Prklad 1 Navrhnite truktru odvodench tried od zkladnej
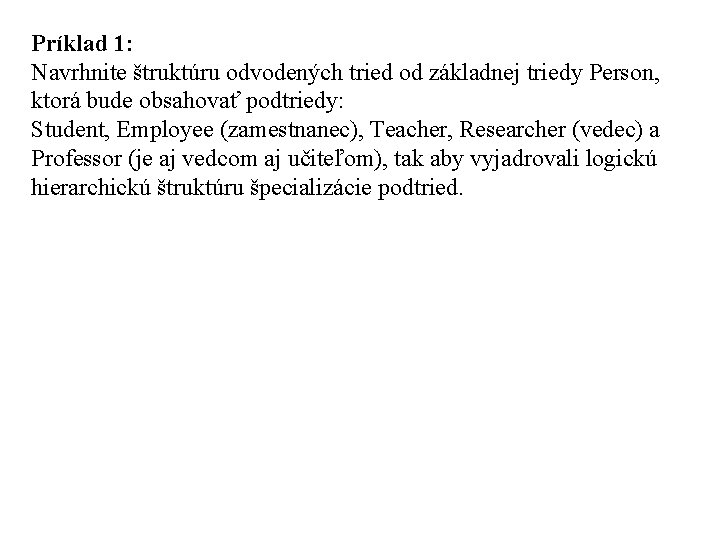
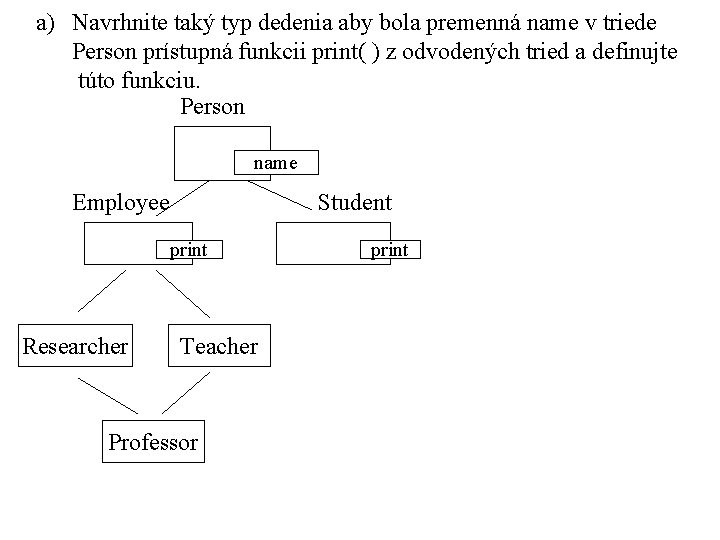
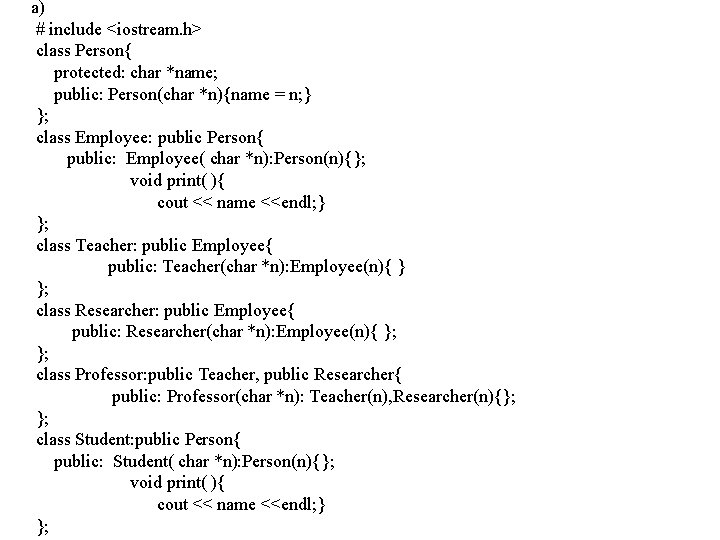
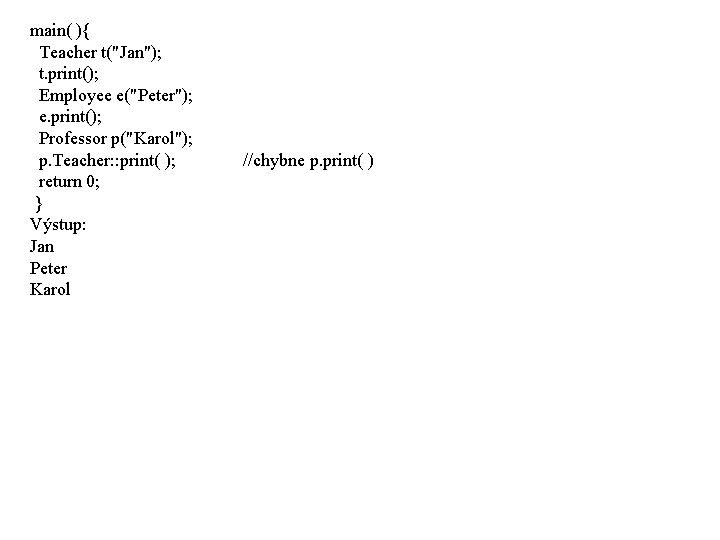
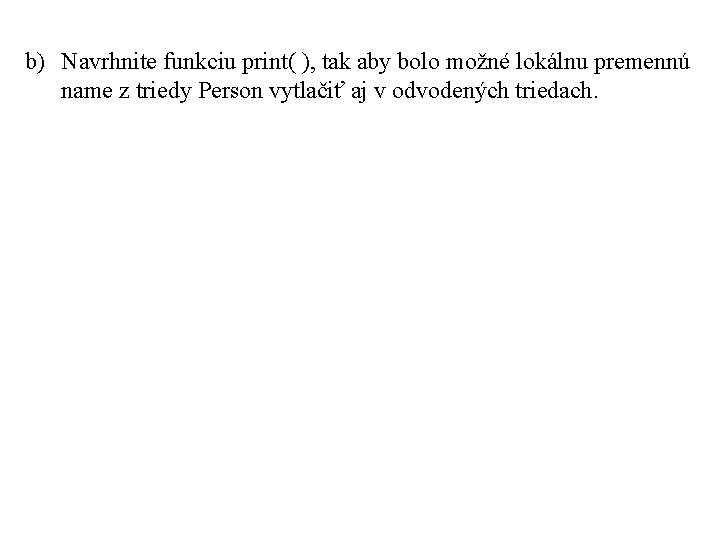
