1 Chapter 4 Control Structures Part 1 Outline
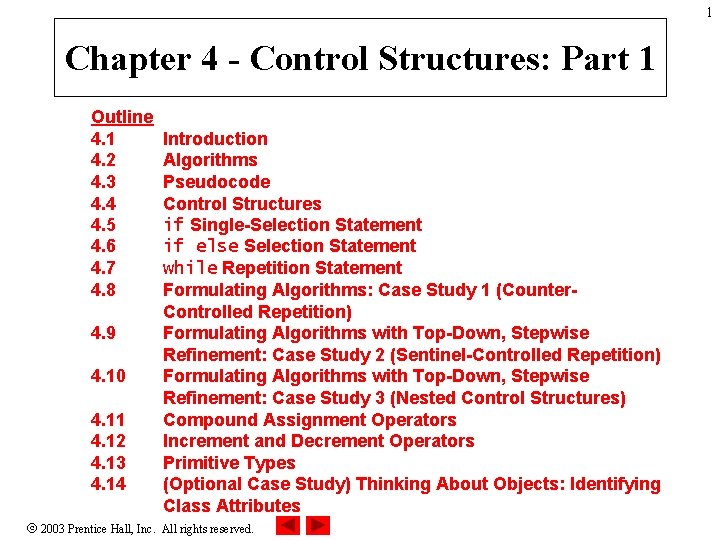
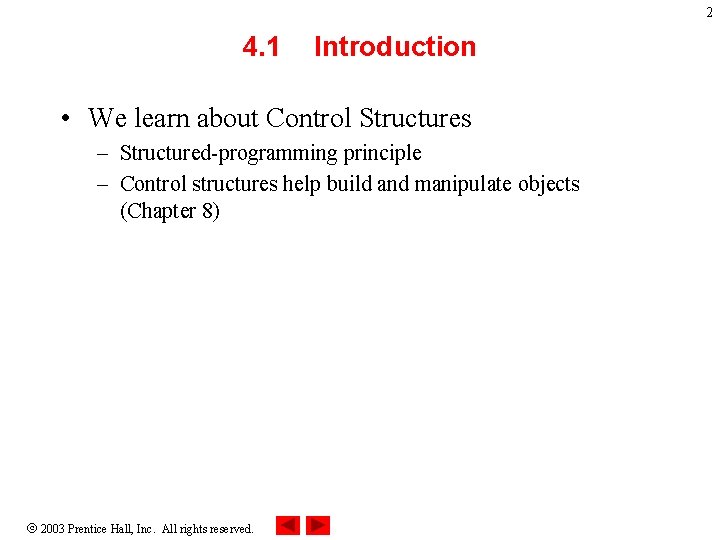
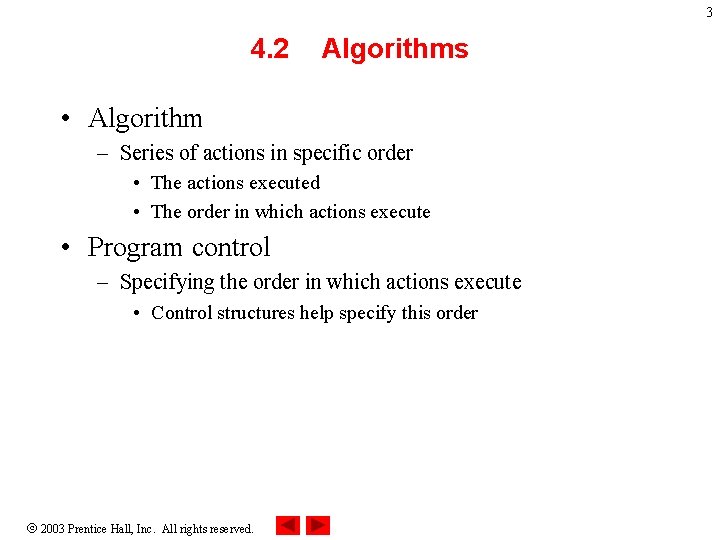
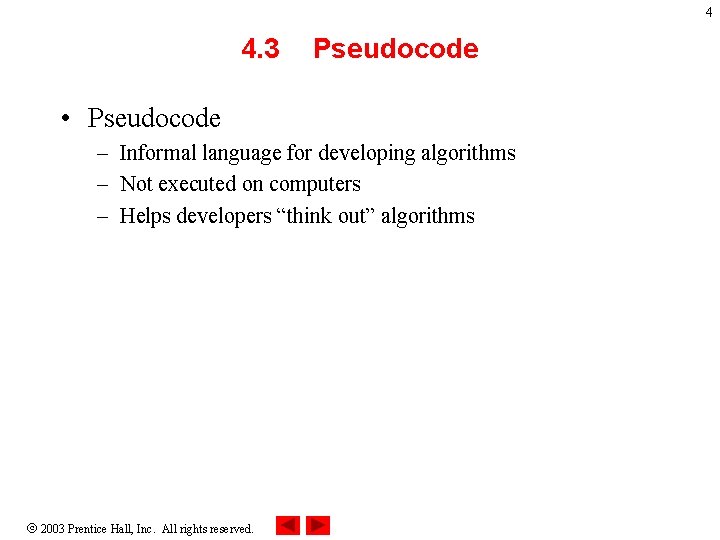
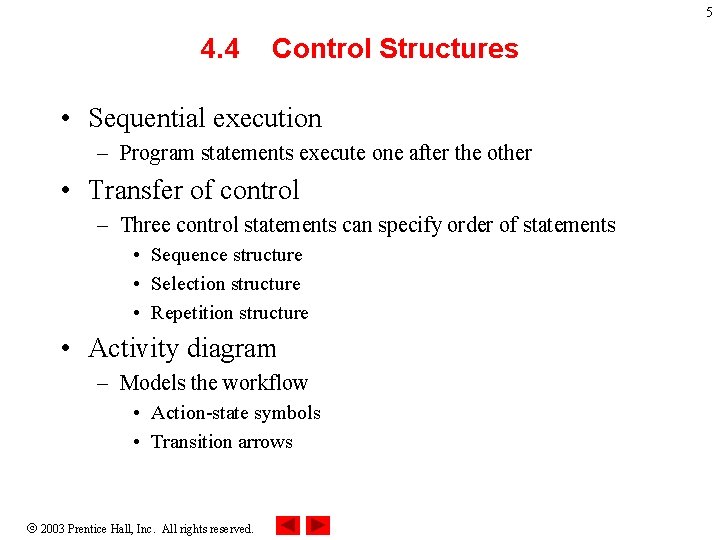
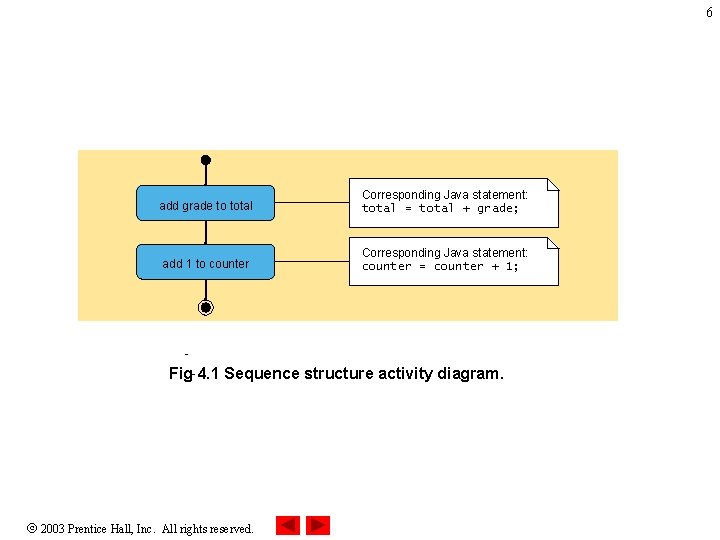
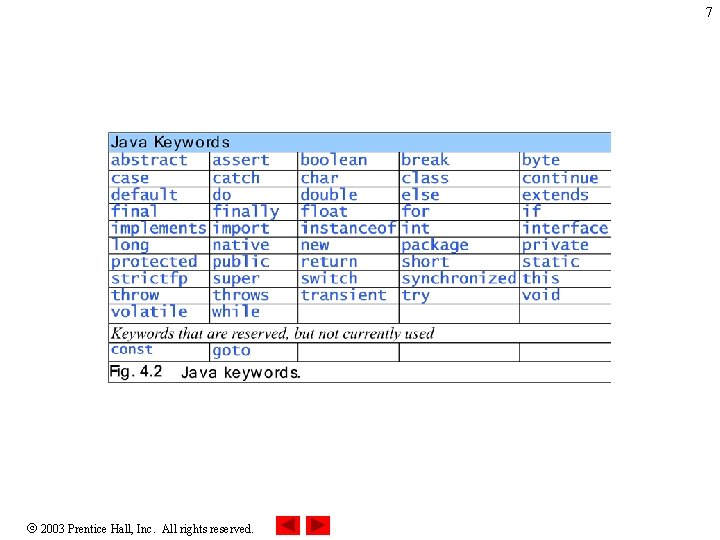
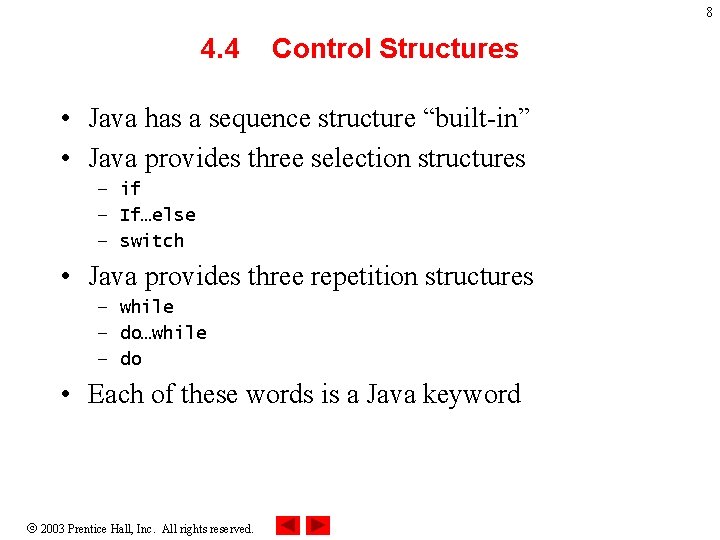
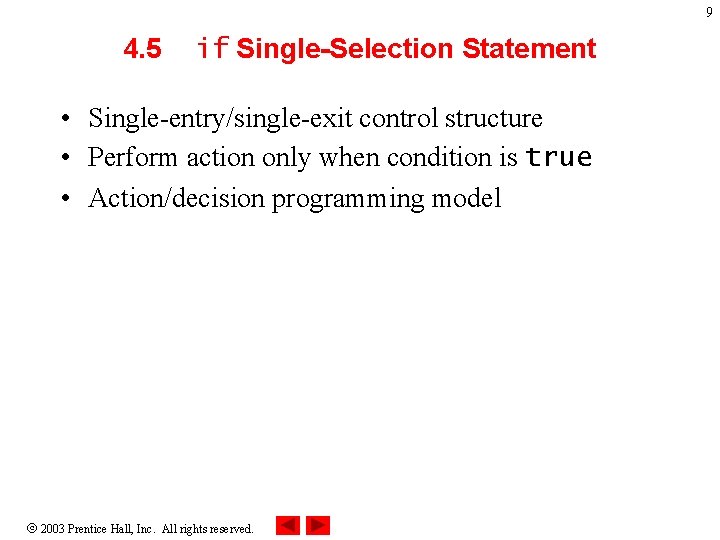
![10 [grade >= 60] print “Passed” [grade < 60] Fig 4. 3 if single-selections 10 [grade >= 60] print “Passed” [grade < 60] Fig 4. 3 if single-selections](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-10.jpg)
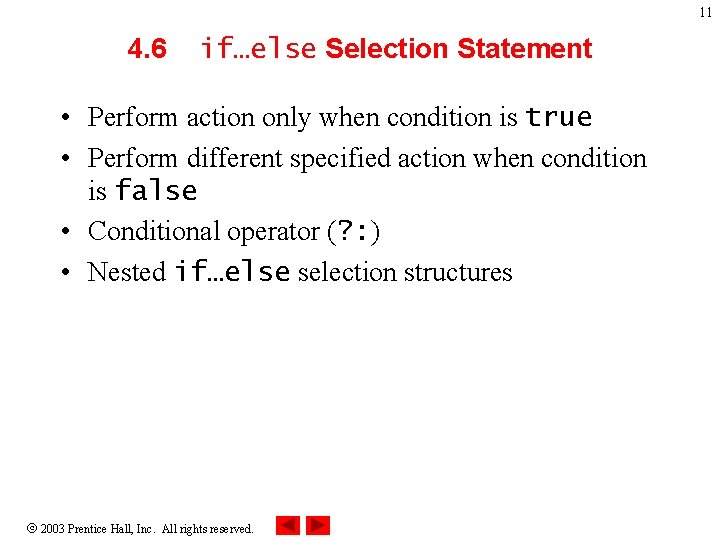
![12 [grade < 60] print “Failed” [grade >= 60] print “Passed” Fig 4. 4 12 [grade < 60] print “Failed” [grade >= 60] print “Passed” Fig 4. 4](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-12.jpg)
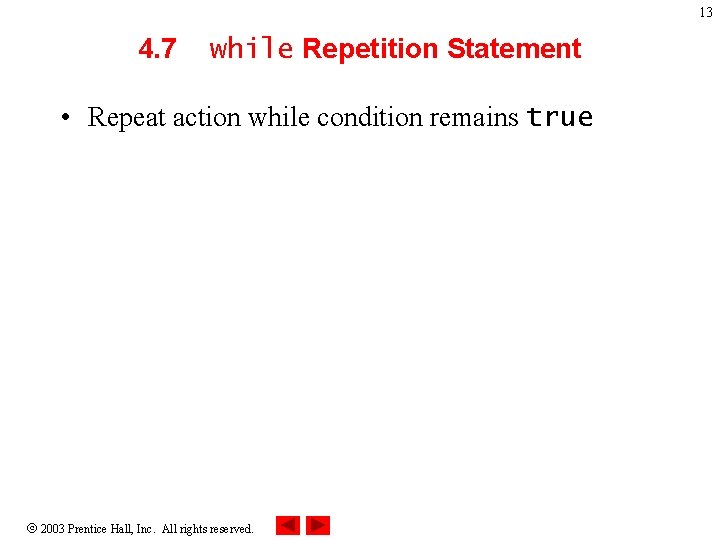
