Python I Skill Session What is a Python
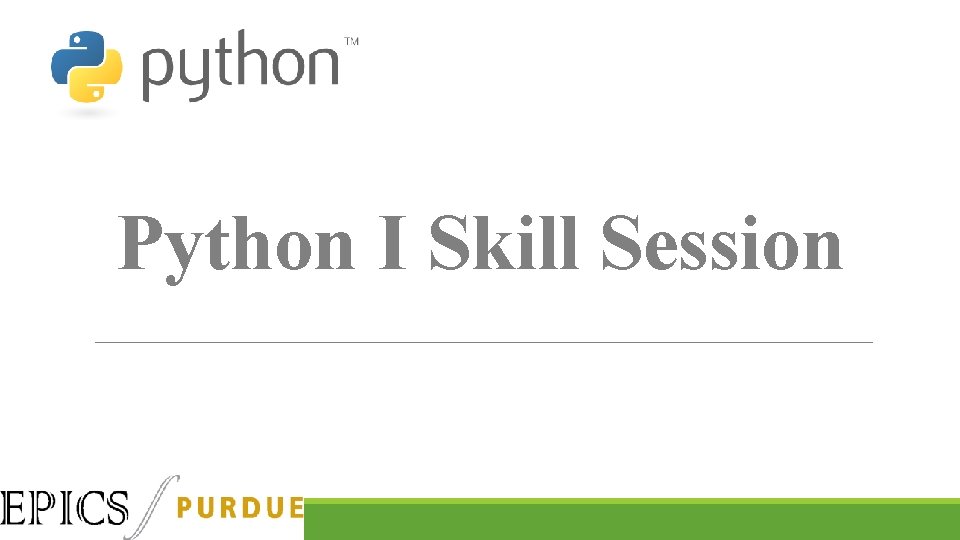
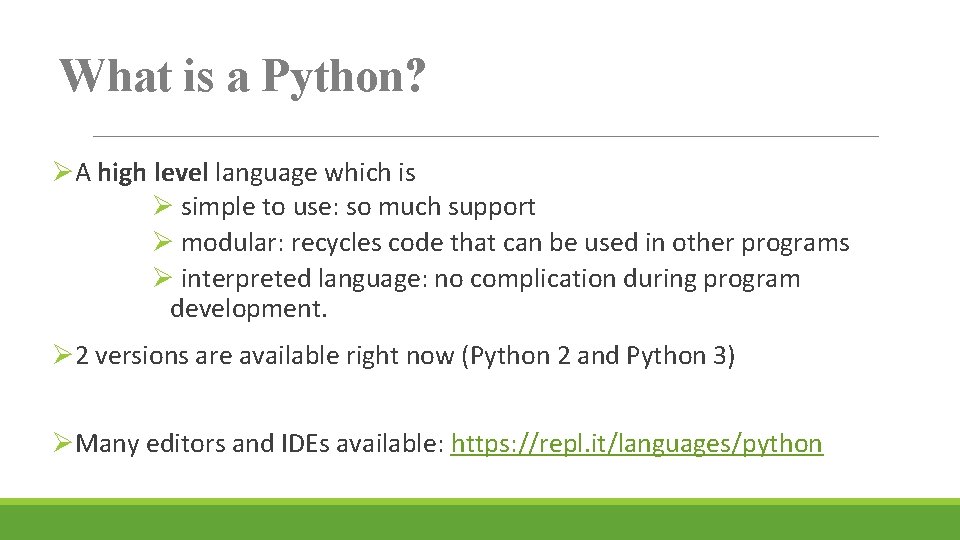
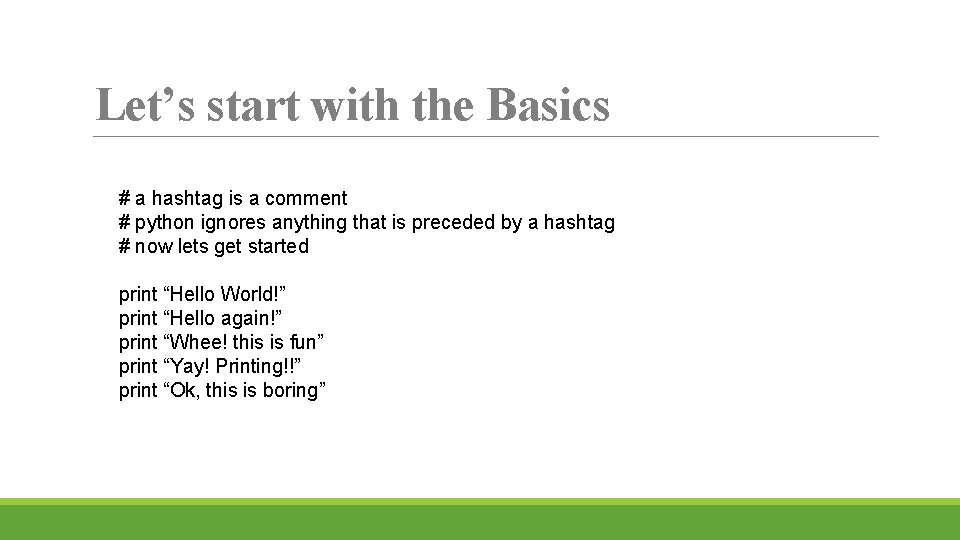
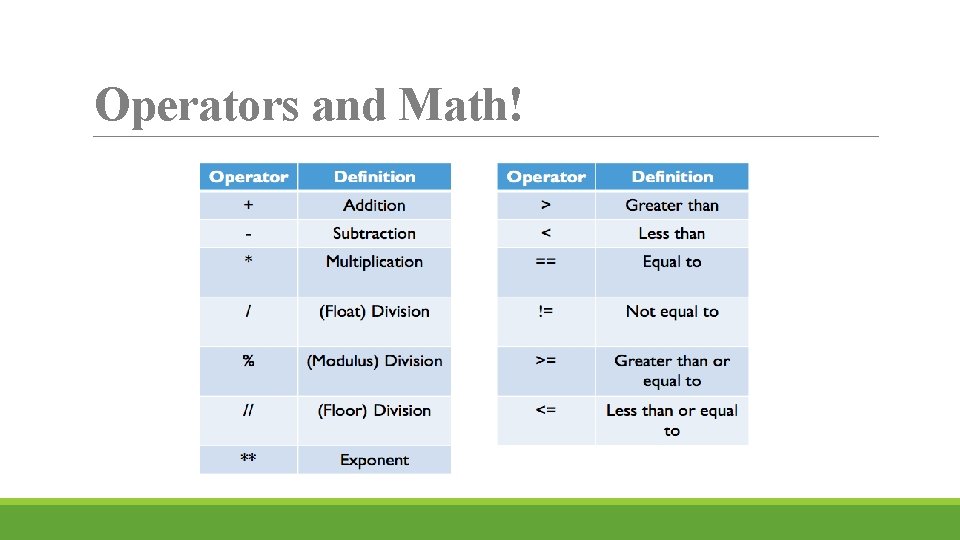
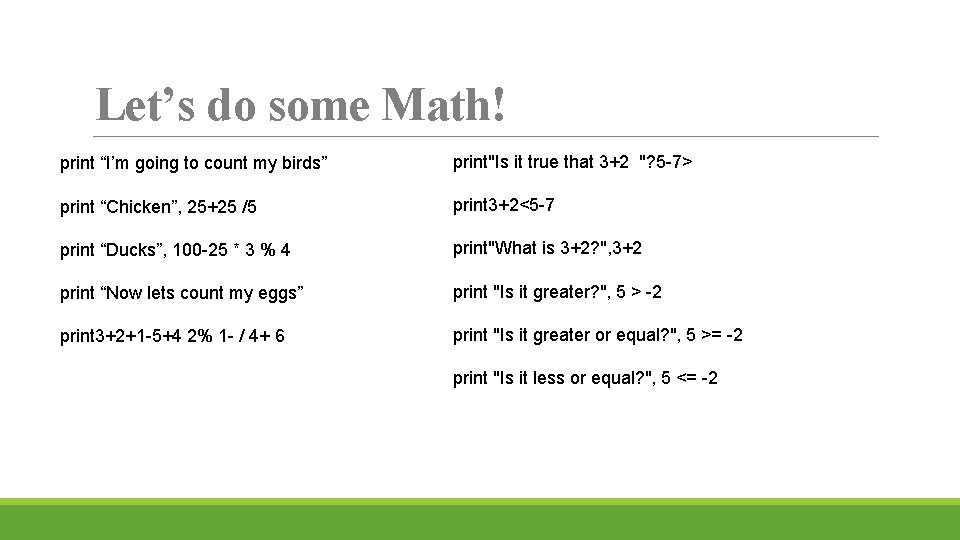
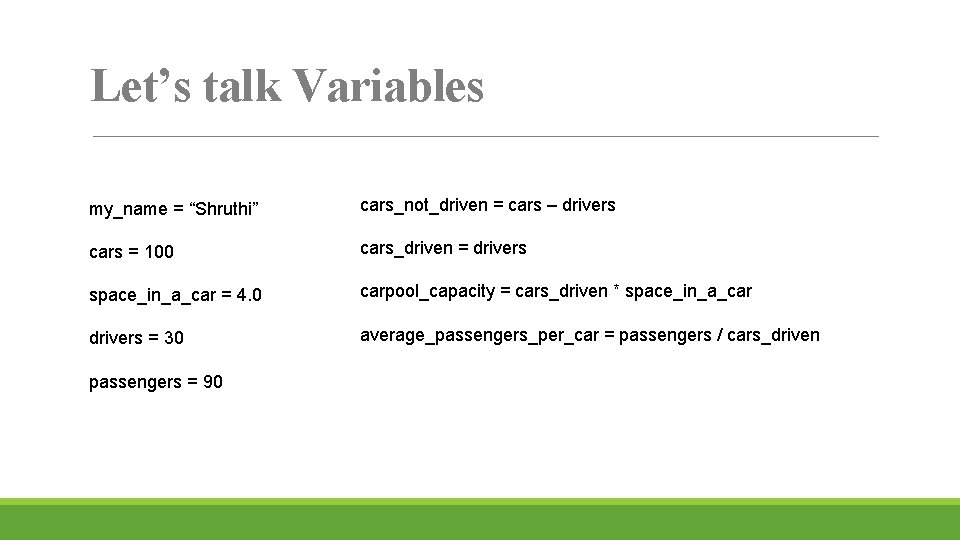
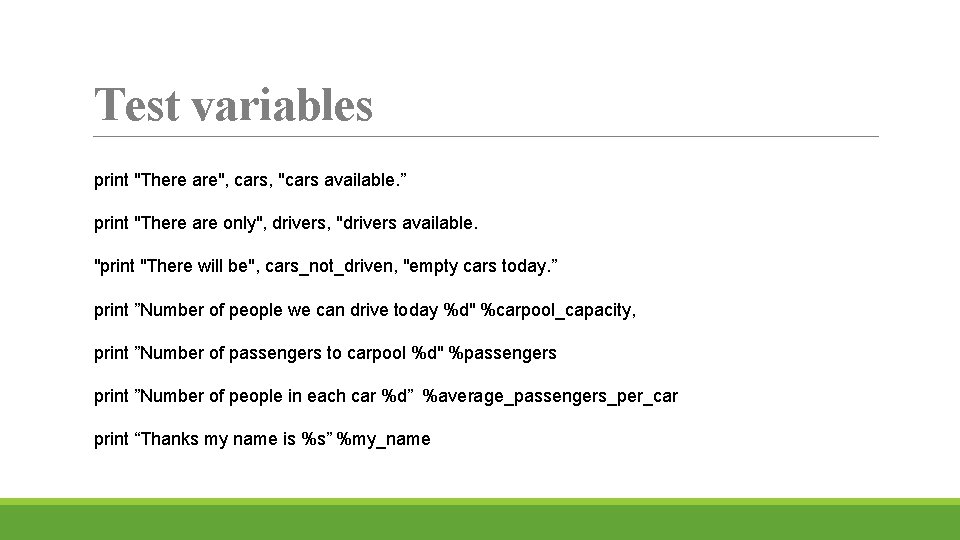
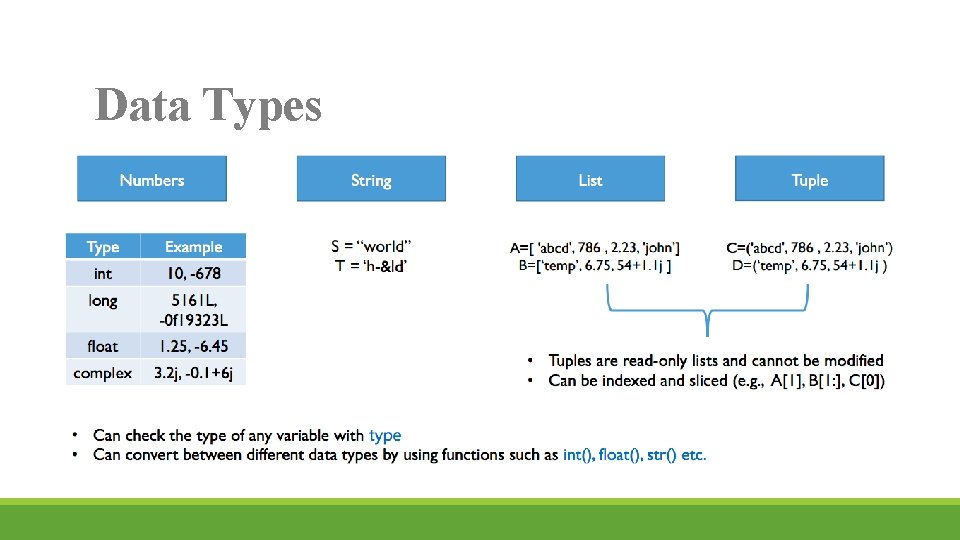
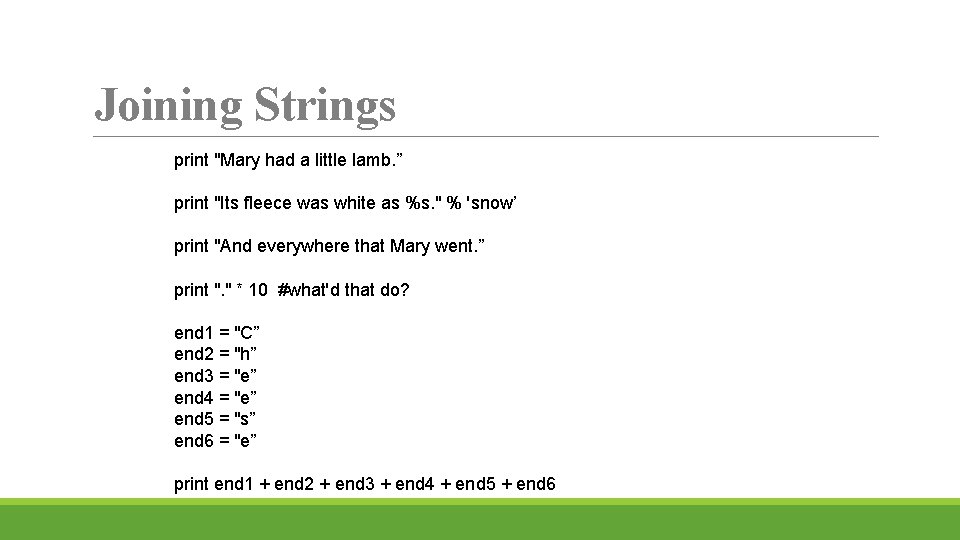
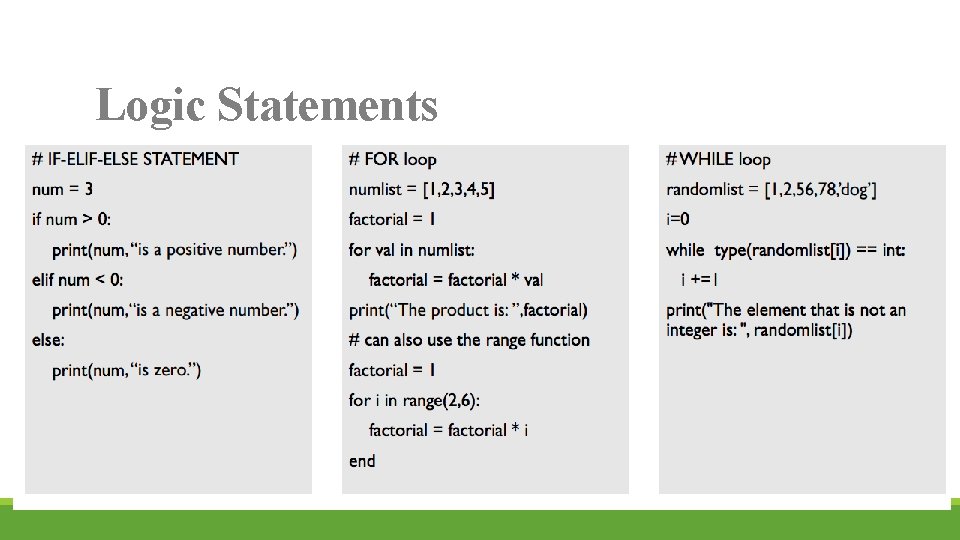
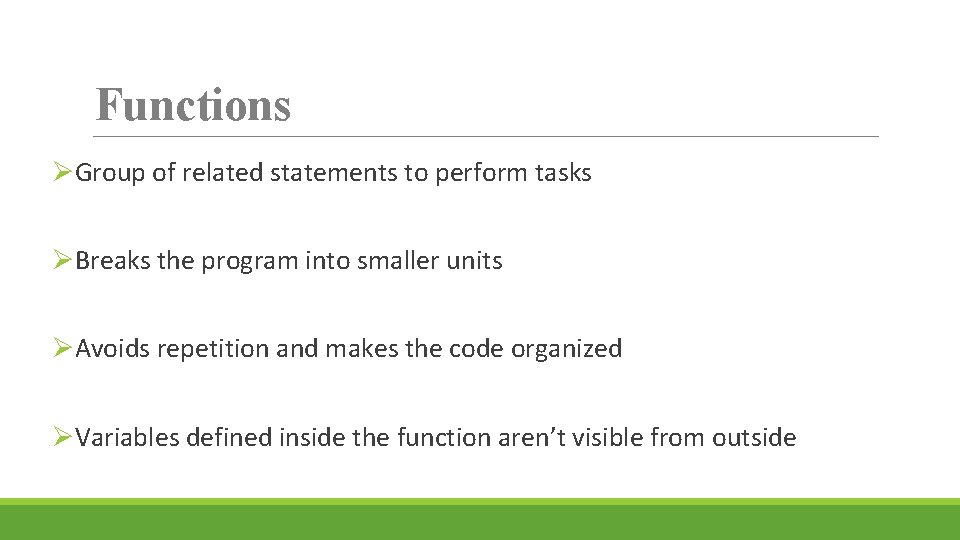
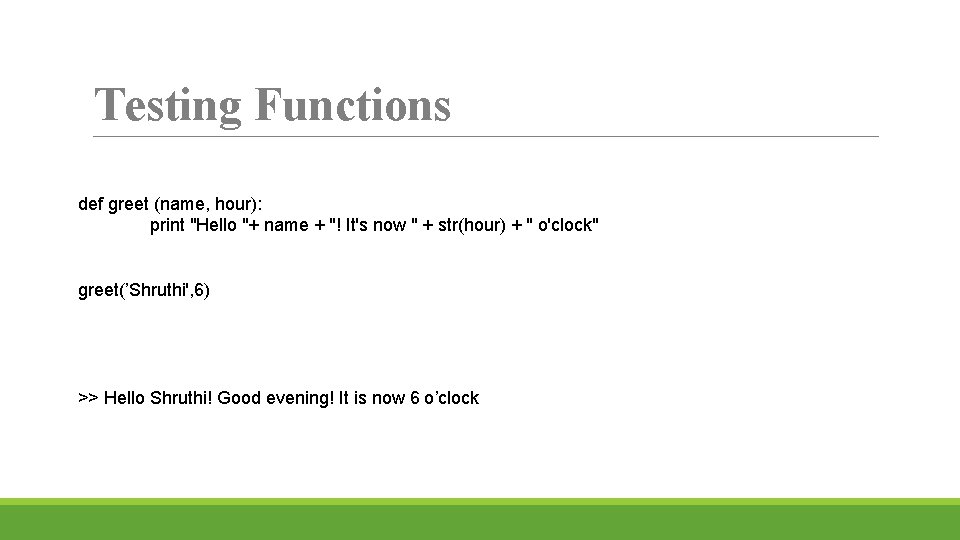
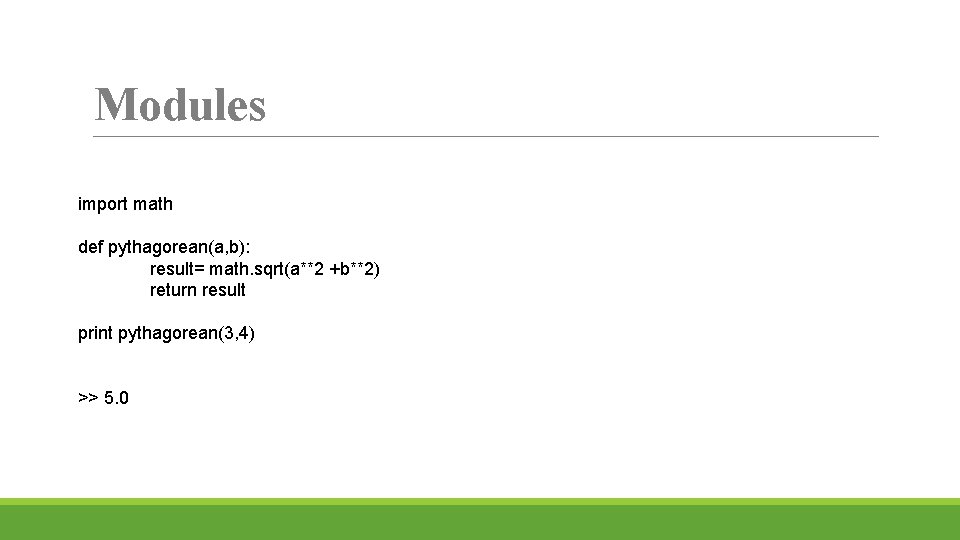
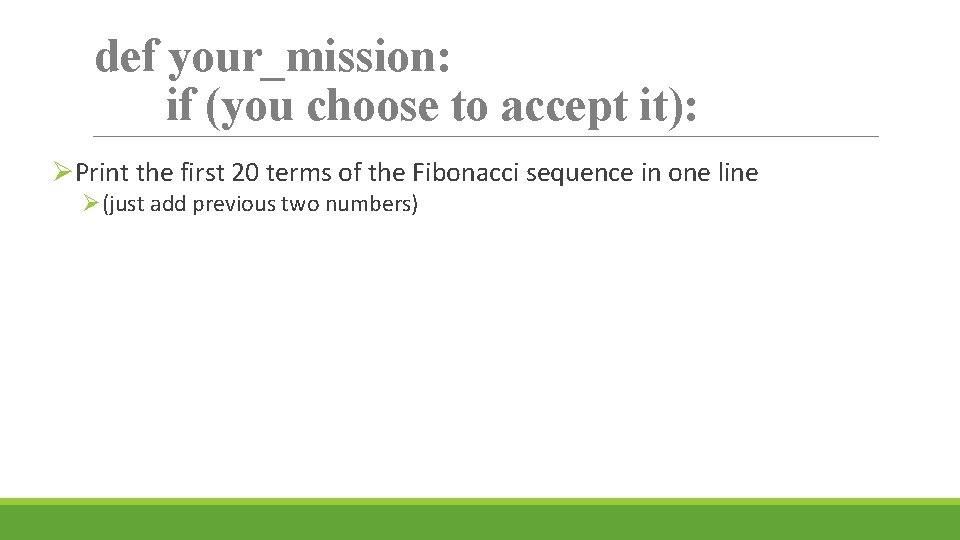
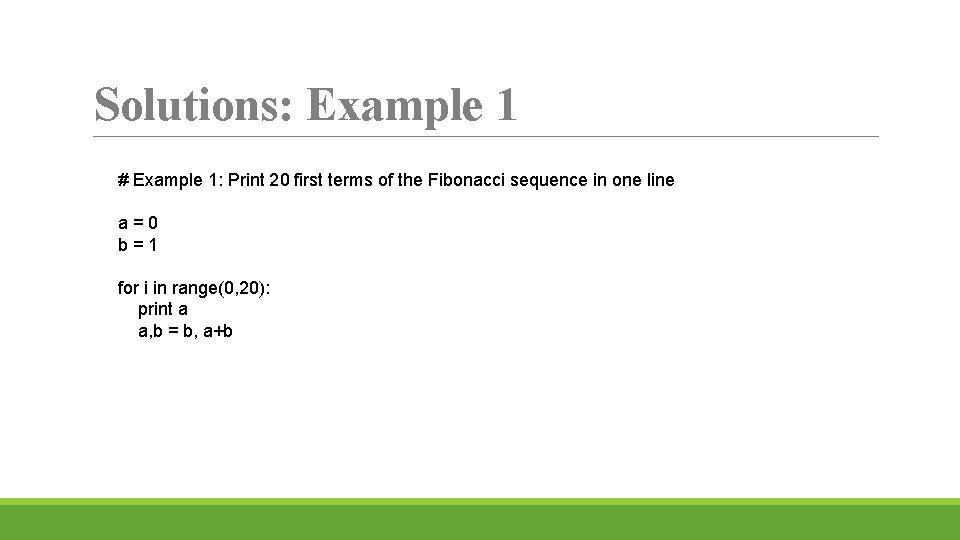
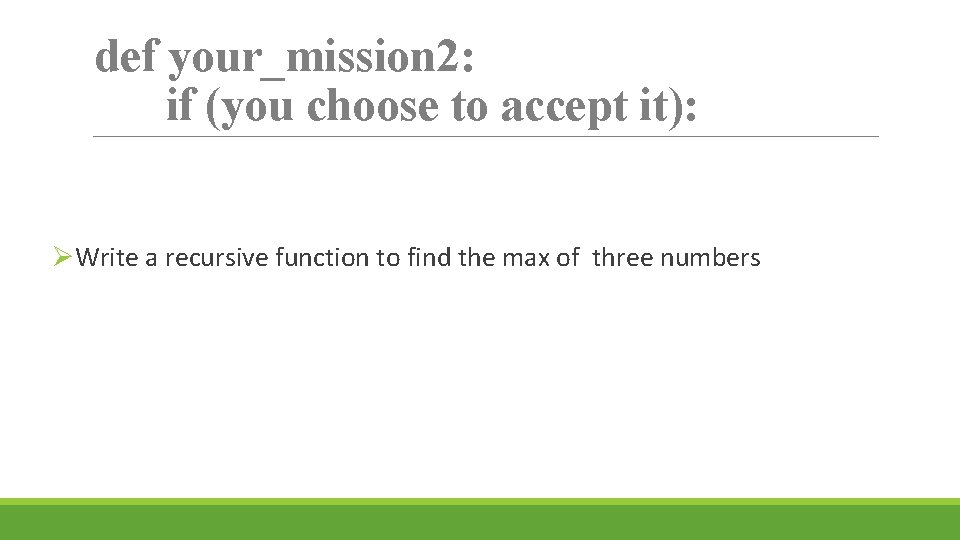
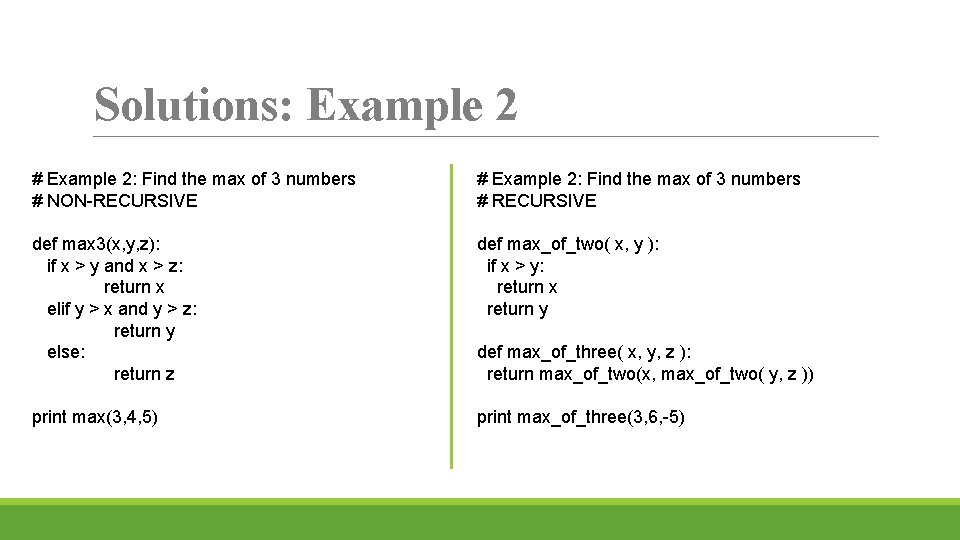
