Recall Some Algorithms Lots of Data Structures Arrays
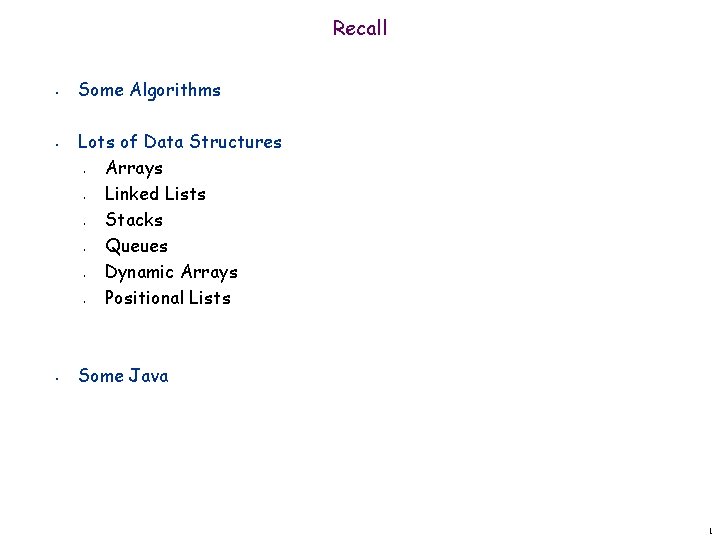
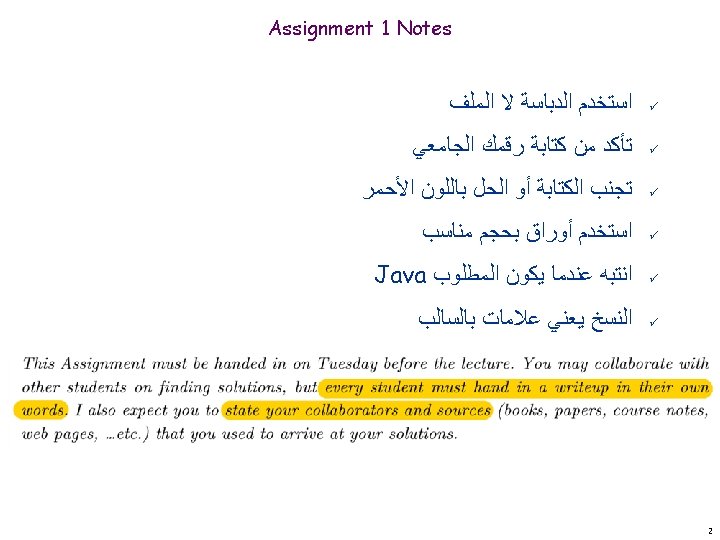
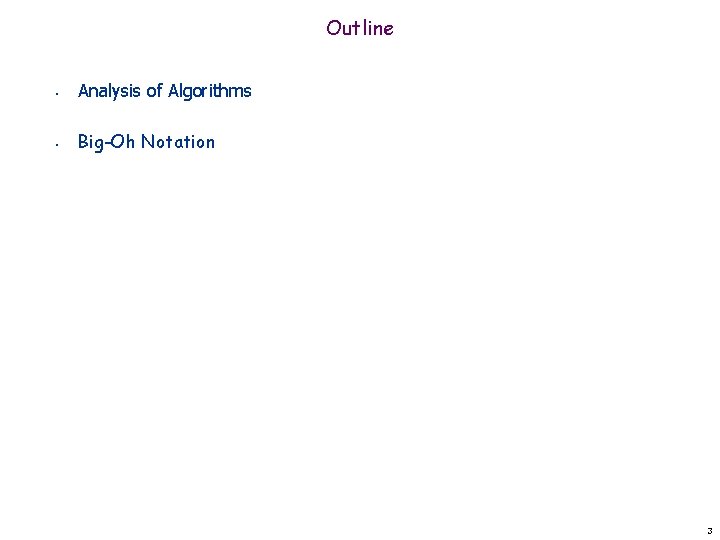
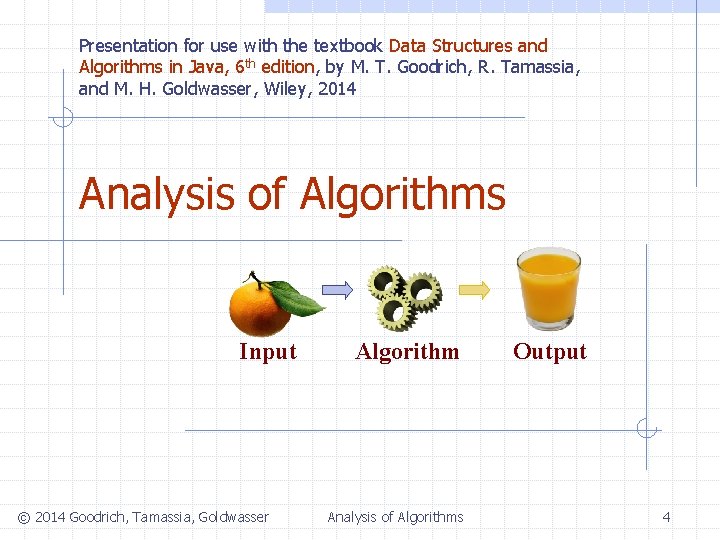
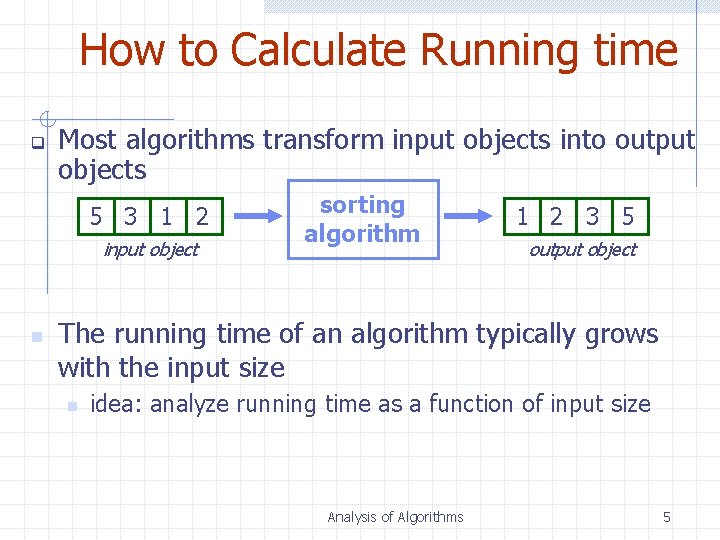
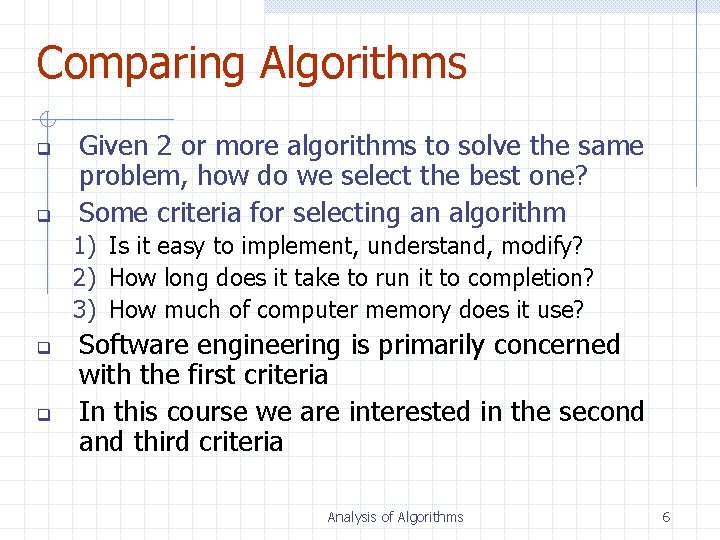
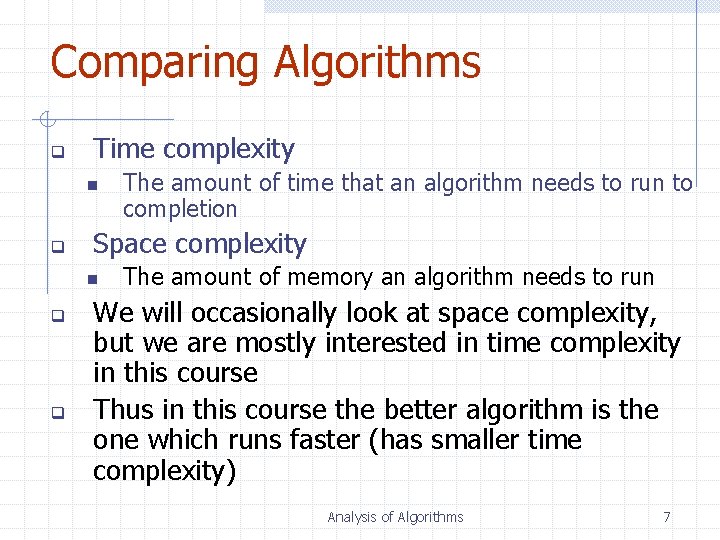
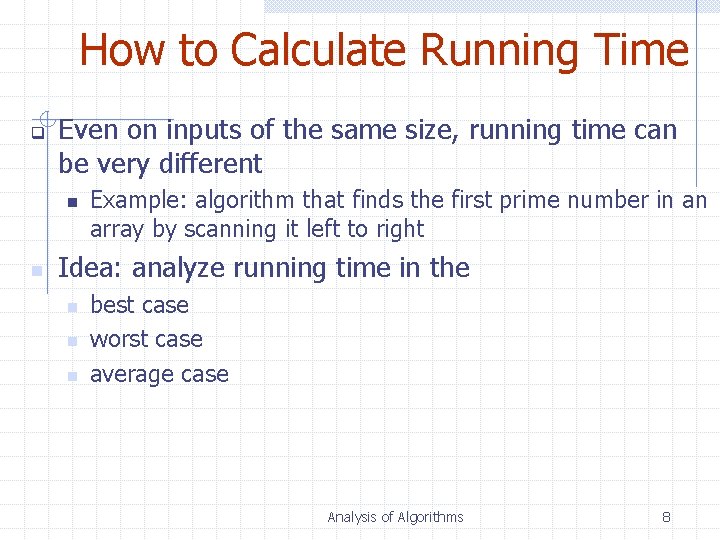
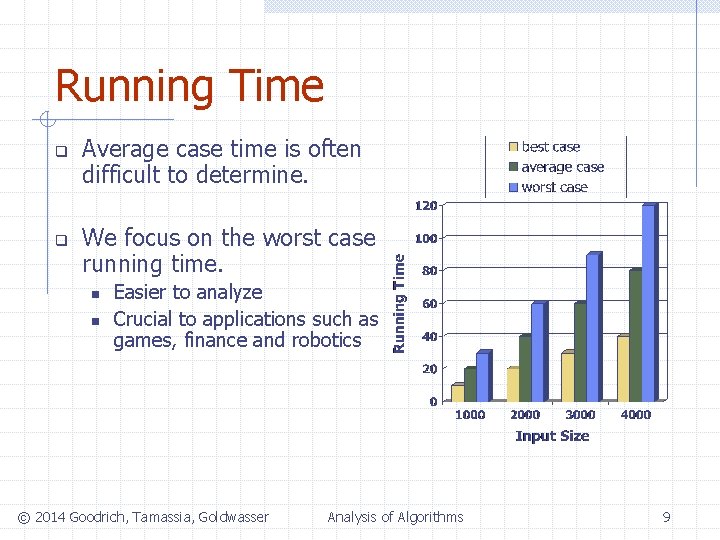
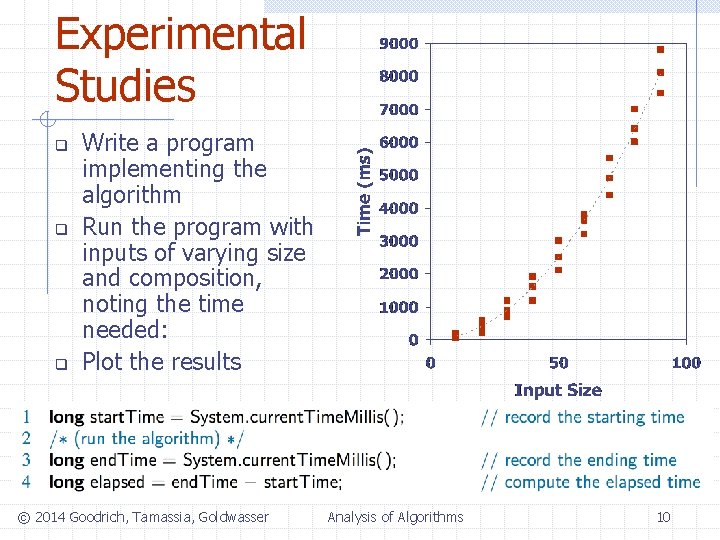
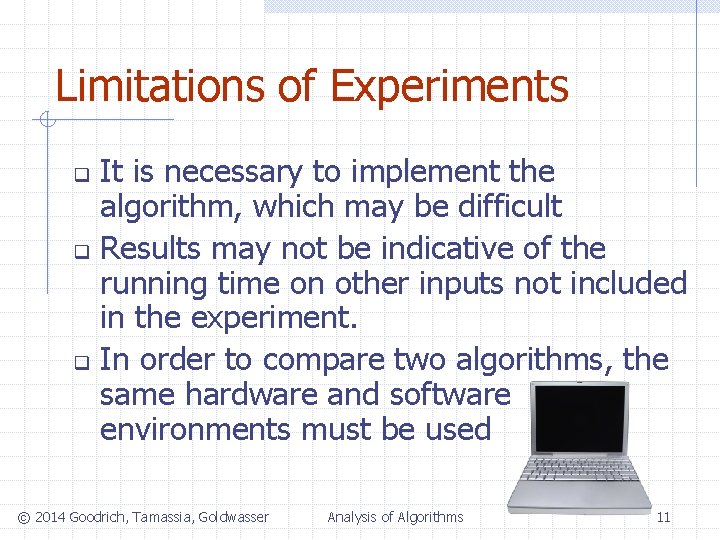
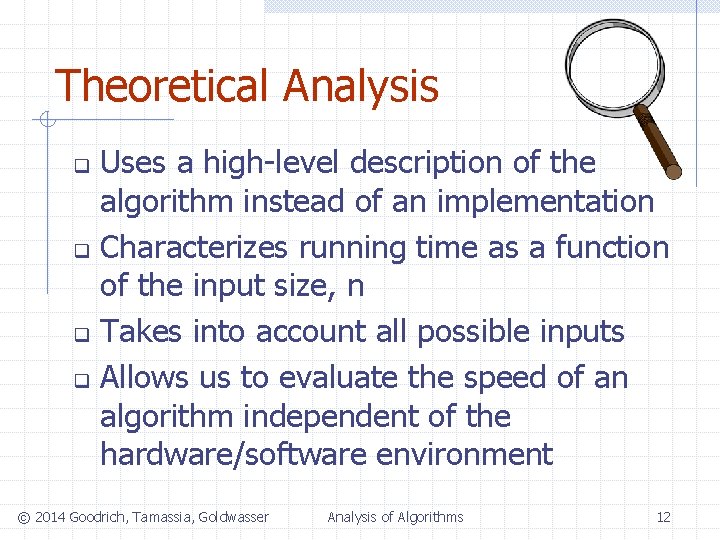
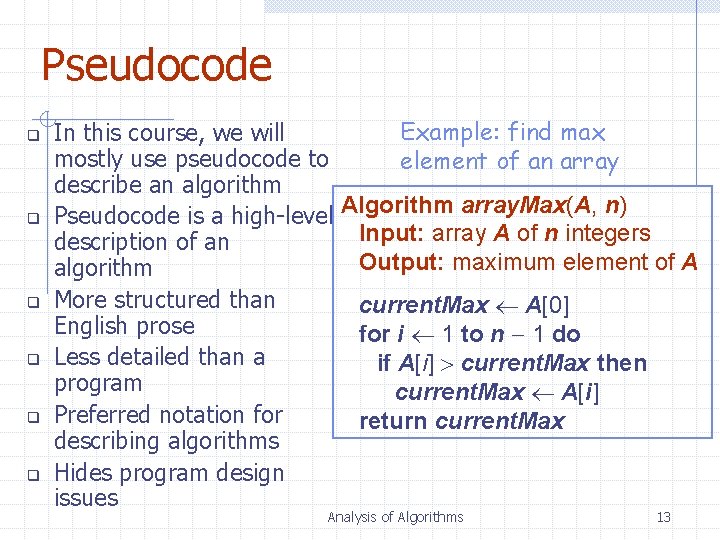
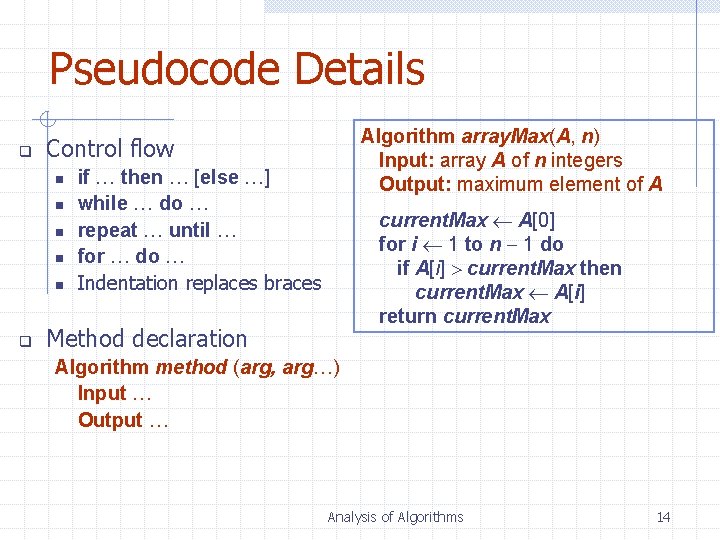
![Pseudocode Details q Method call var. method (arg [, arg…]) q Return value return Pseudocode Details q Method call var. method (arg [, arg…]) q Return value return](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-15.jpg)
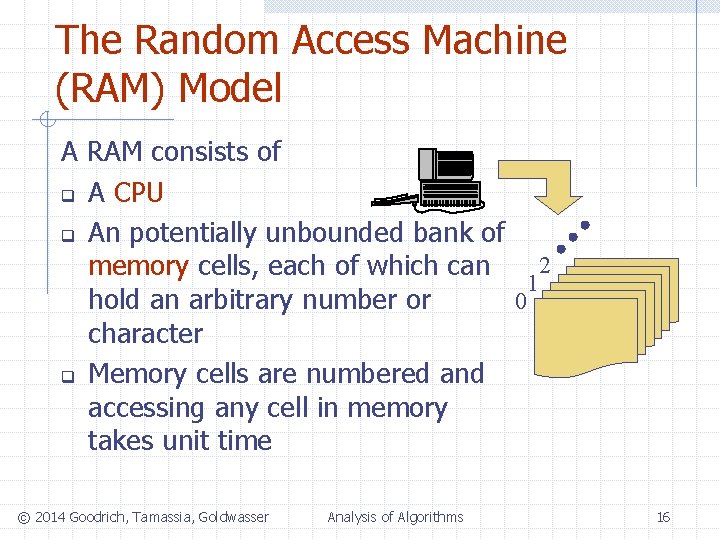
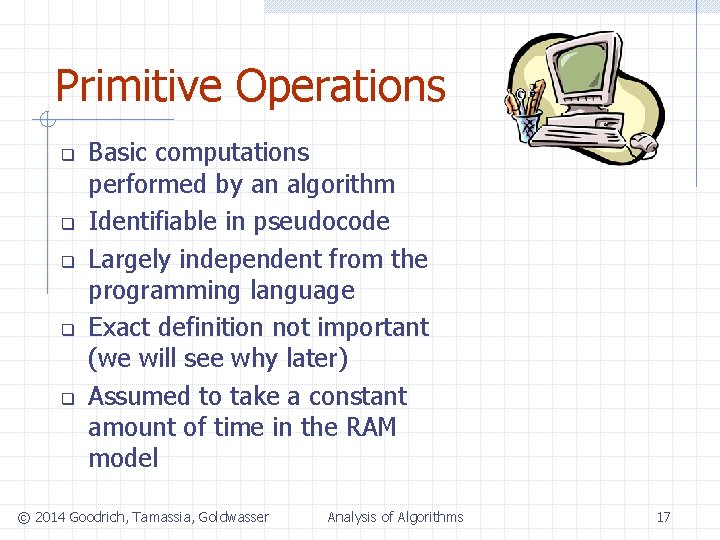
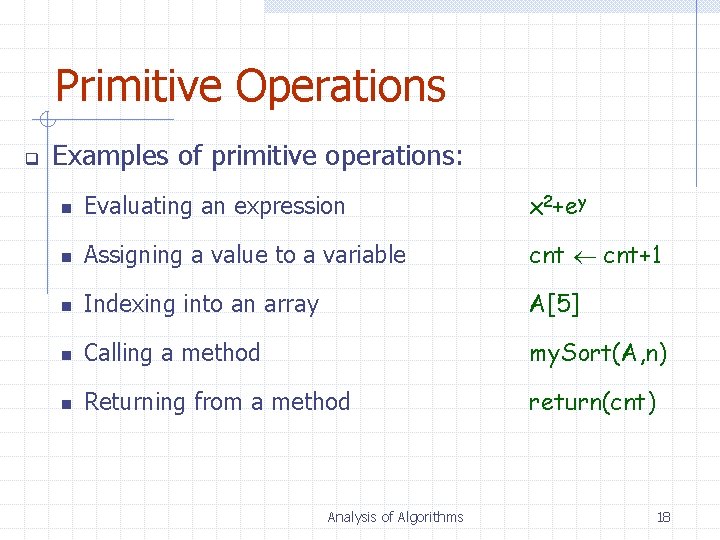
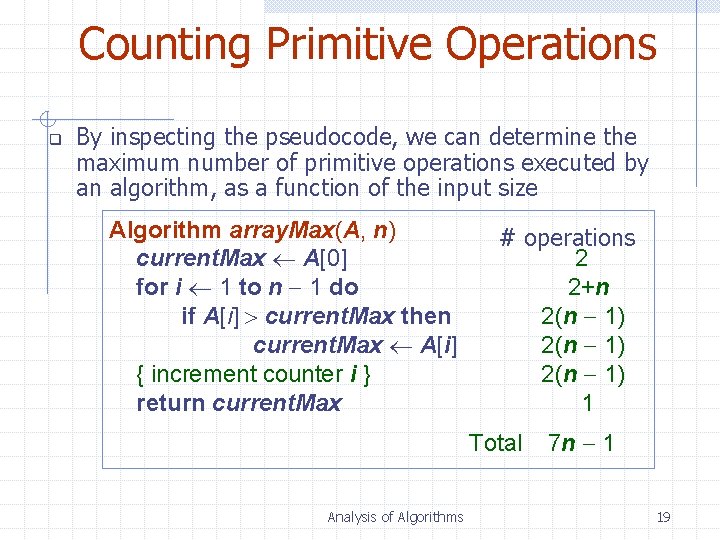
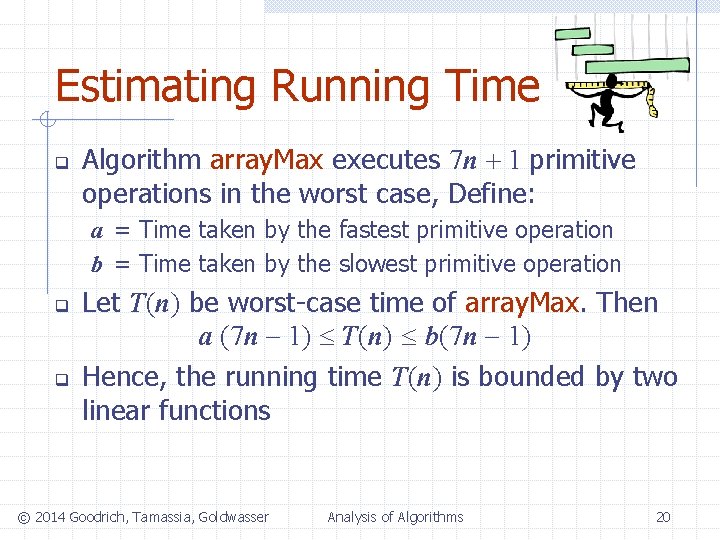
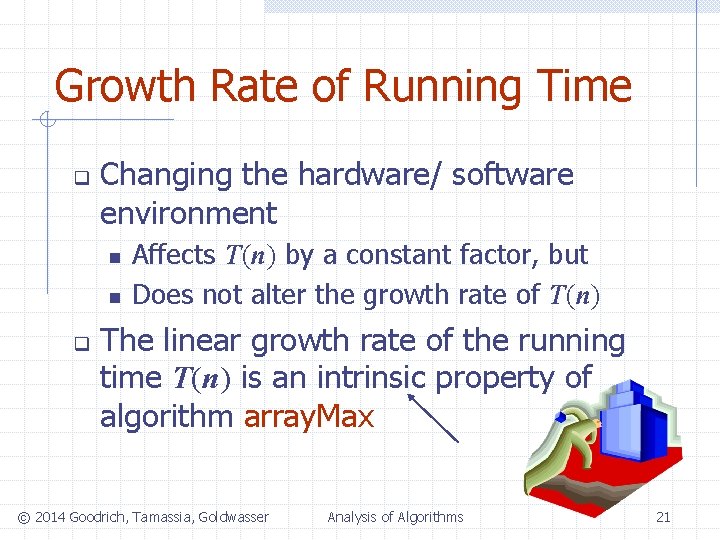
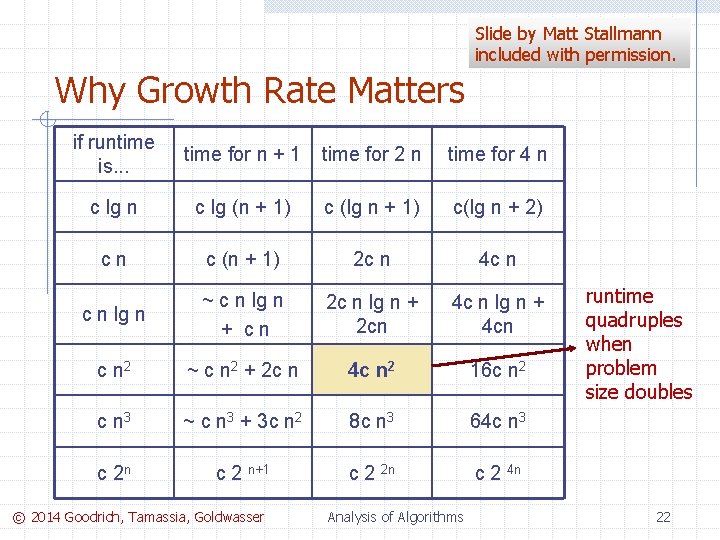
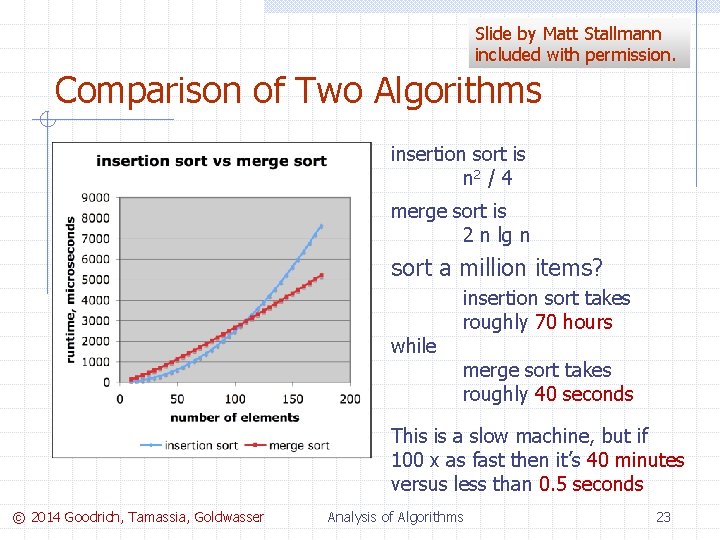
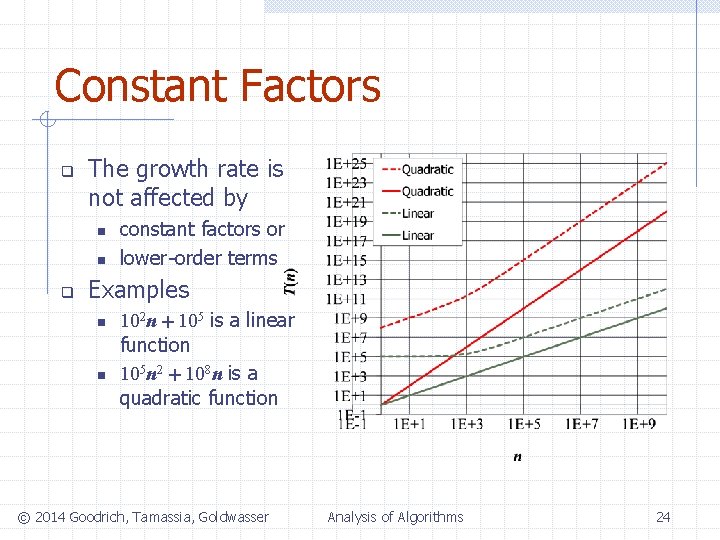
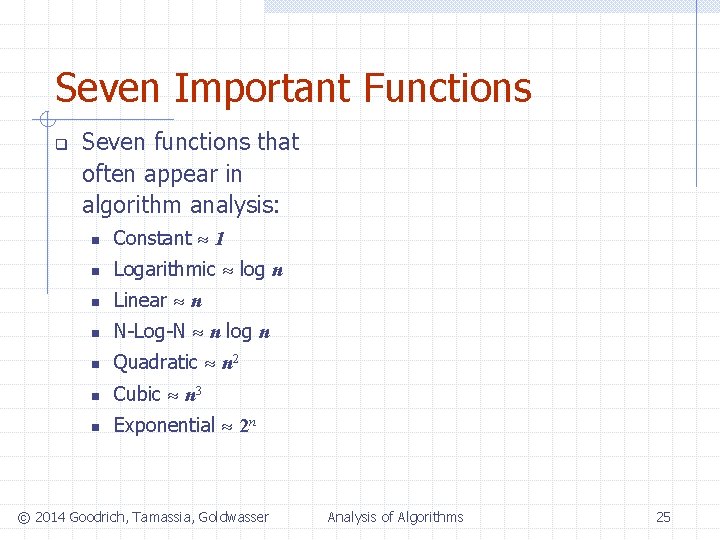
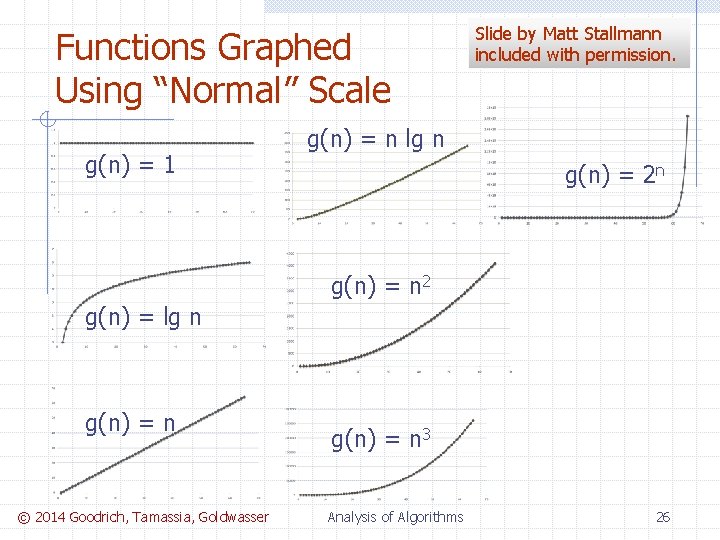
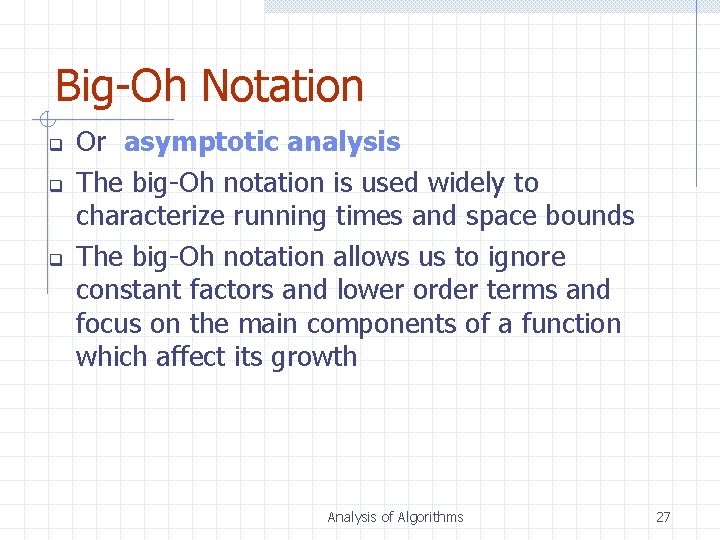
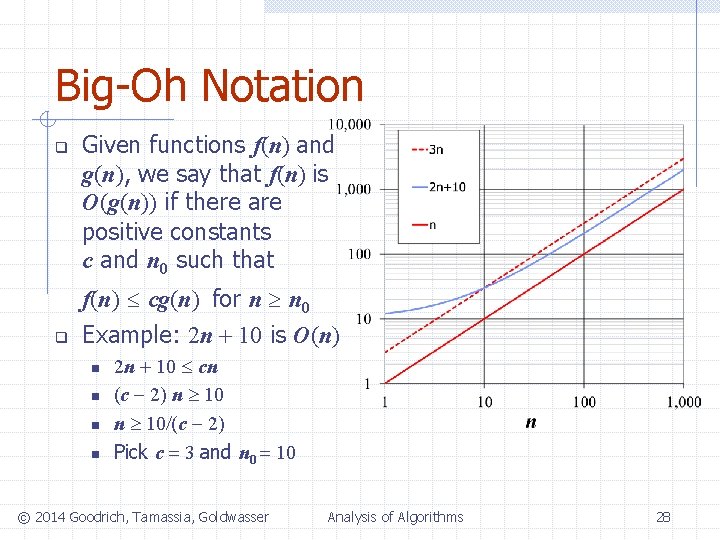
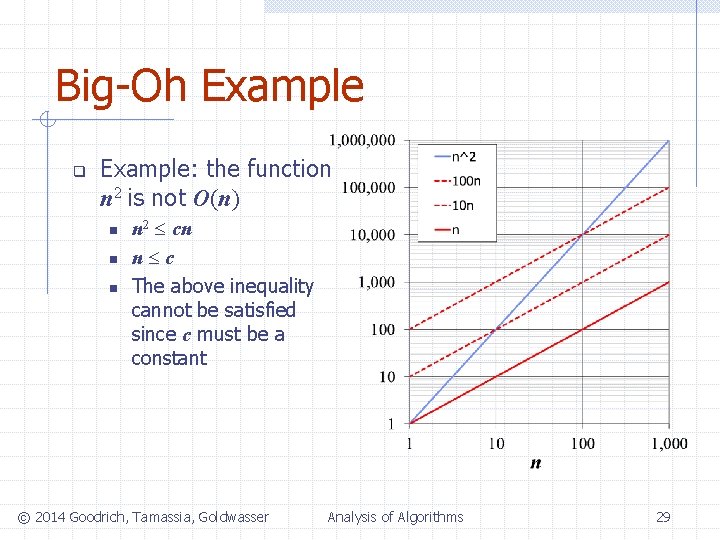
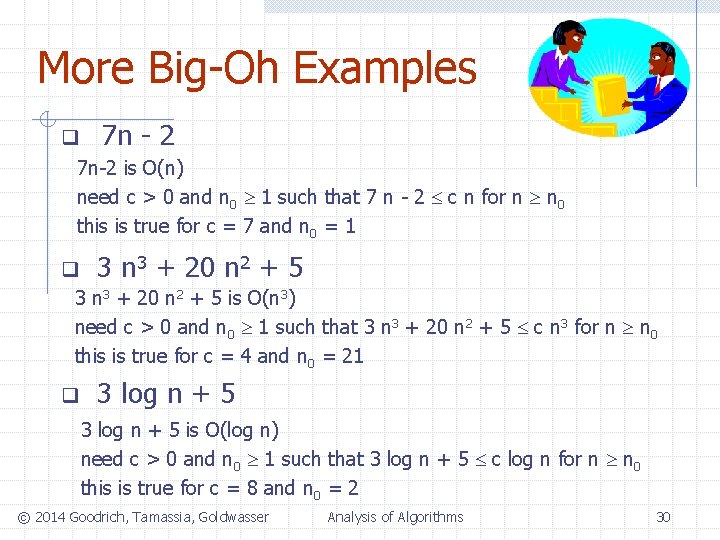
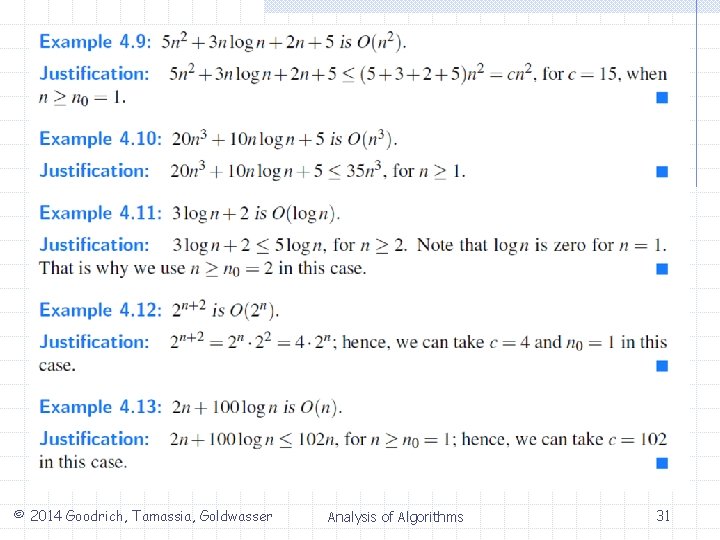
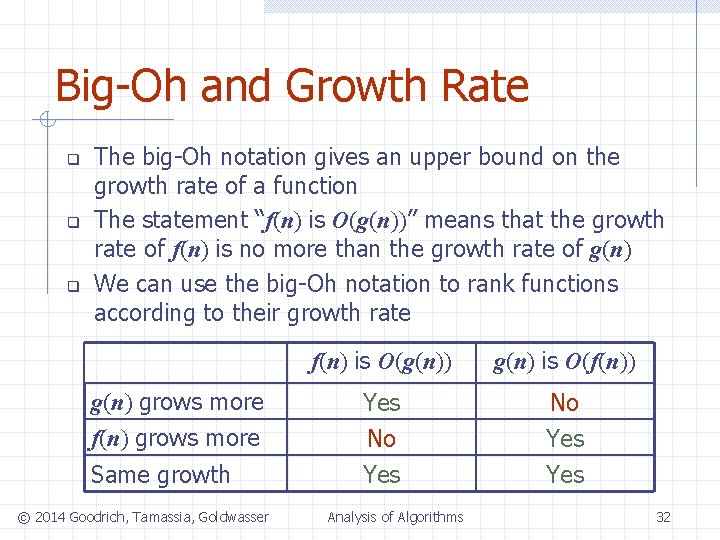
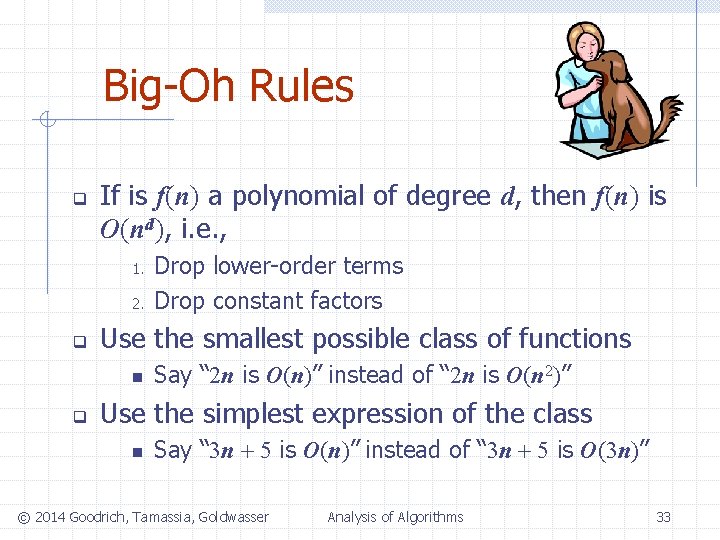
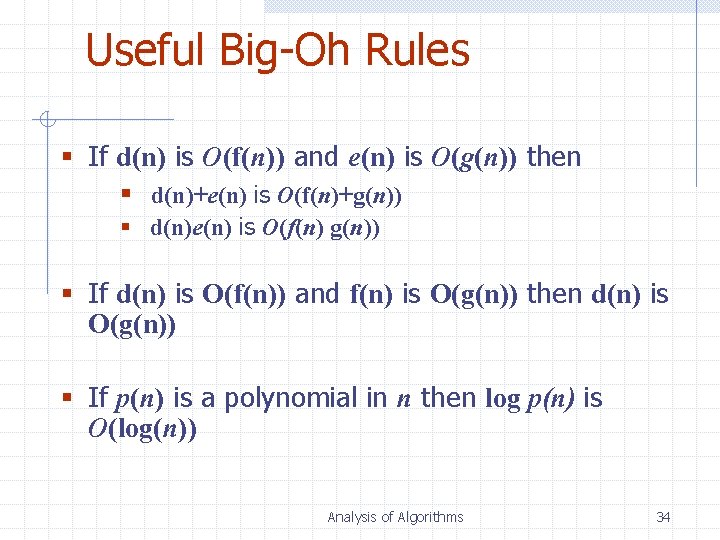
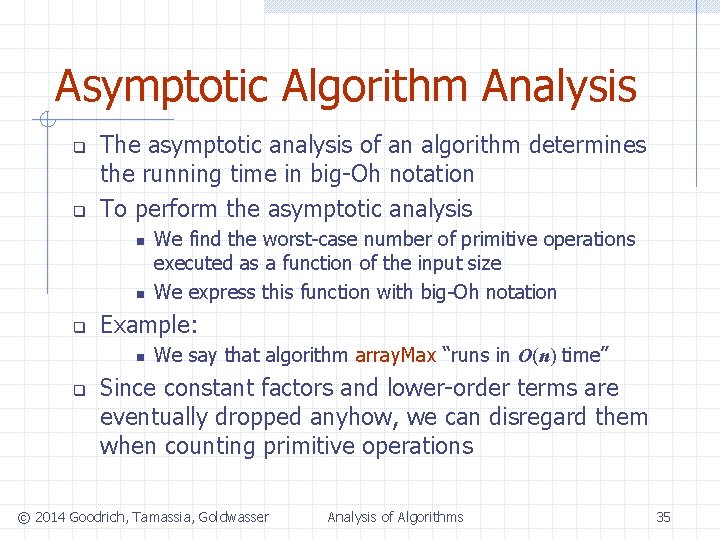
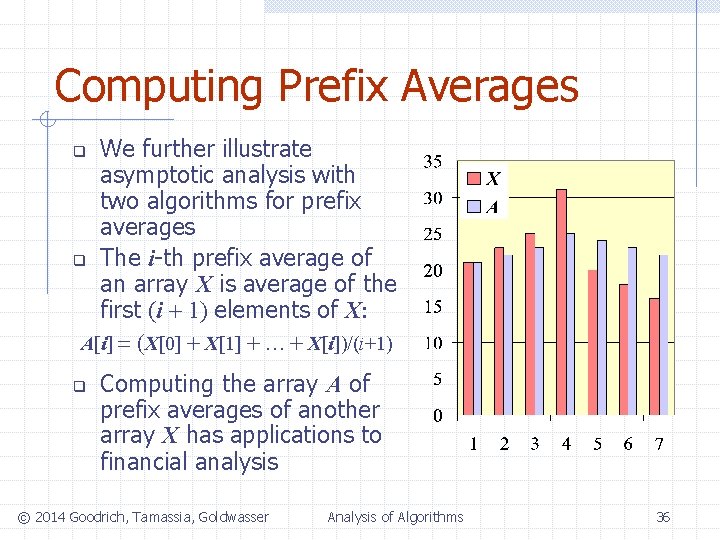
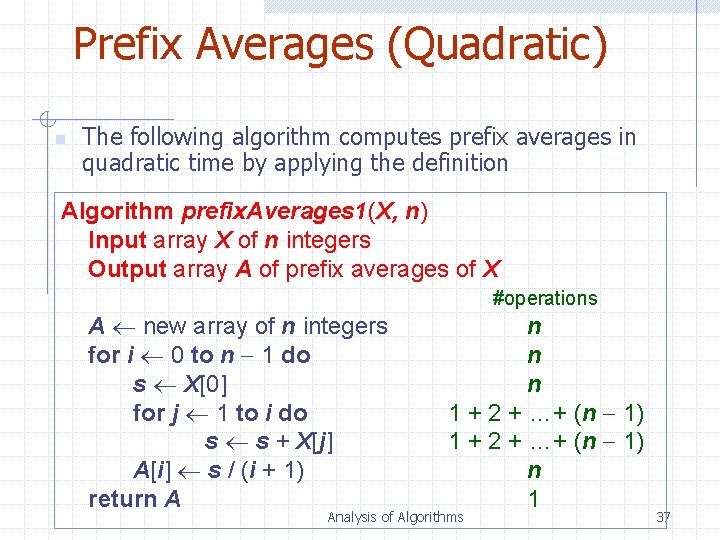
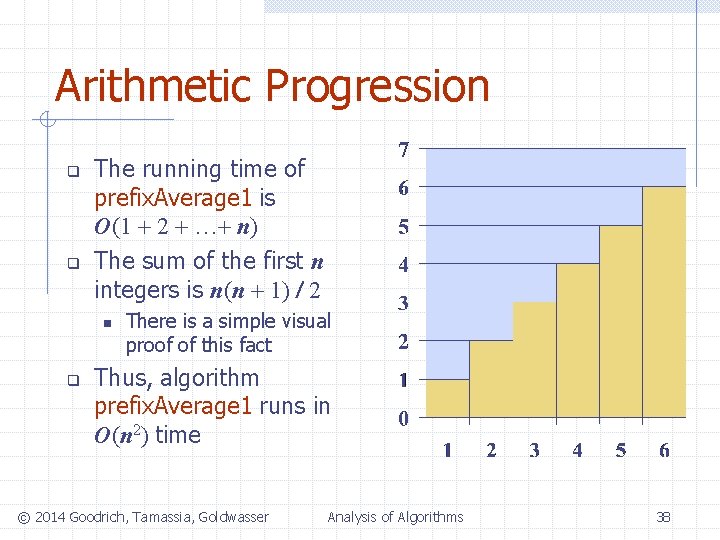
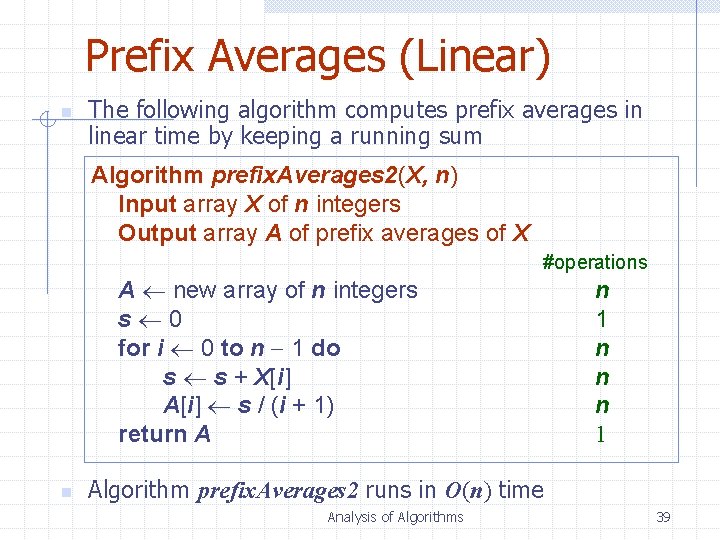
![More Examples Algorithm Sum. Triple. Array(X, n) Input triple array X[ ][ ][ ] More Examples Algorithm Sum. Triple. Array(X, n) Input triple array X[ ][ ][ ]](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-40.jpg)
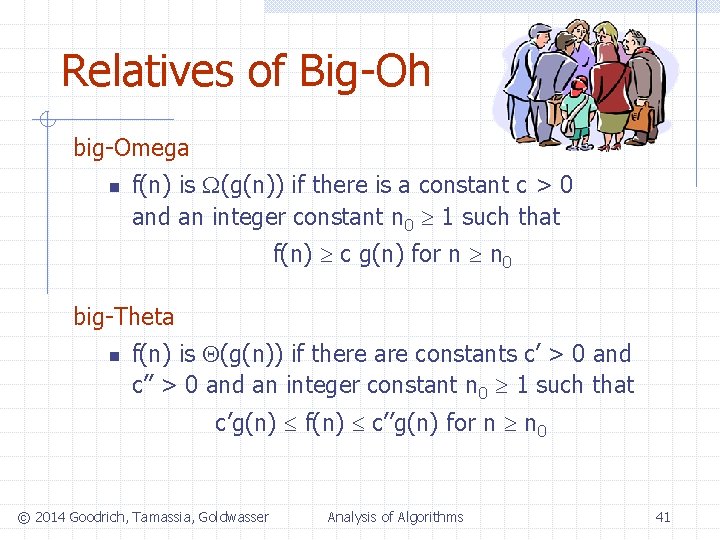
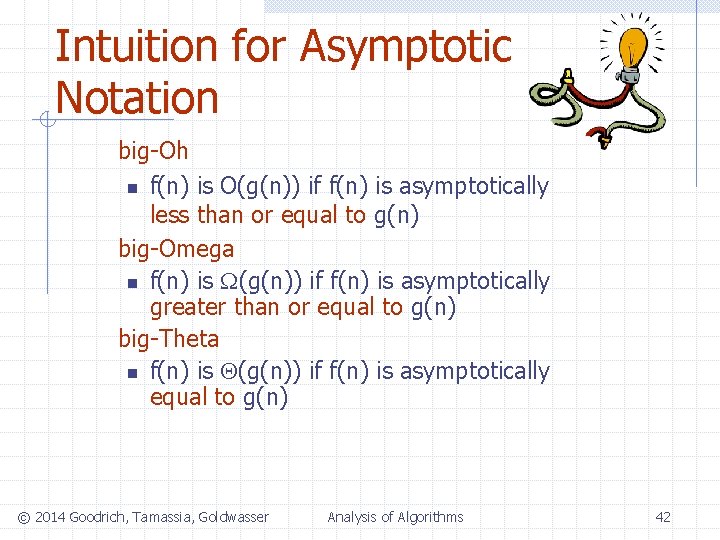
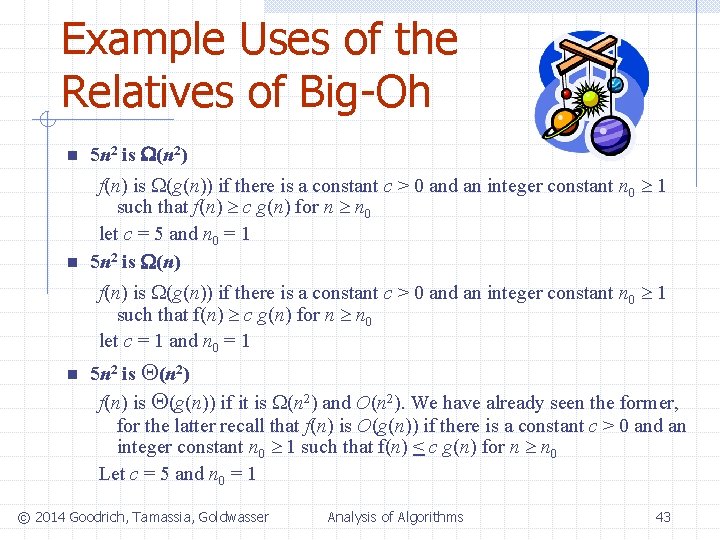
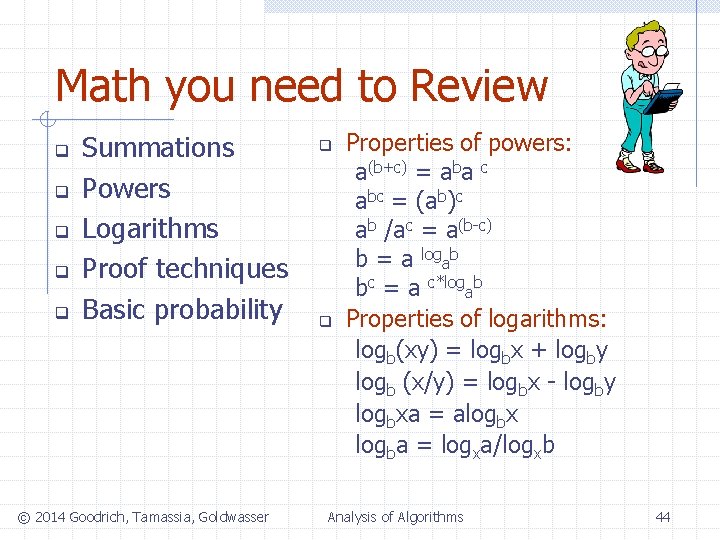
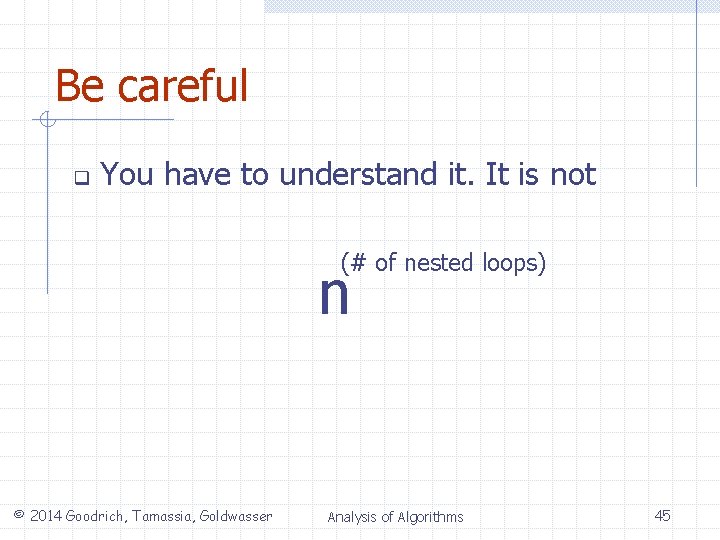
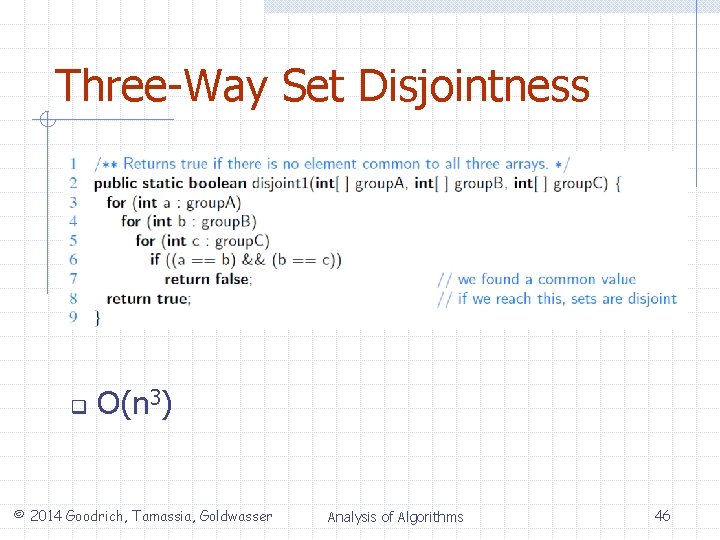
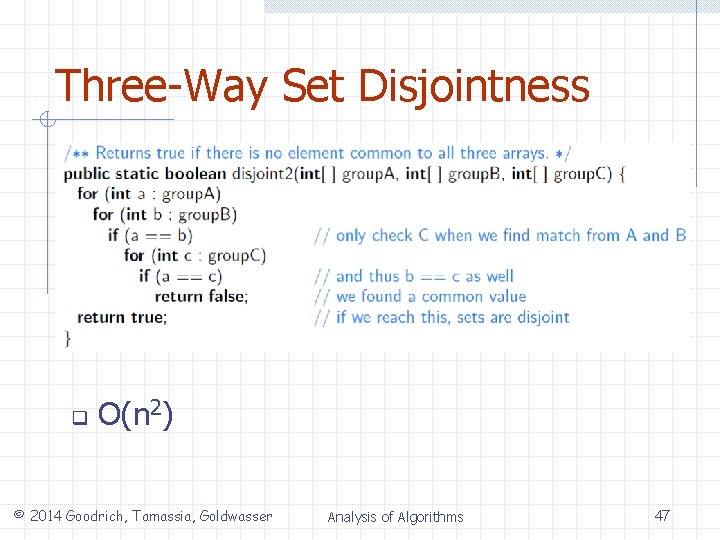
![Reading • [G] Chapter 4 • If you feel you need more then read Reading • [G] Chapter 4 • If you feel you need more then read](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-48.jpg)
- Slides: 48
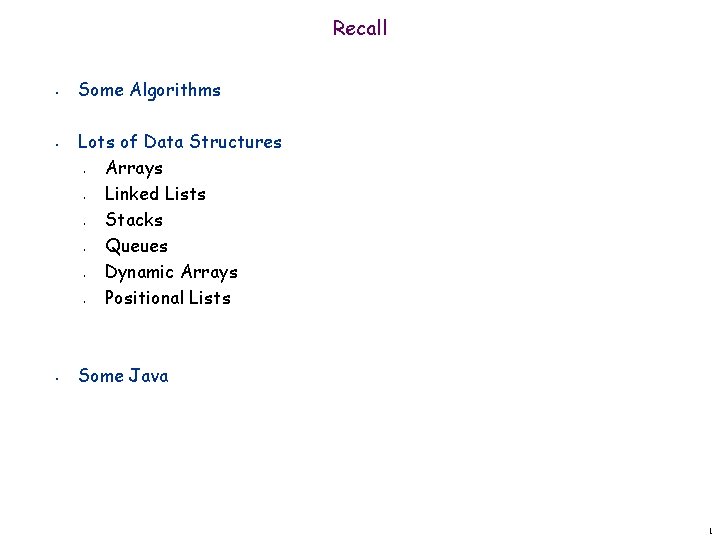