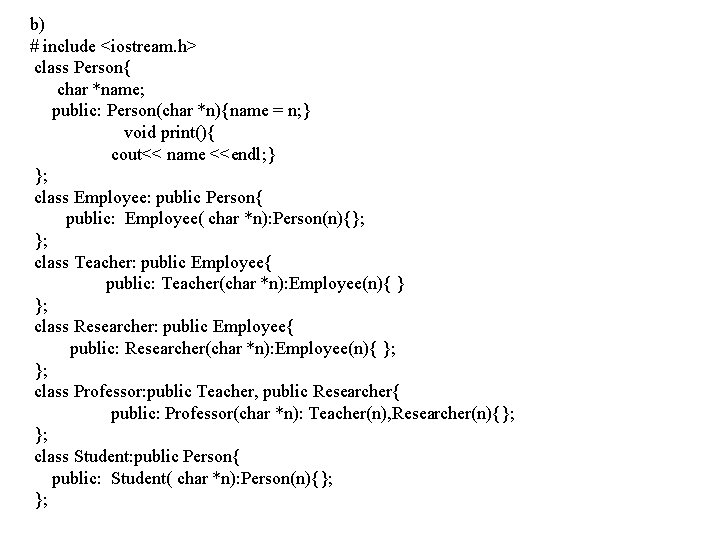
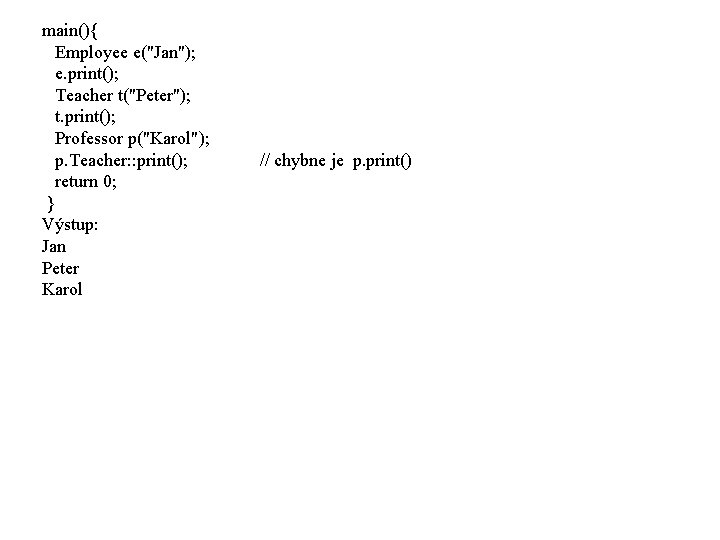
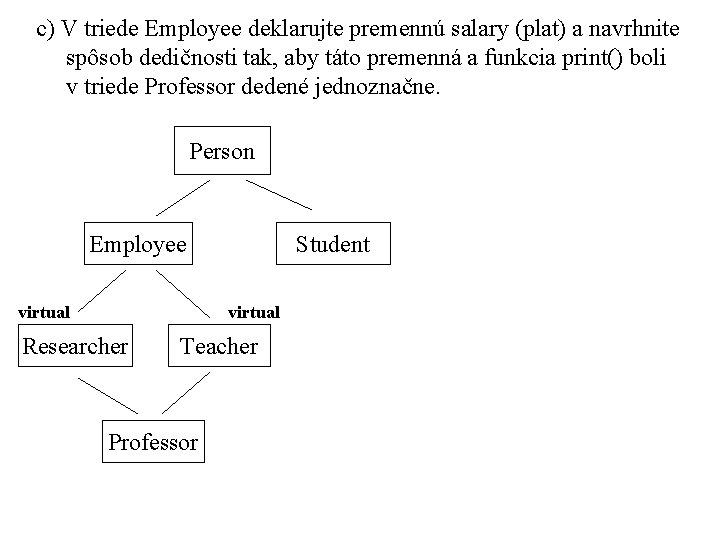
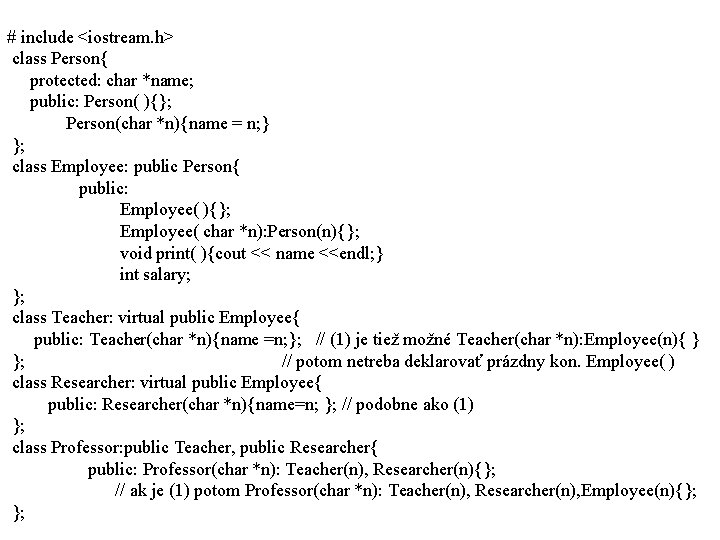
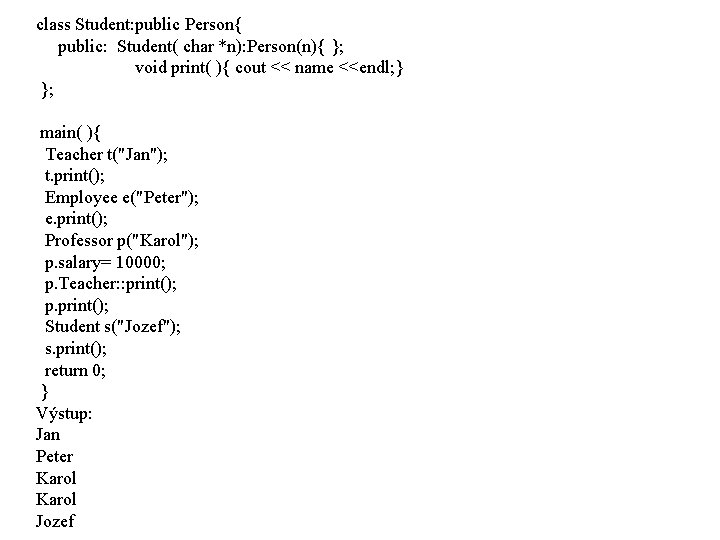
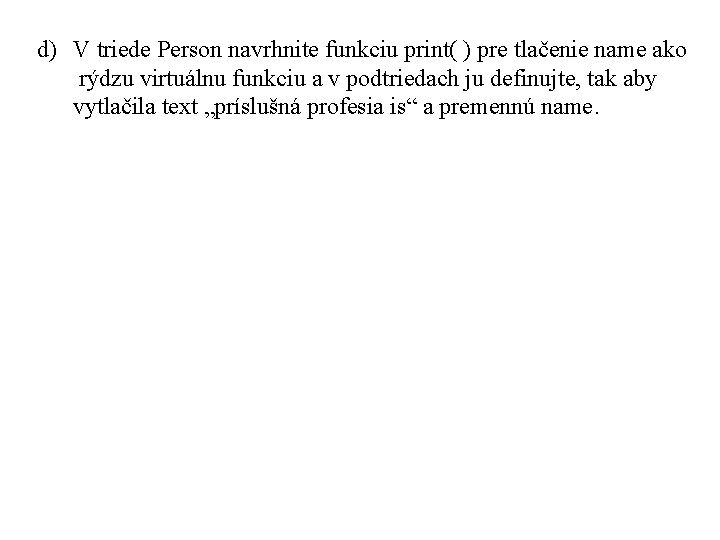
