C 3 0 Tom Roeder CS 215 2006
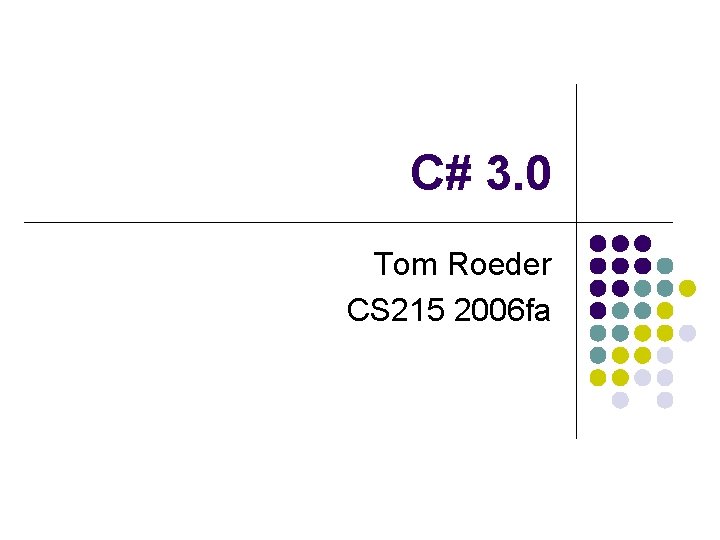
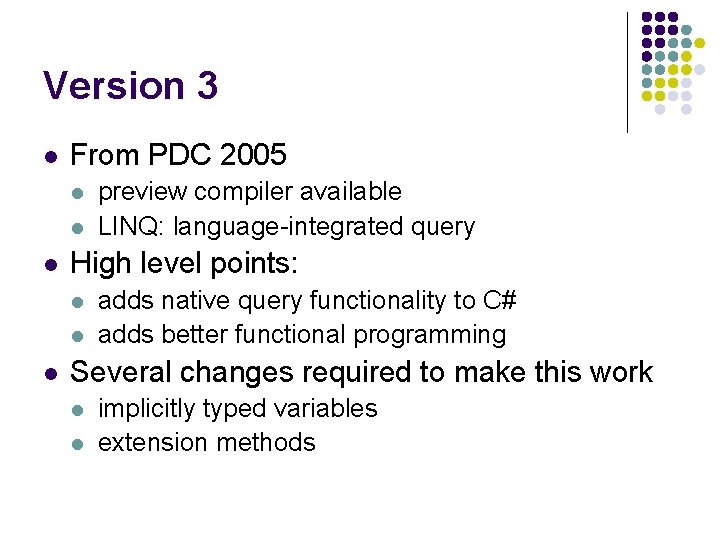
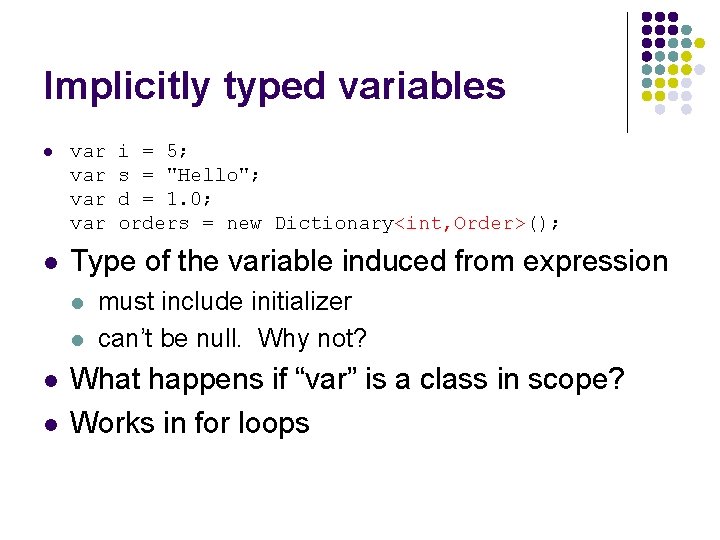
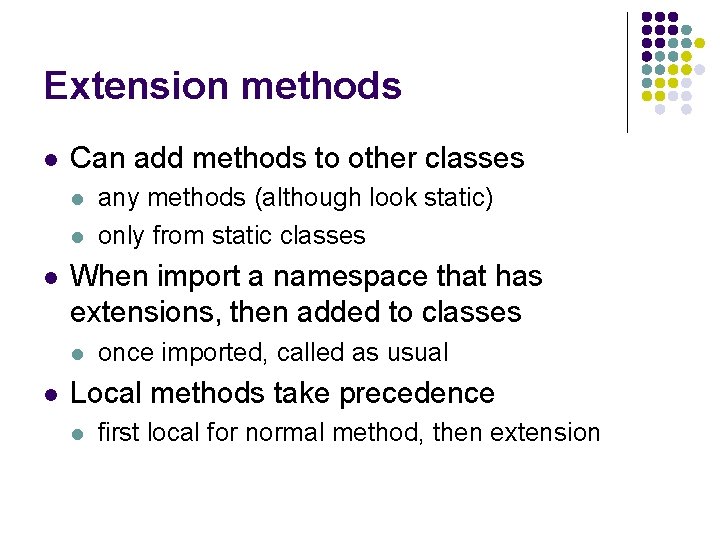
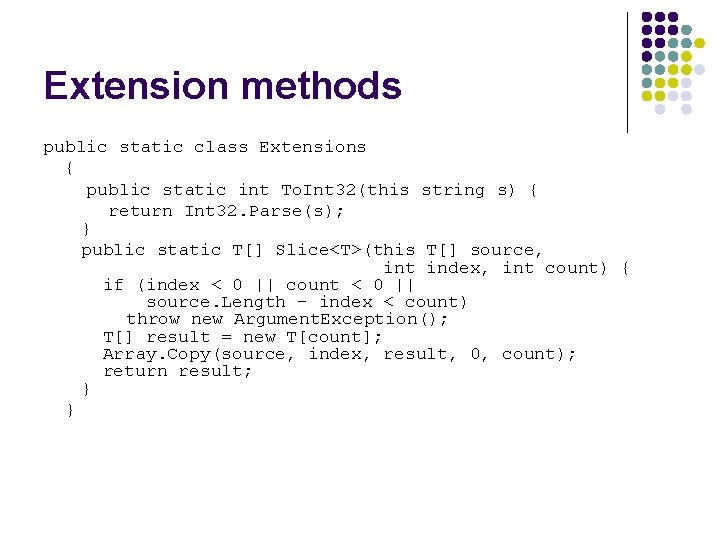
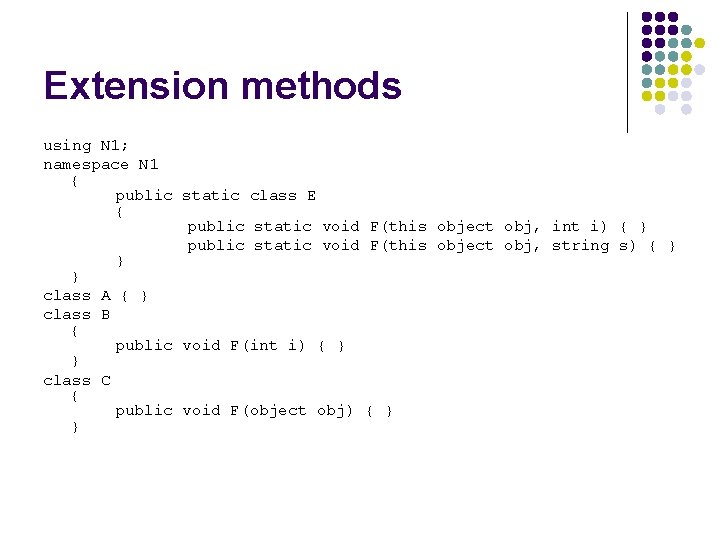
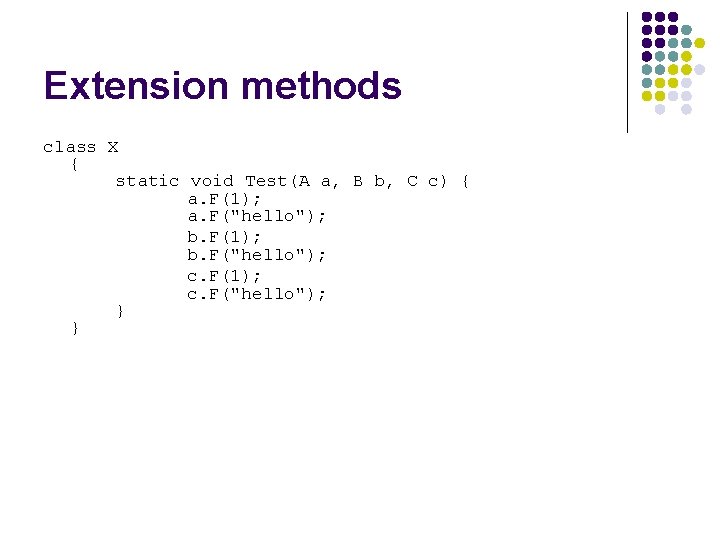
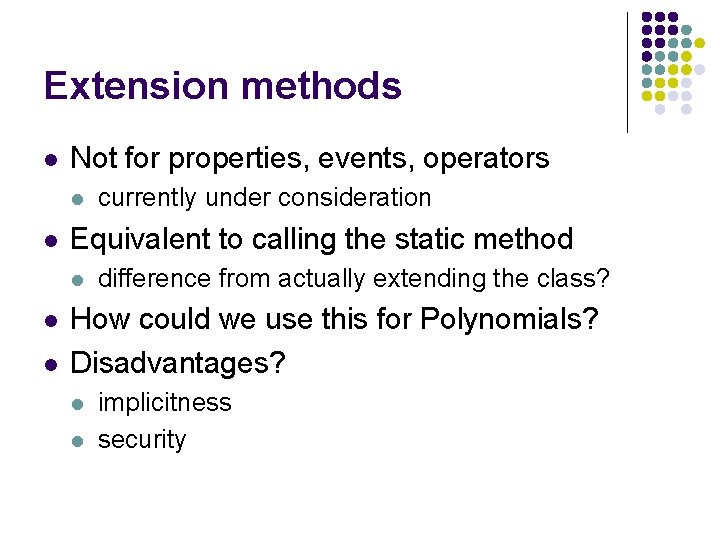
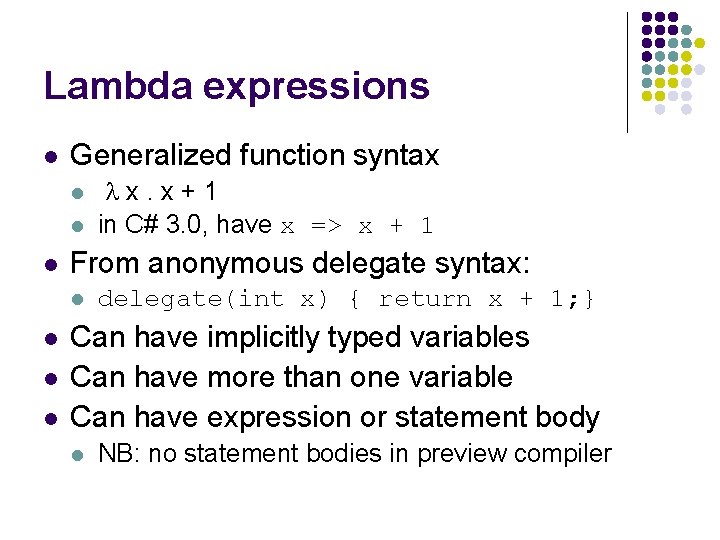
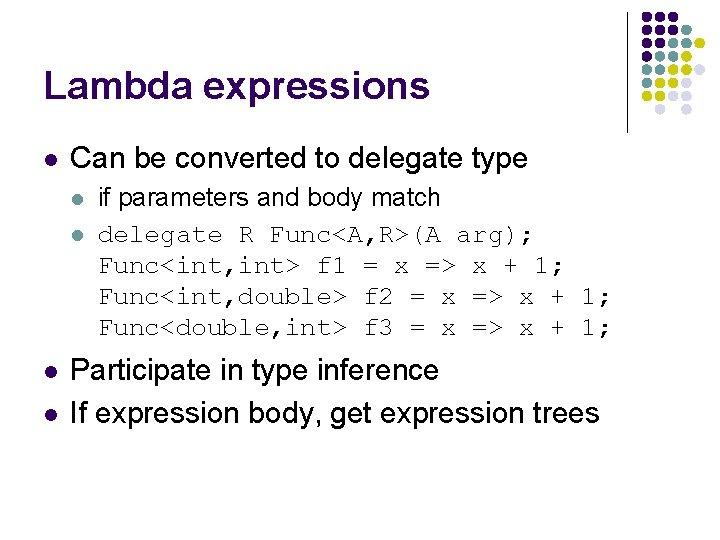
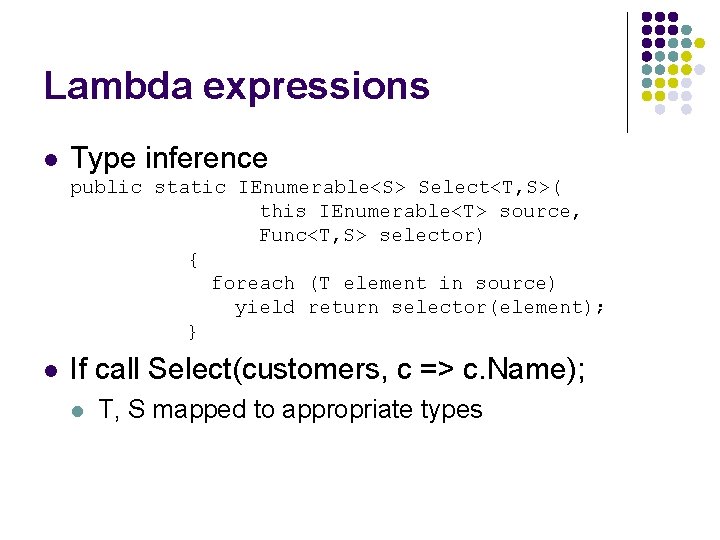
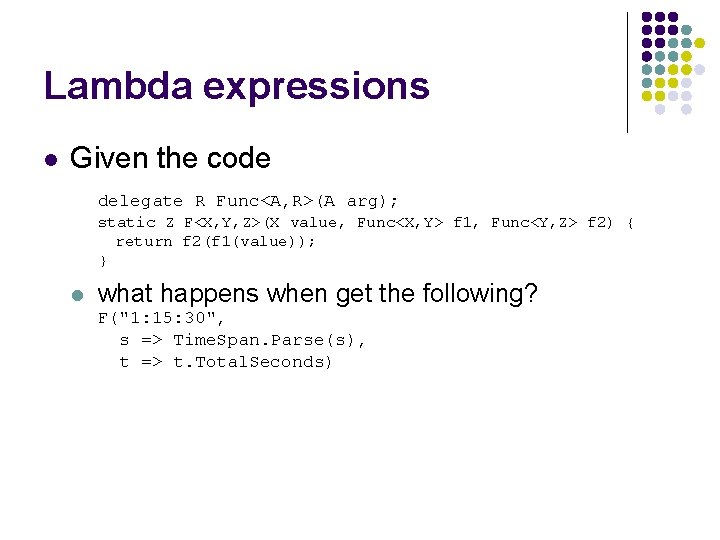
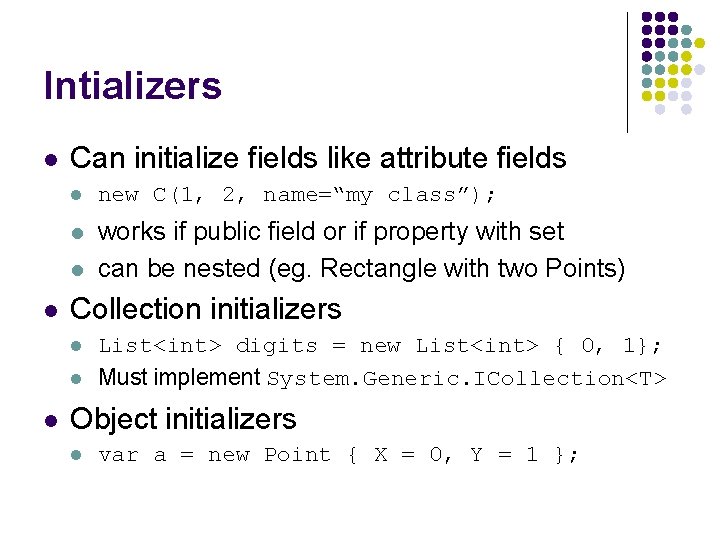
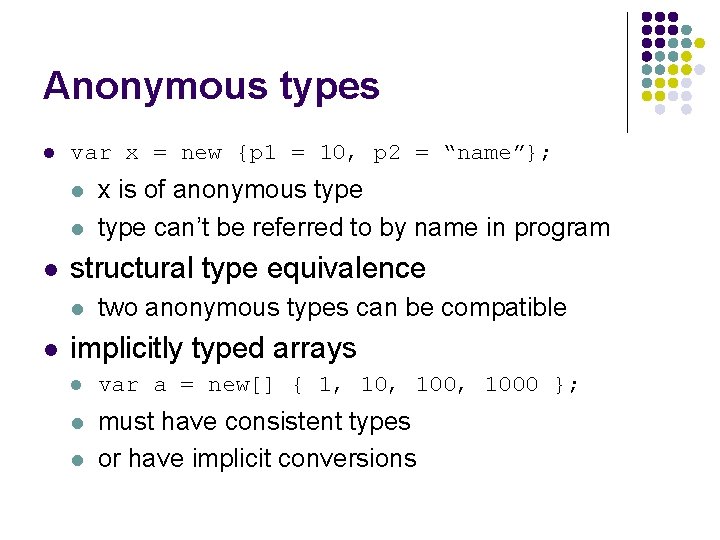
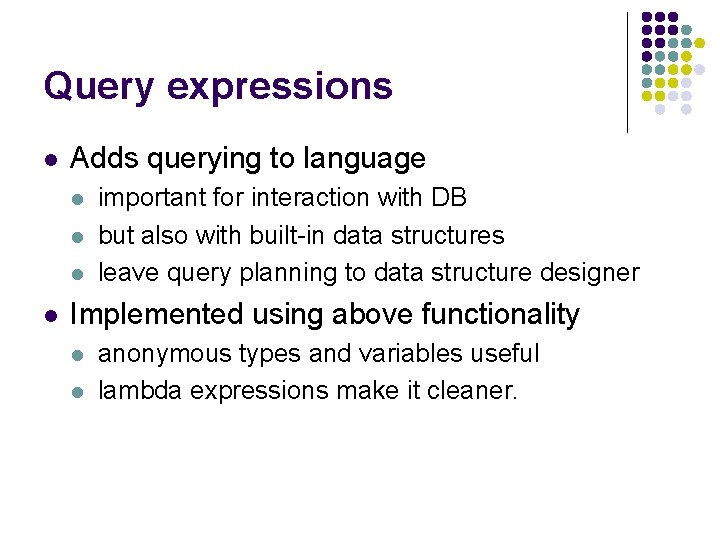
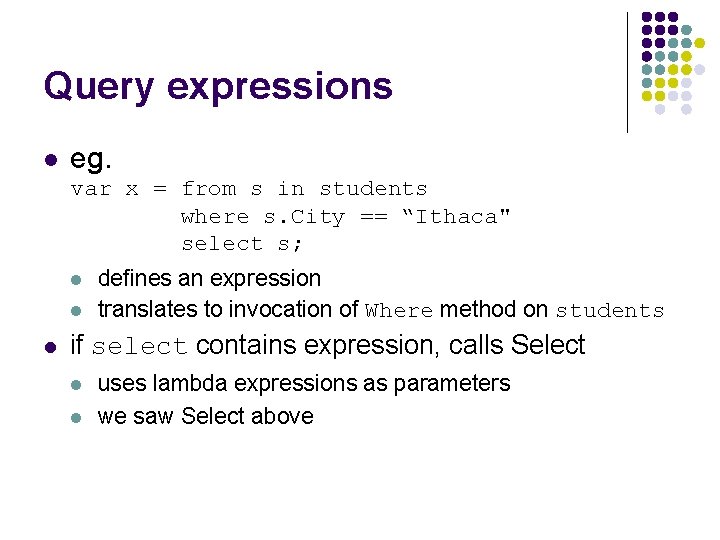
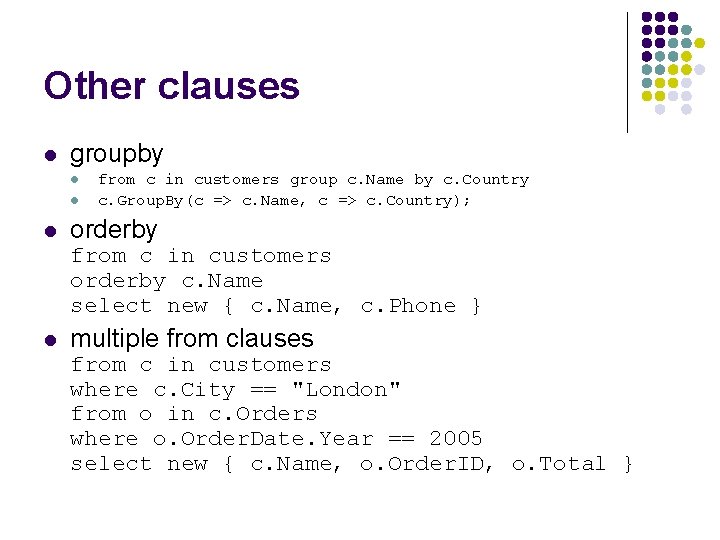
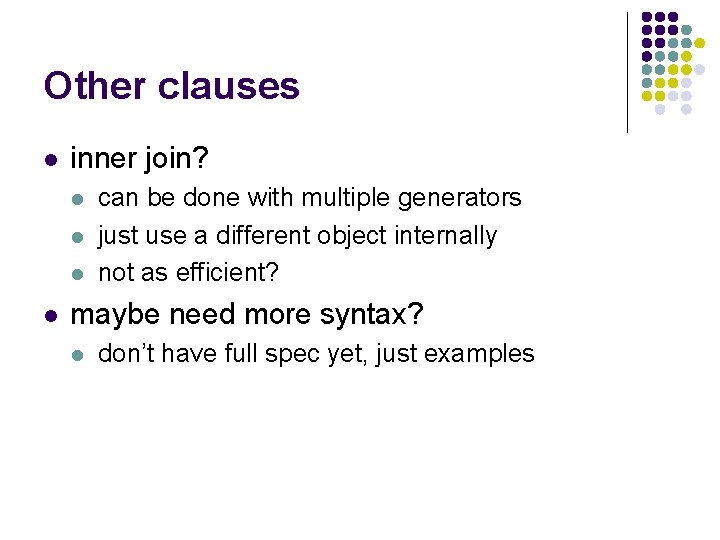
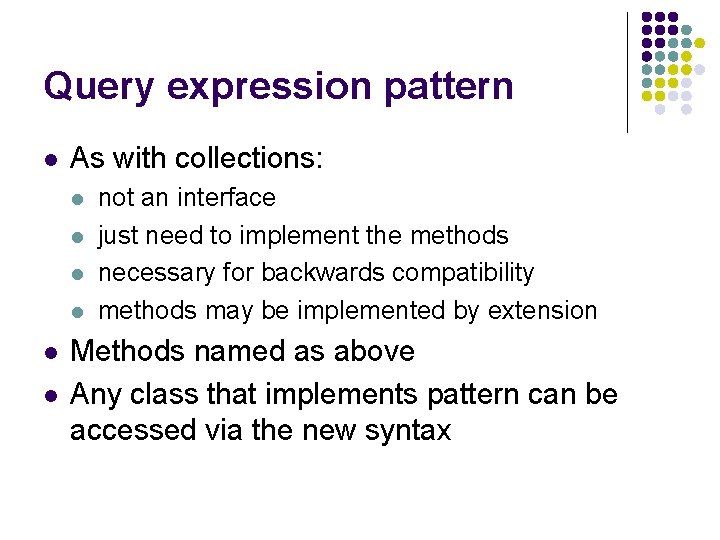
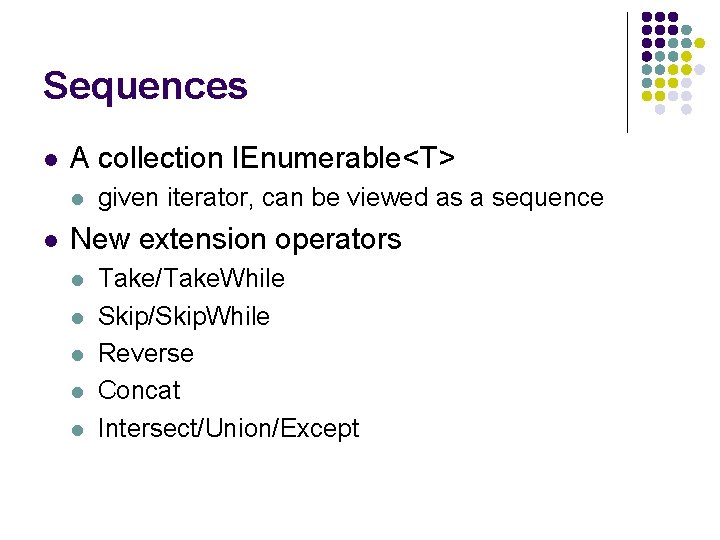
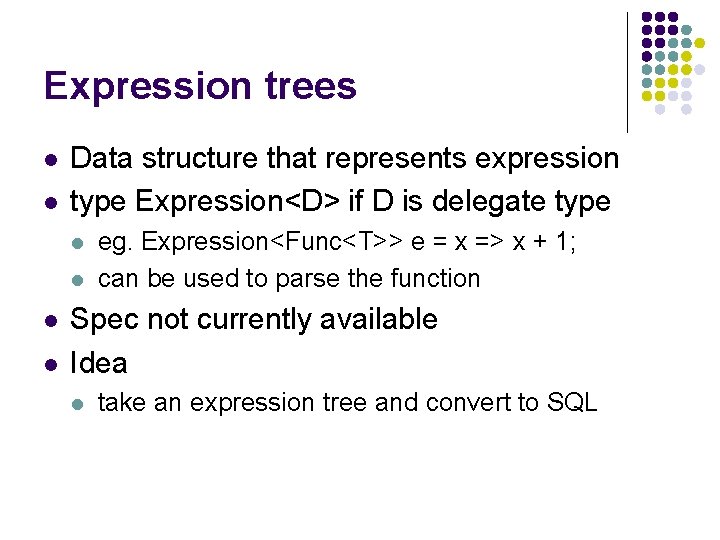
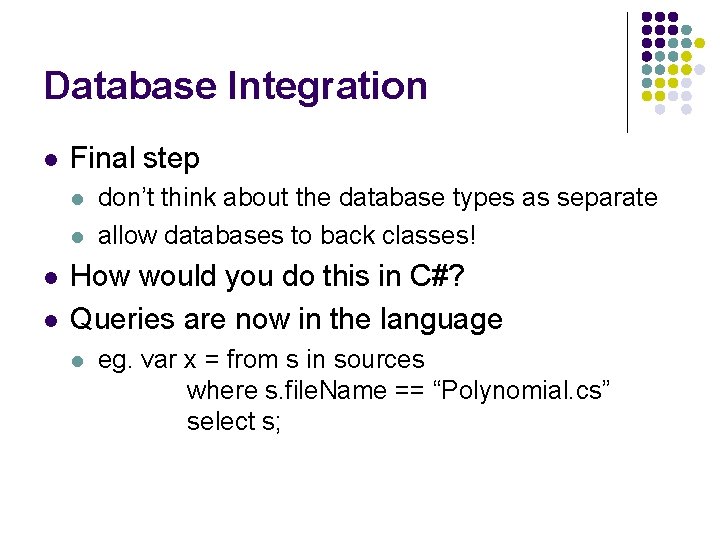