Recall • • Some Algorithms Lots of Data Structures Arrays Linked Lists Stacks Queues Dynamic Arrays Positional Lists • • Some Java 1
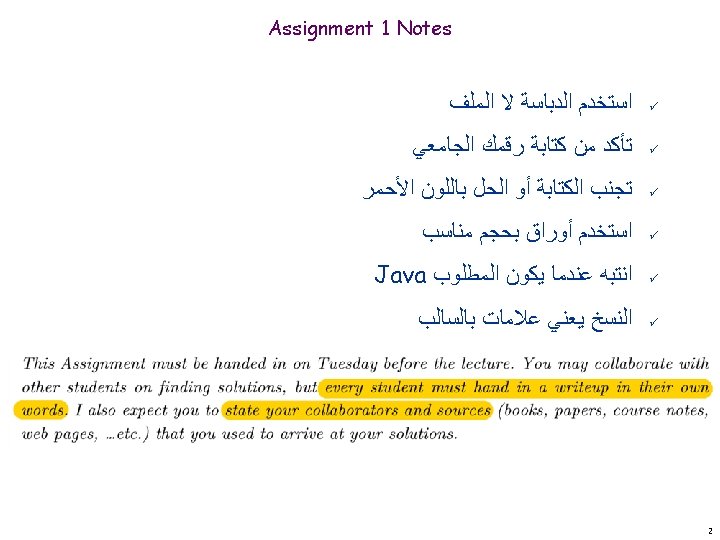
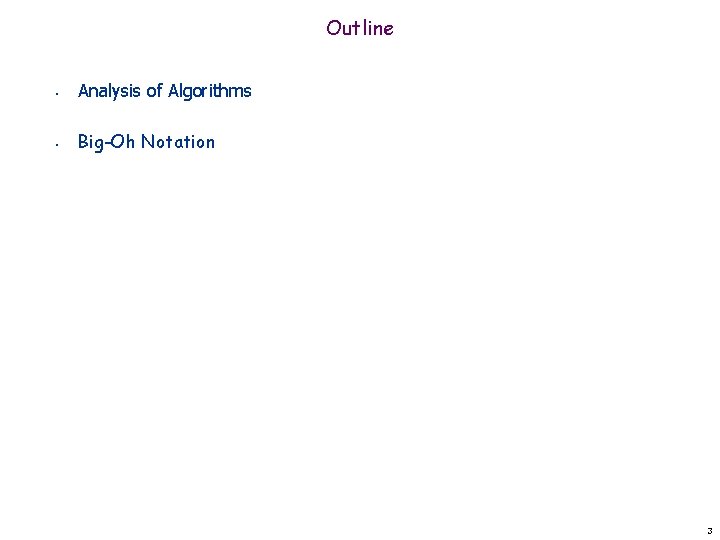
Outline • Analysis of Algorithms • Big-Oh Notation 3
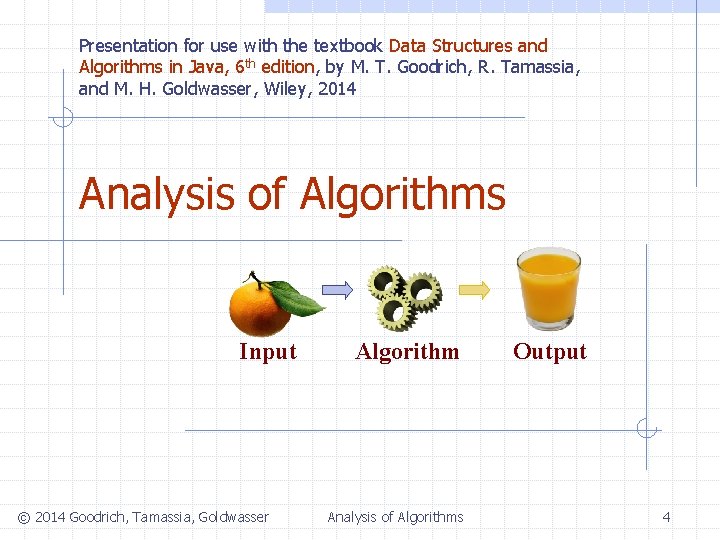
Presentation for use with the textbook Data Structures and Algorithms in Java, 6 th edition, by M. T. Goodrich, R. Tamassia, and M. H. Goldwasser, Wiley, 2014 Analysis of Algorithms Input © 2014 Goodrich, Tamassia, Goldwasser Algorithm Analysis of Algorithms Output 4
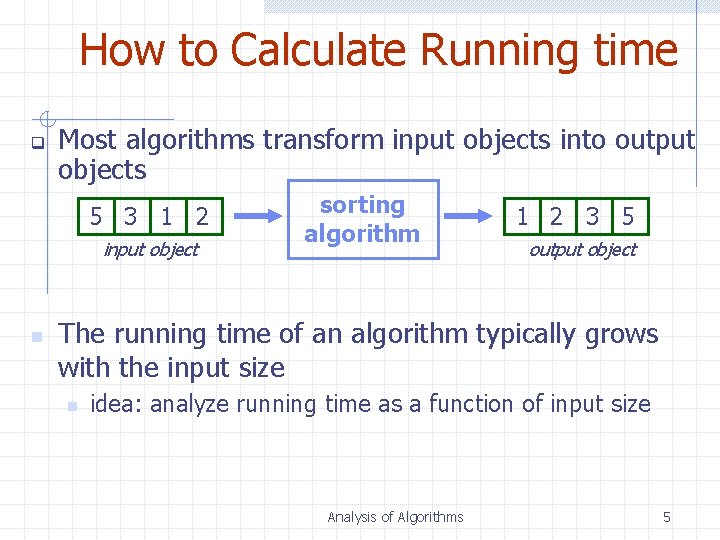
How to Calculate Running time q Most algorithms transform input objects into output objects 5 3 1 2 input object n sorting algorithm 1 2 3 5 output object The running time of an algorithm typically grows with the input size n idea: analyze running time as a function of input size Analysis of Algorithms 5
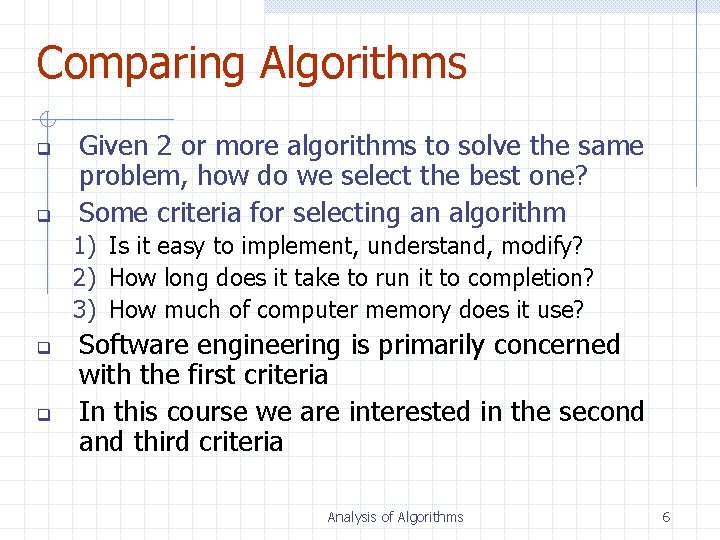
Comparing Algorithms q q Given 2 or more algorithms to solve the same problem, how do we select the best one? Some criteria for selecting an algorithm 1) Is it easy to implement, understand, modify? 2) How long does it take to run it to completion? 3) How much of computer memory does it use? q q Software engineering is primarily concerned with the first criteria In this course we are interested in the second and third criteria Analysis of Algorithms 6
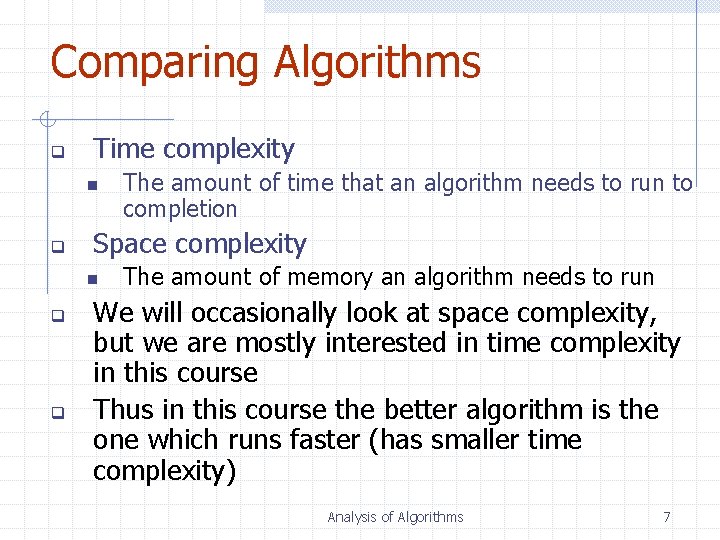
Comparing Algorithms q Time complexity n q Space complexity n q q The amount of time that an algorithm needs to run to completion The amount of memory an algorithm needs to run We will occasionally look at space complexity, but we are mostly interested in time complexity in this course Thus in this course the better algorithm is the one which runs faster (has smaller time complexity) Analysis of Algorithms 7
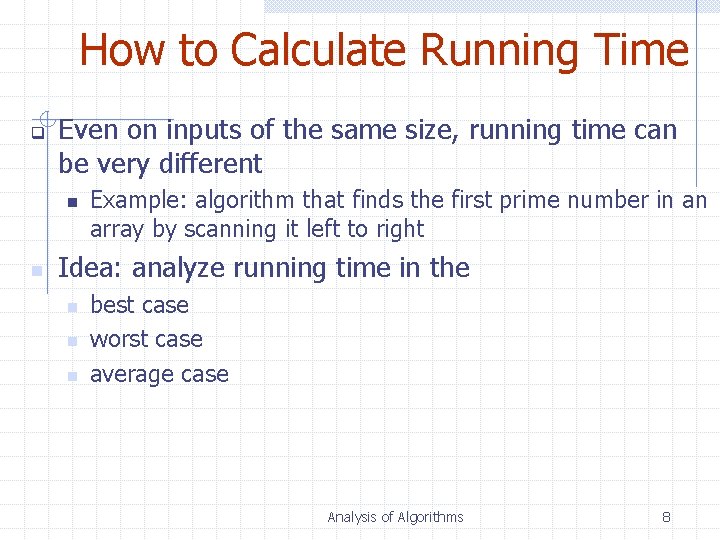
How to Calculate Running Time q Even on inputs of the same size, running time can be very different n n Example: algorithm that finds the first prime number in an array by scanning it left to right Idea: analyze running time in the n n n best case worst case average case Analysis of Algorithms 8
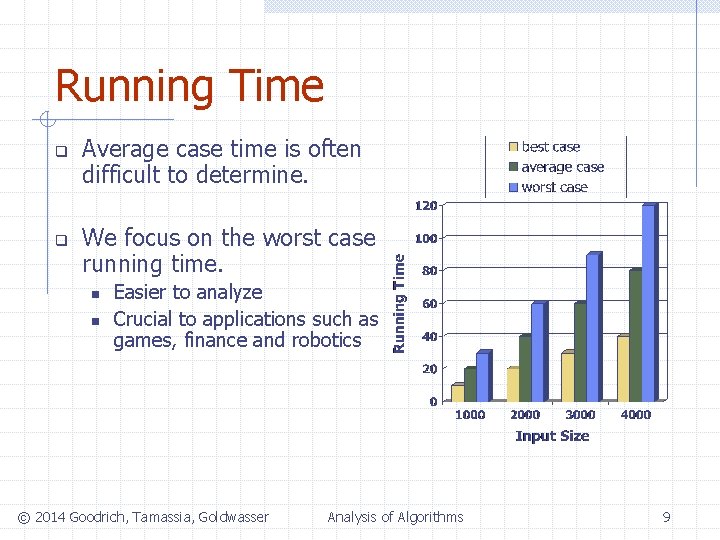
Running Time q q Average case time is often difficult to determine. We focus on the worst case running time. n n Easier to analyze Crucial to applications such as games, finance and robotics © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 9
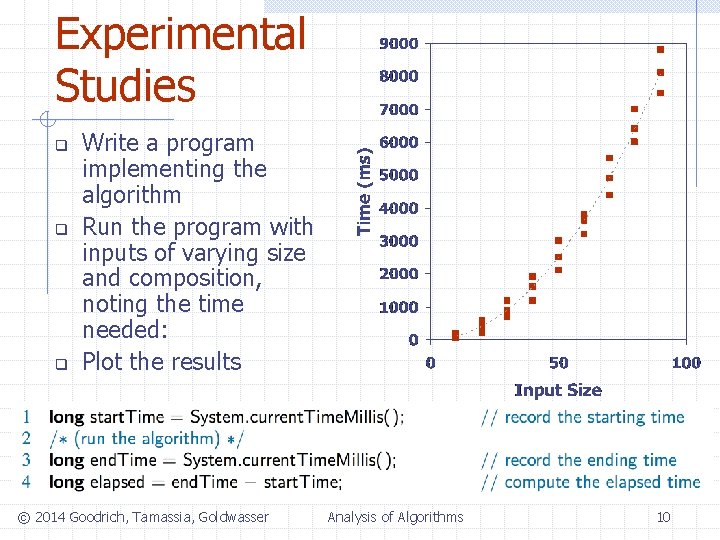
Experimental Studies q q q Write a program implementing the algorithm Run the program with inputs of varying size and composition, noting the time needed: Plot the results © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 10
