1 Chapter 5 Control Structures Part 2 Outline
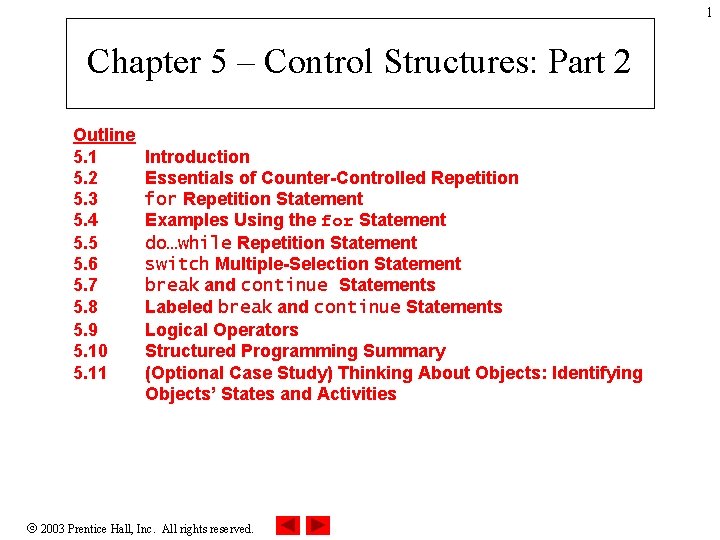
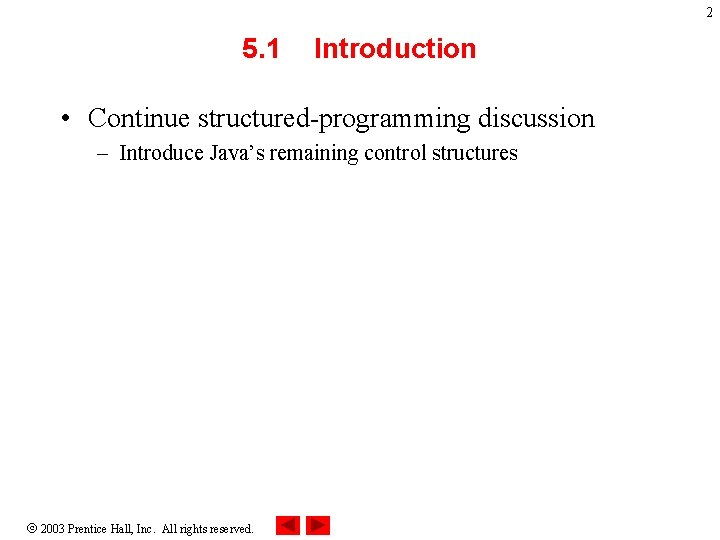
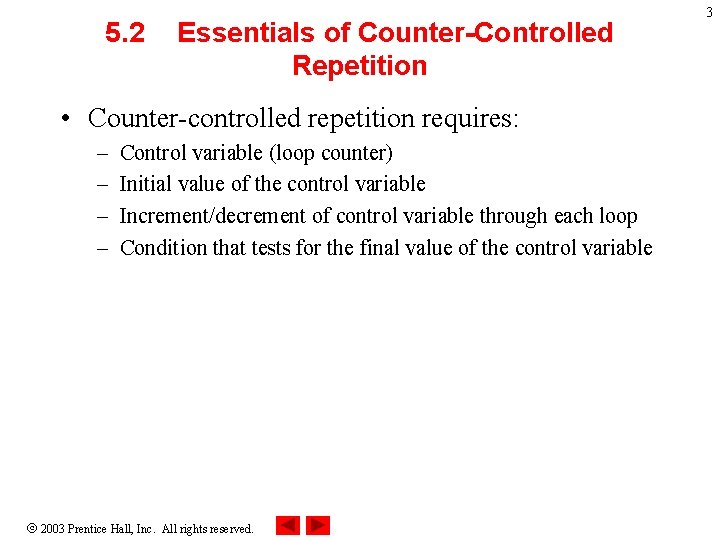
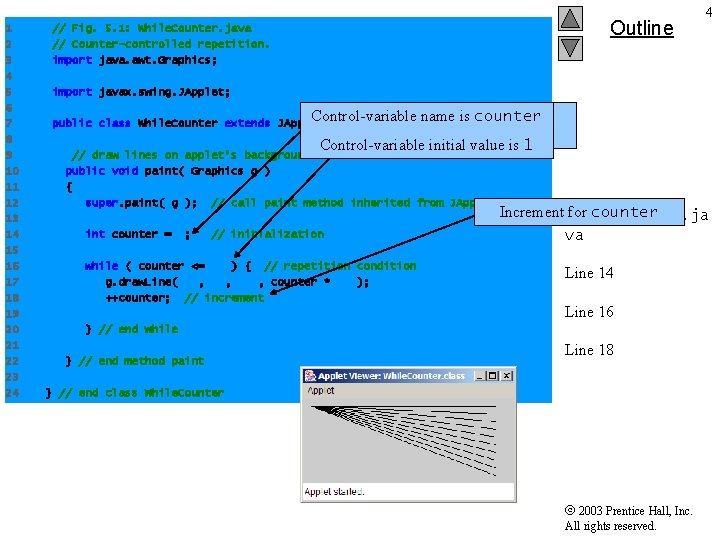
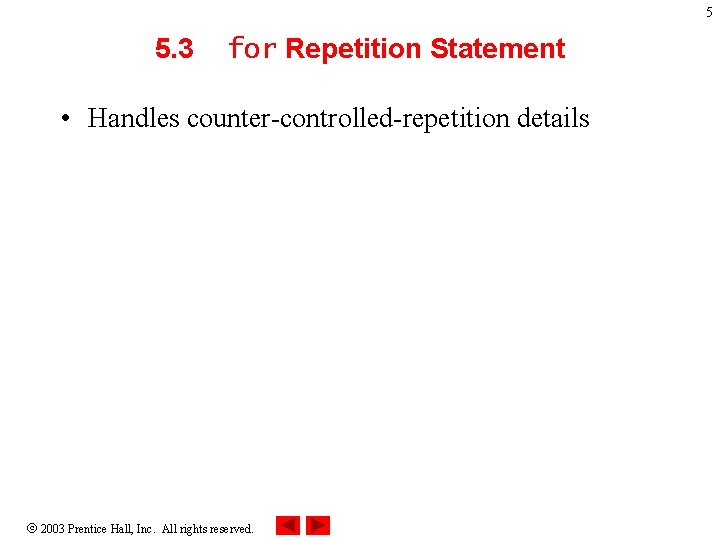
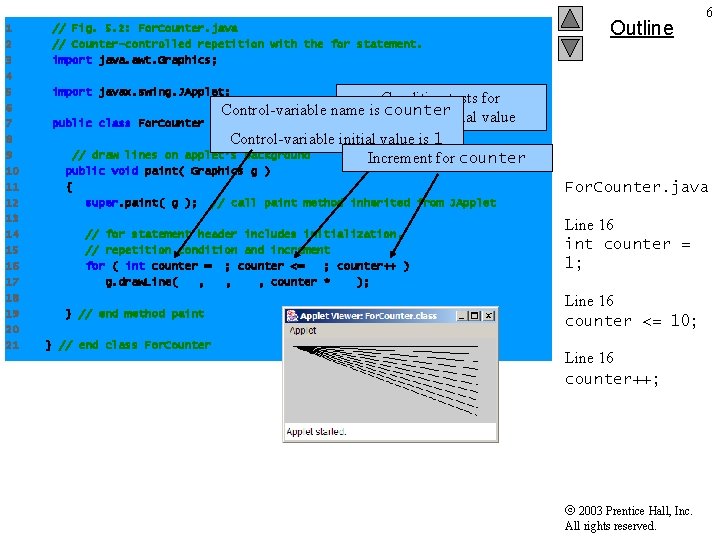
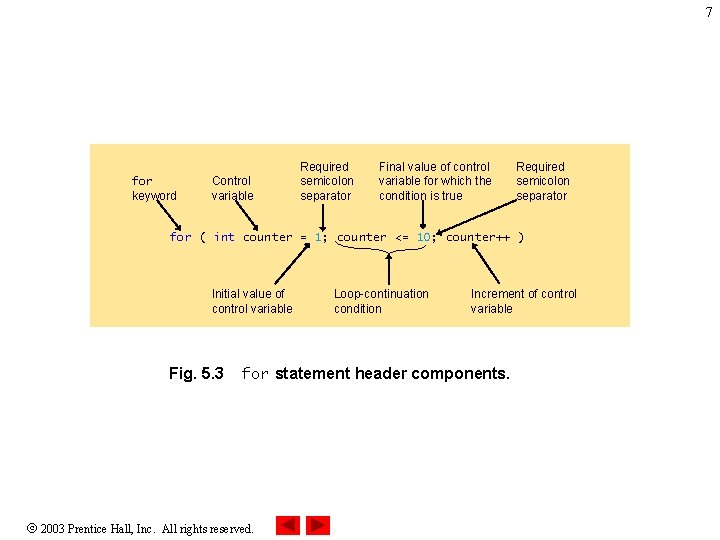
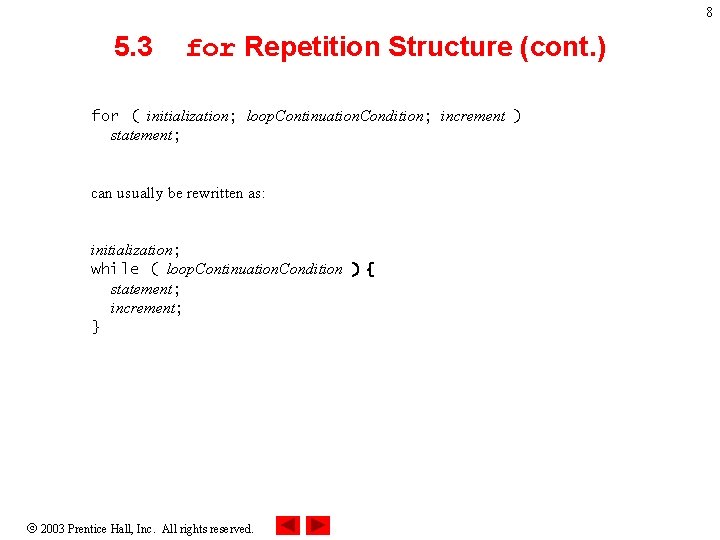
![9 Establish initial value of control variable int counter = 1 [counter <= 10] 9 Establish initial value of control variable int counter = 1 [counter <= 10]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-9.jpg)
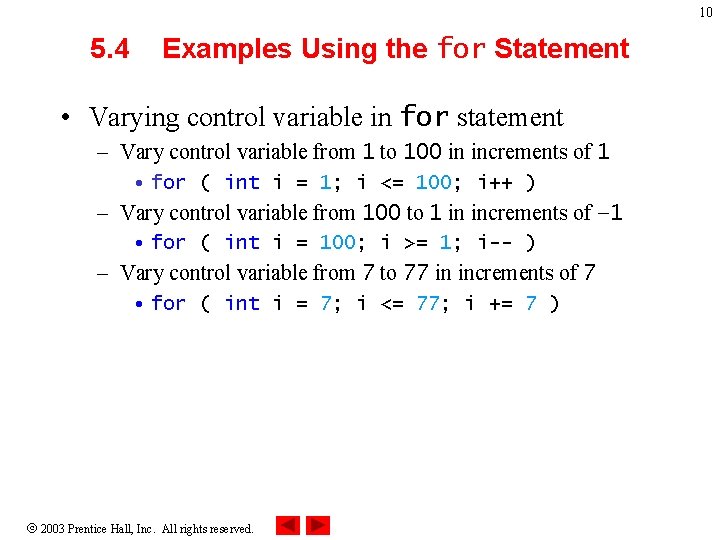
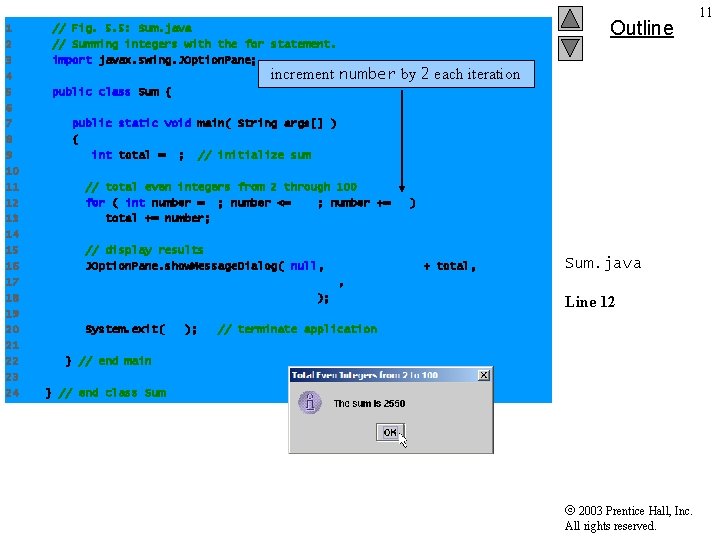
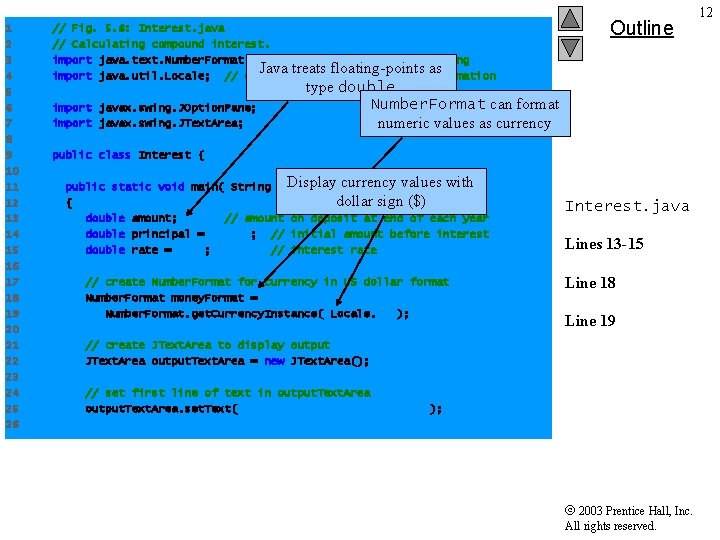
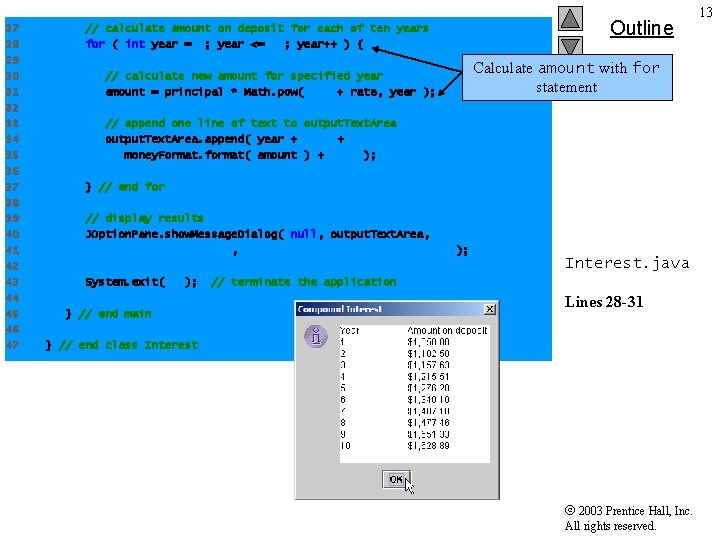
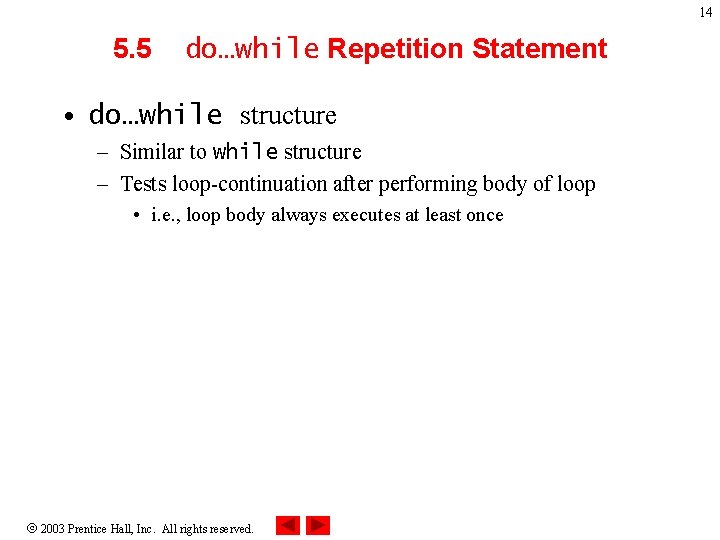
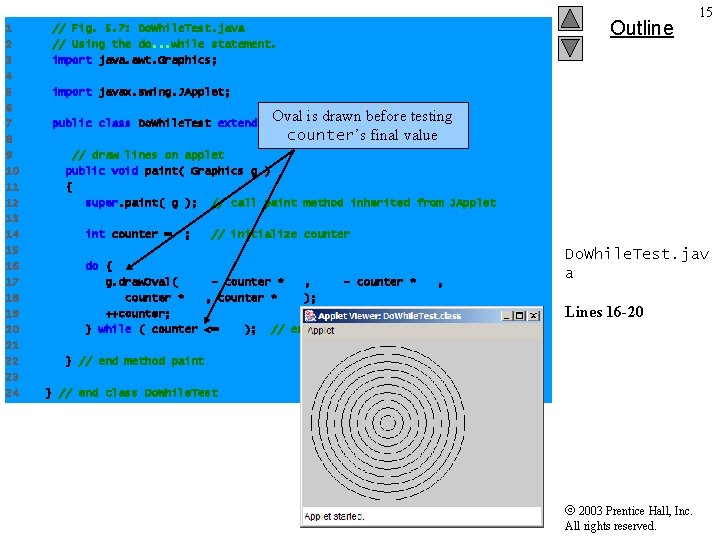
![16 action state [true] condition [false] Fig. 5. 8 do…while repetition statement activity diagram. 16 action state [true] condition [false] Fig. 5. 8 do…while repetition statement activity diagram.](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-16.jpg)
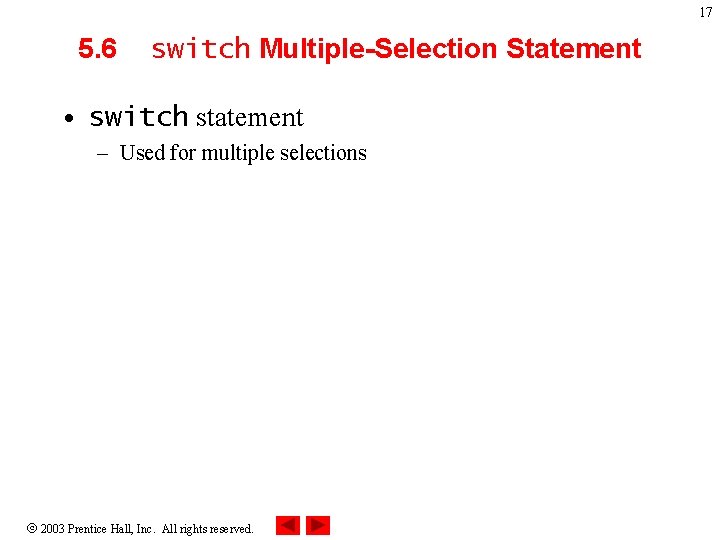
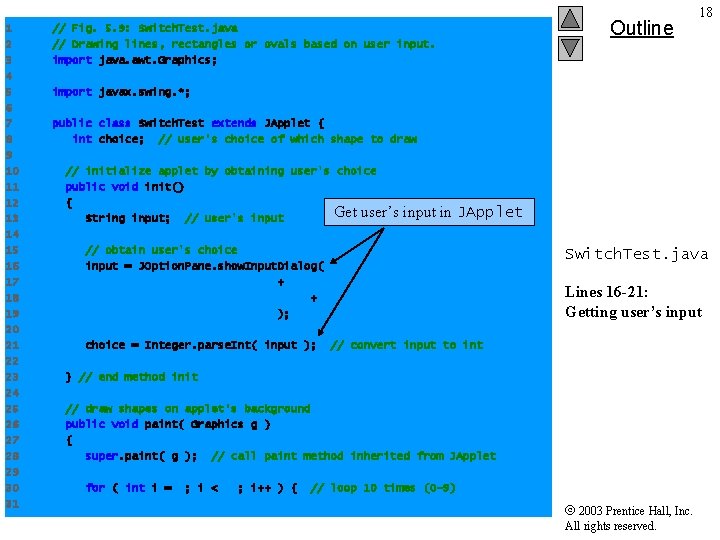
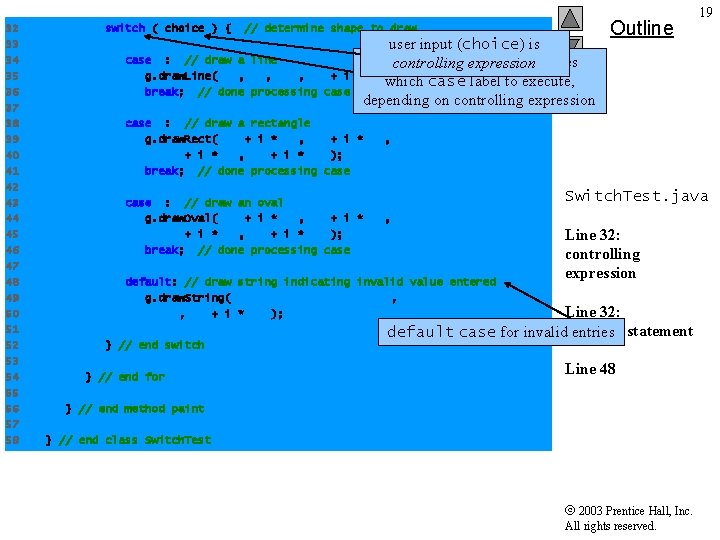
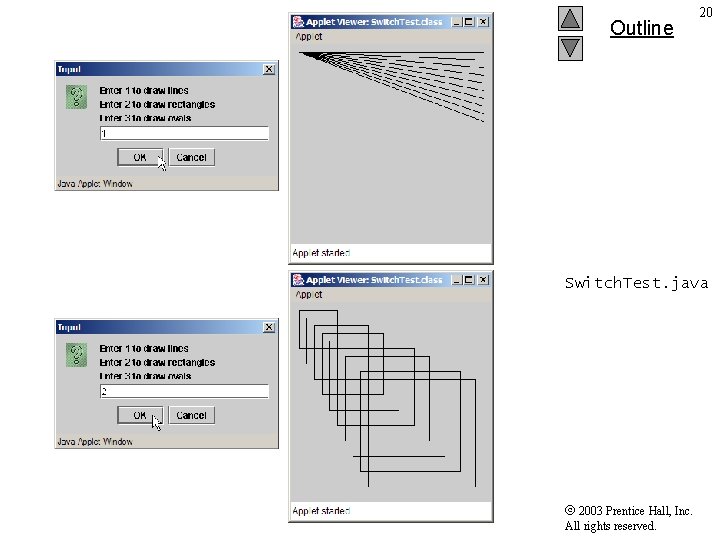
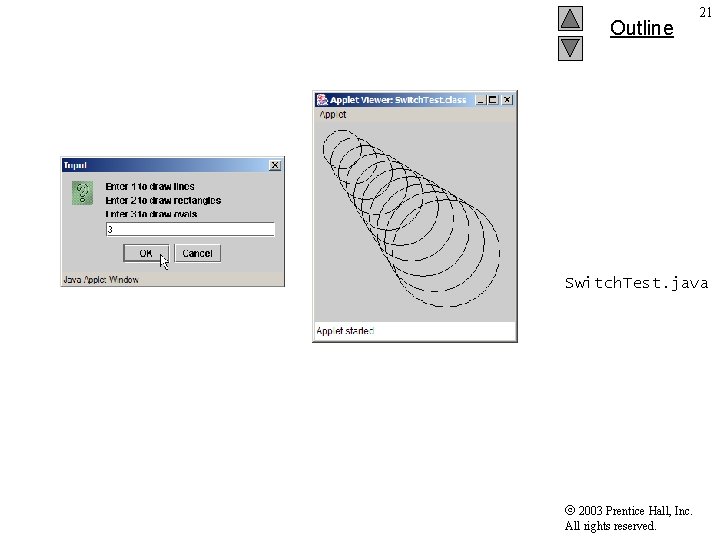
![22 [true] case a action(s) break case b action(s) break case z action(s) break 22 [true] case a action(s) break case b action(s) break case z action(s) break](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-22.jpg)
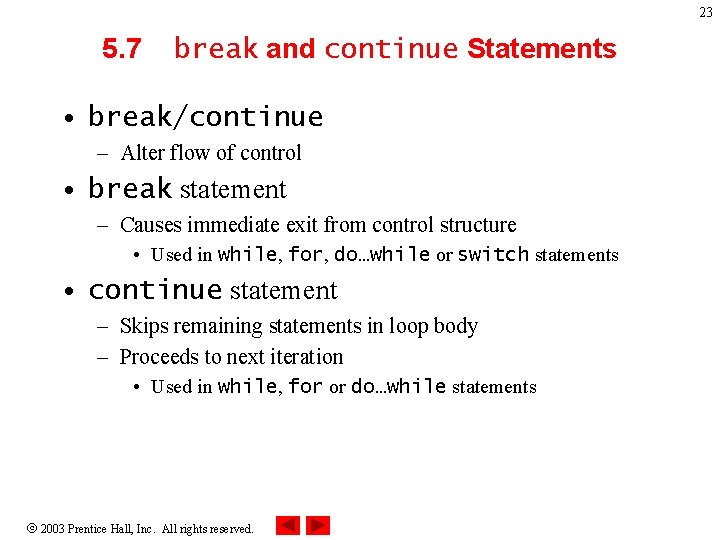
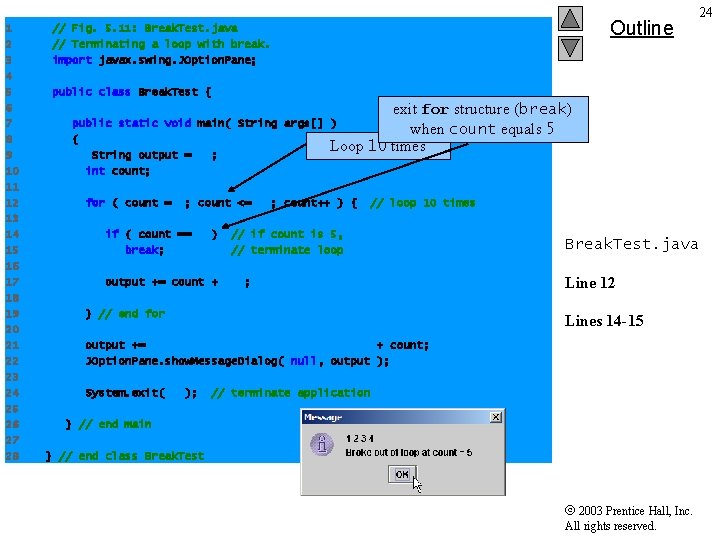