![DLinq Attributes l [Table(Name="DVDTable")] public class DVD { [Column(Id = true)] public string Title; DLinq Attributes l [Table(Name="DVDTable")] public class DVD { [Column(Id = true)] public string Title;](https://slidetodoc.com/presentation_image_h2/72d1bfc3fe03d47317b96ec484ecfcc5/image-23.jpg)
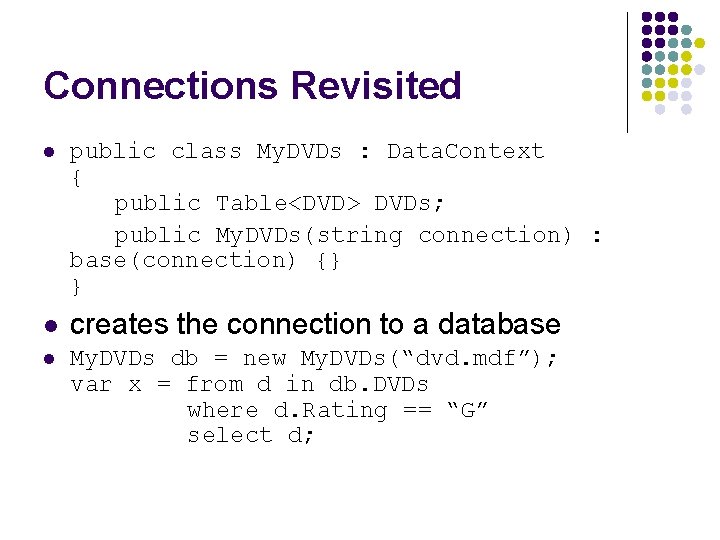
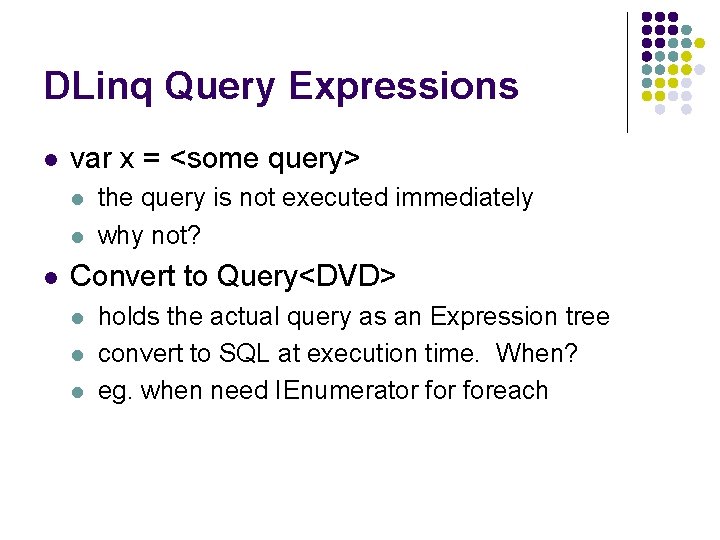
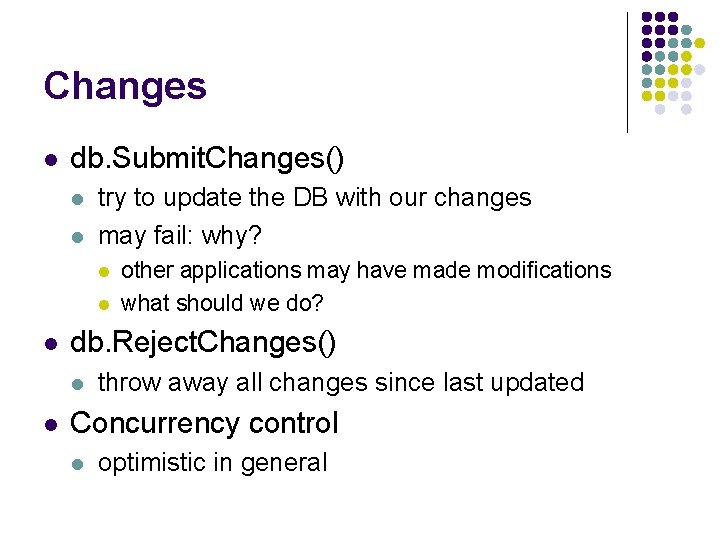
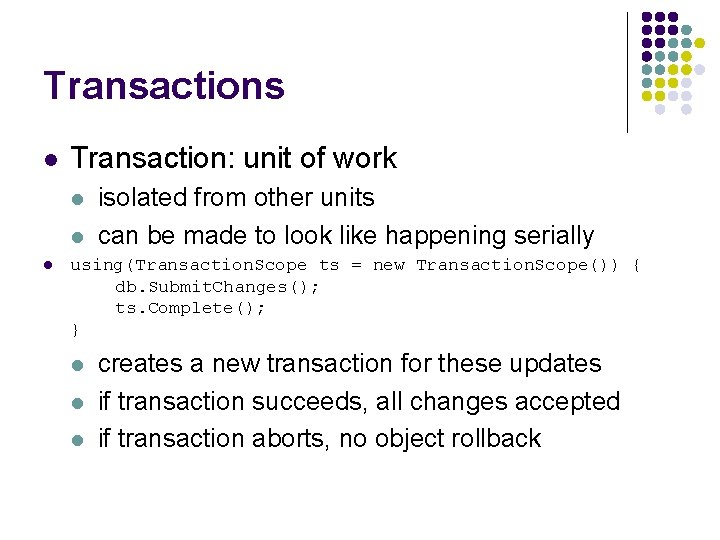
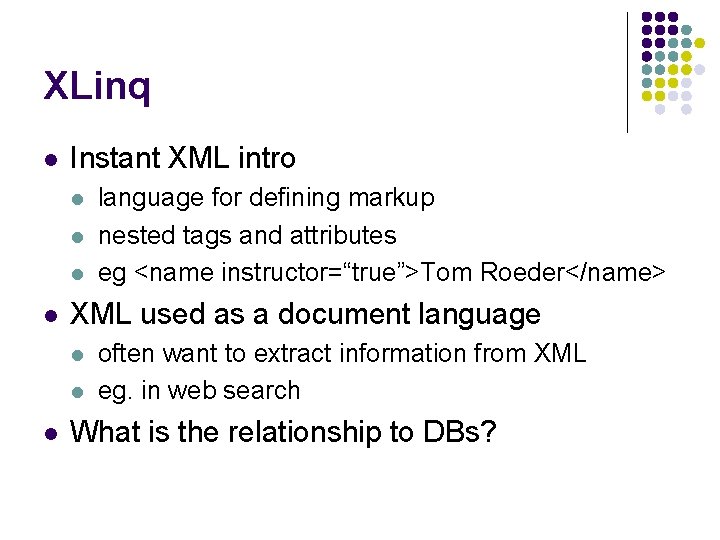
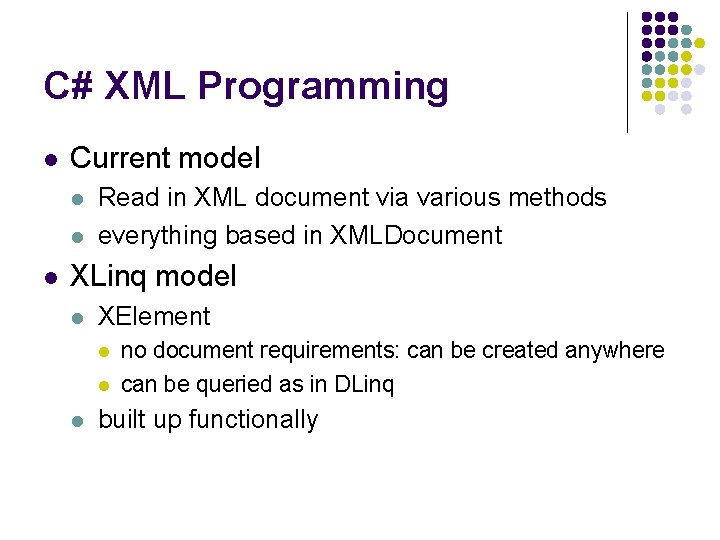
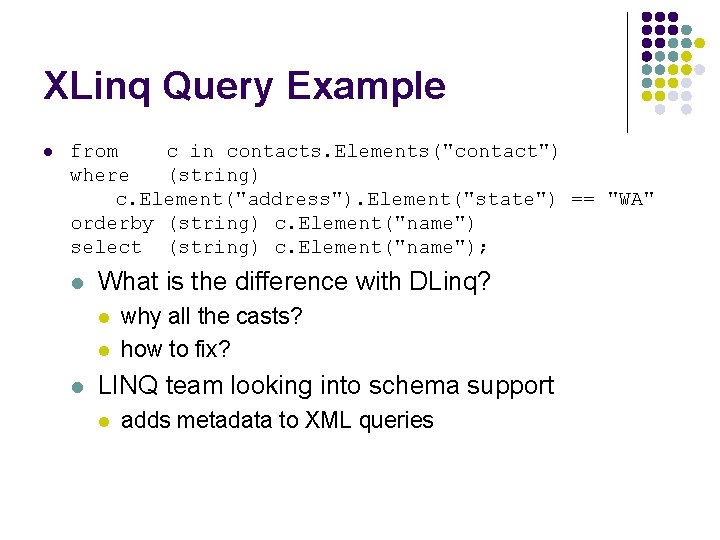
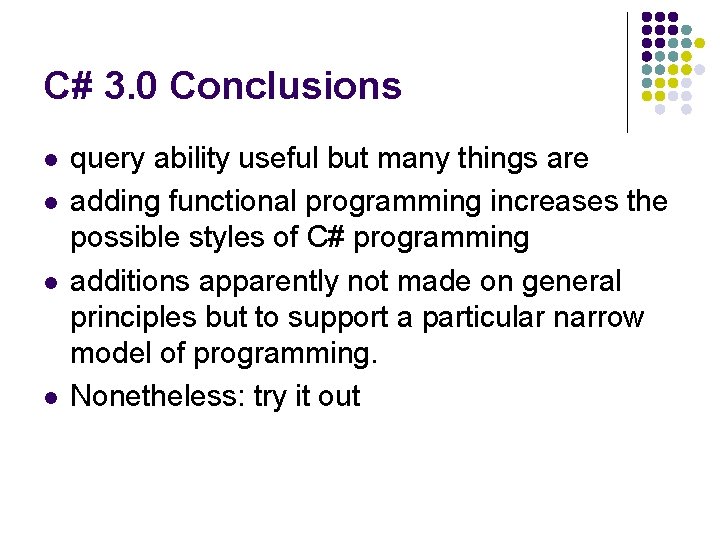
- Slides: 31
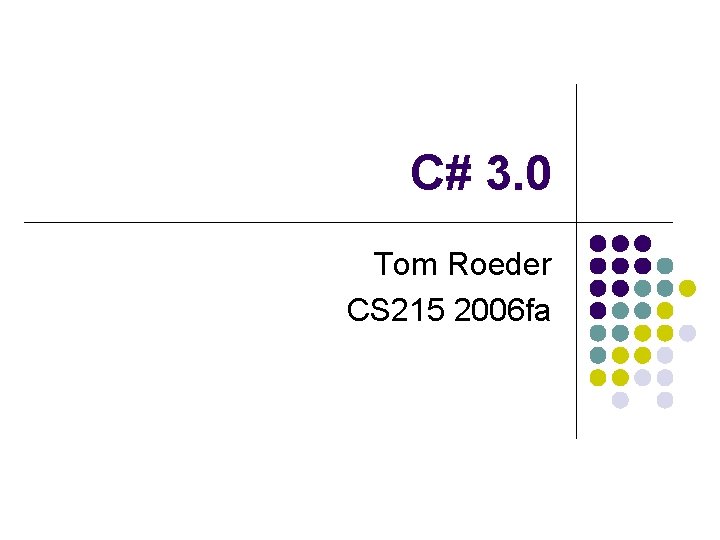
C# 3. 0 Tom Roeder CS 215 2006 fa
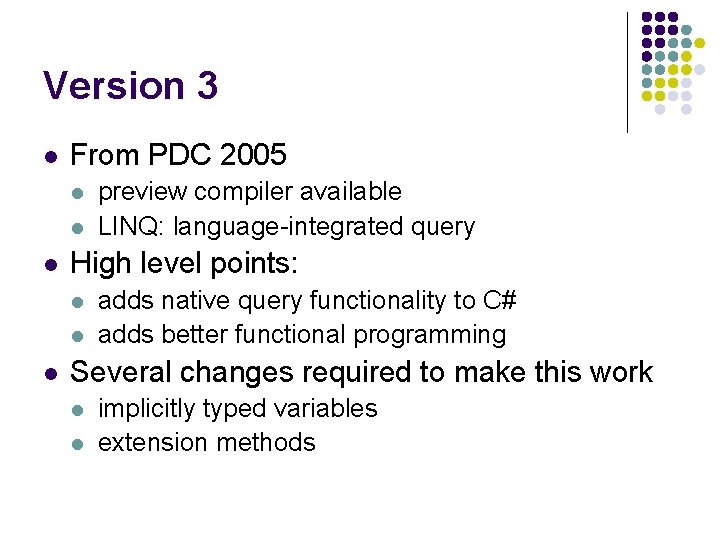
Version 3 l From PDC 2005 l l l High level points: l l l preview compiler available LINQ: language-integrated query adds native query functionality to C# adds better functional programming Several changes required to make this work l l implicitly typed variables extension methods
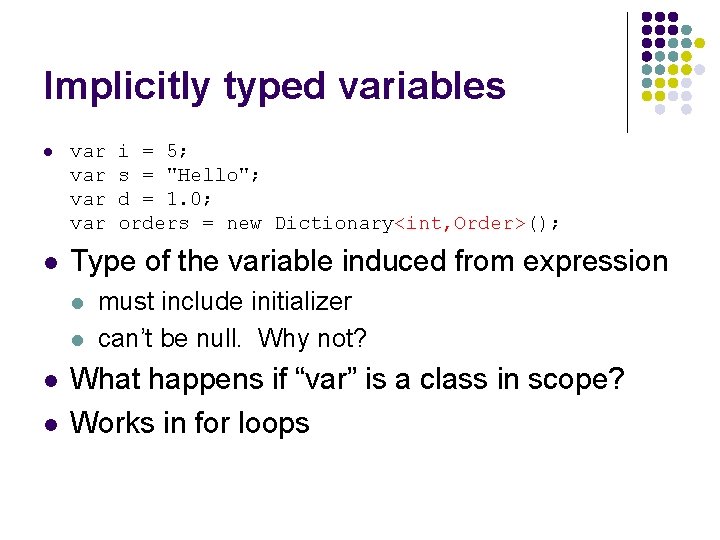
