Vladimir Misic vmcs rit edu Data structures collections
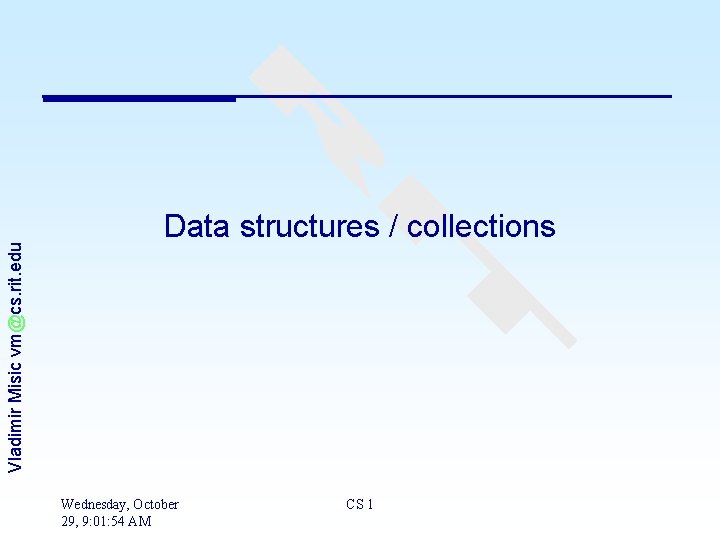
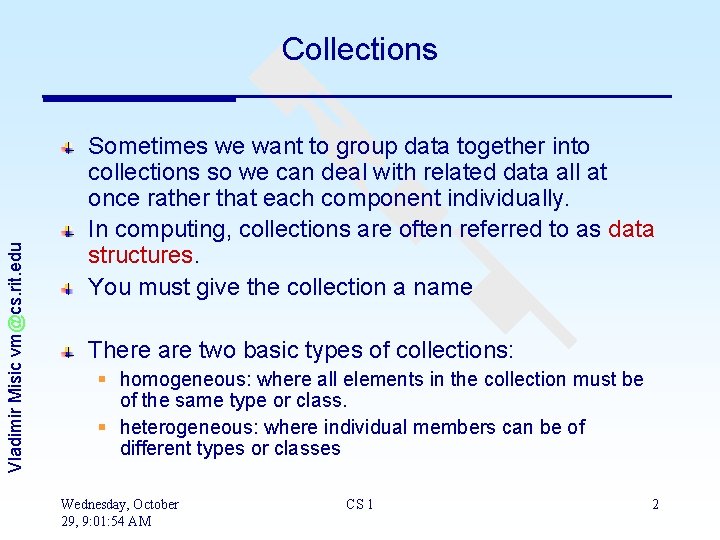
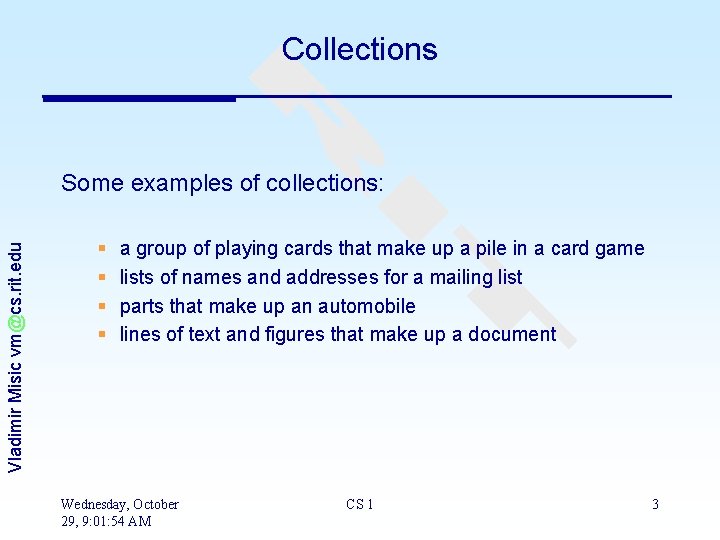
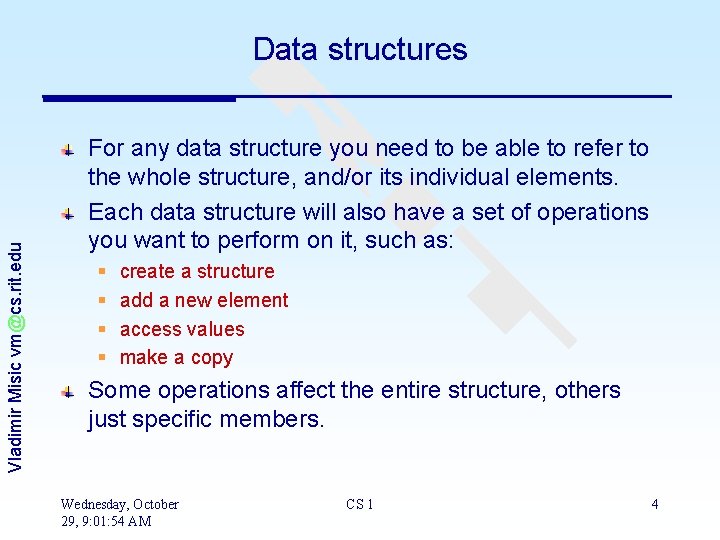
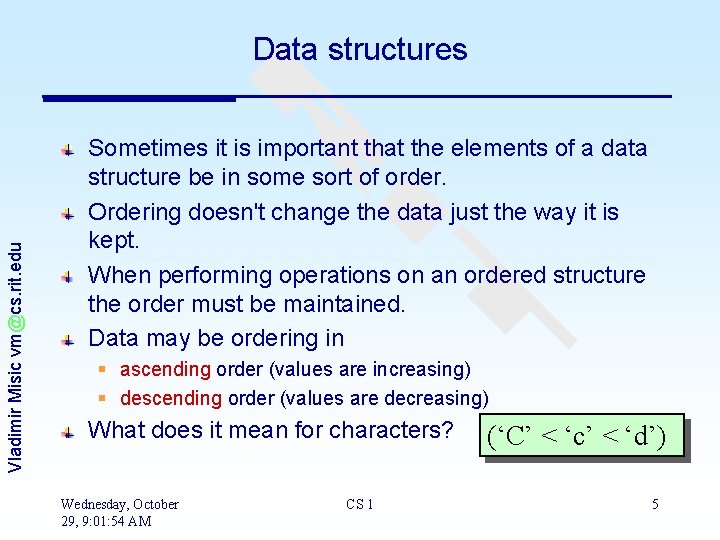
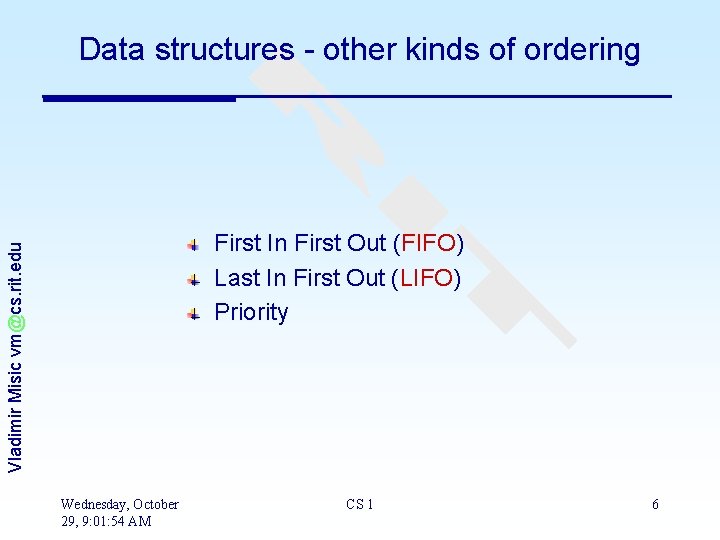
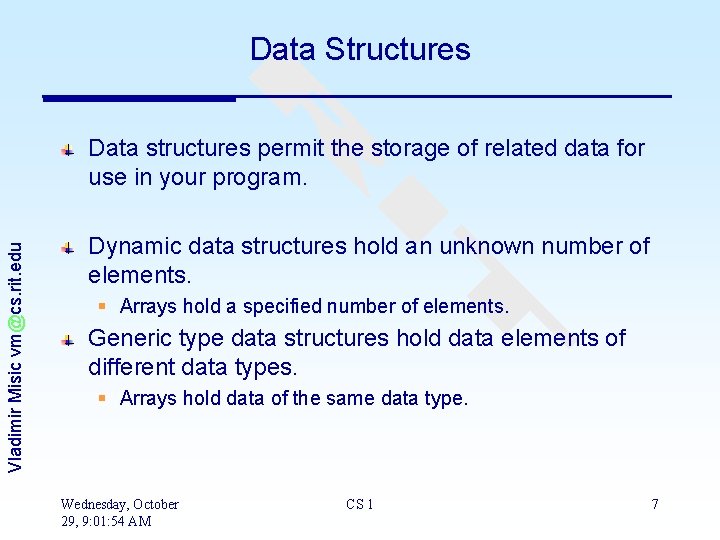
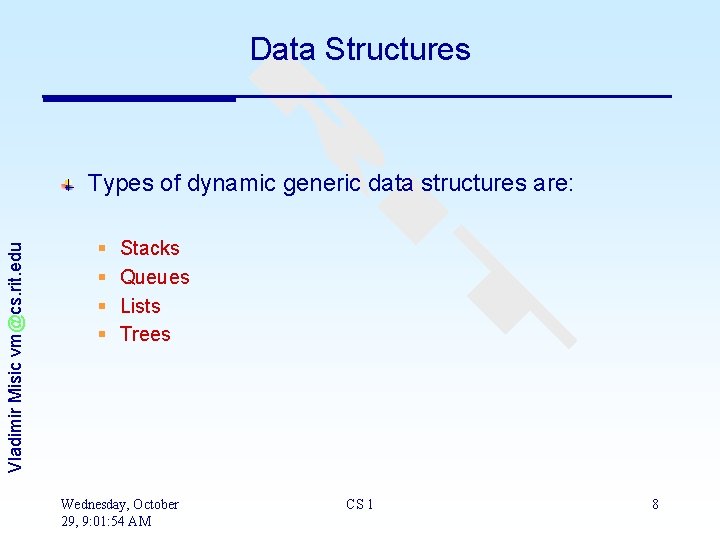
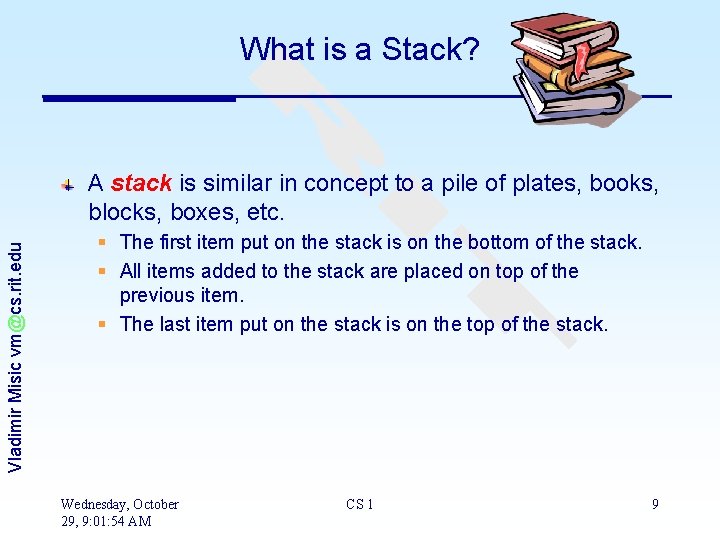
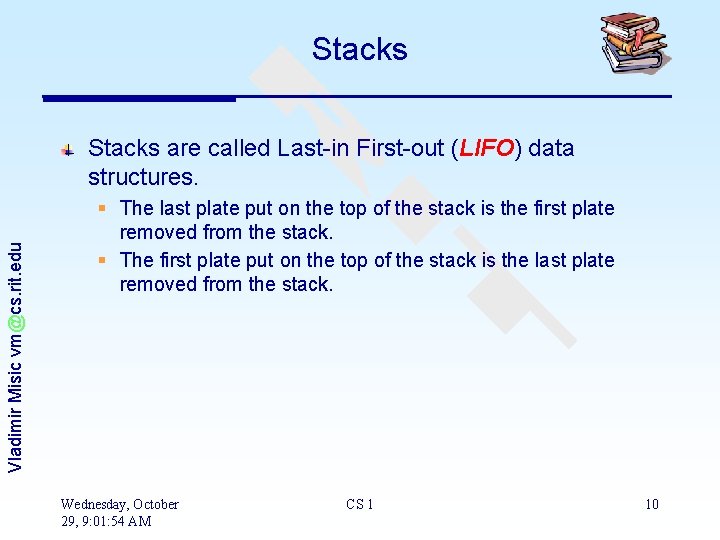
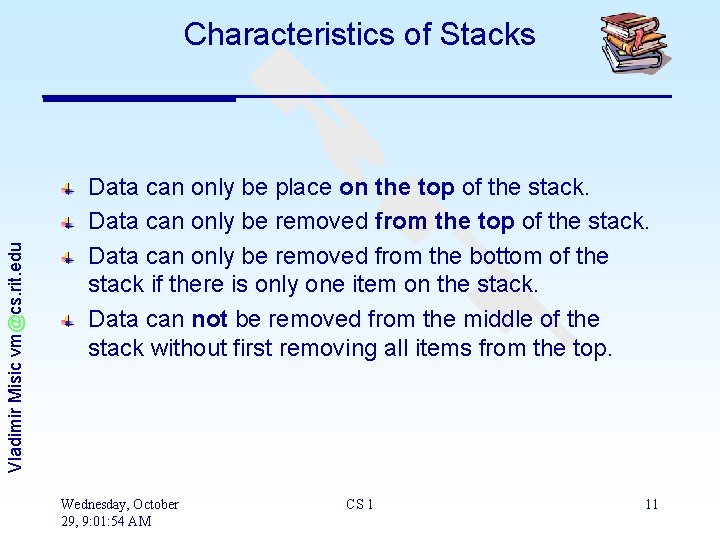
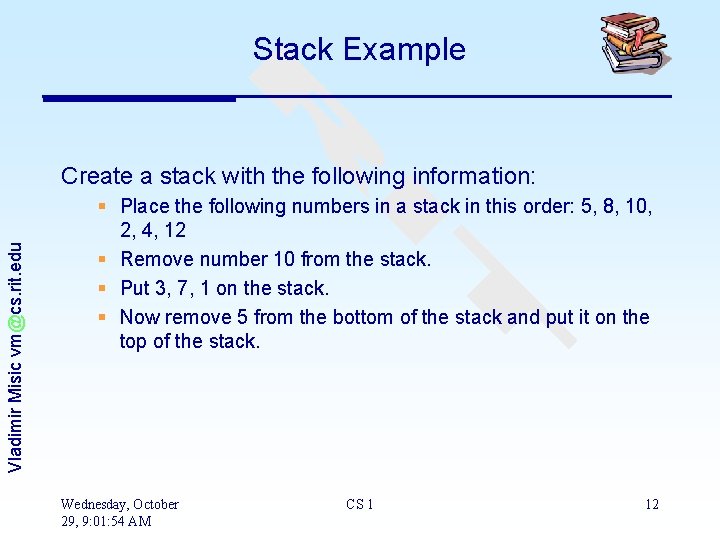
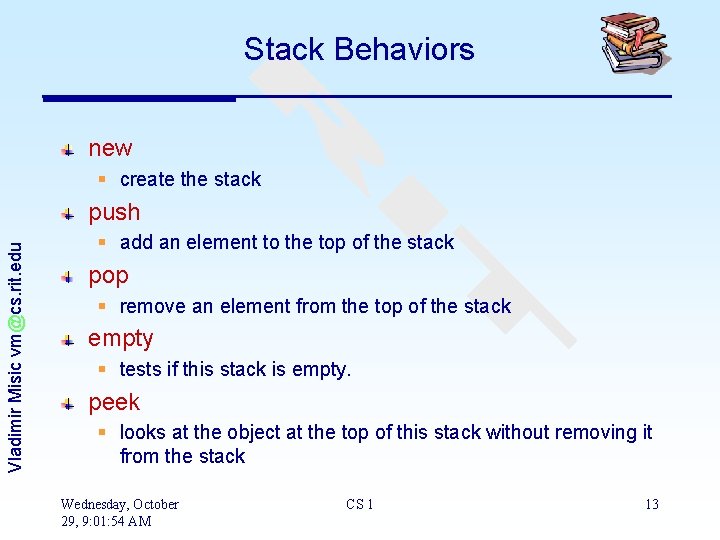
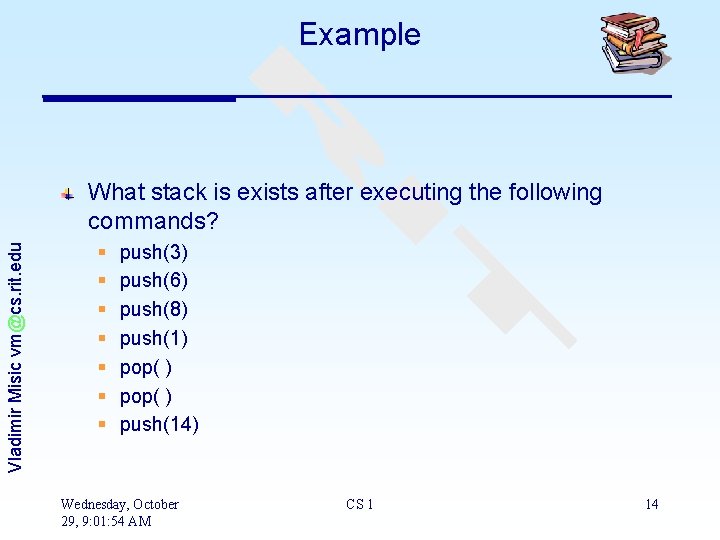
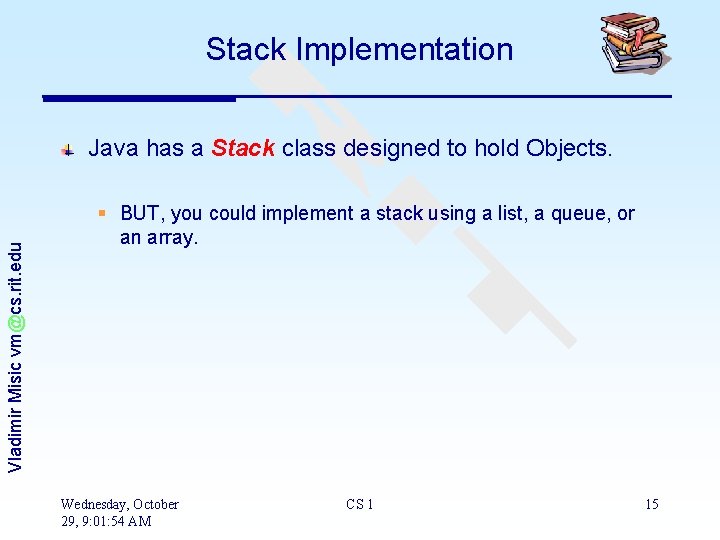
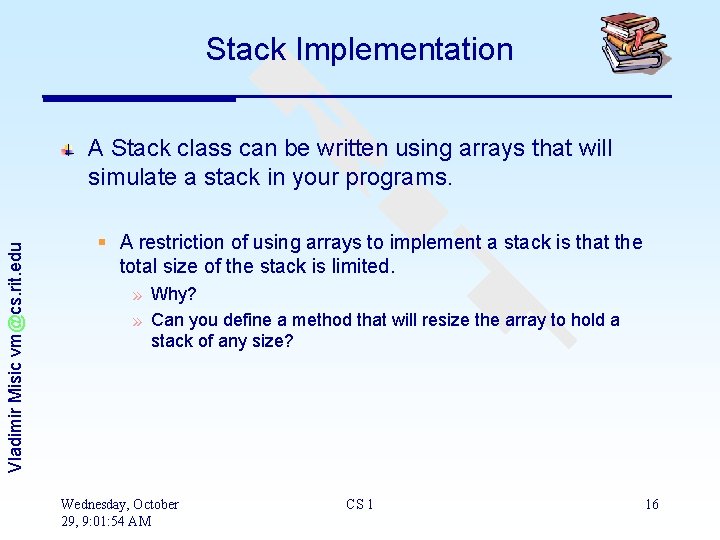
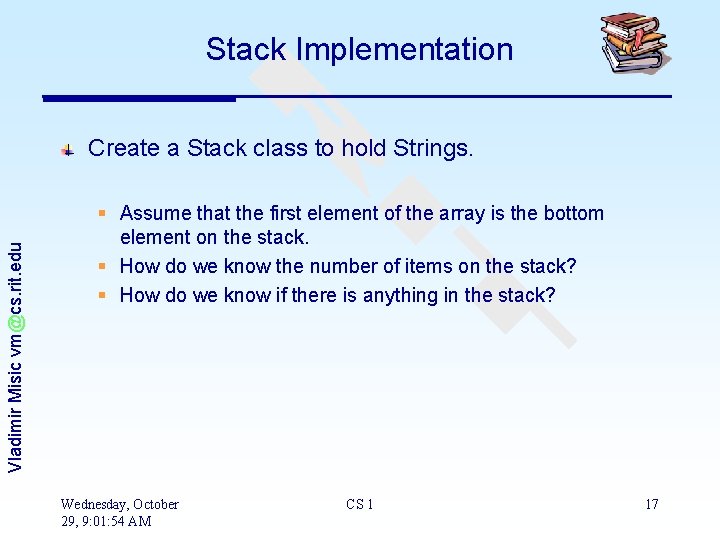
![Stack Implementation import java. io. *; class My. Stack { private String [] stack; Stack Implementation import java. io. *; class My. Stack { private String [] stack;](https://slidetodoc.com/presentation_image_h2/5acf0f9ef24299e833cafb50f66b473f/image-18.jpg)
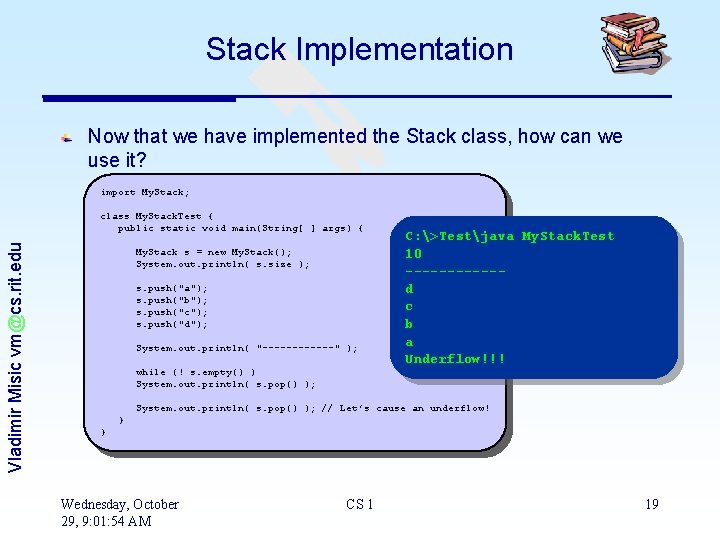
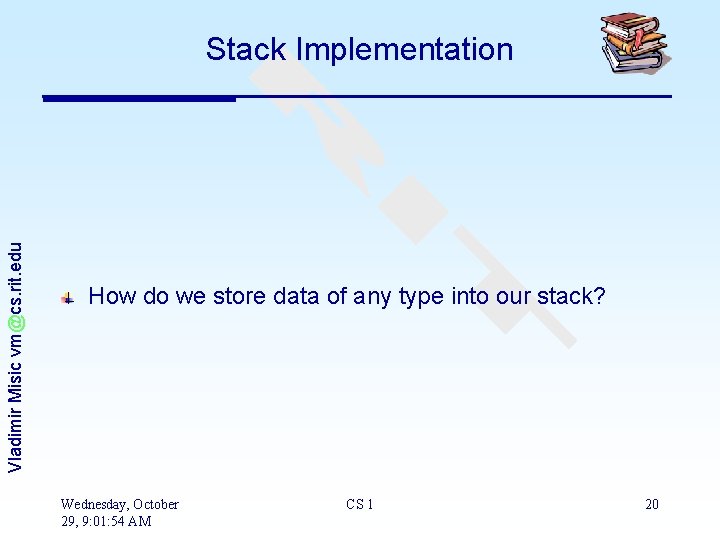
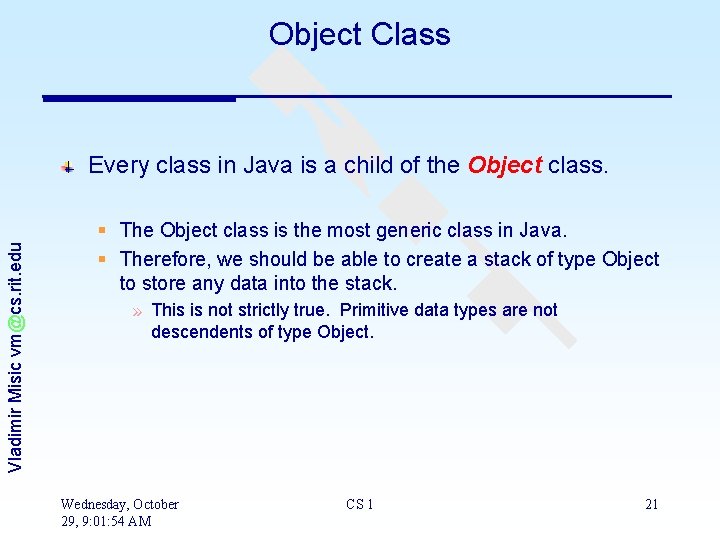
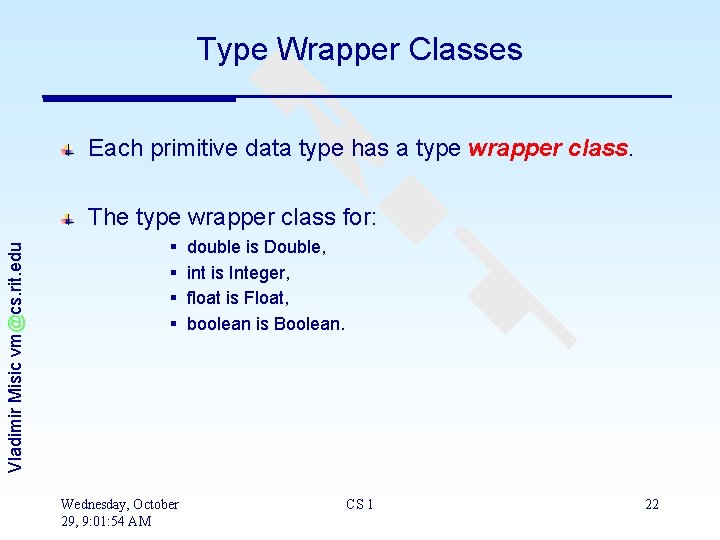
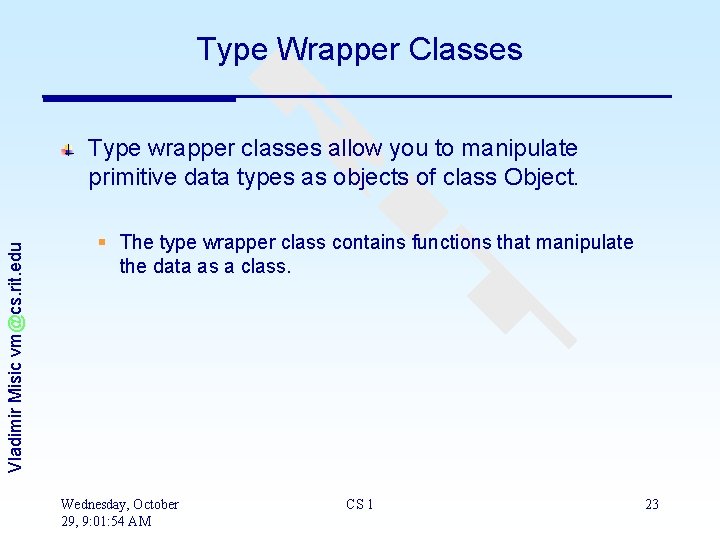
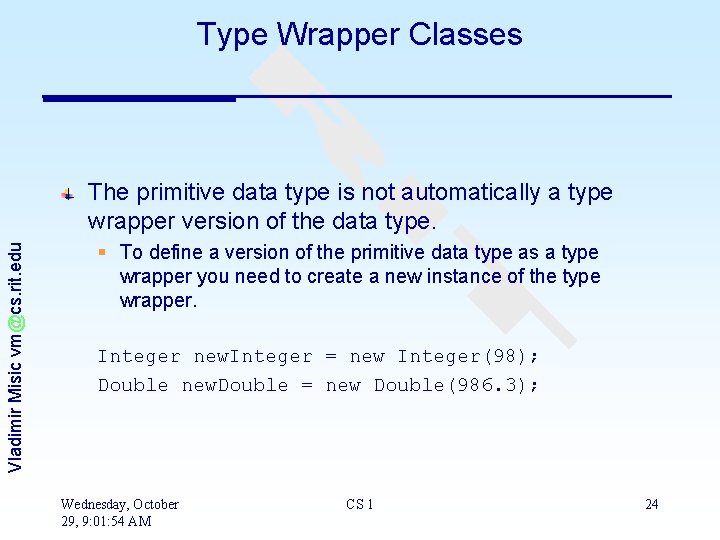
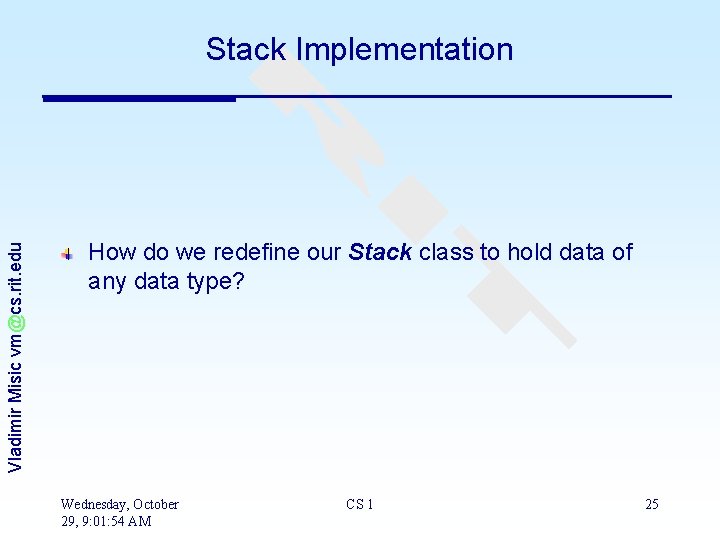
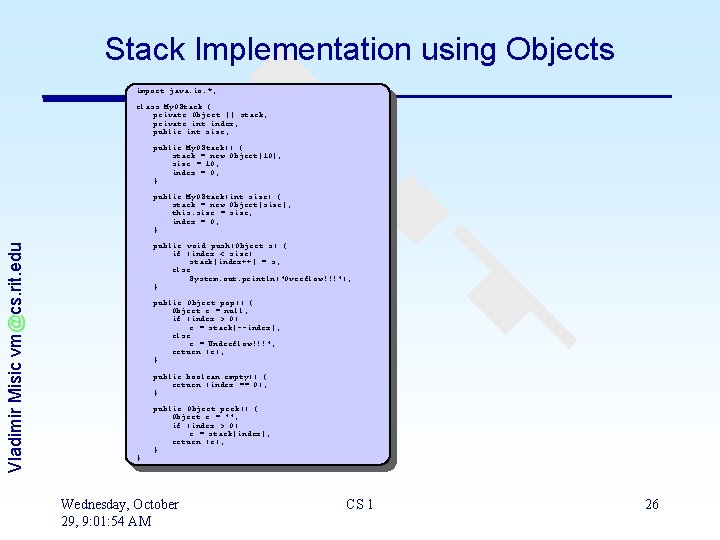
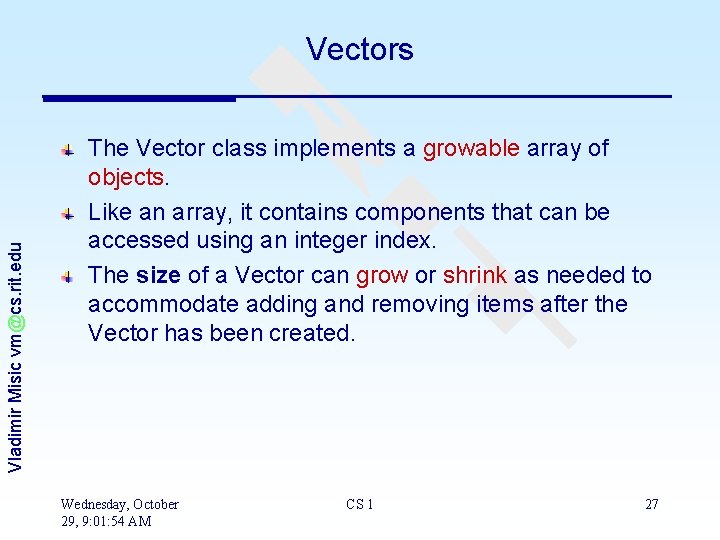
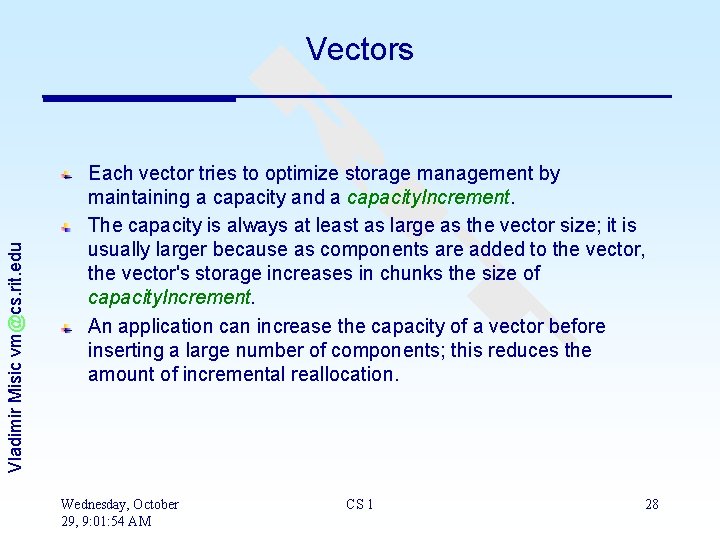
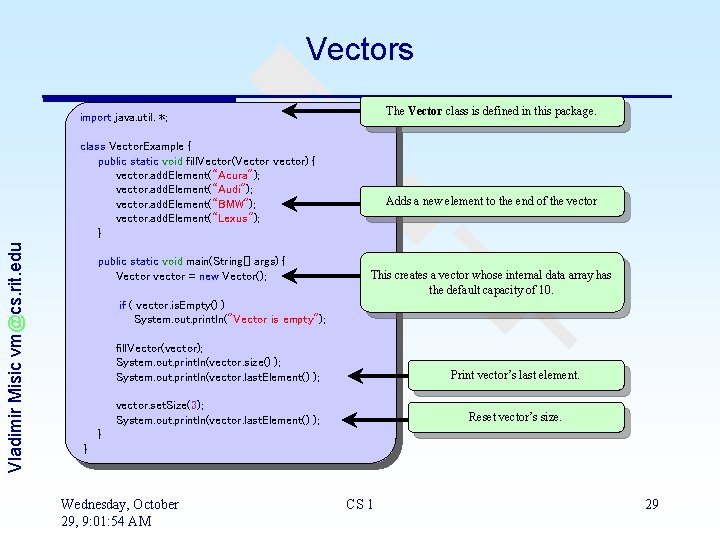
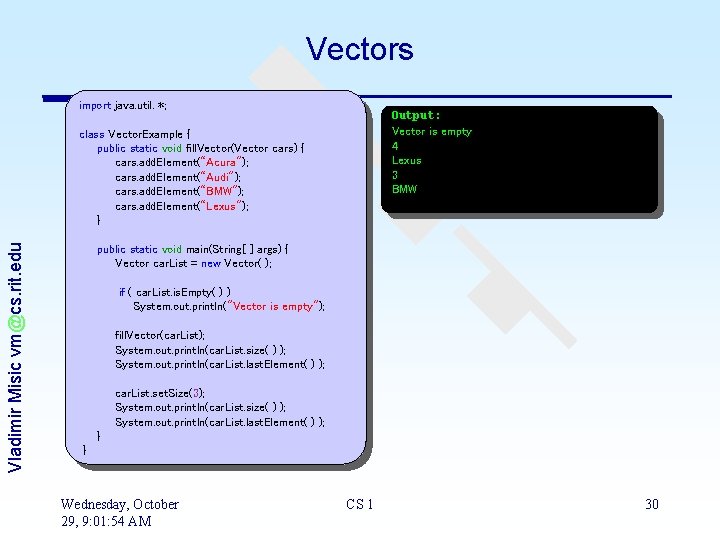
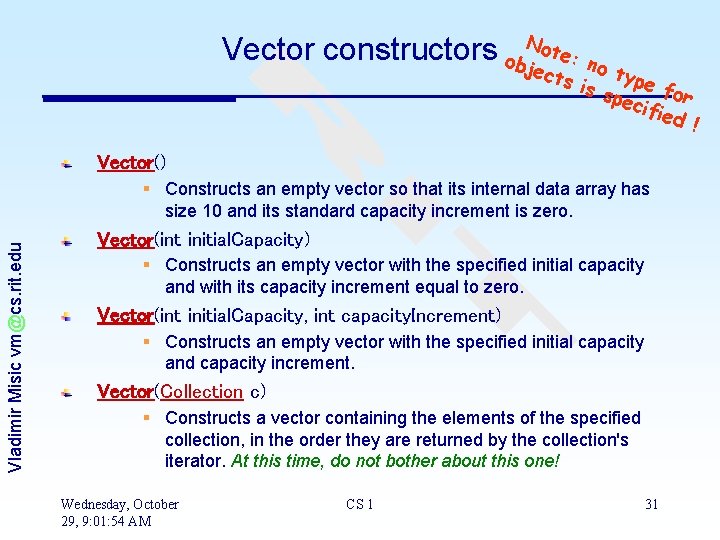
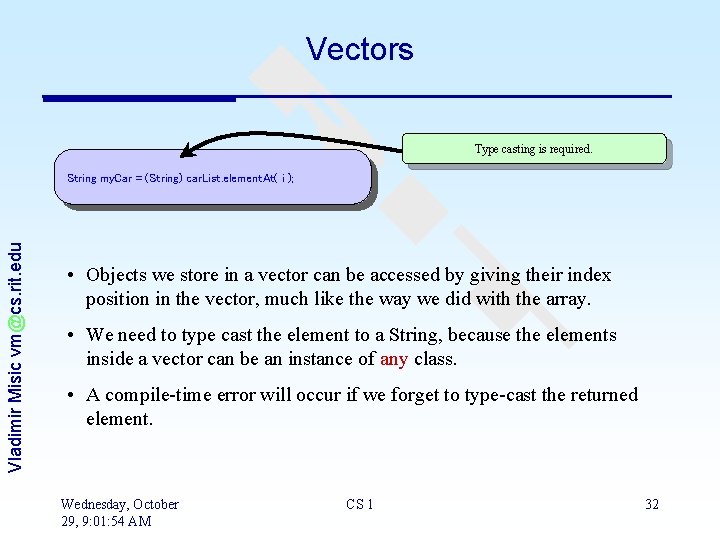
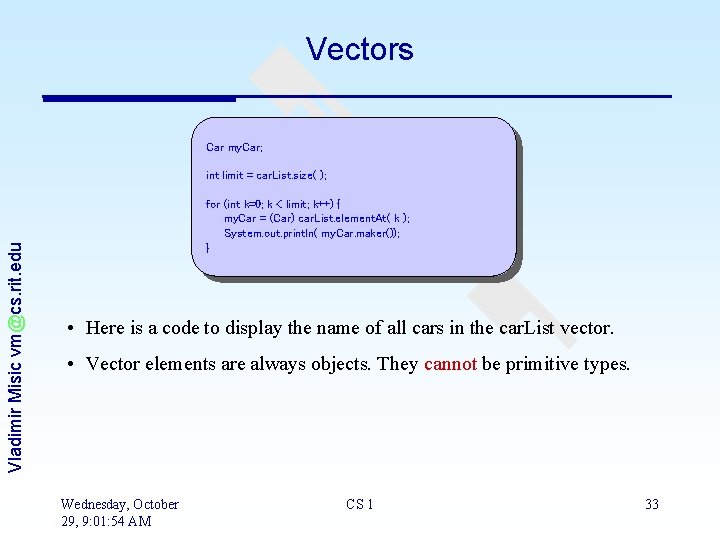
- Slides: 33
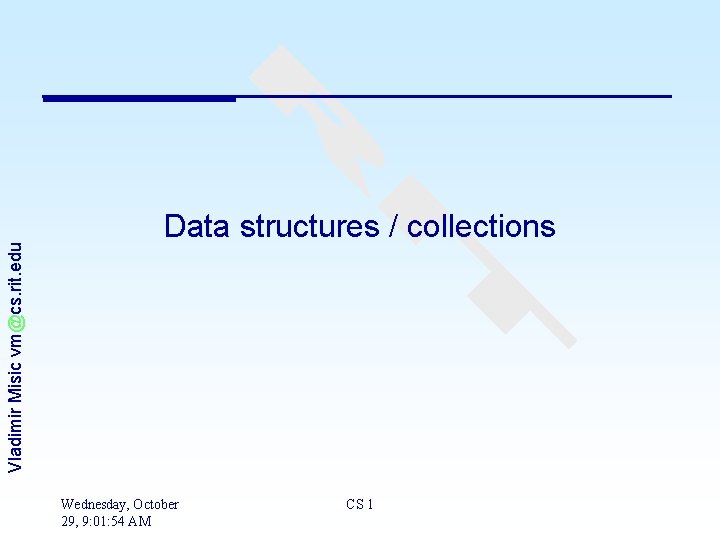
Vladimir Misic vm@cs. rit. edu Data structures / collections Wednesday, October 29, 9: 01: 54 AM CS 1
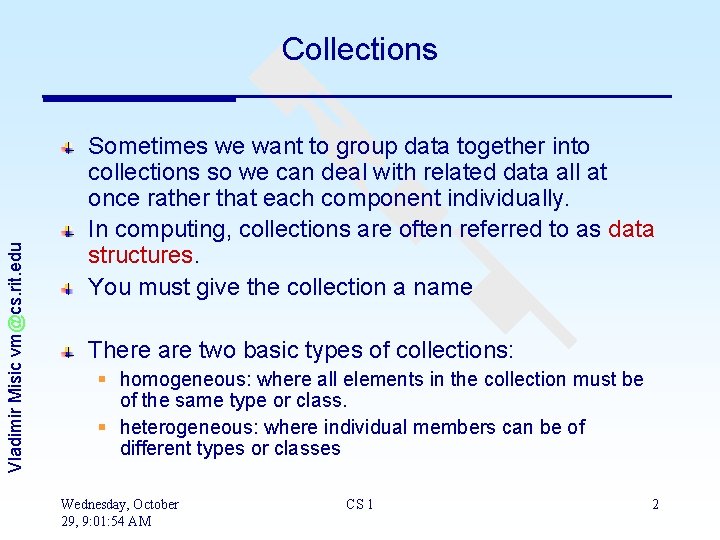
