1 CHAPTER 9 VECTORS 6415 Nyhoff ADTs Data
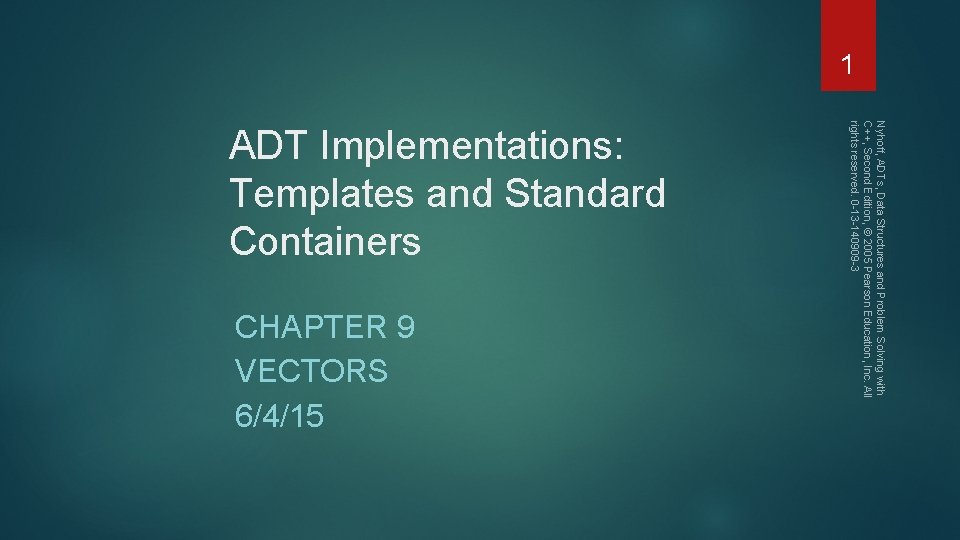
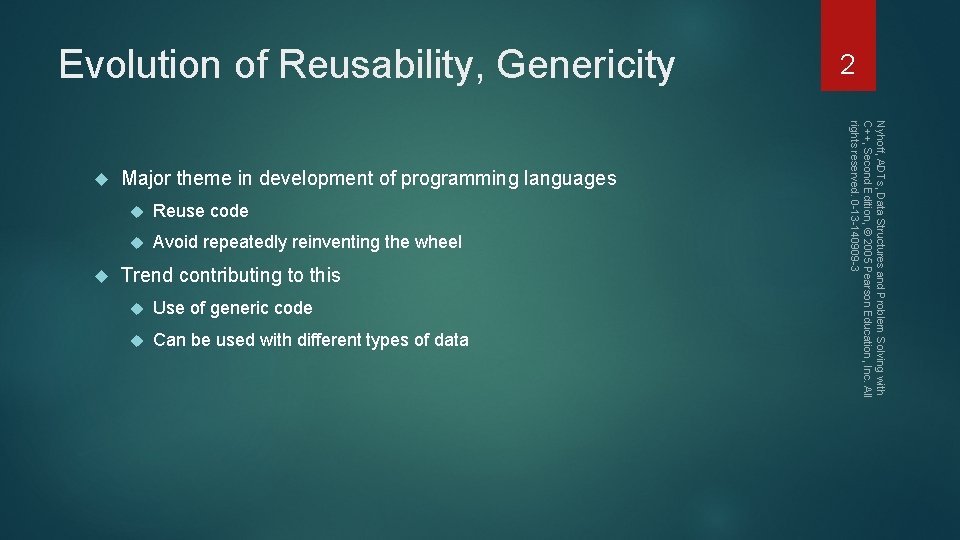
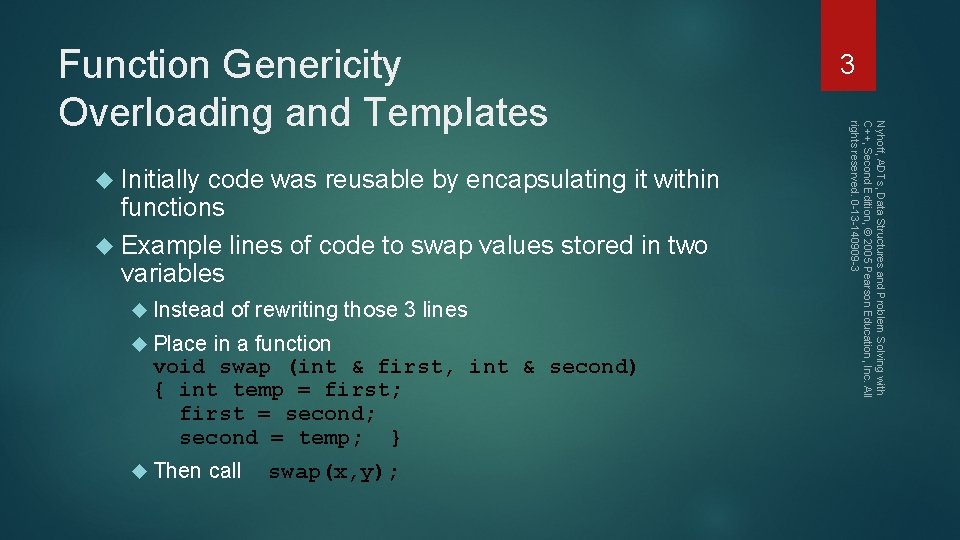
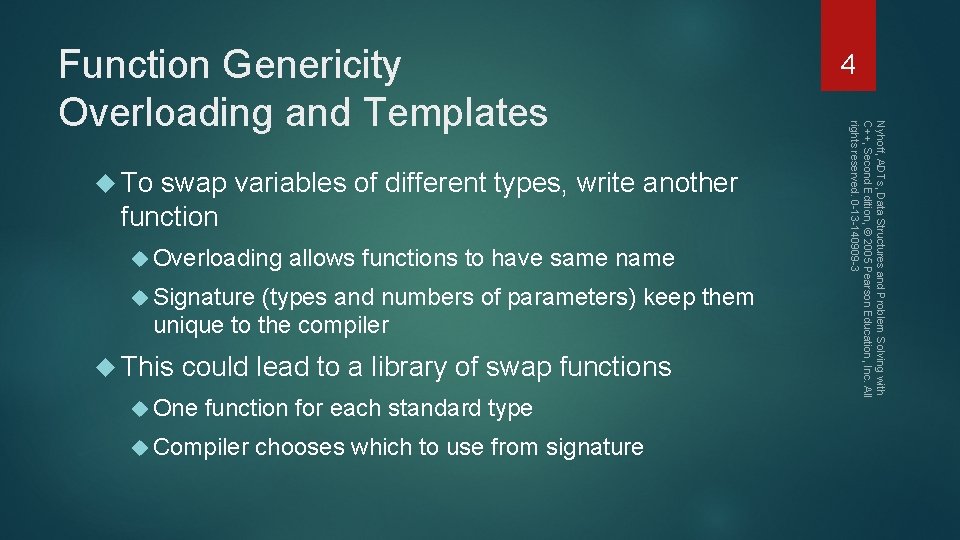
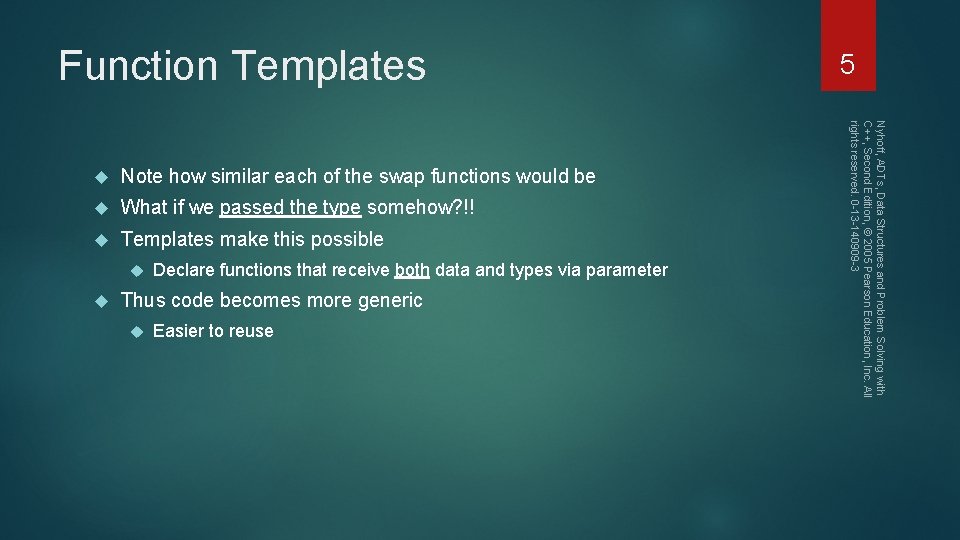
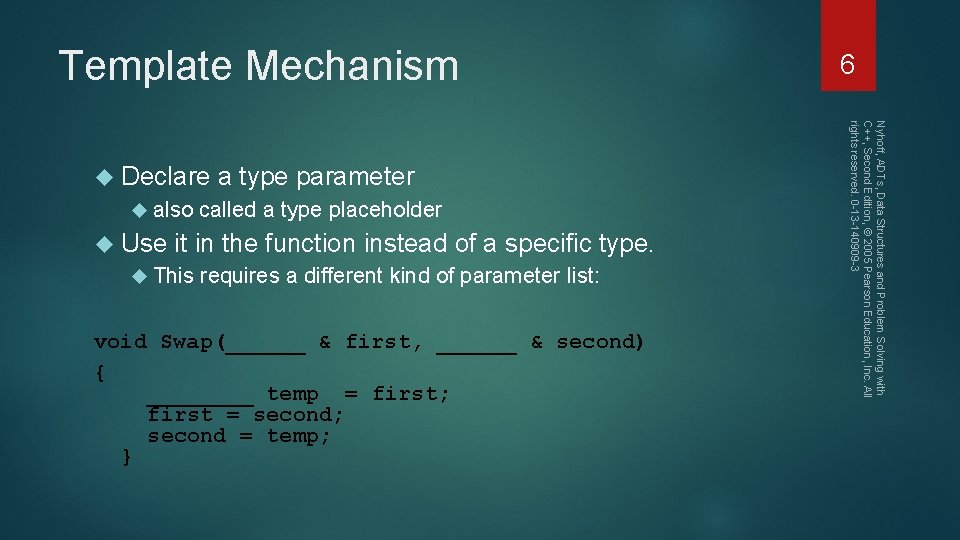
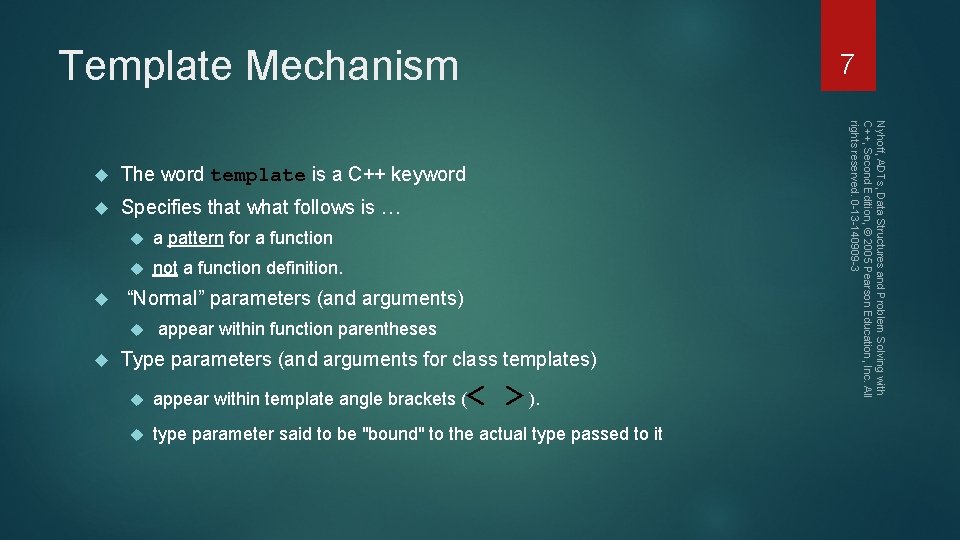
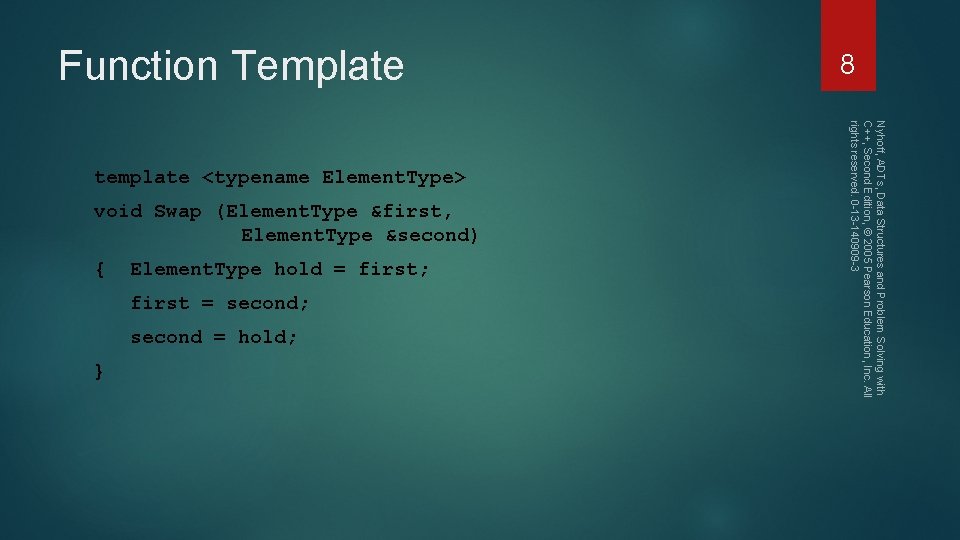
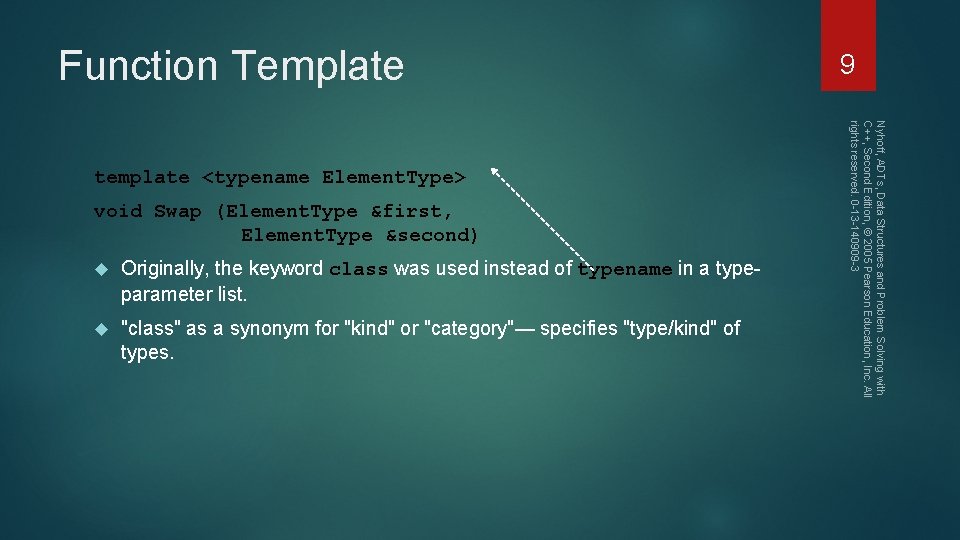
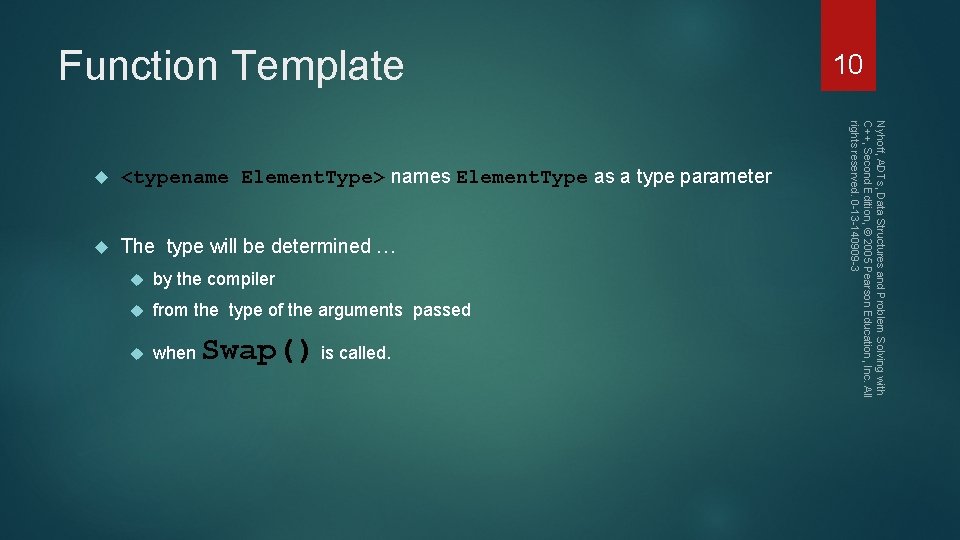
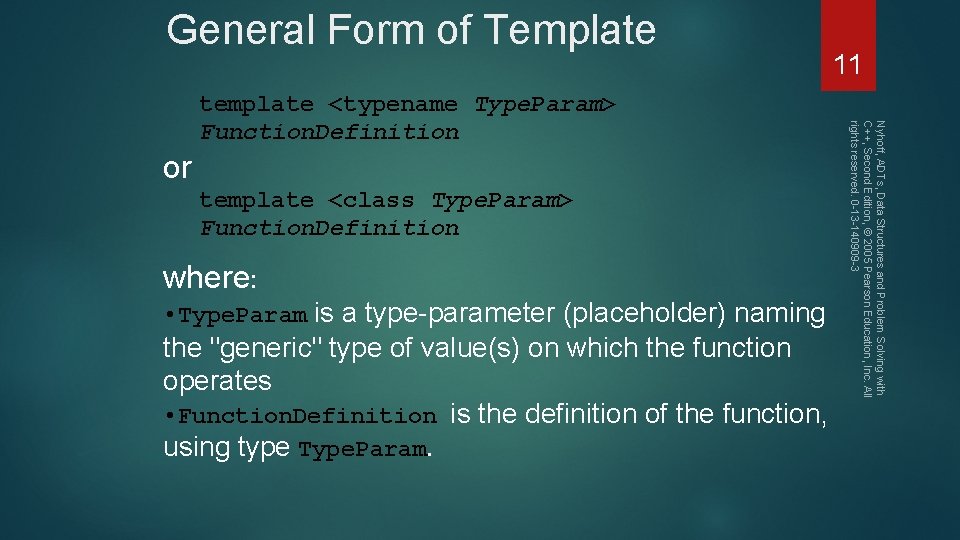
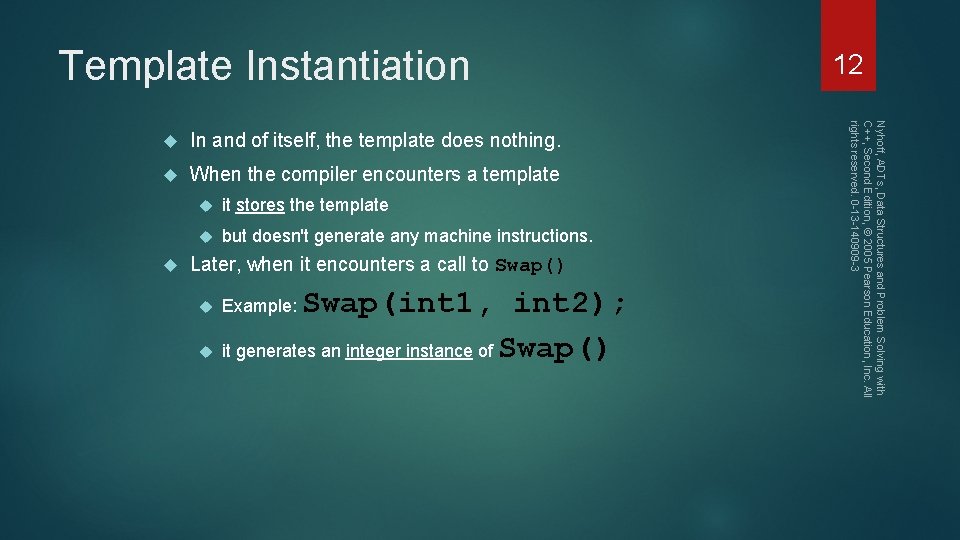
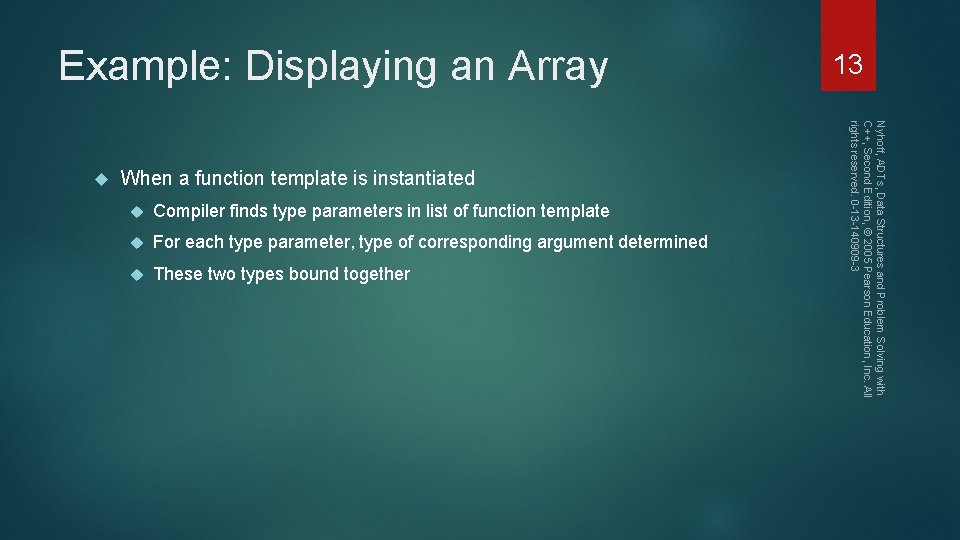
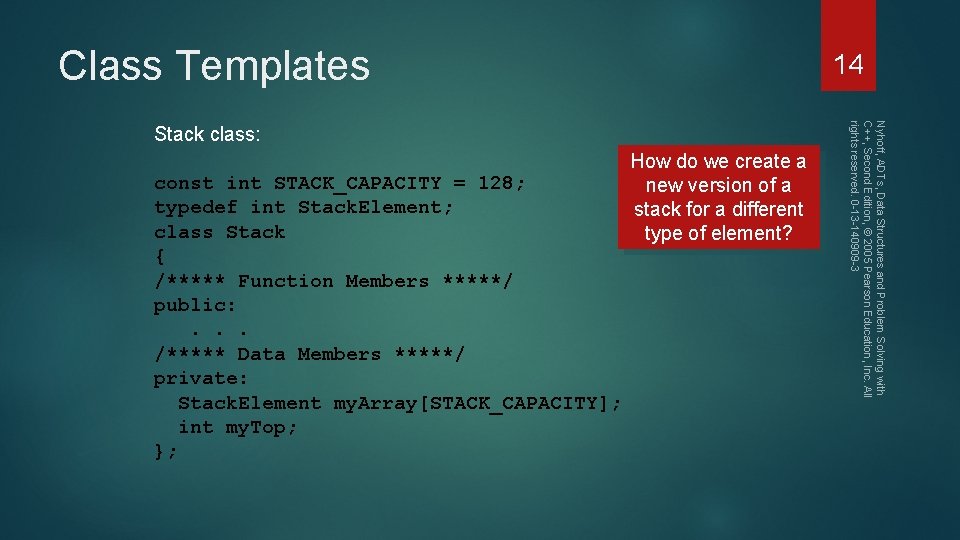
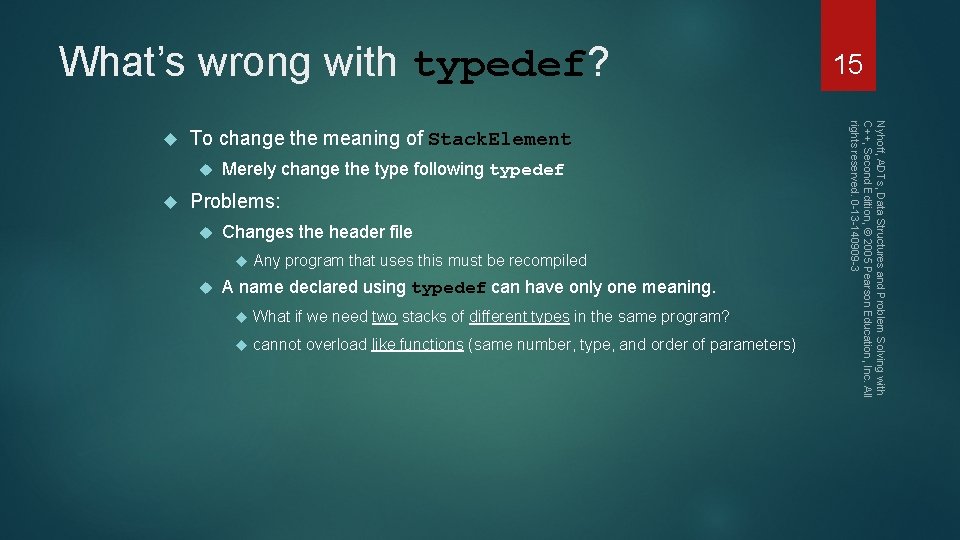
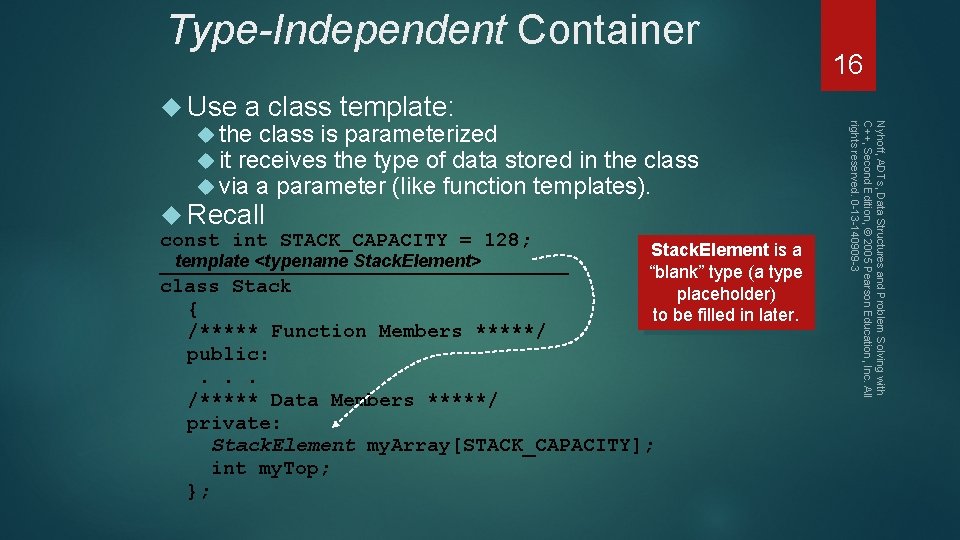
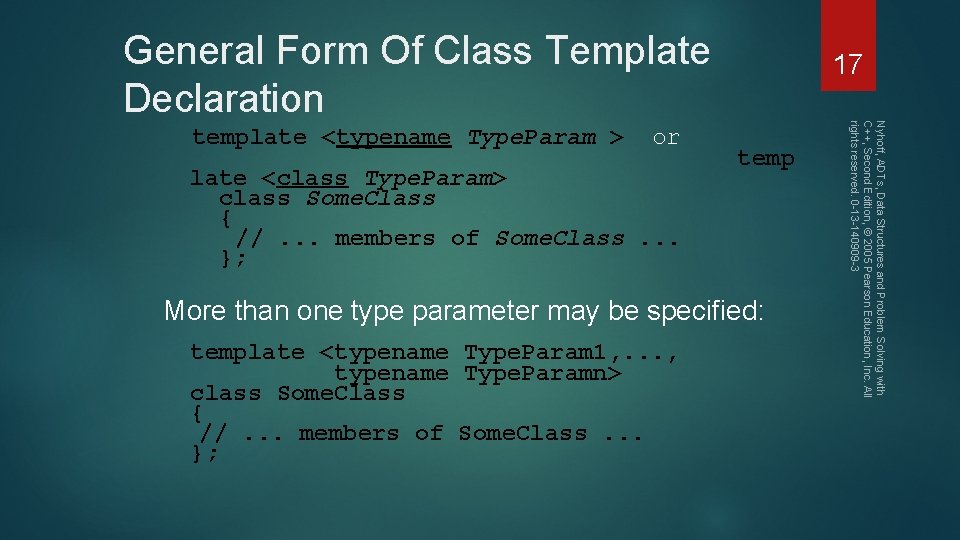
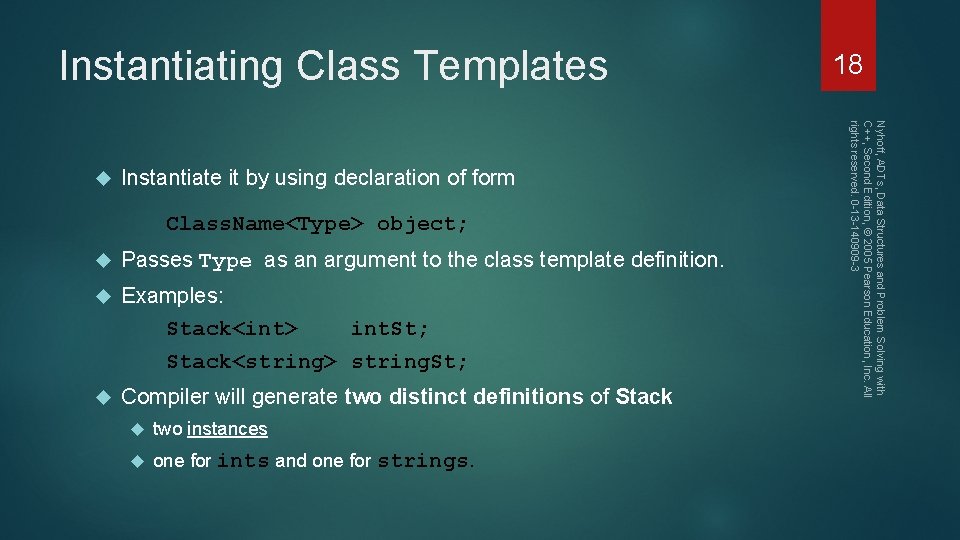
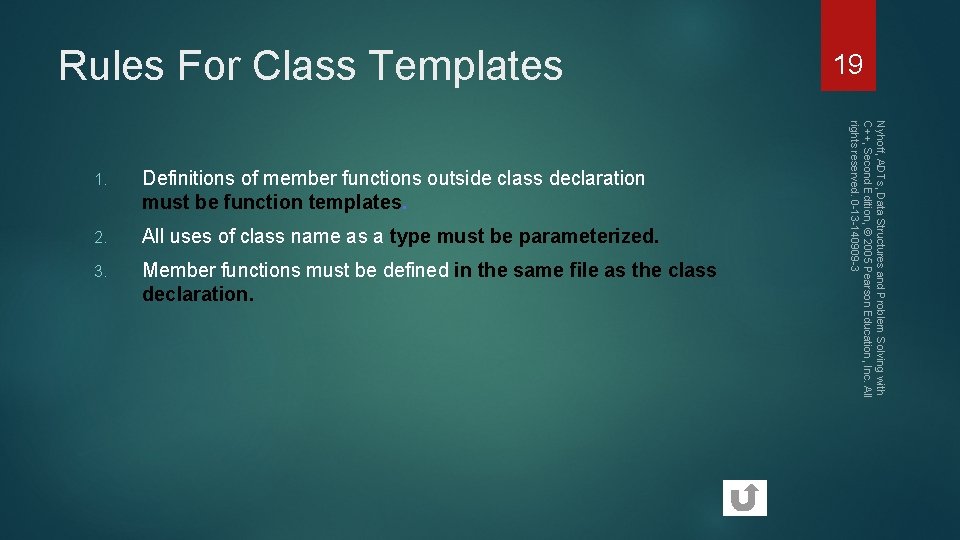
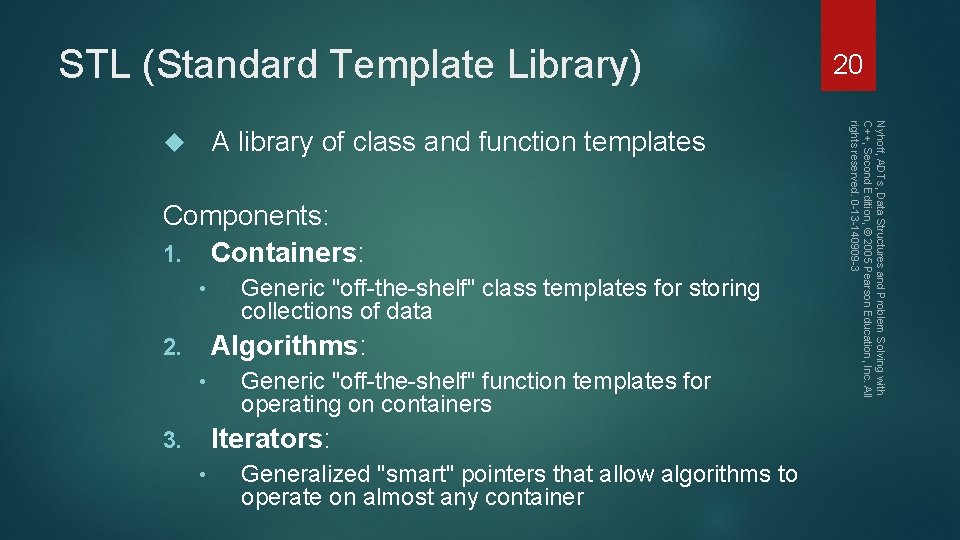
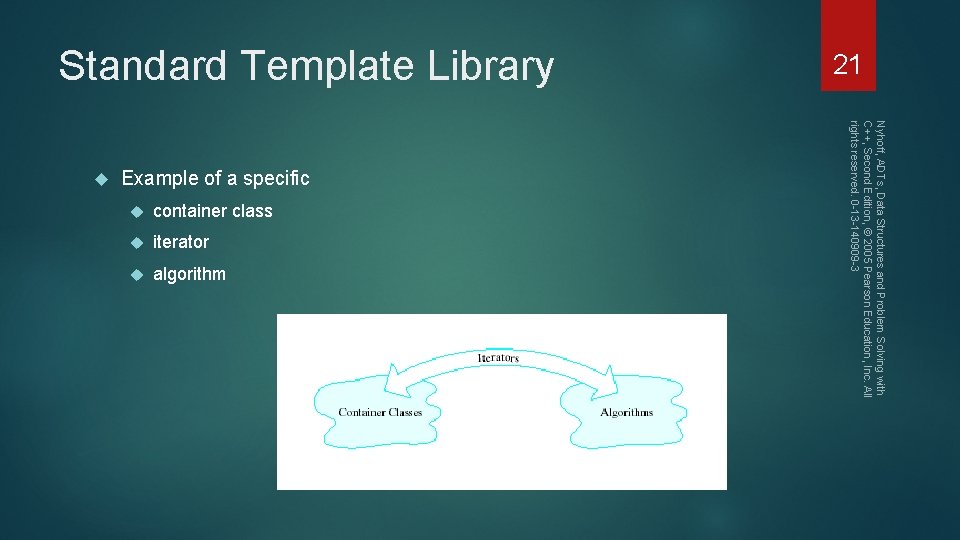
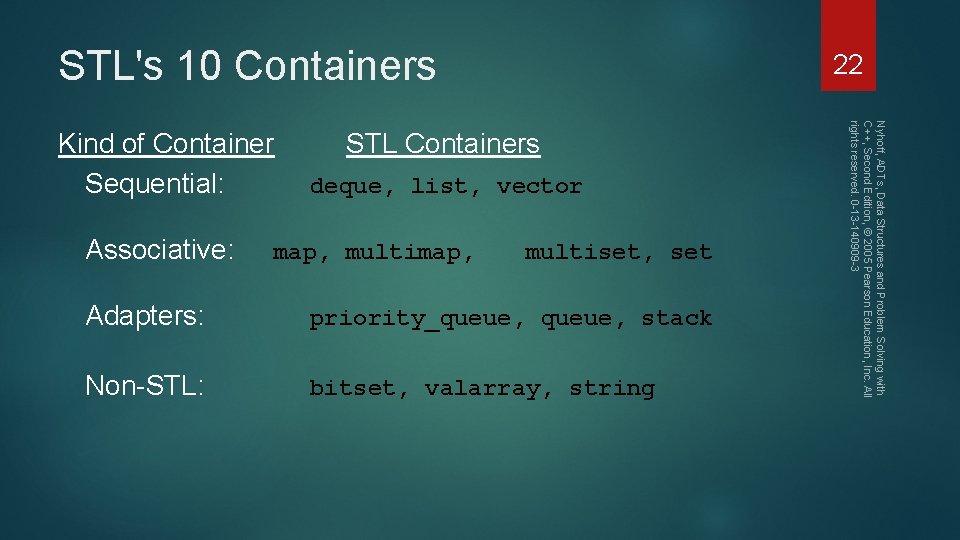
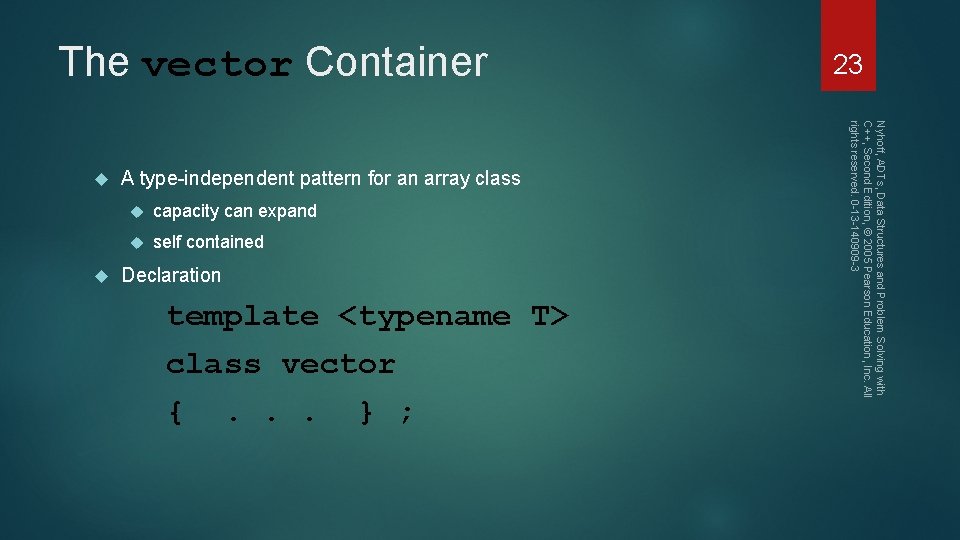
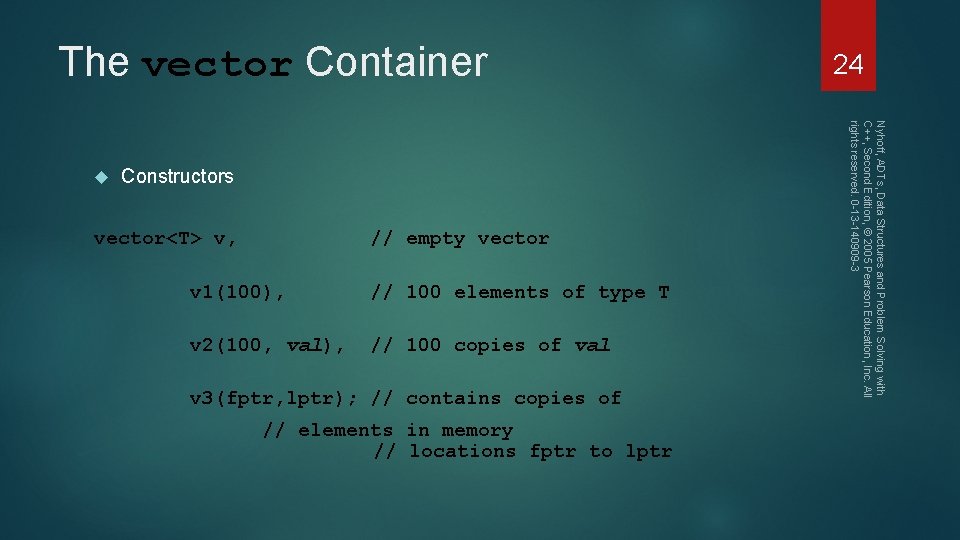
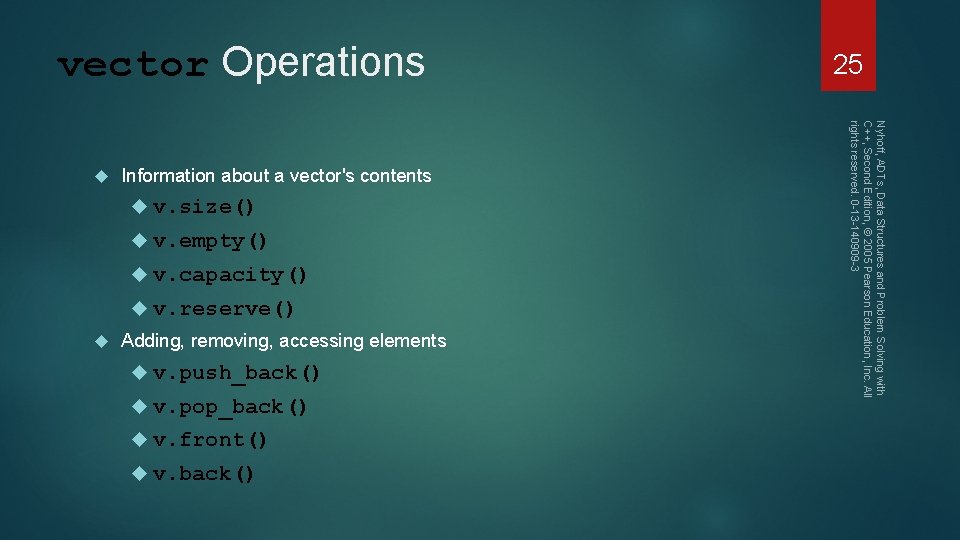
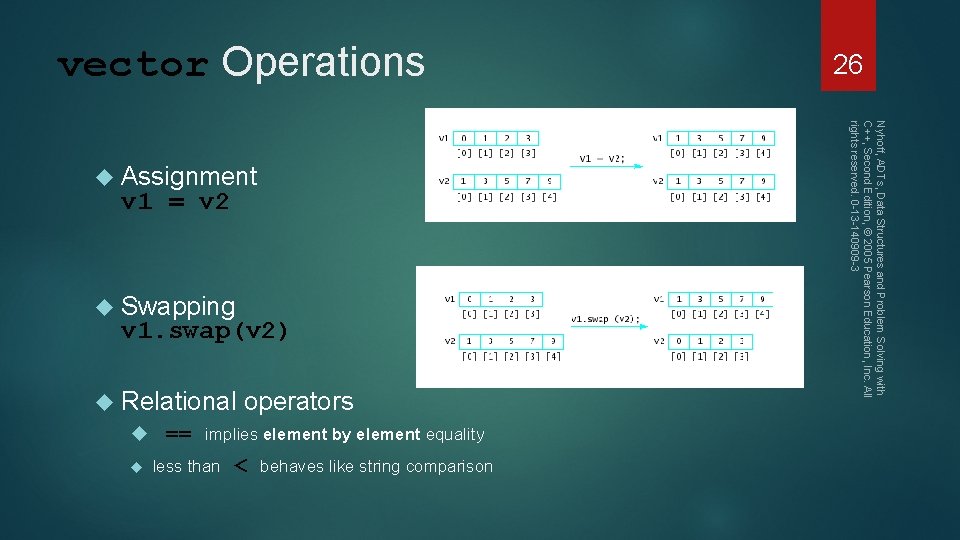
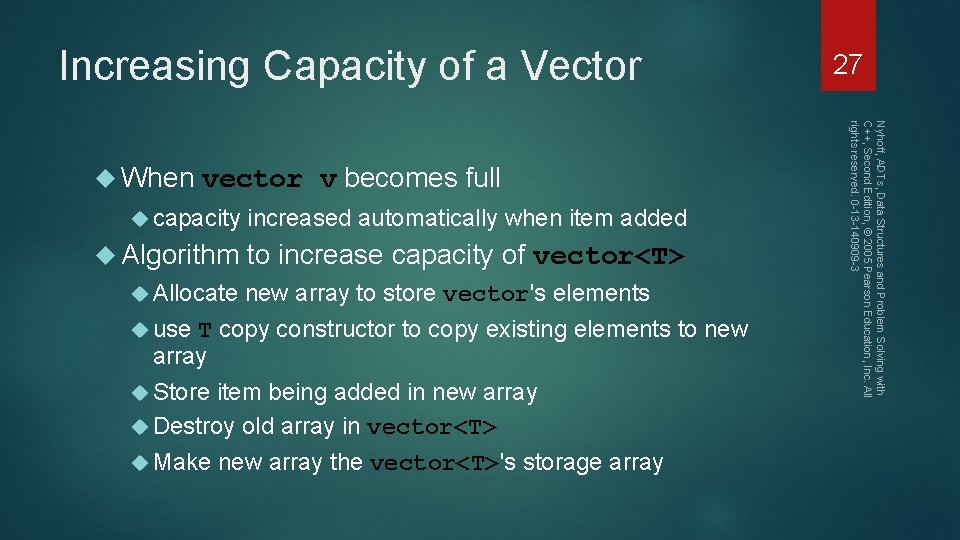
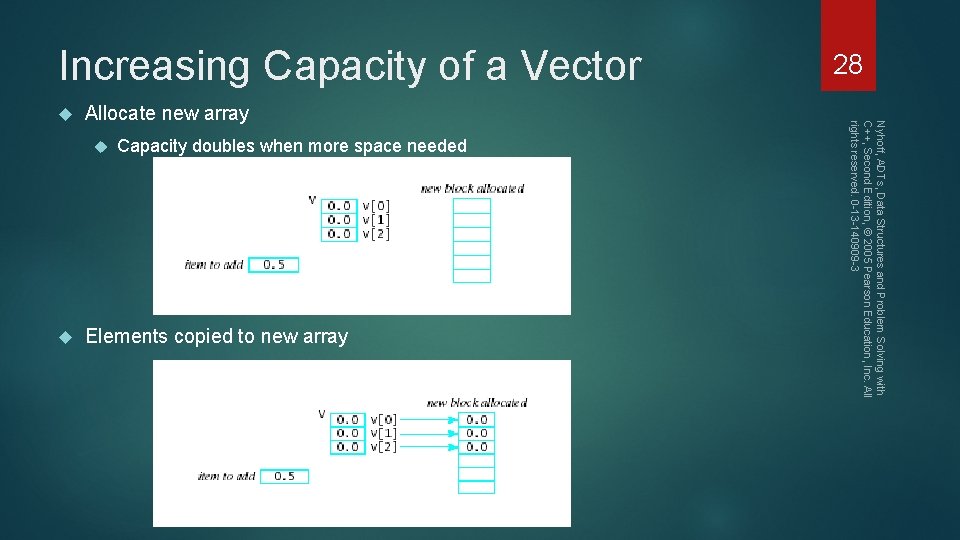
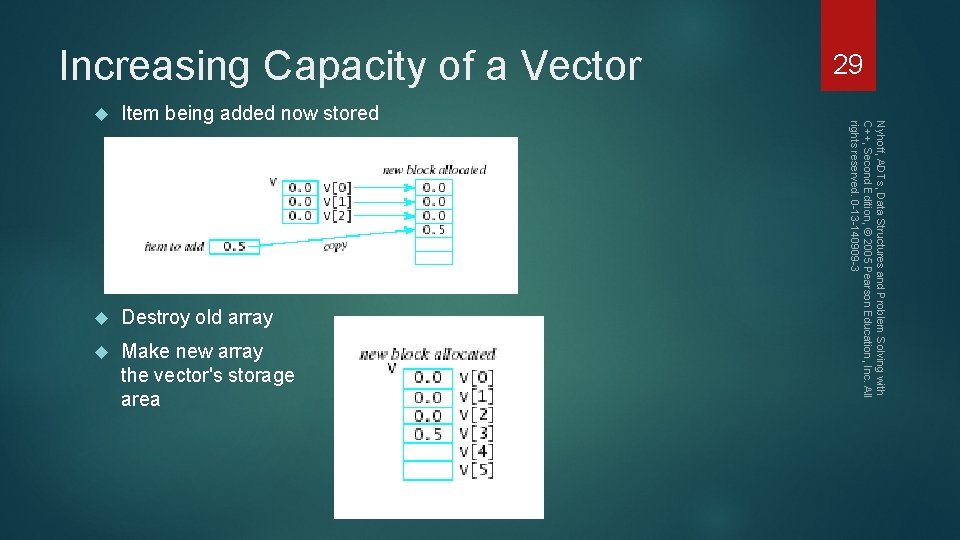
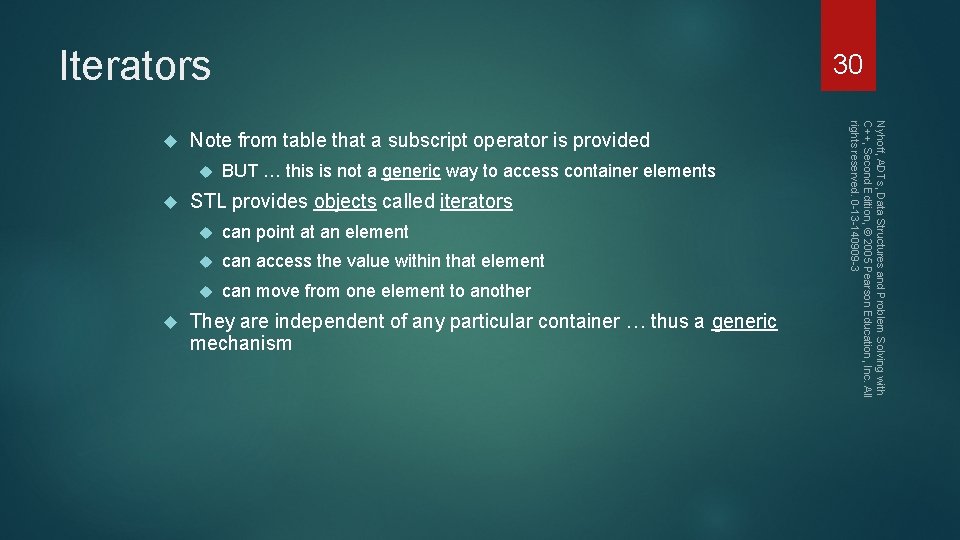
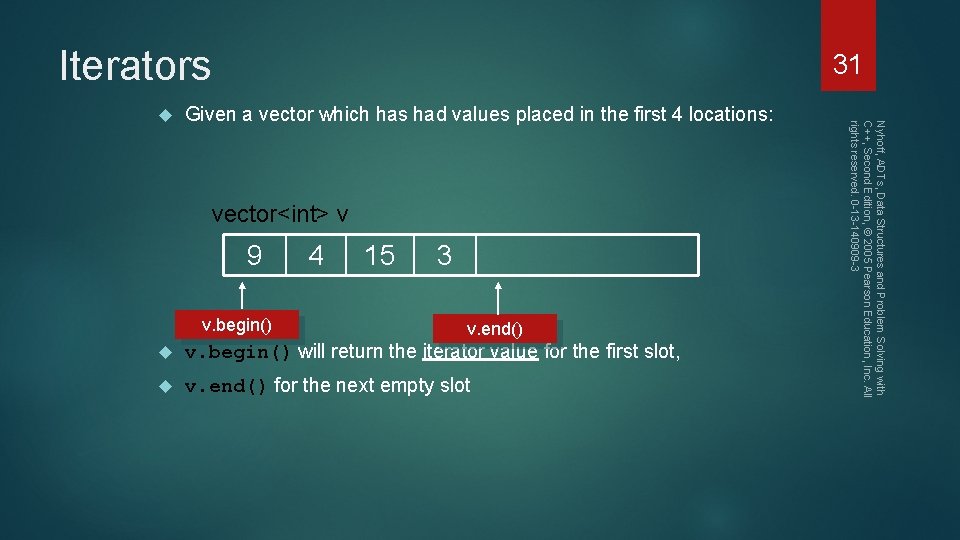
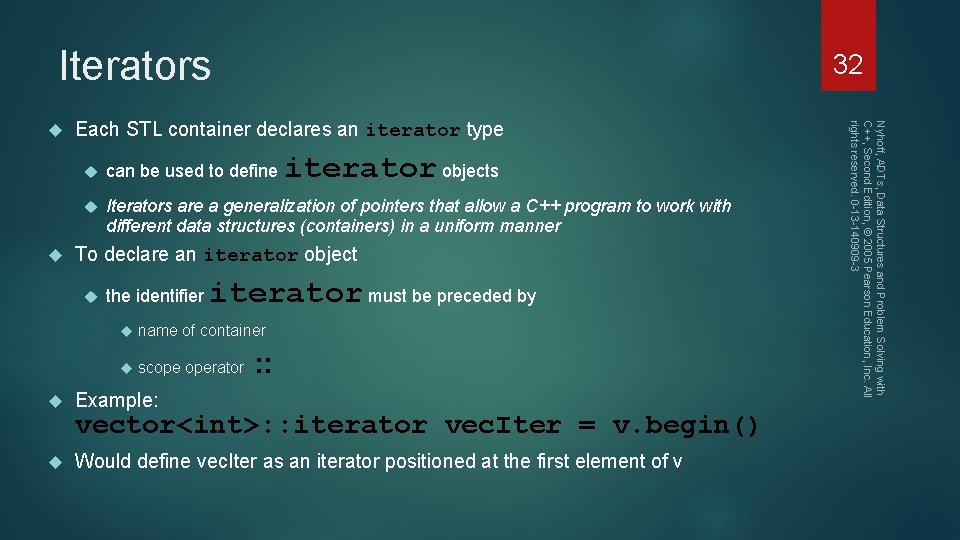
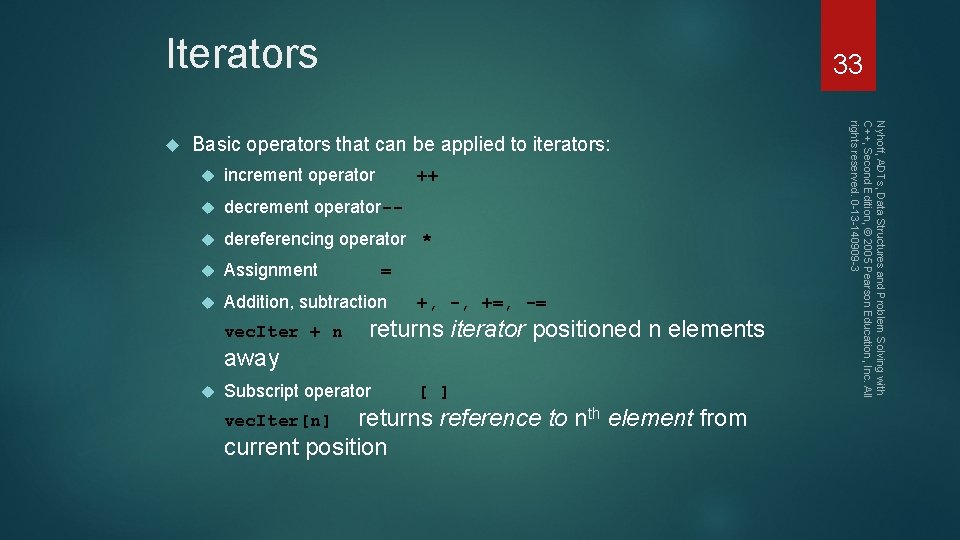
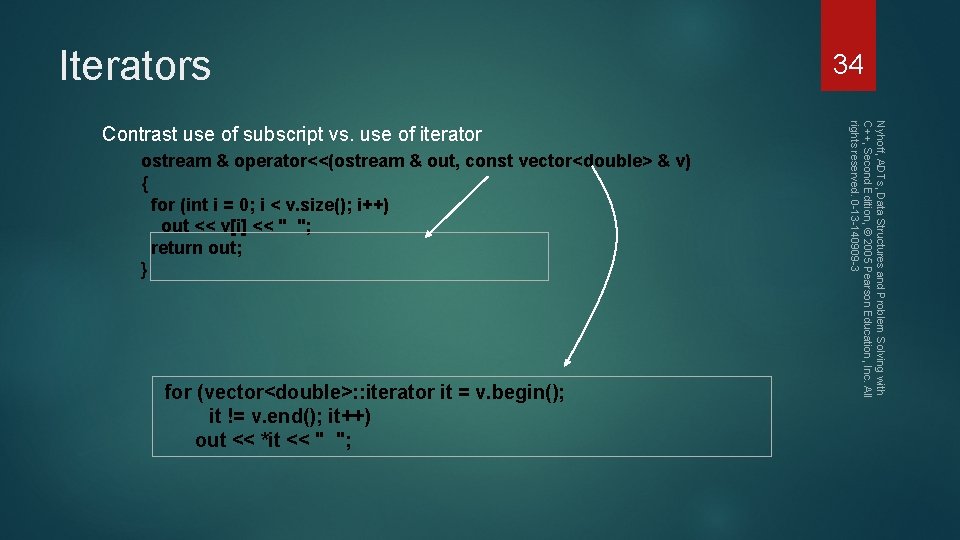
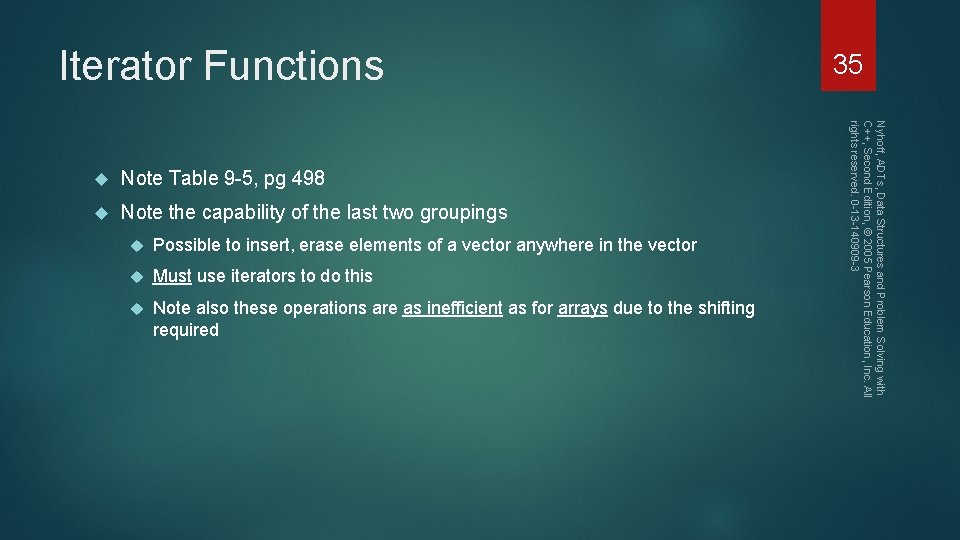
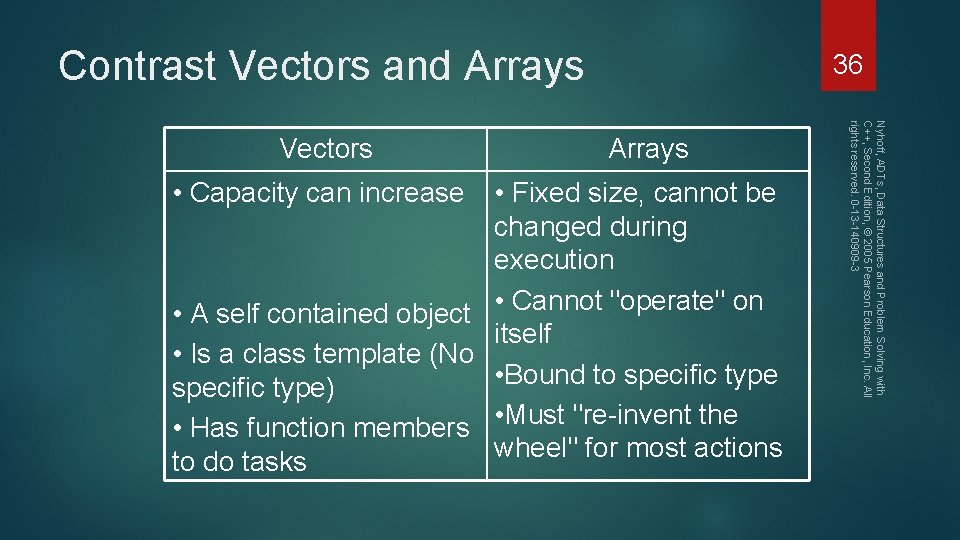
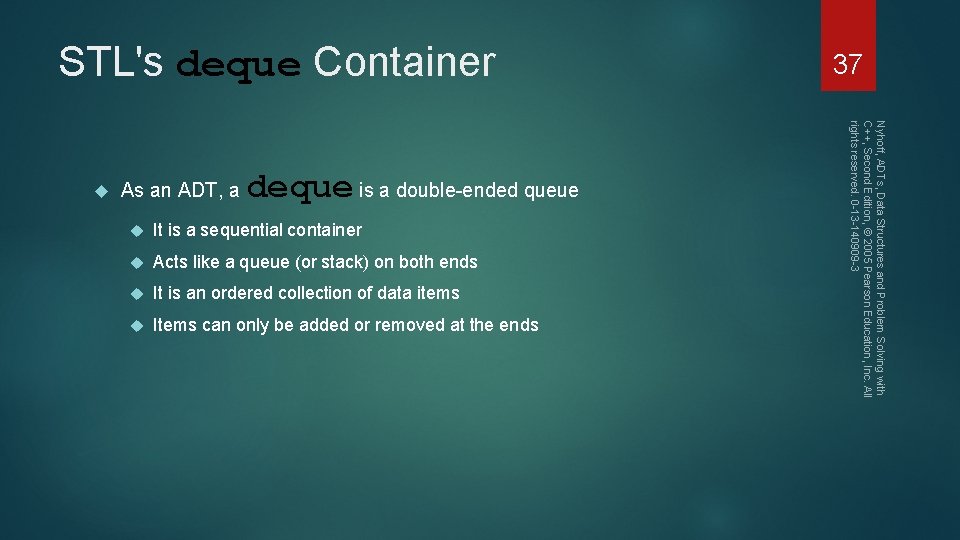
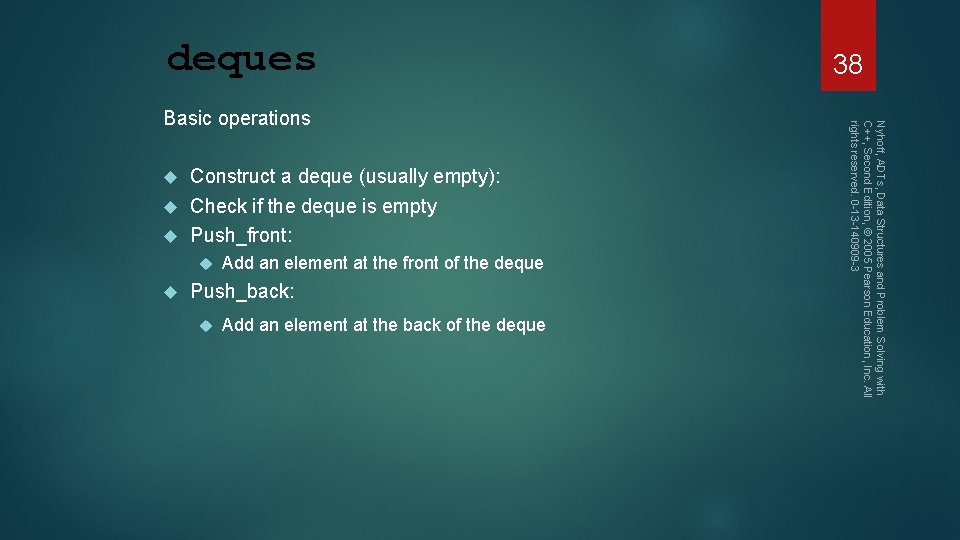
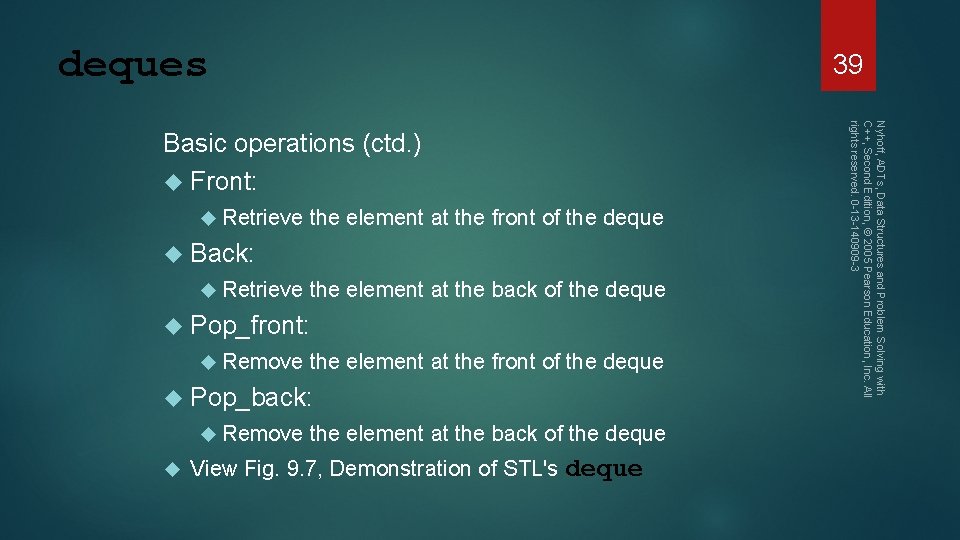
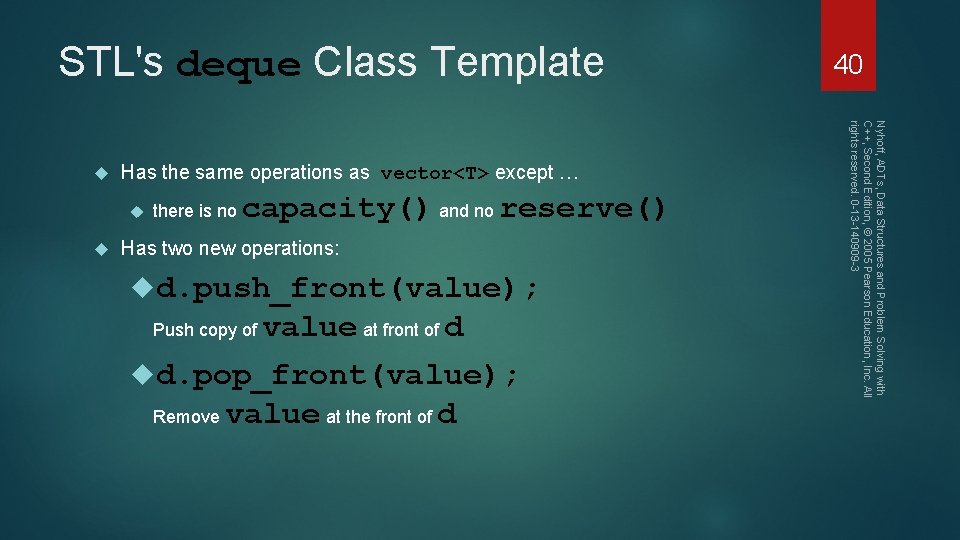
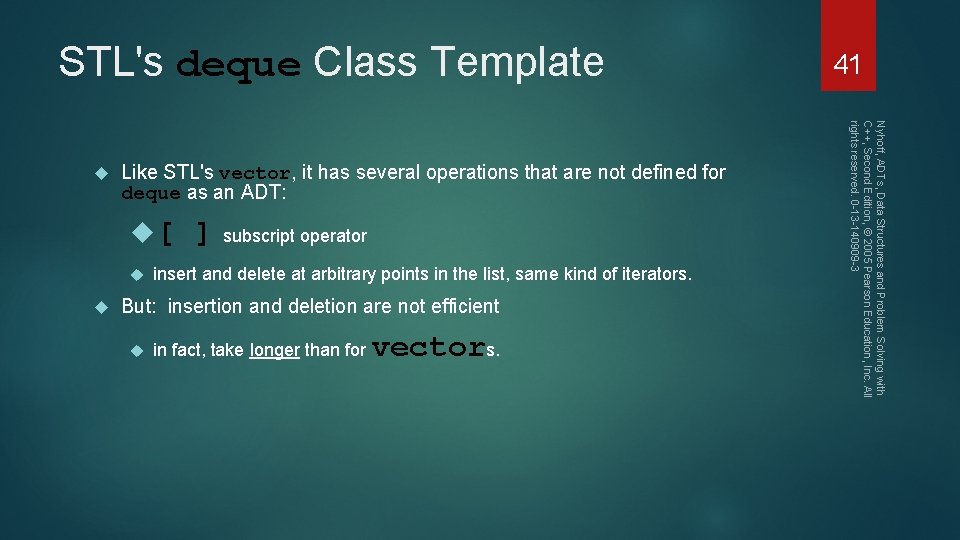
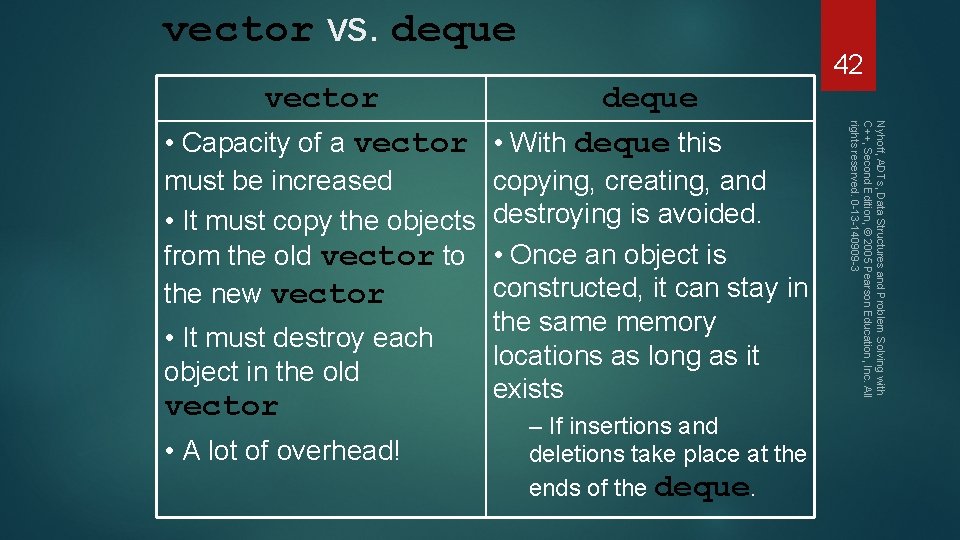
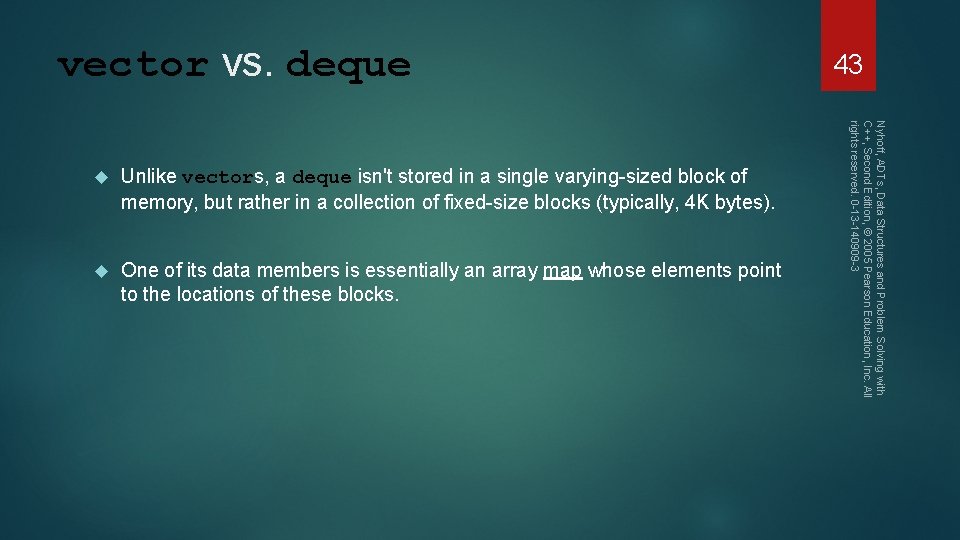
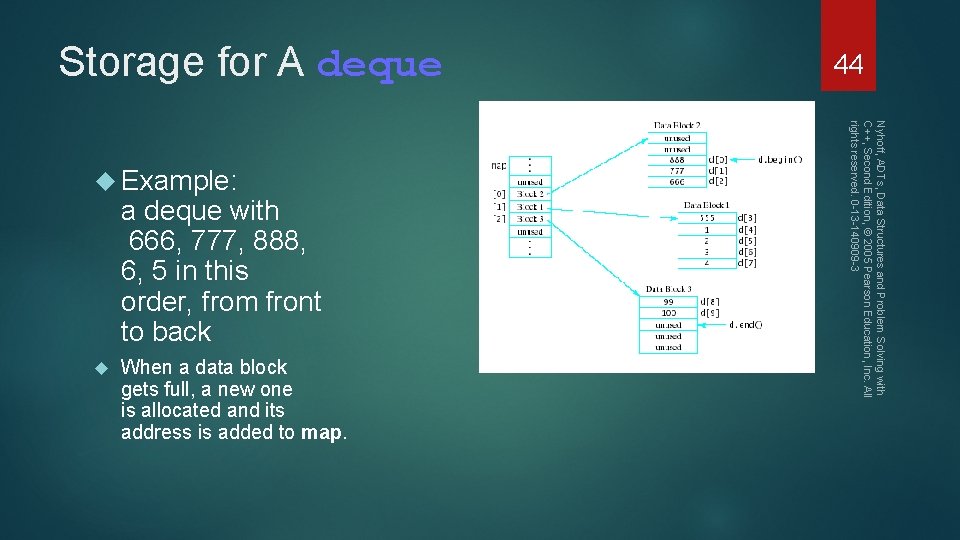
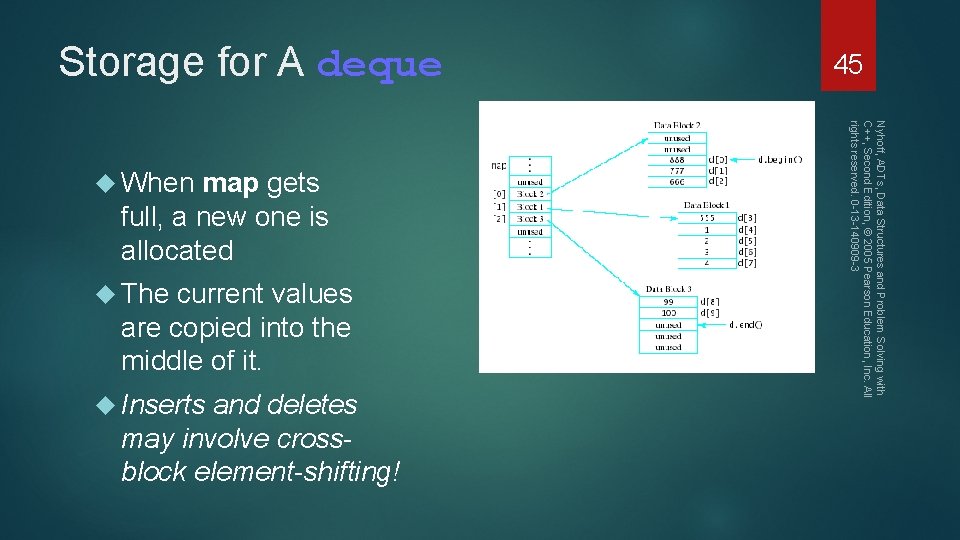
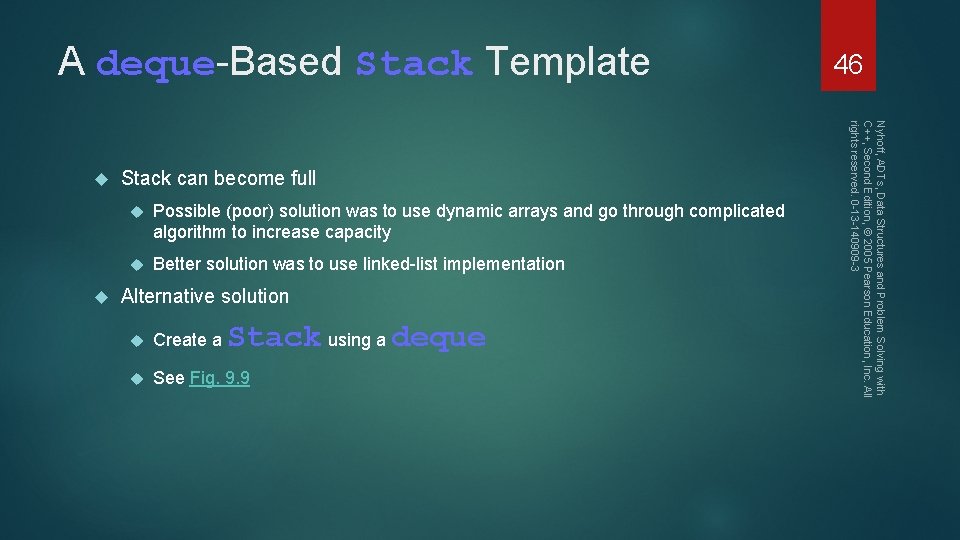
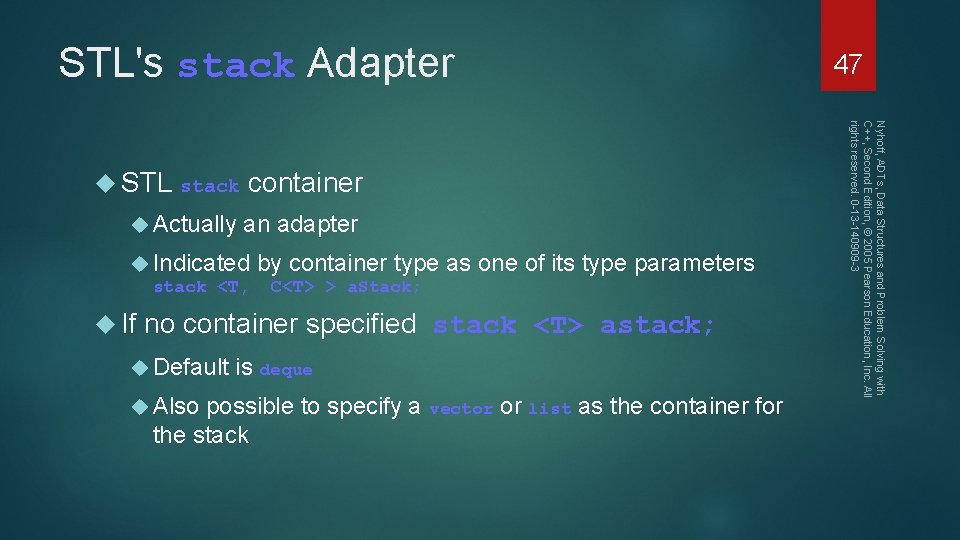
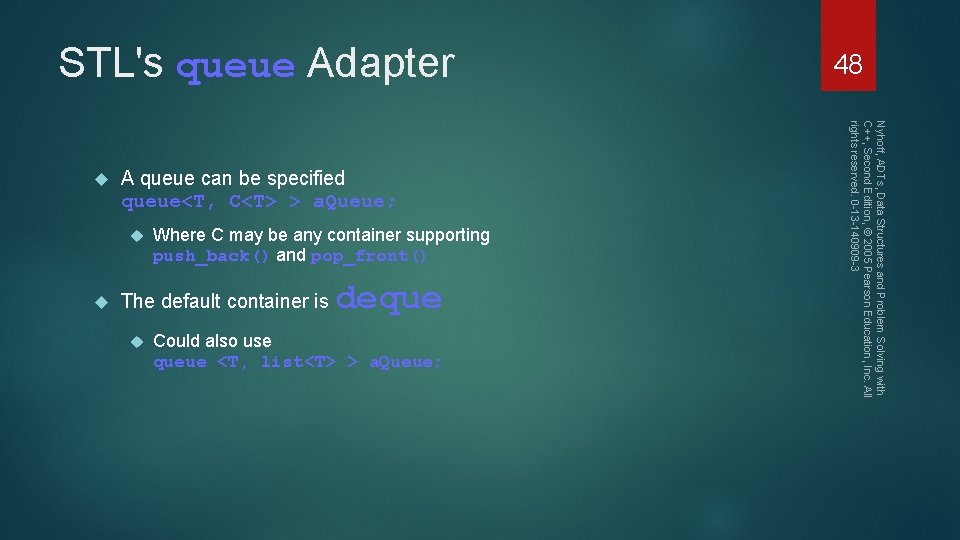
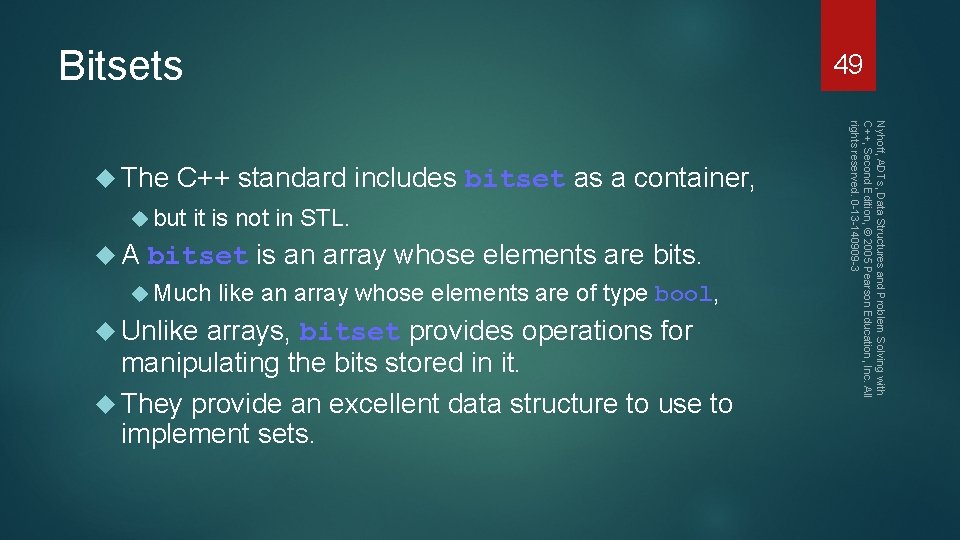
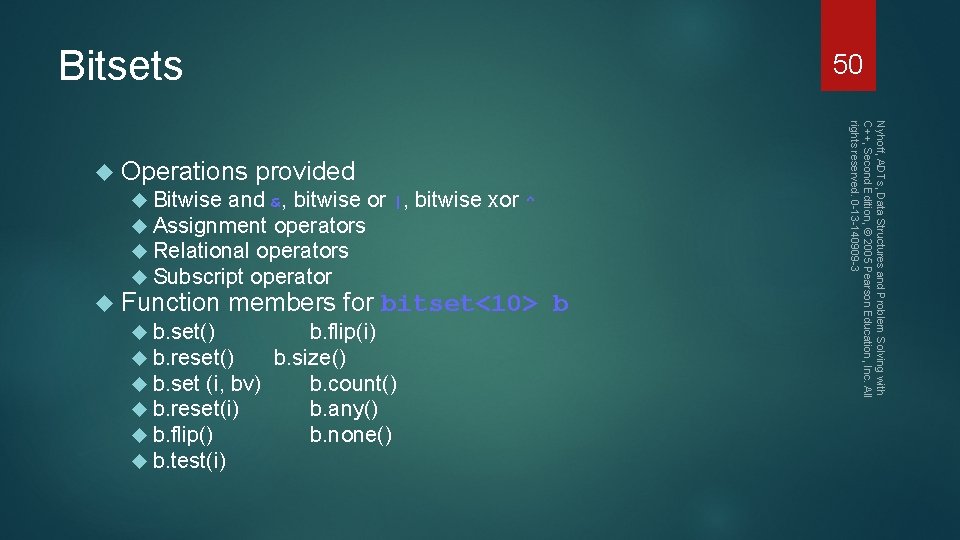
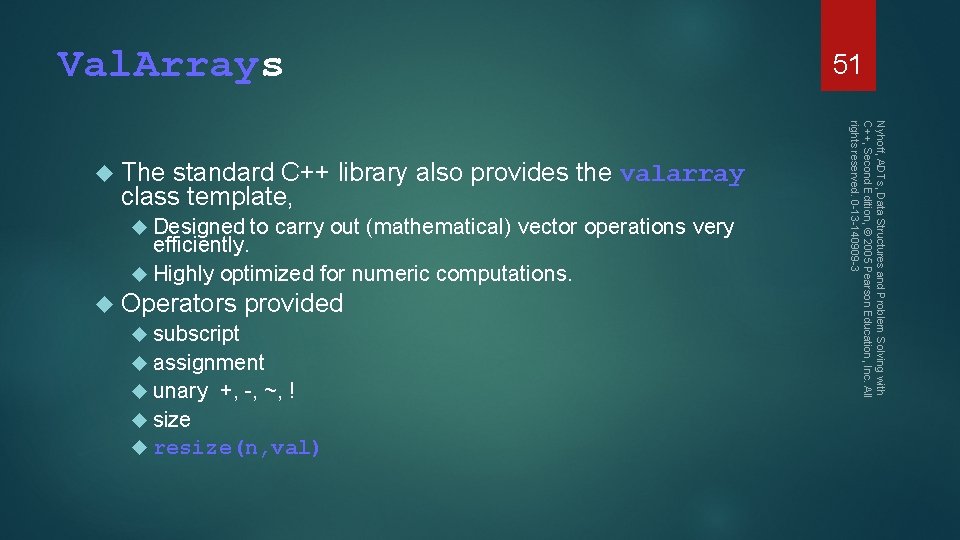
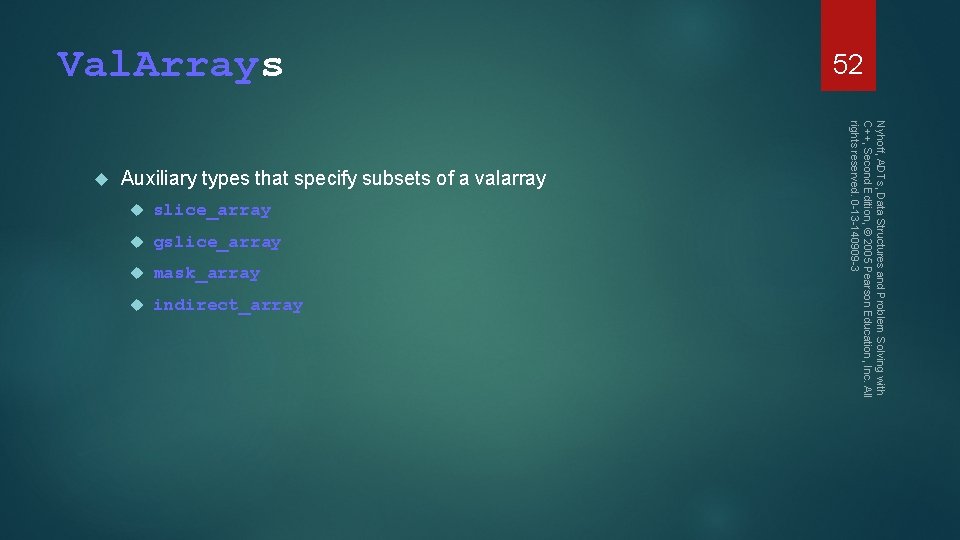
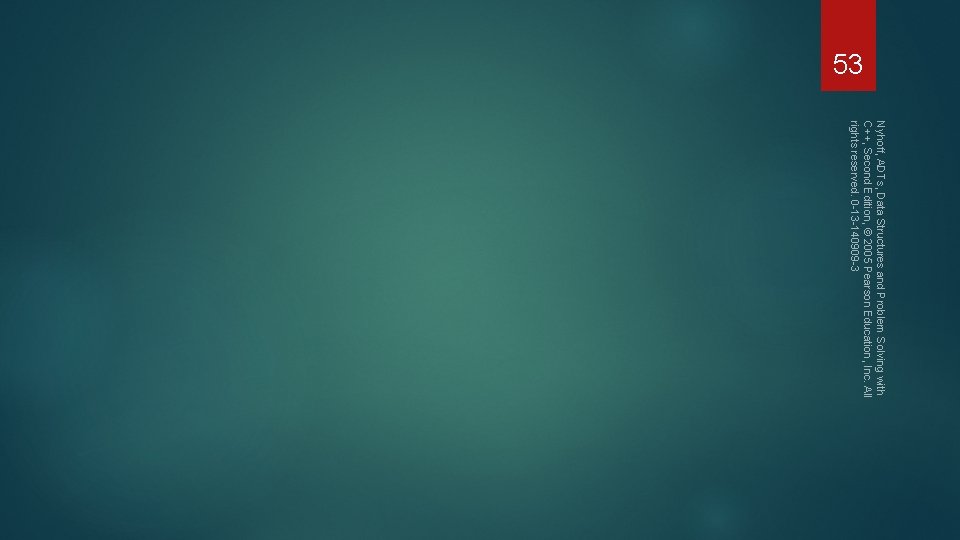
- Slides: 53
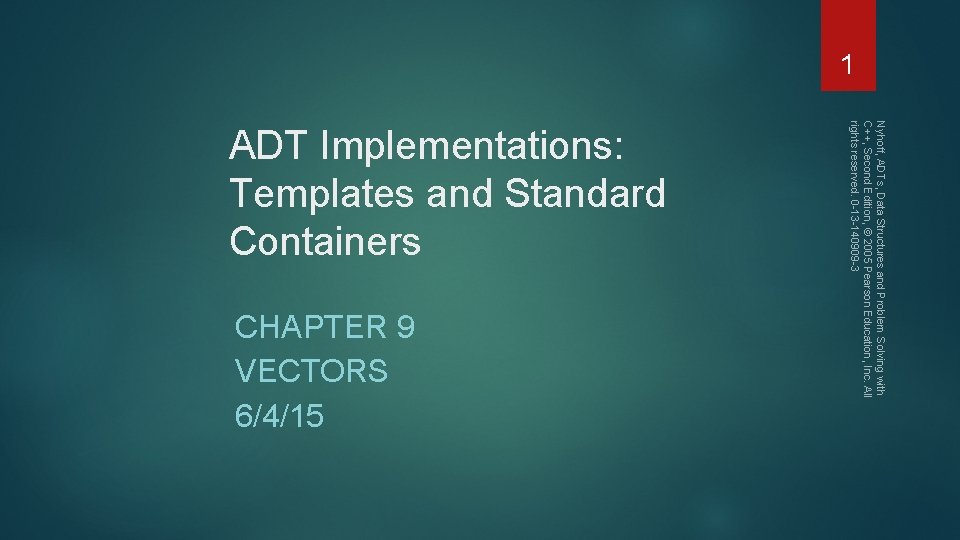
1 CHAPTER 9 VECTORS 6/4/15 Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 ADT Implementations: Templates and Standard Containers
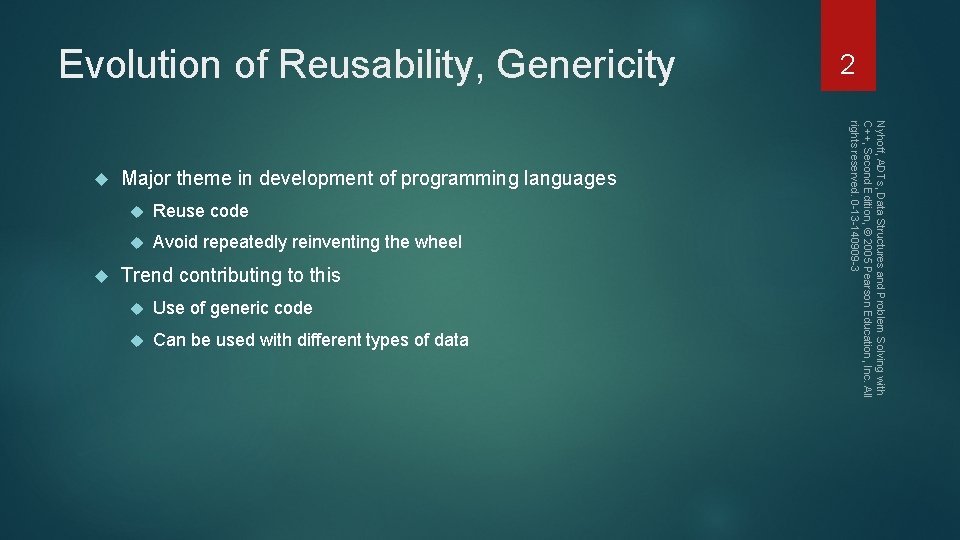
Evolution of Reusability, Genericity Major theme in development of programming languages Reuse code Avoid repeatedly reinventing the wheel Trend contributing to this Use of generic code Can be used with different types of data Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 2
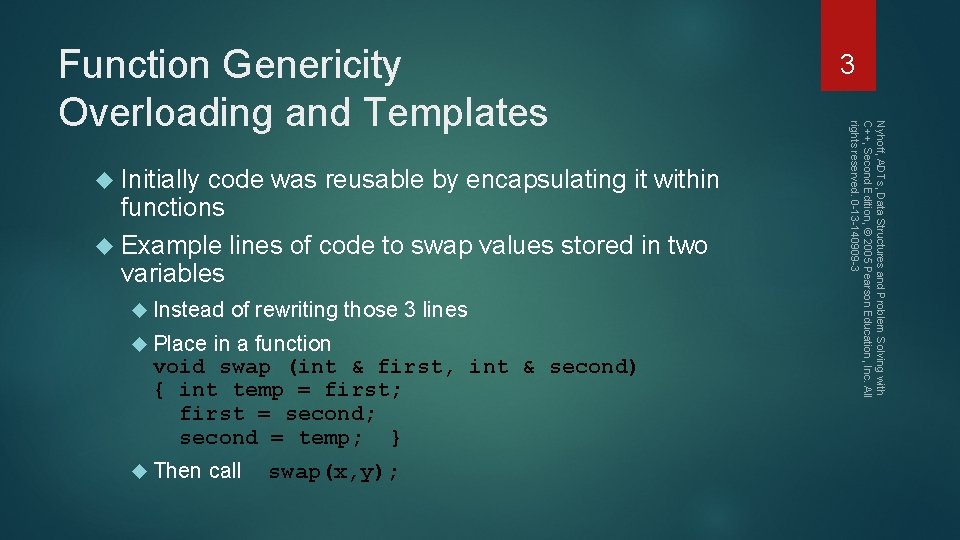