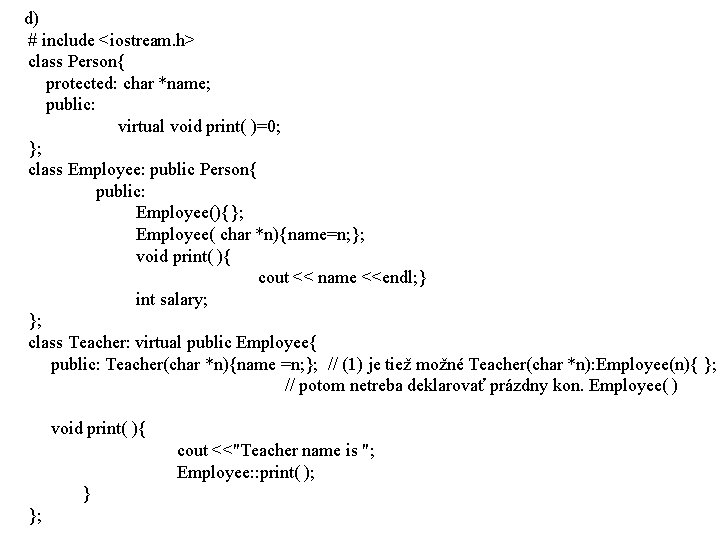
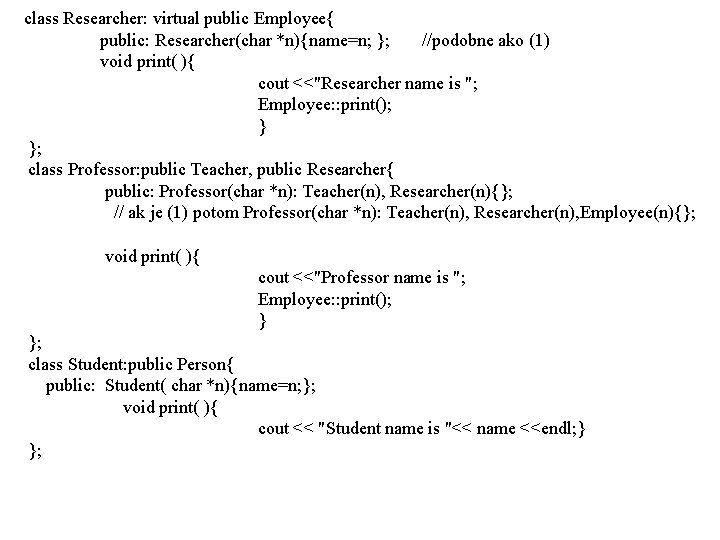
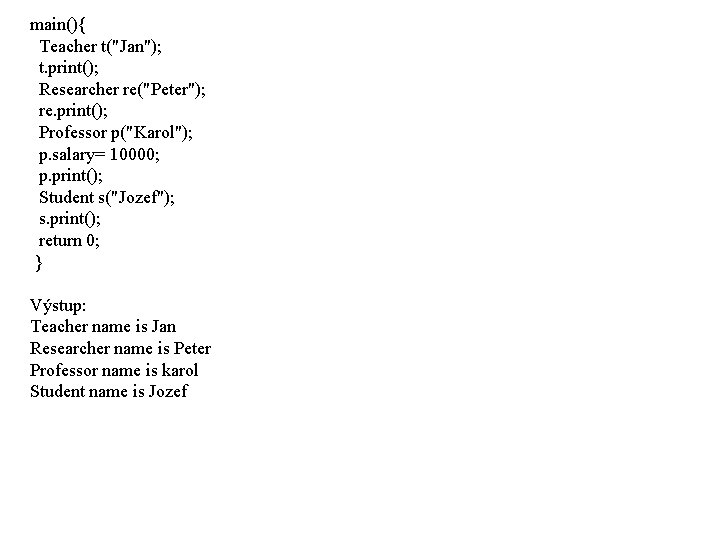
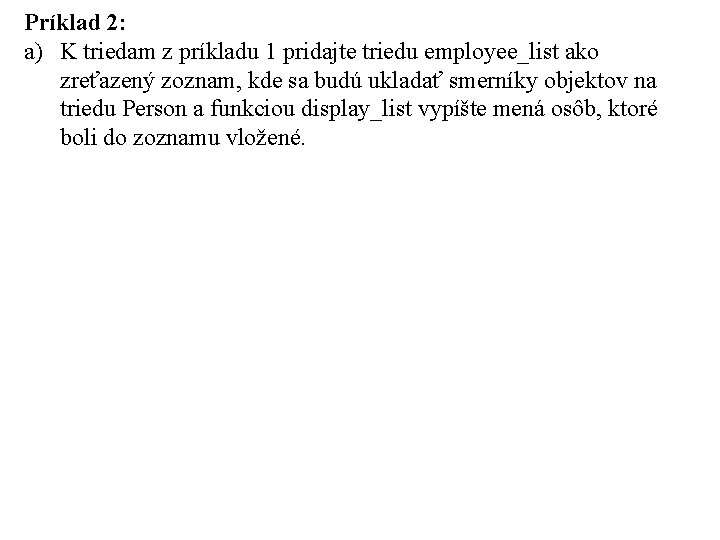
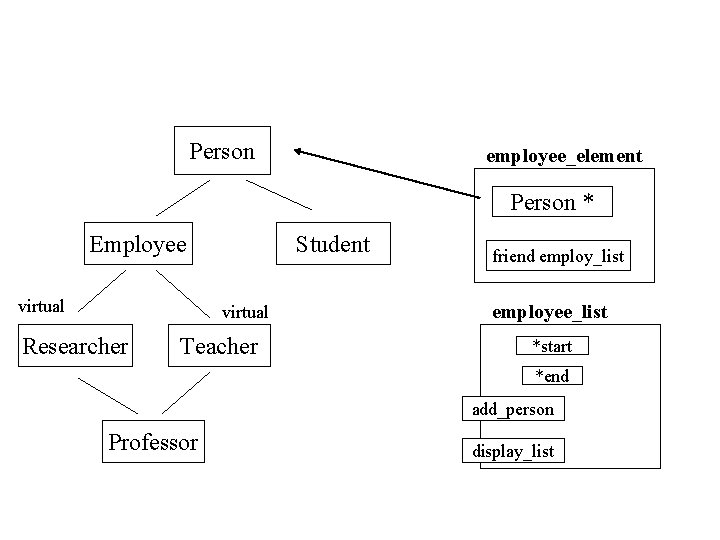
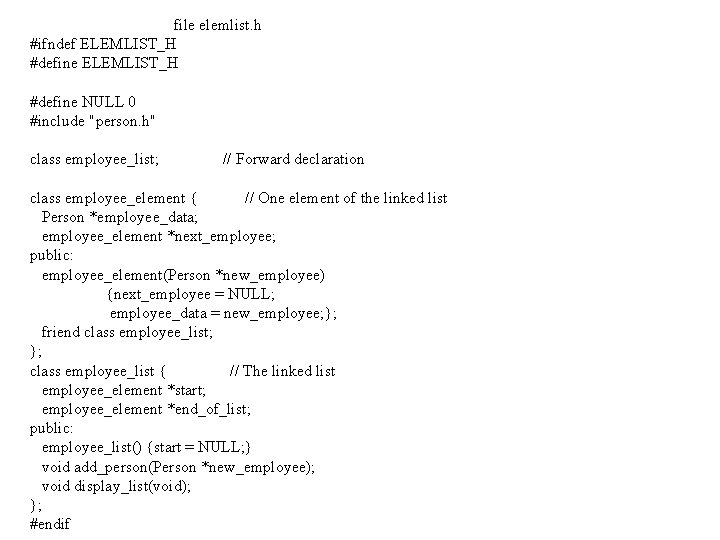
