Arrays Arrays are data structures that hold data
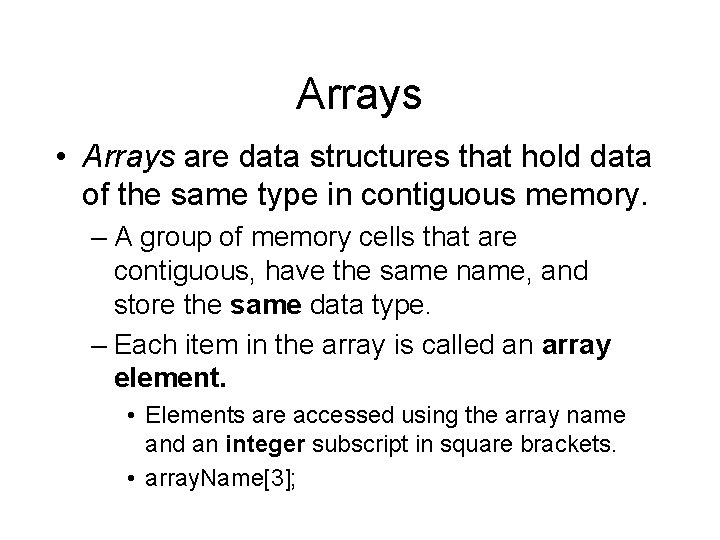
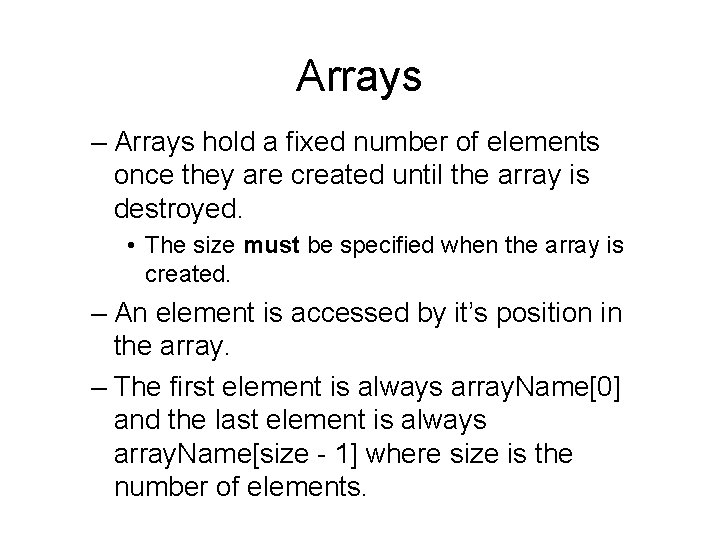
![Arrays double array. Of. Numbers[] = new double[13]; 0 1 2 3 4 5 Arrays double array. Of. Numbers[] = new double[13]; 0 1 2 3 4 5](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-3.jpg)
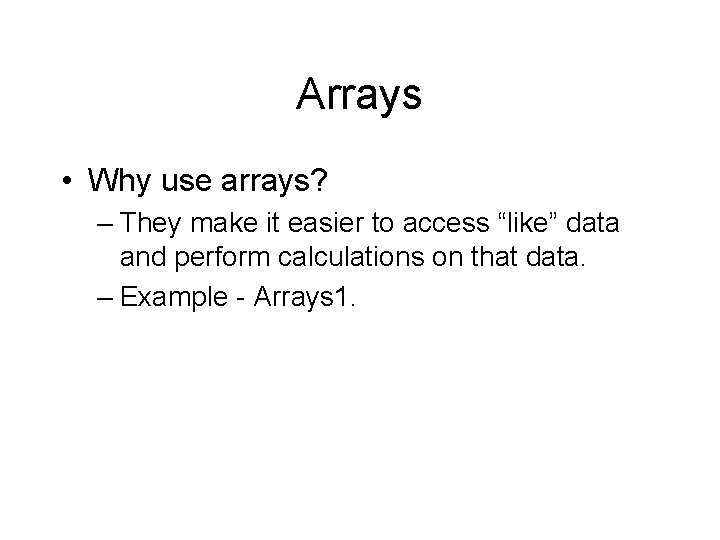
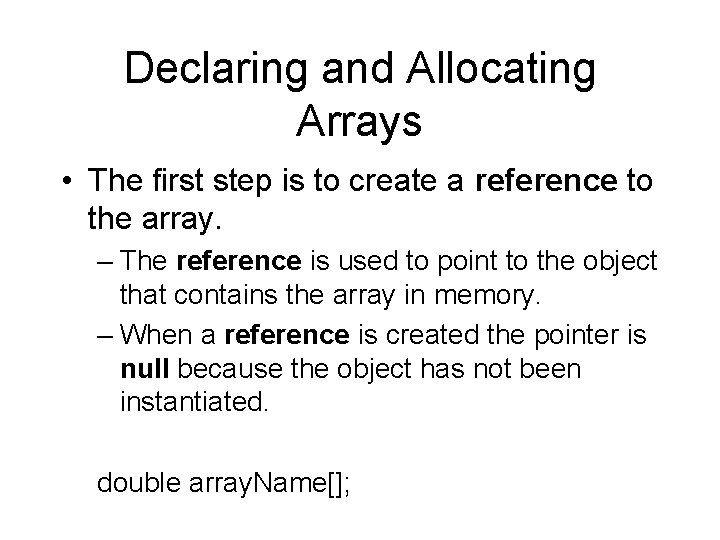
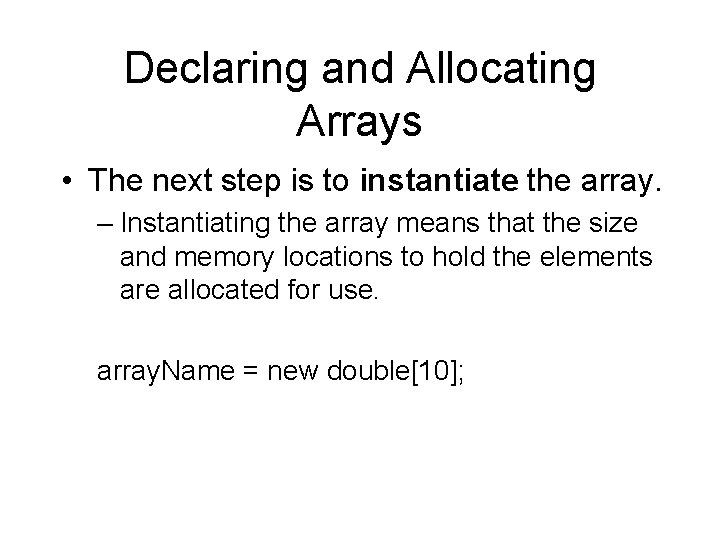
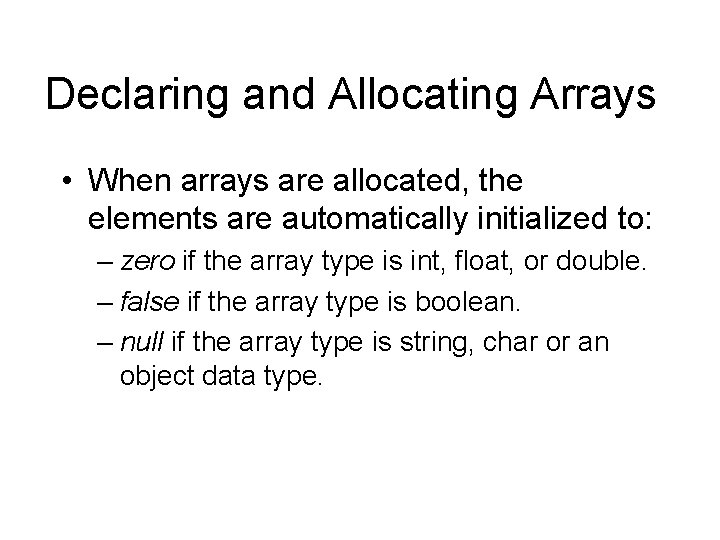
![Declaring and Allocating Arrays double array. Of. Numbers[] = new double[13]; double array. Of. Declaring and Allocating Arrays double array. Of. Numbers[] = new double[13]; double array. Of.](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-8.jpg)
