Arrays Chapter 8 Arrays Hold Multiple Values n
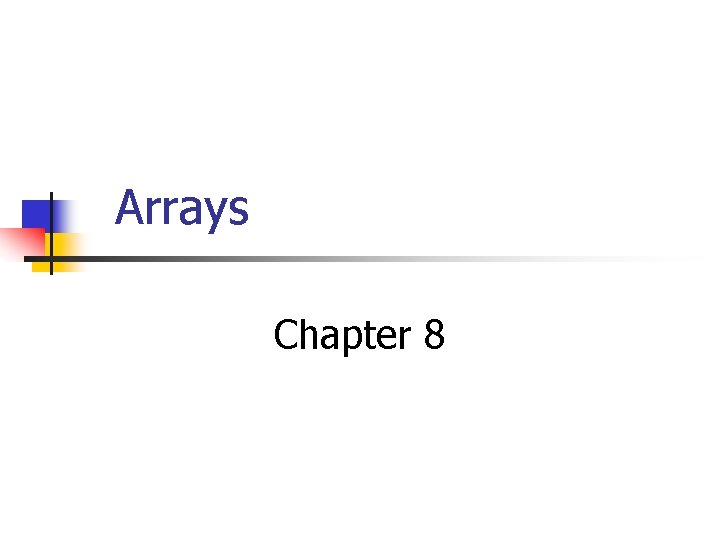
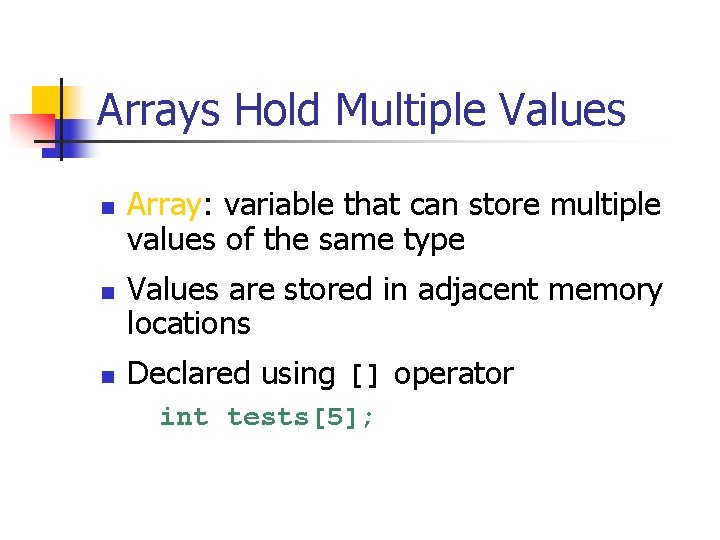
![Array Storage in Memory n The definition int tests[5]; allocates the following memory first Array Storage in Memory n The definition int tests[5]; allocates the following memory first](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-3.jpg)
![Array Terminology In the definition int tests[5]; n int is the data type of Array Terminology In the definition int tests[5]; n int is the data type of](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-4.jpg)
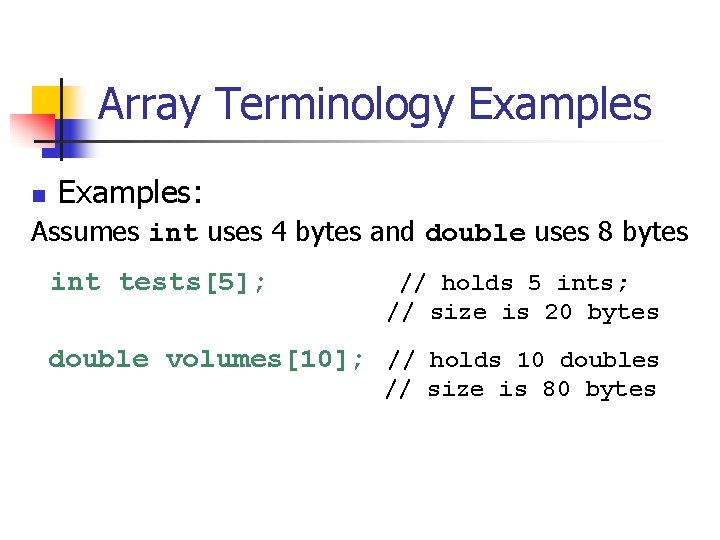
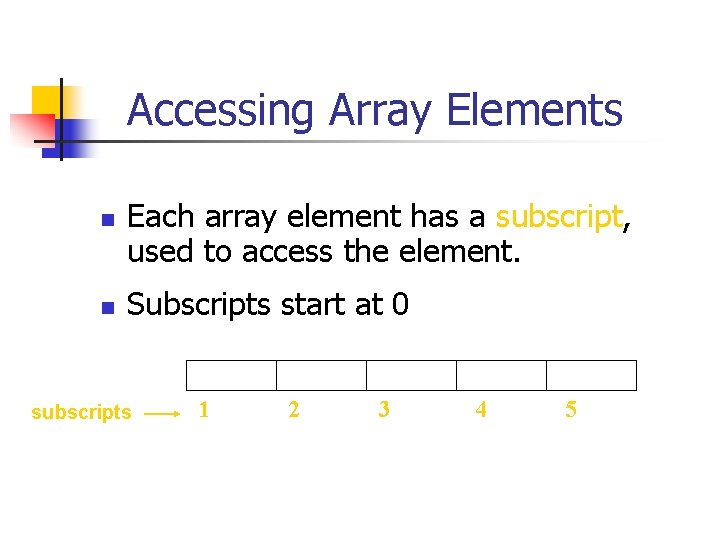
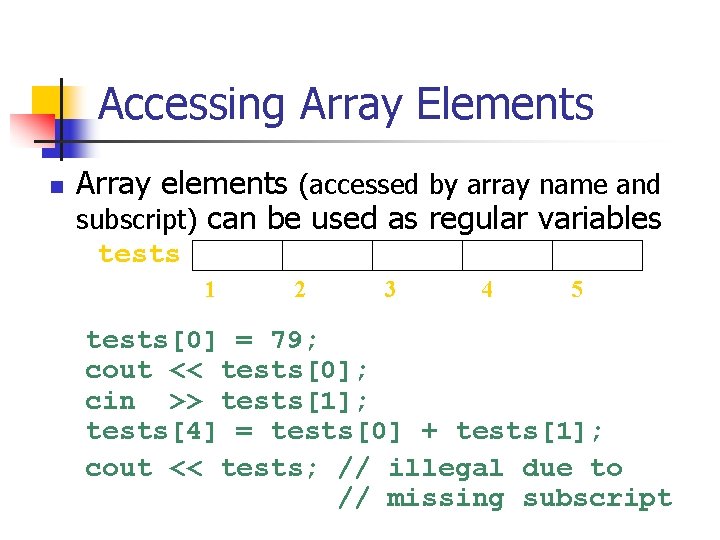
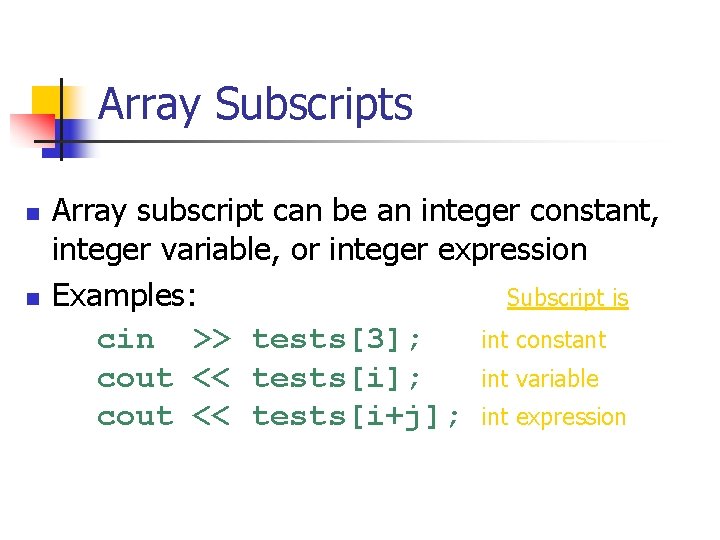
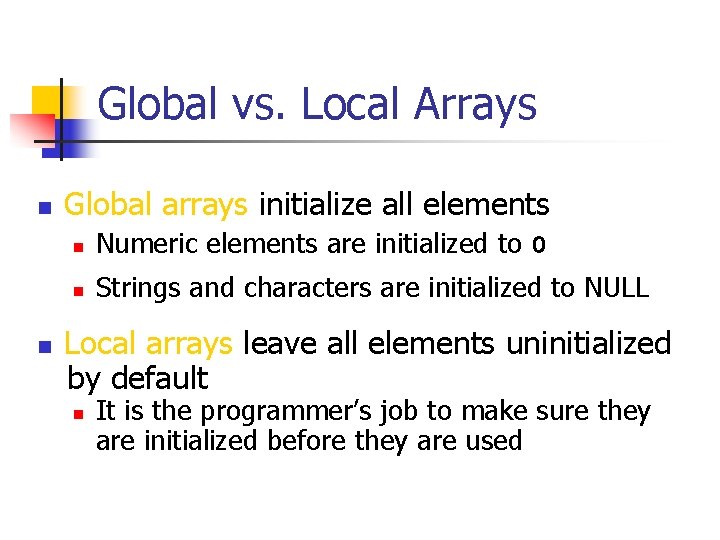
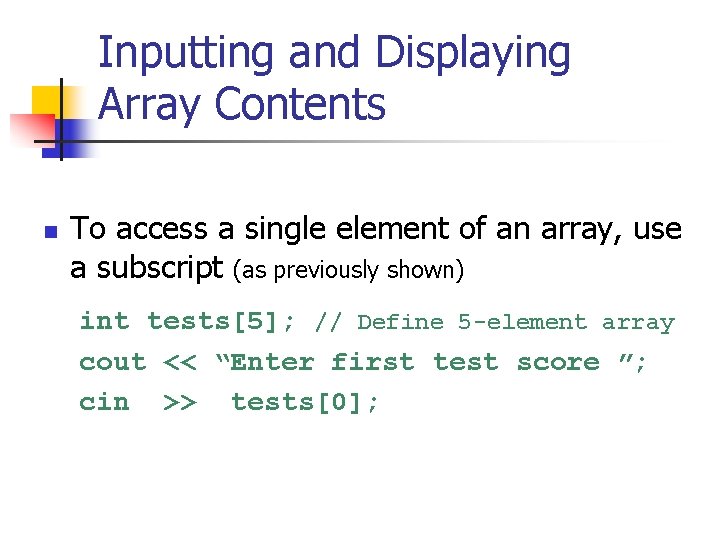
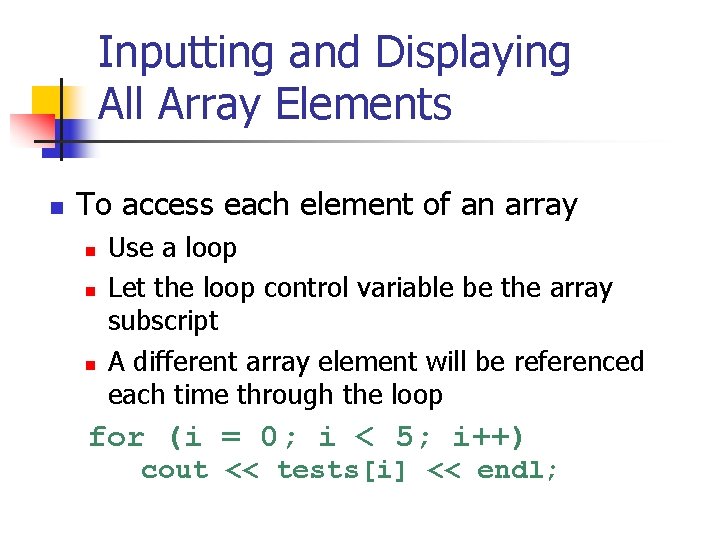
![Getting Array Data from a File int sales[5]; ifstream datafile; datafile. open("sales. dat"); if Getting Array Data from a File int sales[5]; ifstream datafile; datafile. open("sales. dat"); if](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-12.jpg)
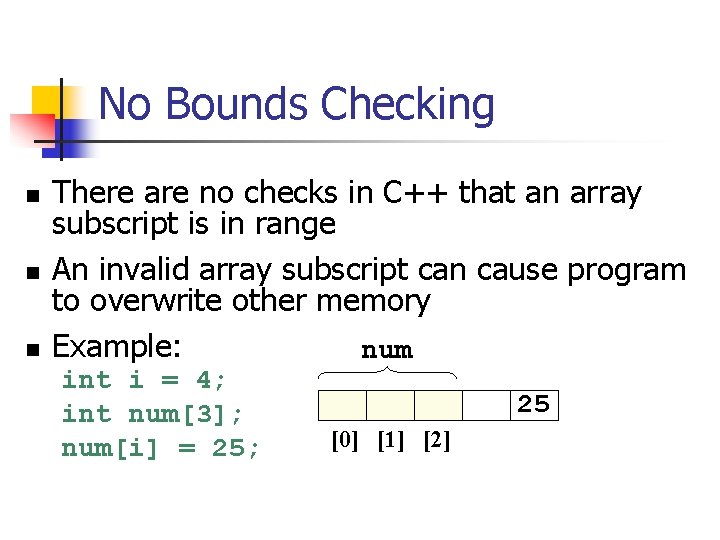
![Array Initialization n Can be initialized during program execution with assignment statements tests[0] = Array Initialization n Can be initialized during program execution with assignment statements tests[0] =](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-14.jpg)
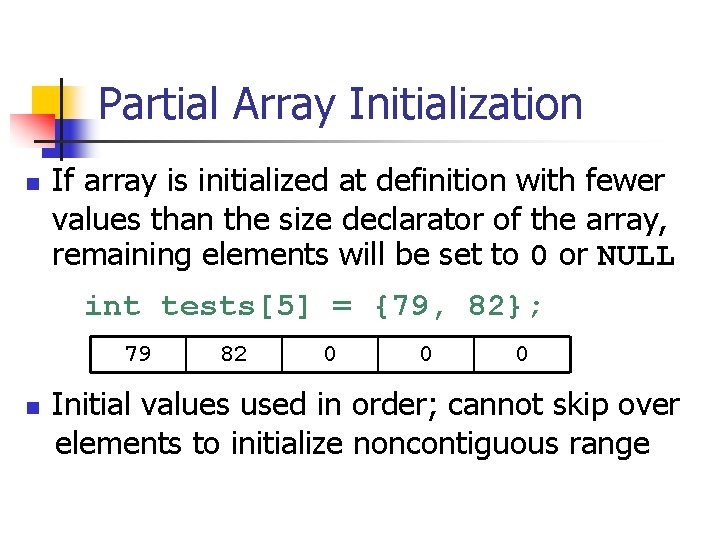
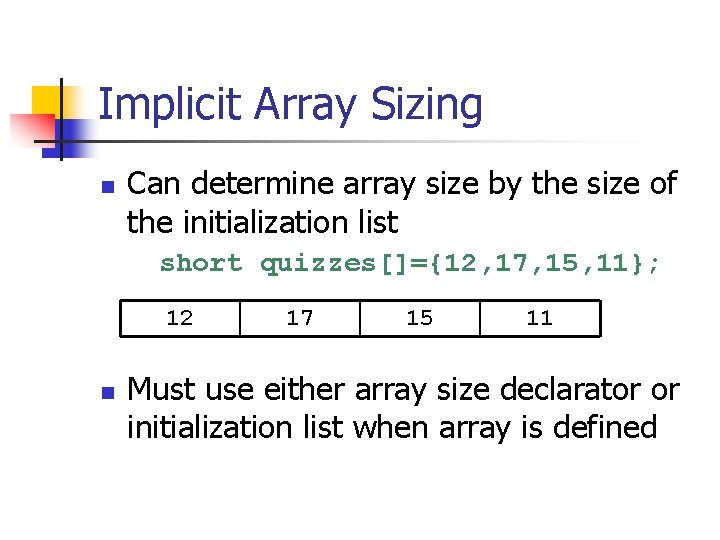
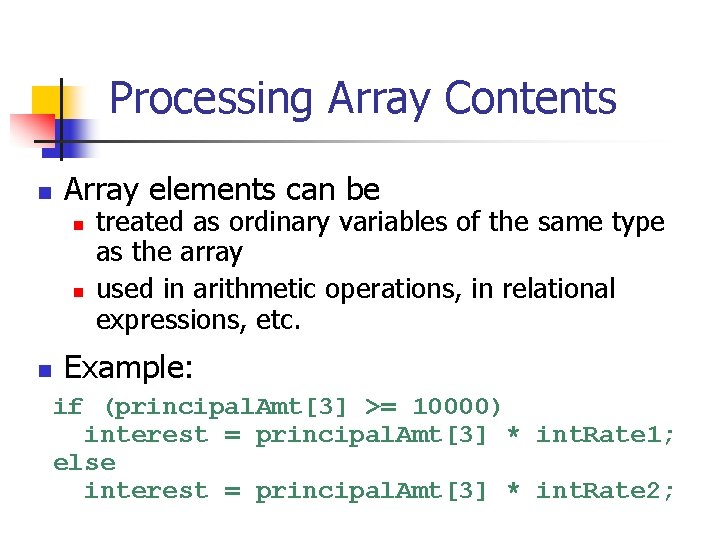
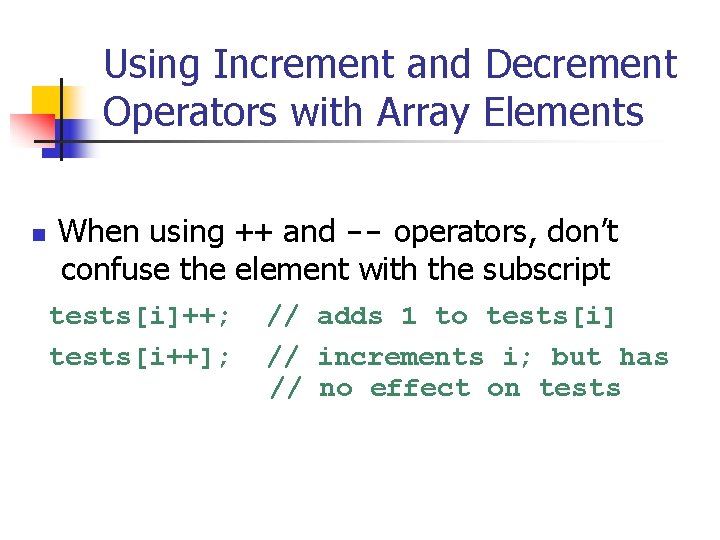
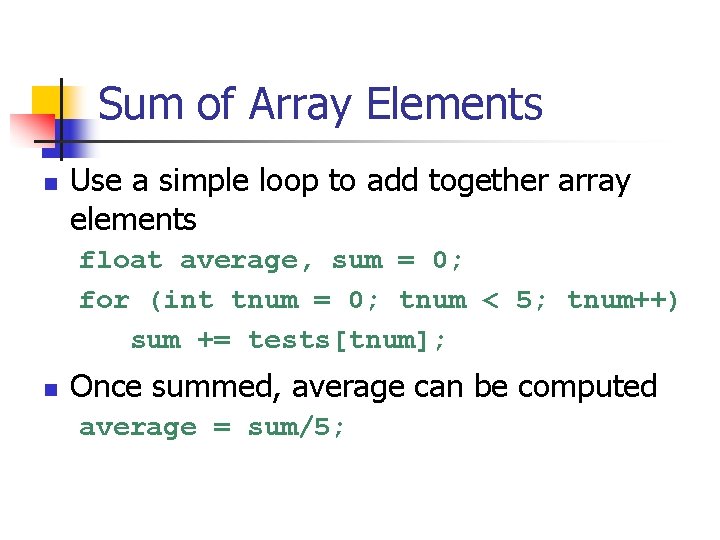
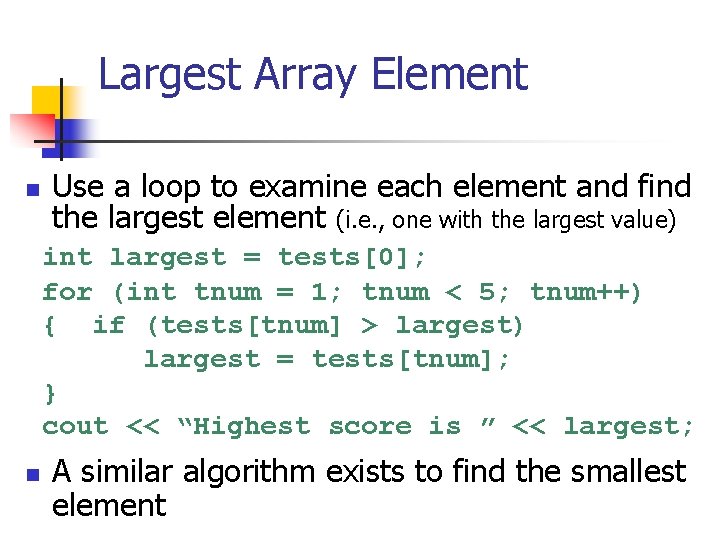
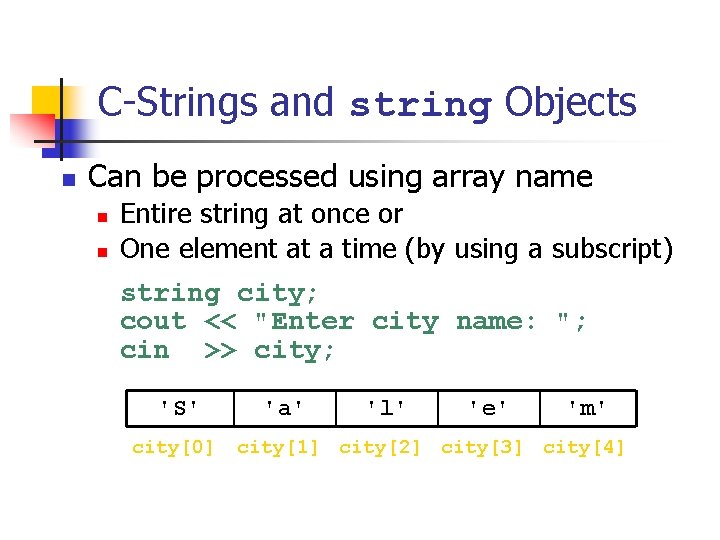
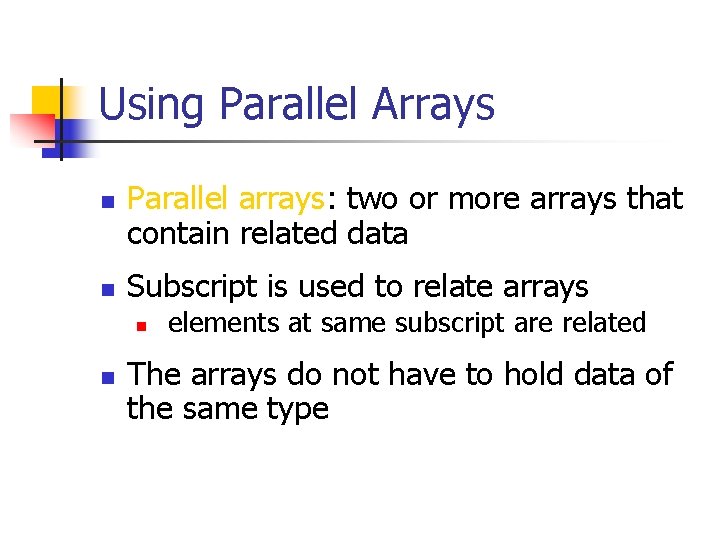
![Parallel Array Example int size = 5; string name[size]; // student name float average[size]; Parallel Array Example int size = 5; string name[size]; // student name float average[size];](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-23.jpg)
![Parallel Array Processing int size = 5; string name[size]; // student name float average[size]; Parallel Array Processing int size = 5; string name[size]; // student name float average[size];](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-24.jpg)
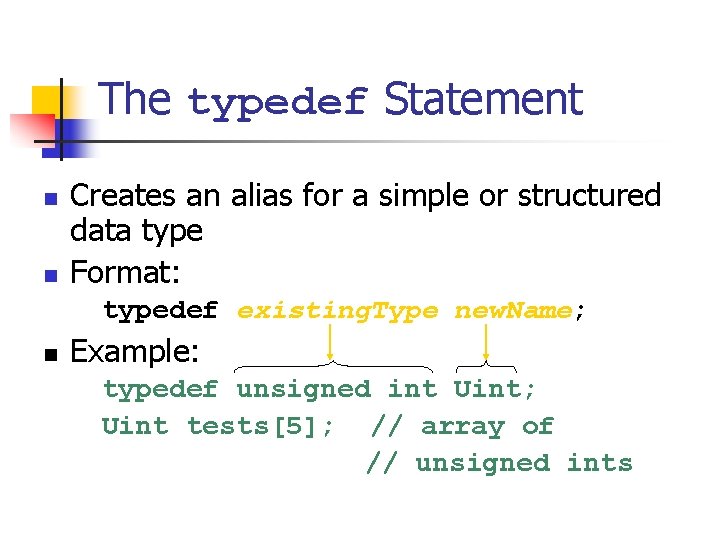
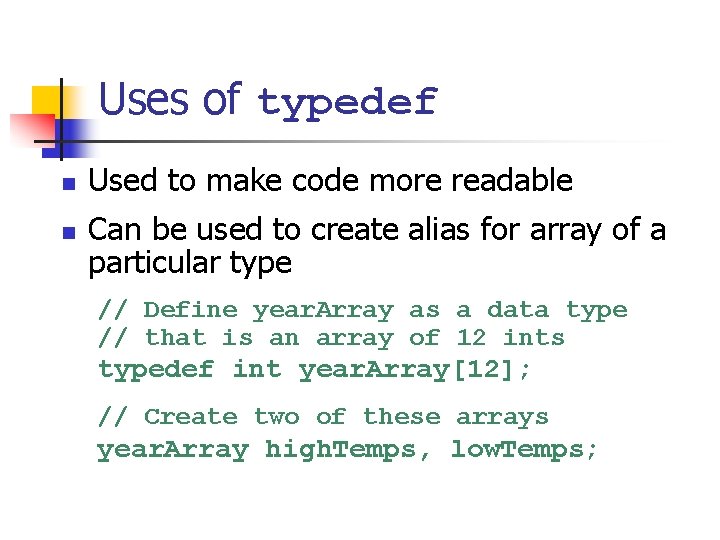
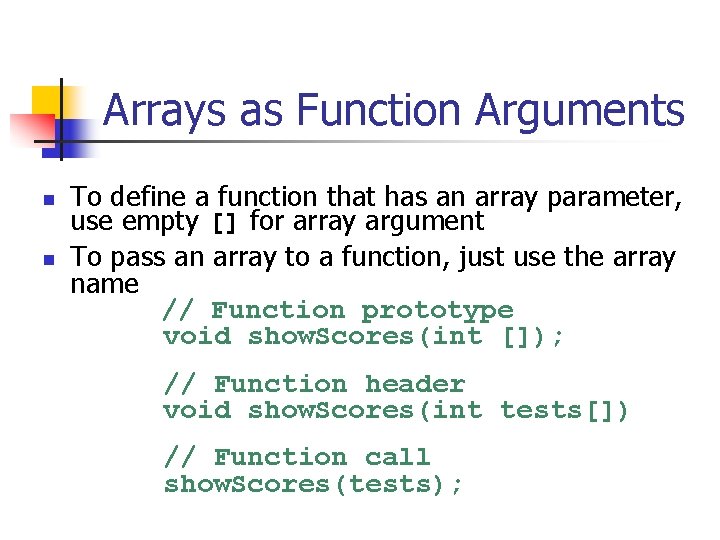
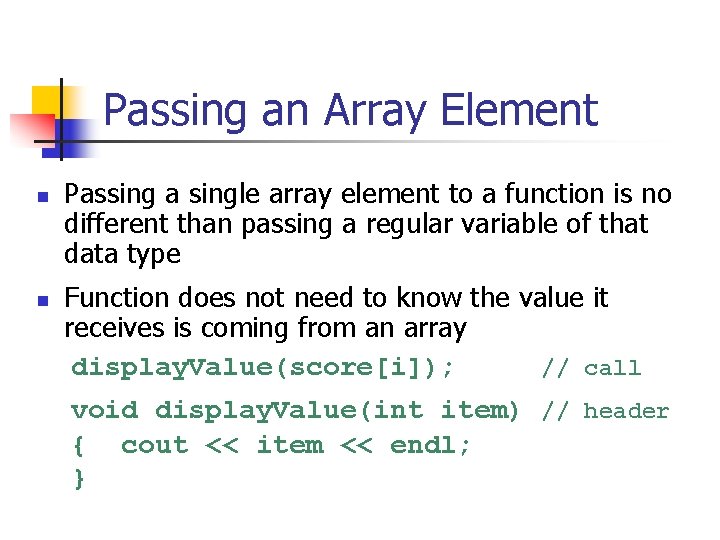
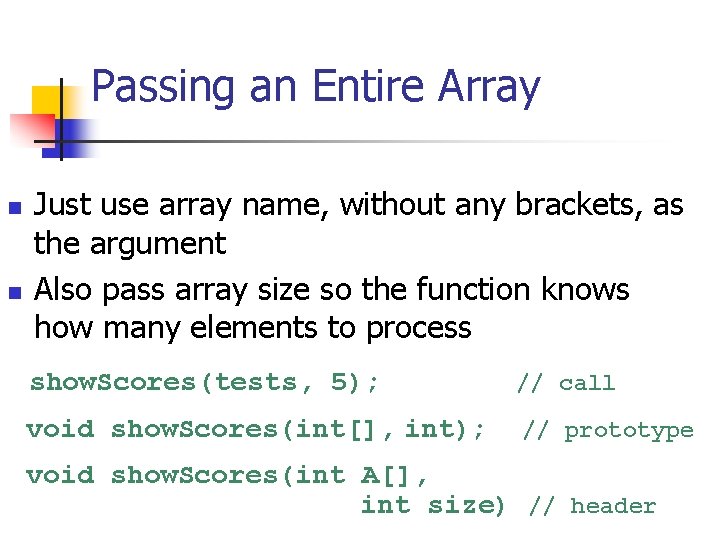
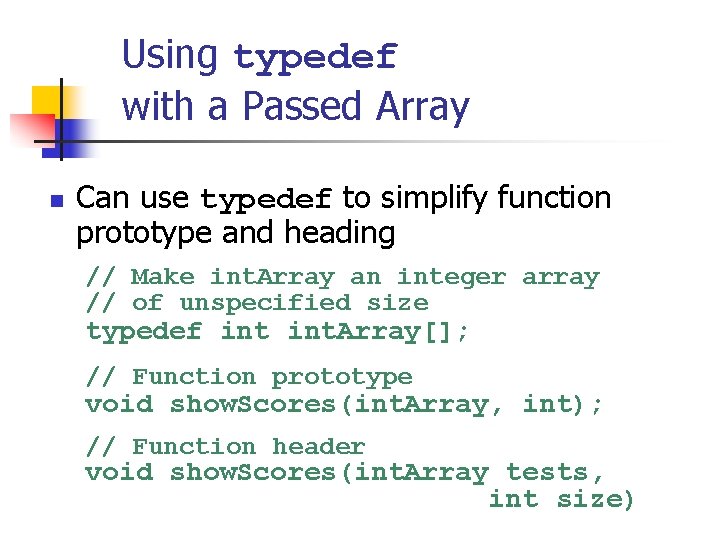
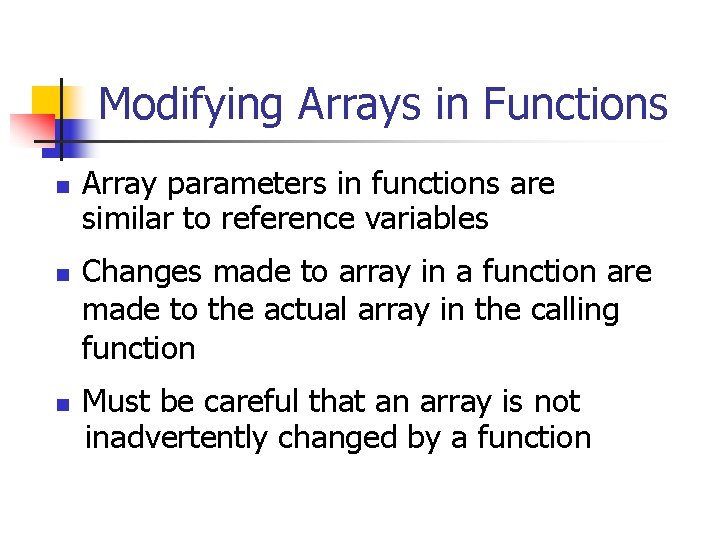
