Serversideness Douglas Crockford Yahoo Inc Server Side Java
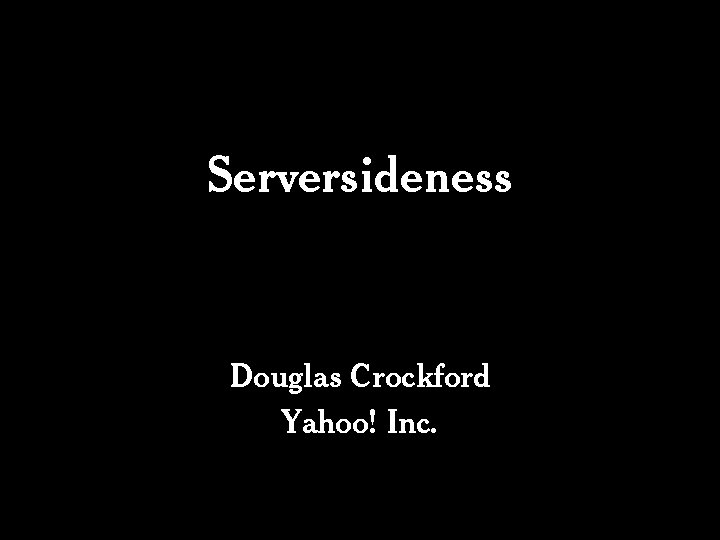
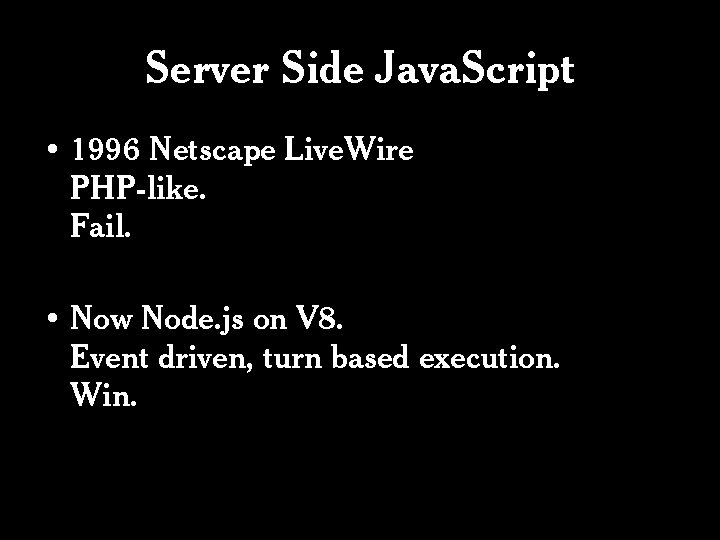
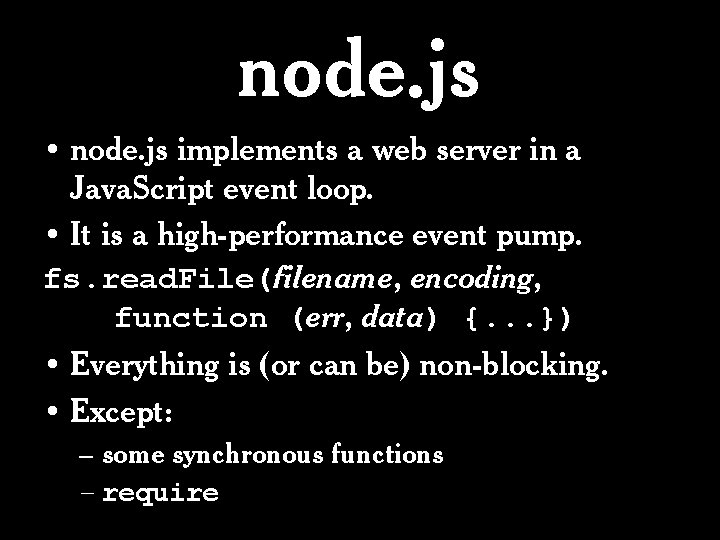
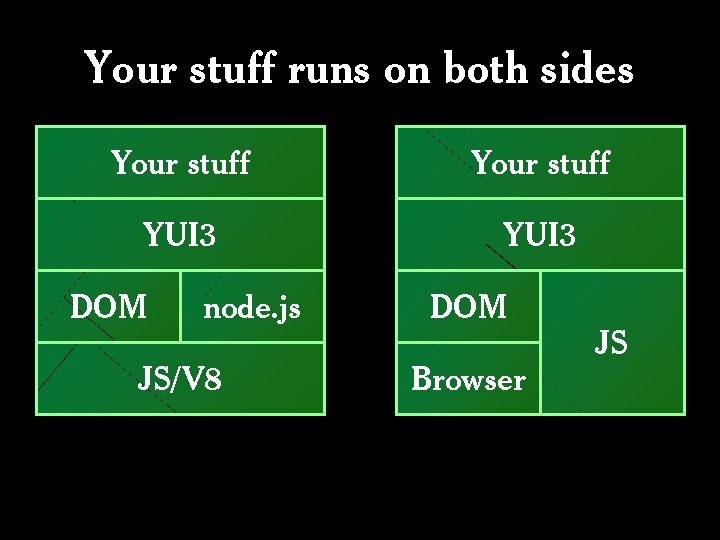
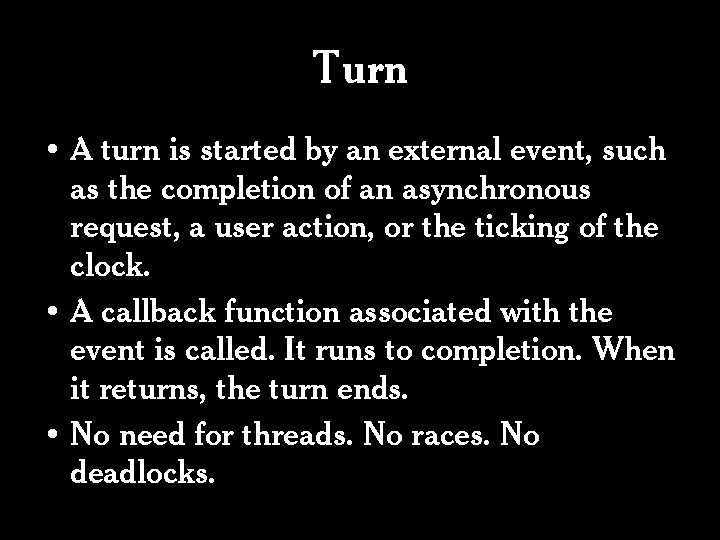
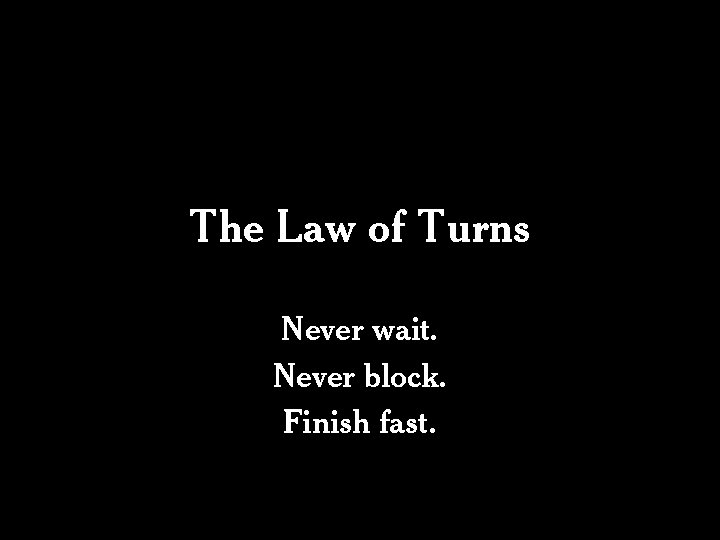
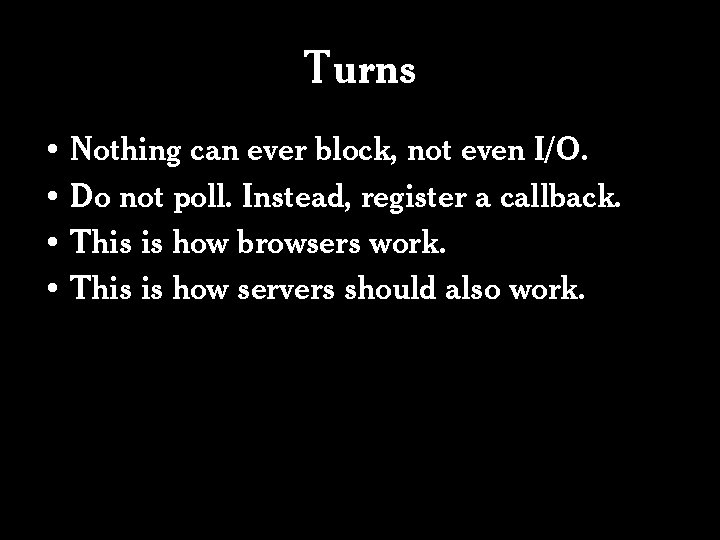
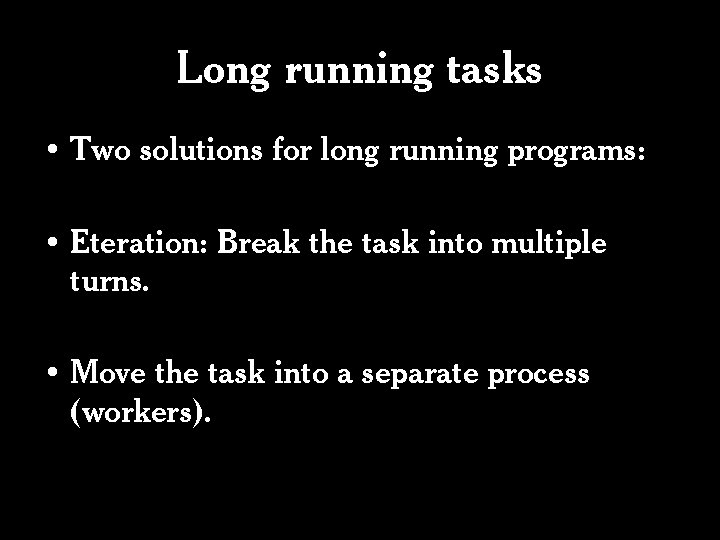
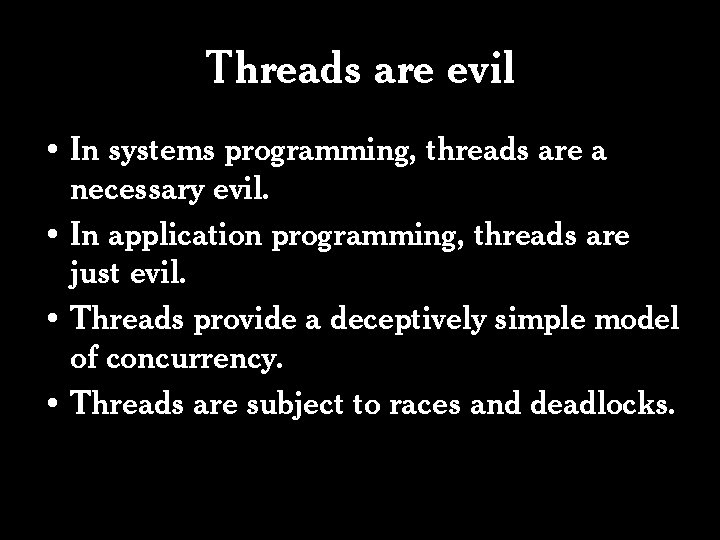
![Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length] Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length]](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-10.jpg)
![Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length] Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length]](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-11.jpg)
![my_array[my_array. length] = 'a'; length_a = my_array. length; my_array[length_a] = 'a'; if (length_a >= my_array[my_array. length] = 'a'; length_a = my_array. length; my_array[length_a] = 'a'; if (length_a >=](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-12.jpg)