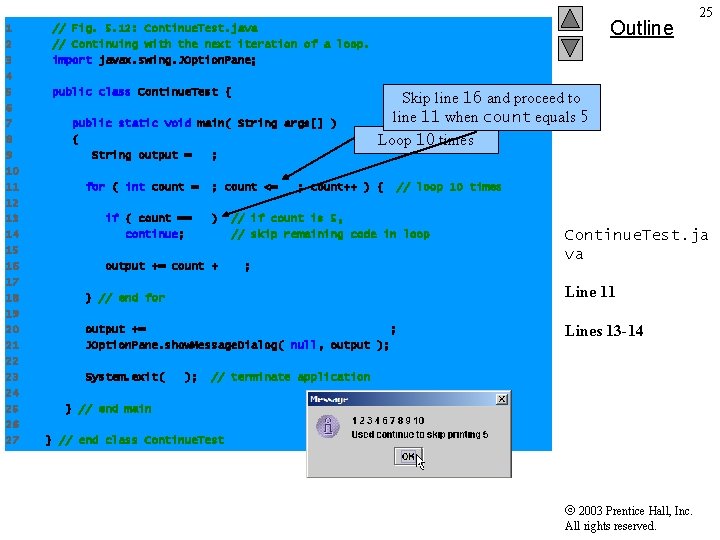
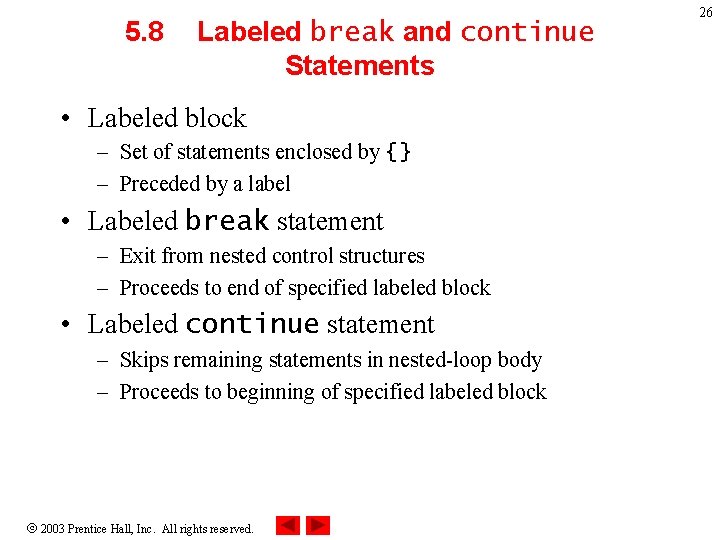
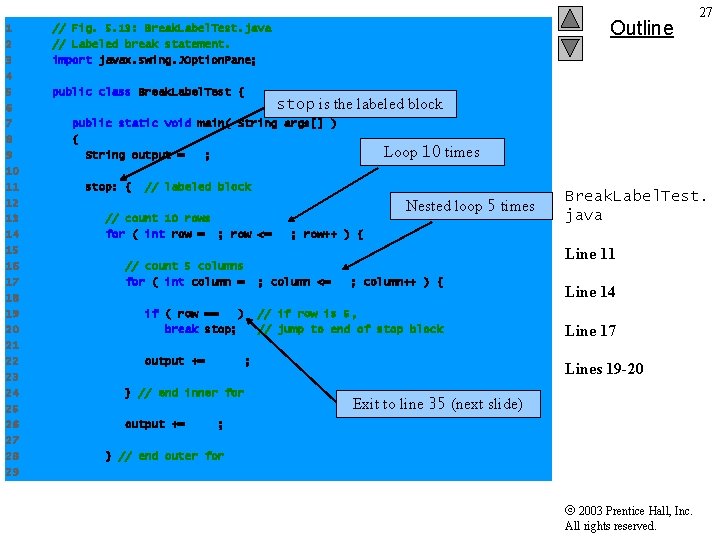
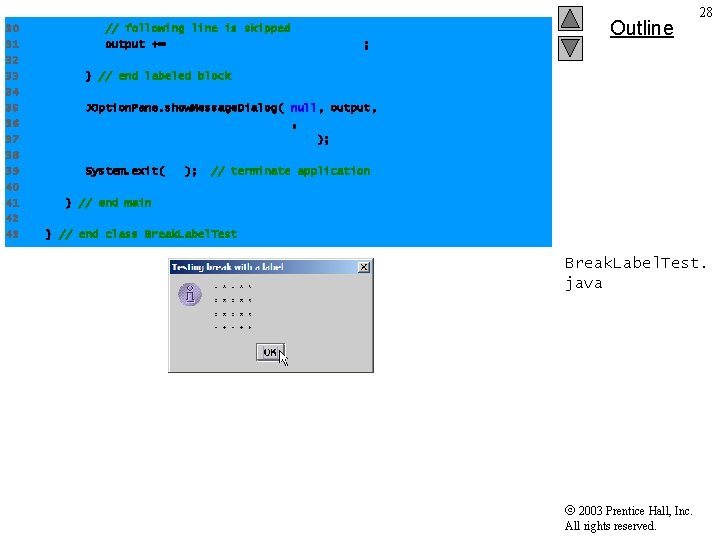
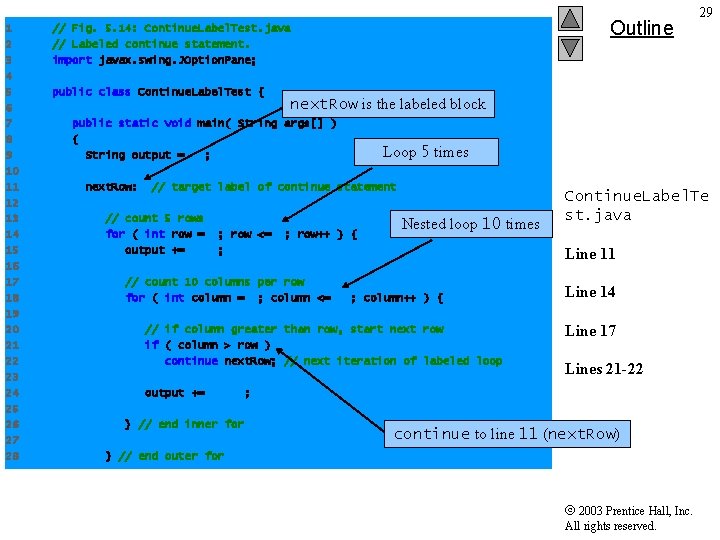
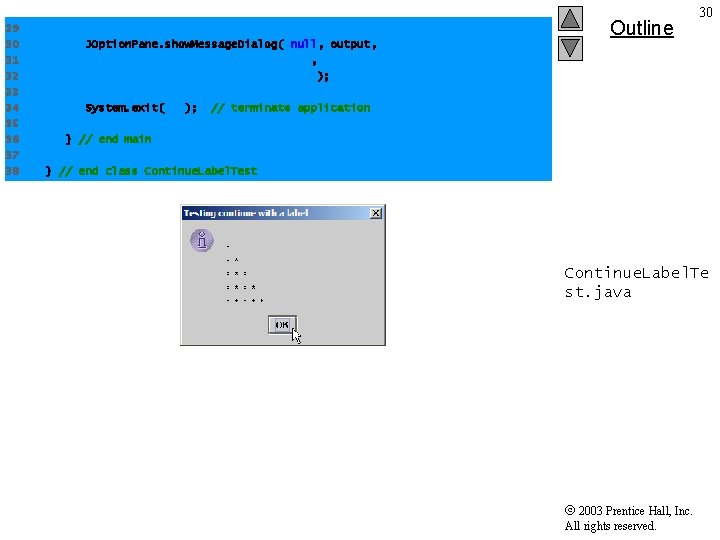
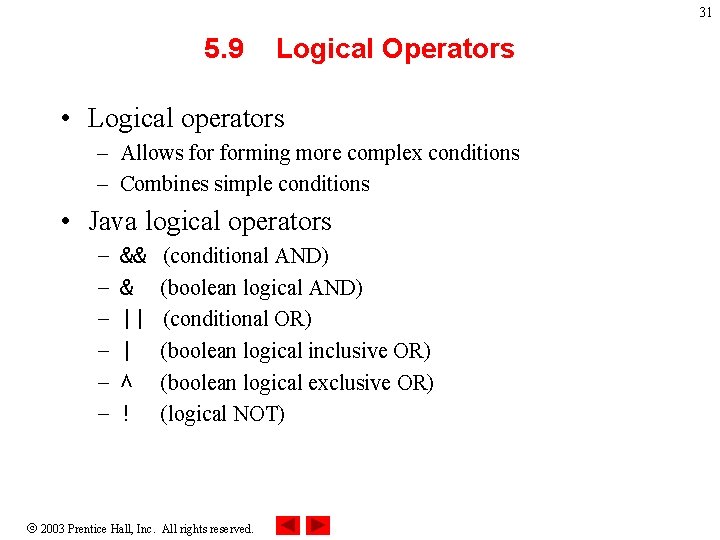
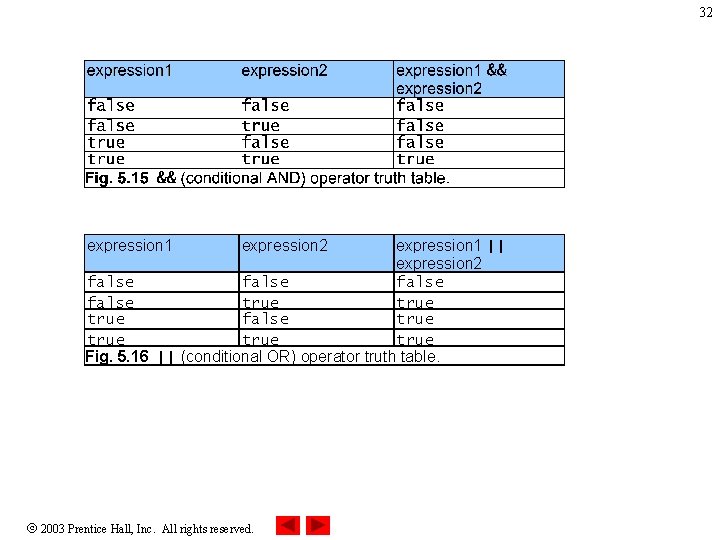
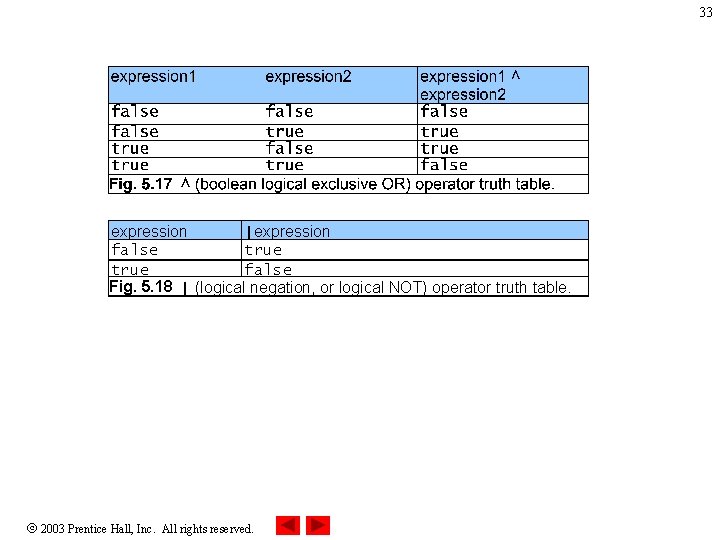
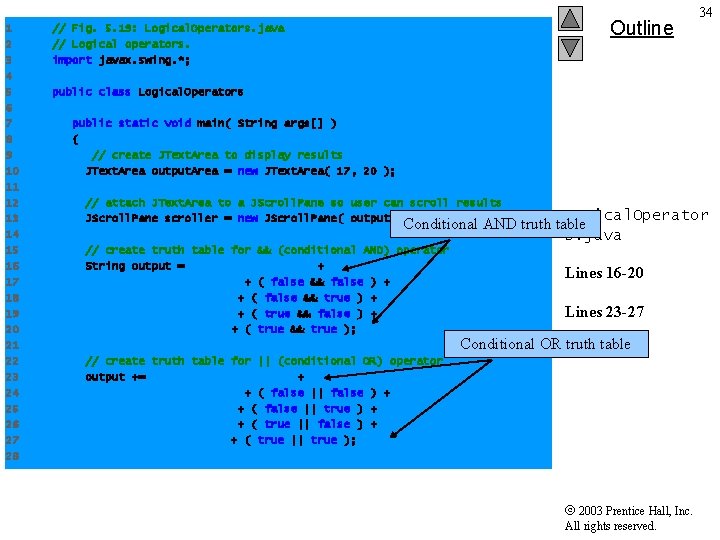
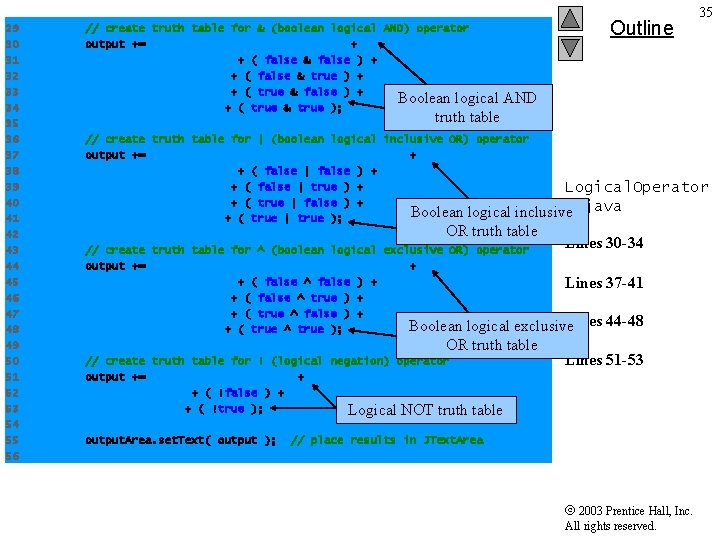
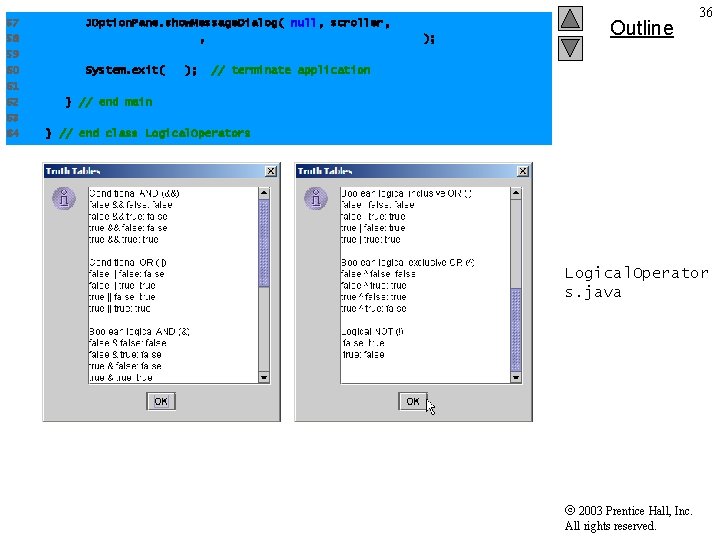
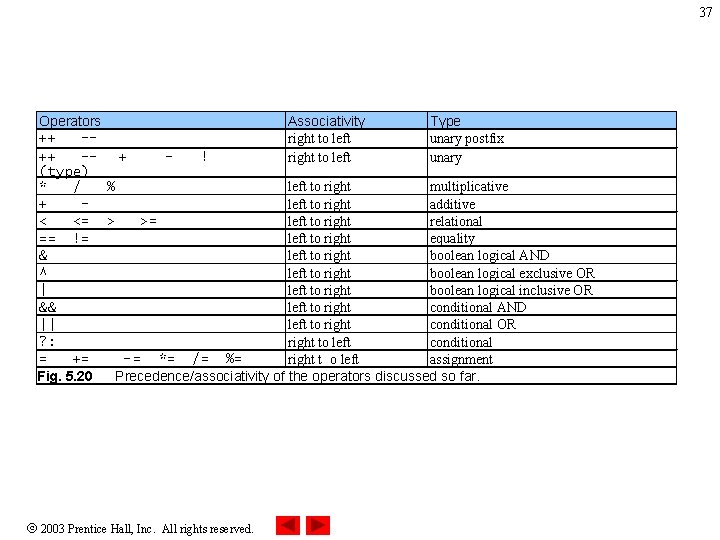
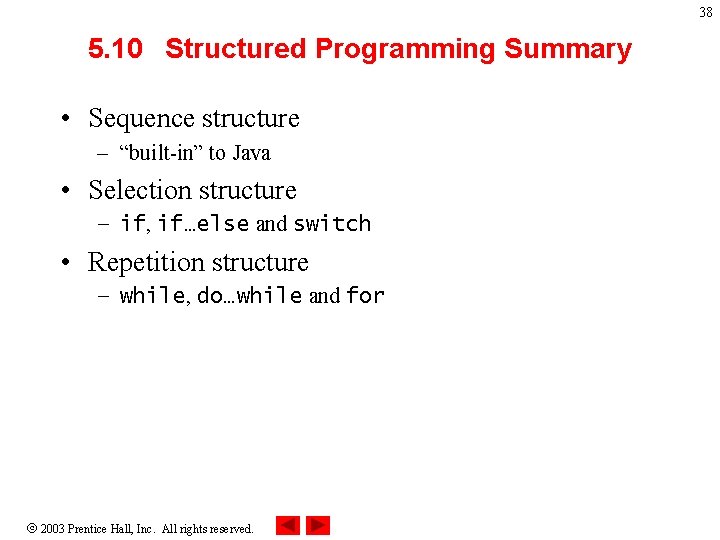
![39 Sequence Selection if statement (single selection) switch statement (multiple selection) [t] [f] [t] 39 Sequence Selection if statement (single selection) switch statement (multiple selection) [t] [f] [t]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-39.jpg)
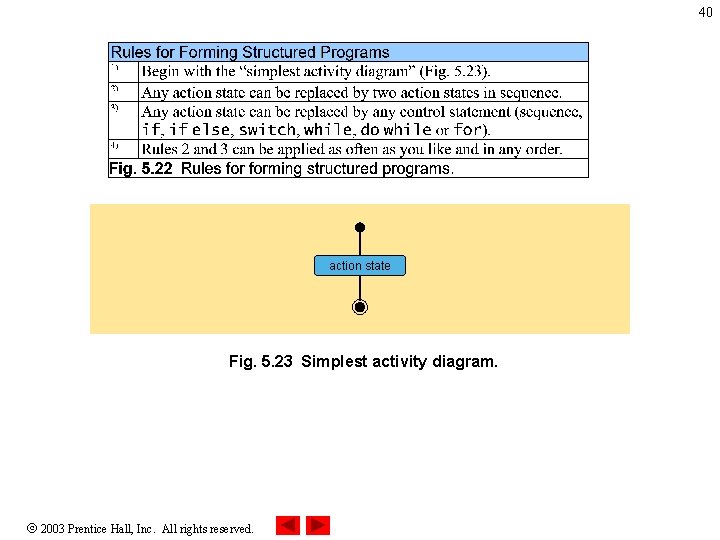
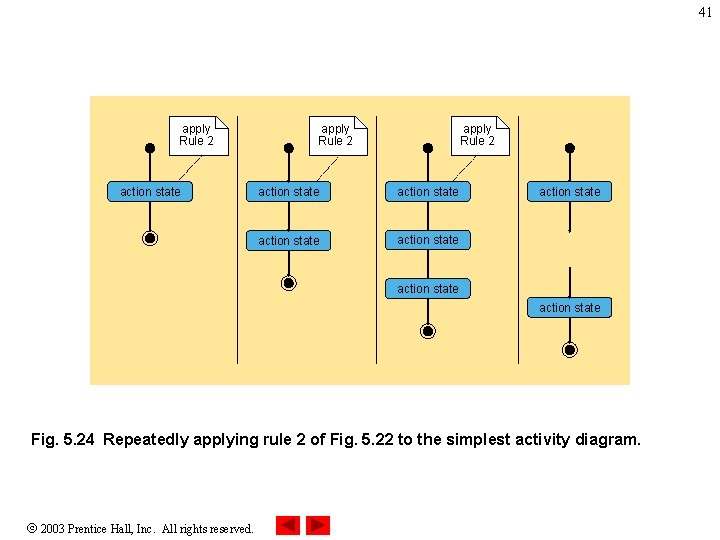
![42 apply Rule 3 action state [f] [f] action state [t] action state apply 42 apply Rule 3 action state [f] [f] action state [t] action state apply](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-42.jpg)
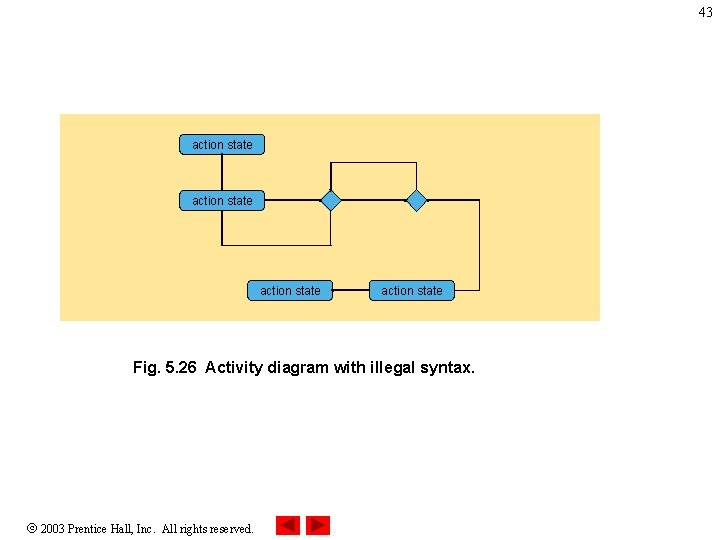
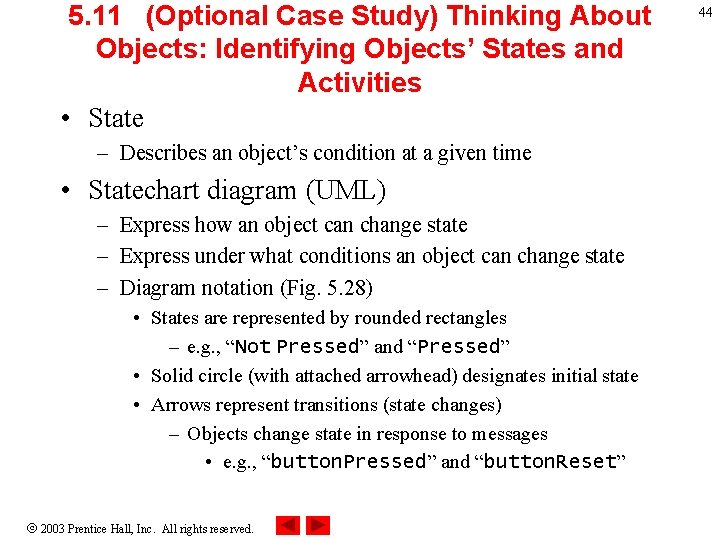
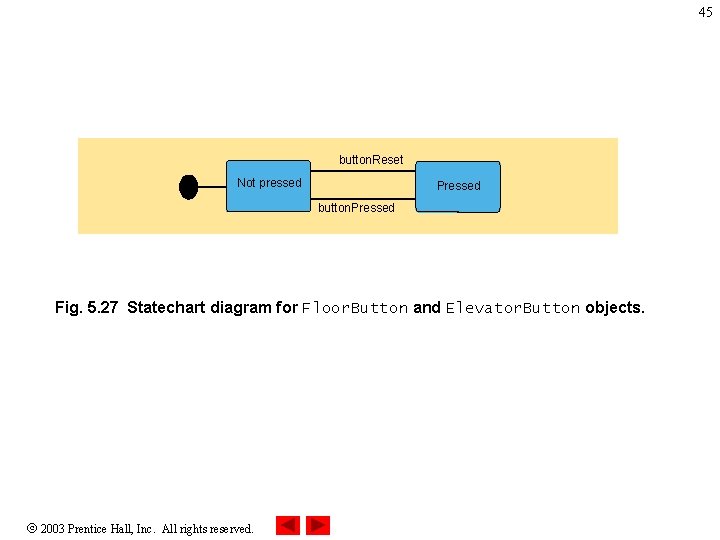
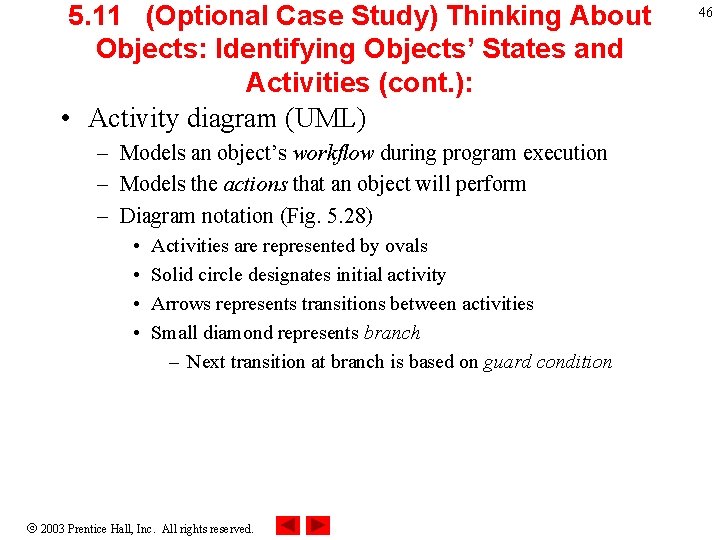
![47 move toward floor button [floor door closed] press floor button [floor door open] 47 move toward floor button [floor door closed] press floor button [floor door open]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-47.jpg)
