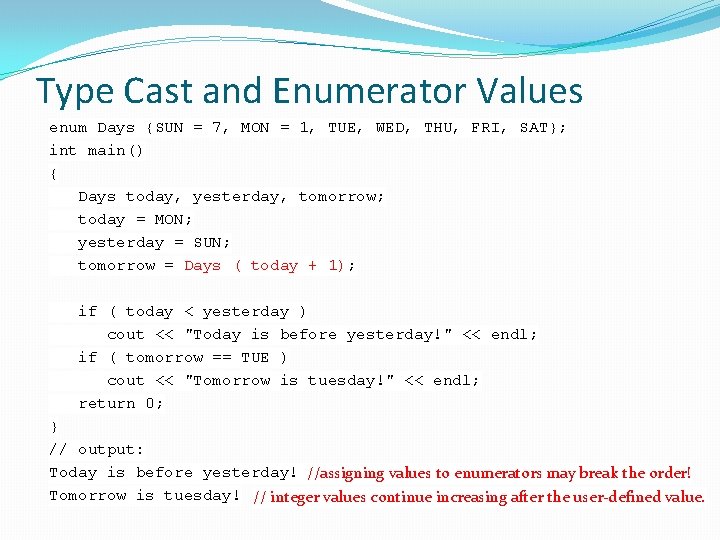
Type Cast and Enumerator Values enum Days {SUN = 7, MON = 1, TUE, WED, THU, FRI, SAT}; int main() { Days today, yesterday, tomorrow; today = MON; yesterday = SUN; tomorrow = Days ( today + 1); if ( today < yesterday ) cout << "Today is before yesterday!" << endl; if ( tomorrow == TUE ) cout << "Tomorrow is tuesday!" << endl; return 0; } // output: Today is before yesterday! //assigning values to enumerators may break the order! Tomorrow is tuesday! // integer values continue increasing after the user-defined value.
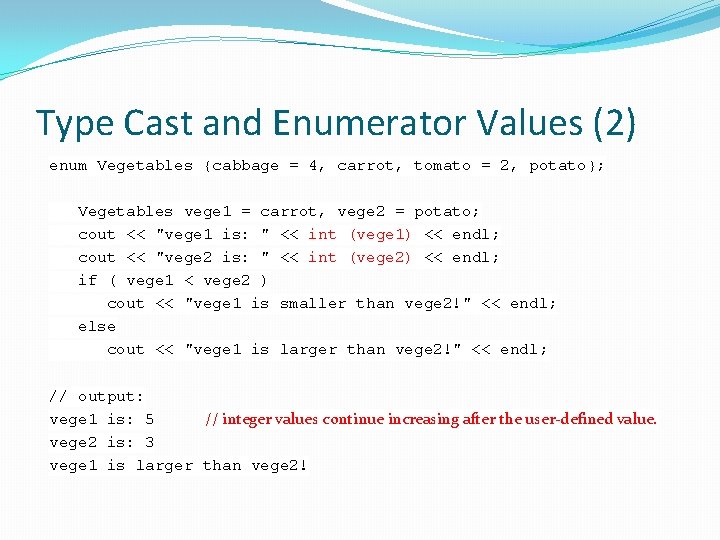
Type Cast and Enumerator Values (2) enum Vegetables {cabbage = 4, carrot, tomato = 2, potato}; Vegetables vege 1 = carrot, vege 2 = potato; cout << "vege 1 is: " << int (vege 1) << endl; cout << "vege 2 is: " << int (vege 2) << endl; if ( vege 1 < vege 2 ) cout << "vege 1 is smaller than vege 2!" << endl; else cout << "vege 1 is larger than vege 2!" << endl; // output: vege 1 is: 5 // integer values continue increasing after the user-defined value. vege 2 is: 3 vege 1 is larger than vege 2!
