104 Lecture Topics Overflow and underflow Logical operations
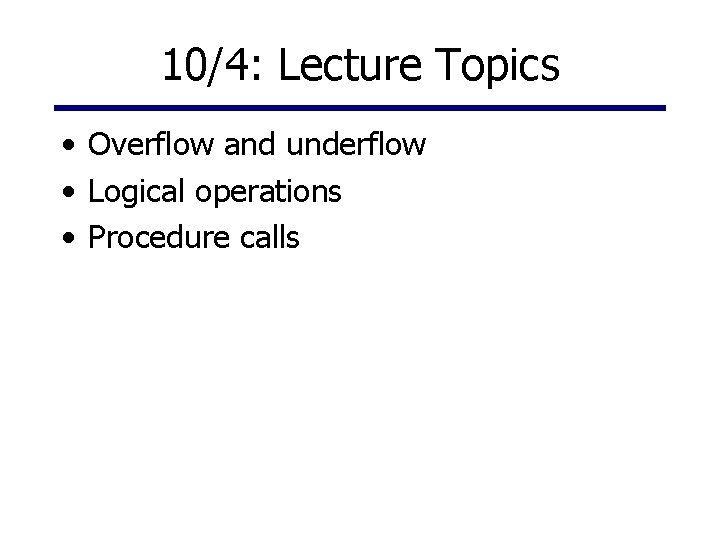
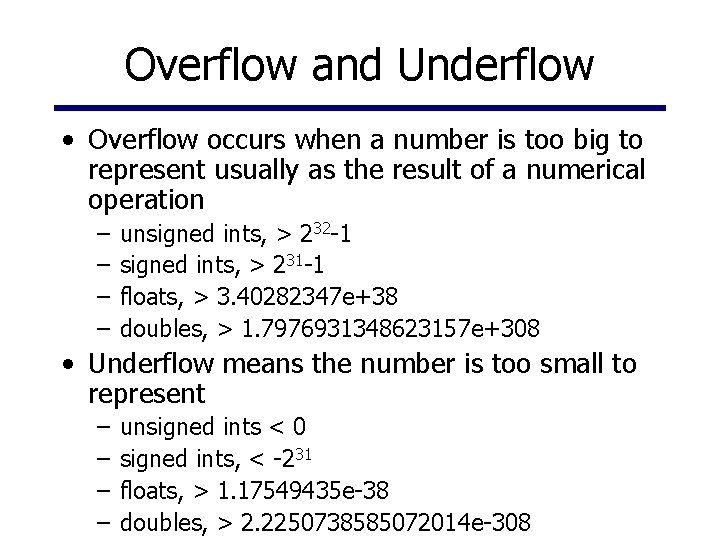
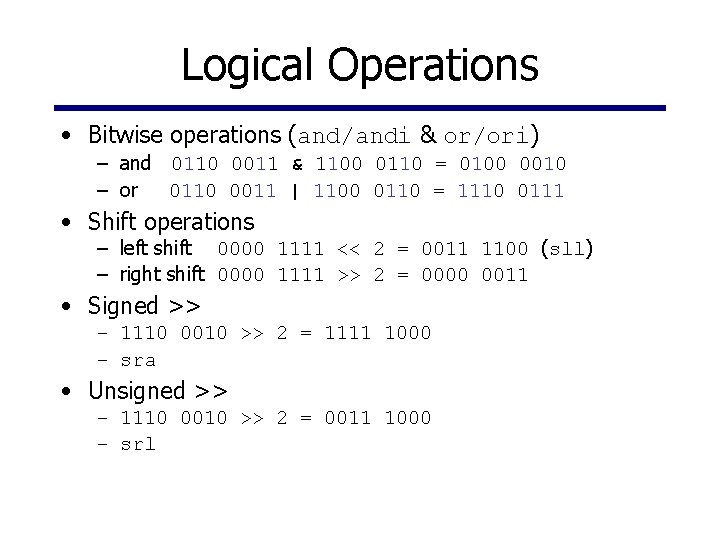
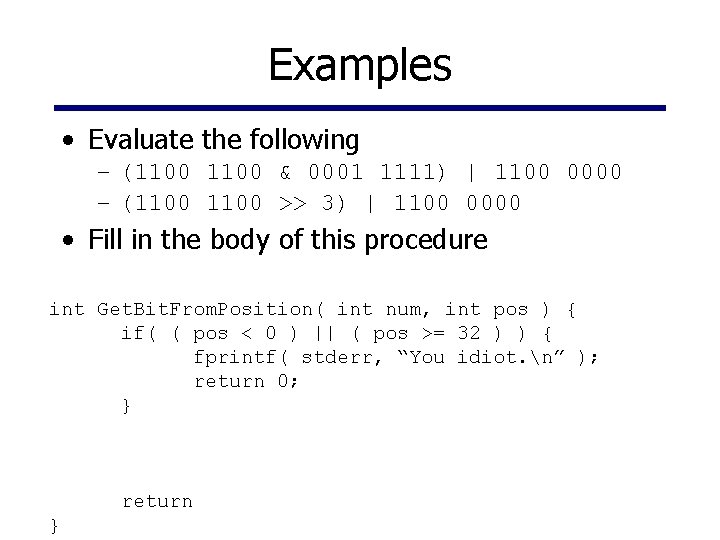
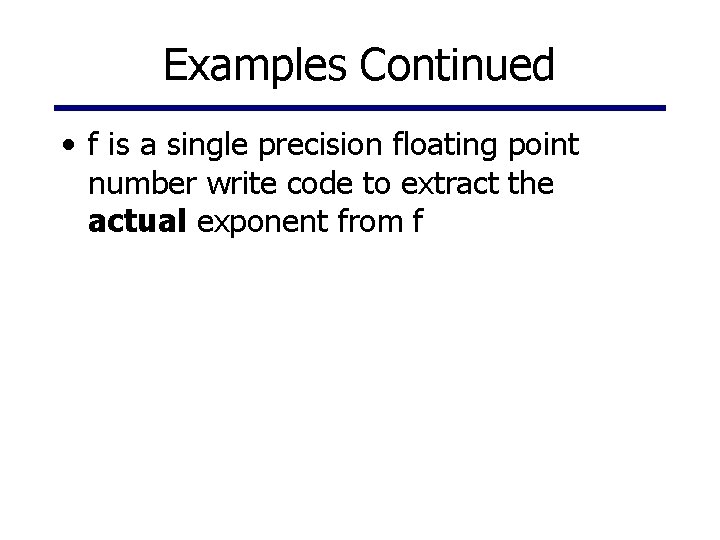
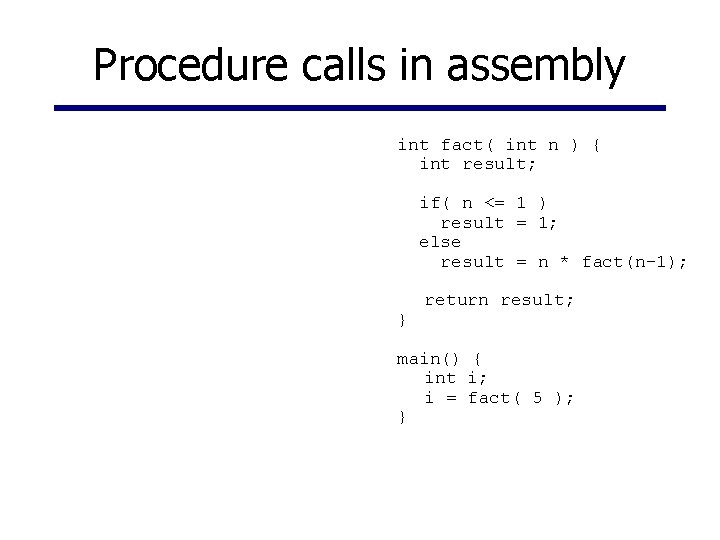
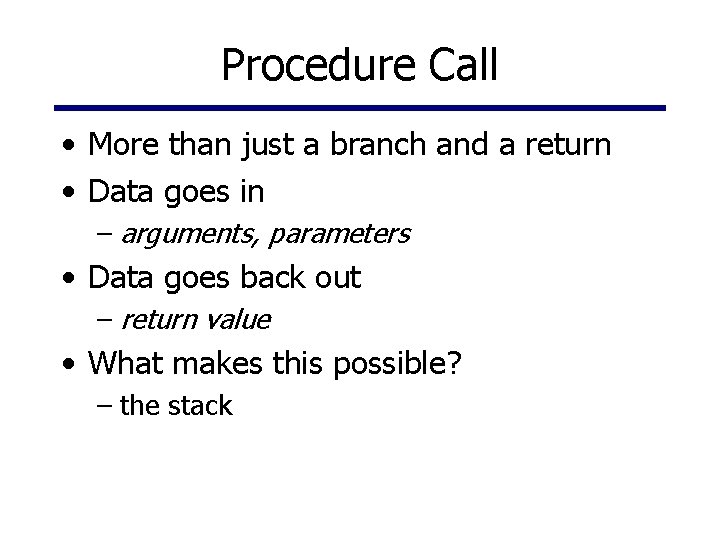
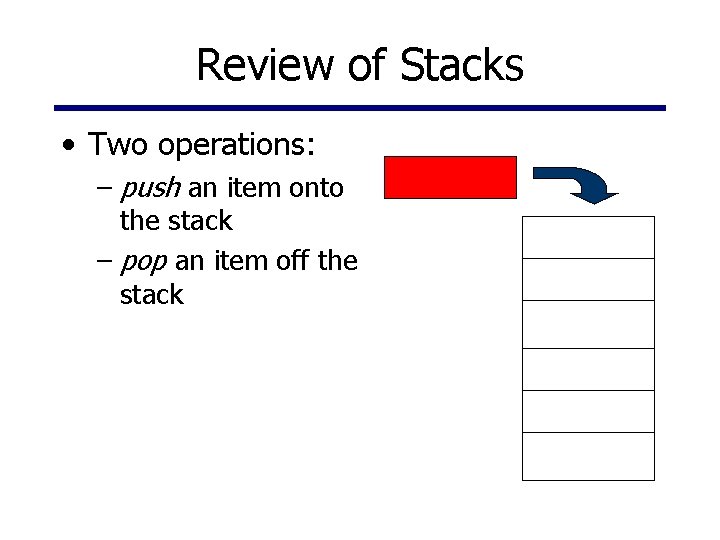
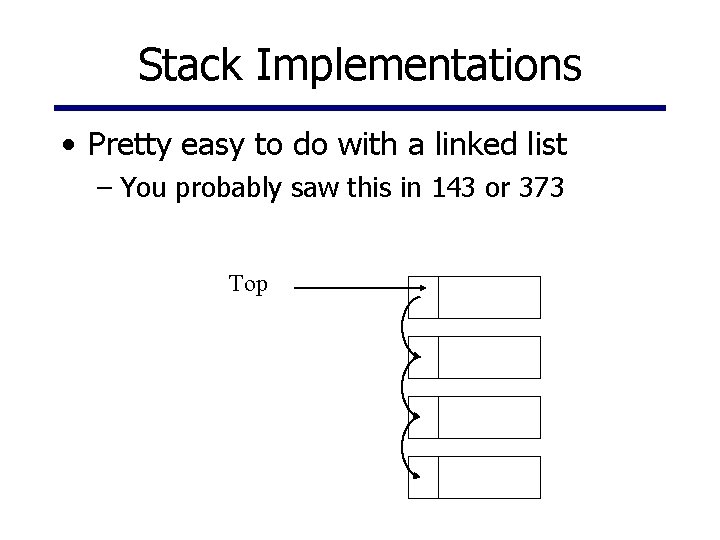
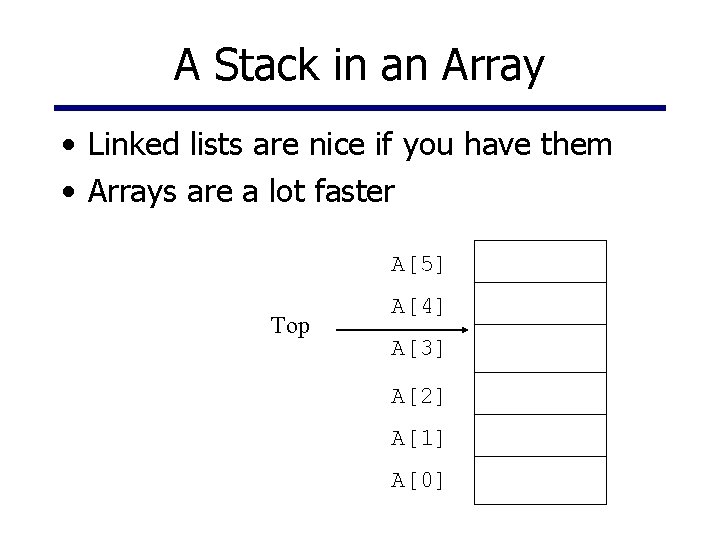
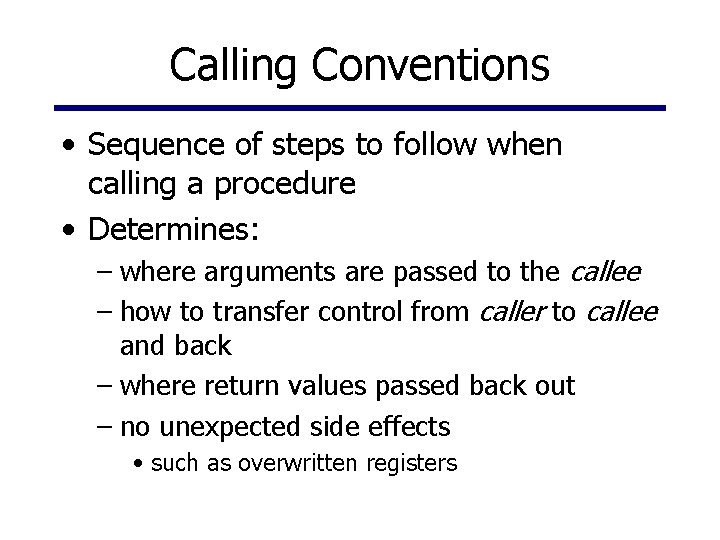
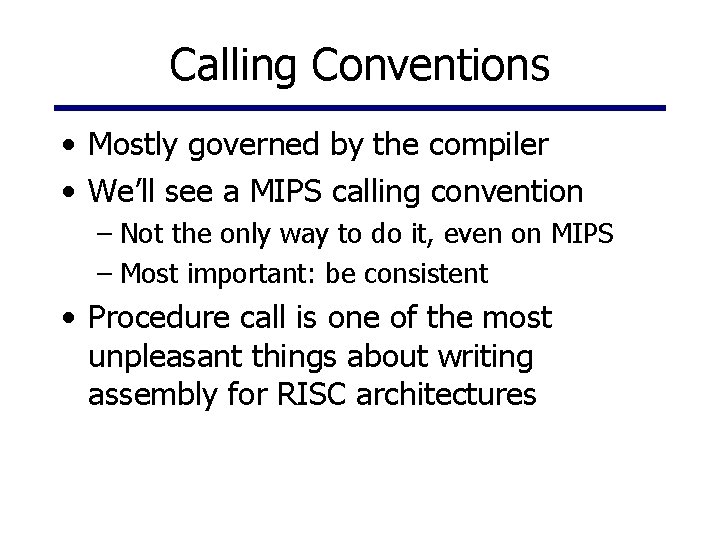
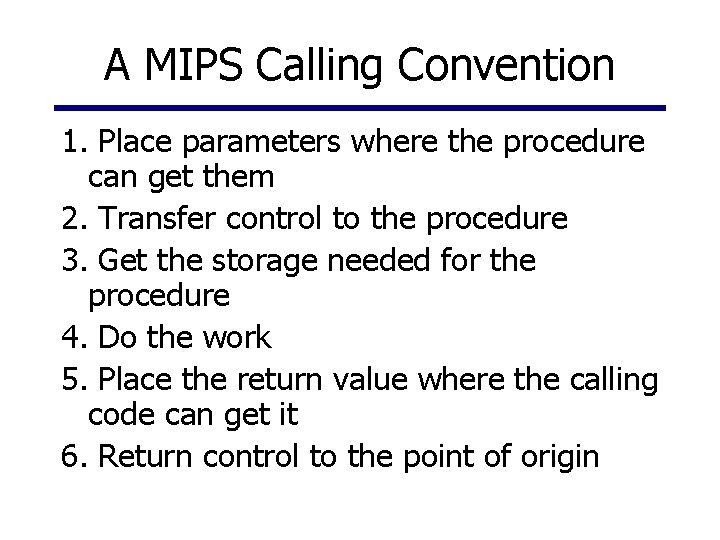
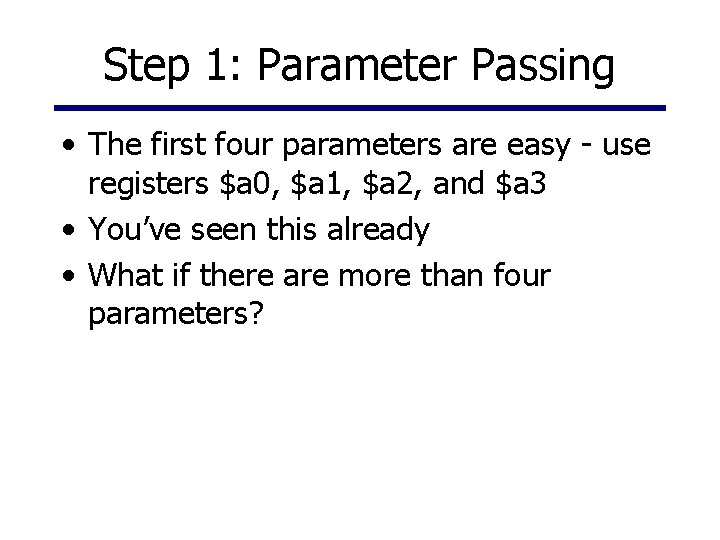
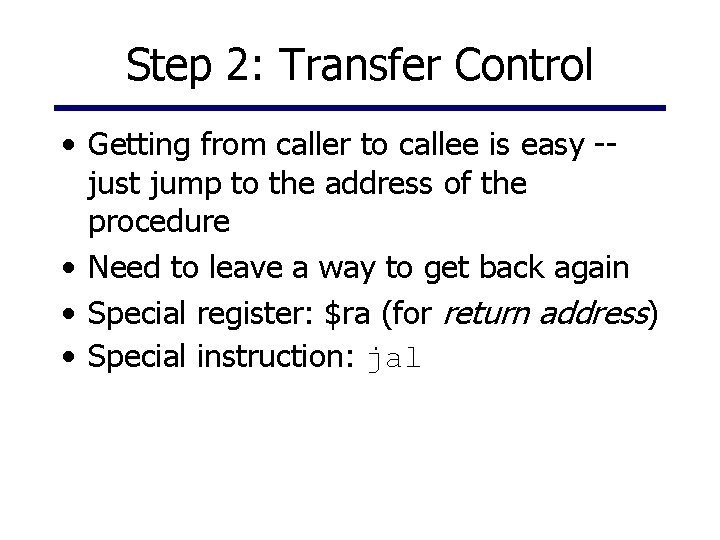
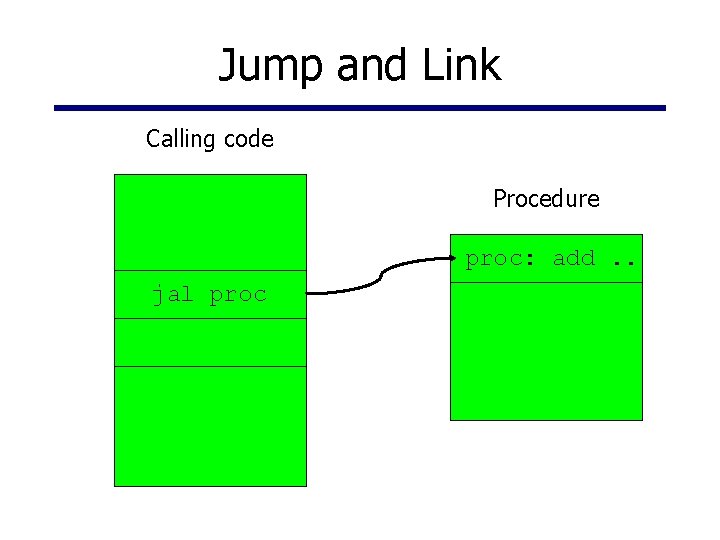
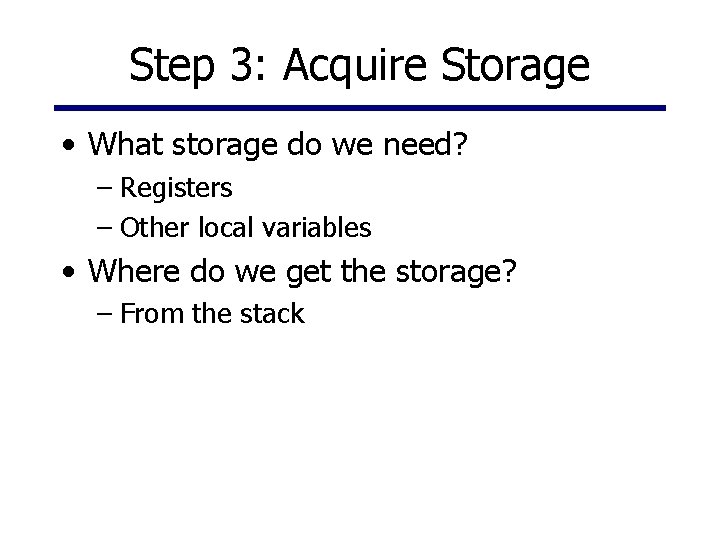
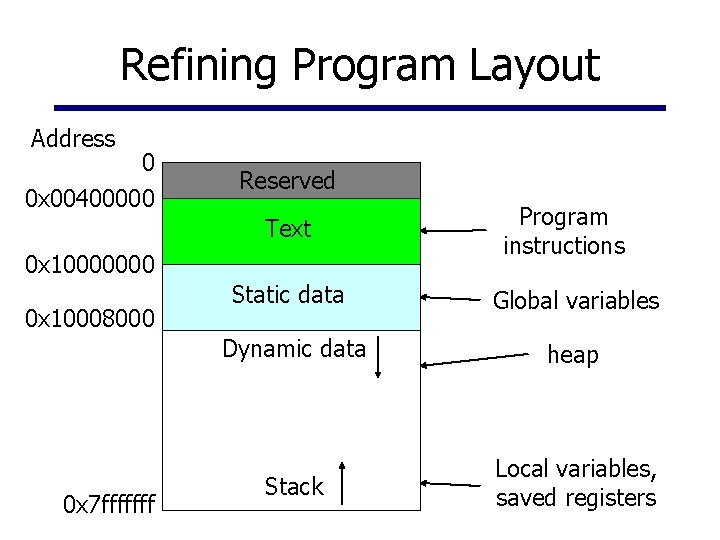
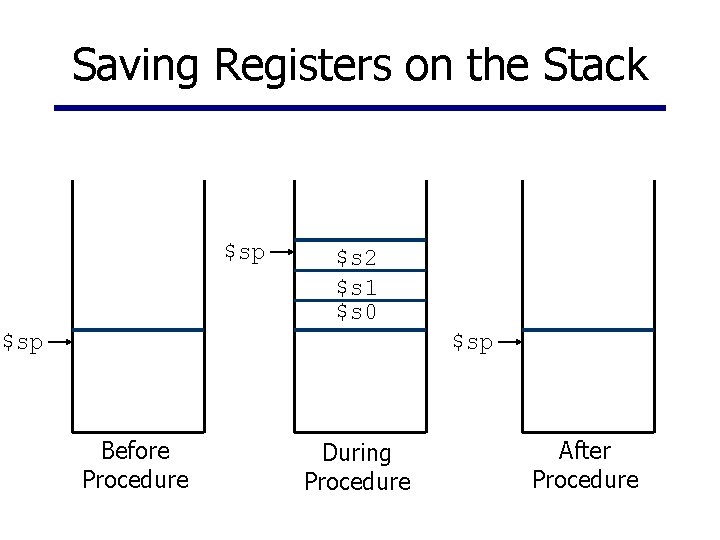
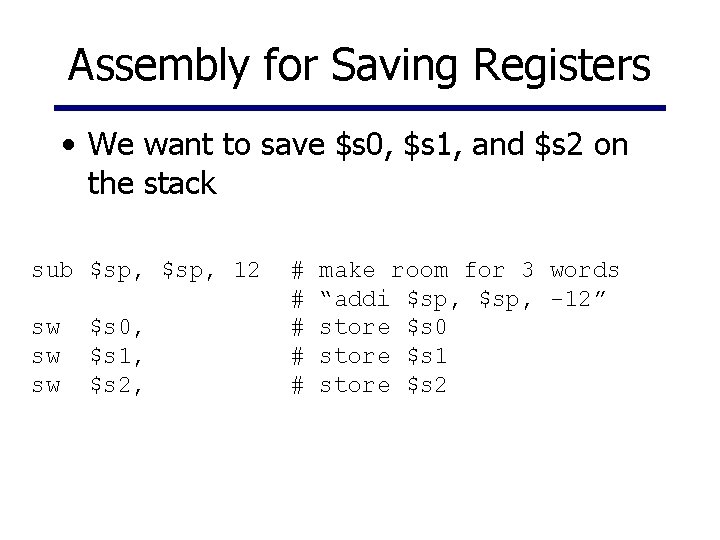
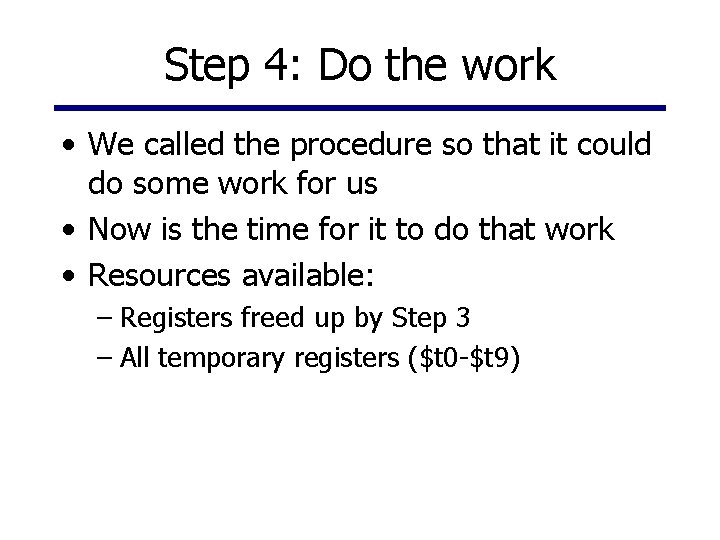
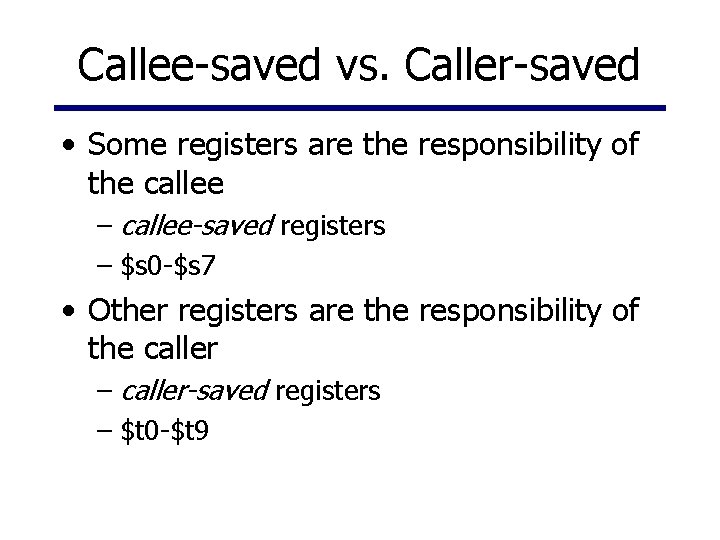