![48 [floor button pressed] [elevator button pressed] set summoned to false close elevator door 48 [floor button pressed] [elevator button pressed] set summoned to false close elevator door](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-48.jpg)
- Slides: 48
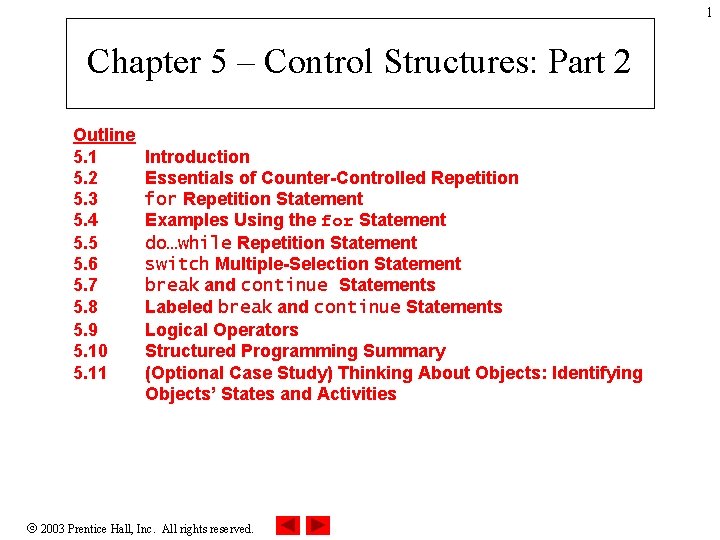
1 Chapter 5 – Control Structures: Part 2 Outline 5. 1 5. 2 5. 3 5. 4 5. 5 5. 6 5. 7 5. 8 5. 9 5. 10 5. 11 Introduction Essentials of Counter-Controlled Repetition for Repetition Statement Examples Using the for Statement do…while Repetition Statement switch Multiple-Selection Statement break and continue Statements Labeled break and continue Statements Logical Operators Structured Programming Summary (Optional Case Study) Thinking About Objects: Identifying Objects’ States and Activities 2003 Prentice Hall, Inc. All rights reserved.
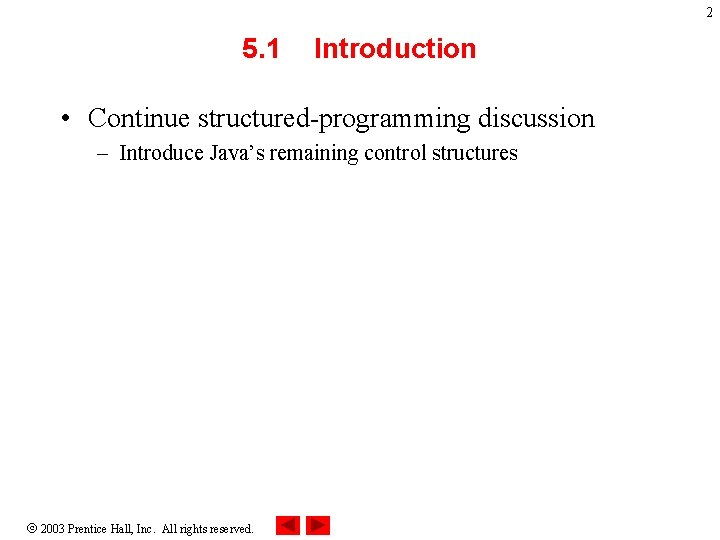
2 5. 1 Introduction • Continue structured-programming discussion – Introduce Java’s remaining control structures 2003 Prentice Hall, Inc. All rights reserved.
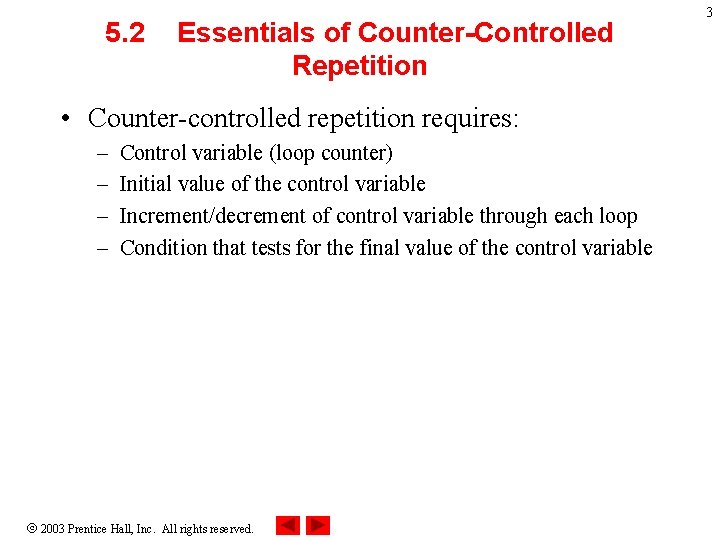
5. 2 Essentials of Counter-Controlled Repetition • Counter-controlled repetition requires: – – Control variable (loop counter) Initial value of the control variable Increment/decrement of control variable through each loop Condition that tests for the final value of the control variable 2003 Prentice Hall, Inc. All rights reserved. 3
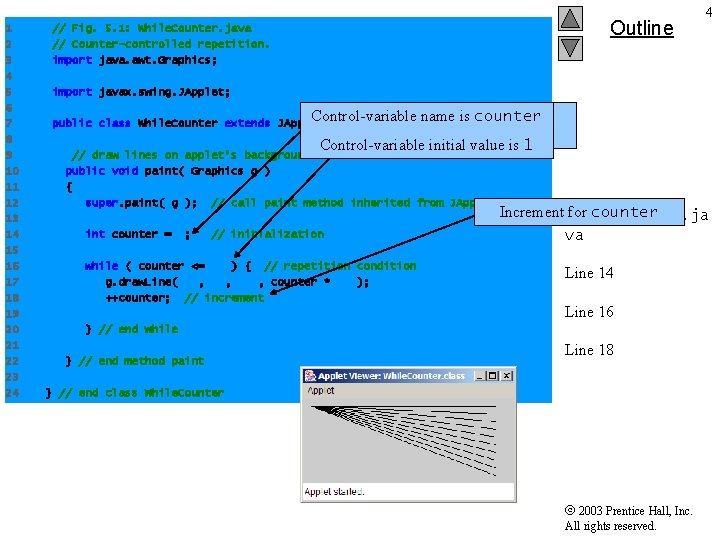
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 Outline // Fig. 5. 