c Templates What is a template Templates are
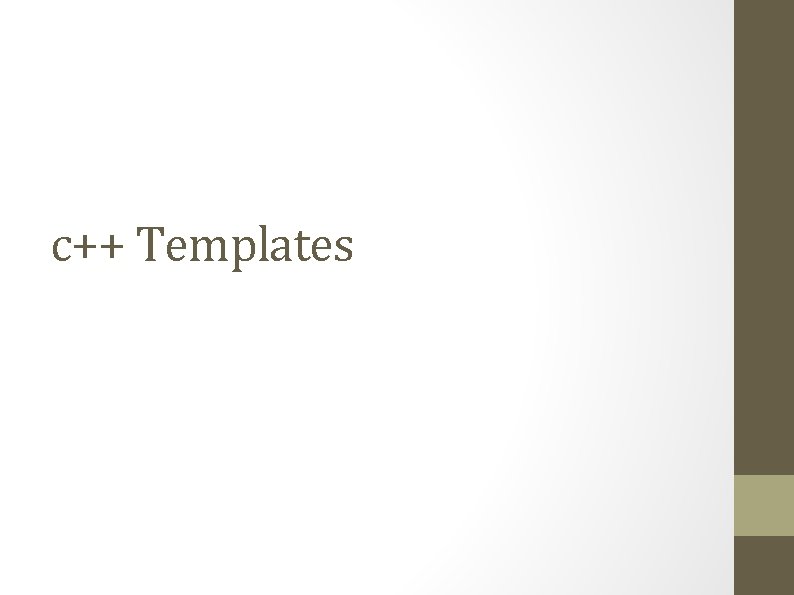
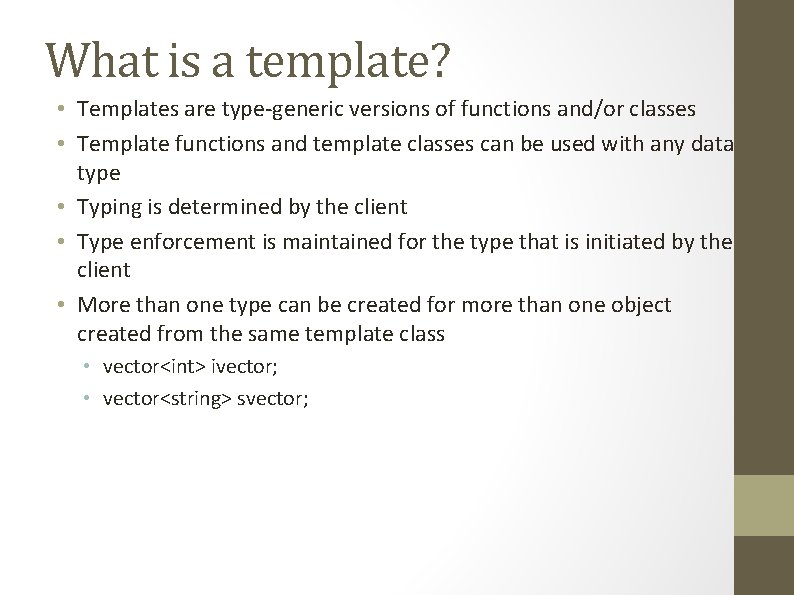
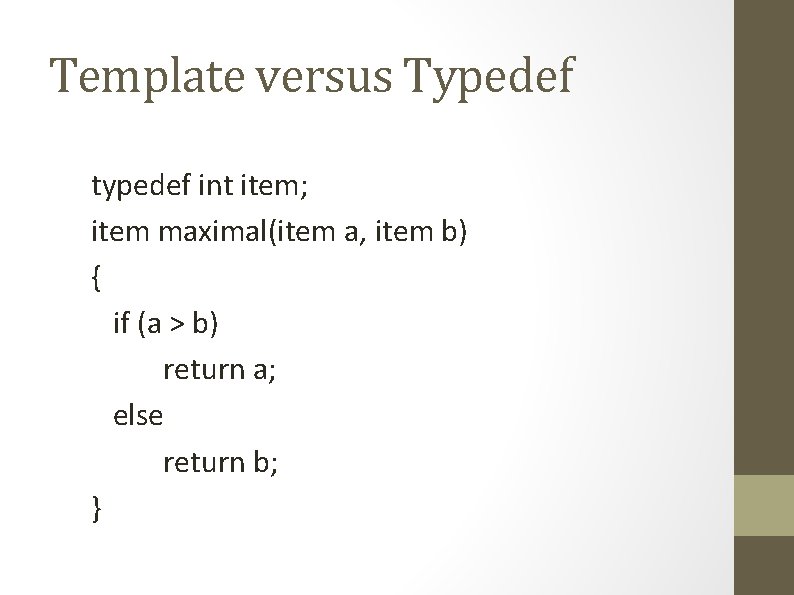
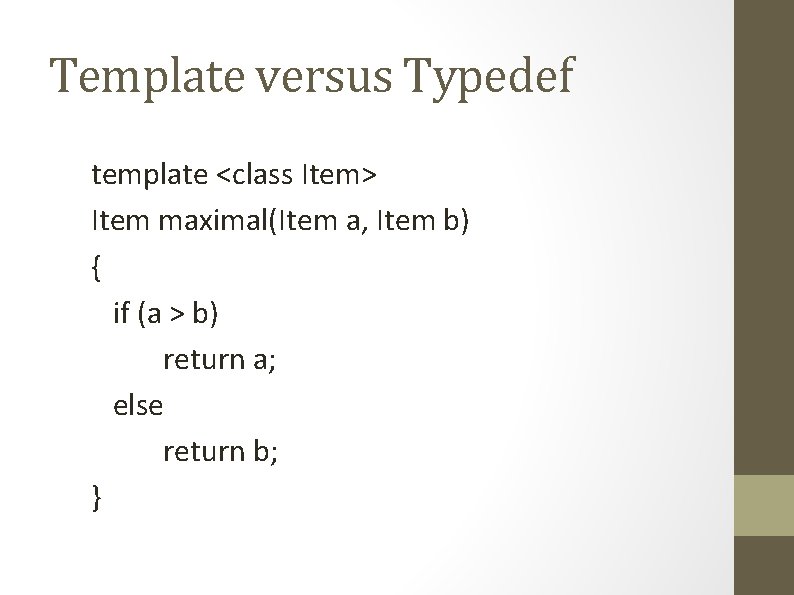
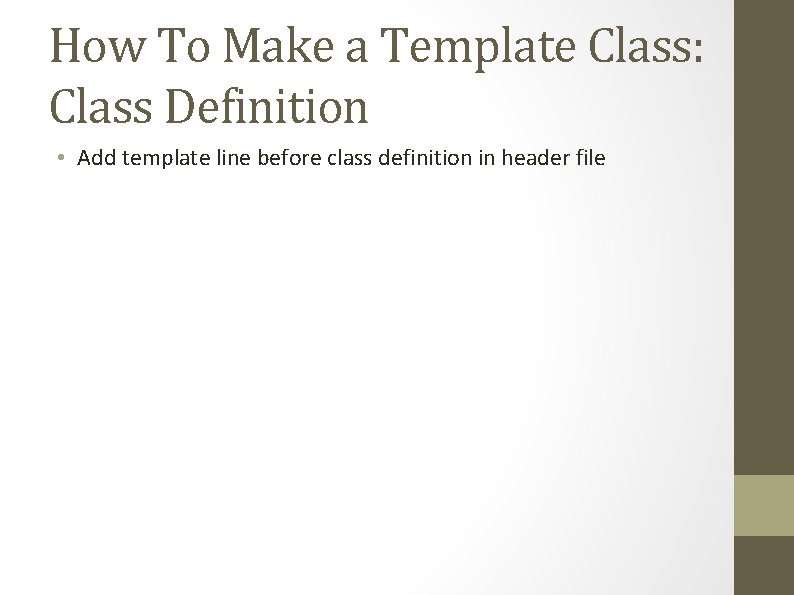
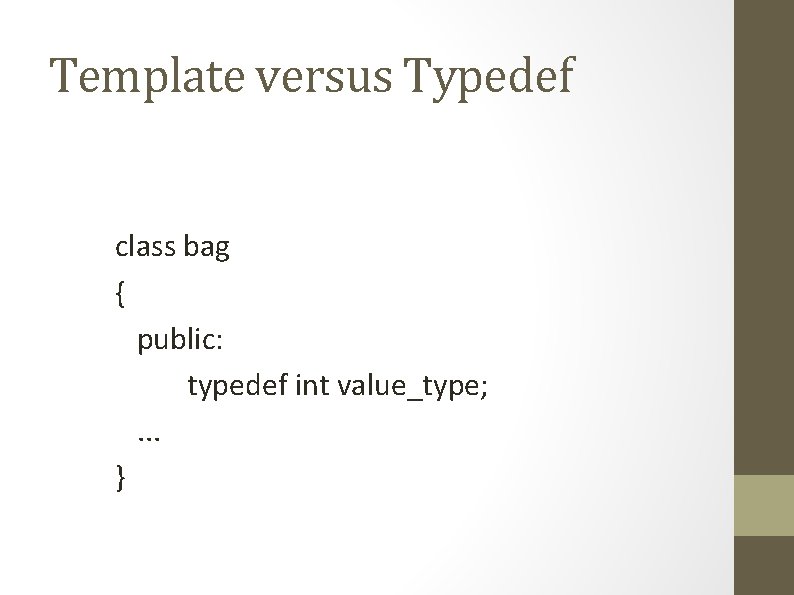
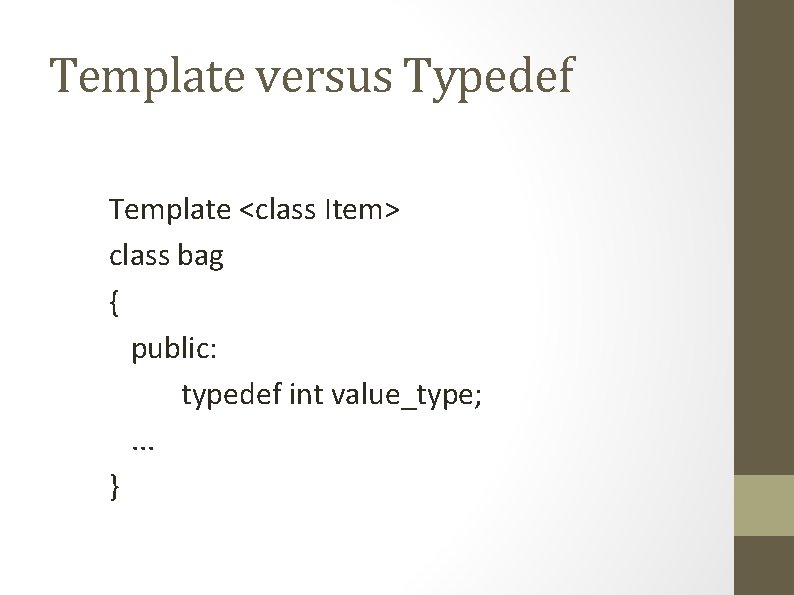
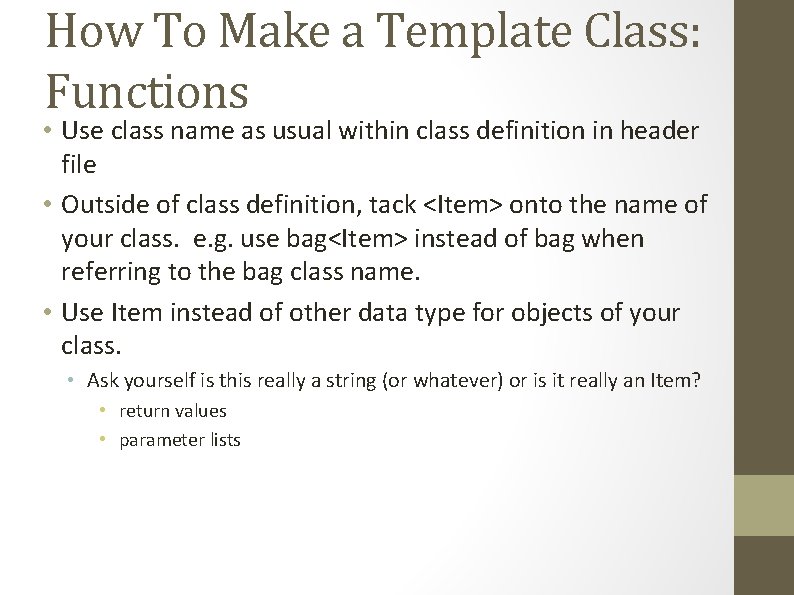
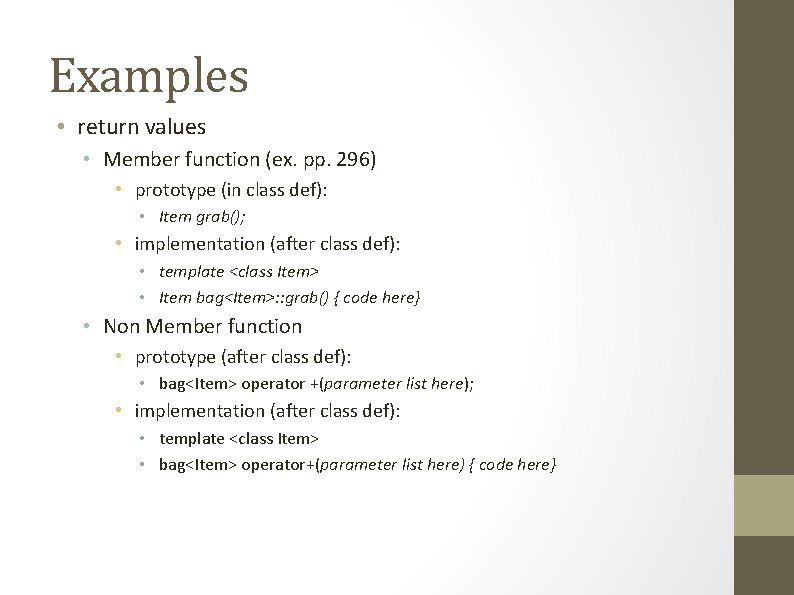
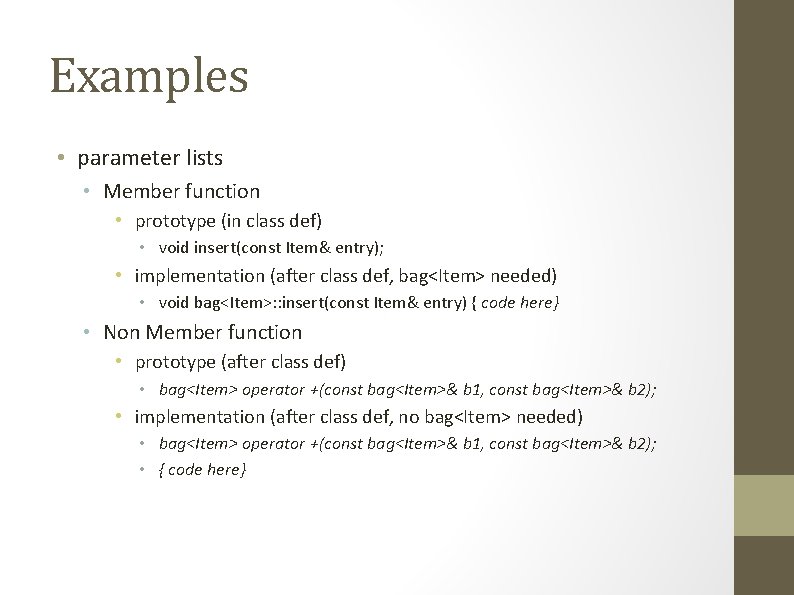
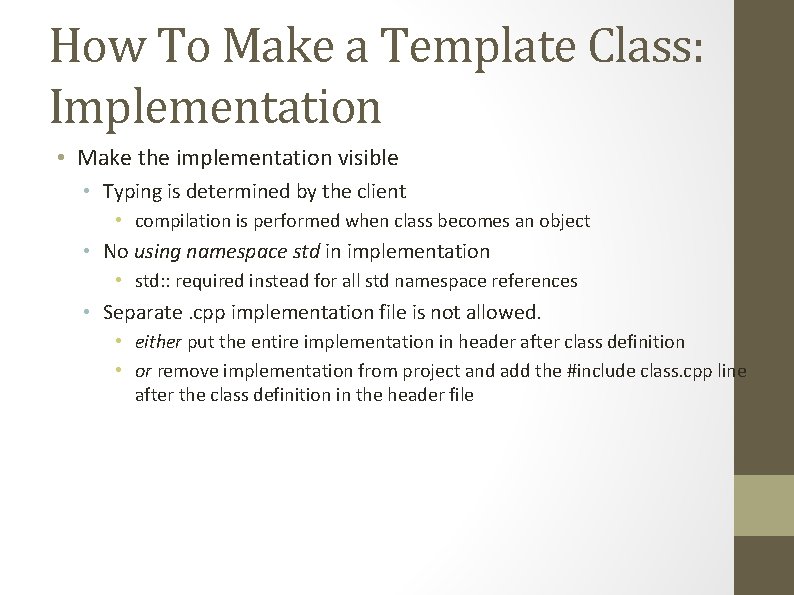
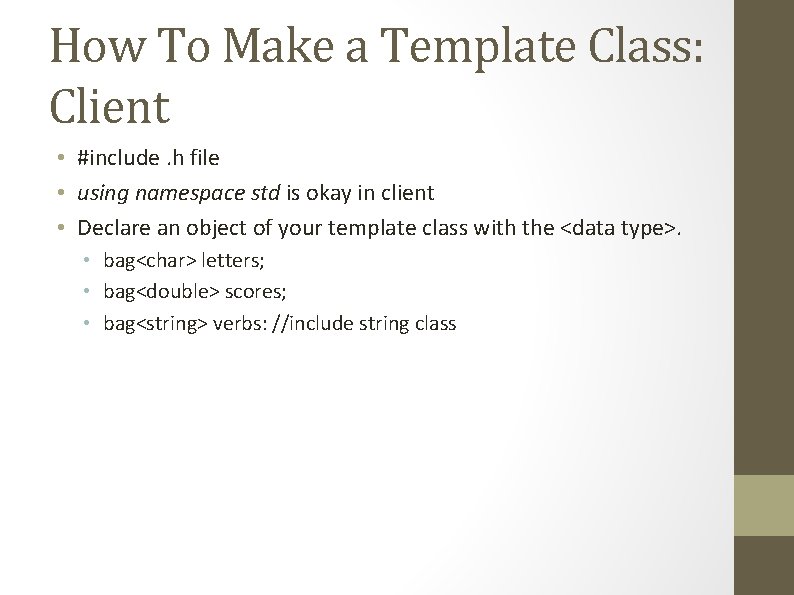
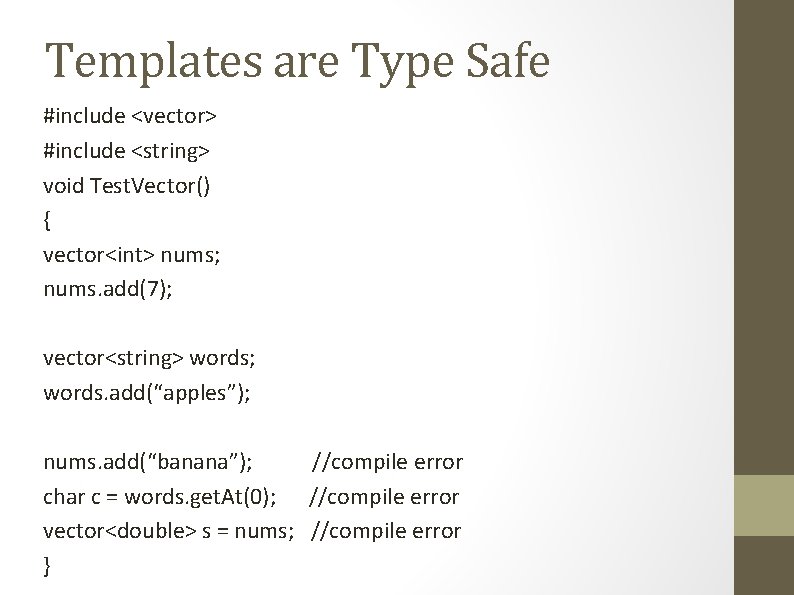
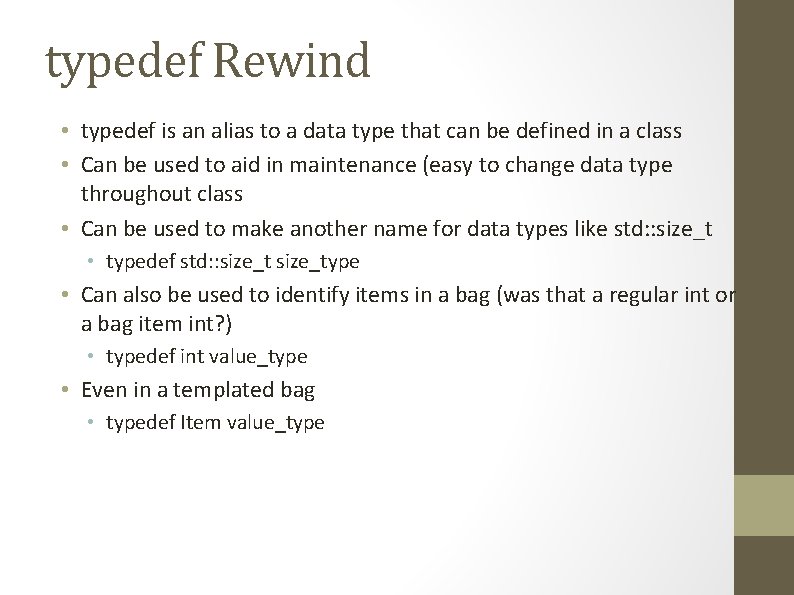
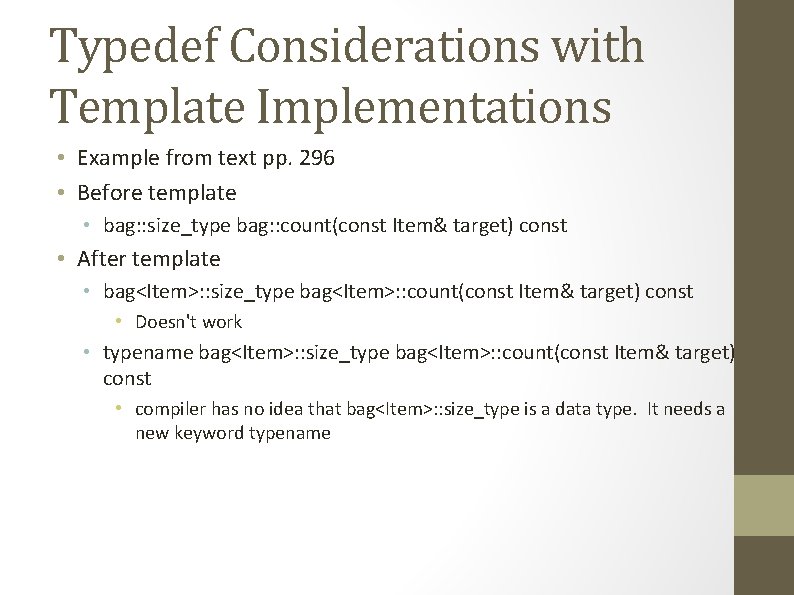
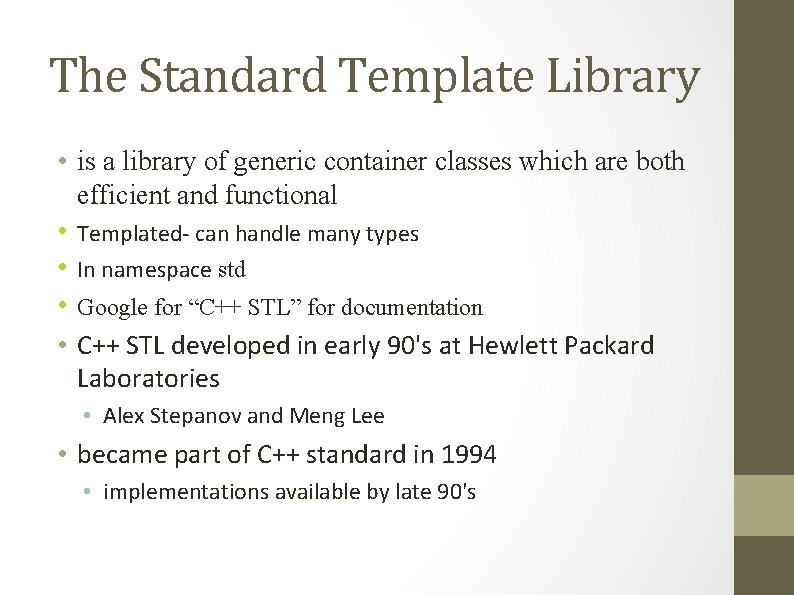
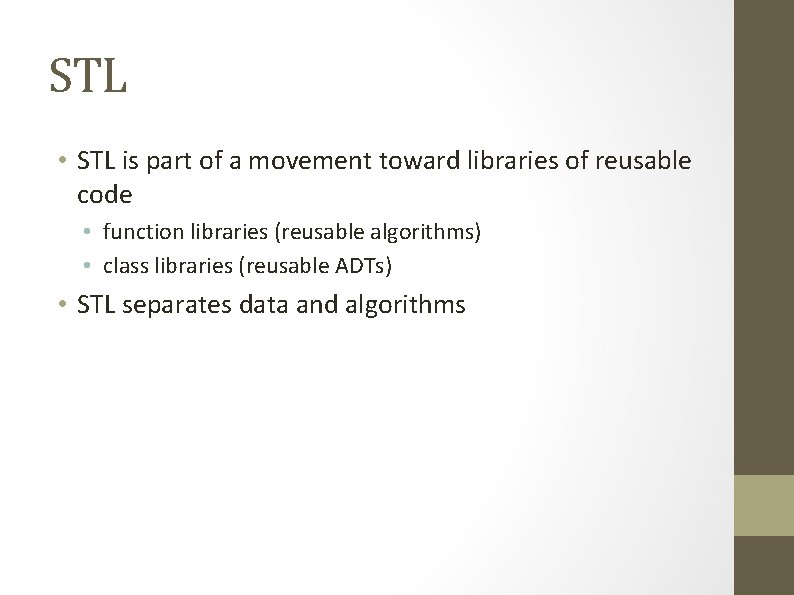
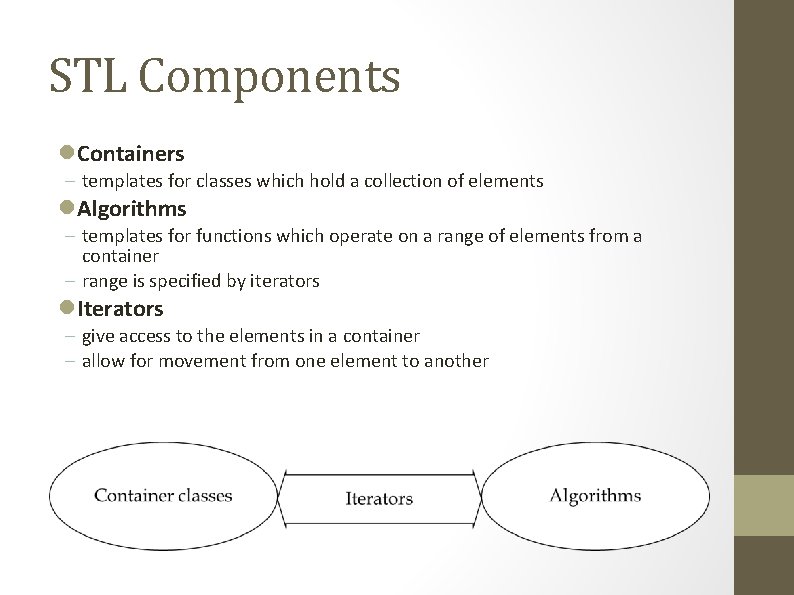
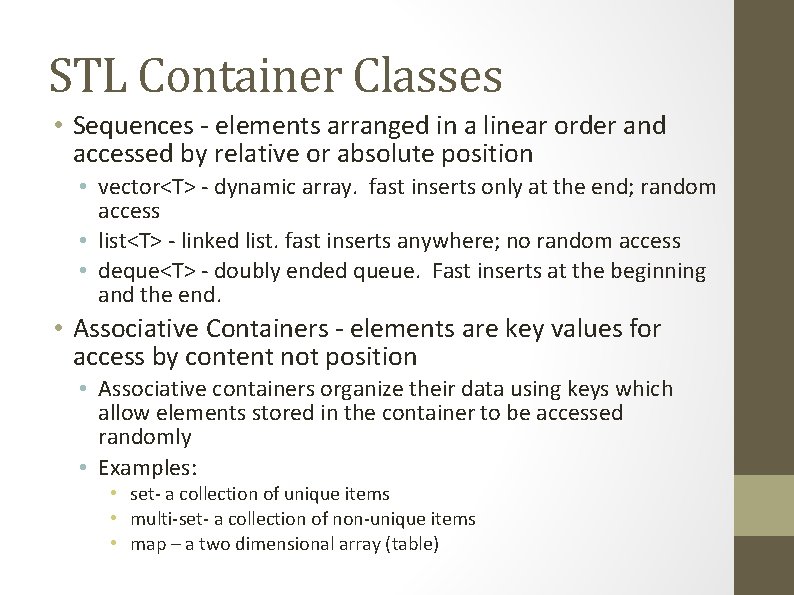
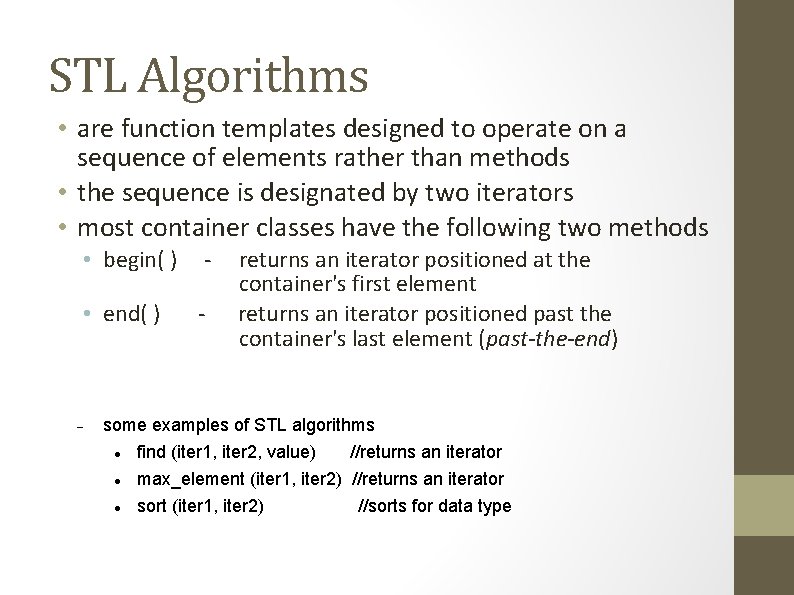
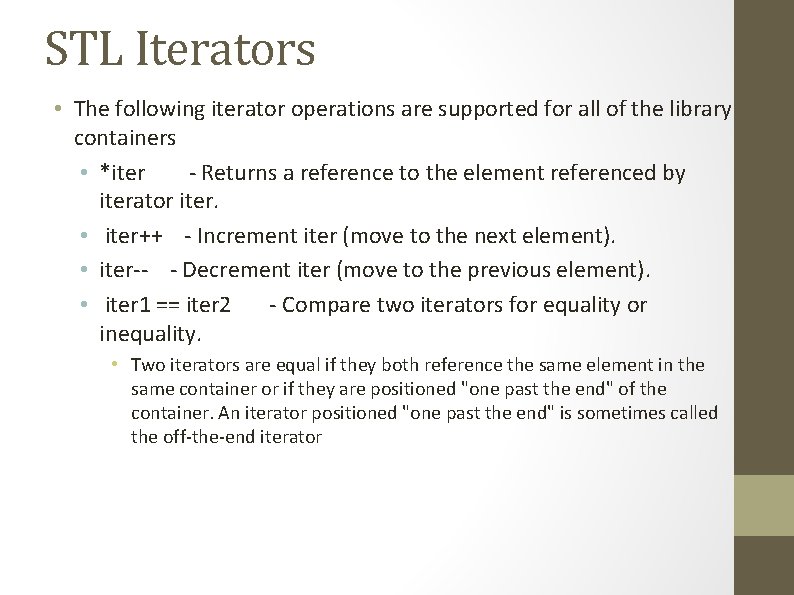
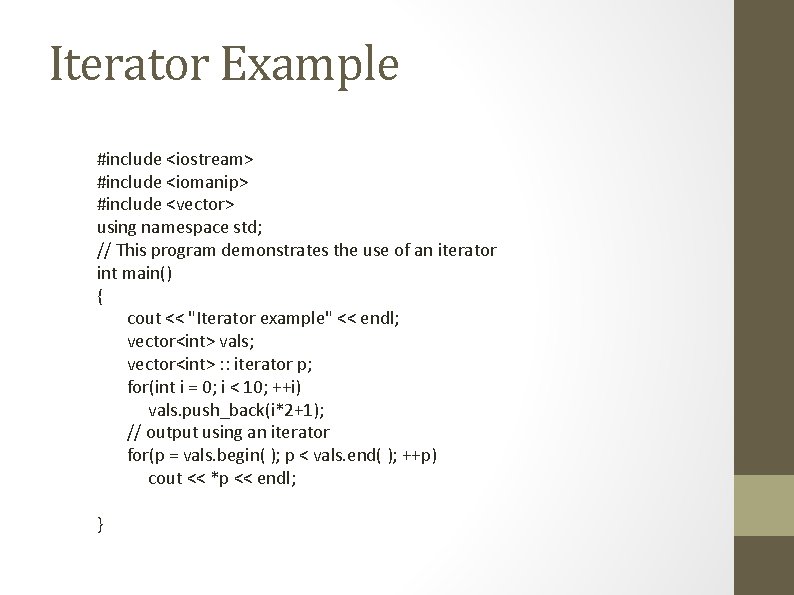
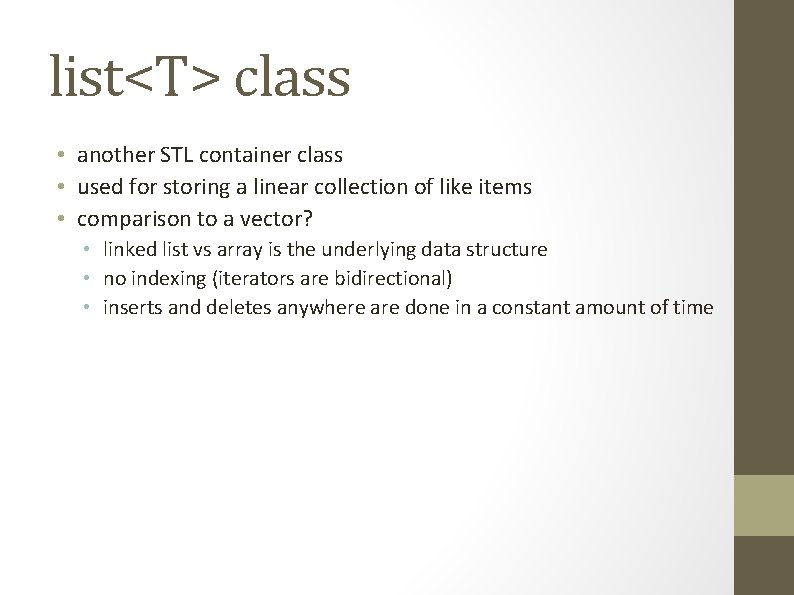
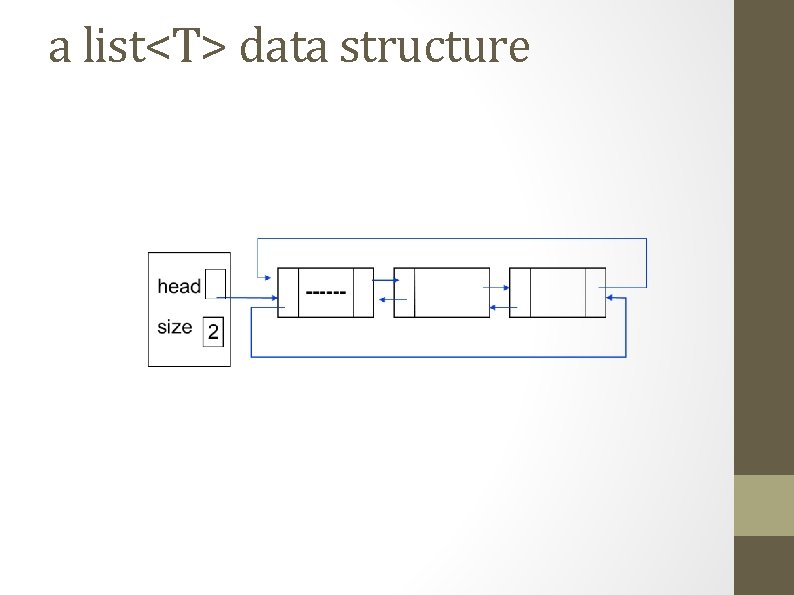
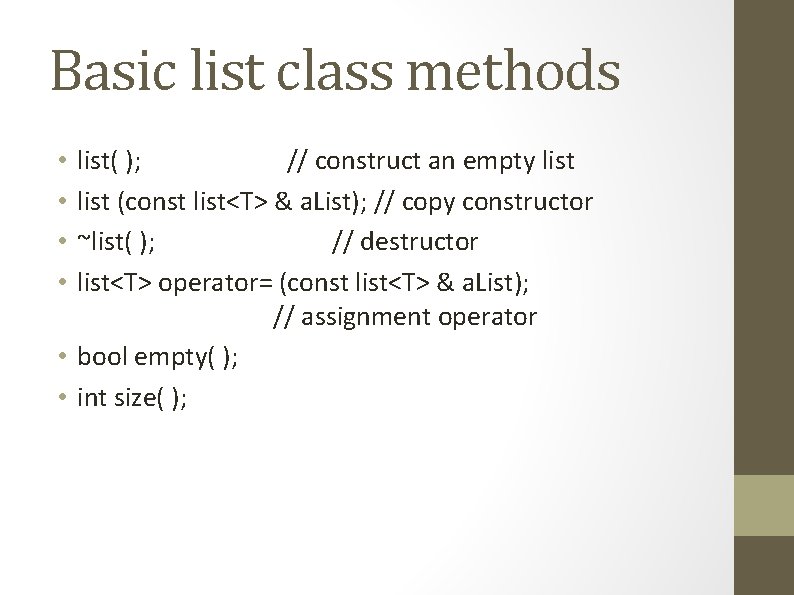
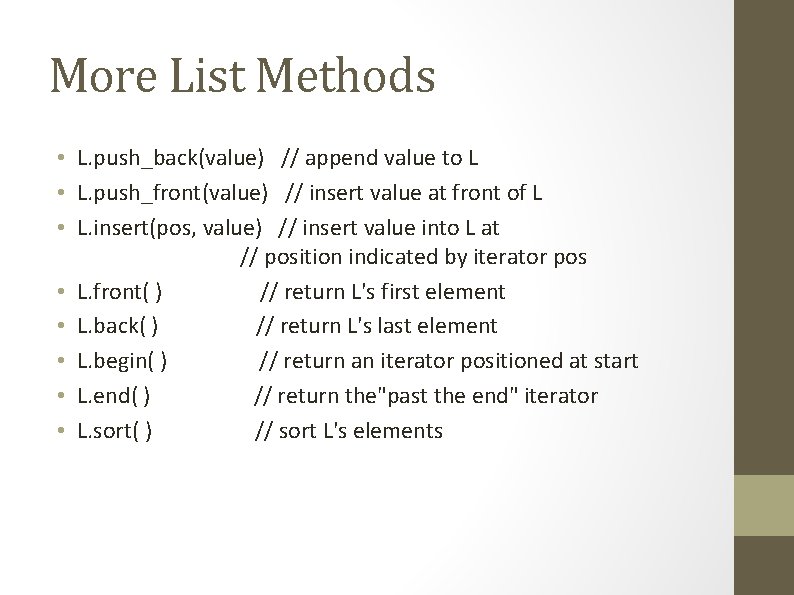
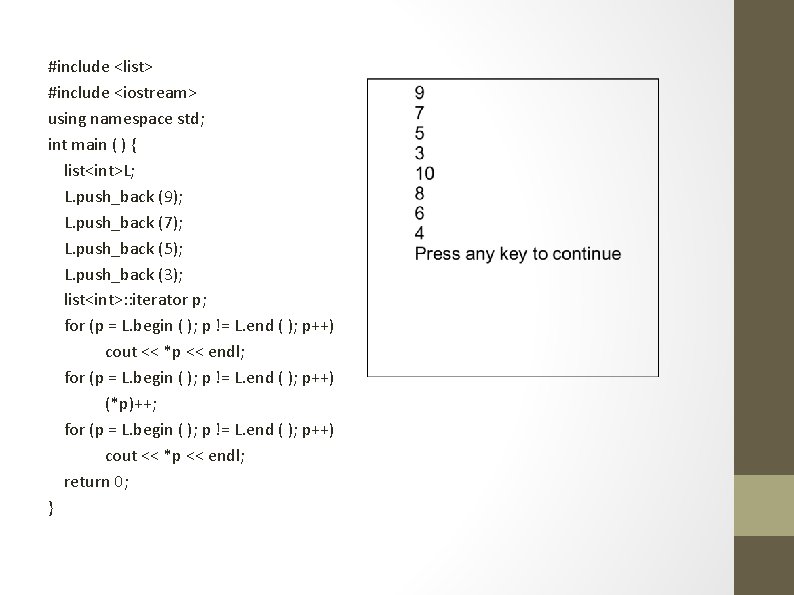
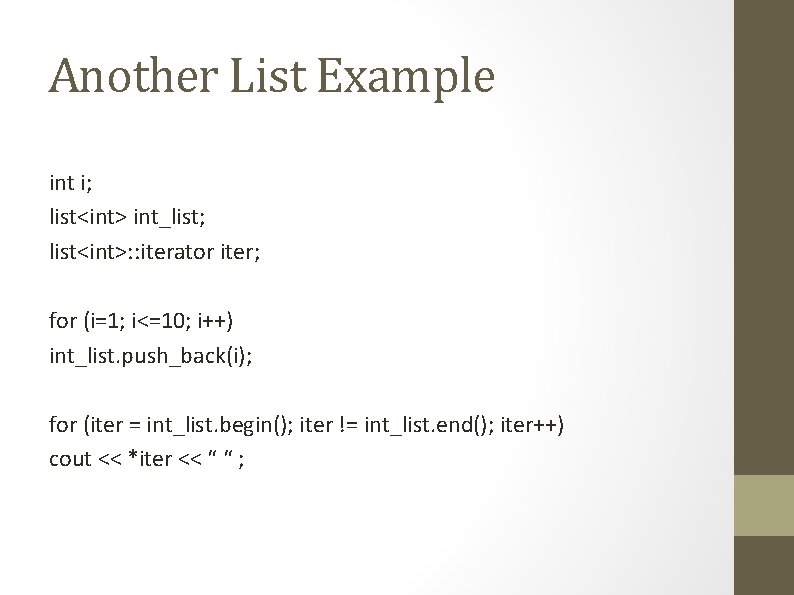
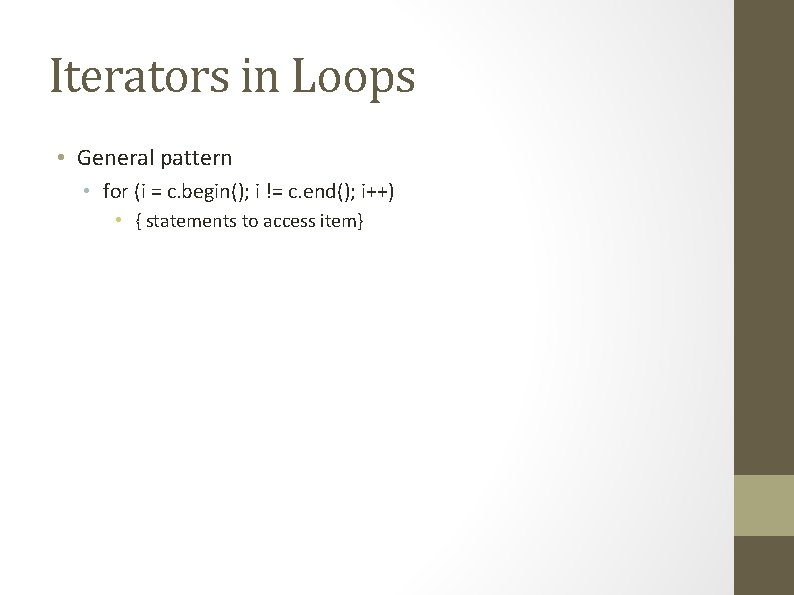
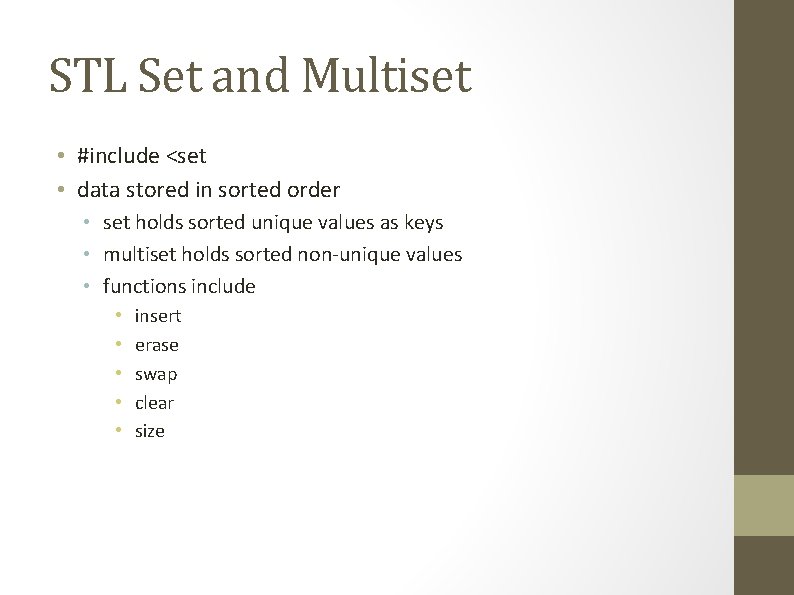
- Slides: 30
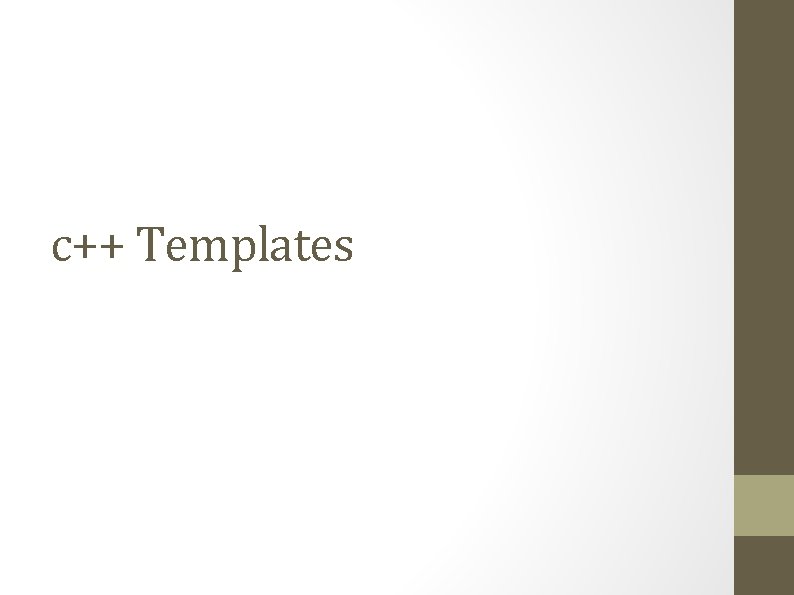
c++ Templates
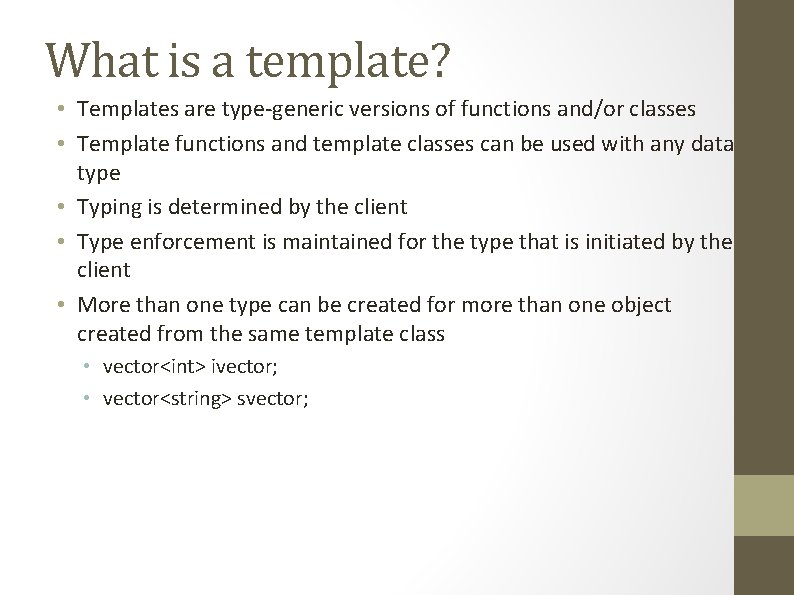