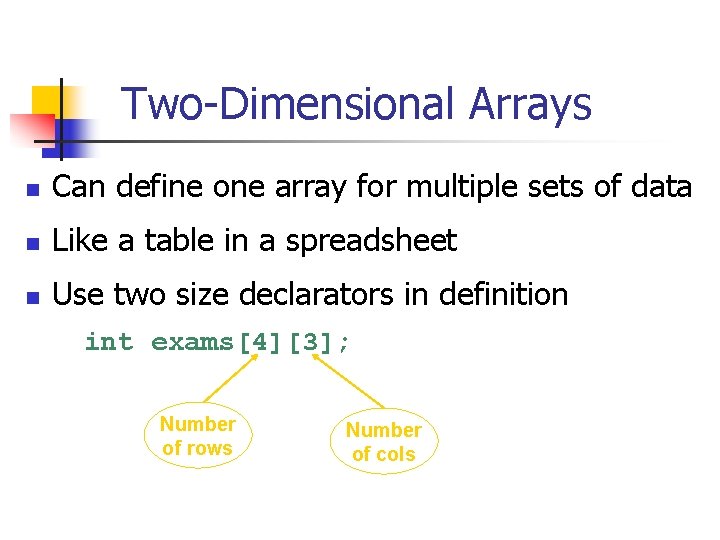
![Two-Dimensional Array Representation int exams[4][3]; columns exams[0][0] exams[0][1] exams[0][2] r o w s exams[1][0] Two-Dimensional Array Representation int exams[4][3]; columns exams[0][0] exams[0][1] exams[0][2] r o w s exams[1][0]](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-33.jpg)
![Initialization at Definition n Two-dimensional arrays are initialized row-by-row int exams[2][2] = { {84, Initialization at Definition n Two-dimensional arrays are initialized row-by-row int exams[2][2] = { {84,](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-34.jpg)
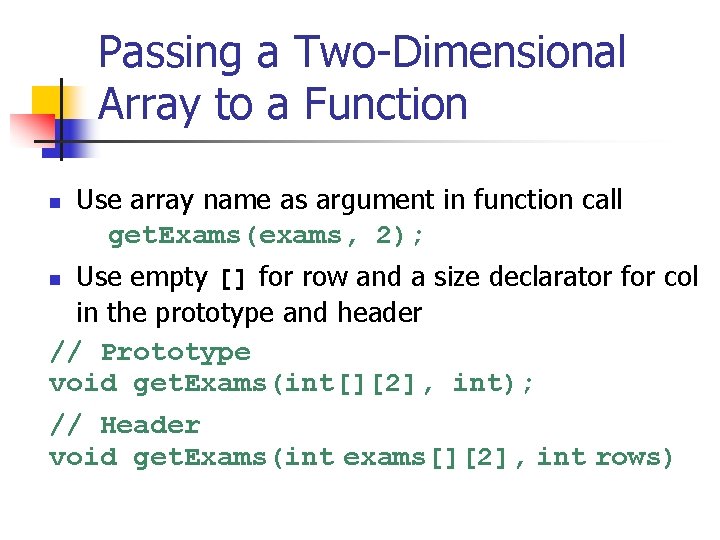
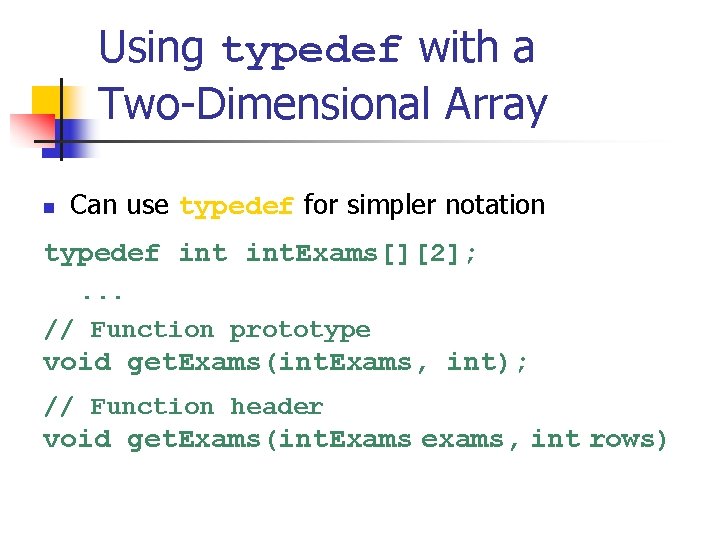
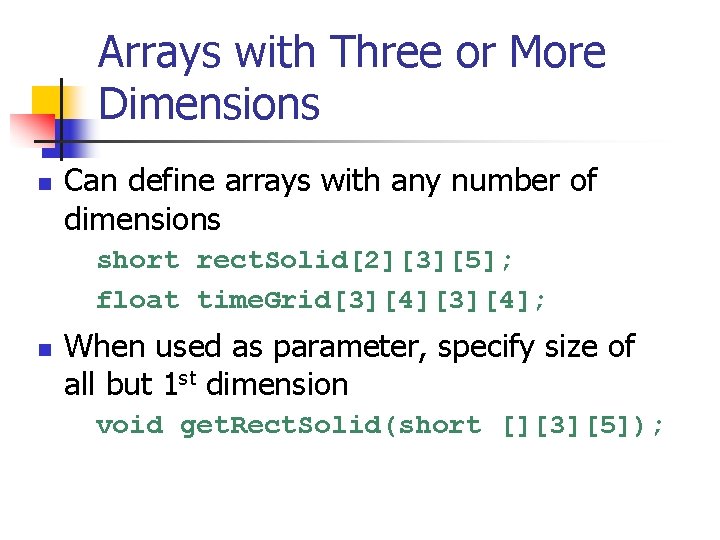
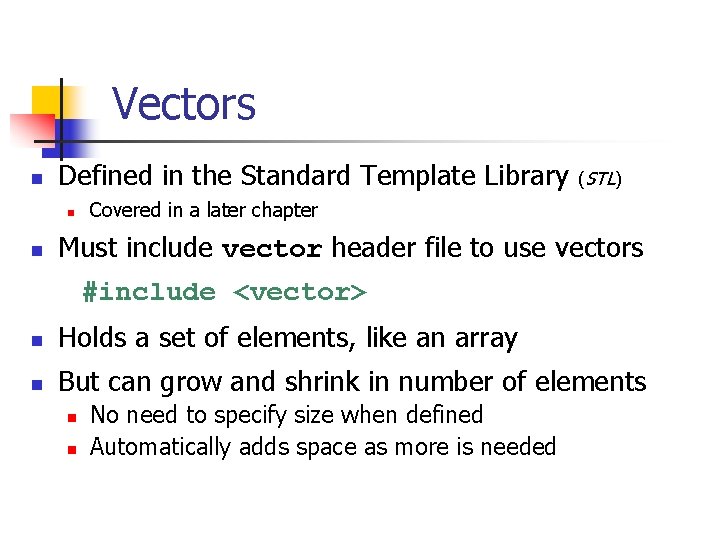
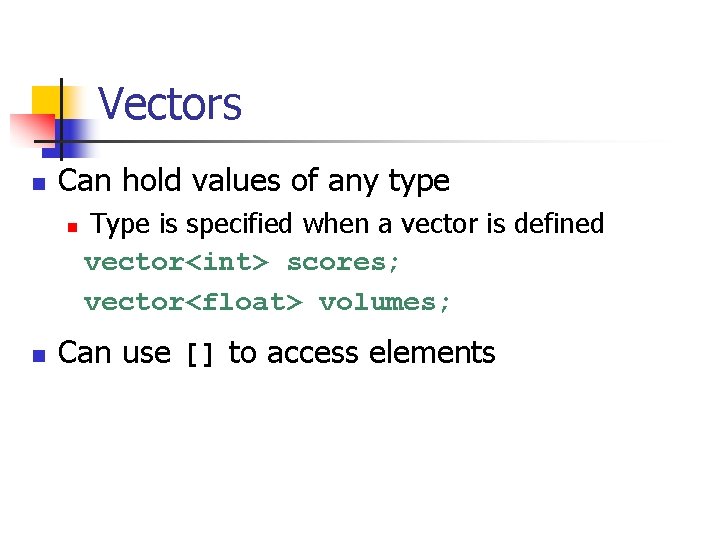
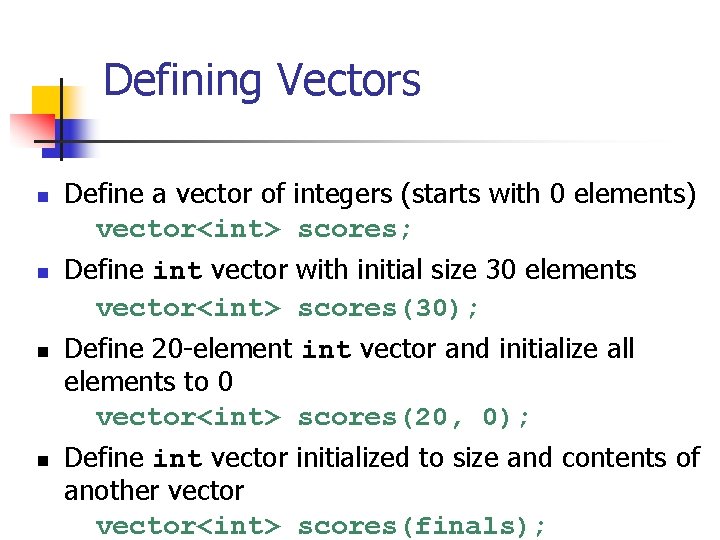
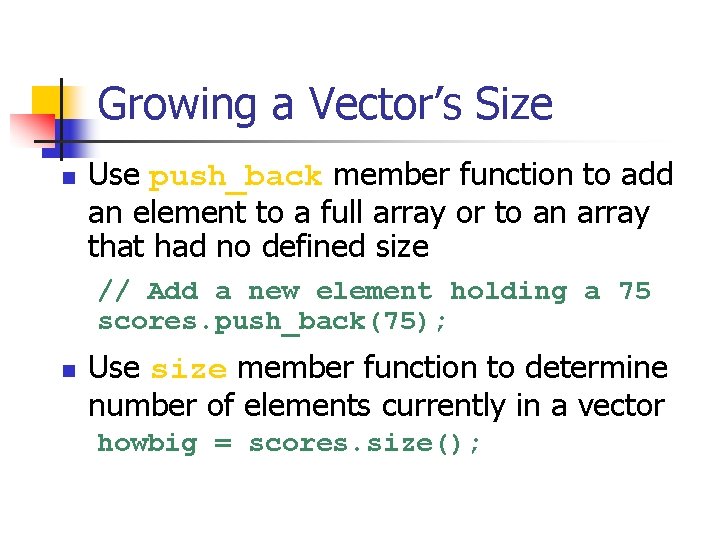
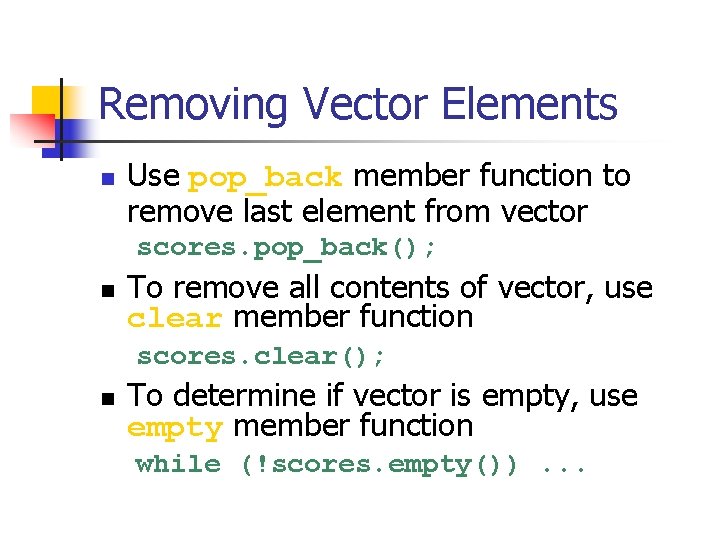
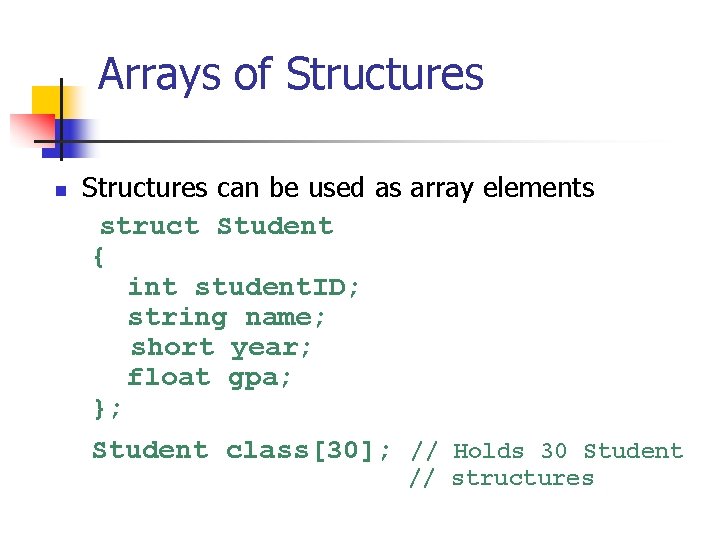
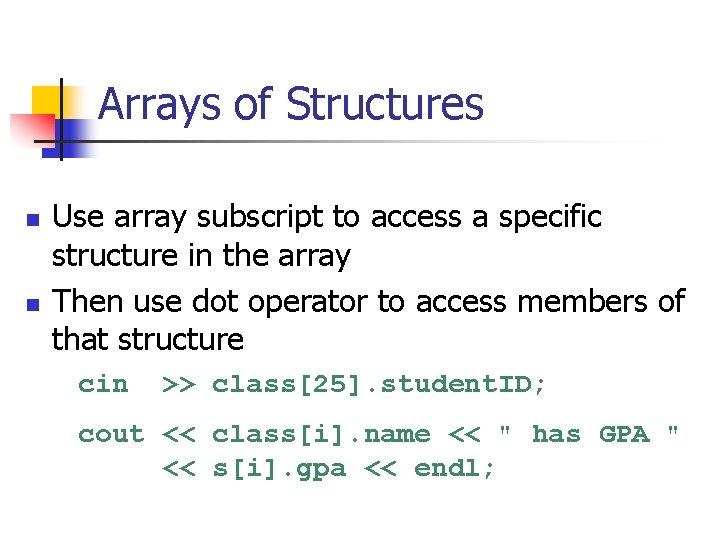
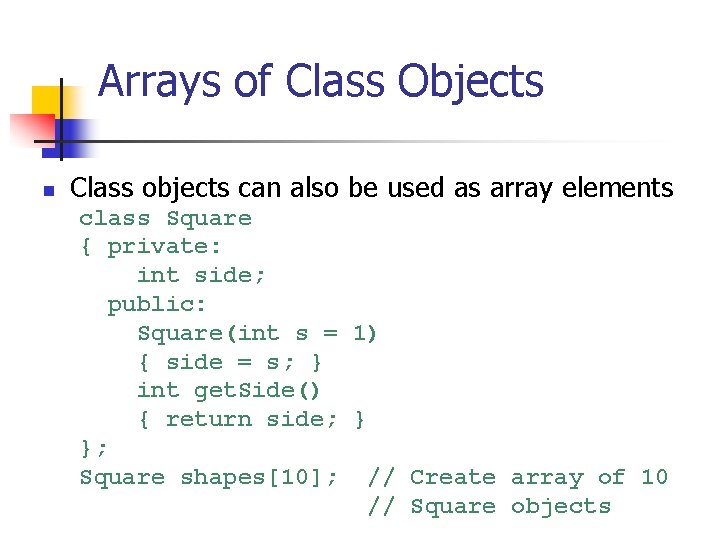
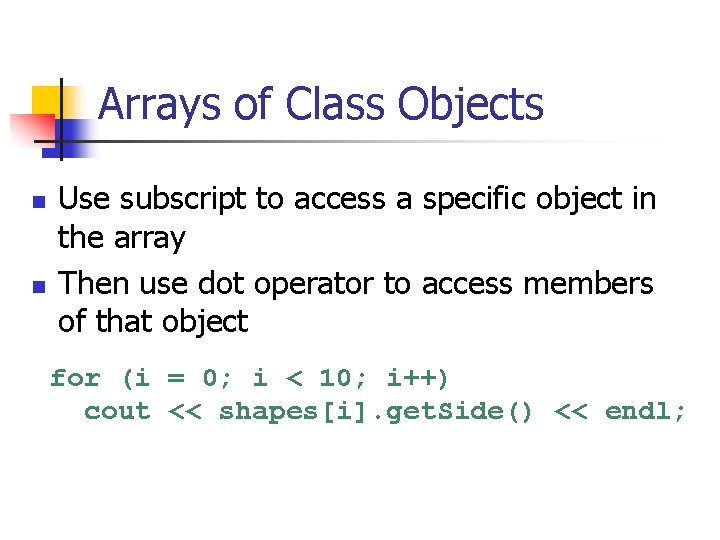
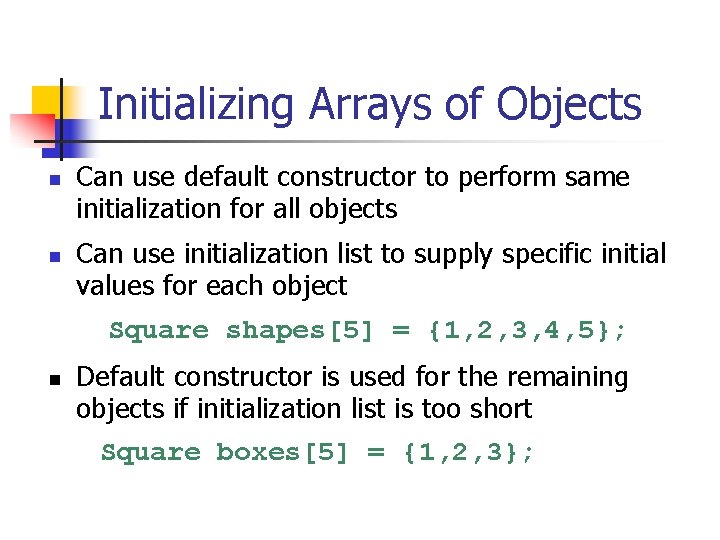
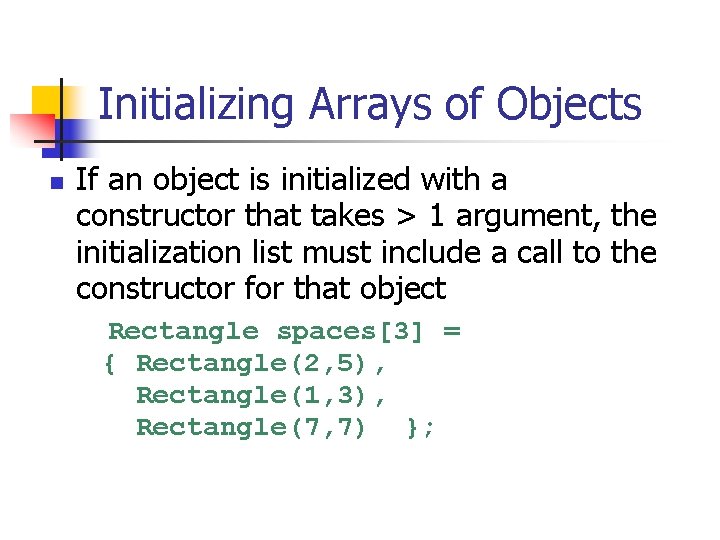
- Slides: 48
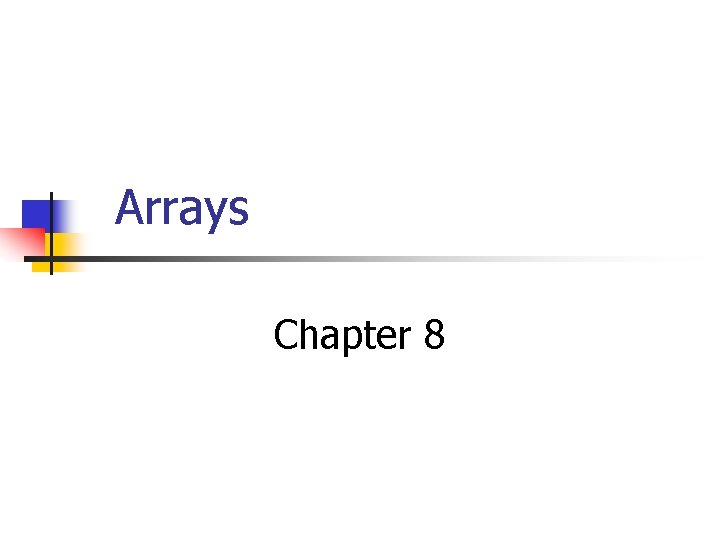
Arrays Chapter 8
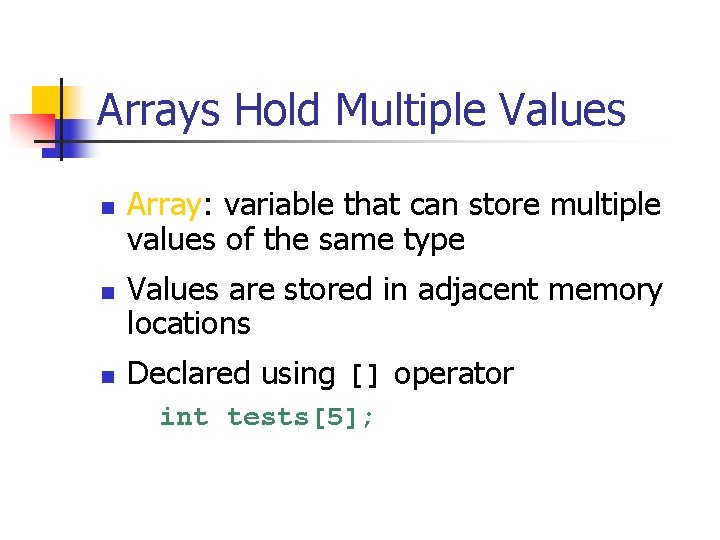
Arrays Hold Multiple Values n n n Array: variable that can store multiple values of the same type Values are stored in adjacent memory locations Declared using [] operator int tests[5];
![Array Storage in Memory n The definition int tests5 allocates the following memory first Array Storage in Memory n The definition int tests[5]; allocates the following memory first](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-3.jpg)
Array Storage in Memory n The definition int tests[5]; allocates the following memory first second third fourth fifth element element
![Array Terminology In the definition int tests5 n int is the data type of Array Terminology In the definition int tests[5]; n int is the data type of](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-4.jpg)
Array Terminology In the definition int tests[5]; n int is the data type of the array elements n n n tests is the name of the array 5, in [5], is the size declarator. It shows the number of elements in the array. The size of an array is the number of bytes allocated for it (number of elements) * (bytes needed for each element)
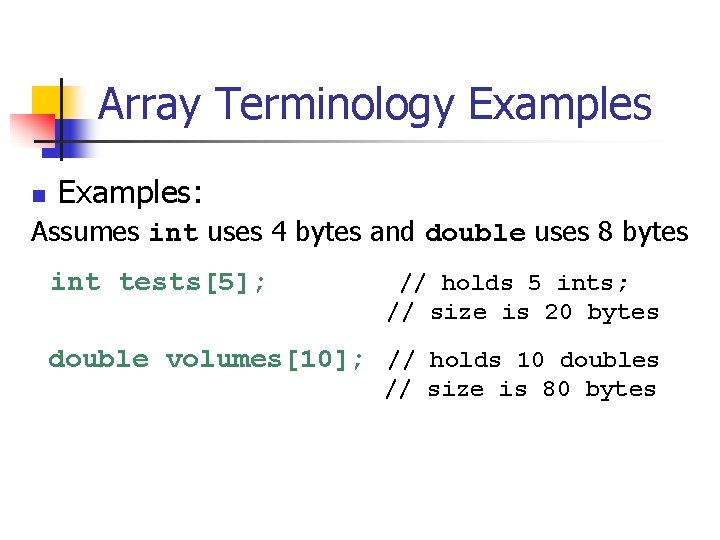