![Declaring and Allocating Arrays Point array. Of. Points[] = new Point[15]; Point array. Of. Declaring and Allocating Arrays Point array. Of. Points[] = new Point[15]; Point array. Of.](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-9.jpg)
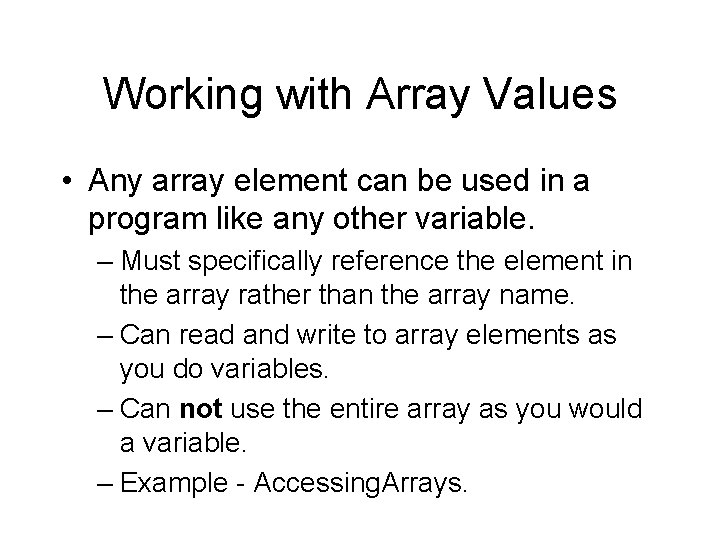
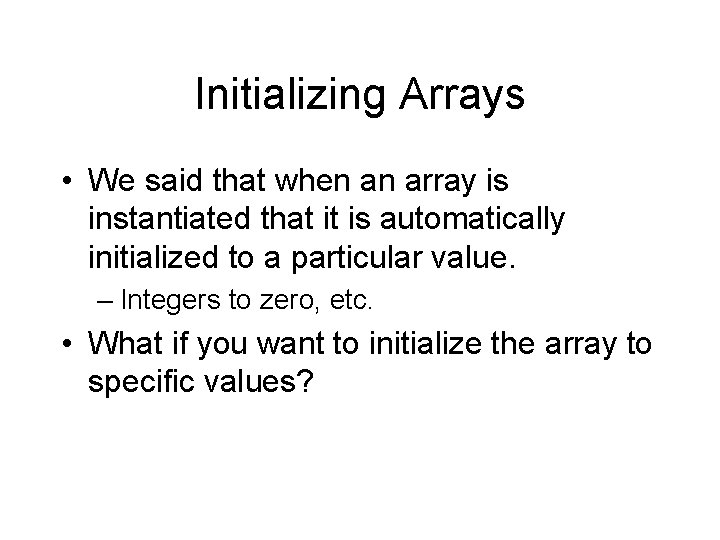
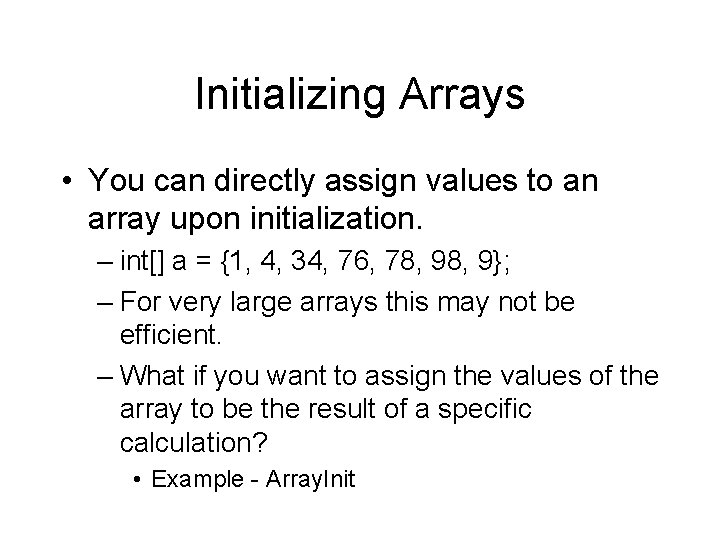
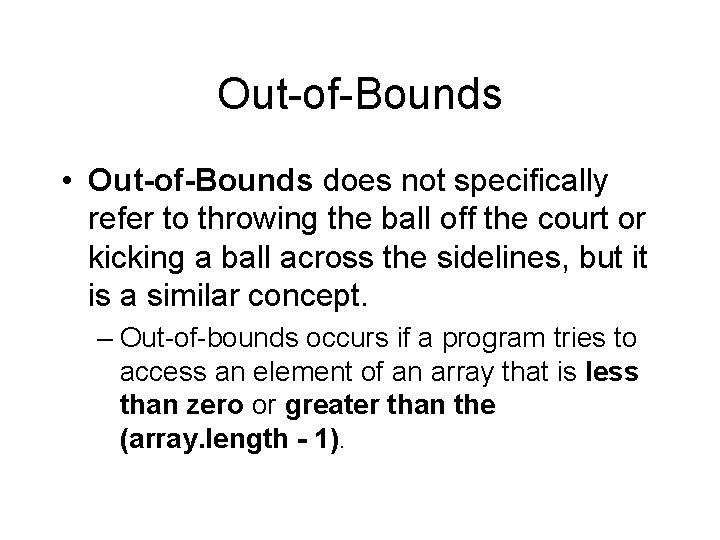
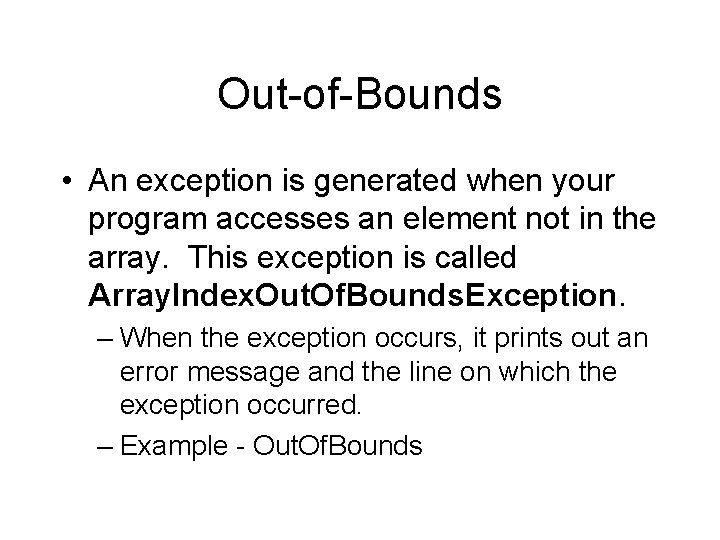
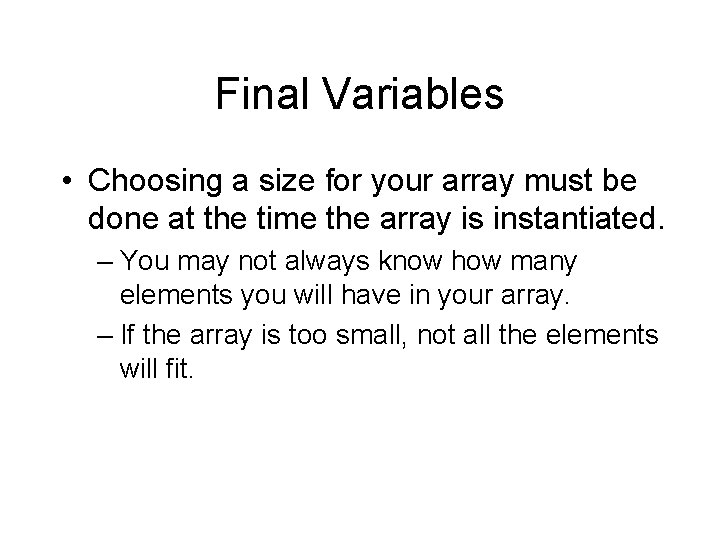
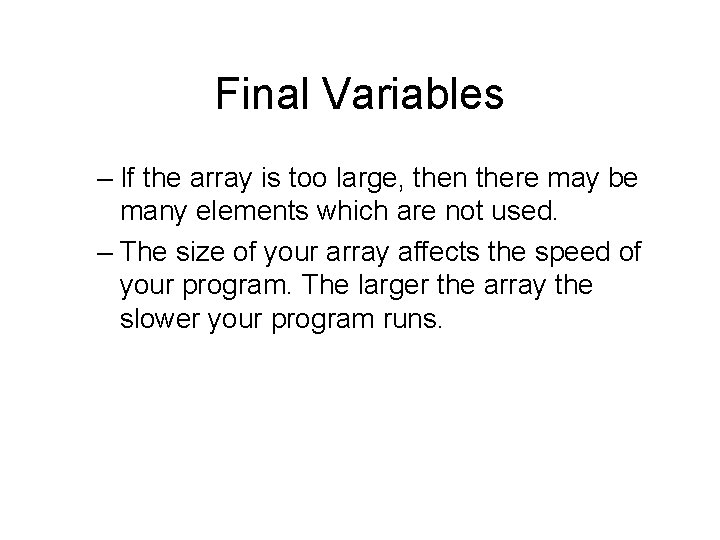
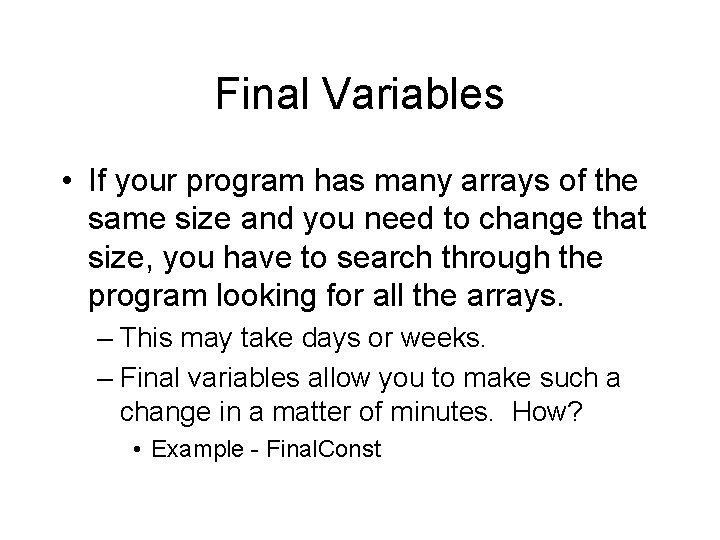
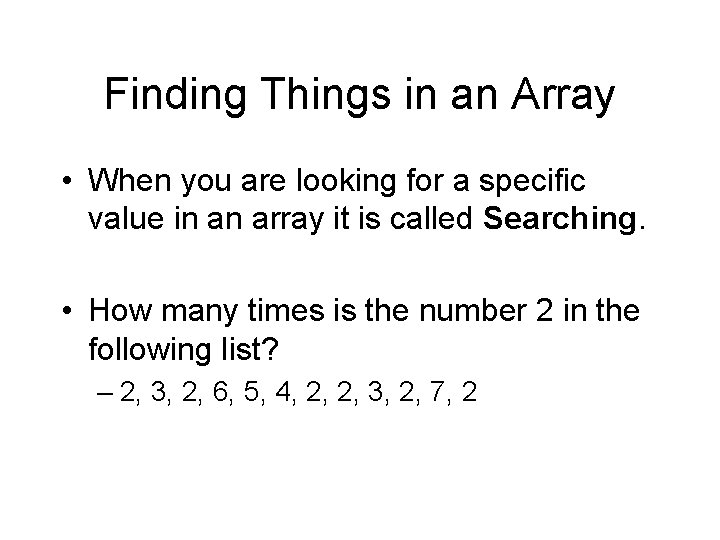
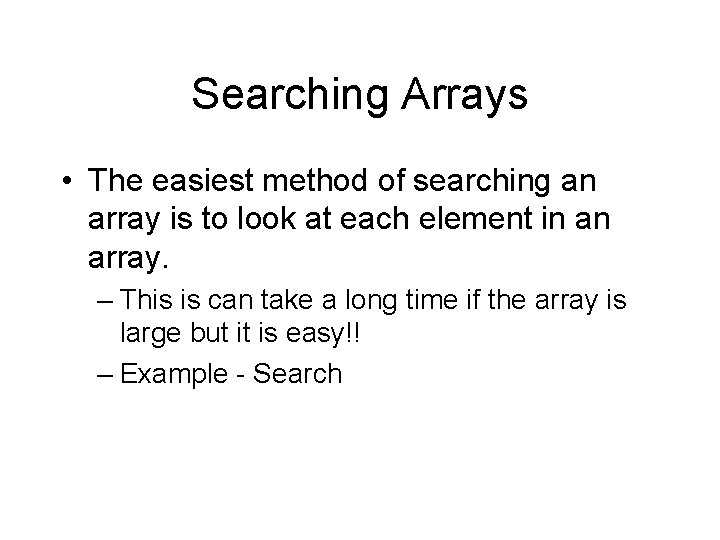
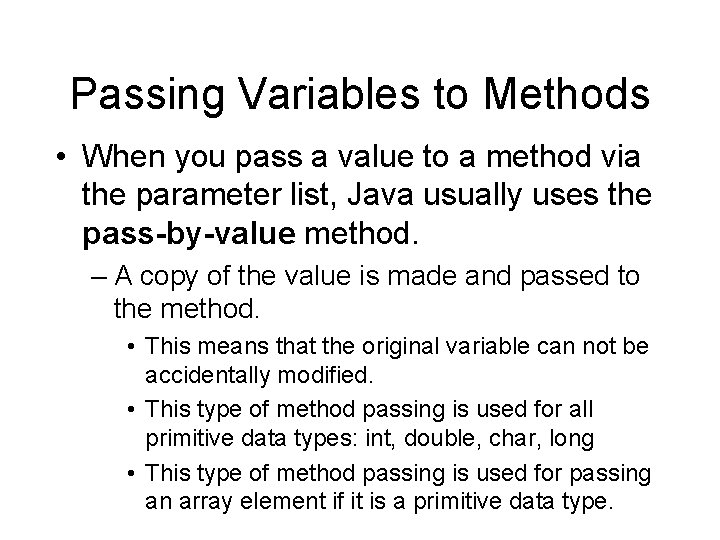
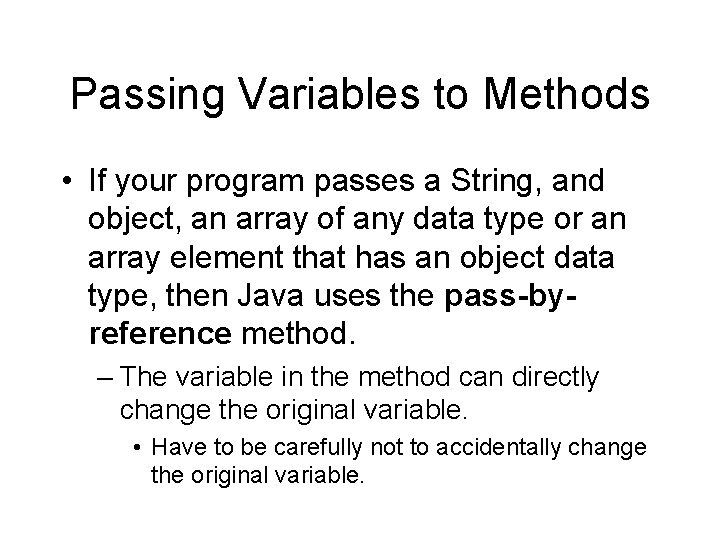
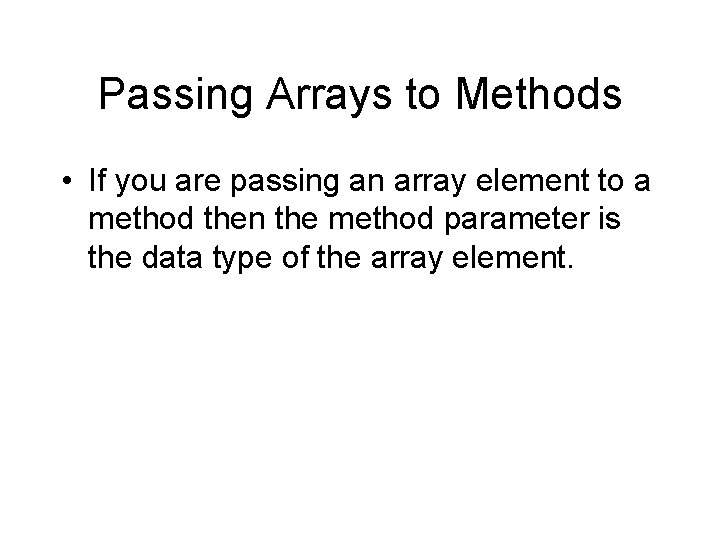
![Passing Arrays to Methods int numbers[] = {5, 6, 34, 45}; Point points[] = Passing Arrays to Methods int numbers[] = {5, 6, 34, 45}; Point points[] =](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-23.jpg)