1: While. Counter. java // Counter-controlled repetition. import java. awt. Graphics; 4 import javax. swing. JApplet; Control-variable. Condition name is counter tests for counter’s final value Control-variable initial value is 1 public class While. Counter extends JApplet { // draw lines on applet’s background public void paint( Graphics g ) { super. paint( g ); // call paint method inherited from JApplet int counter = 1; // initialization while ( counter <= 10 ) { // repetition condition g. draw. Line( 10, 250, counter * 10 ); ++counter; // increment Increment While. Counter. ja for counter va Line 14 Line 16 } // end while } // end method paint Line 18 } // end class While. Counter 2003 Prentice Hall, Inc. All rights reserved.
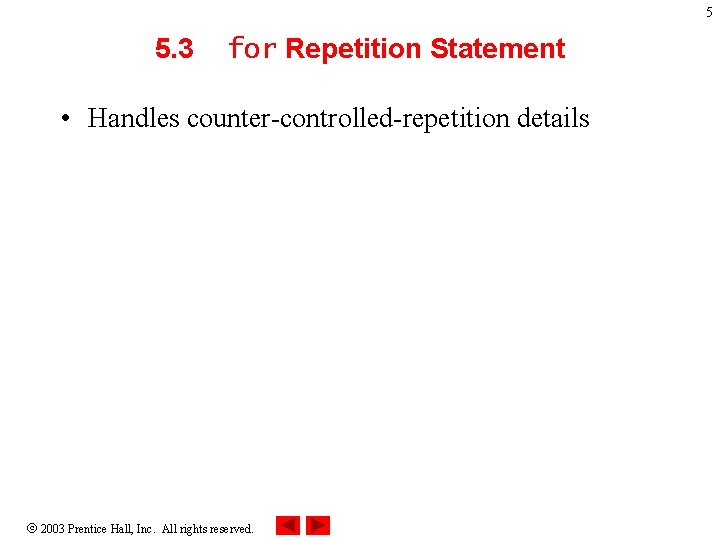
5 5. 3 for Repetition Statement • Handles counter-controlled-repetition details 2003 Prentice Hall, Inc. All rights reserved.
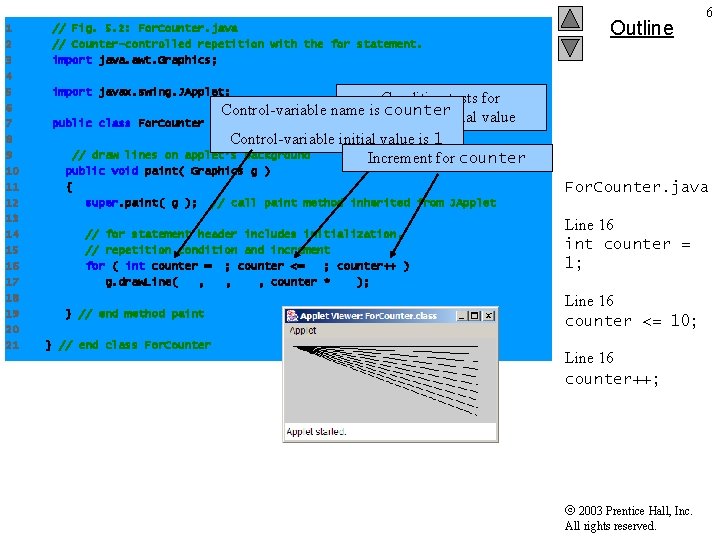
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 // Fig. 5. 2: For. Counter. java // Counter-controlled repetition with the for statement. import java. awt. Graphics; Outline 6 import javax. swing. JApplet; Condition tests for Control-variable namecounter’s is counterfinal value public class For. Counter extends JApplet { Control-variable initial value is 1 applet’s background Increment for counter // draw lines on public void paint( Graphics g ) { super. paint( g ); // call paint method inherited from JApplet // for statement header includes initialization, // repetition condition and increment for ( int counter = 1; counter <= 10; counter++ ) g. draw. Line( 10, 250, counter * 10 ); } // end method paint } // end class For. Counter. java Line 16 int counter = 1; Line 16 counter <= 10; Line 16 counter++; 2003 Prentice Hall, Inc. All rights reserved.