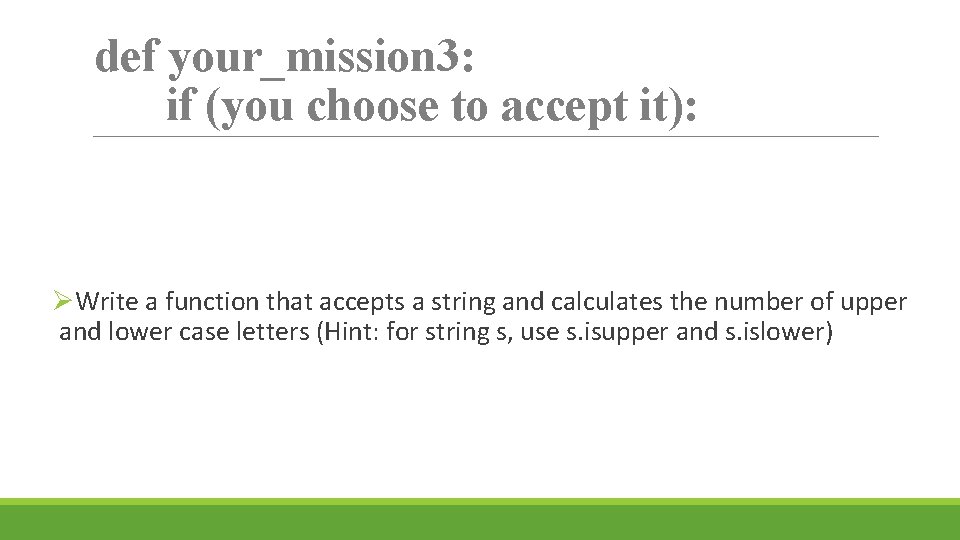
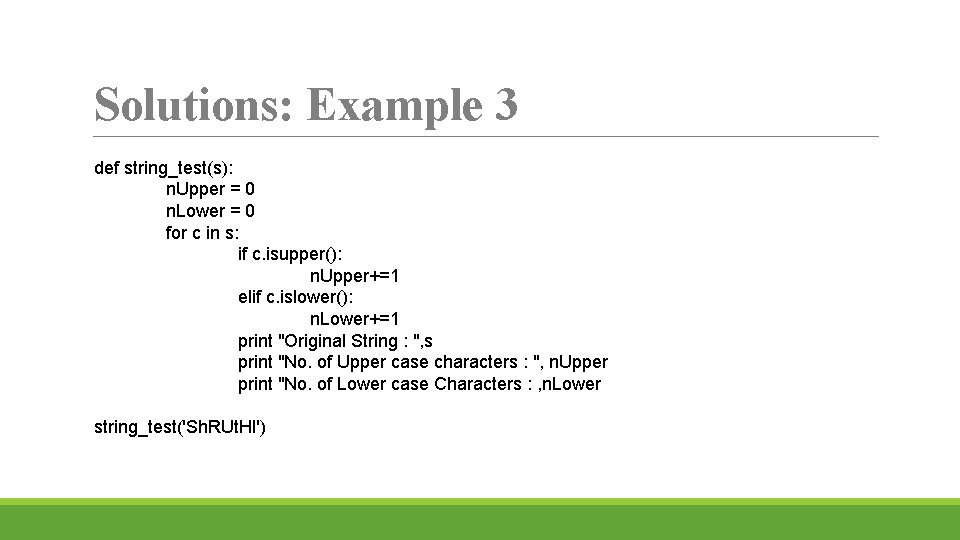
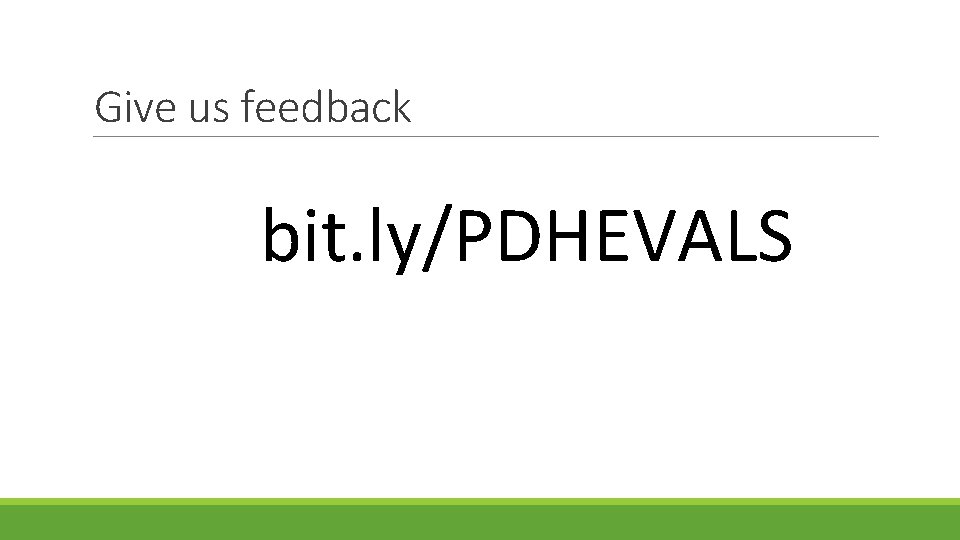
- Slides: 20
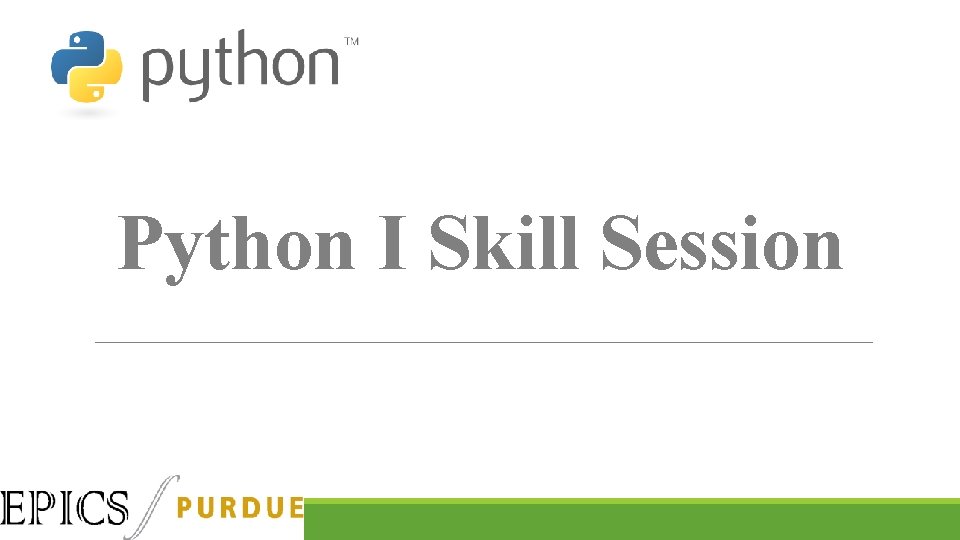
Python I Skill Session
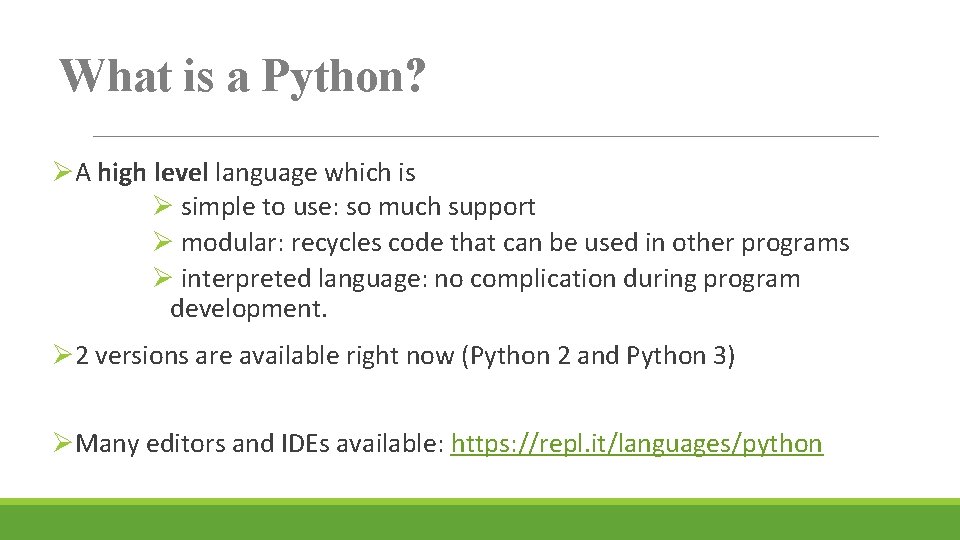
What is a Python? ØA high level language which is Ø simple to use: so much support Ø modular: recycles code that can be used in other programs Ø interpreted language: no complication during program development. Ø 2 versions are available right now (Python 2 and Python 3) ØMany editors and IDEs available: https: //repl. it/languages/python
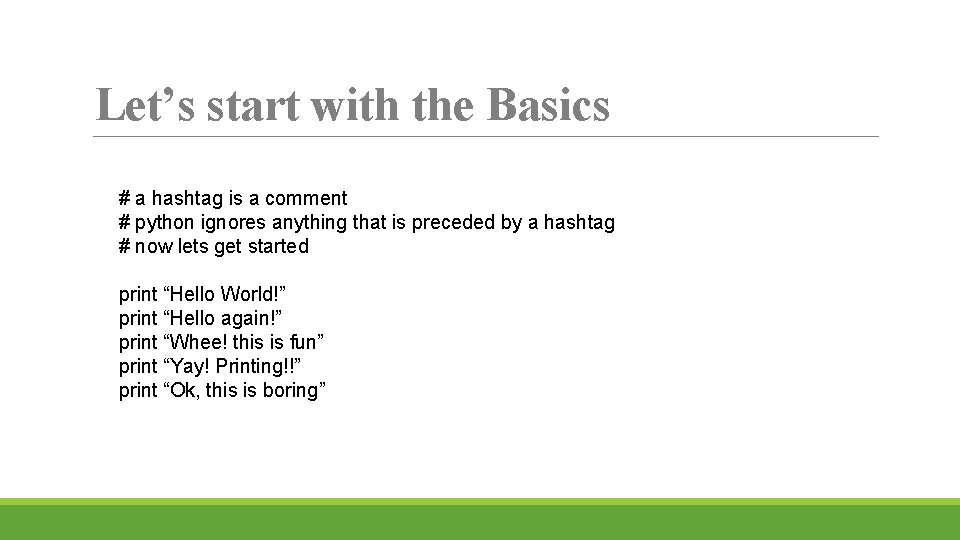
Let’s start with the Basics # a hashtag is a comment # python ignores anything that is preceded by a hashtag # now lets get started print “Hello World!” print “Hello again!” print “Whee! this is fun” print “Yay! Printing!!” print “Ok, this is boring”
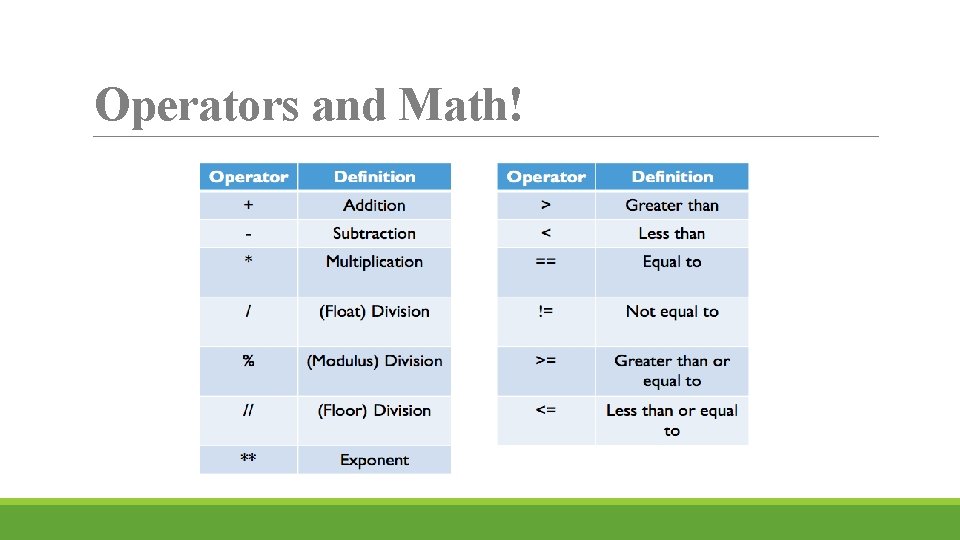
Operators and Math!
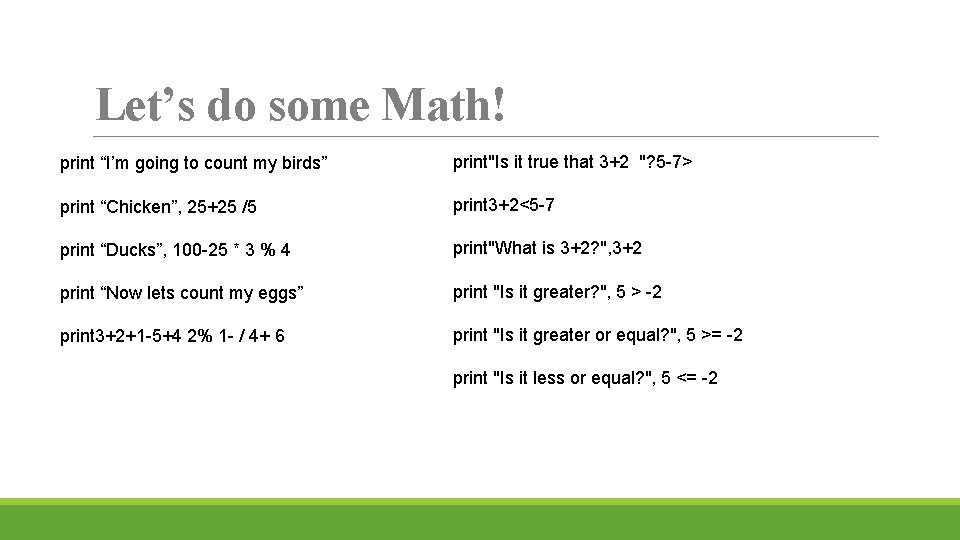
Let’s do some Math! print “I’m going to count my birds” print"Is it true that 3+2 "? 5 -7> print “Chicken”, 25+25 /5 print 3+2<5 -7 print “Ducks”, 100 -25 * 3 % 4 print"What is 3+2? ", 3+2 print “Now lets count my eggs” print "Is it greater? ", 5 > -2 print 3+2+1 -5+4 2% 1 - / 4+ 6 print "Is it greater or equal? ", 5 >= -2 print "Is it less or equal? ", 5 <= -2
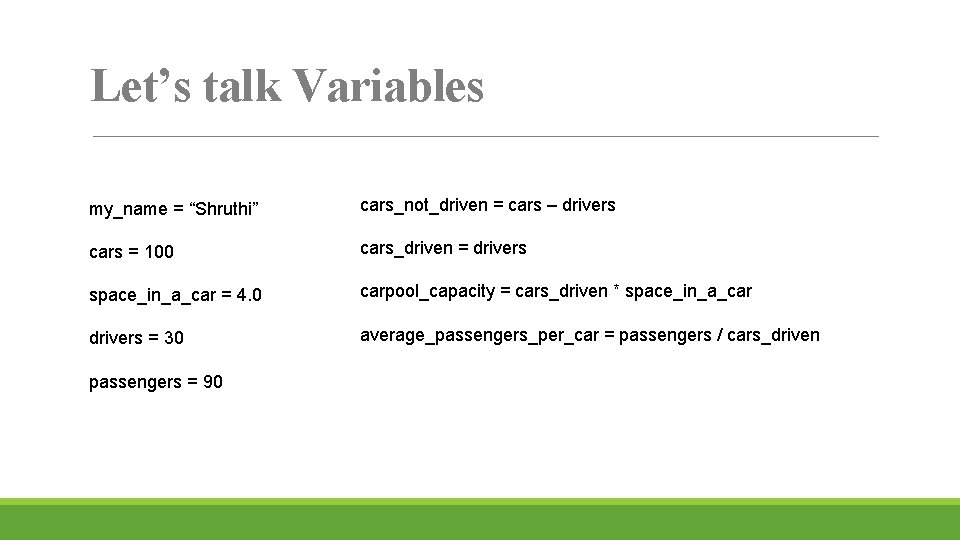
Let’s talk Variables my_name = “Shruthi” cars_not_driven = cars – drivers cars = 100 cars_driven = drivers space_in_a_car = 4. 