What is a template? • Templates are type-generic versions of functions and/or classes • Template functions and template classes can be used with any data type • Typing is determined by the client • Type enforcement is maintained for the type that is initiated by the client • More than one type can be created for more than one object created from the same template class • vector<int> ivector; • vector<string> svector;
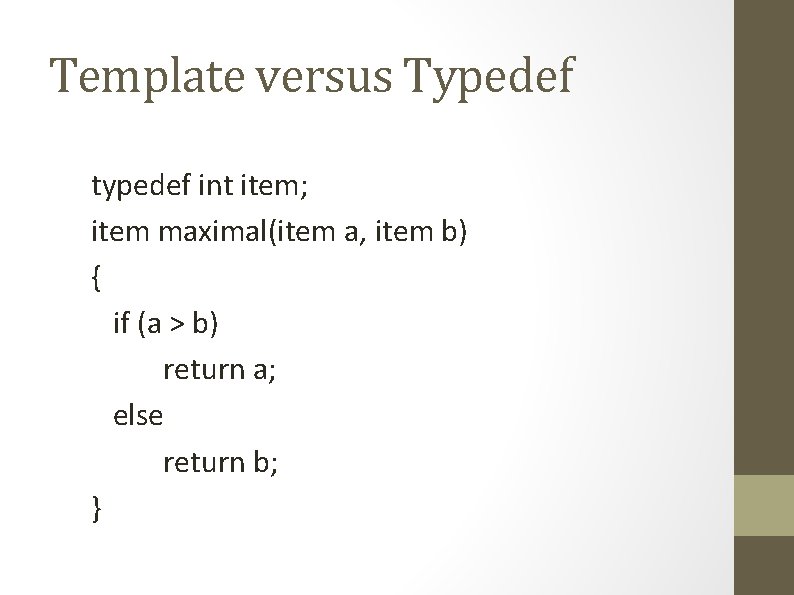
Template versus Typedef typedef int item; item maximal(item a, item b) { if (a > b) return a; else return b; }
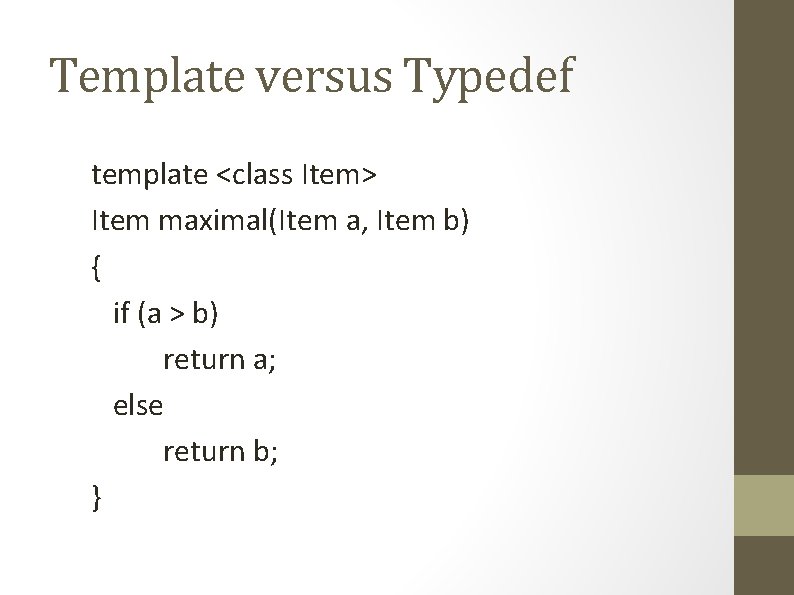
Template versus Typedef template <class Item> Item maximal(Item a, Item b) { if (a > b) return a; else return b; }
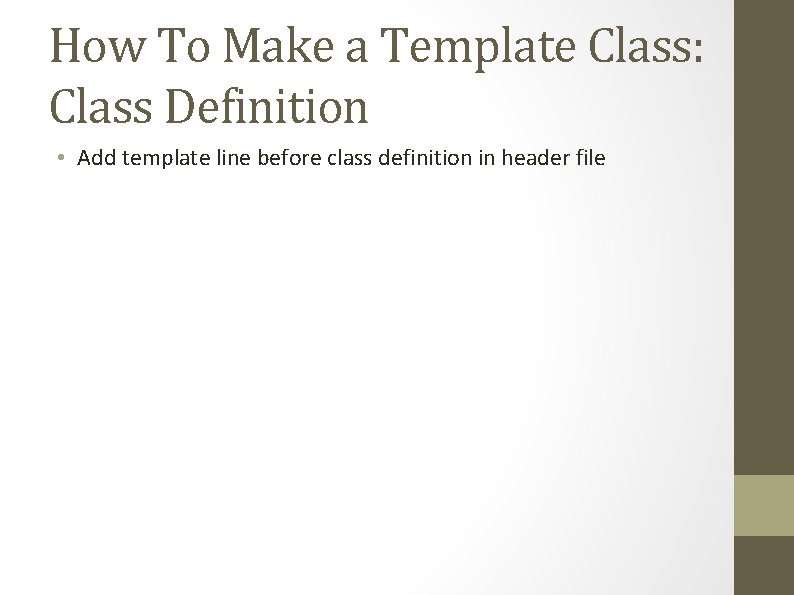
How To Make a Template Class: Class Definition • Add template line before class definition in header file
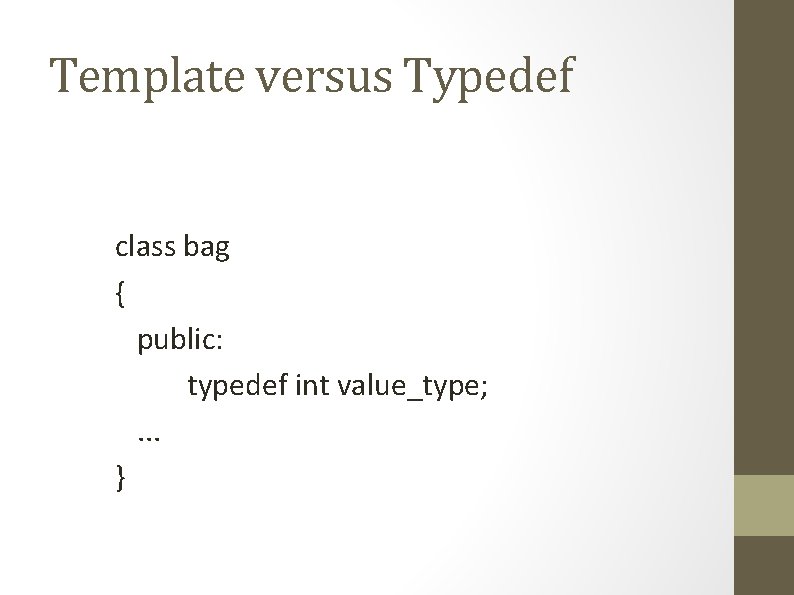
Template versus Typedef class bag { public: typedef int value_type; . . . }
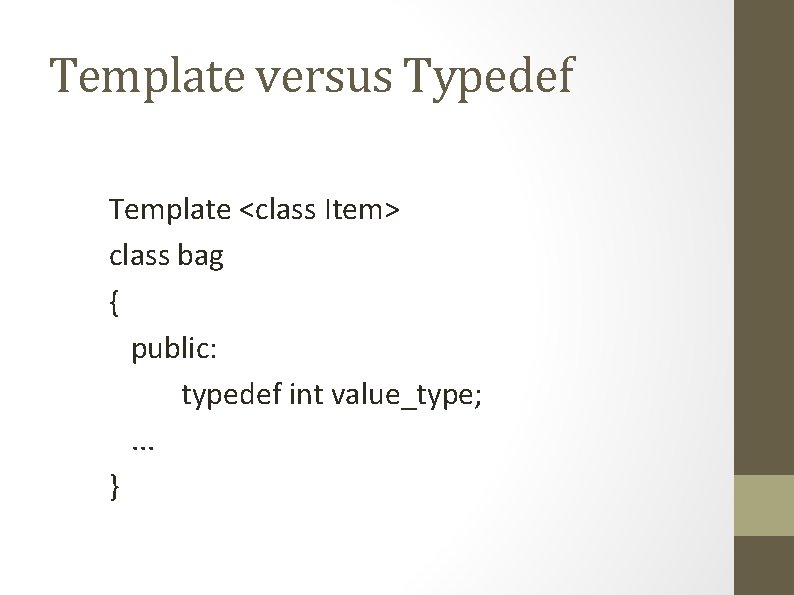
Template versus Typedef Template <class Item> class bag { public: typedef int value_type; . . . }
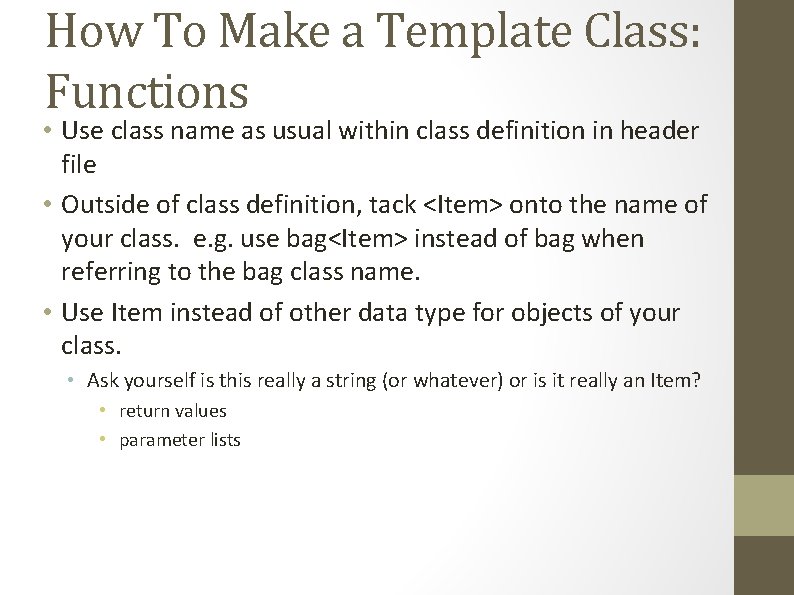
How To Make a Template Class: Functions • Use class name as usual within class definition in header file • Outside of class definition, tack <Item> onto the name of your class. e. g. use bag<Item> instead of bag when referring to the bag class name. • Use Item instead of other data type for objects of your class. • Ask yourself is this really a string (or whatever) or is it really an Item? • return values • parameter lists
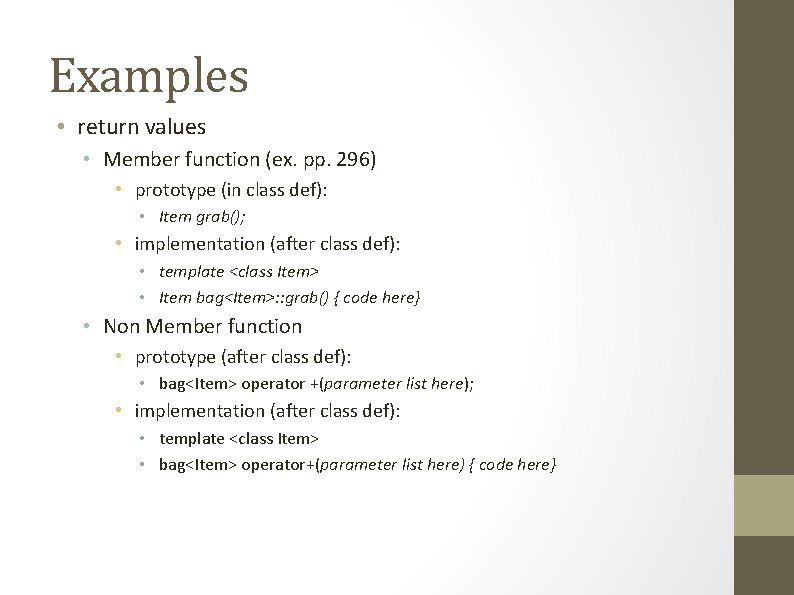
