Reflection Conversions and Exceptions Tom Roeder CS 215
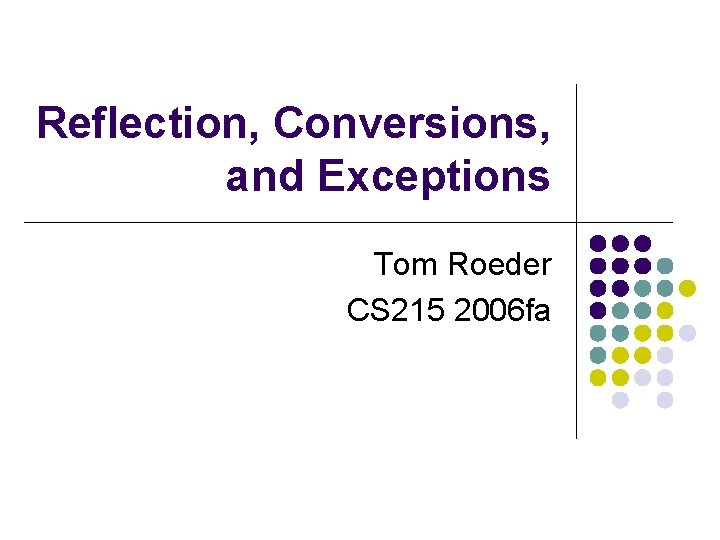
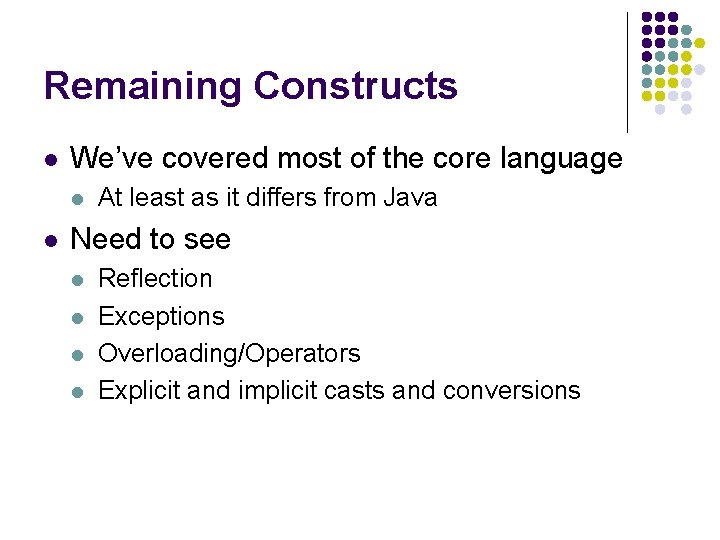
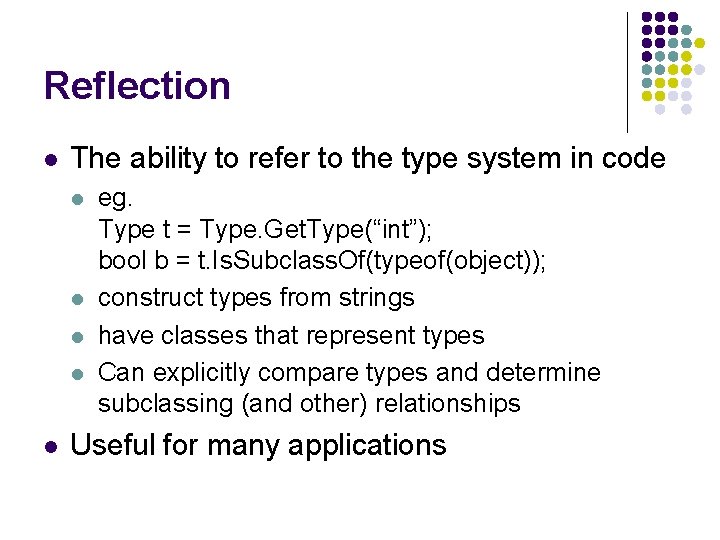
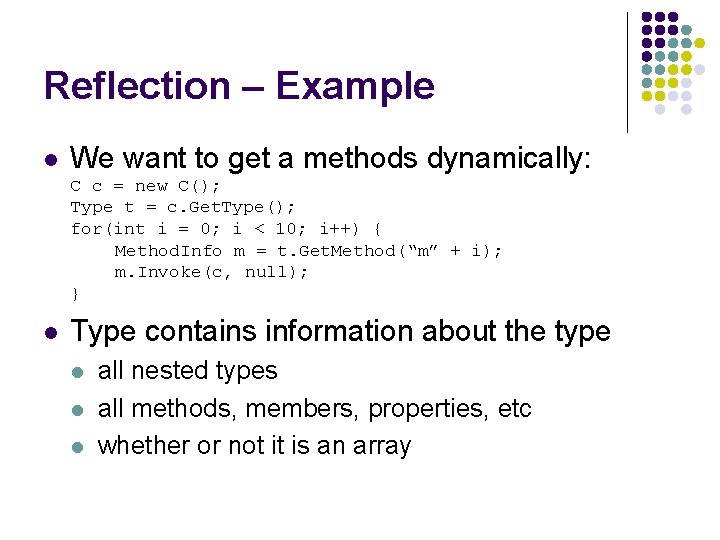
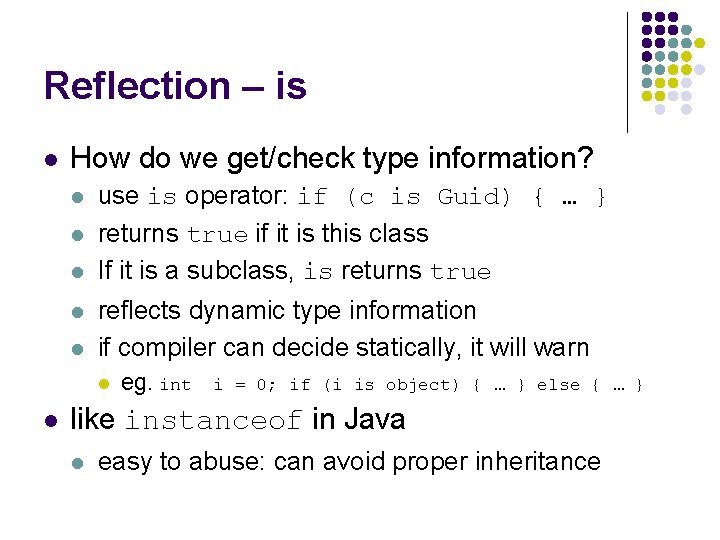
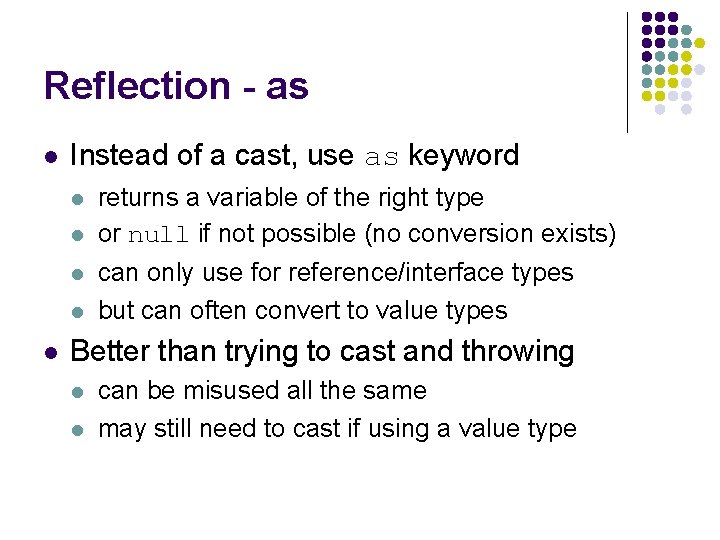
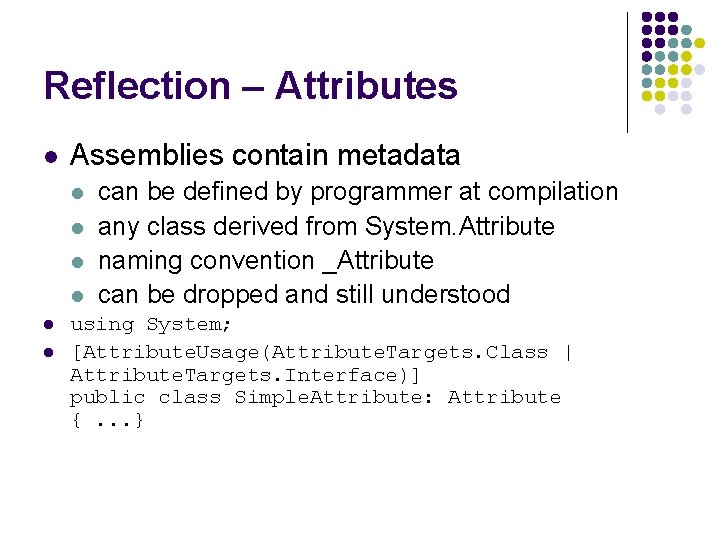
![Reflection – Attributes l [Simple] class Class 1 {. . . } [Simple] interface Reflection – Attributes l [Simple] class Class 1 {. . . } [Simple] interface](https://slidetodoc.com/presentation_image_h2/9aff4dfbda3c43c3f05eaeb91328e026/image-8.jpg)
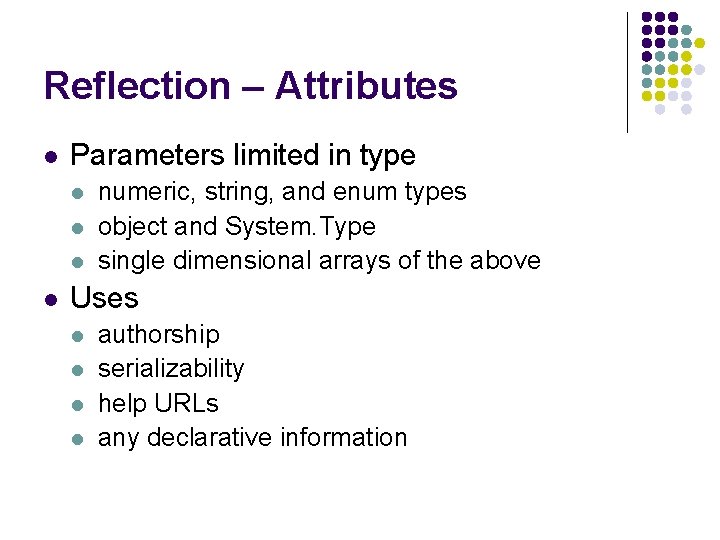
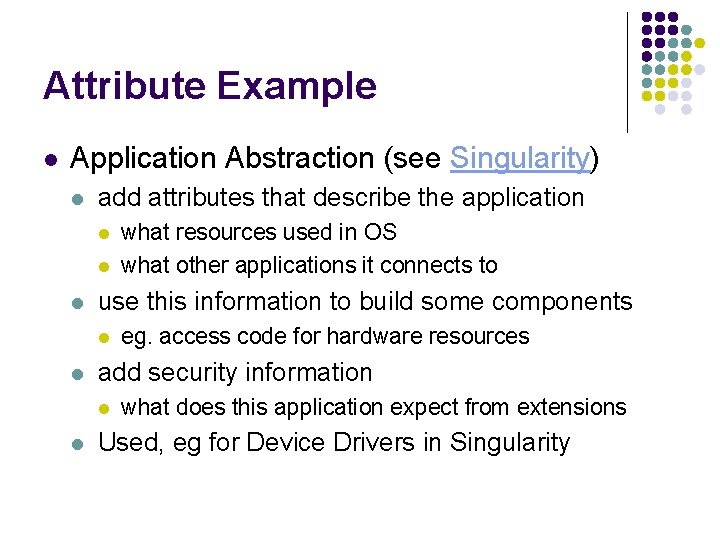
![Attribute Example [Driver. Category] [Signature("/pci/03/00/5333/8811")] class S 3 Trio. Config: Driver. Category. Declaration { Attribute Example [Driver. Category] [Signature("/pci/03/00/5333/8811")] class S 3 Trio. Config: Driver. Category. Declaration {](https://slidetodoc.com/presentation_image_h2/9aff4dfbda3c43c3f05eaeb91328e026/image-11.jpg)
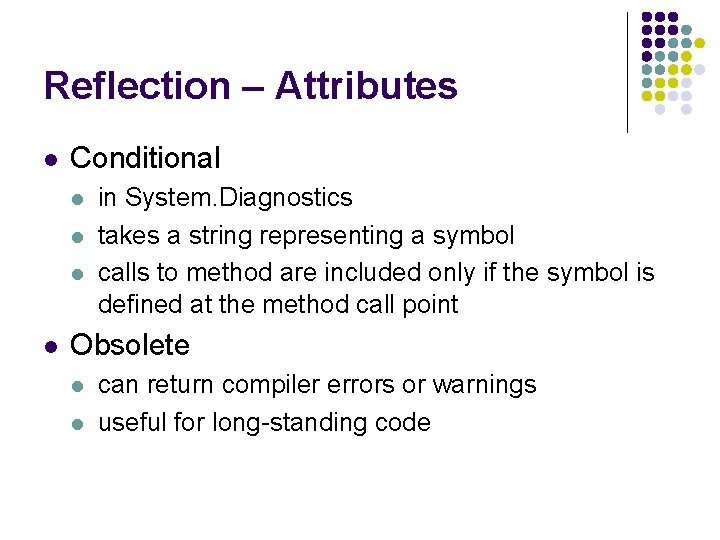
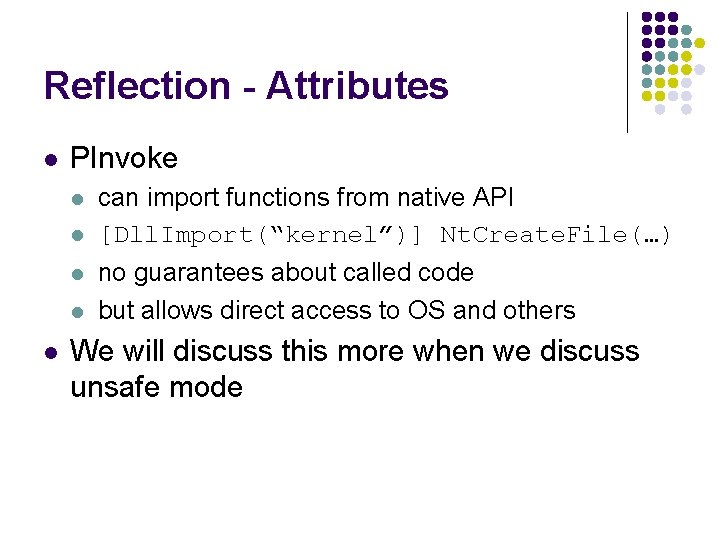
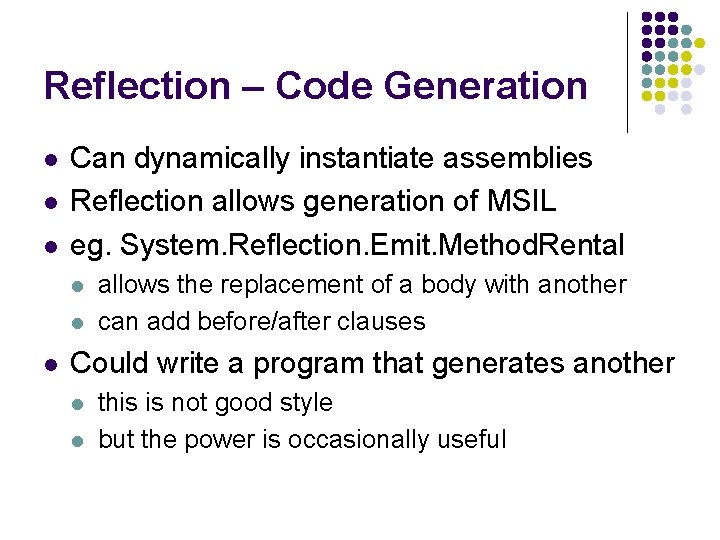
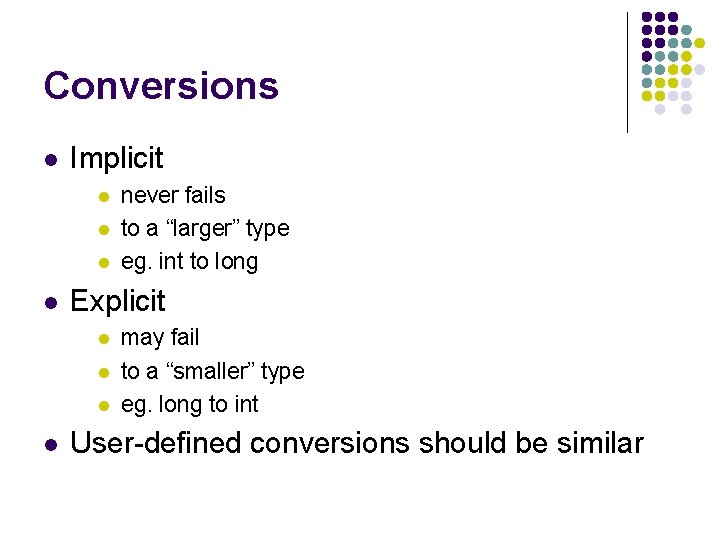
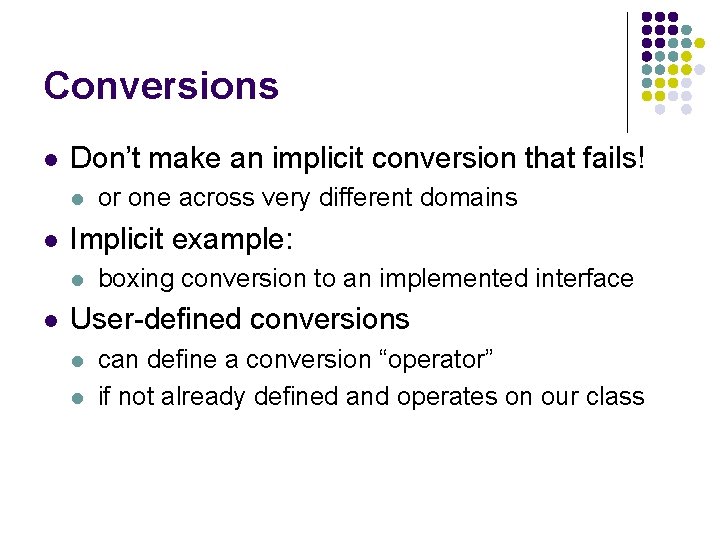