![my_array[my_array. length] = 'a'; my_array[my_array. length] = 'b'; length_a = my_array. length; length_b = my_array[my_array. length] = 'a'; my_array[my_array. length] = 'b'; length_a = my_array. length; length_b =](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-13.jpg)
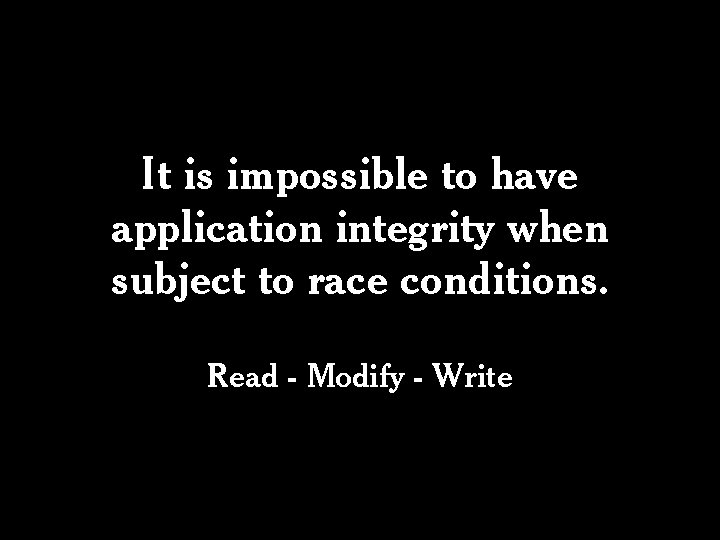
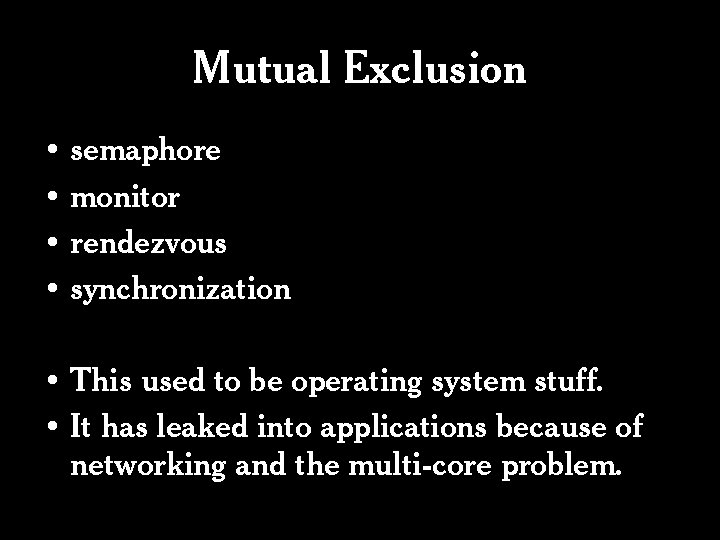
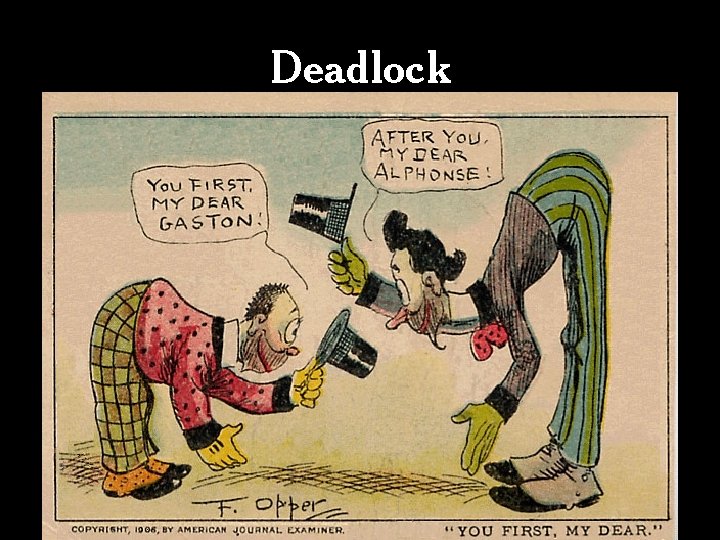
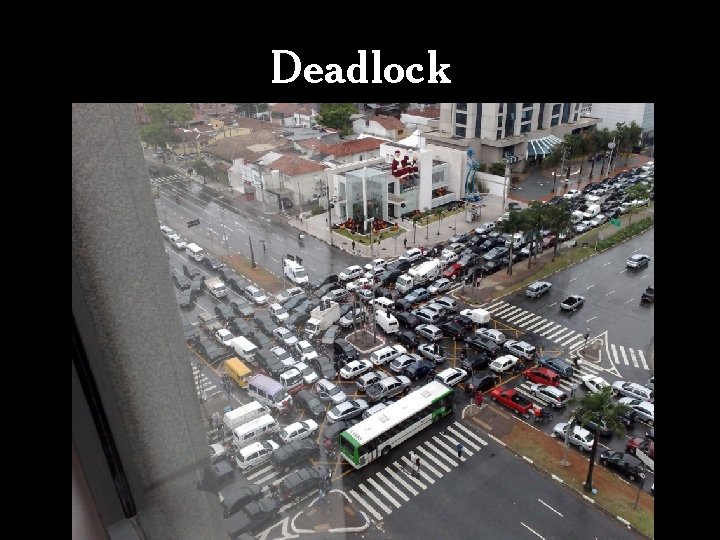
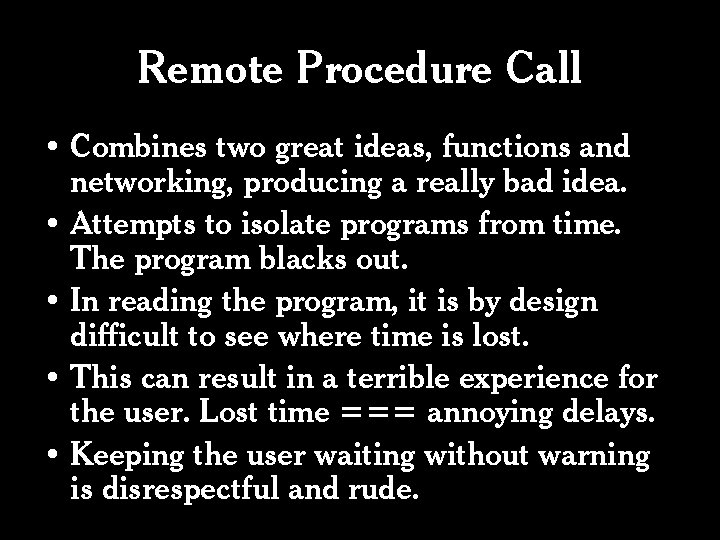
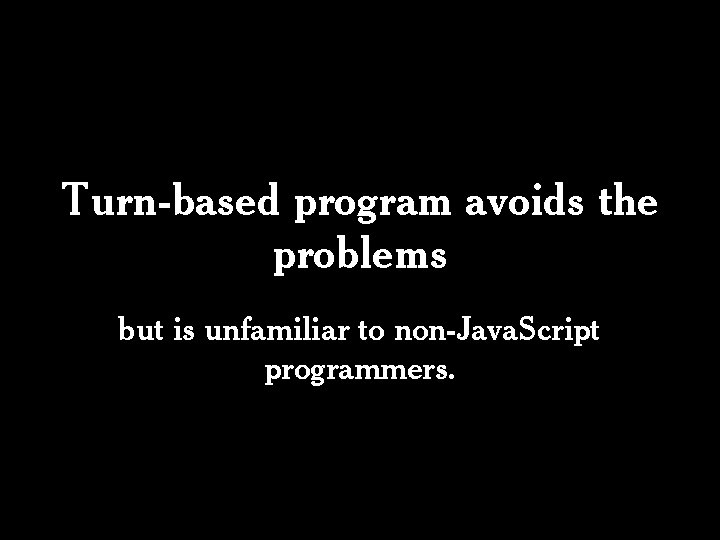
![Quiz 1 function funky(o) { o = null; } var x = []; funky(x); Quiz 1 function funky(o) { o = null; } var x = []; funky(x);](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-20.jpg)
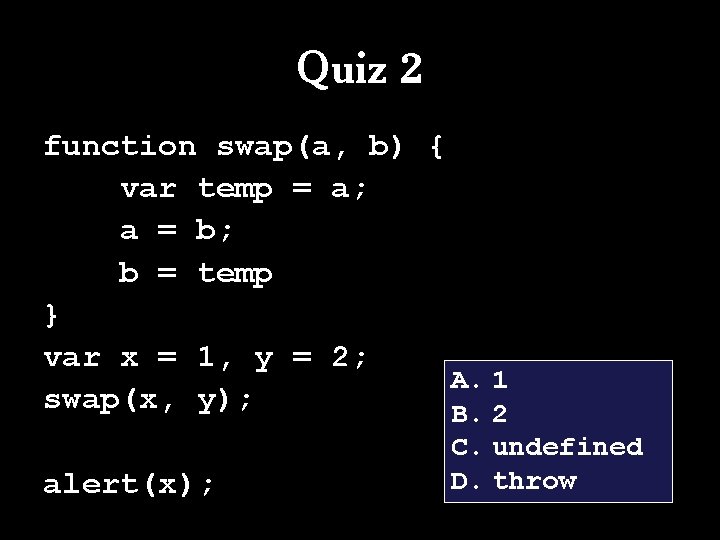
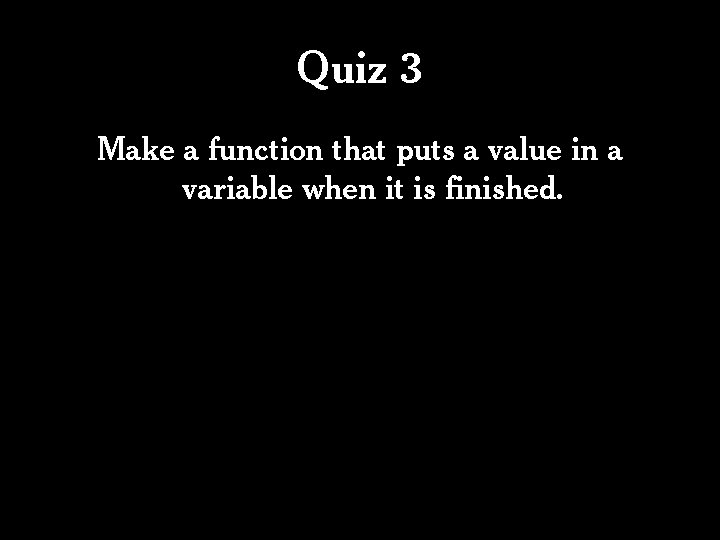
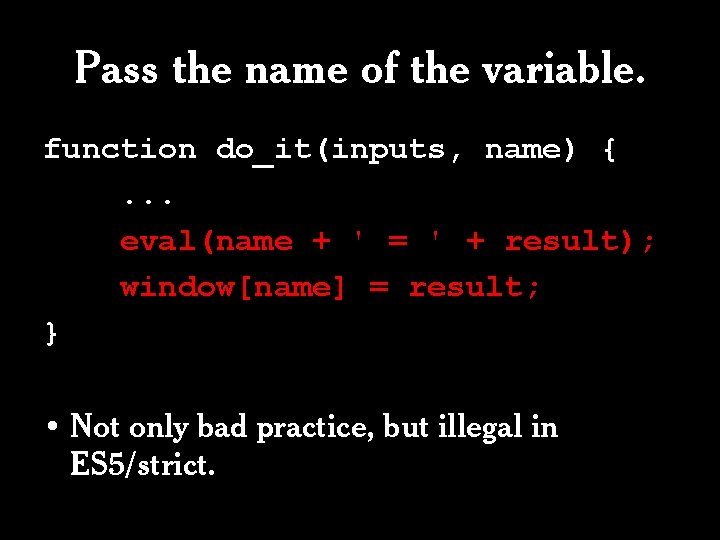
![function do_it(inputs, obj, name) {. . . obj[name] = result; } • Gives do-it function do_it(inputs, obj, name) {. . . obj[name] = result; } • Gives do-it](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-24.jpg)