Implicitly typed variables l l var var Type of the variable induced from expression l l i = 5; s = "Hello"; d = 1. 0; orders = new Dictionary<int, Order>(); must include initializer can’t be null. Why not? What happens if “var” is a class in scope? Works in for loops
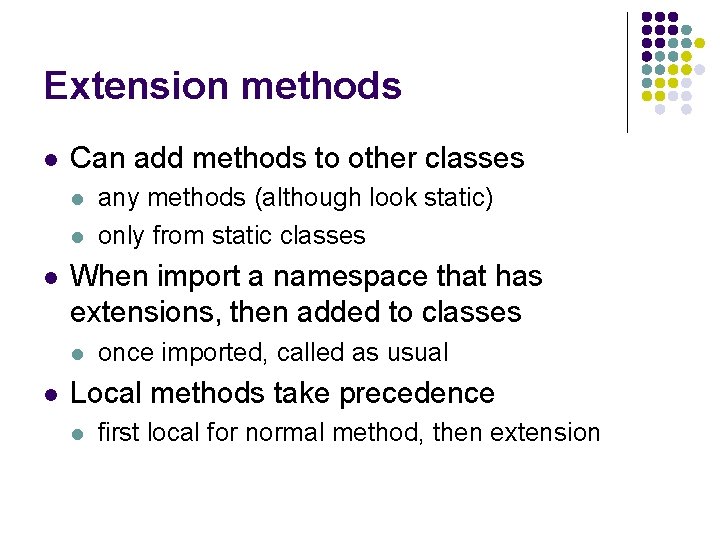
Extension methods l Can add methods to other classes l l l When import a namespace that has extensions, then added to classes l l any methods (although look static) only from static classes once imported, called as usual Local methods take precedence l first local for normal method, then extension
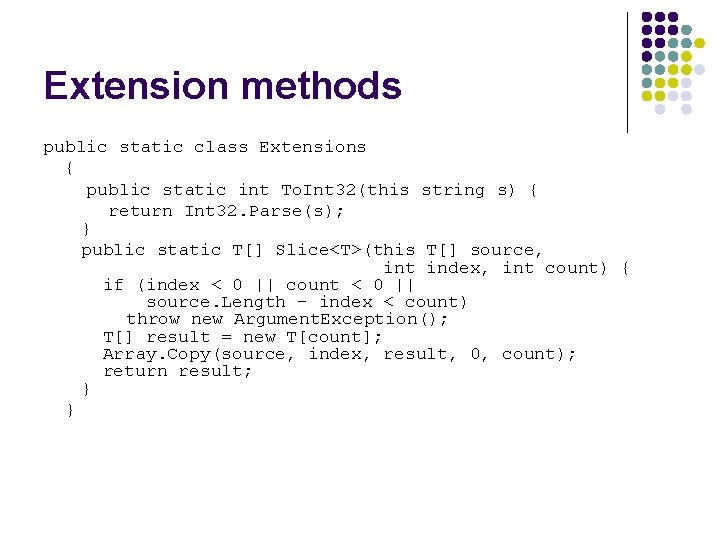
Extension methods public static class Extensions { public static int To. Int 32(this string s) { return Int 32. Parse(s); } public static T[] Slice<T>(this T[] source, int index, int count) { if (index < 0 || count < 0 || source. Length – index < count) throw new Argument. Exception(); T[] result = new T[count]; Array. Copy(source, index, result, 0, count); return result; } }
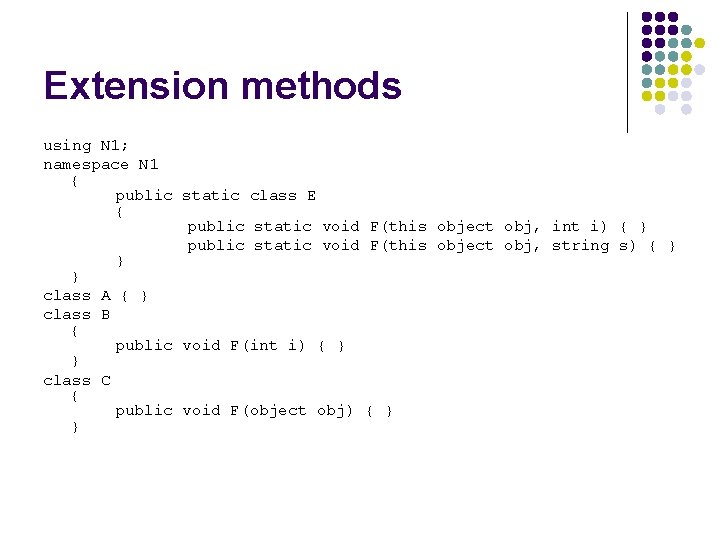