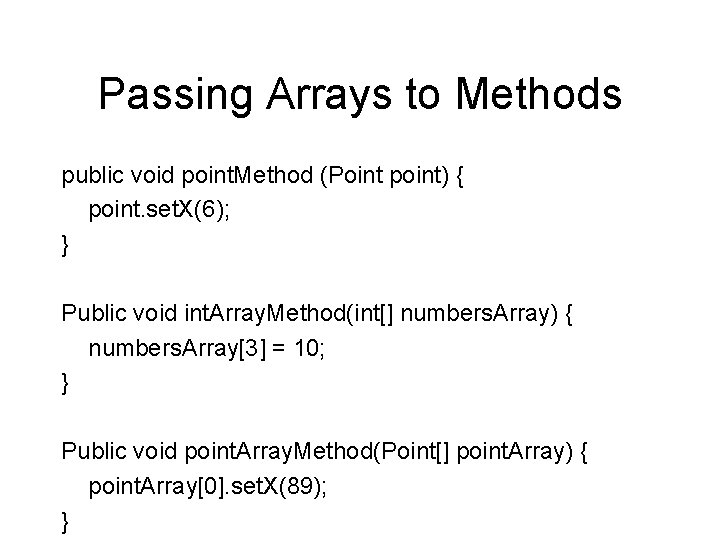
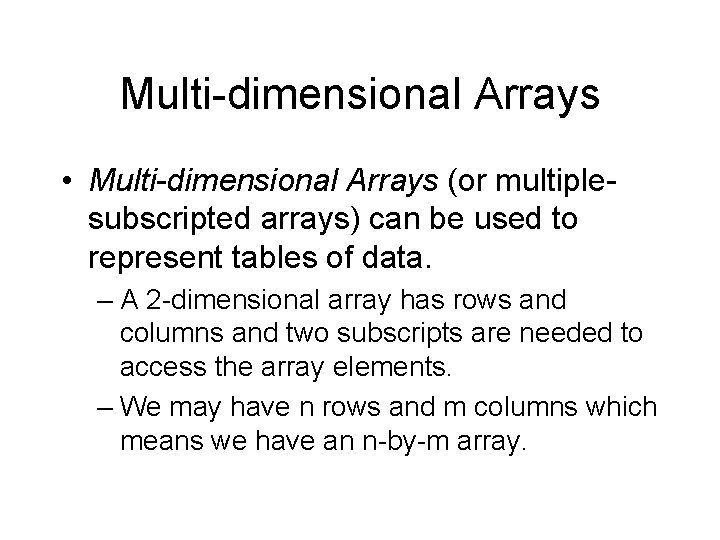
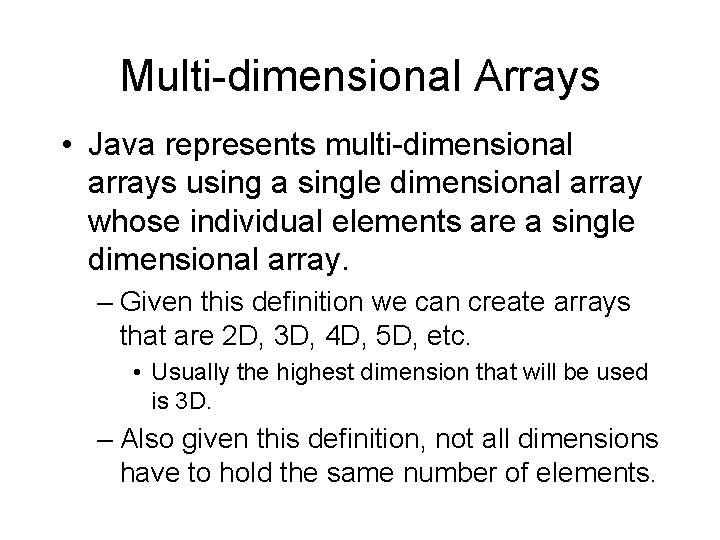
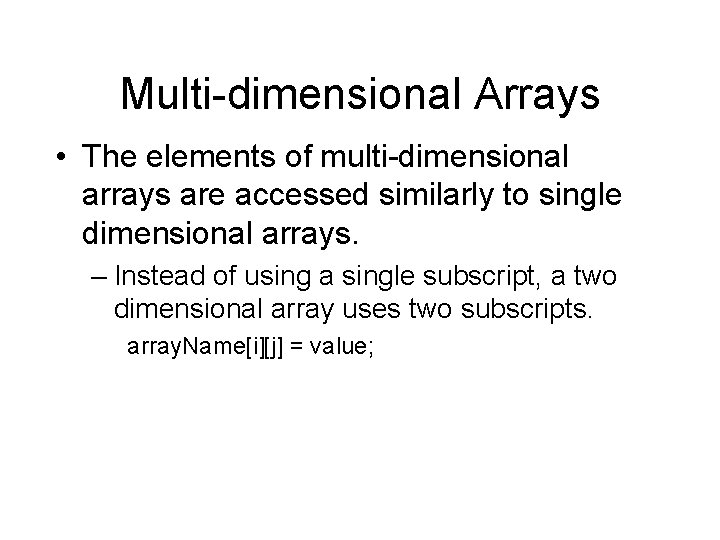
![Multi-dimensional Arrays Int array. Of. Nums[][] = new int[3][4]; Col 0 Col 1 Col Multi-dimensional Arrays Int array. Of. Nums[][] = new int[3][4]; Col 0 Col 1 Col](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-28.jpg)
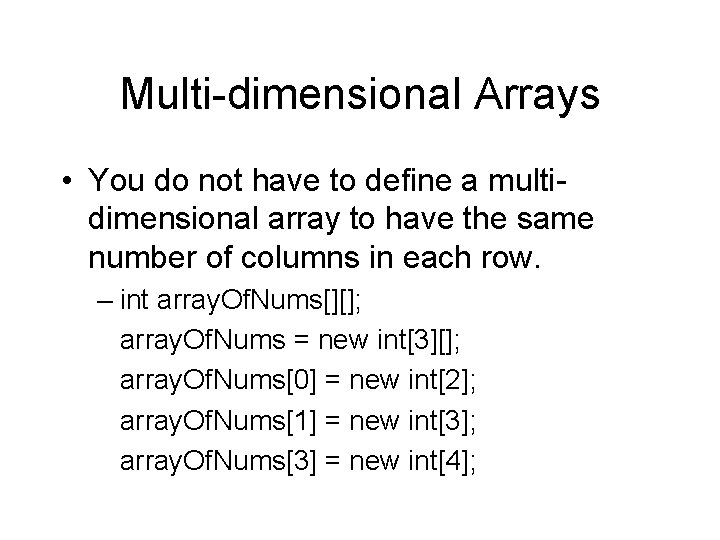
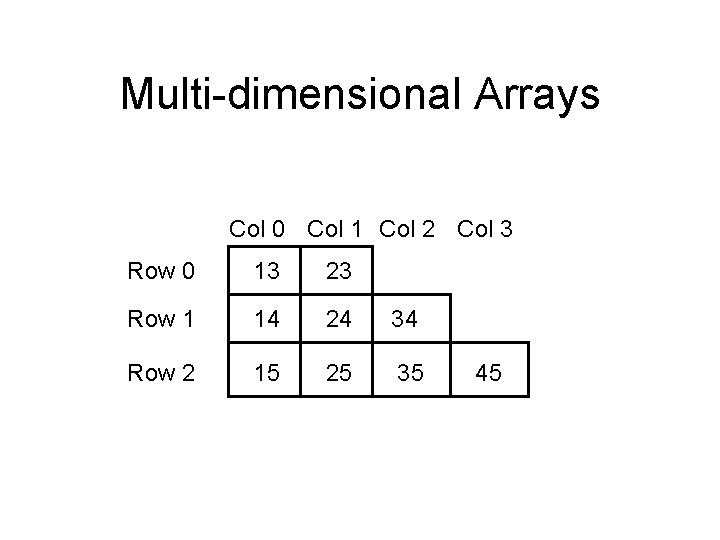
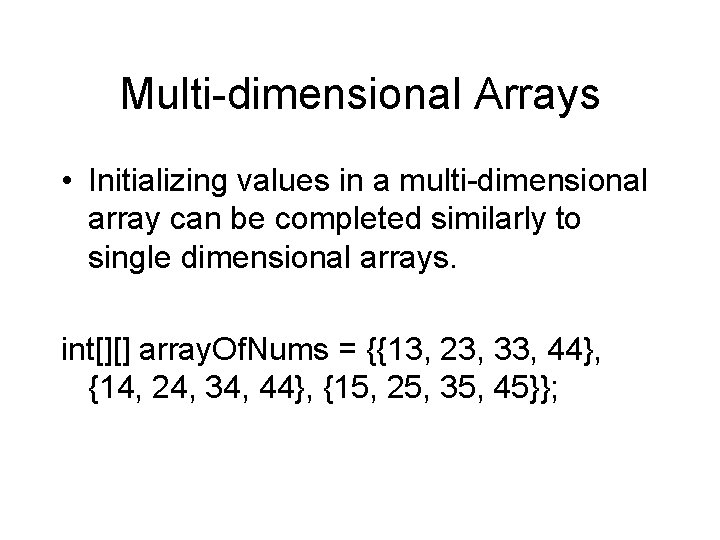
![Multi-dimensional Arrays double numbers[][] = new double[6][5]; for (int i = 0; i < Multi-dimensional Arrays double numbers[][] = new double[6][5]; for (int i = 0; i <](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-32.jpg)