![14 merge decision [product <= 1000] double product value [product > 1000] Corresponding Java 14 merge decision [product <= 1000] double product value [product > 1000] Corresponding Java](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-14.jpg)
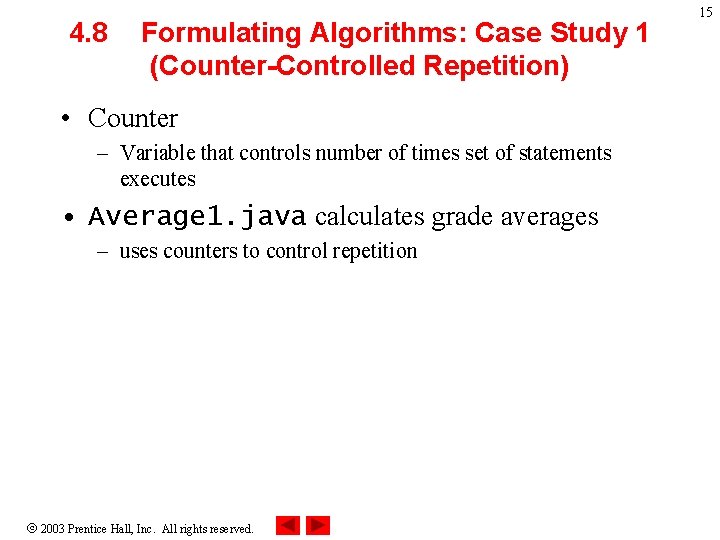
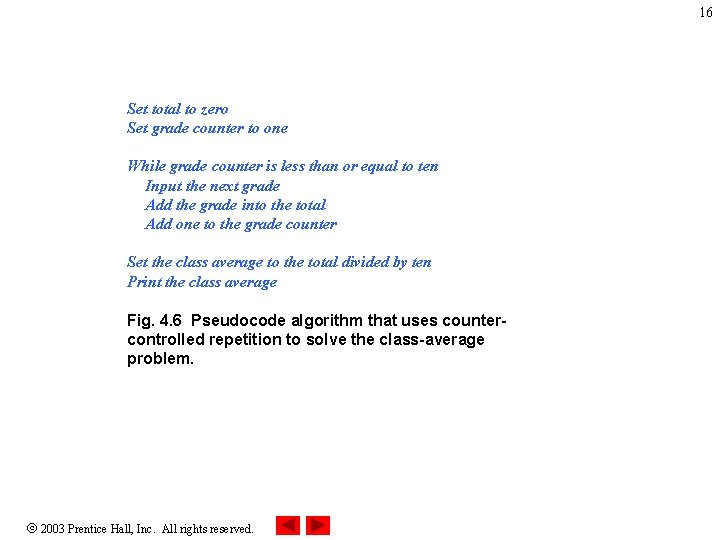
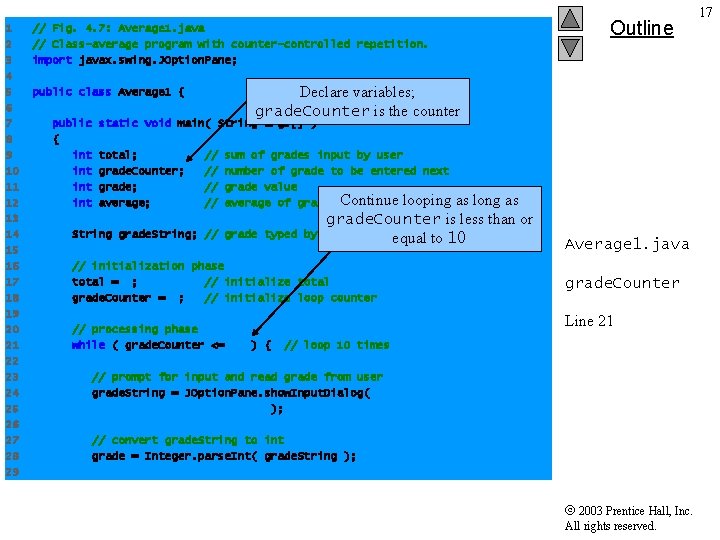
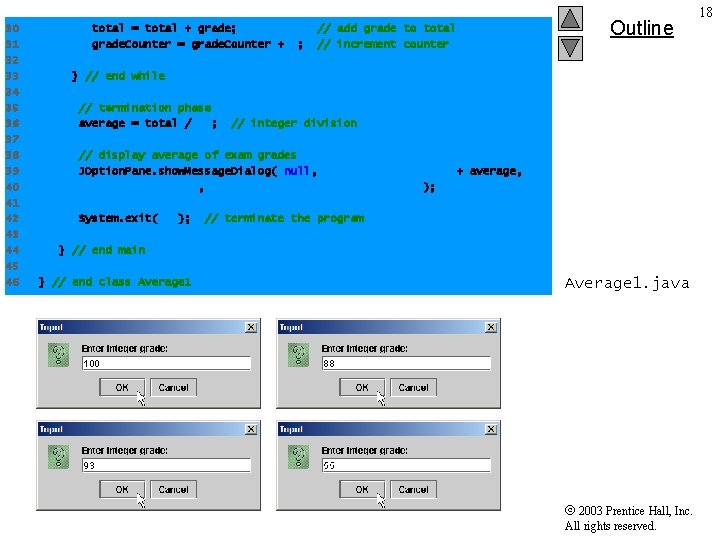
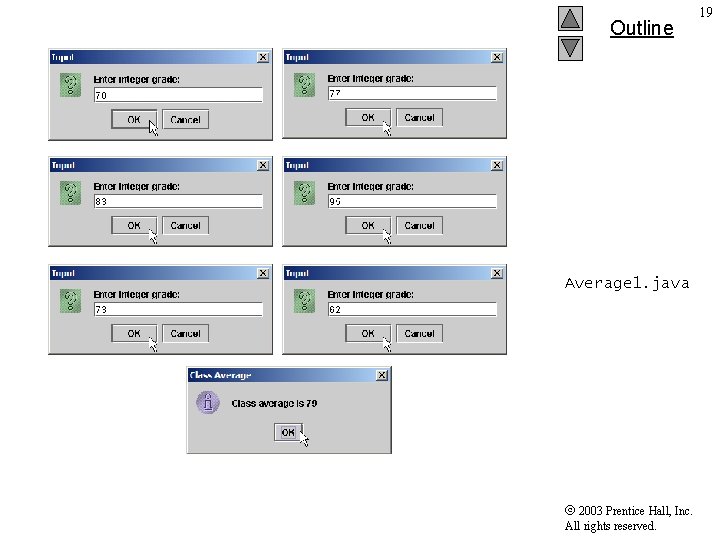
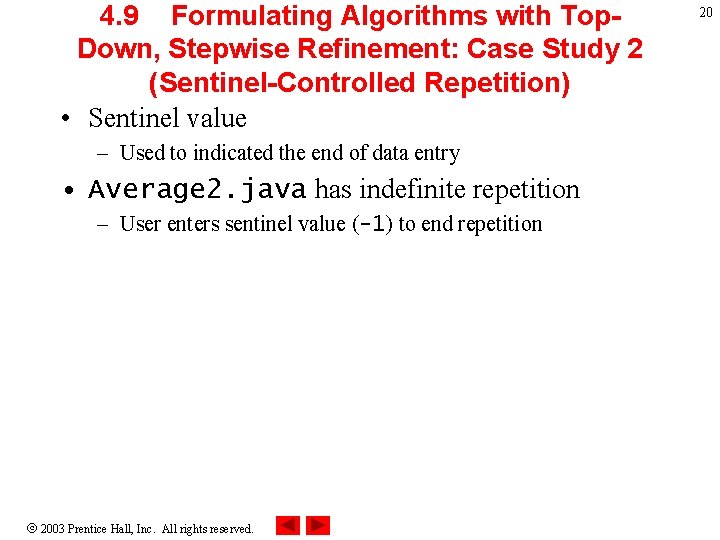
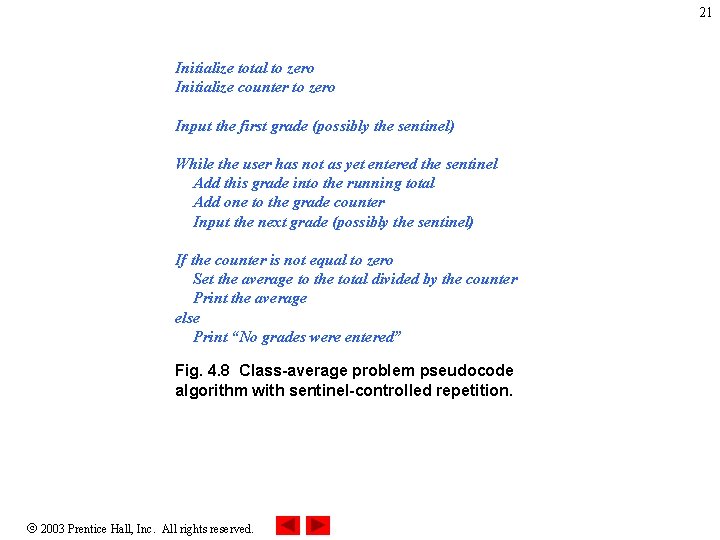
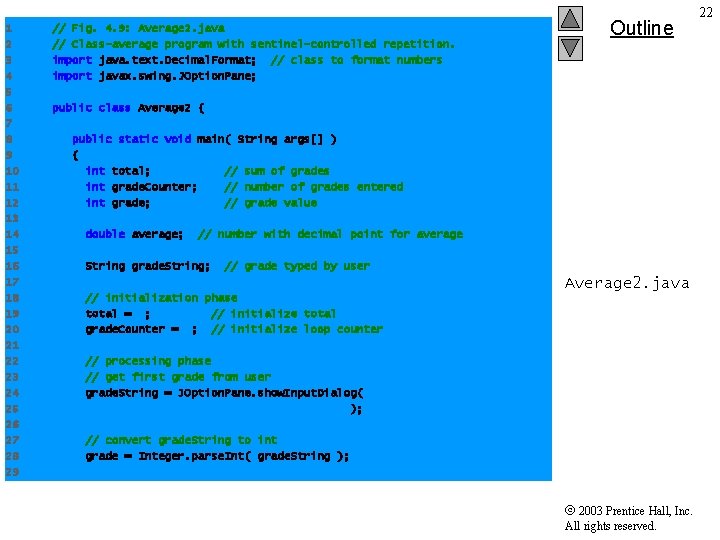
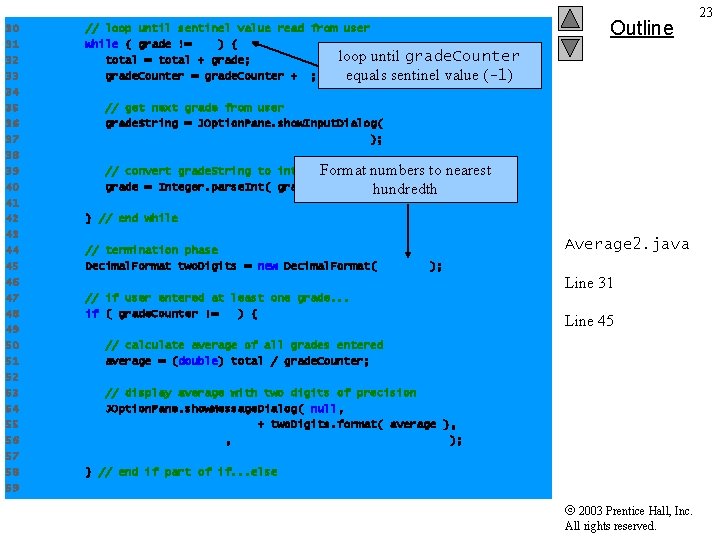
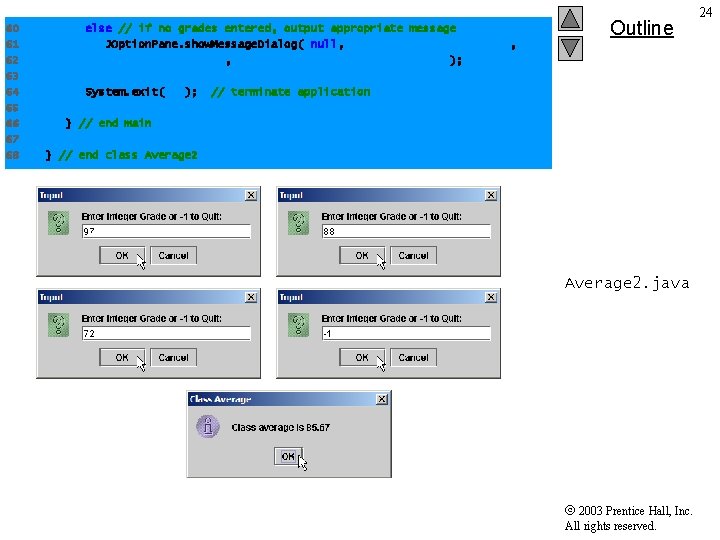
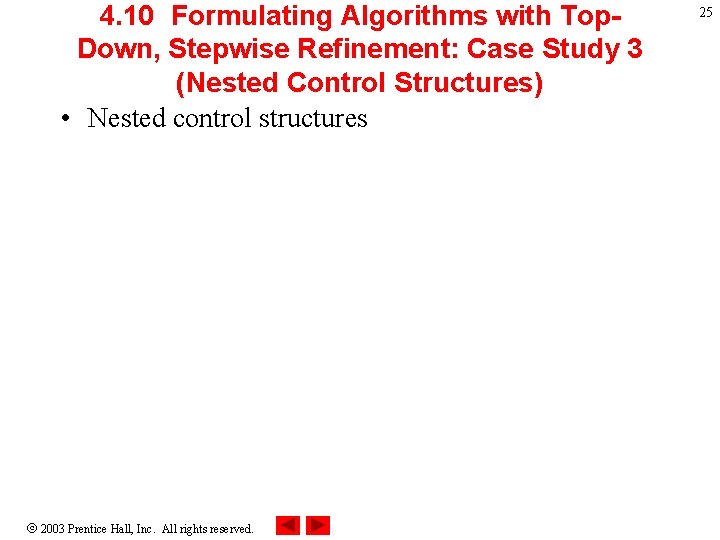
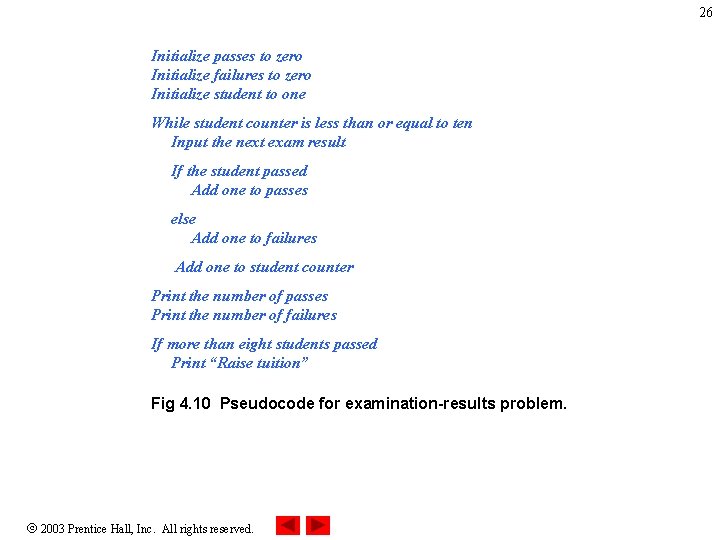
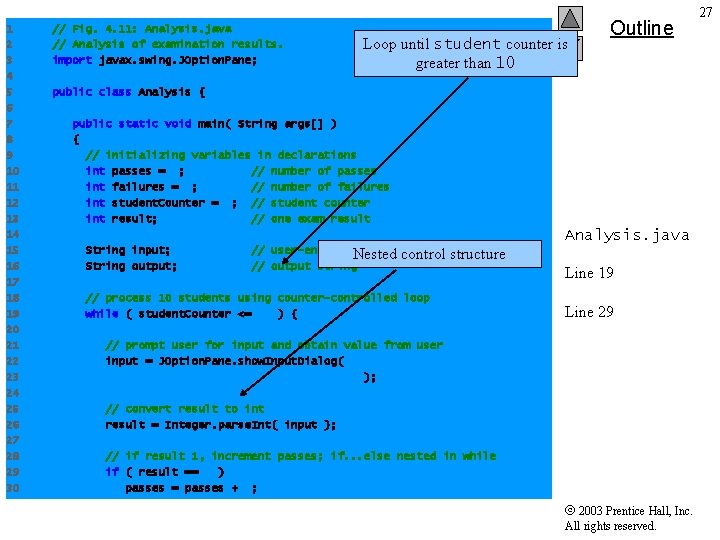
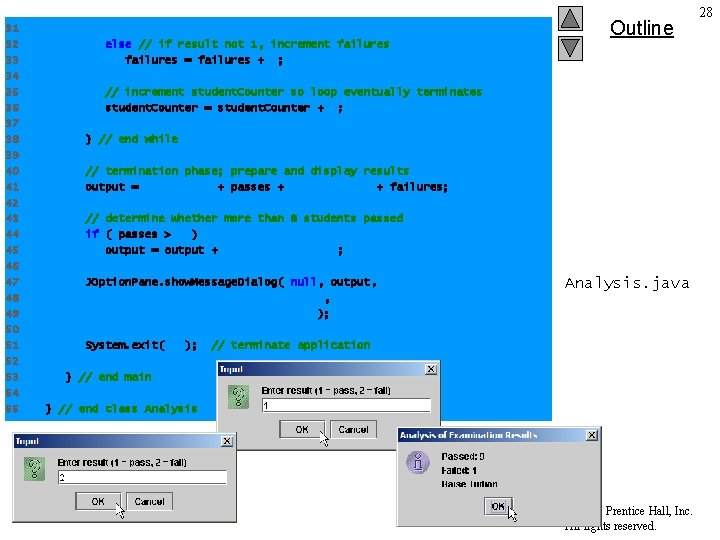
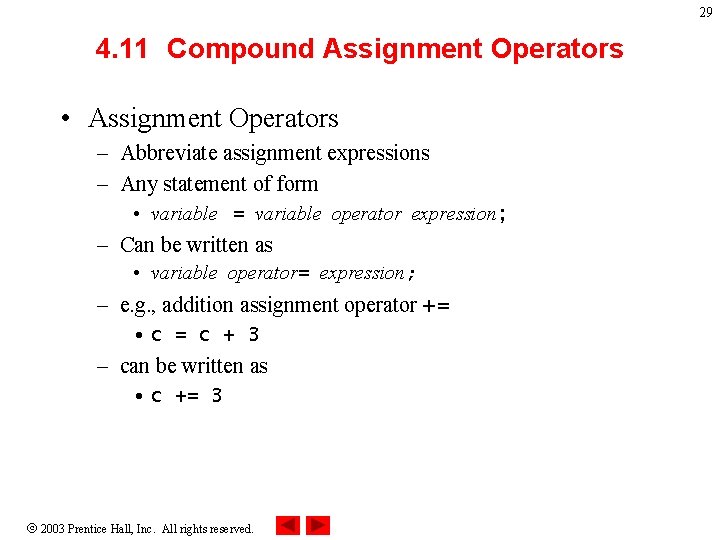
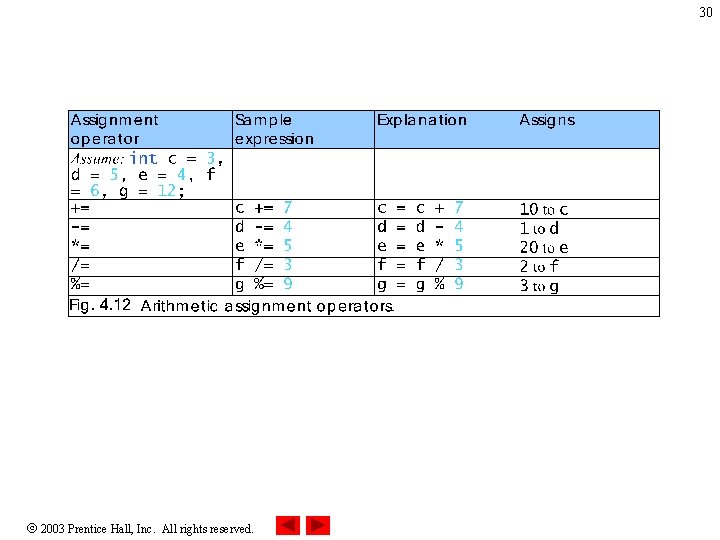
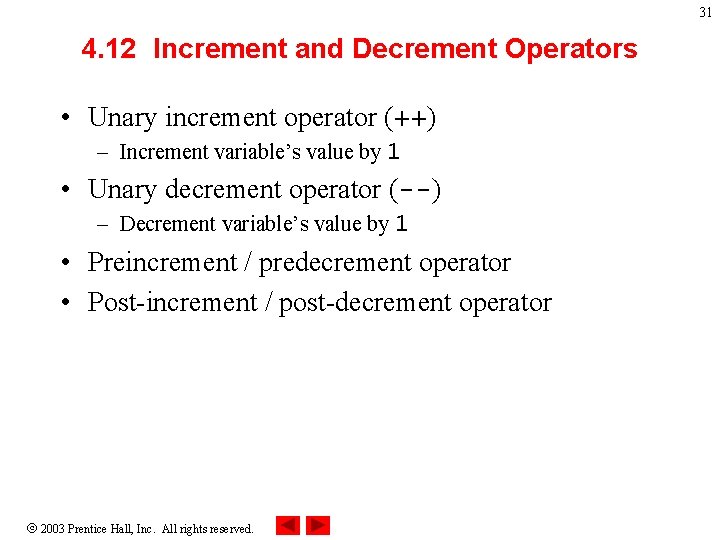
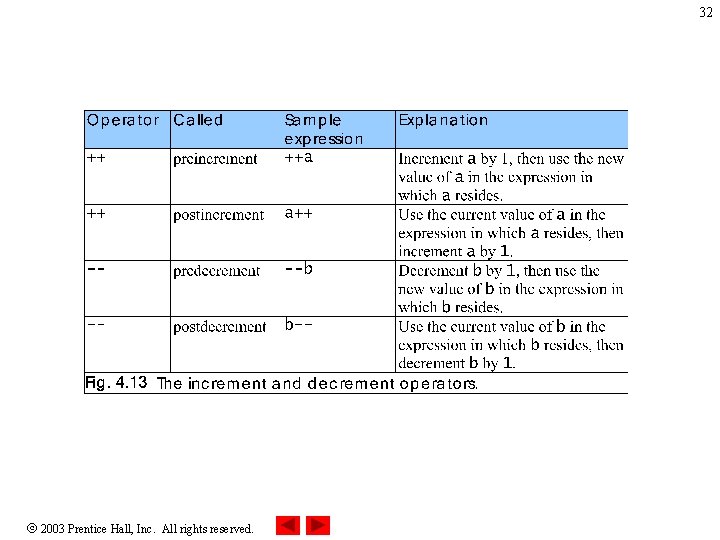