Examples • return values • Member function (ex. pp. 296) • prototype (in class def): • Item grab(); • implementation (after class def): • template <class Item> • Item bag<Item>: : grab() { code here} • Non Member function • prototype (after class def): • bag<Item> operator +(parameter list here); • implementation (after class def): • template <class Item> • bag<Item> operator+(parameter list here) { code here}
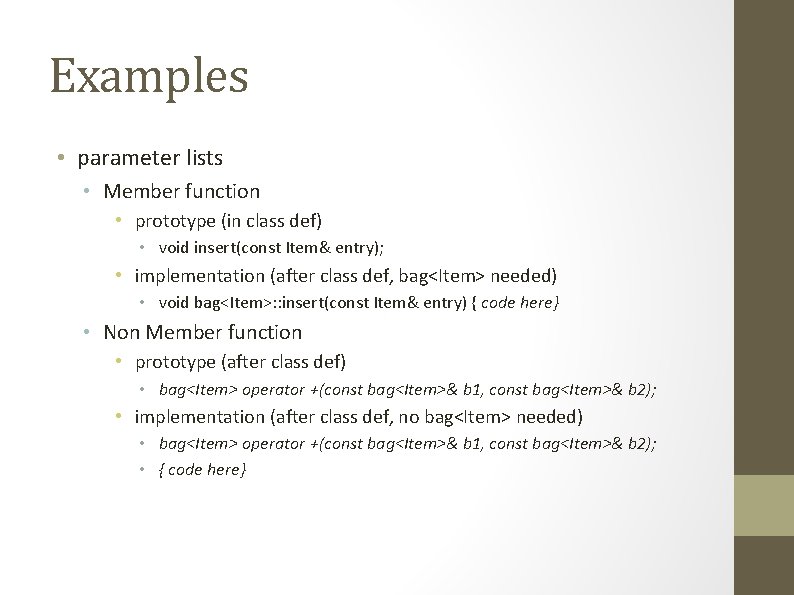
Examples • parameter lists • Member function • prototype (in class def) • void insert(const Item& entry); • implementation (after class def, bag<Item> needed) • void bag<Item>: : insert(const Item& entry) { code here} • Non Member function • prototype (after class def) • bag<Item> operator +(const bag<Item>& b 1, const bag<Item>& b 2); • implementation (after class def, no bag<Item> needed) • bag<Item> operator +(const bag<Item>& b 1, const bag<Item>& b 2); • { code here}
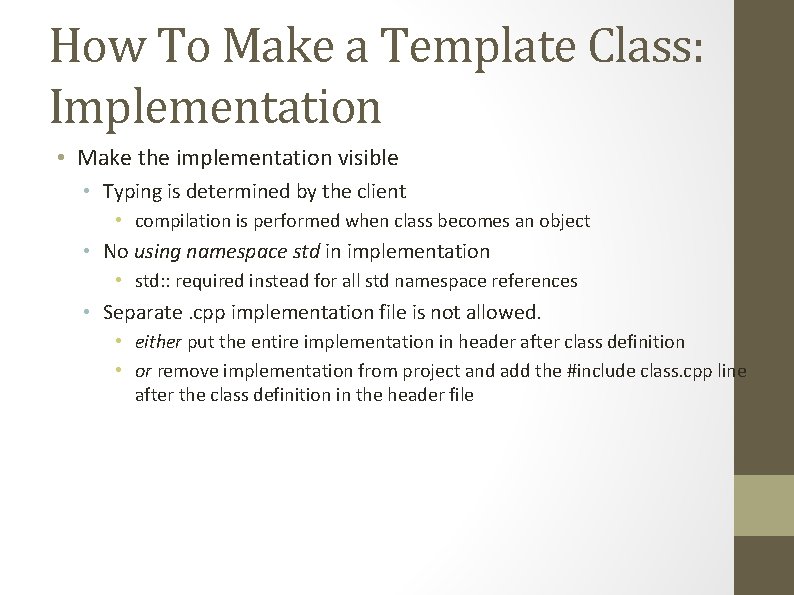
How To Make a Template Class: Implementation • Make the implementation visible • Typing is determined by the client • compilation is performed when class becomes an object • No using namespace std in implementation • std: : required instead for all std namespace references • Separate. cpp implementation file is not allowed. • either put the entire implementation in header after class definition • or remove implementation from project and add the #include class. cpp line after the class definition in the header file
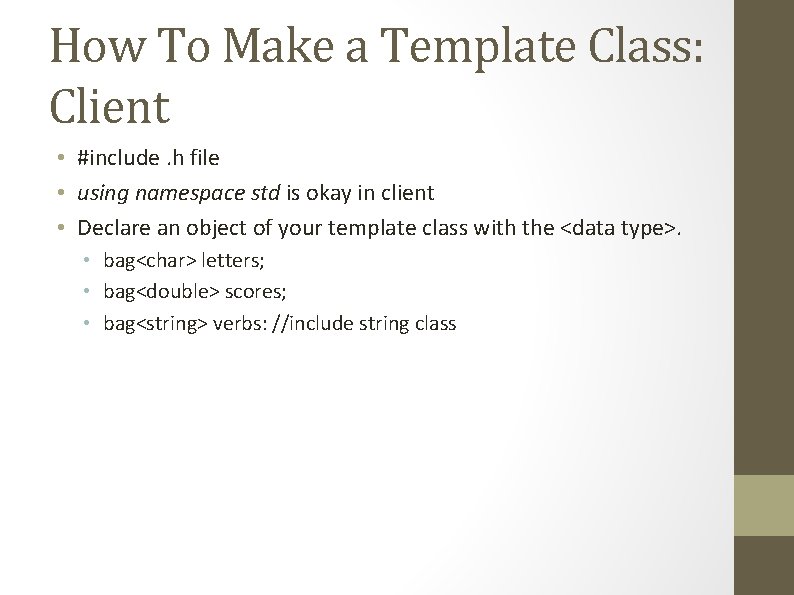
