SORTING Sorting is the process of rearranging a
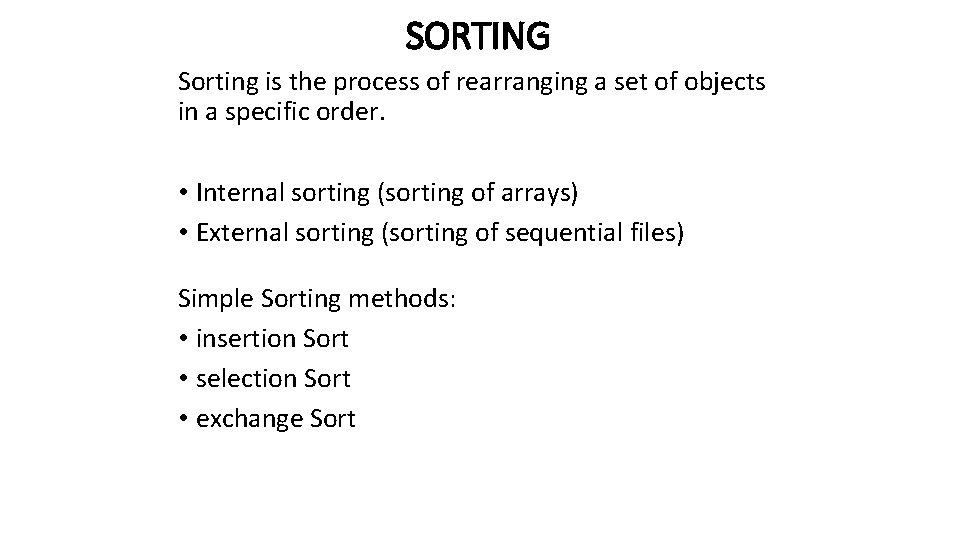
![Insertion sort The items are divided into a sorted sequence a[0]. . . a[i-1] Insertion sort The items are divided into a sorted sequence a[0]. . . a[i-1]](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-2.jpg)
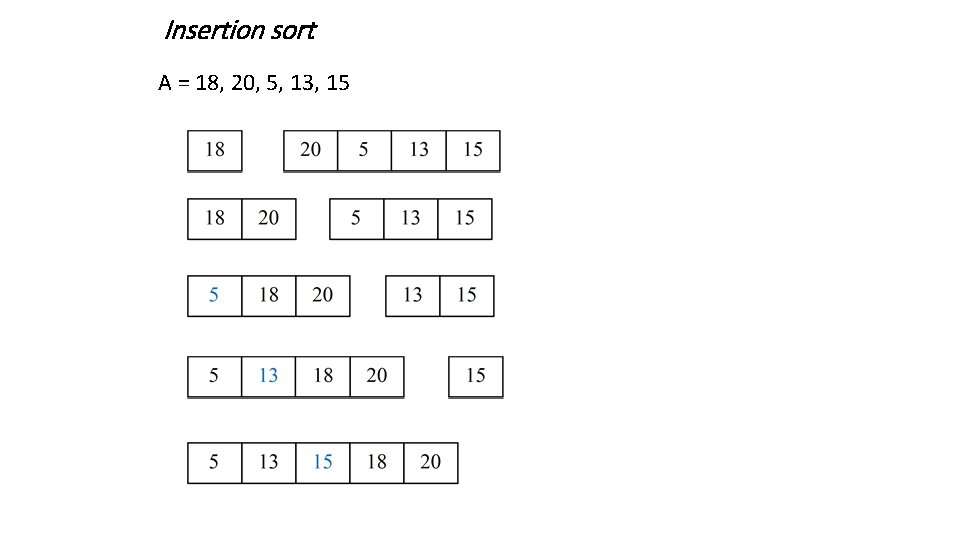
![Insertion sort for (i=1; i<n; i++) { j=i; temp=a[i]; while(j>0 && temp<a[j-1]) { a[j]=a[j-1]; Insertion sort for (i=1; i<n; i++) { j=i; temp=a[i]; while(j>0 && temp<a[j-1]) { a[j]=a[j-1];](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-4.jpg)
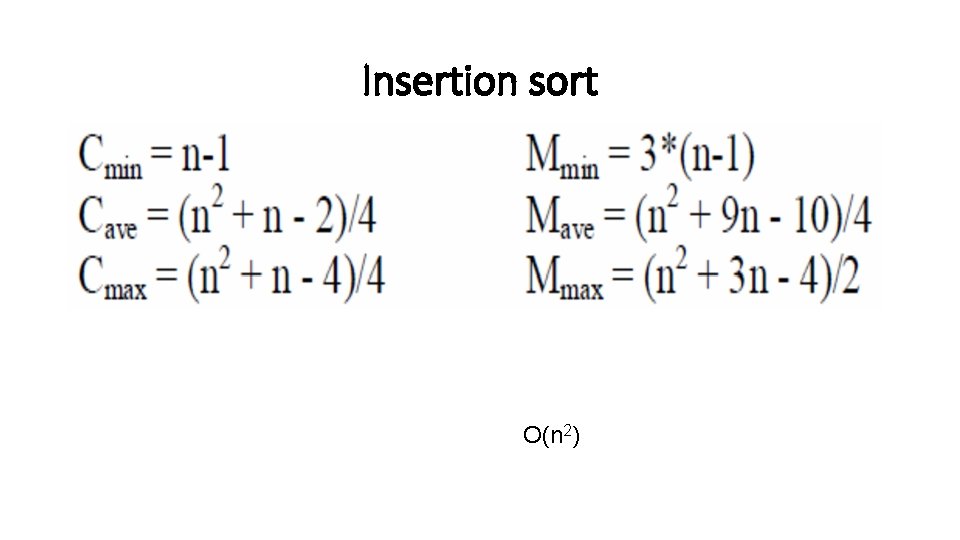