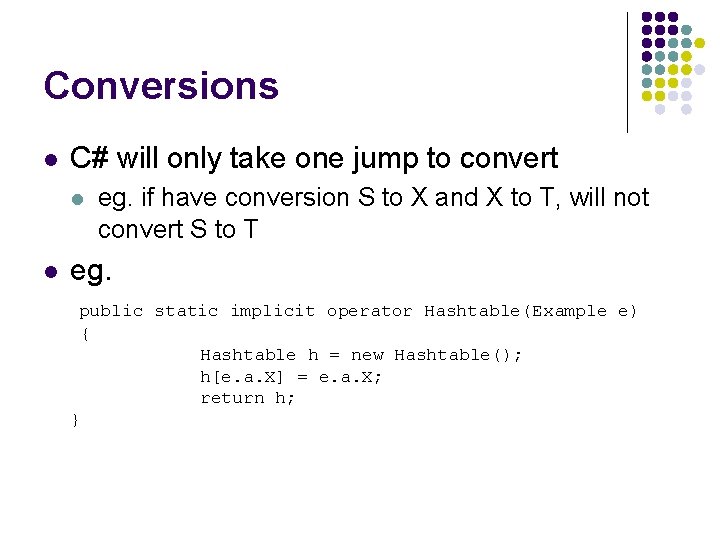
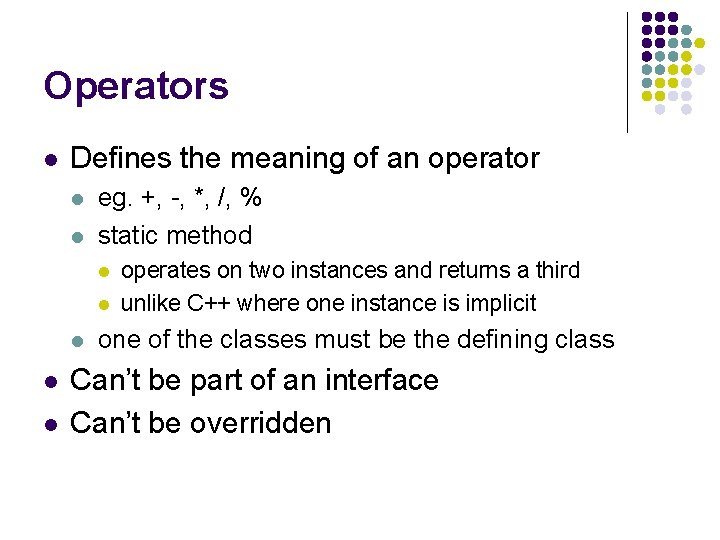
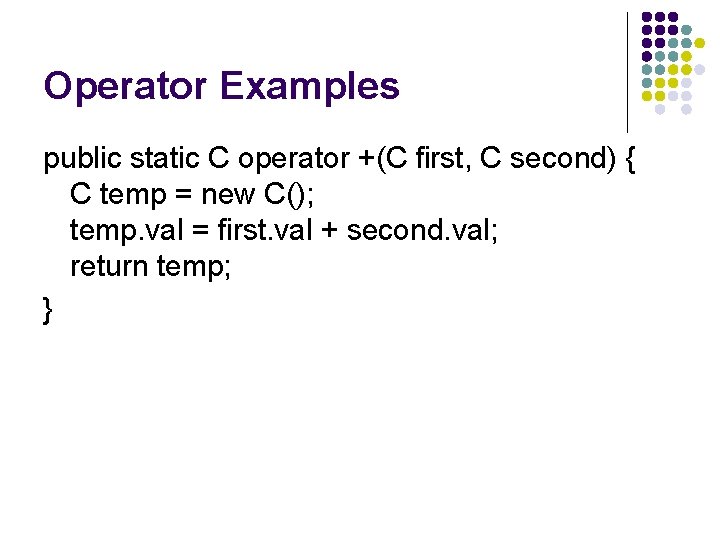
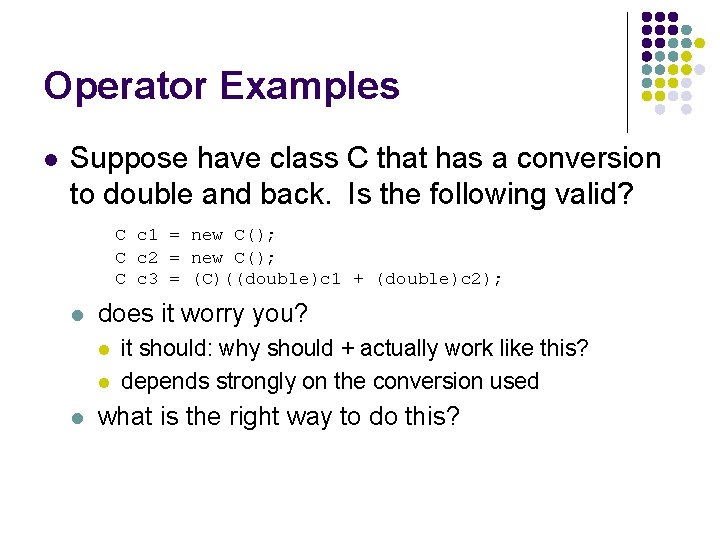
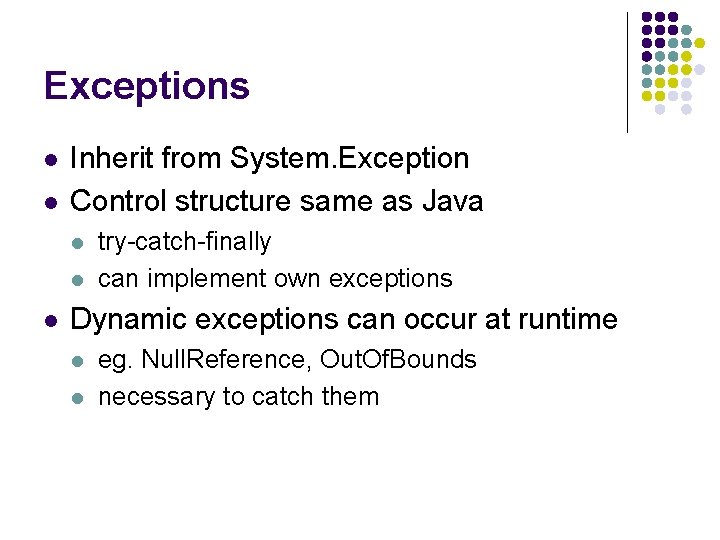
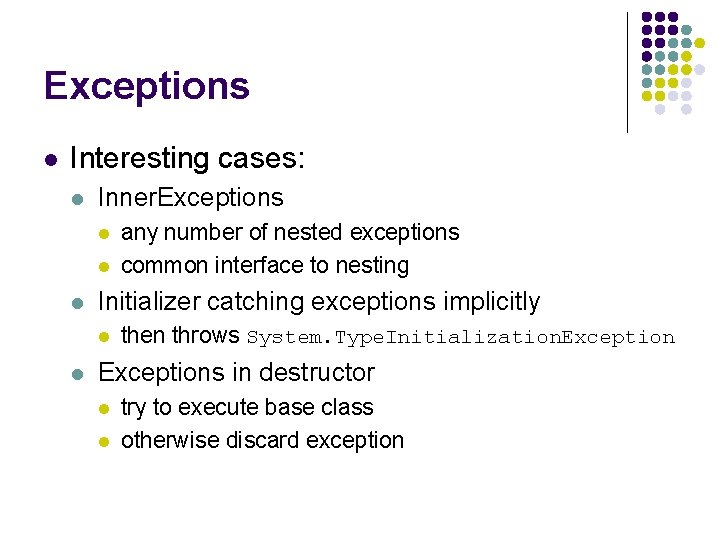
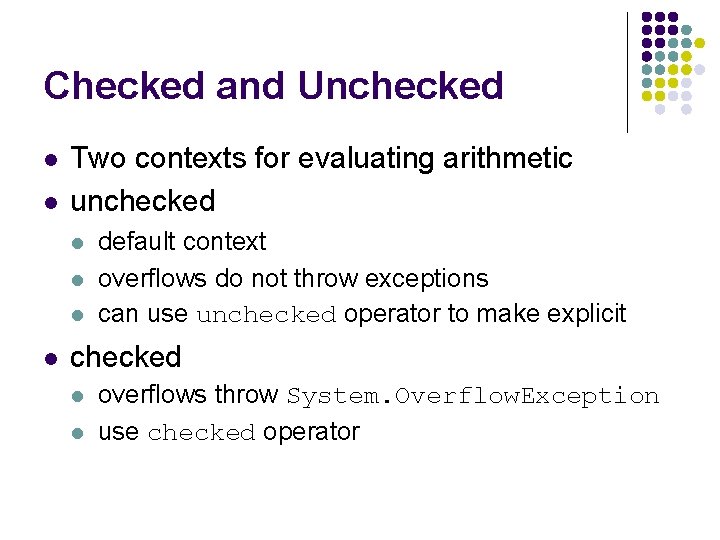
- Slides: 23
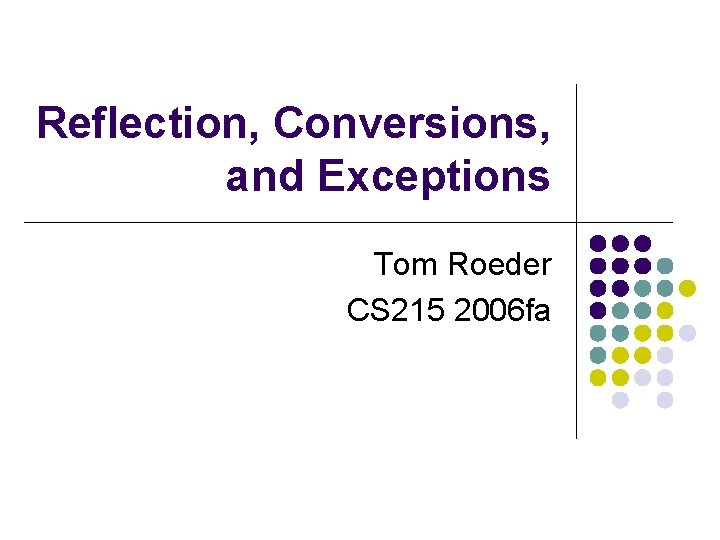
Reflection, Conversions, and Exceptions Tom Roeder CS 215 2006 fa
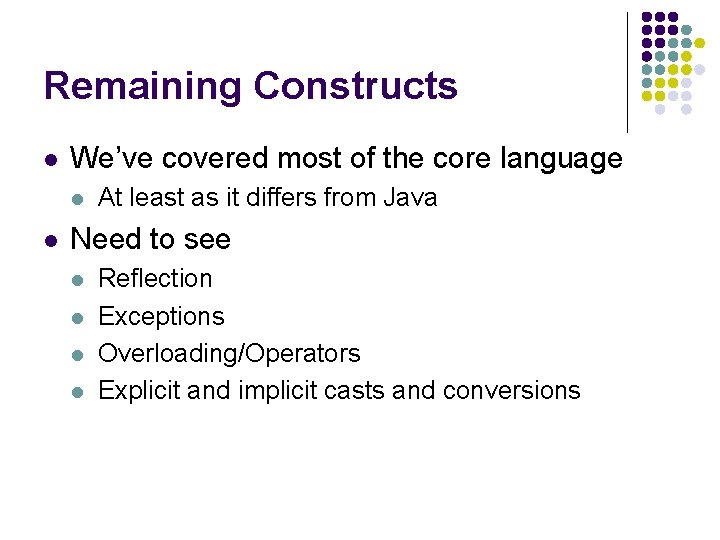
Remaining Constructs l We’ve covered most of the core language l l At least as it differs from Java Need to see l l Reflection Exceptions Overloading/Operators Explicit and implicit casts and conversions
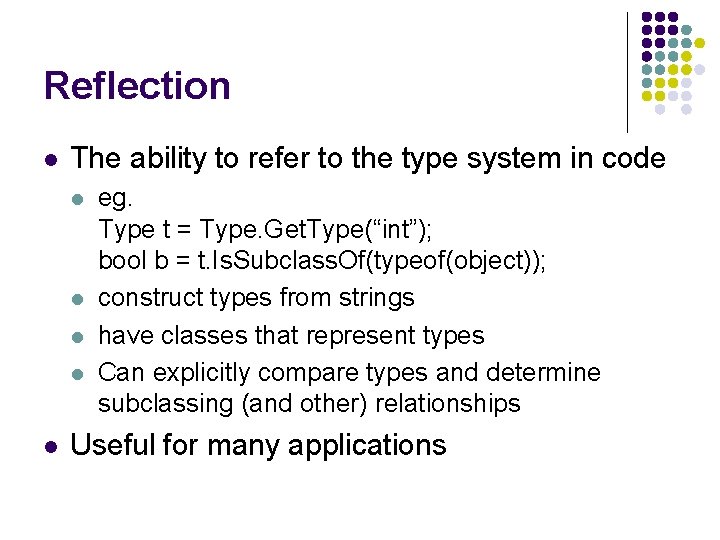
Reflection l The ability to refer to the type system in code l l l eg. Type t = Type. Get. Type(“int”); bool b = t. Is. Subclass. Of(typeof(object)); construct types from strings have classes that represent types Can explicitly compare types and determine subclassing (and other) relationships Useful for many applications
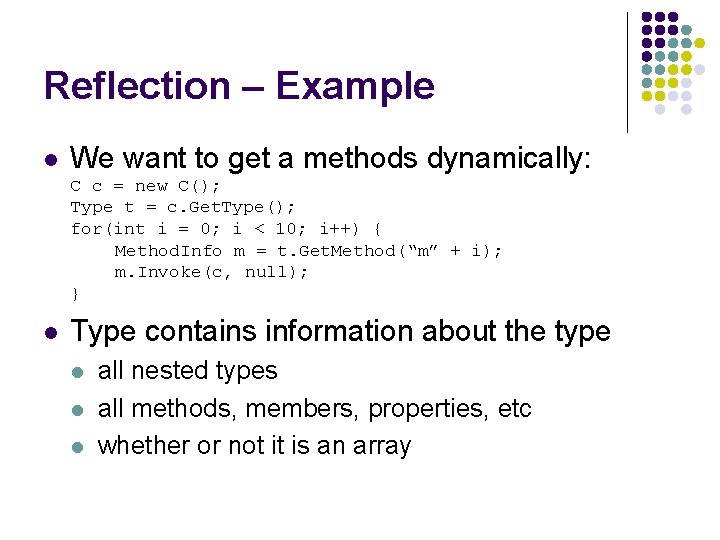
Reflection – Example l We want to get a methods dynamically: C c = new C(); Type t = c. Get. Type(); for(int i = 0; i < 10; i++) { Method. Info m = t. Get. Method(“m” + i); m. Invoke(c, null); } l Type contains information about the type l l l all nested types all methods, members, properties, etc whether or not it is an array