Initially code was reusable by encapsulating it within functions Example lines of code to swap values stored in two variables Instead of rewriting those 3 lines Place in a function void swap (int & first, int & second) { int temp = first; first = second; second = temp; } Then call swap(x, y); 3 Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Function Genericity Overloading and Templates
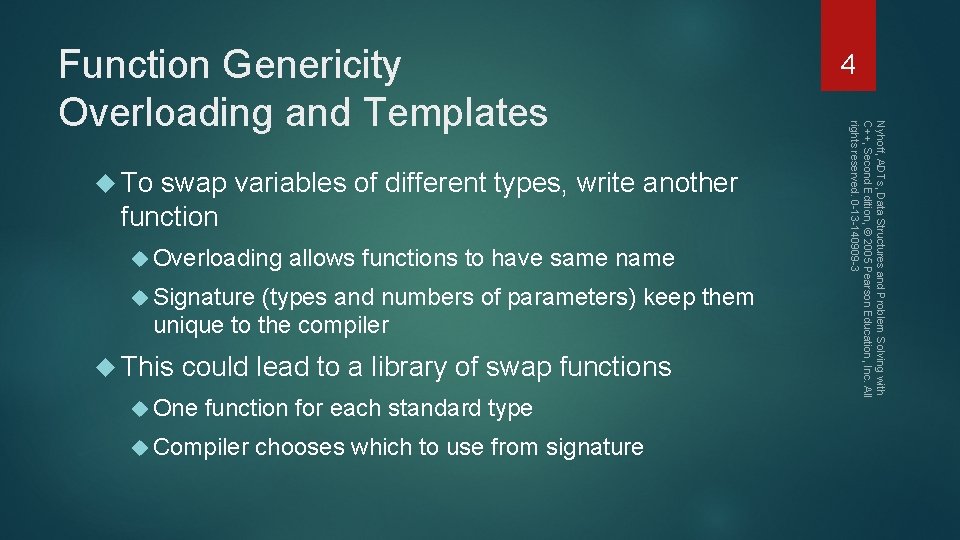
To swap variables of different types, write another function Overloading allows functions to have same name Signature (types and numbers of parameters) keep them unique to the compiler This could lead to a library of swap functions One function for each standard type Compiler chooses which to use from signature 4 Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Function Genericity Overloading and Templates
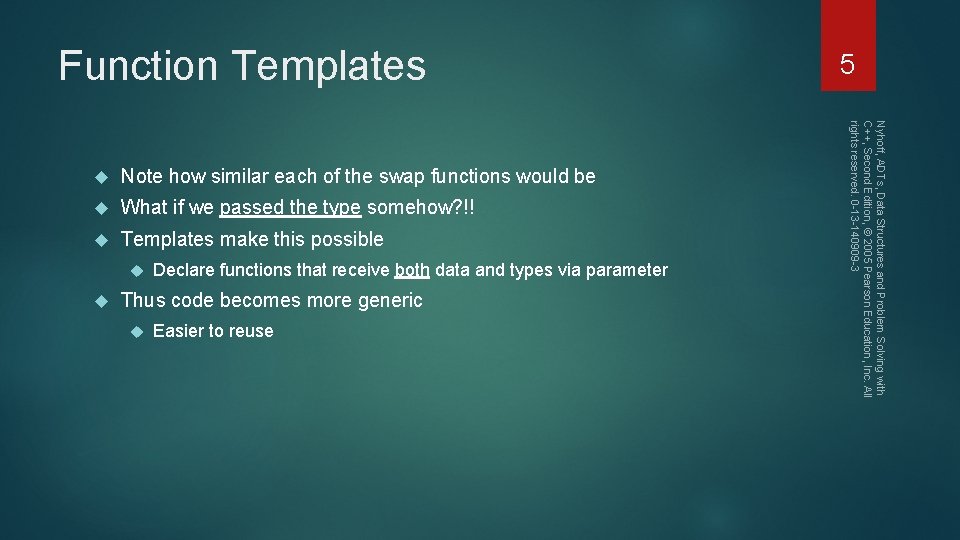
Function Templates Note how similar each of the swap functions would be What if we passed the type somehow? !! Templates make this possible Declare functions that receive both data and types via parameter Thus code becomes more generic Easier to reuse Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 5
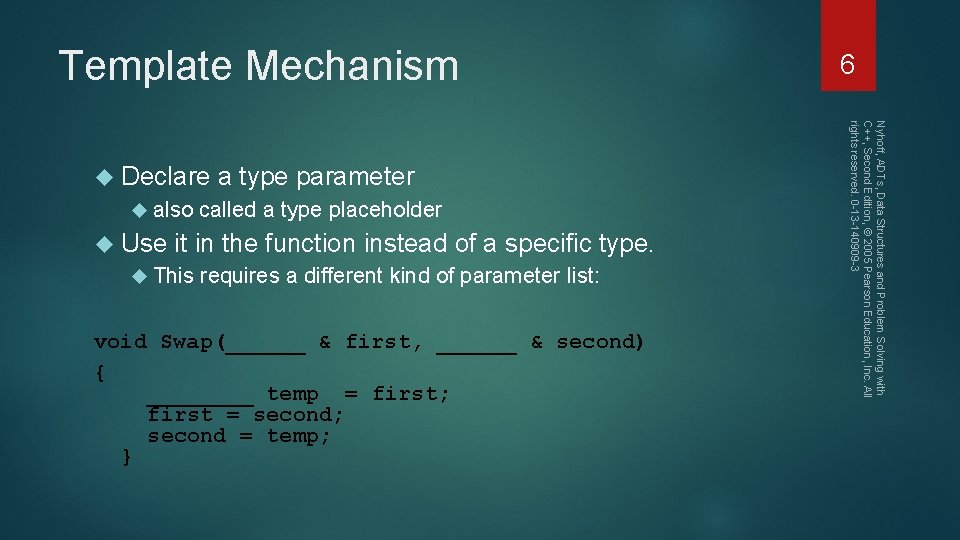
Template Mechanism Use it in the function instead of a specific type. This requires a different kind of parameter list: void Swap(______ & first, ______ & second) { ____ temp = first; first = second; second = temp; } Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Declare a type parameter also called a type placeholder 6
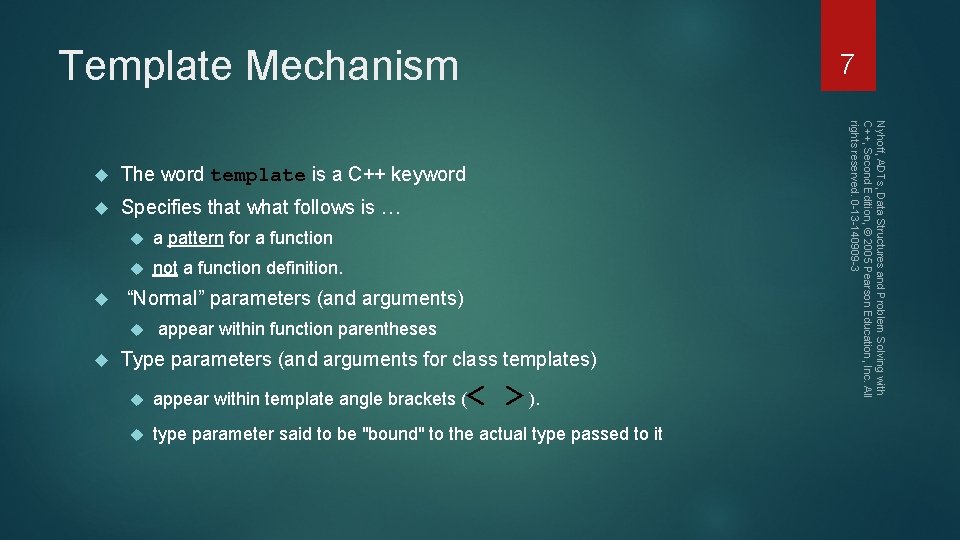
Template Mechanism The word template is a C++ keyword Specifies that what follows is … a pattern for a function not a function definition. “Normal” parameters (and arguments) appear within function parentheses Type parameters (and arguments for class templates) < > ). appear within template angle brackets ( type parameter said to be "bound" to the actual type passed to it Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 7
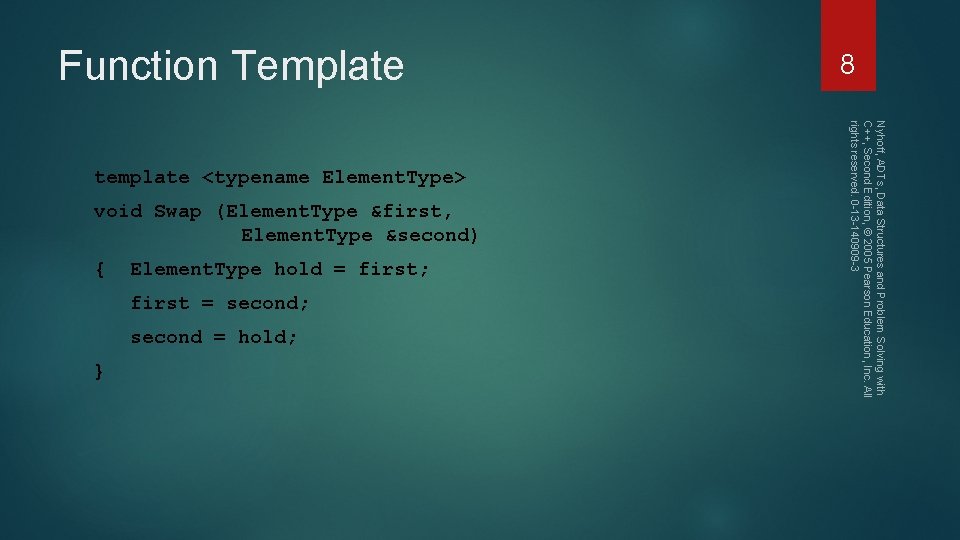
Function Template void Swap (Element. Type &first, Element. Type &second) { Element. Type hold = first; first = second; second = hold; } Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 template <typename Element. Type> 8
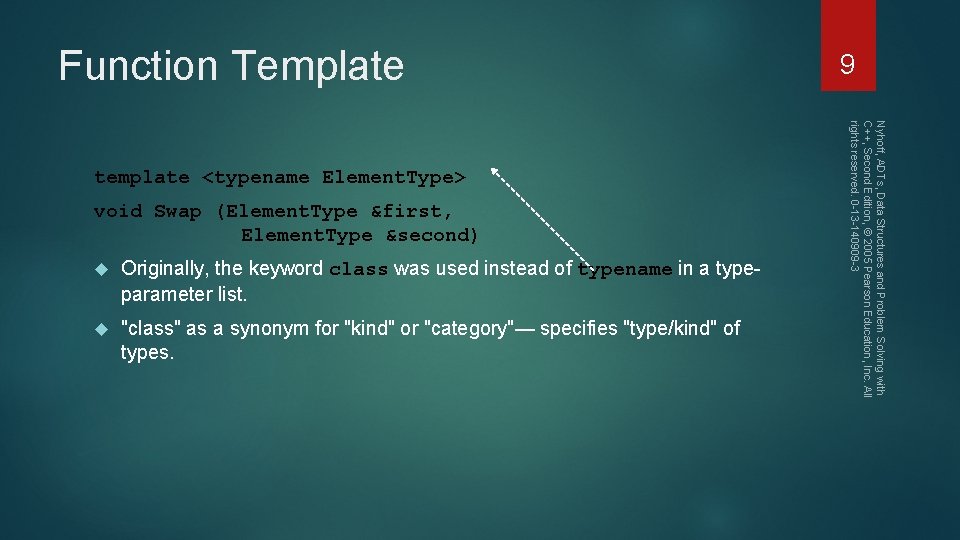
Function Template void Swap (Element. Type &first, Element. Type &second) Originally, the keyword class was used instead of typename in a typeparameter list. "class" as a synonym for "kind" or "category"— specifies "type/kind" of types. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 template <typename Element. Type> 9
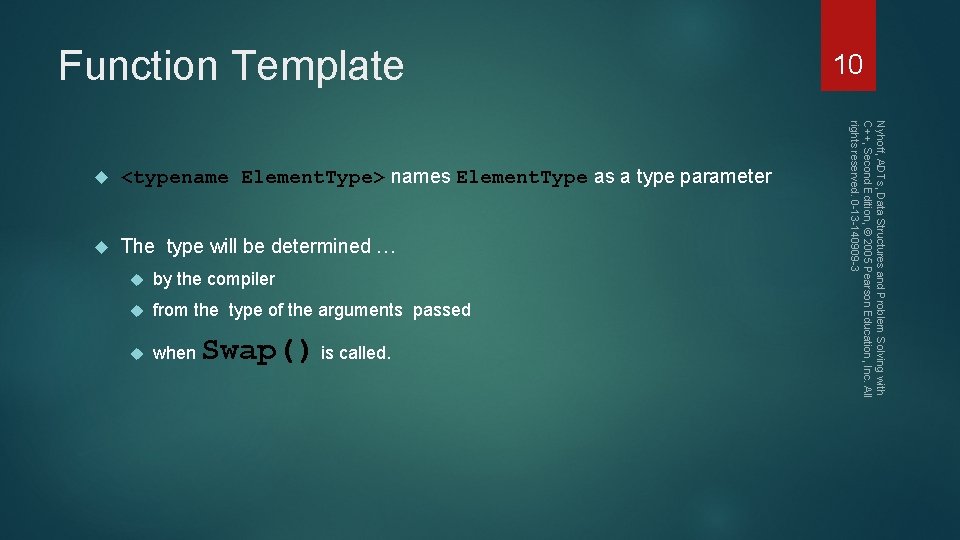
Function Template <typename Element. Type> names Element. Type as a type parameter The type will be determined … by the compiler from the type of the arguments passed when Swap() is called. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 10