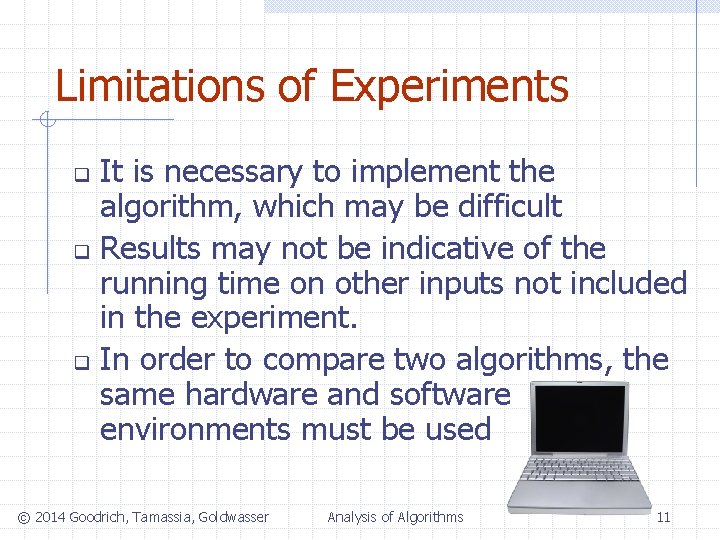
Limitations of Experiments It is necessary to implement the algorithm, which may be difficult q Results may not be indicative of the running time on other inputs not included in the experiment. q In order to compare two algorithms, the same hardware and software environments must be used q © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 11
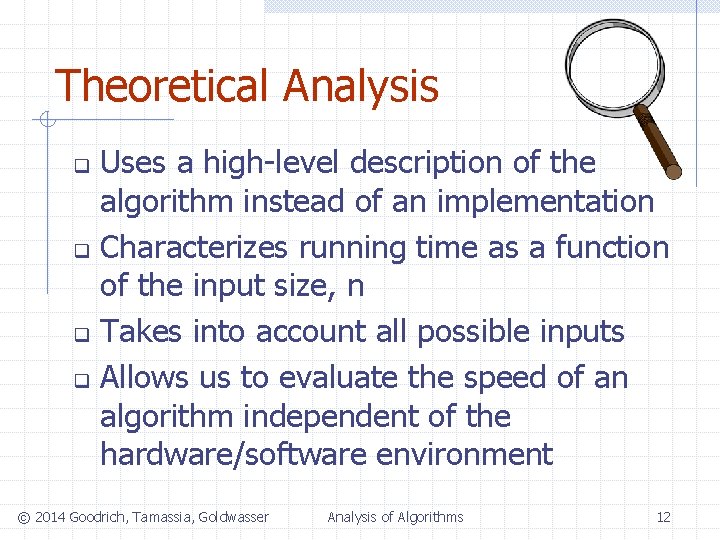
Theoretical Analysis Uses a high-level description of the algorithm instead of an implementation q Characterizes running time as a function of the input size, n q Takes into account all possible inputs q Allows us to evaluate the speed of an algorithm independent of the hardware/software environment q © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 12
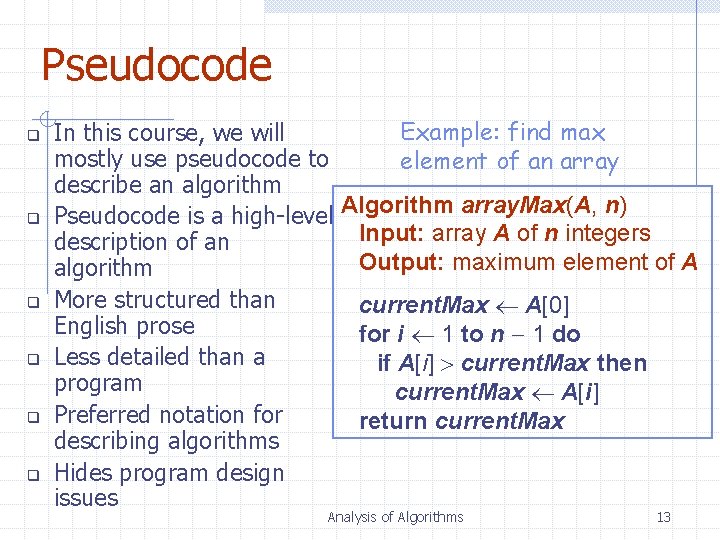
Pseudocode q q q Example: find max In this course, we will mostly use pseudocode to element of an array describe an algorithm Pseudocode is a high-level Algorithm array. Max(A, n) Input: array A of n integers description of an Output: maximum element of A algorithm More structured than current. Max A[0] English prose for i 1 to n 1 do Less detailed than a if A[i] current. Max then program current. Max A[i] Preferred notation for return current. Max describing algorithms Hides program design issues Analysis of Algorithms 13
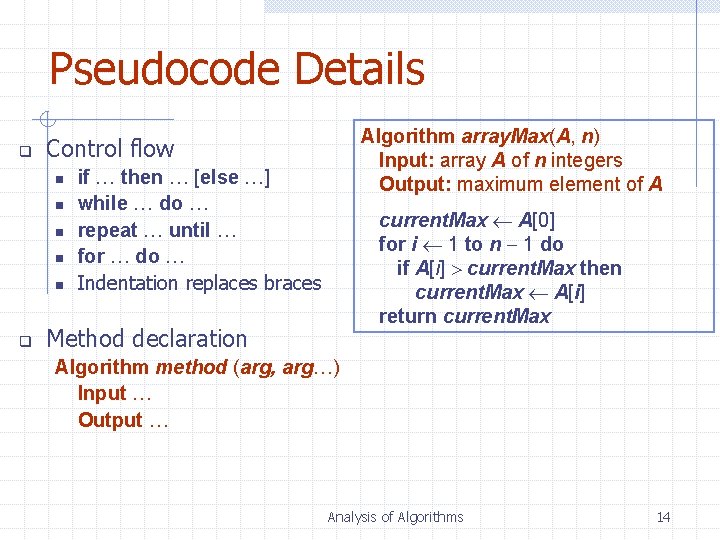
Pseudocode Details q Control flow n n n q Algorithm array. Max(A, n) Input: array A of n integers Output: maximum element of A if … then … [else …] while … do … repeat … until … for … do … Indentation replaces braces current. Max A[0] for i 1 to n 1 do if A[i] current. Max then current. Max A[i] return current. Max Method declaration Algorithm method (arg, arg…) Input … Output … Analysis of Algorithms 14
![Pseudocode Details q Method call var method arg arg q Return value return Pseudocode Details q Method call var. method (arg [, arg…]) q Return value return](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-15.jpg)
Pseudocode Details q Method call var. method (arg [, arg…]) q Return value return expression q Expressions Assignment (like in Java) Equality testing (like in Java) n 2 superscripts and other mathematical formatting allowed Algorithm array. Max(A, n) Input: array A of n integers Output: maximum element of A current. Max A[0] for i 1 to n 1 do if A[i] current. Max then current. Max A[i] return current. Max Analysis of Algorithms 15
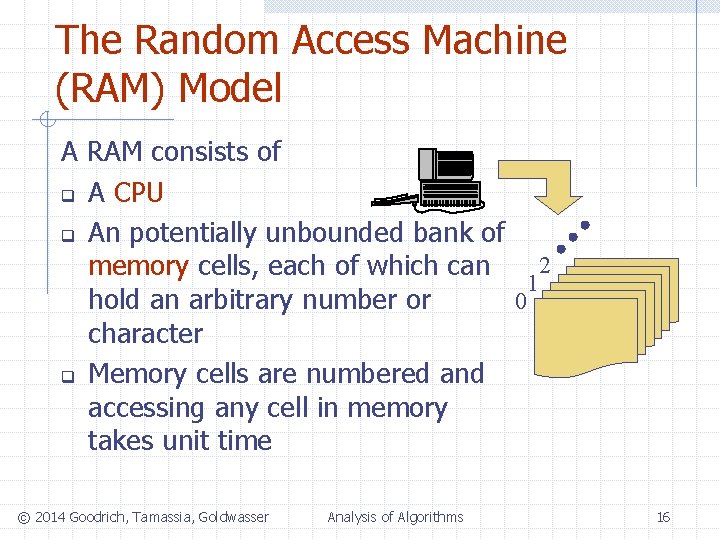