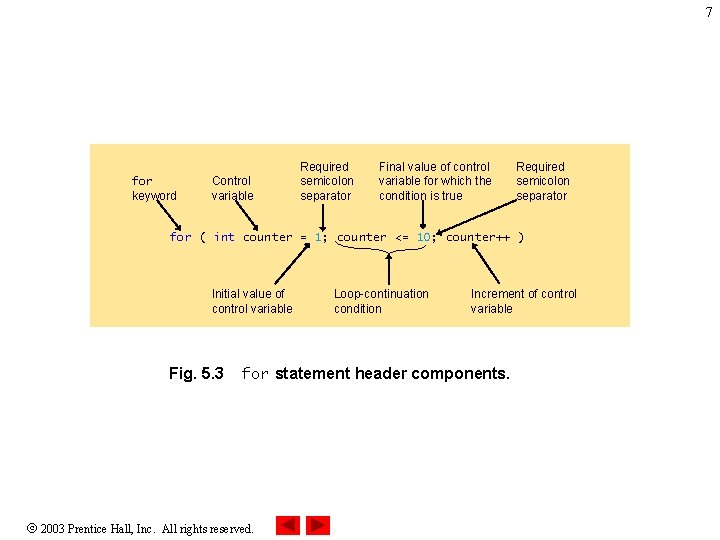
7 for keyword Control variable Required semicolon separator Final value of control variable for which the condition is true Required semicolon separator for ( int counter = 1; counter <= 10; counter++ ) Initial value of control variable Fig. 5. 3 Loop-continuation condition Increment of control variable for statement header components. 2003 Prentice Hall, Inc. All rights reserved.
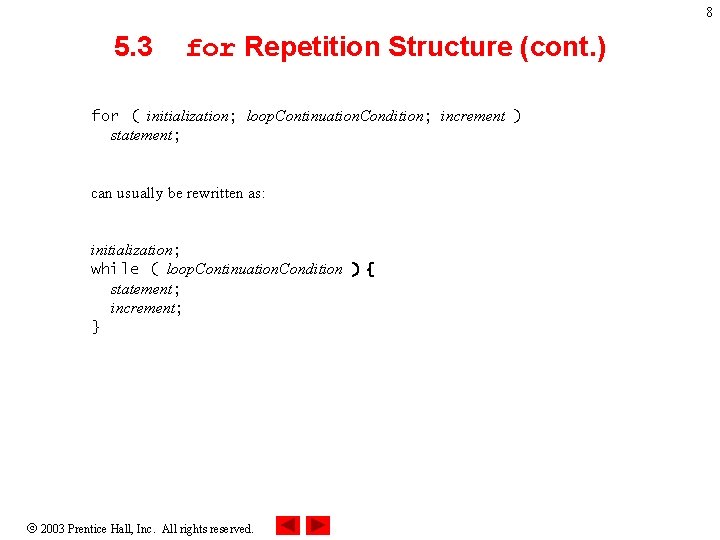
8 5. 3 for Repetition Structure (cont. ) for ( initialization; loop. Continuation. Condition; increment ) statement; can usually be rewritten as: initialization; while ( loop. Continuation. Condition ) { statement; increment; } 2003 Prentice Hall, Inc. All rights reserved.
![9 Establish initial value of control variable int counter 1 counter 10 9 Establish initial value of control variable int counter = 1 [counter <= 10]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-9.jpg)
9 Establish initial value of control variable int counter = 1 [counter <= 10] Draw a line on the applet Increment the control variable [counter > 10] Determine whether the final value of control variable has been reached Fig. 5. 4 g. draw. Line( 10, 250, counter * 10 ); for statement activity diagram. 2003 Prentice Hall, Inc. All rights reserved. counter++
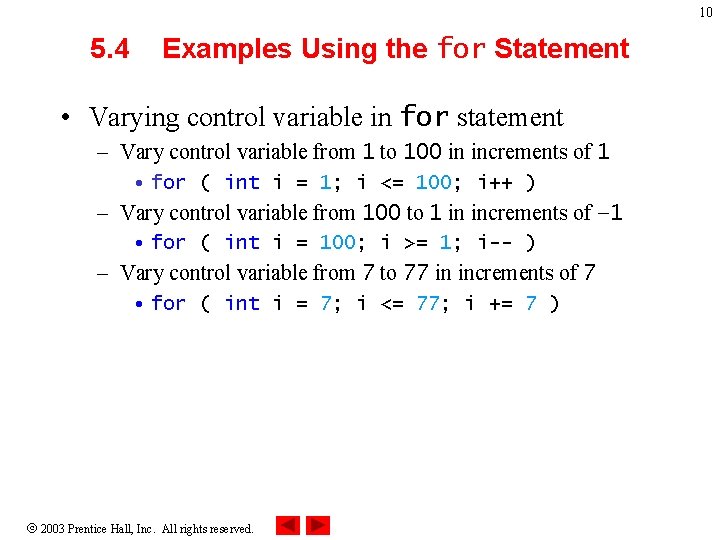
10 5. 4 Examples Using the for Statement • Varying control variable in for statement – Vary control variable from 1 to 100 in increments of 1 • for ( int i = 1; i <= 100; i++ ) – Vary control variable from 100 to 1 in increments of – 1 • for ( int i = 100; i >= 1; i-- ) – Vary control variable from 7 to 77 in increments of 7 • for ( int i = 7; i <= 77; i += 7 ) 2003 Prentice Hall, Inc. All rights reserved.
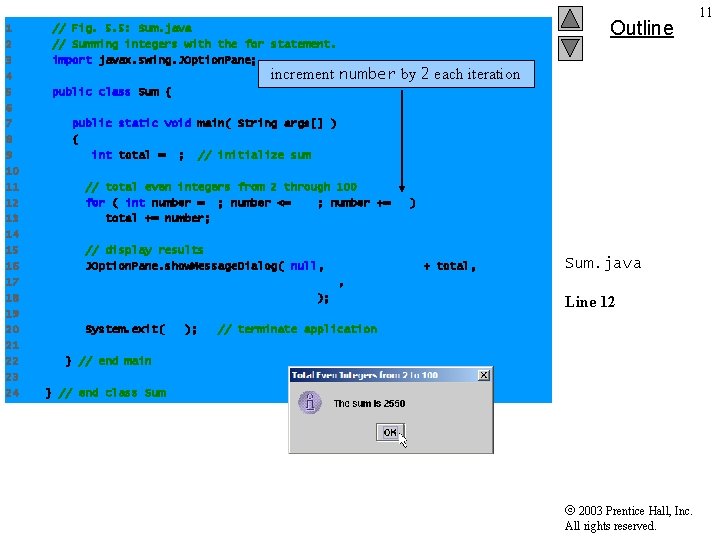
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 // Fig. 5. 5: Sum. java // Summing integers with the for statement. import javax. swing. JOption. Pane; Outline increment number by 2 each iteration public class Sum { public static void main( String args[] ) { int total = 0; // initialize sum // total even integers from 2 through 100 for ( int number = 2; number <= 100; number += 2 ) total += number; // display results JOption. Pane. show. Message. Dialog( null, "The sum is " + total, "Total Even Integers from 2 to 100", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); Sum. java Line 12 // terminate application } // end main } // end class Sum 2003 Prentice Hall, Inc. All rights reserved. 11
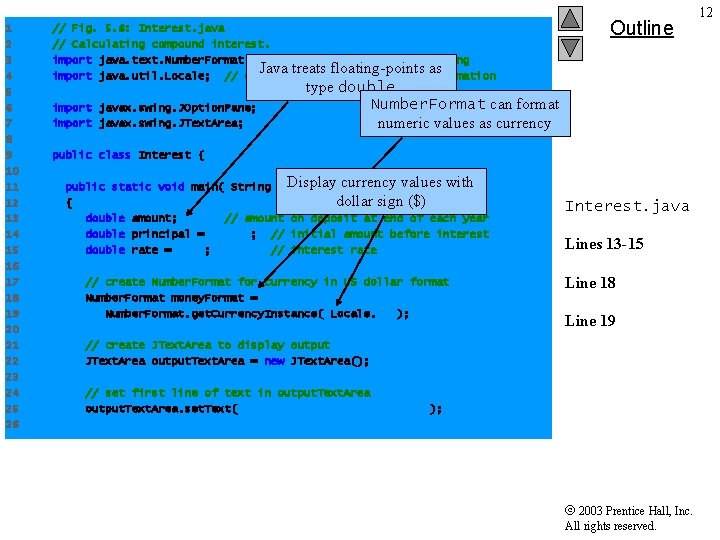
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // Fig. 5. 6: Interest. java // Calculating compound interest. import java. text. Number. Format; // class for numeric formatting Java treats floating-points as import java. util. Locale; // class for country-specific information import javax. swing. JOption. Pane; import javax. swing. JText. Area; Outline type double Number. Format can format numeric values as currency public class Interest { Display public static void main( String args[] ) currency values with { dollar sign ($) double amount; // amount on deposit at end of each year double principal = 1000. 0; // initial amount before interest double rate = 0. 05; // interest rate // create Number. Format for currency in US dollar format Number. Format money. Format = Number. Format. get. Currency. Instance( Locale. US ); Interest. java Lines 13 -15 Line 18 Line 19 // create JText. Area to display output JText. Area output. Text. Area = new JText. Area(); // set first line of text in output. Text. Area. set. Text( "Yeart. Amount on depositn" ); 2003 Prentice Hall, Inc. All rights reserved. 12
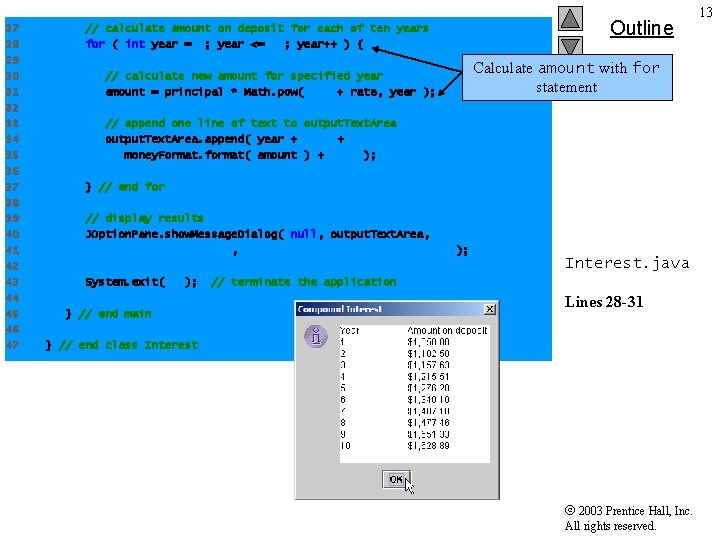
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 // calculate amount on deposit for each of ten years for ( int year = 1; year <= 10; year++ ) { // calculate new amount for specified year amount = principal * Math. pow( 1. 0 + rate, year ); Outline Calculate amount with for statement // append one line of text to output. Text. Area. append( year + "t" + money. Format. format( amount ) + "n" ); } // end for // display results JOption. Pane. show. Message. Dialog( null, output. Text. Area, "Compound Interest", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); } // end main Interest. java // terminate the application Lines 28 -31 } // end class Interest 2003 Prentice Hall, Inc. All rights reserved. 13
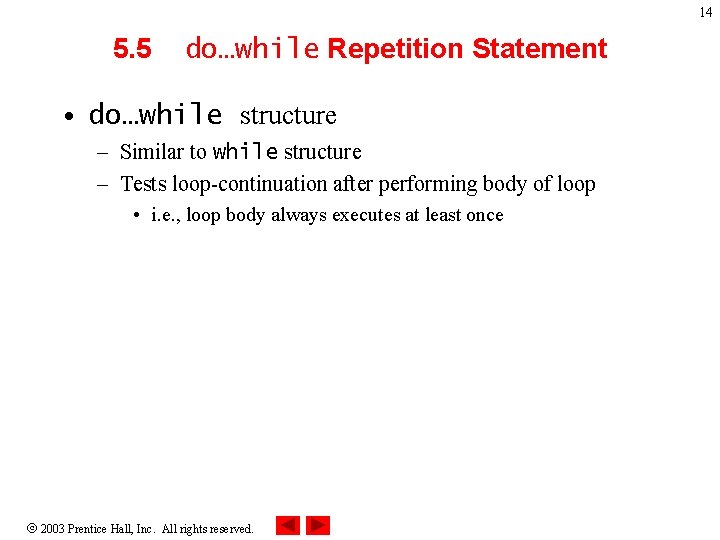
14 5. 5 do…while Repetition Statement • do…while structure – Similar to while structure – Tests loop-continuation after performing body of loop • i. e. , loop body always executes at least once 2003 Prentice Hall, Inc. All rights reserved.
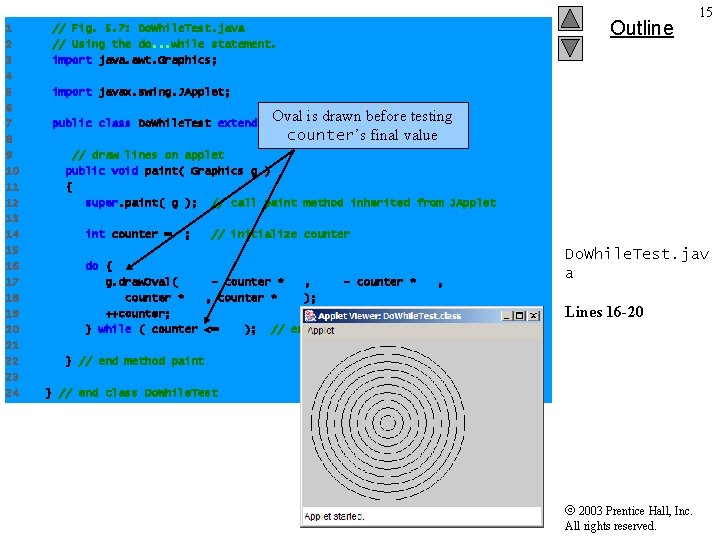
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 // Fig. 5. 7: Do. While. Test. java // Using the do. . . while statement. import java. awt. Graphics; Outline 15 import javax. swing. JApplet; Oval is drawn before testing counter’s final value public class Do. While. Test extends JApplet { // draw lines on applet public void paint( Graphics g ) { super. paint( g ); // call paint method inherited from JApplet int counter = 1; // initialize counter do { g. draw. Oval( 110 - counter * 10, counter * 20, counter * 20 ); ++counter; } while ( counter <= 10 ); // end do. . . while Do. While. Test. jav a Lines 16 -20 } // end method paint } // end class Do. While. Test 2003 Prentice Hall, Inc. All rights reserved.
![16 action state true condition false Fig 5 8 dowhile repetition statement activity diagram 16 action state [true] condition [false] Fig. 5. 8 do…while repetition statement activity diagram.](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-16.jpg)
16 action state [true] condition [false] Fig. 5. 8 do…while repetition statement activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
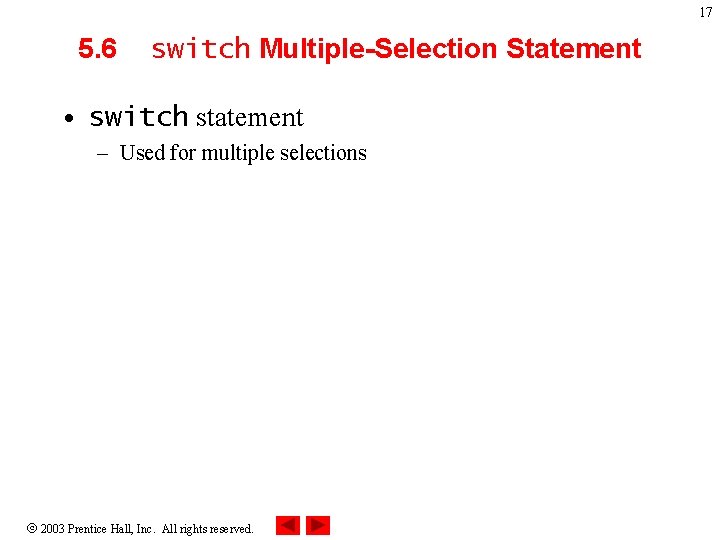
17 5. 6 switch Multiple-Selection Statement • switch statement – Used for multiple selections 2003 Prentice Hall, Inc. All rights reserved.