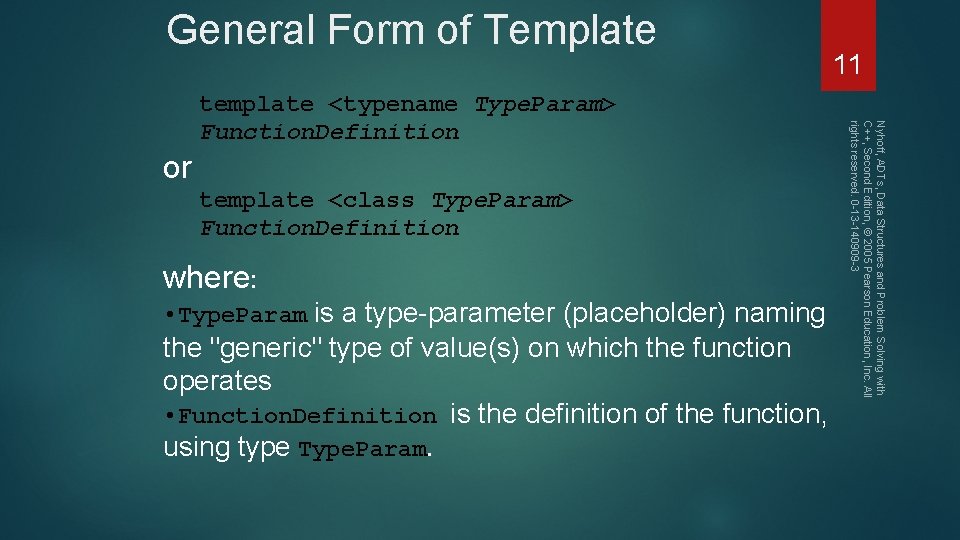
General Form of Template or template <class Type. Param> Function. Definition where: • Type. Param is a type-parameter (placeholder) naming the "generic" type of value(s) on which the function operates • Function. Definition is the definition of the function, using type Type. Param. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 template <typename Type. Param> Function. Definition 11
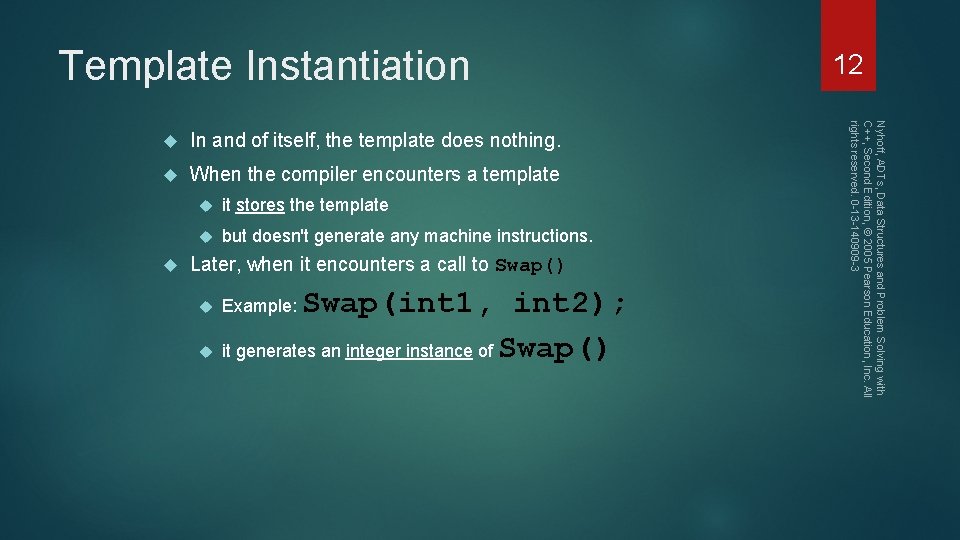
Template Instantiation In and of itself, the template does nothing. When the compiler encounters a template it stores the template but doesn't generate any machine instructions. Later, when it encounters a call to Swap() Swap(int 1, int 2); it generates an integer instance of Swap() Example: Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 12
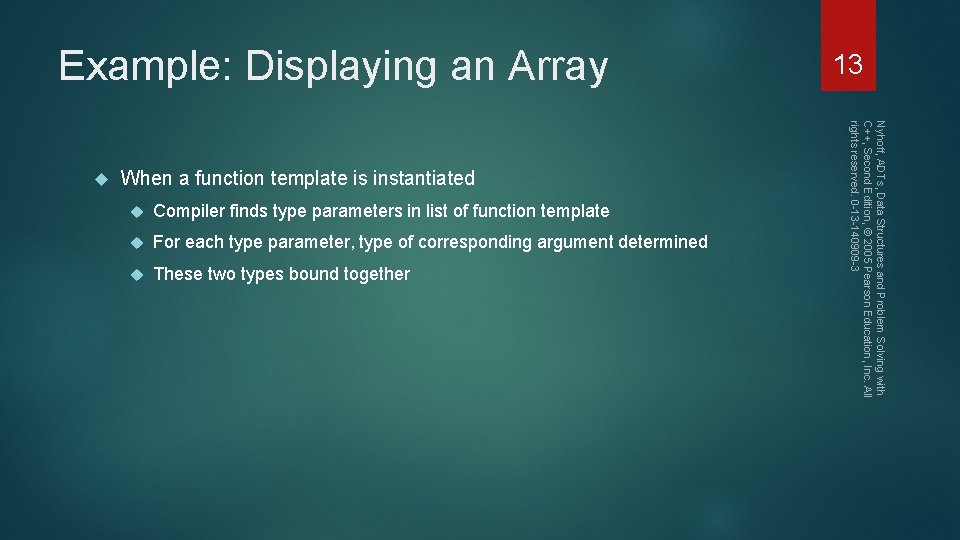
Example: Displaying an Array When a function template is instantiated Compiler finds type parameters in list of function template For each type parameter, type of corresponding argument determined These two types bound together Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 13
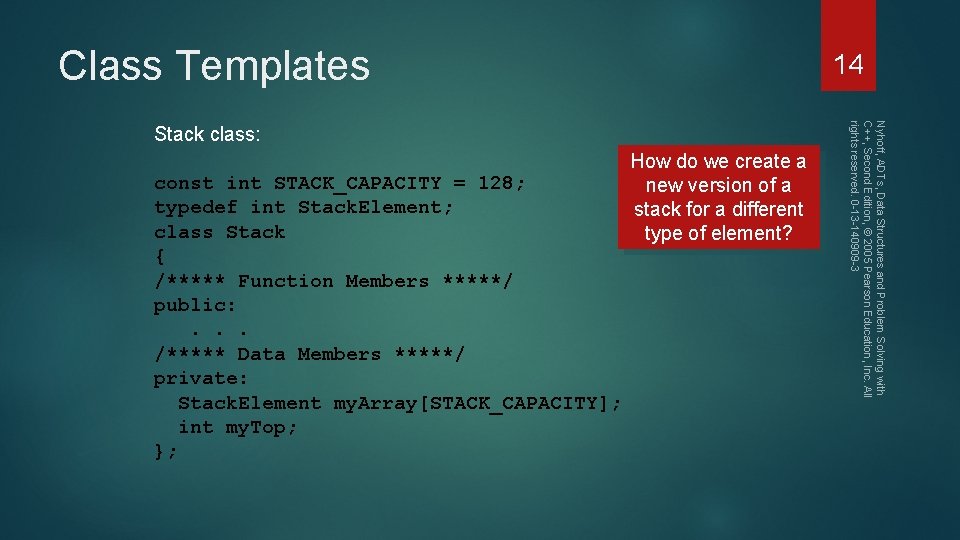
Class Templates 14 const int STACK_CAPACITY = 128; typedef int Stack. Element; class Stack { /***** Function Members *****/ public: . . . /***** Data Members *****/ private: Stack. Element my. Array[STACK_CAPACITY]; int my. Top; }; How do we create a new version of a stack for a different type of element? Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Stack class:
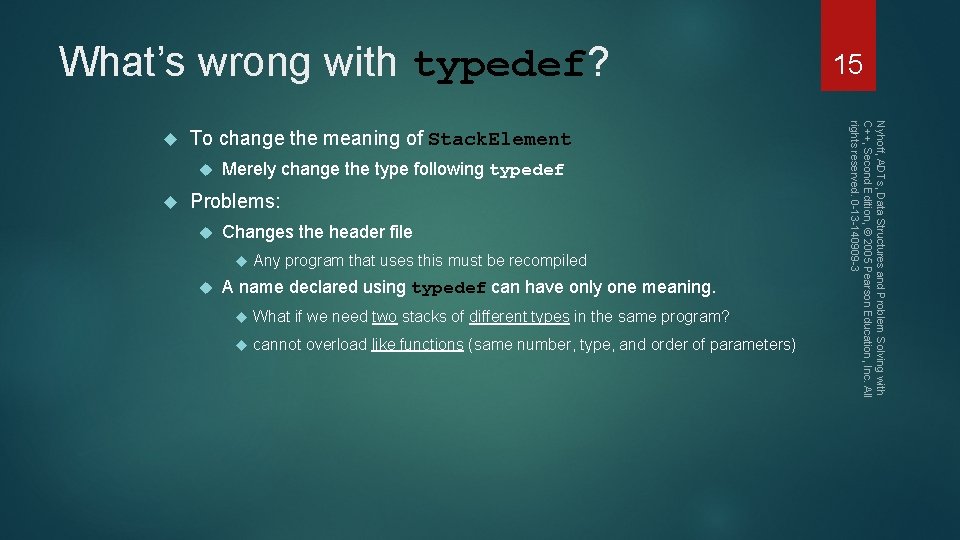
What’s wrong with typedef? To change the meaning of Stack. Element Merely change the type following typedef Problems: Changes the header file Any program that uses this must be recompiled A name declared using typedef can have only one meaning. What if we need two stacks of different types in the same program? cannot overload like functions (same number, type, and order of parameters) Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 15
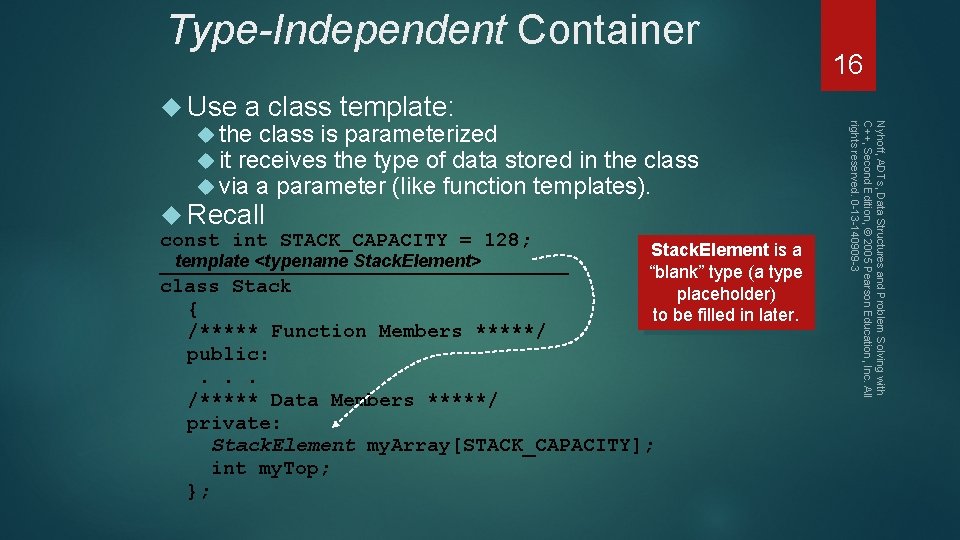
Type-Independent Container const int STACK_CAPACITY = 128; Stack. Element is a template <typename Stack. Element> _________________ “blank” type (a type class Stack placeholder) { to be filled in later. /***** Function Members *****/ public: . . . /***** Data Members *****/ private: Stack. Element my. Array[STACK_CAPACITY]; int my. Top; }; Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Use a class template: the class is parameterized it receives the type of data stored in the class via a parameter (like function templates). Recall 16
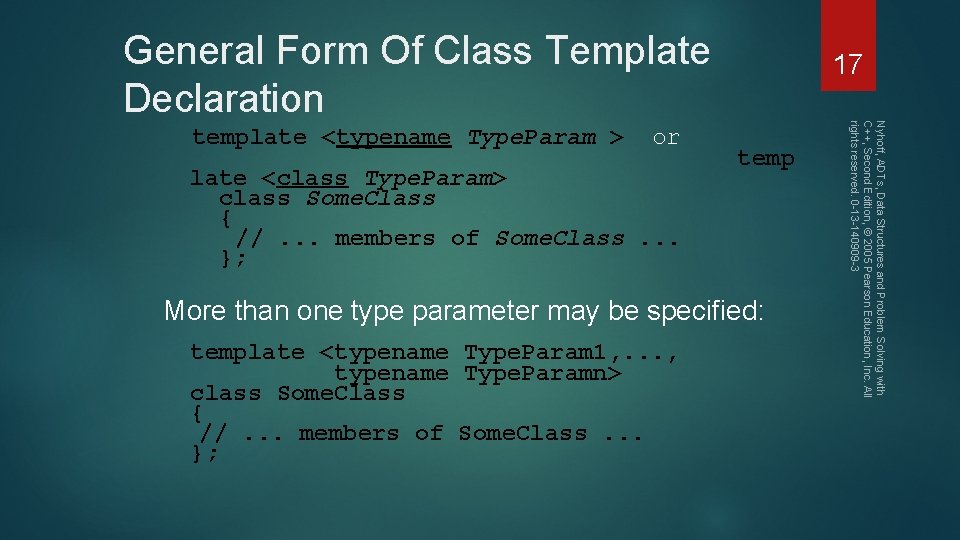