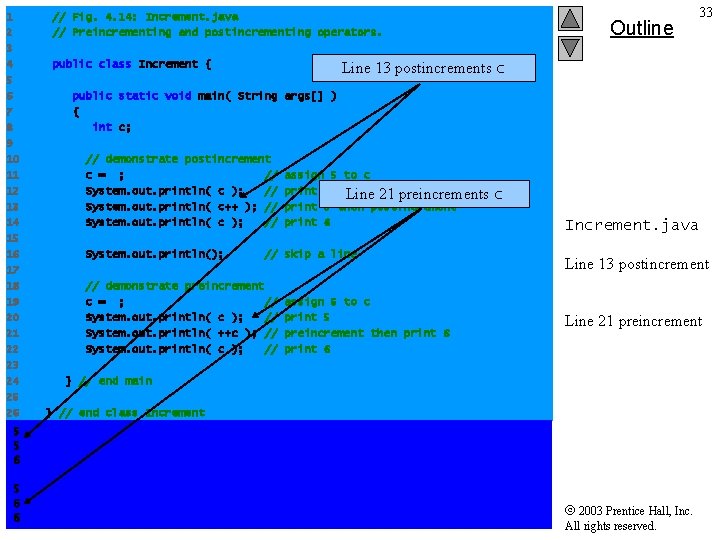
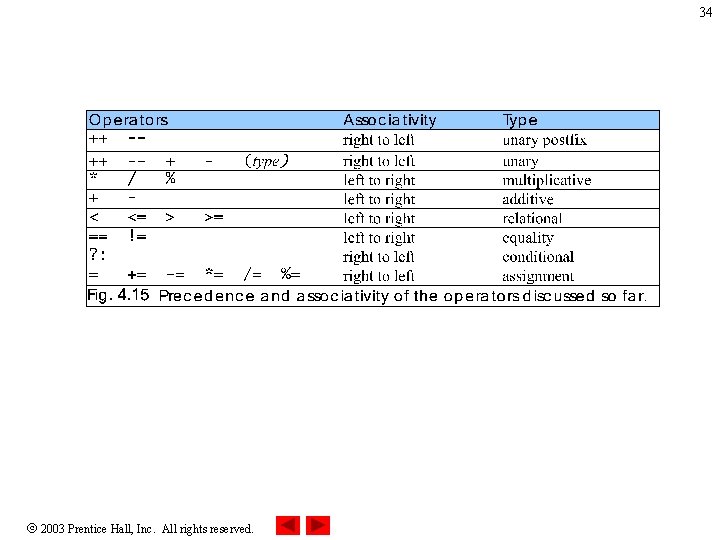
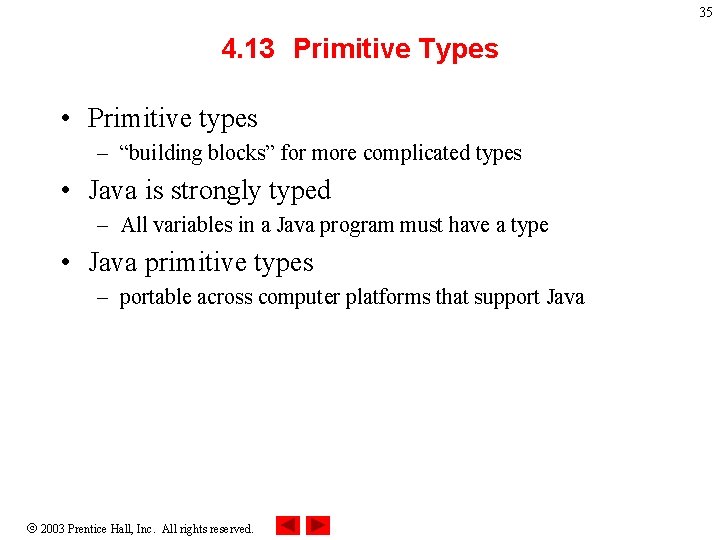
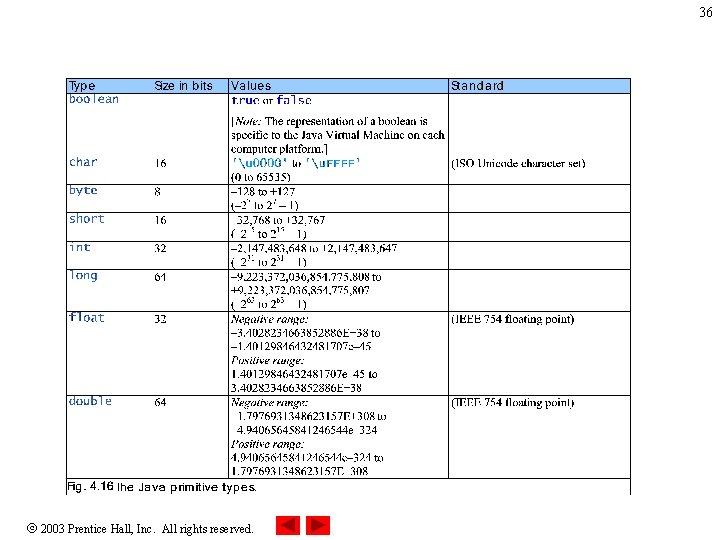
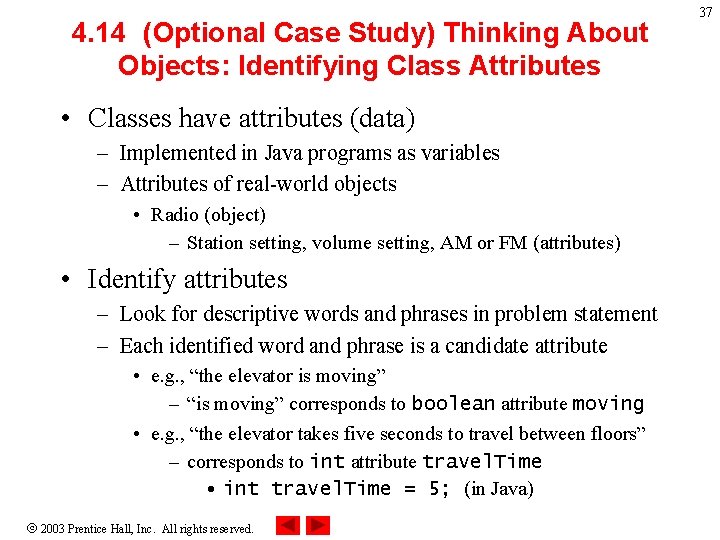
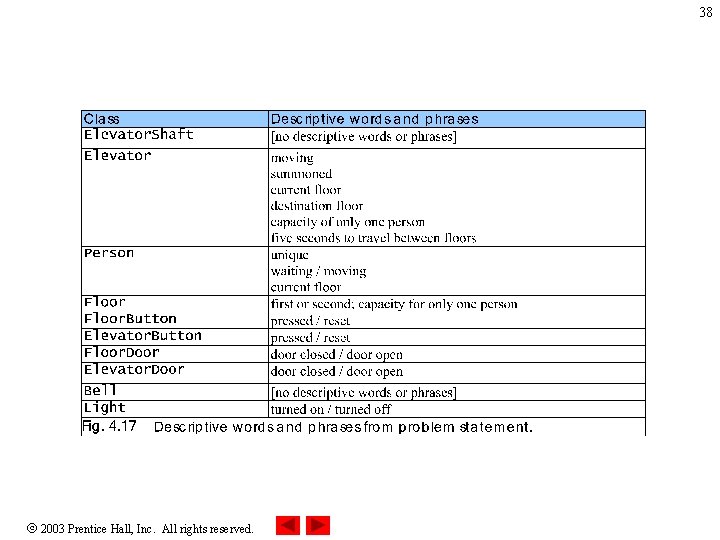
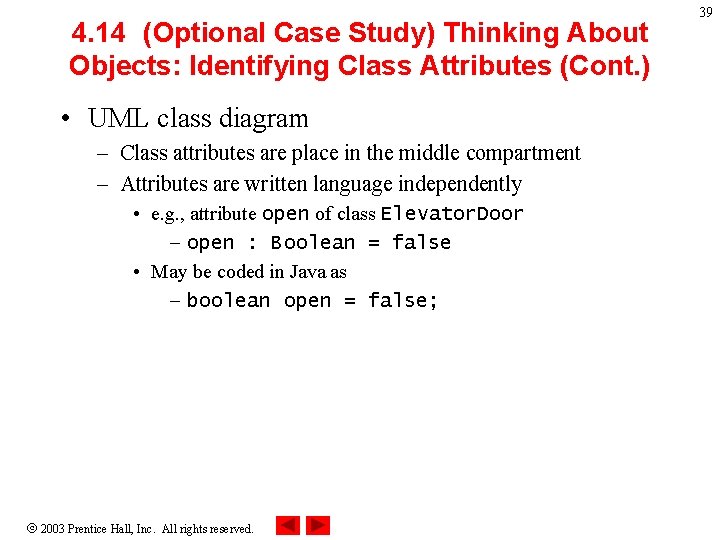
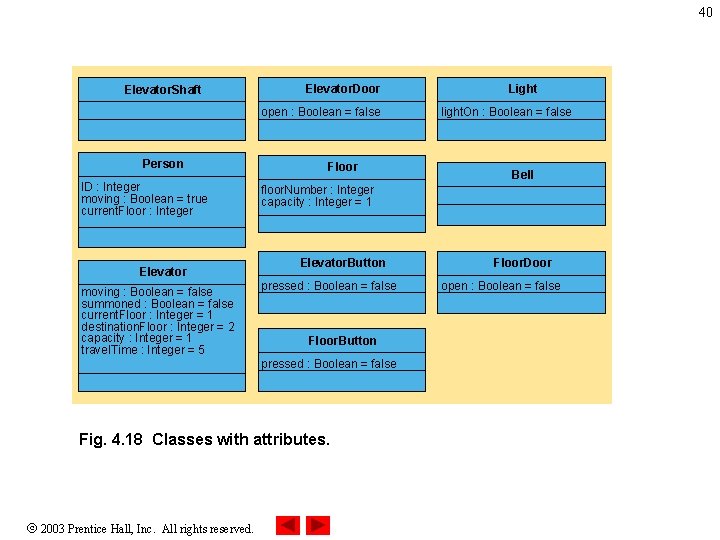
- Slides: 40
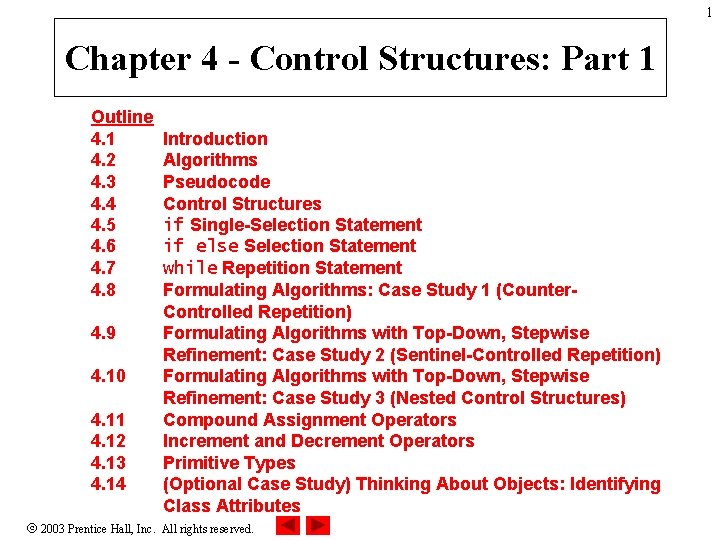
1 Chapter 4 - Control Structures: Part 1 Outline 4. 1 4. 2 4. 3 4. 4 4. 5 4. 6 4. 7 4. 8 4. 9 4. 10 4. 11 4. 12 4. 13 4. 14 Introduction Algorithms Pseudocode Control Structures if Single-Selection Statement if else Selection Statement while Repetition Statement Formulating Algorithms: Case Study 1 (Counter. Controlled Repetition) Formulating Algorithms with Top-Down, Stepwise Refinement: Case Study 2 (Sentinel-Controlled Repetition) Formulating Algorithms with Top-Down, Stepwise Refinement: Case Study 3 (Nested Control Structures) Compound Assignment Operators Increment and