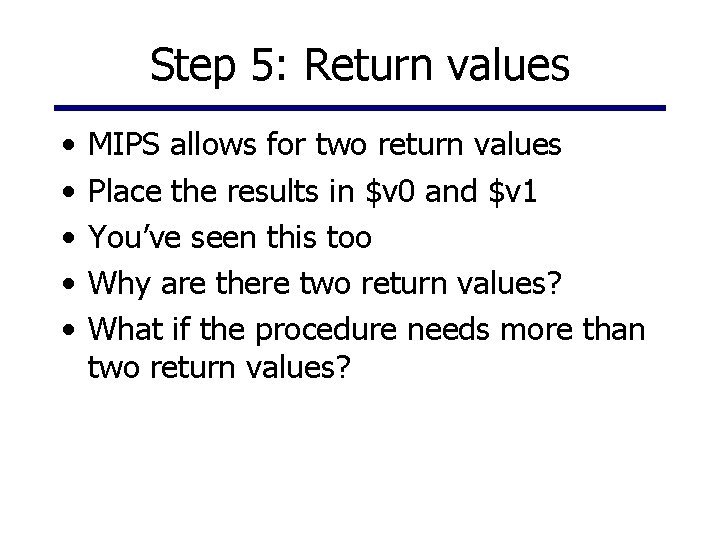
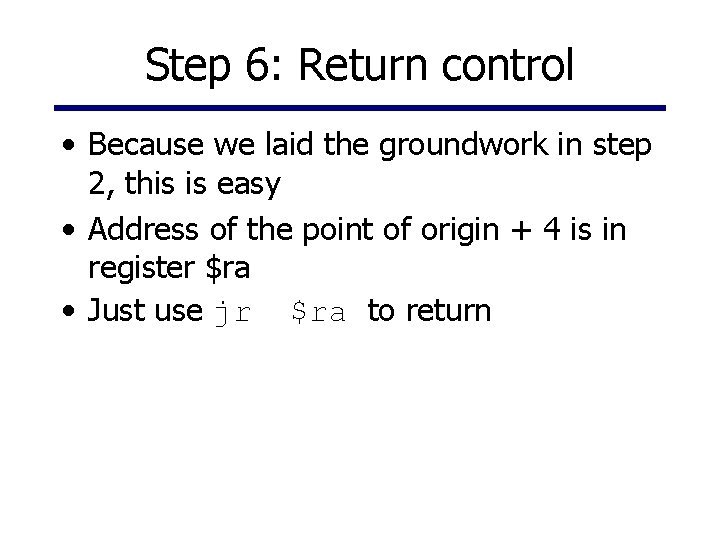
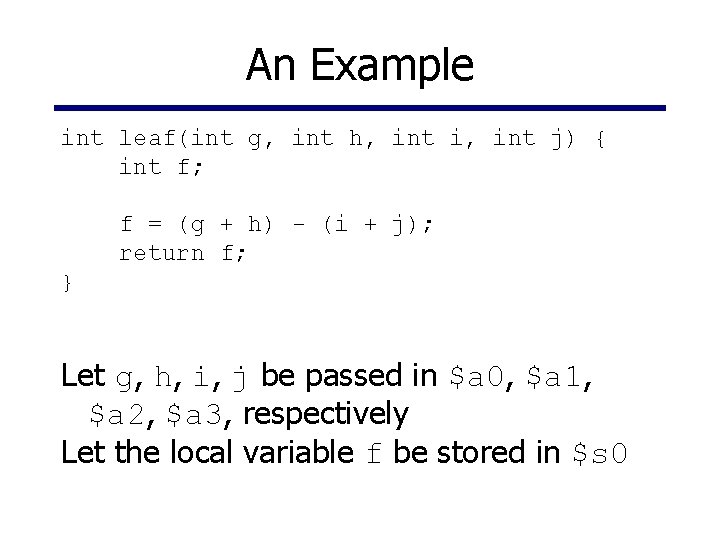
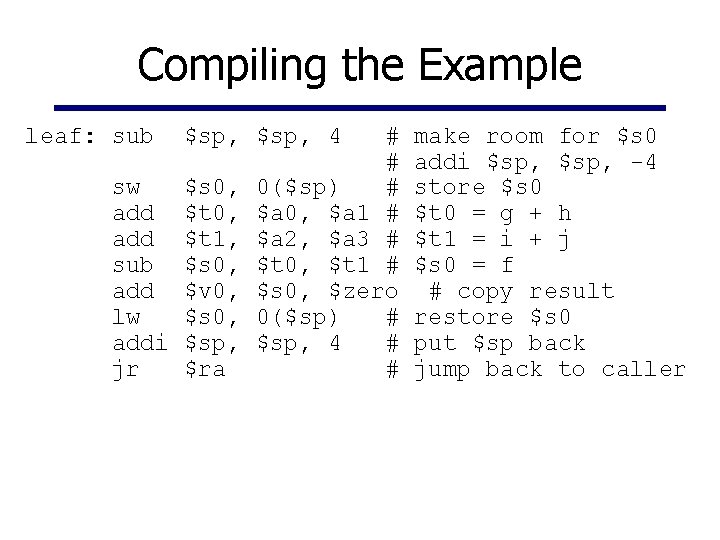
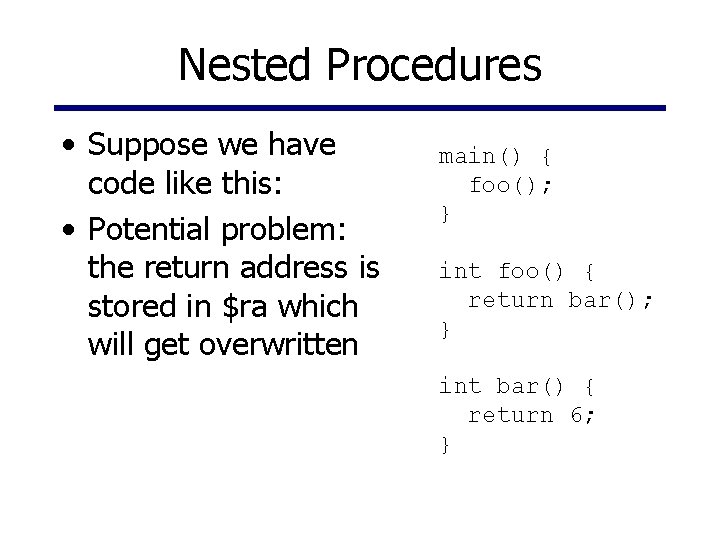
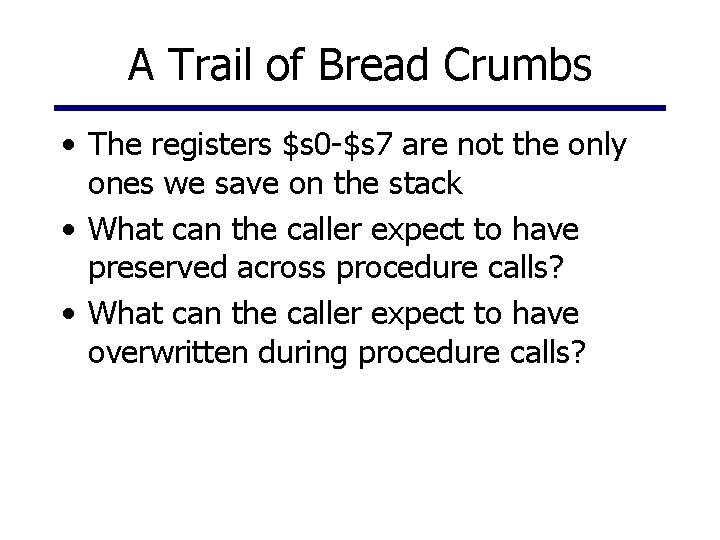
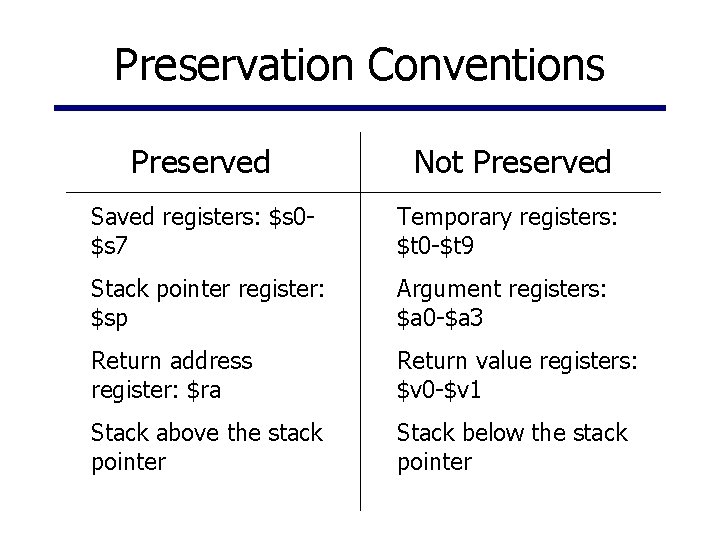
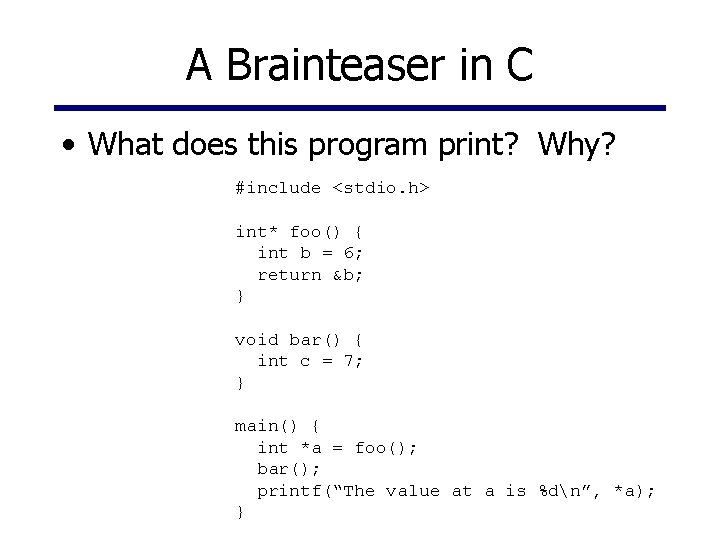
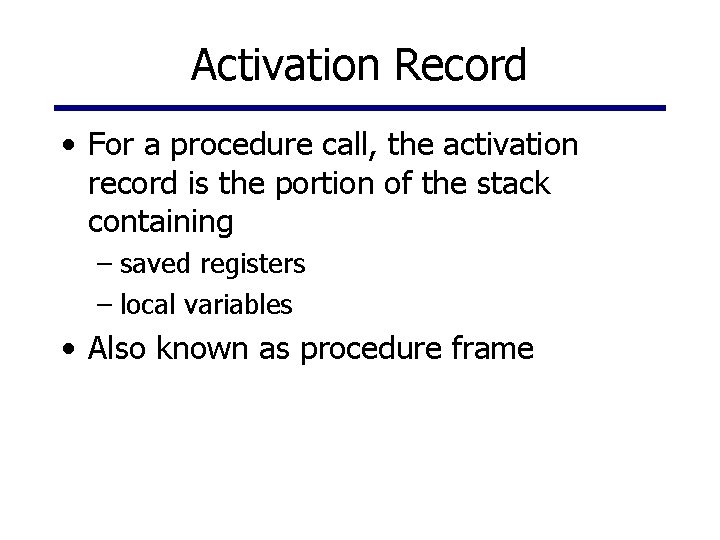
- Slides: 31
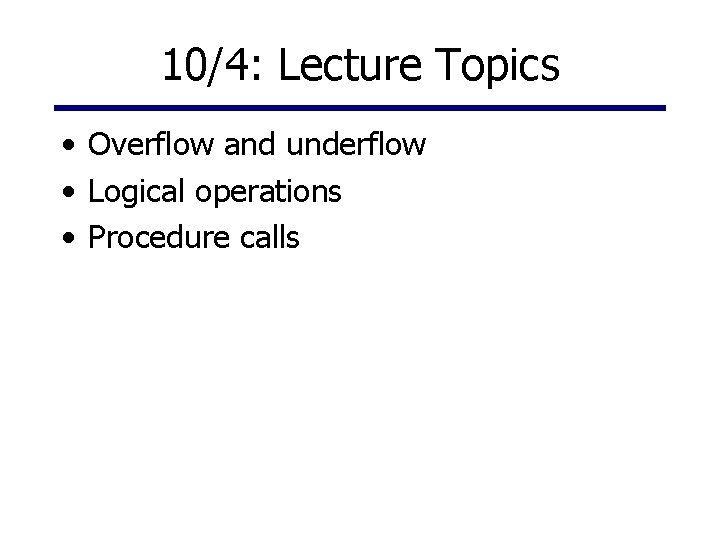
10/4: Lecture Topics • Overflow and underflow • Logical operations • Procedure calls
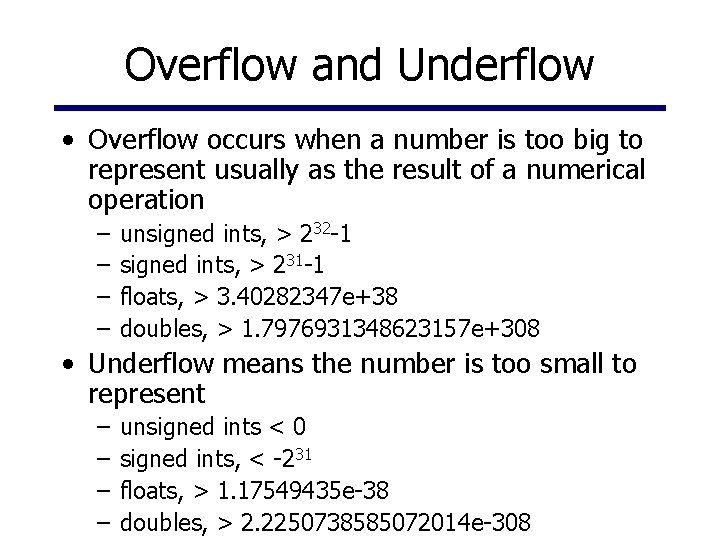
Overflow and Underflow • Overflow occurs when a number is too big to represent usually as the result of a numerical operation – – unsigned ints, > 232 -1 signed ints, > 231 -1 floats, > 3. 40282347 e+38 doubles, > 1. 7976931348623157 e+308 • Underflow means the number is too small to represent – – unsigned ints < 0 signed ints, < -231 floats, > 1. 17549435 e-38 doubles, > 2. 2250738585072014 e-308