Vladimir Misic vm@cs. rit. edu Collections Sometimes we want to group data together into collections so we can deal with related data all at once rather that each component individually. In computing, collections are often referred to as data structures. You must give the collection a name There are two basic types of collections: § homogeneous: where all elements in the collection must be of the same type or class. § heterogeneous: where individual members can be of different types or classes Wednesday, October 29, 9: 01: 54 AM CS 1 2
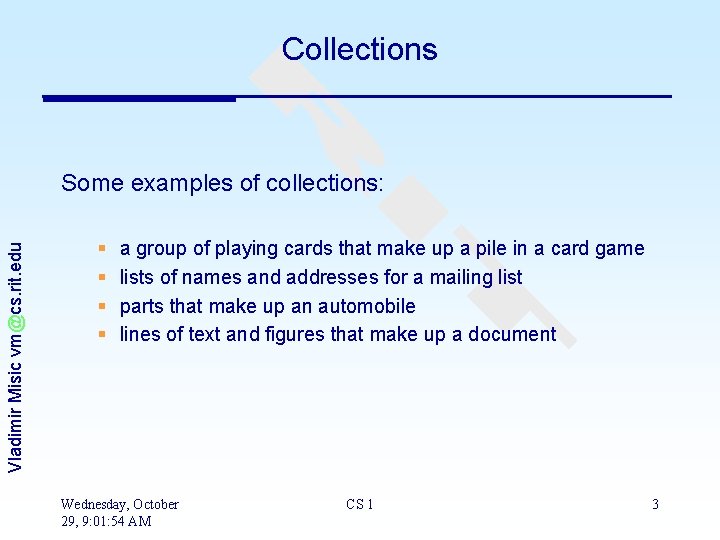
Collections Vladimir Misic vm@cs. rit. edu Some examples of collections: § § a group of playing cards that make up a pile in a card game lists of names and addresses for a mailing list parts that make up an automobile lines of text and figures that make up a document Wednesday, October 29, 9: 01: 54 AM CS 1 3
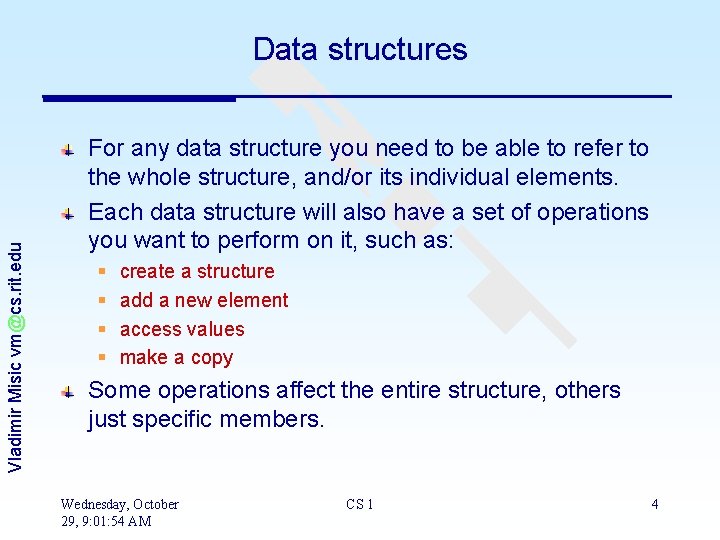
Vladimir Misic vm@cs. rit. edu Data structures For any data structure you need to be able to refer to the whole structure, and/or its individual elements. Each data structure will also have a set of operations you want to perform on it, such as: § § create a structure add a new element access values make a copy Some operations affect the entire structure, others just specific members. Wednesday, October 29, 9: 01: 54 AM CS 1 4
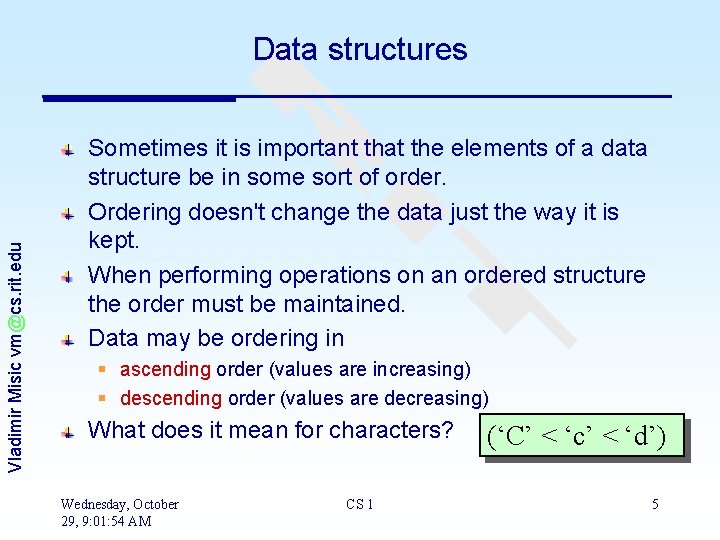
Vladimir Misic vm@cs. rit. edu Data structures Sometimes it is important that the elements of a data structure be in some sort of order. Ordering doesn't change the data just the way it is kept. When performing operations on an ordered structure the order must be maintained. Data may be ordering in § ascending order (values are increasing) § descending order (values are decreasing) What does it mean for characters? Wednesday, October 29, 9: 01: 54 AM CS 1 (‘C’ < ‘c’ < ‘d’) 5
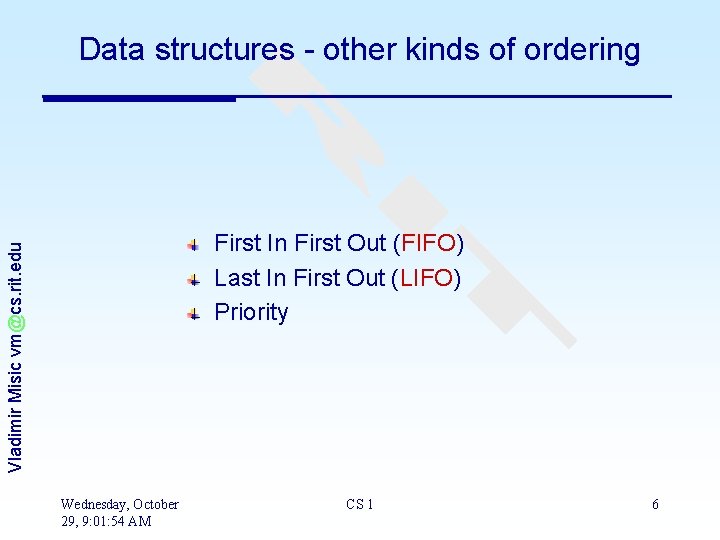
Data structures - other kinds of ordering Vladimir Misic vm@cs. rit. edu First In First Out (FIFO) Last In First Out (LIFO) Priority Wednesday, October 29, 9: 01: 54 AM CS 1 6
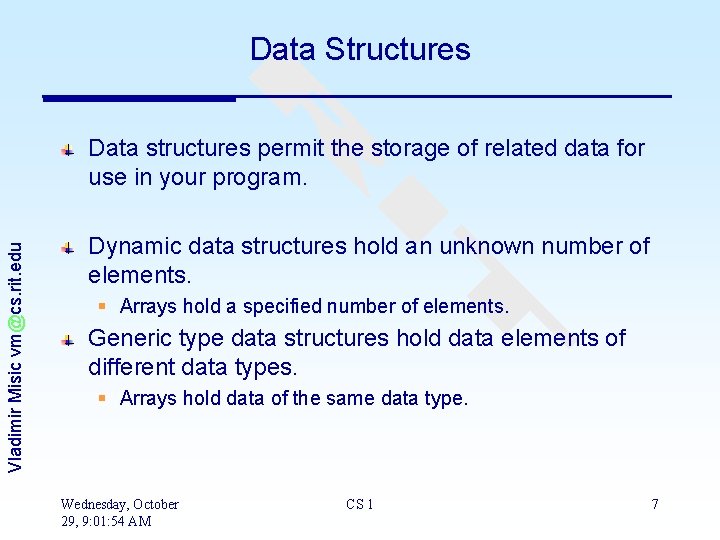