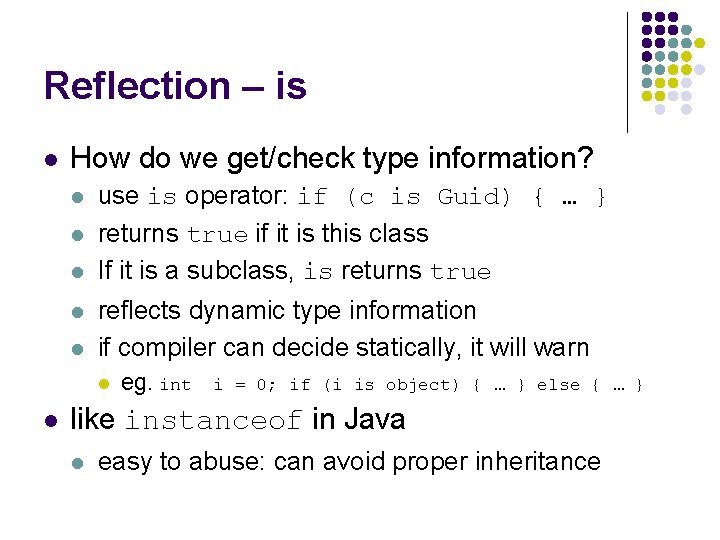
Reflection – is l How do we get/check type information? l l l use is operator: if (c is Guid) { … } returns true if it is this class If it is a subclass, is returns true reflects dynamic type information if compiler can decide statically, it will warn l l eg. int i = 0; if (i is object) { … } else { … } like instanceof in Java l easy to abuse: can avoid proper inheritance
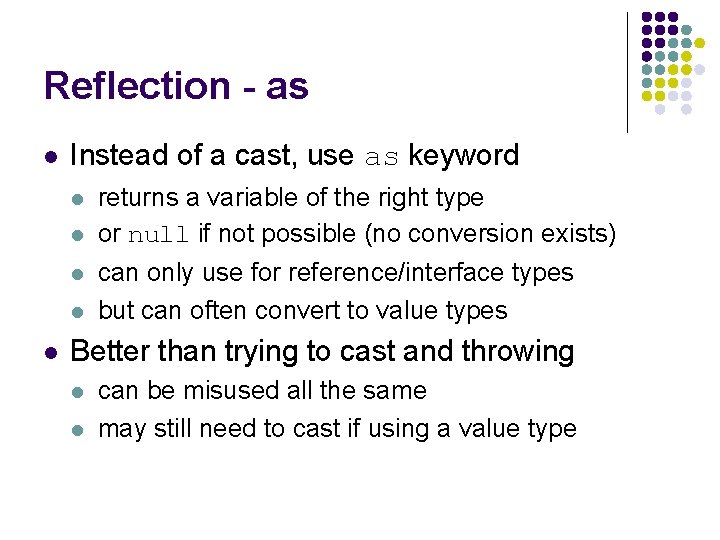
Reflection - as l Instead of a cast, use as keyword l l l returns a variable of the right type or null if not possible (no conversion exists) can only use for reference/interface types but can often convert to value types Better than trying to cast and throwing l l can be misused all the same may still need to cast if using a value type
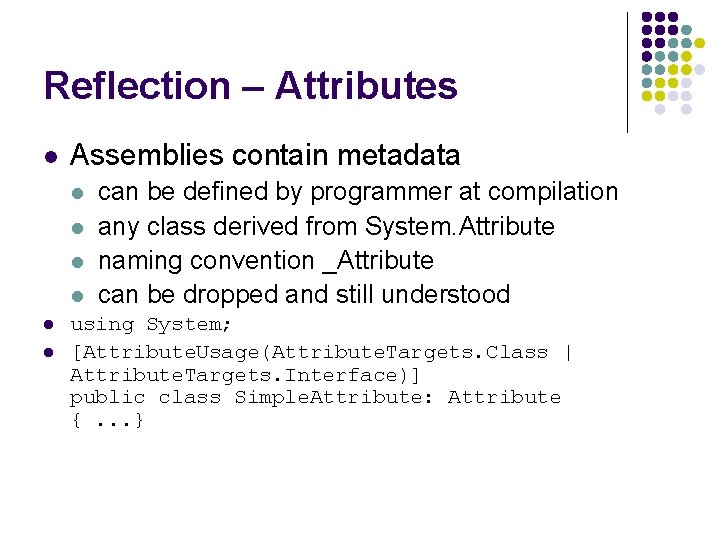
Reflection – Attributes l Assemblies contain metadata l l l can be defined by programmer at compilation any class derived from System. Attribute naming convention _Attribute can be dropped and still understood using System; [Attribute. Usage(Attribute. Targets. Class | Attribute. Targets. Interface)] public class Simple. Attribute: Attribute {. . . }