0 carpool_capacity = cars_driven * space_in_a_car drivers = 30 average_passengers_per_car = passengers / cars_driven passengers = 90
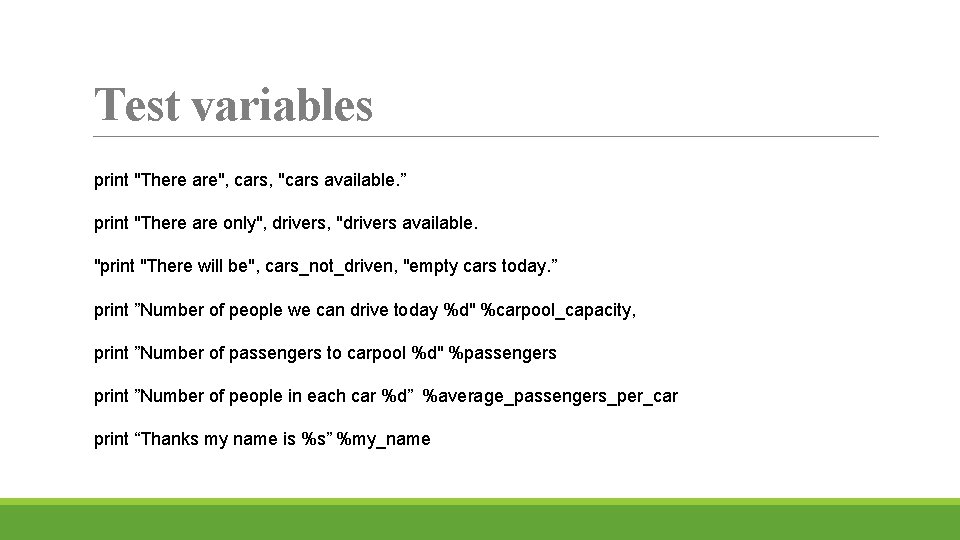
Test variables print "There are", cars, "cars available. ” print "There are only", drivers, "drivers available. "print "There will be", cars_not_driven, "empty cars today. ” print ”Number of people we can drive today %d" %carpool_capacity, print ”Number of passengers to carpool %d" %passengers print ”Number of people in each car %d” %average_passengers_per_car print “Thanks my name is %s” %my_name
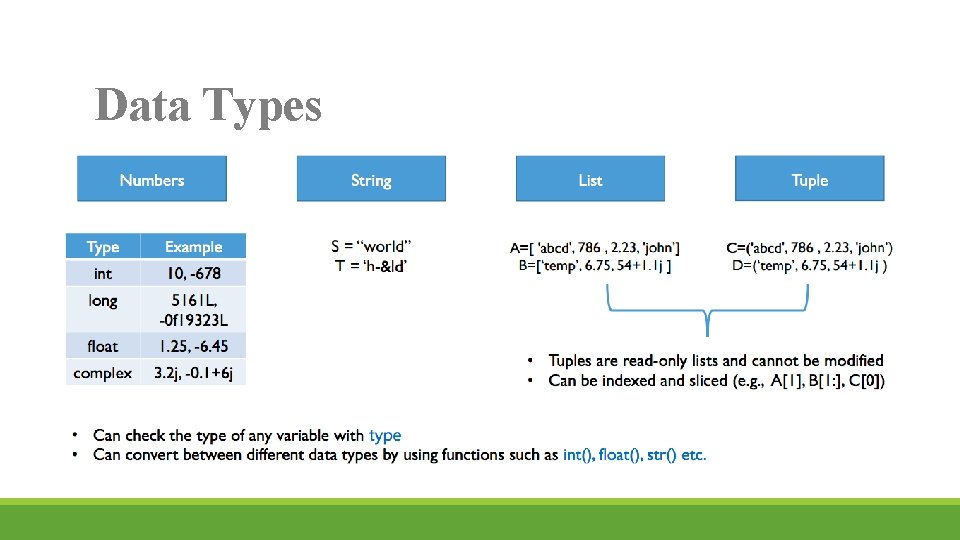
Data Types
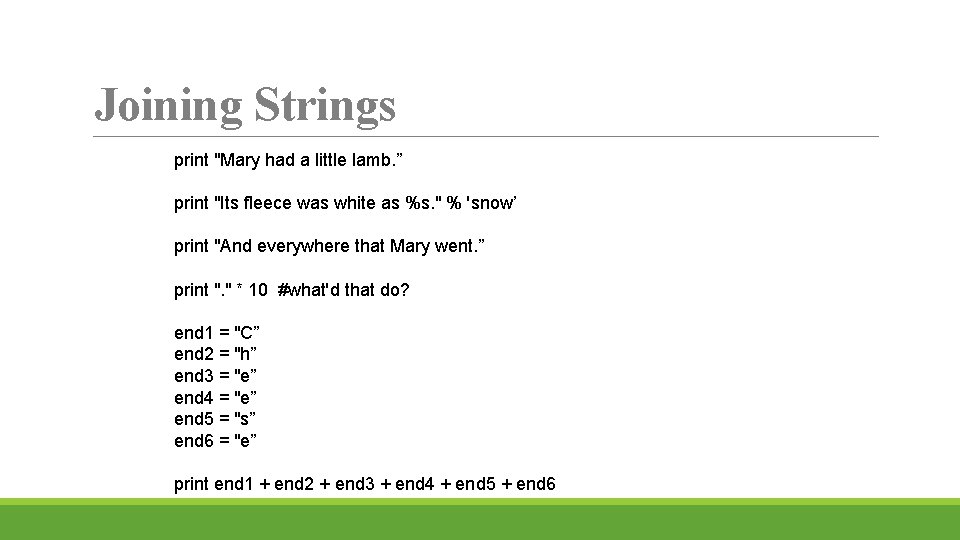