![Binary insertion sort void binsort(int a[], int n) {int item; for (int i=1; i<n; Binary insertion sort void binsort(int a[], int n) {int item; for (int i=1; i<n;](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-6.jpg)
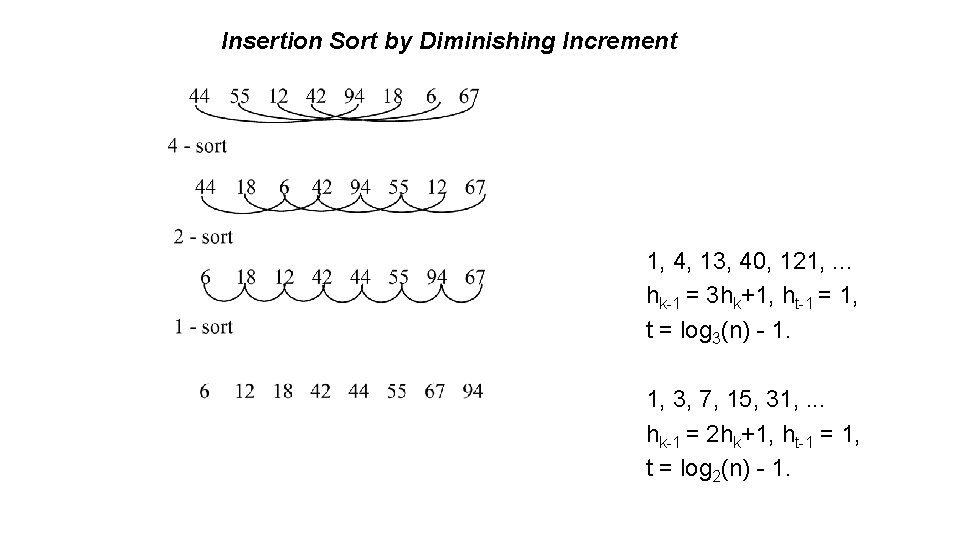
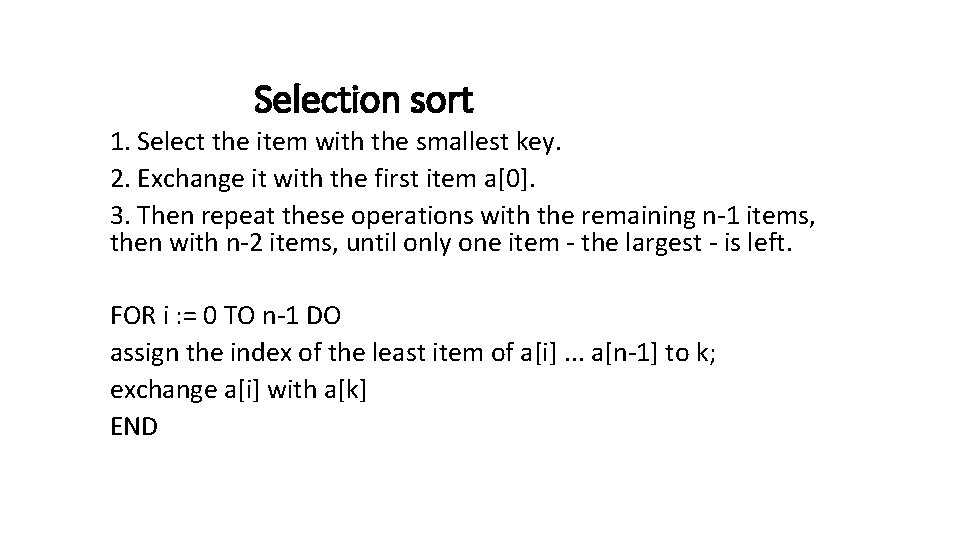
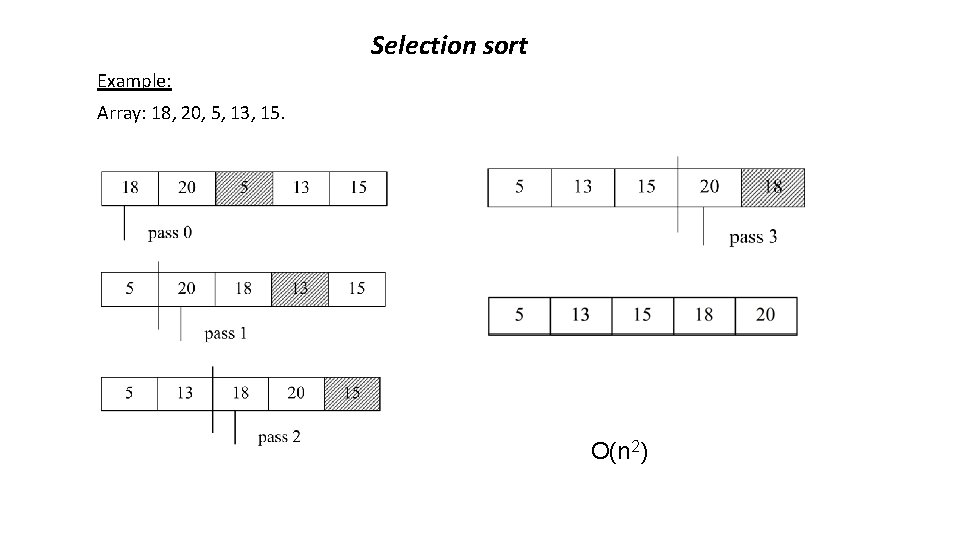
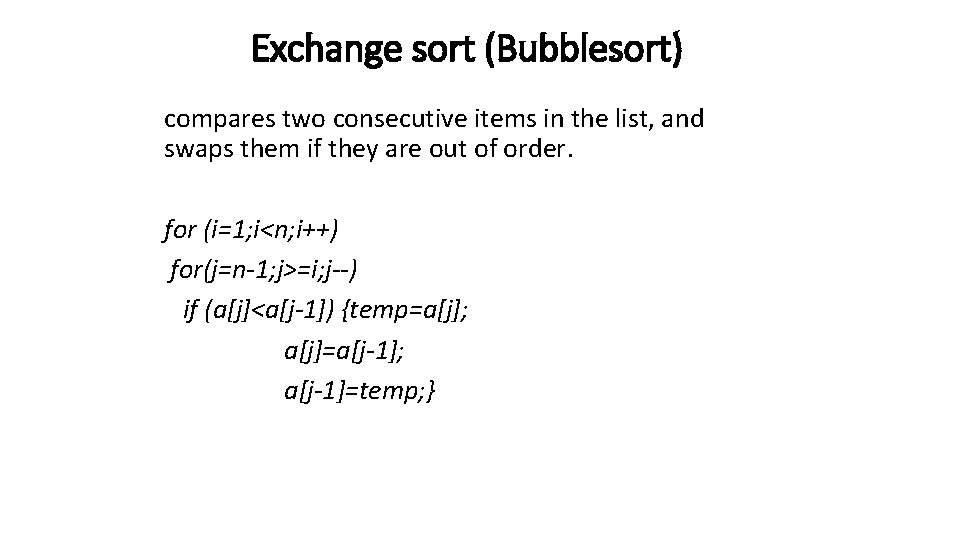
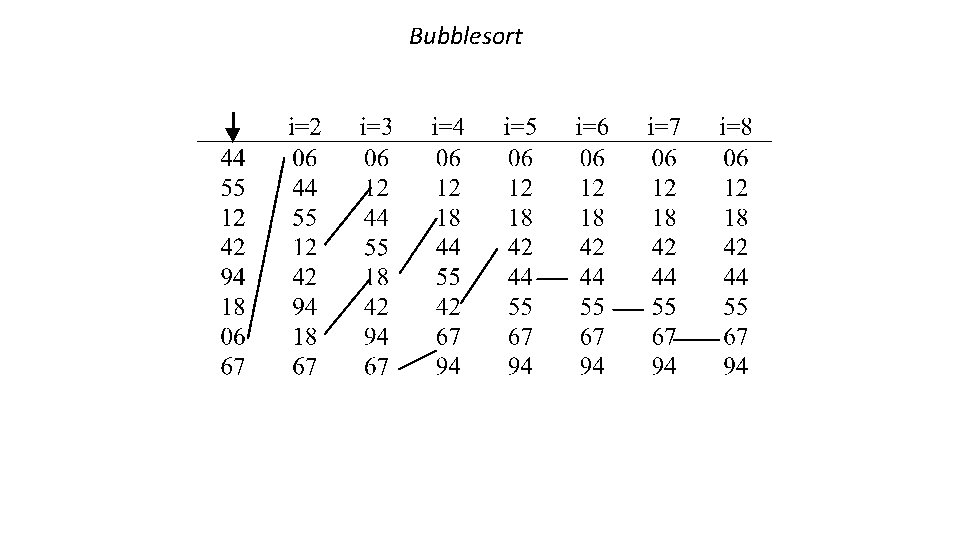
![int ilast; i=n-1; while (i>0) { ilast=0; for(j=0; j<i; j++) if (a[j]>a[j+1]) {temp=a[j]; a[j]=a[j+1]; int ilast; i=n-1; while (i>0) { ilast=0; for(j=0; j<i; j++) if (a[j]>a[j+1]) {temp=a[j]; a[j]=a[j+1];](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-12.jpg)