![Reflection Attributes l Simple class Class 1 Simple interface Reflection – Attributes l [Simple] class Class 1 {. . . } [Simple] interface](https://slidetodoc.com/presentation_image_h2/9aff4dfbda3c43c3f05eaeb91328e026/image-8.jpg)
Reflection – Attributes l [Simple] class Class 1 {. . . } [Simple] interface Interface 1 {. . . } l Multi-use or single-use attributes l l l depends on Allow. Multiple Positional and named parameters l l constructors define positional parameters non-static public RW fields define named
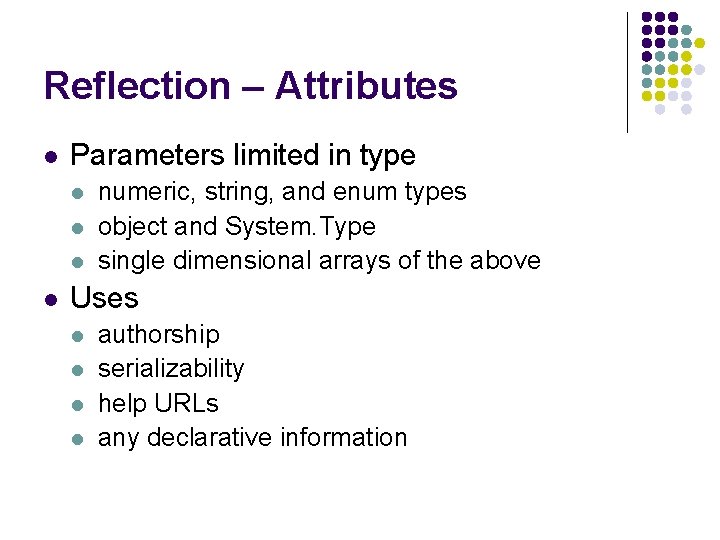
Reflection – Attributes l Parameters limited in type l l numeric, string, and enum types object and System. Type single dimensional arrays of the above Uses l l authorship serializability help URLs any declarative information
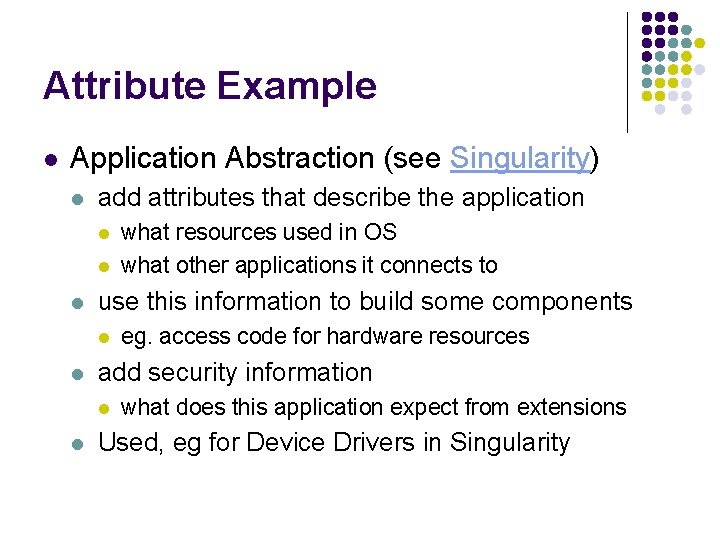
Attribute Example l Application Abstraction (see Singularity) l add attributes that describe the application l l l use this information to build some components l l eg. access code for hardware resources add security information l l what resources used in OS what other applications it connects to what does this application expect from extensions Used, eg for Device Drivers in Singularity
