ch 012 POSIX Threads Ju Hong Taek Computer
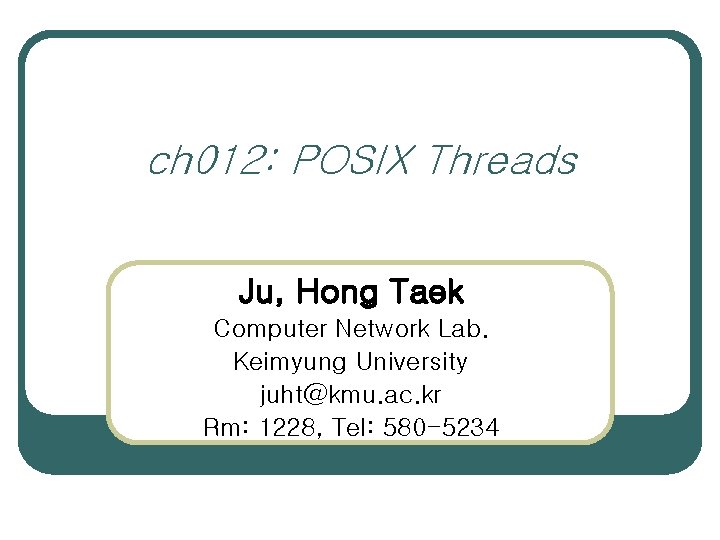
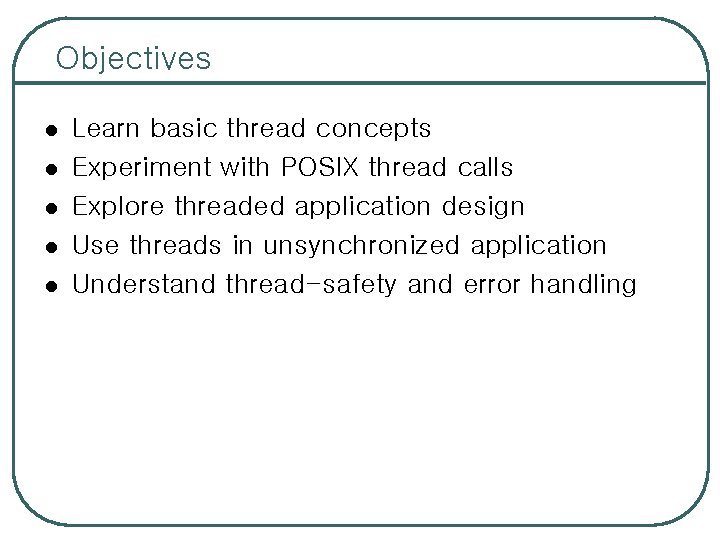
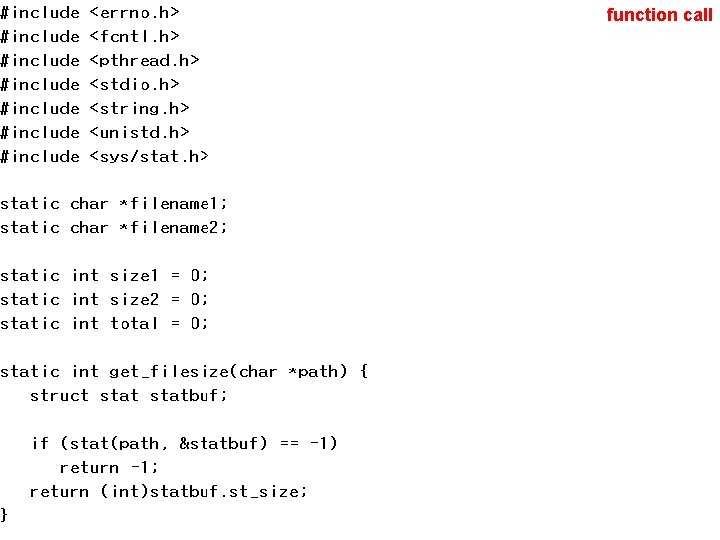
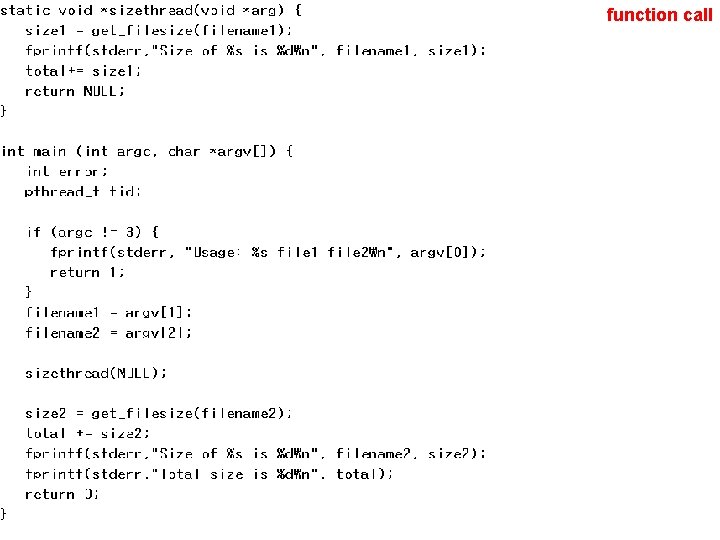
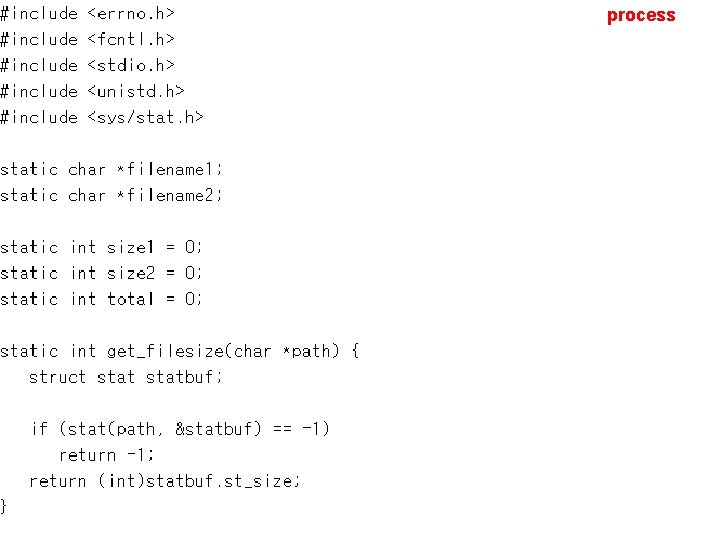
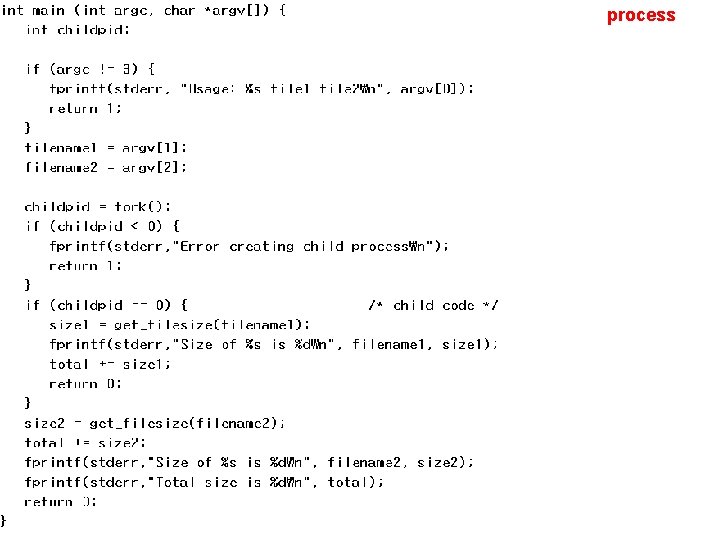
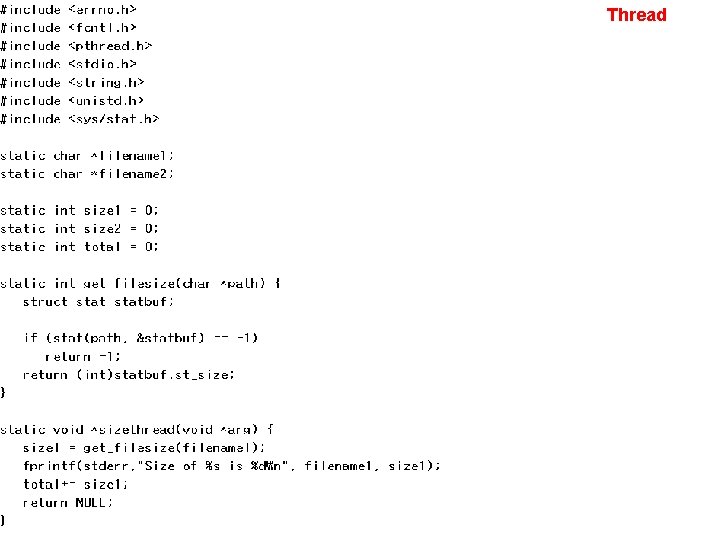
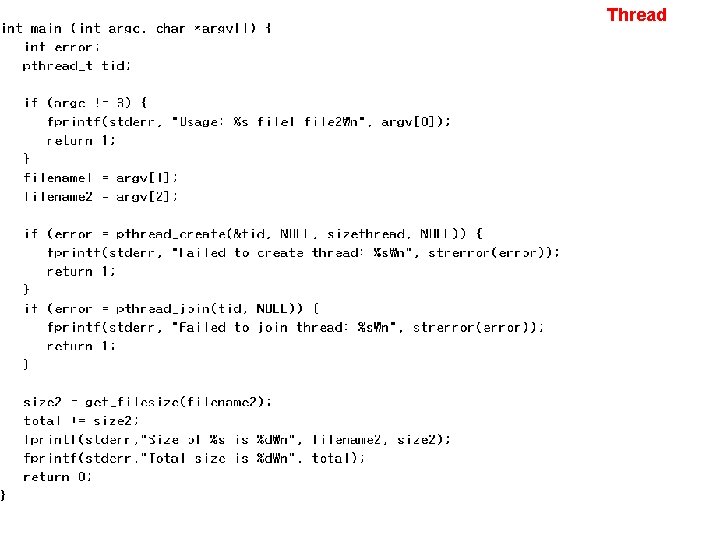
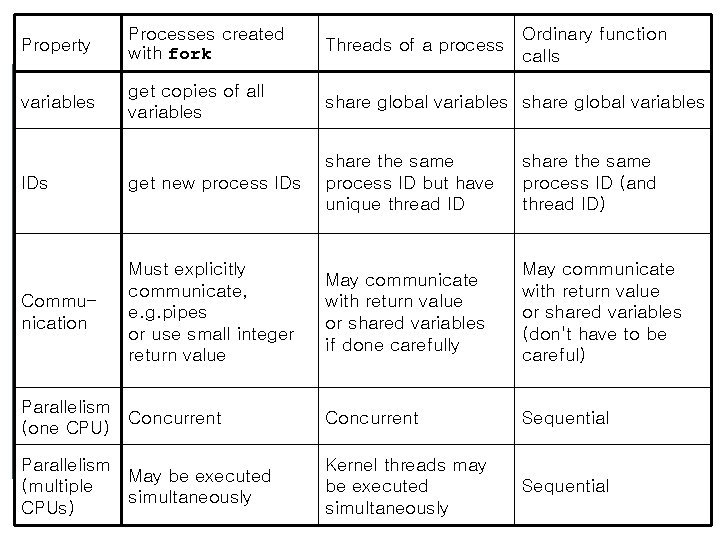
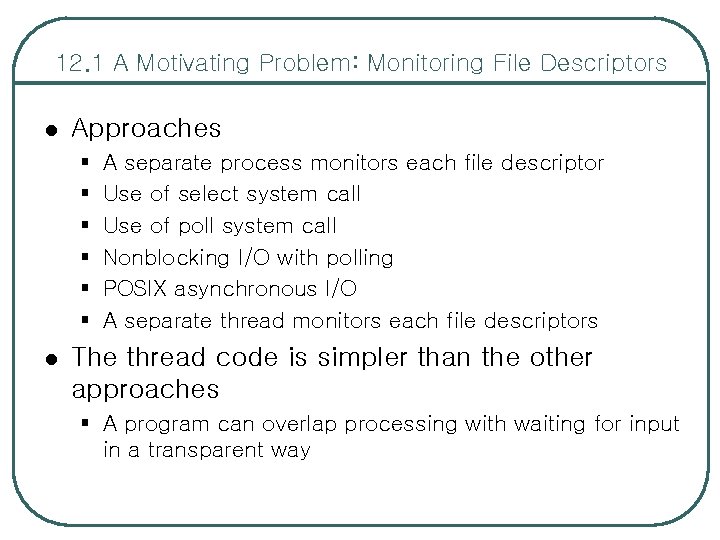
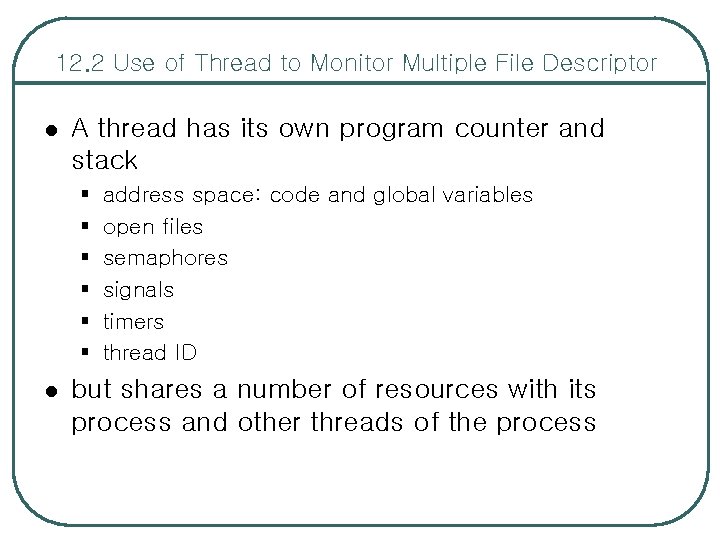
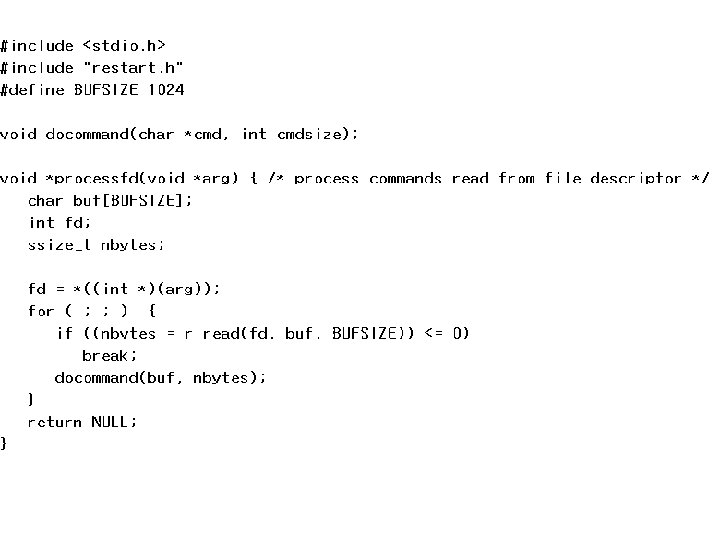
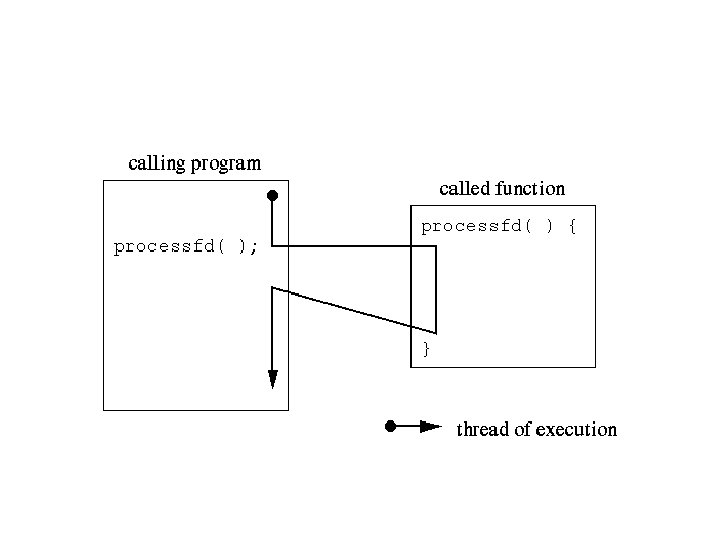
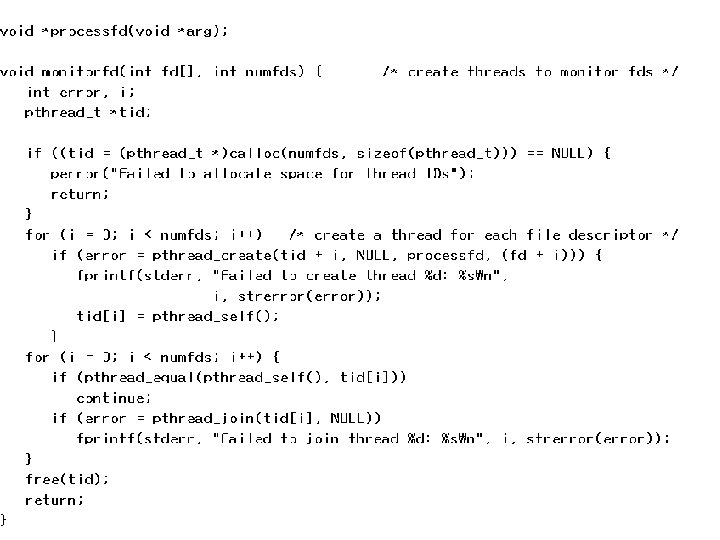
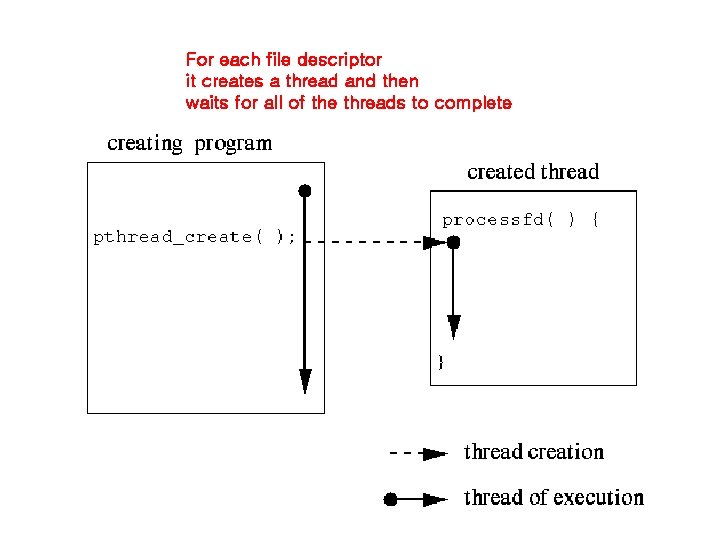
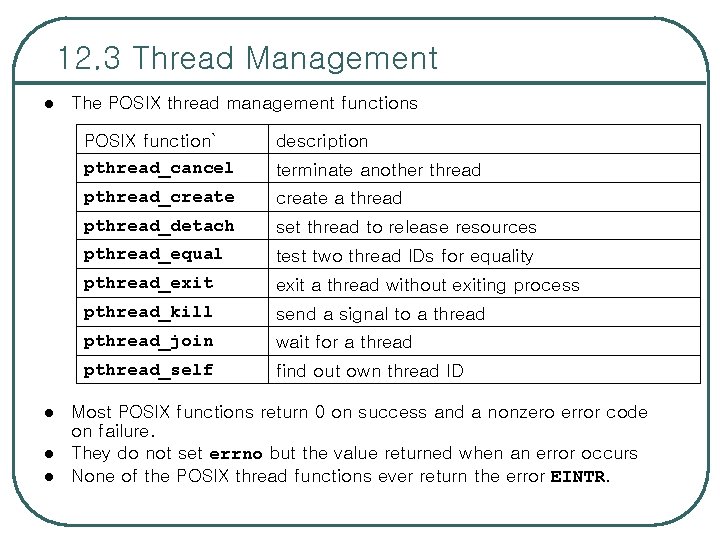
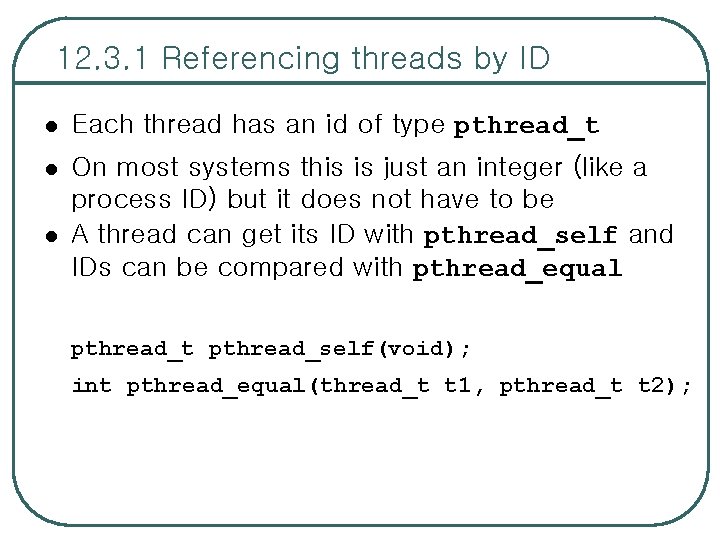
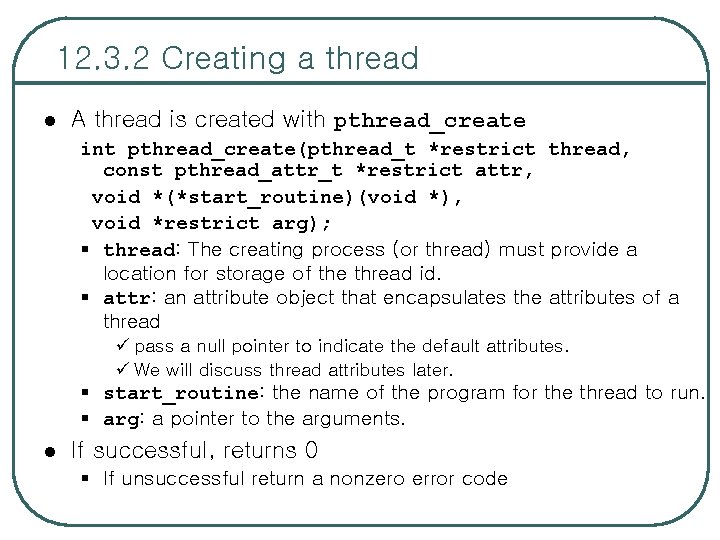
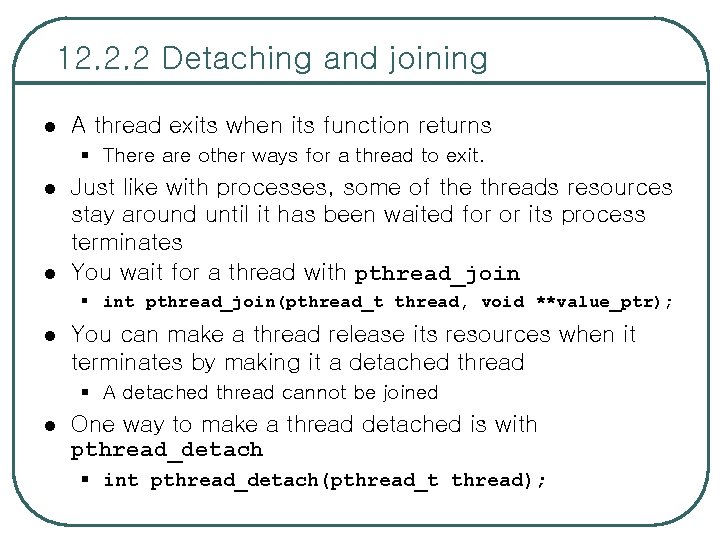
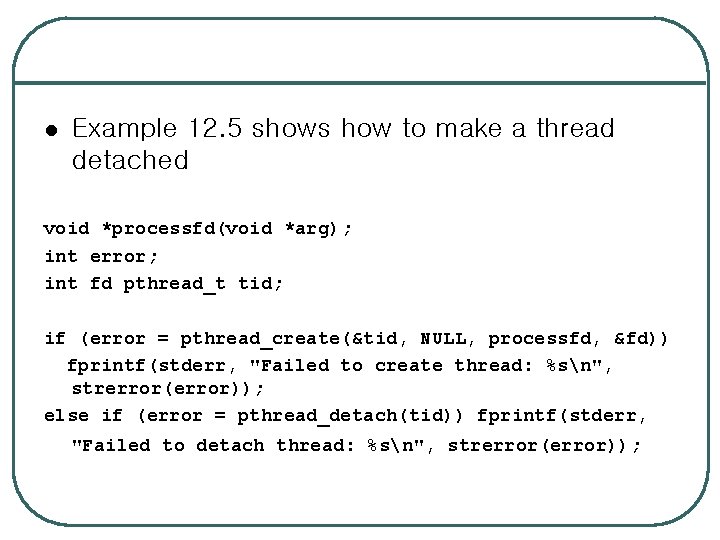
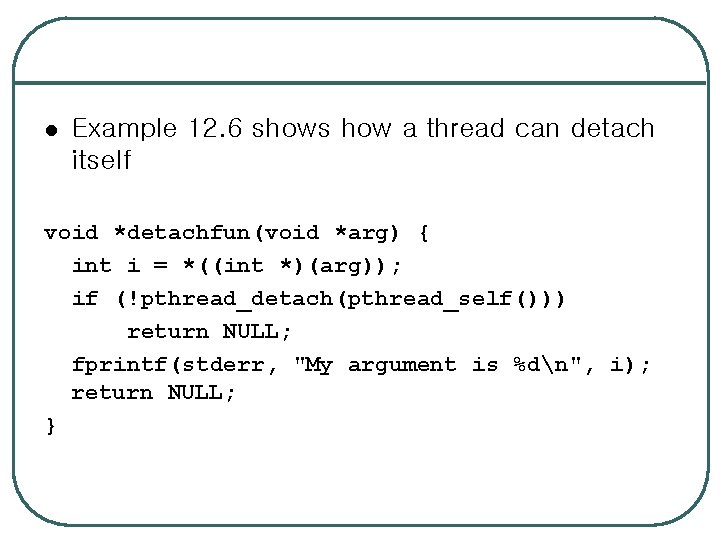
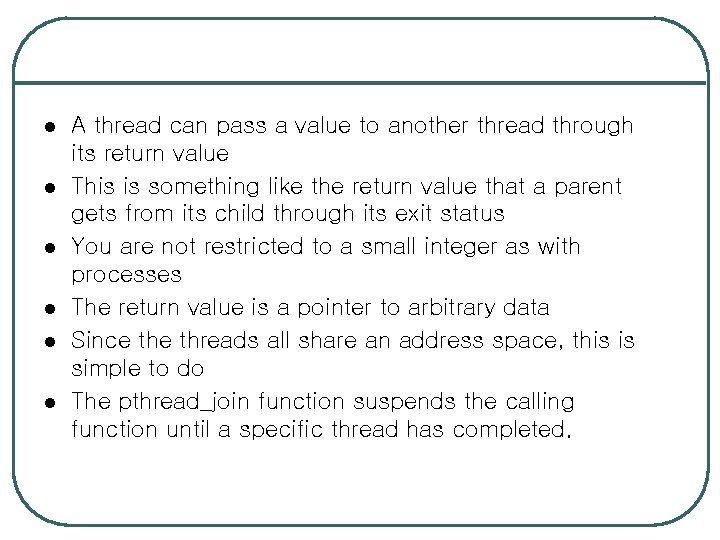
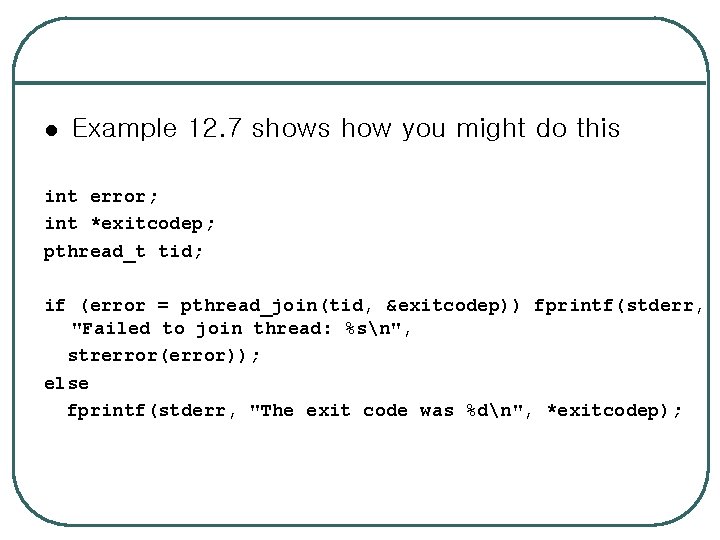
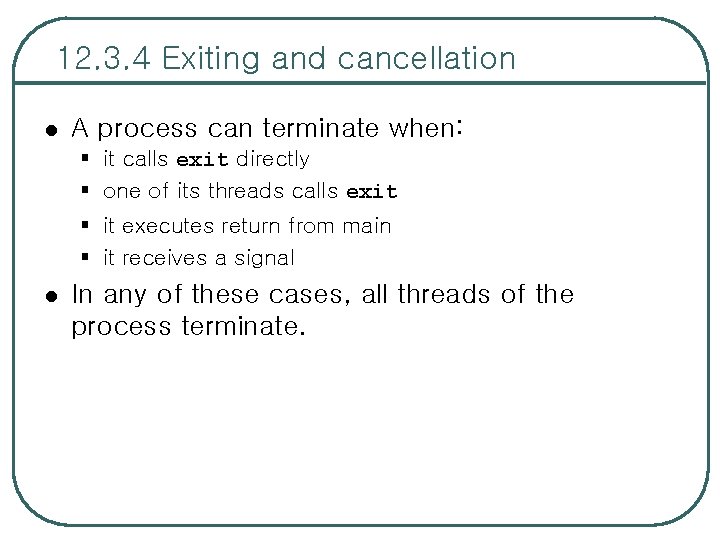
