Visual C 2005 IDE Enhancements Dan Fernandez C
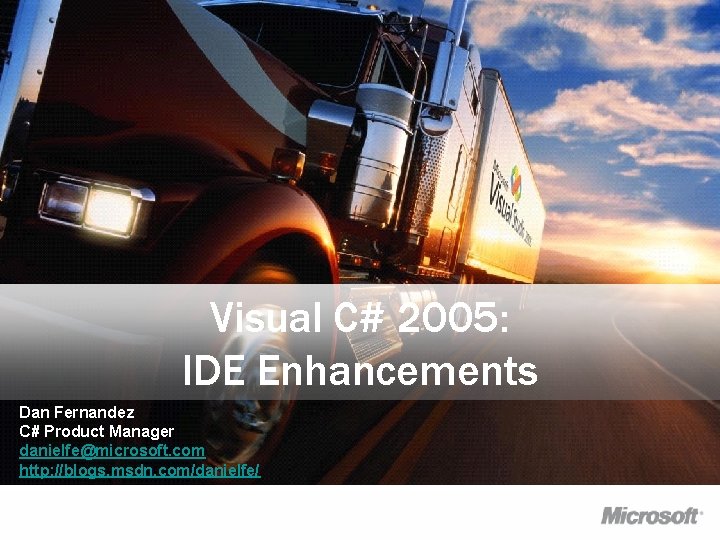
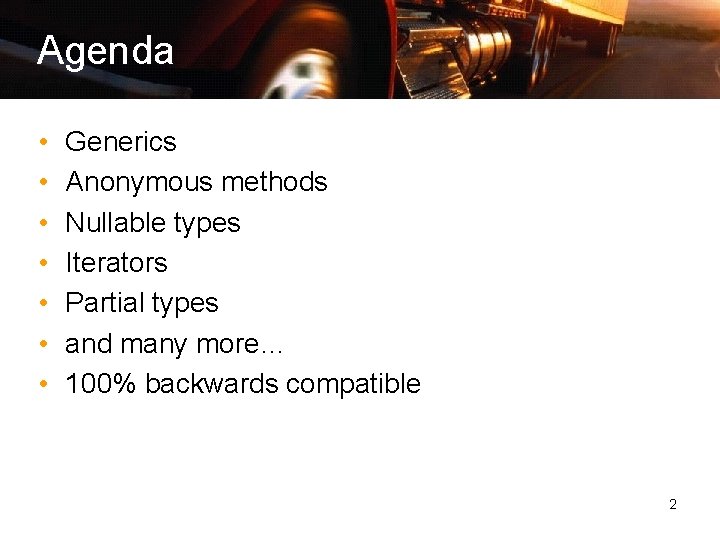
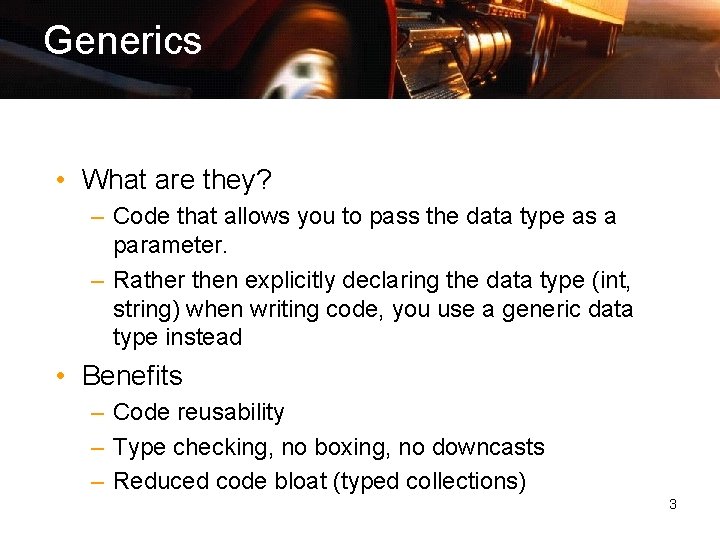
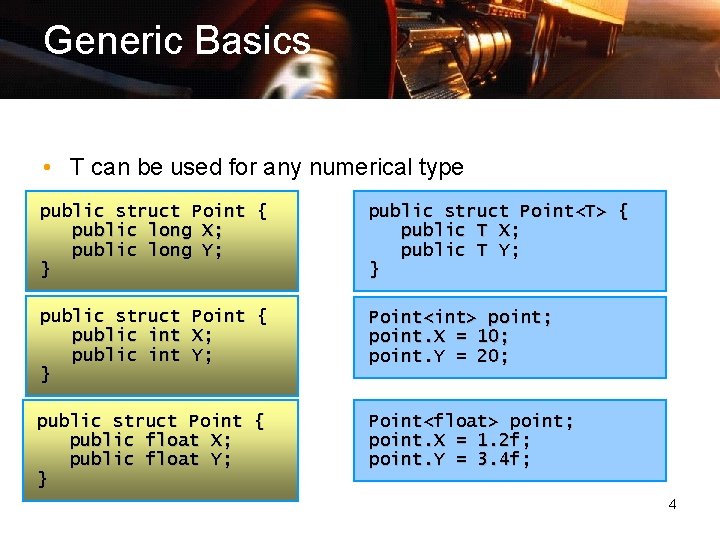
![Generics public class List<T> { private object[] T[] elements; private int count; public void Generics public class List<T> { private object[] T[] elements; private int count; public void](https://slidetodoc.com/presentation_image_h2/a591608569aaee5c2324891a2776bb88/image-5.jpg)
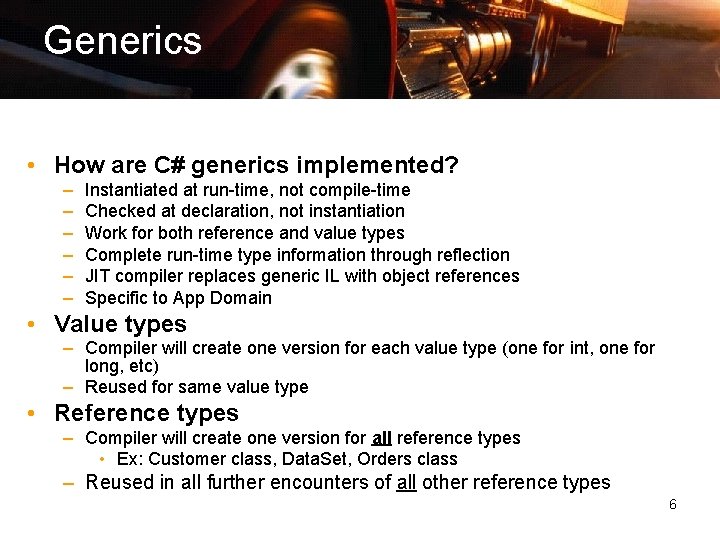
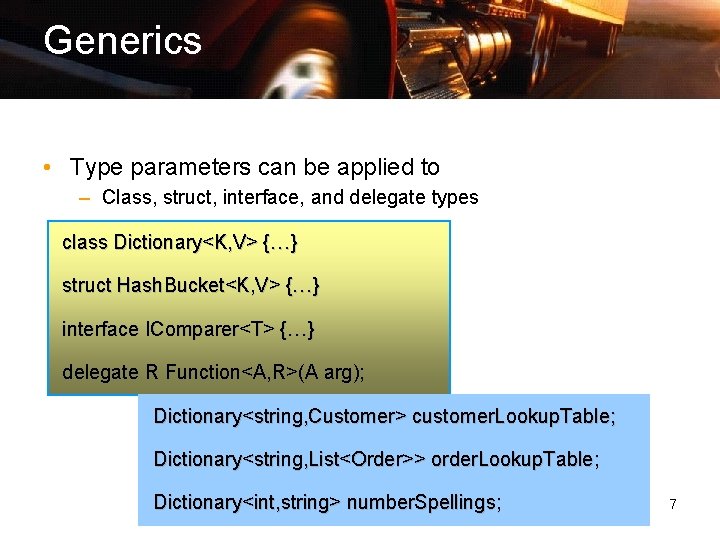
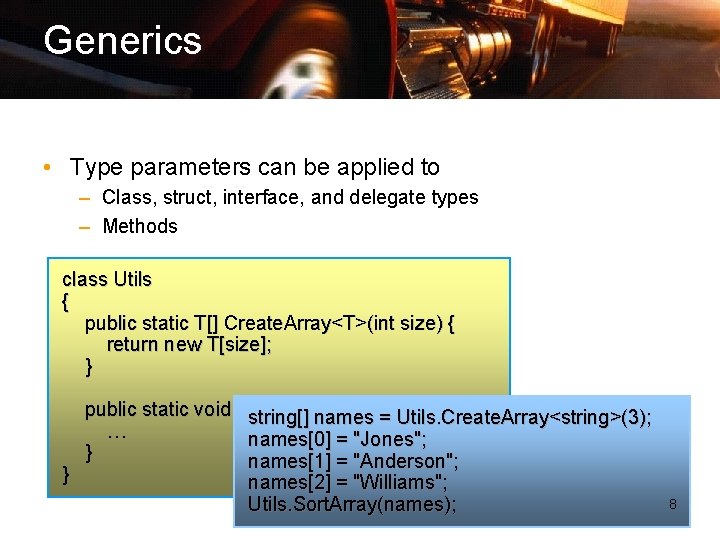
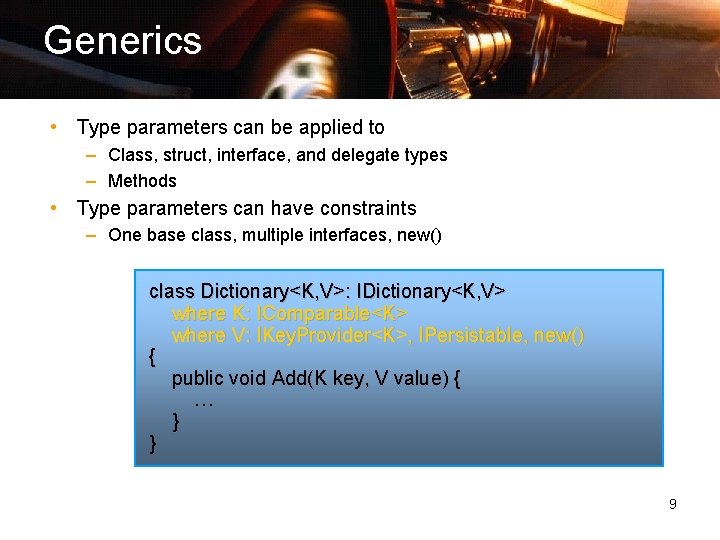
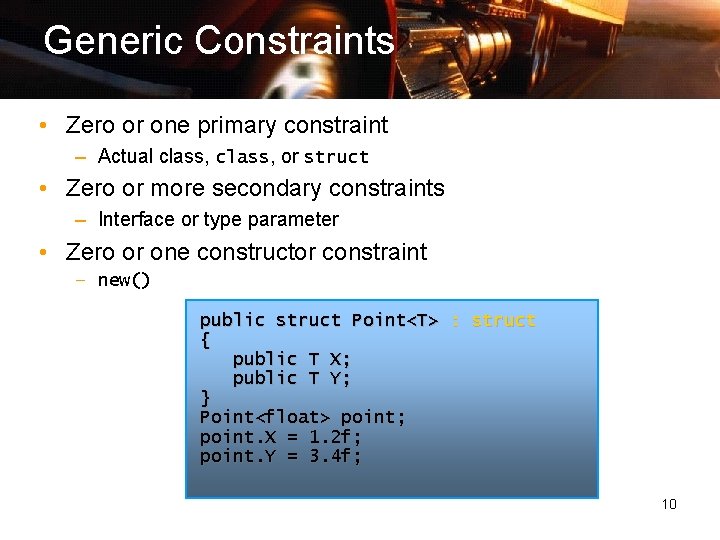
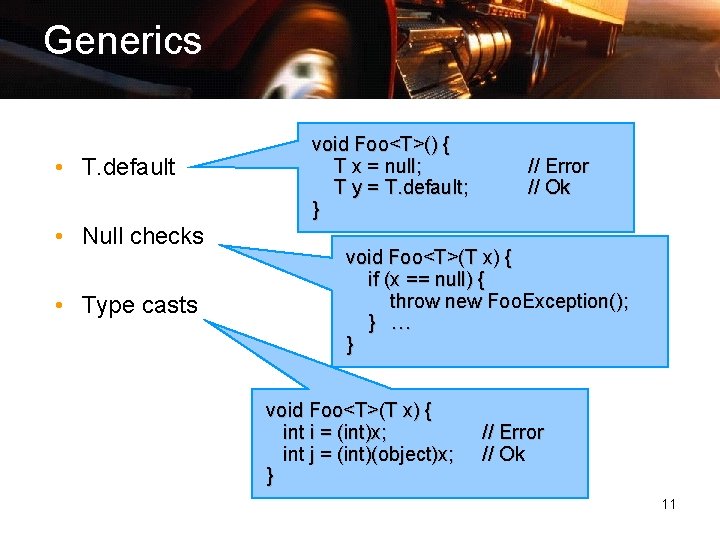
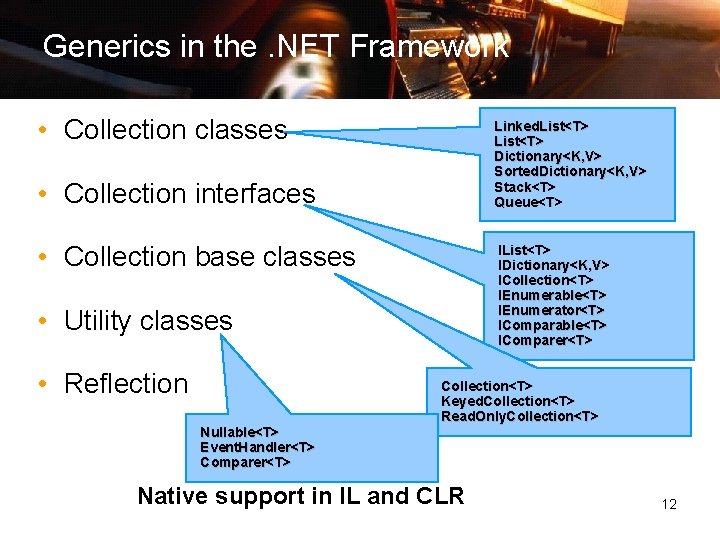
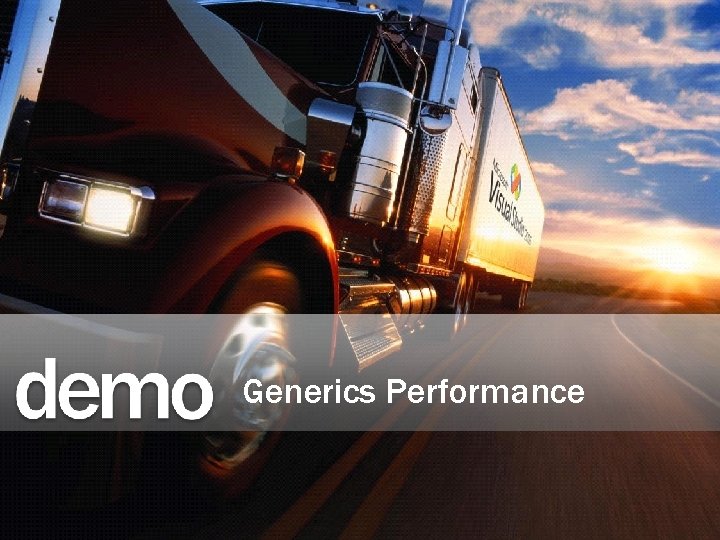
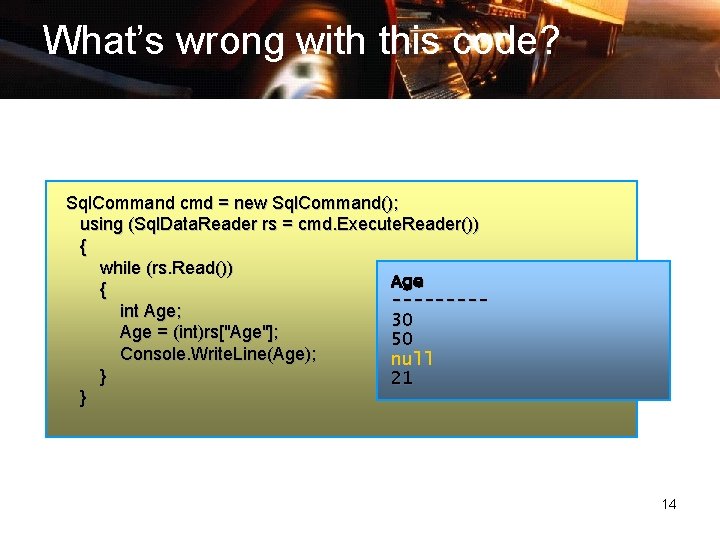
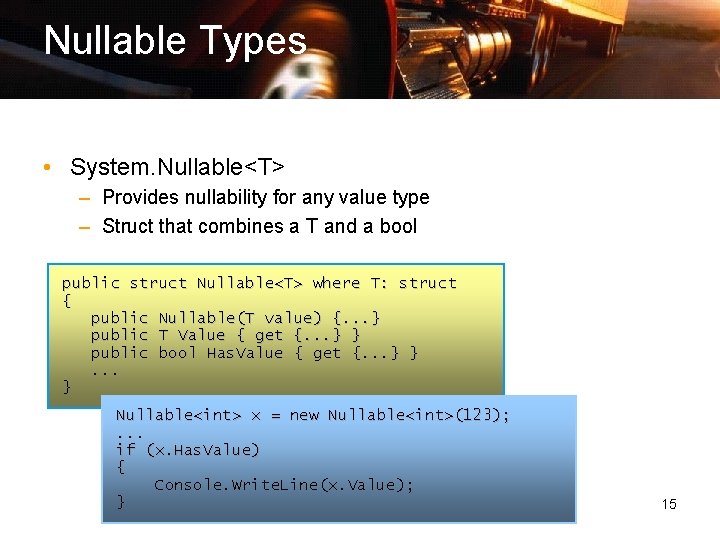
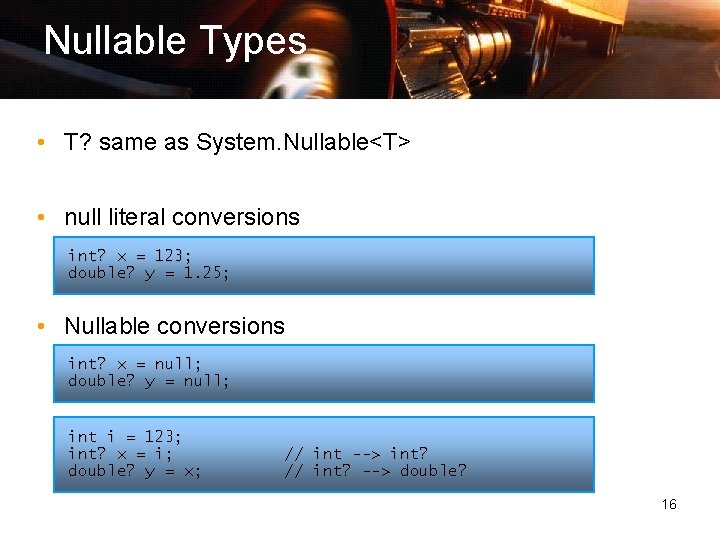
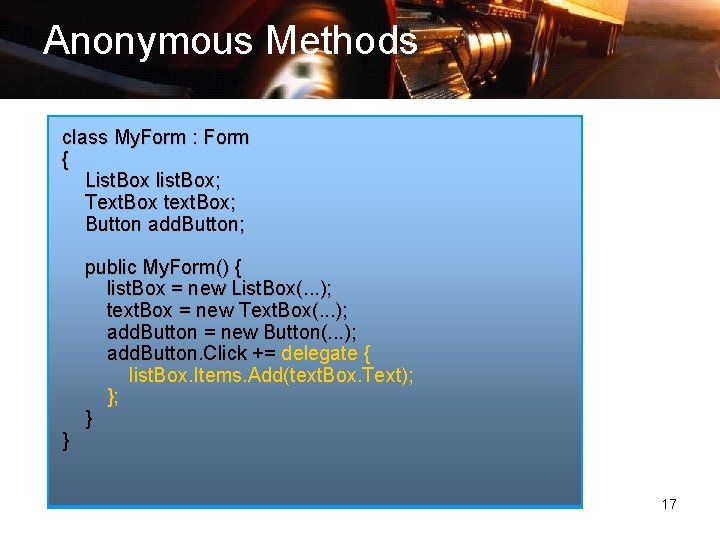
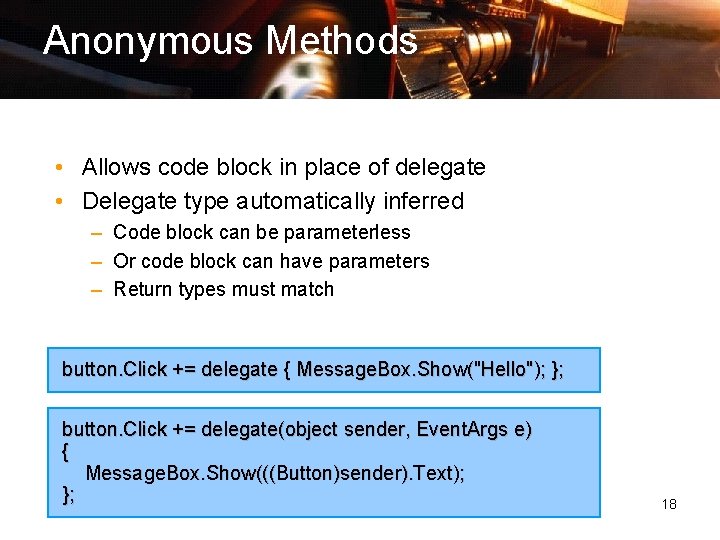
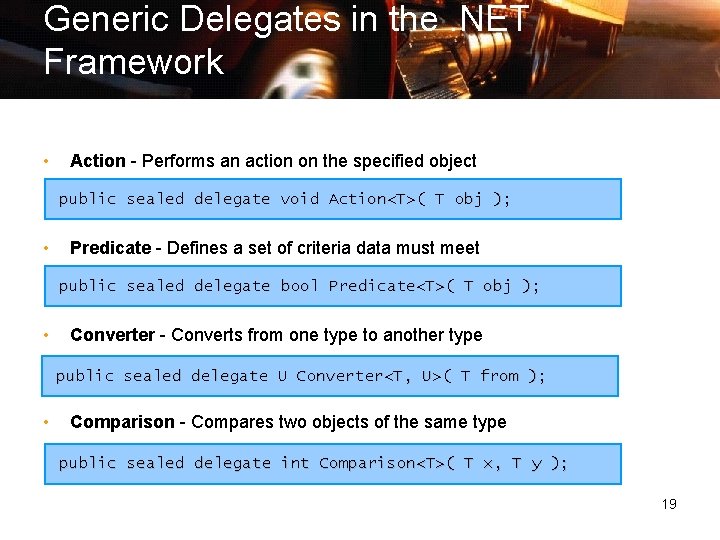
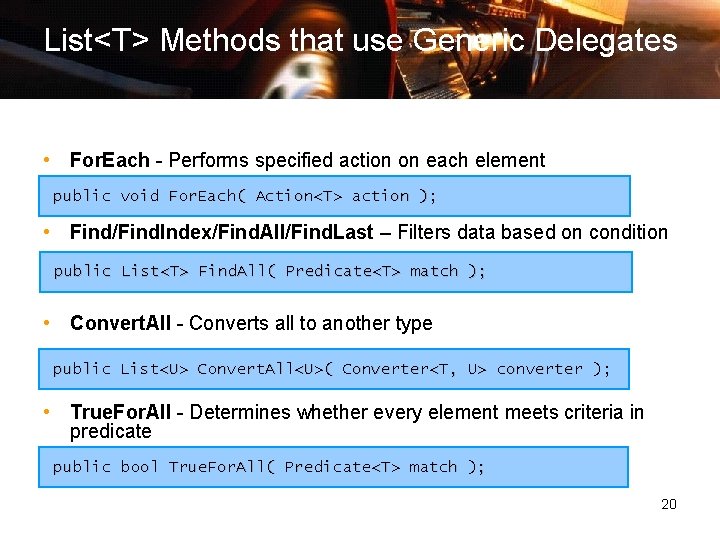
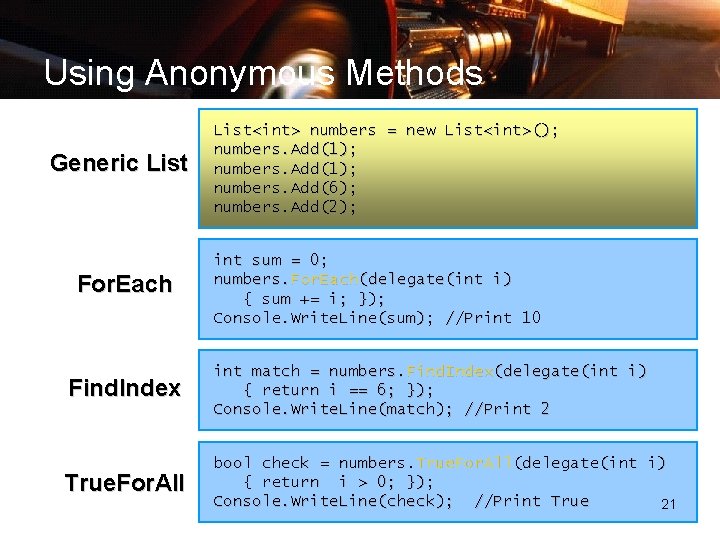
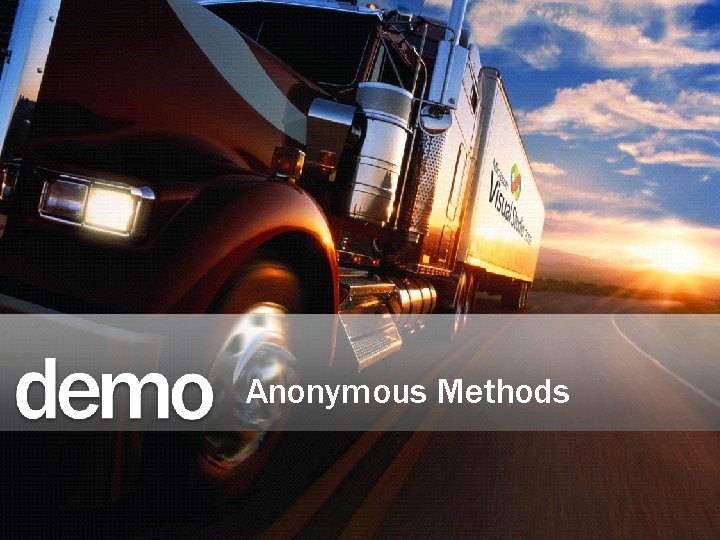
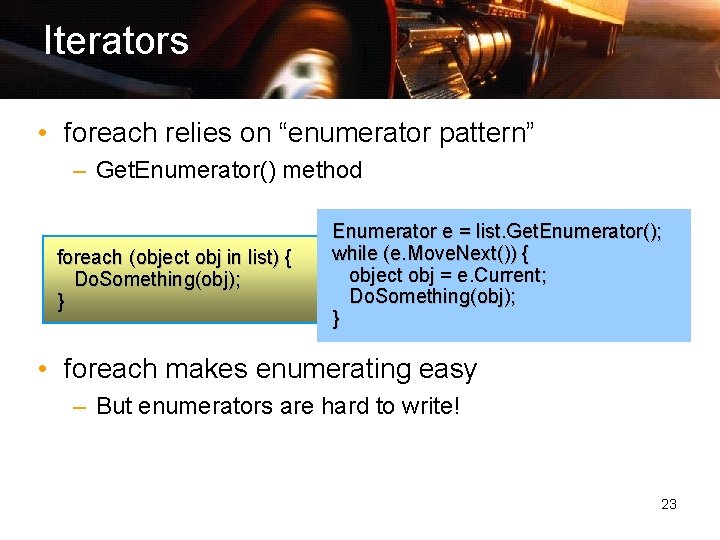
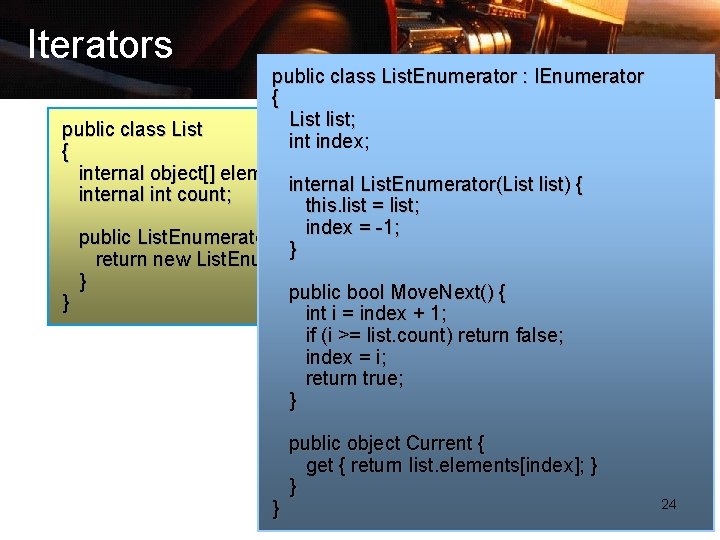
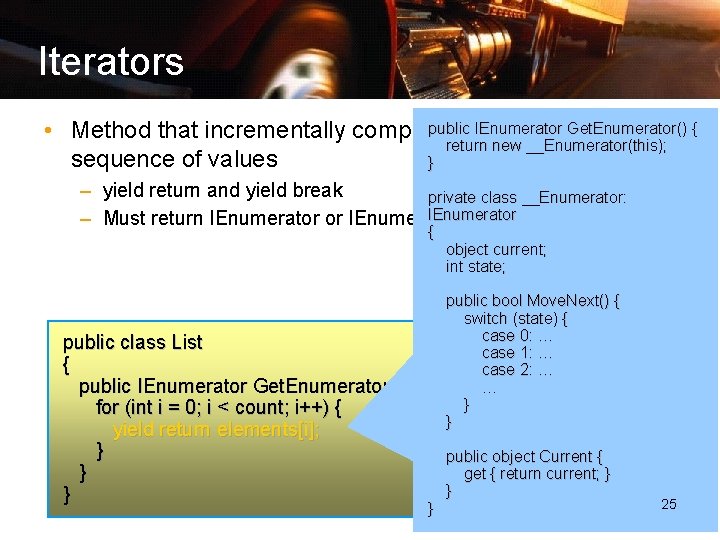
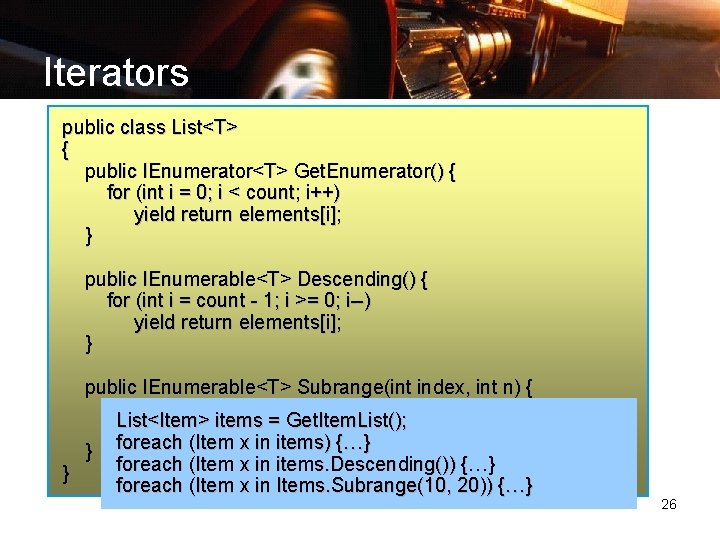
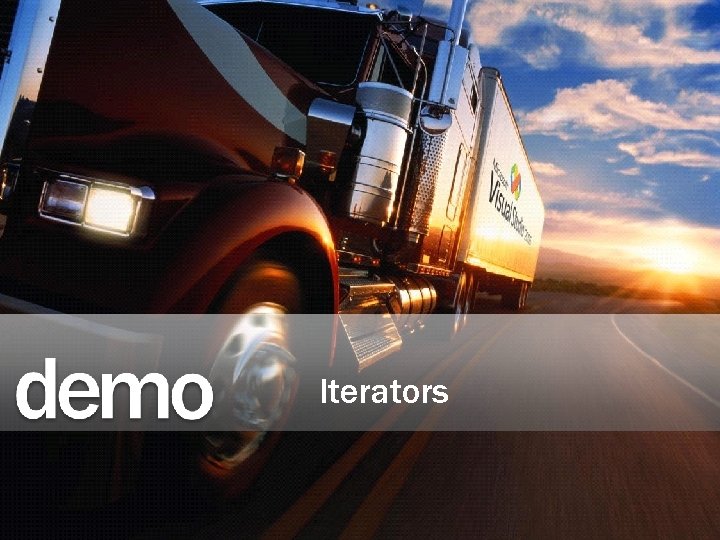
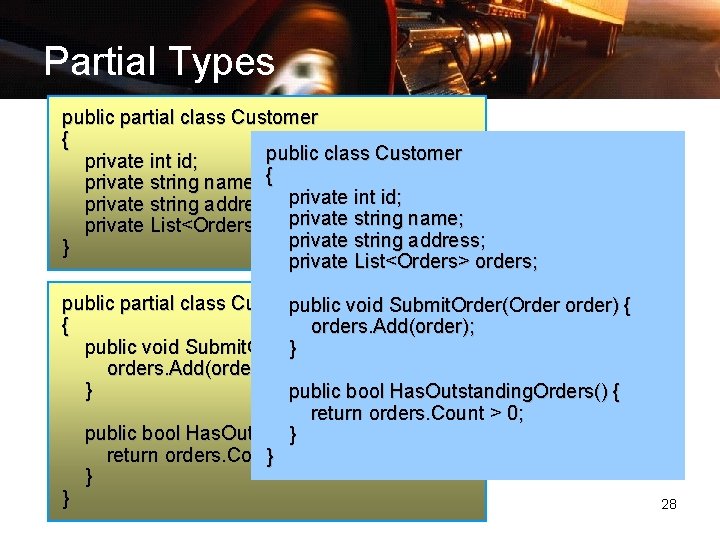
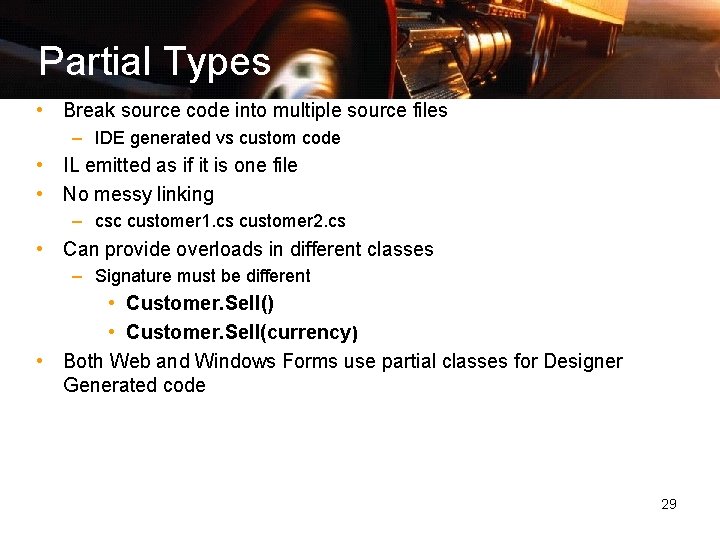
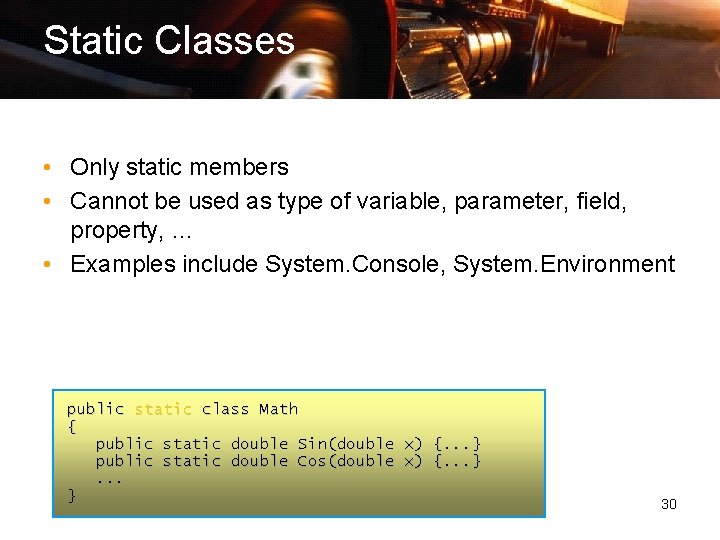
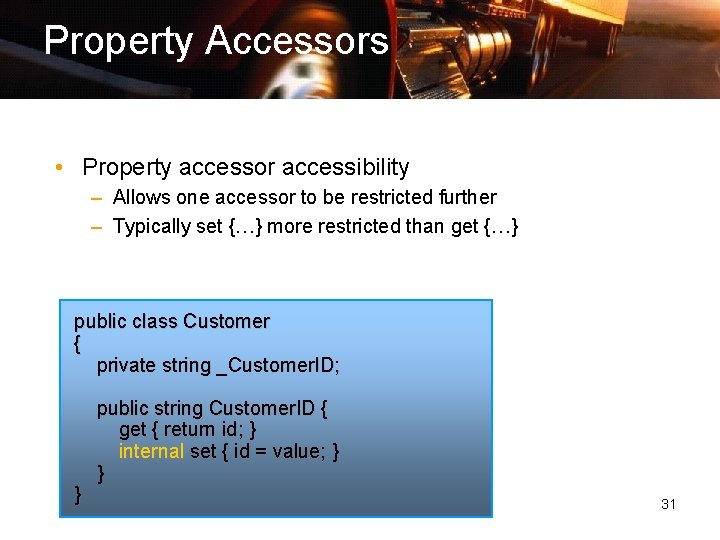
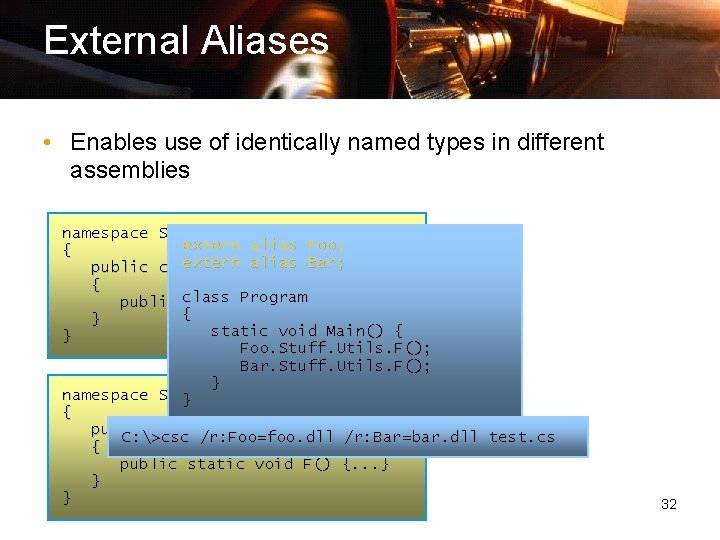
![Inline Warning Control • #pragma warning using System; class Program { [Obsolete] static void Inline Warning Control • #pragma warning using System; class Program { [Obsolete] static void](https://slidetodoc.com/presentation_image_h2/a591608569aaee5c2324891a2776bb88/image-33.jpg)
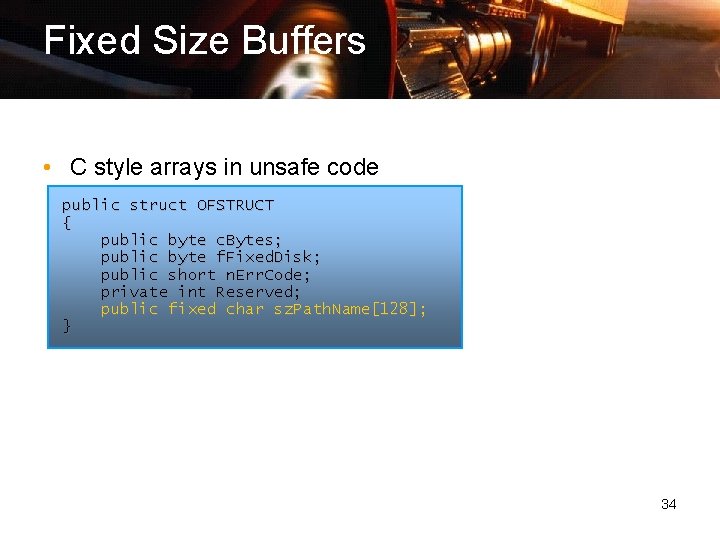
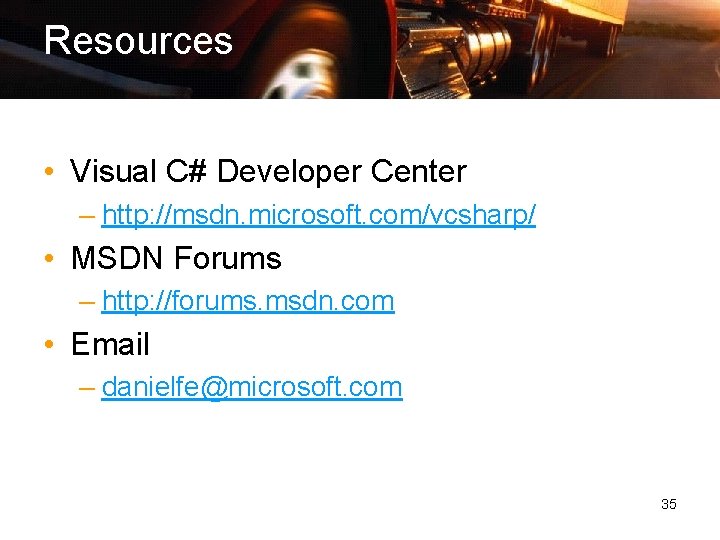
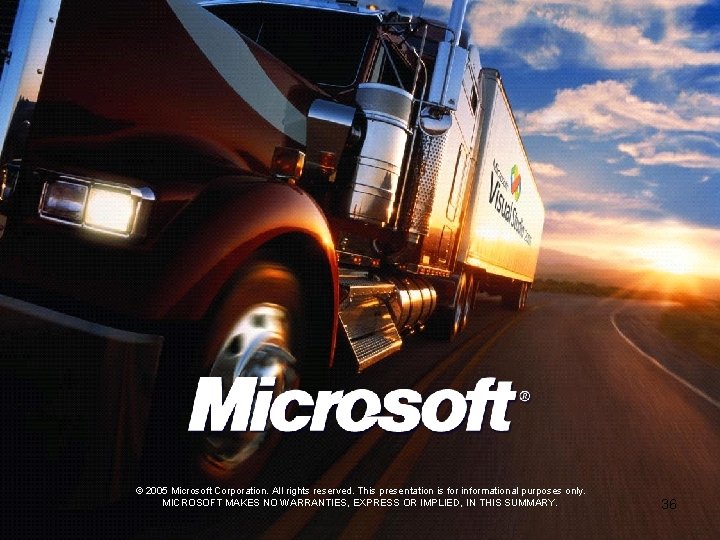
- Slides: 36
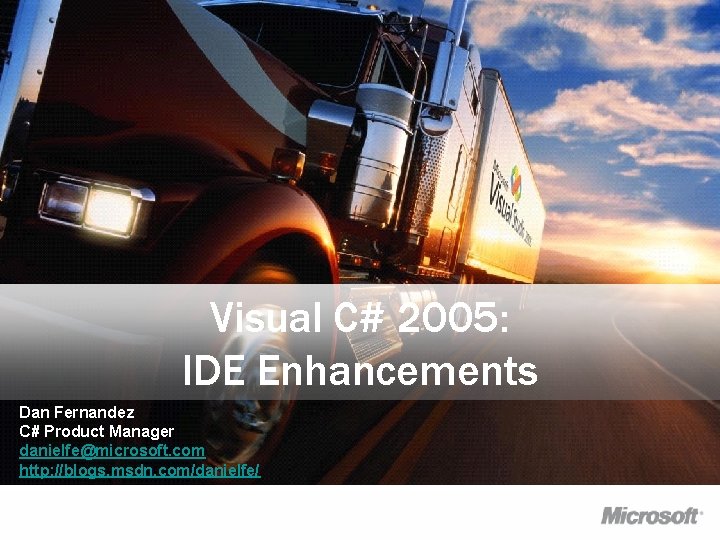
Visual C# 2005: IDE Enhancements Dan Fernandez C# Product Manager danielfe@microsoft. com http: //blogs. msdn. com/danielfe/