![Attribute Example Driver Category Signaturepci030053338811 class S 3 Trio Config Driver Category Declaration Attribute Example [Driver. Category] [Signature("/pci/03/00/5333/8811")] class S 3 Trio. Config: Driver. Category. Declaration {](https://slidetodoc.com/presentation_image_h2/9aff4dfbda3c43c3f05eaeb91328e026/image-11.jpg)
Attribute Example [Driver. Category] [Signature("/pci/03/00/5333/8811")] class S 3 Trio. Config: Driver. Category. Declaration { // Hardware resources from PCI config [Io. Memory. Range(0, Default = 0 xf 8000000, Length = 0 x 400000)] internal readonly Io. Memory. Range frame. Buffer; // Fixed hardware resources [Io. Fixed. Memory. Range(Base = 0 xb 8000, Length = 0 x 8000)] internal readonly Io. Memory. Range text. Buffer;
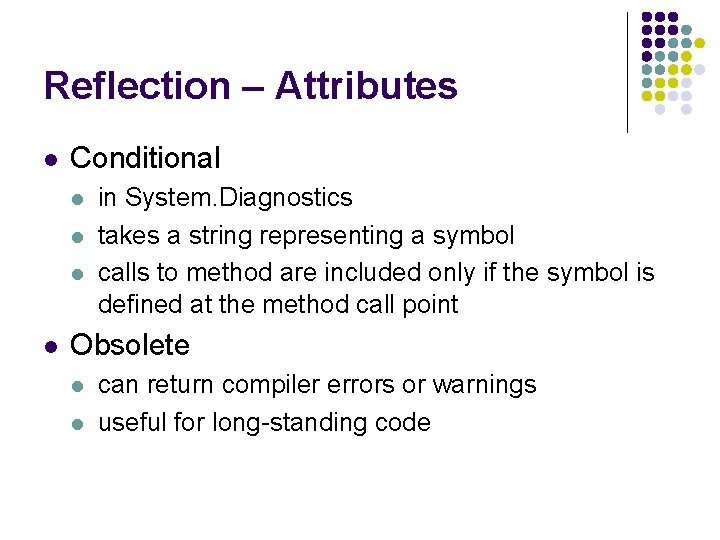
Reflection – Attributes l Conditional l l in System. Diagnostics takes a string representing a symbol calls to method are included only if the symbol is defined at the method call point Obsolete l l can return compiler errors or warnings useful for long-standing code
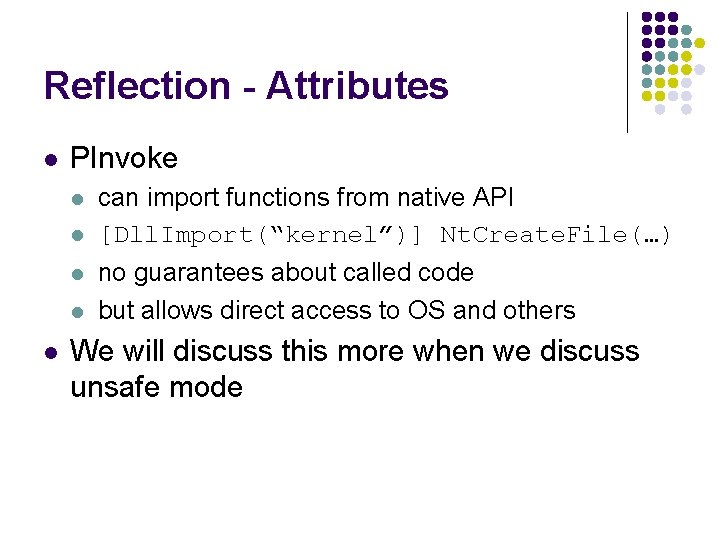