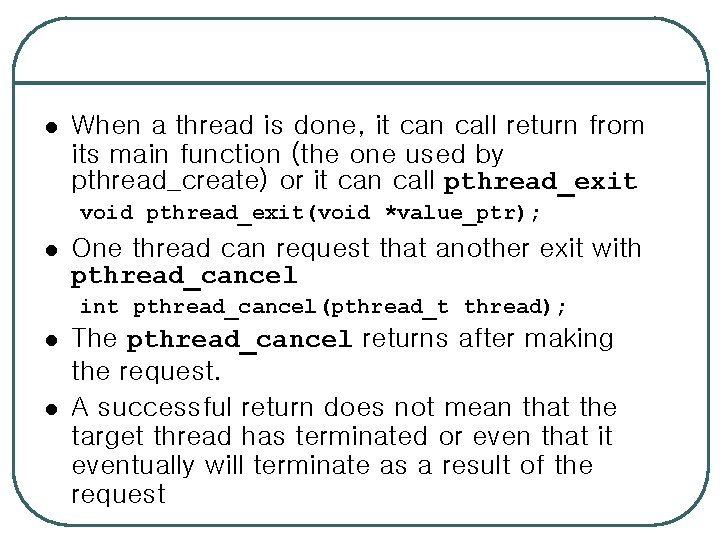
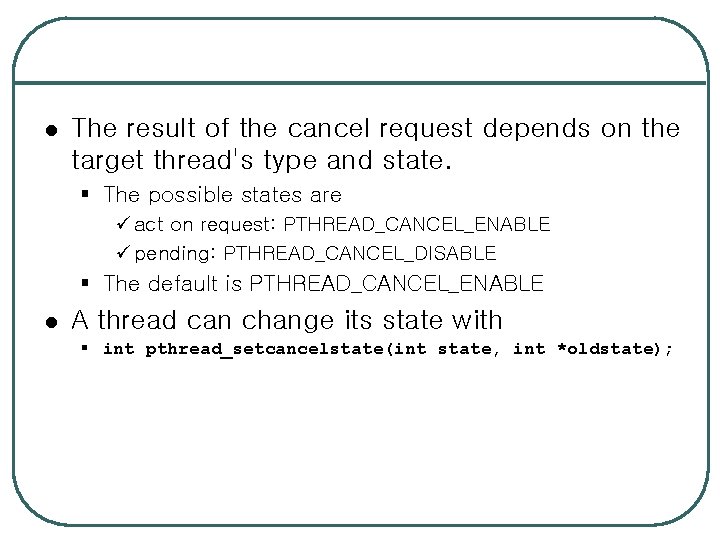
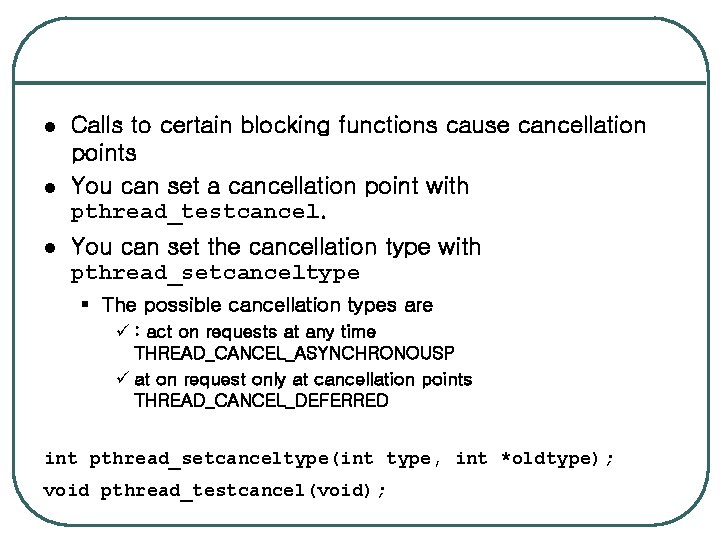
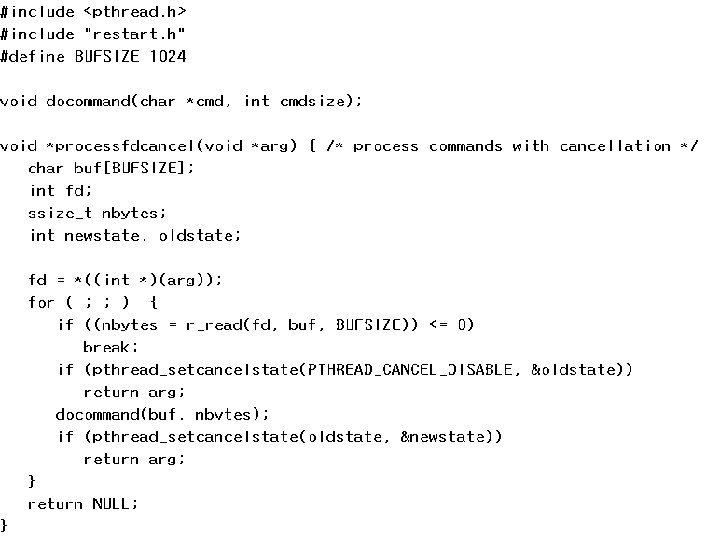
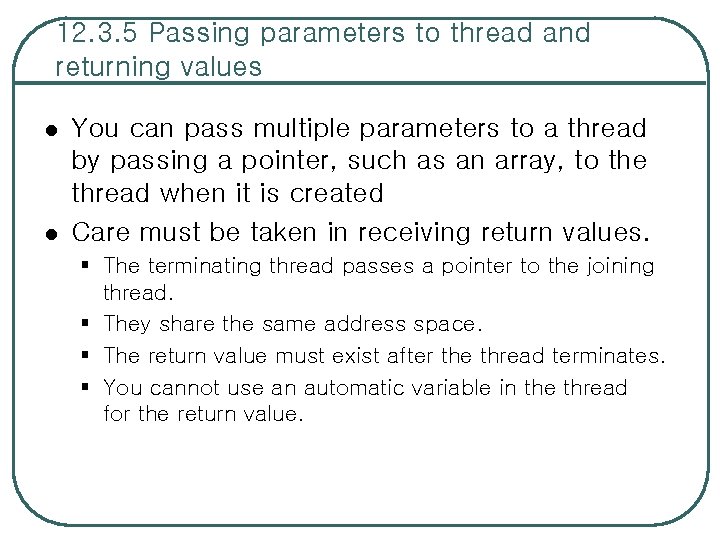
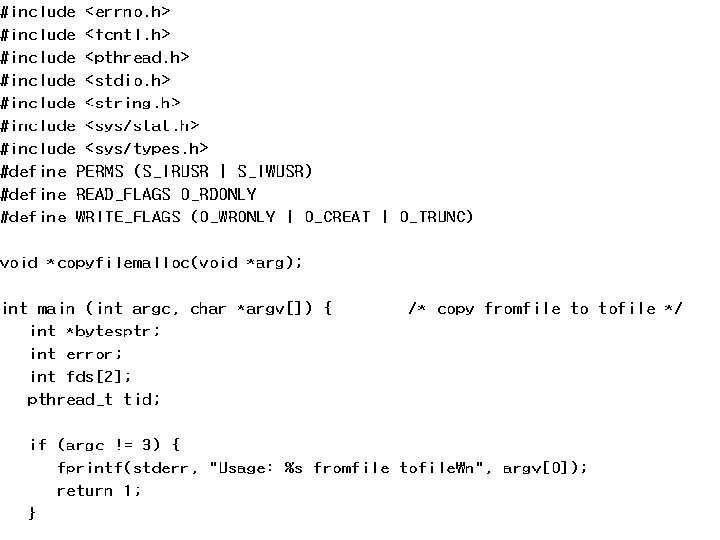
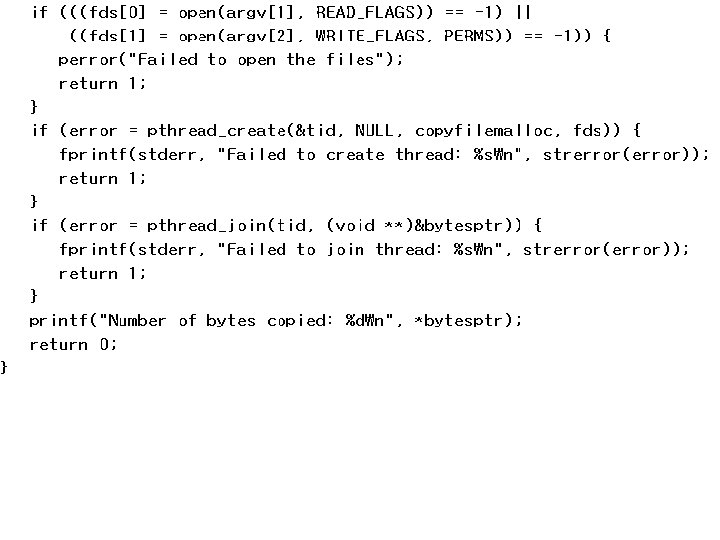
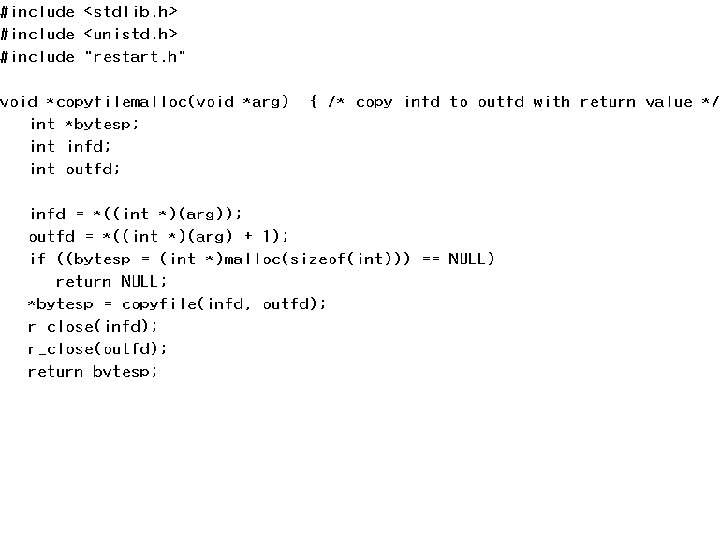
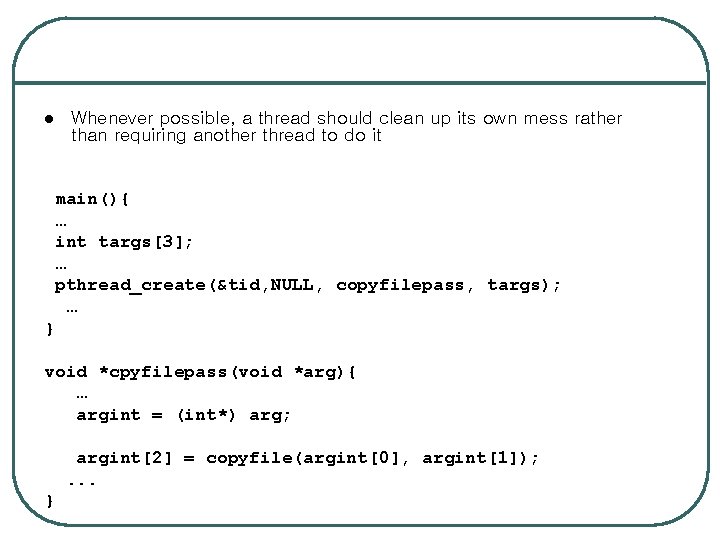
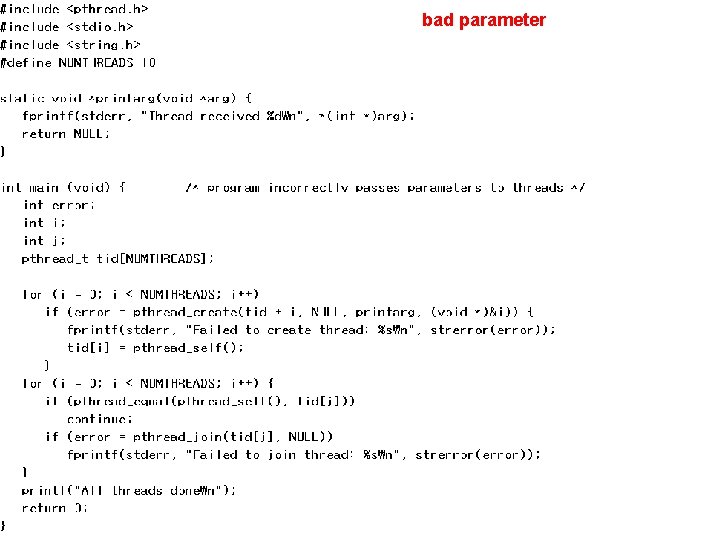
![void *whichexit(void *arg) { int n; int np 1[1]; int *np 2; char s void *whichexit(void *arg) { int n; int np 1[1]; int *np 2; char s](https://slidetodoc.com/presentation_image_h2/8f5d3095748c4a2e9a35450258782bfe/image-35.jpg)
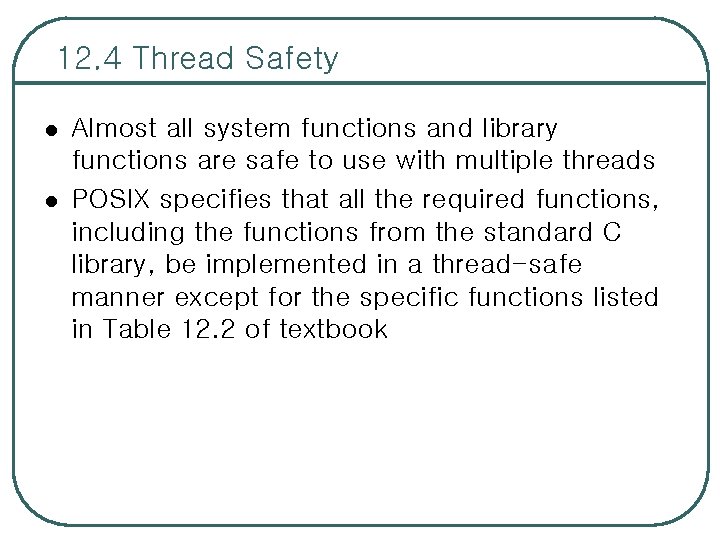
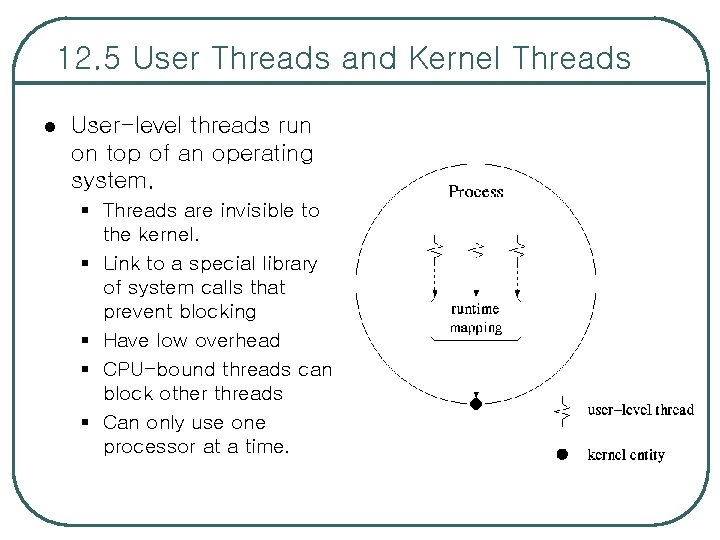
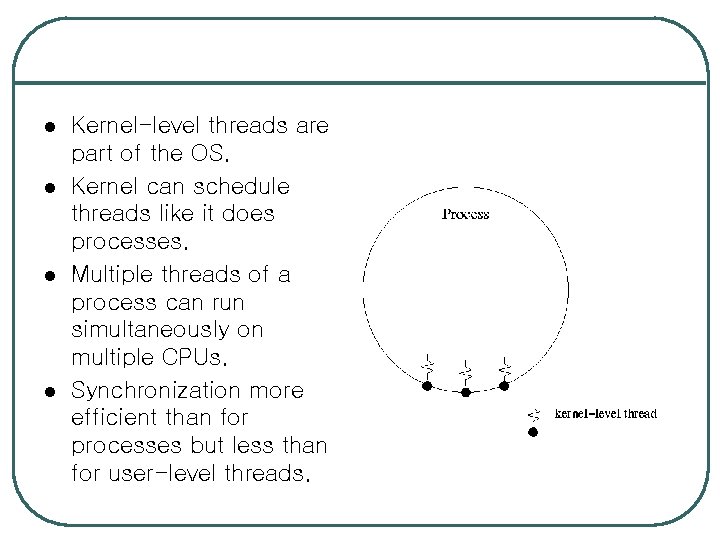
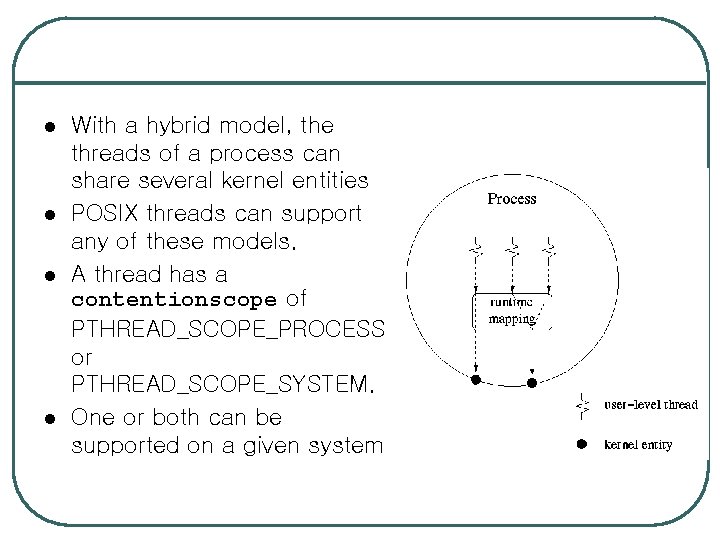
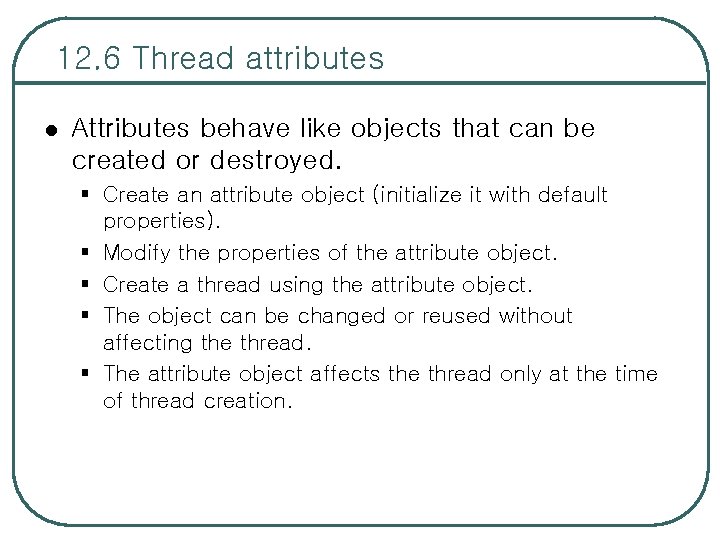
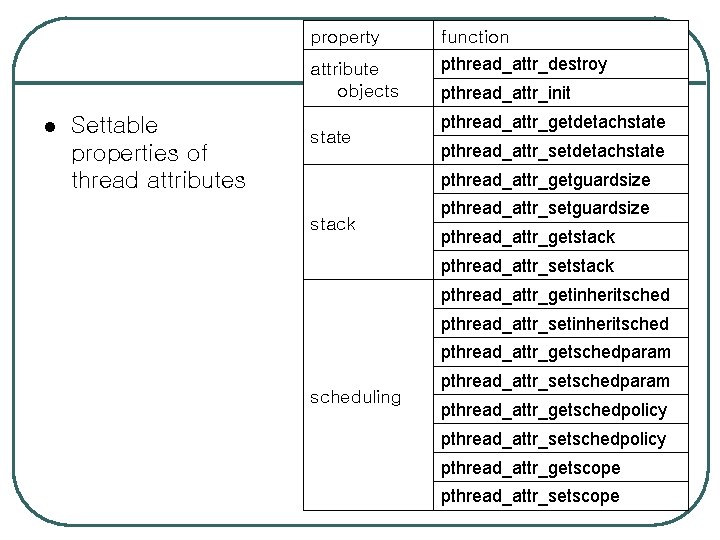
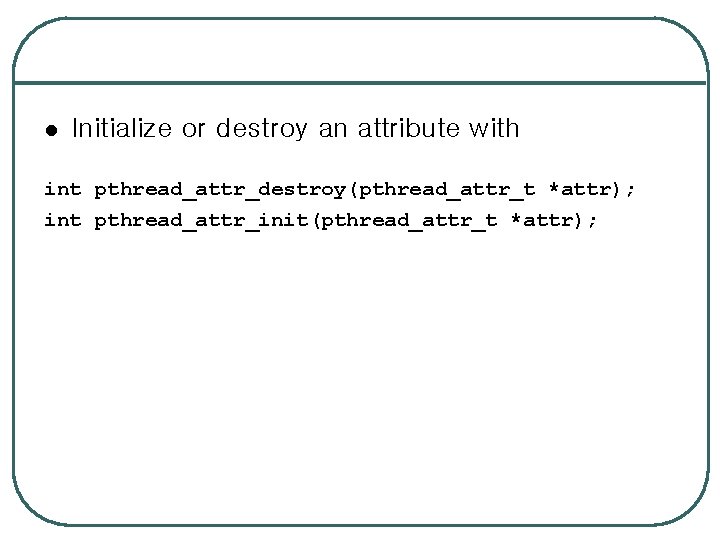
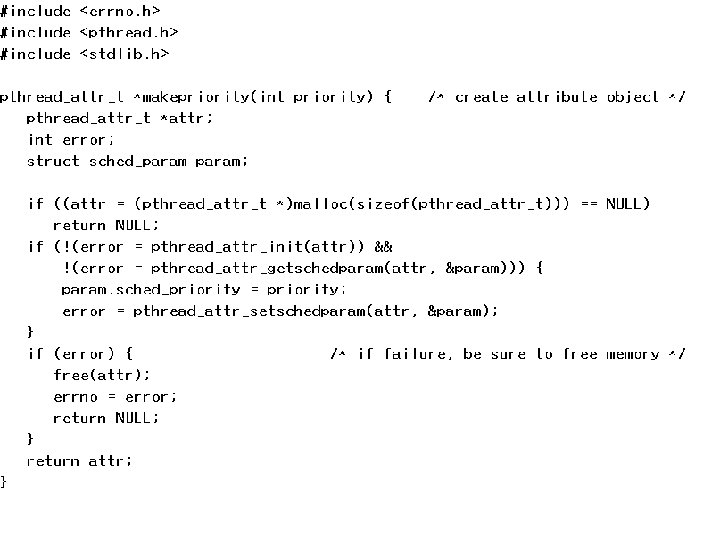
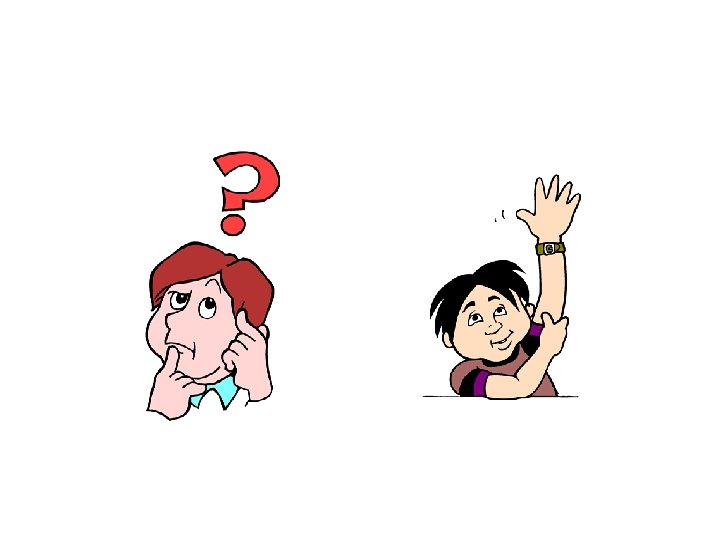
- Slides: 44