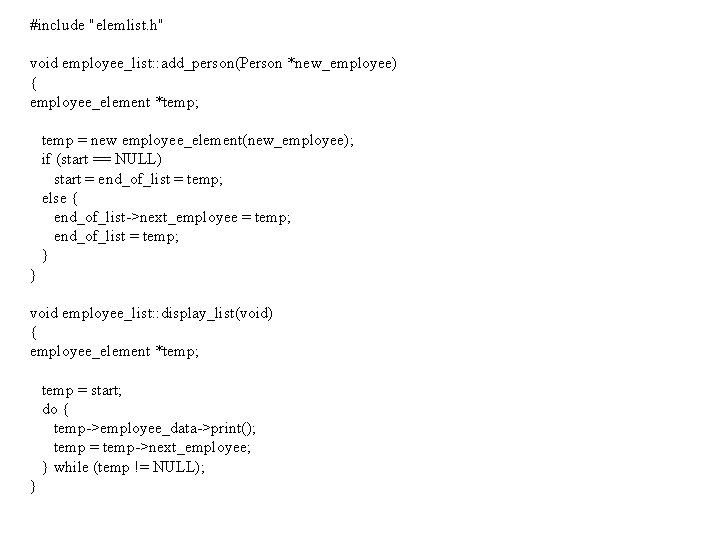
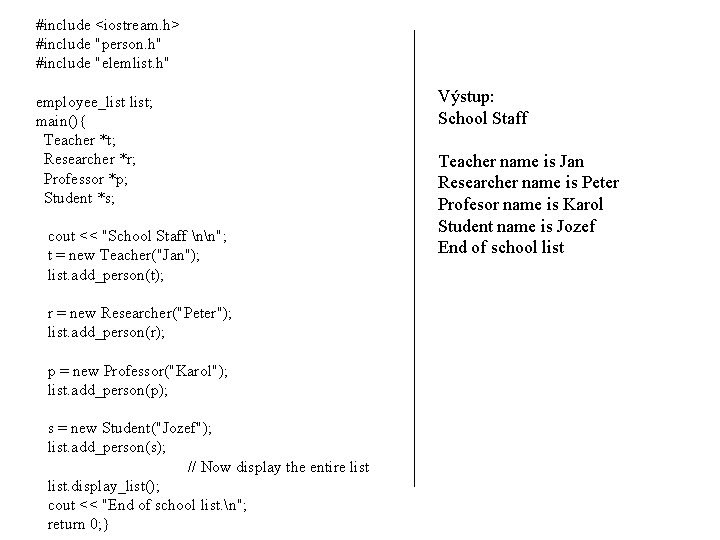
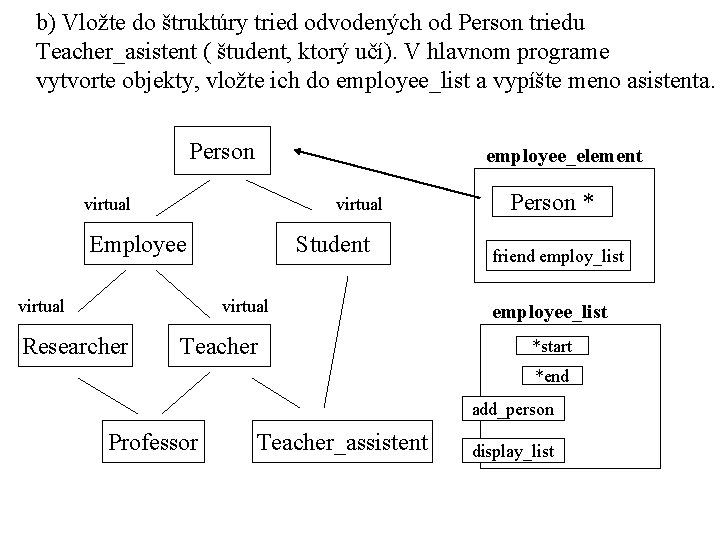
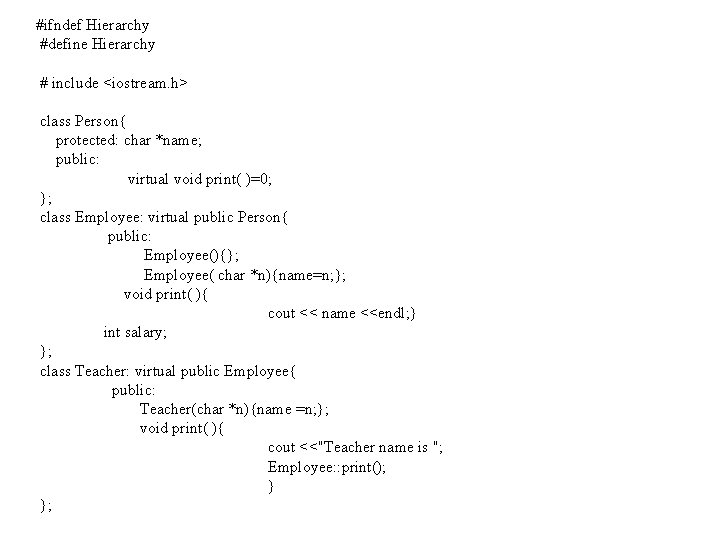
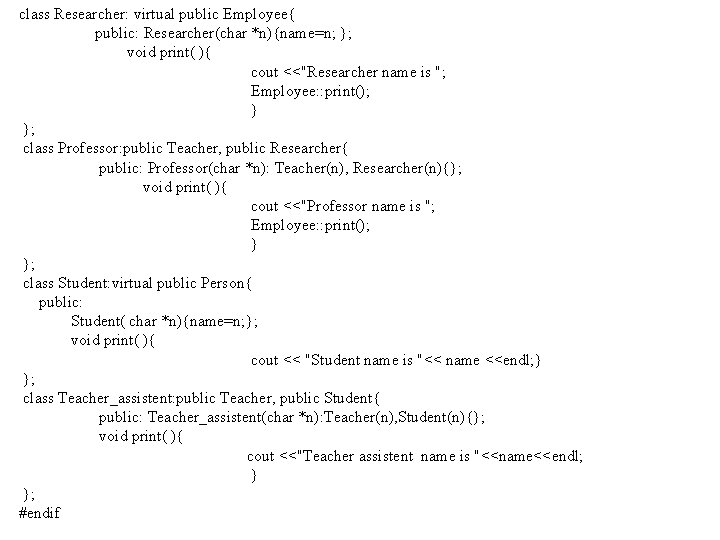
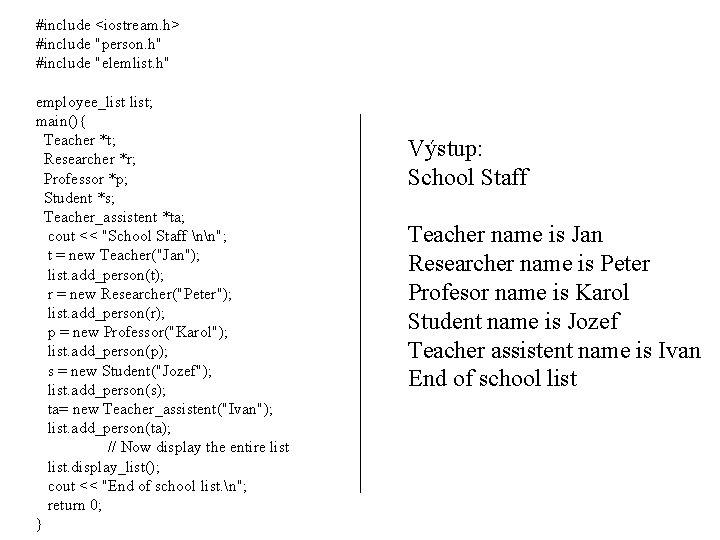