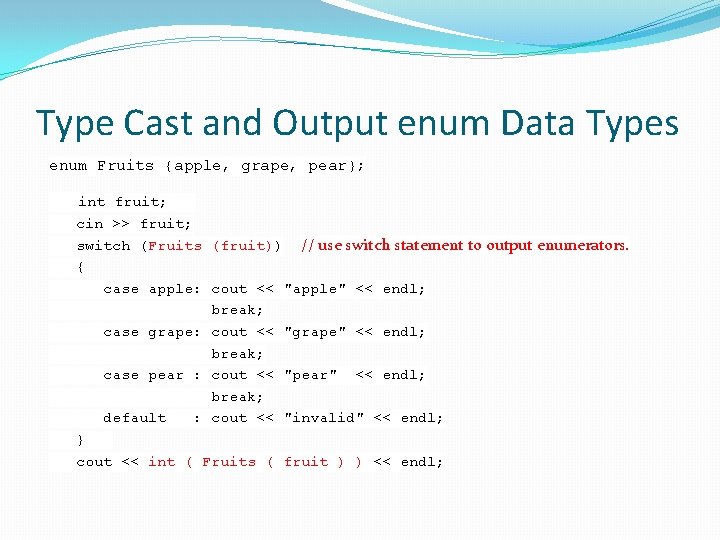
Type Cast and Output enum Data Types enum Fruits {apple, grape, pear}; int fruit; cin >> fruit; switch (Fruits (fruit)) // use switch statement to output enumerators. { case apple: cout << "apple" << endl; break; case grape: cout << "grape" << endl; break; case pear : cout << "pear" << endl; break; default : cout << "invalid" << endl; } cout << int ( Fruits ( fruit ) ) << endl;
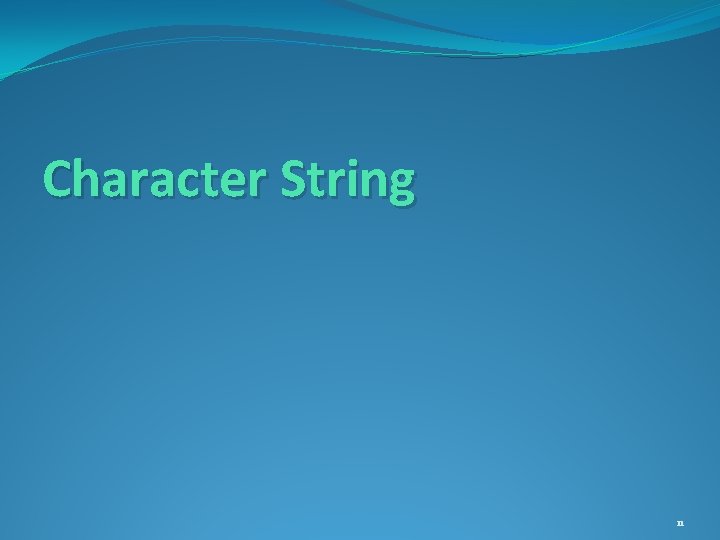
Character String 11
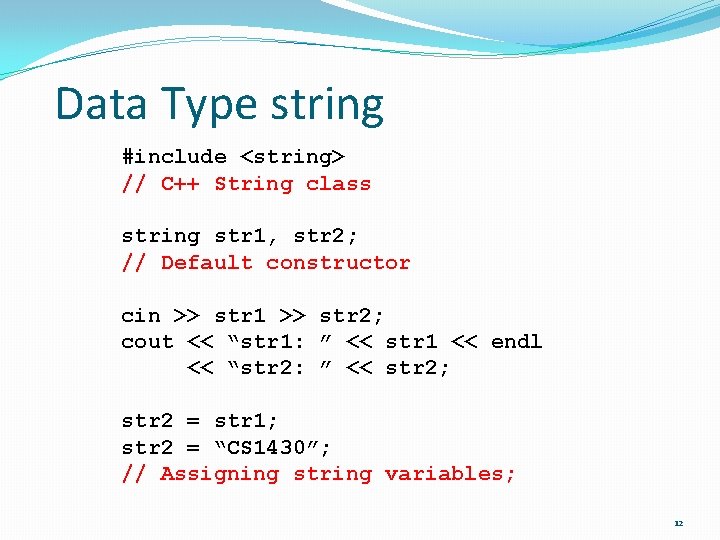
Data Type string #include <string> // C++ String class string str 1, str 2; // Default constructor cin >> str 1 >> str 2; cout << “str 1: ” << str 1 << endl << “str 2: ” << str 2; str 2 = str 1; str 2 = “CS 1430”; // Assigning string variables; 12