The Random Access Machine (RAM) Model A RAM consists of q A CPU q An potentially unbounded bank of 2 memory cells, each of which can 1 0 hold an arbitrary number or character q Memory cells are numbered and accessing any cell in memory takes unit time © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 16
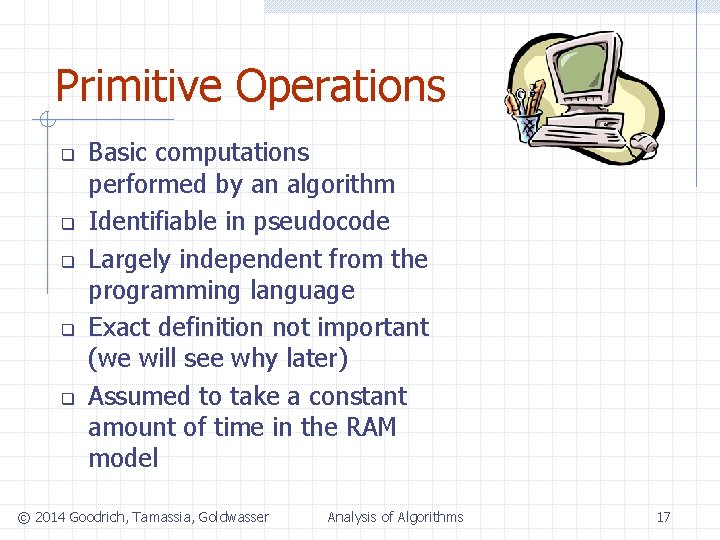
Primitive Operations q q q Basic computations performed by an algorithm Identifiable in pseudocode Largely independent from the programming language Exact definition not important (we will see why later) Assumed to take a constant amount of time in the RAM model © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 17
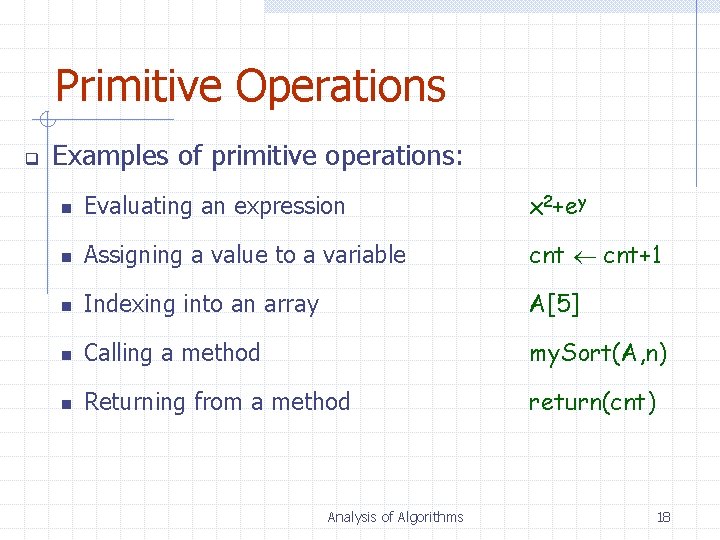
Primitive Operations q Examples of primitive operations: n Evaluating an expression x 2+ey n Assigning a value to a variable cnt+1 n Indexing into an array A[5] n Calling a method my. Sort(A, n) n Returning from a method return(cnt) Analysis of Algorithms 18
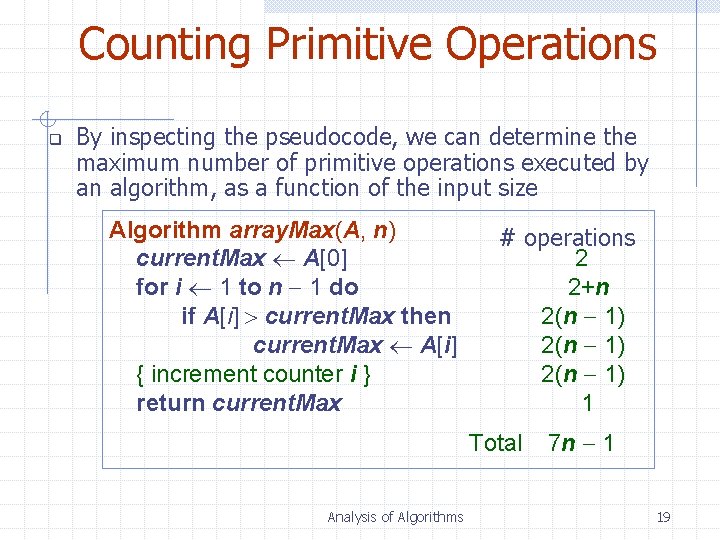
Counting Primitive Operations q By inspecting the pseudocode, we can determine the maximum number of primitive operations executed by an algorithm, as a function of the input size Algorithm array. Max(A, n) current. Max A[0] for i 1 to n 1 do if A[i] current. Max then current. Max A[i] { increment counter i } return current. Max # operations 2 2+n 2(n 1) 1 Total Analysis of Algorithms 7 n 1 19
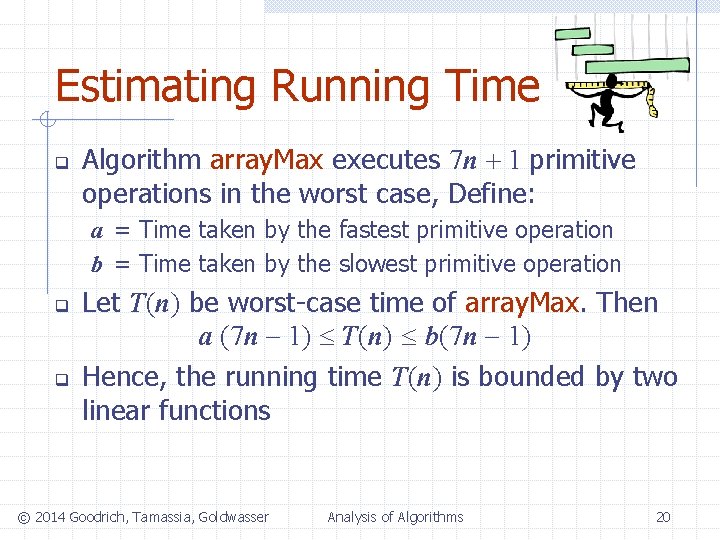
Estimating Running Time q Algorithm array. Max executes 7 n + 1 primitive operations in the worst case, Define: a = Time taken by the fastest primitive operation b = Time taken by the slowest primitive operation q q Let T(n) be worst-case time of array. Max. Then a (7 n 1) T(n) b(7 n 1) Hence, the running time T(n) is bounded by two linear functions © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 20
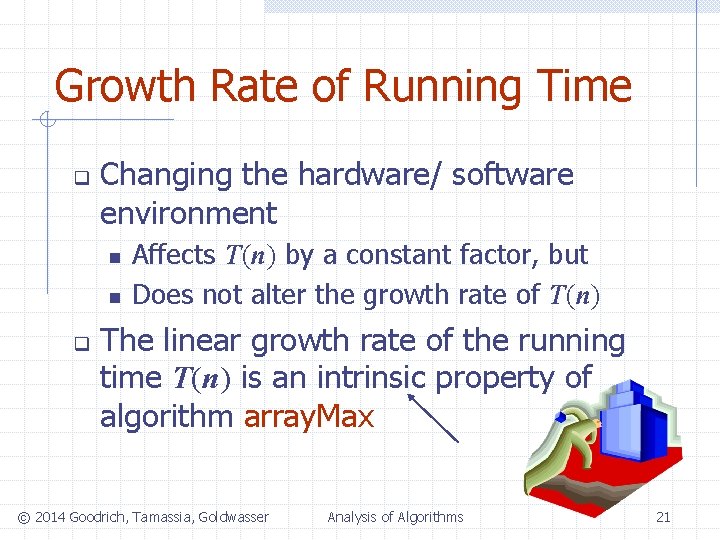
Growth Rate of Running Time q Changing the hardware/ software environment n n q Affects T(n) by a constant factor, but Does not alter the growth rate of T(n) The linear growth rate of the running time T(n) is an intrinsic property of algorithm array. Max © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 21
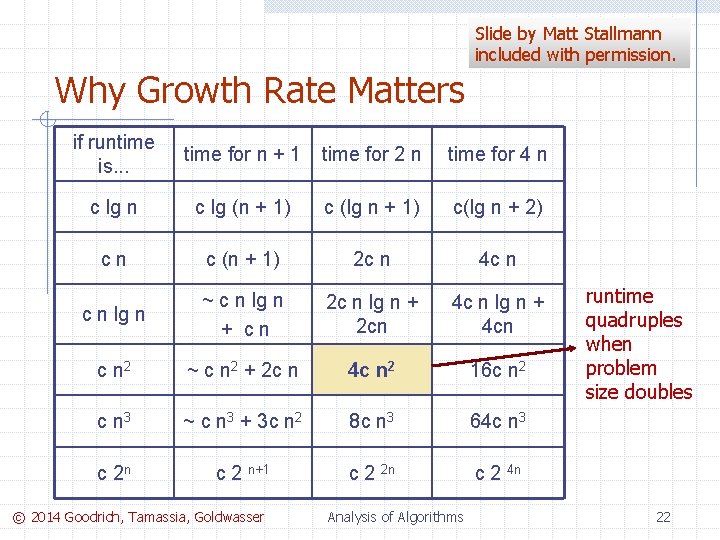
Slide by Matt Stallmann included with permission. Why Growth Rate Matters if runtime is. . . time for n + 1 time for 2 n time for 4 n c lg (n + 1) c (lg n + 1) c(lg n + 2) cn c (n + 1) 2 c n 4 c n lg n ~ c n lg n + cn 2 c n lg n + 2 cn 4 c n lg n + 4 cn c n 2 ~ c n 2 + 2 c n 4 c n 2 16 c n 2 c n 3 ~ c n 3 + 3 c n 2 8 c n 3 64 c n 3 c 2 n c 2 n+1 c 2 2 n c 2 4 n © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms runtime quadruples when problem size doubles 22