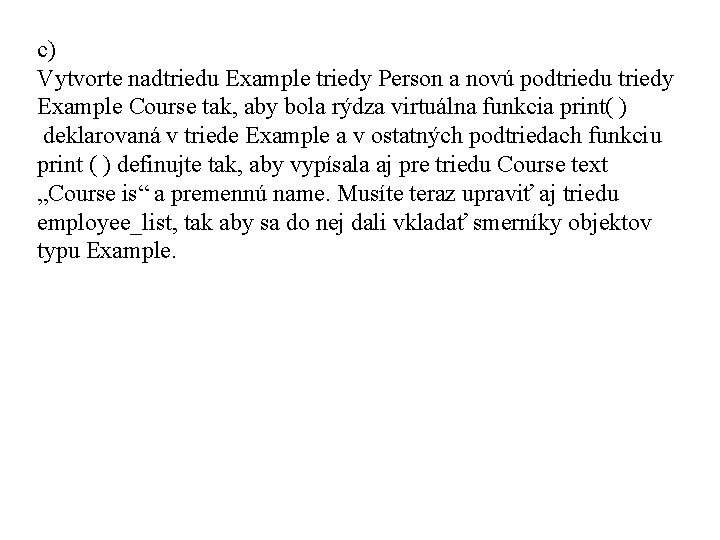
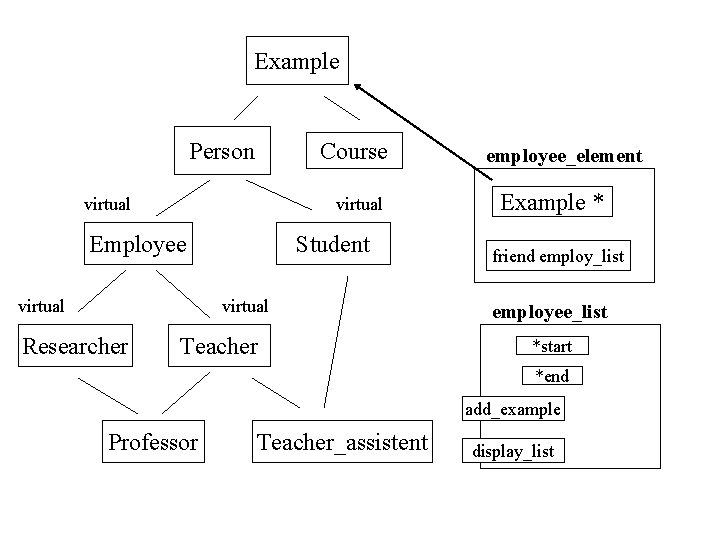
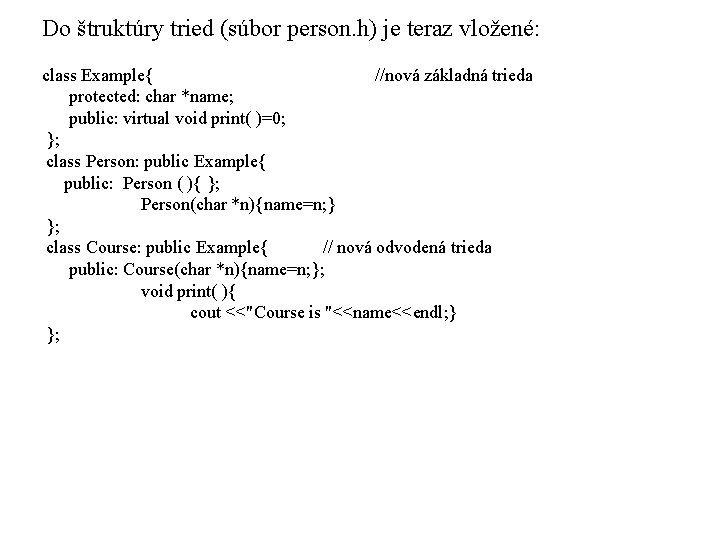
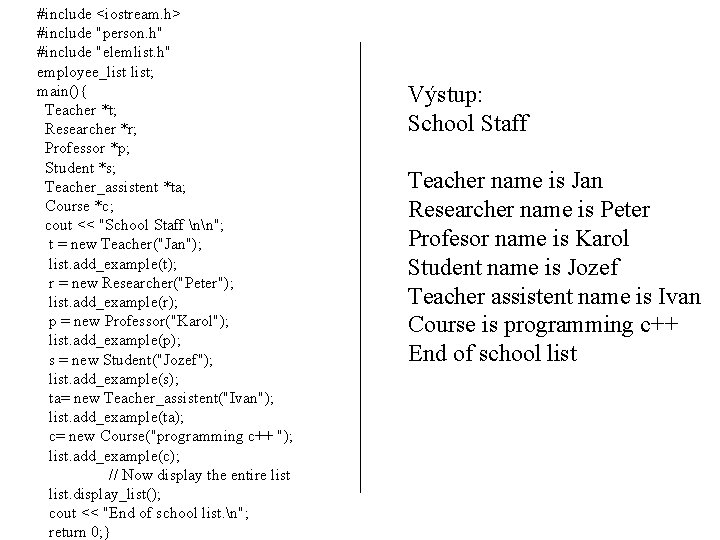
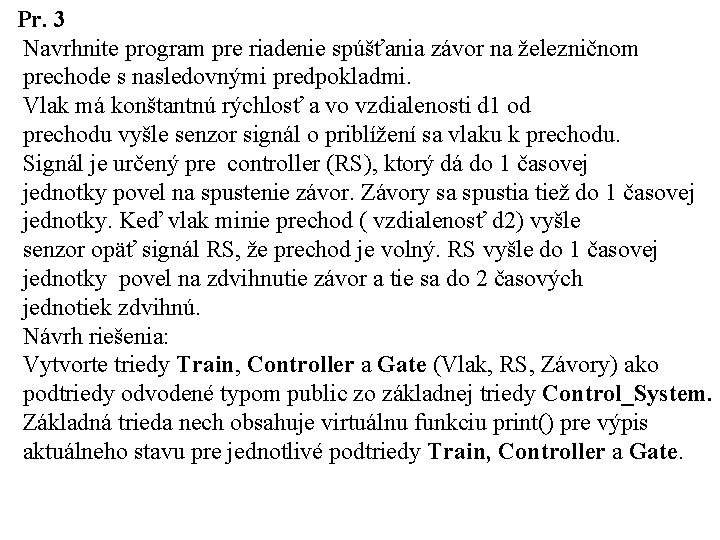
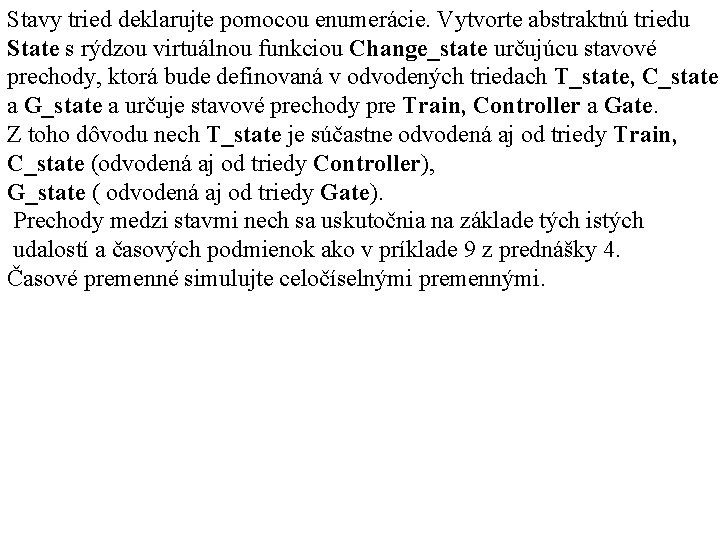