Array Terminology Examples n Examples: Assumes int uses 4 bytes and double uses 8 bytes int tests[5]; // holds 5 ints; // size is 20 bytes double volumes[10]; // holds 10 doubles // size is 80 bytes
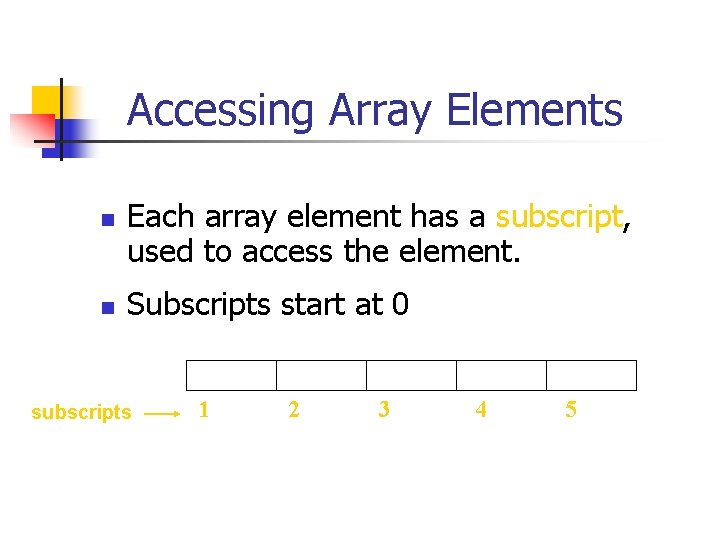
Accessing Array Elements n n Each array element has a subscript, used to access the element. Subscripts start at 0 subscripts 1 2 3 4 5
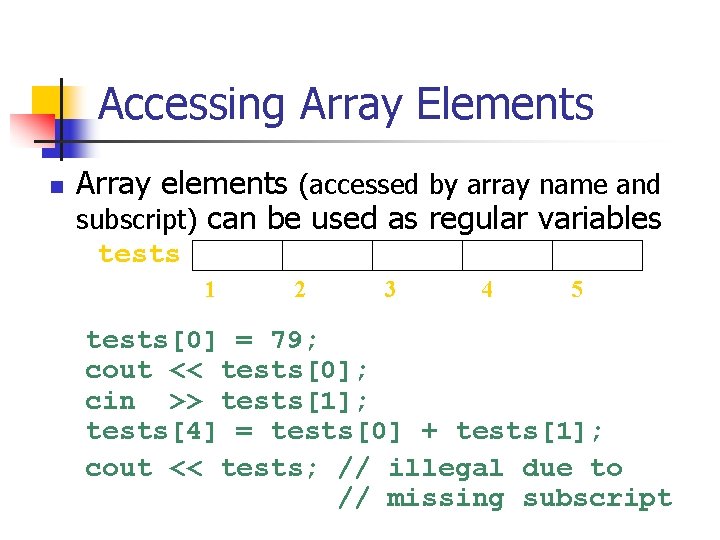
Accessing Array Elements n Array elements (accessed by array name and subscript) can be used as regular variables tests 1 2 3 4 5 tests[0] = 79; cout << tests[0]; cin >> tests[1]; tests[4] = tests[0] + tests[1]; cout << tests; // illegal due to // missing subscript
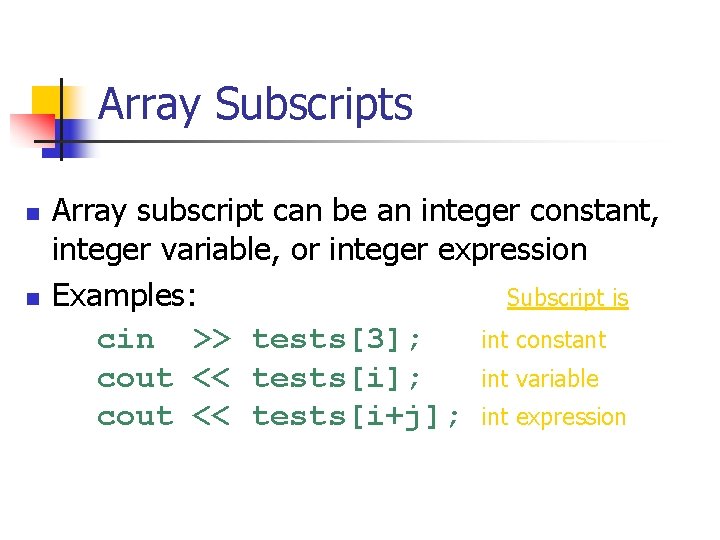
Array Subscripts n n Array subscript can be an integer constant, integer variable, or integer expression Examples: Subscript is cin >> tests[3]; int constant cout << tests[i]; int variable cout << tests[i+j]; int expression
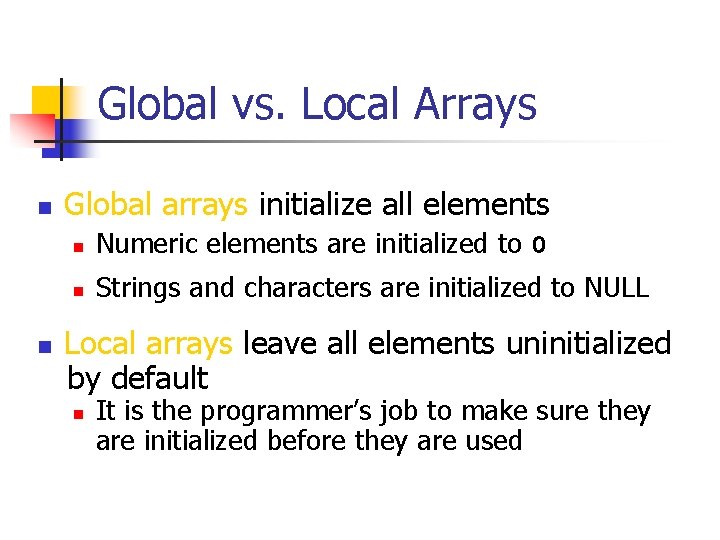
Global vs. Local Arrays n n Global arrays initialize all elements n Numeric elements are initialized to 0 n Strings and characters are initialized to NULL Local arrays leave all elements uninitialized by default n It is the programmer’s job to make sure they are initialized before they are used
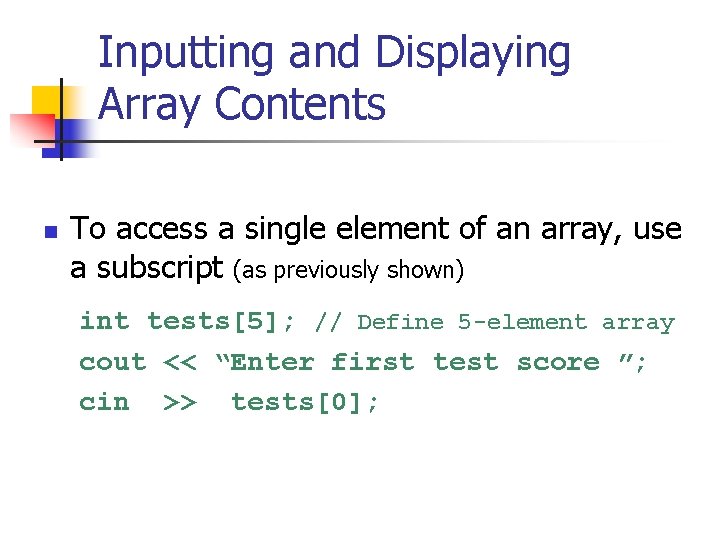
Inputting and Displaying Array Contents n To access a single element of an array, use a subscript (as previously shown) int tests[5]; // Define 5 -element array cout << “Enter first test score ”; cin >> tests[0];
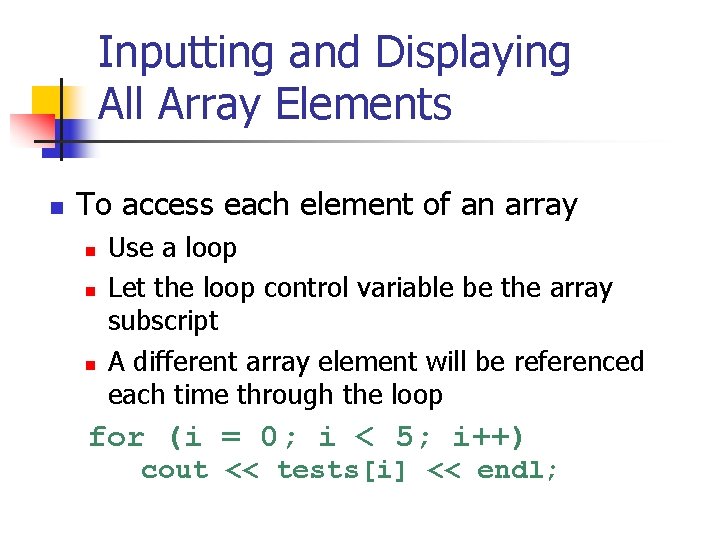
Inputting and Displaying All Array Elements n To access each element of an array n n n Use a loop Let the loop control variable be the array subscript A different array element will be referenced each time through the loop for (i = 0; i < 5; i++) cout << tests[i] << endl;
![Getting Array Data from a File int sales5 ifstream datafile datafile opensales dat if Getting Array Data from a File int sales[5]; ifstream datafile; datafile. open("sales. dat"); if](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-12.jpg)
Getting Array Data from a File int sales[5]; ifstream datafile; datafile. open("sales. dat"); if (!datafile) cout << "Error opening data filen"; else { // Input daily sales for (int day = 0; day < 5; day++) datafile >> sales[day]; datafile. close(); }
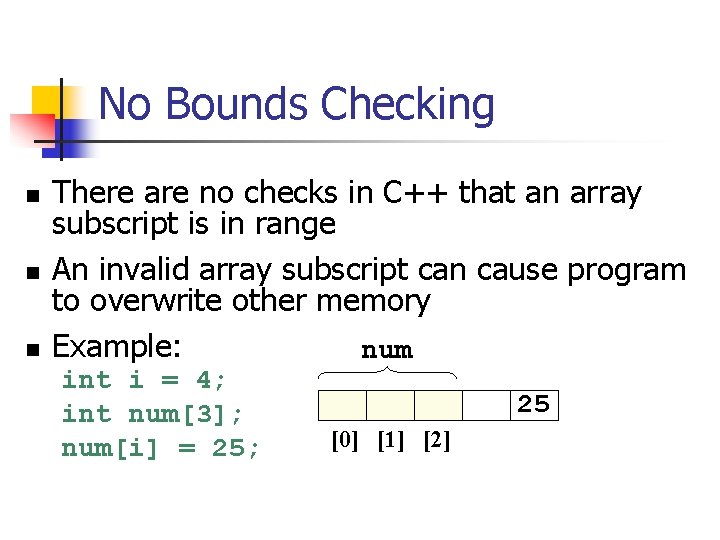
No Bounds Checking n n n There are no checks in C++ that an array subscript is in range An invalid array subscript can cause program to overwrite other memory Example: num int i = 4; int num[3]; num[i] = 25; 25 [0] [1] [2]