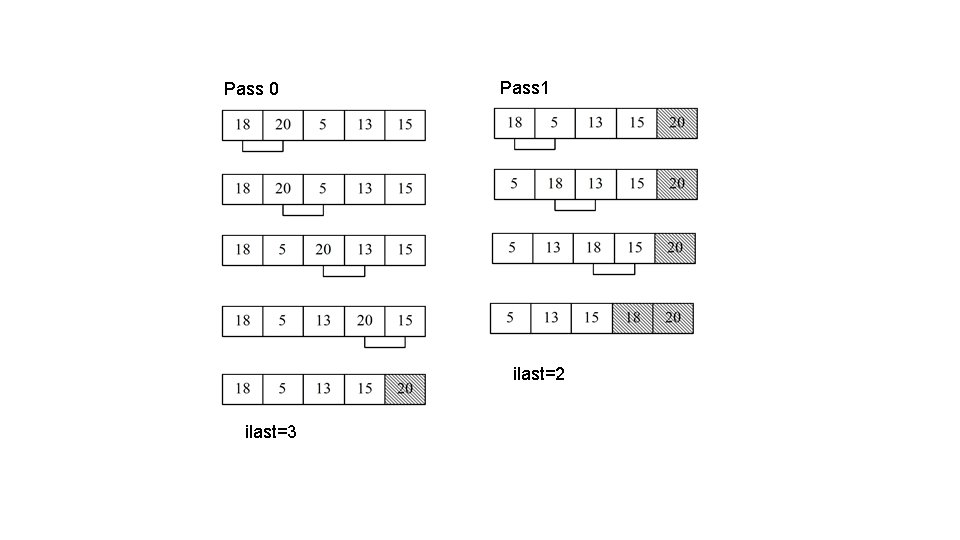
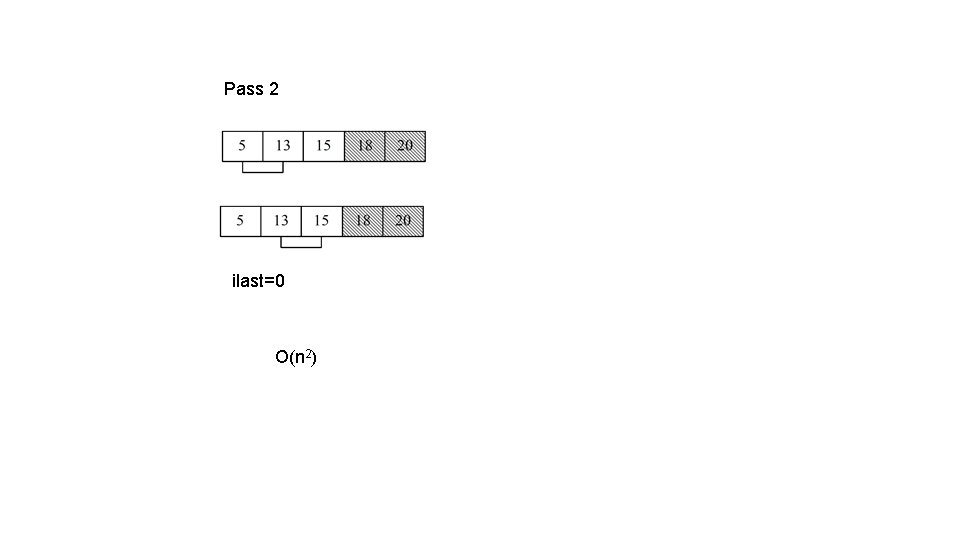
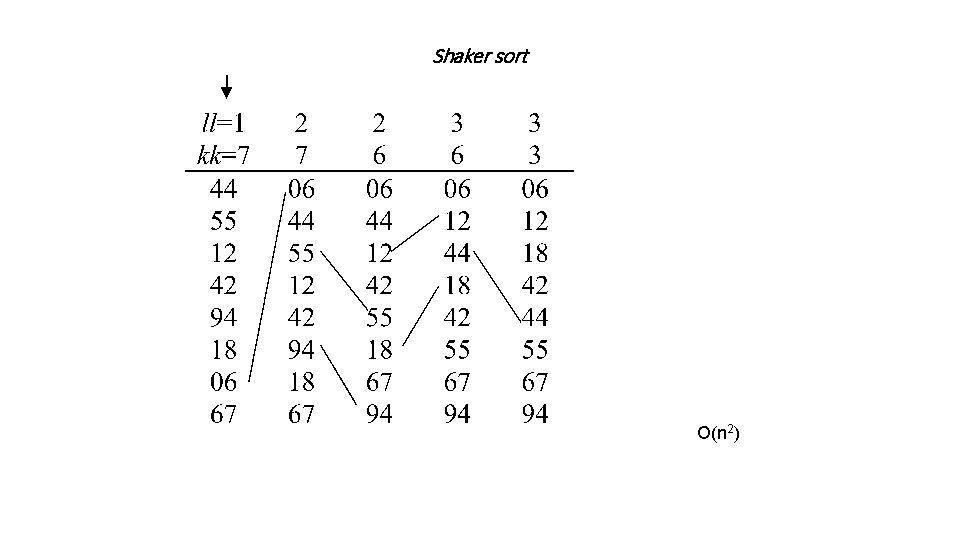
- Slides: 15
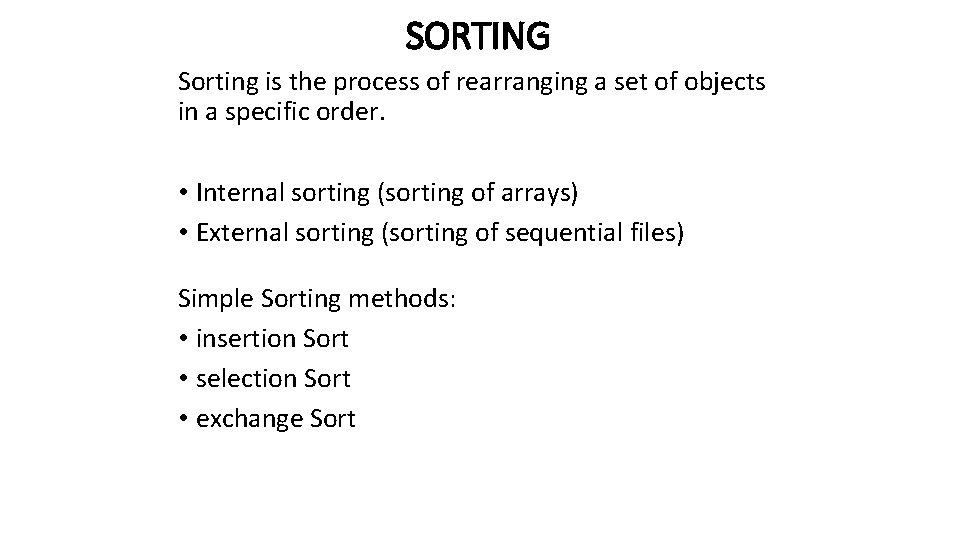
SORTING Sorting is the process of rearranging a set of objects in a specific order. • Internal sorting (sorting of arrays) • External sorting (sorting of sequential files) Simple Sorting methods: • insertion Sort • selection Sort • exchange Sort
![Insertion sort The items are divided into a sorted sequence a0 ai1 Insertion sort The items are divided into a sorted sequence a[0]. . . a[i-1]](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-2.jpg)