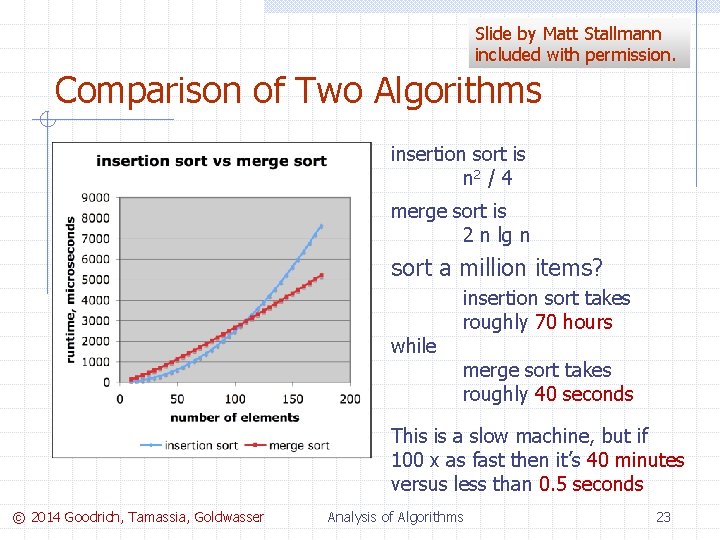
Slide by Matt Stallmann included with permission. Comparison of Two Algorithms insertion sort is n 2 / 4 merge sort is 2 n lg n sort a million items? while insertion sort takes roughly 70 hours merge sort takes roughly 40 seconds This is a slow machine, but if 100 x as fast then it’s 40 minutes versus less than 0. 5 seconds © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 23
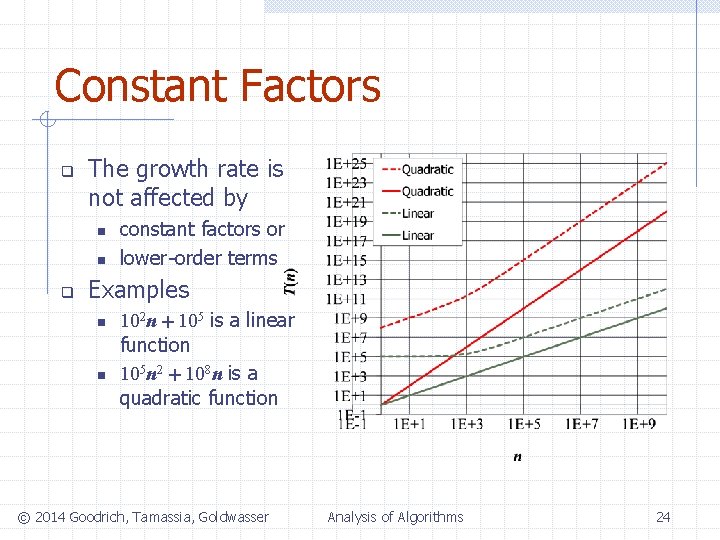
Constant Factors q The growth rate is not affected by n n q constant factors or lower-order terms Examples n n 102 n + 105 is a linear function 105 n 2 + 108 n is a quadratic function © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 24
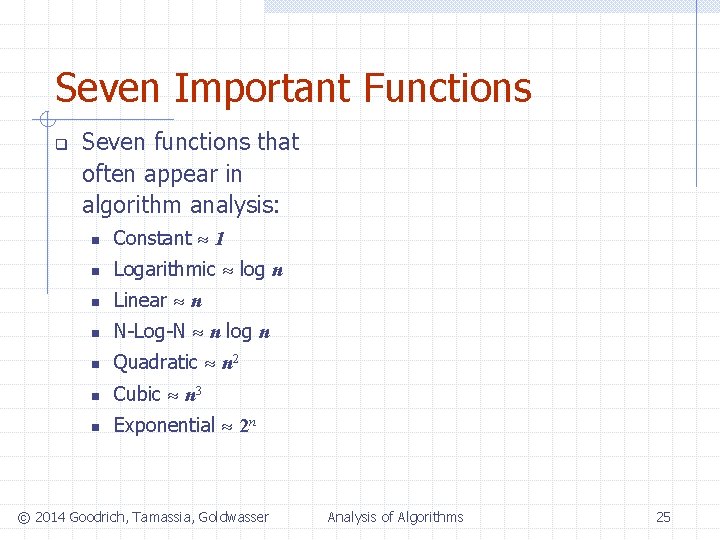
Seven Important Functions q Seven functions that often appear in algorithm analysis: n Constant 1 n Logarithmic log n n Linear n n N-Log-N n log n n Quadratic n 2 n Cubic n 3 n Exponential 2 n © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 25
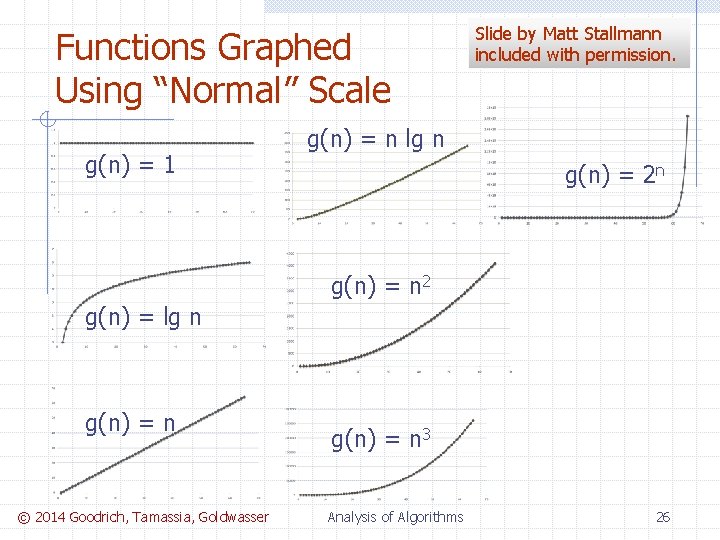
Functions Graphed Using “Normal” Scale g(n) = 1 Slide by Matt Stallmann included with permission. g(n) = n lg n g(n) = 2 n g(n) = n 2 g(n) = lg n g(n) = n © 2014 Goodrich, Tamassia, Goldwasser g(n) = n 3 Analysis of Algorithms 26
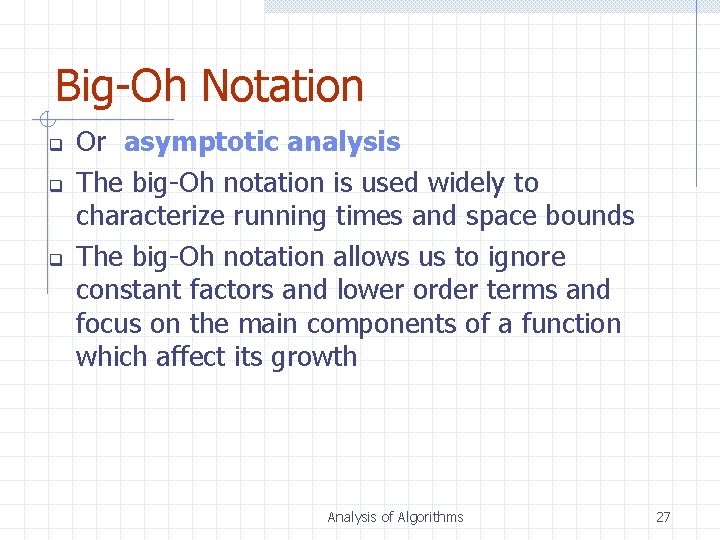
Big-Oh Notation q q q Or asymptotic analysis The big-Oh notation is used widely to characterize running times and space bounds The big-Oh notation allows us to ignore constant factors and lower order terms and focus on the main components of a function which affect its growth Analysis of Algorithms 27
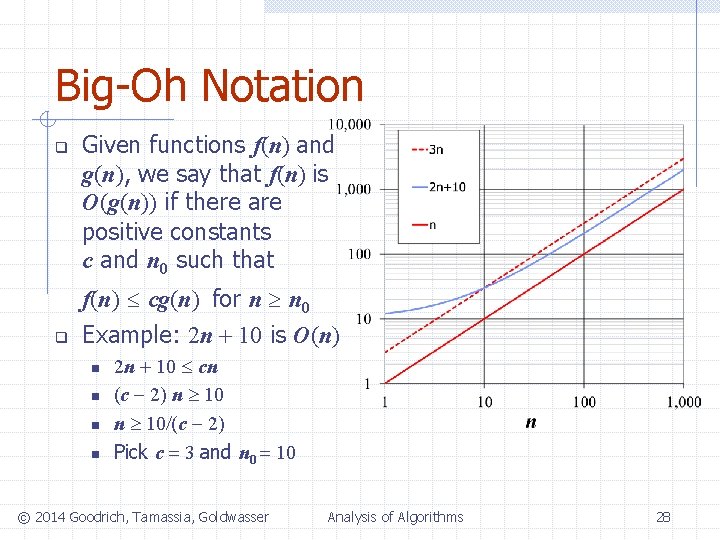
Big-Oh Notation q q Given functions f(n) and g(n), we say that f(n) is O(g(n)) if there are positive constants c and n 0 such that f(n) cg(n) for n n 0 Example: 2 n + 10 is O(n) n n 2 n + 10 cn (c 2) n 10/(c 2) Pick c 3 and n 0 10 © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 28
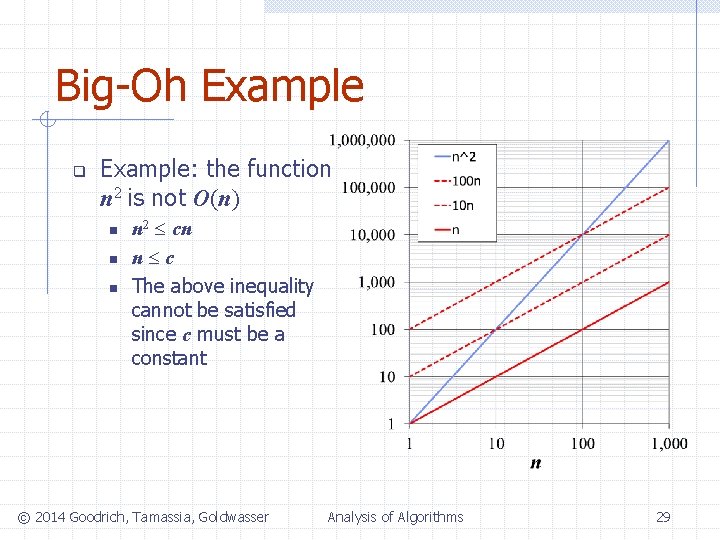
Big-Oh Example q Example: the function n 2 is not O(n) n n 2 cn n c The above inequality cannot be satisfied since c must be a constant © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 29
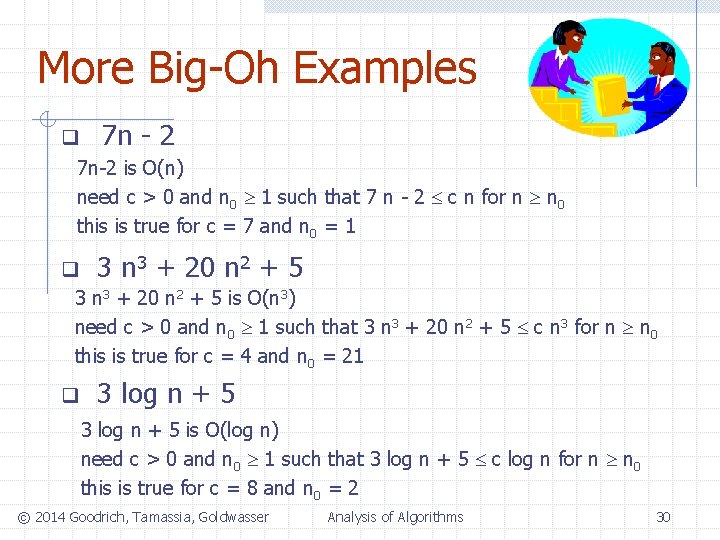
More Big-Oh Examples q 7 n - 2 7 n-2 is O(n) need c > 0 and n 0 1 such that 7 n - 2 c n for n n 0 this is true for c = 7 and n 0 = 1 q 3 n 3 + 20 n 2 + 5 is O(n 3) need c > 0 and n 0 1 such that 3 n 3 + 20 n 2 + 5 c n 3 for n n 0 this is true for c = 4 and n 0 = 21 q 3 log n + 5 is O(log n) need c > 0 and n 0 1 such that 3 log n + 5 c log n for n n 0 this is true for c = 8 and n 0 = 2 © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 30