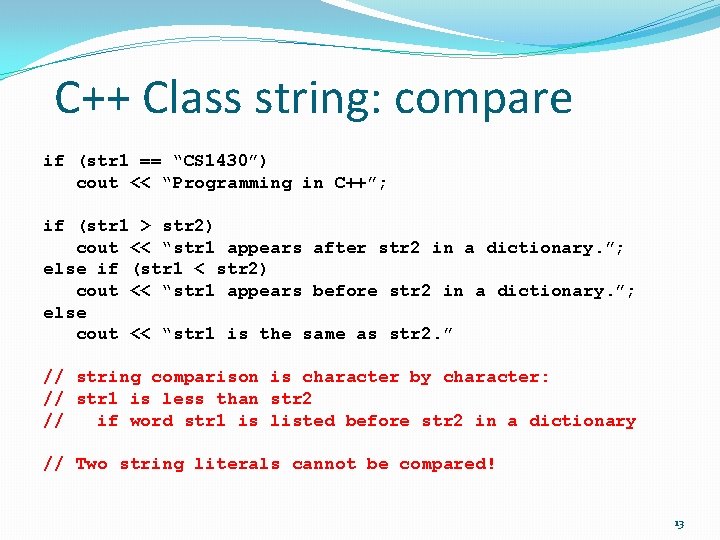
C++ Class string: compare if (str 1 == “CS 1430”) cout << “Programming in C++”; if (str 1 > str 2) cout << “str 1 appears after str 2 in a dictionary. ”; else if (str 1 < str 2) cout << “str 1 appears before str 2 in a dictionary. ”; else cout << “str 1 is the same as str 2. ” // string comparison is character by character: // str 1 is less than str 2 // if word str 1 is listed before str 2 in a dictionary // Two string literals cannot be compared! 13
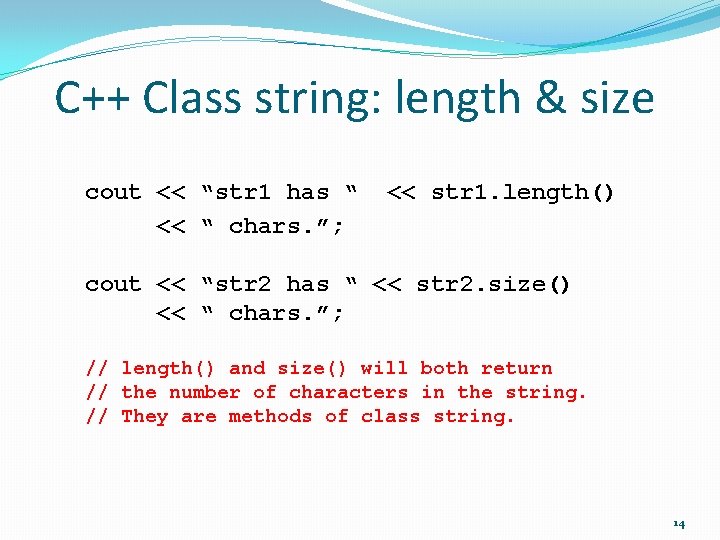
C++ Class string: length & size cout << “str 1 has “ << “ chars. ”; << str 1. length() cout << “str 2 has “ << str 2. size() << “ chars. ”; // length() and size() will both return // the number of characters in the string. // They are methods of class string. 14