![Array Initialization n Can be initialized during program execution with assignment statements tests0 Array Initialization n Can be initialized during program execution with assignment statements tests[0] =](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-14.jpg)
Array Initialization n Can be initialized during program execution with assignment statements tests[0] = 79; tests[1] = 82; // etc. n Can be initialized at array definition with an initialization list int tests[5] = {79, 82, 91, 77, 84};
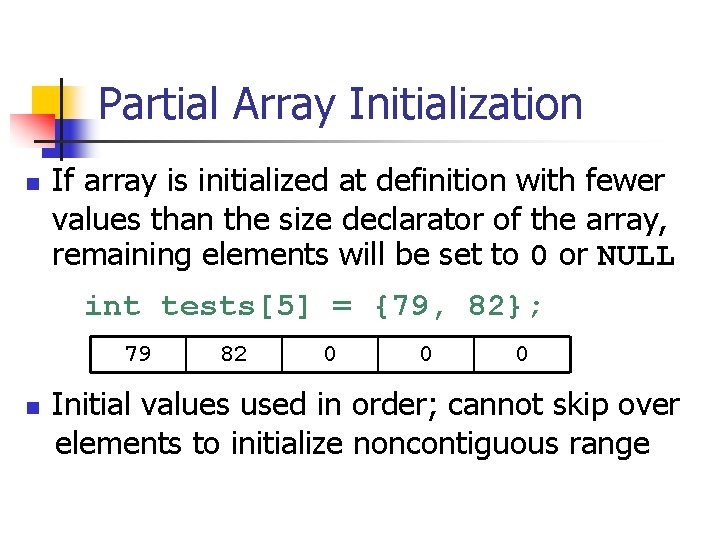
Partial Array Initialization n If array is initialized at definition with fewer values than the size declarator of the array, remaining elements will be set to 0 or NULL int tests[5] = {79, 82}; 79 n 82 0 0 0 Initial values used in order; cannot skip over elements to initialize noncontiguous range
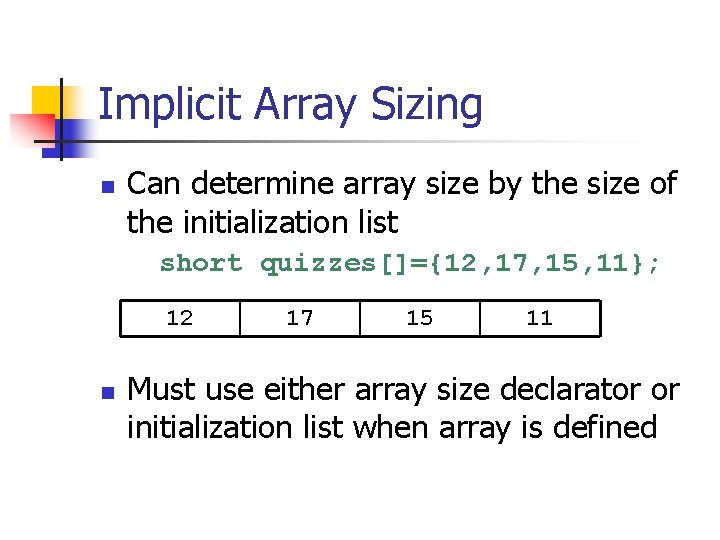
Implicit Array Sizing n Can determine array size by the size of the initialization list short quizzes[]={12, 17, 15, 11}; 12 n 17 15 11 Must use either array size declarator or initialization list when array is defined
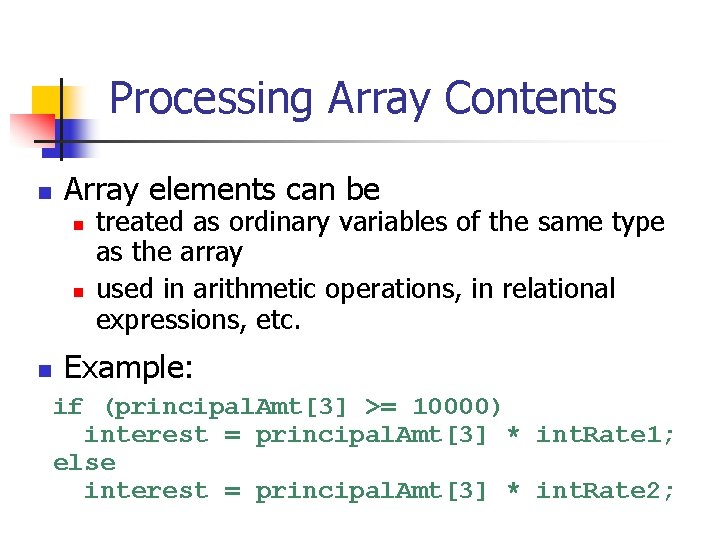
Processing Array Contents n Array elements can be n n n treated as ordinary variables of the same type as the array used in arithmetic operations, in relational expressions, etc. Example: if (principal. Amt[3] >= 10000) interest = principal. Amt[3] * int. Rate 1; else interest = principal. Amt[3] * int. Rate 2;
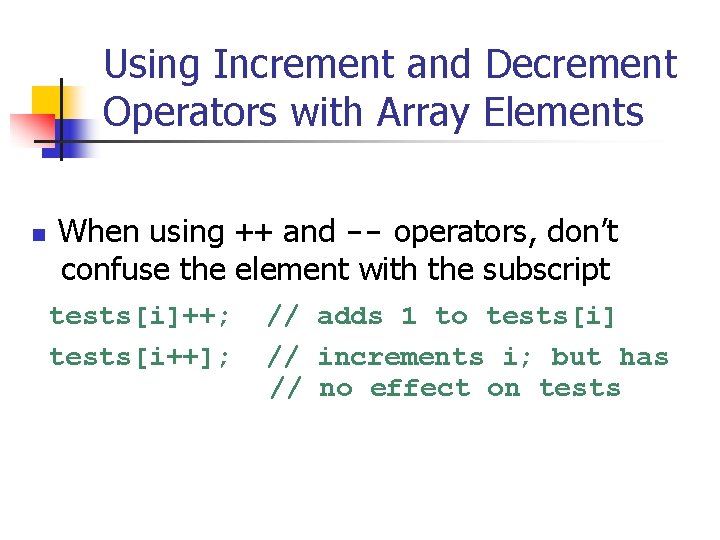
Using Increment and Decrement Operators with Array Elements n When using ++ and -- operators, don’t confuse the element with the subscript tests[i]++; tests[i++]; // adds 1 to tests[i] // increments i; but has // no effect on tests
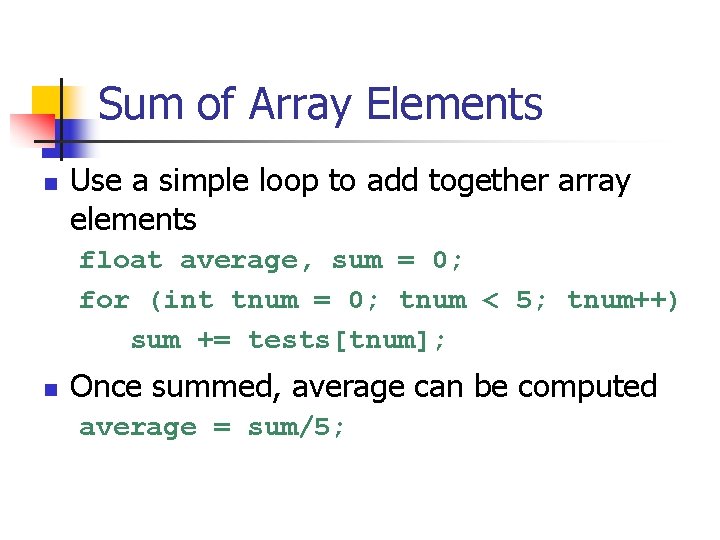
Sum of Array Elements n Use a simple loop to add together array elements float average, sum = 0; for (int tnum = 0; tnum < 5; tnum++) sum += tests[tnum]; n Once summed, average can be computed average = sum/5;
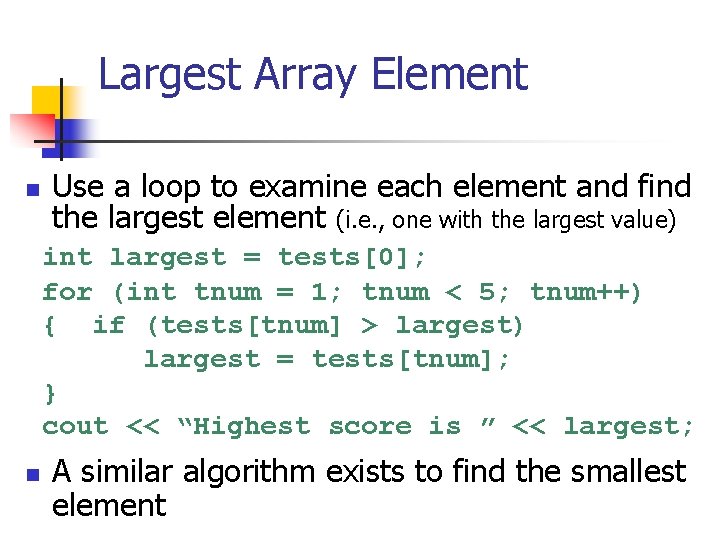
Largest Array Element n Use a loop to examine each element and find the largest element (i. e. , one with the largest value) int largest = tests[0]; for (int tnum = 1; tnum < 5; tnum++) { if (tests[tnum] > largest) largest = tests[tnum]; } cout << “Highest score is ” << largest; n A similar algorithm exists to find the smallest element
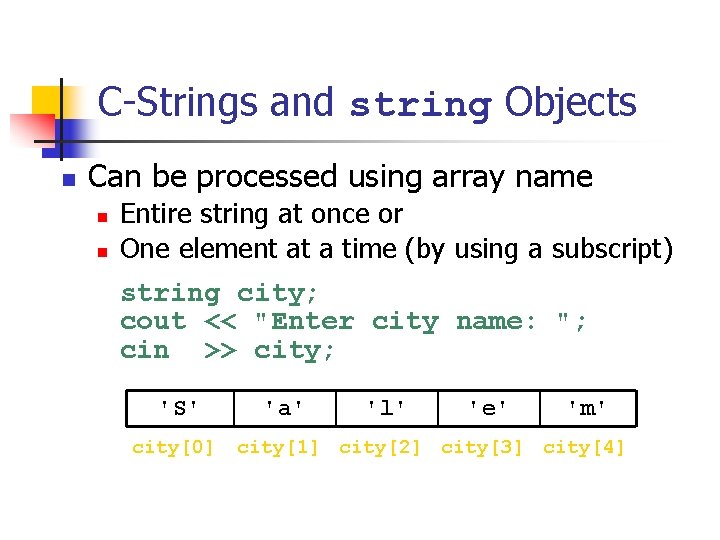
C-Strings and string Objects n Can be processed using array name n n Entire string at once or One element at a time (by using a subscript) string city; cout << "Enter city name: "; cin >> city; 'S' city[0] 'a' 'l' 'e' 'm' city[1] city[2] city[3] city[4]