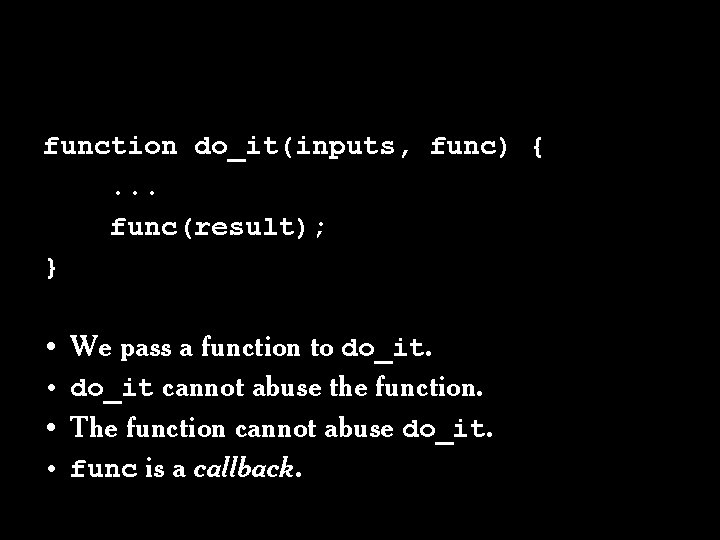
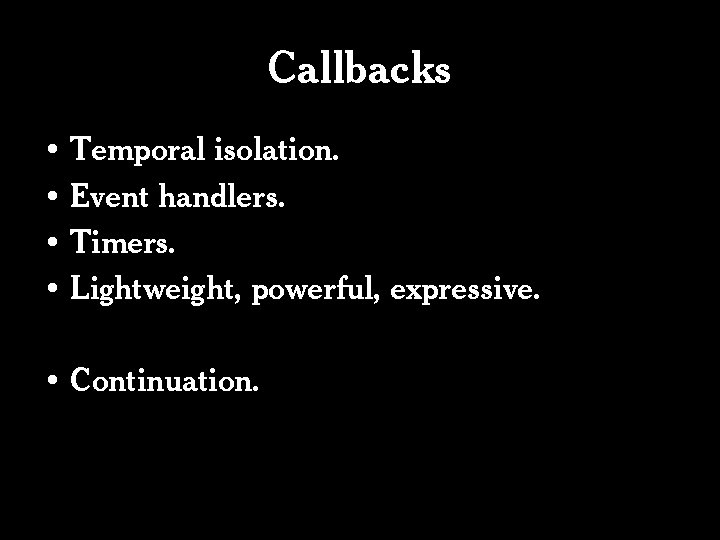
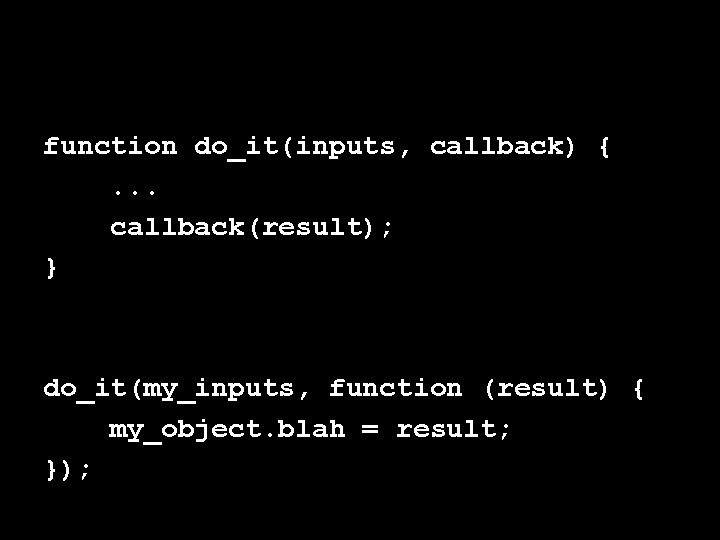
![Generalize. function storer(obj, name) { return function (result) { obj[name] = result; }; } Generalize. function storer(obj, name) { return function (result) { obj[name] = result; }; }](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-28.jpg)
![function storer_maker(obj) { return function(name) { return function (result) { obj[name] = result; }; function storer_maker(obj) { return function(name) { return function (result) { obj[name] = result; };](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-29.jpg)
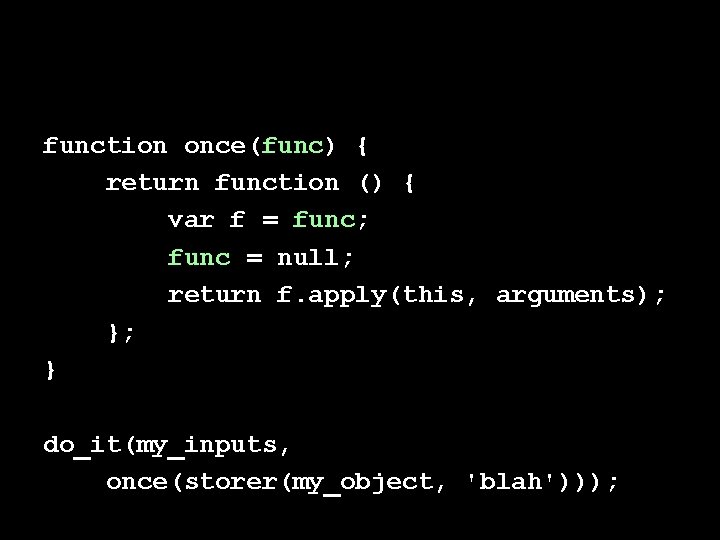
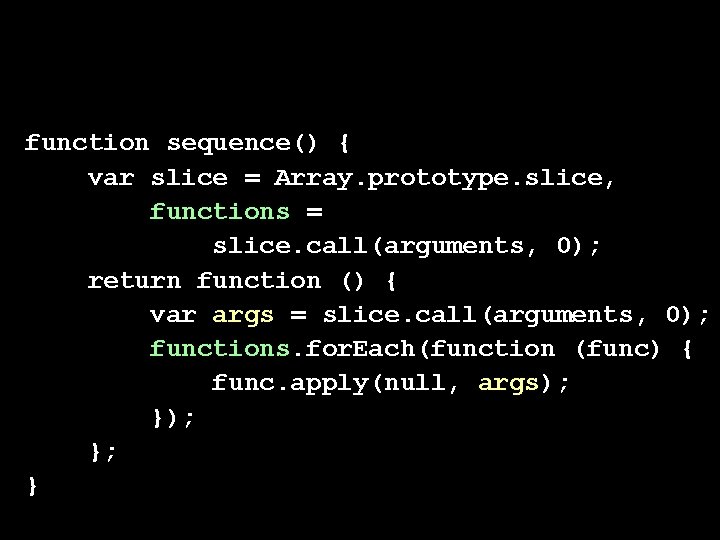
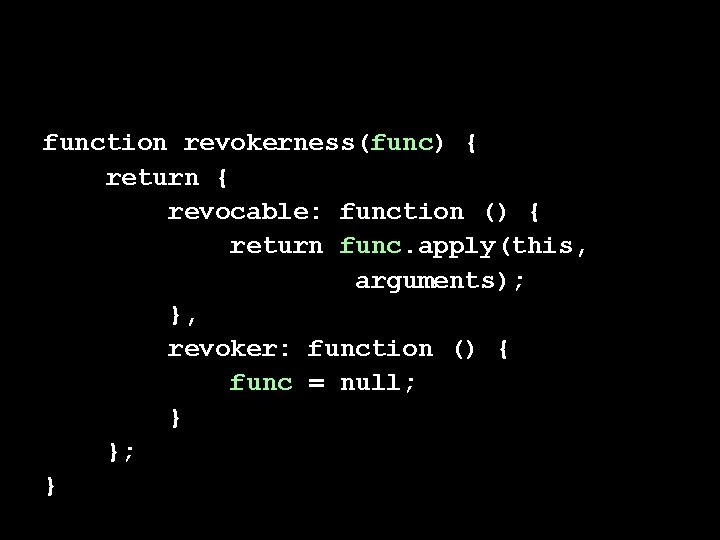
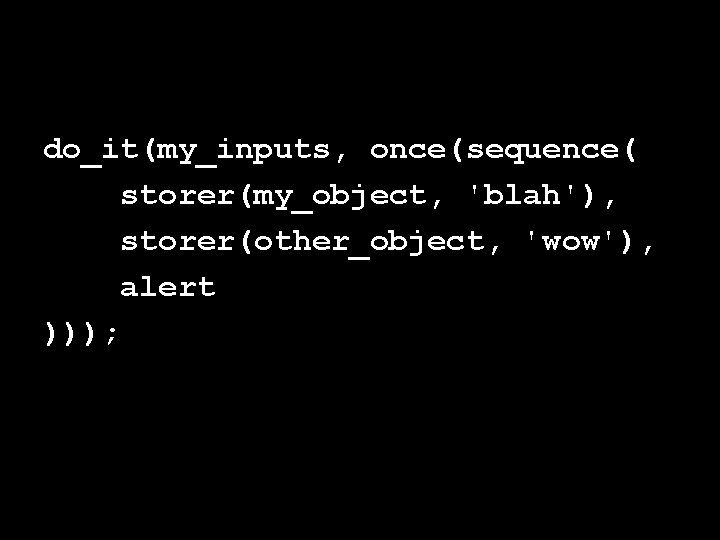
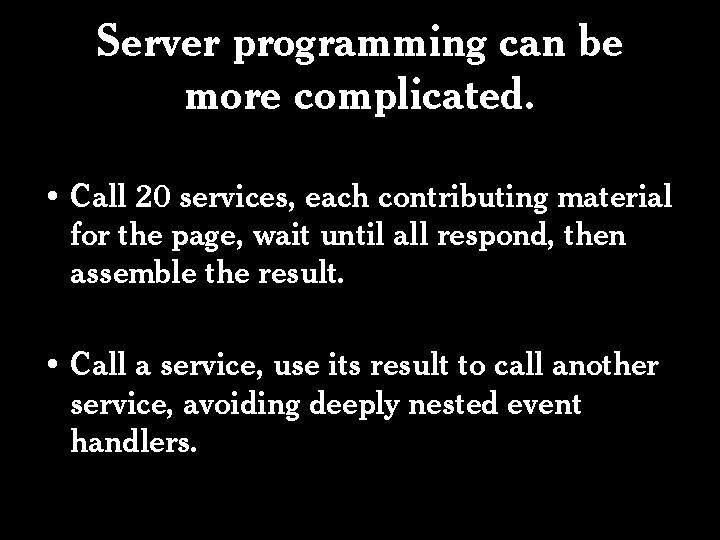
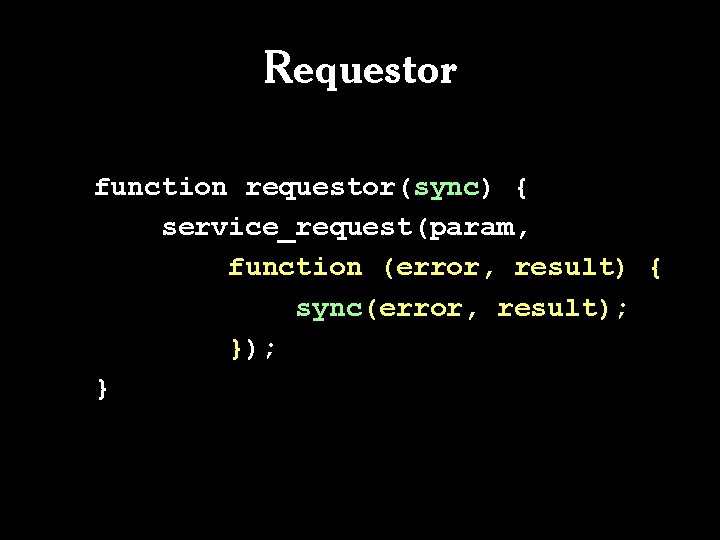
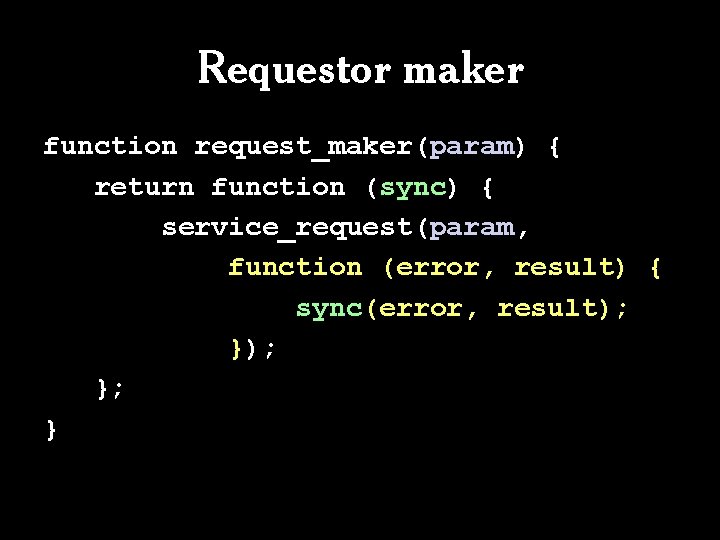