General Form Of Class Template Declaration or late <class Type. Param> class Some. Class { //. . . members of Some. Class. . . }; temp More than one type parameter may be specified: template <typename Type. Param 1, . . . , typename Type. Paramn> class Some. Class { //. . . members of Some. Class. . . }; Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 template <typename Type. Param > 17
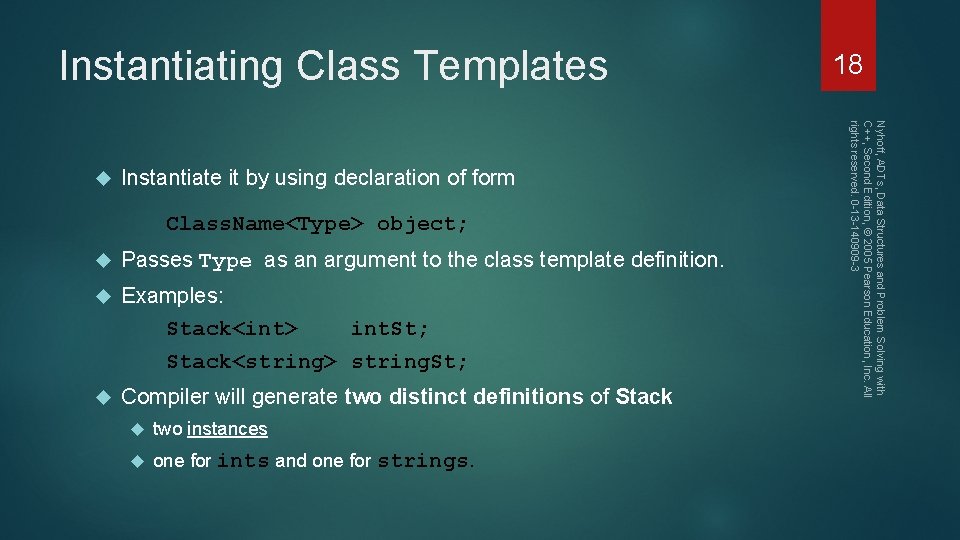
Instantiating Class Templates Instantiate it by using declaration of form Class. Name<Type> object; Passes Type as an argument to the class template definition. Examples: Stack<int> int. St; Stack<string> string. St; Compiler will generate two distinct definitions of Stack two instances one for ints and one for strings. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 18
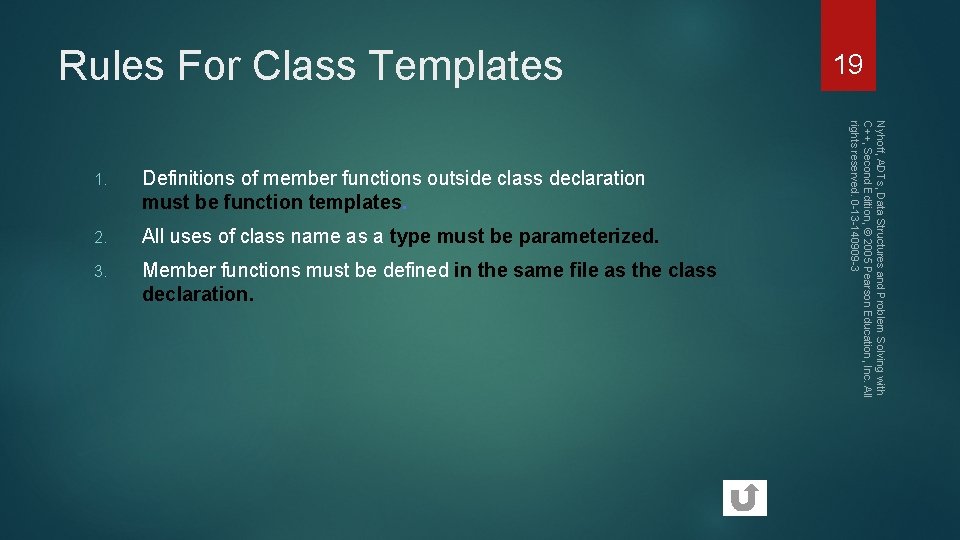
Rules For Class Templates Definitions of member functions outside class declaration must be function templates. 2. All uses of class name as a type must be parameterized. 3. Member functions must be defined in the same file as the class declaration. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 1. 19
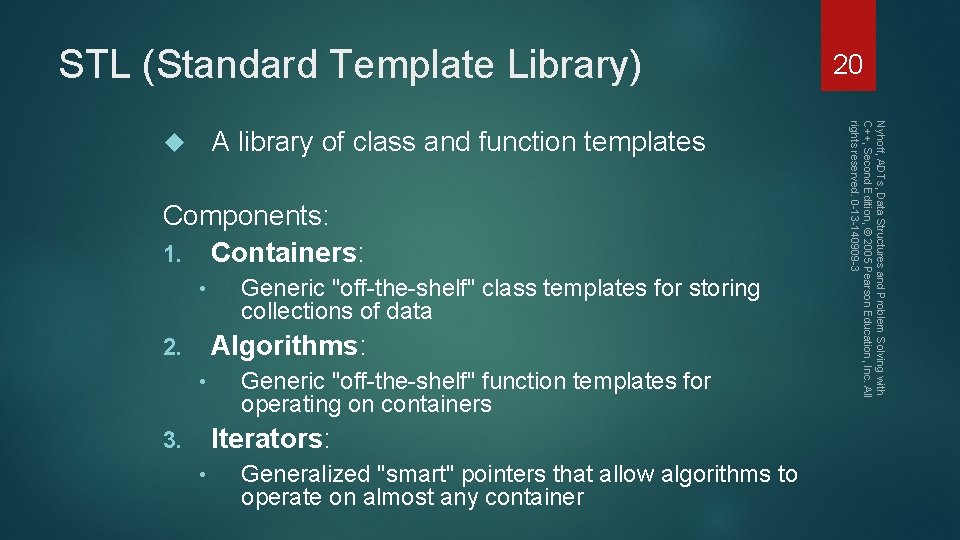
STL (Standard Template Library) Components: 1. Containers: • Generic "off-the-shelf" class templates for storing collections of data Algorithms: 2. • Generic "off-the-shelf" function templates for operating on containers Iterators: 3. • Generalized "smart" pointers that allow algorithms to operate on almost any container Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 A library of class and function templates 20
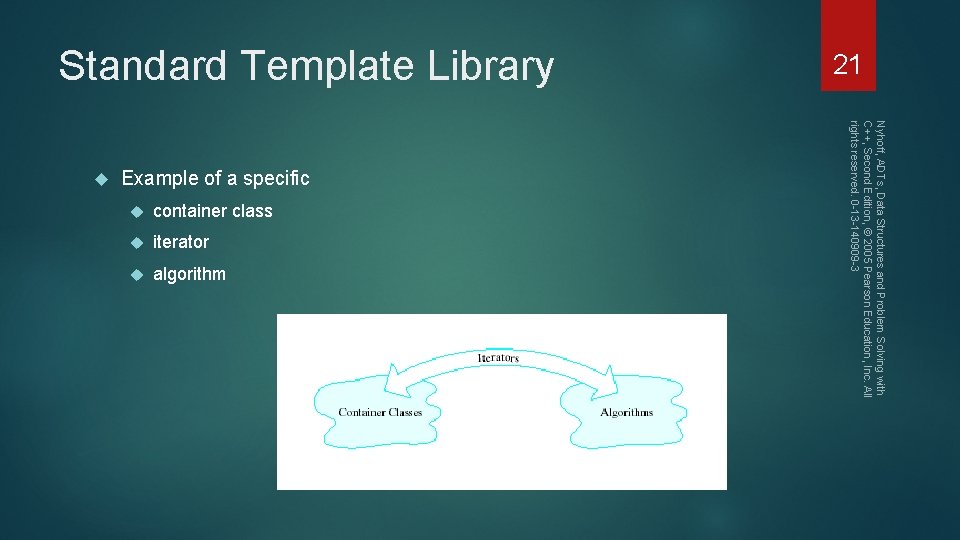
container class iterator algorithm Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Example of a specific 21 Standard Template Library
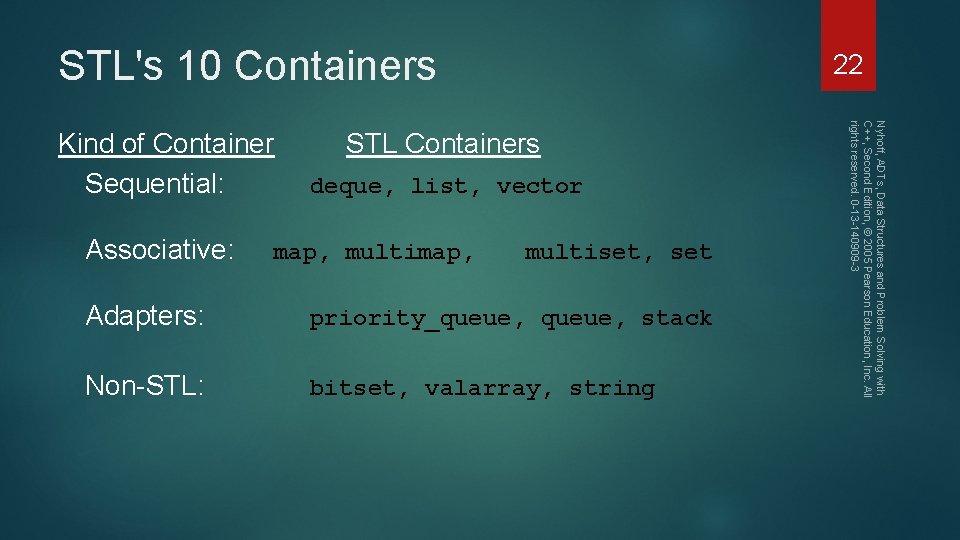
STL's 10 Containers Associative: STL Containers deque, list, vector map, multimap, multiset, set Adapters: priority_queue, stack Non-STL: bitset, valarray, string Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Kind of Container Sequential: 22
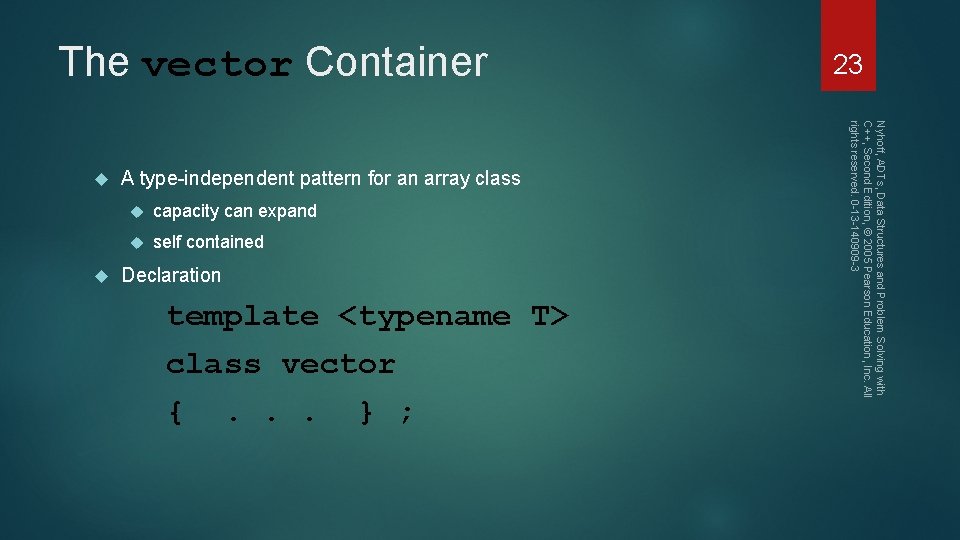
The vector Container A type-independent pattern for an array class capacity can expand self contained Declaration template <typename T> class vector { . . . } ; Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 23
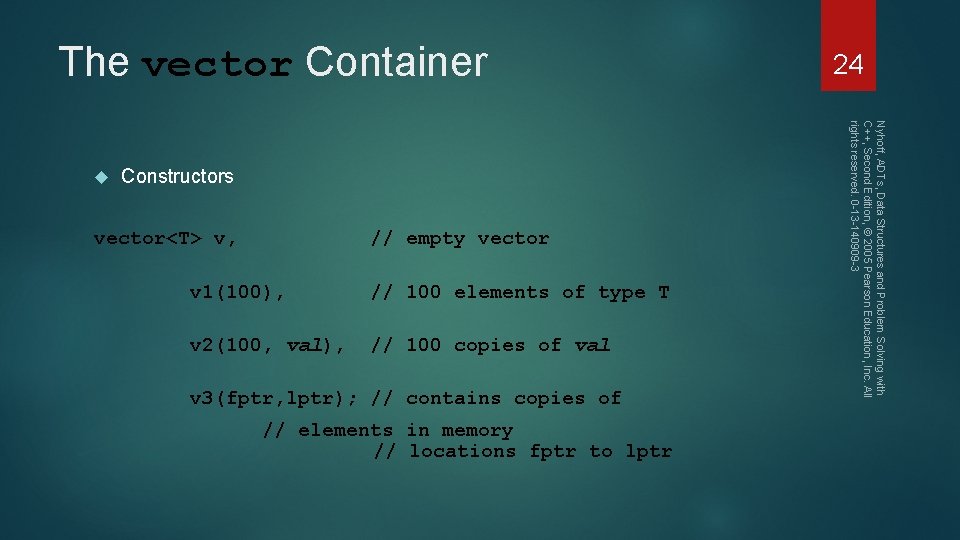
The vector Container Constructors vector<T> v, // empty vector v 1(100), // 100 elements of type T v 2(100, val), // 100 copies of val v 3(fptr, lptr); // contains copies of // elements in memory // locations fptr to lptr Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 24
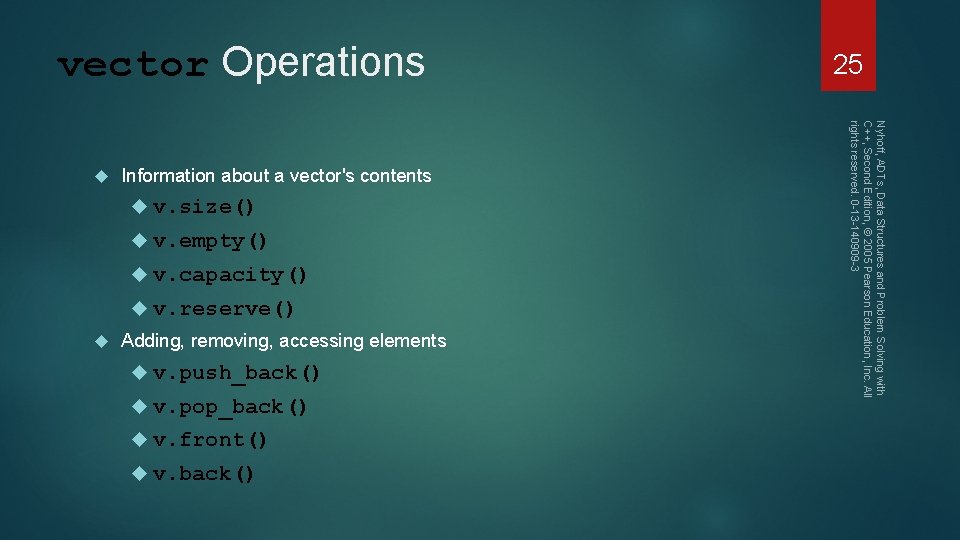
vector Operations Information about a vector's contents v. size() v. empty() v. capacity() v. reserve() Adding, removing, accessing elements v. push_back() v. pop_back() v. front() v. back() Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 25