Decrement Operators Primitive Types (Optional Case Study) Thinking About Objects: Identifying Class Attributes 2003 Prentice Hall, Inc. All rights reserved.
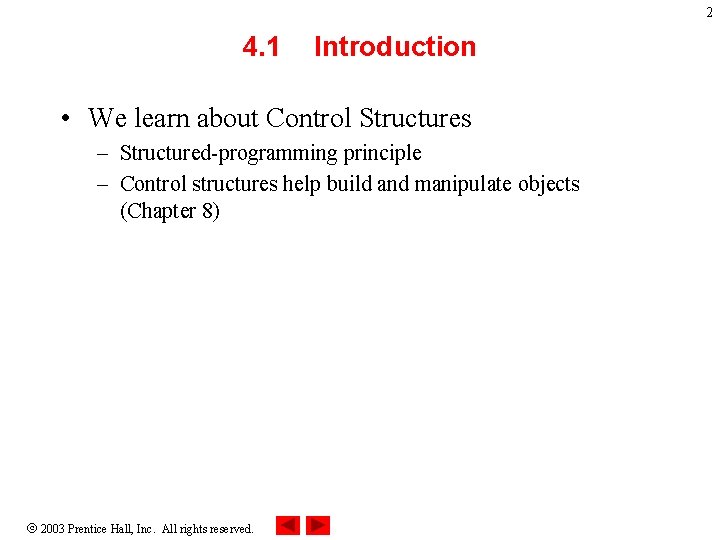
2 4. 1 Introduction • We learn about Control Structures – Structured-programming principle – Control structures help build and manipulate objects (Chapter 8) 2003 Prentice Hall, Inc. All rights reserved.
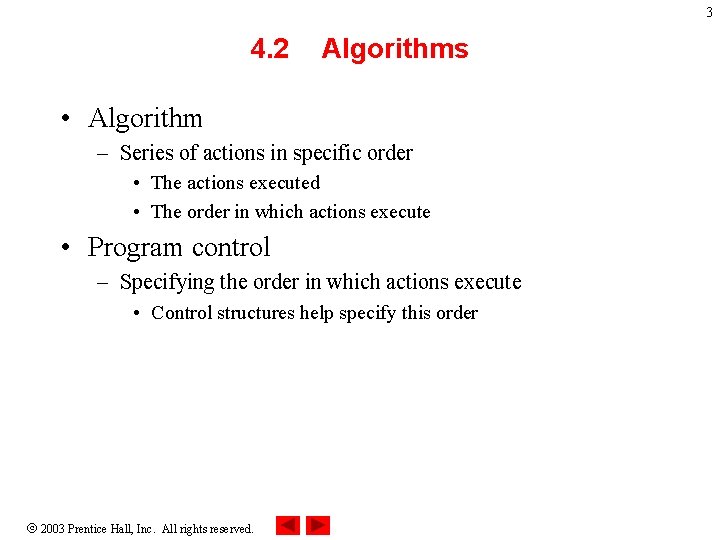
3 4. 2 Algorithms • Algorithm – Series of actions in specific order • The actions executed • The order in which actions execute • Program control – Specifying the order in which actions execute • Control structures help specify this order 2003 Prentice Hall, Inc. All rights reserved.
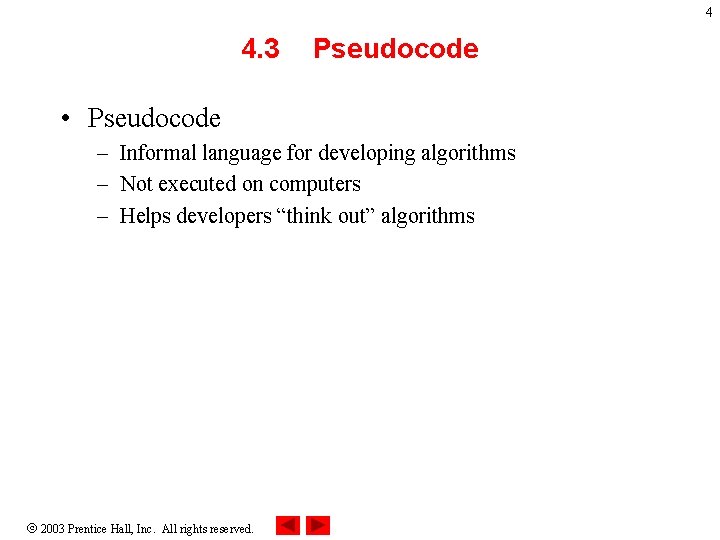
4 4. 3 Pseudocode • Pseudocode – Informal language for developing algorithms – Not executed on computers – Helps developers “think out” algorithms 2003 Prentice Hall, Inc. All rights reserved.