- Slides: 32
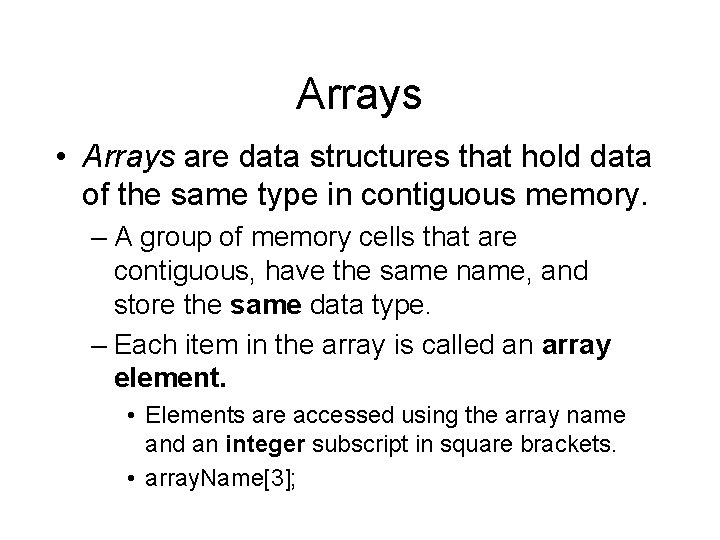
Arrays • Arrays are data structures that hold data of the same type in contiguous memory. – A group of memory cells that are contiguous, have the same name, and store the same data type. – Each item in the array is called an array element. • Elements are accessed using the array name and an integer subscript in square brackets. • array. Name[3];
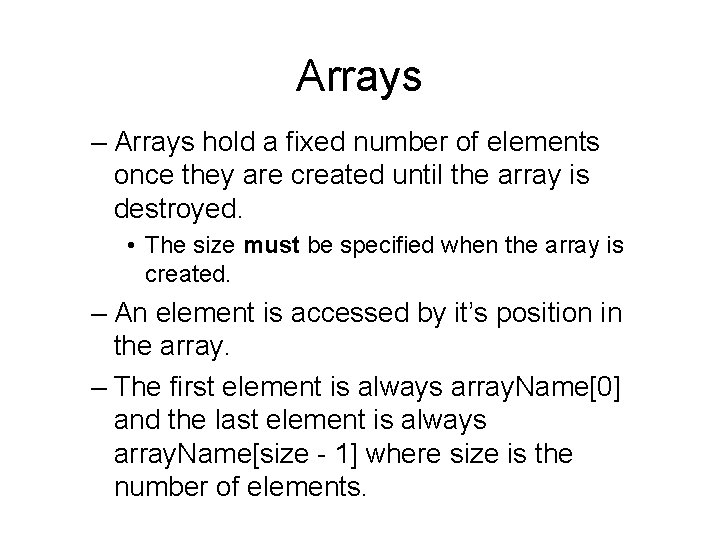
Arrays – Arrays hold a fixed number of elements once they are created until the array is destroyed. • The size must be specified when the array is created. – An element is accessed by it’s position in the array. – The first element is always array. Name[0] and the last element is always array. Name[size - 1] where size is the number of elements.