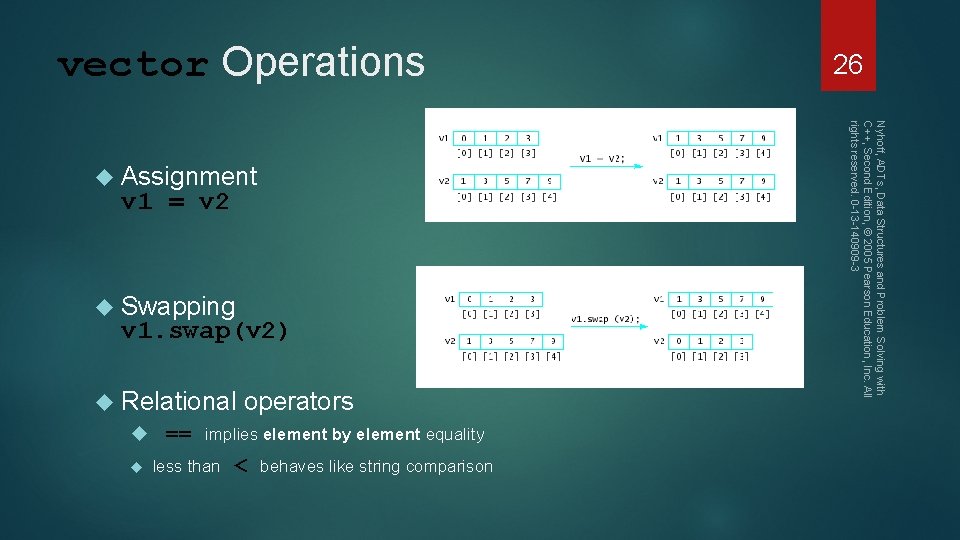
vector Operations v 1 = v 2 Swapping v 1. swap(v 2) Relational operators == implies element by element equality less than < behaves like string comparison Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Assignment 26
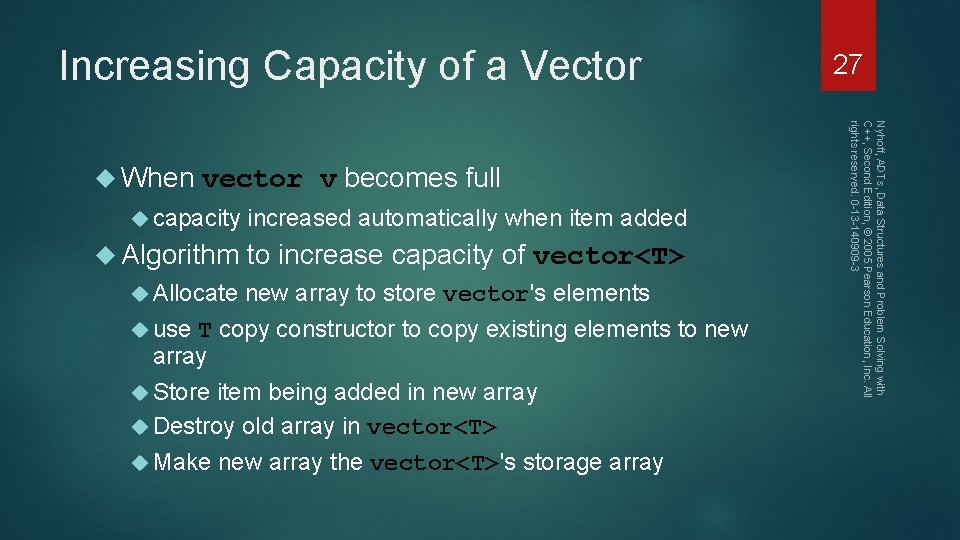
Increasing Capacity of a Vector v becomes full capacity increased automatically when item added Algorithm to increase capacity of vector<T> Allocate new array to store vector's elements use T copy constructor to copy existing elements to new array Store item being added in new array Destroy old array in vector<T> Make new array the vector<T>'s storage array Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 When 27
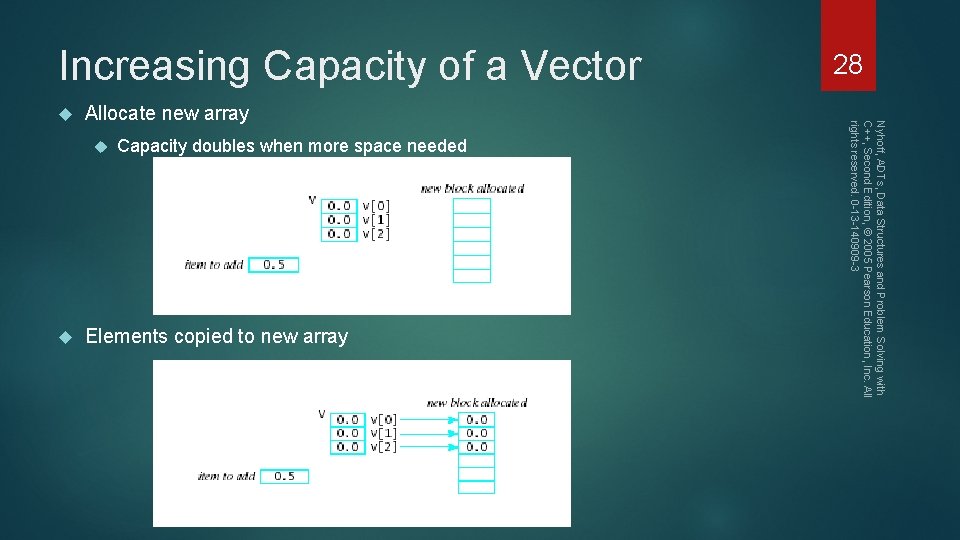
Capacity doubles when more space needed Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Elements copied to new array Allocate new array 28 Increasing Capacity of a Vector
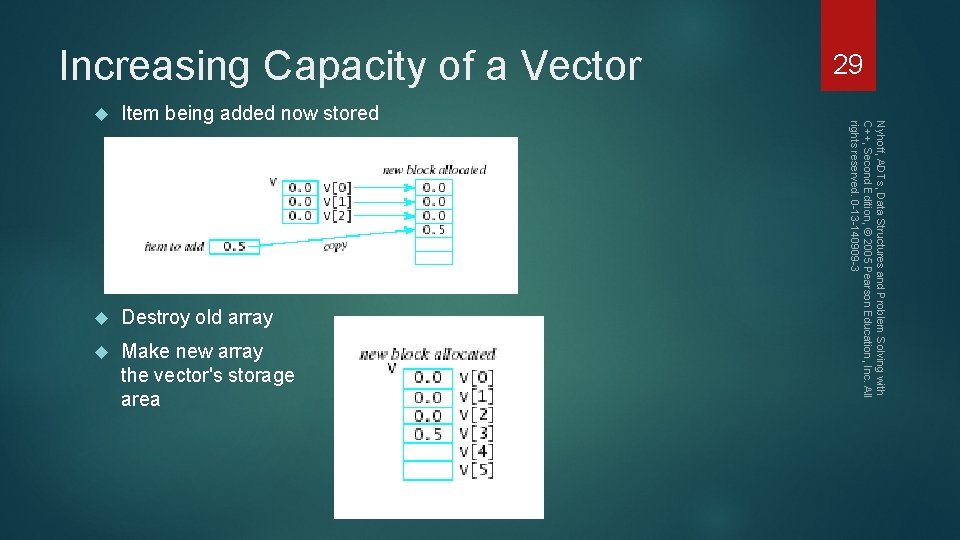
Item being added now stored Destroy old array Make new array the vector's storage area Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 29 Increasing Capacity of a Vector
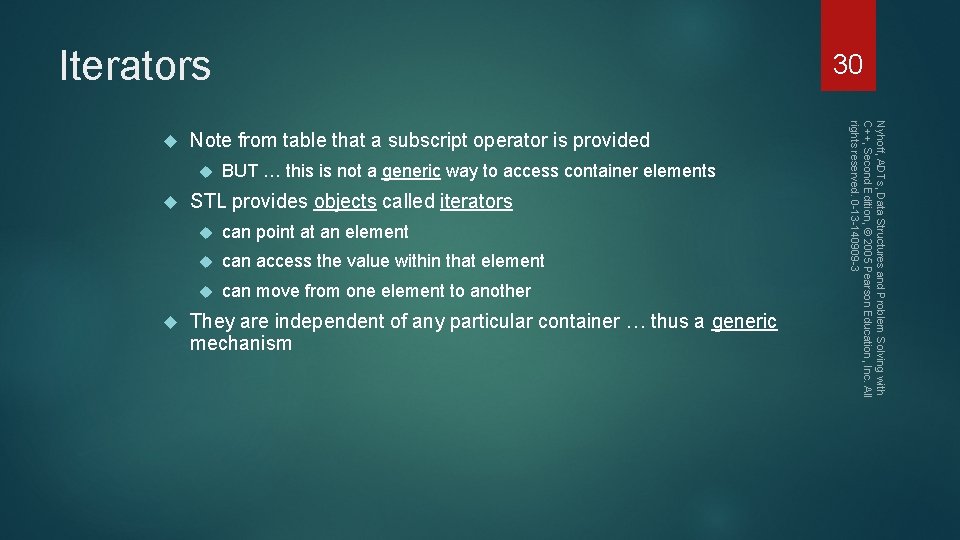
Iterators Note from table that a subscript operator is provided BUT … this is not a generic way to access container elements STL provides objects called iterators can point at an element can access the value within that element can move from one element to another They are independent of any particular container … thus a generic mechanism Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 30
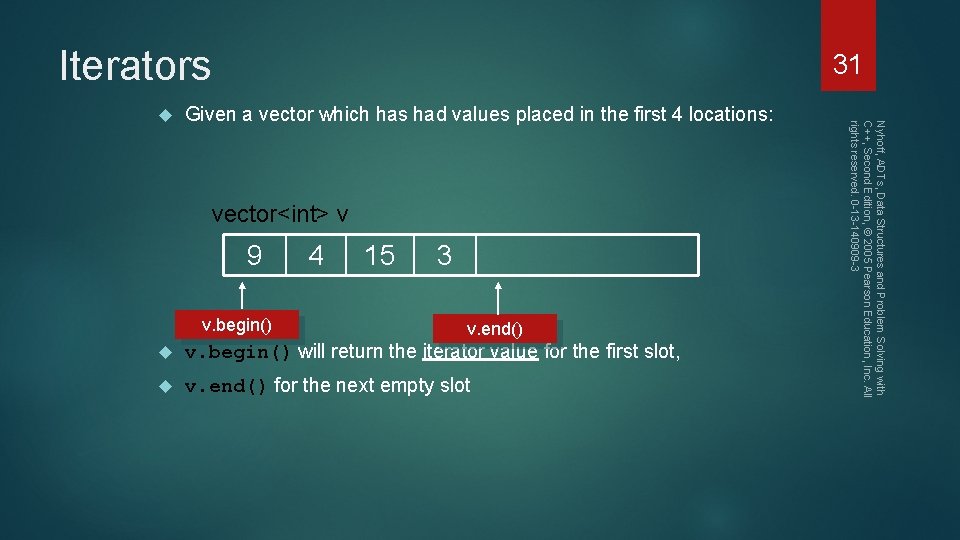
Iterators Given a vector which has had values placed in the first 4 locations: vector<int> v 9 v. begin() 4 15 3 v. end() v. begin() will return the iterator value for the first slot, v. end() for the next empty slot Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 31
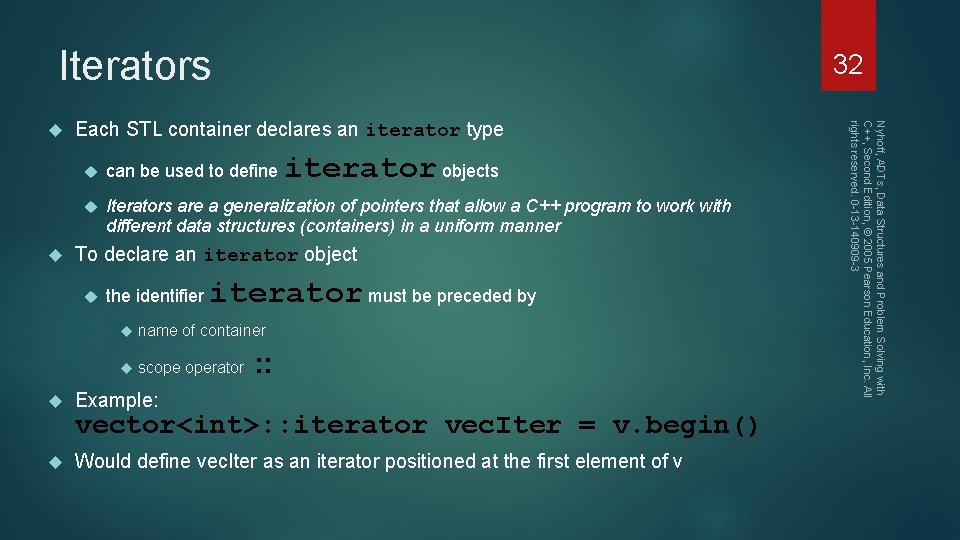
Iterators Each STL container declares an iterator type iterator objects can be used to define Iterators are a generalization of pointers that allow a C++ program to work with different data structures (containers) in a uniform manner To declare an iterator object the identifier iterator must be preceded by name of container scope operator : : Example: Would define vec. Iter as an iterator positioned at the first element of v vector<int>: : iterator vec. Iter = v. begin() Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 32
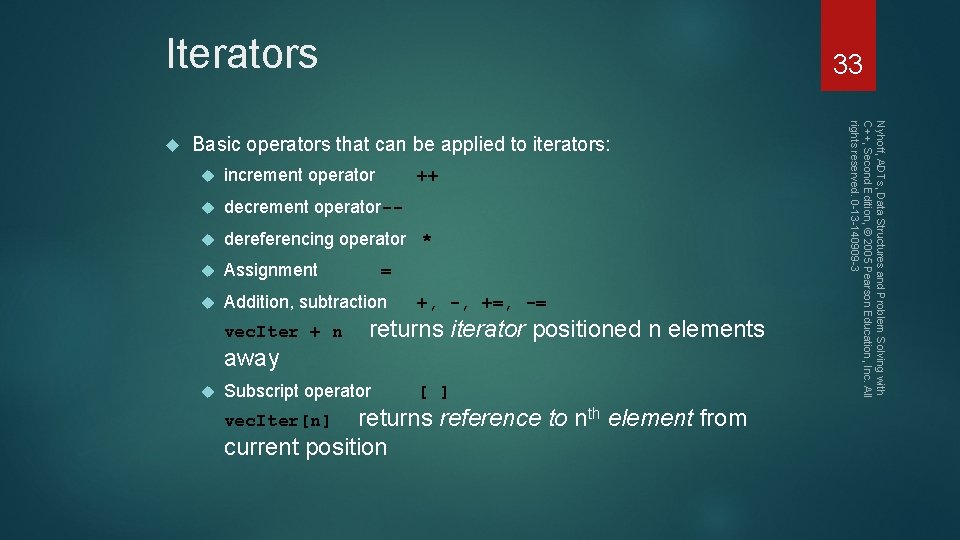
Iterators Basic operators that can be applied to iterators: increment operator decrement operator-- dereferencing operator * Assignment Addition, subtraction vec. Iter + n ++ = +, -, +=, -= returns iterator positioned n elements away Subscript operator [ ] returns reference to nth element from current position vec. Iter[n] Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 33