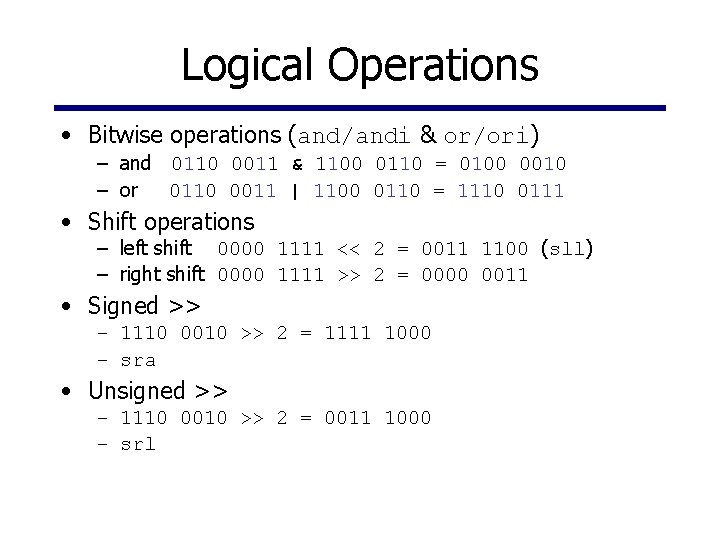
Logical Operations • Bitwise operations (and/andi & or/ori) – and 0110 0011 & 1100 0110 = 0100 0010 – or 0110 0011 | 1100 0110 = 1110 0111 • Shift operations – left shift 0000 1111 << 2 = 0011 1100 (sll) – right shift 0000 1111 >> 2 = 0000 0011 • Signed >> – 1110 0010 >> 2 = 1111 1000 – sra • Unsigned >> – 1110 0010 >> 2 = 0011 1000 – srl
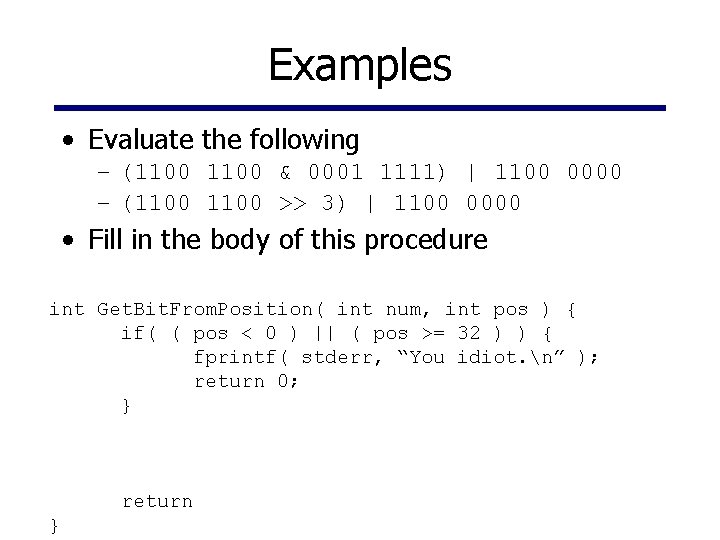
Examples • Evaluate the following – (1100 & 0001 1111) | 1100 0000 – (1100 >> 3) | 1100 0000 • Fill in the body of this procedure int Get. Bit. From. Position( int num, int pos ) { if( ( pos < 0 ) || ( pos >= 32 ) ) { fprintf( stderr, “You idiot. n” ); return 0; } return }
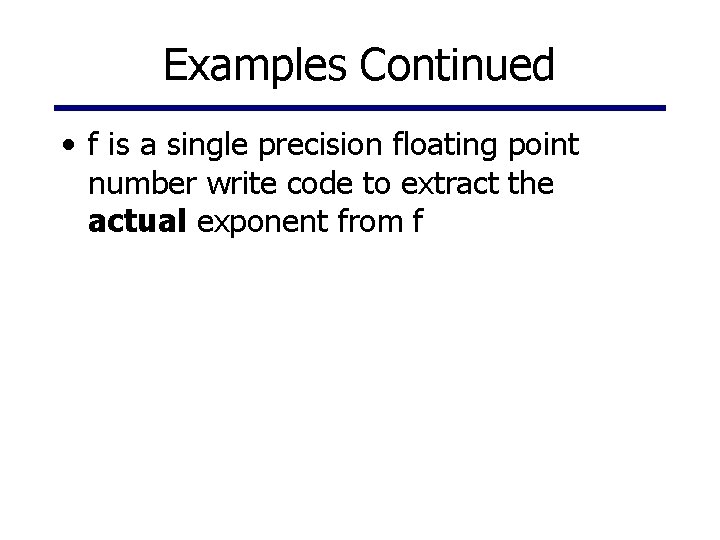
Examples Continued • f is a single precision floating point number write code to extract the actual exponent from f
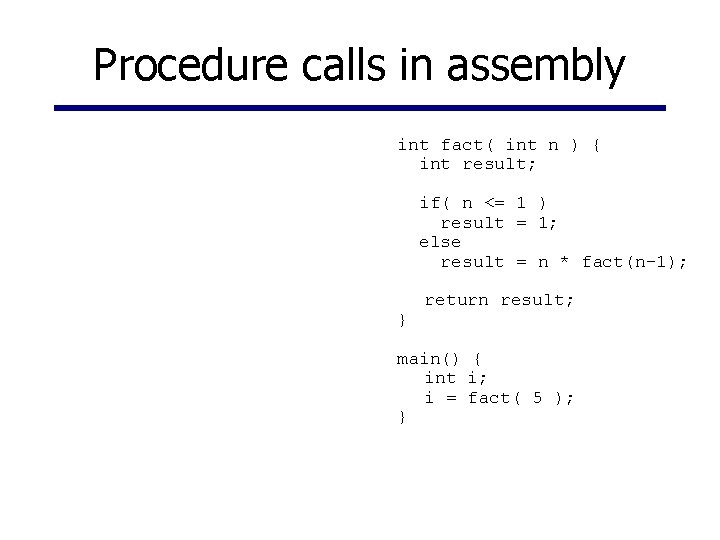