![Arrays double array Of Numbers new double13 0 1 2 3 4 5 Arrays double array. Of. Numbers[] = new double[13]; 0 1 2 3 4 5](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-3.jpg)
Arrays double array. Of. Numbers[] = new double[13]; 0 1 2 3 4 5 6 7 8 9 10 11 12 4. 5 4. 2 9. 9 8. 2 4. 5 0. 0 0. 1 4. 5 5. 2 0. 3 2. 0 1. 3 7. 8 array. Of. Numbers. length;
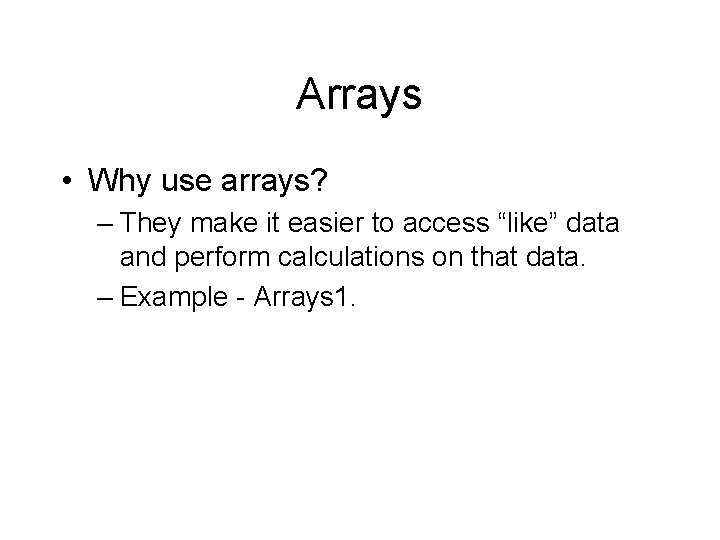
Arrays • Why use arrays? – They make it easier to access “like” data and perform calculations on that data. – Example - Arrays 1.
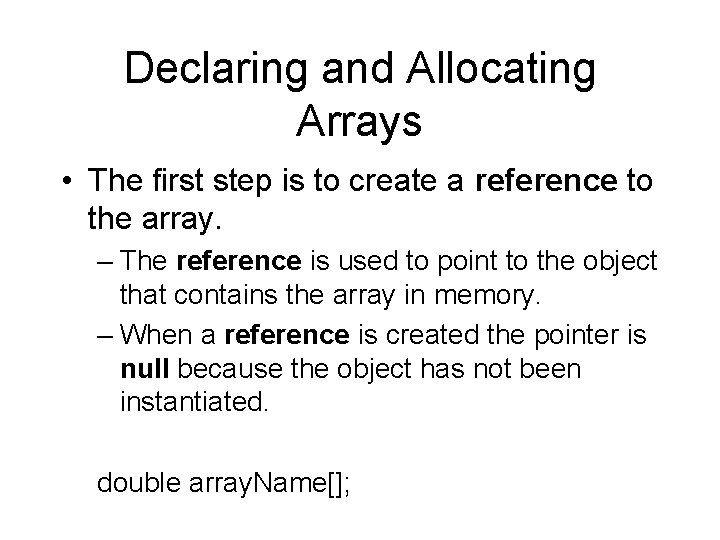
Declaring and Allocating Arrays • The first step is to create a reference to the array. – The reference is used to point to the object that contains the array in memory. – When a reference is created the pointer is null because the object has not been instantiated. double array. Name[];
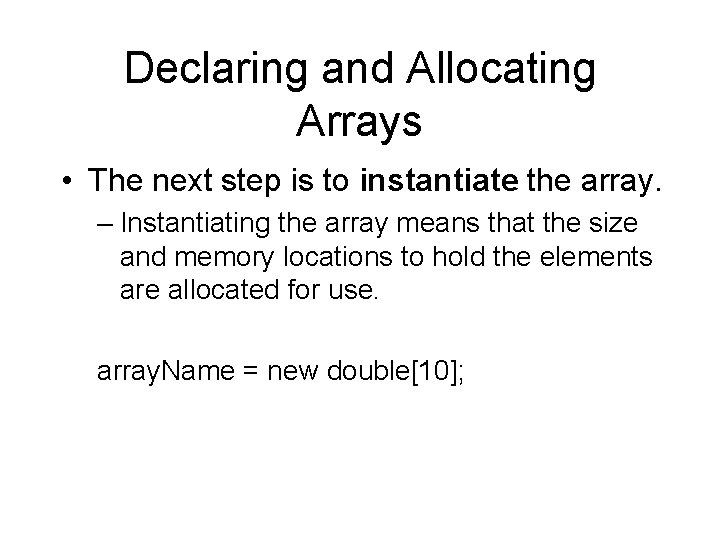
Declaring and Allocating Arrays • The next step is to instantiate the array. – Instantiating the array means that the size and memory locations to hold the elements are allocated for use. array. Name = new double[10];
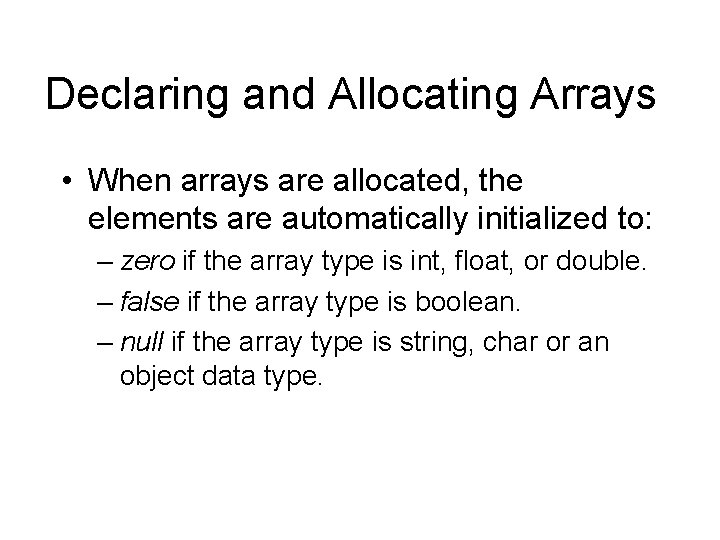
Declaring and Allocating Arrays • When arrays are allocated, the elements are automatically initialized to: – zero if the array type is int, float, or double. – false if the array type is boolean. – null if the array type is string, char or an object data type.
![Declaring and Allocating Arrays double array Of Numbers new double13 double array Of Declaring and Allocating Arrays double array. Of. Numbers[] = new double[13]; double array. Of.](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-8.jpg)
Declaring and Allocating Arrays double array. Of. Numbers[] = new double[13]; double array. Of. Numbers[]; array. Of. Numbers = new double[13]; double[] array. Of. Numbers = {1. 2, 3. 4, 5. 3, 2. 1, 3. 4, 2. 4};
![Declaring and Allocating Arrays Point array Of Points new Point15 Point array Of Declaring and Allocating Arrays Point array. Of. Points[] = new Point[15]; Point array. Of.](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-9.jpg)
Declaring and Allocating Arrays Point array. Of. Points[] = new Point[15]; Point array. Of. Points[]; array. Of. Points = new Point[15];
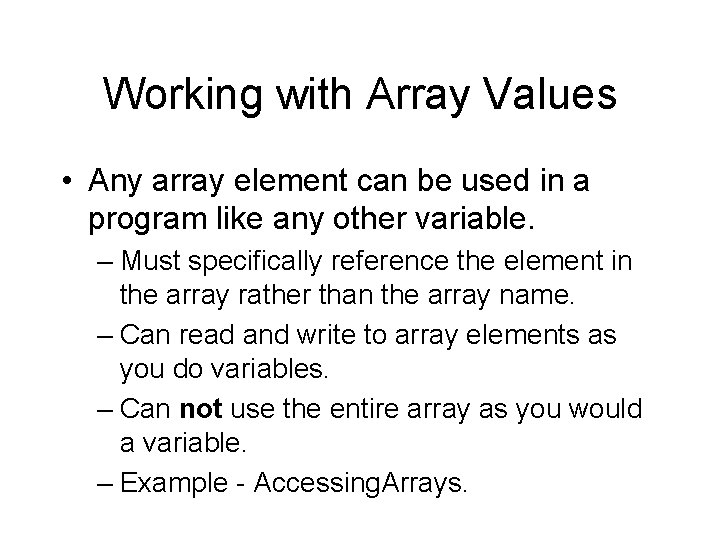
Working with Array Values • Any array element can be used in a program like any other variable. – Must specifically reference the element in the array rather than the array name. – Can read and write to array elements as you do variables. – Can not use the entire array as you would a variable. – Example - Accessing. Arrays.
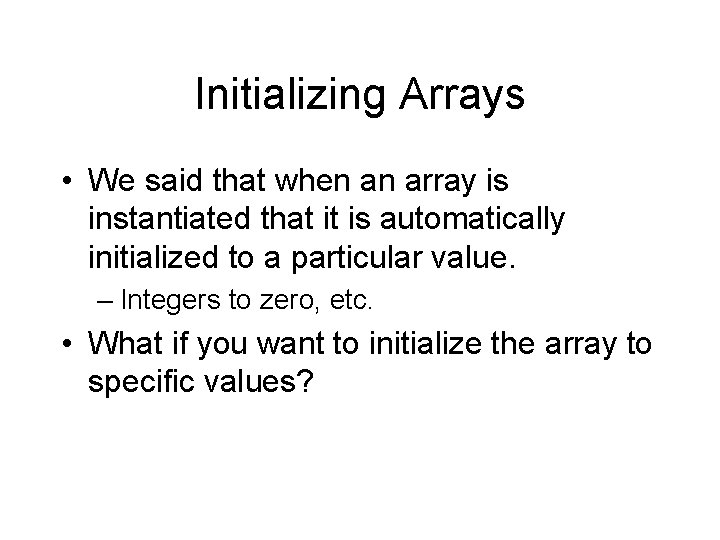
Initializing Arrays • We said that when an array is instantiated that it is automatically initialized to a particular value. – Integers to zero, etc. • What if you want to initialize the array to specific values?