Extension methods using N 1; namespace N 1 { public static class E { public static void F(this object obj, int i) { } public static void F(this object obj, string s) { } } } class A { } class B { public void F(int i) { } } class C { public void F(object obj) { } }
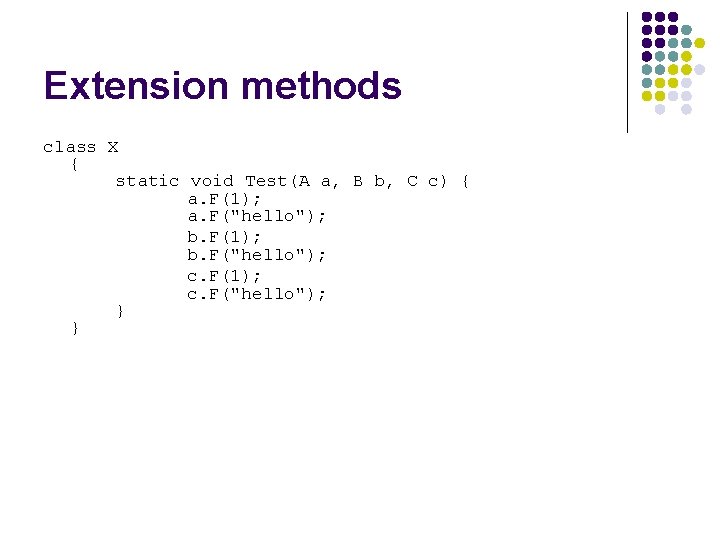
Extension methods class X { static void Test(A a, B b, C c) { a. F(1); a. F("hello"); b. F(1); b. F("hello"); c. F(1); c. F("hello"); } }
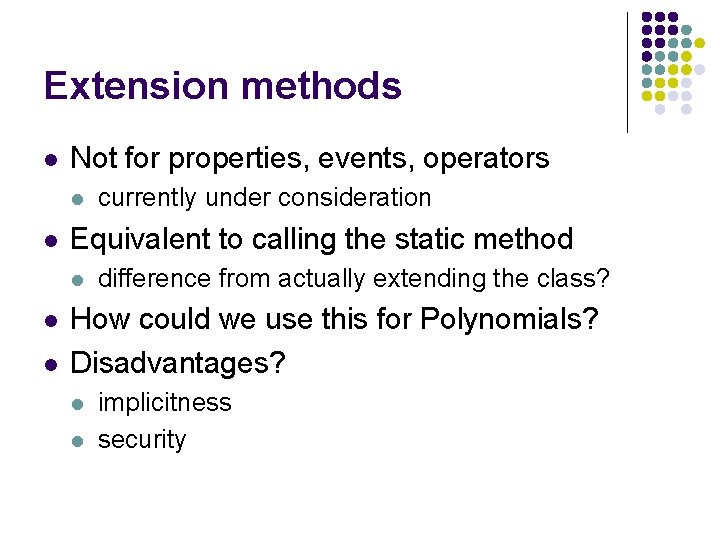
Extension methods l Not for properties, events, operators l l Equivalent to calling the static method l l l currently under consideration difference from actually extending the class? How could we use this for Polynomials? Disadvantages? l l implicitness security
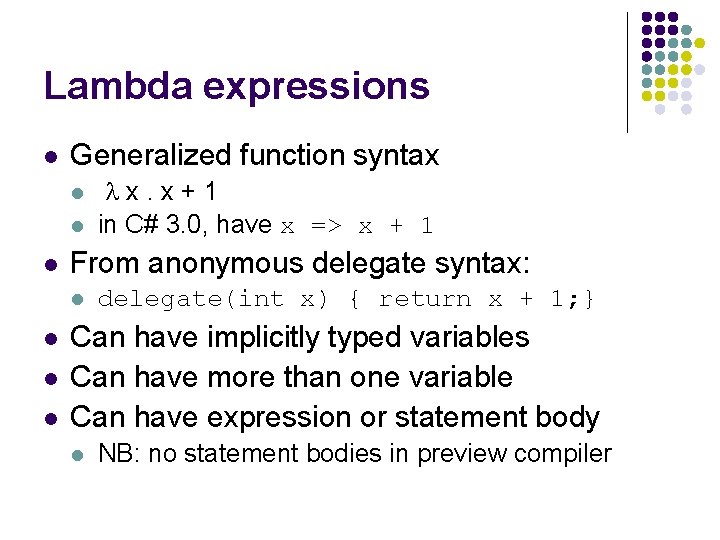
Lambda expressions l Generalized function syntax l l l From anonymous delegate syntax: l l x. x+1 in C# 3. 0, have x => x + 1 delegate(int x) { return x + 1; } Can have implicitly typed variables Can have more than one variable Can have expression or statement body l NB: no statement bodies in preview compiler