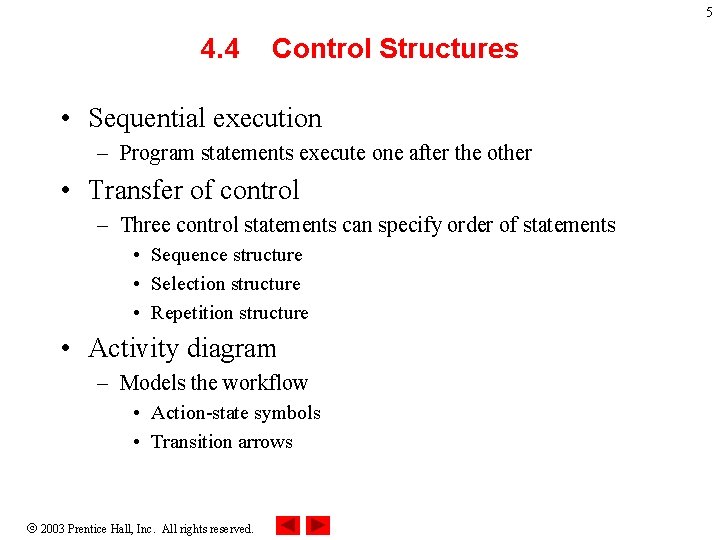
5 4. 4 Control Structures • Sequential execution – Program statements execute one after the other • Transfer of control – Three control statements can specify order of statements • Sequence structure • Selection structure • Repetition structure • Activity diagram – Models the workflow • Action-state symbols • Transition arrows 2003 Prentice Hall, Inc. All rights reserved.
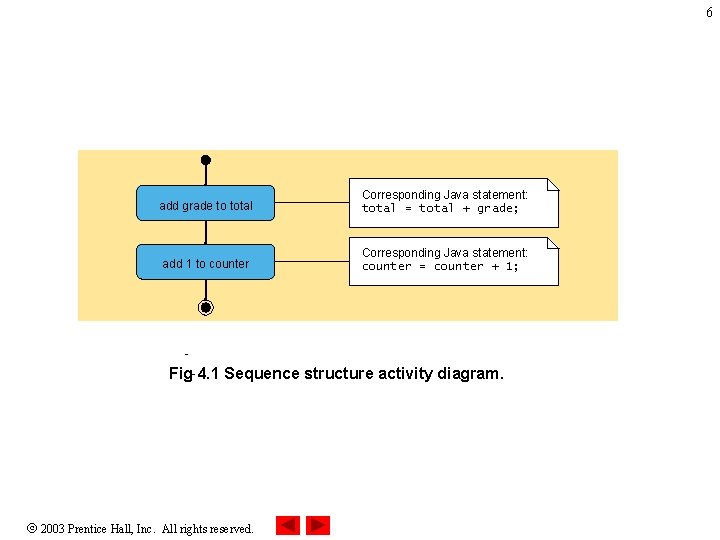
6 add grade to total Corresponding Java statement: total = total + grade; add 1 to counter Corresponding Java statement: counter = counter + 1; Fig 4. 1 Sequence structure activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
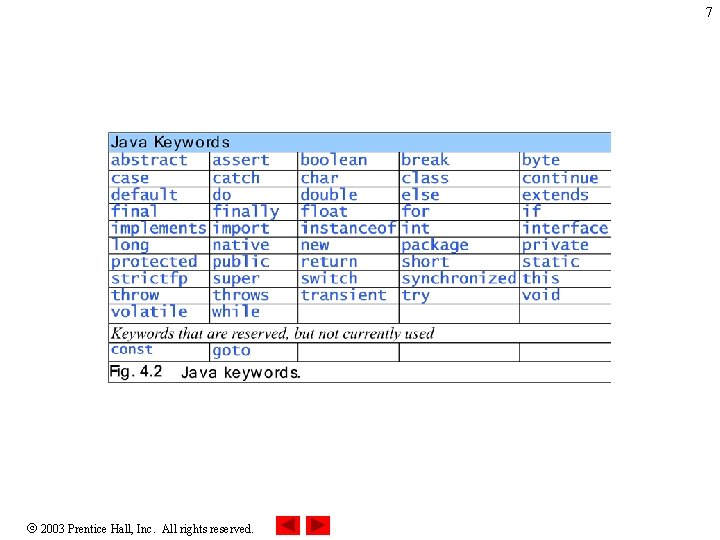
7 2003 Prentice Hall, Inc. All rights reserved.
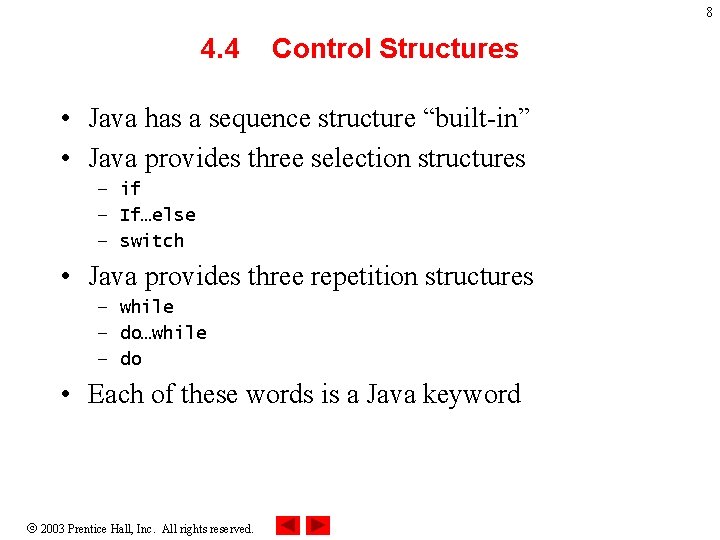
8 4. 4 Control Structures • Java has a sequence structure “built-in” • Java provides three selection structures – if – If…else – switch • Java provides three repetition structures – while – do…while – do • Each of these words is a Java keyword 2003 Prentice Hall, Inc. All rights reserved.
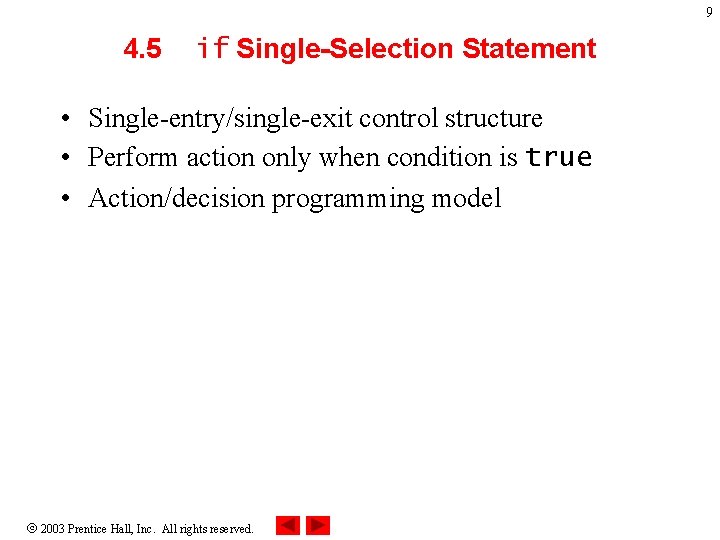
9 4. 5 if Single-Selection Statement • Single-entry/single-exit control structure • Perform action only when condition is true • Action/decision programming model 2003 Prentice Hall, Inc. All rights reserved.
![10 grade 60 print Passed grade 60 Fig 4 3 if singleselections 10 [grade >= 60] print “Passed” [grade < 60] Fig 4. 3 if single-selections](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-10.jpg)
10 [grade >= 60] print “Passed” [grade < 60] Fig 4. 3 if single-selections statement activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
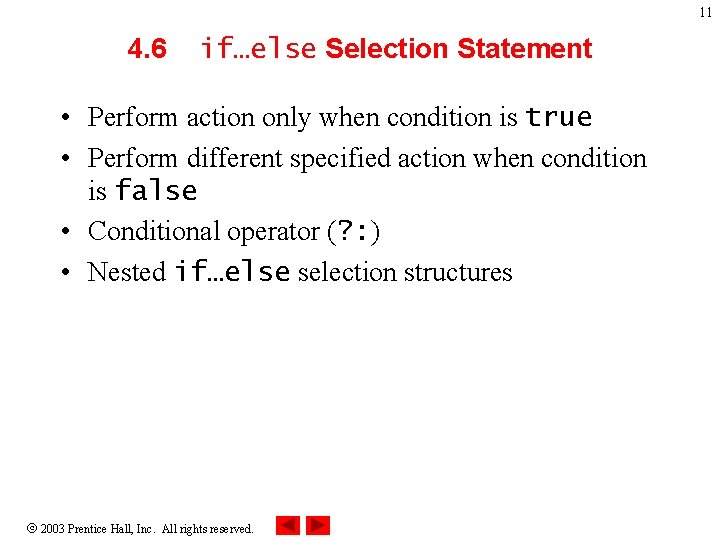
11 4. 6 if…else Selection Statement • Perform action only when condition is true • Perform different specified action when condition is false • Conditional operator (? : ) • Nested if…else selection structures 2003 Prentice Hall, Inc. All rights reserved.
![12 grade 60 print Failed grade 60 print Passed Fig 4 4 12 [grade < 60] print “Failed” [grade >= 60] print “Passed” Fig 4. 4](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-12.jpg)
12 [grade < 60] print “Failed” [grade >= 60] print “Passed” Fig 4. 4 if…else double-selections statement activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
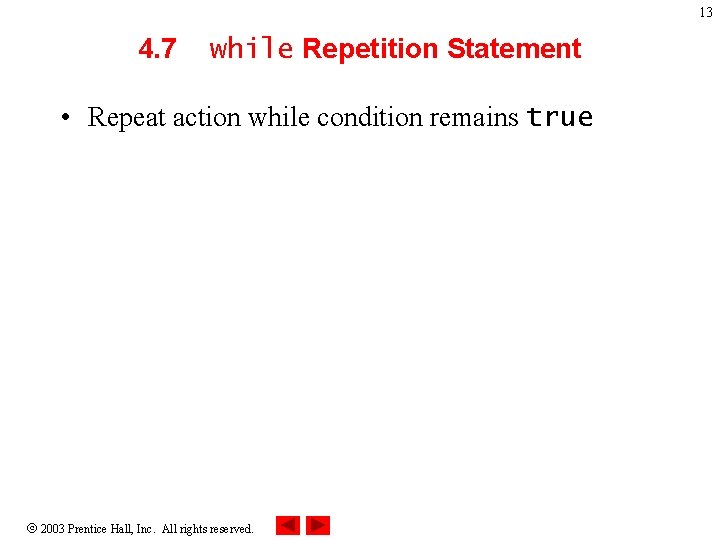
13 4. 7 while Repetition Statement • Repeat action while condition remains true 2003 Prentice Hall, Inc. All rights reserved.
![14 merge decision product 1000 double product value product 1000 Corresponding Java 14 merge decision [product <= 1000] double product value [product > 1000] Corresponding Java](https://slidetodoc.com/presentation_image_h2/66effcdfa78b3c8bb88ebad9ccff89b1/image-14.jpg)
14 merge decision [product <= 1000] double product value [product > 1000] Corresponding Java statement: product = 2 * product; Fig 4. 5 while repetition statement activity diagram. 2003 Prentice Hall, Inc. All rights reserved.
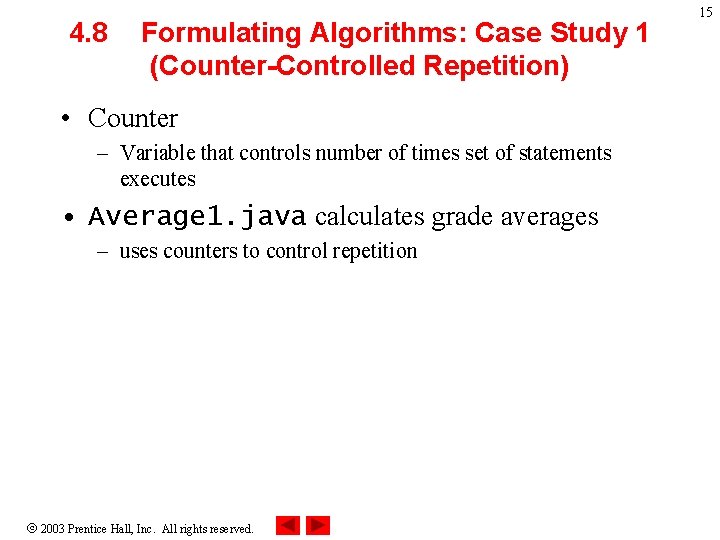
4. 8 Formulating Algorithms: Case Study 1 (Counter-Controlled Repetition) • Counter – Variable that controls number of times set of statements executes • Average 1. java calculates grade averages – uses counters to control repetition 2003 Prentice Hall, Inc. All rights reserved. 15
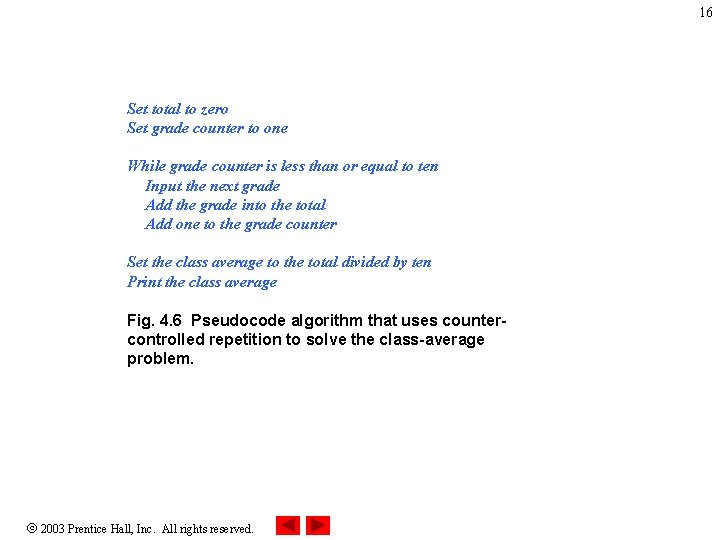
16 Set total to zero Set grade counter to one While grade counter is less than or equal to ten Input the next grade Add the grade into the total Add one to the grade counter Set the class average to the total divided by ten Print the class average Fig. 4. 6 Pseudocode algorithm that uses countercontrolled repetition to solve the class-average problem. 2003 Prentice Hall, Inc. All rights reserved.