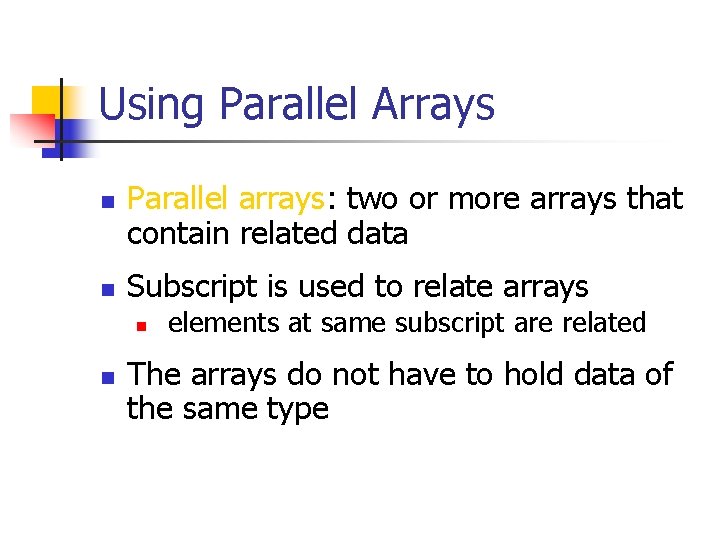
Using Parallel Arrays n n Parallel arrays: two or more arrays that contain related data Subscript is used to relate arrays n n elements at same subscript are related The arrays do not have to hold data of the same type
![Parallel Array Example int size 5 string namesize student name float averagesize Parallel Array Example int size = 5; string name[size]; // student name float average[size];](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-23.jpg)
Parallel Array Example int size = 5; string name[size]; // student name float average[size]; // course average char grade[size]; // course grade name 0 1 2 3 4 average 0 1 2 3 4 grade 0 1 2 3 4
![Parallel Array Processing int size 5 string namesize student name float averagesize Parallel Array Processing int size = 5; string name[size]; // student name float average[size];](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-24.jpg)
Parallel Array Processing int size = 5; string name[size]; // student name float average[size]; // course average char grade[size]; // course grade. . . for (int i = 0; i < size; i++) cout << " Student: " << name[i] << " Average: " << average[i] << " Grade: " << grade[i] << endl;
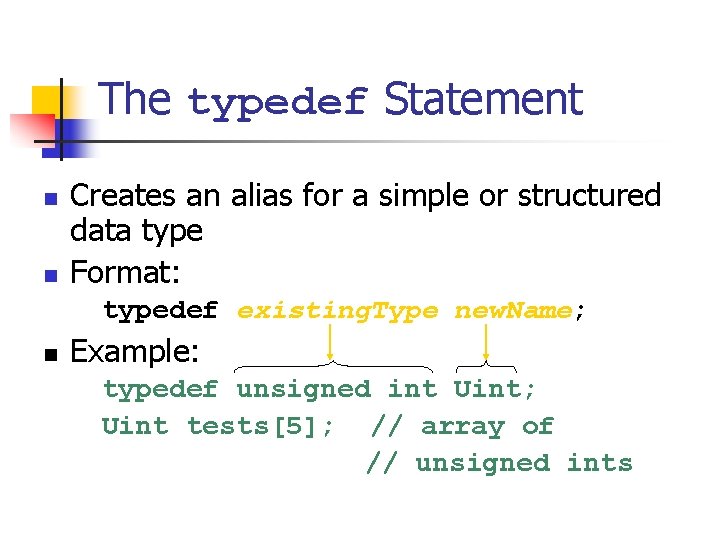
The typedef Statement n n Creates an alias for a simple or structured data type Format: typedef existing. Type new. Name; n Example: typedef unsigned int Uint; Uint tests[5]; // array of // unsigned ints
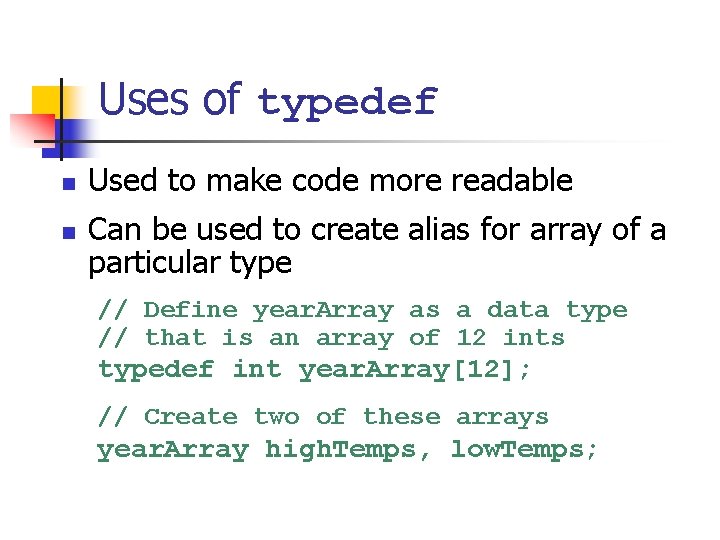
Uses of typedef n n Used to make code more readable Can be used to create alias for array of a particular type // Define year. Array as a data type // that is an array of 12 ints typedef int year. Array[12]; // Create two of these arrays year. Array high. Temps, low. Temps;
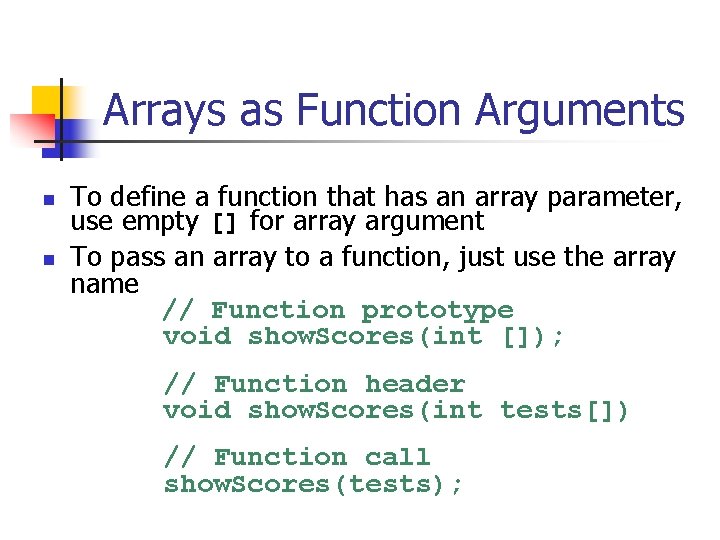
Arrays as Function Arguments n n To define a function that has an array parameter, use empty [] for array argument To pass an array to a function, just use the array name // Function prototype void show. Scores(int []); // Function header void show. Scores(int tests[]) // Function call show. Scores(tests);
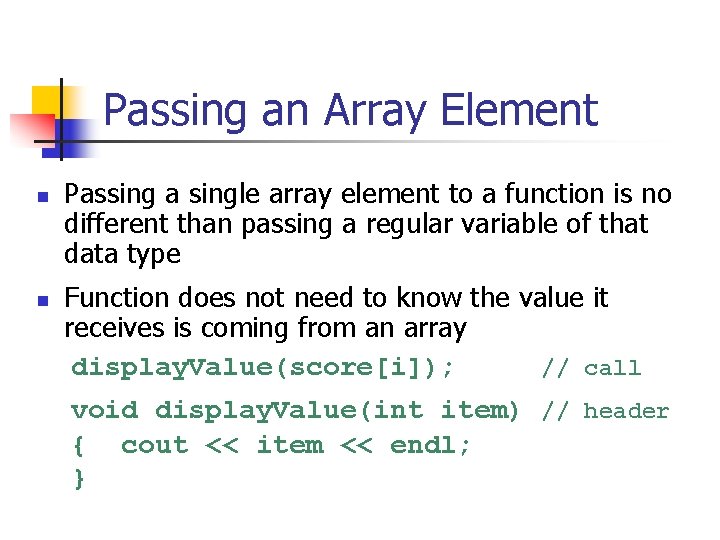
Passing an Array Element n n Passing a single array element to a function is no different than passing a regular variable of that data type Function does not need to know the value it receives is coming from an array display. Value(score[i]); // call void display. Value(int item) // header { cout << item << endl; }