Data Structures Vladimir Misic vm@cs. rit. edu Data structures permit the storage of related data for use in your program. Dynamic data structures hold an unknown number of elements. § Arrays hold a specified number of elements. Generic type data structures hold data elements of different data types. § Arrays hold data of the same data type. Wednesday, October 29, 9: 01: 54 AM CS 1 7
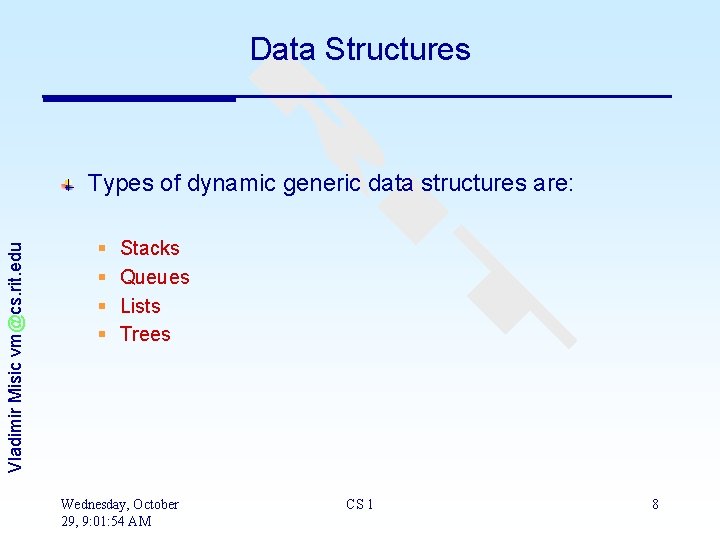
Data Structures Vladimir Misic vm@cs. rit. edu Types of dynamic generic data structures are: § § Stacks Queues Lists Trees Wednesday, October 29, 9: 01: 54 AM CS 1 8
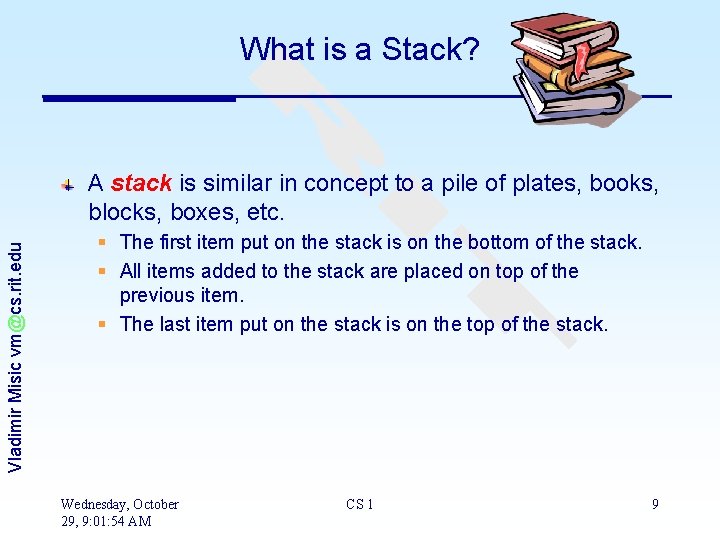
What is a Stack? Vladimir Misic vm@cs. rit. edu A stack is similar in concept to a pile of plates, books, blocks, boxes, etc. § The first item put on the stack is on the bottom of the stack. § All items added to the stack are placed on top of the previous item. § The last item put on the stack is on the top of the stack. Wednesday, October 29, 9: 01: 54 AM CS 1 9
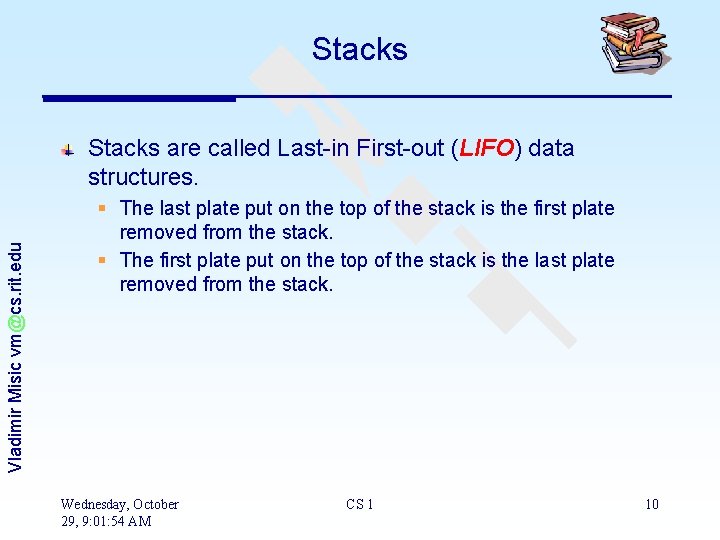
Stacks Vladimir Misic vm@cs. rit. edu Stacks are called Last-in First-out (LIFO) data structures. § The last plate put on the top of the stack is the first plate removed from the stack. § The first plate put on the top of the stack is the last plate removed from the stack. Wednesday, October 29, 9: 01: 54 AM CS 1 10
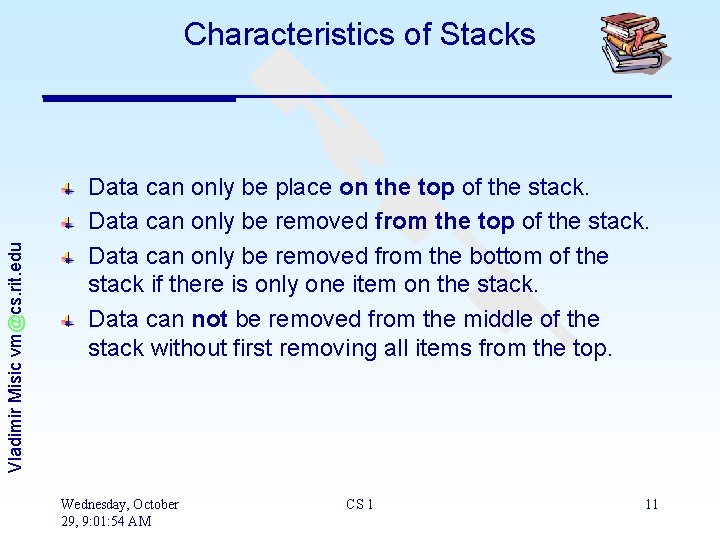
Vladimir Misic vm@cs. rit. edu Characteristics of Stacks Data can only be place on the top of the stack. Data can only be removed from the bottom of the stack if there is only one item on the stack. Data can not be removed from the middle of the stack without first removing all items from the top. Wednesday, October 29, 9: 01: 54 AM CS 1 11
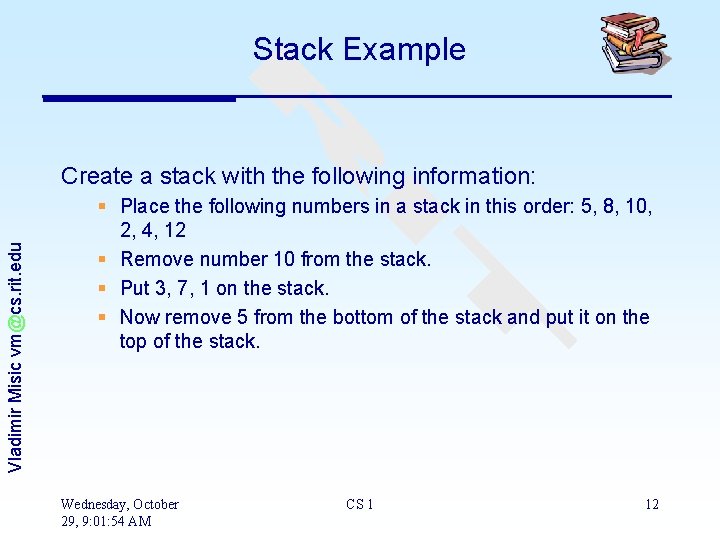
Stack Example Vladimir Misic vm@cs. rit. edu Create a stack with the following information: § Place the following numbers in a stack in this order: 5, 8, 10, 2, 4, 12 § Remove number 10 from the stack. § Put 3, 7, 1 on the stack. § Now remove 5 from the bottom of the stack and put it on the top of the stack. Wednesday, October 29, 9: 01: 54 AM CS 1 12
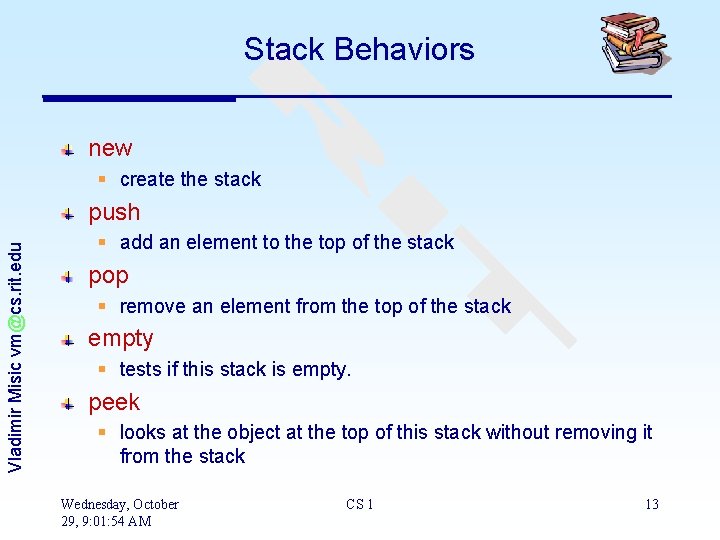