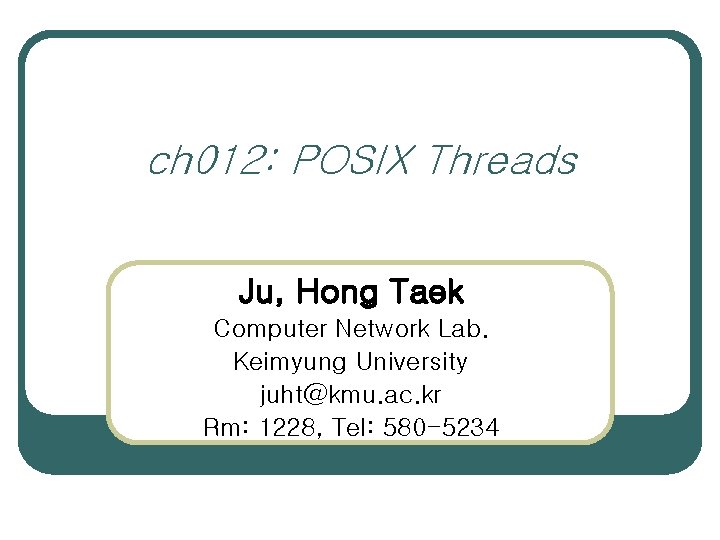
ch 012: POSIX Threads Ju, Hong Taek Computer Network Lab. Keimyung University juht@kmu. ac. kr Rm: 1228, Tel: 580 -5234
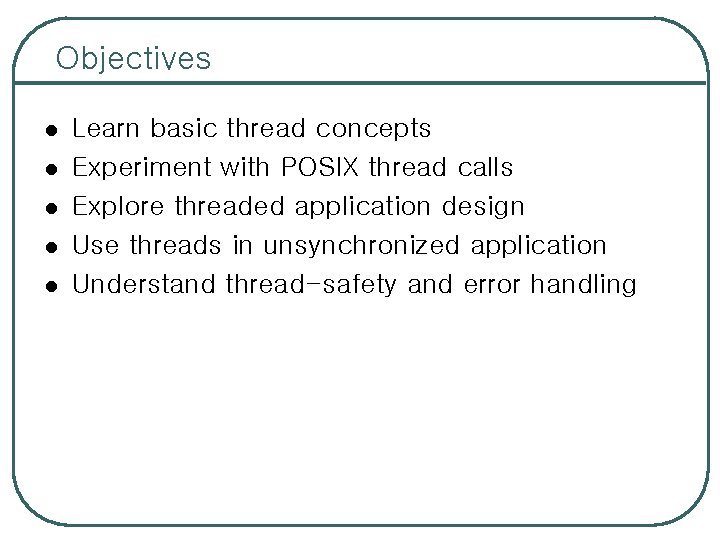
Objectives l l l Learn basic thread concepts Experiment with POSIX thread calls Explore threaded application design Use threads in unsynchronized application Understand thread-safety and error handling
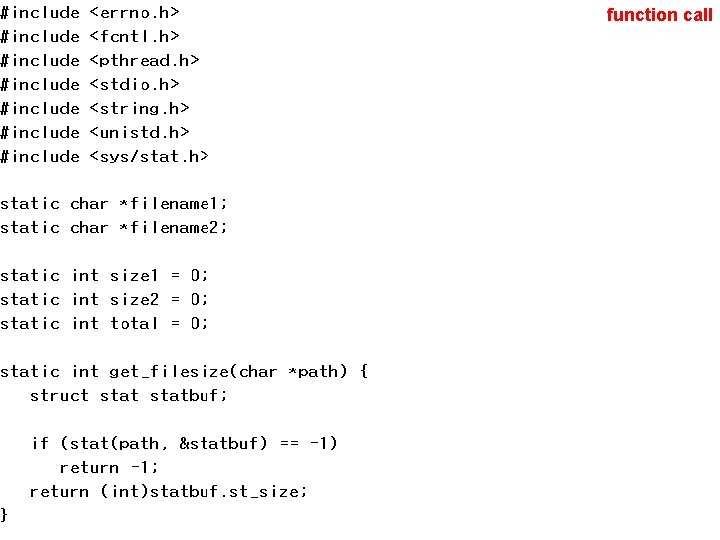
function call
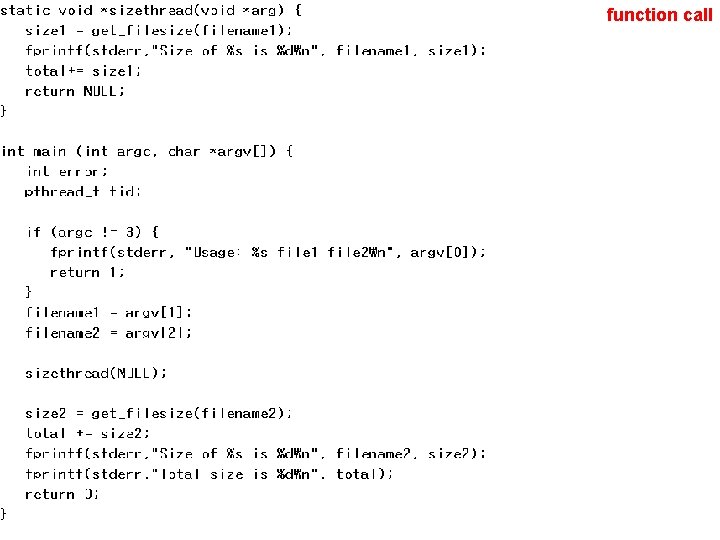
function call
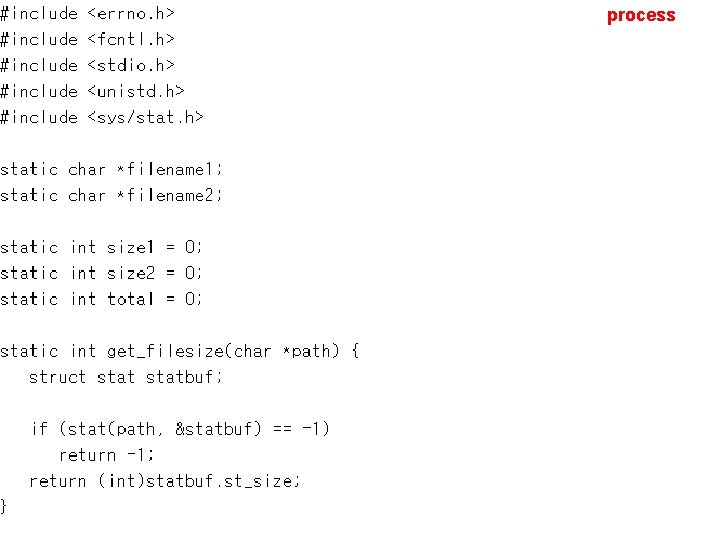
process
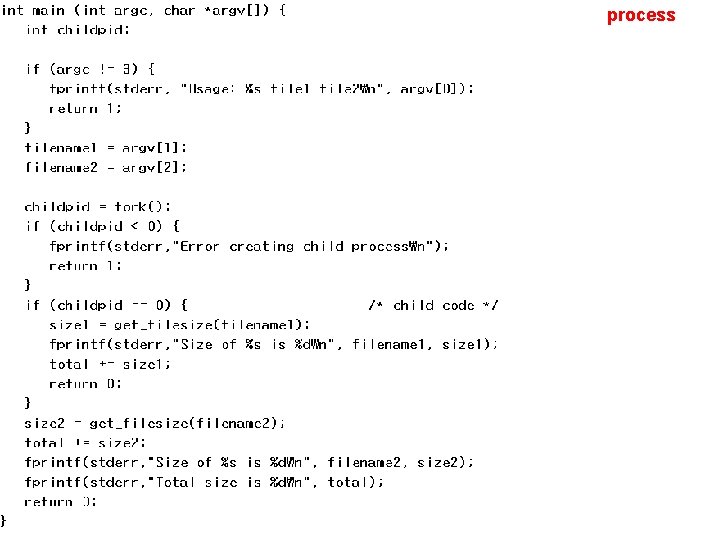
process
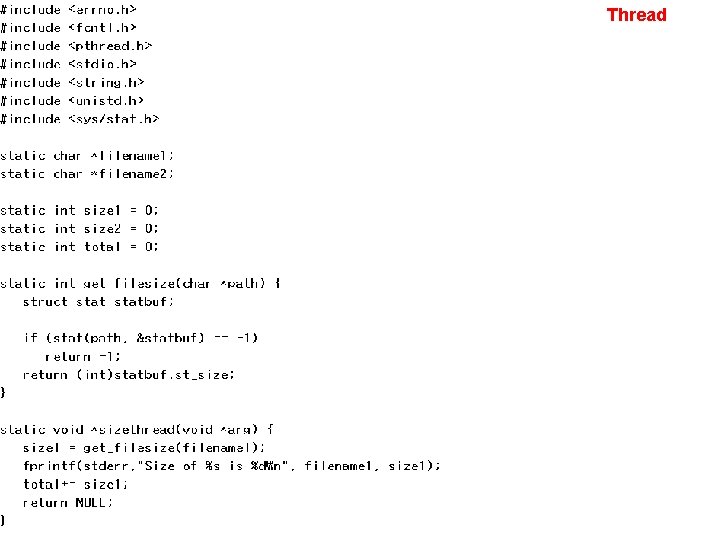
Thread
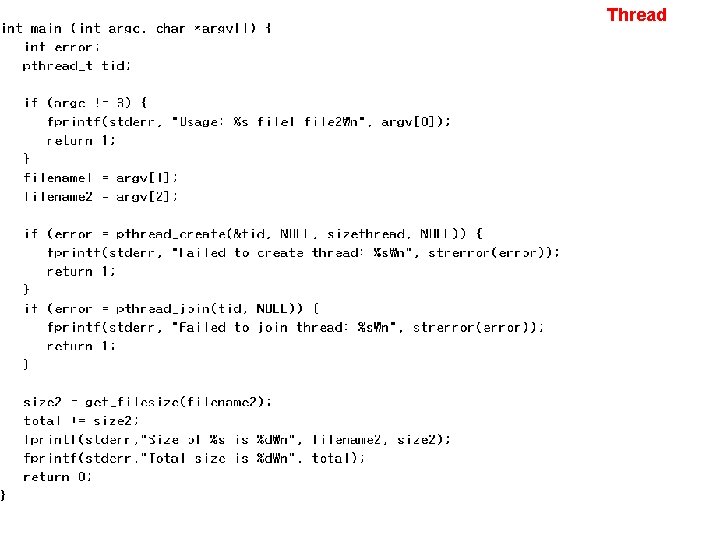
Thread
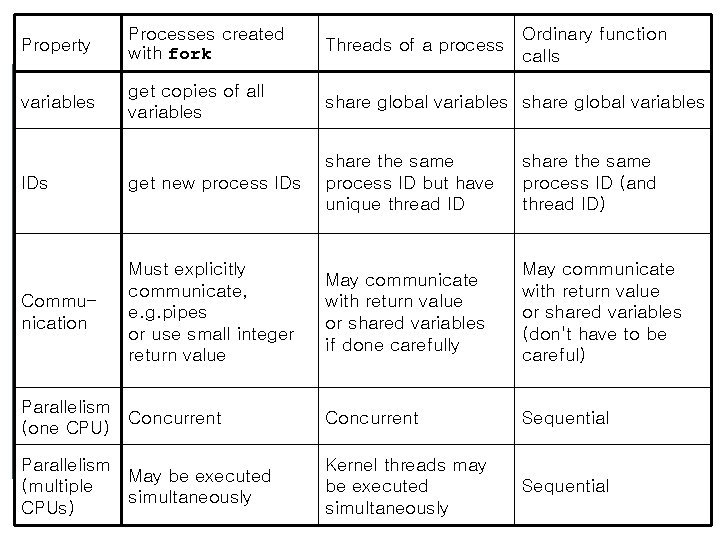
Ordinary function calls Property Processes created with fork Threads of a process variables get copies of all variables share global variables IDs get new process IDs share the same process ID but have unique thread ID share the same process ID (and thread ID) Communication Must explicitly