Insertion sort The items are divided into a sorted sequence a[0]. . . a[i-1] and a source sequence a[i]. . . a[n-1]. FOR i : = 1 TO n-1 DO x : = a[i]; insert x at the appropriate place in a[0]. . . a[i] END
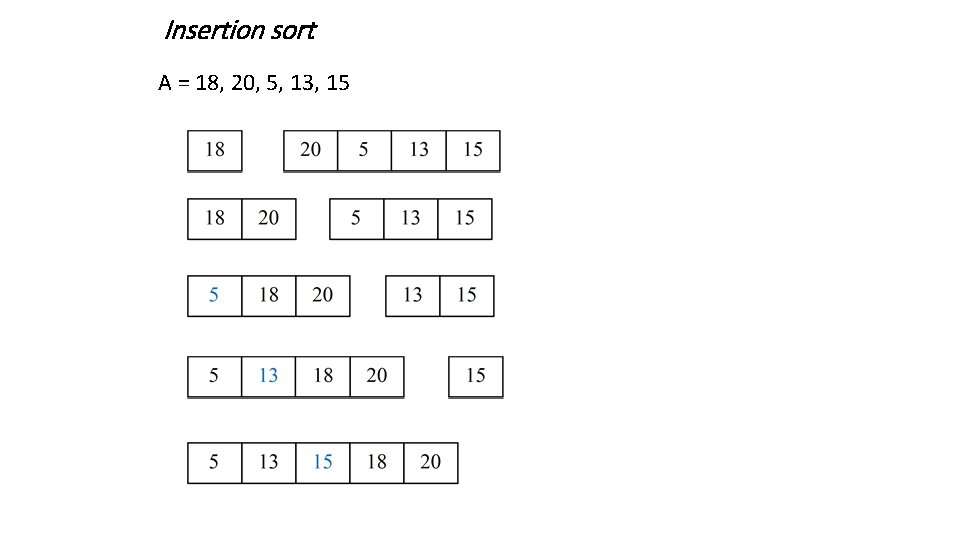
Insertion sort А = 18, 20, 5, 13, 15
![Insertion sort for i1 in i ji tempai whilej0 tempaj1 ajaj1 Insertion sort for (i=1; i<n; i++) { j=i; temp=a[i]; while(j>0 && temp<a[j-1]) { a[j]=a[j-1];](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-4.jpg)
Insertion sort for (i=1; i<n; i++) { j=i; temp=a[i]; while(j>0 && temp<a[j-1]) { a[j]=a[j-1]; j--; } a[j]=temp; } //i determines sequence a[0]. . . a[i] //moving // insert