How To Make a Template Class: Client • #include. h file • using namespace std is okay in client • Declare an object of your template class with the <data type>. • bag<char> letters; • bag<double> scores; • bag<string> verbs: //include string class
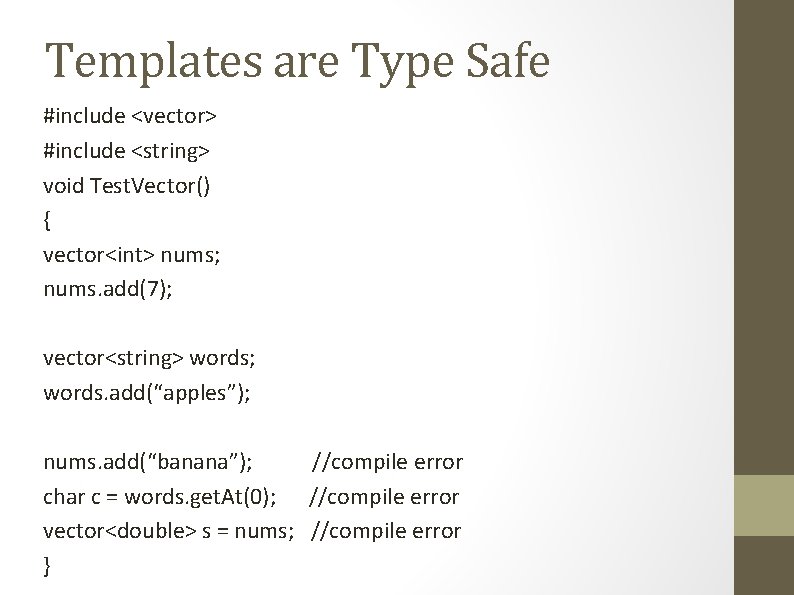
Templates are Type Safe #include <vector> #include <string> void Test. Vector() { vector<int> nums; nums. add(7); vector<string> words; words. add(“apples”); nums. add(“banana”); //compile error char c = words. get. At(0); //compile error vector<double> s = nums; //compile error }
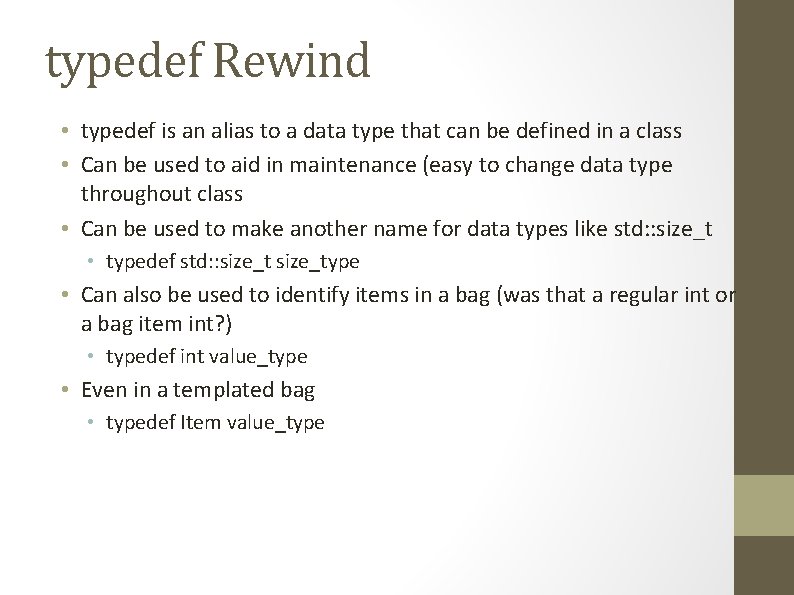
typedef Rewind • typedef is an alias to a data type that can be defined in a class • Can be used to aid in maintenance (easy to change data type throughout class • Can be used to make another name for data types like std: : size_t • typedef std: : size_type • Can also be used to identify items in a bag (was that a regular int or a bag item int? ) • typedef int value_type • Even in a templated bag • typedef Item value_type
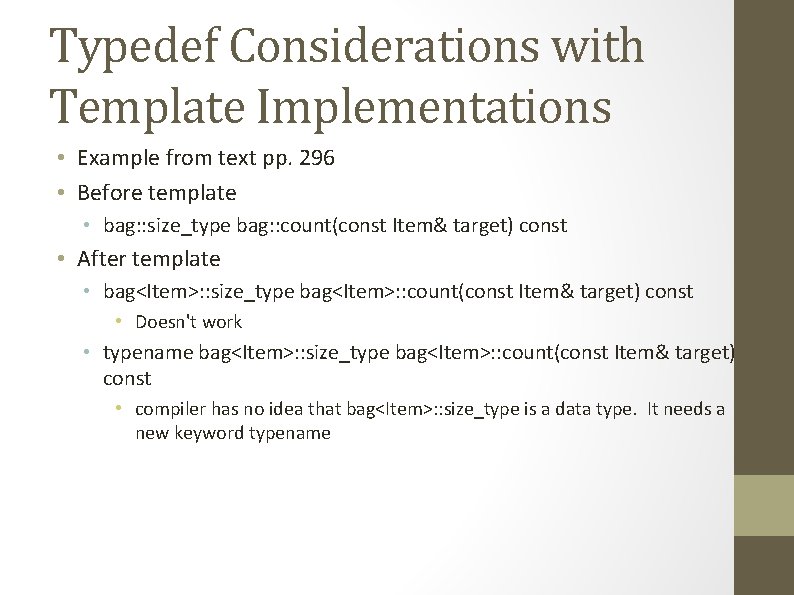
Typedef Considerations with Template Implementations • Example from text pp. 296 • Before template • bag: : size_type bag: : count(const Item& target) const • After template • bag<Item>: : size_type bag<Item>: : count(const Item& target) const • Doesn't work • typename bag<Item>: : size_type bag<Item>: : count(const Item& target) const • compiler has no idea that bag<Item>: : size_type is a data type. It needs a new keyword typename
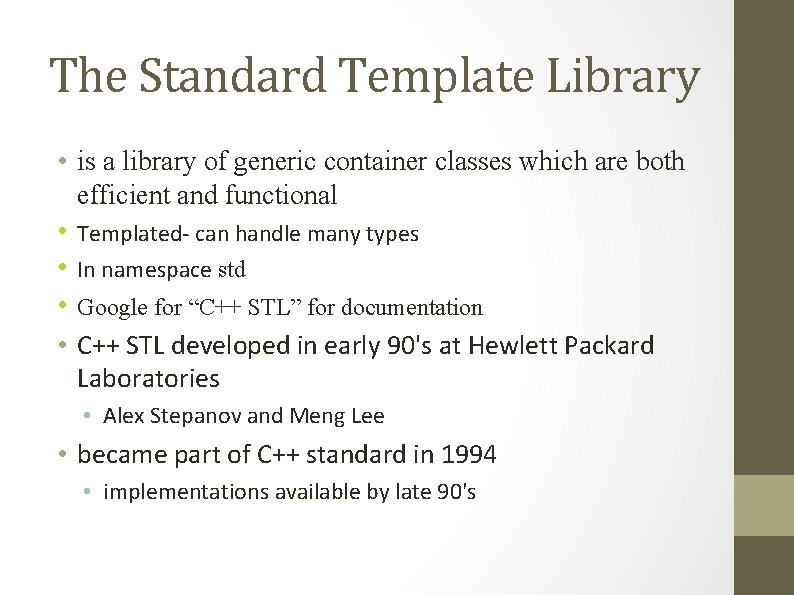