Reflection - Attributes l PInvoke l l l can import functions from native API [Dll. Import(“kernel”)] Nt. Create. File(…) no guarantees about called code but allows direct access to OS and others We will discuss this more when we discuss unsafe mode
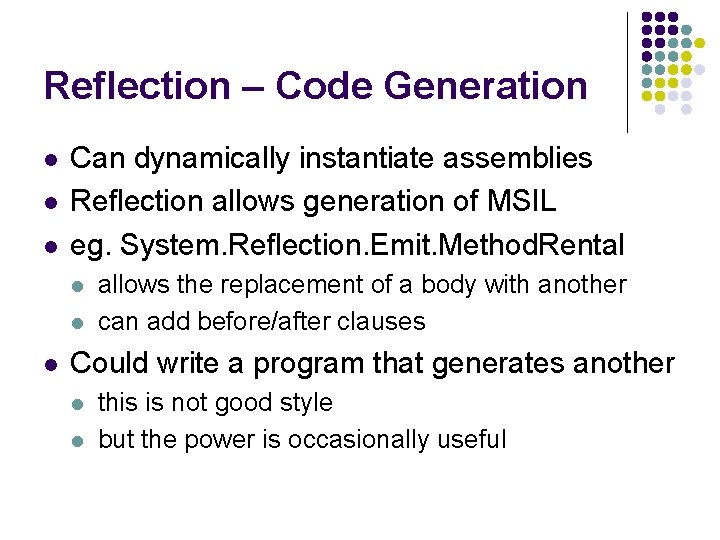
Reflection – Code Generation l l l Can dynamically instantiate assemblies Reflection allows generation of MSIL eg. System. Reflection. Emit. Method. Rental l allows the replacement of a body with another can add before/after clauses Could write a program that generates another l l this is not good style but the power is occasionally useful
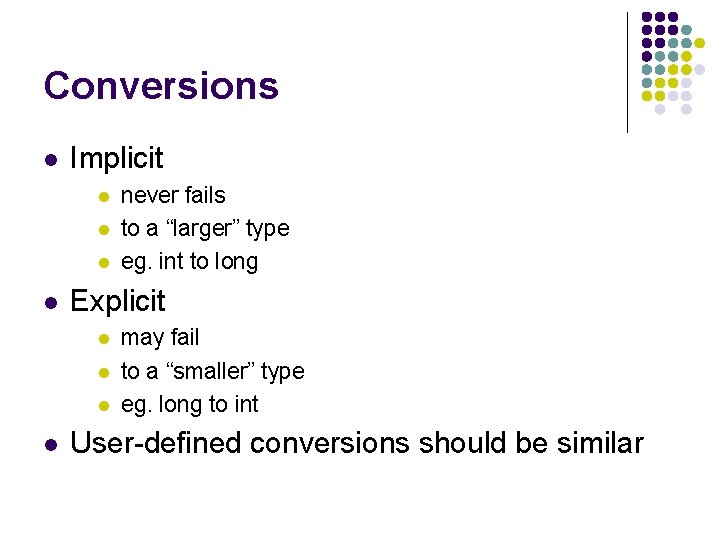
Conversions l Implicit l l Explicit l l never fails to a “larger” type eg. int to long may fail to a “smaller” type eg. long to int User-defined conversions should be similar
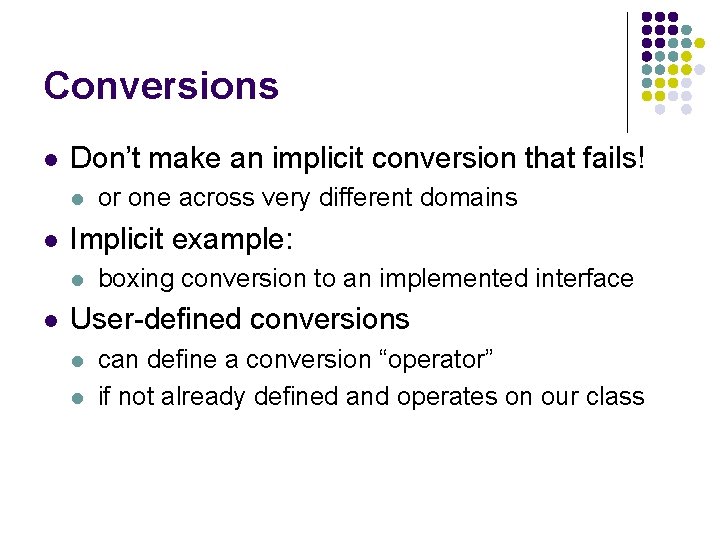
Conversions l Don’t make an implicit conversion that fails! l l Implicit example: l l or one across very different domains boxing conversion to an implemented interface User-defined conversions l l can define a conversion “operator” if not already defined and operates on our class
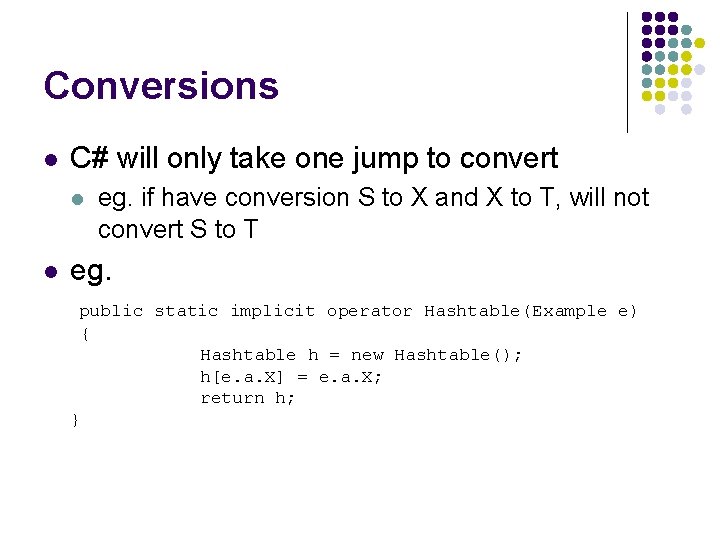