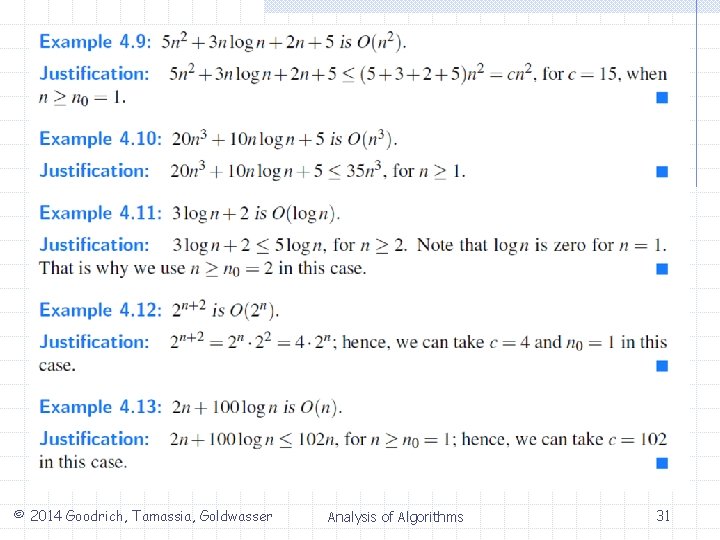
© 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 31
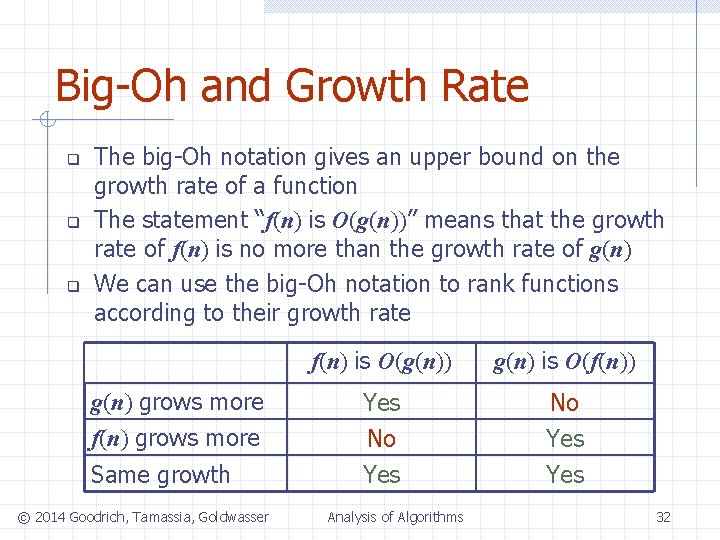
Big-Oh and Growth Rate q q q The big-Oh notation gives an upper bound on the growth rate of a function The statement “f(n) is O(g(n))” means that the growth rate of f(n) is no more than the growth rate of g(n) We can use the big-Oh notation to rank functions according to their growth rate g(n) grows more f(n) grows more Same growth © 2014 Goodrich, Tamassia, Goldwasser f(n) is O(g(n)) g(n) is O(f(n)) Yes No Yes Analysis of Algorithms 32
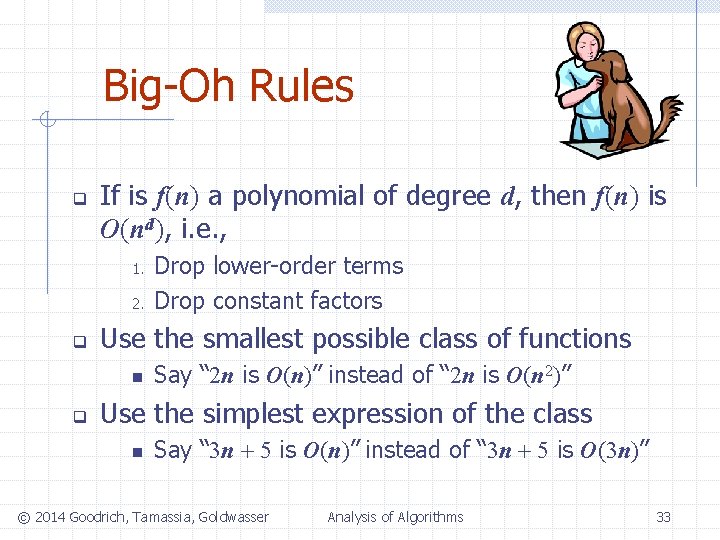
Big-Oh Rules q If is f(n) a polynomial of degree d, then f(n) is O(nd), i. e. , 1. 2. q Use the smallest possible class of functions n q Drop lower-order terms Drop constant factors Say “ 2 n is O(n)” instead of “ 2 n is O(n 2)” Use the simplest expression of the class n Say “ 3 n + 5 is O(n)” instead of “ 3 n + 5 is O(3 n)” © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 33
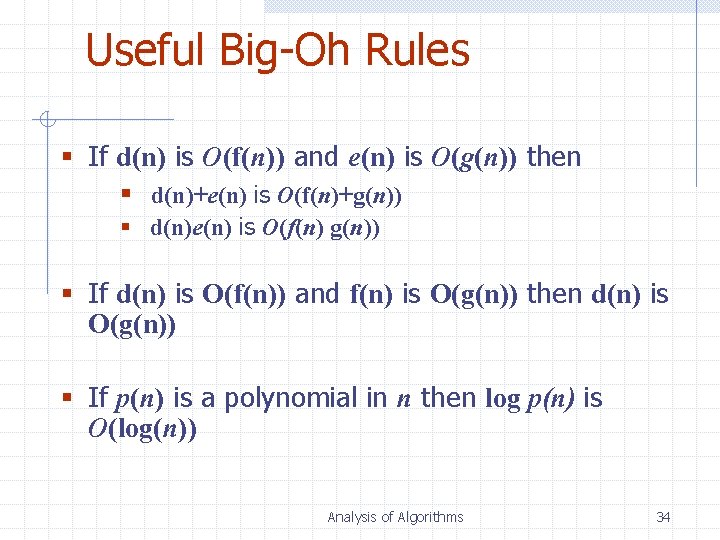
Useful Big-Oh Rules § If d(n) is O(f(n)) and e(n) is O(g(n)) then § d(n)+e(n) is O(f(n)+g(n)) § d(n)e(n) is O(f(n) g(n)) § If d(n) is O(f(n)) and f(n) is O(g(n)) then d(n) is O(g(n)) § If p(n) is a polynomial in n then log p(n) is O(log(n)) Analysis of Algorithms 34
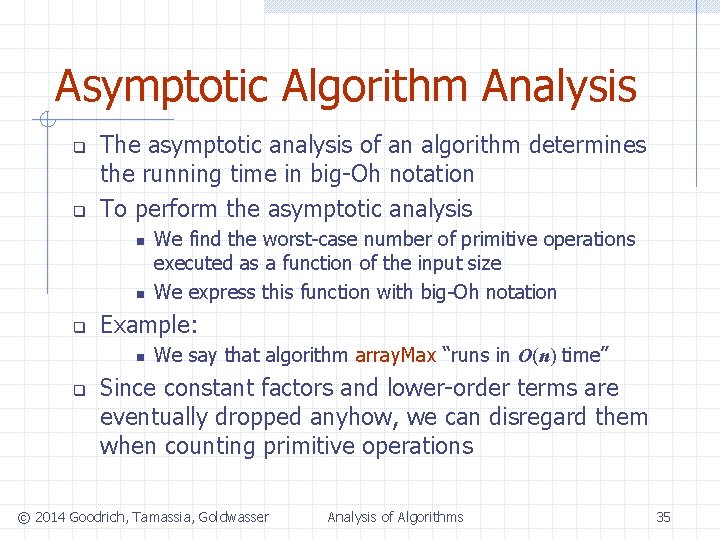
Asymptotic Algorithm Analysis q q The asymptotic analysis of an algorithm determines the running time in big-Oh notation To perform the asymptotic analysis n n q Example: n q We find the worst-case number of primitive operations executed as a function of the input size We express this function with big-Oh notation We say that algorithm array. Max “runs in O(n) time” Since constant factors and lower-order terms are eventually dropped anyhow, we can disregard them when counting primitive operations © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 35
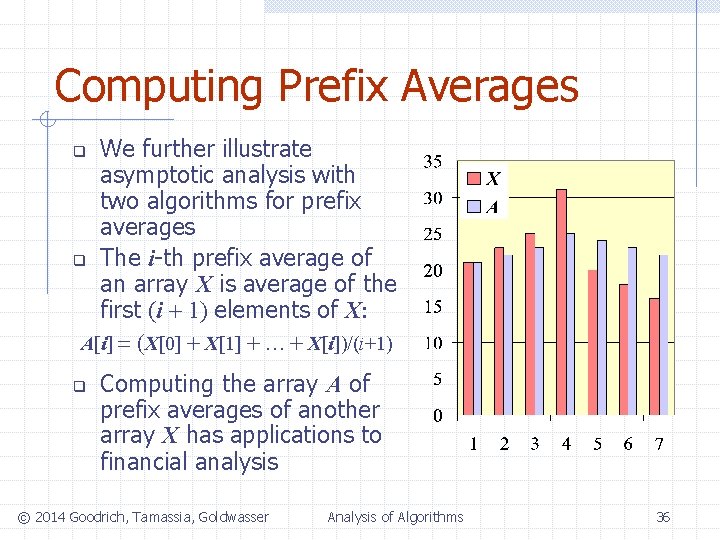
Computing Prefix Averages We further illustrate asymptotic analysis with two algorithms for prefix averages q The i-th prefix average of an array X is average of the first (i + 1) elements of X: A[i] (X[0] + X[1] + … + X[i])/(i+1) q q Computing the array A of prefix averages of another array X has applications to financial analysis © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 36
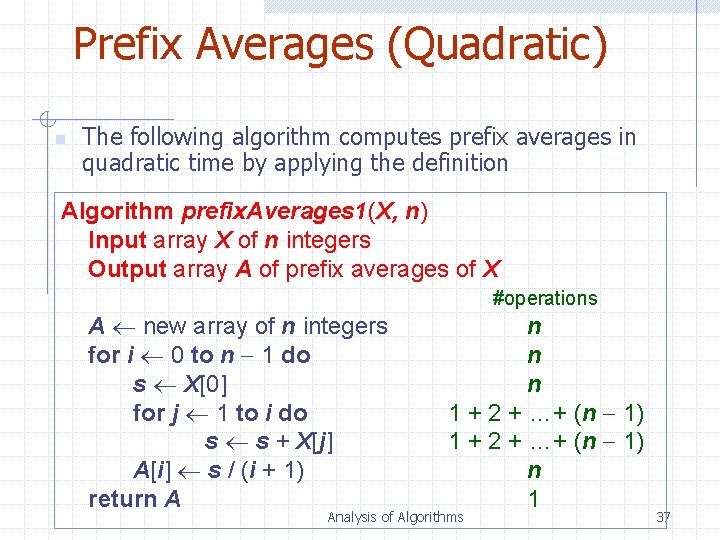