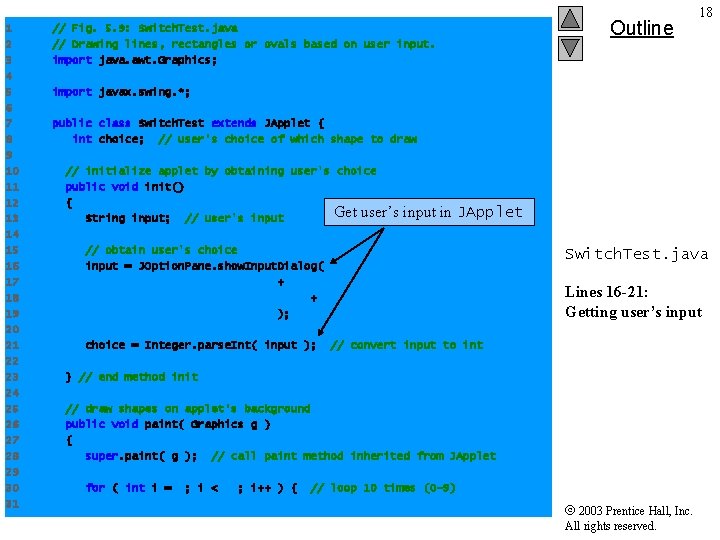
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 // Fig. 5. 9: Switch. Test. java // Drawing lines, rectangles or ovals based on user input. import java. awt. Graphics; Outline 18 import javax. swing. *; public class Switch. Test extends JApplet { int choice; // user's choice of which shape to draw // initialize applet by obtaining user's choice public void init() { Get user’s String input; // user's input in JApplet // obtain user's choice input = JOption. Pane. show. Input. Dialog( "Enter 1 to draw linesn" + "Enter 2 to draw rectanglesn" + "Enter 3 to draw ovalsn" ); choice = Integer. parse. Int( input ); Switch. Test. java Lines 16 -21: Getting user’s input // convert input to int } // end method init // draw shapes on applet's background public void paint( Graphics g ) { super. paint( g ); // call paint method inherited from JApplet for ( int i = 0; i < 10; i++ ) { // loop 10 times (0 -9) 2003 Prentice Hall, Inc. All rights reserved.
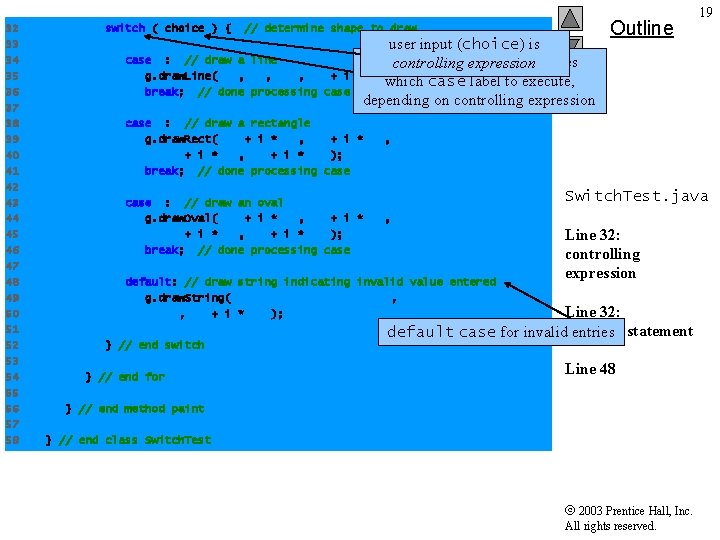
32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 switch ( choice ) { Outline // determine shape to draw user input (choice) is 19 case 1: // draw a line switch statement determines controlling expression g. draw. Line( 10, 250, 10 + i * 10 ); which case label to execute, break; // done processing case depending on controlling expression case 2: // draw a rectangle g. draw. Rect( 10 + i * 10, 50 + i * 10 ); break; // done processing case 3: // draw an oval g. draw. Oval( 10 + i * 10, 50 + i * 10 ); break; // done processing case default: // draw string indicating invalid value entered g. draw. String( "Invalid value entered", 10, 20 + i * 15 ); } // end switch } // end for Switch. Test. java Line 32: controlling expression Line 32: default case for invalidswitch entries statement Line 48 } // end method paint } // end class Switch. Test 2003 Prentice Hall, Inc. All rights reserved.
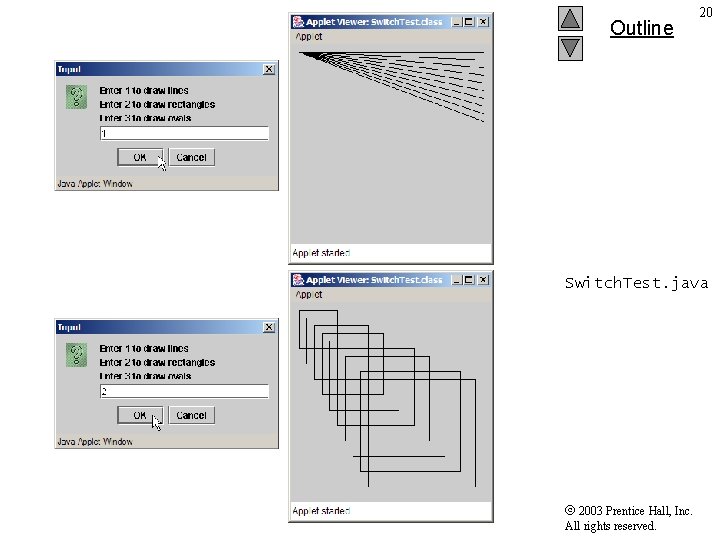
Outline 20 Switch. Test. java 2003 Prentice Hall, Inc. All rights reserved.
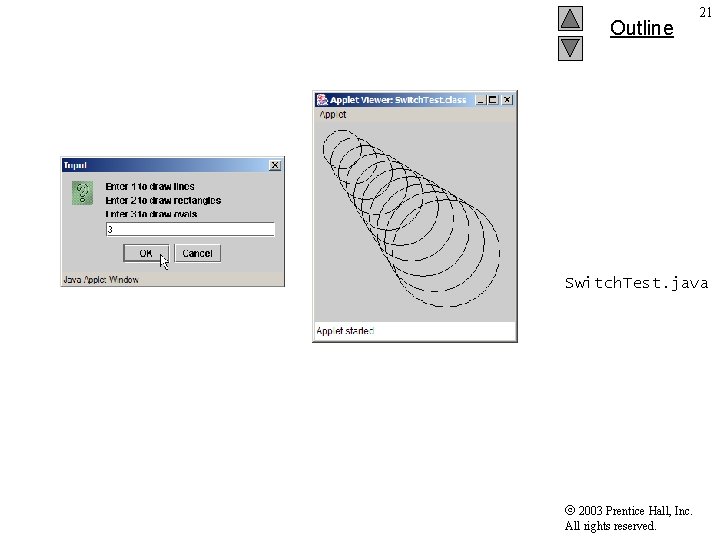
Outline 21 Switch. Test. java 2003 Prentice Hall, Inc. All rights reserved.
![22 true case a actions break case b actions break case z actions break 22 [true] case a action(s) break case b action(s) break case z action(s) break](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-22.jpg)
22 [true] case a action(s) break case b action(s) break case z action(s) break [false] [true] case b [false] . . . [true] case z [false] default action(s) Fig. 5. 10 switch multiple-selection statement activity diagram with break statements. 2003 Prentice Hall, Inc. All rights reserved.
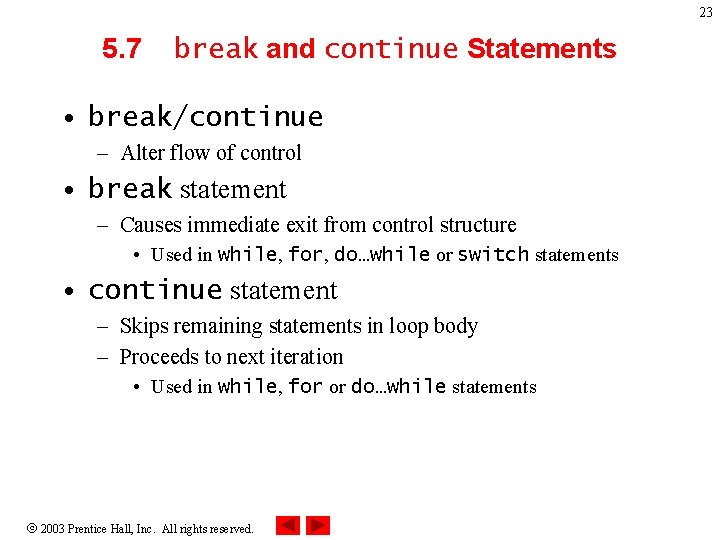
23 5. 7 break and continue Statements • break/continue – Alter flow of control • break statement – Causes immediate exit from control structure • Used in while, for, do…while or switch statements • continue statement – Skips remaining statements in loop body – Proceeds to next iteration • Used in while, for or do…while statements 2003 Prentice Hall, Inc. All rights reserved.
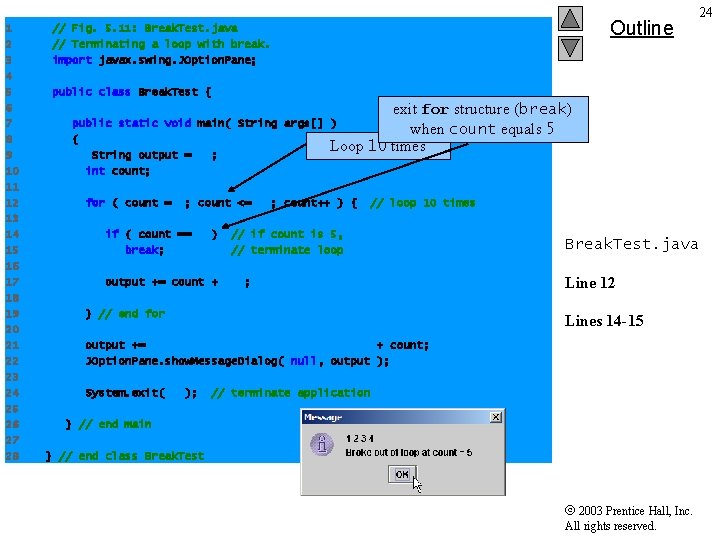
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 Outline // Fig. 5. 11: Break. Test. java // Terminating a loop with break. import javax. swing. JOption. Pane; 24 public class Break. Test { public static void main( String args[] ) { Loop String output = ""; int count; exit for structure (break) when count equals 5 10 times for ( count = 1; count <= 10; count++ ) { if ( count == 5 ) break; // loop 10 times // if count is 5, // terminate loop output += count + " "; } // end for Break. Test. java Line 12 Lines 14 -15 output += "n. Broke out of loop at count = " + count; JOption. Pane. show. Message. Dialog( null, output ); System. exit( 0 ); // terminate application } // end main } // end class Break. Test 2003 Prentice Hall, Inc. All rights reserved.
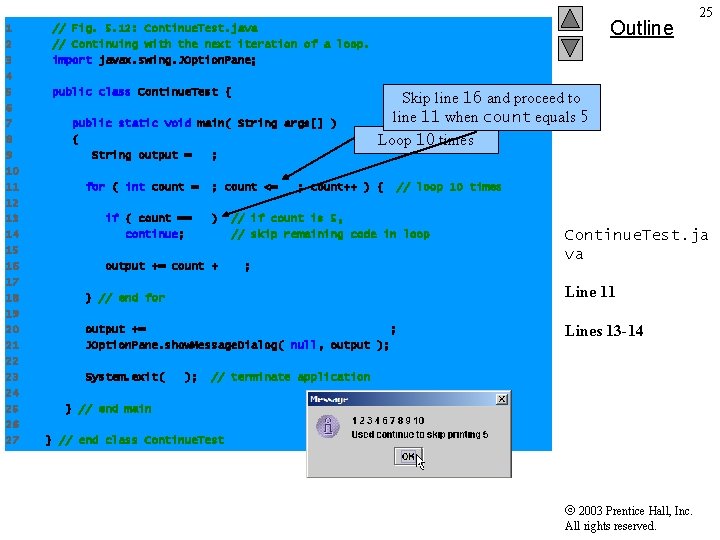
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 Outline // Fig. 5. 12: Continue. Test. java // Continuing with the next iteration of a loop. import javax. swing. JOption. Pane; public class Continue. Test { public static void main( String args[] ) { String output = ""; Skip line 16 and proceed to line 11 when count equals 5 Loop 10 times for ( int count = 1; count <= 10; count++ ) { if ( count == 5 ) continue; // loop 10 times // if count is 5, // skip remaining code in loop output += count + " "; Continue. Test. ja va Line 11 } // end for output += "n. Used continue to skip printing 5"; JOption. Pane. show. Message. Dialog( null, output ); System. exit( 0 ); 25 Lines 13 -14 // terminate application } // end main } // end class Continue. Test 2003 Prentice Hall, Inc. All rights reserved.
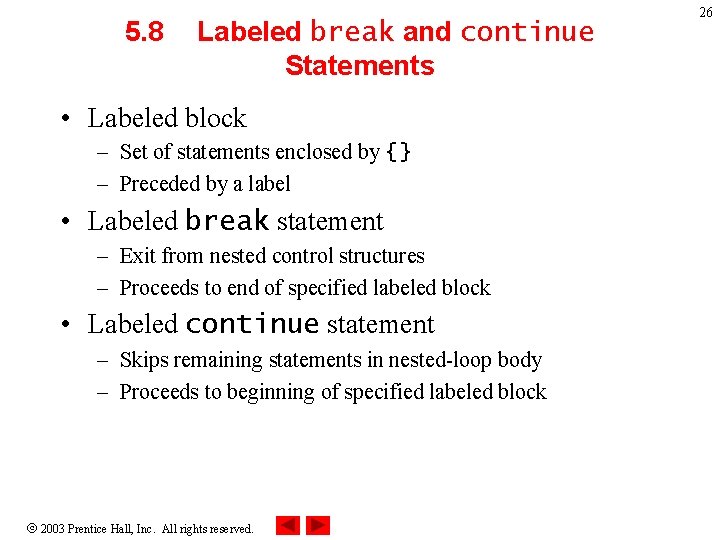
5. 8 Labeled break and continue Statements • Labeled block – Set of statements enclosed by {} – Preceded by a label • Labeled break statement – Exit from nested control structures – Proceeds to end of specified labeled block • Labeled continue statement – Skips remaining statements in nested-loop body – Proceeds to beginning of specified labeled block 2003 Prentice Hall, Inc. All rights reserved. 26
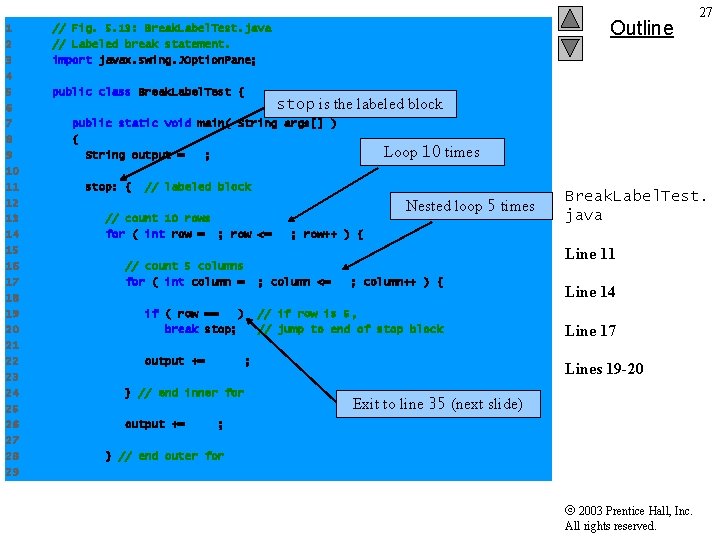
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 Outline // Fig. 5. 13: Break. Label. Test. java // Labeled break statement. import javax. swing. JOption. Pane; public class Break. Label. Test { stop is the labeled block public static void main( String args[] ) { String output = ""; stop: { Loop 10 times // labeled block // count 10 rows for ( int row = 1; row <= 10; row++ ) { Nested loop 5 times // count 5 columns for ( int column = 1; column <= 5 ; column++ ) { if ( row == 5 ) break stop; output += "* // if row is 5, // jump to end of stop block "; } // end inner for 27 Break. Label. Test. java Line 11 Line 14 Line 17 Lines 19 -20 Exit to line 35 (next slide) output += "n"; } // end outer for 2003 Prentice Hall, Inc. All rights reserved.