Joining Strings print "Mary had a little lamb. ” print "Its fleece was white as %s. " % 'snow’ print "And everywhere that Mary went. ” print ". " * 10 #what'd that do? end 1 = "C” end 2 = "h” end 3 = "e” end 4 = "e” end 5 = "s” end 6 = "e” print end 1 + end 2 + end 3 + end 4 + end 5 + end 6
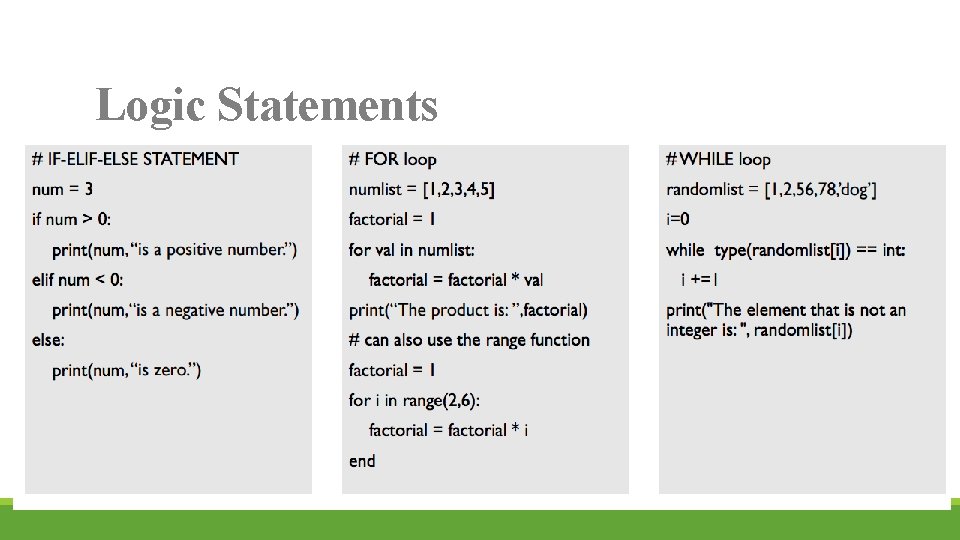
Logic Statements
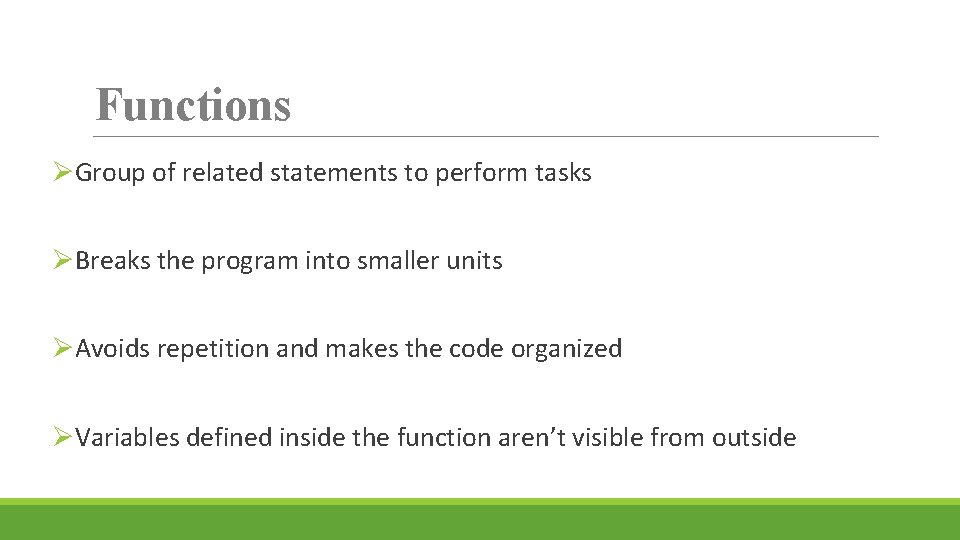
Functions ØGroup of related statements to perform tasks ØBreaks the program into smaller units ØAvoids repetition and makes the code organized ØVariables defined inside the function aren’t visible from outside
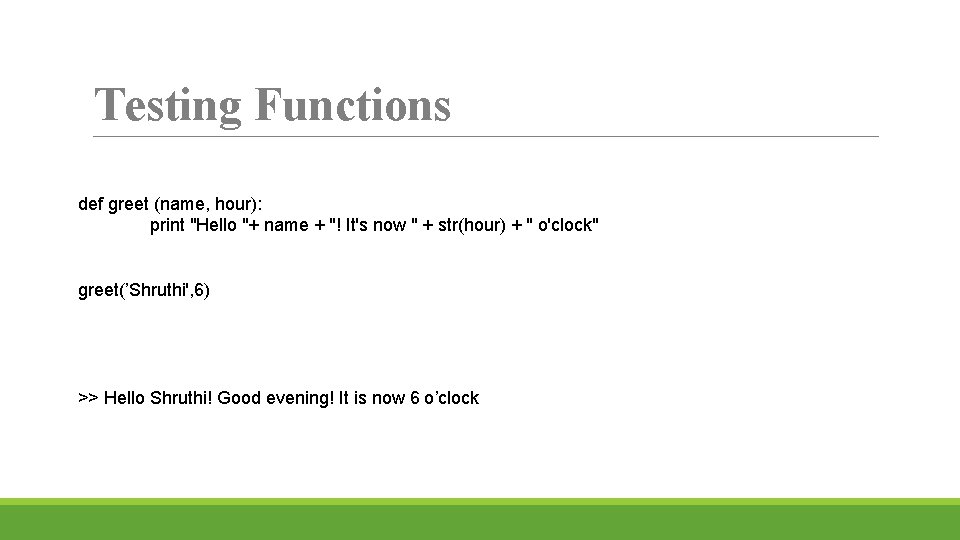
Testing Functions def greet (name, hour): print "Hello "+ name + "! It's now " + str(hour) + " o'clock" greet(’Shruthi', 6) >> Hello Shruthi! Good evening! It is now 6 o’clock