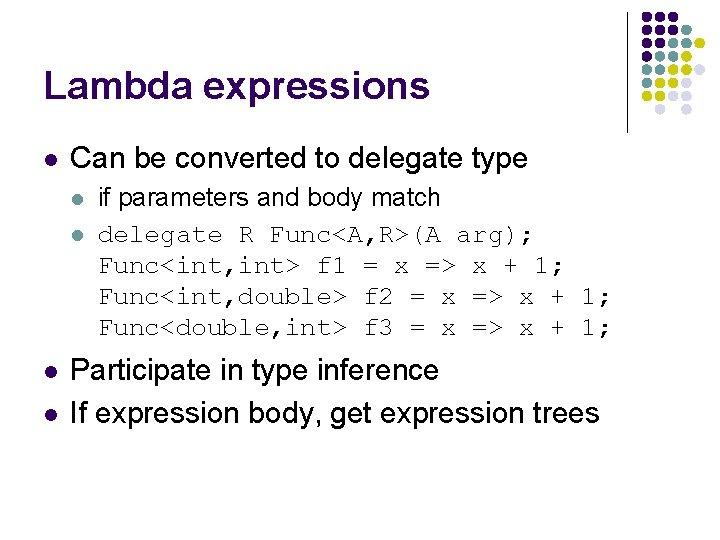
Lambda expressions l Can be converted to delegate type l l if parameters and body match delegate R Func<A, R>(A arg); Func<int, int> f 1 = x => x + 1; Func<int, double> f 2 = x => x + 1; Func<double, int> f 3 = x => x + 1; Participate in type inference If expression body, get expression trees
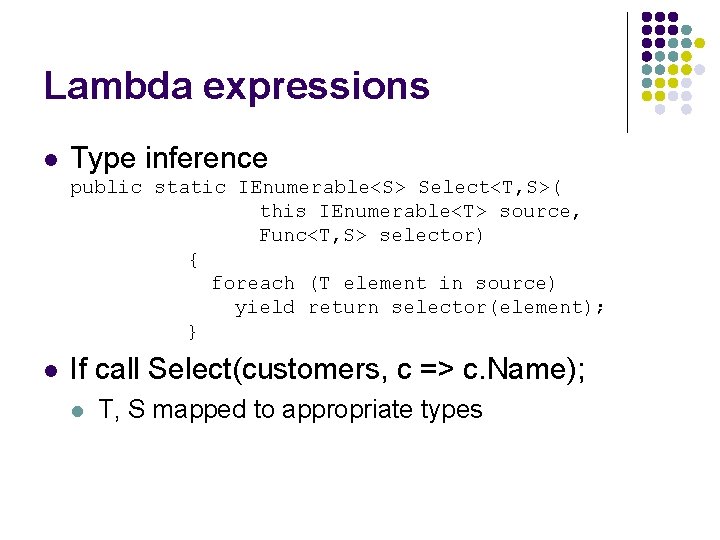
Lambda expressions l Type inference public static IEnumerable<S> Select<T, S>( this IEnumerable<T> source, Func<T, S> selector) { foreach (T element in source) yield return selector(element); } l If call Select(customers, c => c. Name); l T, S mapped to appropriate types
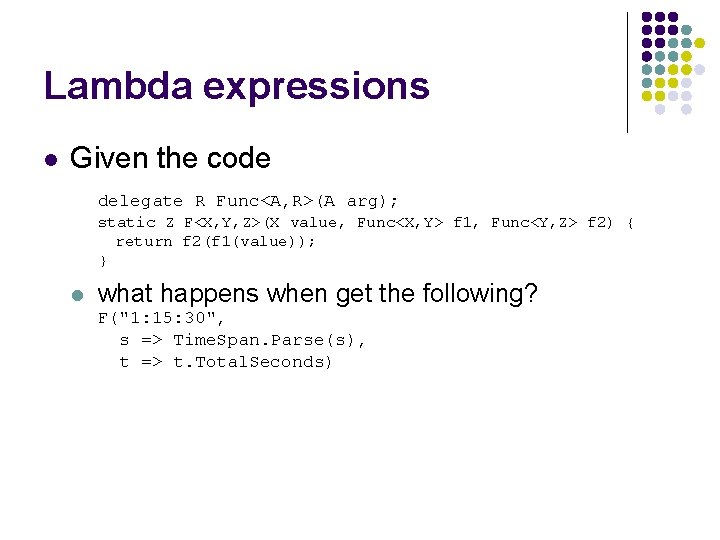
Lambda expressions l Given the code delegate R Func<A, R>(A arg); static Z F<X, Y, Z>(X value, Func<X, Y> f 1, Func<Y, Z> f 2) { return f 2(f 1(value)); } l what happens when get the following? F("1: 15: 30", s => Time. Span. Parse(s), t => t. Total. Seconds)
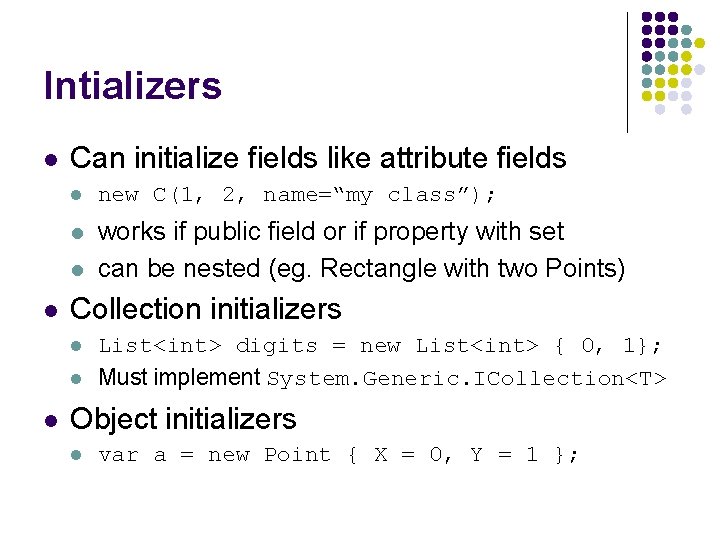