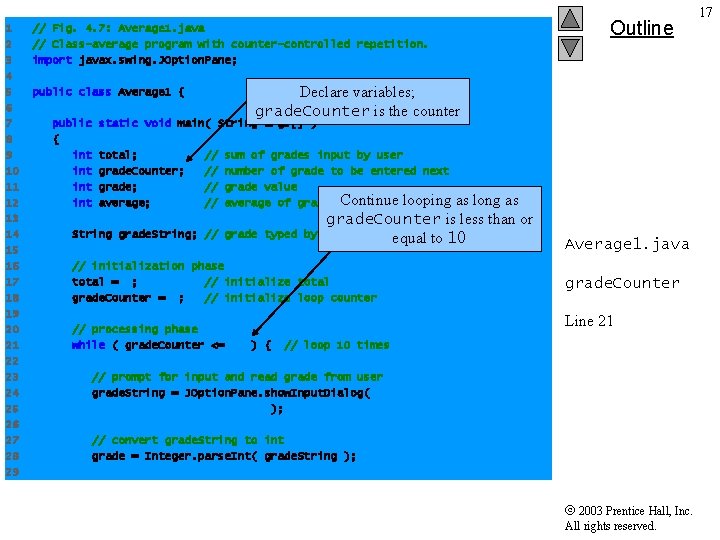
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 // Fig. 4. 7: Average 1. java // Class-average program with counter-controlled repetition. import javax. swing. JOption. Pane; Declare variables; grade. Counter is the counter public class Average 1 { public { int int Outline static void main( String args[] ) total; grade. Counter; grade; average; // // sum of grades input by user number of grade to be entered next grade value average of grades Continue looping String grade. String; // grade typed by as long as grade. Counter is less than or user equal to 10 // initialization phase total = 0; // initialize total grade. Counter = 1; // initialize loop counter // processing phase while ( grade. Counter <= 10 ) { Average 1. java grade. Counter Line 21 // loop 10 times // prompt for input and read grade from user grade. String = JOption. Pane. show. Input. Dialog( "Enter integer grade: " ); // convert grade. String to int grade = Integer. parse. Int( grade. String ); 2003 Prentice Hall, Inc. All rights reserved. 17
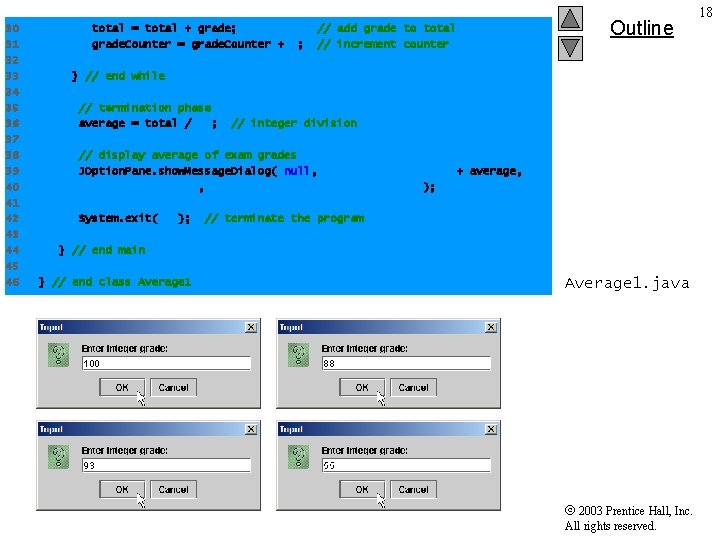
30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 total = total + grade; grade. Counter = grade. Counter + 1; // add grade to total // increment counter Outline } // end while // termination phase average = total / 10; // integer division // display average of exam grades JOption. Pane. show. Message. Dialog( null, "Class average is " + average, "Class Average", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); // terminate the program } // end main } // end class Average 1. java 2003 Prentice Hall, Inc. All rights reserved. 18
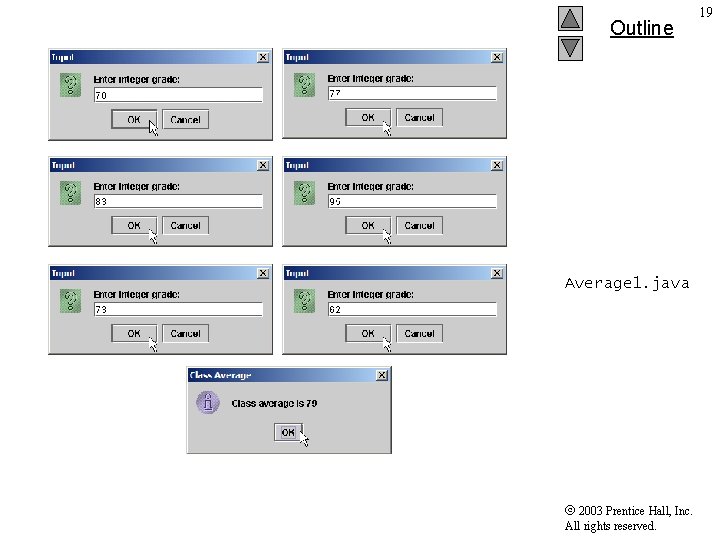
Outline Average 1. java 2003 Prentice Hall, Inc. All rights reserved. 19
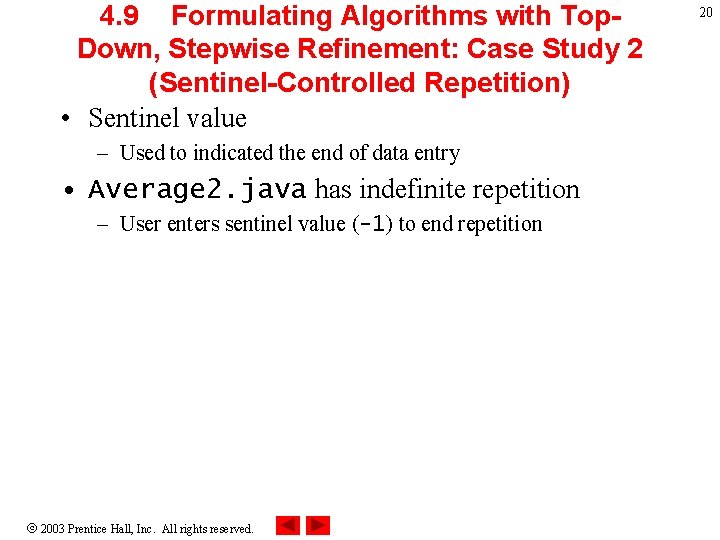
4. 9 Formulating Algorithms with Top. Down, Stepwise Refinement: Case Study 2 (Sentinel-Controlled Repetition) • Sentinel value – Used to indicated the end of data entry • Average 2. java has indefinite repetition – User enters sentinel value (-1) to end repetition 2003 Prentice Hall, Inc. All rights reserved. 20
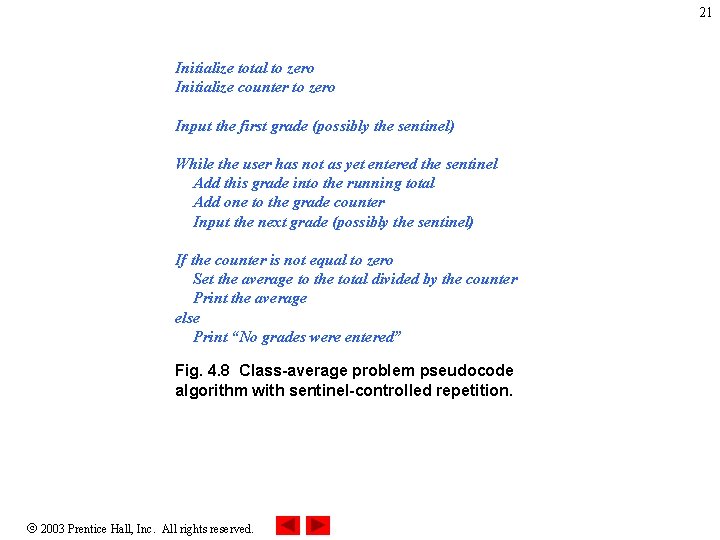
21 Initialize total to zero Initialize counter to zero Input the first grade (possibly the sentinel) While the user has not as yet entered the sentinel Add this grade into the running total Add one to the grade counter Input the next grade (possibly the sentinel) If the counter is not equal to zero Set the average to the total divided by the counter Print the average else Print “No grades were entered” Fig. 4. 8 Class-average problem pseudocode algorithm with sentinel-controlled repetition. 2003 Prentice Hall, Inc. All rights reserved.
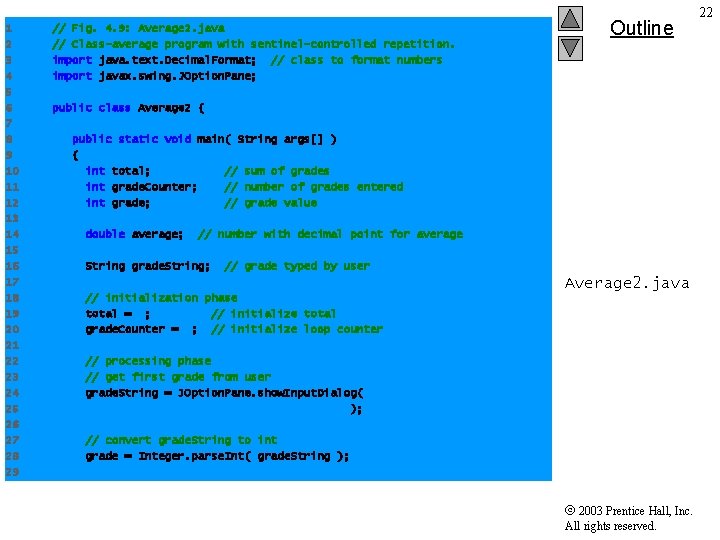
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 // Fig. 4. 9: Average 2. java // Class-average program with sentinel-controlled repetition. import java. text. Decimal. Format; // class to format numbers import javax. swing. JOption. Pane; Outline public class Average 2 { public static void main( String args[] ) { int total; // sum of grades int grade. Counter; // number of grades entered int grade; // grade value double average; // number with decimal point for average String grade. String; // grade typed by user Average 2. java // initialization phase total = 0; // initialize total grade. Counter = 0; // initialize loop counter // processing phase // get first grade from user grade. String = JOption. Pane. show. Input. Dialog( "Enter Integer Grade or -1 to Quit: " ); // convert grade. String to int grade = Integer. parse. Int( grade. String ); 2003 Prentice Hall, Inc. All rights reserved. 22
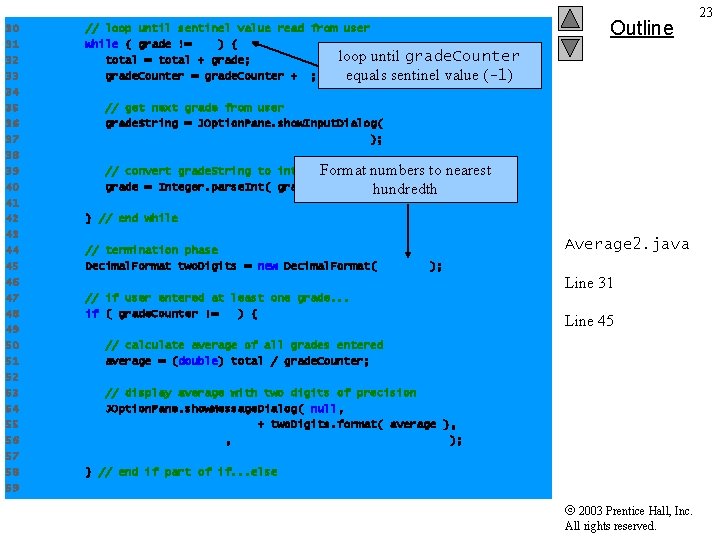
30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 // loop until sentinel value read from user while ( grade != -1 ) { total = total + grade; //loop add until grade. Counter to total grade. Counter = grade. Counter + 1; // equals increment counter sentinel value (-1) Outline // get next grade from user grade. String = JOption. Pane. show. Input. Dialog( "Enter Integer Grade or -1 to Quit: " ); // convert grade. String to int Format numbers to grade = Integer. parse. Int( grade. String ); hundredth nearest } // end while // termination phase Decimal. Format two. Digits = new Decimal. Format( "0. 00" ); Average 2. java Line 31 // if user entered at least one grade. . . if ( grade. Counter != 0 ) { Line 45 // calculate average of all grades entered average = (double) total / grade. Counter; // display average with two digits of precision JOption. Pane. show. Message. Dialog( null, "Class average is " + two. Digits. format( average ), "Class Average", JOption. Pane. INFORMATION_MESSAGE ); } // end if part of if. . . else 2003 Prentice Hall, Inc. All rights reserved. 23
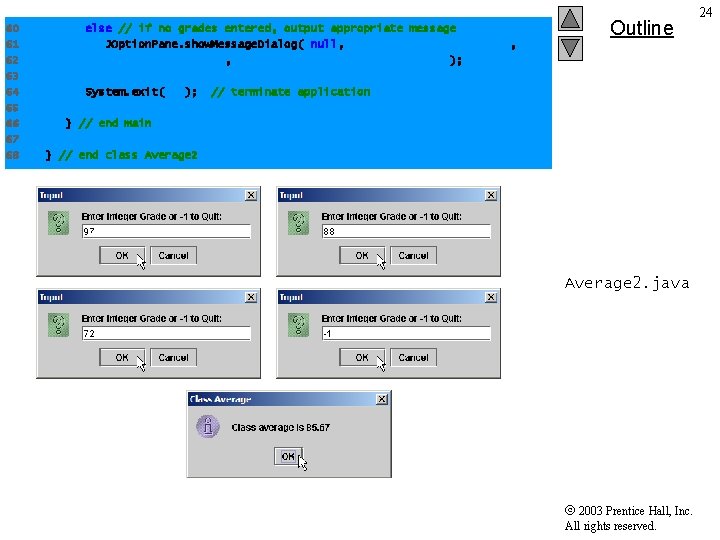
60 61 62 63 64 65 66 67 68 else // if no grades entered, output appropriate message JOption. Pane. show. Message. Dialog( null, "No grades were entered", "Class Average", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); Outline // terminate application } // end main } // end class Average 2. java 2003 Prentice Hall, Inc. All rights reserved. 24
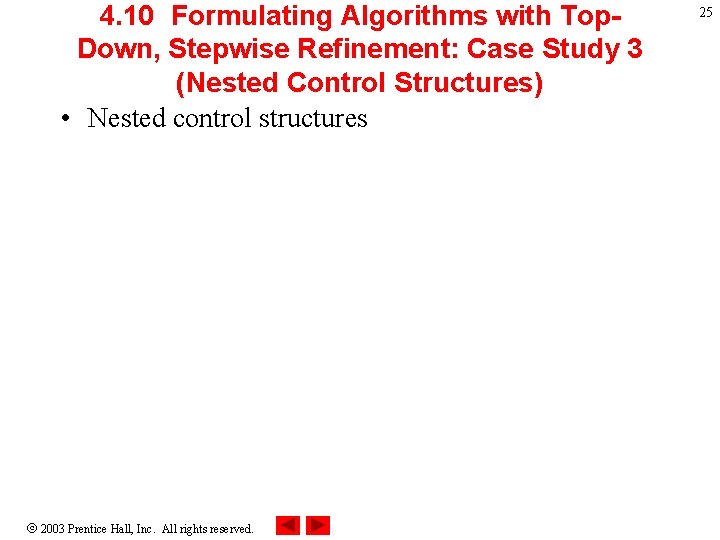
4. 10 Formulating Algorithms with Top. Down, Stepwise Refinement: Case Study 3 (Nested Control Structures) • Nested control structures 2003 Prentice Hall, Inc. All rights reserved. 25
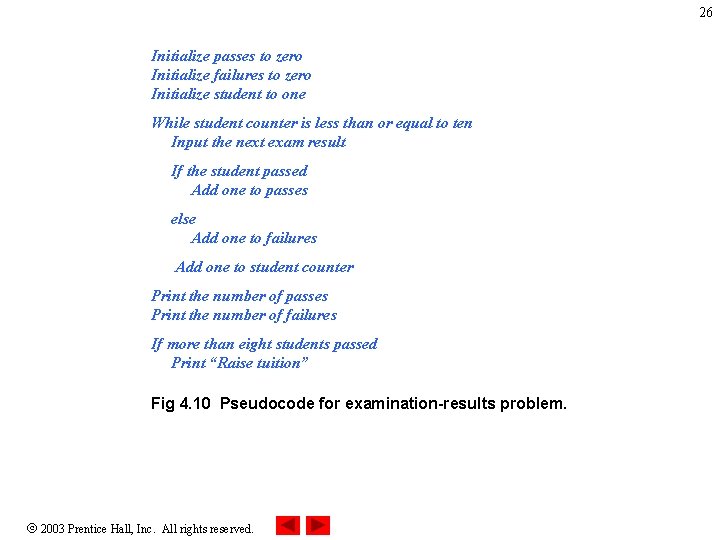
26 Initialize passes to zero Initialize failures to zero Initialize student to one While student counter is less than or equal to ten Input the next exam result If the student passed Add one to passes else Add one to failures Add one to student counter Print the number of passes Print the number of failures If more than eight students passed Print “Raise tuition” Fig 4. 10 Pseudocode for examination-results problem. 2003 Prentice Hall, Inc. All rights reserved.