communicate, e. g. pipes or use small integer return value May communicate with return value or shared variables if done carefully May communicate with return value or shared variables (don't have to be careful) Parallelism Concurrent (one CPU) Concurrent Sequential Parallelism May be executed (multiple simultaneously CPUs) Kernel threads may be executed simultaneously Sequential
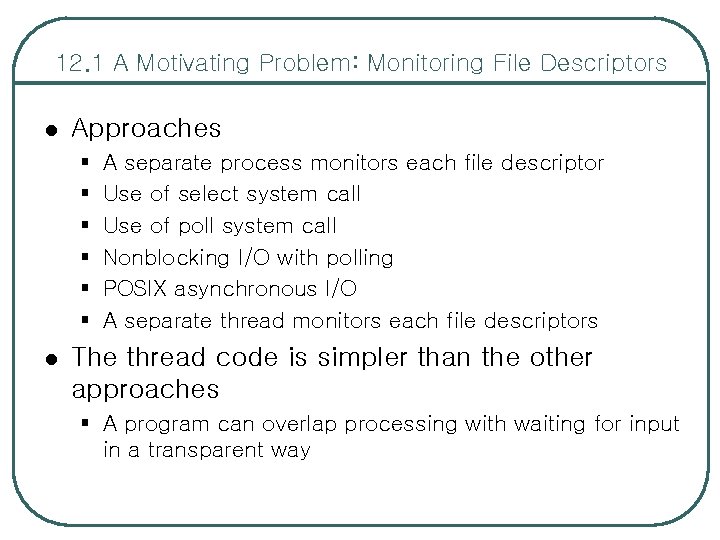
12. 1 A Motivating Problem: Monitoring File Descriptors l Approaches § § § l A separate process monitors each file descriptor Use of select system call Use of poll system call Nonblocking I/O with polling POSIX asynchronous I/O A separate thread monitors each file descriptors The thread code is simpler than the other approaches § A program can overlap processing with waiting for input in a transparent way
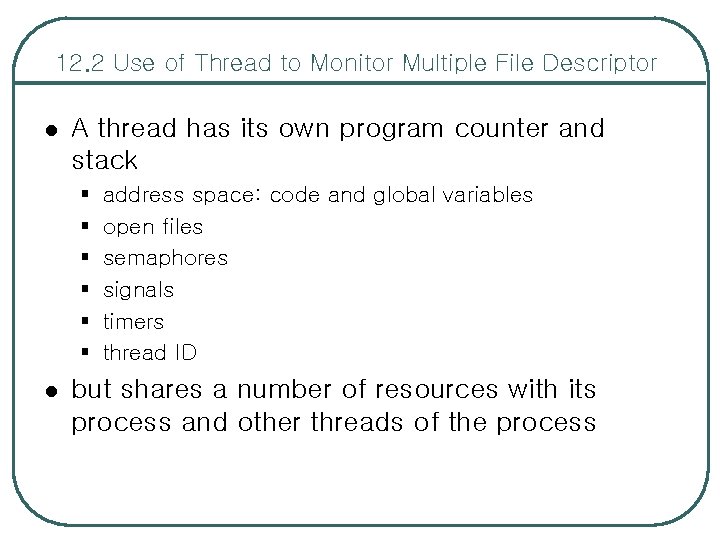
12. 2 Use of Thread to Monitor Multiple File Descriptor l A thread has its own program counter and stack § § § l address space: code and global variables open files semaphores signals timers thread ID but shares a number of resources with its process and other threads of the process