Prefix Averages (Quadratic) n The following algorithm computes prefix averages in quadratic time by applying the definition Algorithm prefix. Averages 1(X, n) Input array X of n integers Output array A of prefix averages of X #operations A new array of n integers for i 0 to n 1 do s X[0] for j 1 to i do s s + X[j] A[i] s / (i + 1) return A n n n 1 + 2 + …+ (n 1) n 1 Analysis of Algorithms 37
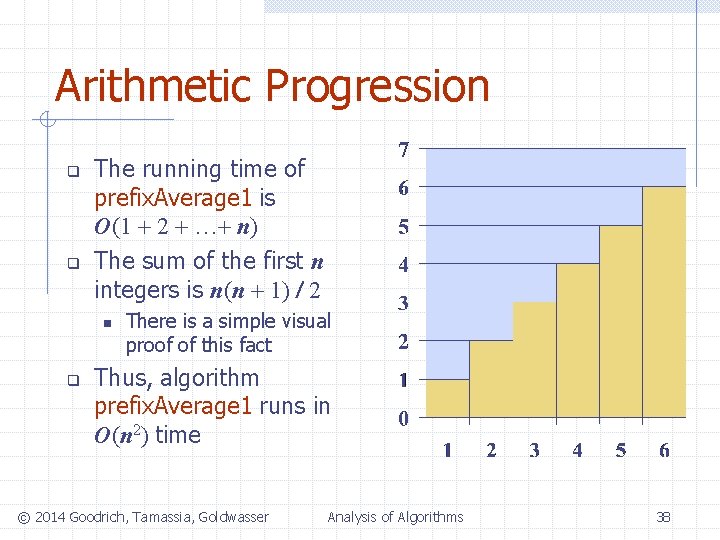
Arithmetic Progression q q The running time of prefix. Average 1 is O(1 + 2 + …+ n) The sum of the first n integers is n(n + 1) / 2 n q There is a simple visual proof of this fact Thus, algorithm prefix. Average 1 runs in O(n 2) time © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 38
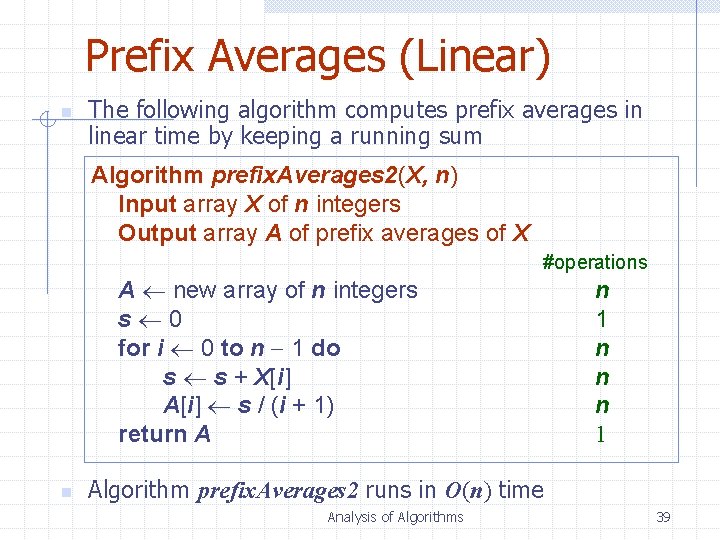
Prefix Averages (Linear) n The following algorithm computes prefix averages in linear time by keeping a running sum Algorithm prefix. Averages 2(X, n) Input array X of n integers Output array A of prefix averages of X #operations A new array of n integers s 0 for i 0 to n 1 do s s + X[i] A[i] s / (i + 1) return A n n 1 n n n 1 Algorithm prefix. Averages 2 runs in O(n) time Analysis of Algorithms 39
![More Examples Algorithm Sum Triple ArrayX n Input triple array X More Examples Algorithm Sum. Triple. Array(X, n) Input triple array X[ ][ ][ ]](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-40.jpg)
More Examples Algorithm Sum. Triple. Array(X, n) Input triple array X[ ][ ][ ] of n by n integers Output sum of elements of X #operations s 0 1 for i 0 to n 1 do n for j 0 to n 1 do n+n+…+n=n 2 for k 0 to n 1 do n 2+…+n 2 = n 3 s s + X[i][j][k] n 2+…+n 2 = n 3 return s 1 n Algorithm Sum. Triple. Array runs in O(n 3) time Analysis of Algorithms 40
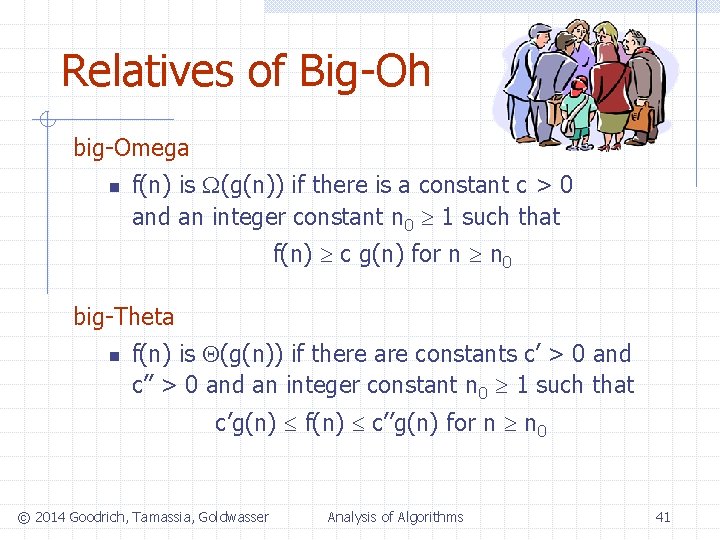
Relatives of Big-Oh big-Omega n f(n) is (g(n)) if there is a constant c > 0 and an integer constant n 0 1 such that f(n) c g(n) for n n 0 big-Theta n f(n) is (g(n)) if there are constants c’ > 0 and c’’ > 0 and an integer constant n 0 1 such that c’g(n) f(n) c’’g(n) for n n 0 © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 41
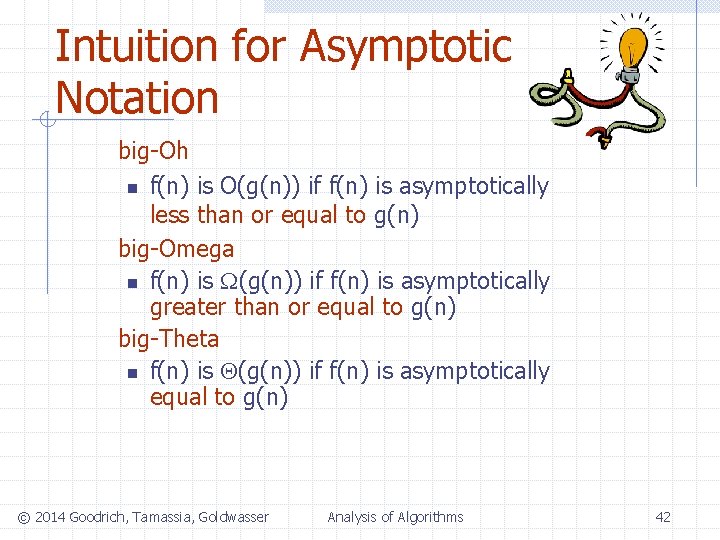
Intuition for Asymptotic Notation big-Oh n f(n) is O(g(n)) if f(n) is asymptotically less than or equal to g(n) big-Omega n f(n) is (g(n)) if f(n) is asymptotically greater than or equal to g(n) big-Theta n f(n) is (g(n)) if f(n) is asymptotically equal to g(n) © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 42
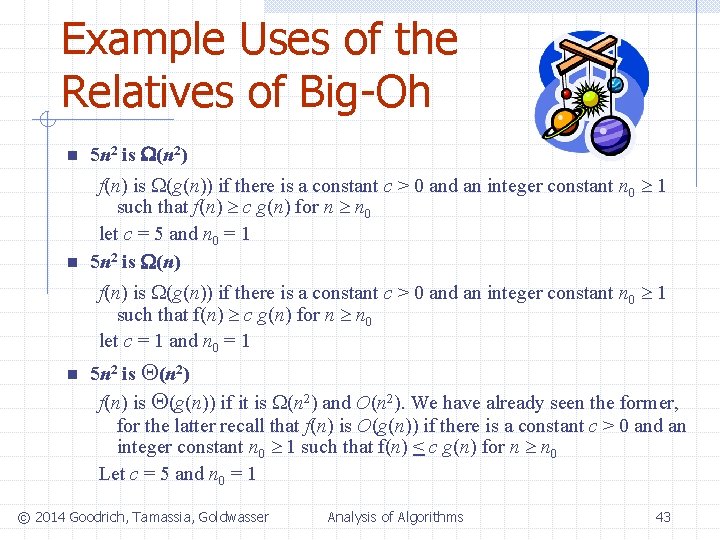
Example Uses of the Relatives of Big-Oh n 5 n 2 is (n 2) n f(n) is (g(n)) if there is a constant c > 0 and an integer constant n 0 1 such that f(n) c g(n) for n n 0 let c = 5 and n 0 = 1 5 n 2 is (n) f(n) is (g(n)) if there is a constant c > 0 and an integer constant n 0 1 such that f(n) c g(n) for n n 0 let c = 1 and n 0 = 1 n 5 n 2 is (n 2) f(n) is (g(n)) if it is (n 2) and O(n 2). We have already seen the former, for the latter recall that f(n) is O(g(n)) if there is a constant c > 0 and an integer constant n 0 1 such that f(n) < c g(n) for n n 0 Let c = 5 and n 0 = 1 © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 43