Stack Behaviors new § create the stack Vladimir Misic vm@cs. rit. edu push § add an element to the top of the stack pop § remove an element from the top of the stack empty § tests if this stack is empty. peek § looks at the object at the top of this stack without removing it from the stack Wednesday, October 29, 9: 01: 54 AM CS 1 13
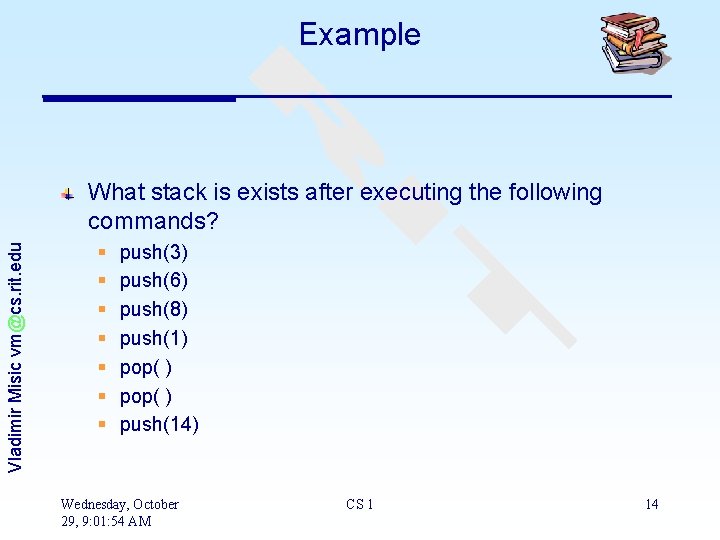
Example Vladimir Misic vm@cs. rit. edu What stack is exists after executing the following commands? § § § § push(3) push(6) push(8) push(1) pop( ) push(14) Wednesday, October 29, 9: 01: 54 AM CS 1 14
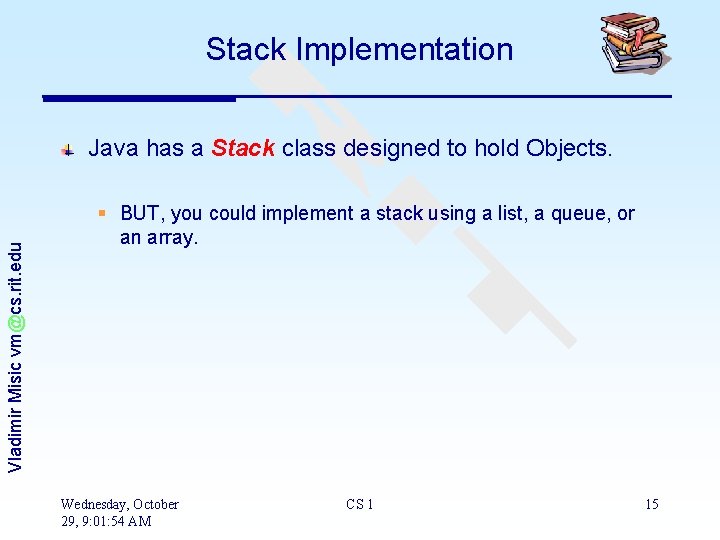
Stack Implementation Vladimir Misic vm@cs. rit. edu Java has a Stack class designed to hold Objects. § BUT, you could implement a stack using a list, a queue, or an array. Wednesday, October 29, 9: 01: 54 AM CS 1 15
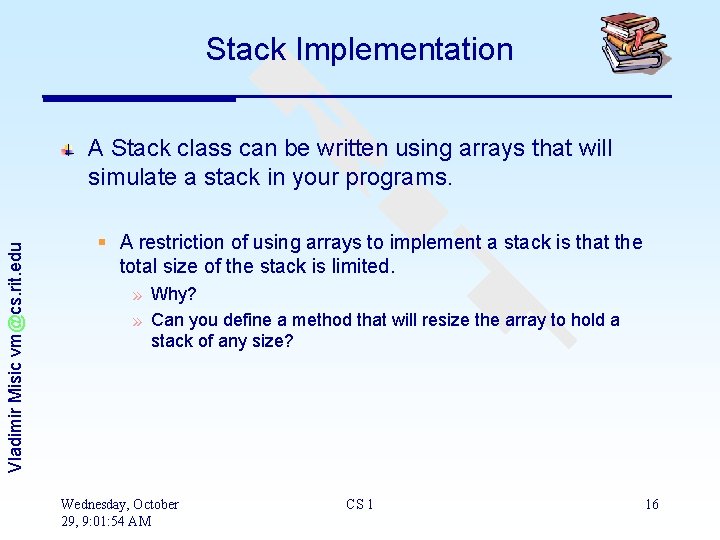
Stack Implementation Vladimir Misic vm@cs. rit. edu A Stack class can be written using arrays that will simulate a stack in your programs. § A restriction of using arrays to implement a stack is that the total size of the stack is limited. » Why? » Can you define a method that will resize the array to hold a stack of any size? Wednesday, October 29, 9: 01: 54 AM CS 1 16
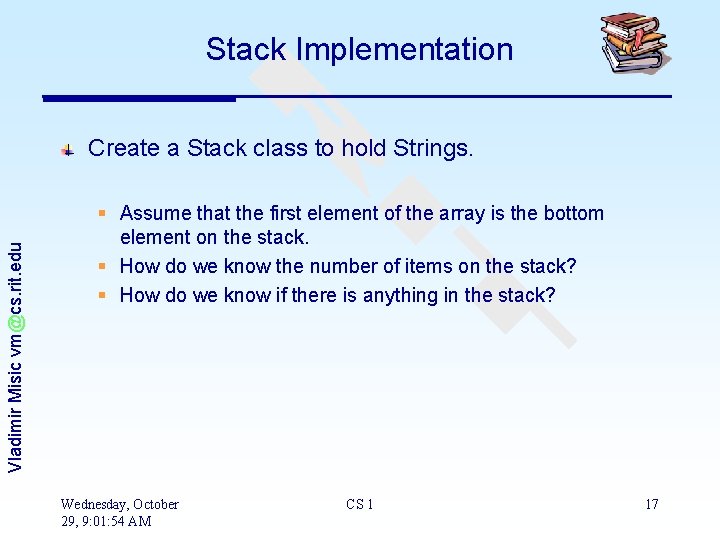
Stack Implementation Vladimir Misic vm@cs. rit. edu Create a Stack class to hold Strings. § Assume that the first element of the array is the bottom element on the stack. § How do we know the number of items on the stack? § How do we know if there is anything in the stack? Wednesday, October 29, 9: 01: 54 AM CS 1 17
![Stack Implementation import java io class My Stack private String stack Stack Implementation import java. io. *; class My. Stack { private String [] stack;](https://slidetodoc.com/presentation_image_h2/5acf0f9ef24299e833cafb50f66b473f/image-18.jpg)