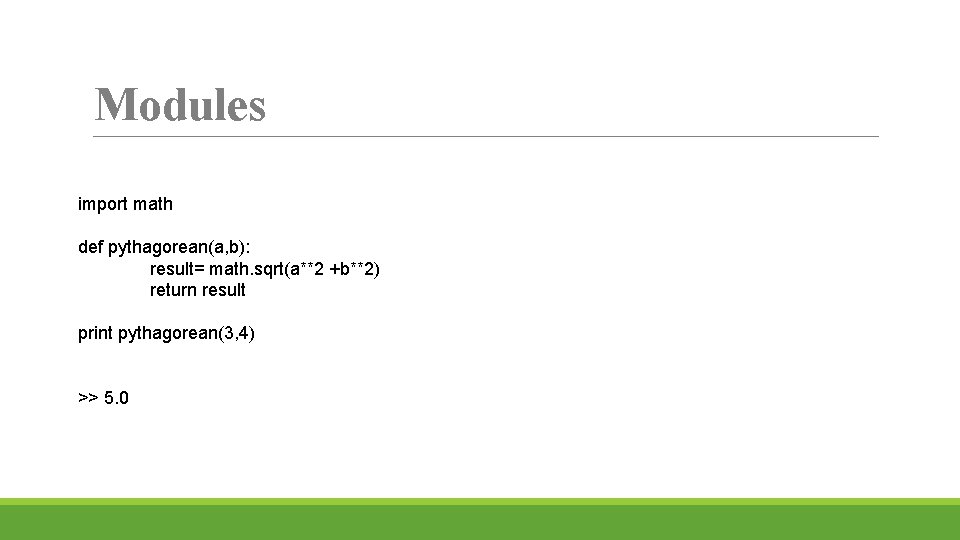
Modules import math def pythagorean(a, b): result= math. sqrt(a**2 +b**2) return result print pythagorean(3, 4) >> 5. 0
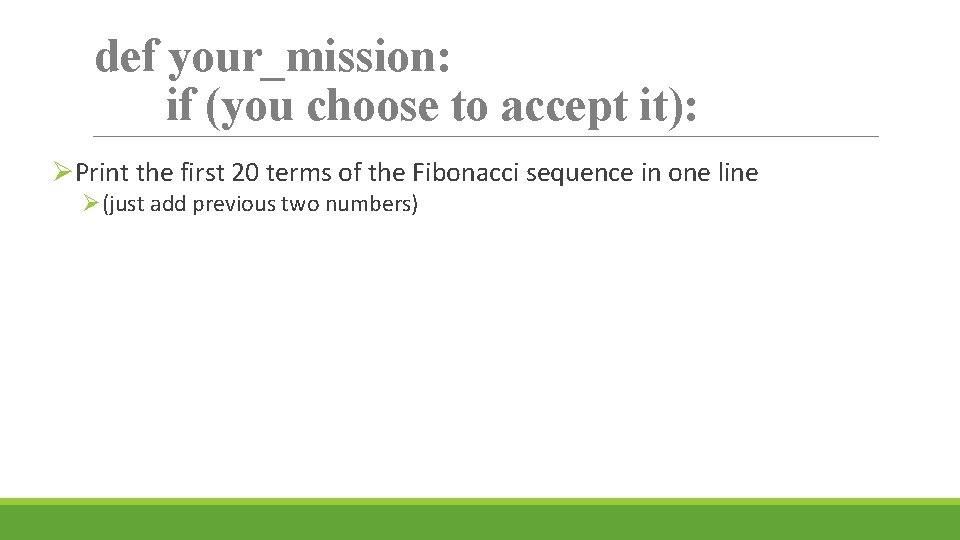
def your_mission: if (you choose to accept it): ØPrint the first 20 terms of the Fibonacci sequence in one line Ø(just add previous two numbers)
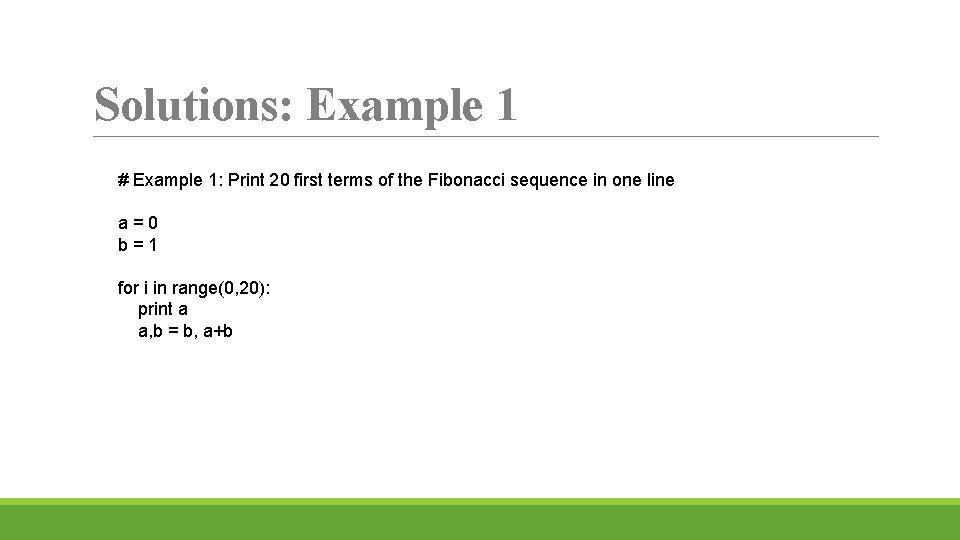
Solutions: Example 1 # Example 1: Print 20 first terms of the Fibonacci sequence in one line a=0 b=1 for i in range(0, 20): print a a, b = b, a+b
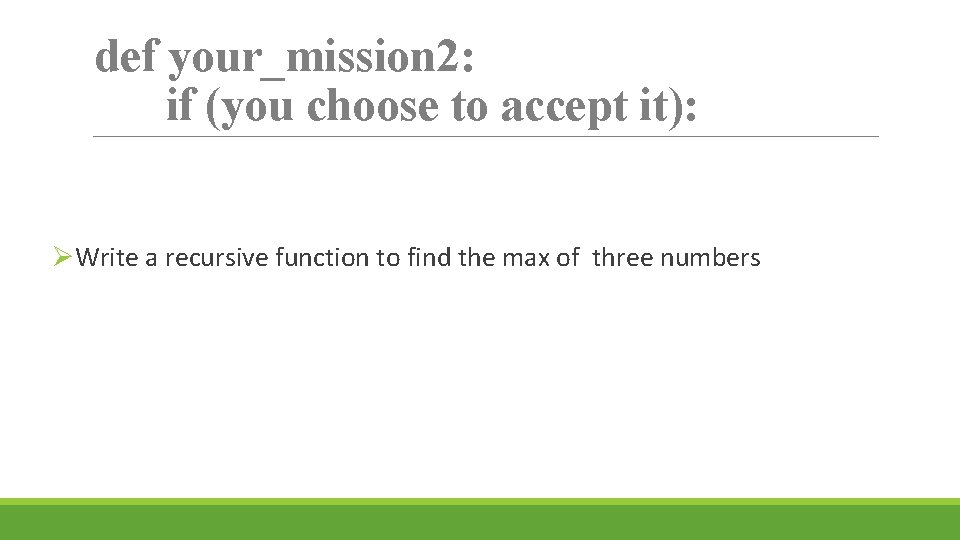
def your_mission 2: if (you choose to accept it): ØWrite a recursive function to find the max of three numbers
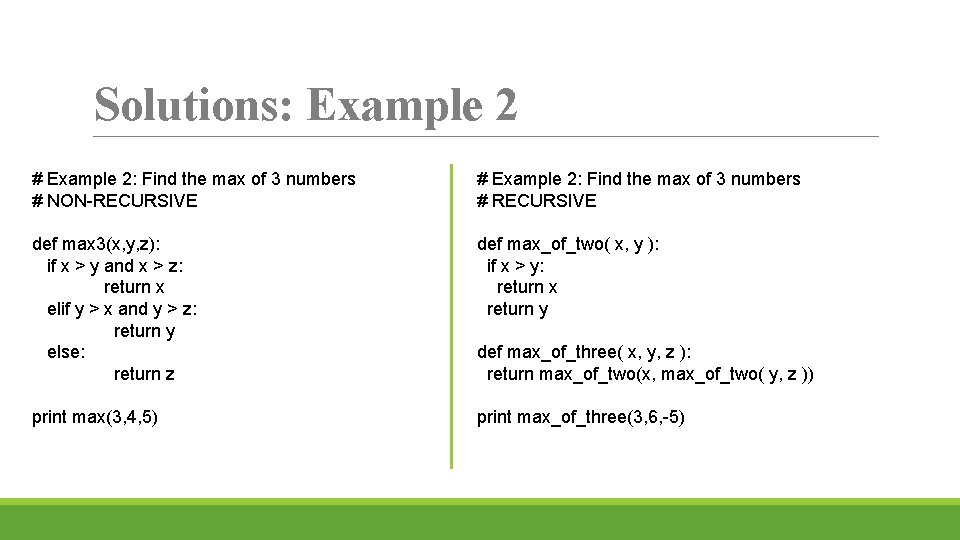