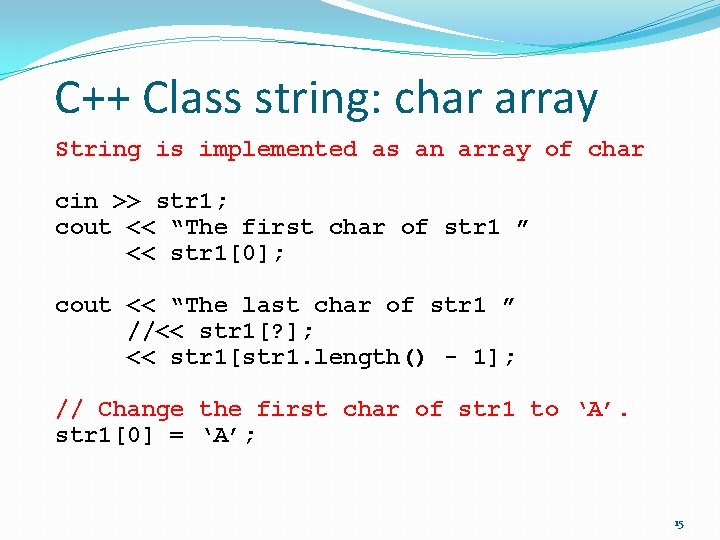
C++ Class string: char array String is implemented as an array of char cin >> str 1; cout << “The first char of str 1 ” << str 1[0]; cout << “The last char of str 1 ” //<< str 1[? ]; << str 1[str 1. length() - 1]; // Change the first char of str 1 to ‘A’. str 1[0] = ‘A’; 15
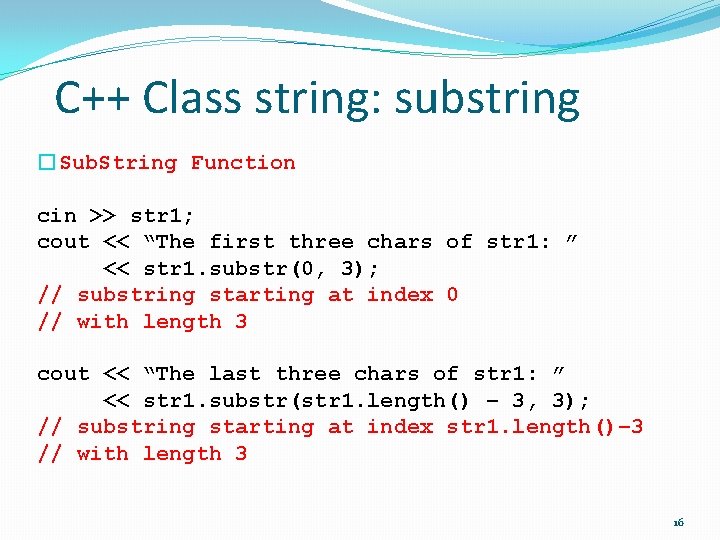
C++ Class string: substring �Sub. String Function cin >> str 1; cout << “The first three chars of str 1: ” << str 1. substr(0, 3); // substring starting at index 0 // with length 3 cout << “The last three chars of str 1: ” << str 1. substr(str 1. length() – 3, 3); // substring starting at index str 1. length()– 3 // with length 3 16
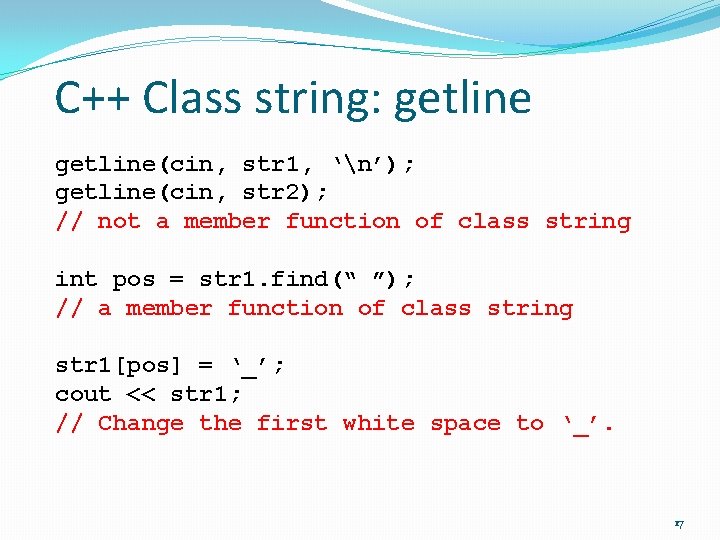