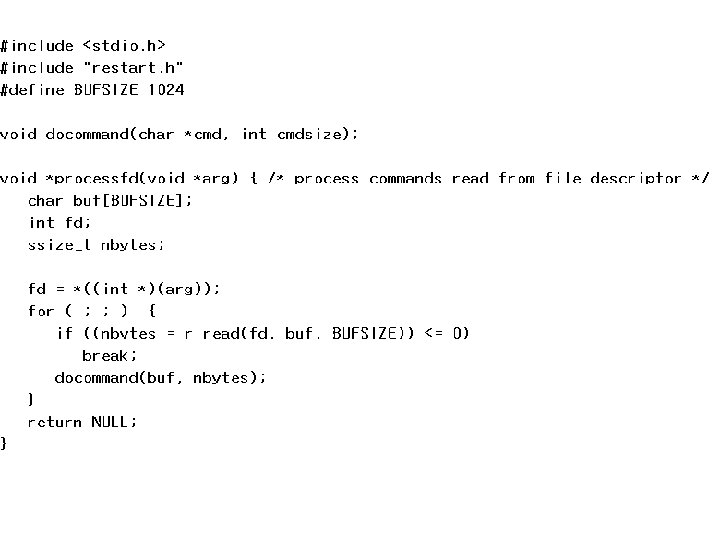
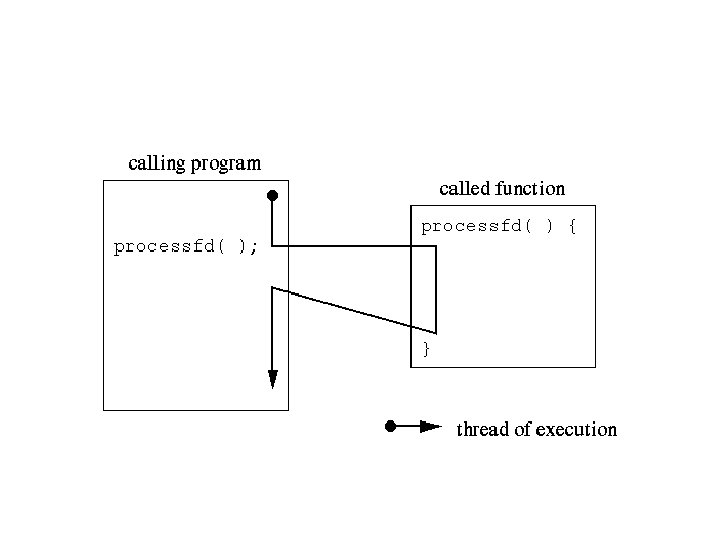
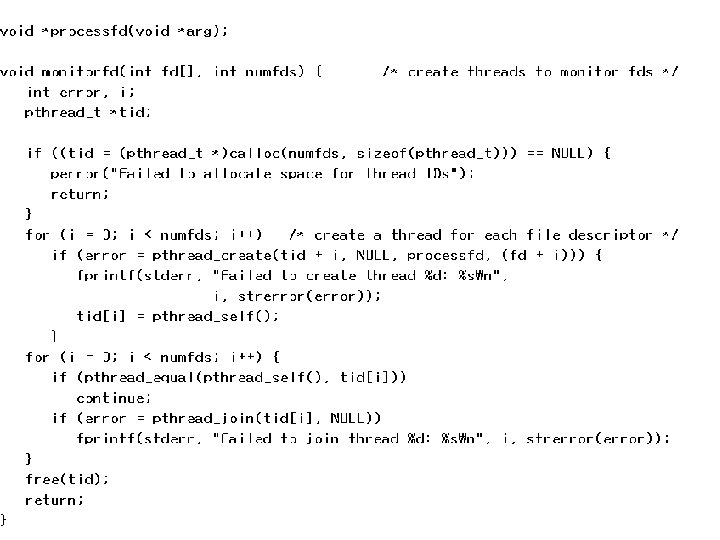
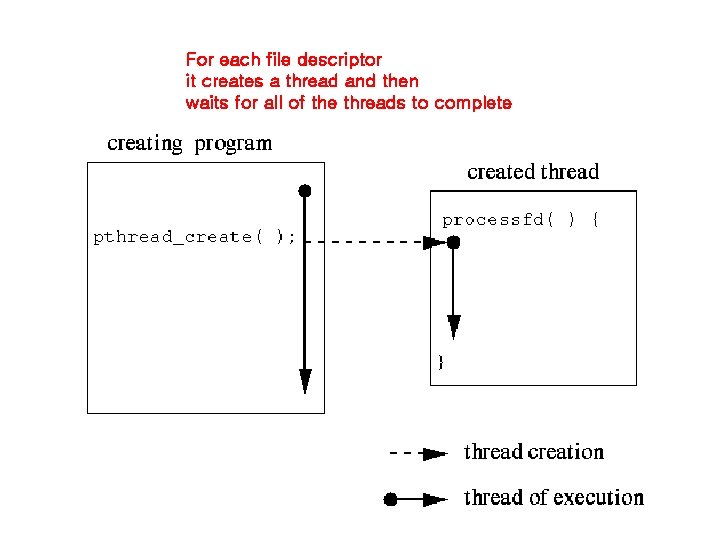
For each file descriptor it creates a thread and then waits for all of the threads to complete
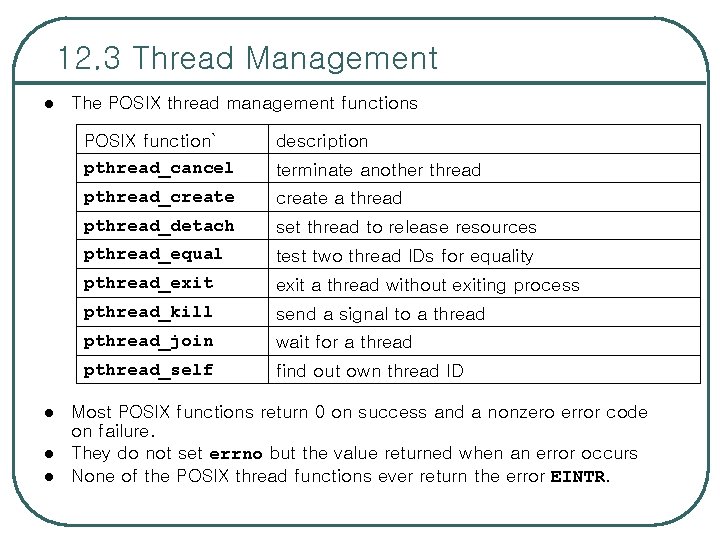
12. 3 Thread Management l l The POSIX thread management functions POSIX function` pthread_cancel description pthread_create a thread pthread_detach set thread to release resources pthread_equal test two thread IDs for equality pthread_exit a thread without exiting process pthread_kill send a signal to a thread pthread_join wait for a thread pthread_self find out own thread ID terminate another thread Most POSIX functions return 0 on success and a nonzero error code on failure. They do not set errno but the value returned when an error occurs None of the POSIX thread functions ever return the error EINTR.