Procedure calls in assembly int fact( int n ) { int result; if( n <= 1 ) result = 1; else result = n * fact(n-1); } return result; main() { int i; i = fact( 5 ); }
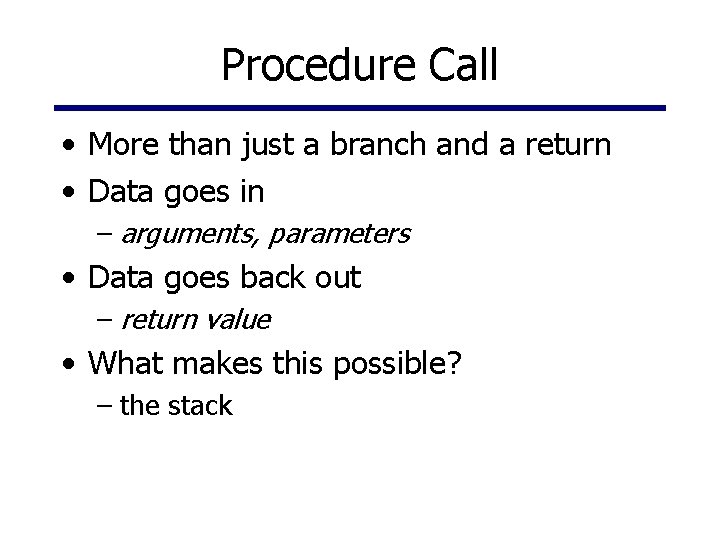
Procedure Call • More than just a branch and a return • Data goes in – arguments, parameters • Data goes back out – return value • What makes this possible? – the stack
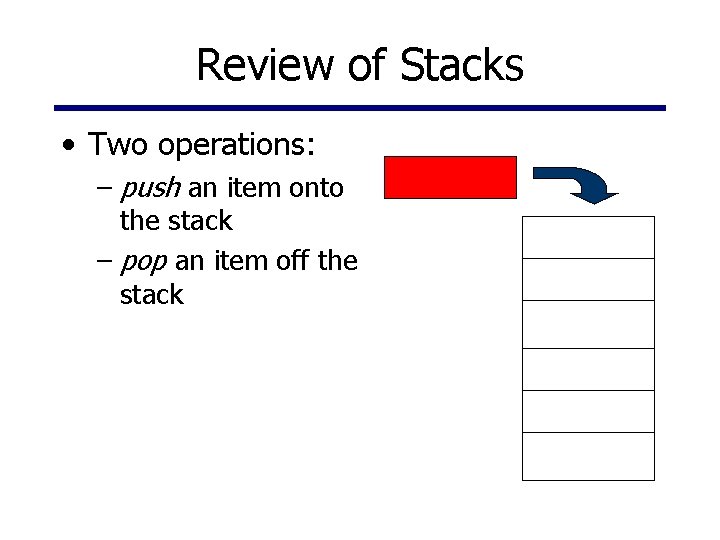
Review of Stacks • Two operations: – push an item onto the stack – pop an item off the stack
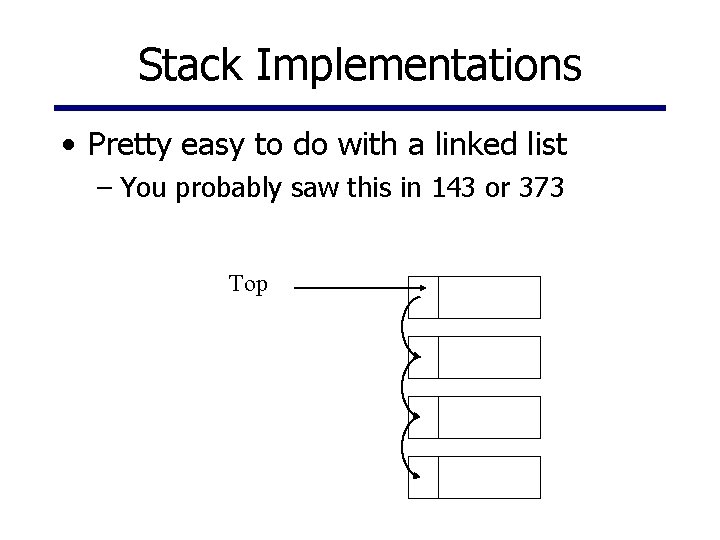
Stack Implementations • Pretty easy to do with a linked list – You probably saw this in 143 or 373 Top
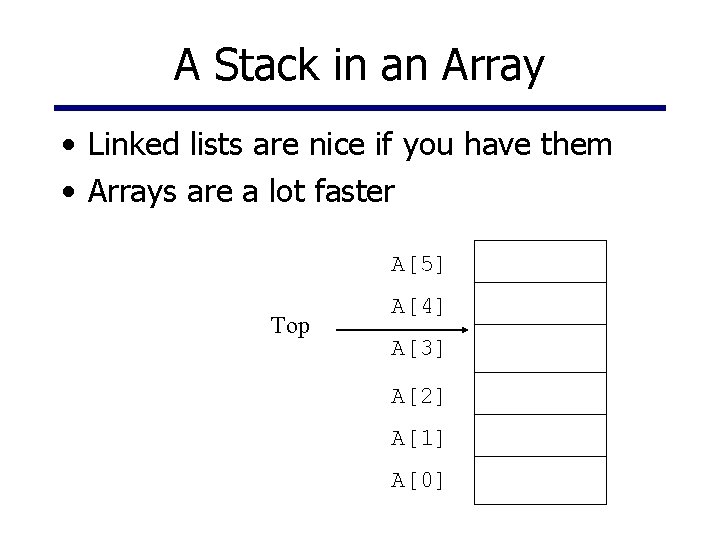
A Stack in an Array • Linked lists are nice if you have them • Arrays are a lot faster A[5] Top A[4] A[3] A[2] A[1] A[0]
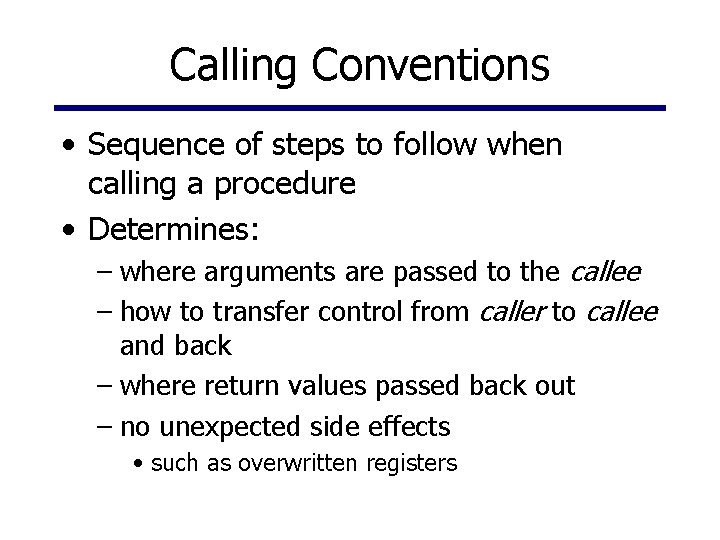
Calling Conventions • Sequence of steps to follow when calling a procedure • Determines: – where arguments are passed to the callee – how to transfer control from caller to callee and back – where return values passed back out – no unexpected side effects • such as overwritten registers