Solutions: Example 2 # Example 2: Find the max of 3 numbers # NON-RECURSIVE # Example 2: Find the max of 3 numbers # RECURSIVE def max 3(x, y, z): if x > y and x > z: return x elif y > x and y > z: return y else: return z def max_of_two( x, y ): if x > y: return x return y print max(3, 4, 5) print max_of_three(3, 6, -5) def max_of_three( x, y, z ): return max_of_two(x, max_of_two( y, z ))
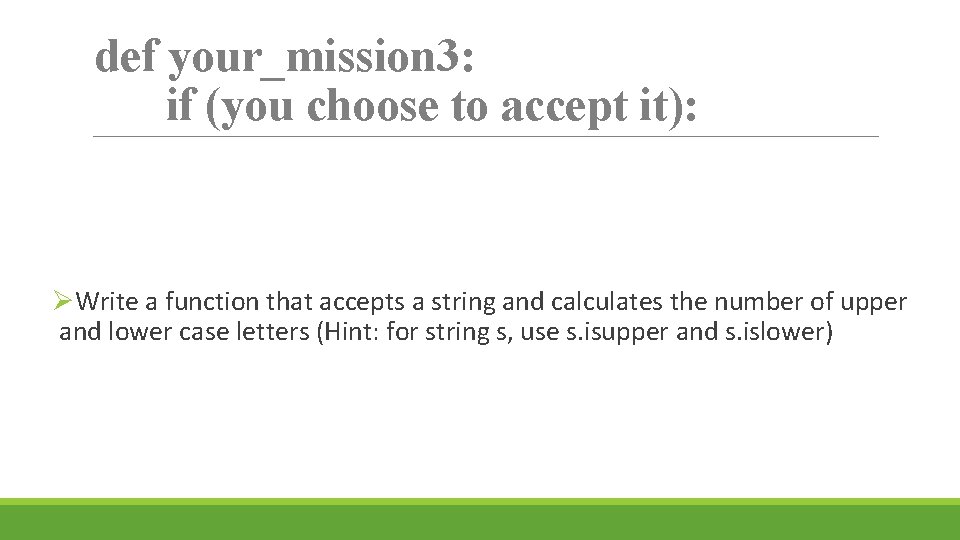
def your_mission 3: if (you choose to accept it): ØWrite a function that accepts a string and calculates the number of upper and lower case letters (Hint: for string s, use s. isupper and s. islower)
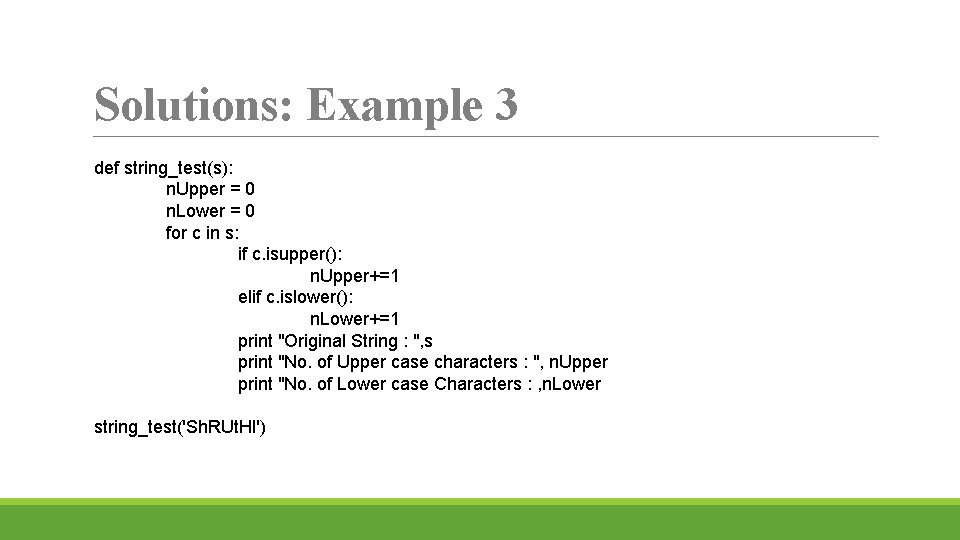