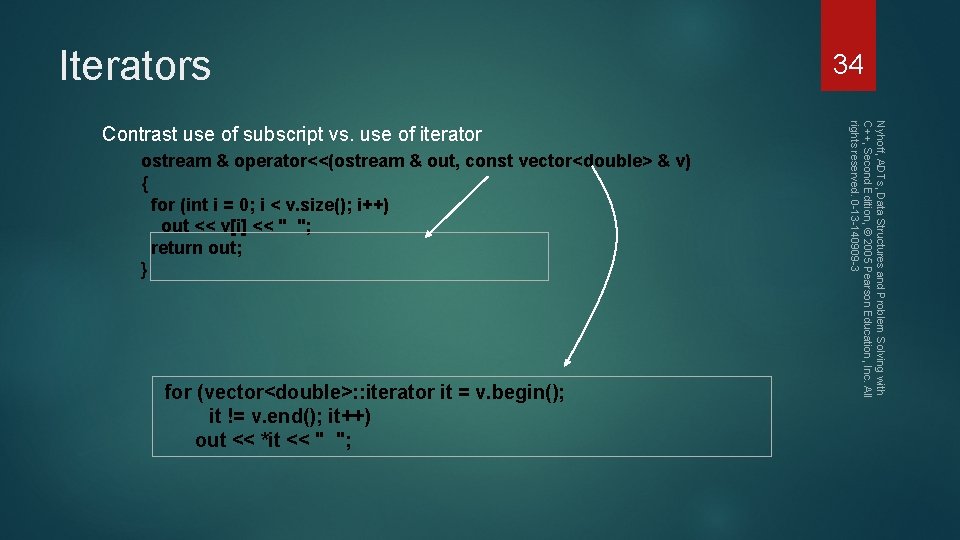
Iterators ostream & operator<<(ostream & out, const vector<double> & v) { for (int i = 0; i < v. size(); i++) out << v[i] << " "; return out; } for (vector<double>: : iterator it = v. begin(); it != v. end(); it++) out << *it << " "; Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Contrast use of subscript vs. use of iterator 34
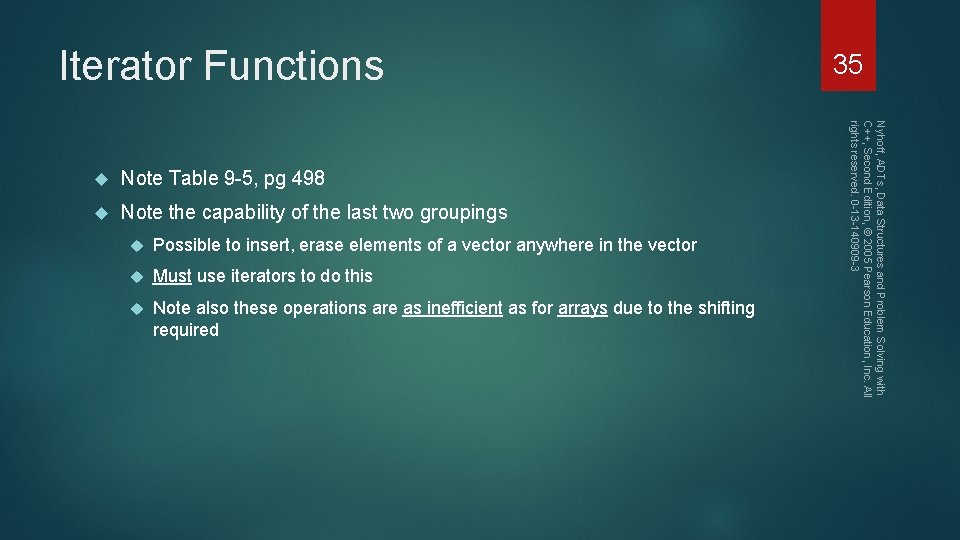
Iterator Functions Note Table 9 -5, pg 498 Note the capability of the last two groupings Possible to insert, erase elements of a vector anywhere in the vector Must use iterators to do this Note also these operations are as inefficient as for arrays due to the shifting required Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 35
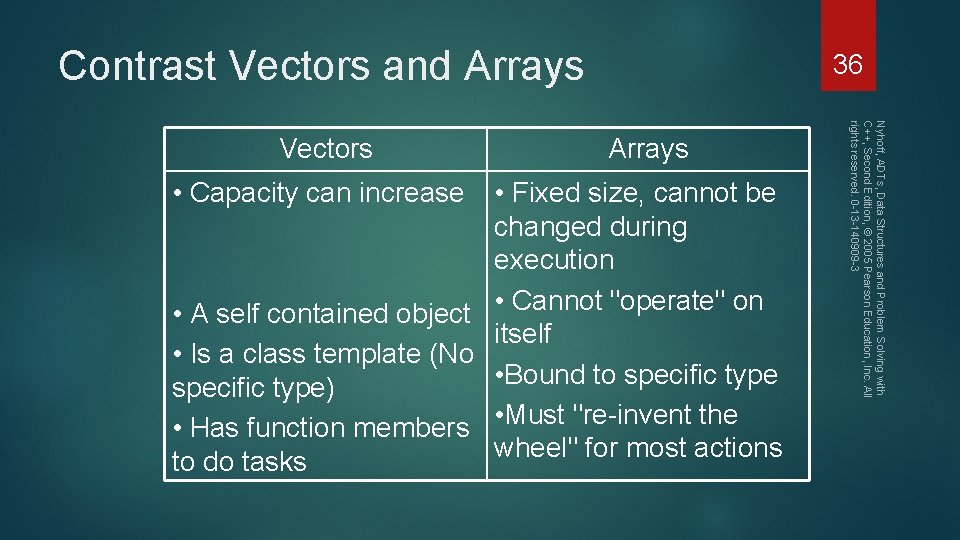
Contrast Vectors and Arrays • Capacity can increase Arrays • Fixed size, cannot be changed during execution • A self contained object • Cannot "operate" on itself • Is a class template (No • Bound to specific type) • Must "re-invent the • Has function members wheel" for most actions to do tasks Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Vectors 36
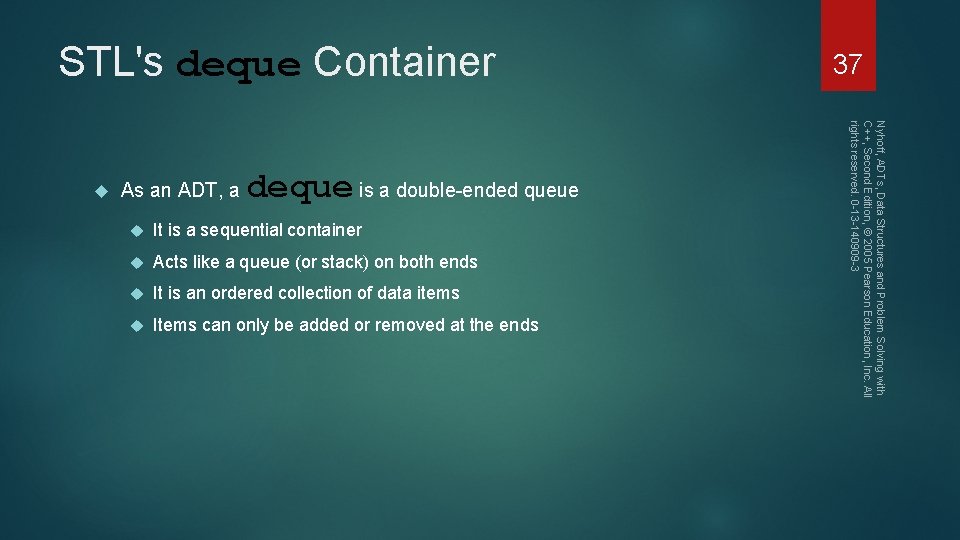
STL's deque Container As an ADT, a deque is a double-ended queue It is a sequential container Acts like a queue (or stack) on both ends It is an ordered collection of data items Items can only be added or removed at the ends Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 37
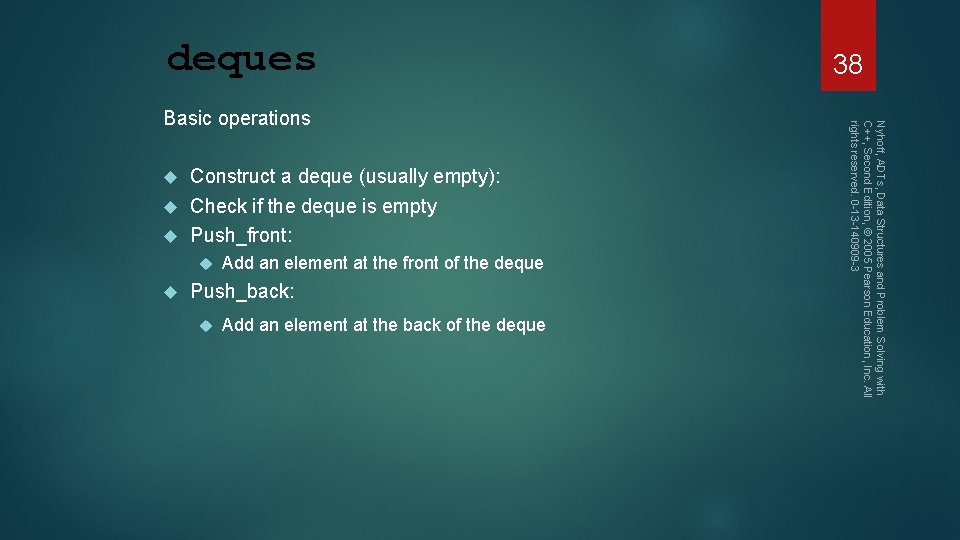
deques Construct a deque (usually empty): Check if the deque is empty Push_front: Add an element at the front of the deque Push_back: Add an element at the back of the deque Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Basic operations 38
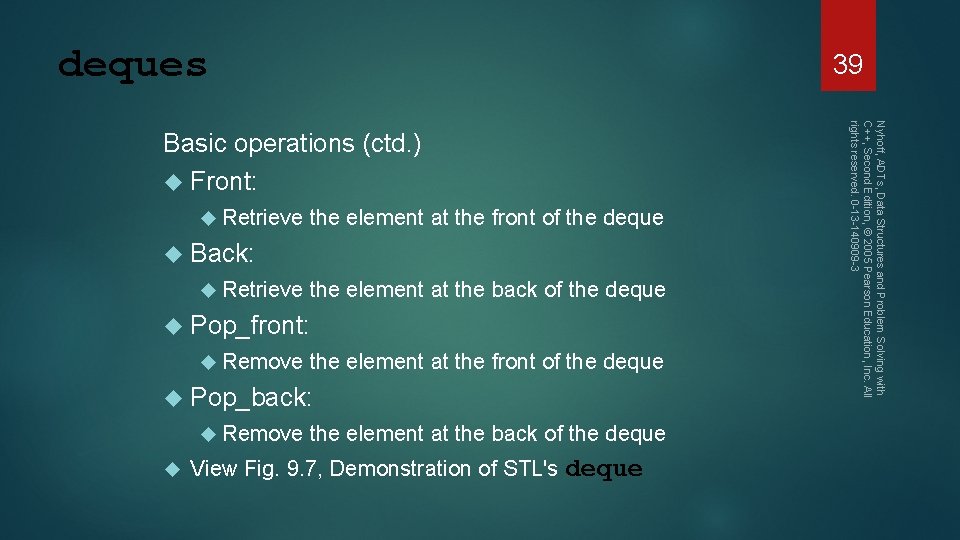
deques 39 Retrieve the element at the front of the deque Back: Retrieve the element at the back of the deque Pop_front: Remove the element at the front of the deque Pop_back: Remove the element at the back of the deque View Fig. 9. 7, Demonstration of STL's deque Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Basic operations (ctd. ) Front:
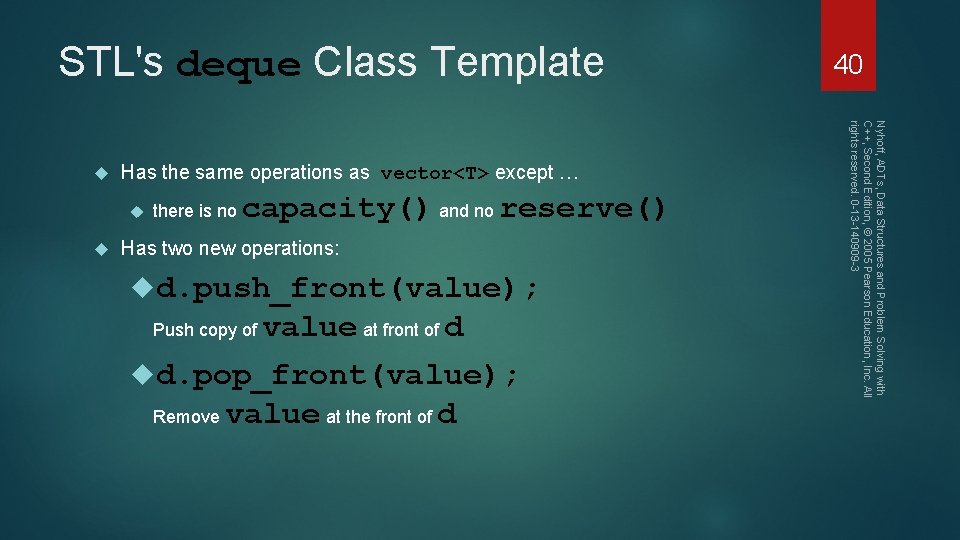
STL's deque Class Template Has the same operations as vector<T> except … there is no capacity() and no reserve() Has two new operations: d. push_front(value); Push copy of value at front of d d. pop_front(value); Remove value at the front of d Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 40
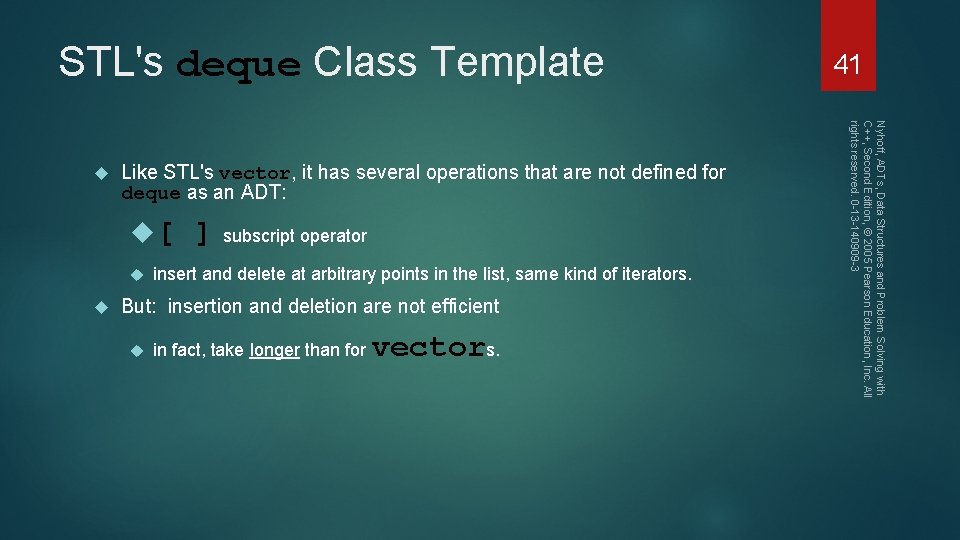
STL's deque Class Template Like STL's vector, it has several operations that are not defined for deque as an ADT: [ ] subscript operator insert and delete at arbitrary points in the list, same kind of iterators. But: insertion and deletion are not efficient in fact, take longer than for vectors. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 41