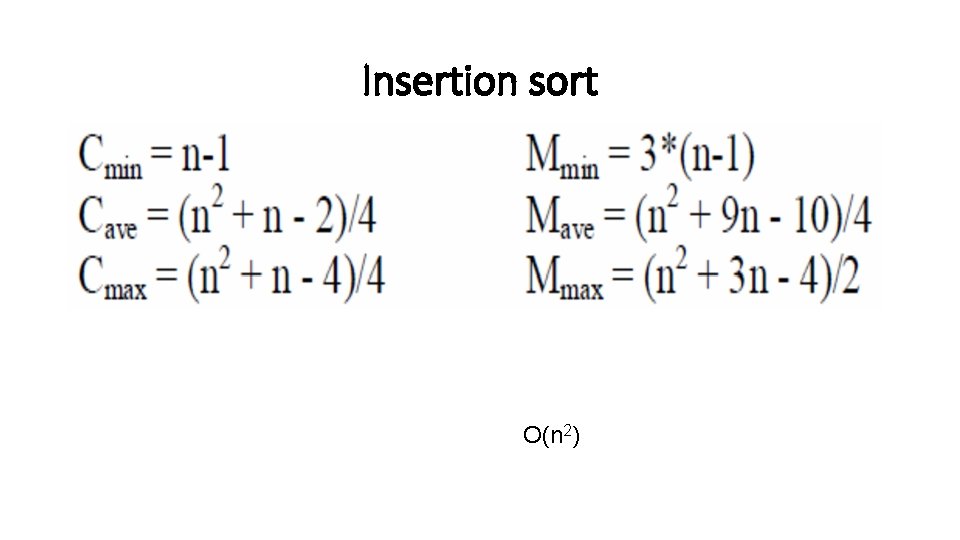
Insertion sort O(n 2)
![Binary insertion sort void binsortint a int n int item for int i1 in Binary insertion sort void binsort(int a[], int n) {int item; for (int i=1; i<n;](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-6.jpg)
Binary insertion sort void binsort(int a[], int n) {int item; for (int i=1; i<n; i++) { item=a[i]; int l=0; int r=i-1; while (l<=r) { int m=(l+r)/2; if (item<a[m]) r=m-1; else l=m+1; } for (int j=i-1; j>=l; j--) a[j+1]=a[j]; a[l]=item; }} O(n 2)
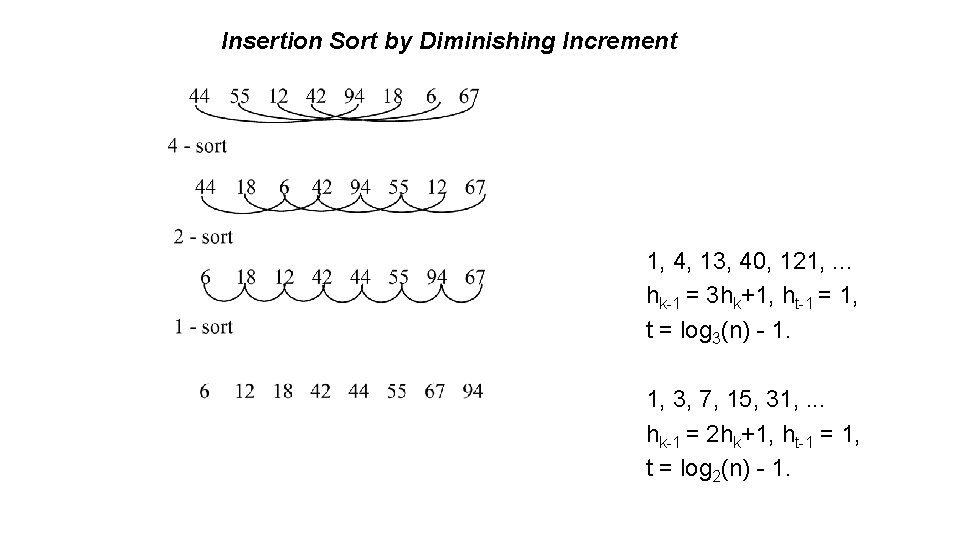
