Stacks and Queues Processing Sequences of Elements Soft
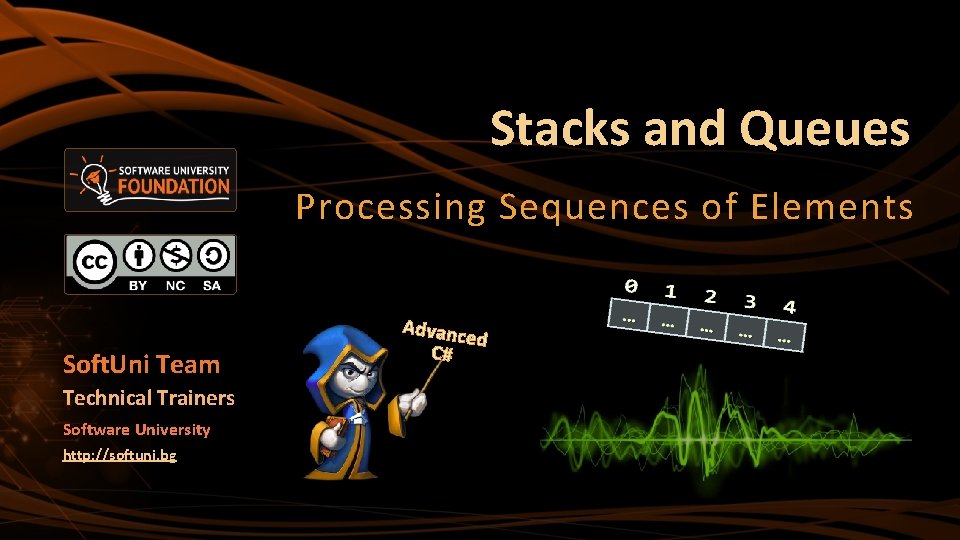
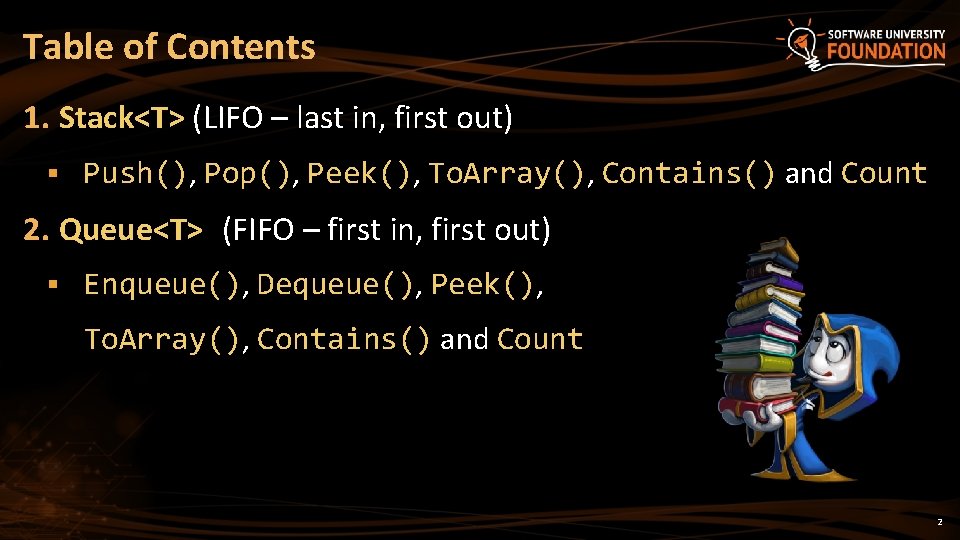
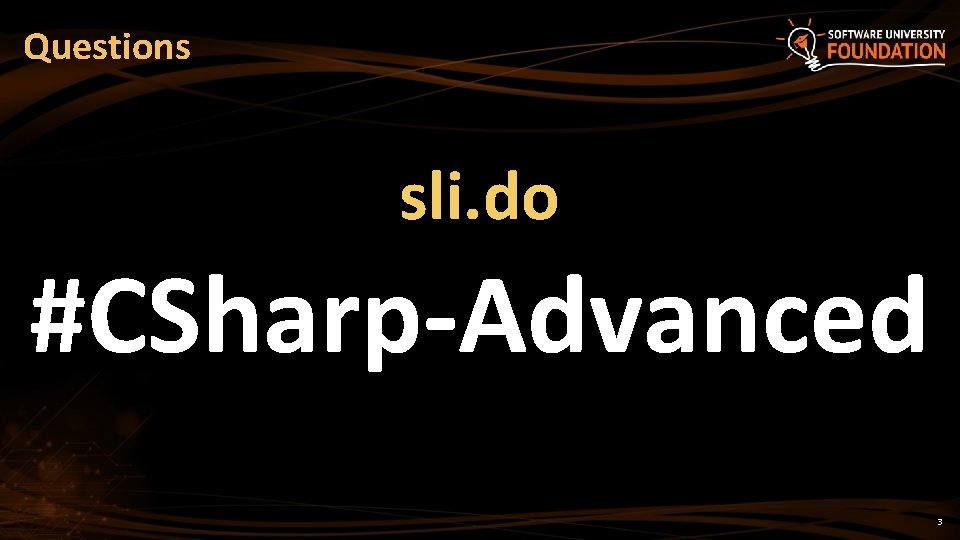
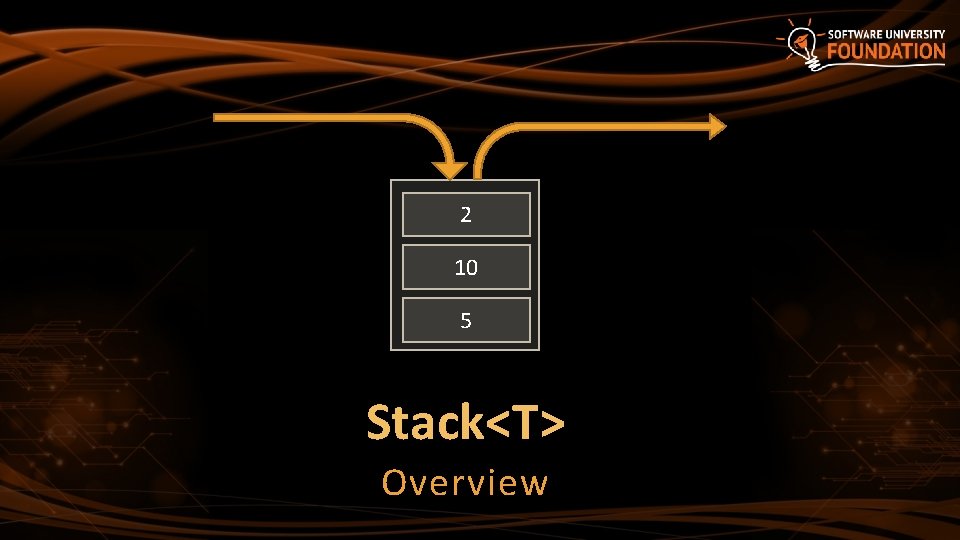
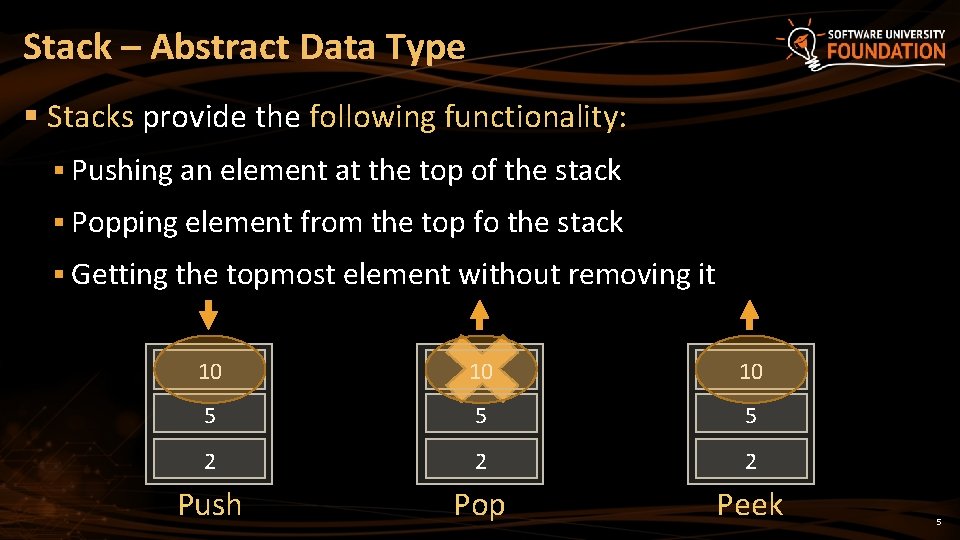
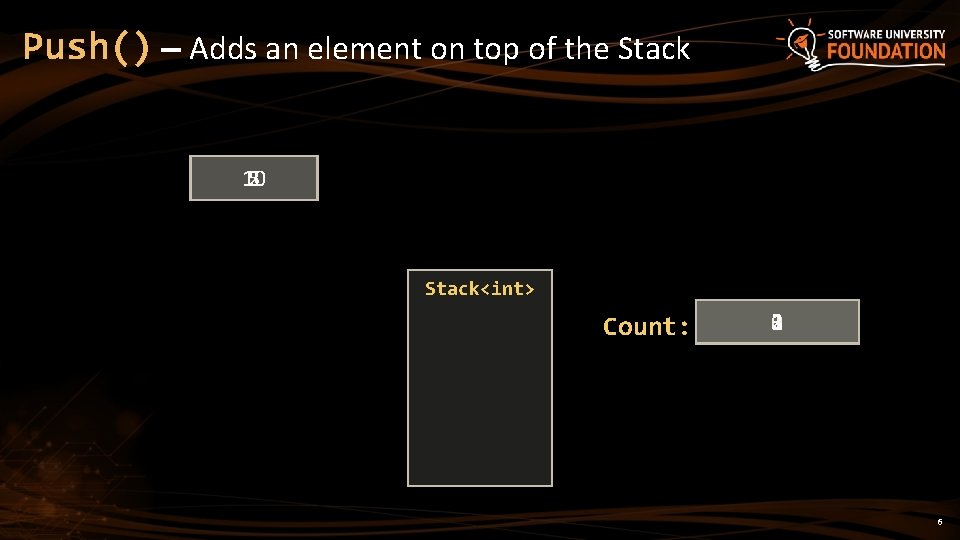
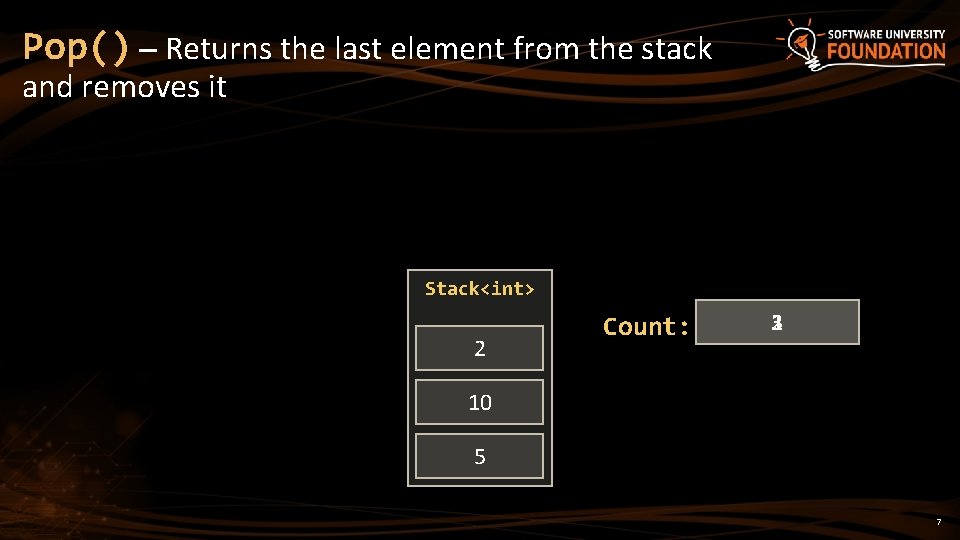
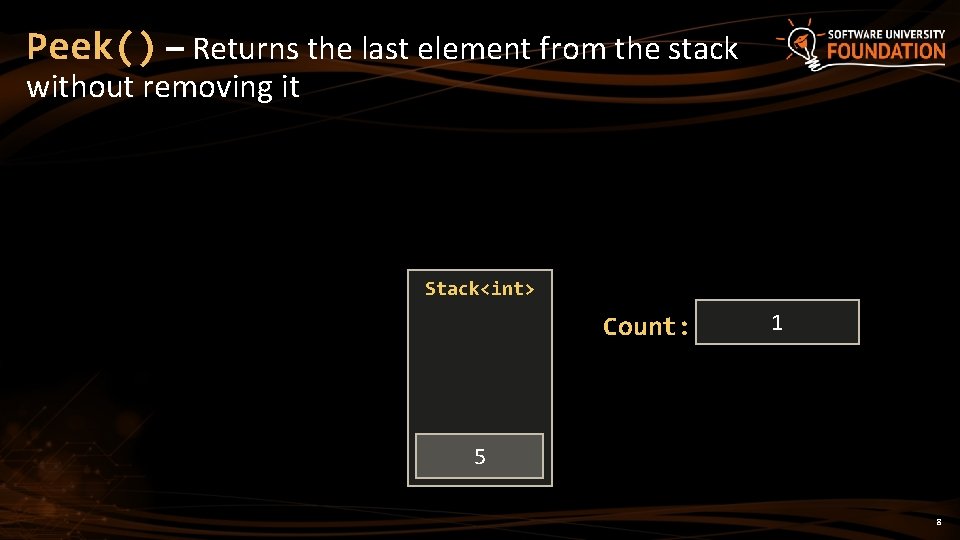
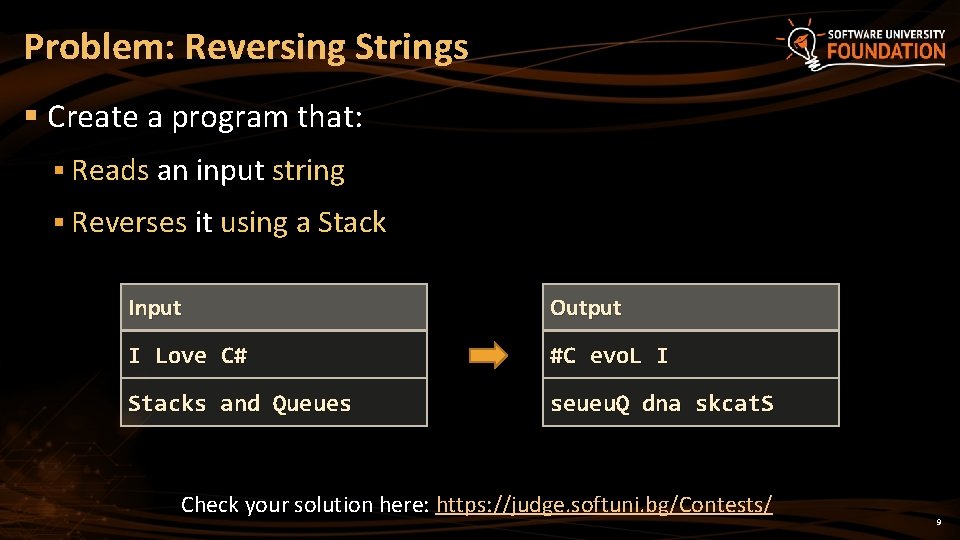
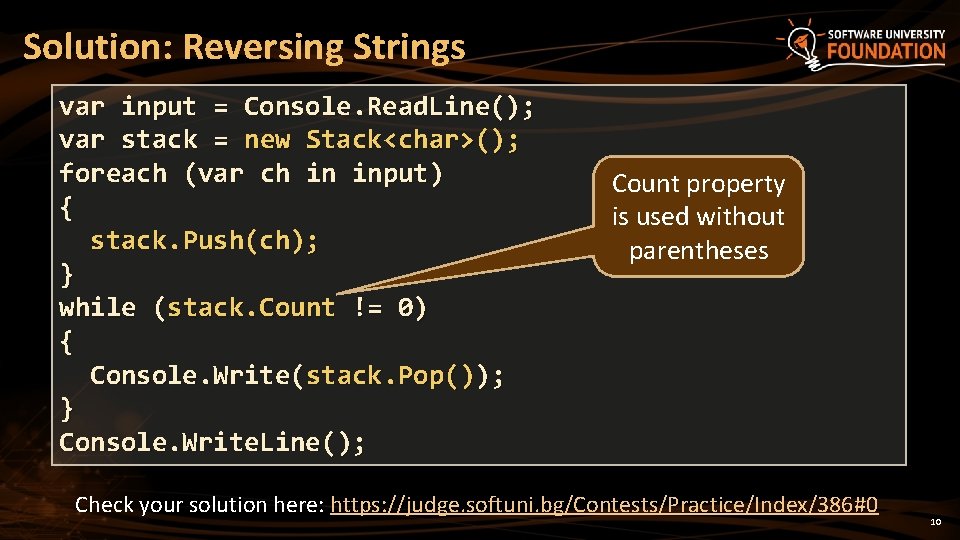
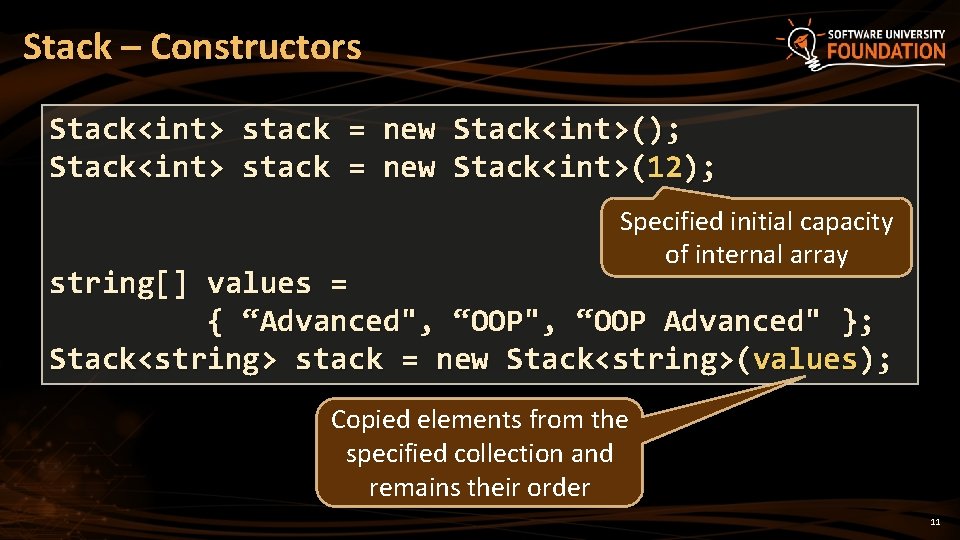
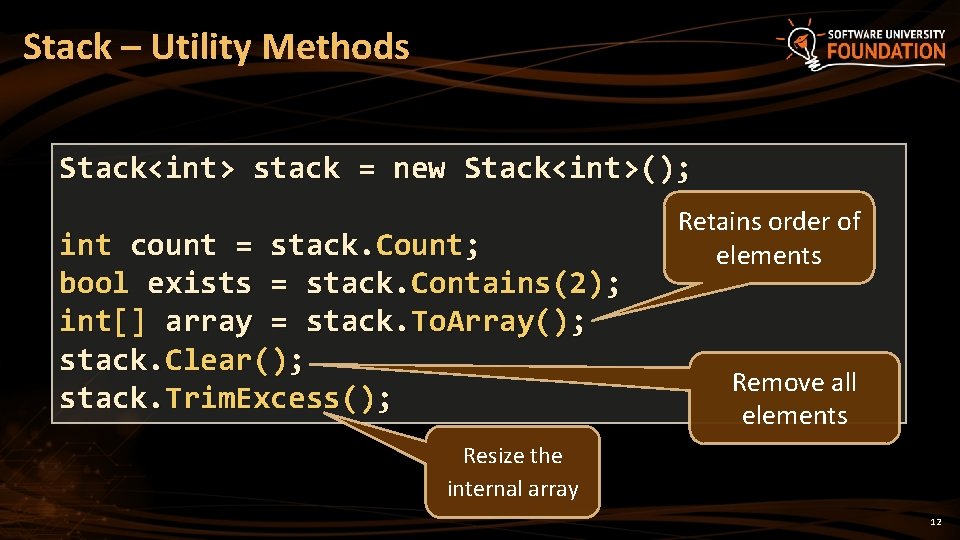
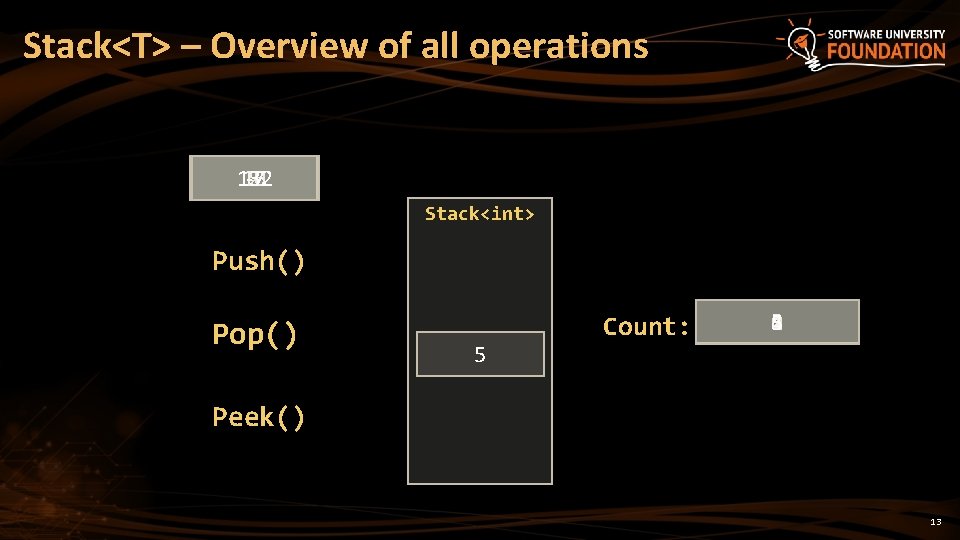
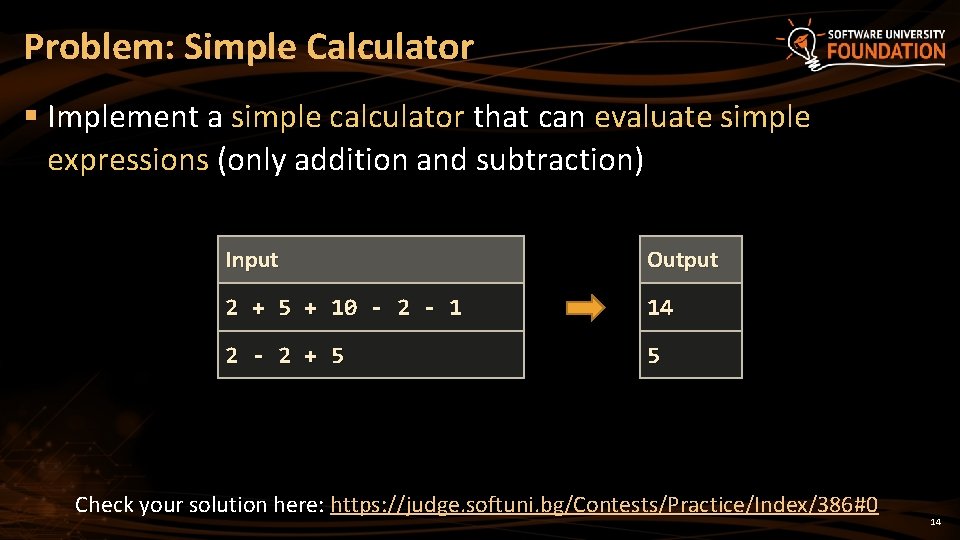
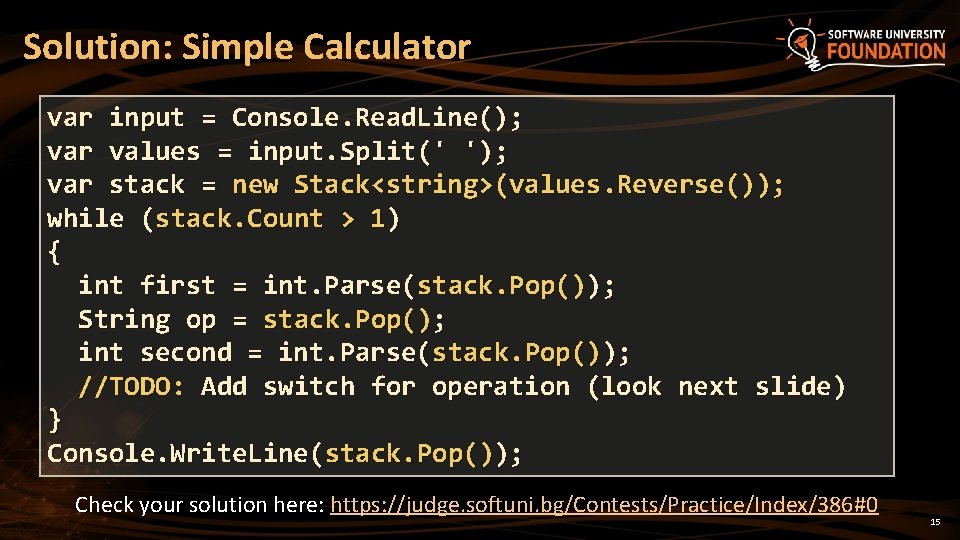
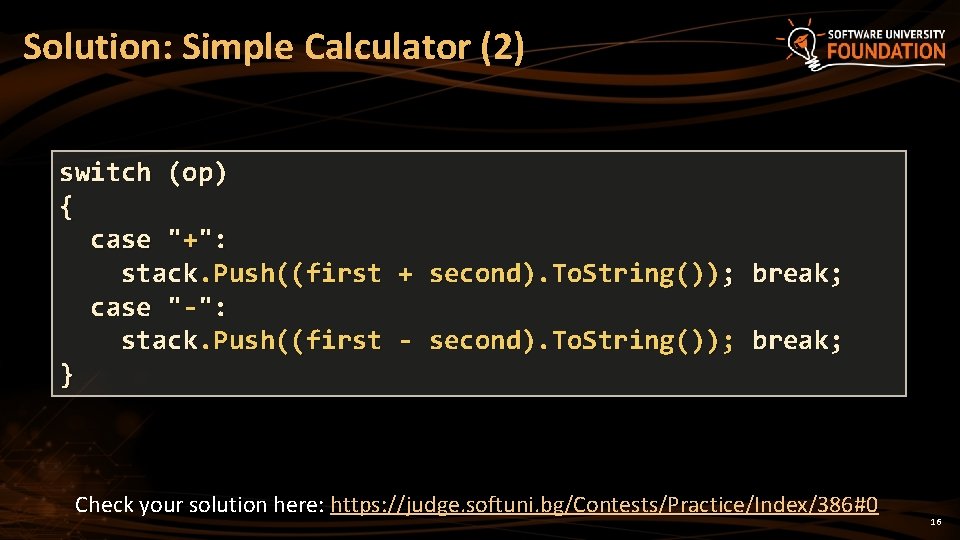
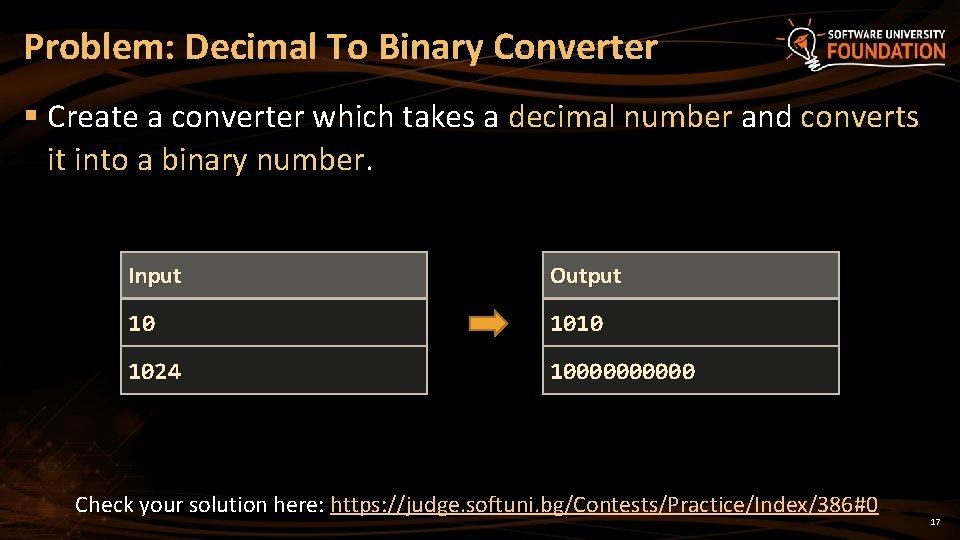
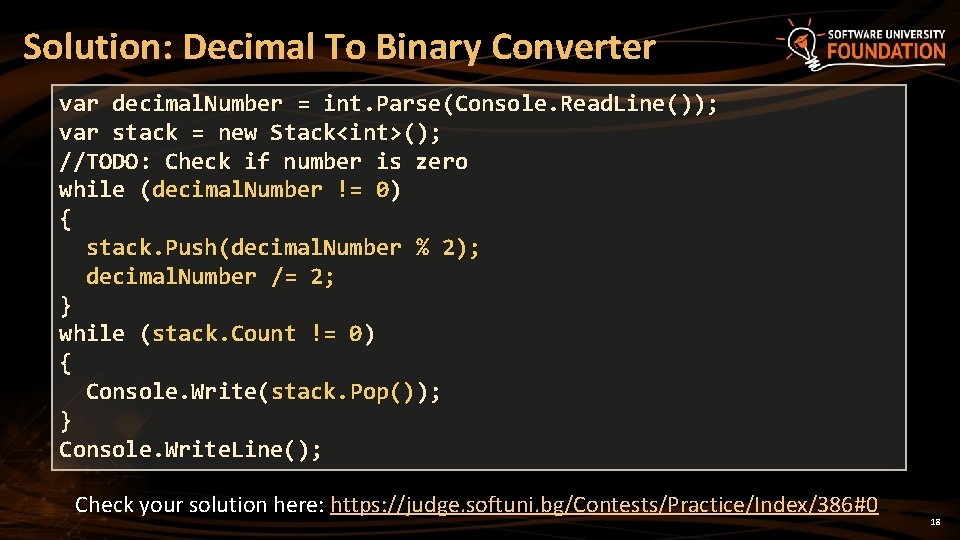
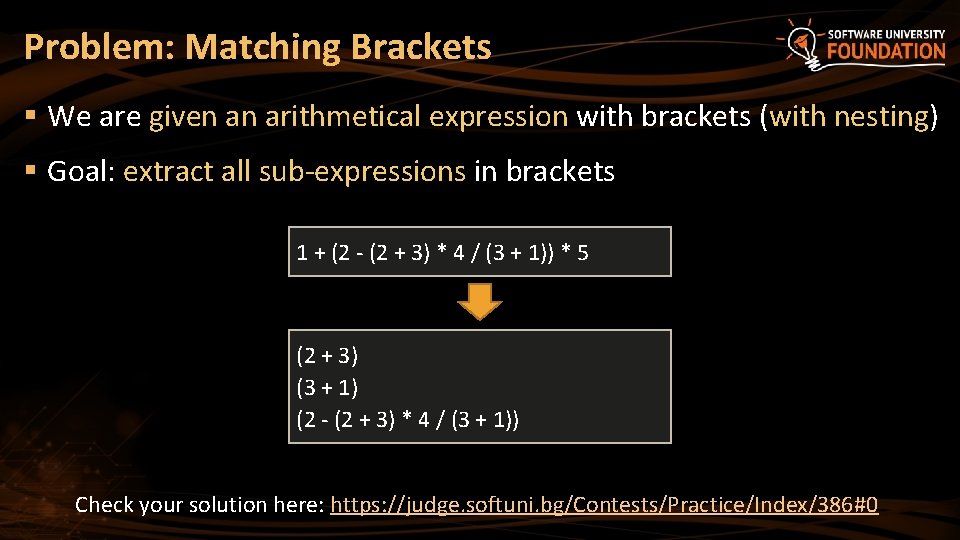
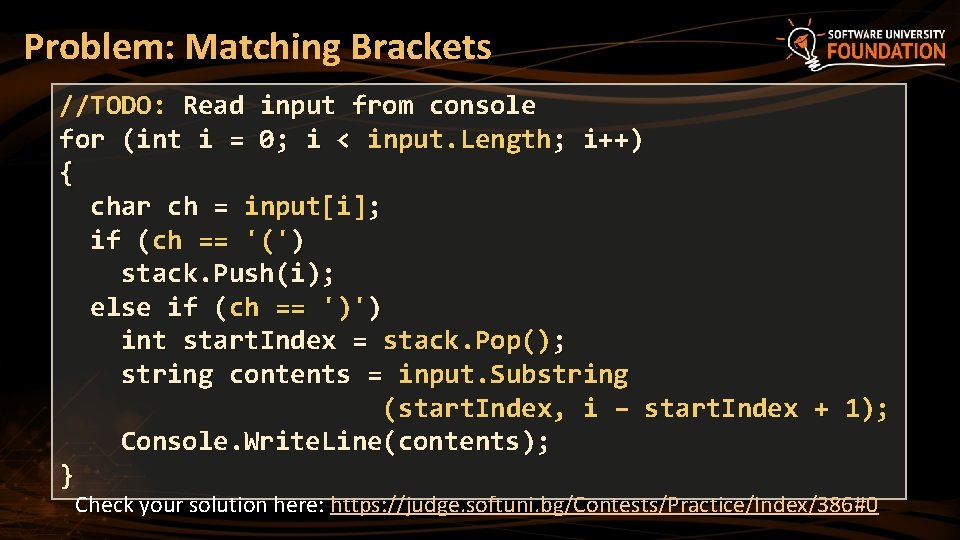
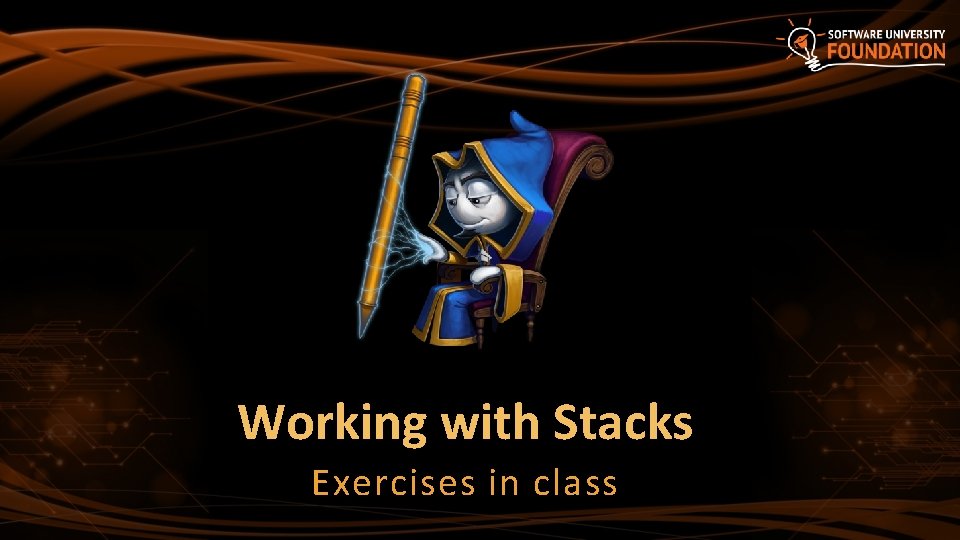
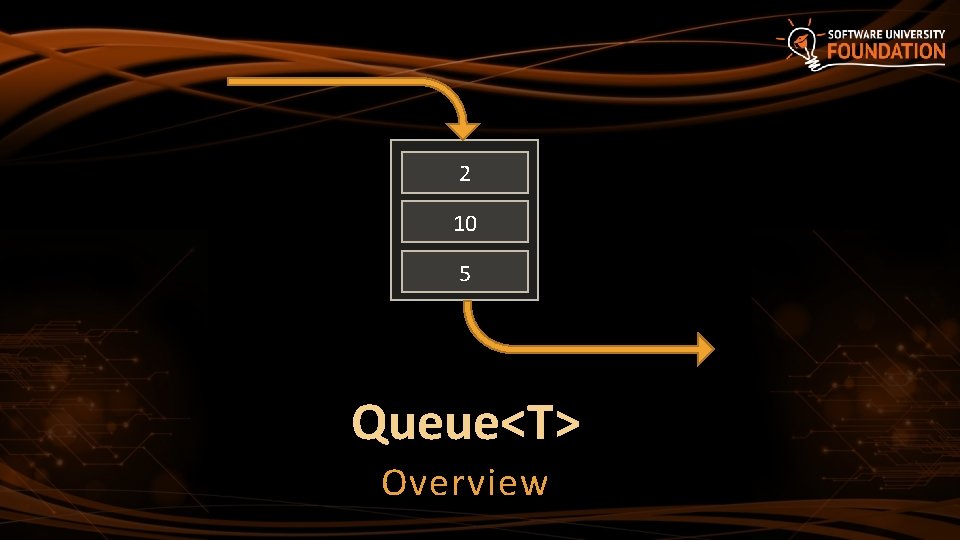
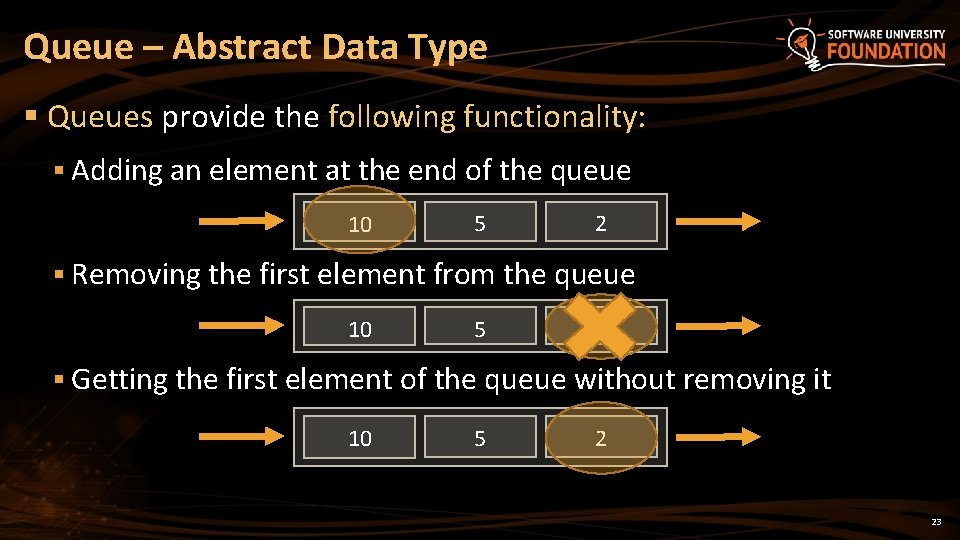
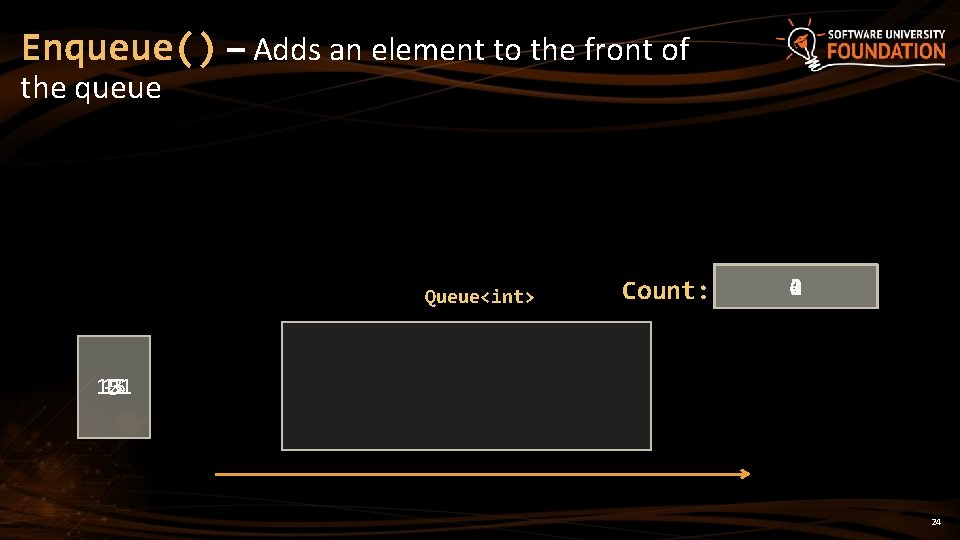
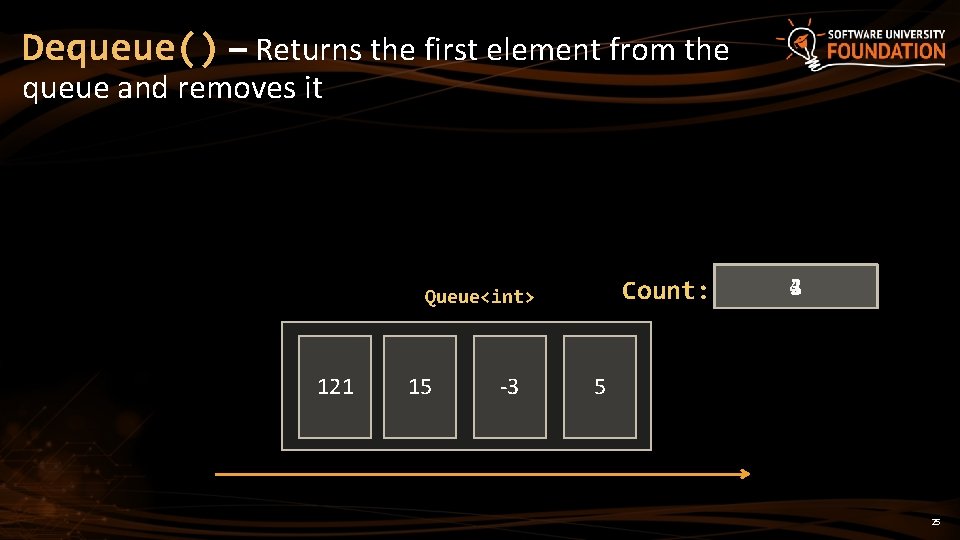
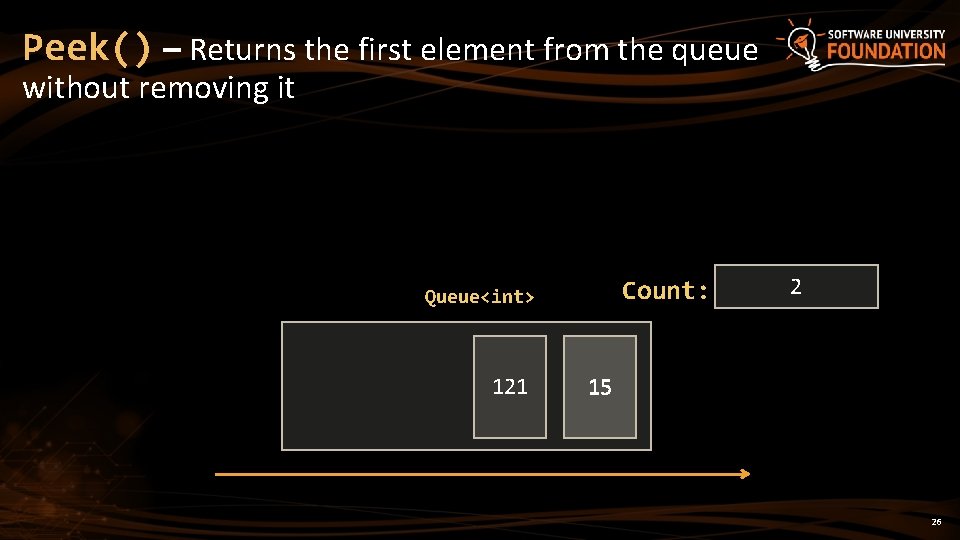
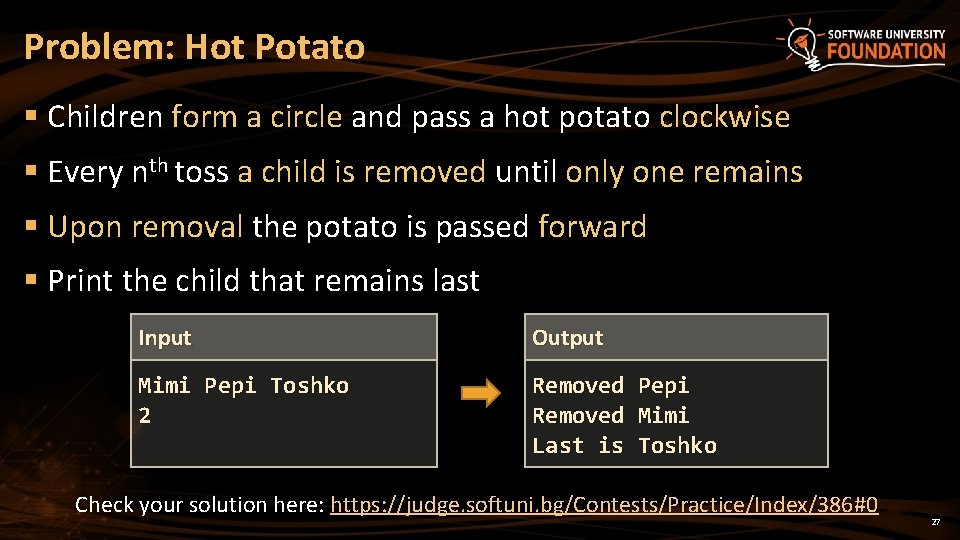
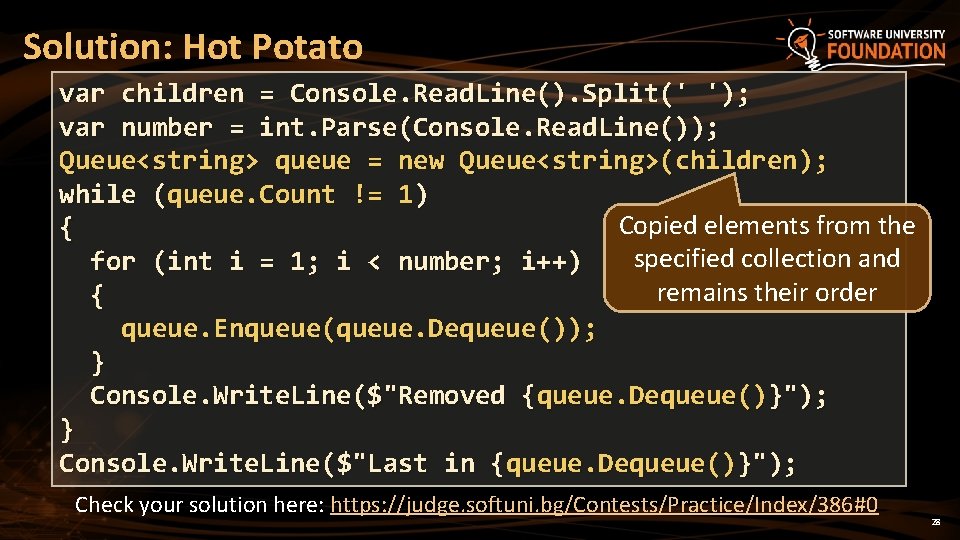
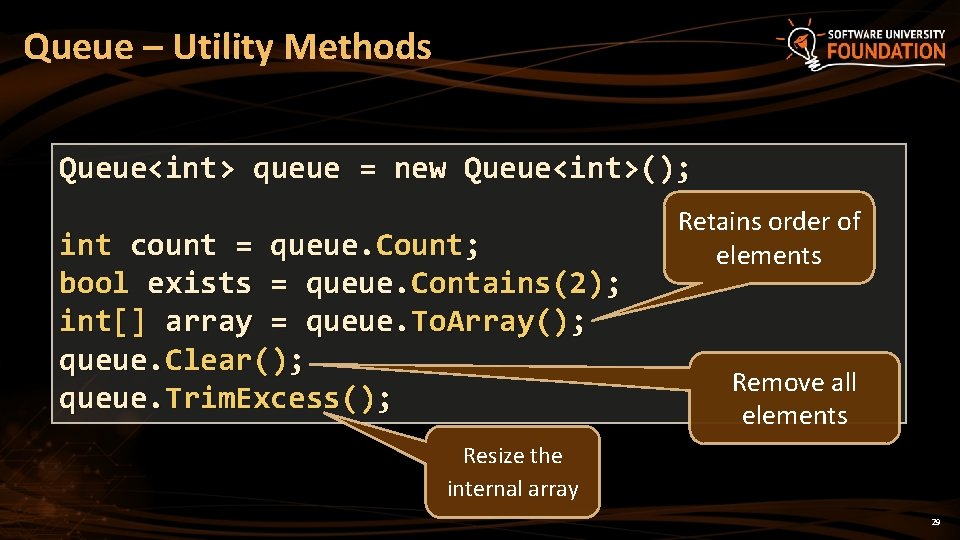
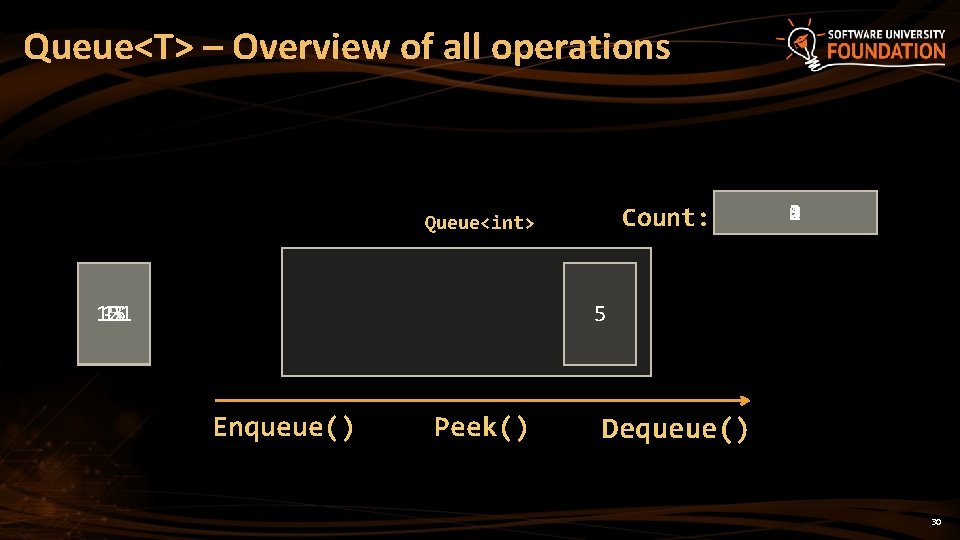
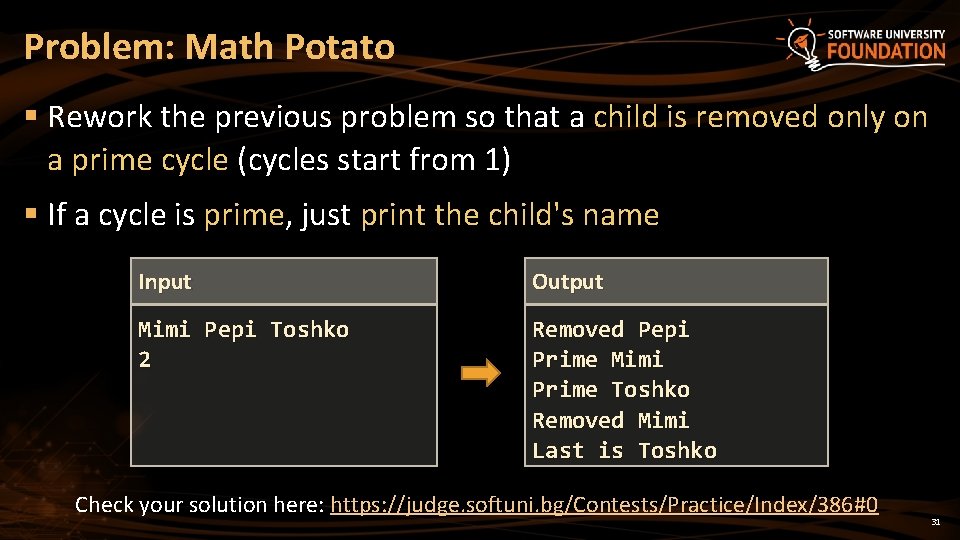
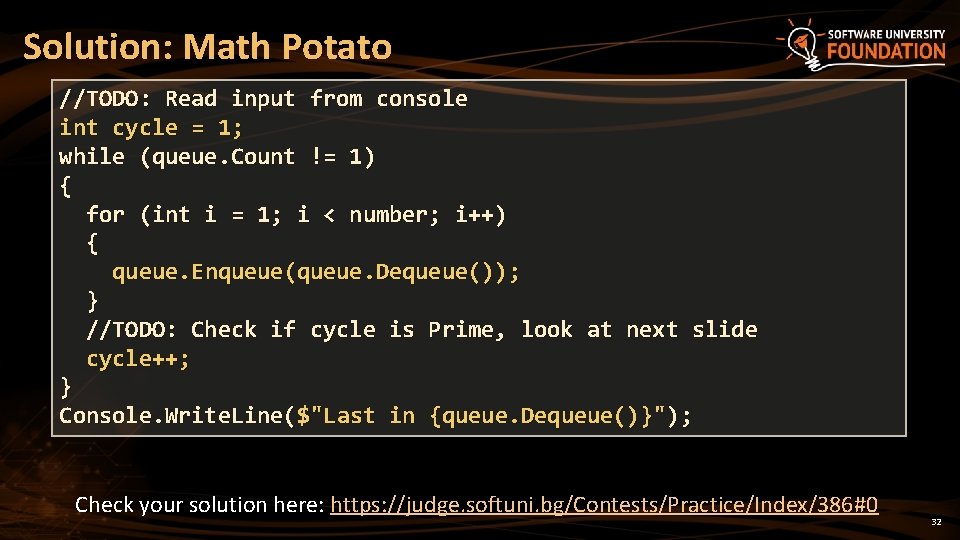
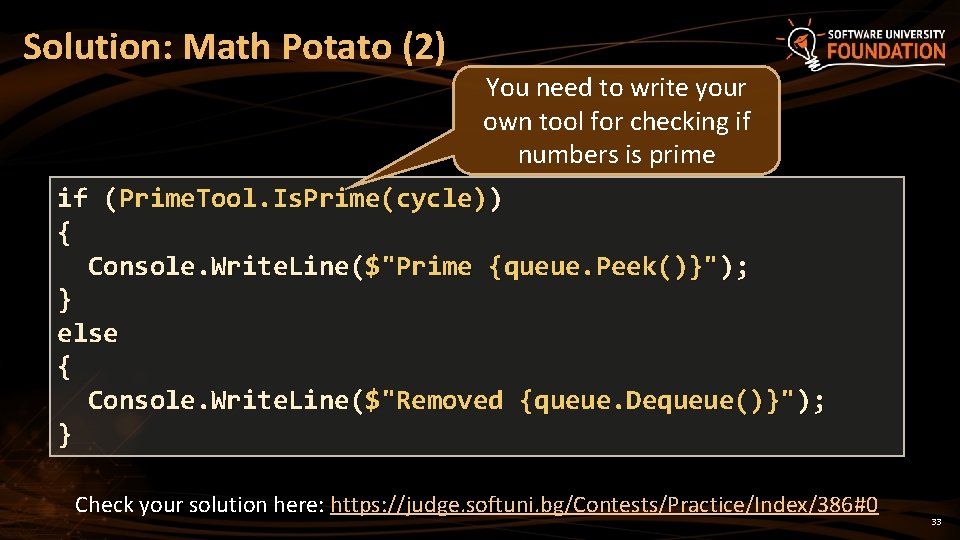
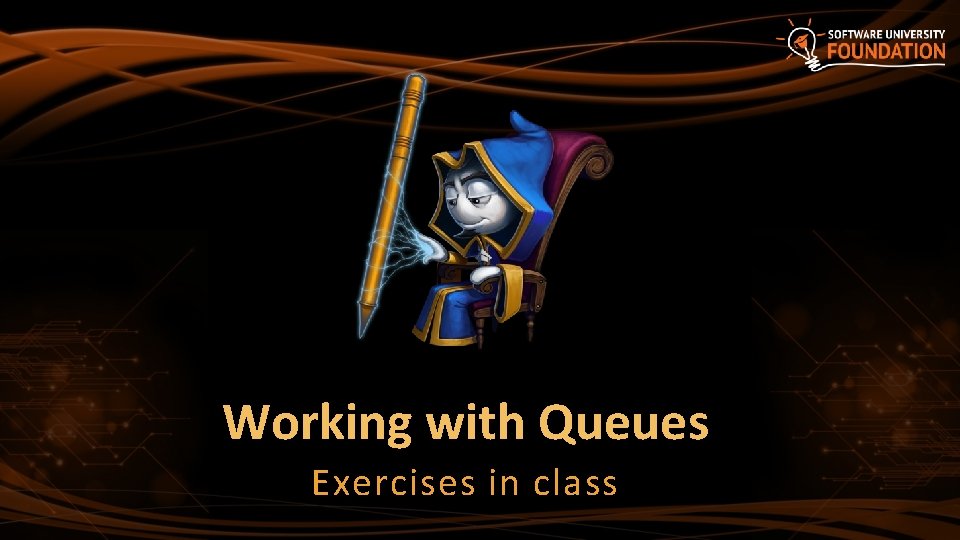
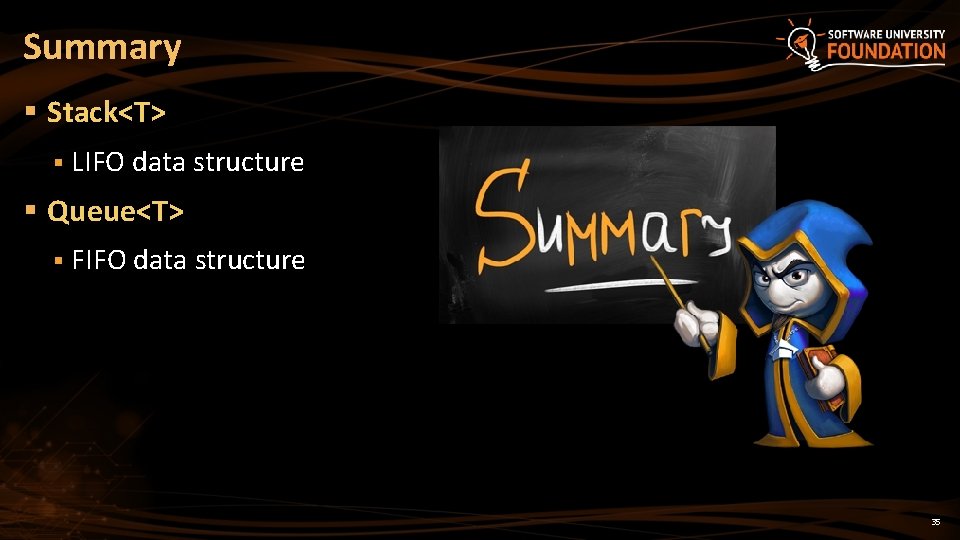
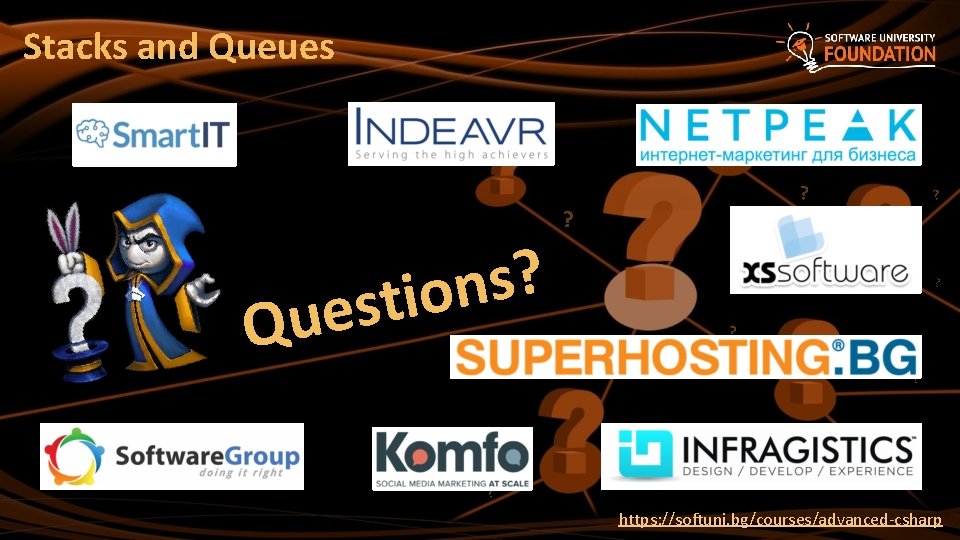