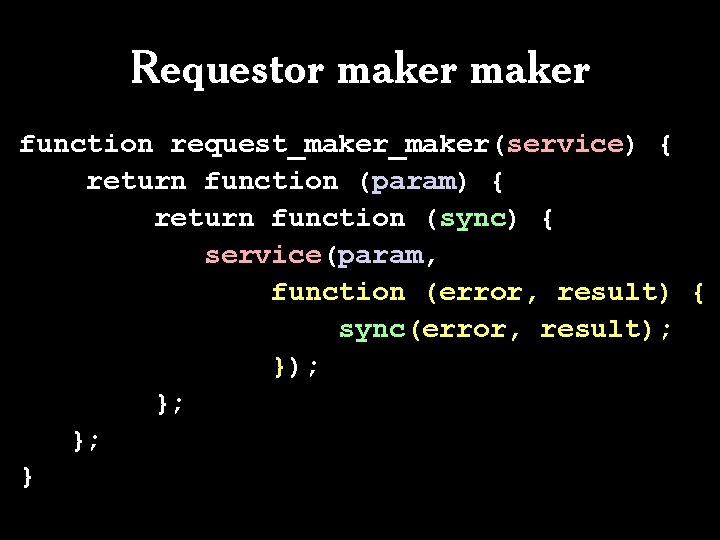
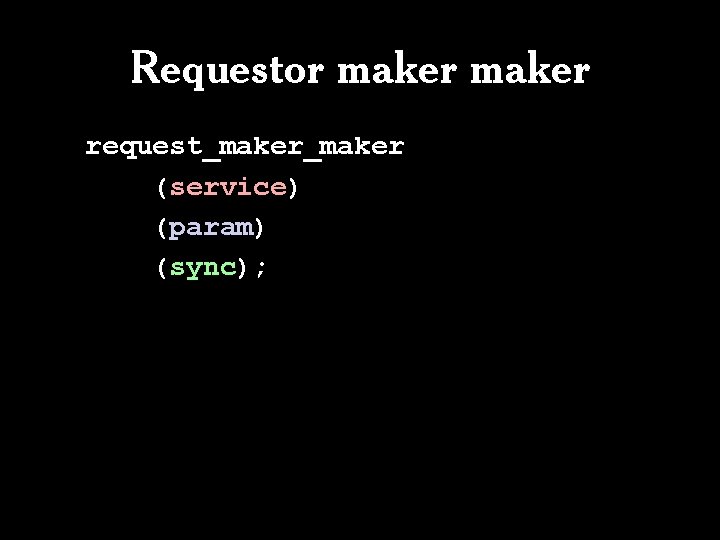
![Composition par([requestor…], sync, timeout); seq([requestor…], sync, timeout); map([requestor…], sync, timeout); Composition par([requestor…], sync, timeout); seq([requestor…], sync, timeout); map([requestor…], sync, timeout);](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-39.jpg)
![Composition function makers paror([requestor…], timeout) seqor([requestor…], timeout) mapor([requestor…], timeout) Composition function makers paror([requestor…], timeout) seqor([requestor…], timeout) mapor([requestor…], timeout)](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-40.jpg)
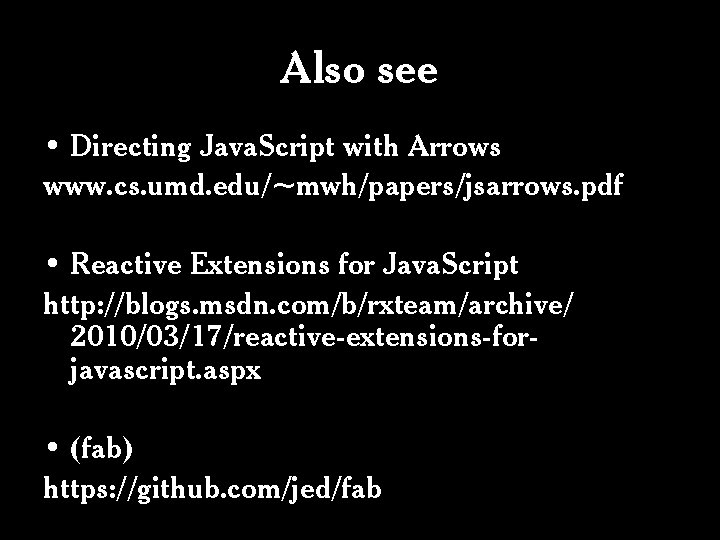
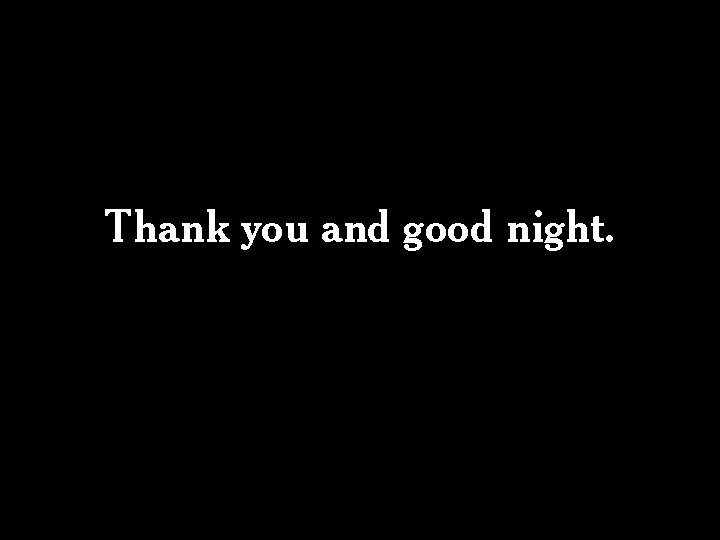
- Slides: 42
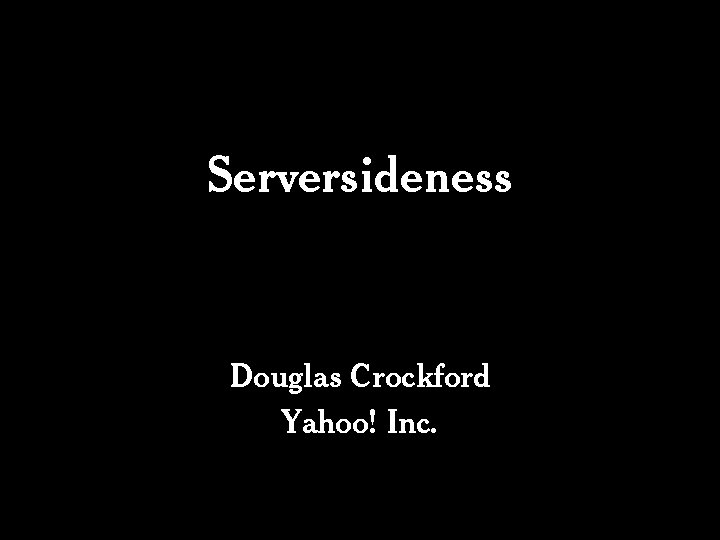
Serversideness Douglas Crockford Yahoo! Inc.
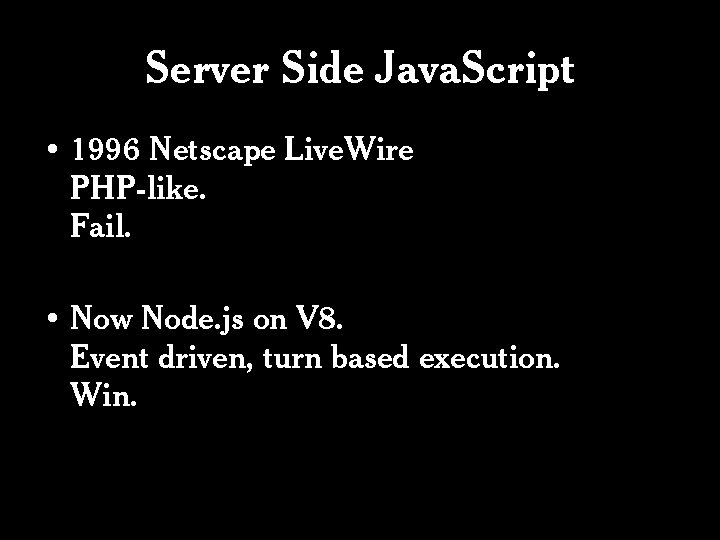
Server Side Java. Script • 1996 Netscape Live. Wire PHP-like. Fail. • Now Node. js on V 8. Event driven, turn based execution. Win.