Insertion Sort by Diminishing Increment 1, 4, 13, 40, 121, . . . hk-1 = 3 hk+1, ht-1 = 1, t = log 3(n) - 1. 1, 3, 7, 15, 31, . . . hk-1 = 2 hk+1, ht-1 = 1, t = log 2(n) - 1.
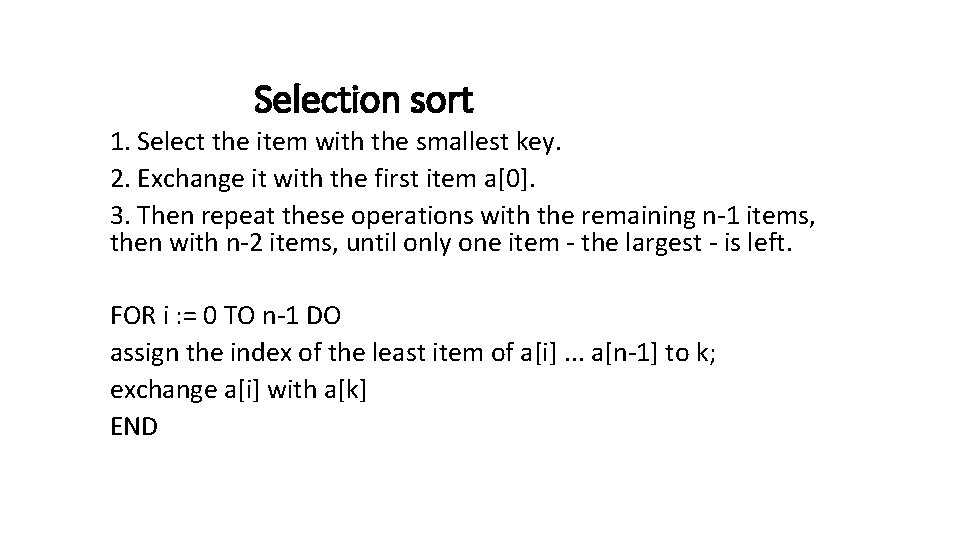
Selection sort 1. Select the item with the smallest key. 2. Exchange it with the first item a[0]. 3. Then repeat these operations with the remaining n-1 items, then with n-2 items, until only one item - the largest - is left. FOR i : = 0 TO n-1 DO assign the index of the least item of a[i]. . . a[n-1] to k; exchange a[i] with a[k] END
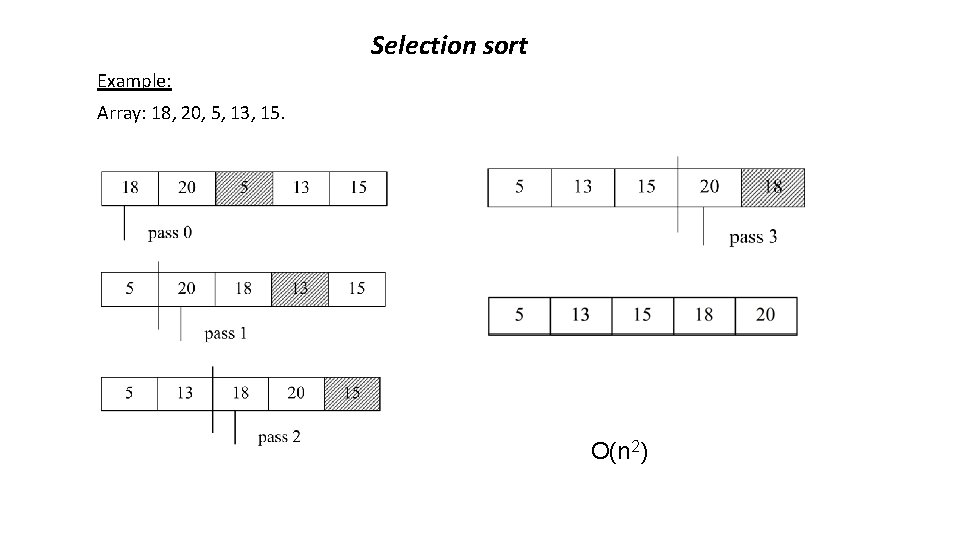
Selection sort Example: Array: 18, 20, 5, 13, 15. O(n 2)