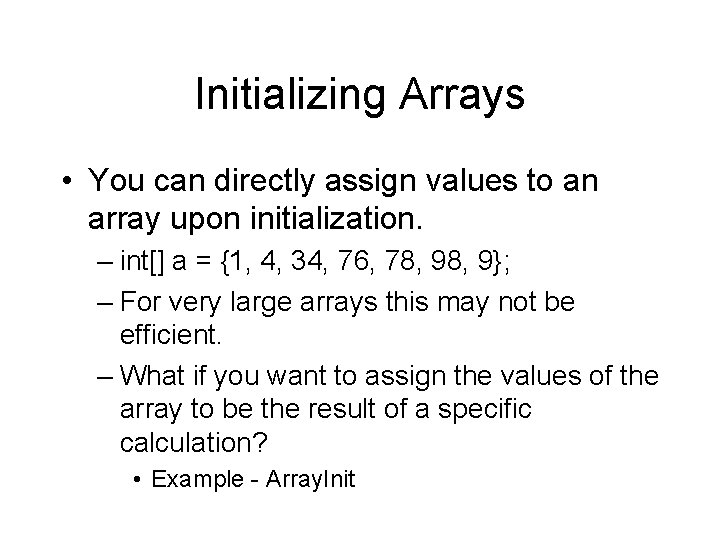
Initializing Arrays • You can directly assign values to an array upon initialization. – int[] a = {1, 4, 34, 76, 78, 9}; – For very large arrays this may not be efficient. – What if you want to assign the values of the array to be the result of a specific calculation? • Example - Array. Init
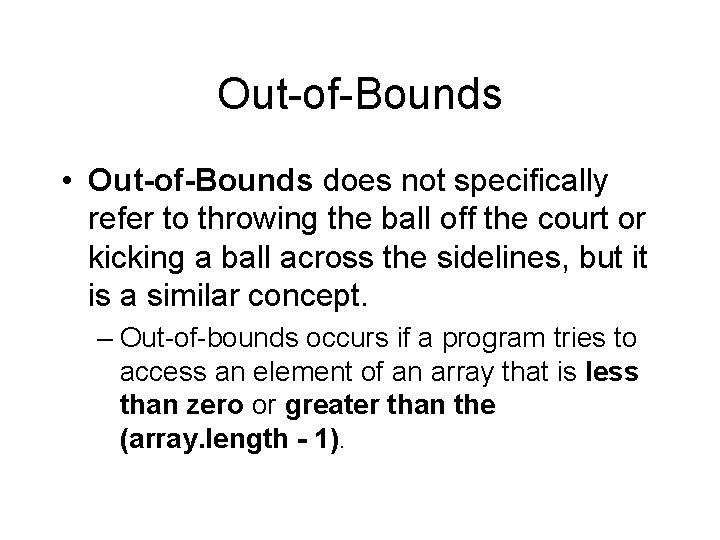
Out-of-Bounds • Out-of-Bounds does not specifically refer to throwing the ball off the court or kicking a ball across the sidelines, but it is a similar concept. – Out-of-bounds occurs if a program tries to access an element of an array that is less than zero or greater than the (array. length - 1).
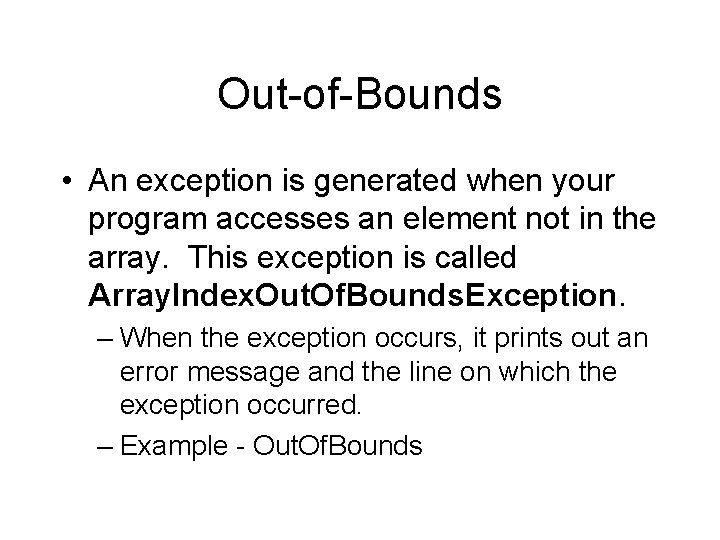
Out-of-Bounds • An exception is generated when your program accesses an element not in the array. This exception is called Array. Index. Out. Of. Bounds. Exception. – When the exception occurs, it prints out an error message and the line on which the exception occurred. – Example - Out. Of. Bounds
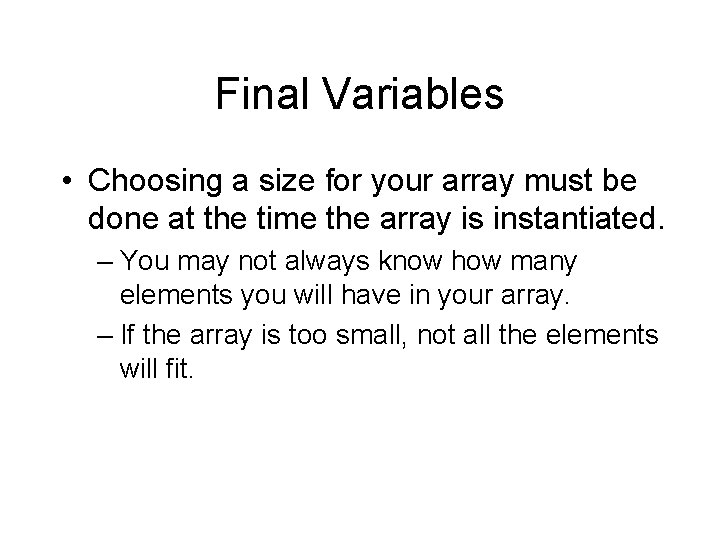
Final Variables • Choosing a size for your array must be done at the time the array is instantiated. – You may not always know how many elements you will have in your array. – If the array is too small, not all the elements will fit.
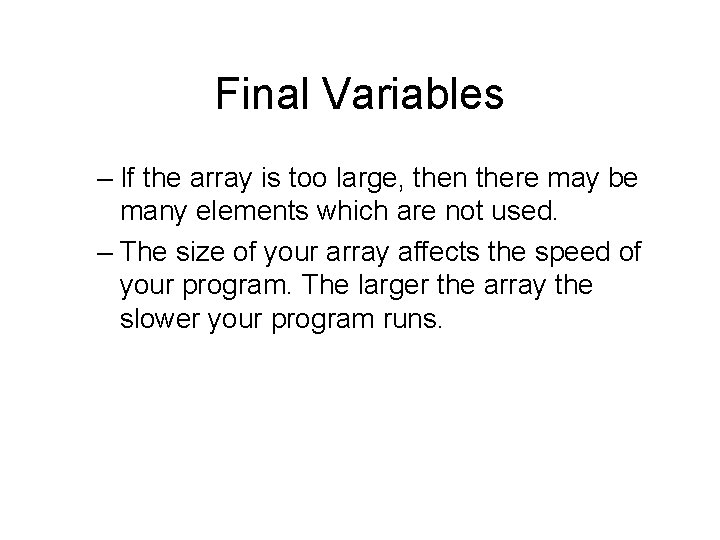
Final Variables – If the array is too large, then there may be many elements which are not used. – The size of your array affects the speed of your program. The larger the array the slower your program runs.