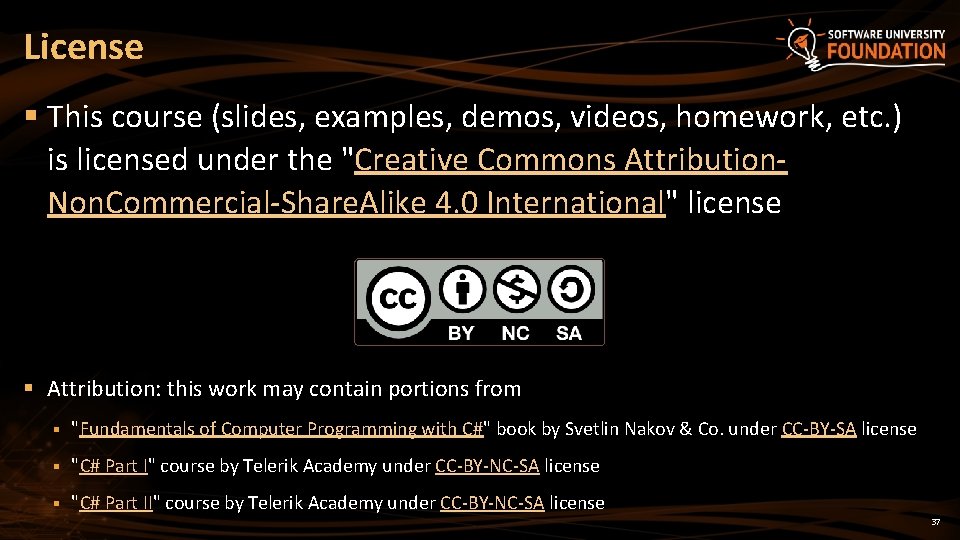
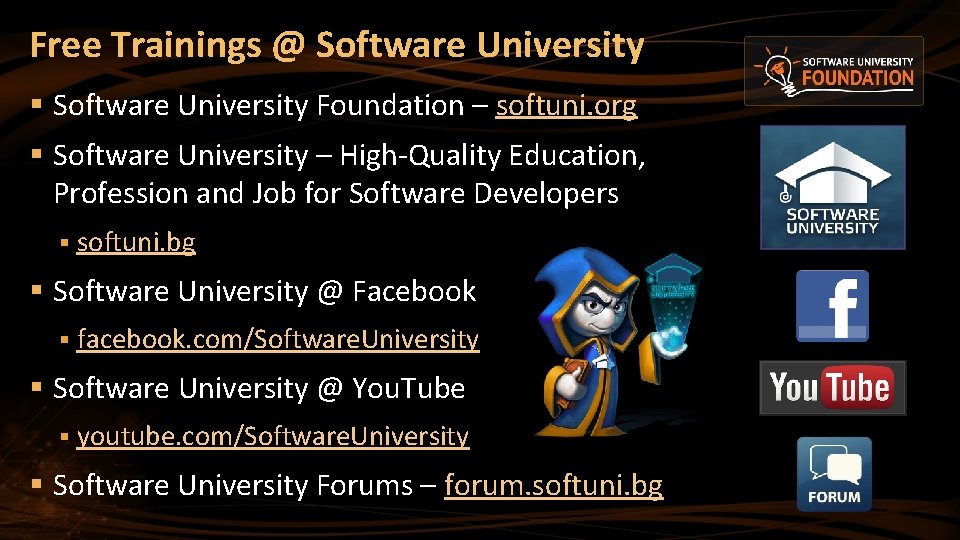
- Slides: 38
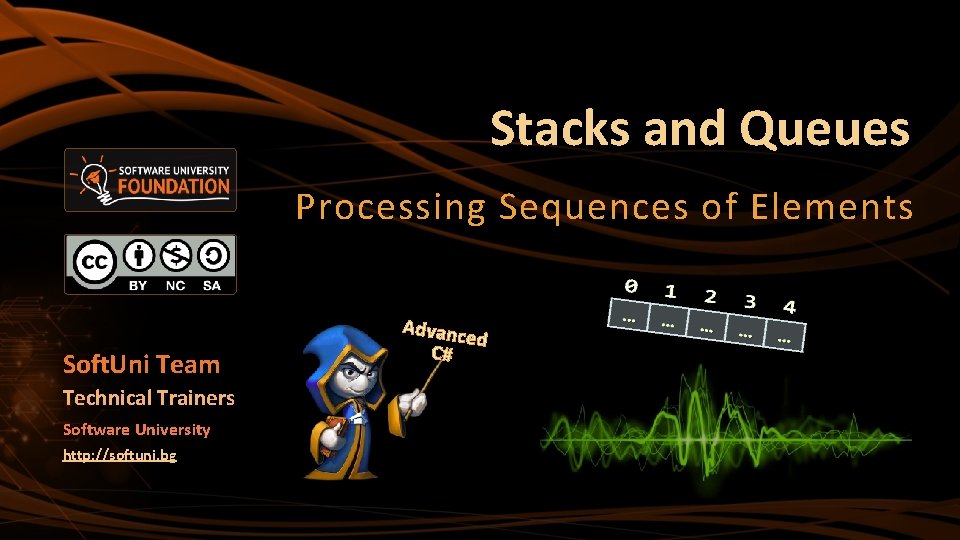
Stacks and Queues Processing Sequences of Elements Soft. Uni Team Technical Trainers Software University http: //softuni. bg Advanc ed C#
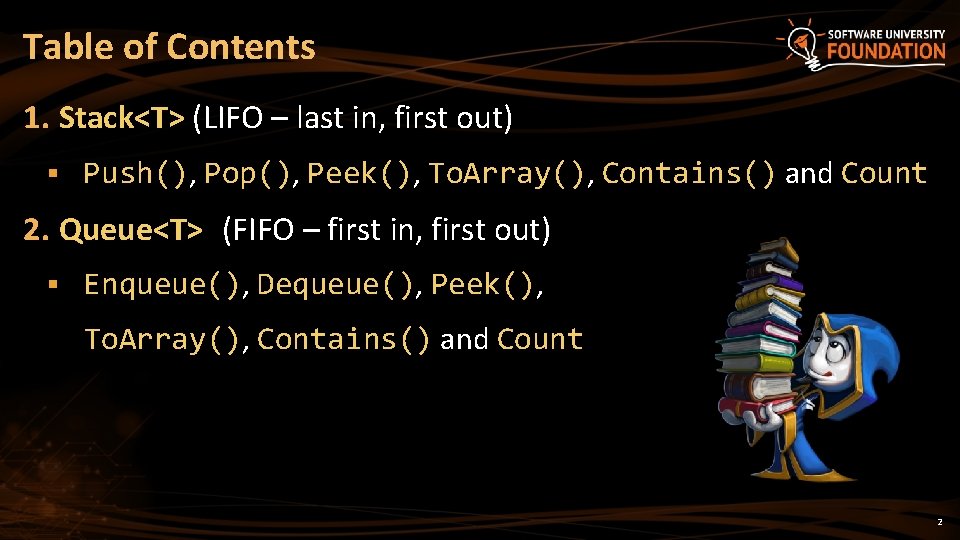
Table of Contents 1. Stack<T> (LIFO – last in, first out) § Push(), Pop(), Peek(), To. Array(), Contains() and Count 2. Queue<T> (FIFO – first in, first out) § Enqueue(), Dequeue(), Peek(), To. Array(), Contains() and Count 2
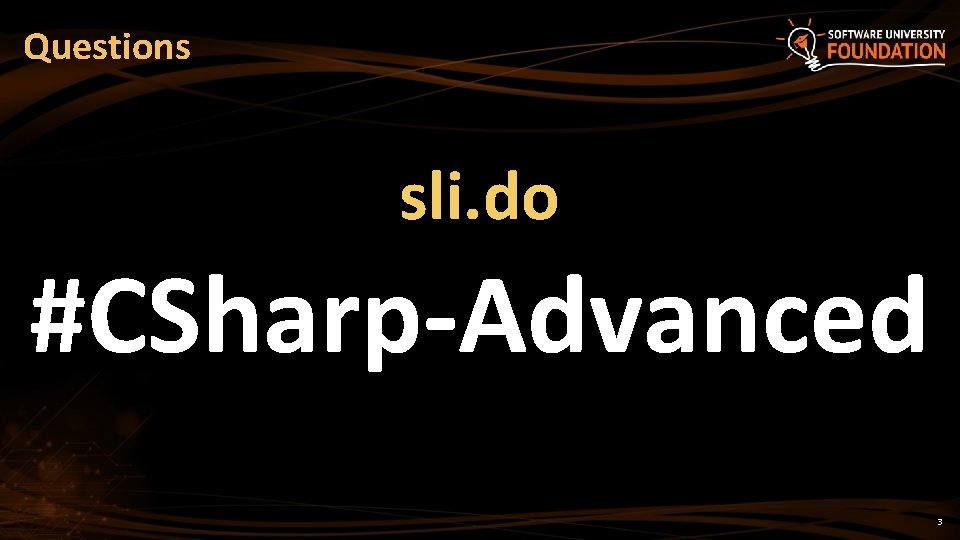
Questions sli. do #CSharp-Advanced 3
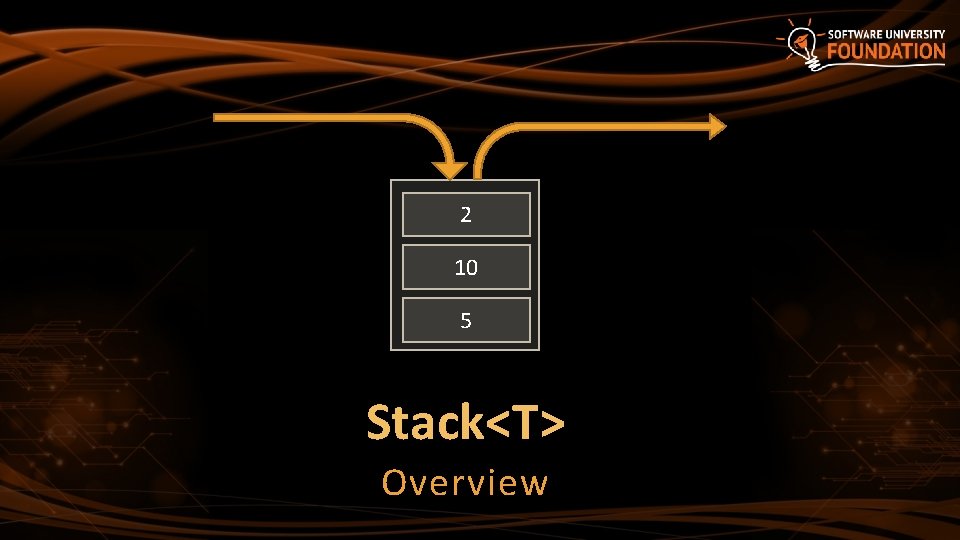
2 10 5 Stack<T> Overview
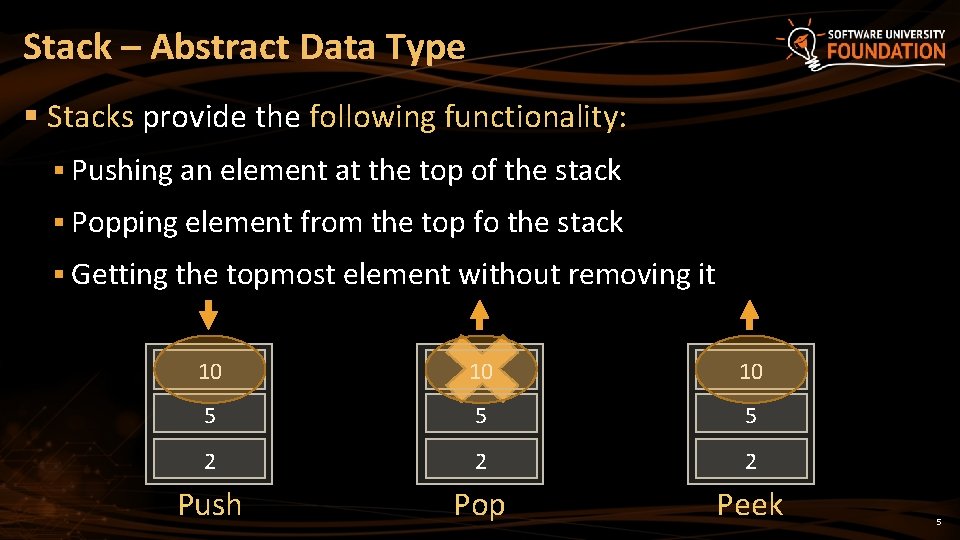
Stack – Abstract Data Type § Stacks provide the following functionality: § Pushing an element at the top of the stack § Popping element from the top fo the stack § Getting the topmost element without removing it 10 10 10 5 5 5 2 2 2 Push Pop Peek 5
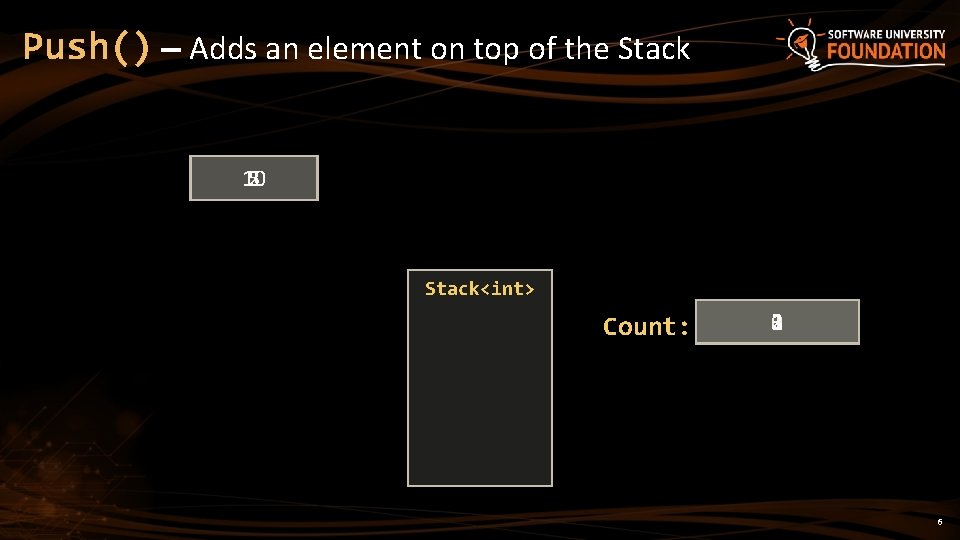
Push() – Adds an element on top of the Stack 10 52 Stack<int> Count: 2 0 3 1 6
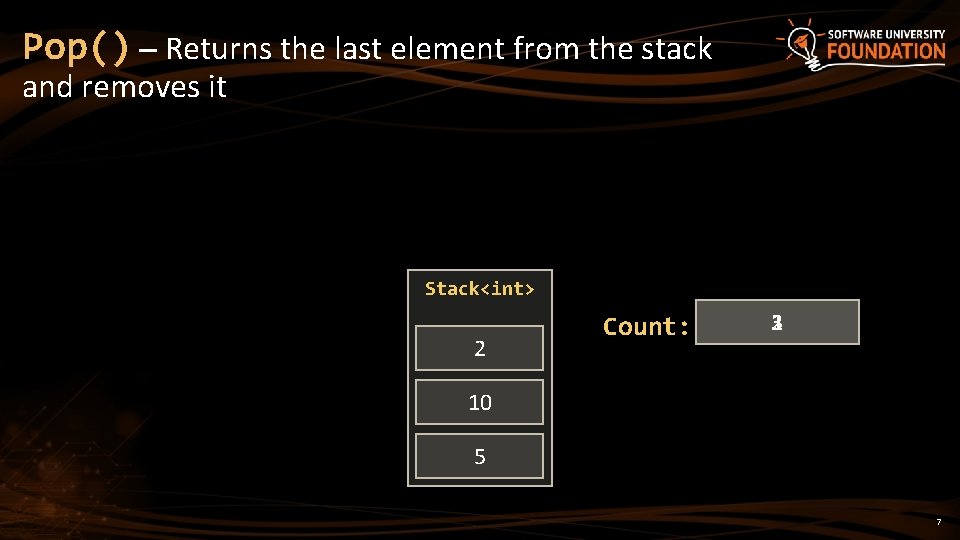
Pop() – Returns the last element from the stack and removes it Stack<int> 2 Count: 2 1 3 10 5 7
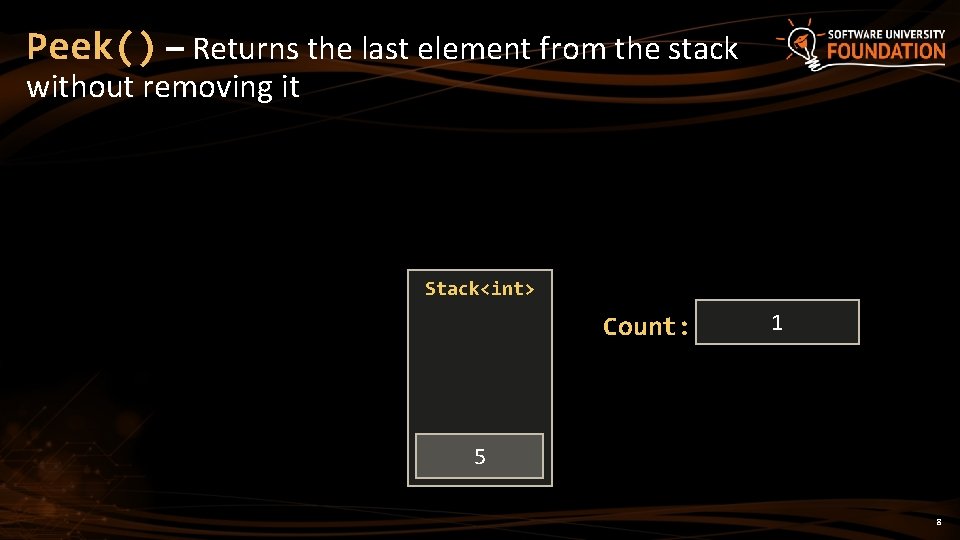
Peek() – Returns the last element from the stack without removing it Stack<int> Count: 1 5 8
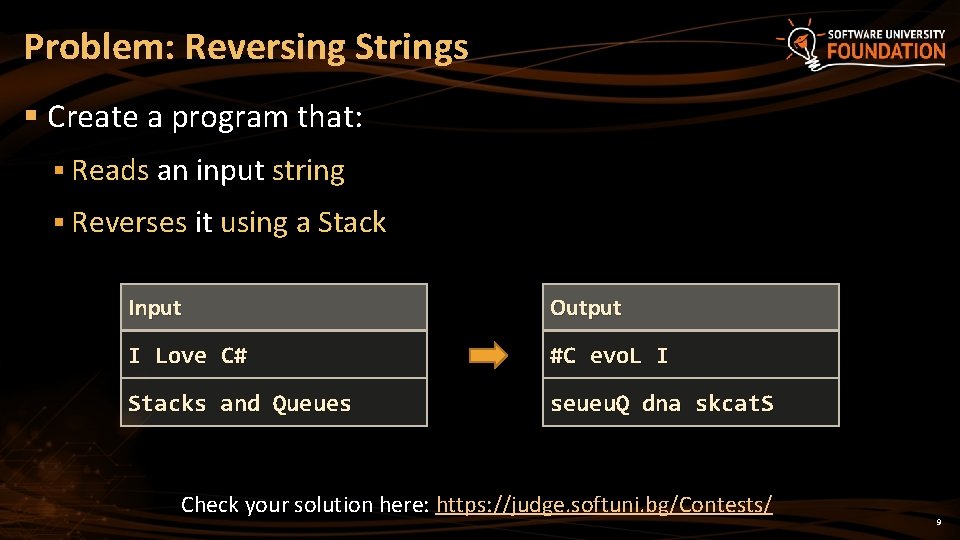
Problem: Reversing Strings § Create a program that: § Reads an input string § Reverses it using a Stack Input Output I Love C# #C evo. L I Stacks and Queues seueu. Q dna skcat. S Check your solution here: https: //judge. softuni. bg/Contests/ 9