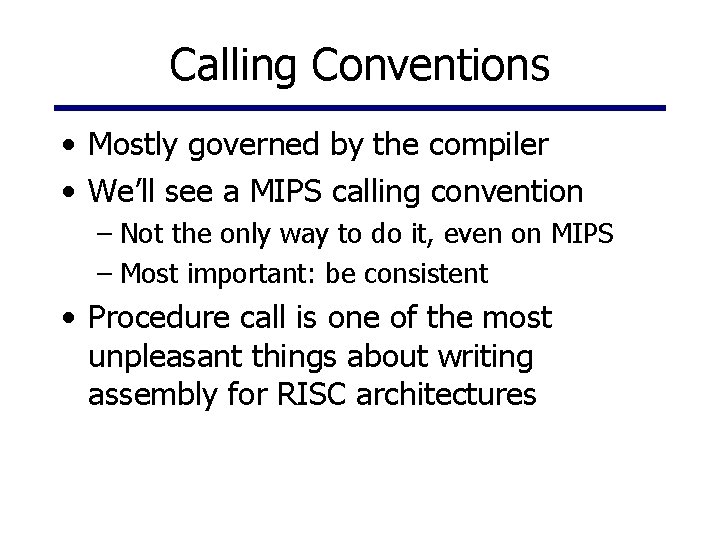
Calling Conventions • Mostly governed by the compiler • We’ll see a MIPS calling convention – Not the only way to do it, even on MIPS – Most important: be consistent • Procedure call is one of the most unpleasant things about writing assembly for RISC architectures
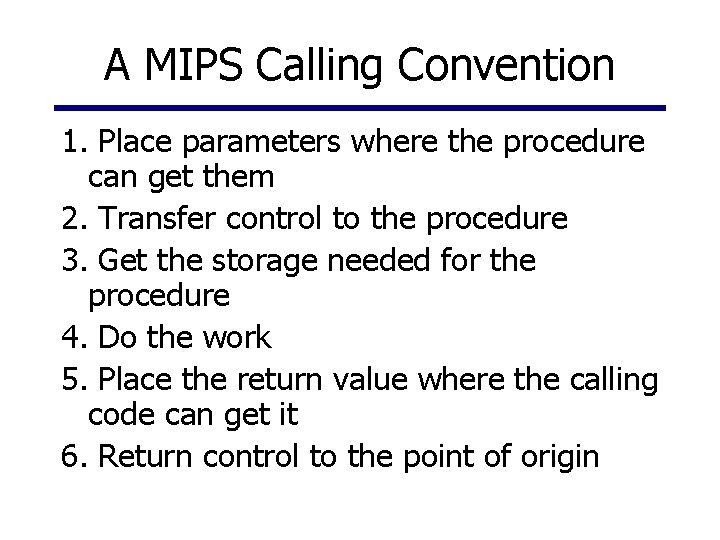
A MIPS Calling Convention 1. Place parameters where the procedure can get them 2. Transfer control to the procedure 3. Get the storage needed for the procedure 4. Do the work 5. Place the return value where the calling code can get it 6. Return control to the point of origin
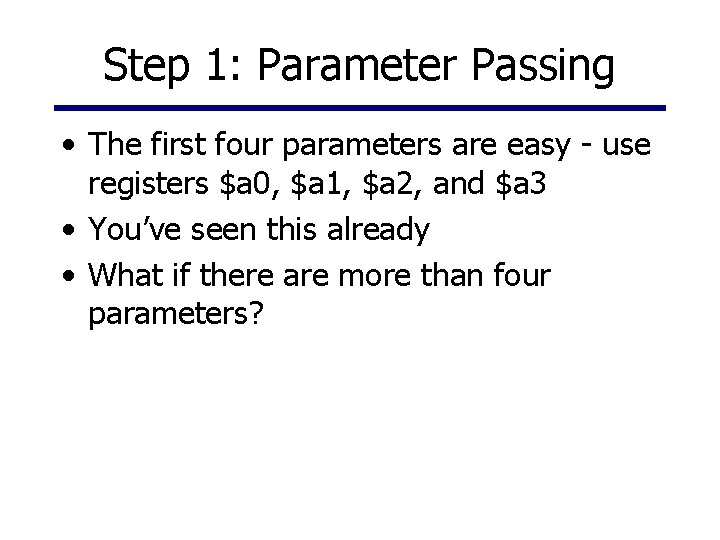
Step 1: Parameter Passing • The first four parameters are easy - use registers $a 0, $a 1, $a 2, and $a 3 • You’ve seen this already • What if there are more than four parameters?