The Standard Template Library • is a library of generic container classes which are both efficient and functional • Templated- can handle many types • In namespace std • Google for “C++ STL” for documentation • C++ STL developed in early 90's at Hewlett Packard Laboratories • Alex Stepanov and Meng Lee • became part of C++ standard in 1994 • implementations available by late 90's
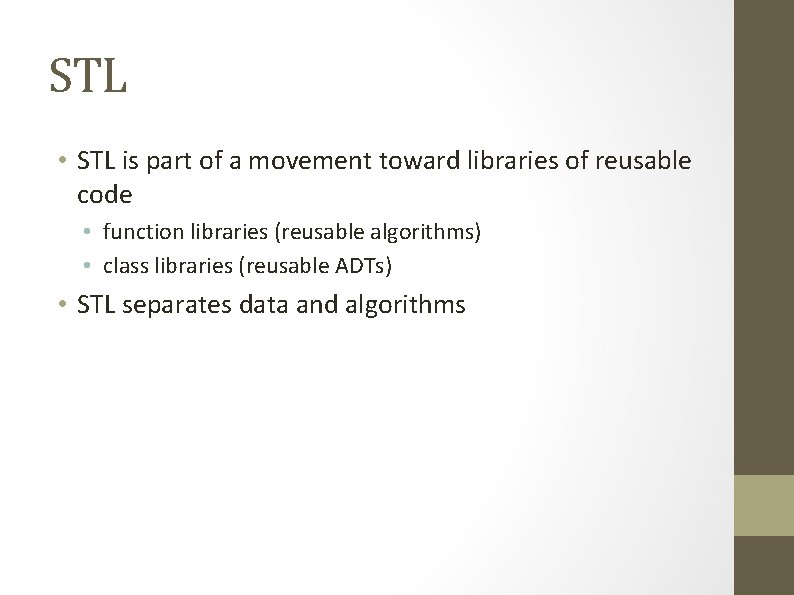
STL • STL is part of a movement toward libraries of reusable code • function libraries (reusable algorithms) • class libraries (reusable ADTs) • STL separates data and algorithms
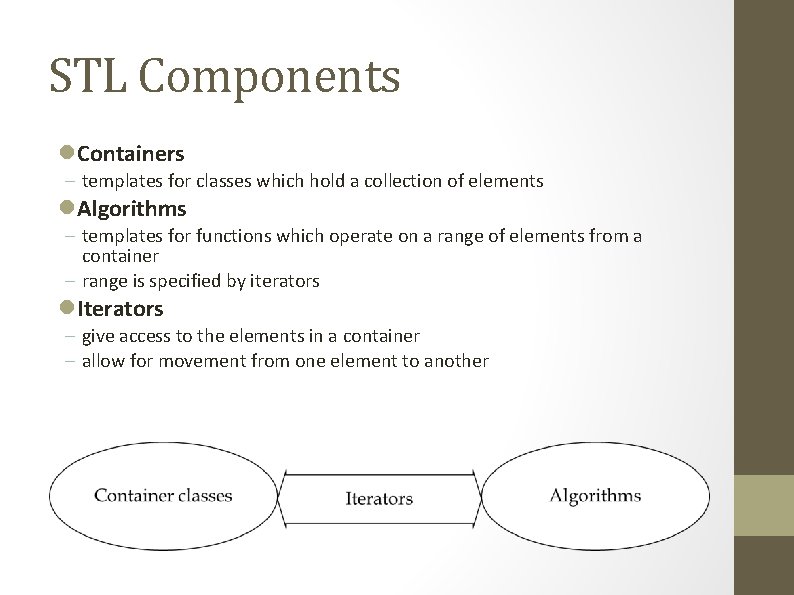
STL Components Containers templates for classes which hold a collection of elements Algorithms templates for functions which operate on a range of elements from a container range is specified by iterators Iterators give access to the elements in a container allow for movement from one element to another
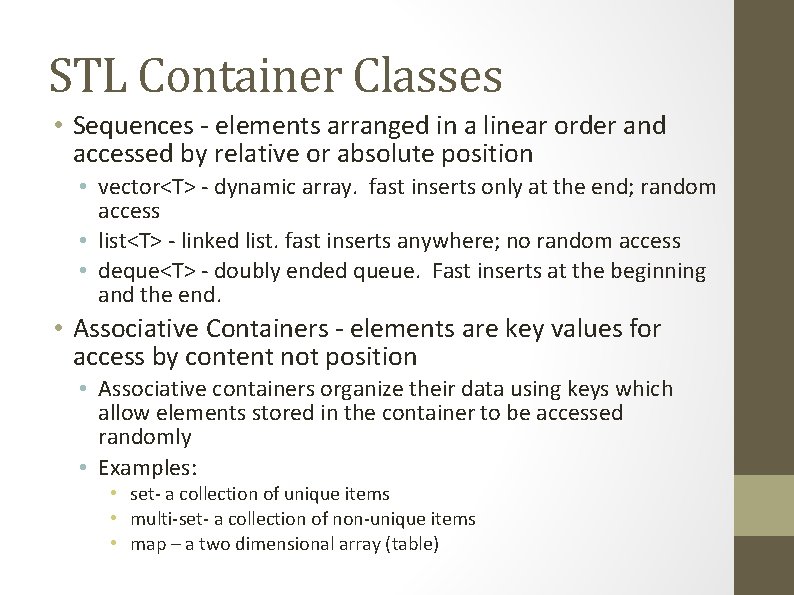
STL Container Classes • Sequences - elements arranged in a linear order and accessed by relative or absolute position • vector<T> - dynamic array. fast inserts only at the end; random access • list<T> - linked list. fast inserts anywhere; no random access • deque<T> - doubly ended queue. Fast inserts at the beginning and the end. • Associative Containers - elements are key values for access by content not position • Associative containers organize their data using keys which allow elements stored in the container to be accessed randomly • Examples: • set- a collection of unique items • multi-set- a collection of non-unique items • map – a two dimensional array (table)