C++ Class string: getline(cin, str 1, ‘n’); getline(cin, str 2); // not a member function of class string int pos = str 1. find(“ ”); // a member function of class string str 1[pos] = ‘_’; cout << str 1; // Change the first white space to ‘_’. 17
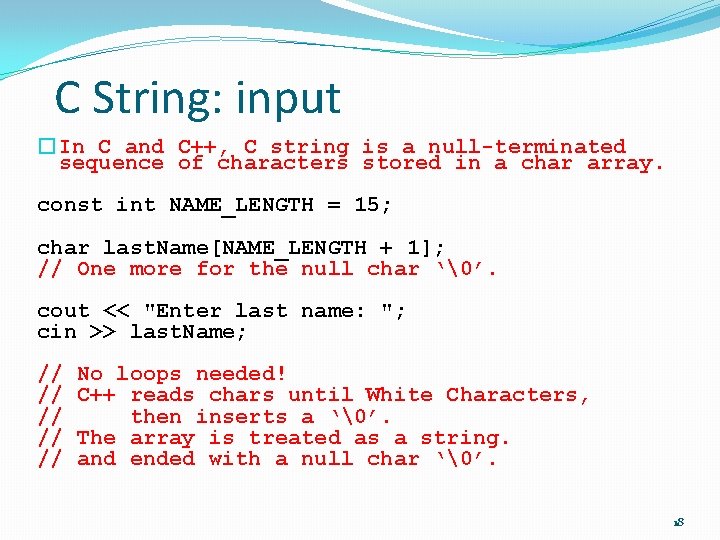
C String: input �In C and C++, C string is a null-terminated sequence of characters stored in a char array. const int NAME_LENGTH = 15; char last. Name[NAME_LENGTH + 1]; // One more for the null char ‘ ’. cout << "Enter last name: "; cin >> last. Name; // // // No loops needed! C++ reads chars until White Characters, then inserts a ‘ ’. The array is treated as a string. and ended with a null char ‘ ’. 18
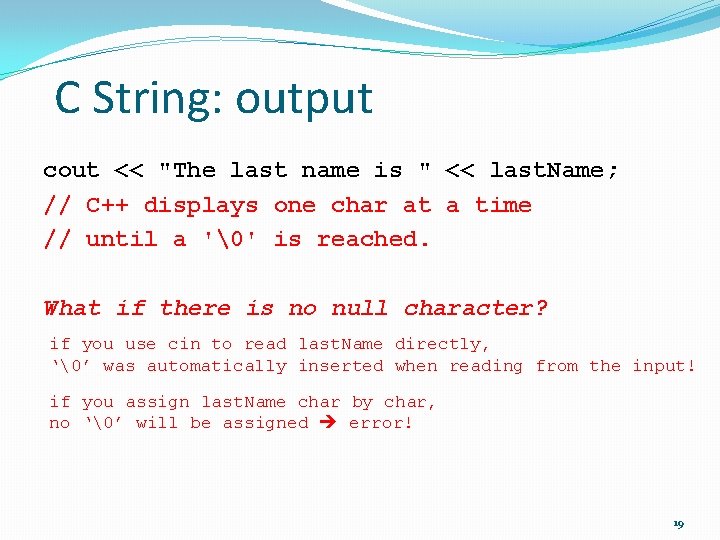
C String: output cout << "The last name is " << last. Name; // C++ displays one char at a time // until a '