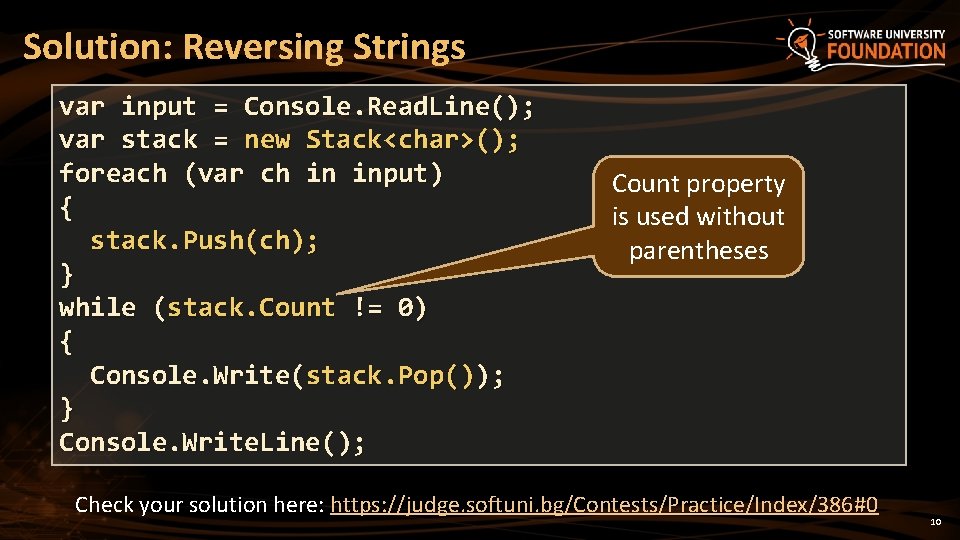
Solution: Reversing Strings var input = Console. Read. Line(); var stack = new Stack<char>(); foreach (var ch in input) { stack. Push(ch); } while (stack. Count != 0) { Console. Write(stack. Pop()); } Console. Write. Line(); Count property is used without parentheses Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 10
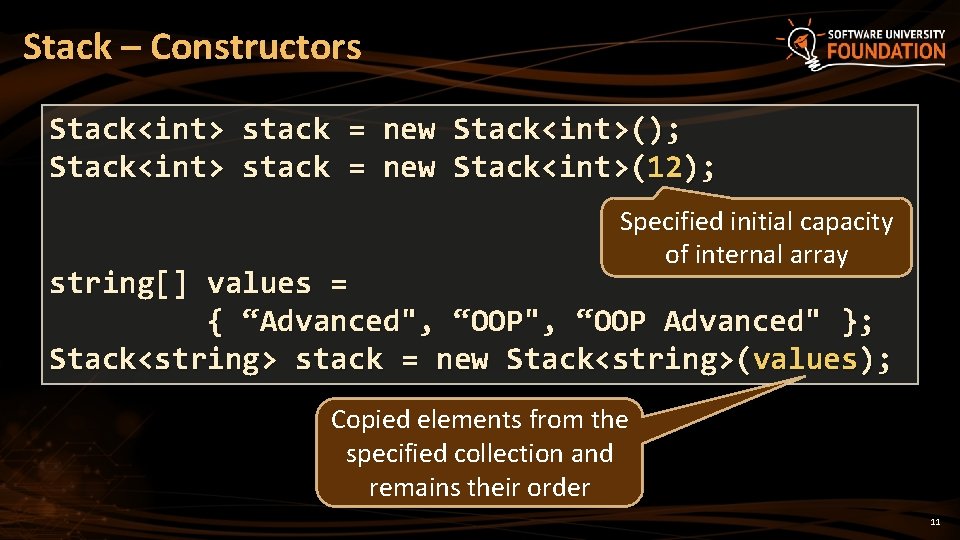
Stack – Constructors Stack<int> stack = new Stack<int>(); Stack<int> stack = new Stack<int>(12); Specified initial capacity of internal array string[] values = { “Advanced", “OOP Advanced" }; Stack<string> stack = new Stack<string>(values); Copied elements from the specified collection and remains their order 11
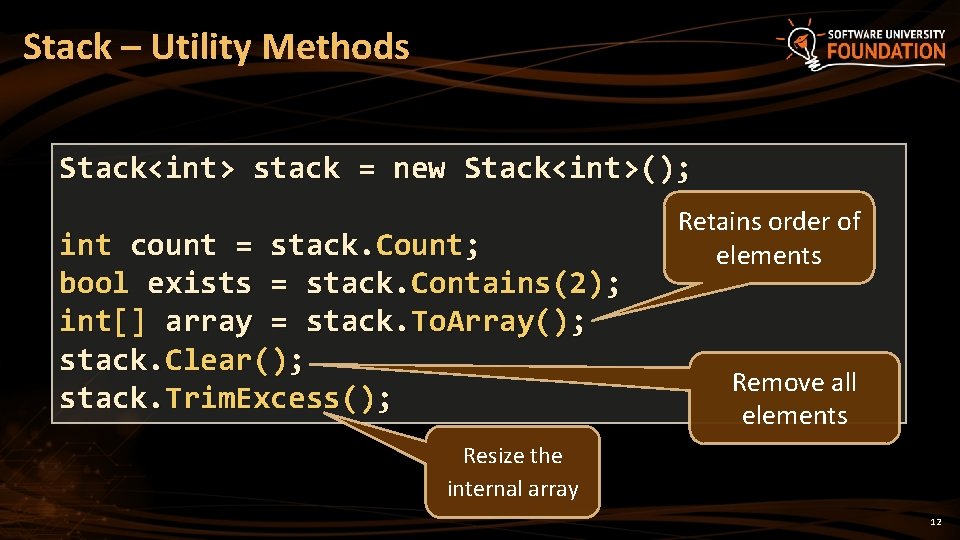
Stack – Utility Methods Stack<int> stack = new Stack<int>(); int count = stack. Count; bool exists = stack. Contains(2); int[] array = stack. To. Array(); stack. Clear(); stack. Trim. Excess(); Retains order of elements Remove all elements Resize the internal array 12
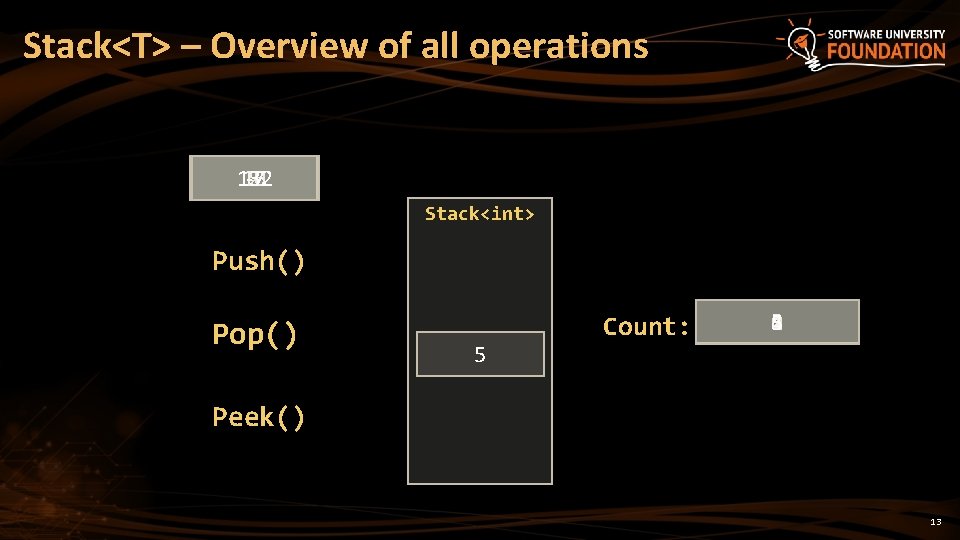
Stack<T> – Overview of all operations 132 10 15 -7 52 Stack<int> Push() Pop() 5 Count: 2 1 0 3 5 4 Peek() 13
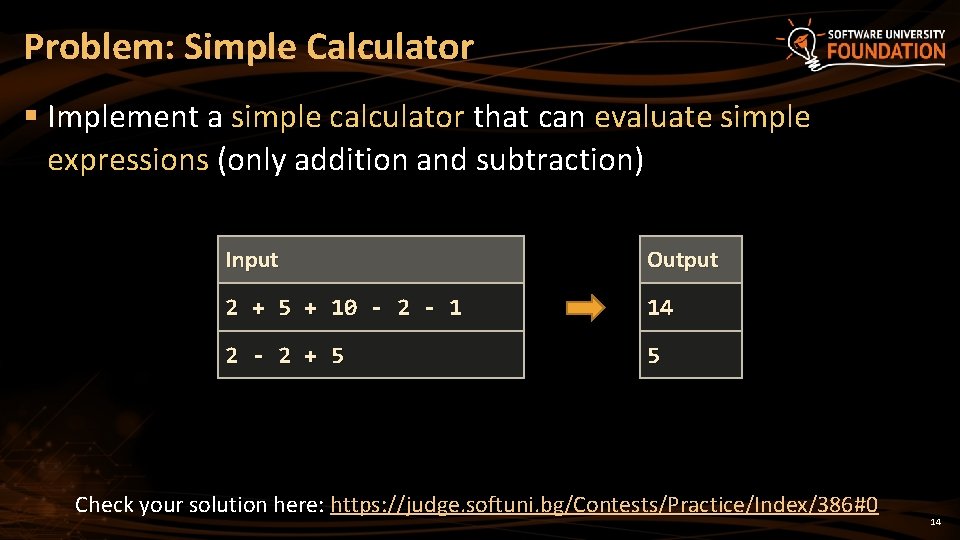
Problem: Simple Calculator § Implement a simple calculator that can evaluate simple expressions (only addition and subtraction) Input Output 2 + 5 + 10 - 2 - 1 14 2 - 2 + 5 5 Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 14