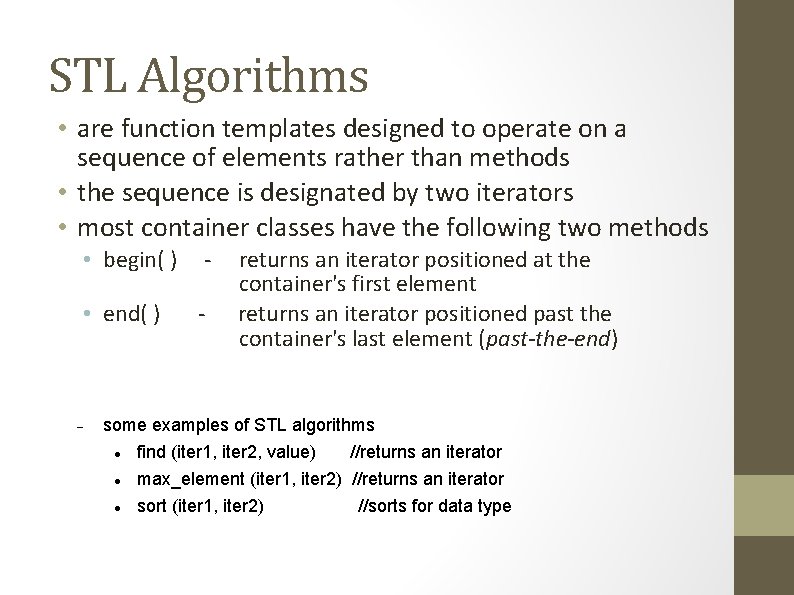
STL Algorithms • are function templates designed to operate on a sequence of elements rather than methods • the sequence is designated by two iterators • most container classes have the following two methods • begin( ) - • end( ) - returns an iterator positioned at the container's first element returns an iterator positioned past the container's last element (past-the-end) some examples of STL algorithms find (iter 1, iter 2, value) //returns an iterator max_element (iter 1, iter 2) //returns an iterator sort (iter 1, iter 2) //sorts for data type
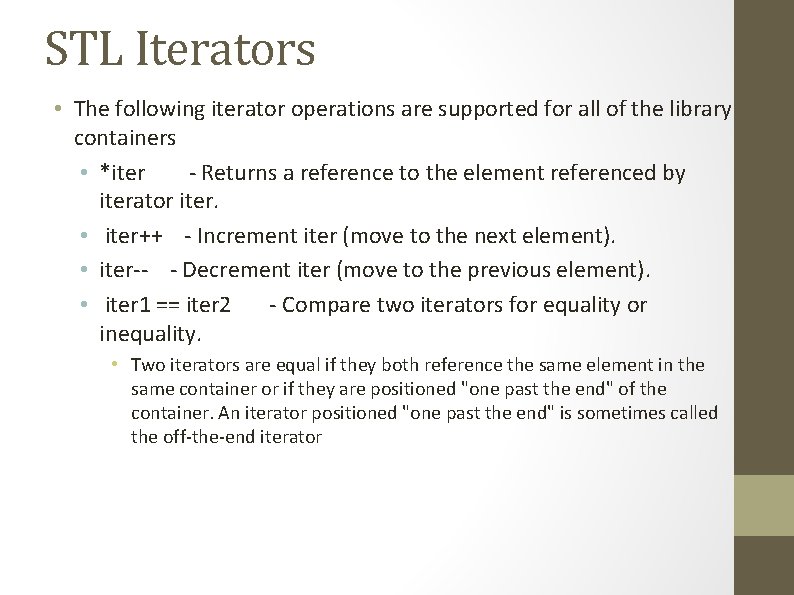
STL Iterators • The following iterator operations are supported for all of the library containers • *iter - Returns a reference to the element referenced by iterator iter. • iter++ - Increment iter (move to the next element). • iter-- - Decrement iter (move to the previous element). • iter 1 == iter 2 - Compare two iterators for equality or inequality. • Two iterators are equal if they both reference the same element in the same container or if they are positioned "one past the end" of the container. An iterator positioned "one past the end" is sometimes called the off-the-end iterator
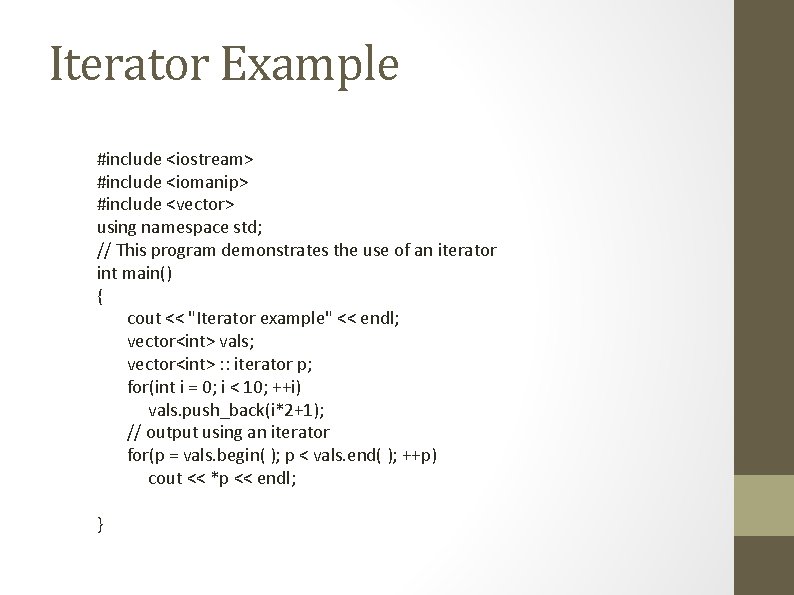
Iterator Example #include <iostream> #include <iomanip> #include <vector> using namespace std; // This program demonstrates the use of an iterator int main() { cout << "Iterator example" << endl; vector<int> vals; vector<int> : : iterator p; for(int i = 0; i < 10; ++i) vals. push_back(i*2+1); // output using an iterator for(p = vals. begin( ); p < vals. end( ); ++p) cout << *p << endl; }