Stack Implementation import java. io. *; class My. Stack { private String [] stack; private int index; public int size; public My. Stack() { this. stack = new String[10]; this. size = 10; this. index = 0; } Vladimir Misic vm@cs. rit. edu public My. Stack(int size) { stack = new String[size]; this. size = size; index = 0; } public void push(String s) { if (index < size) stack[index++] = s; else System. out. println("Overflow!!!"); } public String pop() { String r = ""; if (index > 0) r = stack[--index]; else r = “Underflow!!!"; return (r); } Not e: N sho uld ot 100% h und andle corre o c erfl ow verflo t. We wa bett nd er ! !! public boolean empty() { return (index == 0); } } public String peek() { String r = ""; if (index > 0) r = stack[index-1]; return (r); } Wednesday, October 29, 9: 01: 54 AM CS 1 18
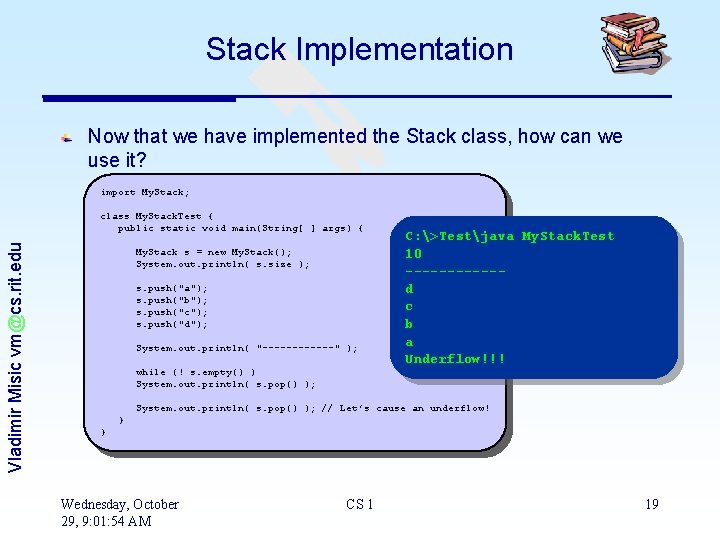
Stack Implementation Now that we have implemented the Stack class, how can we use it? import My. Stack; Vladimir Misic vm@cs. rit. edu class My. Stack. Test { public static void main(String[ ] args) { My. Stack s = new My. Stack(); System. out. println( s. size ); s. push("a"); s. push("b"); s. push("c"); s. push("d"); System. out. println( "------" ); while (! s. empty() ) System. out. println( s. pop() ); C: >Testjava My. Stack. Test 10 ------d c b a Underflow!!! System. out. println( s. pop() ); // Let’s cause an underflow! } } Wednesday, October 29, 9: 01: 54 AM CS 1 19
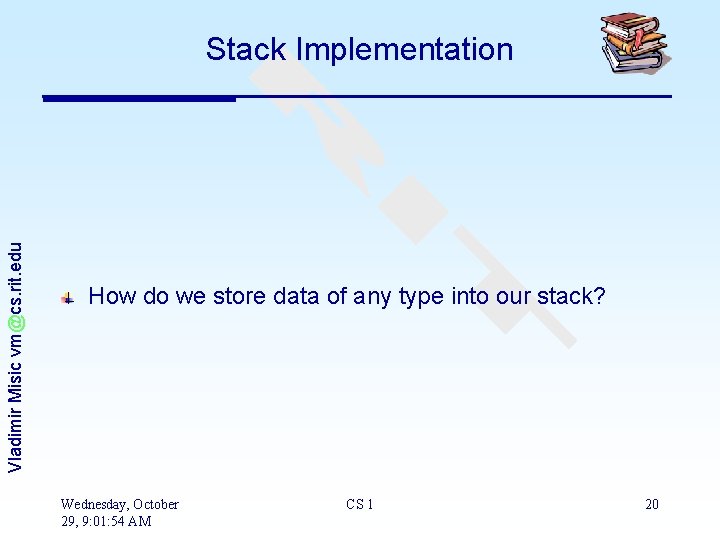
Vladimir Misic vm@cs. rit. edu Stack Implementation How do we store data of any type into our stack? Wednesday, October 29, 9: 01: 54 AM CS 1 20
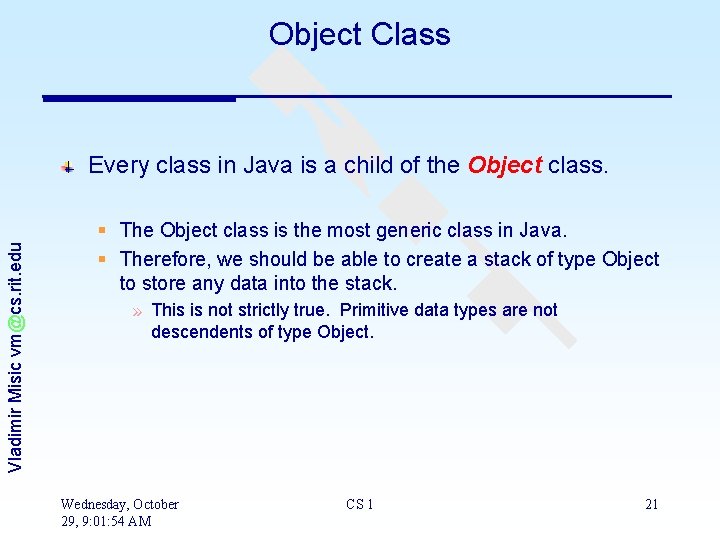
Object Class Vladimir Misic vm@cs. rit. edu Every class in Java is a child of the Object class. § The Object class is the most generic class in Java. § Therefore, we should be able to create a stack of type Object to store any data into the stack. » This is not strictly true. Primitive data types are not descendents of type Object. Wednesday, October 29, 9: 01: 54 AM CS 1 21
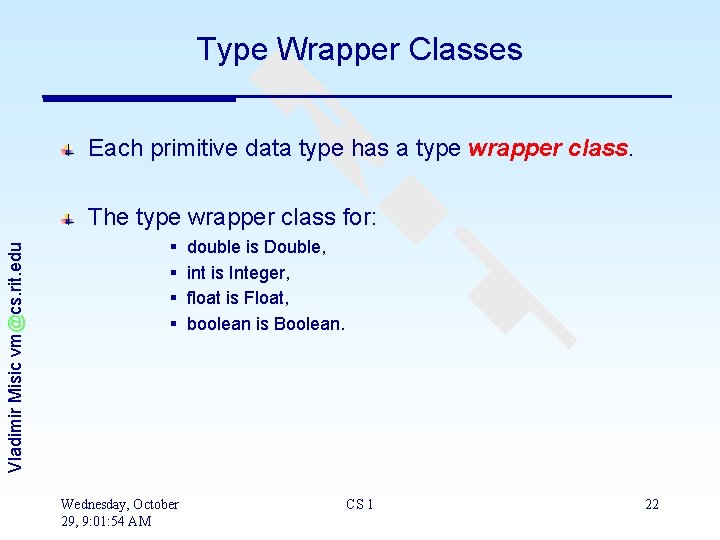
Type Wrapper Classes Each primitive data type has a type wrapper class. Vladimir Misic vm@cs. rit. edu The type wrapper class for: § § Wednesday, October 29, 9: 01: 54 AM double is Double, int is Integer, float is Float, boolean is Boolean. CS 1 22