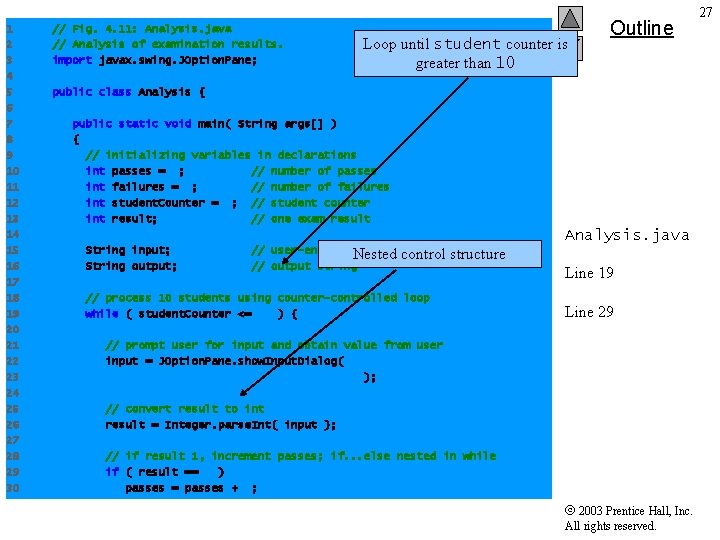
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 // Fig. 4. 11: Analysis. java // Analysis of examination results. import javax. swing. JOption. Pane; Loop until student counter is greater than 10 Outline public class Analysis { public static void main( String args[] ) { // initializing variables in declarations int passes = 0; // number of passes int failures = 0; // number of failures int student. Counter = 1; // student counter int result; // one exam result Analysis. java String input; String output; // user-entered Nested value // output string control structure // process 10 students using counter-controlled loop while ( student. Counter <= 10 ) { Line 19 Line 29 // prompt user for input and obtain value from user input = JOption. Pane. show. Input. Dialog( "Enter result (1 = pass, 2 = fail)" ); // convert result to int result = Integer. parse. Int( input ); // if result 1, increment passes; if. . . else nested in while if ( result == 1 ) passes = passes + 1; 2003 Prentice Hall, Inc. All rights reserved. 27
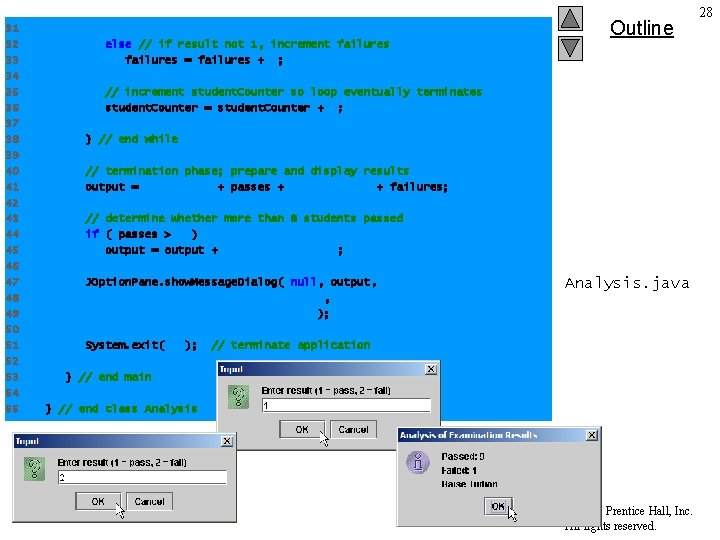
31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 else // if result not 1, increment failures = failures + 1; Outline // increment student. Counter so loop eventually terminates student. Counter = student. Counter + 1; } // end while // termination phase; prepare and display results output = "Passed: " + passes + "n. Failed: " + failures; // determine whether more than 8 students passed if ( passes > 8 ) output = output + "n. Raise Tuition"; JOption. Pane. show. Message. Dialog( null, output, "Analysis of Examination Results", JOption. Pane. INFORMATION_MESSAGE ); System. exit( 0 ); Analysis. java // terminate application } // end main } // end class Analysis 2003 Prentice Hall, Inc. All rights reserved. 28
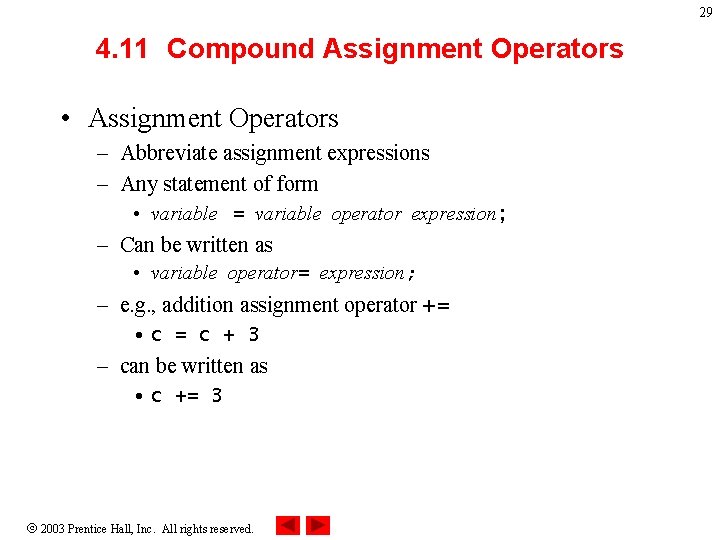
29 4. 11 Compound Assignment Operators • Assignment Operators – Abbreviate assignment expressions – Any statement of form • variable = variable operator expression; – Can be written as • variable operator= expression; – e. g. , addition assignment operator += • c = c + 3 – can be written as • c += 3 2003 Prentice Hall, Inc. All rights reserved.
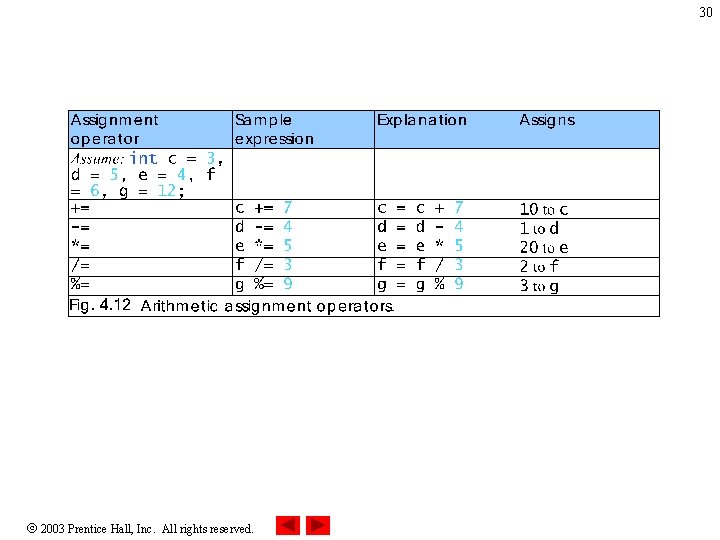
30 2003 Prentice Hall, Inc. All rights reserved.
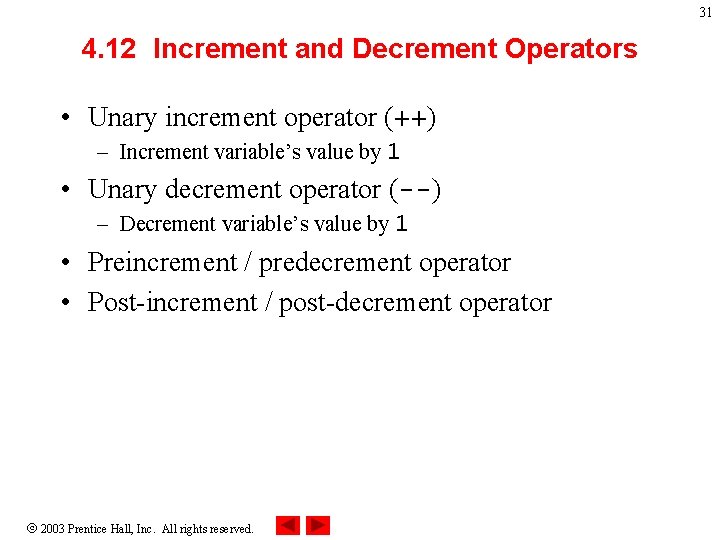
31 4. 12 Increment and Decrement Operators • Unary increment operator (++) – Increment variable’s value by 1 • Unary decrement operator (--) – Decrement variable’s value by 1 • Preincrement / predecrement operator • Post-increment / post-decrement operator 2003 Prentice Hall, Inc. All rights reserved.
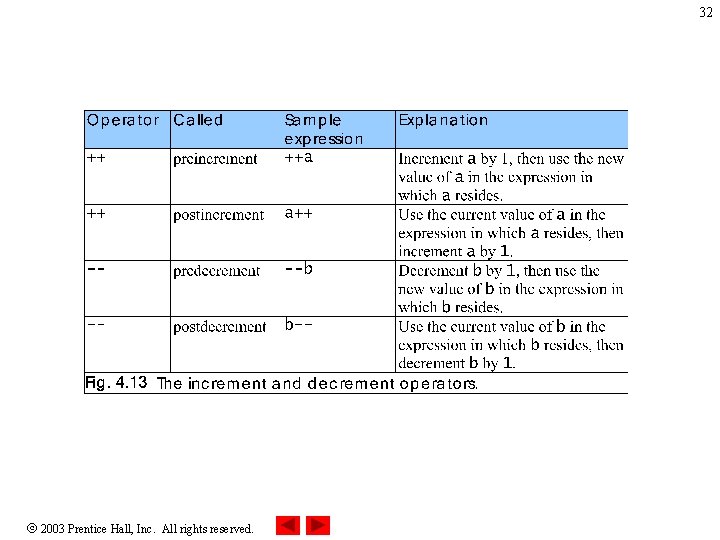
32 2003 Prentice Hall, Inc. All rights reserved.