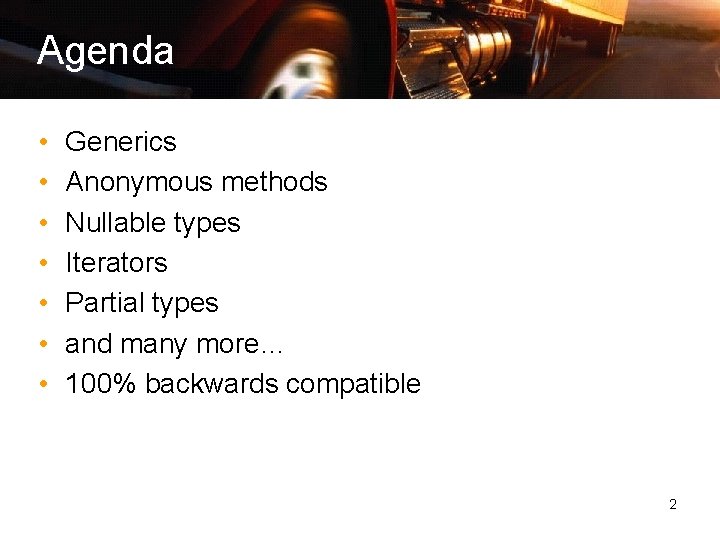
Agenda • • Generics Anonymous methods Nullable types Iterators Partial types and many more… 100% backwards compatible 2
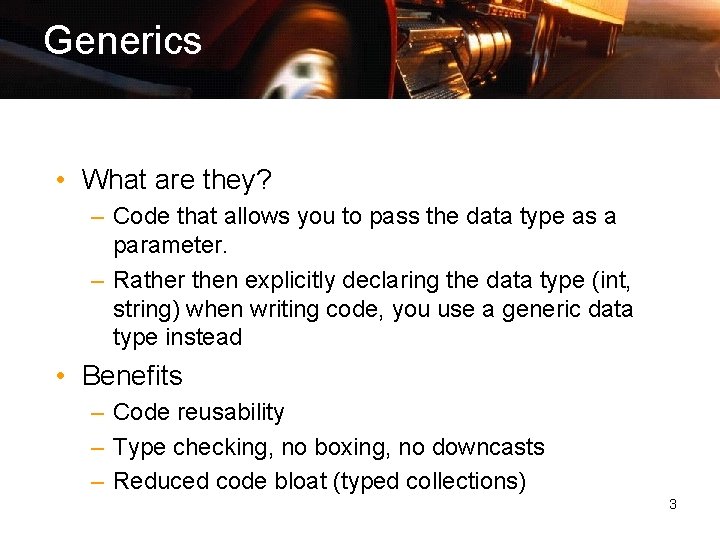
Generics • What are they? – Code that allows you to pass the data type as a parameter. – Rather then explicitly declaring the data type (int, string) when writing code, you use a generic data type instead • Benefits – Code reusability – Type checking, no boxing, no downcasts – Reduced code bloat (typed collections) 3
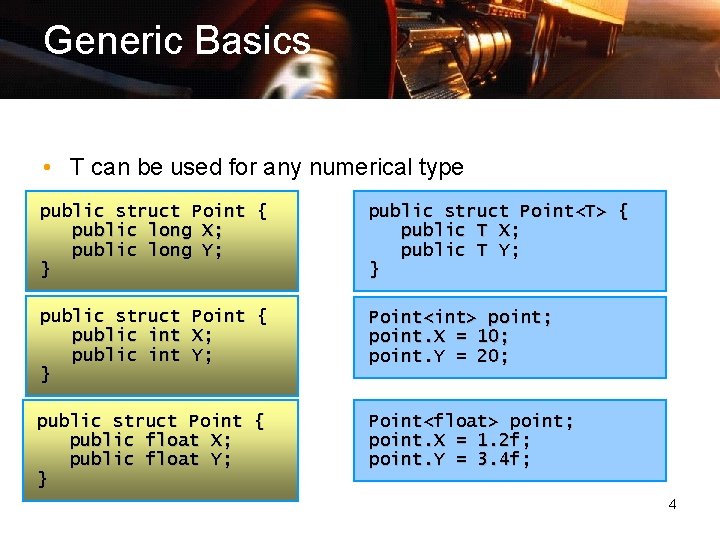
Generic Basics • T can be used for any numerical type public struct Point { public long X; public long Y; } public struct Point<T> { public T X; public T Y; } public struct Point { public int X; public int Y; } Point<int> point; point. X = 10; point. Y = 20; public struct Point { public float X; public float Y; } Point<float> point; point. X = 1. 2 f; point. Y = 3. 4 f; 4
![Generics public class ListT private object T elements private int count public void Generics public class List<T> { private object[] T[] elements; private int count; public void](https://slidetodoc.com/presentation_image_h2/a591608569aaee5c2324891a2776bb88/image-5.jpg)
Generics public class List<T> { private object[] T[] elements; private int count; public void Add(object Add(T element) { if (count == elements. Length) Resize(count * 2); elements[count++] = element; } } public object T this[int index] { get { return elements[index]; List<int. List int}> int. List = new= List(); new List<int>(); set { elements[index] = value; } } int. List. Add(1); // No Argument boxing is boxed int. List. Add(2); // No Argument boxing is boxed public int Count { int. List. Add("Three"); // Compile-time Should be an error get { return count; } } int i = int. List[0]; (int)int. List[0]; // No cast // Cast required 5
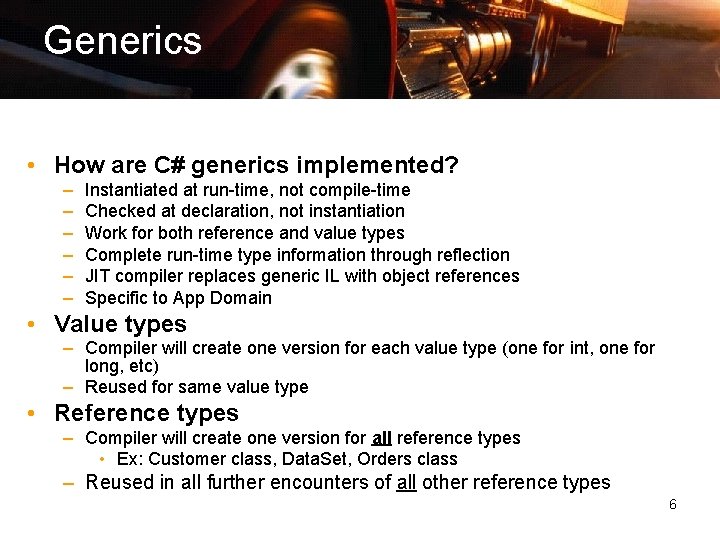