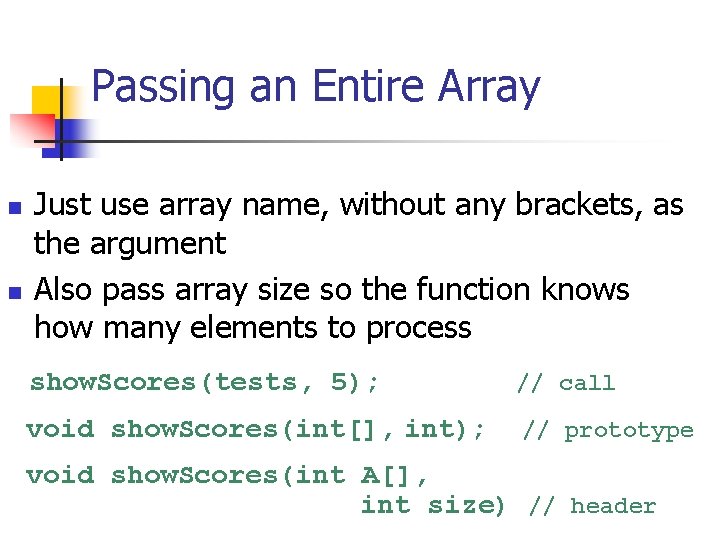
Passing an Entire Array n n Just use array name, without any brackets, as the argument Also pass array size so the function knows how many elements to process show. Scores(tests, 5); // call void show. Scores(int[], int); // prototype void show. Scores(int A[], int size) // header
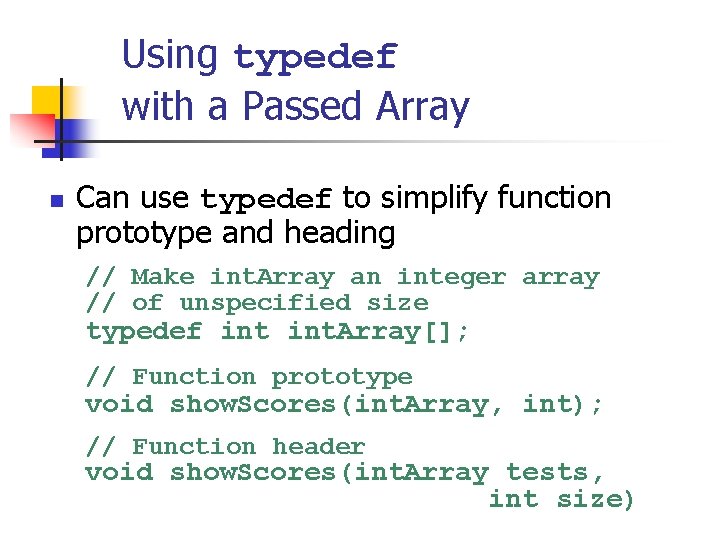
Using typedef with a Passed Array n Can use typedef to simplify function prototype and heading // Make int. Array an integer array // of unspecified size typedef int. Array[]; // Function prototype void show. Scores(int. Array, int); // Function header void show. Scores(int. Array tests, int size)
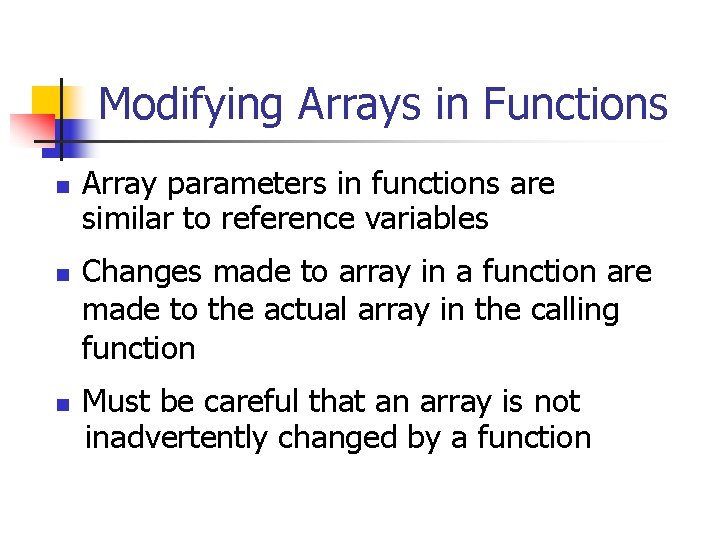
Modifying Arrays in Functions n n n Array parameters in functions are similar to reference variables Changes made to array in a function are made to the actual array in the calling function Must be careful that an array is not inadvertently changed by a function
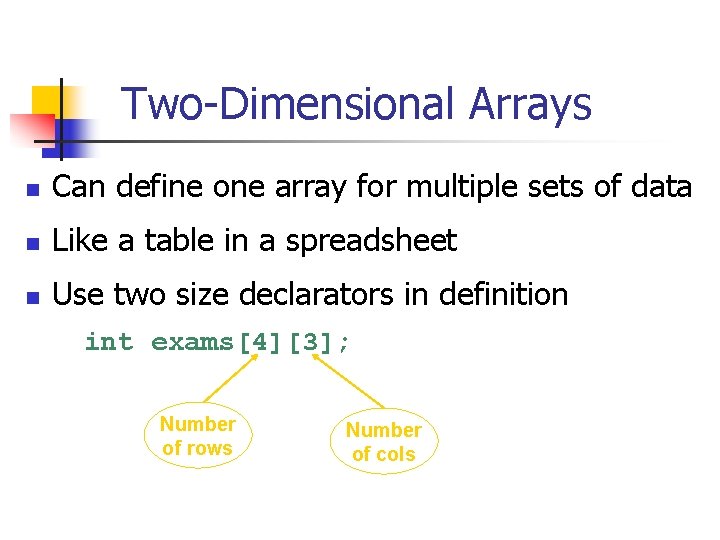
Two-Dimensional Arrays n Can define one array for multiple sets of data n Like a table in a spreadsheet n Use two size declarators in definition int exams[4][3]; Number of rows Number of cols
![TwoDimensional Array Representation int exams43 columns exams00 exams01 exams02 r o w s exams10 Two-Dimensional Array Representation int exams[4][3]; columns exams[0][0] exams[0][1] exams[0][2] r o w s exams[1][0]](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-33.jpg)
Two-Dimensional Array Representation int exams[4][3]; columns exams[0][0] exams[0][1] exams[0][2] r o w s exams[1][0] exams[1][1] exams[1][2] exams[2][0] exams[2][1] exams[2][2] exams[3][0] exams[3][1] exams[3][2] n Use two subscripts to access element exams[2][2] = 86;
![Initialization at Definition n Twodimensional arrays are initialized rowbyrow int exams22 84 Initialization at Definition n Two-dimensional arrays are initialized row-by-row int exams[2][2] = { {84,](https://slidetodoc.com/presentation_image_h2/05b3294023dabc07172dd66ff6dc42a1/image-34.jpg)
Initialization at Definition n Two-dimensional arrays are initialized row-by-row int exams[2][2] = { {84, 78}, {92, 97} }; 84 78 92 97 n Can omit inner { }
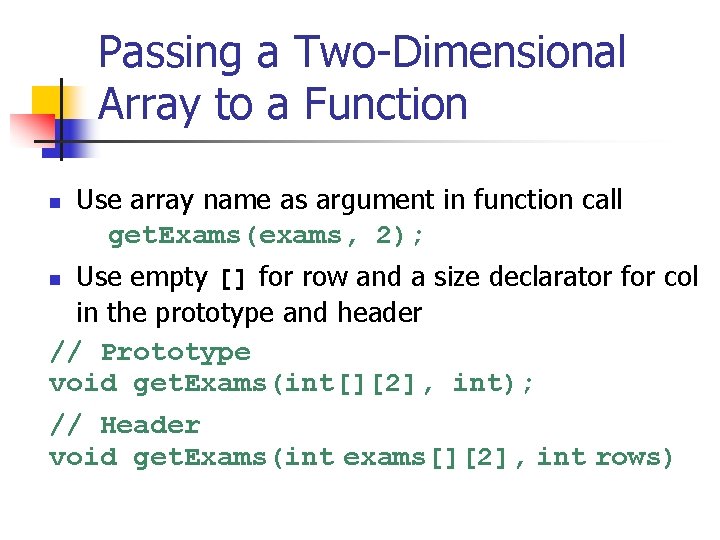
Passing a Two-Dimensional Array to a Function n Use array name as argument in function call get. Exams(exams, 2); Use empty [] for row and a size declarator for col in the prototype and header // Prototype void get. Exams(int[][2], int); n // Header void get. Exams(int exams[][2], int rows)
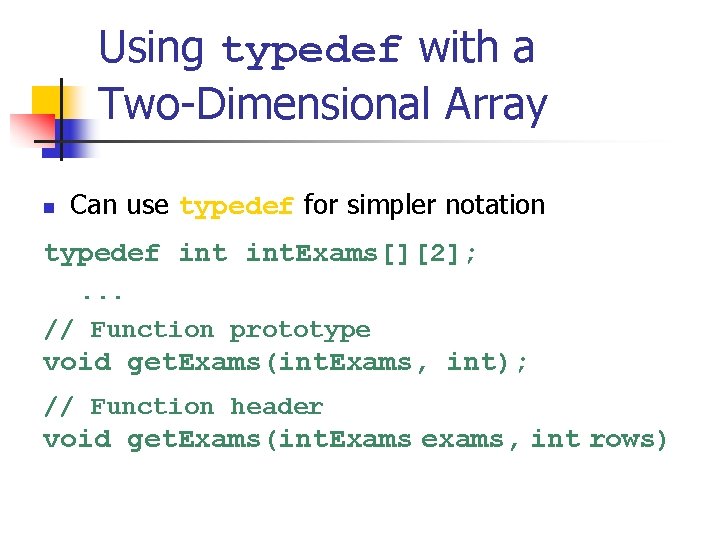