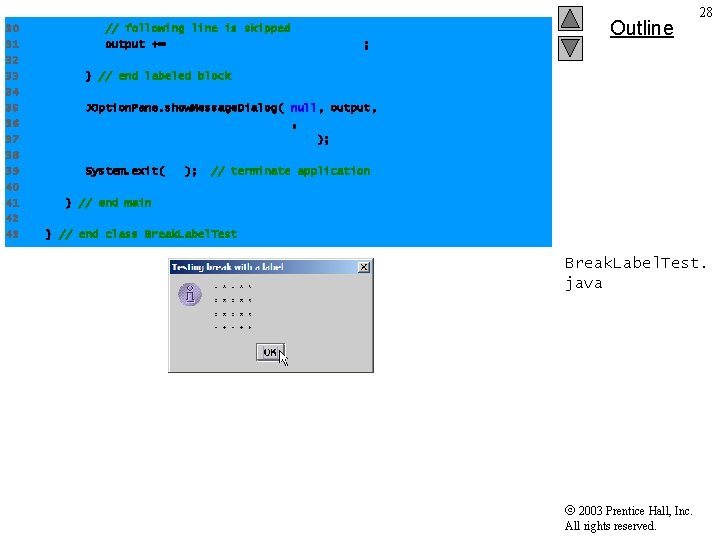
30 31 32 33 34 35 36 37 38 39 40 41 42 43 // following line is skipped output += "n. Loops terminated normally"; Outline 28 } // end labeled block JOption. Pane. show. Message. Dialog( null, output, "Testing break with a label", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); // terminate application } // end main } // end class Break. Label. Test. java 2003 Prentice Hall, Inc. All rights reserved.
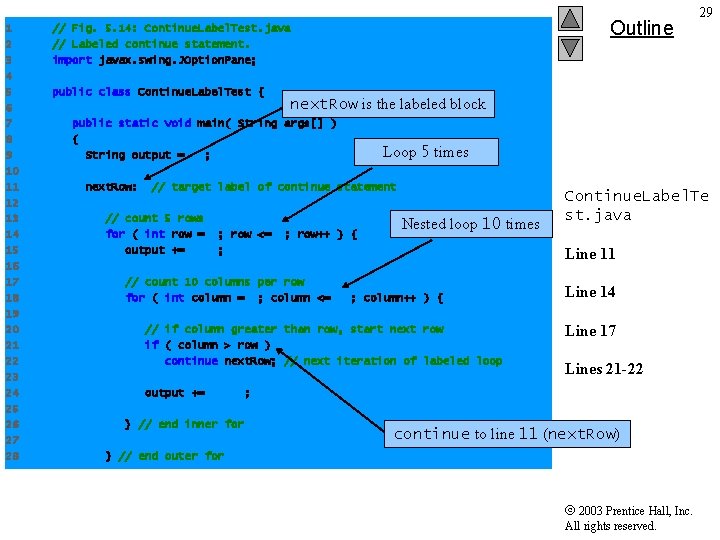
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 Outline // Fig. 5. 14: Continue. Label. Test. java // Labeled continue statement. import javax. swing. JOption. Pane; public class Continue. Label. Test { next. Row is the labeled block public static void main( String args[] ) { String output = ""; next. Row: Loop 5 times // target label of continue statement // count 5 rows for ( int row = 1; row <= 5; row++ ) { output += "n"; Nested loop 10 times Continue. Label. Te st. java Line 11 // count 10 columns per row for ( int column = 1; column <= 10; column++ ) { // if column greater than row, start next row if ( column > row ) continue next. Row; // next iteration of labeled loop output += "* Line 14 Line 17 Lines 21 -22 "; } // end inner for 29 continue to line 11 (next. Row) } // end outer for 2003 Prentice Hall, Inc. All rights reserved.
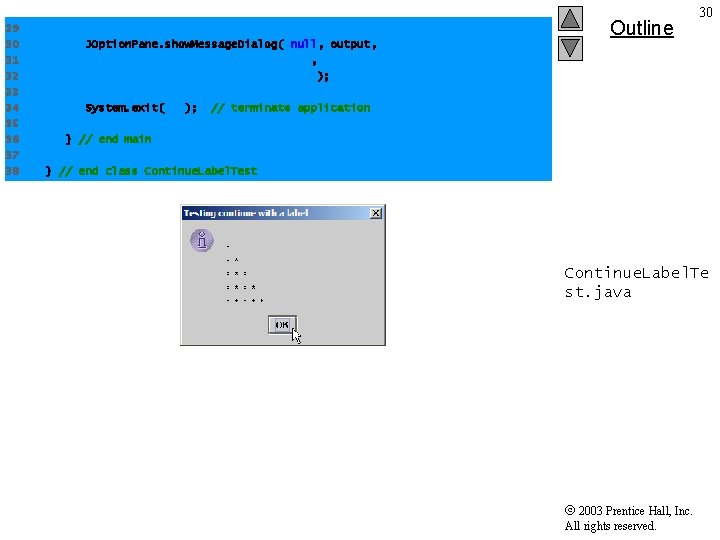
29 30 31 32 33 34 35 36 37 38 JOption. Pane. show. Message. Dialog( null, output, "Testing continue with a label", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); Outline 30 // terminate application } // end main } // end class Continue. Label. Test Continue. Label. Te st. java 2003 Prentice Hall, Inc. All rights reserved.
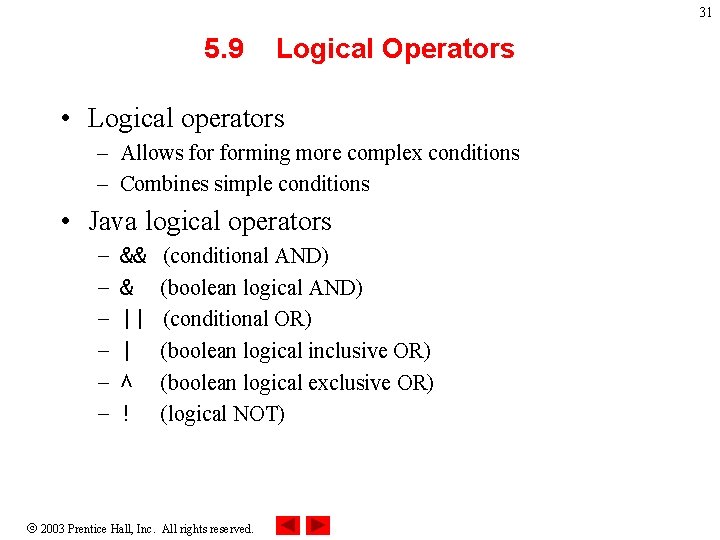
31 5. 9 Logical Operators • Logical operators – Allows forming more complex conditions – Combines simple conditions • Java logical operators – – – && & || | ^ ! (conditional AND) (boolean logical AND) (conditional OR) (boolean logical inclusive OR) (boolean logical exclusive OR) (logical NOT) 2003 Prentice Hall, Inc. All rights reserved.
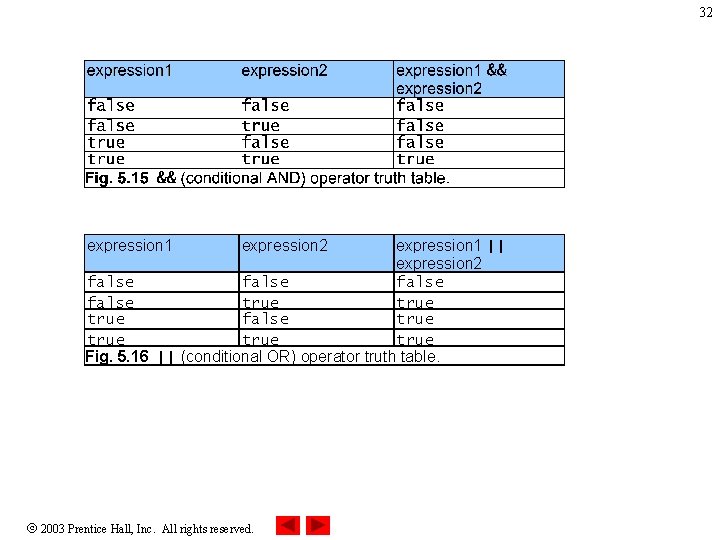
32 expression 1 expression 2 expression 1 || expression 2 false true false true Fig. 5. 16 || (conditional OR) operator truth table. 2003 Prentice Hall, Inc. All rights reserved.
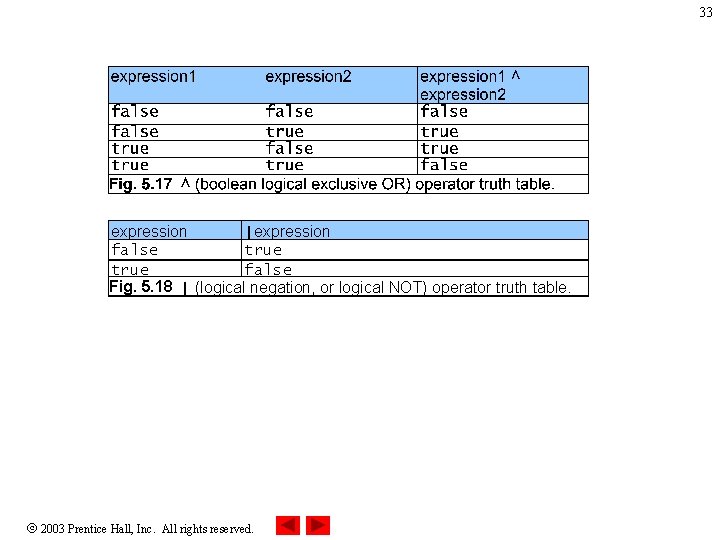
33 expression !expression false true false Fig. 5. 18 ! (logical negation, or logical NOT) operator truth table. 2003 Prentice Hall, Inc. All rights reserved.
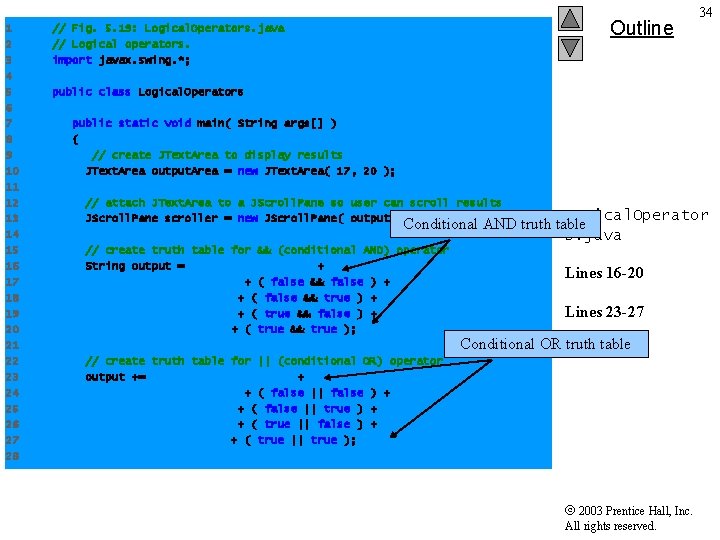
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 Outline // Fig. 5. 19: Logical. Operators. java // Logical operators. import javax. swing. *; 34 public class Logical. Operators public static void main( String args[] ) { // create JText. Area to display results JText. Area output. Area = new JText. Area( 17, 20 ); // attach JText. Area to a JScroll. Pane so user can scroll results JScroll. Pane scroller = new JScroll. Pane( output. Area ); Logical. Operator Conditional AND truth table s. java // create truth table for && (conditional AND) operator String output = "Logical AND (&&)" + "nfalse && false: " + ( false && false ) + "nfalse && true: " + ( false && true ) + "ntrue && false: " + ( true && false ) + "ntrue && true: " + ( true && true ); Lines 16 -20 Lines 23 -27 Conditional OR truth table // create truth table for || (conditional OR) operator output += "nn. Logical OR (||)" + "nfalse || false: " + ( false || false ) + "nfalse || true: " + ( false || true ) + "ntrue || false: " + ( true || false ) + "ntrue || true: " + ( true || true ); 2003 Prentice Hall, Inc. All rights reserved.
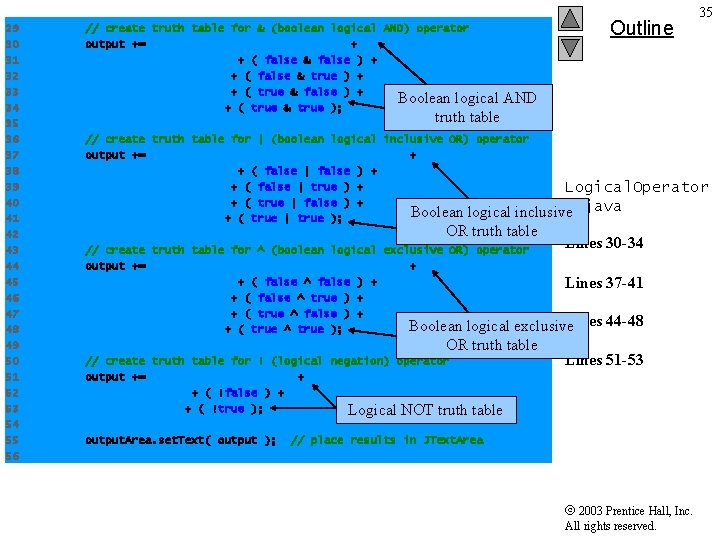
29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 // create truth table for & (boolean logical AND) operator output += "nn. Boolean logical AND (&)" + "nfalse & false: " + ( false & false ) + "nfalse & true: " + ( false & true ) + "ntrue & false: " + ( true & false ) + Boolean logical "ntrue & true: " + ( true & true ); Outline 35 AND truth table // create truth table for | (boolean logical inclusive OR) operator output += "nn. Boolean logical inclusive OR (|)" + "nfalse | false: " + ( false | false ) + "nfalse | true: " + ( false | true ) + Logical. Operator "ntrue | false: " + ( true | false ) + Boolean logical inclusives. java "ntrue | true: " + ( true | true ); OR truth table Lines 30 -34 // create truth table for ^ (boolean logical exclusive OR) operator output += "nn. Boolean logical exclusive OR (^)" + "nfalse ^ false: " + ( false ^ false ) + Lines "nfalse ^ true: " + ( false ^ true ) + "ntrue ^ false: " + ( true ^ false ) + Lines Boolean logical exclusive "ntrue ^ true: " + ( true ^ true ); OR truth table // create truth table for ! (logical negation) operator output += "nn. Logical NOT (!)" + "n!false: " + ( !false ) + "n!true: " + ( !true ); Logical NOT truth output. Area. set. Text( output ); 37 -41 44 -48 Lines 51 -53 table // place results in JText. Area 2003 Prentice Hall, Inc. All rights reserved.
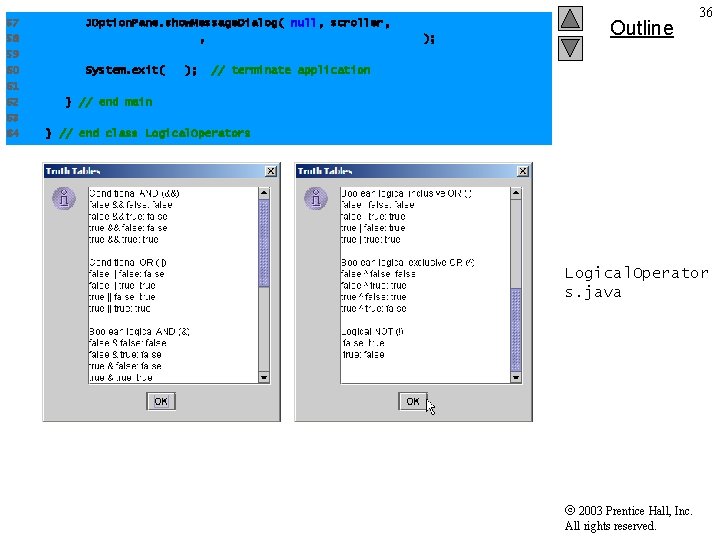
57 58 59 60 61 62 63 64 JOption. Pane. show. Message. Dialog( null, scroller, "Truth Tables", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); Outline 36 // terminate application } // end main } // end class Logical. Operator s. java 2003 Prentice Hall, Inc. All rights reserved.