Generics • How are C# generics implemented? – – – Instantiated at run-time, not compile-time Checked at declaration, not instantiation Work for both reference and value types Complete run-time type information through reflection JIT compiler replaces generic IL with object references Specific to App Domain • Value types – Compiler will create one version for each value type (one for int, one for long, etc) – Reused for same value type • Reference types – Compiler will create one version for all reference types • Ex: Customer class, Data. Set, Orders class – Reused in all further encounters of all other reference types 6
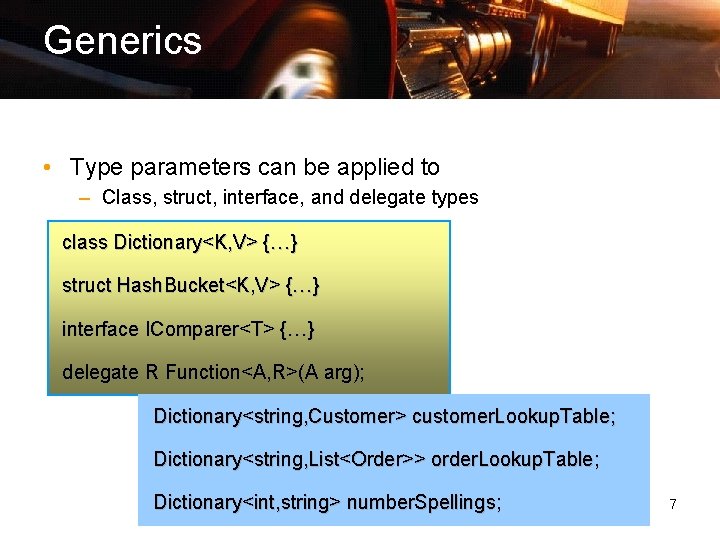
Generics • Type parameters can be applied to – Class, struct, interface, and delegate types class Dictionary<K, V> {…} struct Hash. Bucket<K, V> {…} interface IComparer<T> {…} delegate R Function<A, R>(A arg); Dictionary<string, Customer> customer. Lookup. Table; Dictionary<string, List<Order>> order. Lookup. Table; Dictionary<int, string> number. Spellings; 7
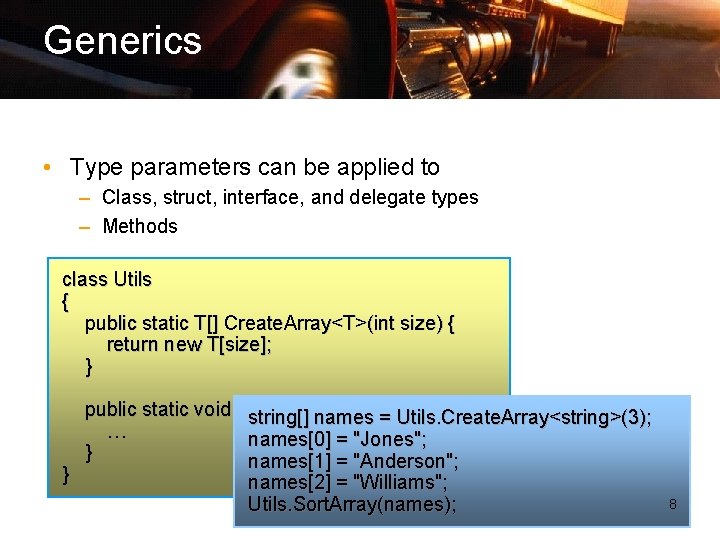
Generics • Type parameters can be applied to – Class, struct, interface, and delegate types – Methods class Utils { public static T[] Create. Array<T>(int size) { return new T[size]; } public static void Sort. Array <T>(T[]= array) { string[] names Utils. Create. Array <string>(3); … names[0] = "Jones"; } names[1] = "Anderson"; } names[2] = "Williams"; Utils. Sort. Array(names); 8
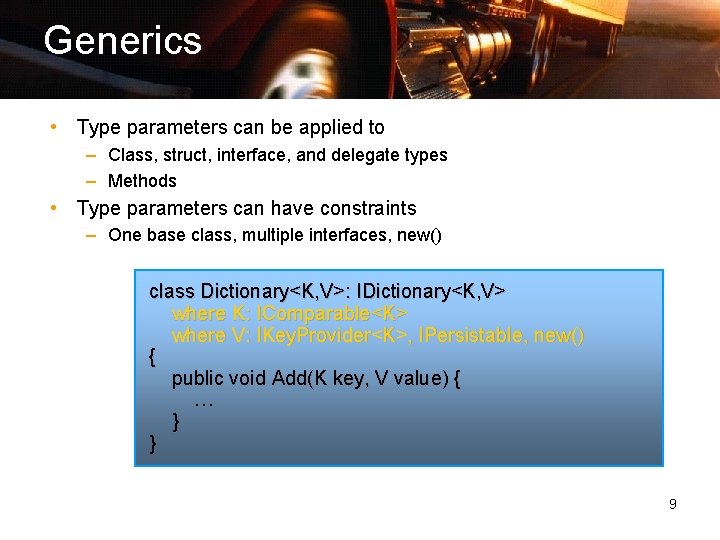
Generics • Type parameters can be applied to – Class, struct, interface, and delegate types – Methods • Type parameters can have constraints – One base class, multiple interfaces, new() class Dictionary<K, V>: Dictionary<K, V> where IDictionary K: IComparable <K, V> { where K: IComparable<K> public void where V: IKey. Provider Add(K key, <K>, V value) IPersistable { , new() { … public if (key. Compare. To(x ((void IComparable)key). Compare. To(x Add(K key, )V== value) 0) {…} { ) == 0) {…} … } } 9
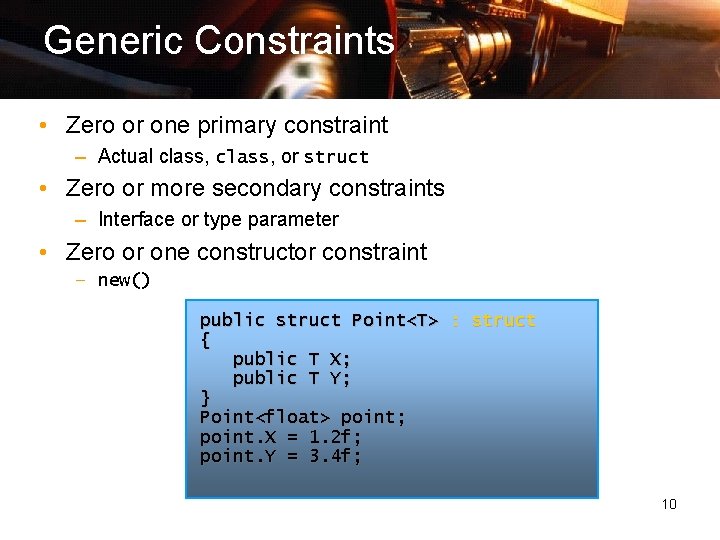
Generic Constraints • Zero or one primary constraint – Actual class, or struct • Zero or more secondary constraints – Interface or type parameter • Zero or one constructor constraint – new() public struct Point<T> : struct { public T X; public T Y; } Point<float> point; point. X = 1. 2 f; point. Y = 3. 4 f; 10