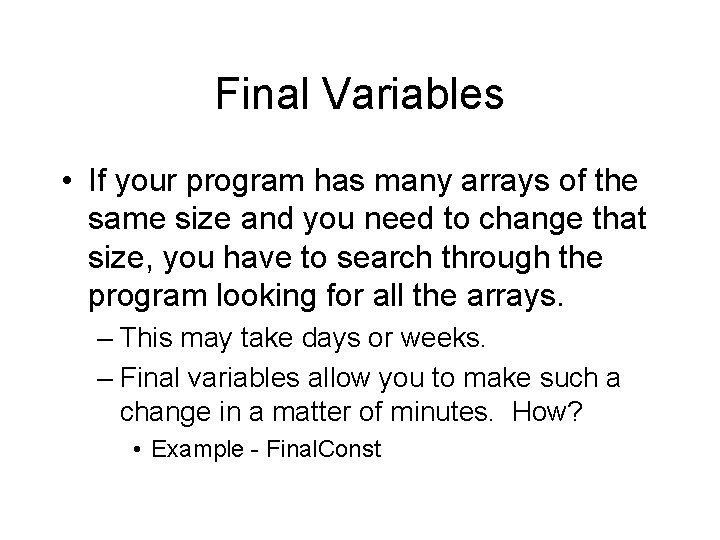
Final Variables • If your program has many arrays of the same size and you need to change that size, you have to search through the program looking for all the arrays. – This may take days or weeks. – Final variables allow you to make such a change in a matter of minutes. How? • Example - Final. Const
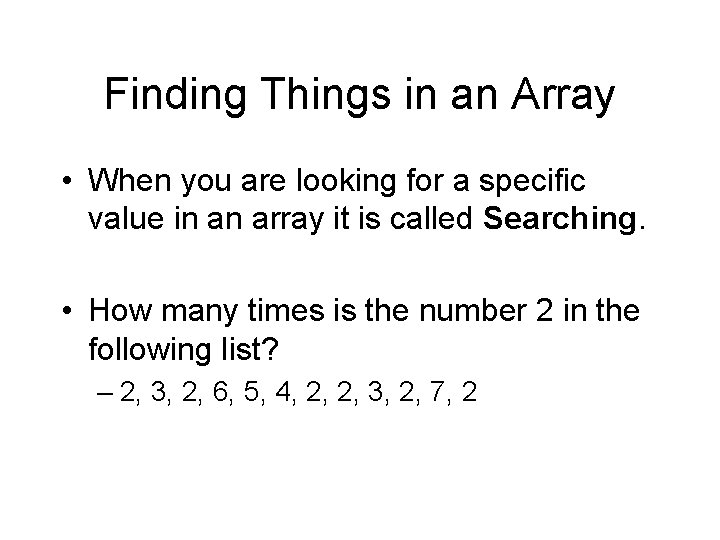
Finding Things in an Array • When you are looking for a specific value in an array it is called Searching. • How many times is the number 2 in the following list? – 2, 3, 2, 6, 5, 4, 2, 2, 3, 2, 7, 2
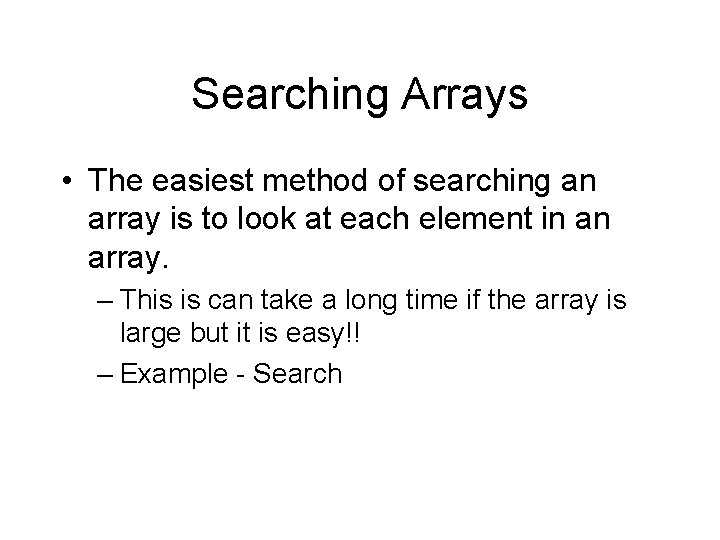
Searching Arrays • The easiest method of searching an array is to look at each element in an array. – This is can take a long time if the array is large but it is easy!! – Example - Search
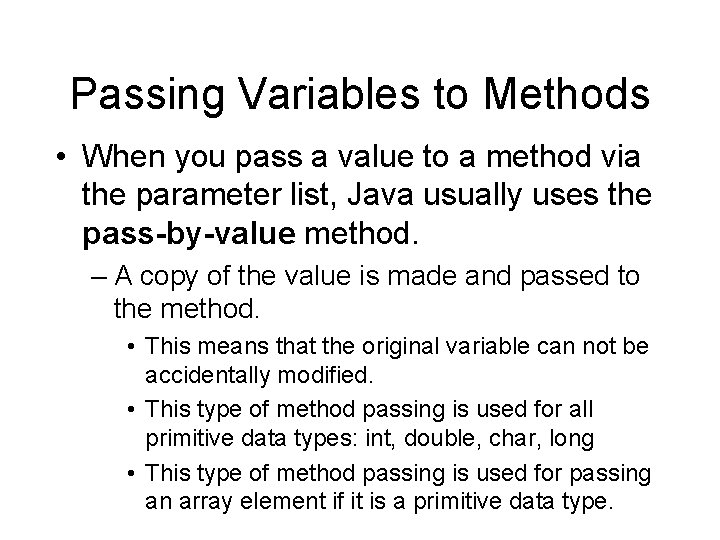
Passing Variables to Methods • When you pass a value to a method via the parameter list, Java usually uses the pass-by-value method. – A copy of the value is made and passed to the method. • This means that the original variable can not be accidentally modified. • This type of method passing is used for all primitive data types: int, double, char, long • This type of method passing is used for passing an array element if it is a primitive data type.
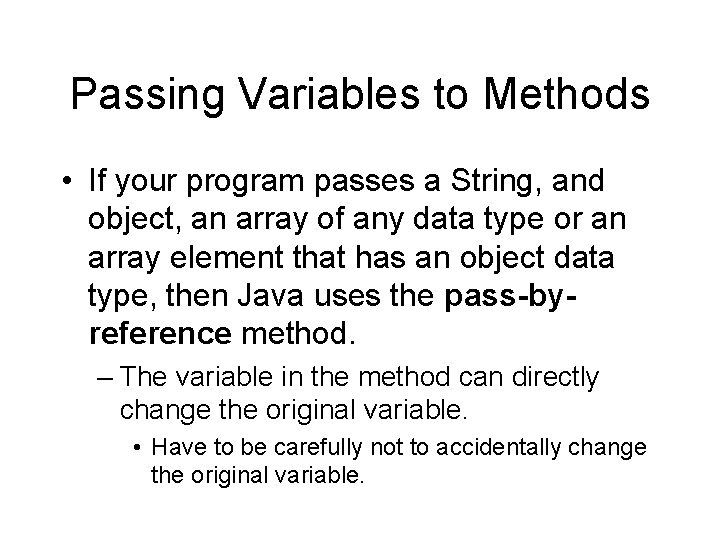
Passing Variables to Methods • If your program passes a String, and object, an array of any data type or an array element that has an object data type, then Java uses the pass-byreference method. – The variable in the method can directly change the original variable. • Have to be carefully not to accidentally change the original variable.
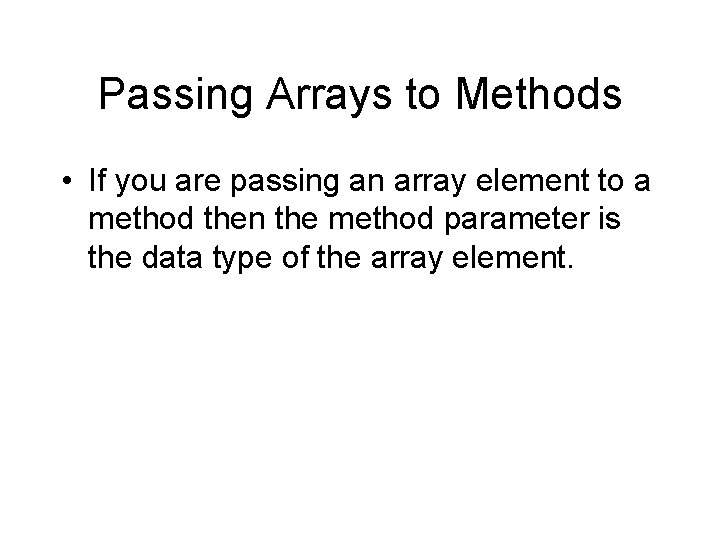
Passing Arrays to Methods • If you are passing an array element to a method then the method parameter is the data type of the array element.
![Passing Arrays to Methods int numbers 5 6 34 45 Point points Passing Arrays to Methods int numbers[] = {5, 6, 34, 45}; Point points[] =](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-23.jpg)
Passing Arrays to Methods int numbers[] = {5, 6, 34, 45}; Point points[] = new Point[4]; int. Method(numbers[1]); point. Method 2(points[2]); int. Array. Method(numbers); point. Array. Method(points); public void int. Method (int number) { number += 4; }
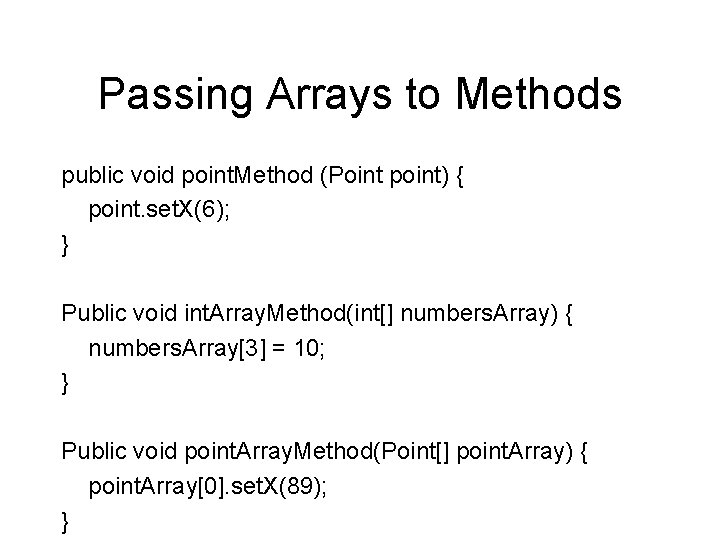
Passing Arrays to Methods public void point. Method (Point point) { point. set. X(6); } Public void int. Array. Method(int[] numbers. Array) { numbers. Array[3] = 10; } Public void point. Array. Method(Point[] point. Array) { point. Array[0]. set. X(89); }
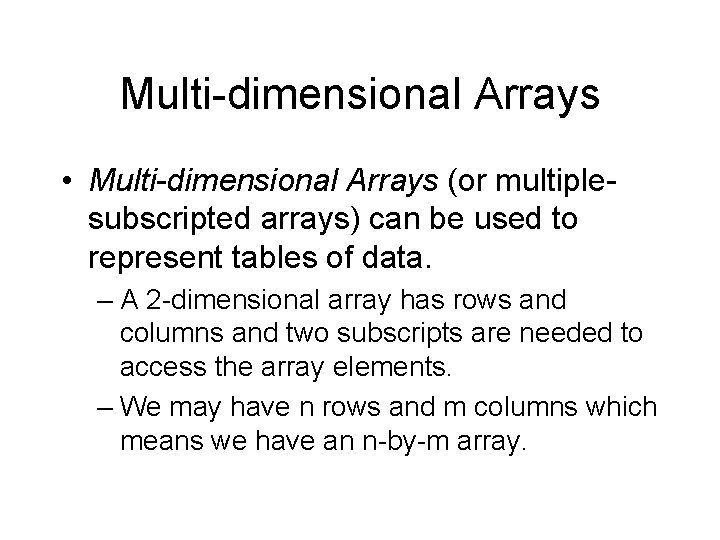
Multi-dimensional Arrays • Multi-dimensional Arrays (or multiplesubscripted arrays) can be used to represent tables of data. – A 2 -dimensional array has rows and columns and two subscripts are needed to access the array elements. – We may have n rows and m columns which means we have an n-by-m array.