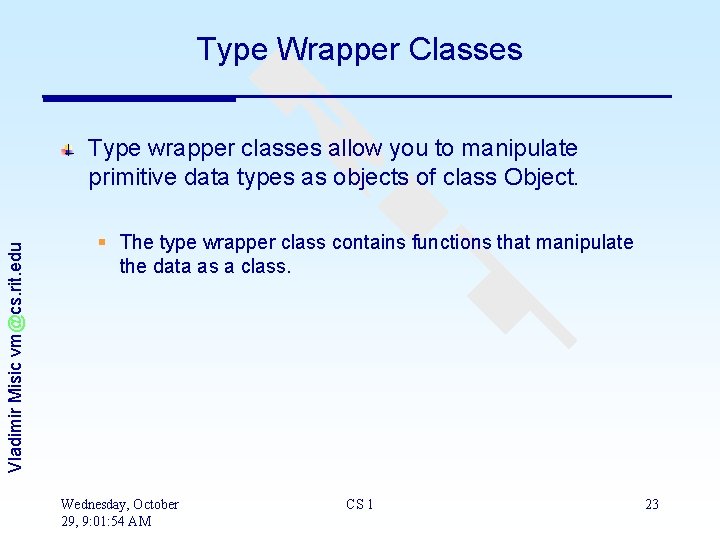
Type Wrapper Classes Vladimir Misic vm@cs. rit. edu Type wrapper classes allow you to manipulate primitive data types as objects of class Object. § The type wrapper class contains functions that manipulate the data as a class. Wednesday, October 29, 9: 01: 54 AM CS 1 23
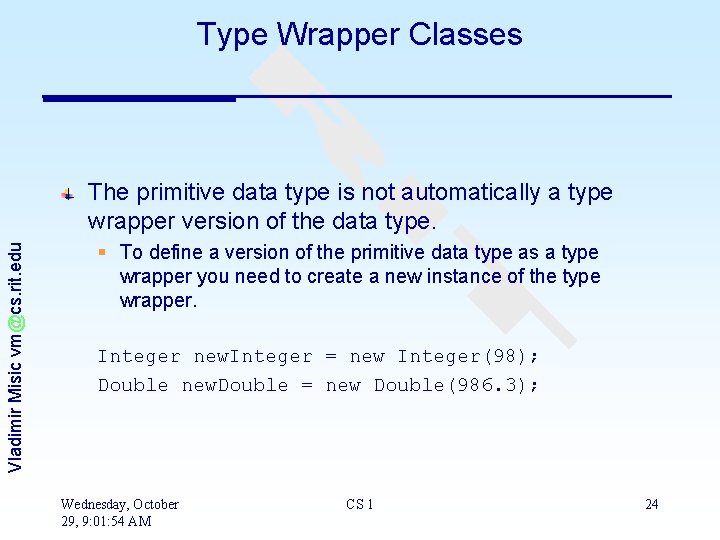
Type Wrapper Classes Vladimir Misic vm@cs. rit. edu The primitive data type is not automatically a type wrapper version of the data type. § To define a version of the primitive data type as a type wrapper you need to create a new instance of the type wrapper. Integer new. Integer = new Integer(98); Double new. Double = new Double(986. 3); Wednesday, October 29, 9: 01: 54 AM CS 1 24
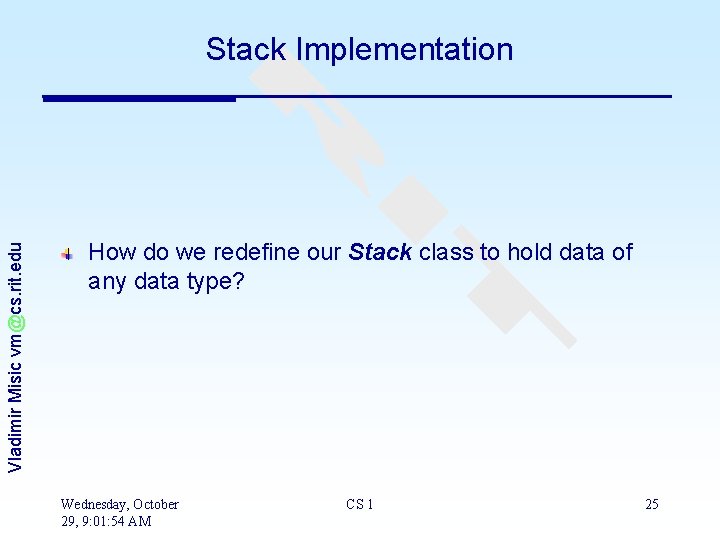
Vladimir Misic vm@cs. rit. edu Stack Implementation How do we redefine our Stack class to hold data of any data type? Wednesday, October 29, 9: 01: 54 AM CS 1 25
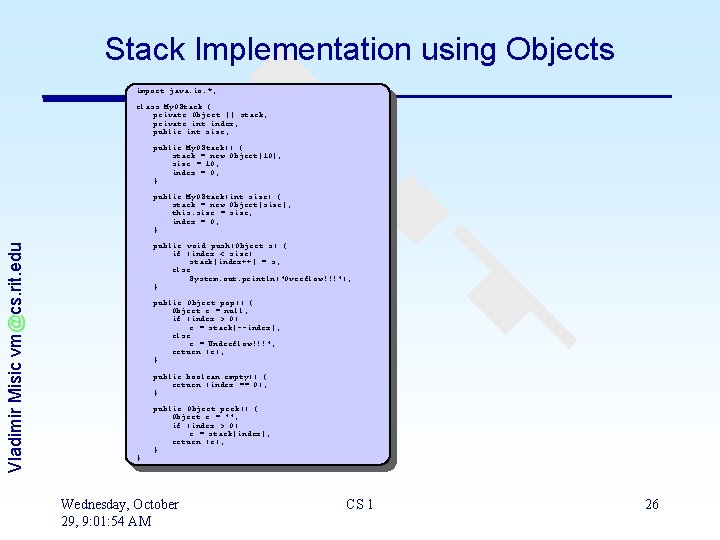
Stack Implementation using Objects import java. io. *; class My. OStack { private Object [] stack; private int index; public int size; public My. OStack() { stack = new Object[10]; size = 10; index = 0; } Vladimir Misic vm@cs. rit. edu public My. OStack(int size) { stack = new Object[size]; this. size = size; index = 0; } public void push(Object s) { if (index < size) stack[index++] = s; else System. out. println("Overflow!!!"); } public Object pop() { Object r = null; if (index > 0) r = stack[--index]; else r = Underflow!!!"; return (r); } public boolean empty() { return (index == 0); } } public Object peek() { Object r = ""; if (index > 0) r = stack[index]; return (r); } Wednesday, October 29, 9: 01: 54 AM CS 1 26
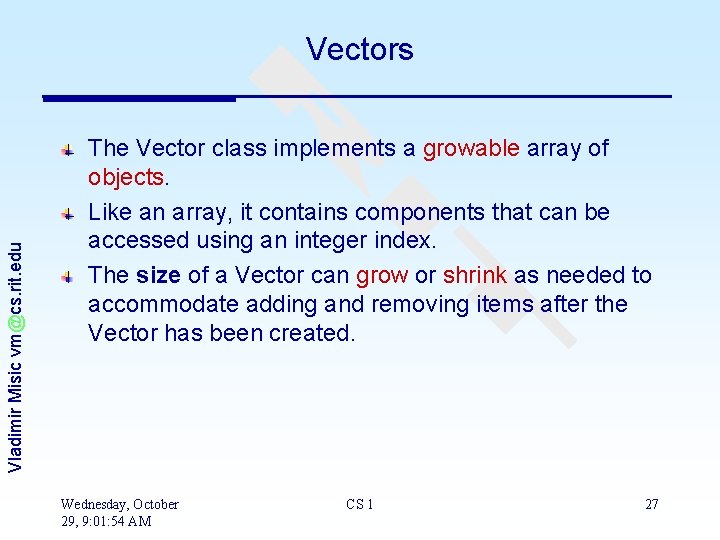
Vladimir Misic vm@cs. rit. edu Vectors The Vector class implements a growable array of objects. Like an array, it contains components that can be accessed using an integer index. The size of a Vector can grow or shrink as needed to accommodate adding and removing items after the Vector has been created. Wednesday, October 29, 9: 01: 54 AM CS 1 27