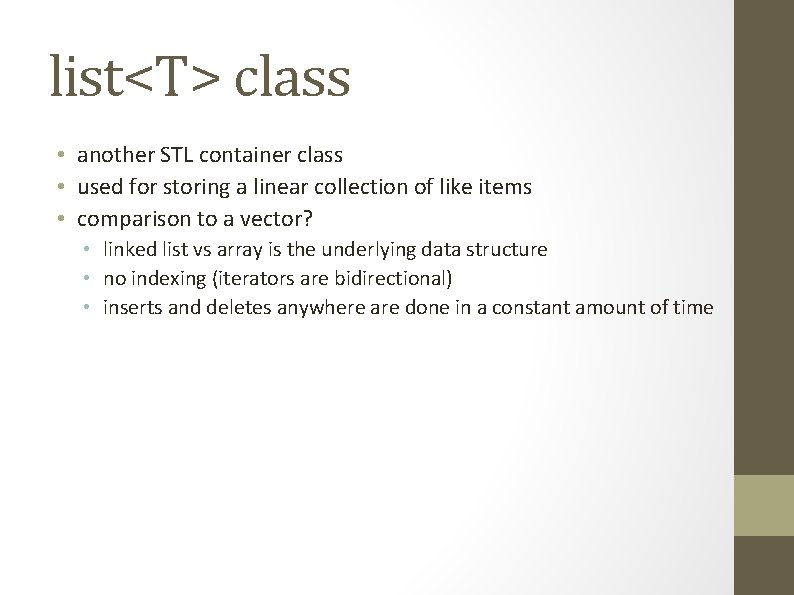
list<T> class • another STL container class • used for storing a linear collection of like items • comparison to a vector? • linked list vs array is the underlying data structure • no indexing (iterators are bidirectional) • inserts and deletes anywhere are done in a constant amount of time
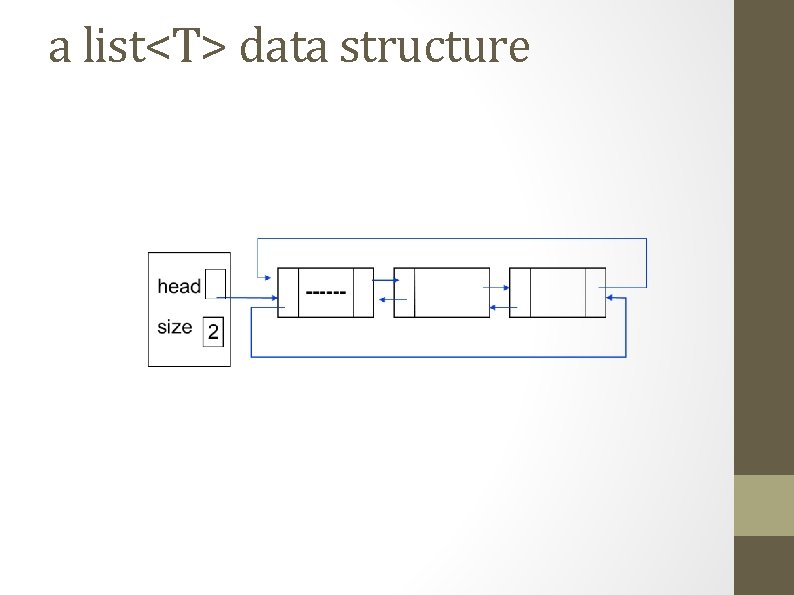
a list<T> data structure
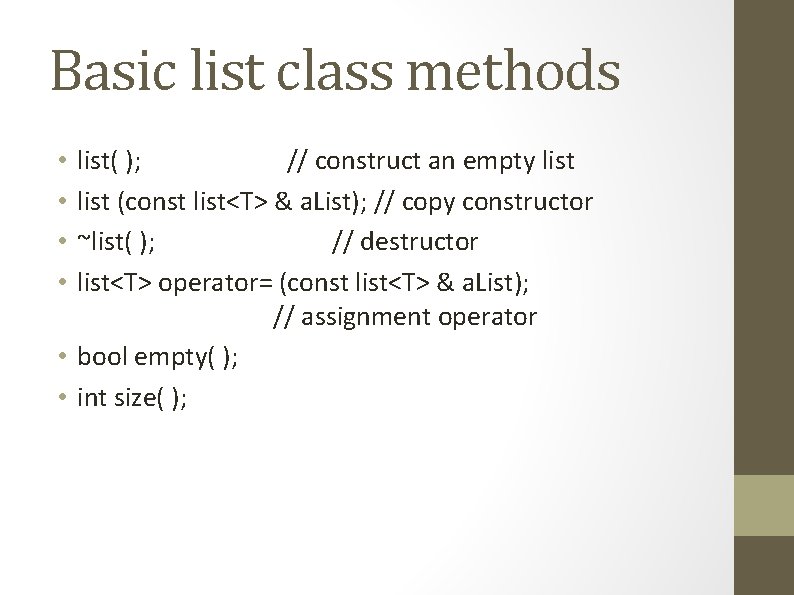
Basic list class methods list( ); // construct an empty list (const list<T> & a. List); // copy constructor ~list( ); // destructor list<T> operator= (const list<T> & a. List); // assignment operator • bool empty( ); • int size( ); • •
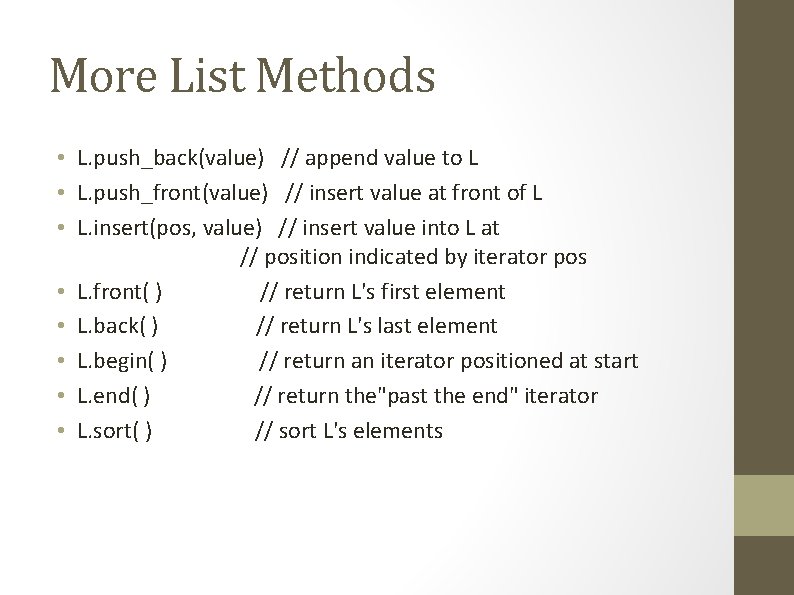
More List Methods • L. push_back(value) // append value to L • L. push_front(value) // insert value at front of L • L. insert(pos, value) // insert value into L at // position indicated by iterator pos • L. front( ) // return L's first element • L. back( ) // return L's last element • L. begin( ) // return an iterator positioned at start • L. end( ) // return the"past the end" iterator • L. sort( ) // sort L's elements
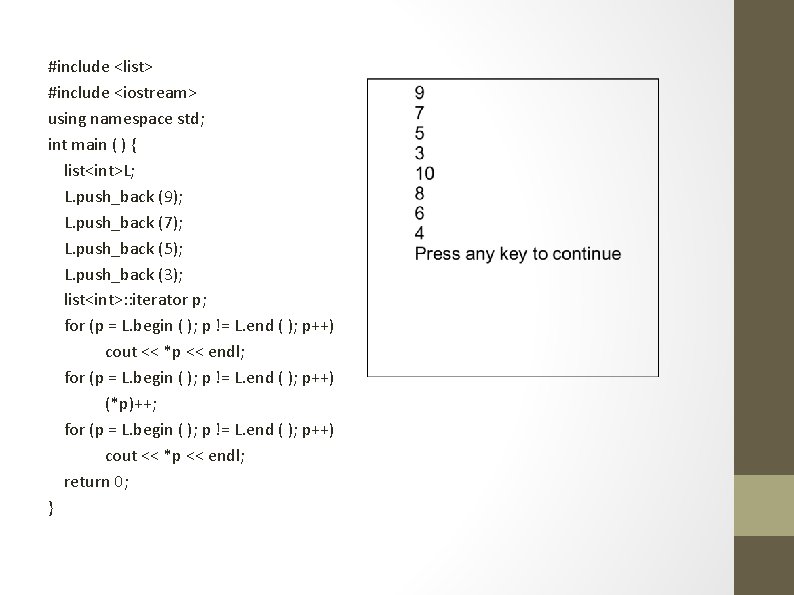
#include <list> #include <iostream> using namespace std; int main ( ) { list<int>L; L. push_back (9); L. push_back (7); L. push_back (5); L. push_back (3); list<int>: : iterator p; for (p = L. begin ( ); p != L. end ( ); p++) cout << *p << endl; for (p = L. begin ( ); p != L. end ( ); p++) (*p)++; for (p = L. begin ( ); p != L. end ( ); p++) cout << *p << endl; return 0; }