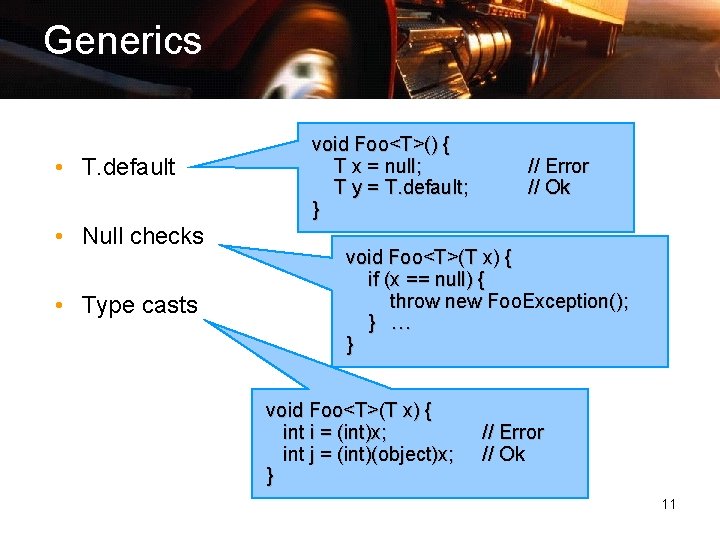
Generics • T. default • Null checks • Type casts void Foo<T>() { T x = null; T y = T. default; } // Error // Ok void Foo<T>(T x) { if (x == null) { throw new Foo. Exception(); } … } void Foo<T>(T x) { int i = (int)x; int j = (int)(object)x; } // Error // Ok 11
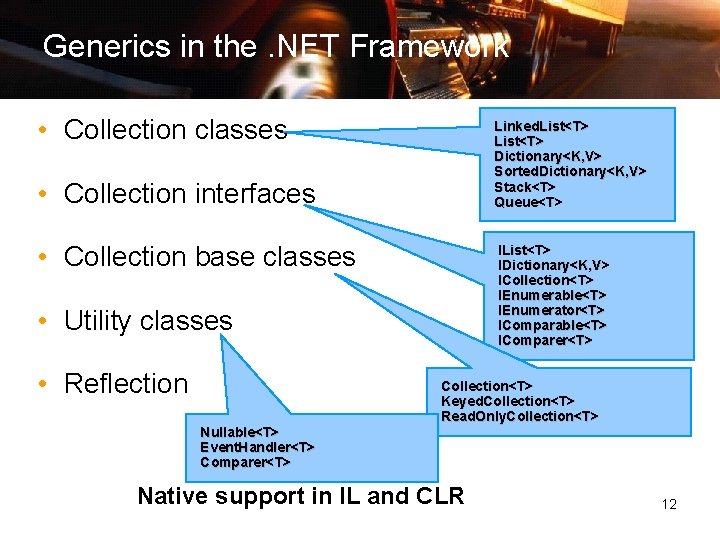
Generics in the. NET Framework • Collection classes Linked. List<T> Dictionary<K, V> Sorted. Dictionary<K, V> Stack<T> Queue<T> • Collection interfaces • Collection base classes IList<T> IDictionary<K, V> ICollection<T> IEnumerable<T> IEnumerator<T> IComparable<T> IComparer<T> • Utility classes • Reflection Nullable<T> Event. Handler<T> Comparer<T> Collection<T> Keyed. Collection<T> Read. Only. Collection<T> Native support in IL and CLR 12
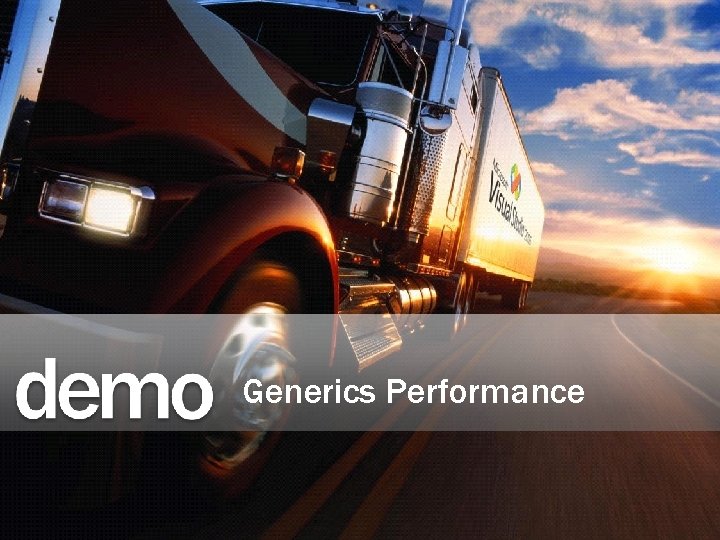
Generics Performance
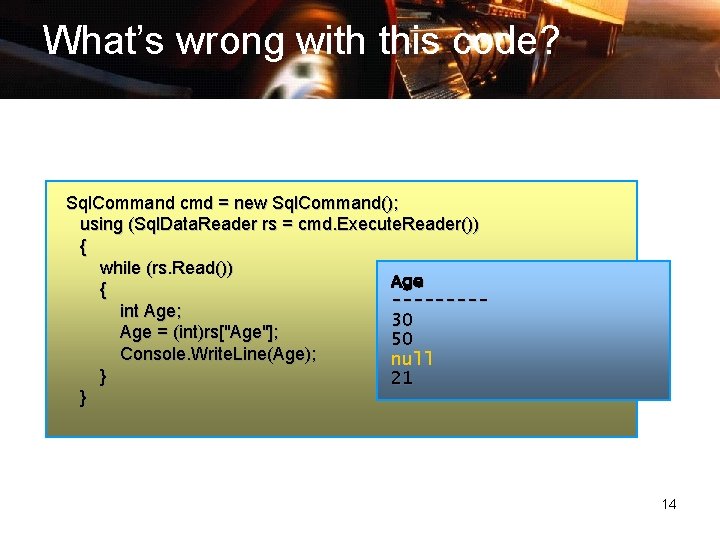
What’s wrong with this code? Sql. Command cmd = new Sql. Command(); using (Sql. Data. Reader rs = cmd. Execute. Reader()) { while (rs. Read()) Age { ----int Age; 30 Age = (int)rs["Age"]; 50 Console. Write. Line(Age); null } 21 } 14
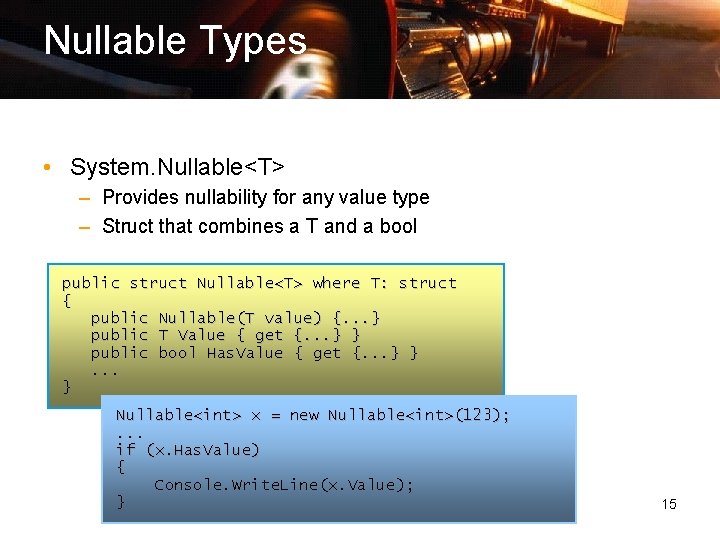
Nullable Types • System. Nullable<T> – Provides nullability for any value type – Struct that combines a T and a bool public struct Nullable<T> where T: struct { public Nullable(T value) {. . . } public T Value { get {. . . } } public bool Has. Value { get {. . . } }. . . } Nullable<int> x = new Nullable<int>(123); . . . if (x. Has. Value) { Console. Write. Line(x. Value); } 15
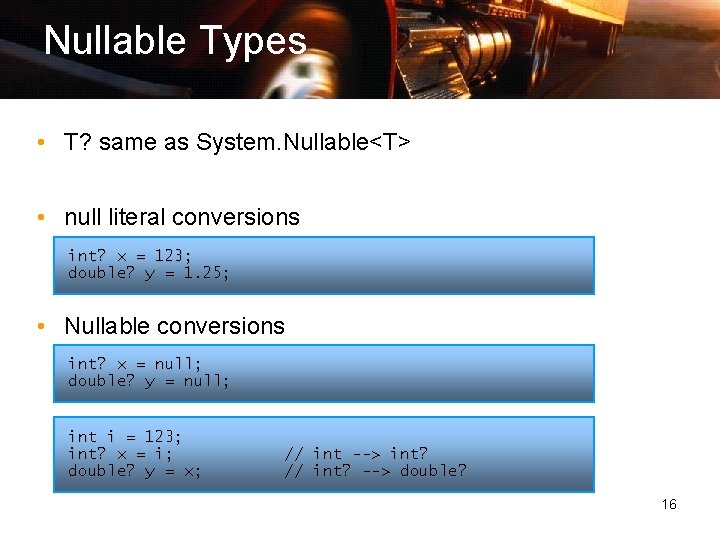
Nullable Types • T? same as System. Nullable<T> • null literal conversions int? x = 123; double? y = 1. 25; • Nullable conversions int? x = null; double? y = null; int i = 123; int? x = i; double? y = x; // int --> int? // int? --> double? 16
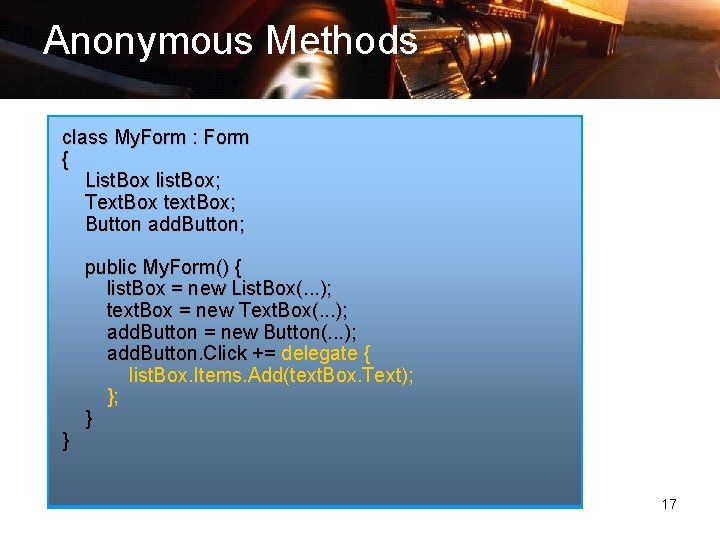
Anonymous Methods class My. Form : Form { List. Box list. Box; Text. Box text. Box; Button add. Button; public My. Form() { list. Box = new List. Box(. . . ); text. Box = new Text. Box(. . . ); add. Button = new Button(. . . ); add. Button. Click += delegate { new Event. Handler(Add. Click ); list. Box. Items. Add(text. Box. Text); } }; }void Add. Click(object sender, Event. Args e) { } list. Box. Items. Add(text. Box. Text); } } 17