Using typedef with a Two-Dimensional Array n Can use typedef for simpler notation typedef int. Exams[][2]; . . . // Function prototype void get. Exams(int. Exams, int); // Function header void get. Exams(int. Exams exams, int rows)
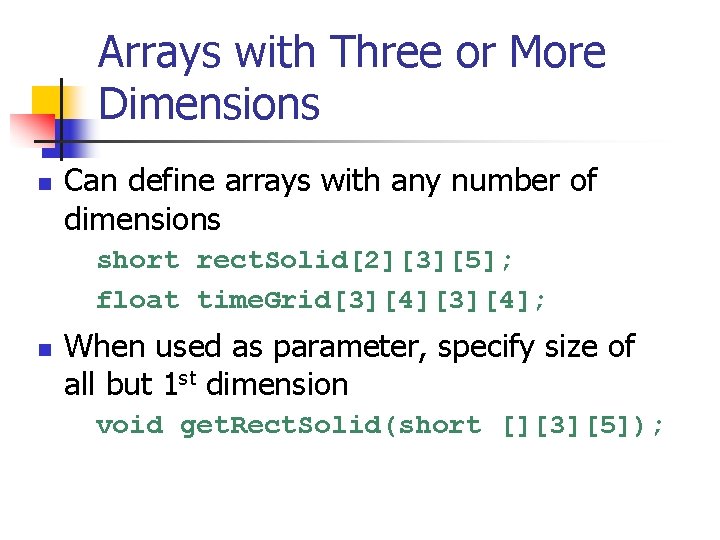
Arrays with Three or More Dimensions n Can define arrays with any number of dimensions short rect. Solid[2][3][5]; float time. Grid[3][4]; n When used as parameter, specify size of all but 1 st dimension void get. Rect. Solid(short [][3][5]);
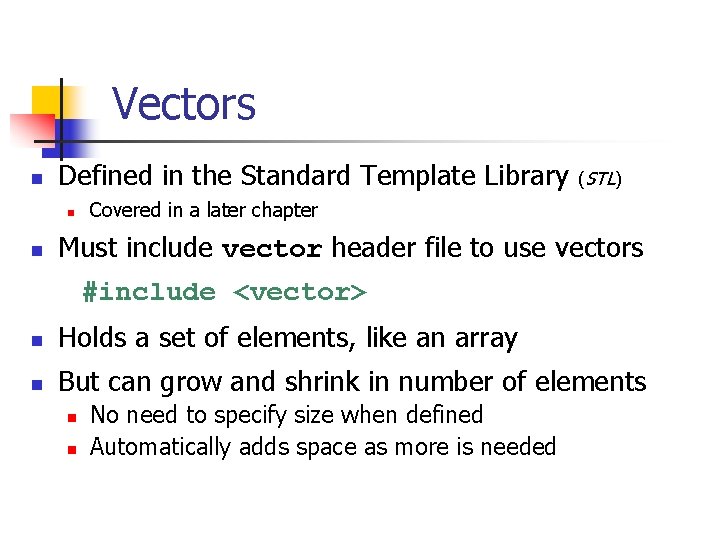
Vectors n Defined in the Standard Template Library n n (STL) Covered in a later chapter Must include vector header file to use vectors #include <vector> n Holds a set of elements, like an array n But can grow and shrink in number of elements n n No need to specify size when defined Automatically adds space as more is needed
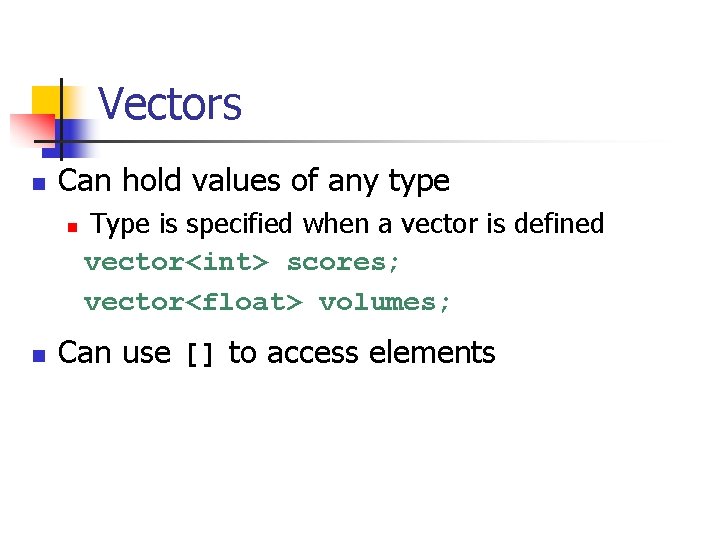
Vectors n Can hold values of any type n n Type is specified when a vector is defined vector<int> scores; vector<float> volumes; Can use [] to access elements
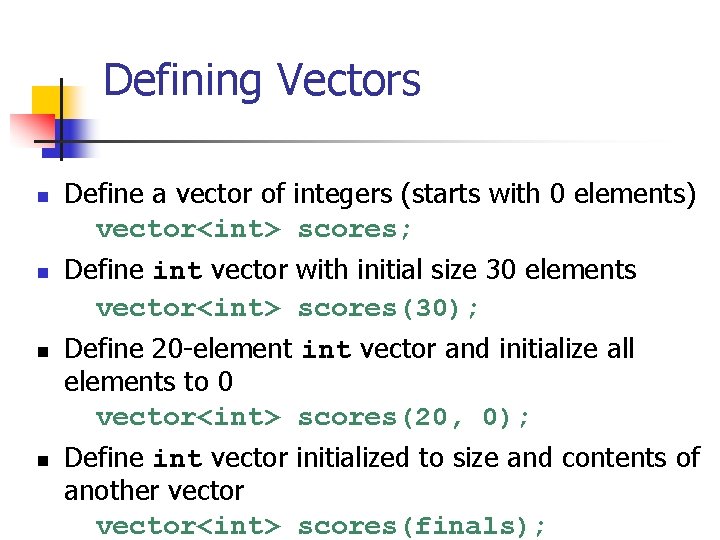
Defining Vectors n n Define a vector of integers (starts with 0 elements) vector<int> scores; Define int vector with initial size 30 elements vector<int> scores(30); Define 20 -element int vector and initialize all elements to 0 vector<int> scores(20, 0); Define int vector initialized to size and contents of another vector<int> scores(finals);
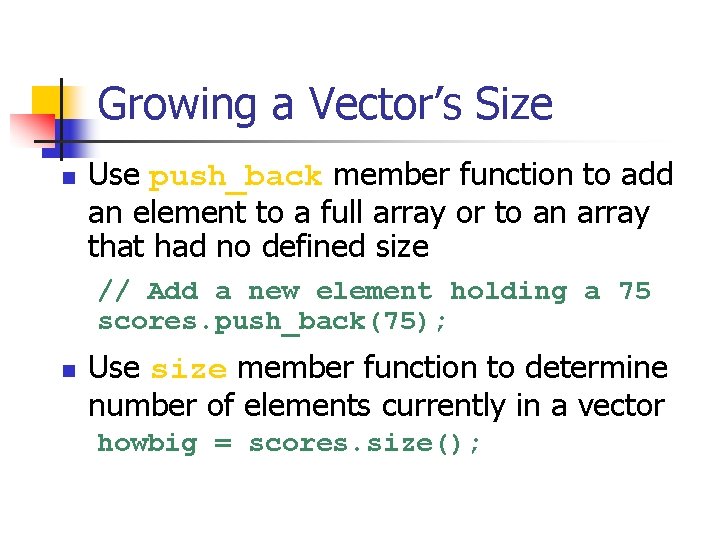
Growing a Vector’s Size n Use push_back member function to add an element to a full array or to an array that had no defined size // Add a new element holding a 75 scores. push_back(75); n Use size member function to determine number of elements currently in a vector howbig = scores. size();
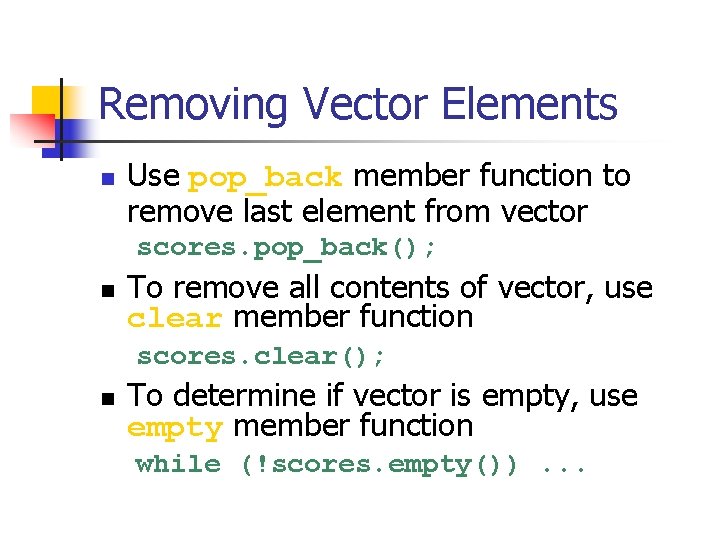
Removing Vector Elements n Use pop_back member function to remove last element from vector scores. pop_back(); n To remove all contents of vector, use clear member function scores. clear(); n To determine if vector is empty, use empty member function while (!scores. empty()). . .
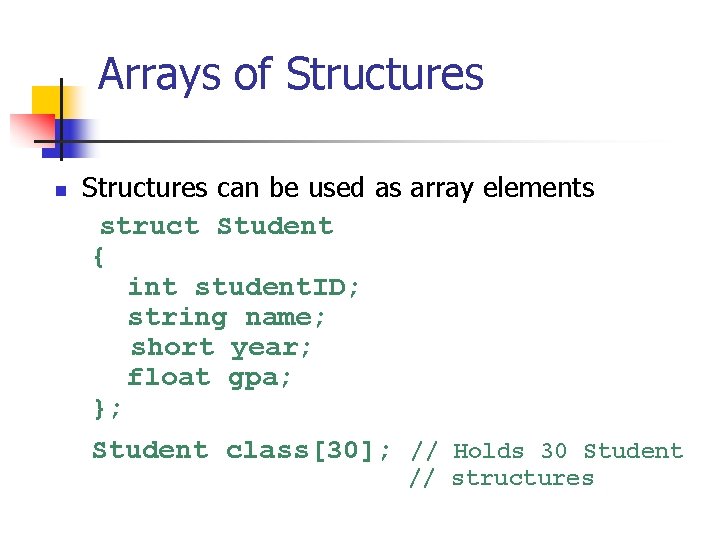
Arrays of Structures n Structures can be used as array elements struct Student { int student. ID; string name; short year; float gpa; }; Student class[30]; // Holds 30 Student // structures
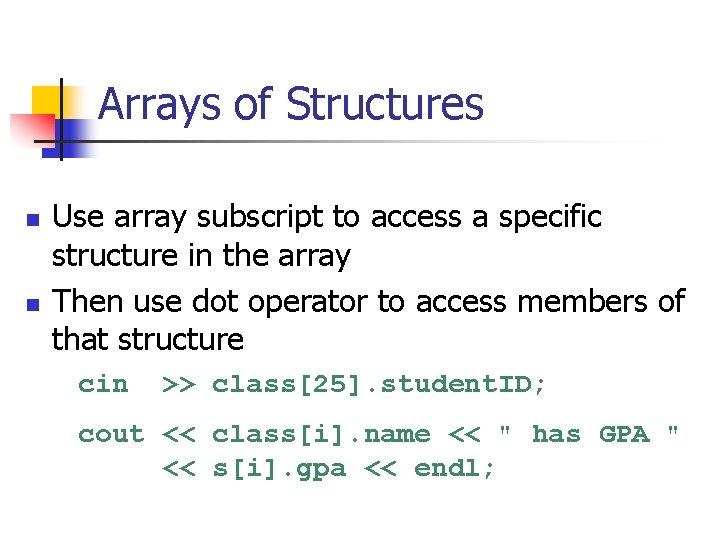
Arrays of Structures n n Use array subscript to access a specific structure in the array Then use dot operator to access members of that structure cin >> class[25]. student. ID; cout << class[i]. name << " has GPA " << s[i]. gpa << endl;