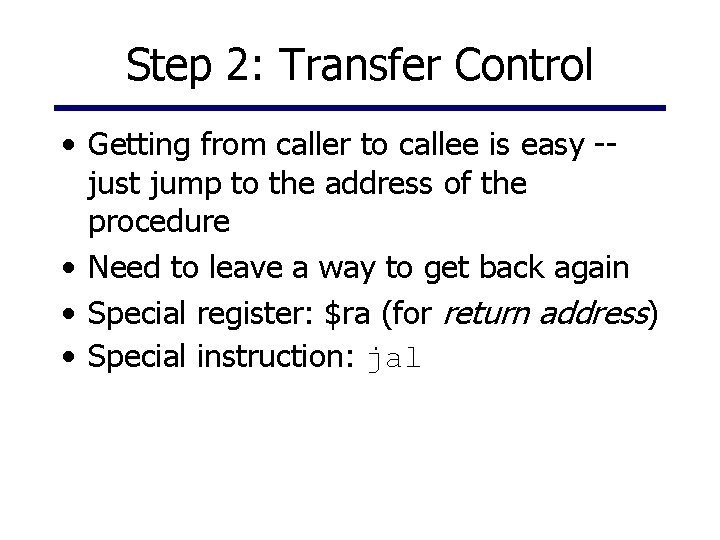
Step 2: Transfer Control • Getting from caller to callee is easy -just jump to the address of the procedure • Need to leave a way to get back again • Special register: $ra (for return address) • Special instruction: jal
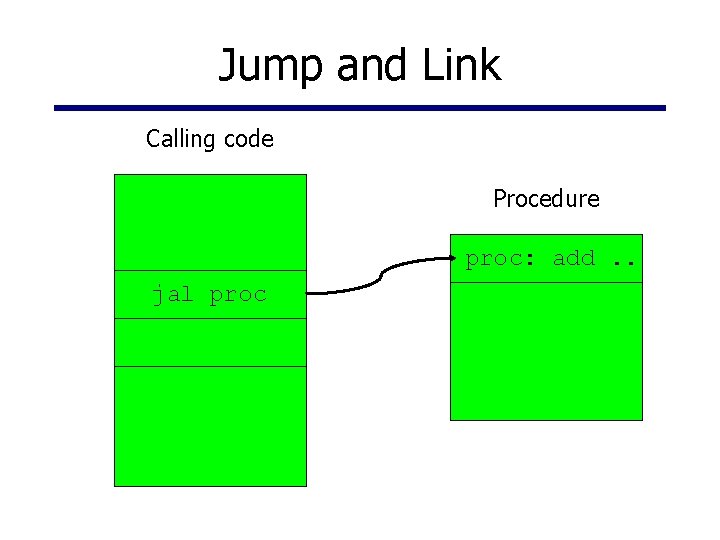
Jump and Link Calling code Procedure proc: add. . jal proc
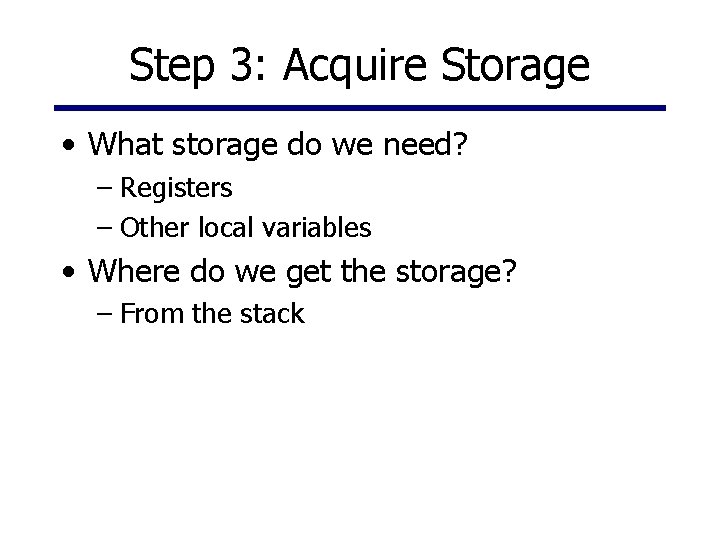
Step 3: Acquire Storage • What storage do we need? – Registers – Other local variables • Where do we get the storage? – From the stack
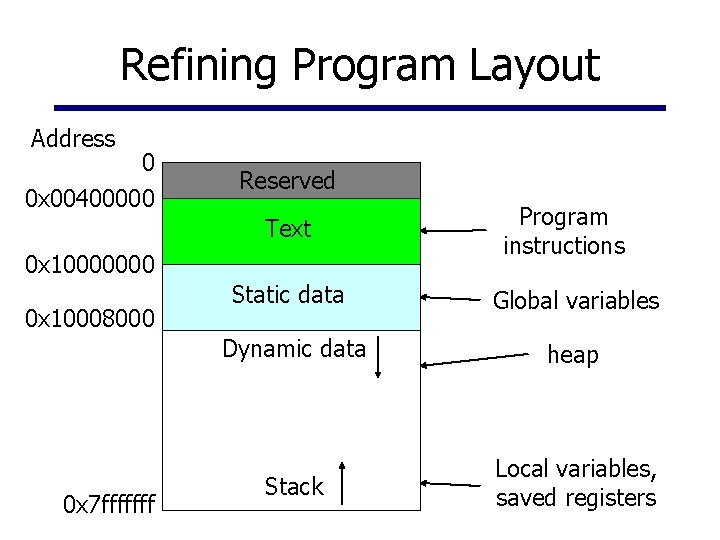
Refining Program Layout Address 0 0 x 00400000 Reserved Text 0 x 10000000 0 x 10008000 0 x 7 fffffff Program instructions Static data Global variables Dynamic data heap Stack Local variables, saved registers
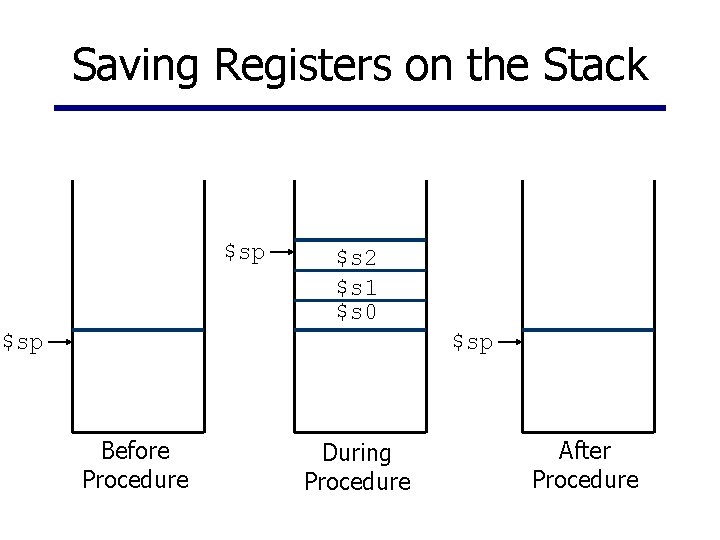
Saving Registers on the Stack $sp $s 2 $s 1 $s 0 $sp Before Procedure During Procedure After Procedure
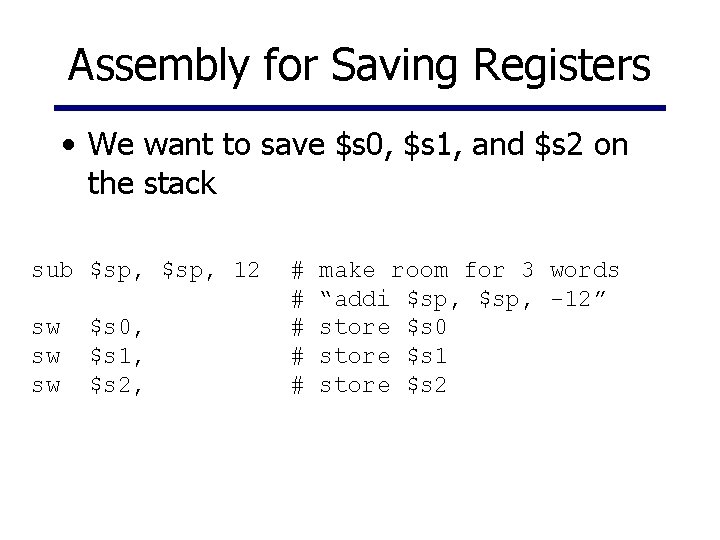