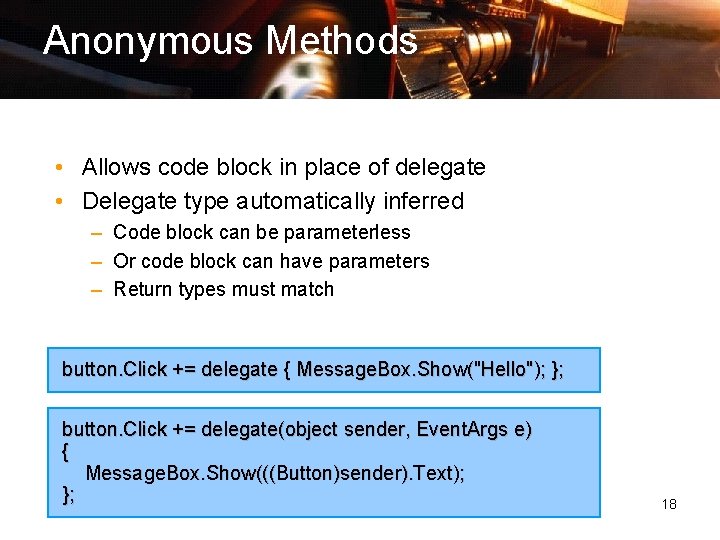
Anonymous Methods • Allows code block in place of delegate • Delegate type automatically inferred – Code block can be parameterless – Or code block can have parameters – Return types must match button. Click += delegate { Message. Box. Show("Hello"); }; button. Click += delegate(object sender, Event. Args e) { Message. Box. Show(((Button)sender). Text); }; 18
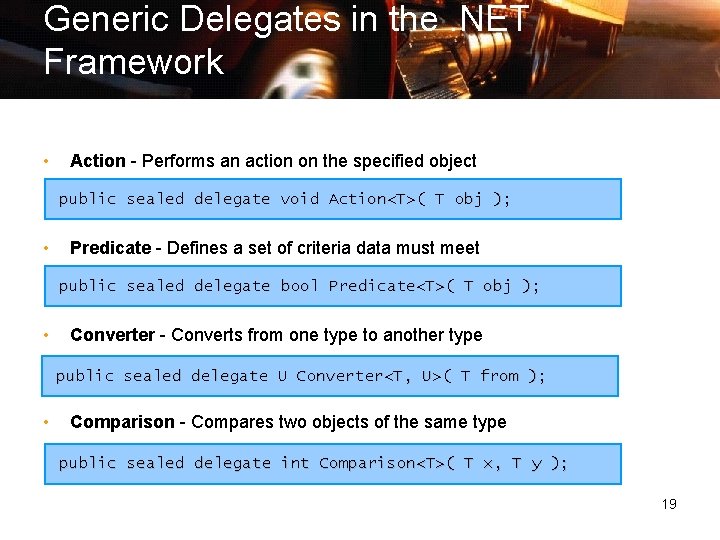
Generic Delegates in the. NET Framework • Action - Performs an action on the specified object public sealed delegate void Action<T>( T obj ); • Predicate - Defines a set of criteria data must meet public sealed delegate bool Predicate<T>( T obj ); • Converter - Converts from one type to another type public sealed delegate U Converter<T, U>( T from ); • Comparison - Compares two objects of the same type public sealed delegate int Comparison<T>( T x, T y ); 19
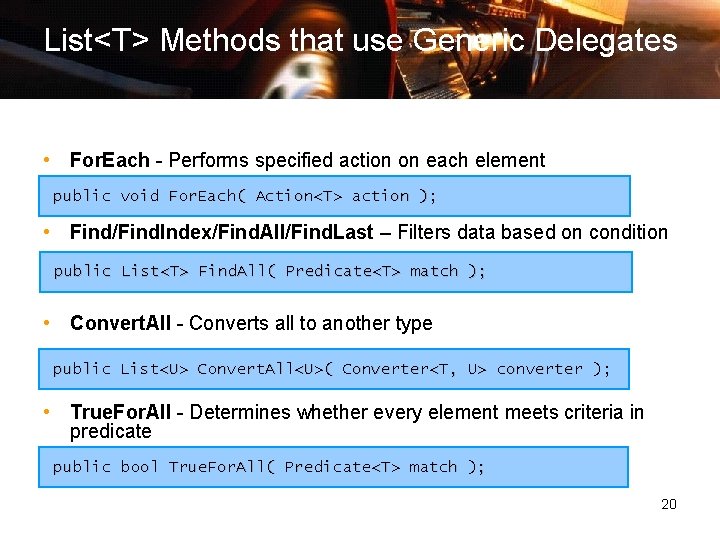
List<T> Methods that use Generic Delegates • For. Each - Performs specified action on each element public void For. Each( Action<T> action ); • Find/Find. Index/Find. All/Find. Last – Filters data based on condition public List<T> Find. All( Predicate<T> match ); • Convert. All - Converts all to another type public List<U> Convert. All<U>( Converter<T, U> converter ); • True. For. All - Determines whether every element meets criteria in predicate public bool True. For. All( Predicate<T> match ); 20
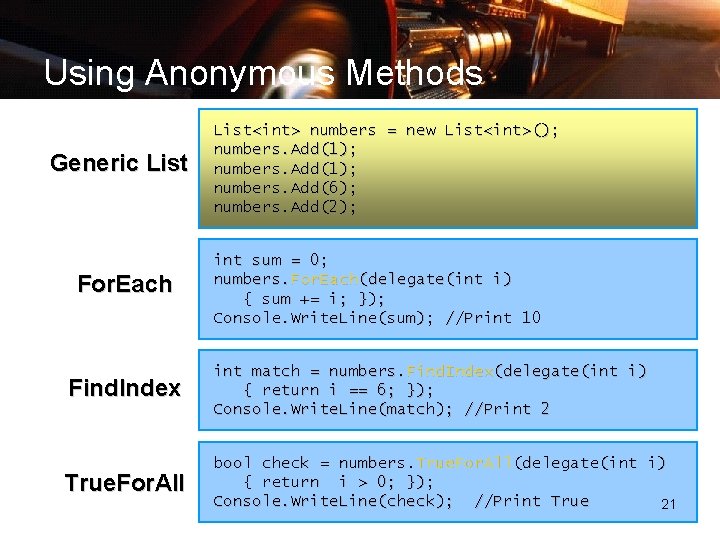
Using Anonymous Methods Generic List For. Each List<int> numbers = new List<int>(); numbers. Add(1); numbers. Add(6); numbers. Add(2); int sum = 0; numbers. For. Each(delegate(int i) { sum += i; }); Console. Write. Line(sum); //Print 10 Find. Index int match = numbers. Find. Index(delegate(int i) { return i == 6; }); Console. Write. Line(match); //Print 2 True. For. All bool check = numbers. True. For. All(delegate(int i) { return i > 0; }); Console. Write. Line(check); //Print True 21