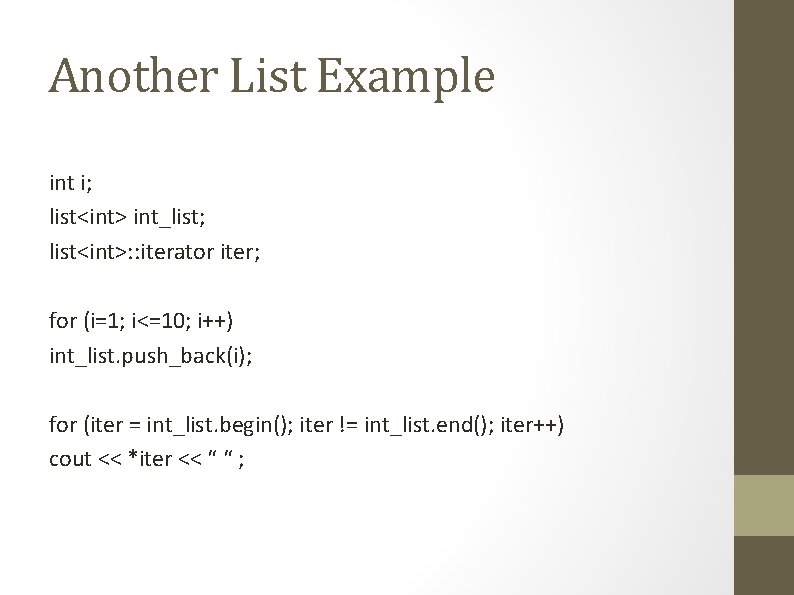
Another List Example int i; list<int> int_list; list<int>: : iterator iter; for (i=1; i<=10; i++) int_list. push_back(i); for (iter = int_list. begin(); iter != int_list. end(); iter++) cout << *iter << “ “ ;
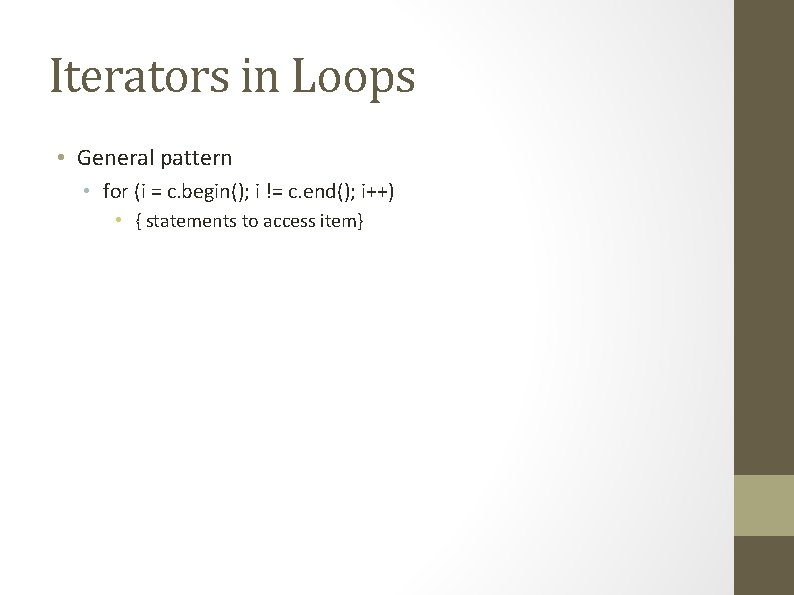
Iterators in Loops • General pattern • for (i = c. begin(); i != c. end(); i++) • { statements to access item}
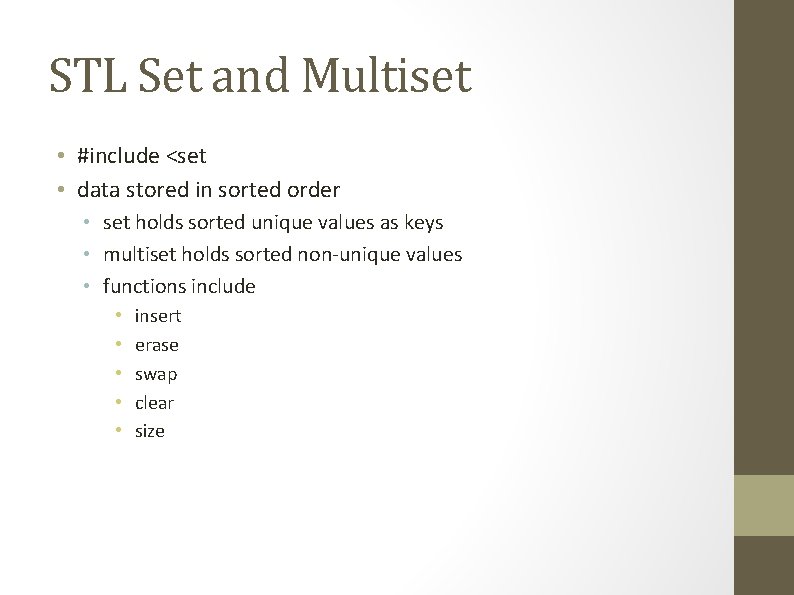
STL Set and Multiset • #include <set • data stored in sorted order • set holds sorted unique values as keys • multiset holds sorted non-unique values • functions include • • • insert erase swap clear size
Antigentest åre
Sebutkan fitur-fitur yang terdapat di panel publisher
Powerpoim
Spotlight effect definition psychology
Cmmi project management
Online planbook
Job to be done template
Pc dmis report templates
Quarterly business review templates
Strategic map
Allppts
247 templates
Naysayer templates
Rotary powerpoint template
Swift ui templates
Islamic powerpoint
Pecha template
Cdc project management templates
Football pitch templates
Emds templates
Tfs process template editor
Templates
Kpi development template
Swimlane template
Edubuzz
Change management heat map template
C generic programming
Free ppt templates allppt.com
Multiscatter
Https://creativemarket.com/
Allppt.com _ free powerpoint templates diagrams and charts