Assembly for Saving Registers • We want to save $s 0, $s 1, and $s 2 on the stack sub $sp, 12 sw sw sw $s 0, $s 1, $s 2, # # # make room for 3 words “addi $sp, -12” store $s 0 store $s 1 store $s 2
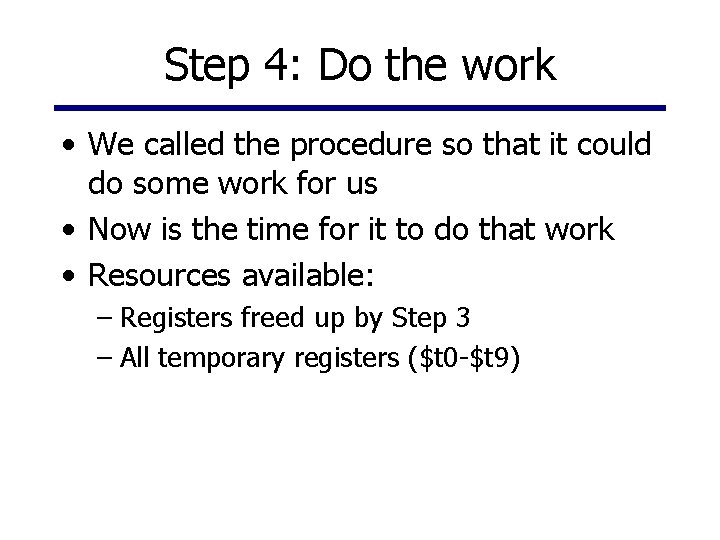
Step 4: Do the work • We called the procedure so that it could do some work for us • Now is the time for it to do that work • Resources available: – Registers freed up by Step 3 – All temporary registers ($t 0 -$t 9)
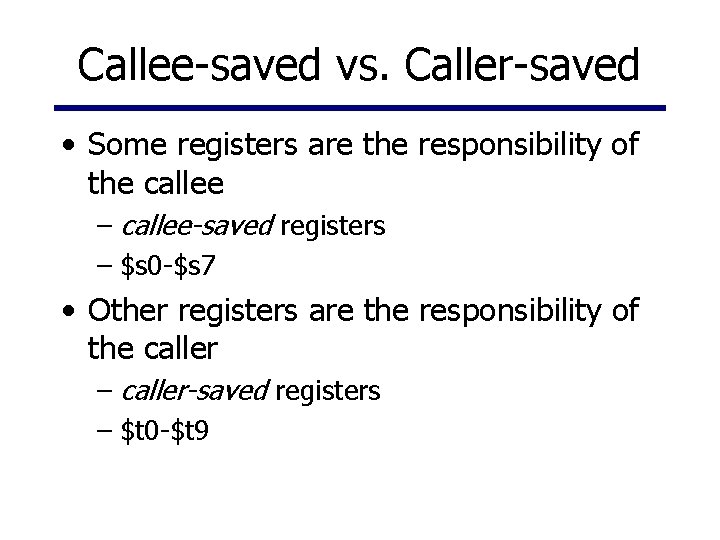
Callee-saved vs. Caller-saved • Some registers are the responsibility of the callee – callee-saved registers – $s 0 -$s 7 • Other registers are the responsibility of the caller – caller-saved registers – $t 0 -$t 9
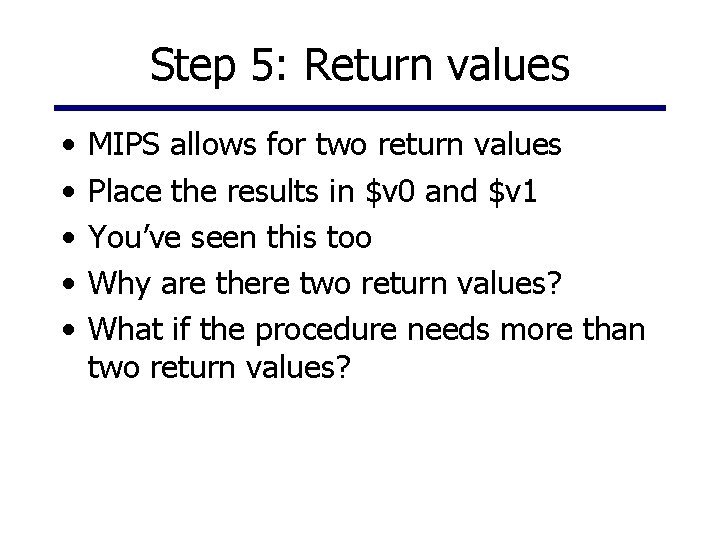
Step 5: Return values • • • MIPS allows for two return values Place the results in $v 0 and $v 1 You’ve seen this too Why are there two return values? What if the procedure needs more than two return values?