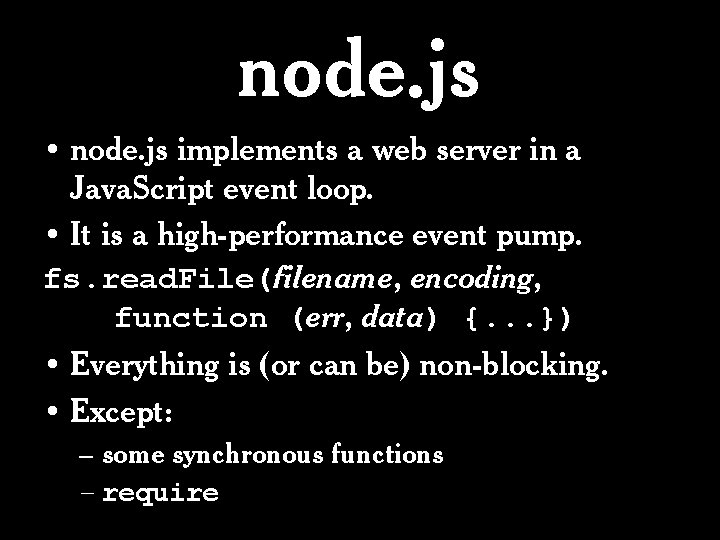
node. js • node. js implements a web server in a Java. Script event loop. • It is a high-performance event pump. fs. read. File(filename, encoding, function (err, data) {. . . }) • Everything is (or can be) non-blocking. • Except: – some synchronous functions – require
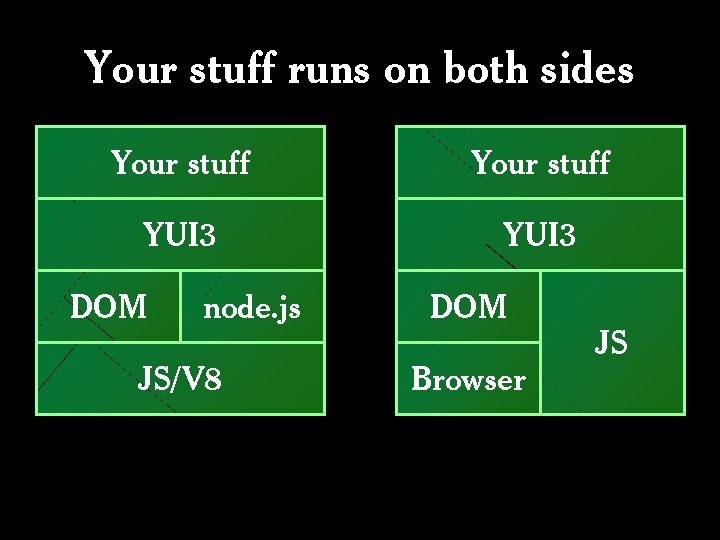
Your stuff runs on both sides Your stuff YUI 3 DOM node. js JS/V 8 DOM Browser JS
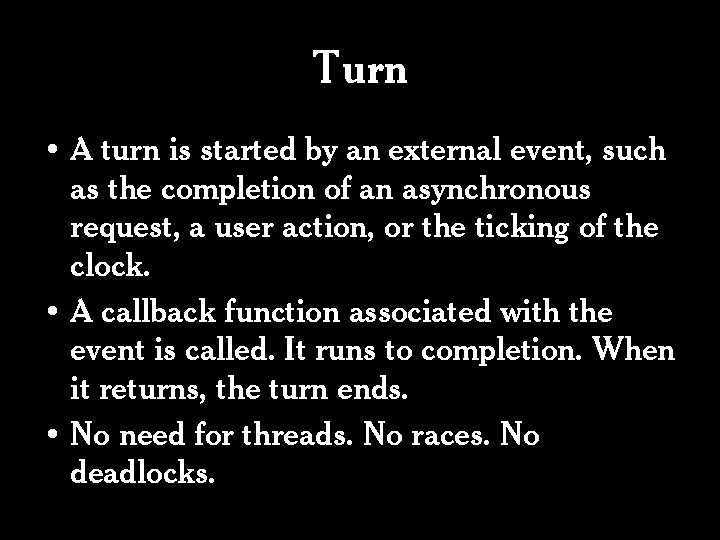
Turn • A turn is started by an external event, such as the completion of an asynchronous request, a user action, or the ticking of the clock. • A callback function associated with the event is called. It runs to completion. When it returns, the turn ends. • No need for threads. No races. No deadlocks.
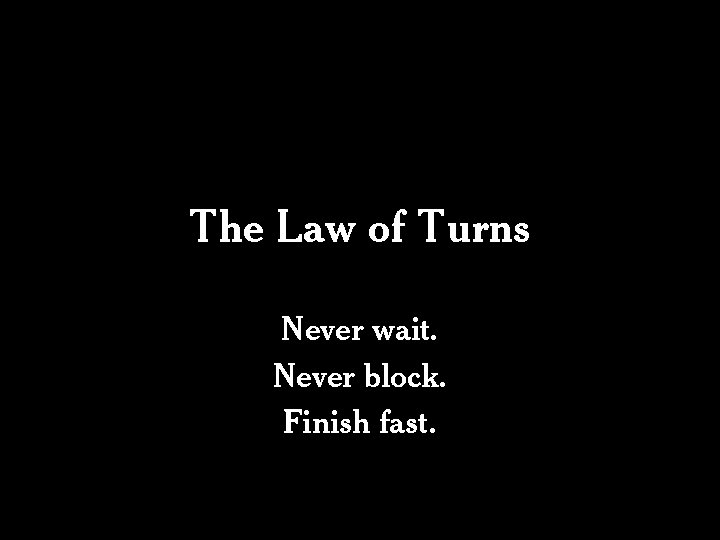
The Law of Turns Never wait. Never block. Finish fast.
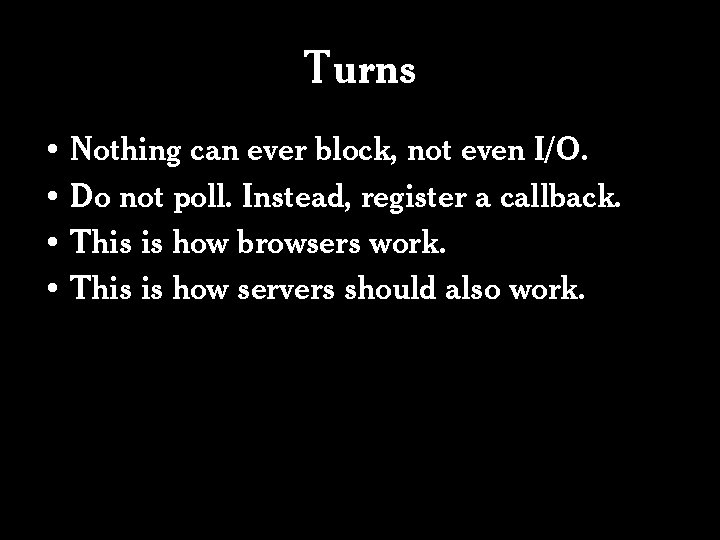
Turns • Nothing can ever block, not even I/O. • Do not poll. Instead, register a callback. • This is how browsers work. • This is how servers should also work.
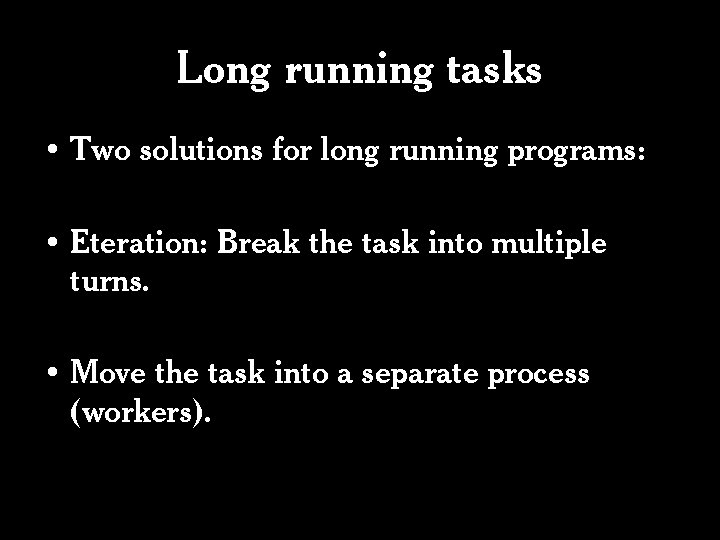
Long running tasks • Two solutions for long running programs: • Eteration: Break the task into multiple turns. • Move the task into a separate process (workers).
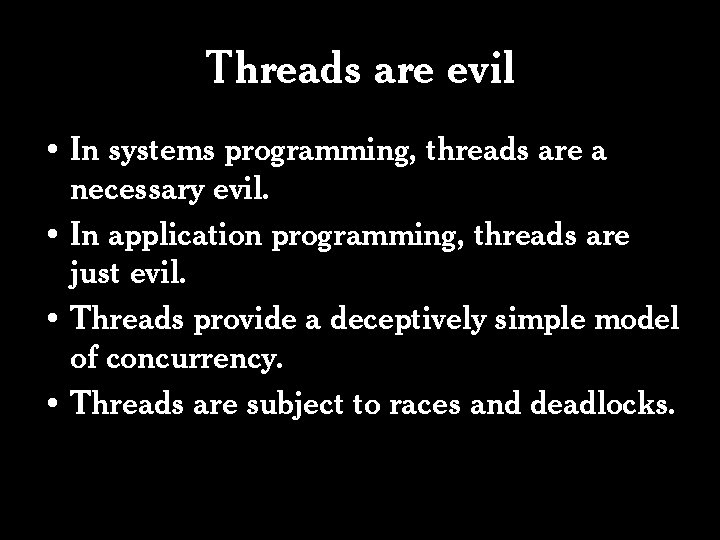
Threads are evil • In systems programming, threads are a necessary evil. • In application programming, threads are just evil. • Threads provide a deceptively simple model of concurrency. • Threads are subject to races and deadlocks.