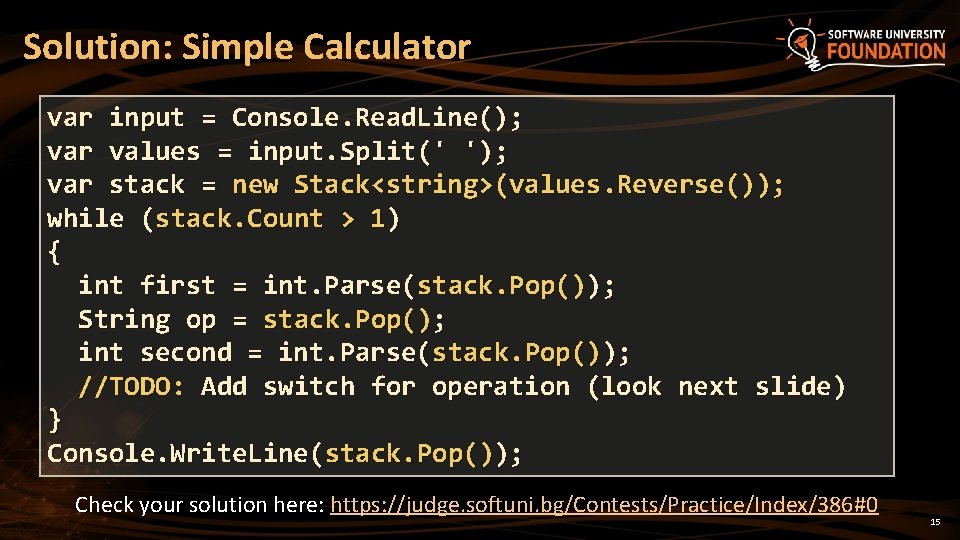
Solution: Simple Calculator var input = Console. Read. Line(); var values = input. Split(' '); var stack = new Stack<string>(values. Reverse()); while (stack. Count > 1) { int first = int. Parse(stack. Pop()); String op = stack. Pop(); int second = int. Parse(stack. Pop()); //TODO: Add switch for operation (look next slide) } Console. Write. Line(stack. Pop()); Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 15
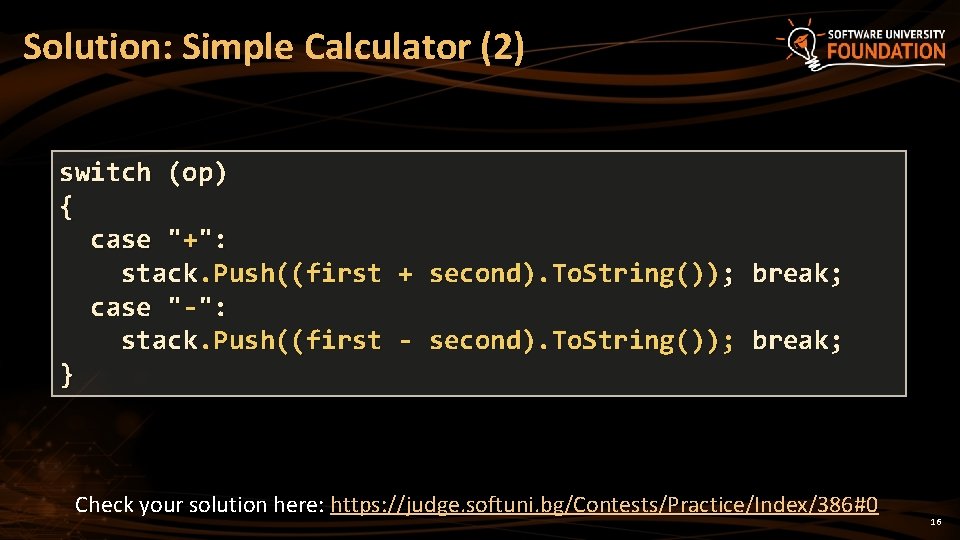
Solution: Simple Calculator (2) switch (op) { case "+": stack. Push((first + second). To. String()); break; case "-": stack. Push((first - second). To. String()); break; } Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 16
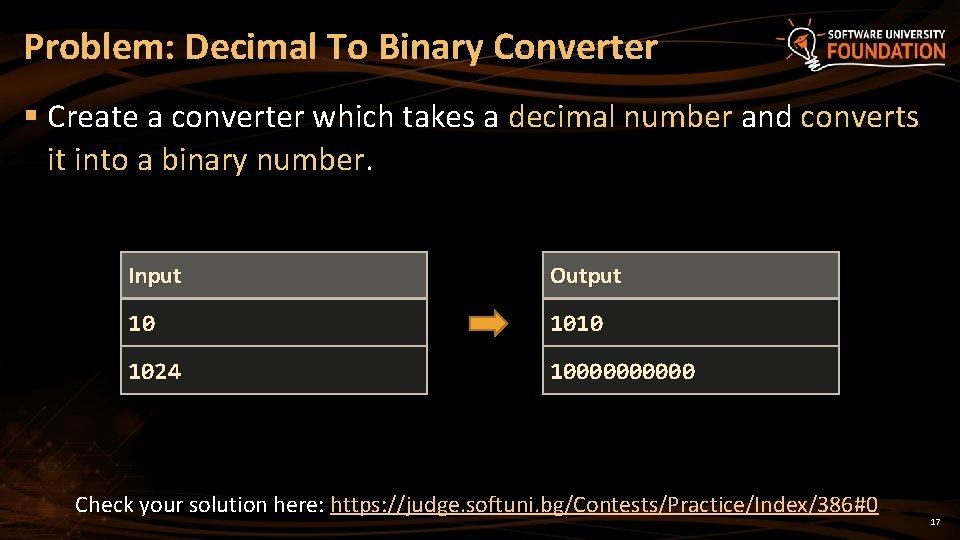
Problem: Decimal To Binary Converter § Create a converter which takes a decimal number and converts it into a binary number. Input Output 10 1024 100000 Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 17
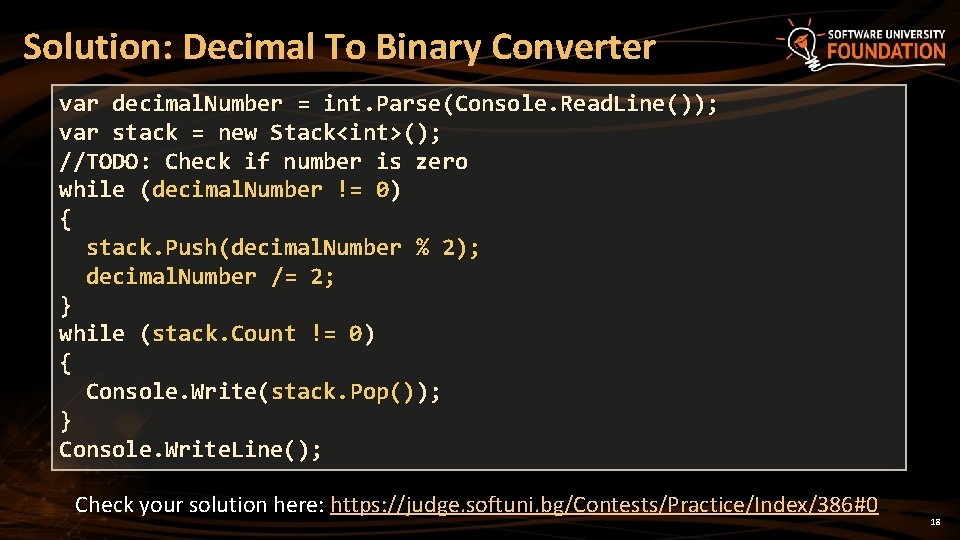
Solution: Decimal To Binary Converter var decimal. Number = int. Parse(Console. Read. Line ()); var stack = new Stack<int>(); //TODO: Check if number is zero while (decimal. Number != 0) { stack. Push(decimal. Number % 2); decimal. Number /= 2; } while (stack. Count != 0) { Console. Write(stack. Pop()); } Console. Write. Line(); Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 18
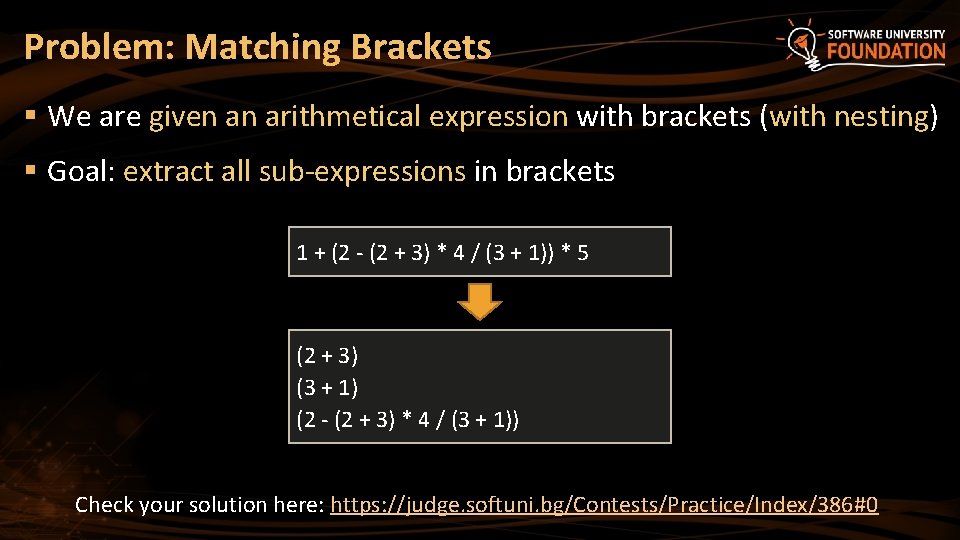