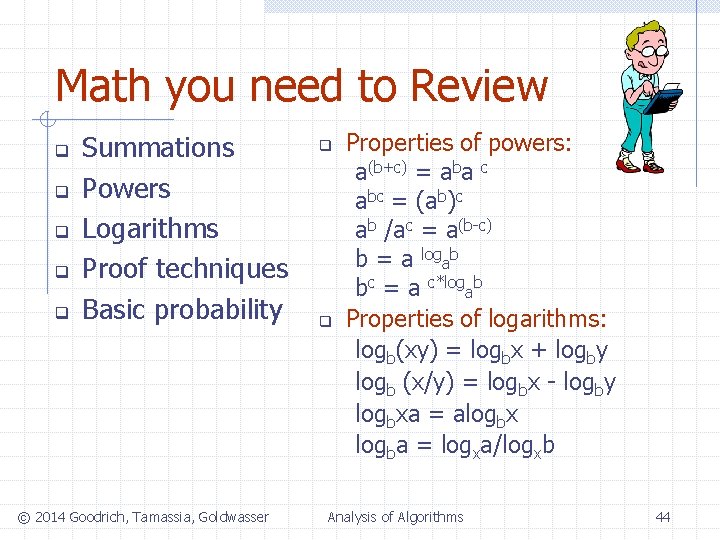
Math you need to Review q q q Summations Powers Logarithms Proof techniques Basic probability © 2014 Goodrich, Tamassia, Goldwasser q q Properties of powers: a(b+c) = aba c abc = (ab)c ab /ac = a(b-c) b = a logab bc = a c*logab Properties of logarithms: logb(xy) = logbx + logby logb (x/y) = logbx - logby logbxa = alogbx logba = logxa/logxb Analysis of Algorithms 44
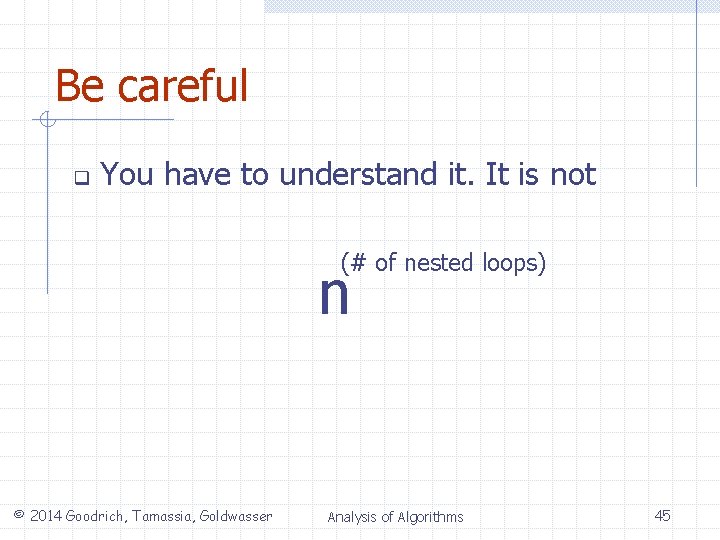
Be careful q You have to understand it. It is not (# of nested loops) n © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 45
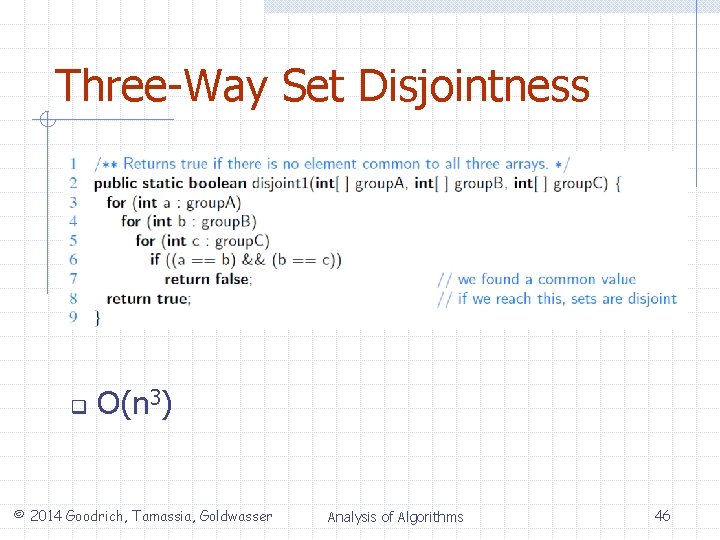
Three-Way Set Disjointness q O(n 3) © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 46
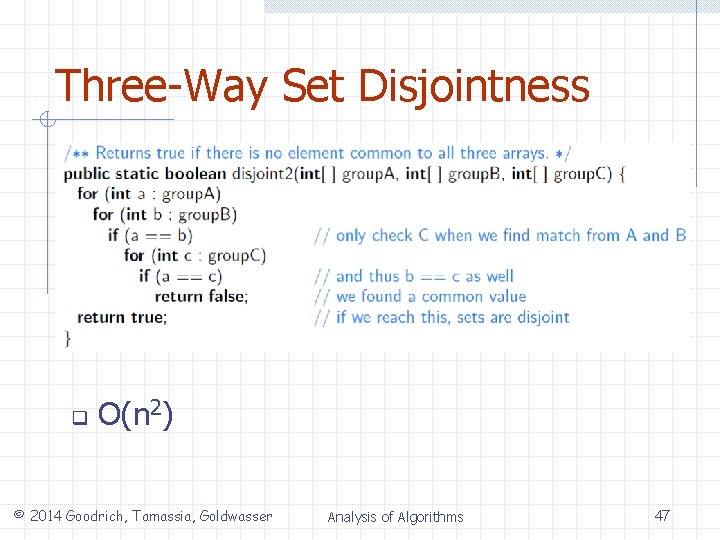
Three-Way Set Disjointness q O(n 2) © 2014 Goodrich, Tamassia, Goldwasser Analysis of Algorithms 47
![Reading G Chapter 4 If you feel you need more then read Reading • [G] Chapter 4 • If you feel you need more then read](https://slidetodoc.com/presentation_image_h2/43c0e015808bf74188f1007b69f6ece2/image-48.jpg)
Reading • [G] Chapter 4 • If you feel you need more then read • • [L] 2. 1 and 2. 2 If you fell you need even more then read • [K] 2. 1 and 2. 2 • If you feel you need even more • [C] Chapter 3 48
Ajit diwan
Cos 423 princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan
Algorithms + data structures = programs
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Information retrieval data structures and algorithms
Data structures and algorithms
Advantages and disadvantages of arrays in data structure
Parallel arrays java
Homologous structures
Recall data structure
Array of arrays c++
Parallel arrays
潘仁義
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Variables unidimensionales ejemplos
Arreglos bidimensionales java
Mips array example
Polynomial representation using array in c
Strings in assembly language
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
I wonder is it possible
Pascal multidimensional array
Mips dynamic array
Creating arrays matlab
Arrays as adt
Java partially filled array
Redundant arrays of independent disks
Python list of arrays
Arrays
Day 3: arrays
Redundant array of inexpensive disks
Small basic array
Disadvantages of array
Microled arrays
Are vectors dynamic arrays
Facts about arrays
They say sometimes you win some