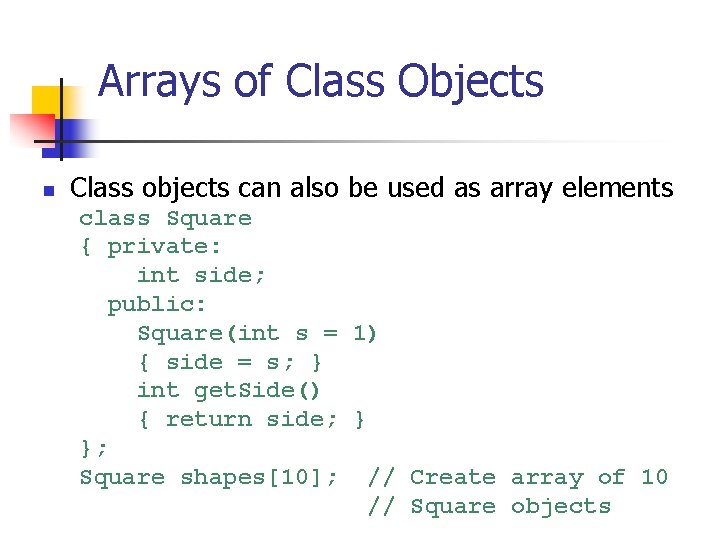
Arrays of Class Objects n Class objects can also be used as array elements class Square { private: int side; public: Square(int s = 1) { side = s; } int get. Side() { return side; } }; Square shapes[10]; // Create array of 10 // Square objects
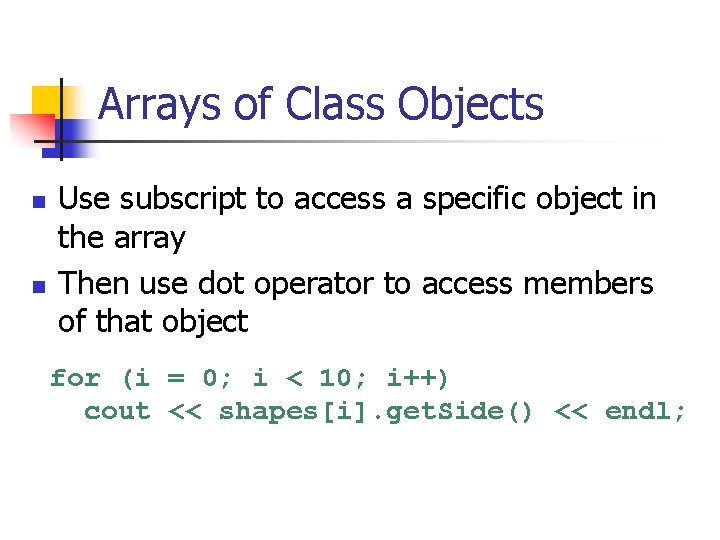
Arrays of Class Objects n n Use subscript to access a specific object in the array Then use dot operator to access members of that object for (i = 0; i < 10; i++) cout << shapes[i]. get. Side() << endl;
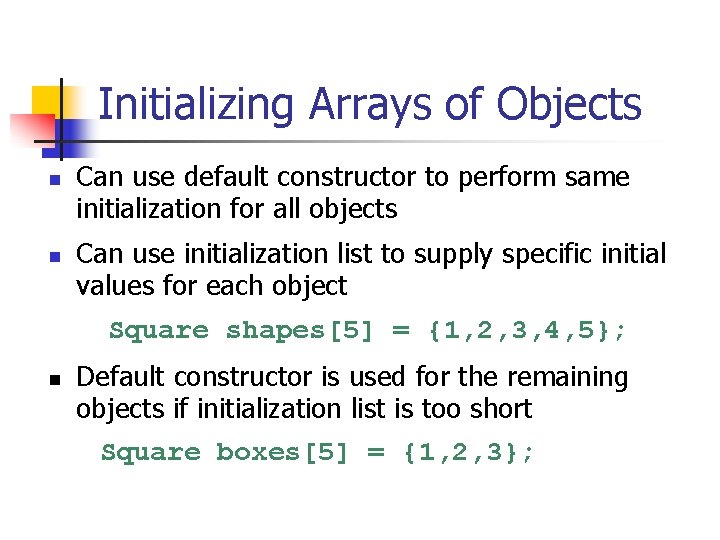
Initializing Arrays of Objects n n Can use default constructor to perform same initialization for all objects Can use initialization list to supply specific initial values for each object Square shapes[5] = {1, 2, 3, 4, 5}; n Default constructor is used for the remaining objects if initialization list is too short Square boxes[5] = {1, 2, 3};
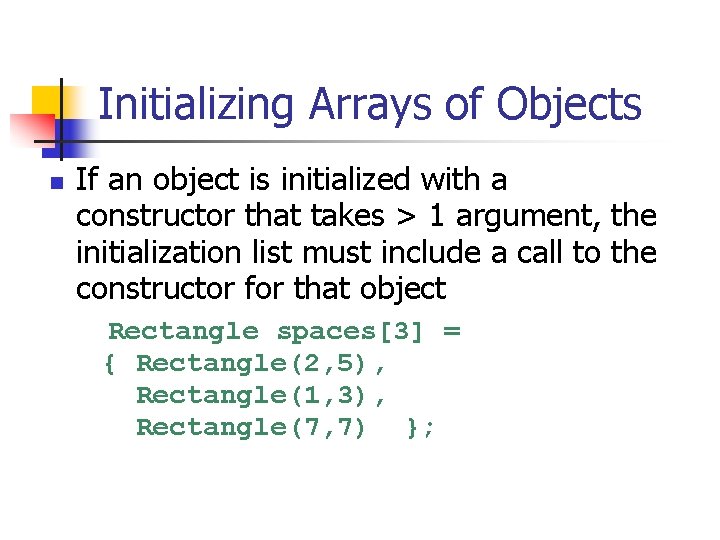
Initializing Arrays of Objects n If an object is initialized with a constructor that takes > 1 argument, the initialization list must include a call to the constructor for that object Rectangle spaces[3] = { Rectangle(2, 5), Rectangle(1, 3), Rectangle(7, 7) };
Western values vs eastern values
Bruce maglino workplace values
An individual's enduring tendency to feel
It is a symbol with two possible values, 0 and 1.
Human values definition
Multiple baseline vs multiple probe design
Example of mimd
Chapter 15 section 2 the second new deal takes hold
Parallel arrays in data structure
Array of arrays c++
Java array operations
Veteork
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Ejemplos de arreglos unidimensionales en java
Arreglos bidimensionales en java
Arrays mips
Polynomial representation using array in c
Assembly array of strings
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Advantages of array
How many arrays in 24
Pascal 2d array
Mips array example
Creating arrays matlab
Arrays as adt
Partially filled arrays
Redundant arrays of independent disks
Python list of arrays
Arrays
Day 3: arrays
Raid redundant array of inexpensive disks
Small basic array examples
Disadvantages of dynamic memory allocation in c
Microled arrays
Are vectors dynamic arrays
Facts about arrays
You were the word in the beginning
Will your anchor hold in the storms of life
Hold your hand up high lyrics
The second coming structure
Sdb student required info mdc hold
Sample and hold spiegazione
Instrument hold line