Problem: Matching Brackets § We are given an arithmetical expression with brackets (with nesting) § Goal: extract all sub-expressions in brackets 1 + (2 - (2 + 3) * 4 / (3 + 1)) * 5 (2 + 3) (3 + 1) (2 - (2 + 3) * 4 / (3 + 1)) Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0
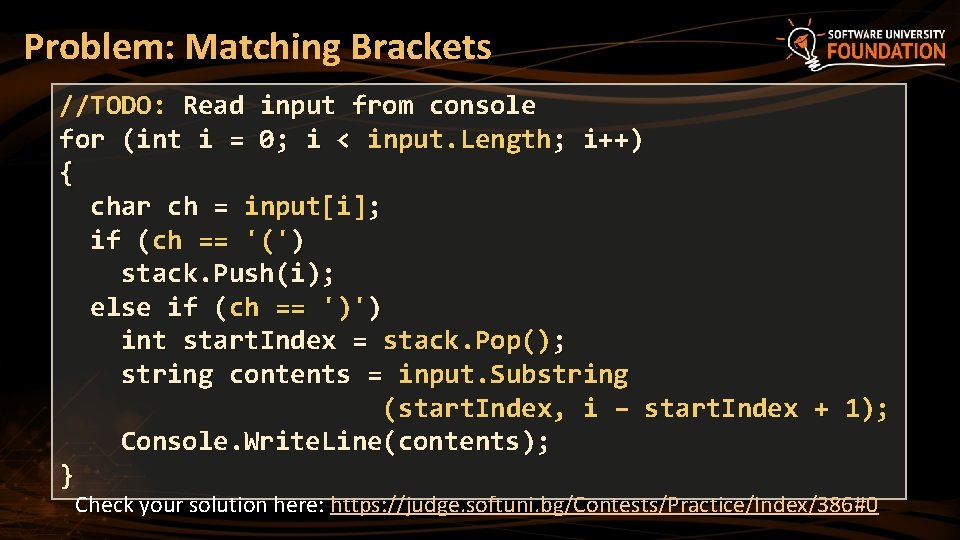
Problem: Matching Brackets //TODO: Read input from console for (int i = 0; i < input. Length; i++) { char ch = input[i]; if (ch == '(') stack. Push(i); else if (ch == ')') int start. Index = stack. Pop(); string contents = input. Substring (start. Index, i – start. Index + 1); Console. Write. Line(contents); } Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0
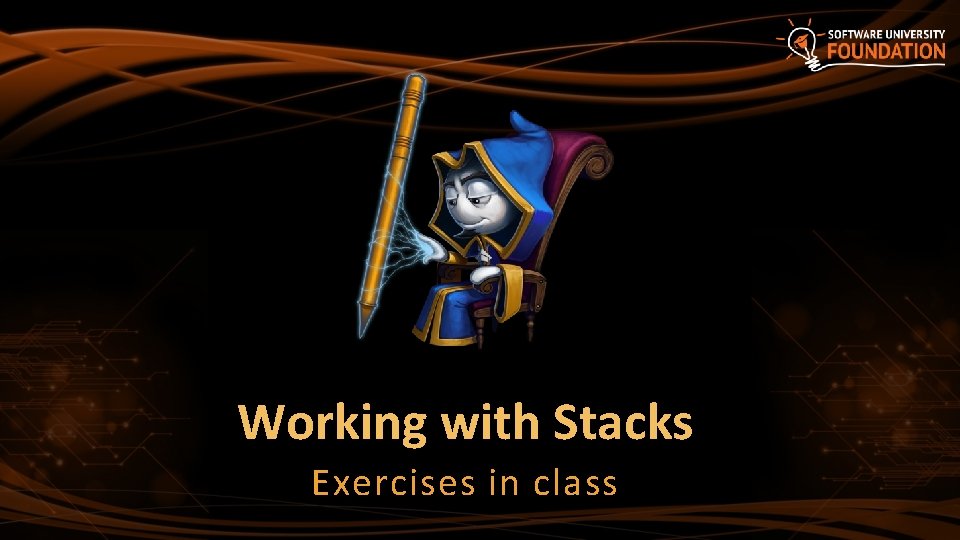
Working with Stacks Exercises in class
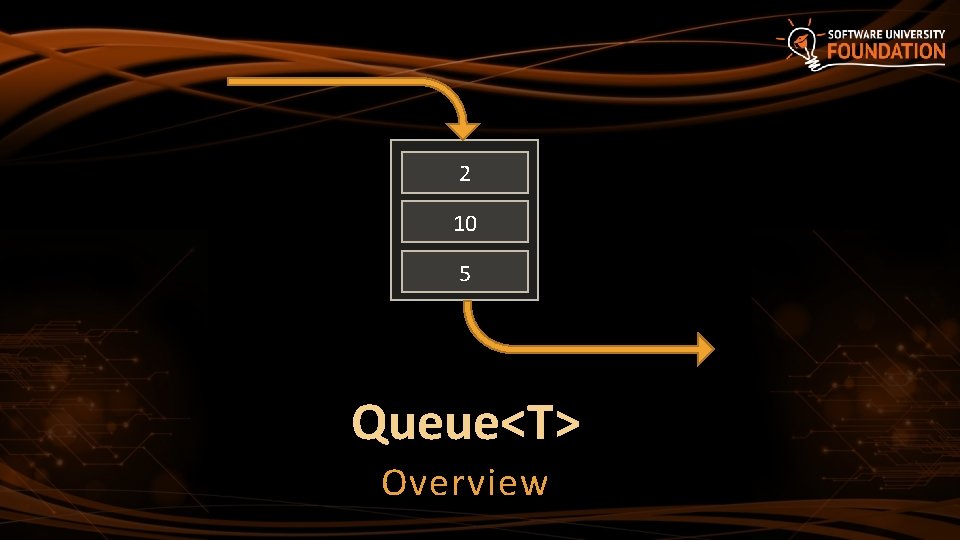
2 10 5 Queue<T> Overview
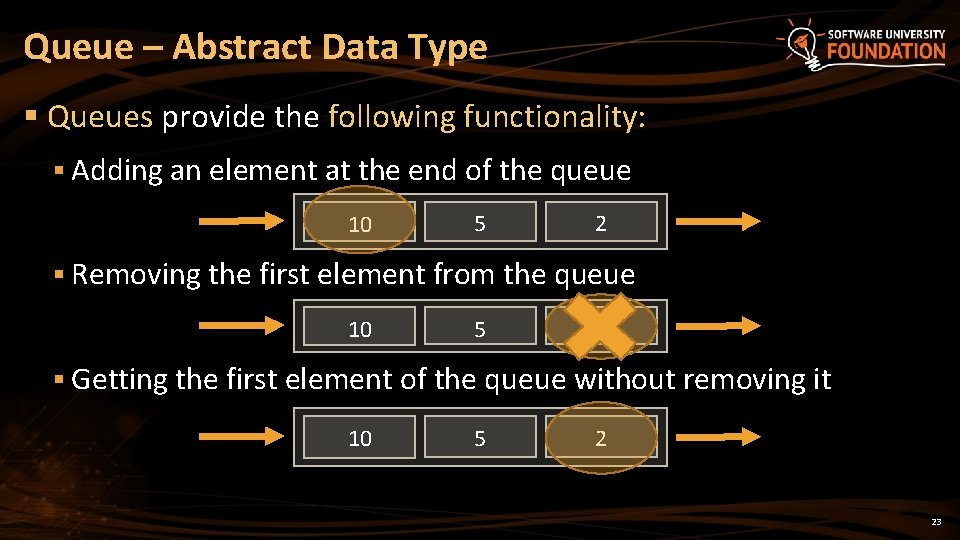
Queue – Abstract Data Type § Queues provide the following functionality: § Adding an element at the end of the queue 10 5 2 § Removing the first element from the queue 10 5 2 § Getting the first element of the queue without removing it 10 5 2 23
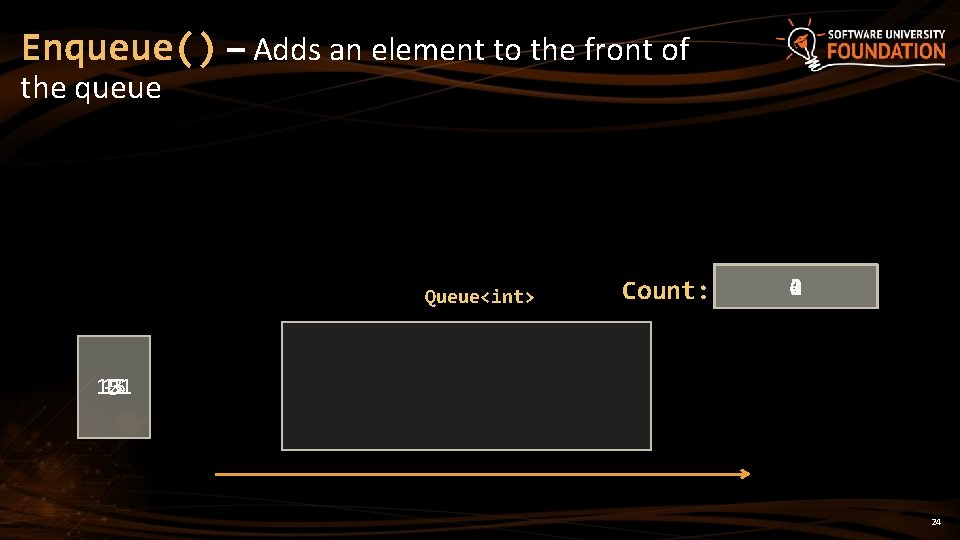
Enqueue() – Adds an element to the front of the queue Queue<int> Count: 43 2 0 1 121 15 -3 5 24
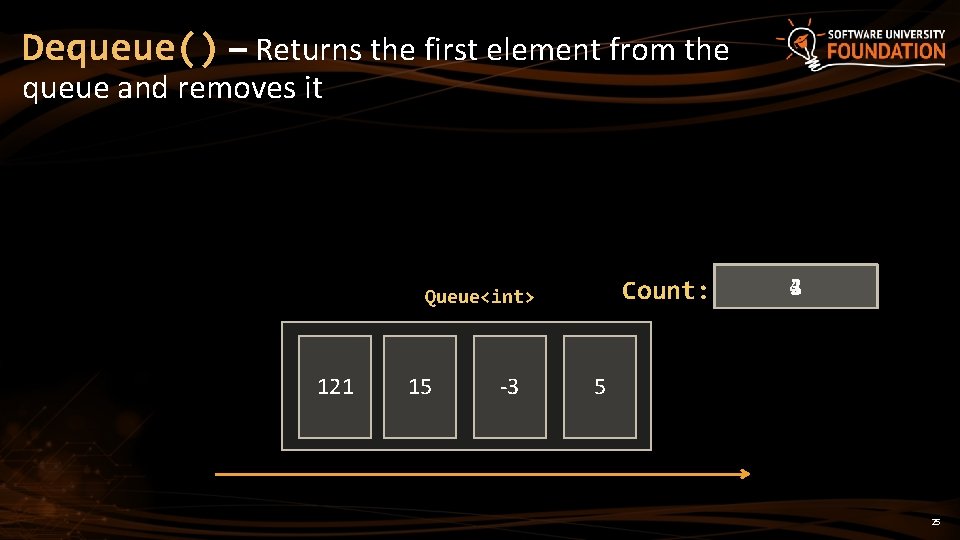
Dequeue() – Returns the first element from the queue and removes it Count: Queue<int> 121 15 -3 42 3 5 25
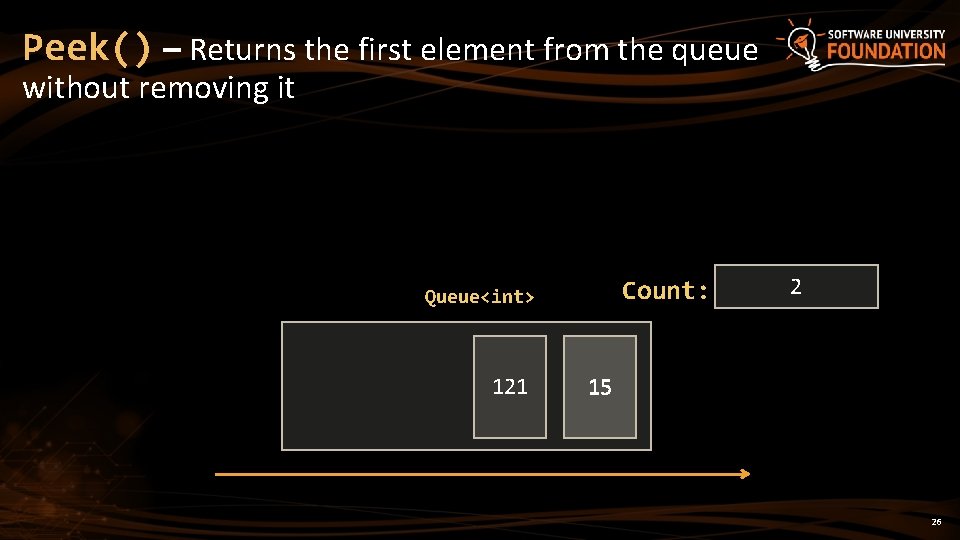
Peek() – Returns the first element from the queue without removing it Count: Queue<int> 121 2 15 26
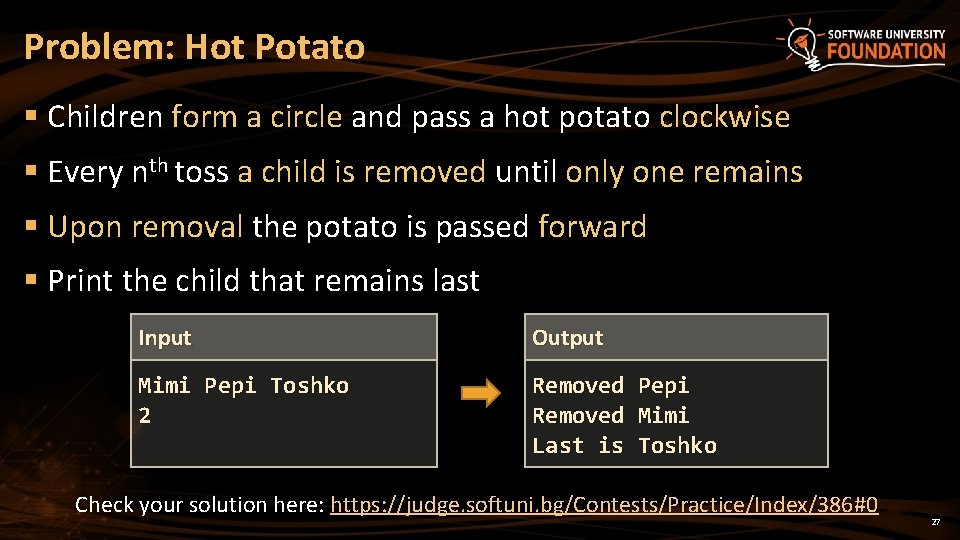