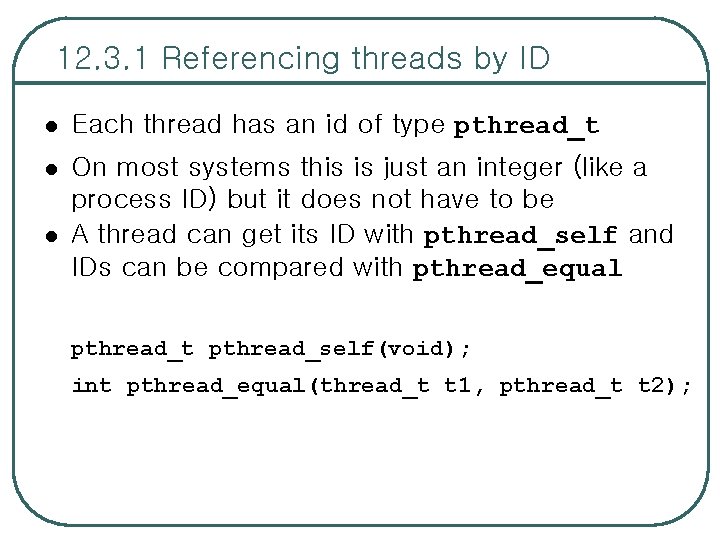
12. 3. 1 Referencing threads by ID l Each thread has an id of type pthread_t l On most systems this is just an integer (like a process ID) but it does not have to be A thread can get its ID with pthread_self and IDs can be compared with pthread_equal l pthread_t pthread_self(void); int pthread_equal(thread_t t 1, pthread_t t 2);
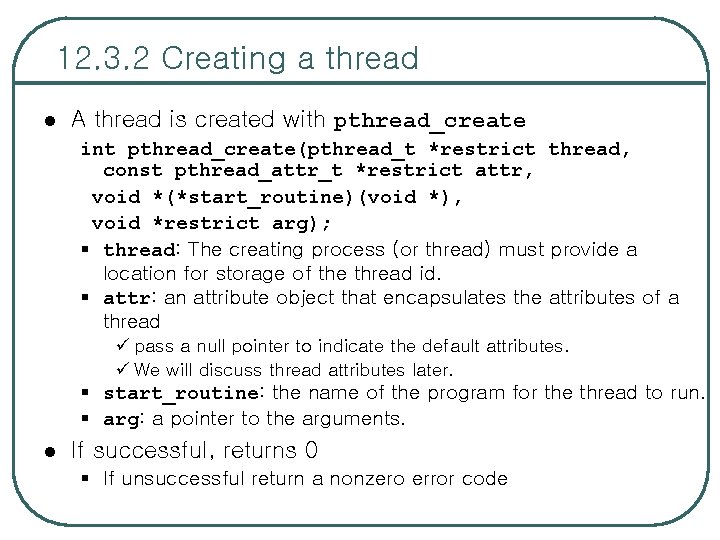
12. 3. 2 Creating a thread l A thread is created with pthread_create int pthread_create(pthread_t *restrict thread, const pthread_attr_t *restrict attr, void *(*start_routine)(void *), void *restrict arg); § thread: The creating process (or thread) must provide a location for storage of the thread id. § attr: an attribute object that encapsulates the attributes of a thread ü pass a null pointer to indicate the default attributes. ü We will discuss thread attributes later. § start_routine: the name of the program for the thread to run. § arg: a pointer to the arguments. l If successful, returns 0 § If unsuccessful return a nonzero error code
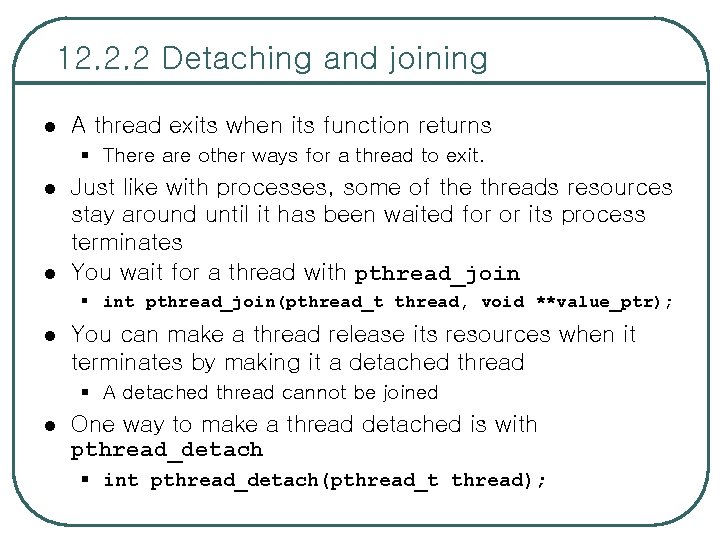
12. 2. 2 Detaching and joining l A thread exits when its function returns § There are other ways for a thread to exit. l l Just like with processes, some of the threads resources stay around until it has been waited for or its process terminates You wait for a thread with pthread_join § int pthread_join(pthread_t thread, void **value_ptr); l You can make a thread release its resources when it terminates by making it a detached thread § A detached thread cannot be joined l One way to make a thread detached is with pthread_detach § int pthread_detach(pthread_t thread);
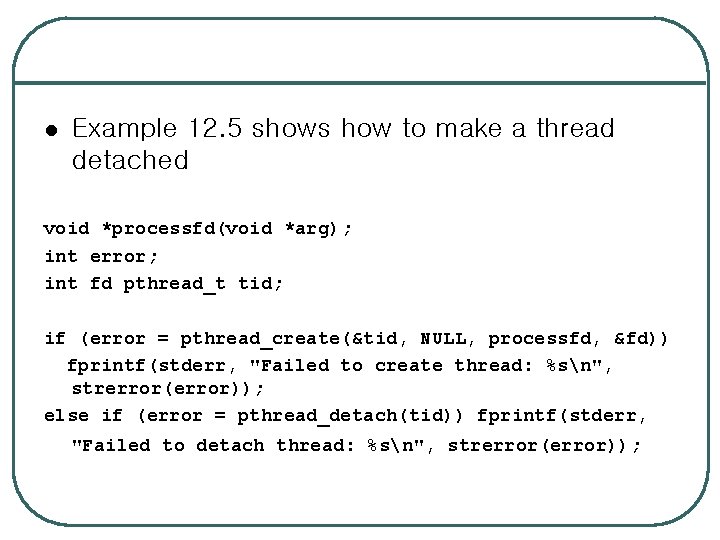
l Example 12. 5 shows how to make a thread detached void *processfd(void *arg); int error; int fd pthread_t tid; if (error = pthread_create(&tid, NULL, processfd, &fd)) fprintf(stderr, "Failed to create thread: %sn", strerror(error)); else if (error = pthread_detach(tid)) fprintf(stderr, "Failed to detach thread: %sn", strerror(error));
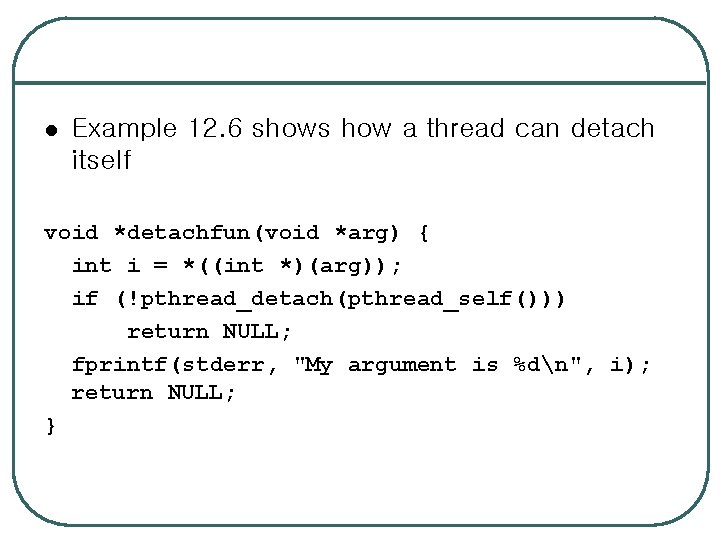
l Example 12. 6 shows how a thread can detach itself void *detachfun(void *arg) { int i = *((int *)(arg)); if (!pthread_detach(pthread_self())) return NULL; fprintf(stderr, "My argument is %dn", i); return NULL; }
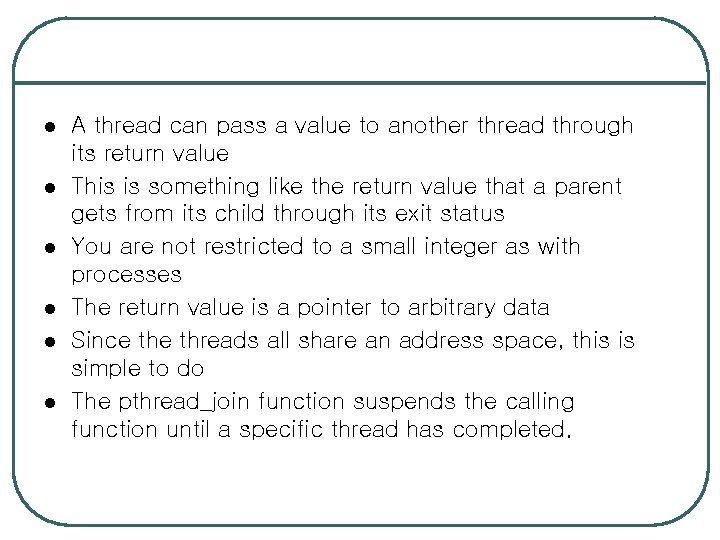
l l l A thread can pass a value to another thread through its return value This is something like the return value that a parent gets from its child through its exit status You are not restricted to a small integer as with processes The return value is a pointer to arbitrary data Since threads all share an address space, this is simple to do The pthread_join function suspends the calling function until a specific thread has completed.
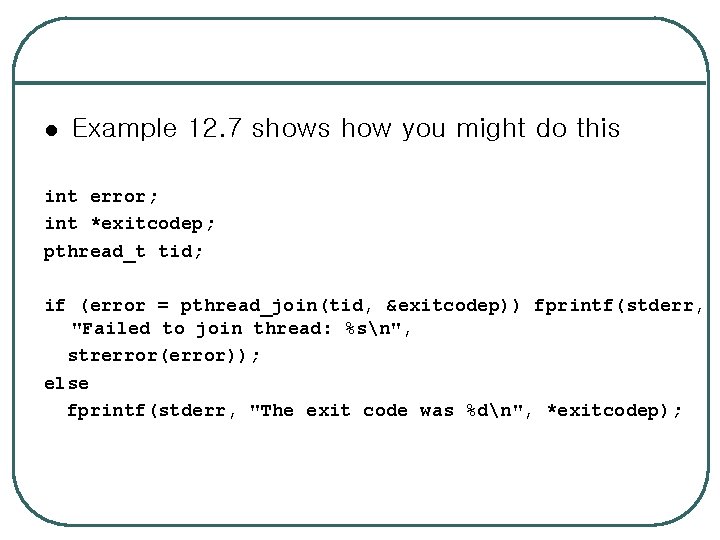
l Example 12. 7 shows how you might do this int error; int *exitcodep; pthread_t tid; if (error = pthread_join(tid, &exitcodep)) fprintf(stderr, "Failed to join thread: %sn", strerror(error)); else fprintf(stderr, "The exit code was %dn", *exitcodep);
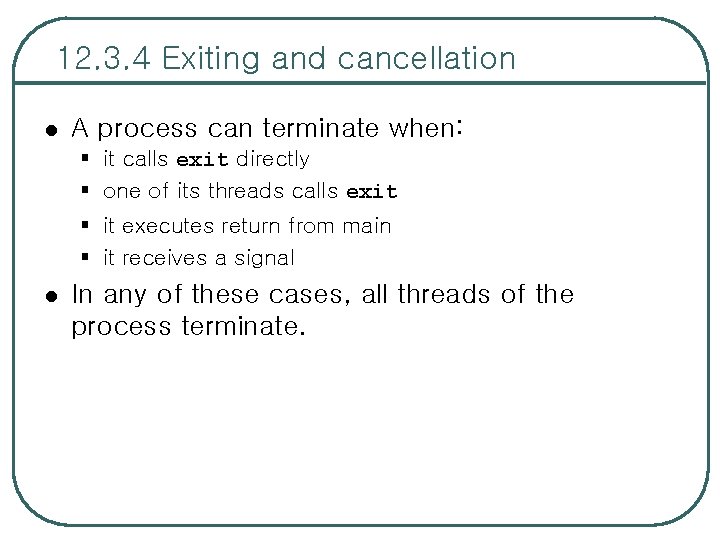
12. 3. 4 Exiting and cancellation l A process can terminate when: § it calls exit directly § one of its threads calls exit § it executes return from main § it receives a signal l In any of these cases, all threads of the process terminate.