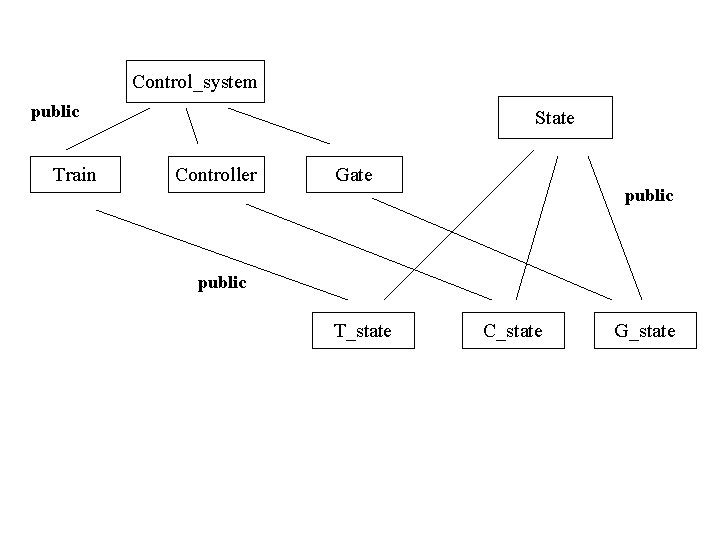
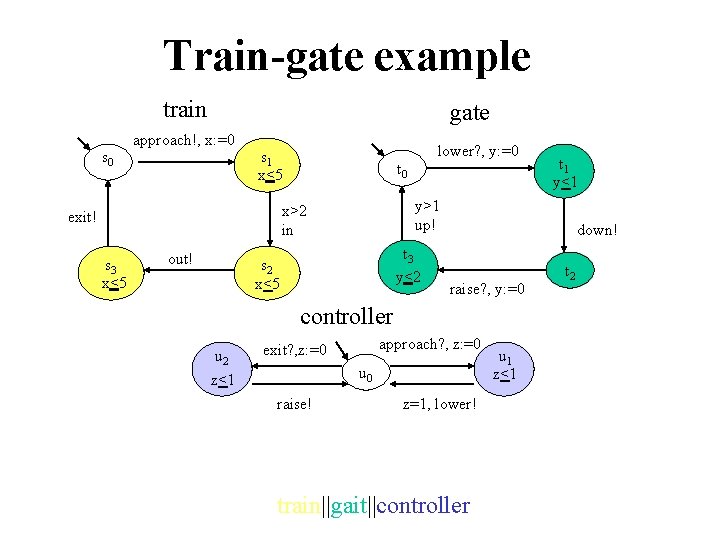
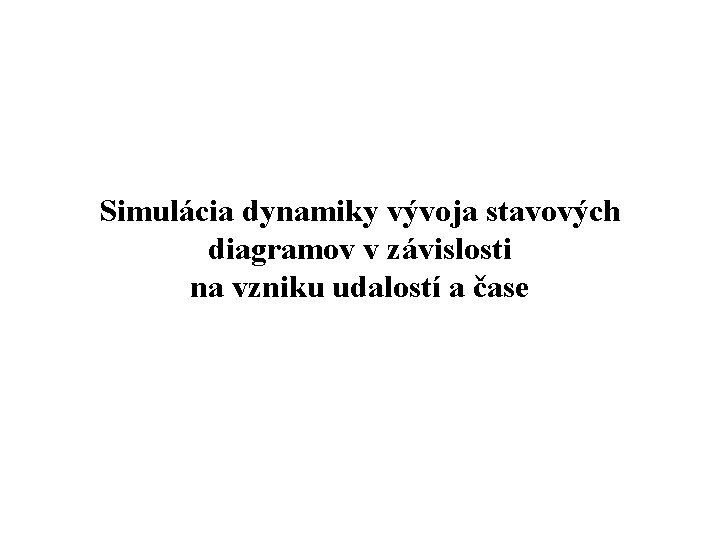
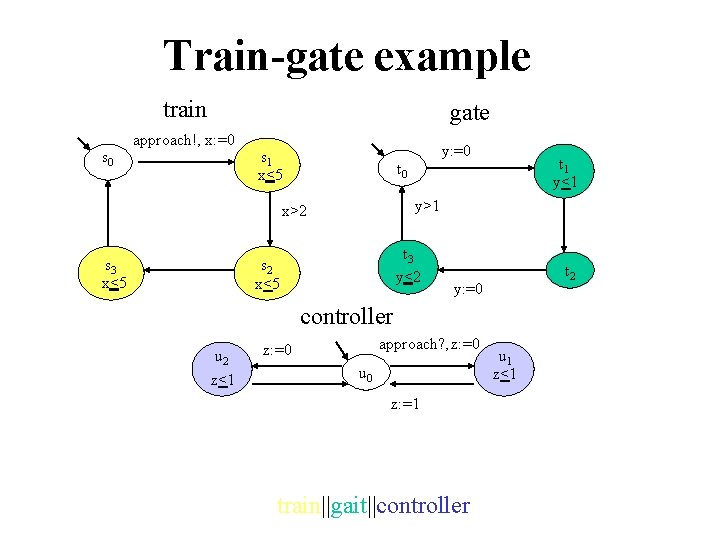
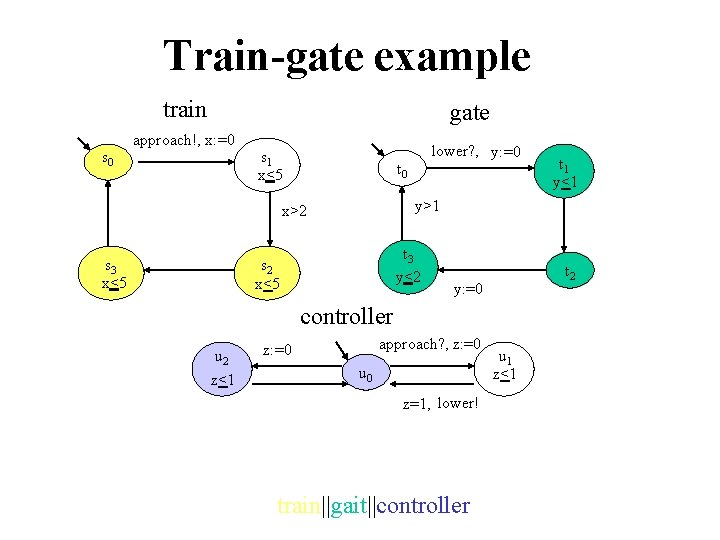
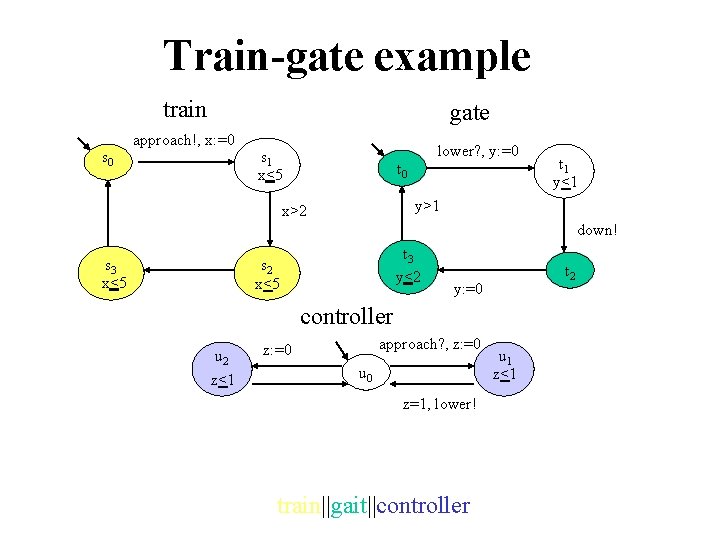