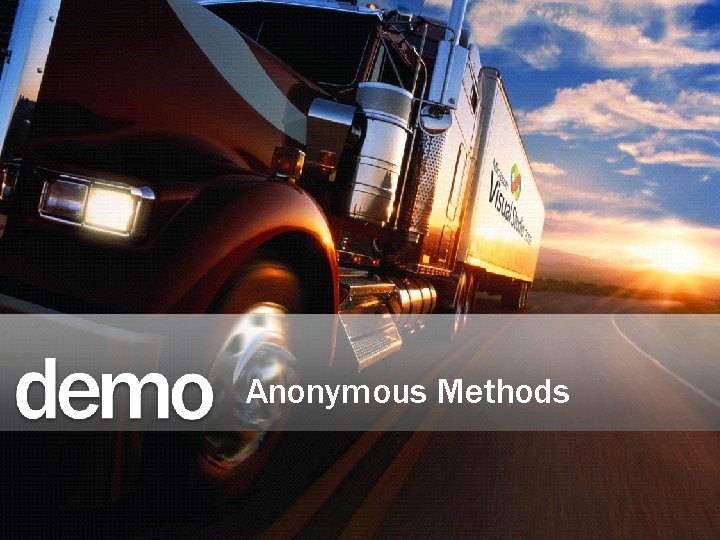
Anonymous Methods
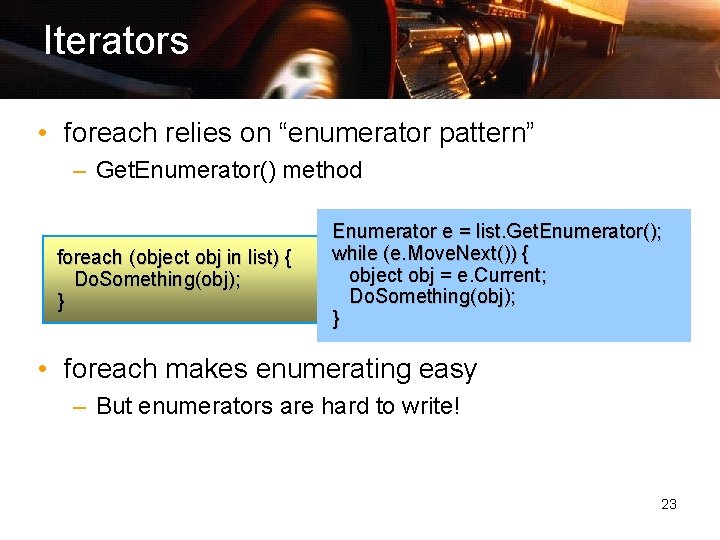
Iterators • foreach relies on “enumerator pattern” – Get. Enumerator() method foreach (object obj in list) { Do. Something(obj); } Enumerator e = list. Get. Enumerator(); while (e. Move. Next()) { object obj = e. Current; Do. Something(obj); } • foreach makes enumerating easy – But enumerators are hard to write! 23
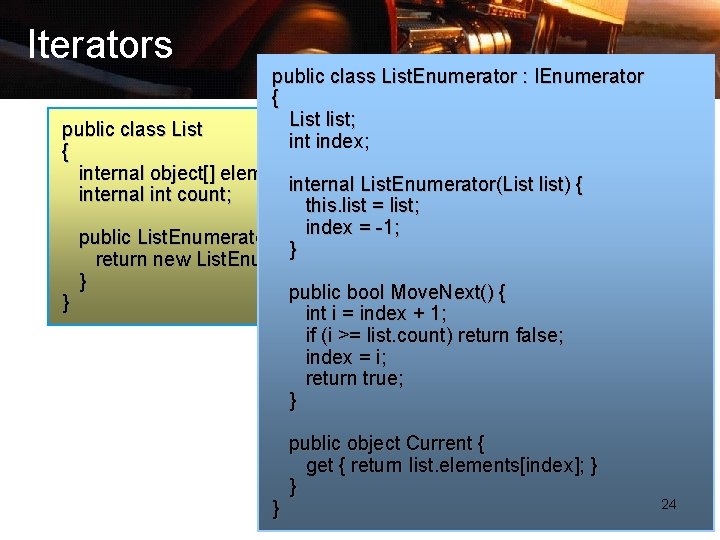
Iterators public class List. Enumerator : IEnumerator { List list; int index; public class List { internal object[] elements; internal List. Enumerator(List list) { internal int count; this. list = list; index = -1; public List. Enumerator Get. Enumerator() { } return new List. Enumerator(this); } public bool Move. Next() { } int i = index + 1; if (i >= list. count) return false; index = i; return true; } } public object Current { get { return list. elements[index]; } } 24
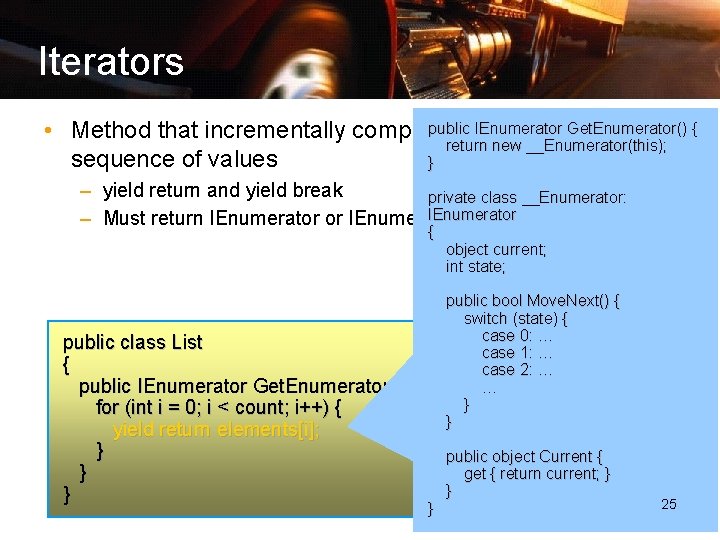
Iterators publicand IEnumerator Get. Enumerator () { • Method that incrementally computes returns a return new __Enumerator(this); sequence of values } – yield return and yield break private class __Enumerator: IEnumerator – Must return IEnumerator or IEnumerable { public class List { public IEnumerator Get. Enumerator() { for (int i = 0; i < count; i++) { yield return elements[i]; } } } object current; int state; public bool Move. Next() { switch (state) { case 0: … case 1: … case 2: … … } } } public object Current { get { return current; } } 25
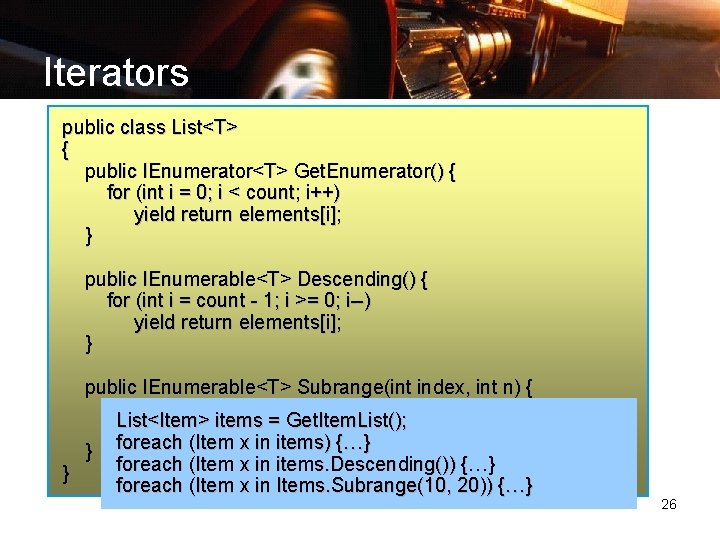
Iterators public class List<T> { public IEnumerator<T> Get. Enumerator() { for (int i = 0; i < count; i++) yield return elements[i]; } public IEnumerable<T> Descending() { for (int i = count - 1; i >= 0; i--) yield return elements[i]; } public IEnumerable<T> Subrange(int index, int n) { for (int i = 0; i < n; i++) List<Item> items = Get. Item. List+(); yield return elements[index i]; foreach (Item x in items) {…} } foreach (Item x in items. Descending()) {…} } foreach (Item x in Items. Subrange(10, 20)) {…} 26
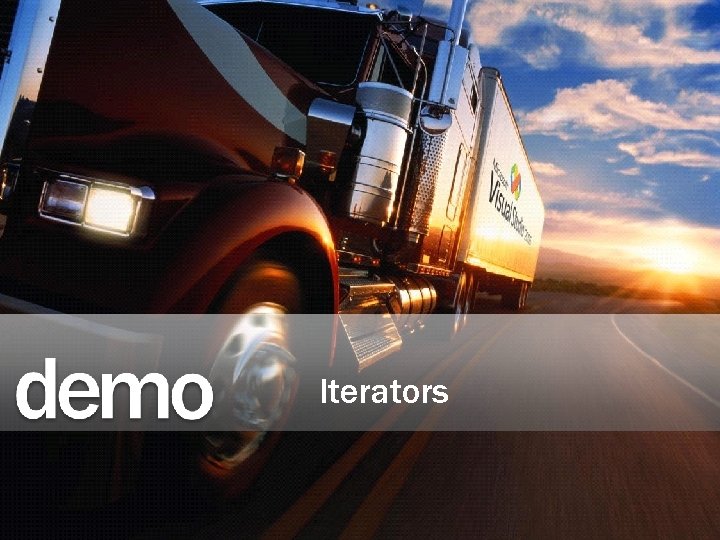
Iterators