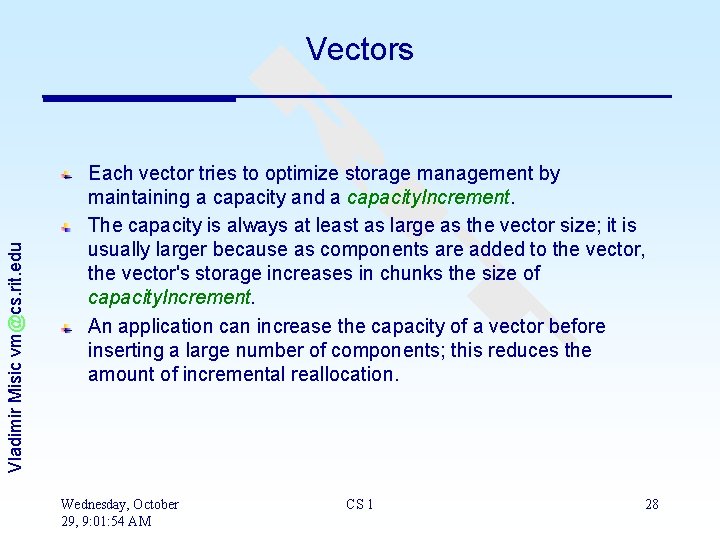
Vladimir Misic vm@cs. rit. edu Vectors Each vector tries to optimize storage management by maintaining a capacity and a capacity. Increment. The capacity is always at least as large as the vector size; it is usually larger because as components are added to the vector, the vector's storage increases in chunks the size of capacity. Increment. An application can increase the capacity of a vector before inserting a large number of components; this reduces the amount of incremental reallocation. Wednesday, October 29, 9: 01: 54 AM CS 1 28
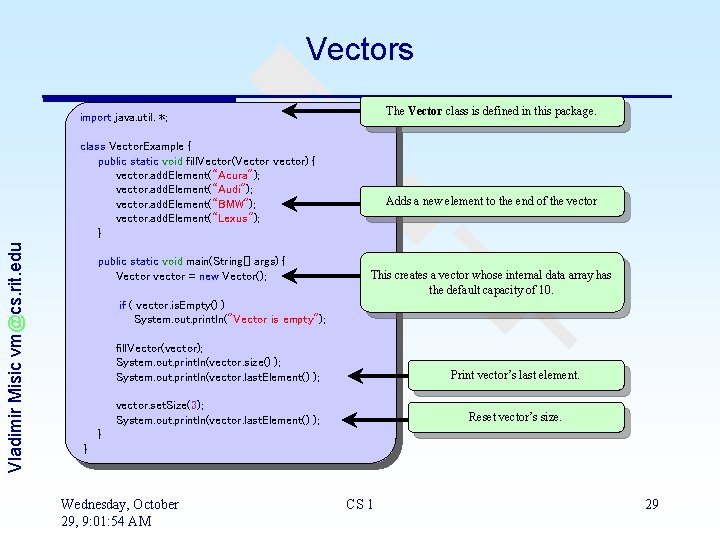
Vectors The Vector class is defined in this package. import java. util. *; Vladimir Misic vm@cs. rit. edu class Vector. Example { public static void fill. Vector(Vector vector) { vector. add. Element(“Acura"); vector. add. Element(“Audi"); vector. add. Element(“BMW"); vector. add. Element(“Lexus"); } public static void main(String[] args) { Vector vector = new Vector(); Adds a new element to the end of the vector This creates a vector whose internal data array has the default capacity of 10. if ( vector. is. Empty() ) System. out. println("Vector is empty"); fill. Vector(vector); System. out. println(vector. size() ); System. out. println(vector. last. Element() ); Print vector’s last element. vector. set. Size(3); System. out. println(vector. last. Element() ); Reset vector’s size. } } Wednesday, October 29, 9: 01: 54 AM CS 1 29
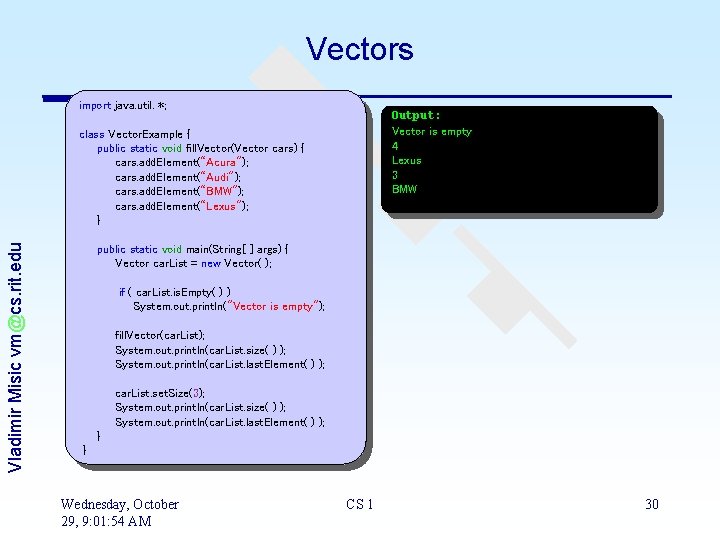
Vectors import java. util. *; Output: Vector is empty 4 Lexus 3 BMW Vladimir Misic vm@cs. rit. edu class Vector. Example { public static void fill. Vector(Vector cars) { cars. add. Element(“Acura"); cars. add. Element(“Audi"); cars. add. Element(“BMW"); cars. add. Element(“Lexus"); } public static void main(String[ ] args) { Vector car. List = new Vector( ); if ( car. List. is. Empty( ) ) System. out. println("Vector is empty"); fill. Vector(car. List); System. out. println(car. List. size( ) ); System. out. println(car. List. last. Element( ) ); car. List. set. Size(3); System. out. println(car. List. size( ) ); System. out. println(car. List. last. Element( ) ); } } Wednesday, October 29, 9: 01: 54 AM CS 1 30