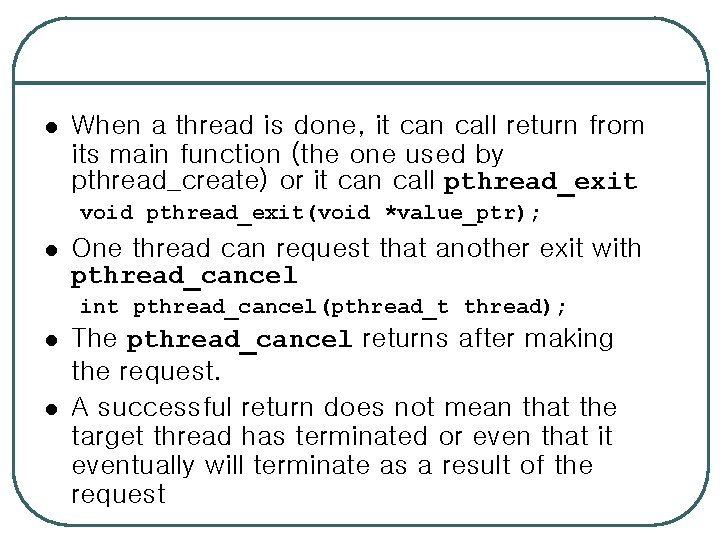
l When a thread is done, it can call return from its main function (the one used by pthread_create) or it can call pthread_exit void pthread_exit(void *value_ptr); l One thread can request that another exit with pthread_cancel int pthread_cancel(pthread_t thread); l l The pthread_cancel returns after making the request. A successful return does not mean that the target thread has terminated or even that it eventually will terminate as a result of the request
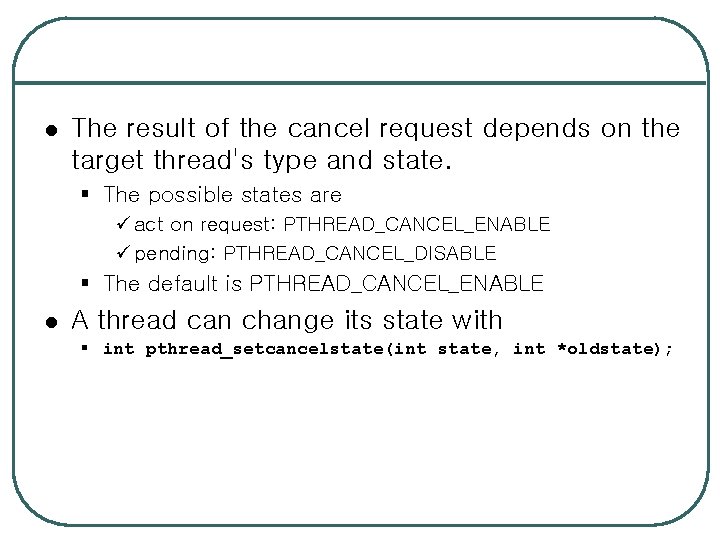
l The result of the cancel request depends on the target thread's type and state. § The possible states are ü act on request: PTHREAD_CANCEL_ENABLE ü pending: PTHREAD_CANCEL_DISABLE § The default is PTHREAD_CANCEL_ENABLE l A thread can change its state with § int pthread_setcancelstate(int state, int *oldstate);
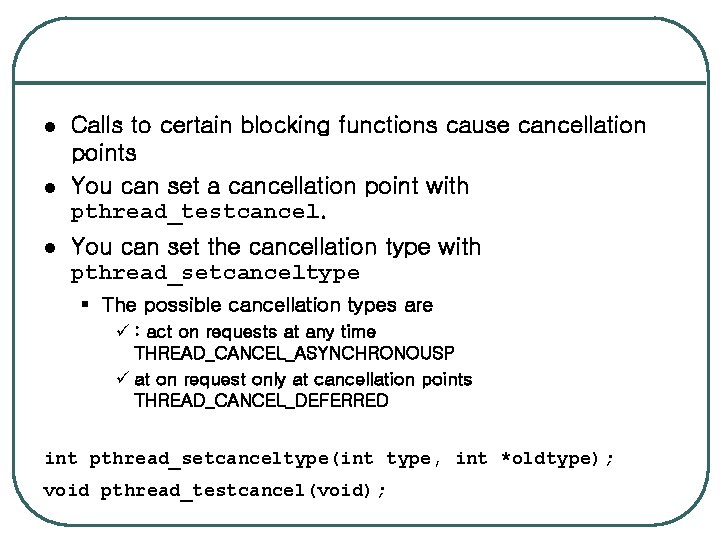
l l l Calls to certain blocking functions cause cancellation points You can set a cancellation point with pthread_testcancel. You can set the cancellation type with pthread_setcanceltype § The possible cancellation types are ü : act on requests at any time THREAD_CANCEL_ASYNCHRONOUSP ü at on request only at cancellation points THREAD_CANCEL_DEFERRED int pthread_setcanceltype(int type, int *oldtype); void pthread_testcancel(void);
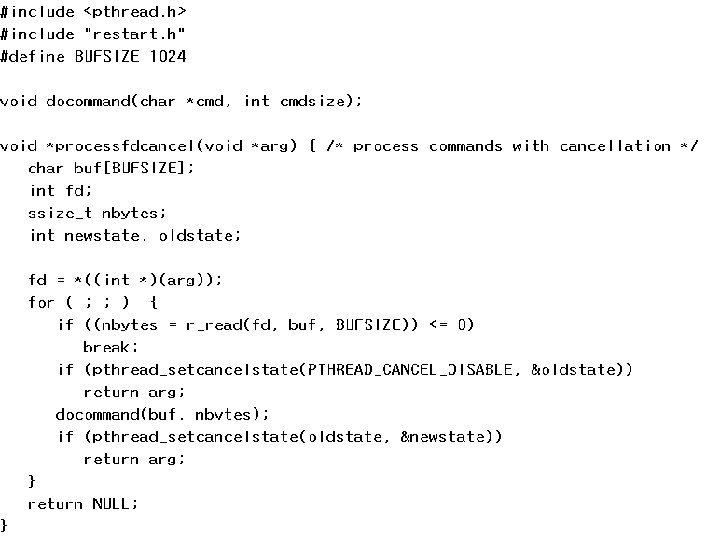
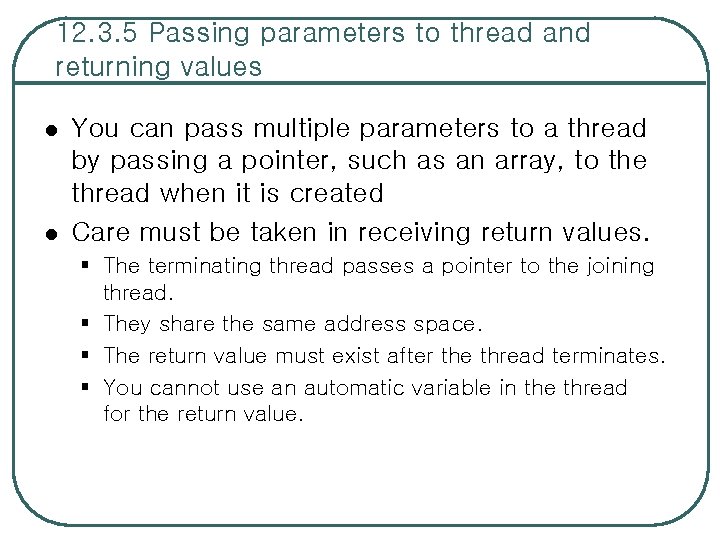
12. 3. 5 Passing parameters to thread and returning values l l You can pass multiple parameters to a thread by passing a pointer, such as an array, to the thread when it is created Care must be taken in receiving return values. § The terminating thread passes a pointer to the joining thread. § They share the same address space. § The return value must exist after the thread terminates. § You cannot use an automatic variable in the thread for the return value.
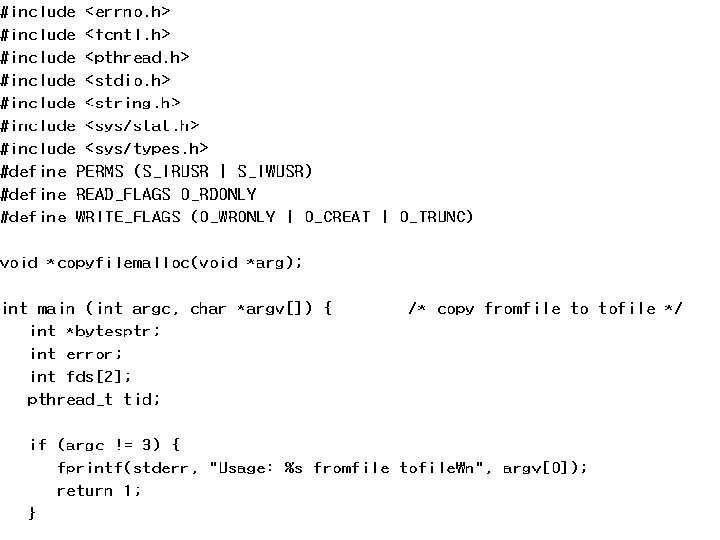
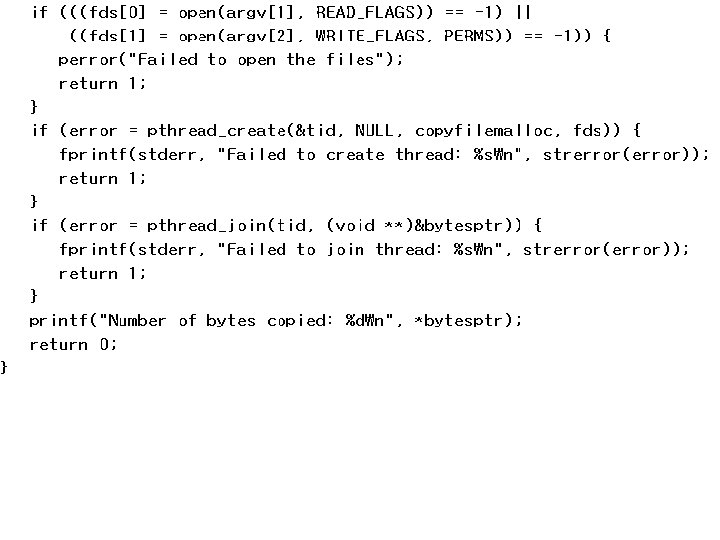
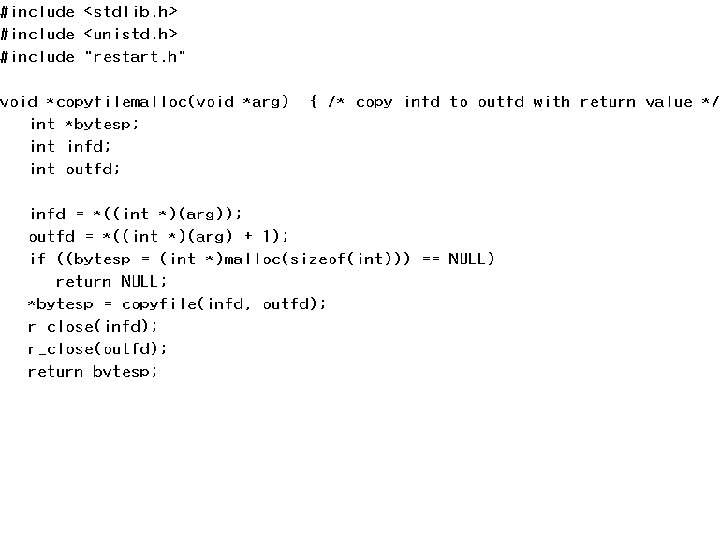
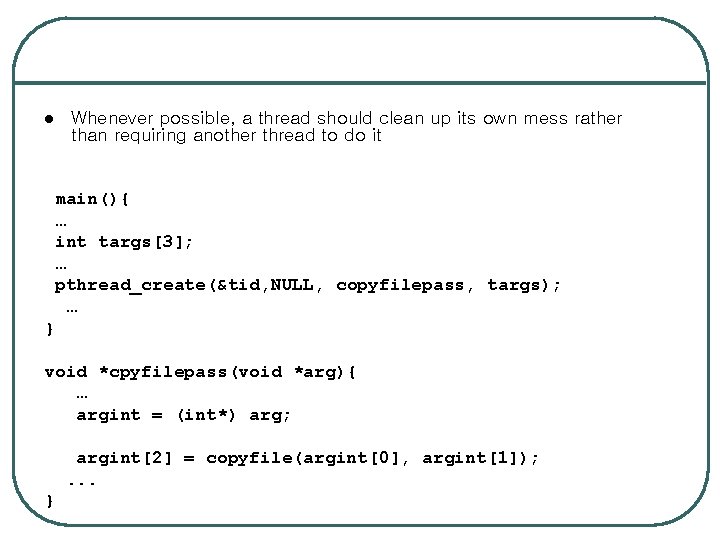
Whenever possible, a thread should clean up its own mess rather than requiring another thread to do it l main(){ … int targs[3]; … pthread_create(&tid, NULL, copyfilepass, targs); … } void *cpyfilepass(void *arg){ … argint = (int*) arg; argint[2] = copyfile(argint[0], argint[1]); . . . }
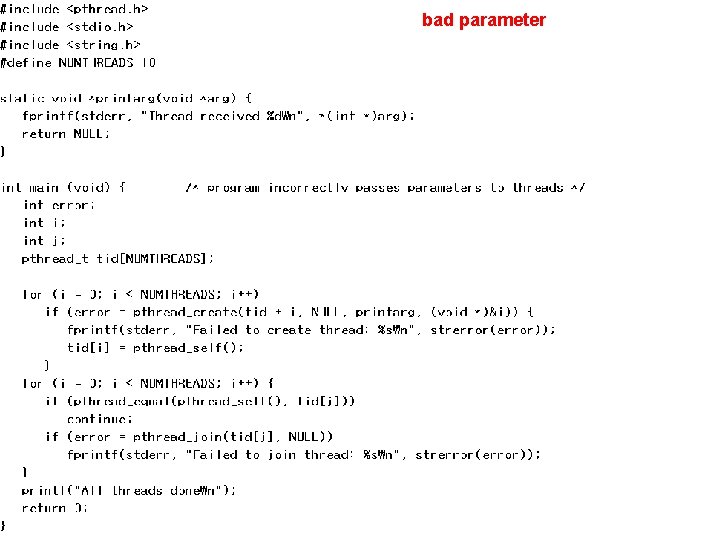
bad parameter
![void whichexitvoid arg int n int np 11 int np 2 char s void *whichexit(void *arg) { int n; int np 1[1]; int *np 2; char s](https://slidetodoc.com/presentation_image_h2/8f5d3095748c4a2e9a35450258782bfe/image-35.jpg)
void *whichexit(void *arg) { int n; int np 1[1]; int *np 2; char s 1[10]; char s 2[] = "I am done"; n = 3; np 1[0] = n; np 2 = (int *)malloc(sizeof(int)); *np 2 = n; strcpy(s 1, "Done"); return(NULL); } Which of the following would be safe replacement for NULL as the parameter to return? 1. n 2. &n 3. (int *)n 4. np 1 5. np 2 6. s 1 7. s 2 8. “this works” 9. strerror(EINTR)
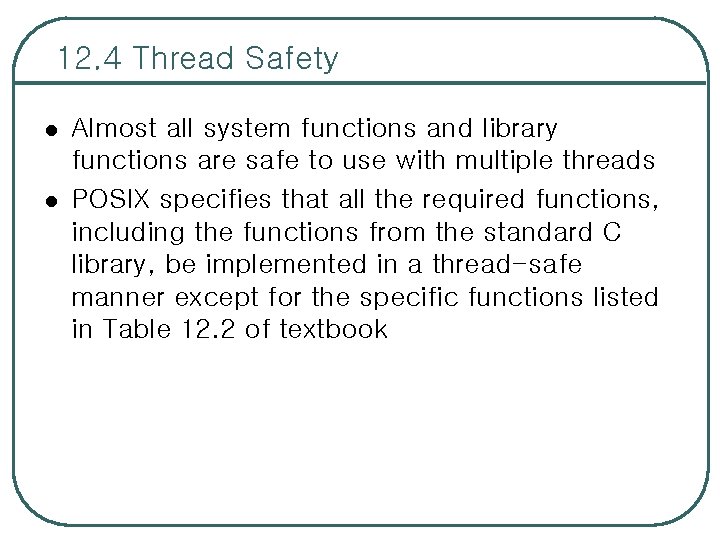
12. 4 Thread Safety l l Almost all system functions and library functions are safe to use with multiple threads POSIX specifies that all the required functions, including the functions from the standard C library, be implemented in a thread-safe manner except for the specific functions listed in Table 12. 2 of textbook
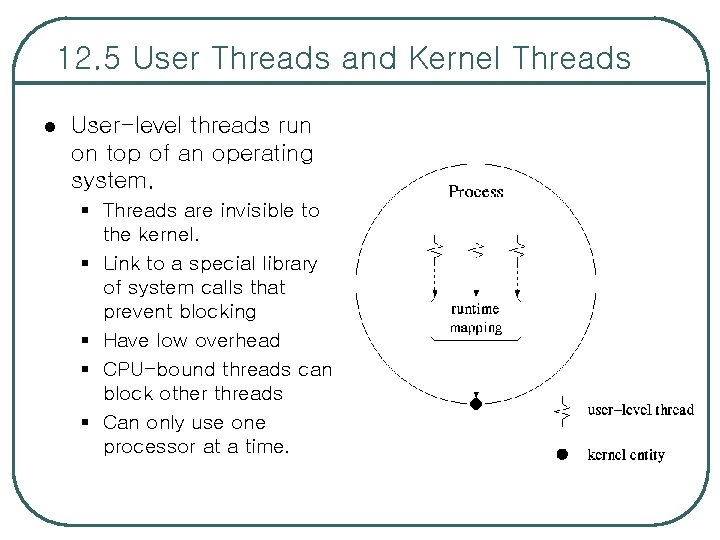
12. 5 User Threads and Kernel Threads l User-level threads run on top of an operating system. § Threads are invisible to the kernel. § Link to a special library of system calls that prevent blocking § Have low overhead § CPU-bound threads can block other threads § Can only use one processor at a time.