Intializers l Can initialize fields like attribute fields l new C(1, 2, name=“my class”); l works if public field or if property with set can be nested (eg. Rectangle with two Points) l l Collection initializers l l l List<int> digits = new List<int> { 0, 1}; Must implement System. Generic. ICollection<T> Object initializers l var a = new Point { X = 0, Y = 1 };
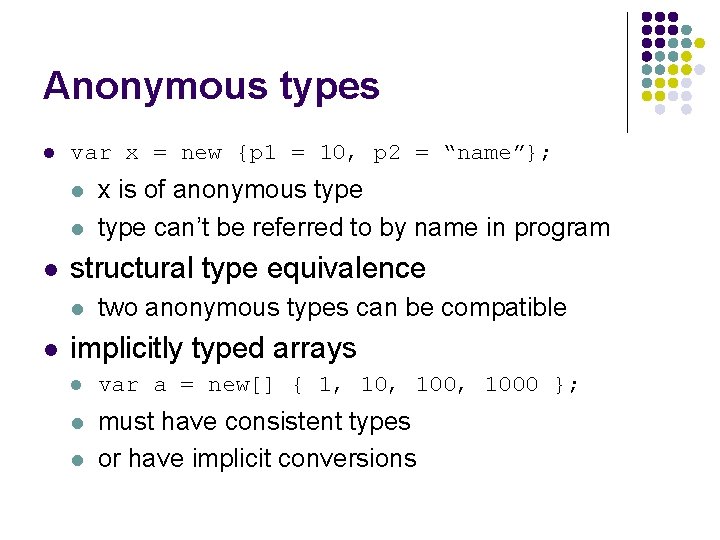
Anonymous types l var x = new {p 1 = 10, p 2 = “name”}; l l l structural type equivalence l l x is of anonymous type can’t be referred to by name in program two anonymous types can be compatible implicitly typed arrays l var a = new[] { 1, 100, 1000 }; l must have consistent types or have implicit conversions l
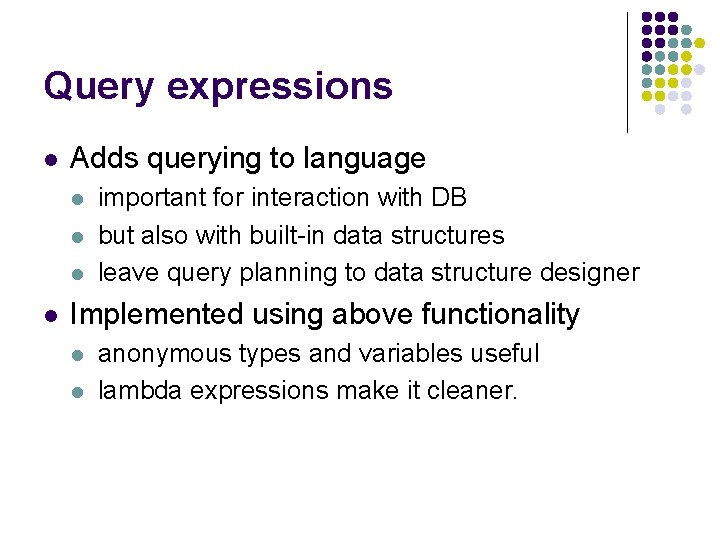
Query expressions l Adds querying to language l l important for interaction with DB but also with built-in data structures leave query planning to data structure designer Implemented using above functionality l l anonymous types and variables useful lambda expressions make it cleaner.