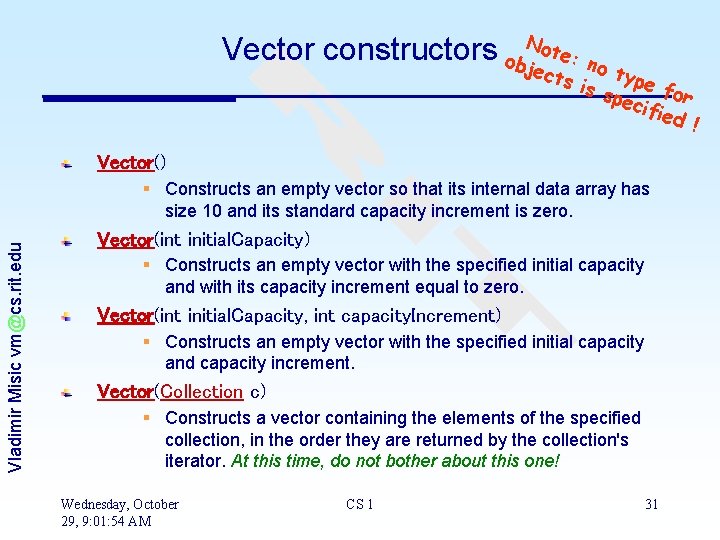
Vector constructors ob. Nj ote: no ect s typ e is s pec for ifie d ! Vector() Vladimir Misic vm@cs. rit. edu § Constructs an empty vector so that its internal data array has size 10 and its standard capacity increment is zero. Vector(int initial. Capacity) § Constructs an empty vector with the specified initial capacity and with its capacity increment equal to zero. Vector(int initial. Capacity, int capacity. Increment) § Constructs an empty vector with the specified initial capacity and capacity increment. Vector(Collection c) § Constructs a vector containing the elements of the specified collection, in the order they are returned by the collection's iterator. At this time, do not bother about this one! Wednesday, October 29, 9: 01: 54 AM CS 1 31
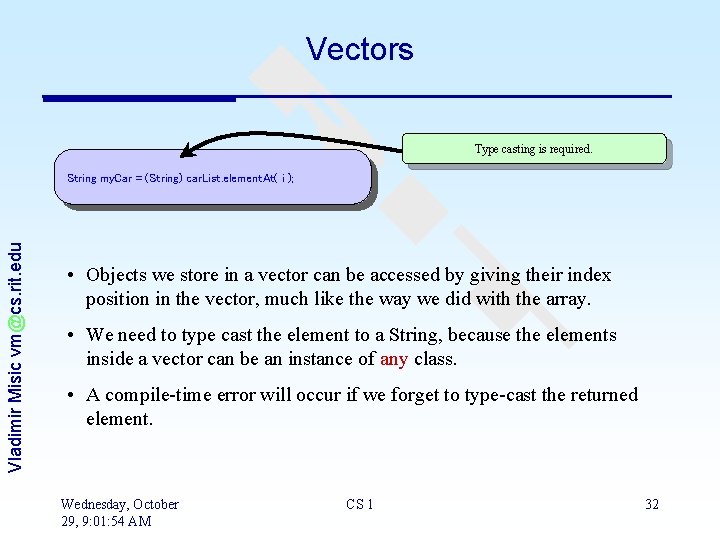
Vectors Type casting is required. Vladimir Misic vm@cs. rit. edu String my. Car = (String) car. List. element. At( i ); • Objects we store in a vector can be accessed by giving their index position in the vector, much like the way we did with the array. • We need to type cast the element to a String, because the elements inside a vector can be an instance of any class. • A compile-time error will occur if we forget to type-cast the returned element. Wednesday, October 29, 9: 01: 54 AM CS 1 32
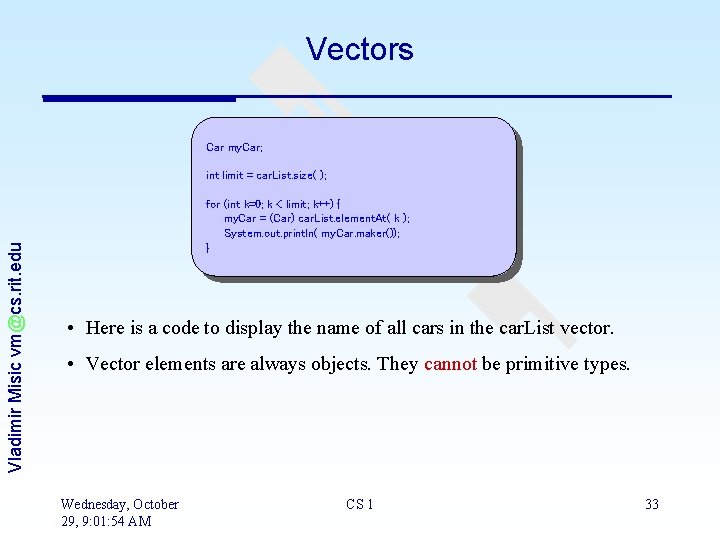
Vectors Car my. Car; Vladimir Misic vm@cs. rit. edu int limit = car. List. size( ); for (int k=0; k < limit; k++) { my. Car = (Car) car. List. element. At( k ); System. out. println( my. Car. maker()); } • Here is a code to display the name of all cars in the car. List vector. • Vector elements are always objects. They cannot be primitive types. Wednesday, October 29, 9: 01: 54 AM CS 1 33