Solutions: Example 3 def string_test(s): n. Upper = 0 n. Lower = 0 for c in s: if c. isupper(): n. Upper+=1 elif c. islower(): n. Lower+=1 print "Original String : ", s print "No. of Upper case characters : ", n. Upper print "No. of Lower case Characters : , n. Lower string_test('Sh. RUt. HI')
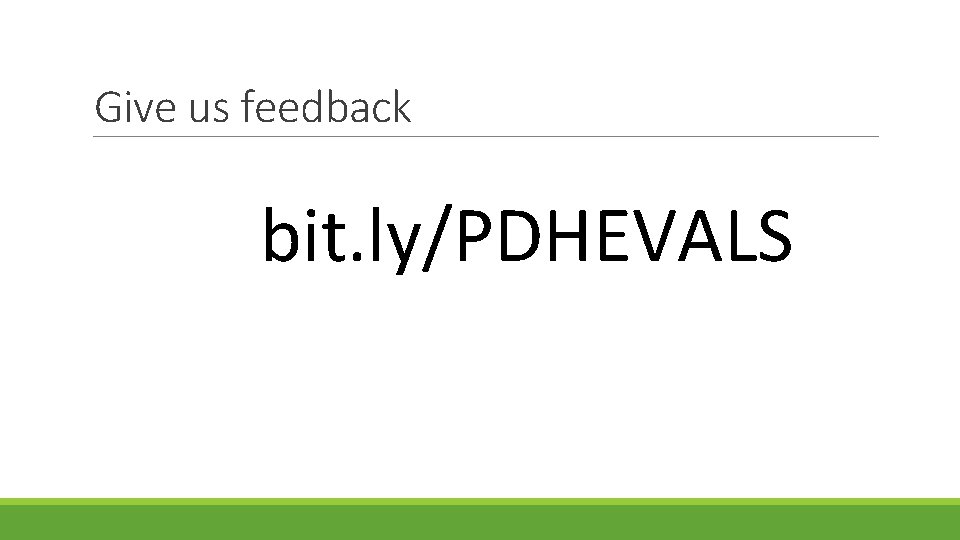
Give us feedback bit. ly/PDHEVALS
Soft skills
Css session
An exercise session has three stages
Practical session definition
Meet the parents session
Prayer for planning session
Balabit vs cyberark
Listening sessions for employees
Session title examples
Cake session
Handshake smu
First cbt session structure
Nexthink user session monitoring
Poster imrad
Brown paper mapping
M&a bo session
Gate candidate site
Define social change
Test session
Coaching session agenda
Application presentation session transport network