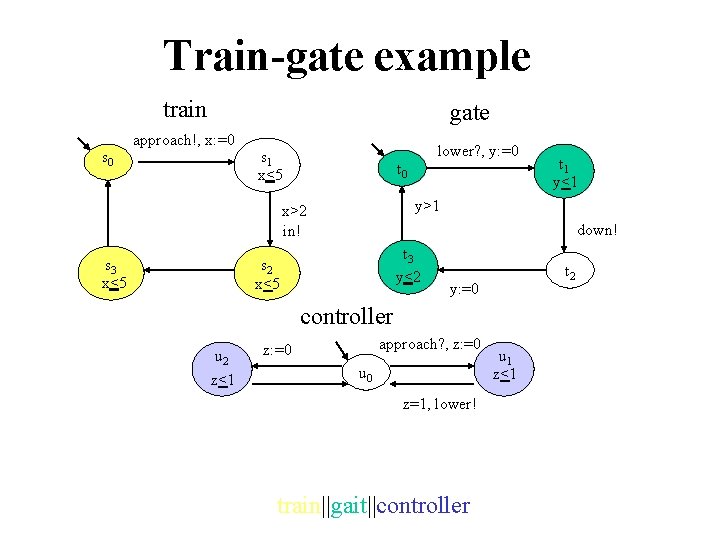
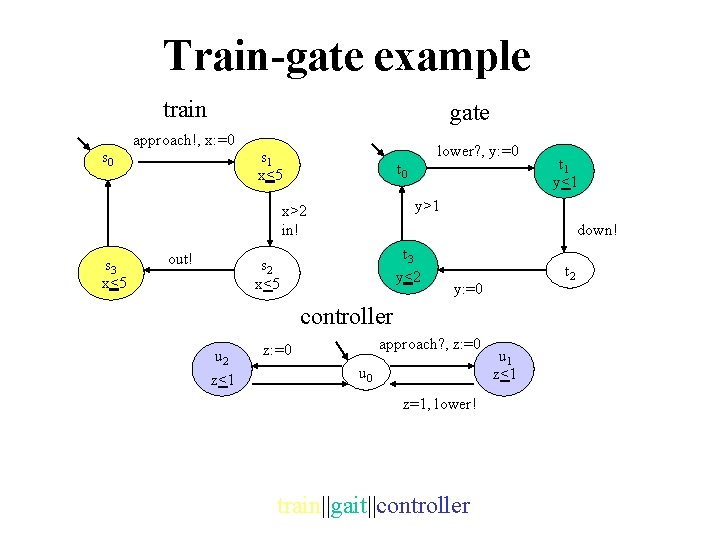
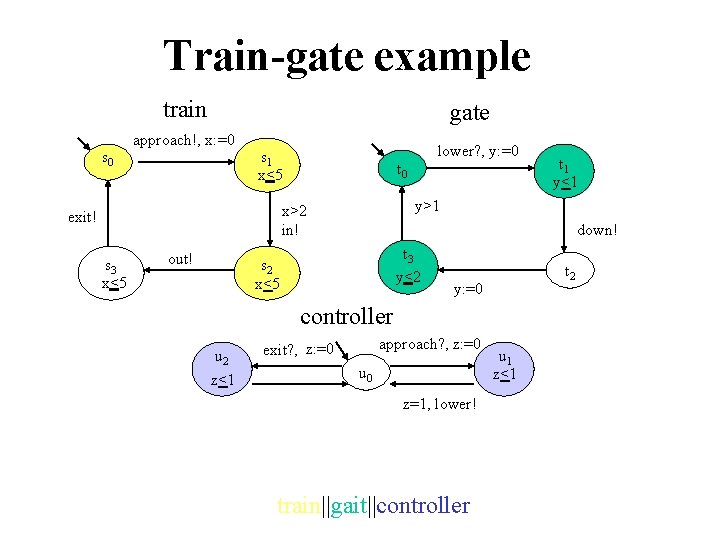
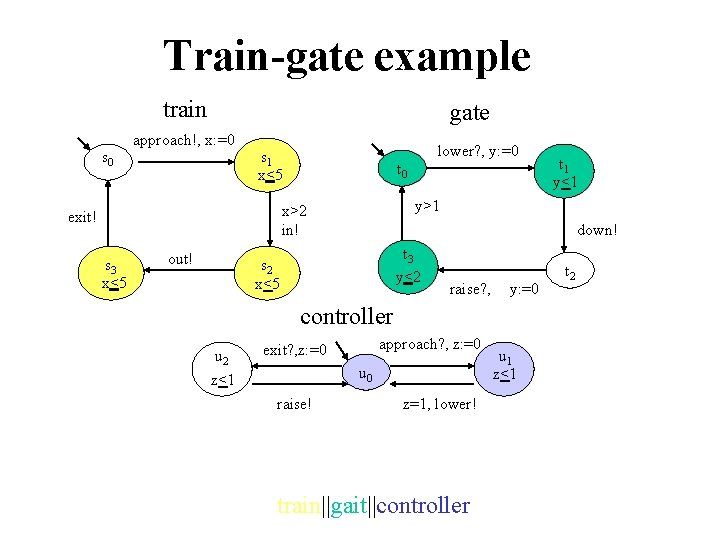
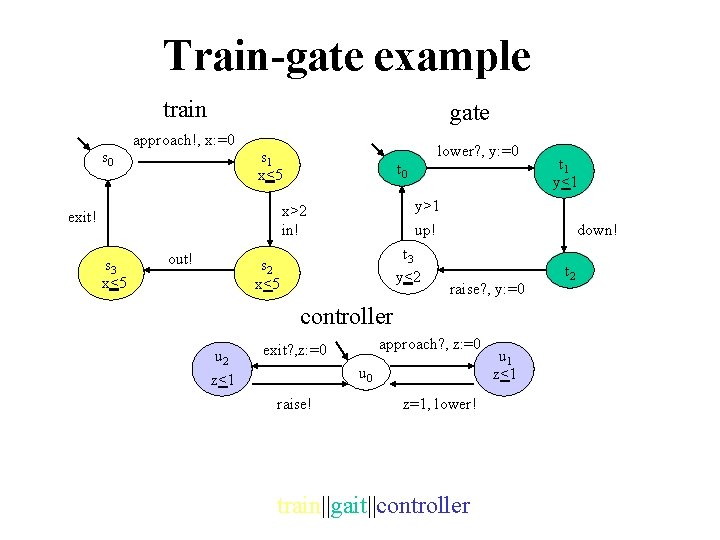
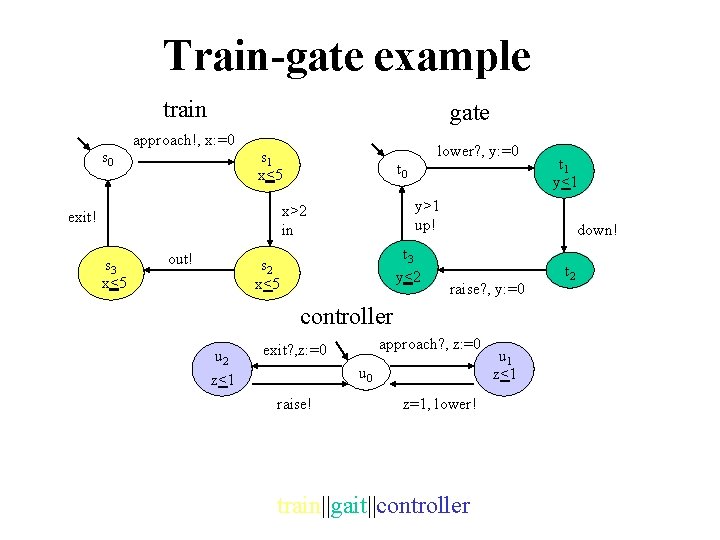