![Two threads myarray 1 myarraymyarray length a 2 myarraymyarray length Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length]](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-10.jpg)
Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length] = 'b'; • ['a', 'b'] • ['b', 'a']
![Two threads myarray 1 myarraymyarray length a 2 myarraymyarray length Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length]](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-11.jpg)
Two threads • my_array = []; 1. my_array[my_array. length] = 'a'; 2. my_array[my_array. length] = 'b'; • • ['a', 'b'] ['b', 'a'] ['b']
![myarraymyarray length a lengtha myarray length myarraylengtha a if lengtha my_array[my_array. length] = 'a'; length_a = my_array. length; my_array[length_a] = 'a'; if (length_a >=](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-12.jpg)
my_array[my_array. length] = 'a'; length_a = my_array. length; my_array[length_a] = 'a'; if (length_a >= my_array. length) { my_array. length = length_a + 1; }
![myarraymyarray length a myarraymyarray length b lengtha myarray length lengthb my_array[my_array. length] = 'a'; my_array[my_array. length] = 'b'; length_a = my_array. length; length_b =](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-13.jpg)
my_array[my_array. length] = 'a'; my_array[my_array. length] = 'b'; length_a = my_array. length; length_b = my_array. length; my_array[length_a] = 'a'; if (length_a >= my_array. length) my_array[length_b] = 'b'; my_array. length = length_a + } if (length_b >= my_array. length) my_array. length = length_b + } { 1;
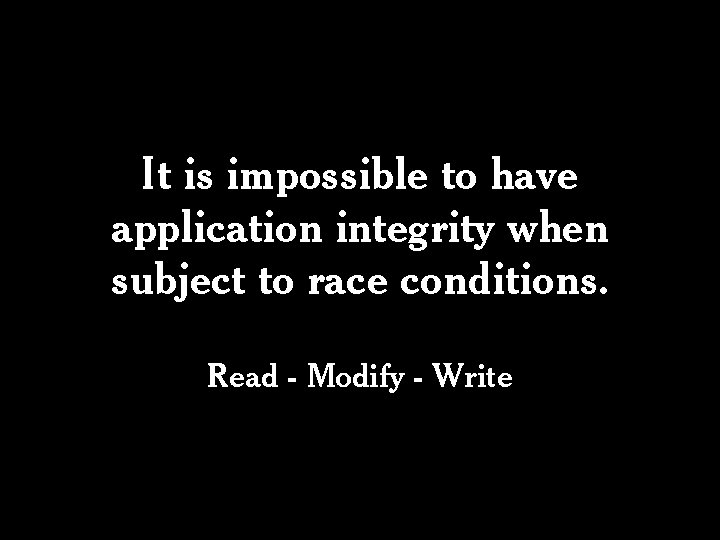
It is impossible to have application integrity when subject to race conditions. Read - Modify - Write
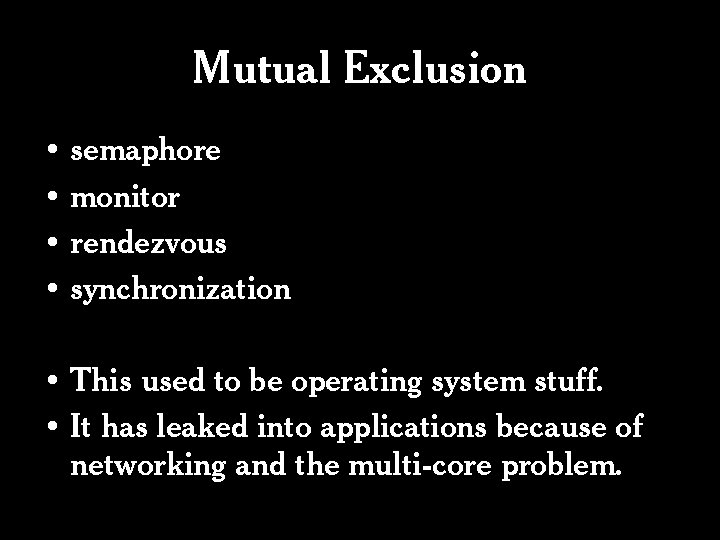
Mutual Exclusion • semaphore • monitor • rendezvous • synchronization • This used to be operating system stuff. • It has leaked into applications because of networking and the multi-core problem.
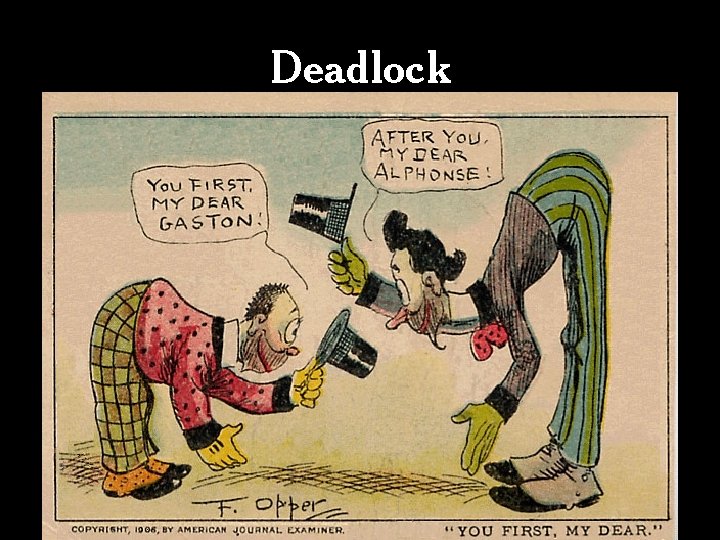
Deadlock
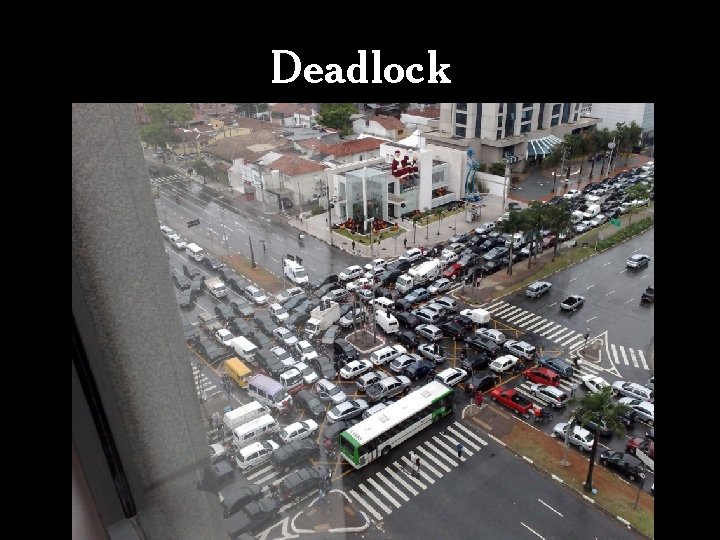
Deadlock
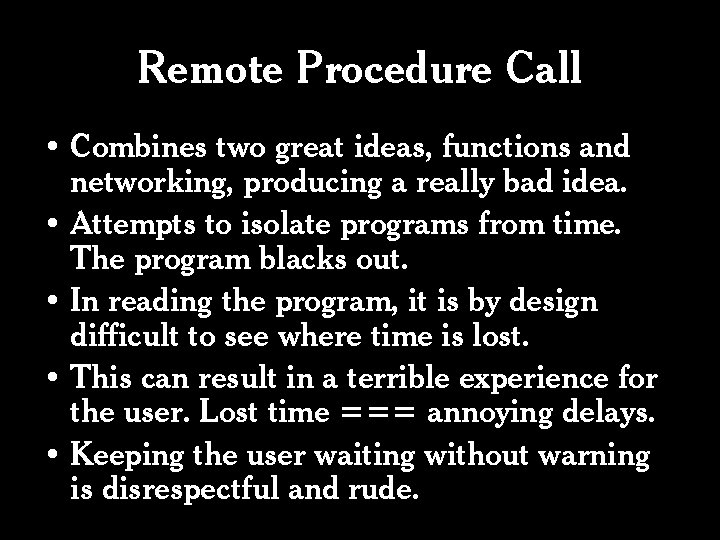
Remote Procedure Call • Combines two great ideas, functions and networking, producing a really bad idea. • Attempts to isolate programs from time. The program blacks out. • In reading the program, it is by design difficult to see where time is lost. • This can result in a terrible experience for the user. Lost time === annoying delays. • Keeping the user waiting without warning is disrespectful and rude.
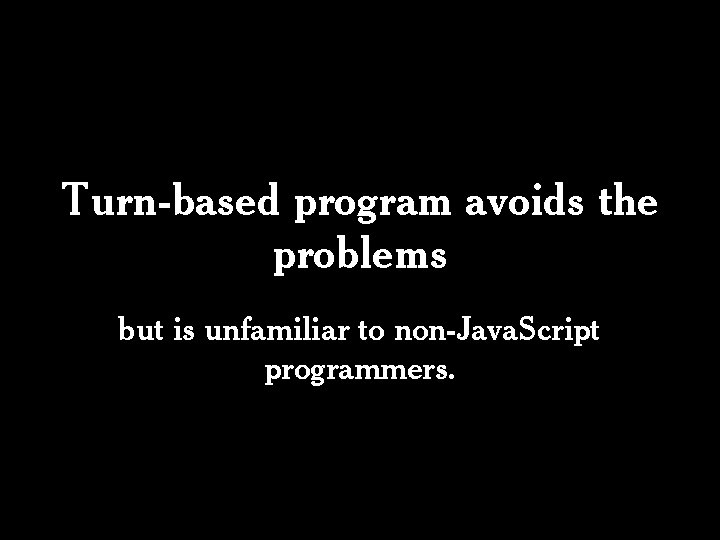
Turn-based program avoids the problems but is unfamiliar to non-Java. Script programmers.
![Quiz 1 function funkyo o null var x funkyx Quiz 1 function funky(o) { o = null; } var x = []; funky(x);](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-20.jpg)
Quiz 1 function funky(o) { o = null; } var x = []; funky(x); alert(x); A. null B. [] C. undefined D. throw
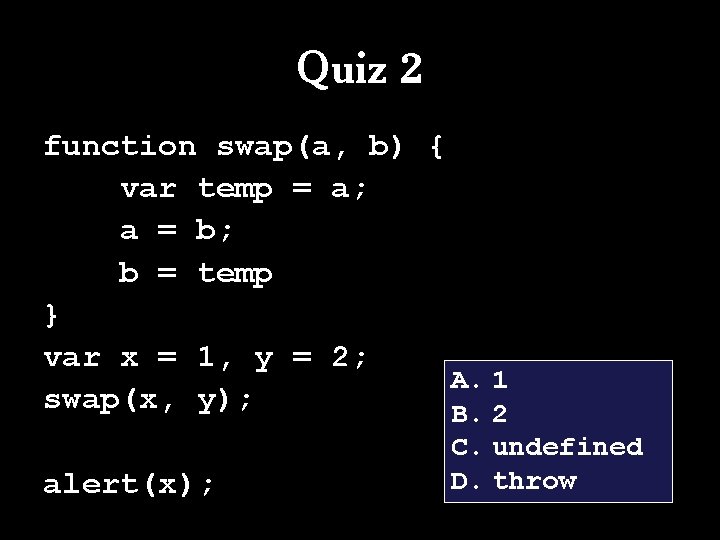
Quiz 2 function swap(a, b) { var temp = a; a = b; b = temp } var x = 1, y = 2; A. 1 swap(x, y); B. 2 alert(x); C. undefined D. throw
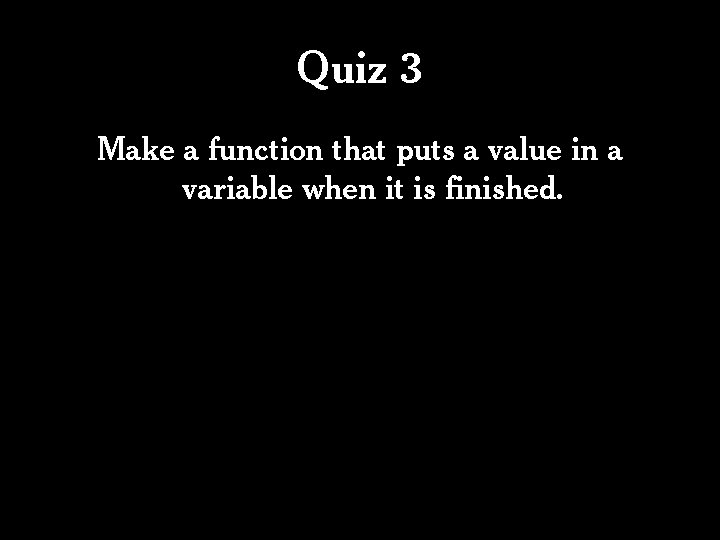
Quiz 3 Make a function that puts a value in a variable when it is finished.
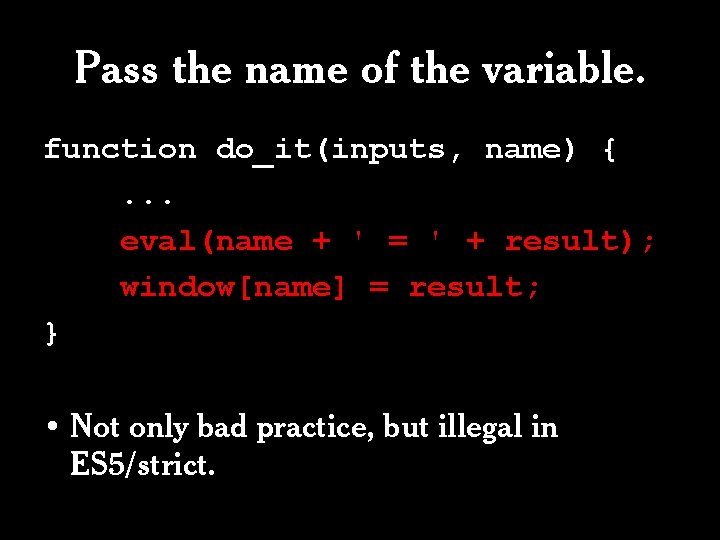
Pass the name of the variable. function do_it(inputs, name) {. . . eval(name + ' = ' + result); window[name] = result; } • Not only bad practice, but illegal in ES 5/strict.