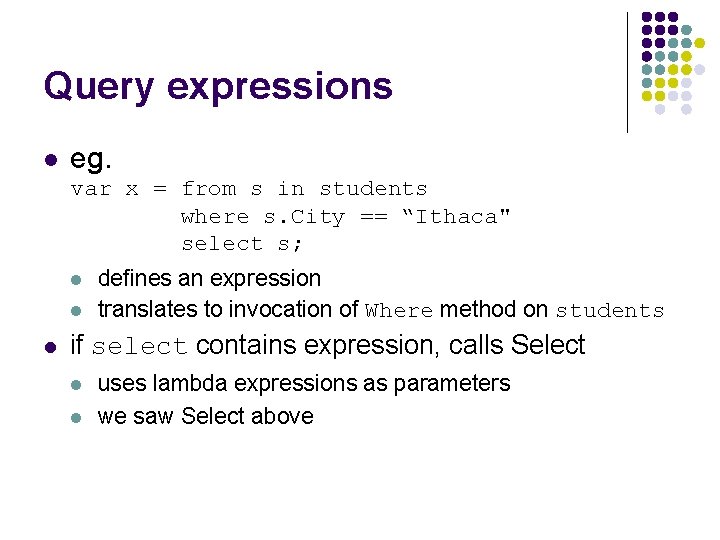
Query expressions l eg. var x = from s in students where s. City == “Ithaca" select s; l l l defines an expression translates to invocation of Where method on students if select contains expression, calls Select l l uses lambda expressions as parameters we saw Select above
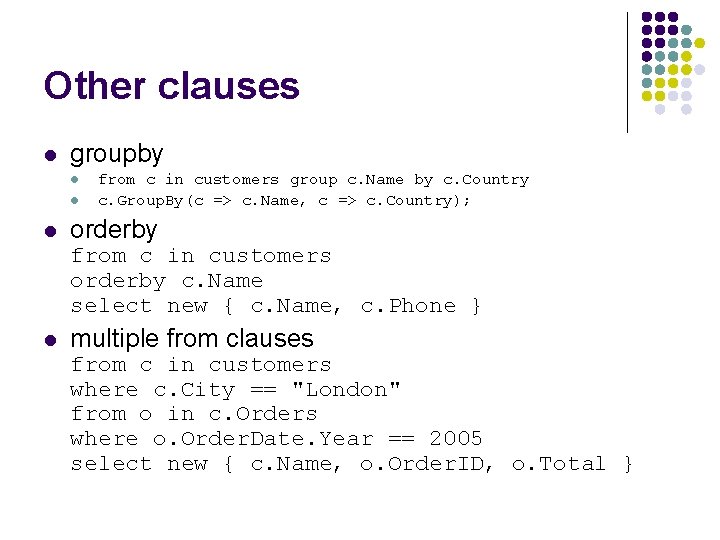
Other clauses l groupby l l l from c in customers group c. Name by c. Country c. Group. By(c => c. Name, c => c. Country); orderby from c in customers orderby c. Name select new { c. Name, c. Phone } l multiple from clauses from c in customers where c. City == "London" from o in c. Orders where o. Order. Date. Year == 2005 select new { c. Name, o. Order. ID, o. Total }
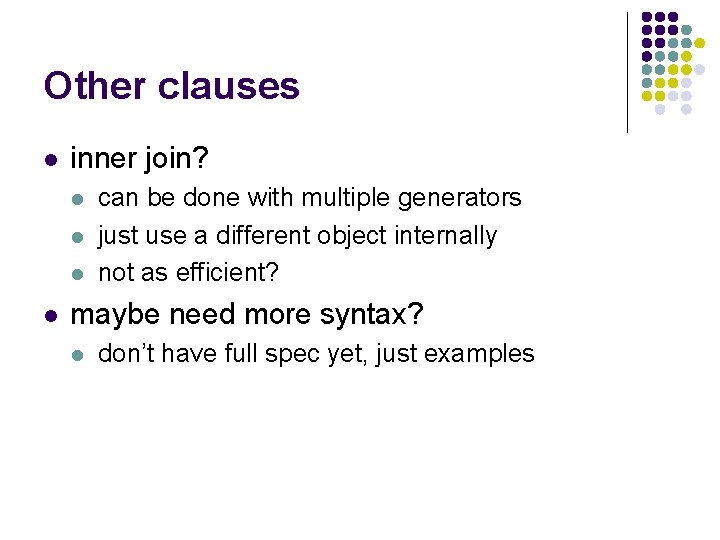
Other clauses l inner join? l l can be done with multiple generators just use a different object internally not as efficient? maybe need more syntax? l don’t have full spec yet, just examples
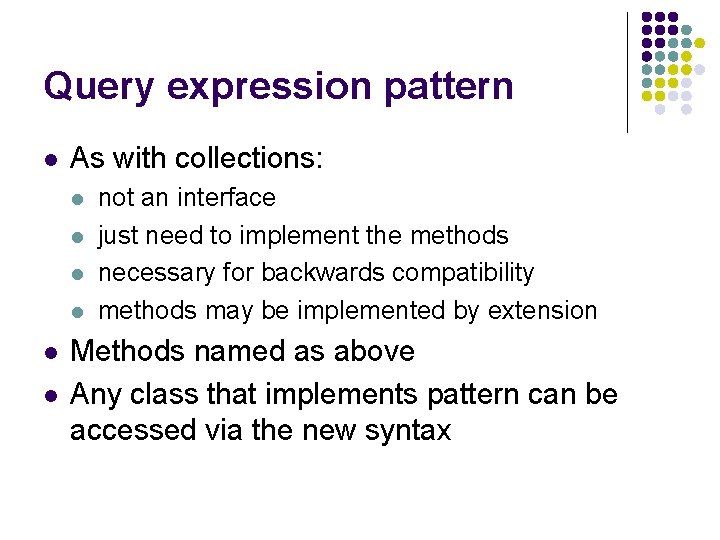
Query expression pattern l As with collections: l l l not an interface just need to implement the methods necessary for backwards compatibility methods may be implemented by extension Methods named as above Any class that implements pattern can be accessed via the new syntax
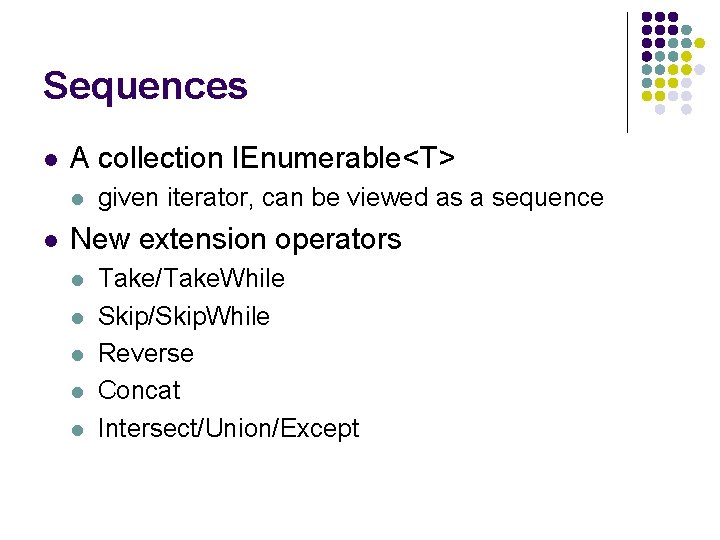
Sequences l A collection IEnumerable<T> l l given iterator, can be viewed as a sequence New extension operators l l l Take/Take. While Skip/Skip. While Reverse Concat Intersect/Union/Except
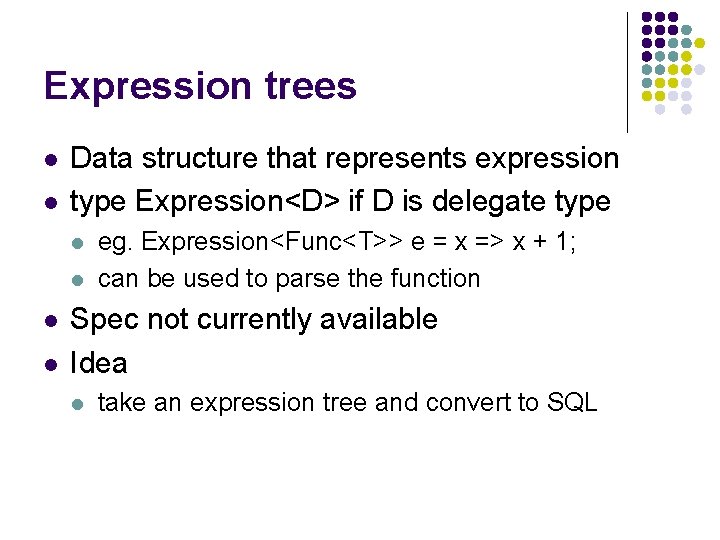
Expression trees l l Data structure that represents expression type Expression<D> if D is delegate type l l eg. Expression<Func<T>> e = x => x + 1; can be used to parse the function Spec not currently available Idea l take an expression tree and convert to SQL
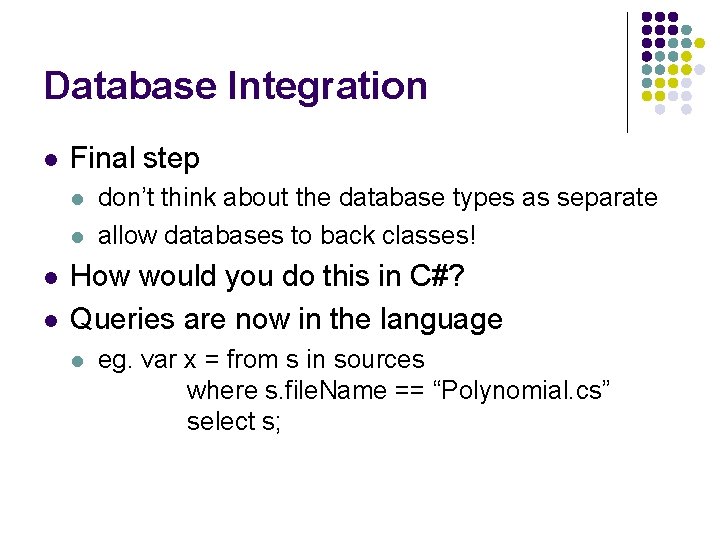
Database Integration l Final step l l don’t think about the database types as separate allow databases to back classes! How would you do this in C#? Queries are now in the language l eg. var x = from s in sources where s. file. Name == “Polynomial. cs” select s;
![DLinq Attributes l TableNameDVDTable public class DVD ColumnId true public string Title DLinq Attributes l [Table(Name="DVDTable")] public class DVD { [Column(Id = true)] public string Title;](https://slidetodoc.com/presentation_image_h2/72d1bfc3fe03d47317b96ec484ecfcc5/image-23.jpg)
DLinq Attributes l [Table(Name="DVDTable")] public class DVD { [Column(Id = true)] public string Title; [Column] public string Rating; }
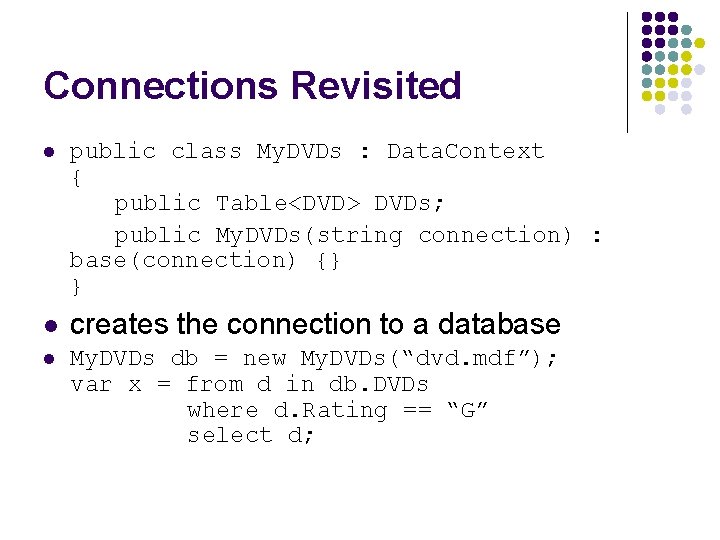
Connections Revisited l public class My. DVDs : Data. Context { public Table<DVD> DVDs; public My. DVDs(string connection) : base(connection) {} } l creates the connection to a database l My. DVDs db = new My. DVDs(“dvd. mdf”); var x = from d in db. DVDs where d. Rating == “G” select d;
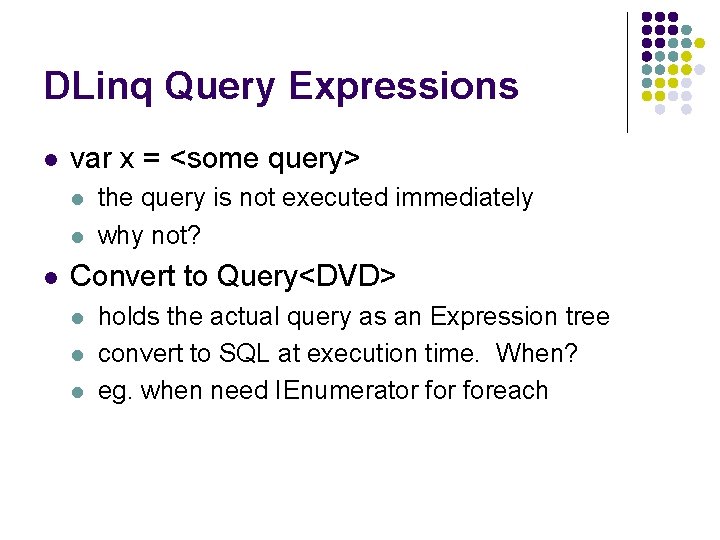