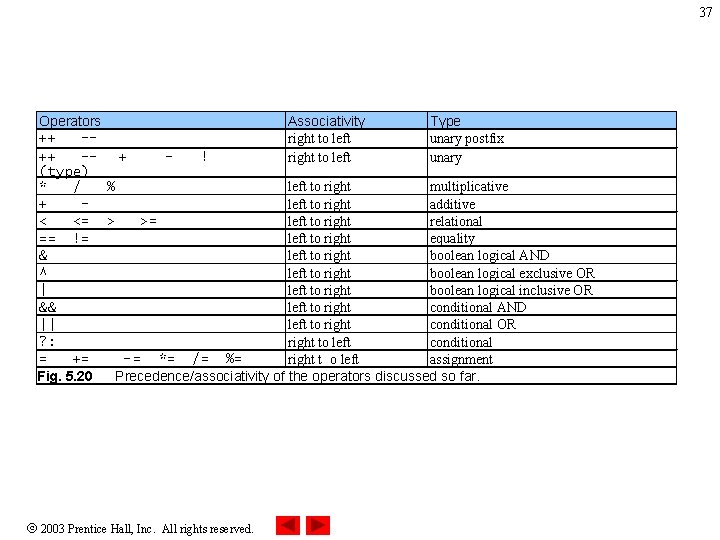
37 Operators Associativity Type ++ -right to left unary postfix ++ -- + ! right to left unary (type) * / % left to right multiplicative + left to right additive < <= > >= left to right relational == != left to right equality & left to right boolean logical AND ^ left to right boolean logical exclusive OR | boolean logical inclusive OR left to right && left to right conditional AND || left to right conditional OR ? : right to left conditional = += - = *= /= %= right t o left assignment Fig. 5. 20 Precedence/associativity of the operators discussed so far. 2003 Prentice Hall, Inc. All rights reserved.
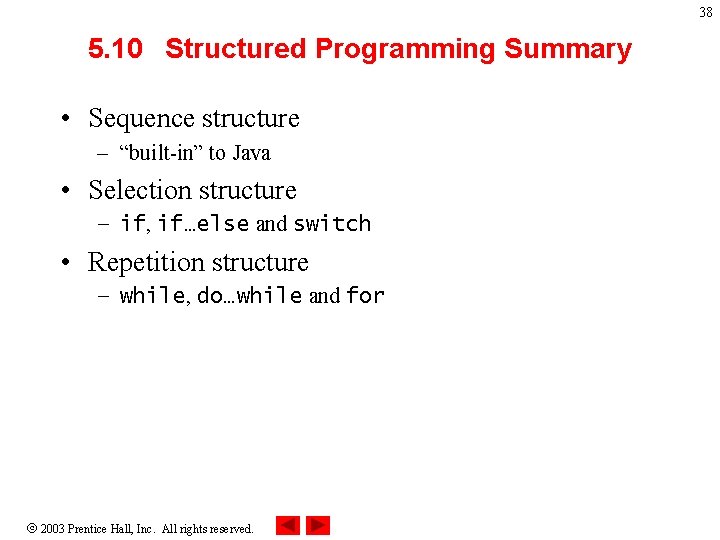
38 5. 10 Structured Programming Summary • Sequence structure – “built-in” to Java • Selection structure – if, if…else and switch • Repetition structure – while, do…while and for 2003 Prentice Hall, Inc. All rights reserved.
![39 Sequence Selection if statement single selection switch statement multiple selection t f t 39 Sequence Selection if statement (single selection) switch statement (multiple selection) [t] [f] [t]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-39.jpg)
39 Sequence Selection if statement (single selection) switch statement (multiple selection) [t] [f] [t] if else statement (double selection) [f] break . . . [t] [f] break default Repetition while statement do while statement for statement [t] [f] Fig. 5. 21 Java’s single-entry/single-exit sequence, selection and repetition statements. 2003 Prentice Hall, Inc. All rights reserved.
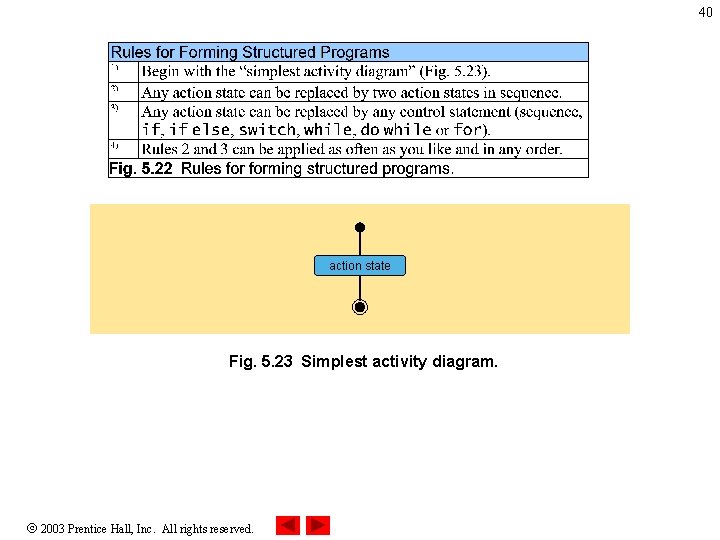
40 action state Fig. 5. 23 Simplest activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
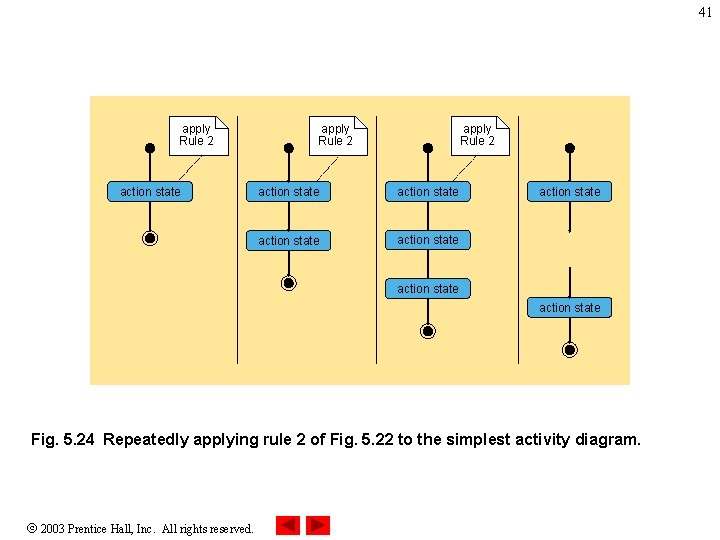
41 apply Rule 2 action state action state . . . action state Fig. 5. 24 Repeatedly applying rule 2 of Fig. 5. 22 to the simplest activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
![42 apply Rule 3 action state f f action state t action state apply 42 apply Rule 3 action state [f] [f] action state [t] action state apply](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-42.jpg)
42 apply Rule 3 action state [f] [f] action state [t] action state apply Rule 3 [t] [f] action state [t] action state Fig. 5. 25 Applying rule 3 of Fig. 5. 22 to the simplest activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
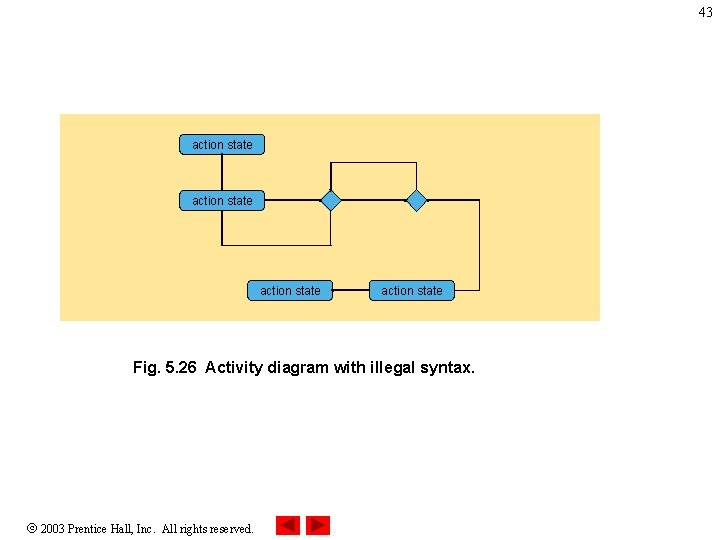
43 action state Fig. 5. 26 Activity diagram with illegal syntax. 2003 Prentice Hall, Inc. All rights reserved.
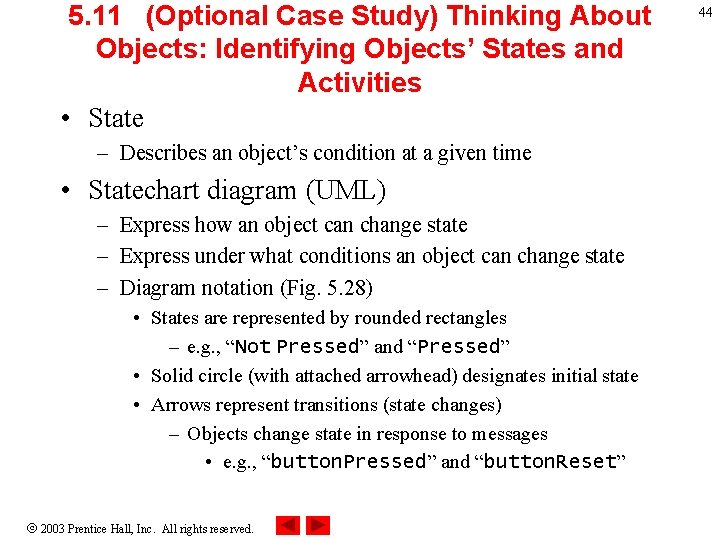
5. 11 (Optional Case Study) Thinking About Objects: Identifying Objects’ States and Activities • State – Describes an object’s condition at a given time • Statechart diagram (UML) – Express how an object can change state – Express under what conditions an object can change state – Diagram notation (Fig. 5. 28) • States are represented by rounded rectangles – e. g. , “Not Pressed” and “Pressed” • Solid circle (with attached arrowhead) designates initial state • Arrows represent transitions (state changes) – Objects change state in response to messages • e. g. , “button. Pressed” and “button. Reset” 2003 Prentice Hall, Inc. All rights reserved. 44
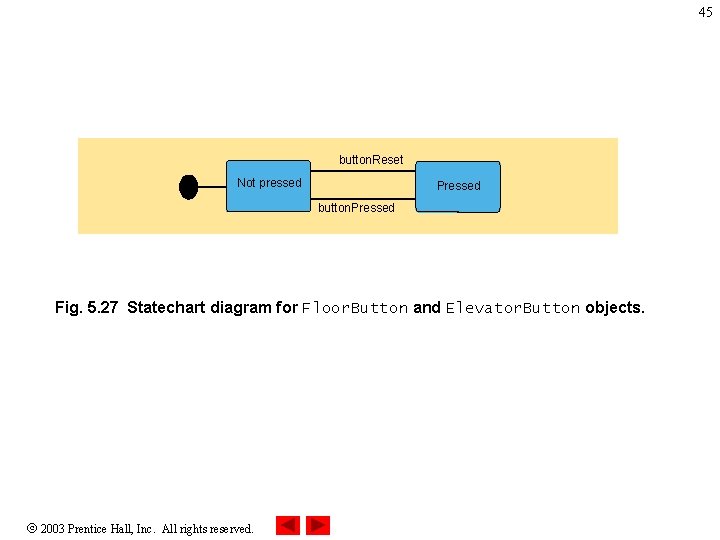
45 button. Reset Not pressed Pressed button. Pressed Fig. 5. 27 Statechart diagram for Floor. Button and Elevator. Button objects. 2003 Prentice Hall, Inc. All rights reserved.
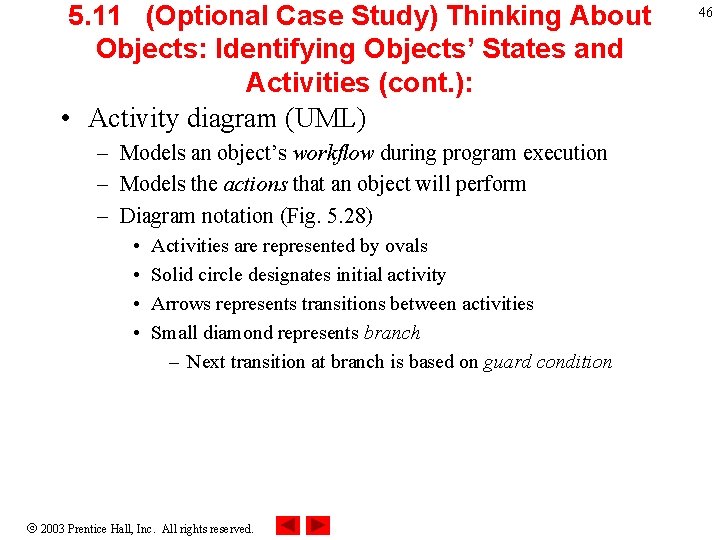
5. 11 (Optional Case Study) Thinking About Objects: Identifying Objects’ States and Activities (cont. ): • Activity diagram (UML) – Models an object’s workflow during program execution – Models the actions that an object will perform – Diagram notation (Fig. 5. 28) • • Activities are represented by ovals Solid circle designates initial activity Arrows represents transitions between activities Small diamond represents branch – Next transition at branch is based on guard condition 2003 Prentice Hall, Inc. All rights reserved. 46
![47 move toward floor button floor door closed press floor button floor door open 47 move toward floor button [floor door closed] press floor button [floor door open]](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-47.jpg)
47 move toward floor button [floor door closed] press floor button [floor door open] wait for door to open [no passenger on elevator] [passenger on elevator] wait for passenger to exit elevator enter elevator press elevator button wait for door to open exit elevator Fig. 5. 28 Activity diagram for a Person object. 2003 Prentice Hall, Inc. All rights reserved.
![48 floor button pressed elevator button pressed set summoned to false close elevator door 48 [floor button pressed] [elevator button pressed] set summoned to false close elevator door](https://slidetodoc.com/presentation_image_h2/4bedd26d35bfbb7d70e7dff8368b1844/image-48.jpg)
48 [floor button pressed] [elevator button pressed] set summoned to false close elevator door [button on destination[elevator idle] floor pressed] [elevator moving] [button on destination floor pressed] move to destination floor reset elevator button [button on current floor pressed] ring bell [button on current floor pressed] set summoned to true open elevator door [summoned] [not summoned] Fig. 5. 29 Activity diagram for the Elevator object. 2003 Prentice Hall, Inc. All rights reserved.
Analogous structure
Quote one topic sentence
Resolution in a story example
Part of story structures
Ap seminar part b outline
What is the name of the first part of an outline?
Hardware and control structures
Statement level control structures
Intro.php?aid=
Control structure in c
Repetition control statement
Types of control structures
Branching instructions in 8086
Statement level control structures
Control structures in php
Iteration control structures
One way selection
Iteration control structures
Entity life history
Pseudocode outline
Visual basic control structures
Support control and movement lesson outline
Lesson outline structure movement and control
Part whole model subtraction
Part to part ratio definition
Part part whole
Technical description examples
Bar parts
The part of a shadow surrounding the darkest part
Part to part variation
Safe driving points balance virginia
Roll drivers ed
Chapter 2 analyzing transactions
Analyzing transactions in a cash control system
Lattice organizational structure
What is a monopolistic competition example
Chapter 7 section 3 structures and organelles
Chapter 16 worksheet the knee and related structures
Chapter 16 worksheet the knee and related structures
Chapter 7 organizational structures
Data structures chapter 1
Chapter 7 market structures vocabulary
Hello market
Chapter 7 section 3 structures and organelles
Translational research institute on pain in later life
Process control and product control
Control volume vs control surface
Stock control e flow control
Control volume vs control surface