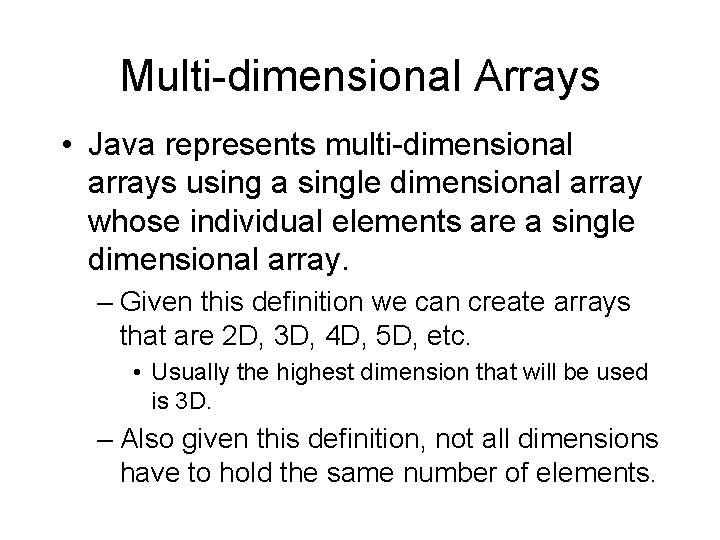
Multi-dimensional Arrays • Java represents multi-dimensional arrays using a single dimensional array whose individual elements are a single dimensional array. – Given this definition we can create arrays that are 2 D, 3 D, 4 D, 5 D, etc. • Usually the highest dimension that will be used is 3 D. – Also given this definition, not all dimensions have to hold the same number of elements.
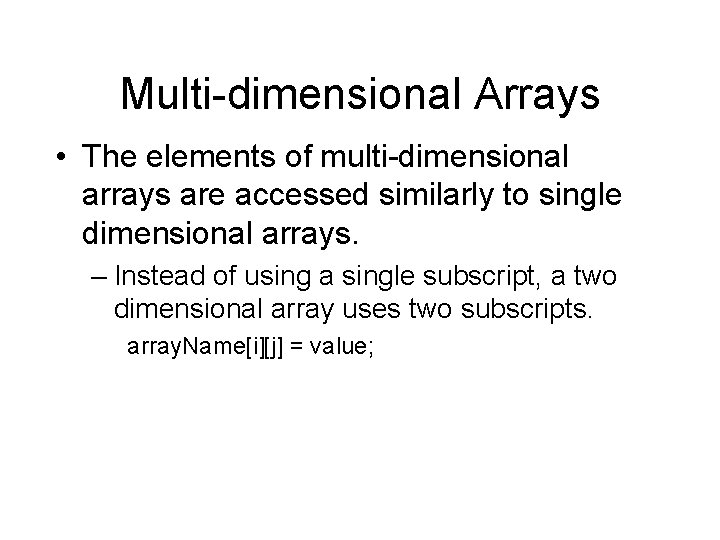
Multi-dimensional Arrays • The elements of multi-dimensional arrays are accessed similarly to single dimensional arrays. – Instead of using a single subscript, a two dimensional array uses two subscripts. array. Name[i][j] = value;
![Multidimensional Arrays Int array Of Nums new int34 Col 0 Col 1 Col Multi-dimensional Arrays Int array. Of. Nums[][] = new int[3][4]; Col 0 Col 1 Col](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-28.jpg)
Multi-dimensional Arrays Int array. Of. Nums[][] = new int[3][4]; Col 0 Col 1 Col 2 Col 3 Row 0 13 23 33 43 Row 1 14 24 34 44 Row 2 15 25 35 45
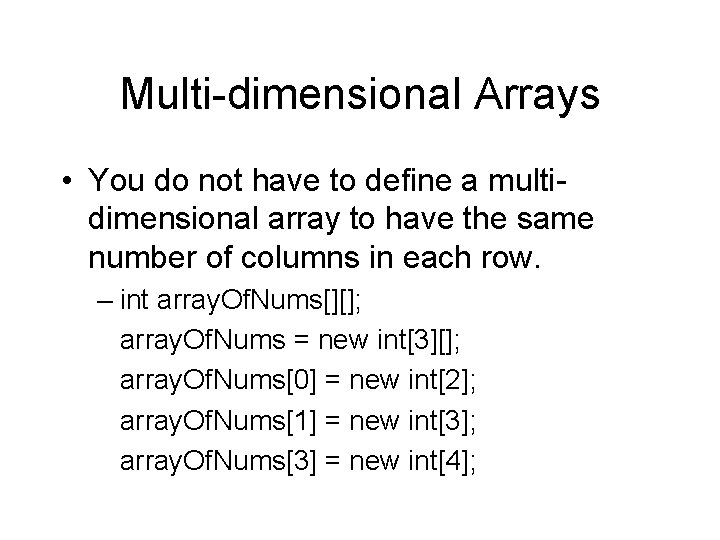
Multi-dimensional Arrays • You do not have to define a multidimensional array to have the same number of columns in each row. – int array. Of. Nums[][]; array. Of. Nums = new int[3][]; array. Of. Nums[0] = new int[2]; array. Of. Nums[1] = new int[3]; array. Of. Nums[3] = new int[4];
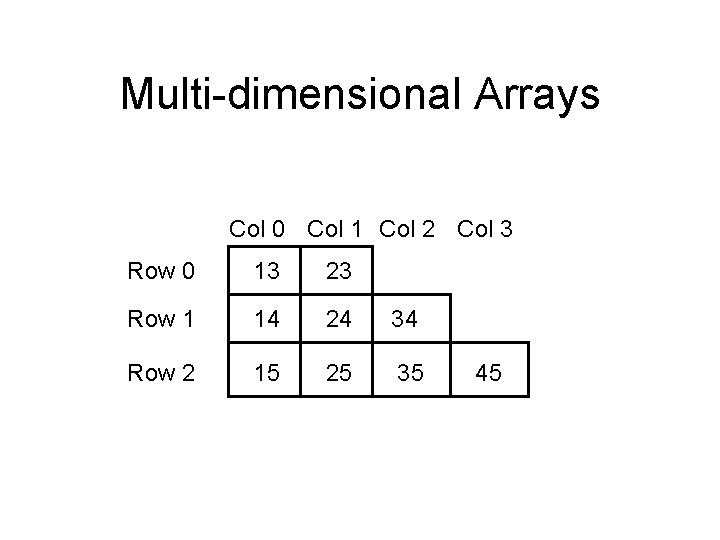
Multi-dimensional Arrays Col 0 Col 1 Col 2 Col 3 Row 0 13 23 Row 1 14 24 34 Row 2 15 25 35 45
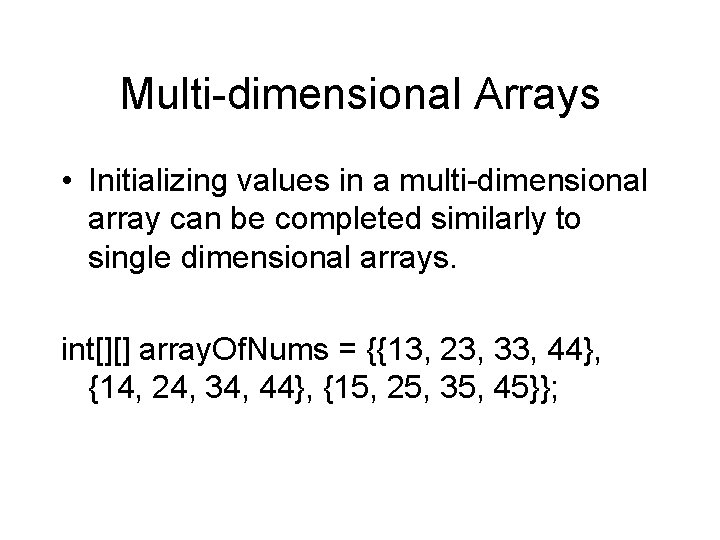
Multi-dimensional Arrays • Initializing values in a multi-dimensional array can be completed similarly to single dimensional arrays. int[][] array. Of. Nums = {{13, 23, 33, 44}, {14, 24, 34, 44}, {15, 25, 35, 45}};
![Multidimensional Arrays double numbers new double65 for int i 0 i Multi-dimensional Arrays double numbers[][] = new double[6][5]; for (int i = 0; i <](https://slidetodoc.com/presentation_image/8de9b868bbc6ef9db2e73d38e56bc578/image-32.jpg)
Multi-dimensional Arrays double numbers[][] = new double[6][5]; for (int i = 0; i < numbers. length; i++) for (int j = 0; j < numbers[0]. length; j++) numbers[i][j] = 2. 0;