Conversions l C# will only take one jump to convert l l eg. if have conversion S to X and X to T, will not convert S to T eg. public static implicit operator Hashtable(Example e) { Hashtable h = new Hashtable(); h[e. a. X] = e. a. X; return h; }
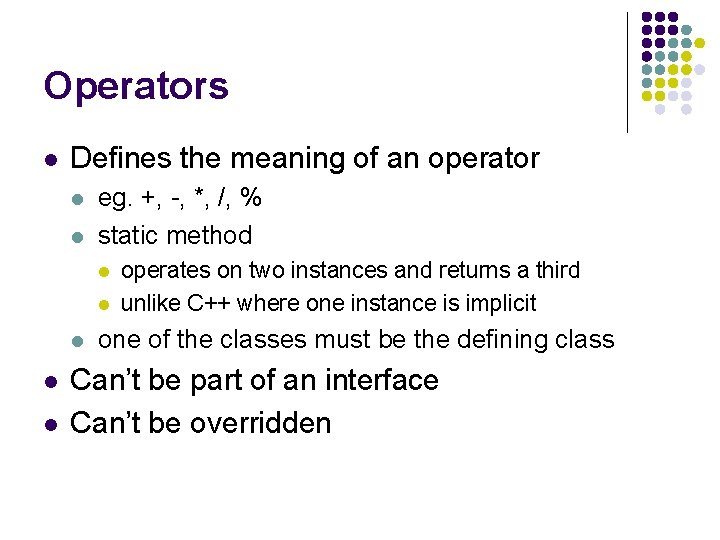
Operators l Defines the meaning of an operator l l eg. +, -, *, /, % static method l l l operates on two instances and returns a third unlike C++ where one instance is implicit one of the classes must be the defining class Can’t be part of an interface Can’t be overridden
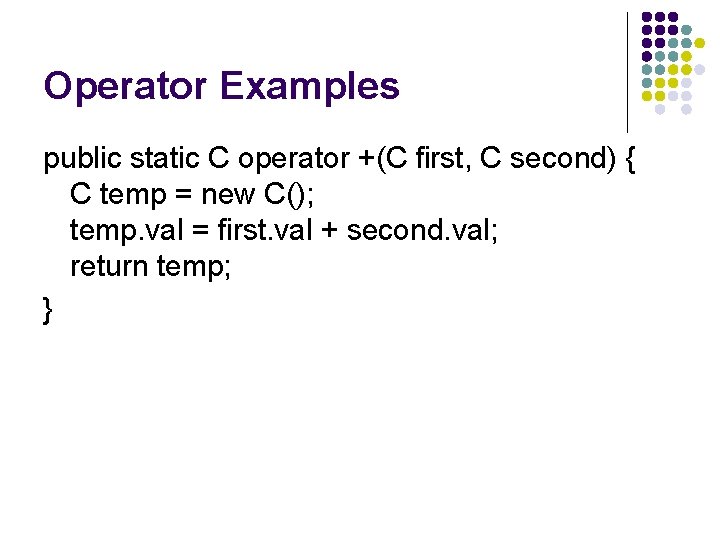
Operator Examples public static C operator +(C first, C second) { C temp = new C(); temp. val = first. val + second. val; return temp; }
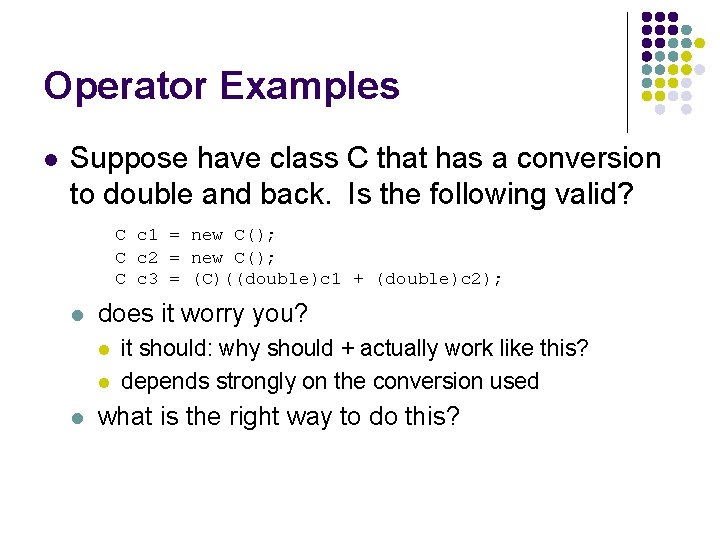
Operator Examples l Suppose have class C that has a conversion to double and back. Is the following valid? C c 1 = new C(); C c 2 = new C(); C c 3 = (C)((double)c 1 + (double)c 2); l does it worry you? l l l it should: why should + actually work like this? depends strongly on the conversion used what is the right way to do this?
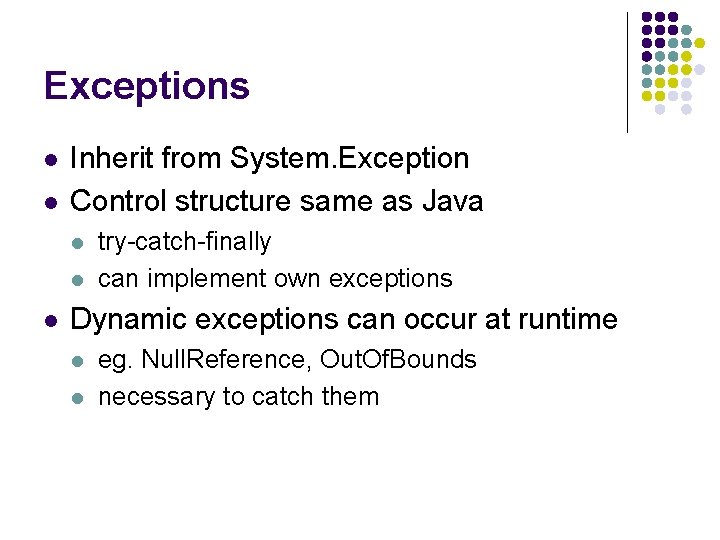
Exceptions l l Inherit from System. Exception Control structure same as Java l l l try-catch-finally can implement own exceptions Dynamic exceptions can occur at runtime l l eg. Null. Reference, Out. Of. Bounds necessary to catch them