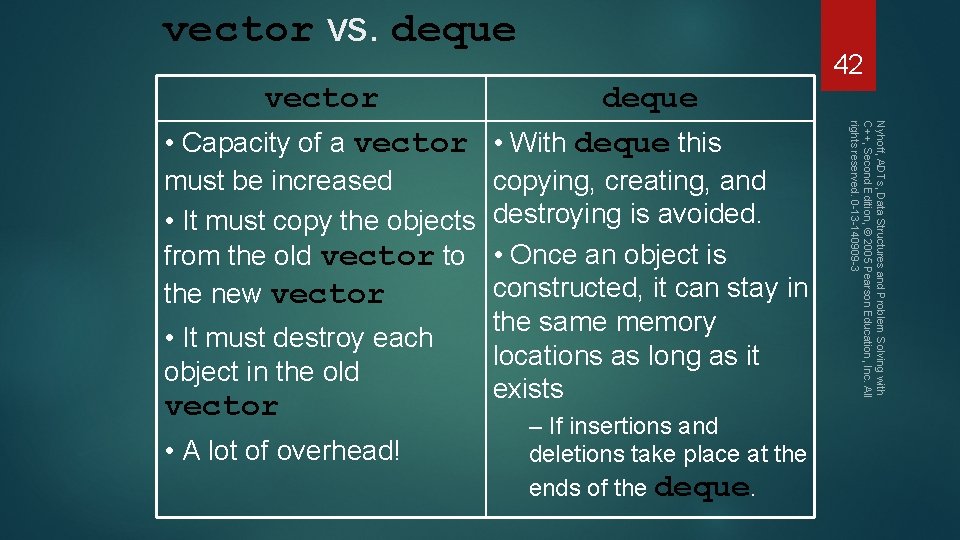
vector vs. deque 42 must be increased • It must copy the objects from the old vector to the new vector • It must destroy each object in the old vector • A lot of overhead! copying, creating, and destroying is avoided. • Once an object is constructed, it can stay in the same memory locations as long as it exists – If insertions and deletions take place at the ends of the deque. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 vector deque • Capacity of a vector • With deque this
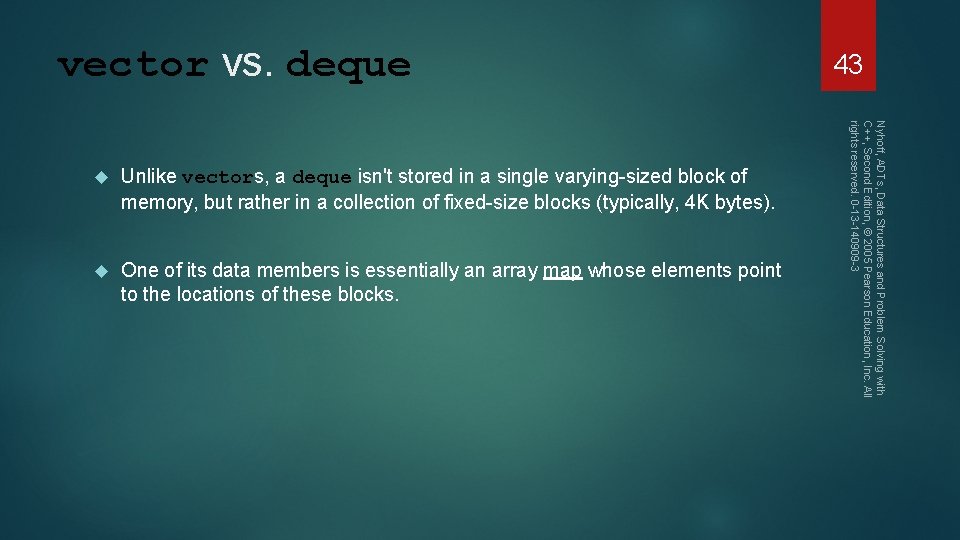
vector vs. deque Unlike vectors, a deque isn't stored in a single varying-sized block of memory, but rather in a collection of fixed-size blocks (typically, 4 K bytes). One of its data members is essentially an array map whose elements point to the locations of these blocks. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 43
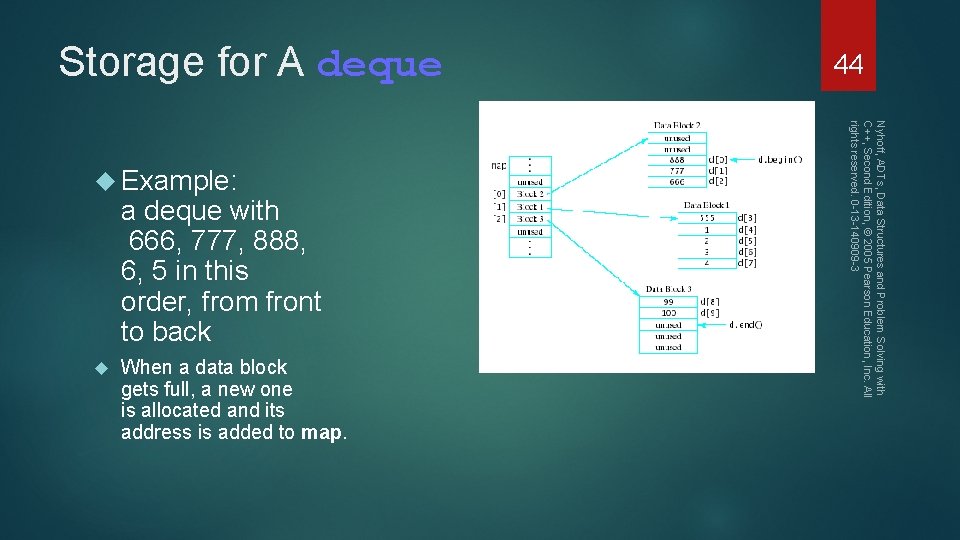
Storage for A deque a deque with 666, 777, 888, 6, 5 in this order, from front to back When a data block gets full, a new one is allocated and its address is added to map. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Example: 44
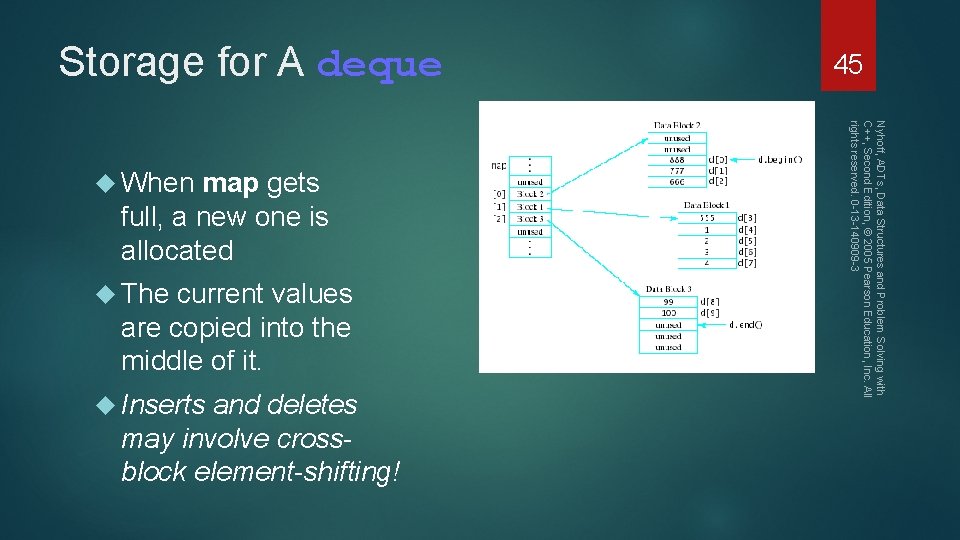
Storage for A deque map gets full, a new one is allocated The current values are copied into the middle of it. Inserts and deletes may involve crossblock element-shifting! Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 When 45
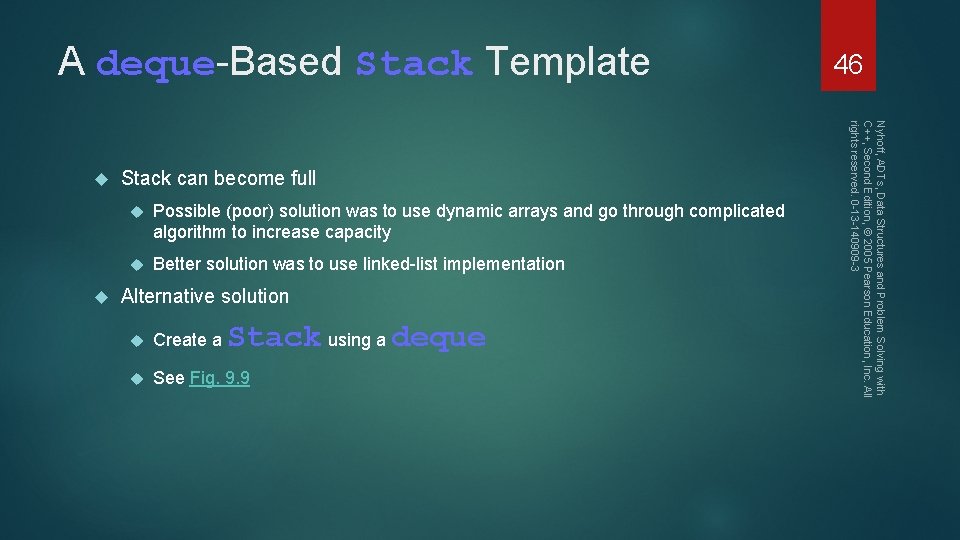
A deque-Based Stack Template Stack can become full Possible (poor) solution was to use dynamic arrays and go through complicated algorithm to increase capacity Better solution was to use linked-list implementation Alternative solution Stack using a deque Create a See Fig. 9. 9 Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 46
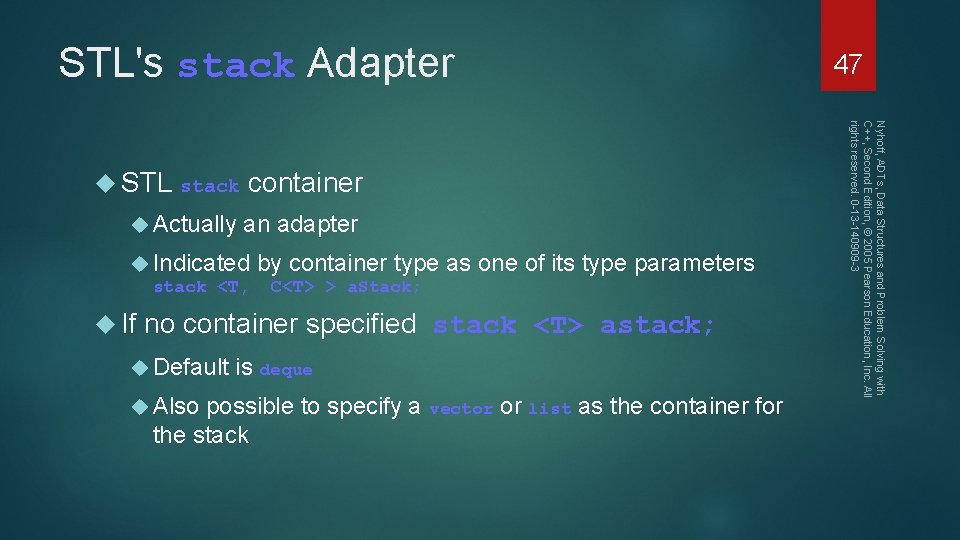
STL's stack Adapter container Actually an adapter Indicated stack <T, If by container type as one of its type parameters C<T> > a. Stack; no container specified stack <T> astack; Default Also is deque possible to specify a vector or list as the container for the stack Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 STL stack 47
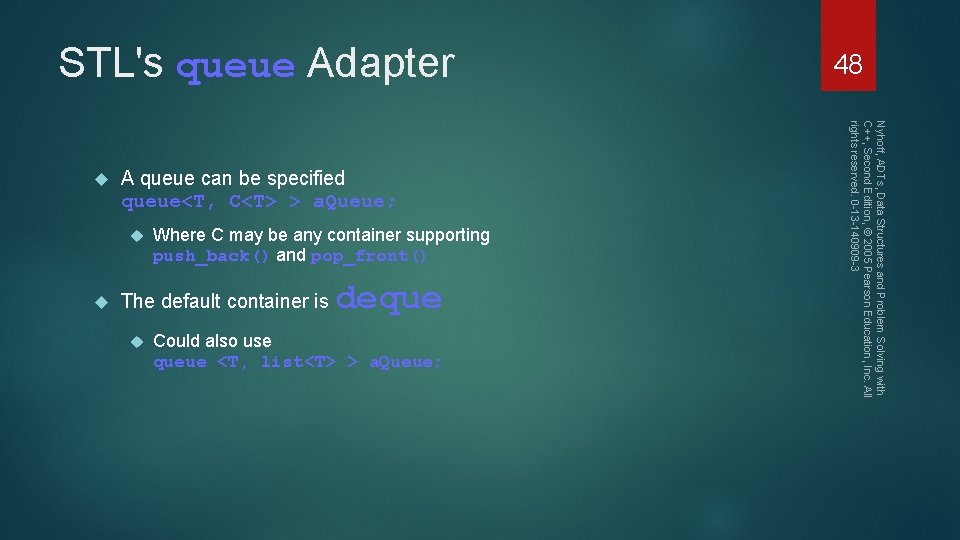
STL's queue Adapter A queue can be specified queue<T, C<T> > a. Queue; Where C may be any container supporting push_back() and pop_front() The default container is deque Could also use queue <T, list<T> > a. Queue; Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 48
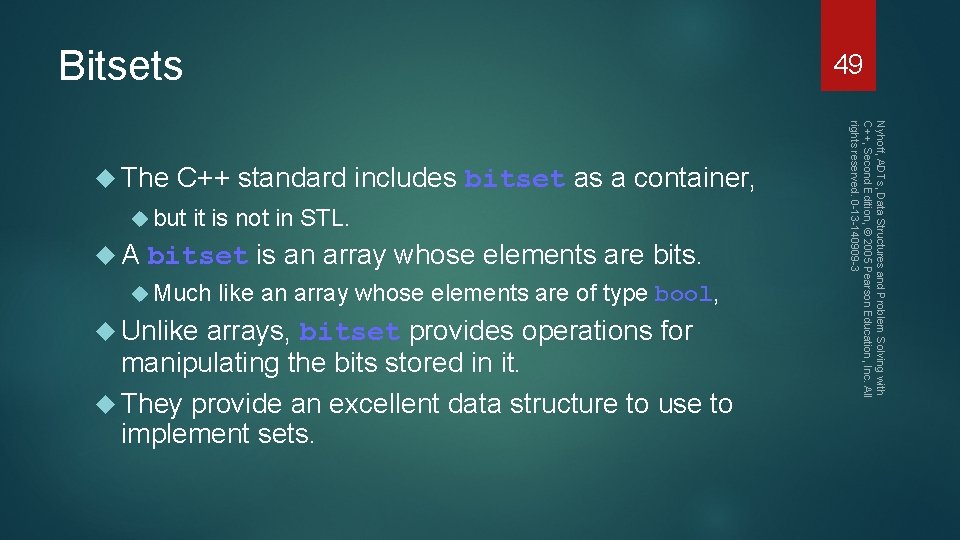