Problem: Hot Potato § Children form a circle and pass a hot potato clockwise § Every nth toss a child is removed until only one remains § Upon removal the potato is passed forward § Print the child that remains last Input Output Mimi Pepi Toshko 2 Removed Pepi Removed Mimi Last is Toshko Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 27
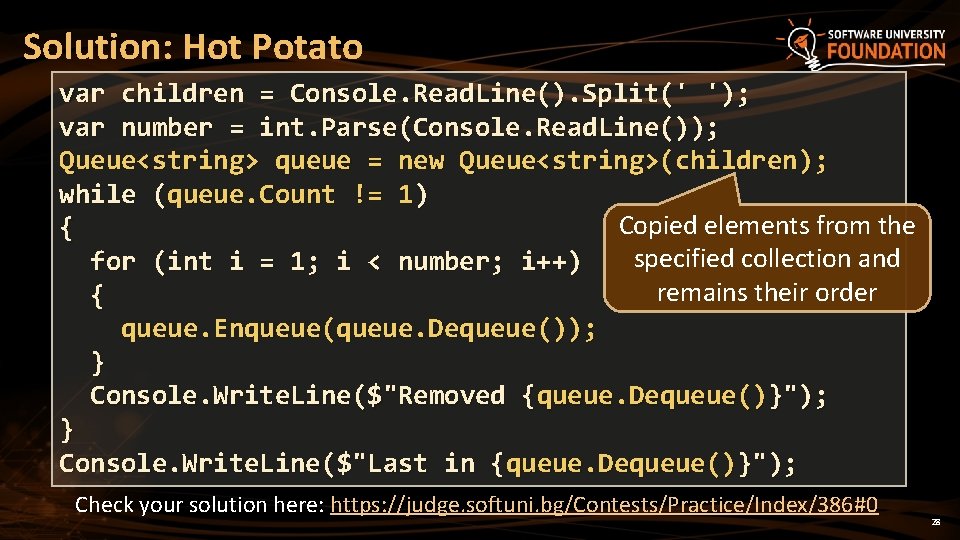
Solution: Hot Potato var children = Console. Read. Line(). Split(' '); var number = int. Parse(Console. Read. Line()); Queue<string> queue = new Queue<string>(children); while (queue. Count != 1) Copied elements from the { specified collection and for (int i = 1; i < number; i++) remains their order { queue. Enqueue(queue. Dequeue()); } Console. Write. Line($"Removed {queue. Dequeue()}"); } Console. Write. Line($"Last in {queue. Dequeue()}"); Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 28
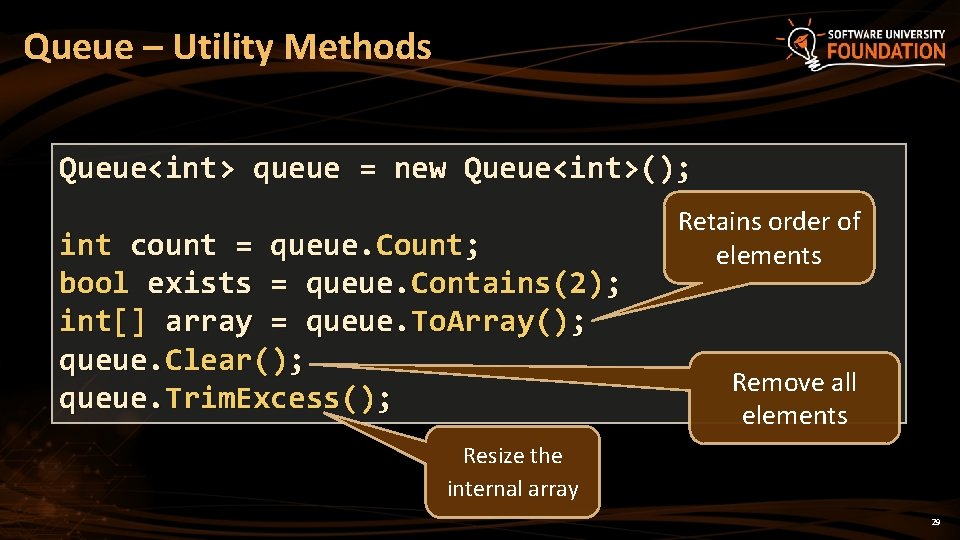
Queue – Utility Methods Queue<int> queue = new Queue<int>(); int count = queue. Count; bool exists = queue. Contains(2); int[] array = queue. To. Array(); queue. Clear(); queue. Trim. Excess(); Retains order of elements Remove all elements Resize the internal array 29
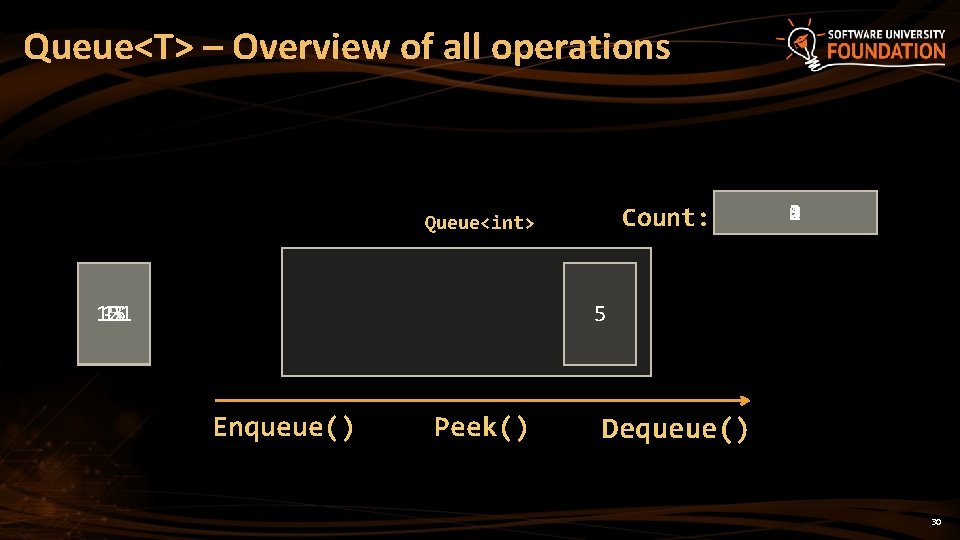
Queue<T> – Overview of all operations Count: Queue<int> 121 15 -3 5 4 2 1 0 3 5 Enqueue() Peek() Dequeue() 30
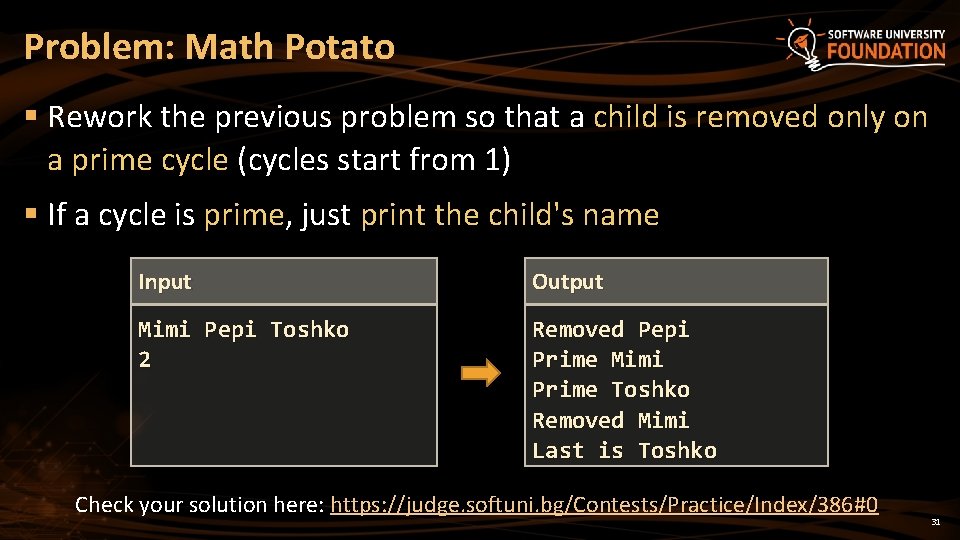
Problem: Math Potato § Rework the previous problem so that a child is removed only on a prime cycle (cycles start from 1) § If a cycle is prime, just print the child's name Input Output Mimi Pepi Toshko 2 Removed Pepi Prime Mimi Prime Toshko Removed Mimi Last is Toshko Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 31