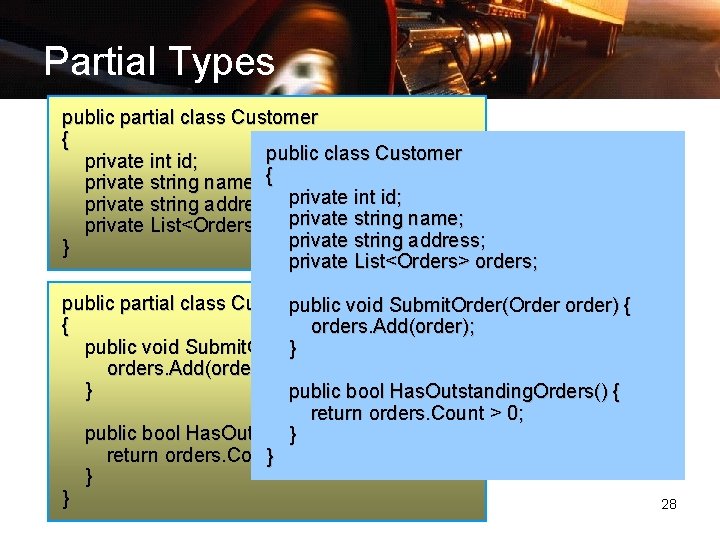
Partial Types public partial class Customer { public class Customer private int id; private string name; { private string address; private int id; private List<Orders> orders ; string name; private string address; } private List<Orders> orders; public partial class Customer public void Submit. Order(Order order) { { orders. Add(order); public void Submit. Order(Order order) { } orders. Add(order); } public bool Has. Outstanding. Orders() { return orders. Count > 0; public bool Has. Outstanding. Orders () { } return orders. Count } > 0; } } 28
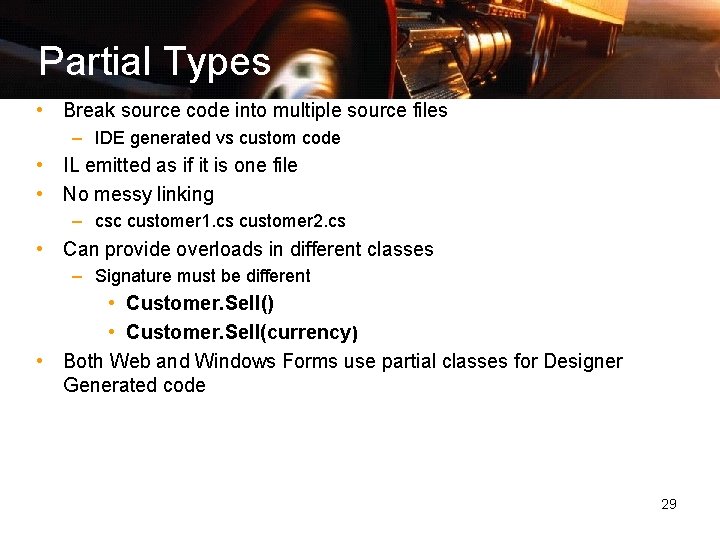
Partial Types • Break source code into multiple source files – IDE generated vs custom code • IL emitted as if it is one file • No messy linking – csc customer 1. cs customer 2. cs • Can provide overloads in different classes – Signature must be different • Customer. Sell() • Customer. Sell(currency) • Both Web and Windows Forms use partial classes for Designer Generated code 29
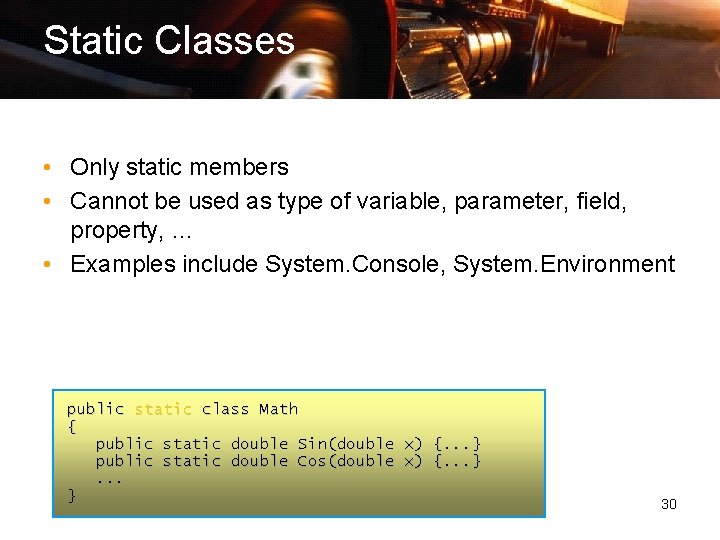
Static Classes • Only static members • Cannot be used as type of variable, parameter, field, property, … • Examples include System. Console, System. Environment public static class Math { public static double Sin(double x) {. . . } public static double Cos(double x) {. . . } 30
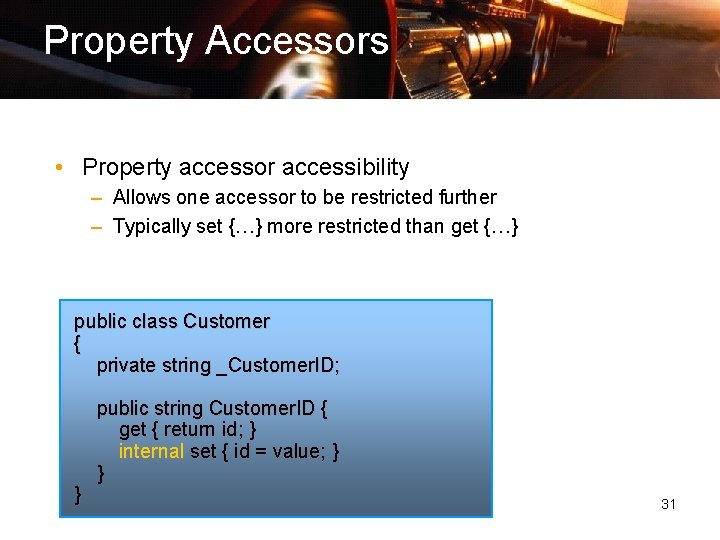
Property Accessors • Property accessor accessibility – Allows one accessor to be restricted further – Typically set {…} more restricted than get {…} public class Customer { private string _Customer. ID; } public string Customer. ID { get { return id; } internal set { id = value; } } 31
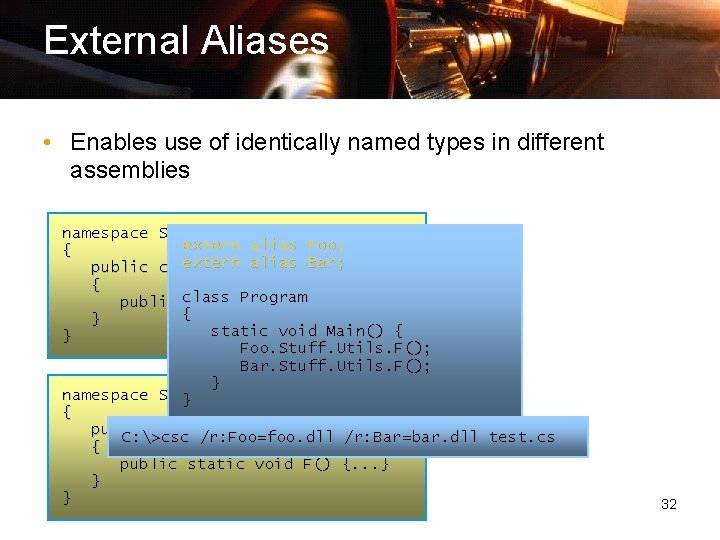
External Aliases • Enables use of identically named types in different assemblies namespace Stuff foo. dll extern alias Foo; { extern alias Bar; public class Utils { public class static. Program void F() {. . . } { } static void Main() { } Foo. Stuff. Utils. F(); Bar. Stuff. Utils. F(); } namespace Stuff bar. dll } { public Utils=foo. dll /r: Bar=bar. dll test. cs C: >class csc /r: Foo { public static void F() {. . . } } } 32