![function doitinputs obj name objname result Gives doit function do_it(inputs, obj, name) {. . . obj[name] = result; } • Gives do-it](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-24.jpg)
function do_it(inputs, obj, name) {. . . obj[name] = result; } • Gives do-it too much authority.
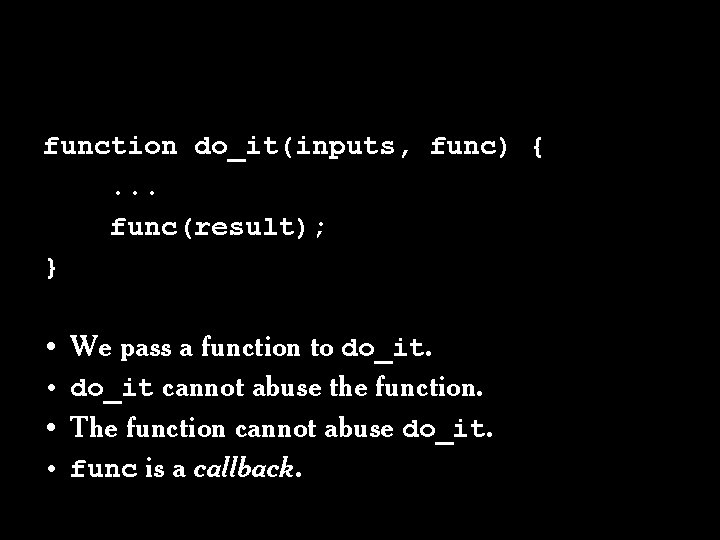
function do_it(inputs, func) {. . . func(result); } • • We pass a function to do_it cannot abuse the function. The function cannot abuse do_it. func is a callback.
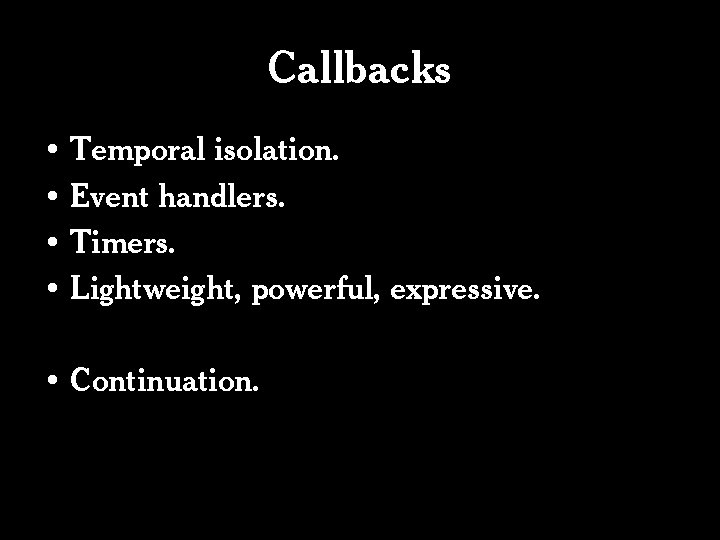
Callbacks • Temporal isolation. • Event handlers. • Timers. • Lightweight, powerful, expressive. • Continuation.
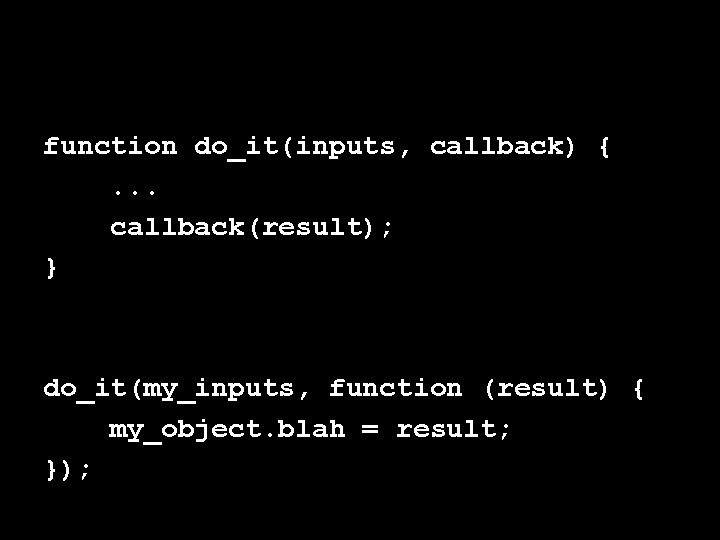
function do_it(inputs, callback) {. . . callback(result); } do_it(my_inputs, function (result) { my_object. blah = result; });
![Generalize function storerobj name return function result objname result Generalize. function storer(obj, name) { return function (result) { obj[name] = result; }; }](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-28.jpg)
Generalize. function storer(obj, name) { return function (result) { obj[name] = result; }; } do_it(inputs, storer(my_object, 'blah'));
![function storermakerobj return functionname return function result objname result function storer_maker(obj) { return function(name) { return function (result) { obj[name] = result; };](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-29.jpg)
function storer_maker(obj) { return function(name) { return function (result) { obj[name] = result; }; }; } my_storer = storer_maker(my_object); do_it(my_inputs, my_storer('blah'));
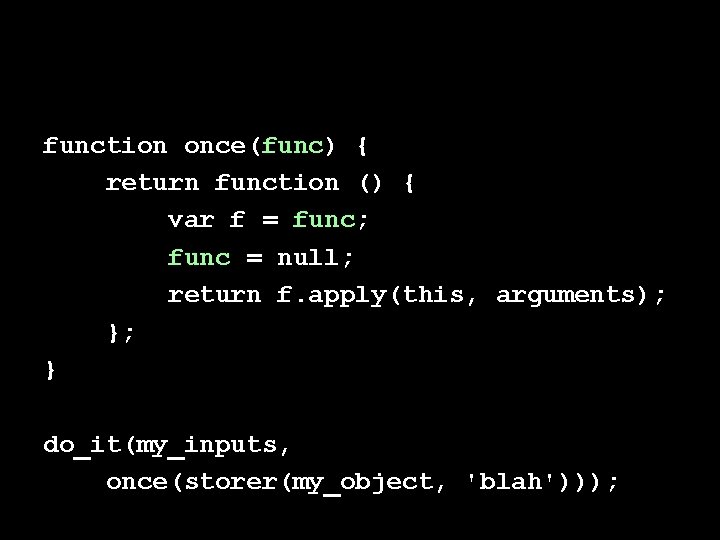
function once(func) { return function () { var f = func; func = null; return f. apply(this, arguments); }; } do_it(my_inputs, once(storer(my_object, 'blah')));
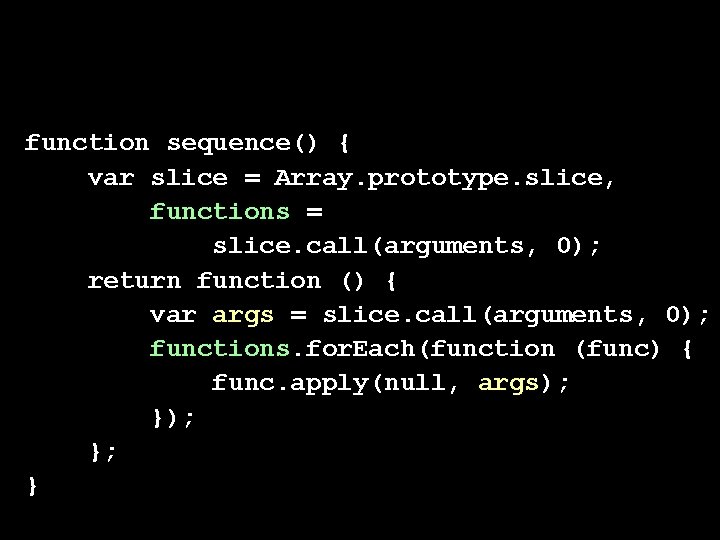
function sequence() { var slice = Array. prototype. slice, functions = slice. call(arguments, 0); return function () { var args = slice. call(arguments, 0); functions. for. Each(function (func) { func. apply(null, args); }; }
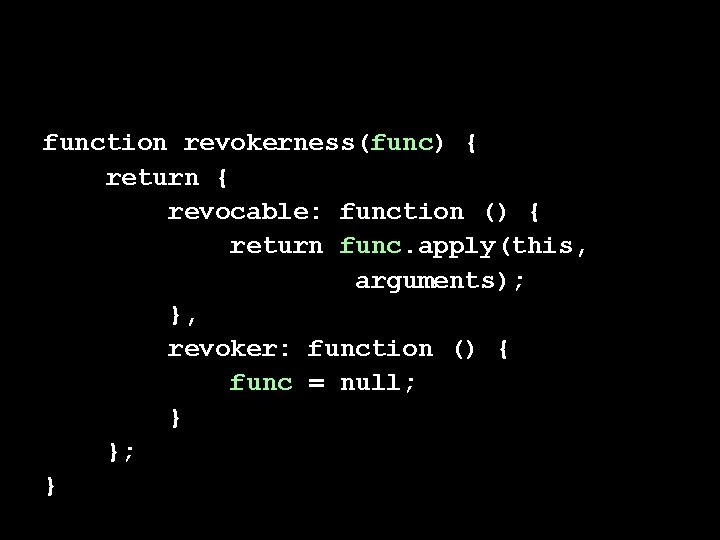
function revokerness(func) { return { revocable: function () { return func. apply(this, arguments); }, revoker: function () { func = null; } }; }
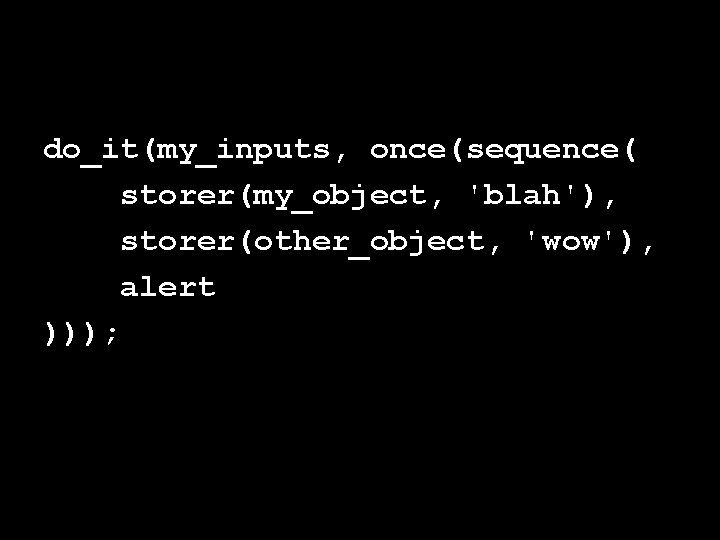
do_it(my_inputs, once(sequence( storer(my_object, 'blah'), storer(other_object, 'wow'), alert )));
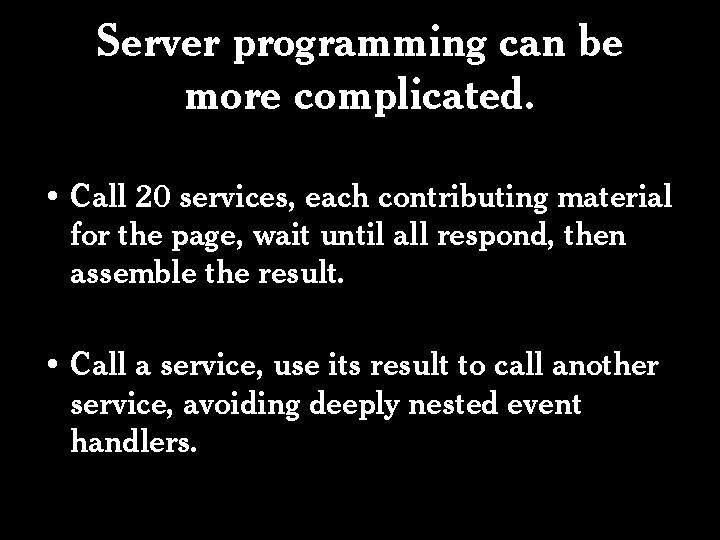
Server programming can be more complicated. • Call 20 services, each contributing material for the page, wait until all respond, then assemble the result. • Call a service, use its result to call another service, avoiding deeply nested event handlers.