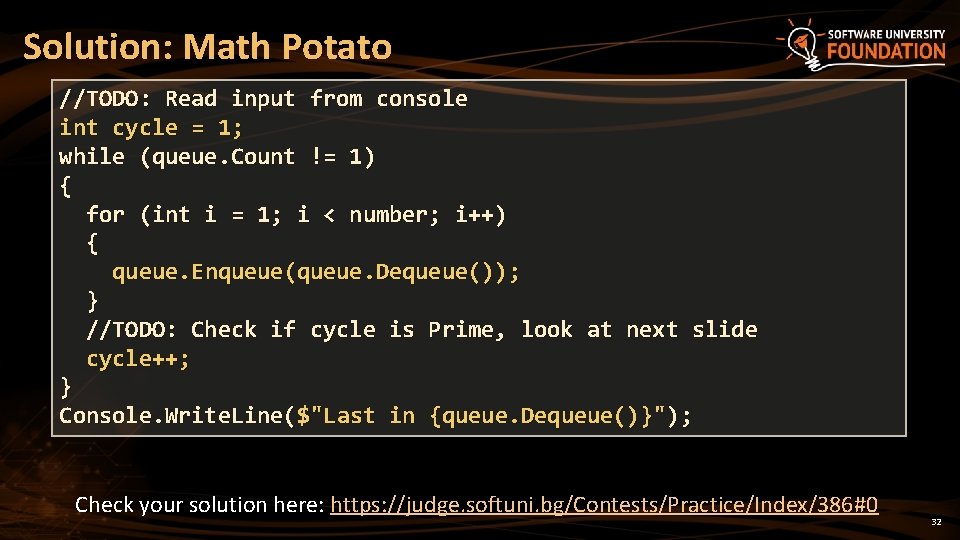
Solution: Math Potato //TODO: Read input from console int cycle = 1; while (queue. Count != 1) { for (int i = 1; i < number; i++) { queue. Enqueue(queue. Dequeue()); } //TODO: Check if cycle is Prime, look at next slide cycle++; } Console. Write. Line($"Last in {queue. Dequeue()}"); Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 32
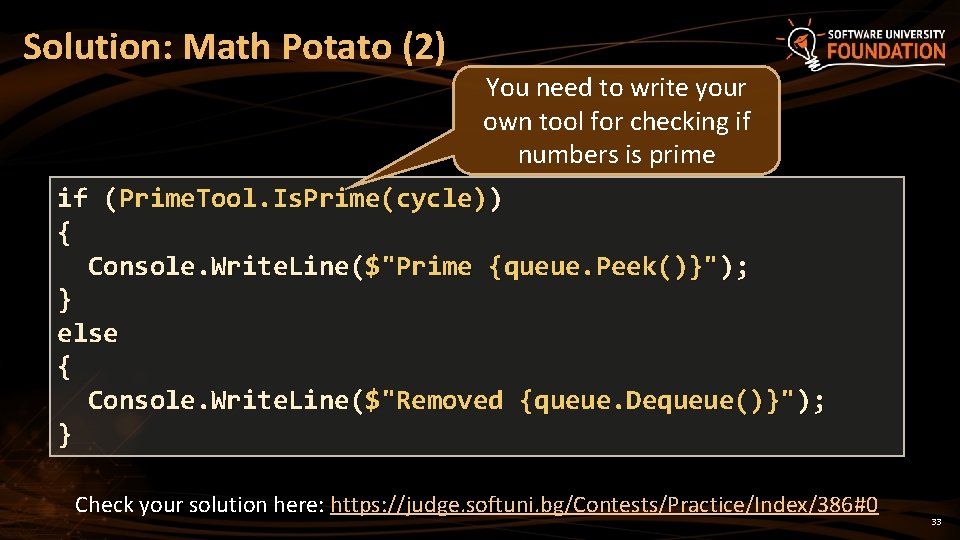
Solution: Math Potato (2) You need to write your own tool for checking if numbers is prime if (Prime. Tool. Is. Prime(cycle)) { Console. Write. Line($"Prime {queue. Peek()}"); } else { Console. Write. Line($"Removed {queue. Dequeue()}"); } Check your solution here: https: //judge. softuni. bg/Contests/Practice/Index/386#0 33
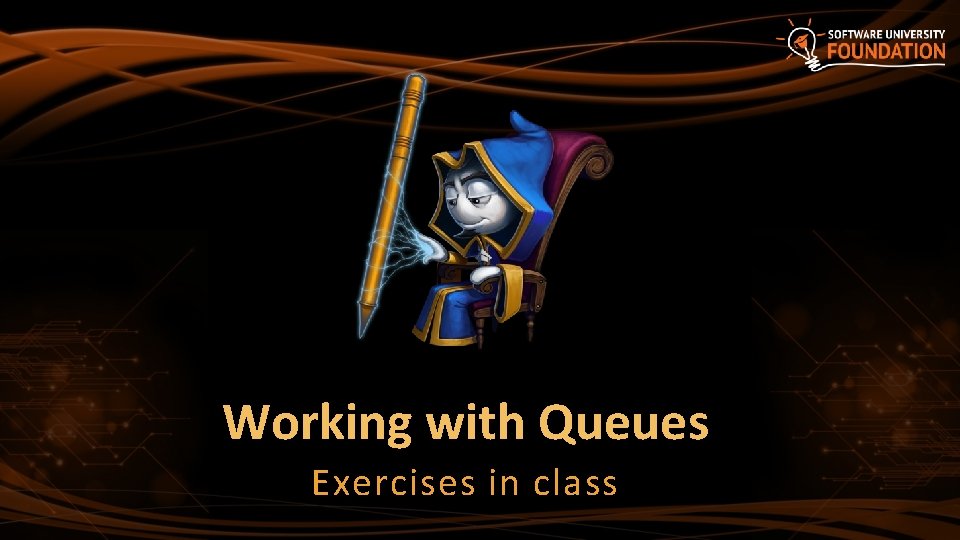
Working with Queues Exercises in class
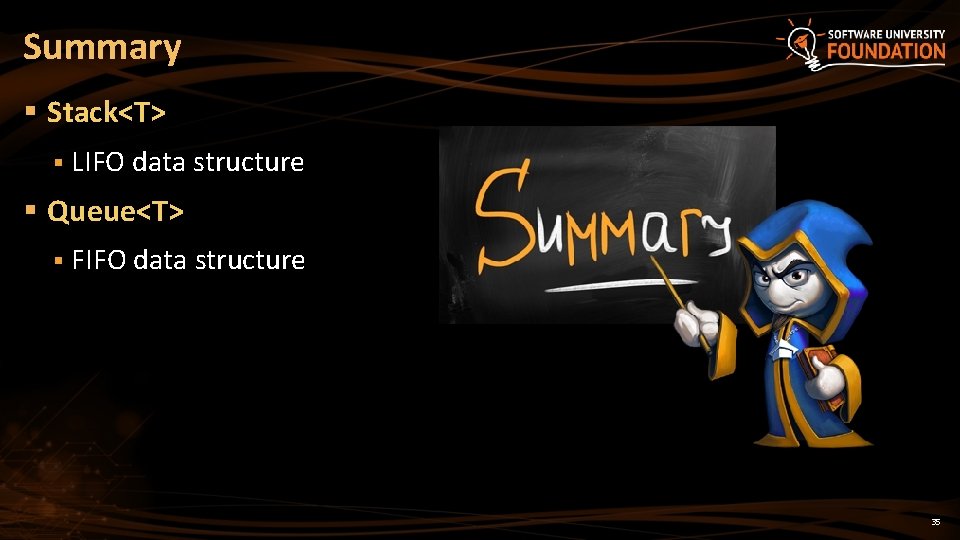
Summary § Stack<T> § LIFO data structure § Queue<T> § FIFO data structure 35
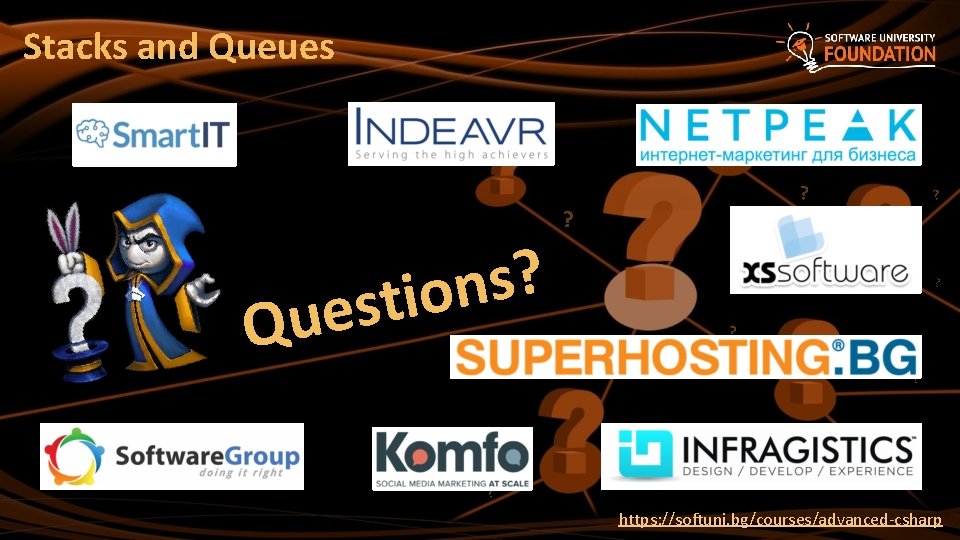
Stacks and Queues ? s n stio e u Q ? ? ? https: //softuni. bg/courses/advanced-csharp
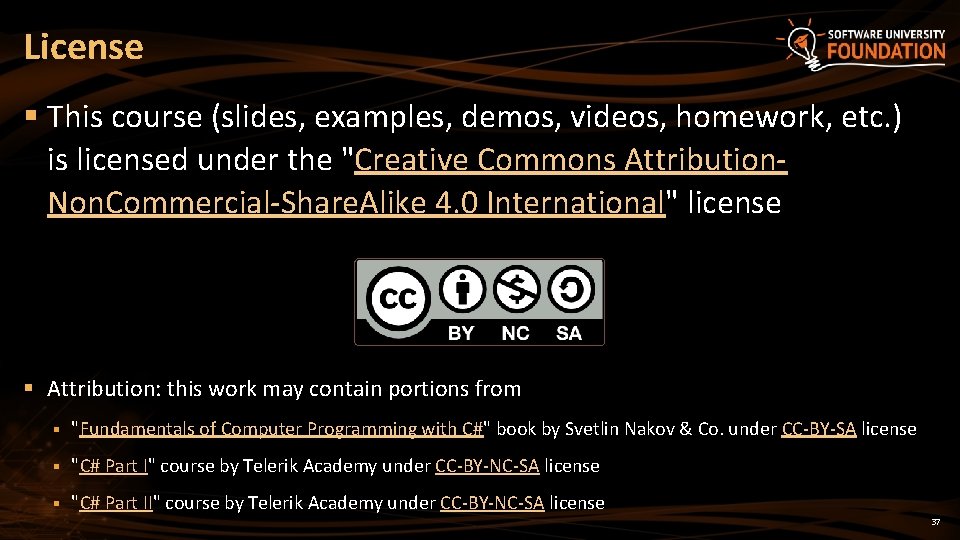
License § This course (slides, examples, demos, videos, homework, etc. ) is licensed under the "Creative Commons Attribution. Non. Commercial-Share. Alike 4. 0 International" license § Attribution: this work may contain portions from § "Fundamentals of Computer Programming with C#" book by Svetlin Nakov & Co. under CC-BY-SA license § "C# Part I" course by Telerik Academy under CC-BY-NC-SA license § "C# Part II" course by Telerik Academy under CC-BY-NC-SA license 37