![Inline Warning Control pragma warning using System class Program Obsolete static void Inline Warning Control • #pragma warning using System; class Program { [Obsolete] static void](https://slidetodoc.com/presentation_image_h2/a591608569aaee5c2324891a2776bb88/image-33.jpg)
Inline Warning Control • #pragma warning using System; class Program { [Obsolete] static void Foo() {} static void Main() { #pragma warning disable 612 Foo(); #pragma warning restore 612 } } 33
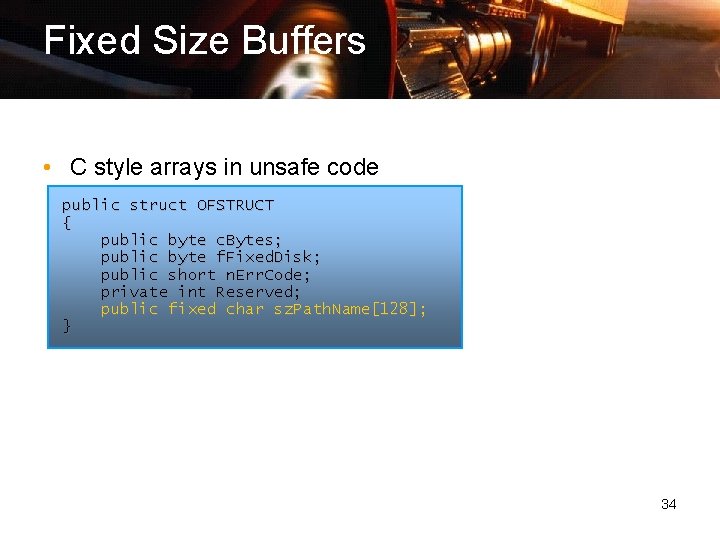
Fixed Size Buffers • C style arrays in unsafe code public struct OFSTRUCT { public byte c. Bytes; public byte f. Fixed. Disk; public short n. Err. Code; private int Reserved; public fixed char sz. Path. Name[128]; } 34
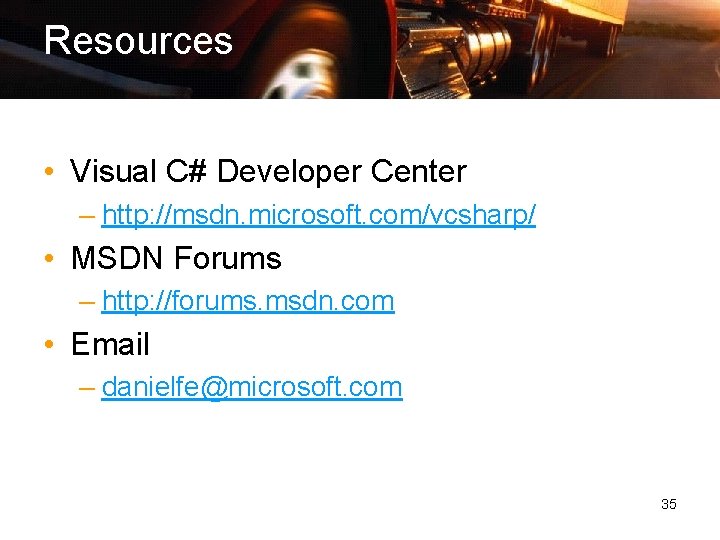
Resources • Visual C# Developer Center – http: //msdn. microsoft. com/vcsharp/ • MSDN Forums – http: //forums. msdn. com • Email – danielfe@microsoft. com 35
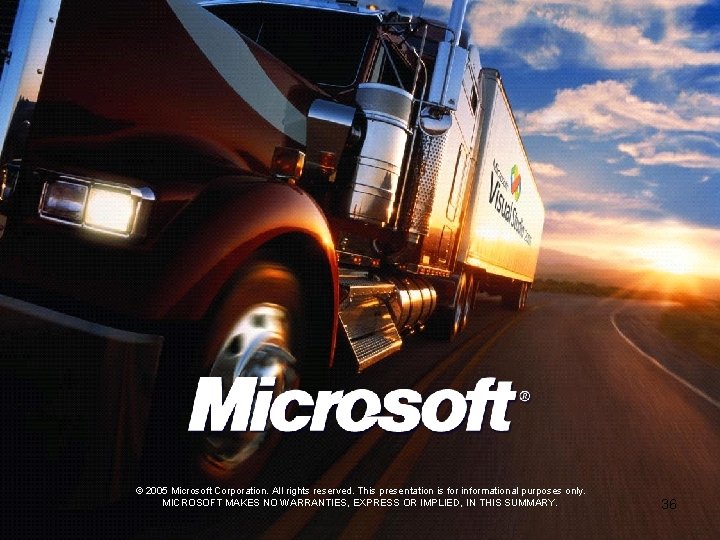
© 2005 Microsoft Corporation. All rights reserved. This presentation is for informational purposes only. MICROSOFT MAKES NO WARRANTIES, EXPRESS OR IMPLIED, IN THIS SUMMARY. 36
Bagaimana anda mendefinisikan ide-ide melalui outline
Apa itu ide pokok
Visual studio 2010 vb express
Contoh bentuk visualisasi statis adalah …
Visual studio 2010 express
Visual studio 2005 tools for office
Visual studio 2010 express
Visual studio 2005 team suite
Vslang
Visual studio 2005
Msdn subscription levels
Visual studio 2005
Visual studio 2005 team
Light cured gel polish may be used on
What is the chemistry behind ml/pp and how does it work?
Advertising vs promotion
What is the chemistry behind monomer liquid and polymer
Francisco fernandez md
Joaquín sagarra fernández-prida
Thalia fernandez
Dr fernandez psychiatrist
Daniel fernández de landa
Isabel fernandez garcia
Candela quiroga fernandez
Ime rudy fernandez
Roberto fernandez md
Sonia fernandez sanchez
Jose tejada
Anthony fernandez
Arman autoportrait robot
Juan manuel martos fernandez
Pedro y adolfo gómez fernández
Asi se siente mexico letra
Javier riancho zarrabeitia
Ernesto fernandez arias
Bolleria fernandez
Antropología y trabajo social