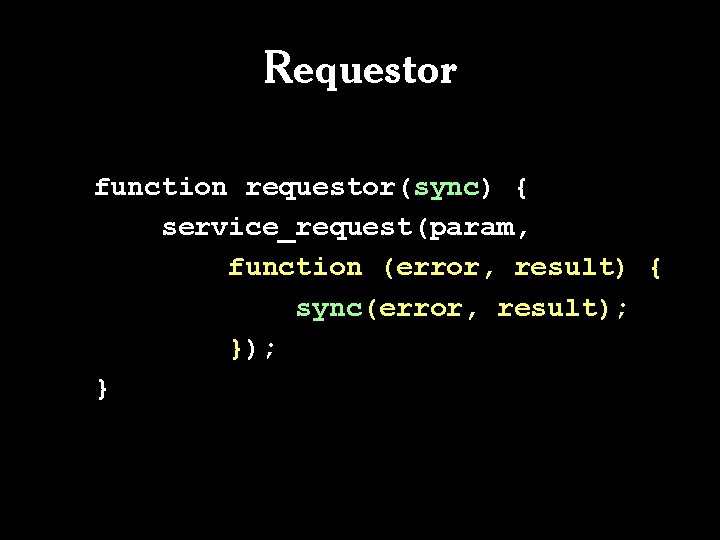
Requestor function requestor(sync) { service_request(param, function (error, result) { sync(error, result); }
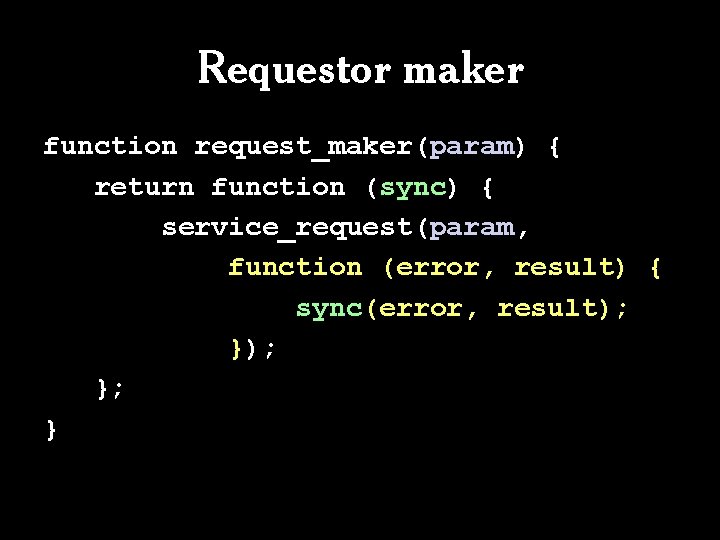
Requestor maker function request_maker(param) { return function (sync) { service_request(param, function (error, result) { sync(error, result); }; }
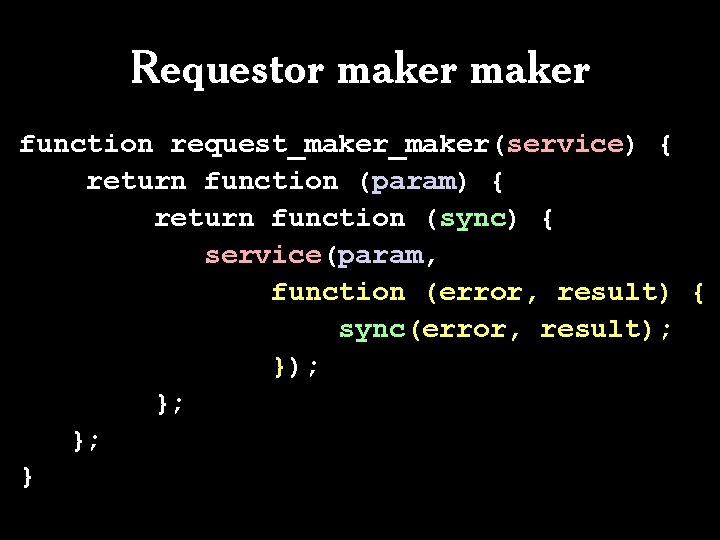
Requestor maker function request_maker(service) { return function (param) { return function (sync) { service(param, function (error, result) { sync(error, result); }; }; }
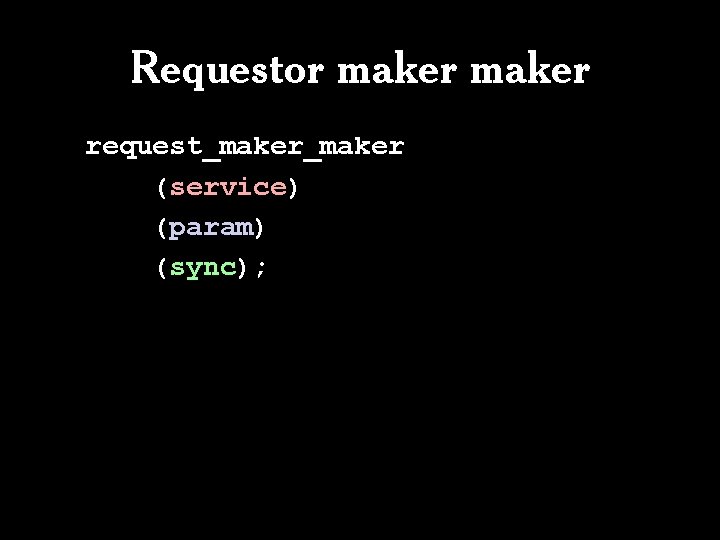
Requestor maker request_maker (service) (param) (sync);
![Composition parrequestor sync timeout seqrequestor sync timeout maprequestor sync timeout Composition par([requestor…], sync, timeout); seq([requestor…], sync, timeout); map([requestor…], sync, timeout);](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-39.jpg)
Composition par([requestor…], sync, timeout); seq([requestor…], sync, timeout); map([requestor…], sync, timeout);
![Composition function makers parorrequestor timeout seqorrequestor timeout maporrequestor timeout Composition function makers paror([requestor…], timeout) seqor([requestor…], timeout) mapor([requestor…], timeout)](https://slidetodoc.com/presentation_image_h2/8adac3f4c42287baf9a7f3c899e5ea17/image-40.jpg)
Composition function makers paror([requestor…], timeout) seqor([requestor…], timeout) mapor([requestor…], timeout)
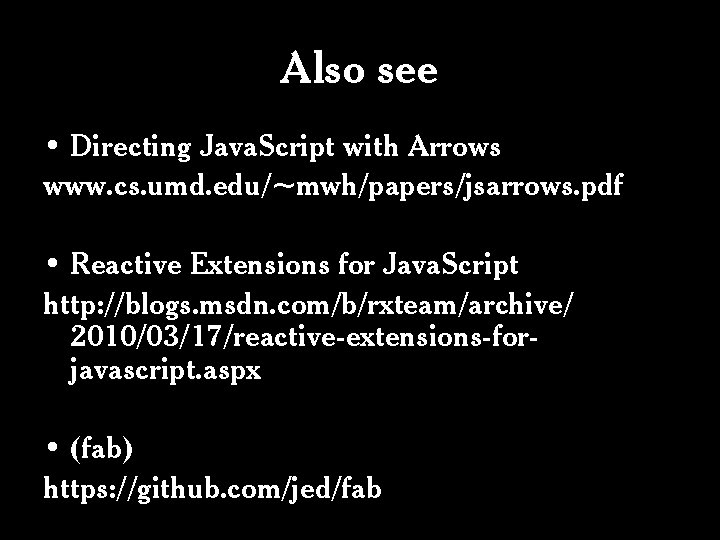
Also see • Directing Java. Script with Arrows www. cs. umd. edu/~mwh/papers/jsarrows. pdf • Reactive Extensions for Java. Script http: //blogs. msdn. com/b/rxteam/archive/ 2010/03/17/reactive-extensions-forjavascript. aspx • (fab) https: //github. com/jed/fab
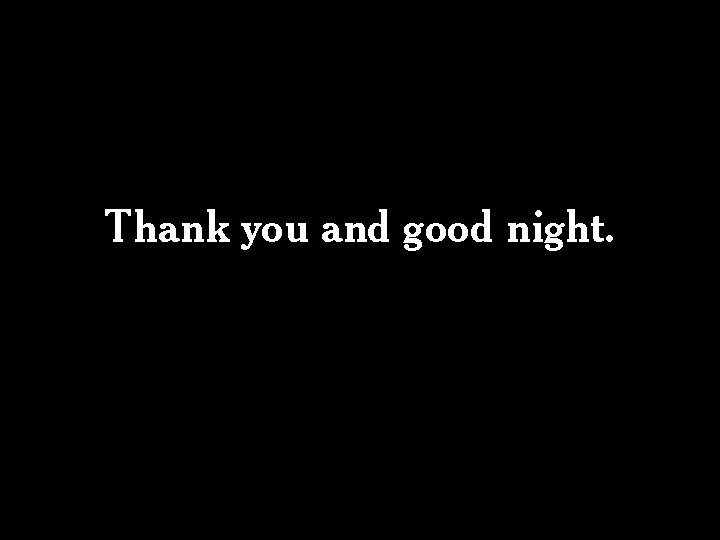
Thank you and good night.
Misty douglas
Prove sss similarity theorem
Lesson 7-4 applying properties of similar triangles
Sss similarity definition
Sss similarity theorem
What is server side programming
Douglas mcgregor the human side of enterprise
Perfect competition side by side graphs
Uil tea side by side
M&a process timeline
Glass will break first on the weaker side, the side:
Side angle side theorem
Two wheels roll side by side
Iso 5817 welding symbols
A thin rectangular plate of sides 60mm
Compensating curve denture
Solclimatic
Side by side stuff
Sin = adjacent or opposite
Mandibular movement
Videocon side by side refrigerator
Red side blue side
Introduction to server side programming
Aam adobe
Php server side includes
Server-side technologies
Azure server side encryption
What is node js server side javascript
Server side
What is jsp file
Pircbot
Java irc server
Android udp client
Java.rmi.server.codebase
Lập trình socket giao tiếp tcp client/server java
Knock knock server java
Java server pages life cycle
Java server pages
Java server pages
Jsp o que é
Java import java.util.*
Java import java.util.*
Import java.awt.* import java.applet.*