Bitsets C++ standard includes bitset as a container, but A it is not in STL. bitset is an array whose elements are bits. Much Unlike an array whose elements are of type bool, arrays, bitset provides operations for manipulating the bits stored in it. They provide an excellent data structure to use to implement sets. Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 The 49
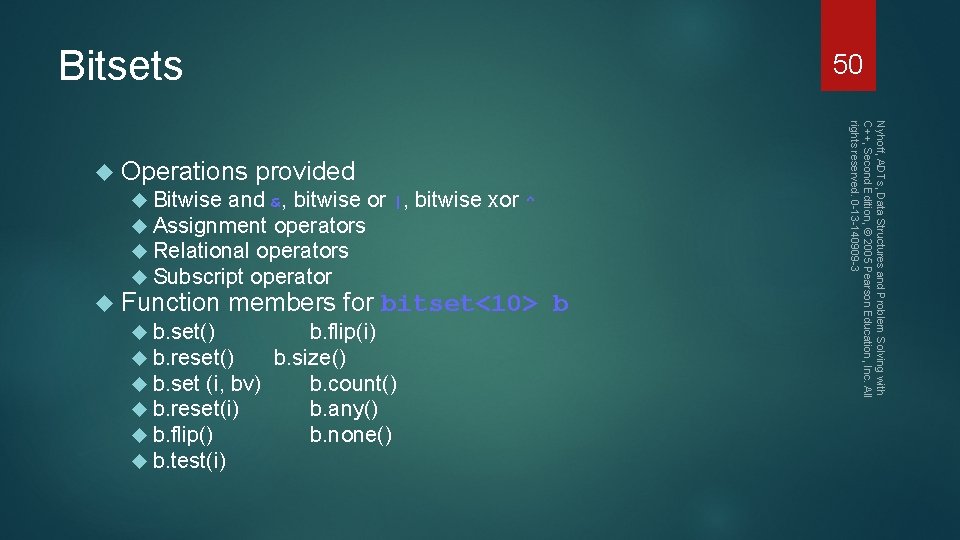
Bitsets b Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Operations provided Bitwise and &, bitwise or |, bitwise xor ^ Assignment operators Relational operators Subscript operator Function members for bitset<10> b. set() b. flip(i) b. reset() b. size() b. set (i, bv) b. count() b. reset(i) b. any() b. flip() b. none() b. test(i) 50
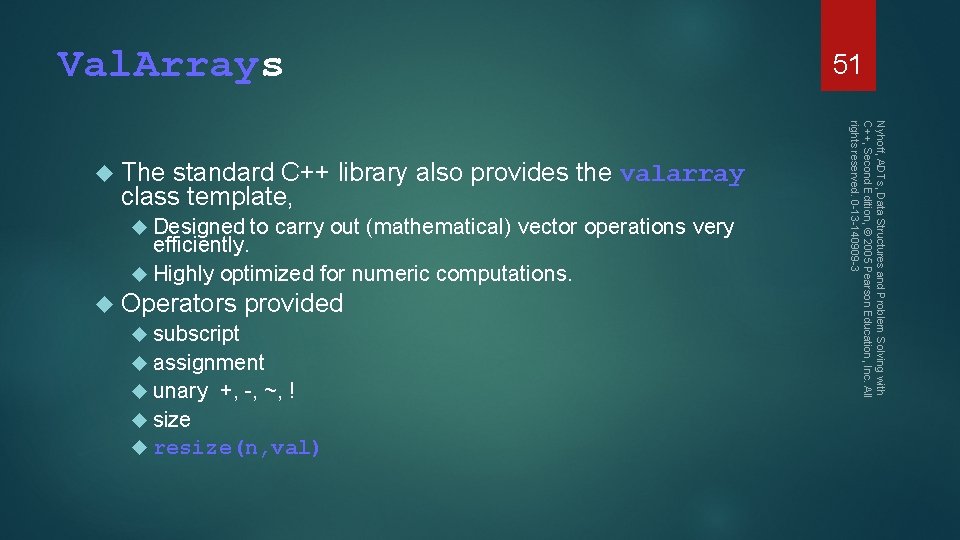
Val. Arrays standard C++ library also provides the valarray class template, Designed to carry out (mathematical) vector operations very efficiently. Highly optimized for numeric computations. Operators provided subscript assignment unary +, -, ~, ! size resize(n, val) Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 The 51
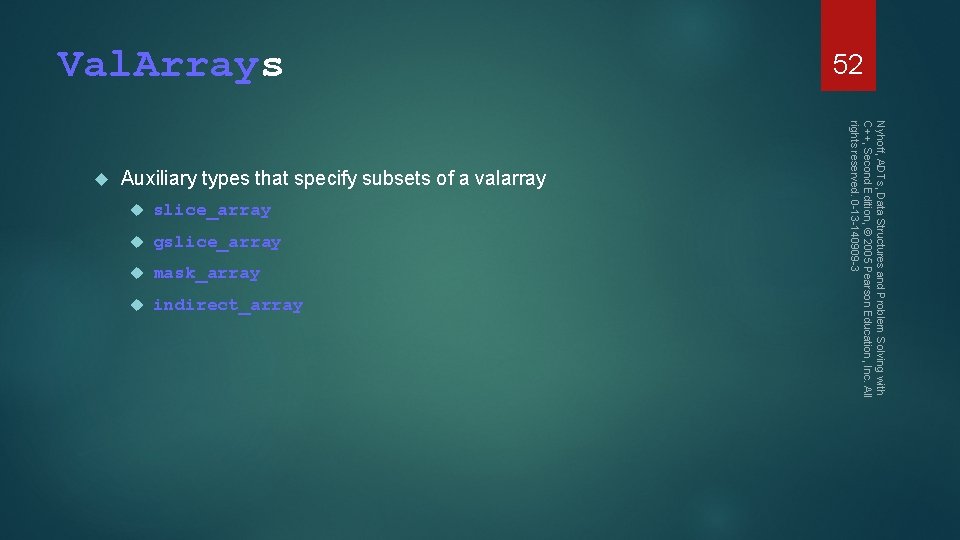
slice_array gslice_array mask_array indirect_array Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3 Auxiliary types that specify subsets of a valarray 52 Val. Arrays
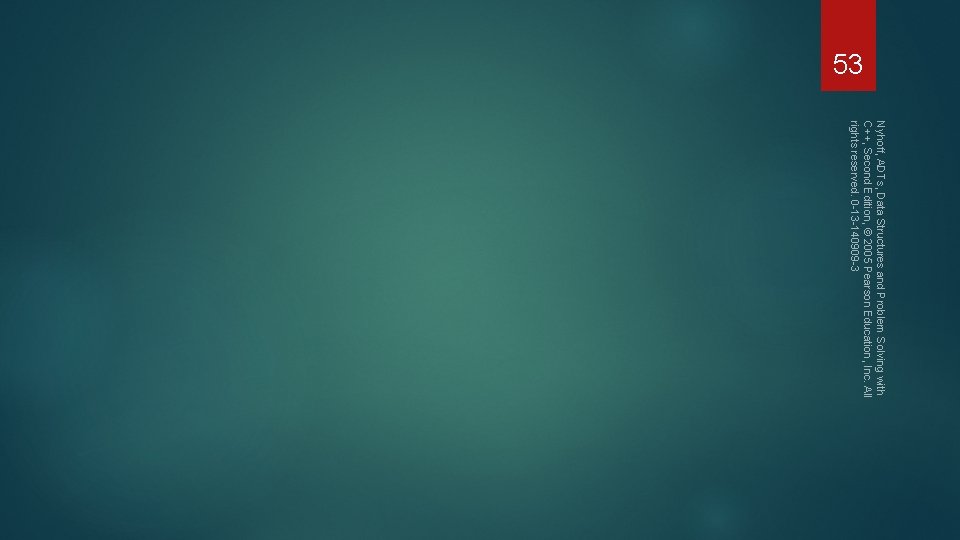
53 Nyhoff, ADTs, Data Structures and Problem Solving with C++, Second Edition, © 2005 Pearson Education, Inc. All rights reserved. 0 -13 -140909 -3
Adts, data structures, and problem solving with c++
Parameterized adts is also known as
Parameterized adts is also known as
Adts ukiah
Engineering mechanics chapter 2
Chapter 3 vectors worksheets
Chapter 12 vectors and the geometry of space
Dot product
What is the name of the quantity represented as i^?
Xss vectors
Example of vectors
Adding multiple vectors
A bear searching for food wanders 35 meters east
Vectors in 2 dimensions
Vectors in one dimension
Vector in component form
Find a unit vector in the direction of the given vector.
Decomposing vectors
Vectors and scalars in physics
Monia
A storm system moves 5000 km due east
Difference between vectors and scalars
The vector has both magnitude and
Cartesian equation
The diagram shows two vectors that point west and north.
Equivalent vectors
What is interdependence according to seven vector model
2d motion equations
Different between scalar and vector quantity
Madas vectors
Madas vectors
Madas vectors
Entropy is scalar or vector
What is this?
What is meant by like and unlike forces
Vab=va-vb relative velocity
Ap physics vectors test
Graphical method of vector addition
Orthogonal set
Operacions amb vectors
Linearly independent
Norm van een vector
Linear algebra
Condition for linearly independent vectors
Intro to vectors
Ib math sl vectors
Vectors higher maths
8-7 vectors
Precalculus vectors worksheet
Geometric representation of vectors
Dr frost
Partial differentiation of vectors
Array concatenation matlab
Vector space example