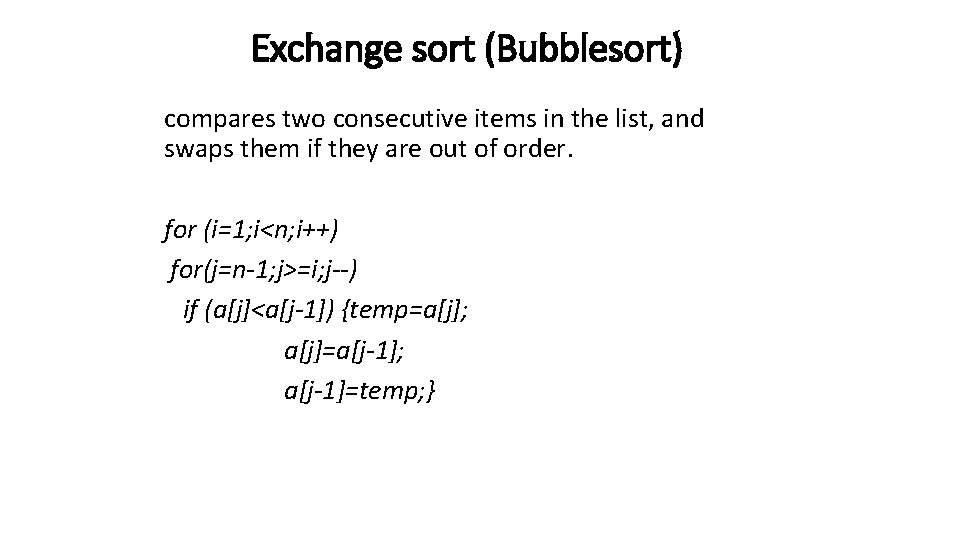
Exchange sort (Bubblesort) compares two consecutive items in the list, and swaps them if they are out of order. for (i=1; i<n; i++) for(j=n-1; j>=i; j--) if (a[j]<a[j-1]) {temp=a[j]; a[j]=a[j-1]; a[j-1]=temp; }
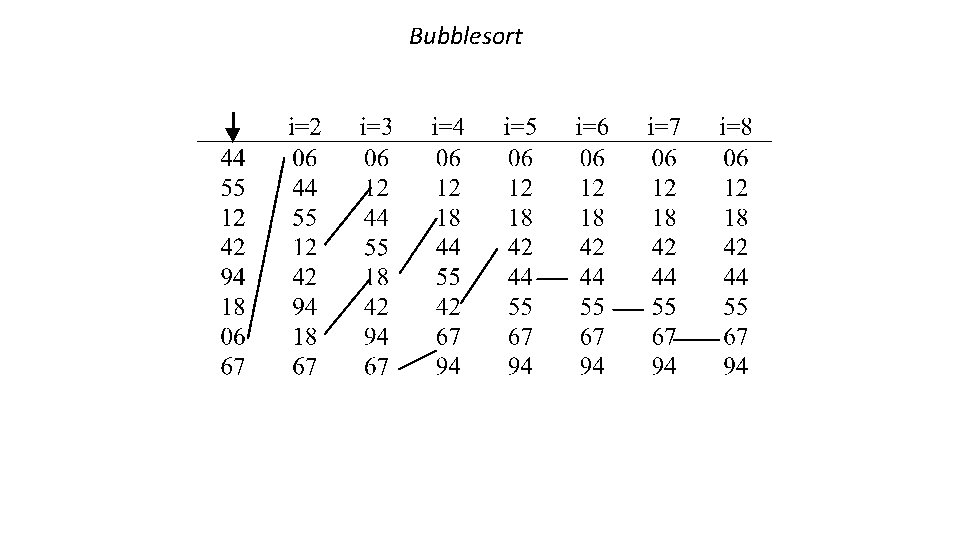
Bubblesort
![int ilast in1 while i0 ilast0 forj0 ji j if ajaj1 tempaj ajaj1 int ilast; i=n-1; while (i>0) { ilast=0; for(j=0; j<i; j++) if (a[j]>a[j+1]) {temp=a[j]; a[j]=a[j+1];](https://slidetodoc.com/presentation_image_h/2b98fd7bdf26f106c3dddc232c4ceea8/image-12.jpg)
int ilast; i=n-1; while (i>0) { ilast=0; for(j=0; j<i; j++) if (a[j]>a[j+1]) {temp=a[j]; a[j]=a[j+1]; a[j+1]=temp; ilast=j; } i=ilast; }