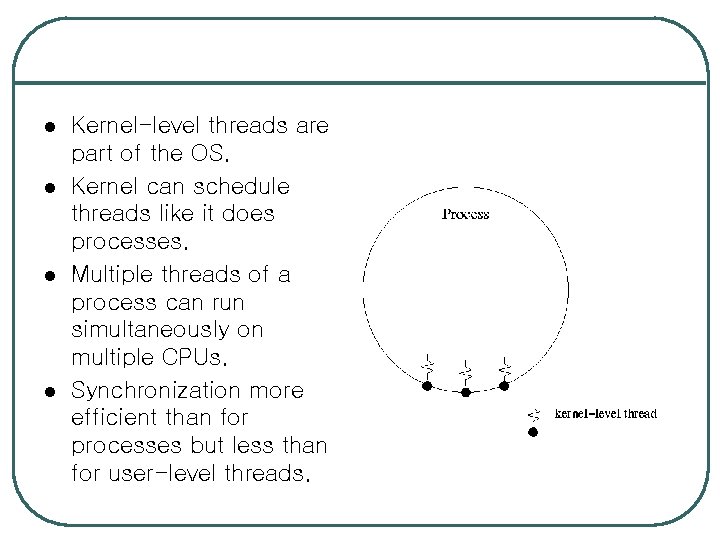
l l Kernel-level threads are part of the OS. Kernel can schedule threads like it does processes. Multiple threads of a process can run simultaneously on multiple CPUs. Synchronization more efficient than for processes but less than for user-level threads.
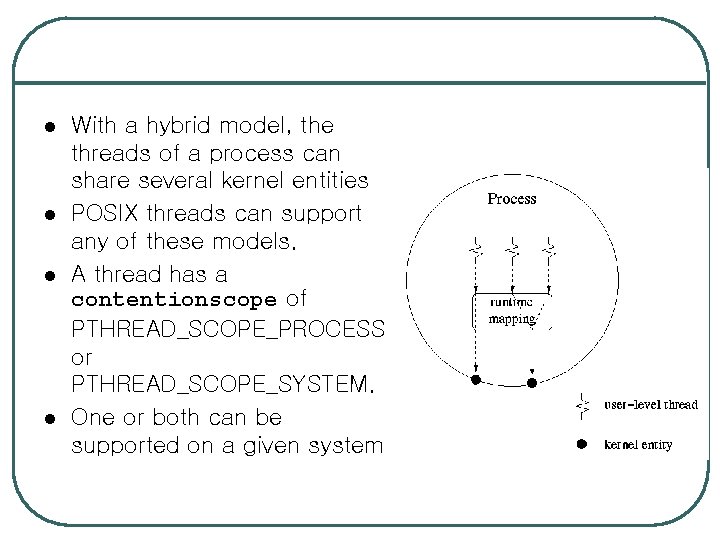
l l With a hybrid model, the threads of a process can share several kernel entities POSIX threads can support any of these models. A thread has a contentionscope of PTHREAD_SCOPE_PROCESS or PTHREAD_SCOPE_SYSTEM. One or both can be supported on a given system
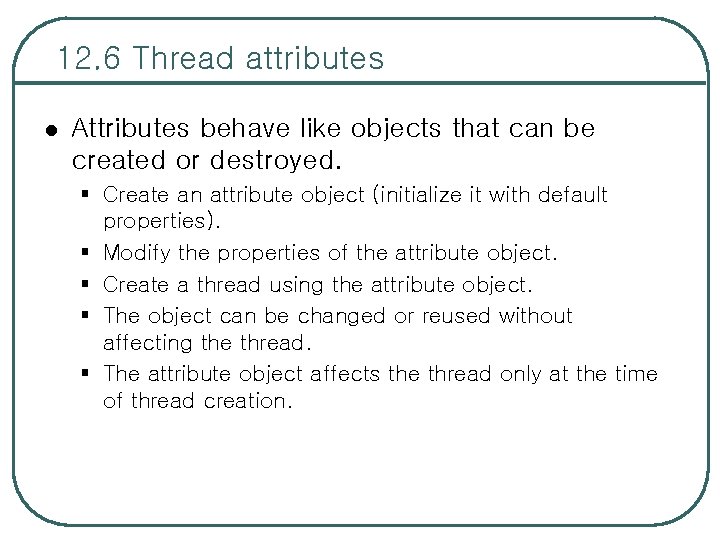
12. 6 Thread attributes l Attributes behave like objects that can be created or destroyed. § Create an attribute object (initialize it with default properties). § Modify the properties of the attribute object. § Create a thread using the attribute object. § The object can be changed or reused without affecting the thread. § The attribute object affects the thread only at the time of thread creation.
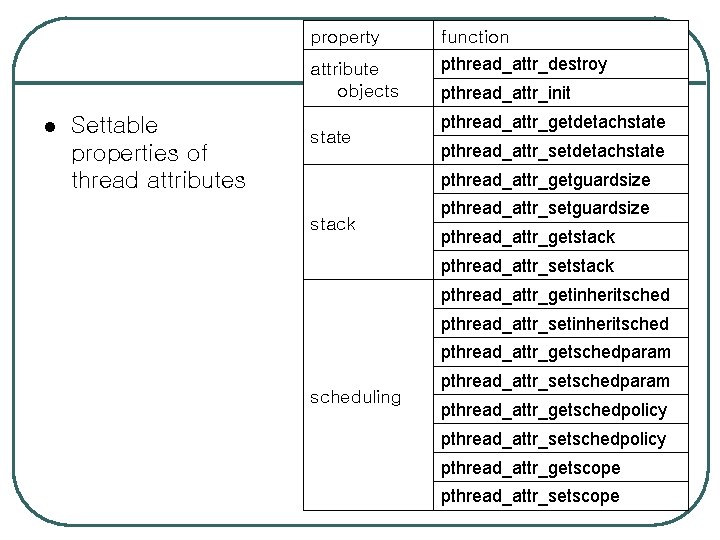
l Settable properties of thread attributes property function attribute objects pthread_attr_destroy state pthread_attr_init pthread_attr_getdetachstate pthread_attr_setdetachstate pthread_attr_getguardsize stack pthread_attr_setguardsize pthread_attr_getstack pthread_attr_setstack pthread_attr_getinheritsched pthread_attr_setinheritsched pthread_attr_getschedparam scheduling pthread_attr_setschedparam pthread_attr_getschedpolicy pthread_attr_setschedpolicy pthread_attr_getscope pthread_attr_setscope
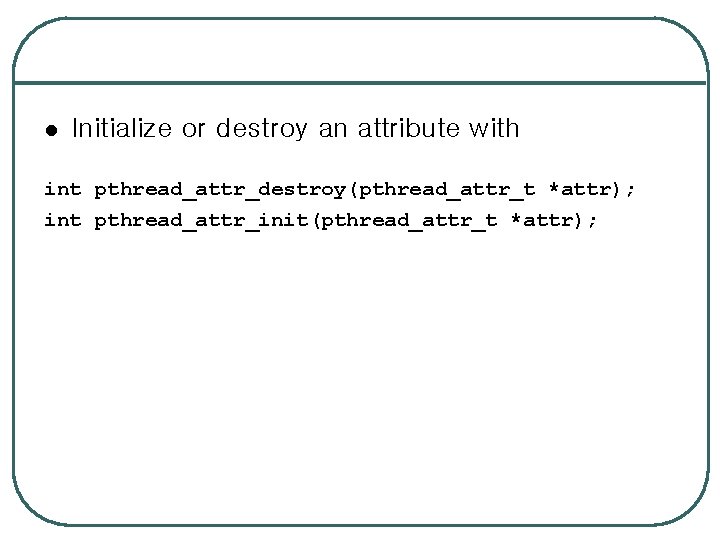
l Initialize or destroy an attribute with int pthread_attr_destroy(pthread_attr_t *attr); int pthread_attr_init(pthread_attr_t *attr);
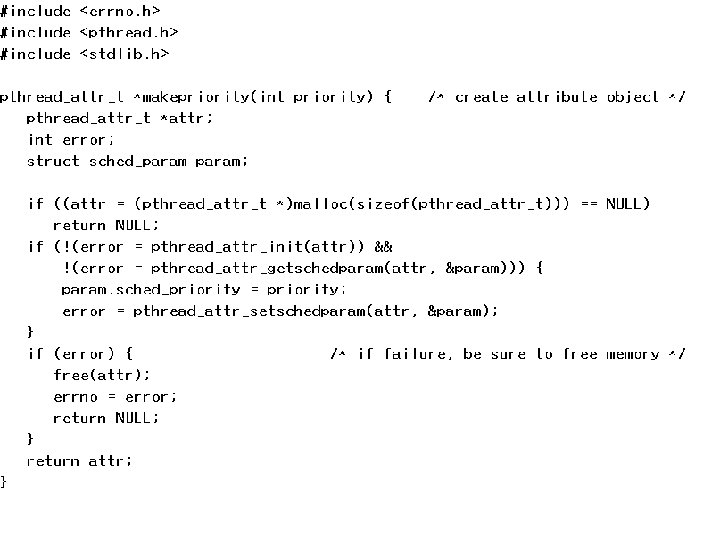
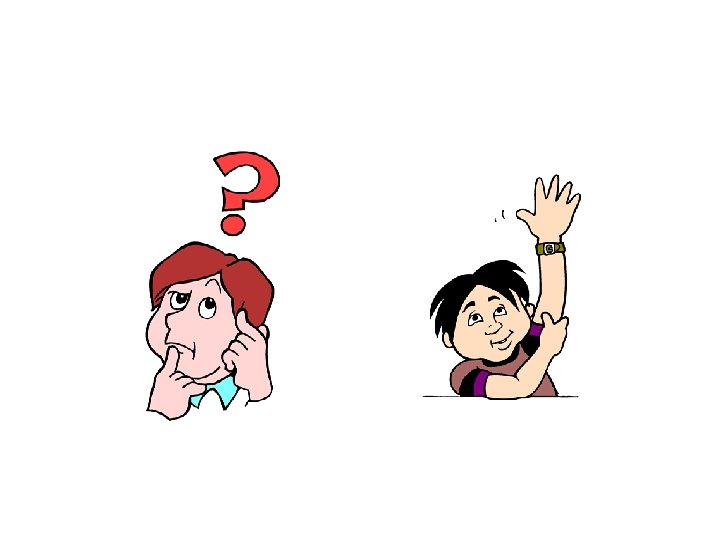
Pthreads
Lightweight thread
Taek lisans vize yenileme
Dr sinem tüzel
Ansi c standards
Posix 4
Posix api
Shared memory in unix
Posix adalah
Mecanismos de comunicación y sincronización de procesos
Hilos posix
Posix filesystem
Zabiegi sanitarne i specjalne
Abc pamec
Nf 01-012
Auc rule 012
Nit dicla 031
Ioit-012
Nom-012-scfi-1994
C11 threads
Java shared memory
Screw thread profile adopted by bis
Process and threads
Flexible flat material made by interlacing yarns
Tipos de escalonamento
Process and threads
Os threads
The vinaya pitaka is a sacred text of
Needle like threads of spongy bone
Takes out sewing mistakes
Golden thread principle
Pintos manual
Threads in operating system
Threads java
Alternanthera red threads
Forum.unity.com/threads/game-over.54735
Threads fiji
Threads consume cpu in best possible manner
Sockets and threads
Forum.unity.com/threads/game-over.54735
Threads vs processes
Sequence diagram threads
Threads cannot be implemented as a library
Threads.h
The material used for cutting tools can be