DLinq Query Expressions l var x = <some query> l l l the query is not executed immediately why not? Convert to Query<DVD> l l l holds the actual query as an Expression tree convert to SQL at execution time. When? eg. when need IEnumerator foreach
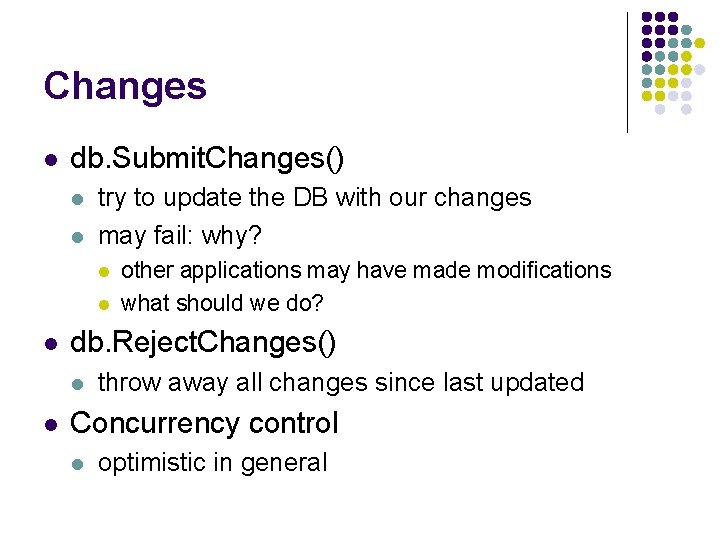
Changes l db. Submit. Changes() l l try to update the DB with our changes may fail: why? l l l db. Reject. Changes() l l other applications may have made modifications what should we do? throw away all changes since last updated Concurrency control l optimistic in general
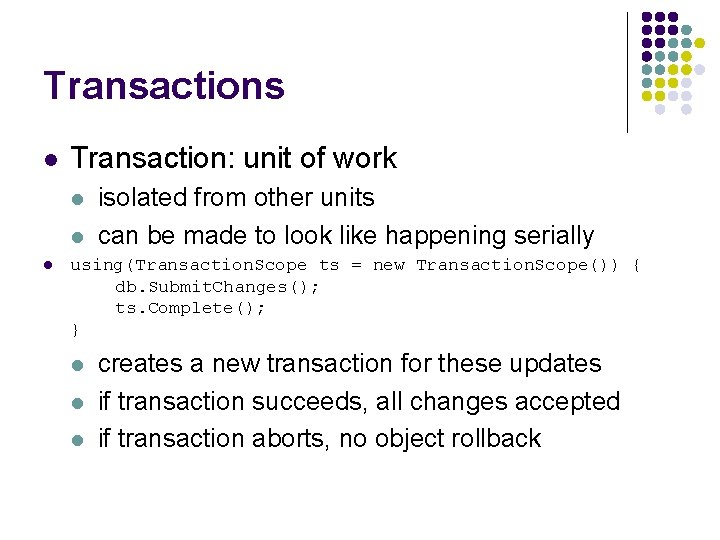
Transactions l Transaction: unit of work l l isolated from other units can be made to look like happening serially l using(Transaction. Scope ts = new Transaction. Scope()) { db. Submit. Changes(); ts. Complete(); } l l l creates a new transaction for these updates if transaction succeeds, all changes accepted if transaction aborts, no object rollback
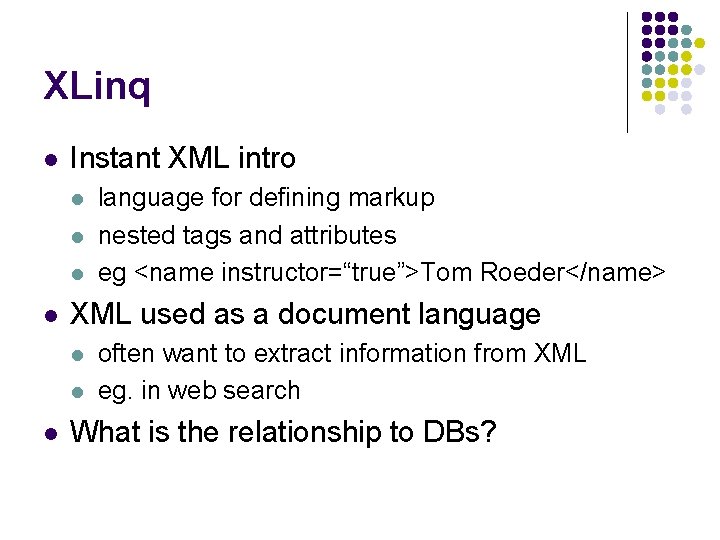
XLinq l Instant XML intro l l XML used as a document language l language for defining markup nested tags and attributes eg <name instructor=“true”>Tom Roeder</name> often want to extract information from XML eg. in web search What is the relationship to DBs?
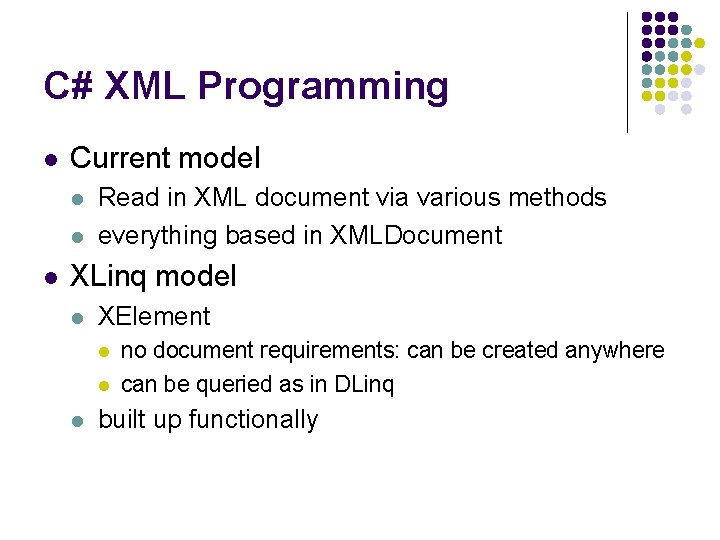
C# XML Programming l Current model l Read in XML document via various methods everything based in XMLDocument XLinq model l XElement l l l no document requirements: can be created anywhere can be queried as in DLinq built up functionally
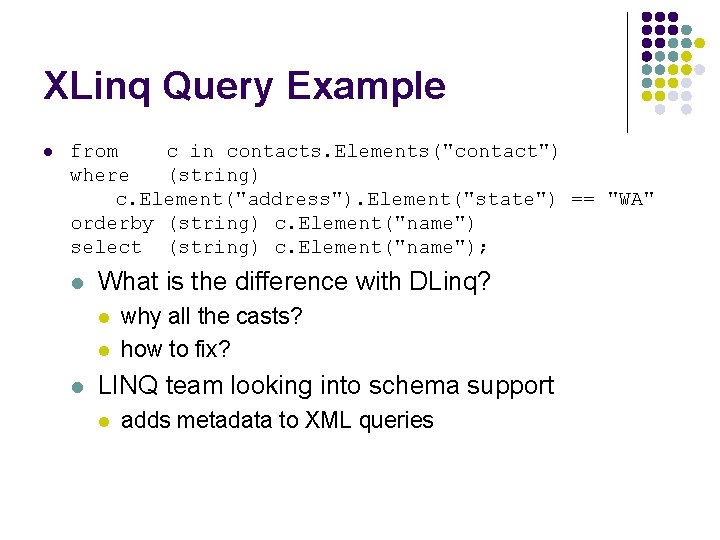
XLinq Query Example l from c in contacts. Elements("contact") where (string) c. Element("address"). Element("state") == "WA" orderby (string) c. Element("name") select (string) c. Element("name"); l What is the difference with DLinq? l l l why all the casts? how to fix? LINQ team looking into schema support l adds metadata to XML queries
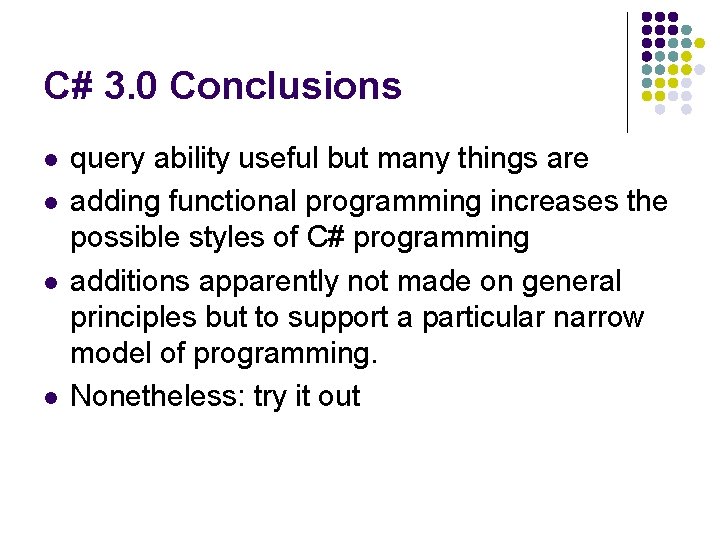
C# 3. 0 Conclusions l l query ability useful but many things are adding functional programming increases the possible styles of C# programming additions apparently not made on general principles but to support a particular narrow model of programming. Nonetheless: try it out
Go 910
Symbolism in the devil and tom walker
Computer arithmetic
Definition work physics
Iso/tc 215
Completed ics 215 form
Guggisberg 10 year development plan
Eecs 478
Dtu-215
239 rounded to the nearest ten
Servsoc/inicio.aspx
Ley 40/215
Tanget ratio
Po box 1220 philadelphia pa 19105 provider phone number
The formula c=5p+215 relates c
Stc 215/1994
Sentenza n. 215/87 della corte costituzionale
Economic 215
Nom 030 stps 2006
Peyton manning cleft lip
Boardworks ltd 2006
Decreto 2323 de 2006
Caltrans standard specifications 2006
Sentencia c-355 de 2006
School year 2005
Lee 2006
Jane eyre cast
Tata persuratan kemenag
Ego pharma birthday 10th february 2006
Geogrphic
2006
King of fighters 2006