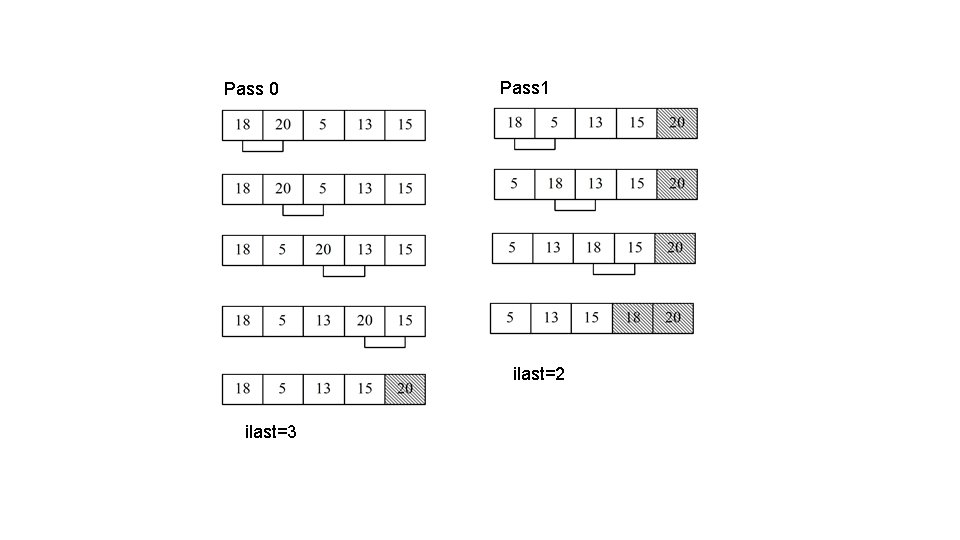
Pass 0 Pass 1 ilast=2 ilast=3
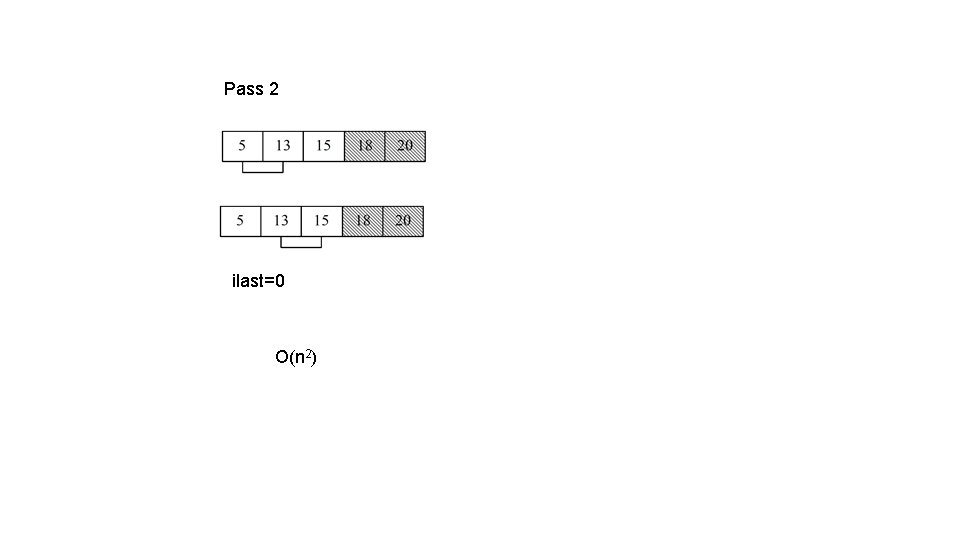
Pass 2 ilast=0 O(n 2)
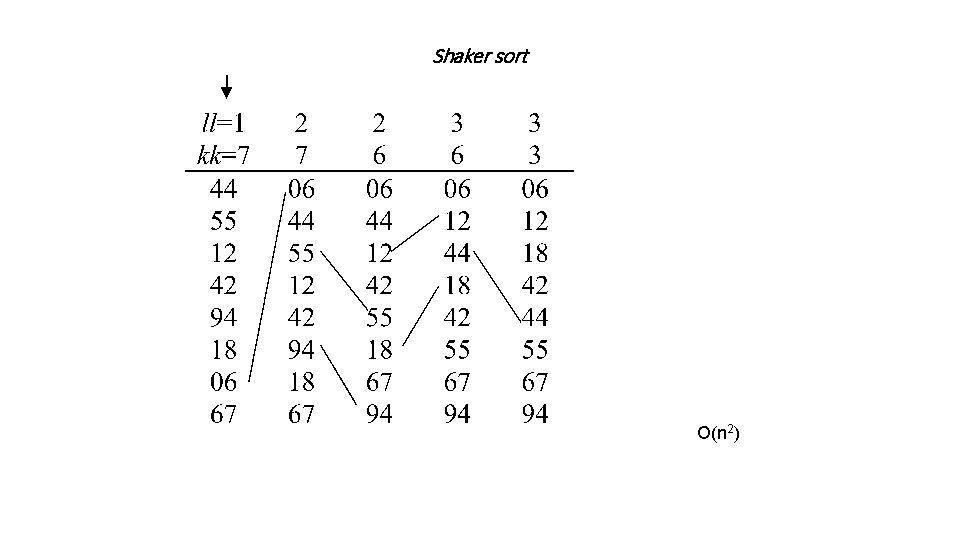
Shaker sort O(n 2)
Internal vs external sorting
Rearranging equations
Rearrangement of atoms
Research is rearranging facts
How to rearrange quadratic equation
Simultaneous equations dr frost
Rearranging kinematic equations
Rearranging equation calculator science
Dr frost quadratic graphs
Rearranging formulae dr frost
Hình ảnh bộ gõ cơ thể búng tay
Lp html
Bổ thể
Tỉ lệ cơ thể trẻ em
Gấu đi như thế nào
Tư thế worm breton