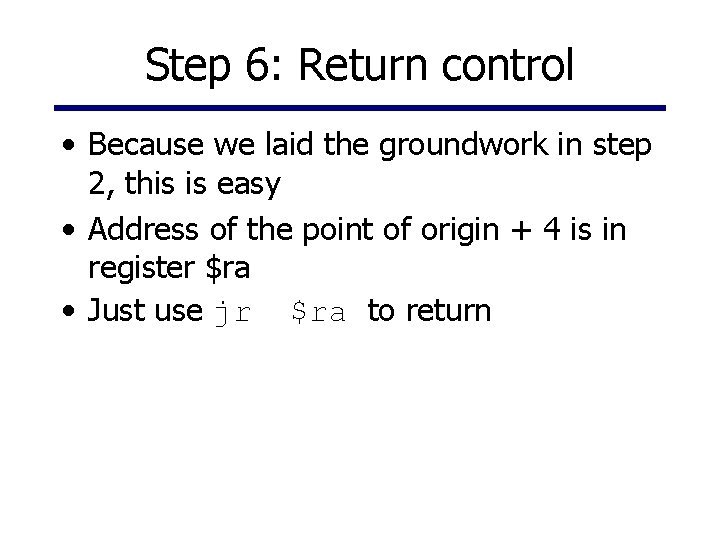
Step 6: Return control • Because we laid the groundwork in step 2, this is easy • Address of the point of origin + 4 is in register $ra • Just use jr $ra to return
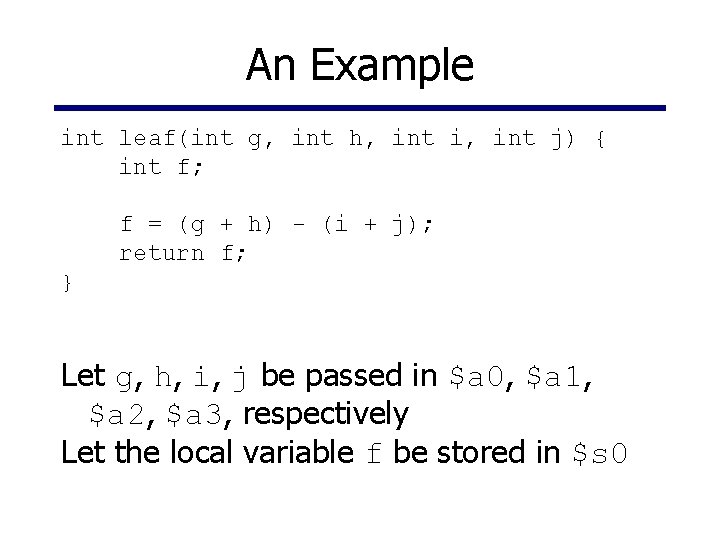
An Example int leaf(int g, int h, int i, int j) { int f; f = (g + h) - (i + j); return f; } Let g, h, i, j be passed in $a 0, $a 1, $a 2, $a 3, respectively Let the local variable f be stored in $s 0
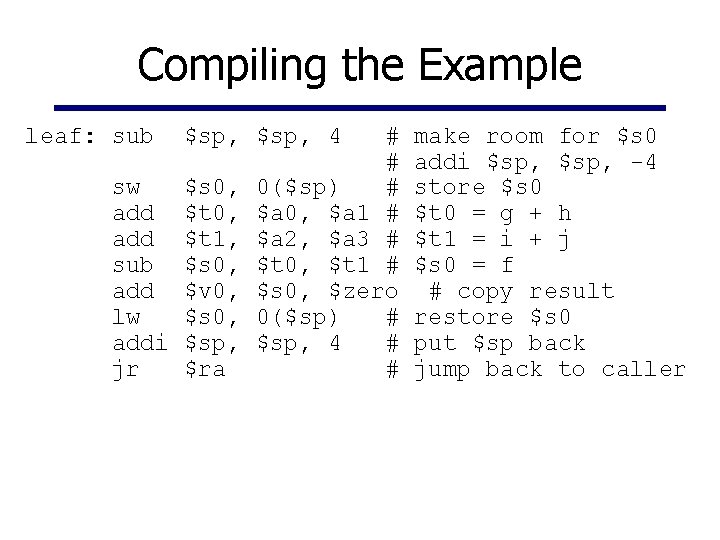
Compiling the Example leaf: sub sw add sub add lw addi jr $sp, 4 $s 0, $t 1, $s 0, $v 0, $sp, $ra # # 0($sp) # $a 0, $a 1 # $a 2, $a 3 # $t 0, $t 1 # $s 0, $zero 0($sp) # $sp, 4 # # make room for $s 0 addi $sp, -4 store $s 0 $t 0 = g + h $t 1 = i + j $s 0 = f # copy result restore $s 0 put $sp back jump back to caller
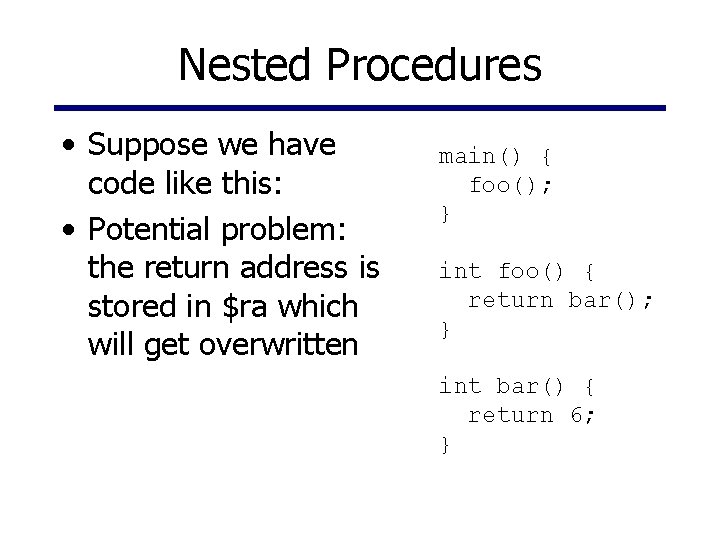
Nested Procedures • Suppose we have code like this: • Potential problem: the return address is stored in $ra which will get overwritten main() { foo(); } int foo() { return bar(); } int bar() { return 6; }
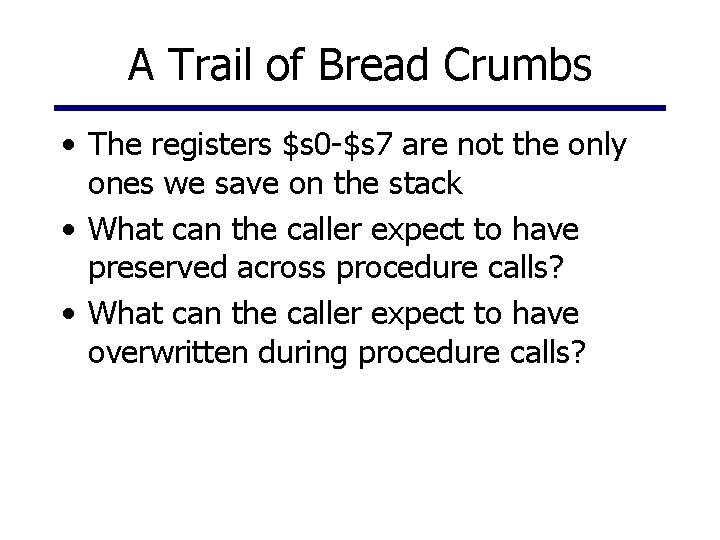
A Trail of Bread Crumbs • The registers $s 0 -$s 7 are not the only ones we save on the stack • What can the caller expect to have preserved across procedure calls? • What can the caller expect to have overwritten during procedure calls?
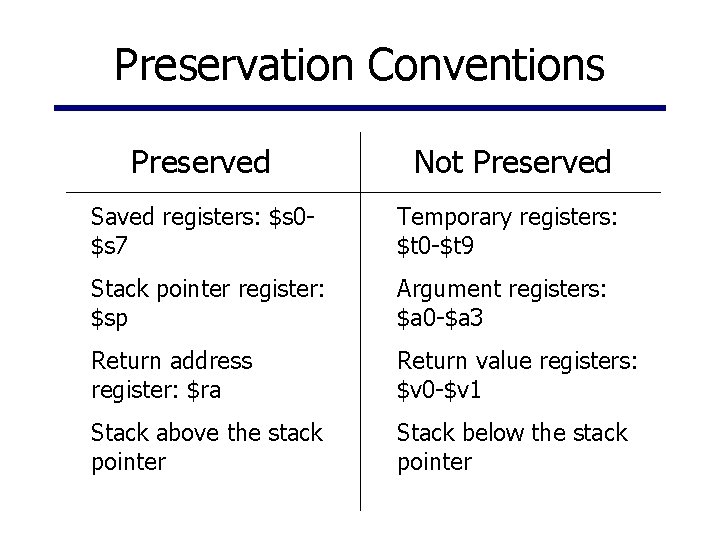
Preservation Conventions Preserved Not Preserved Saved registers: $s 0$s 7 Temporary registers: $t 0 -$t 9 Stack pointer register: $sp Argument registers: $a 0 -$a 3 Return address register: $ra Return value registers: $v 0 -$v 1 Stack above the stack pointer Stack below the stack pointer
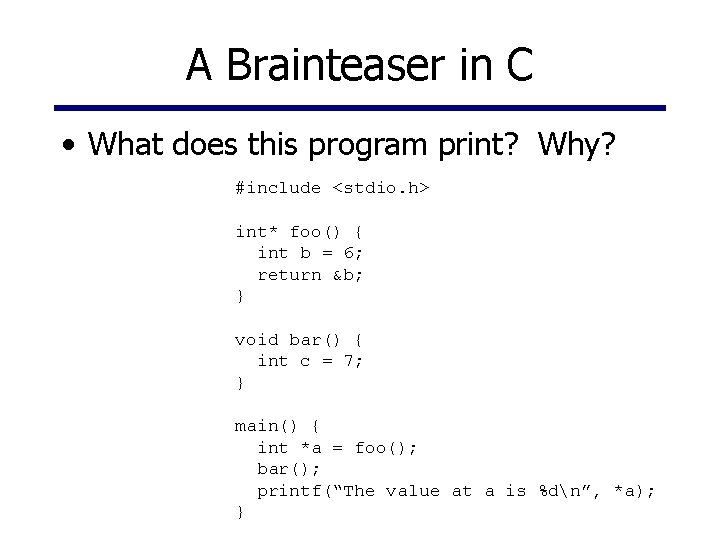
A Brainteaser in C • What does this program print? Why? #include <stdio. h> int* foo() { int b = 6; return &b; } void bar() { int c = 7; } main() { int *a = foo(); bar(); printf(“The value at a is %dn”, *a); }
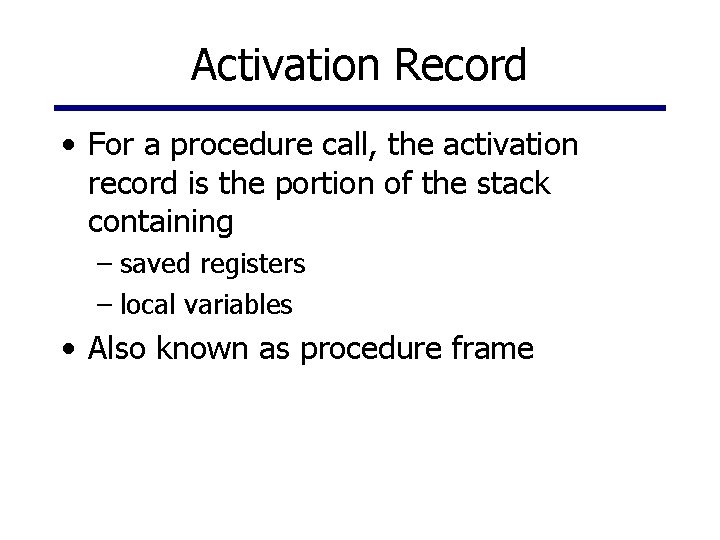
Activation Record • For a procedure call, the activation record is the portion of the stack containing – saved registers – local variables • Also known as procedure frame