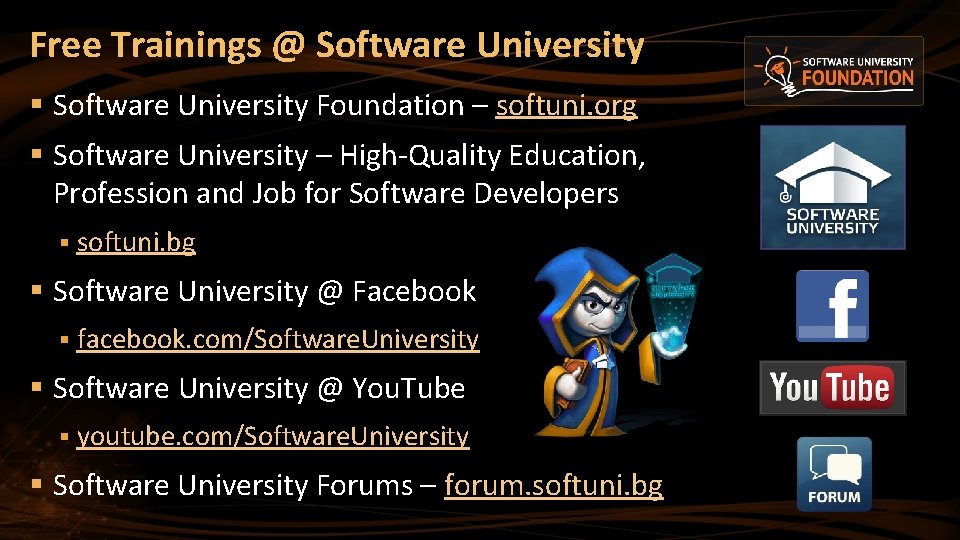
Free Trainings @ Software University § Software University Foundation – softuni. org § Software University – High-Quality Education, Profession and Job for Software Developers § softuni. bg § Software University @ Facebook § facebook. com/Software. University § Software University @ You. Tube § youtube. com/Software. University § Software University Forums – forum. softuni. bg
Empty python
Java stacks and queues
Exercises on stacks and queues
What are stacks
Bays and headlands
Priority queues: quiz
Representation of queues
Message queue in unix
Adaptable priority queue
Message queues in rtos
Applications of priority queues
Queue adalah jenis antrian
Queue head tail
Aganj
Pda
Terrace
Types of stacks
Data structures using java
Stacks+routined
6 stacks
Speed stacks spreads nationally in 1998.
.
Stacks internet
Angle stacks
Point processing and neighbourhood processing
Examples of secondary processing
What is interactive processing
Top down vs bottom up processing
Bottom up processing vs top down processing
Bottom up processing
Fractal image
Histogram processing in digital image processing
Parallel processing vs concurrent processing
A generalization of unsharp masking is
پردازش تصویر
Morphological processing in digital image processing
Bottom down processing
Arithmetic and geometric sequences and series
10-2 practice arithmetic sequences and series answer key