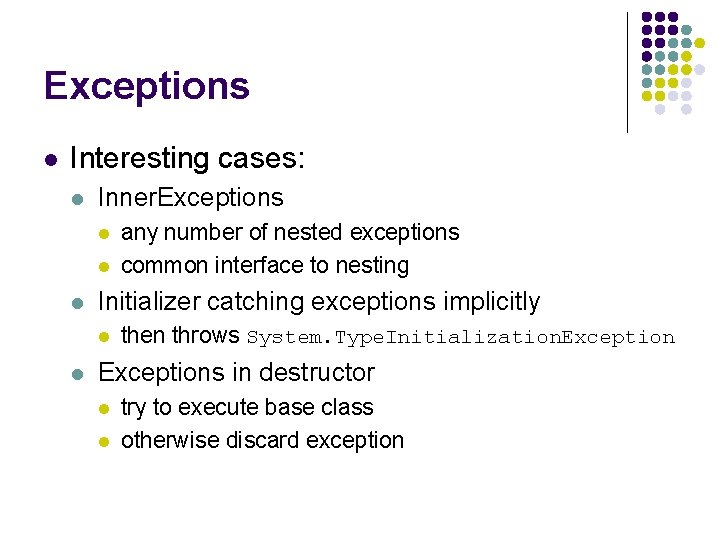
Exceptions l Interesting cases: l Inner. Exceptions l l l Initializer catching exceptions implicitly l l any number of nested exceptions common interface to nesting then throws System. Type. Initialization. Exceptions in destructor l l try to execute base class otherwise discard exception
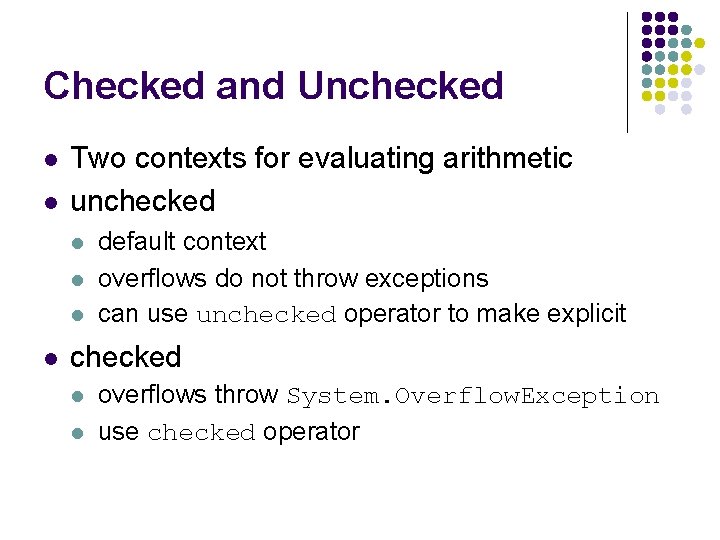
Checked and Unchecked l l Two contexts for evaluating arithmetic unchecked l l default context overflows do not throw exceptions can use unchecked operator to make explicit checked l l overflows throw System. Overflow. Exception use checked operator
Symbols in the devil and tom walker
Diverging lens negative focal length
Go 910
Sentenza n. 215/87 della corte costituzionale
Completed ics 215 form
Http //servsoc/inicio.aspx
Health partners hp connect
10000000/215
Eecs 215 umich
Ley 40/215
The formula c=5p+215 relates c
What is work in physics
Dtu-215
What is the ratio for tangent
Economic 215
Economic 215
Stc 215/1994
Tc 215
Round 703 to the nearest hundred
Manycomparative and superlative
Predefined and non predefined exceptions are raised
Comparative and superlative for badly
Comparatives and superlatives exceptions
Spl exceptions