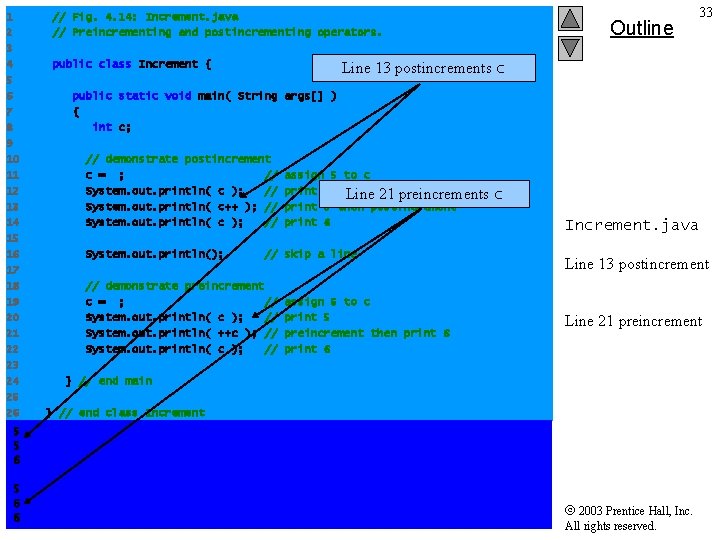
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // Fig. 4. 14: Increment. java // Preincrementing and postincrementing operators. Outline Line 13 postincrements c public class Increment { public static void main( String args[] ) { int c; // demonstrate postincrement c = 5; // System. out. println( c ); // System. out. println( c++ ); // System. out. println( c ); // System. out. println(); assign 5 to c print 5 Line 21 preincrements print 5 then postincrement print 6 // skip a line // demonstrate preincrement c = 5; // System. out. println( c ); // System. out. println( ++c ); // System. out. println( c ); // assign 5 to c print 5 preincrement then print 6 c Increment. java Line 13 postincrement Line 21 preincrement } // end main } // end class Increment 5 5 6 6 33 2003 Prentice Hall, Inc. All rights reserved.
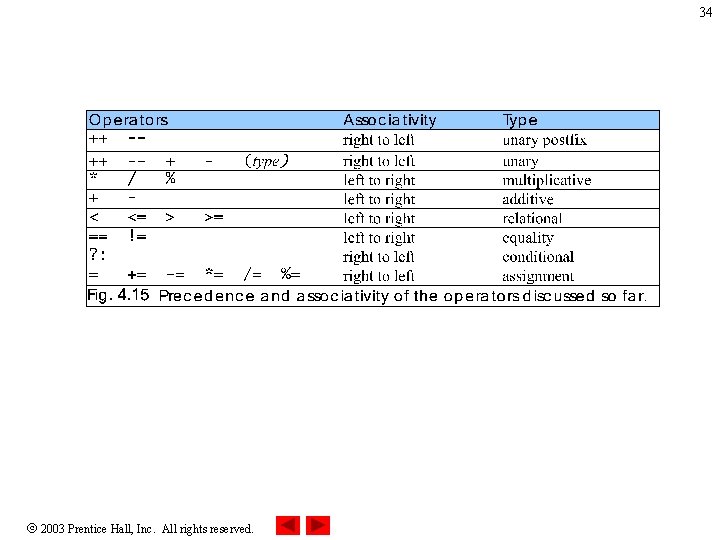
34 2003 Prentice Hall, Inc. All rights reserved.
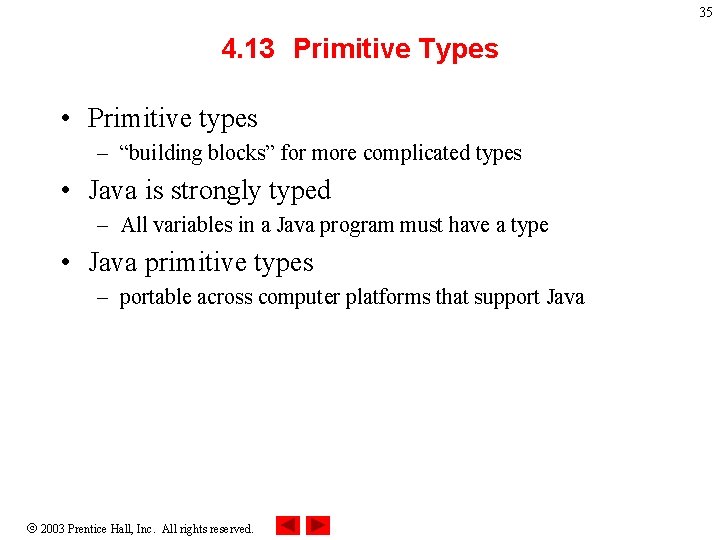
35 4. 13 Primitive Types • Primitive types – “building blocks” for more complicated types • Java is strongly typed – All variables in a Java program must have a type • Java primitive types – portable across computer platforms that support Java 2003 Prentice Hall, Inc. All rights reserved.
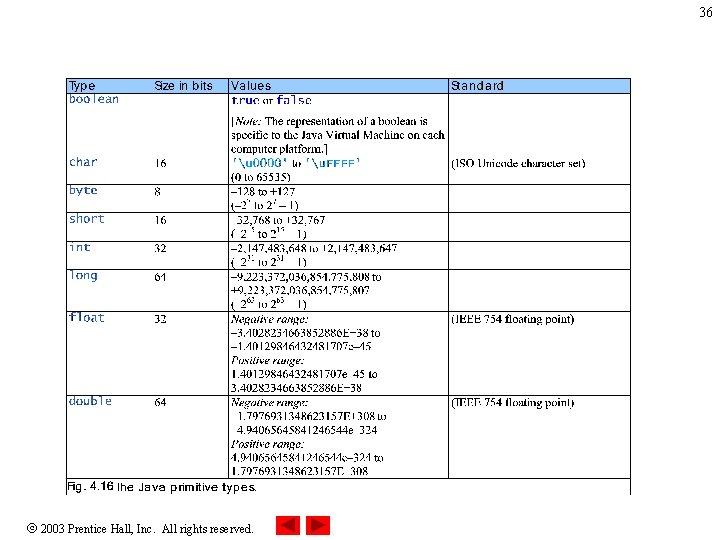
36 2003 Prentice Hall, Inc. All rights reserved.
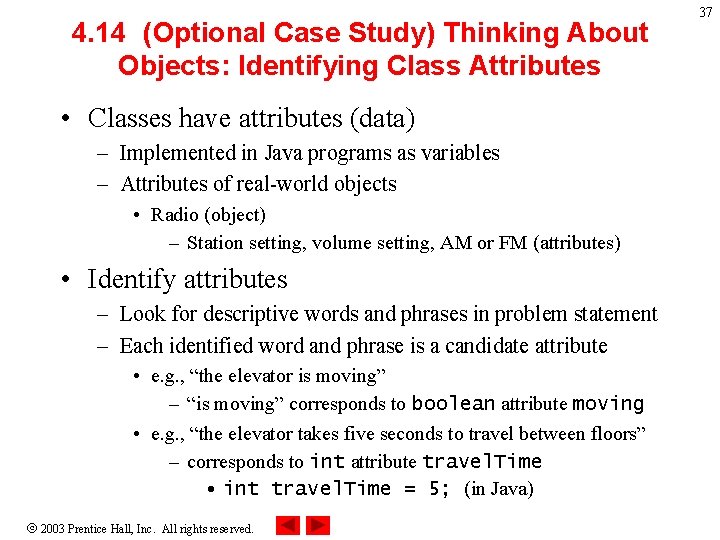
4. 14 (Optional Case Study) Thinking About Objects: Identifying Class Attributes • Classes have attributes (data) – Implemented in Java programs as variables – Attributes of real-world objects • Radio (object) – Station setting, volume setting, AM or FM (attributes) • Identify attributes – Look for descriptive words and phrases in problem statement – Each identified word and phrase is a candidate attribute • e. g. , “the elevator is moving” – “is moving” corresponds to boolean attribute moving • e. g. , “the elevator takes five seconds to travel between floors” – corresponds to int attribute travel. Time • int travel. Time = 5; (in Java) 2003 Prentice Hall, Inc. All rights reserved. 37
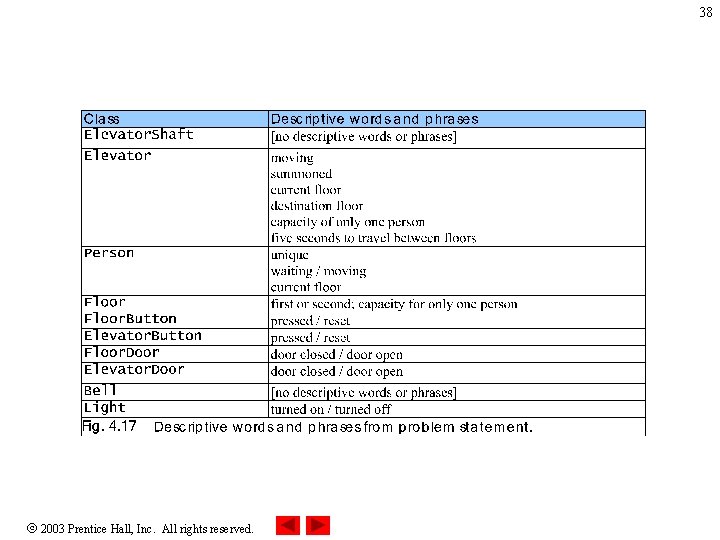
38 2003 Prentice Hall, Inc. All rights reserved.
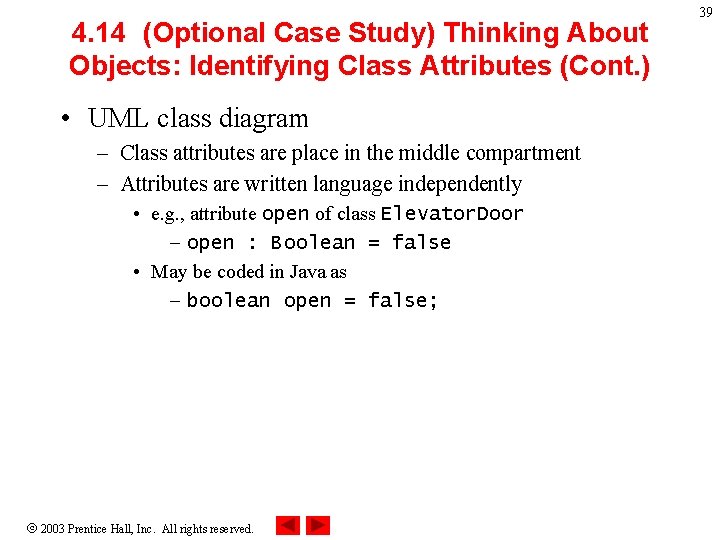
4. 14 (Optional Case Study) Thinking About Objects: Identifying Class Attributes (Cont. ) • UML class diagram – Class attributes are place in the middle compartment – Attributes are written language independently • e. g. , attribute open of class Elevator. Door – open : Boolean = false • May be coded in Java as – boolean open = false; 2003 Prentice Hall, Inc. All rights reserved. 39
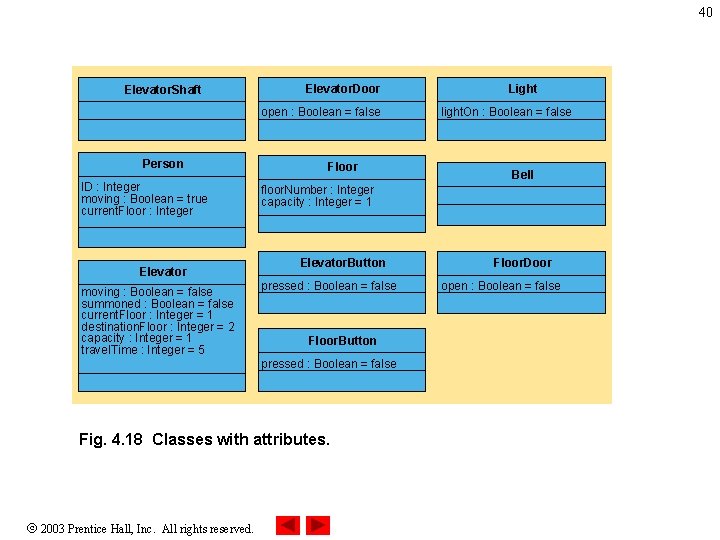
40 Elevator. Shaft Elevator. Door open : Boolean = false Person ID : Integer moving : Boolean = true current. Floor : Integer Elevator moving : Boolean = false summoned : Boolean = false current. Floor : Integer = 1 destination. Floor : Integer = 2 capacity : Integer = 1 travel. Time : Integer = 5 Floor light. On : Boolean = false Bell floor. Number : Integer capacity : Integer = 1 Elevator. Button pressed : Boolean = false Floor. Button pressed : Boolean = false Fig. 4. 18 Classes with attributes. 2003 Prentice Hall, Inc. All rights reserved. Light Floor. Door open : Boolean = false
Give other examples of homologous structures
Topic sentence outline example
Resolution in a story example
Different parts of a story's structure
Ap seminar part b outline
What is the name of the first part of an outline?
Hardware and control structures
Statement level control structures
Control structures in php
Setprecision in c++
Repetition control structure in c++
Types of control structures
Control flow structures
Statement level control structures
Control structures in php
Iteration control structures
Is a while loop a selection structure
Iteration control structures
Iteration control structures
Pseudocode outline
Control structure in visual basic
Support control and movement lesson outline
Lesson outline structure movement and control
Addition symbol
Part to part ratio definition
Brainpop ratios
Technical description meaning
Part part
The phase of the moon you see depends on ______.
Minitab adalah
Module 3 topic 4 basic maneuvering steering and braking
Yaw drivers ed
Analyzing transactions in accounting
Analyzing transactions in a cash control system
Chapter 7 organizational structures
Monopolistic competition example
Chapter 7 section 3 structures and organelles
Makenzie milton injury
Chapter 16 worksheet the knee and related structures
Chapter 7 organizational structures
Data structures chapter 1