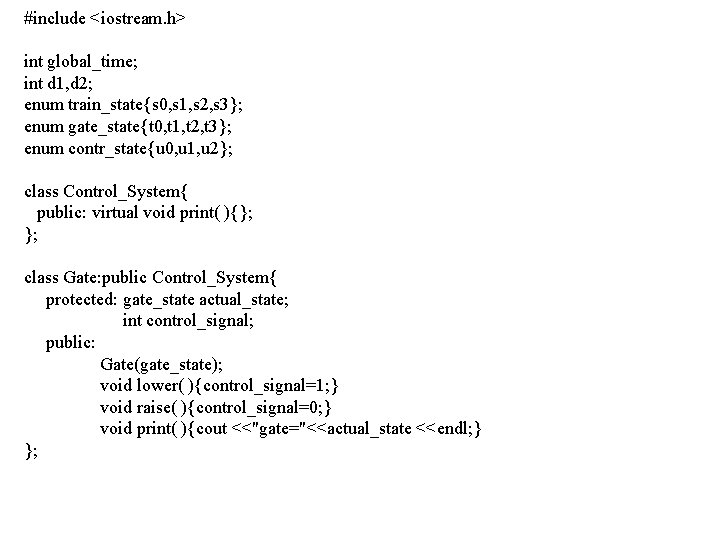
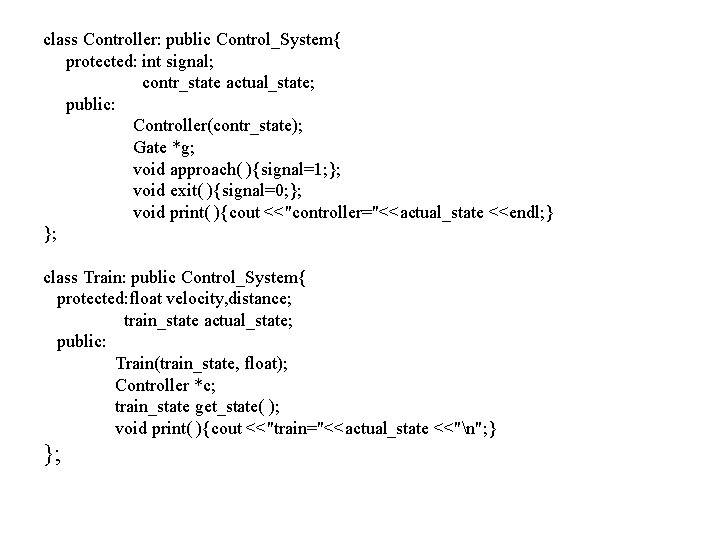
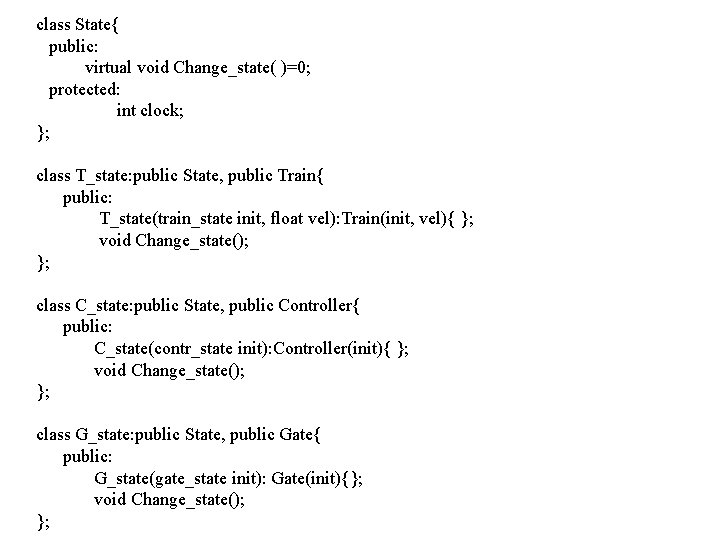
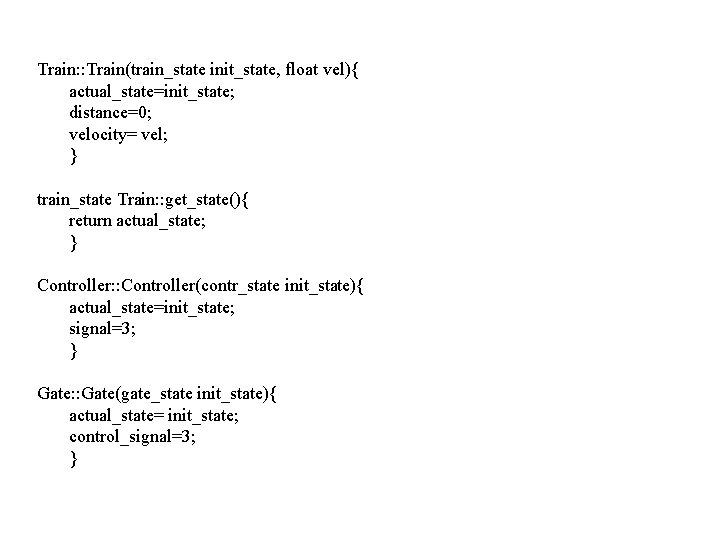
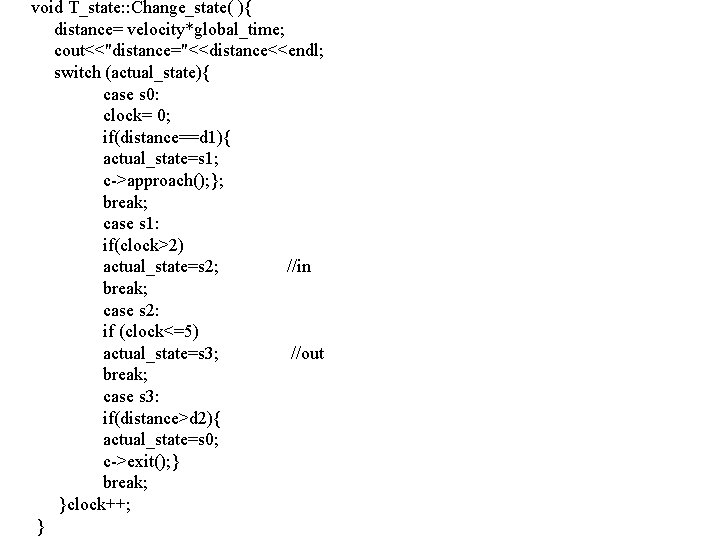
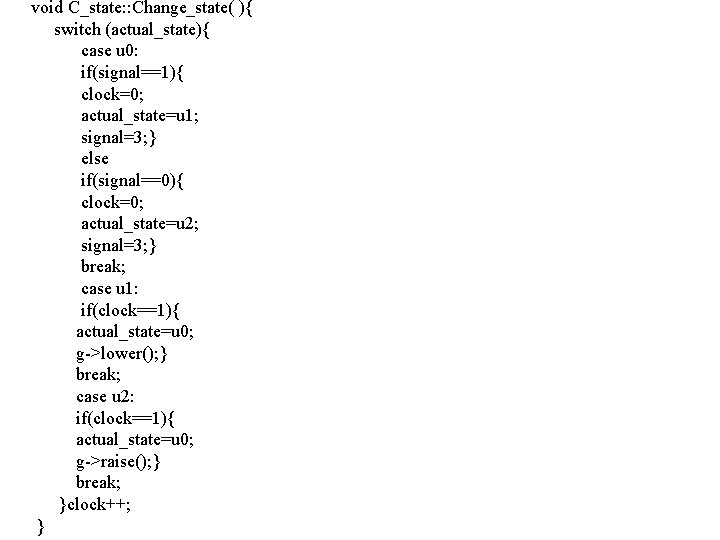
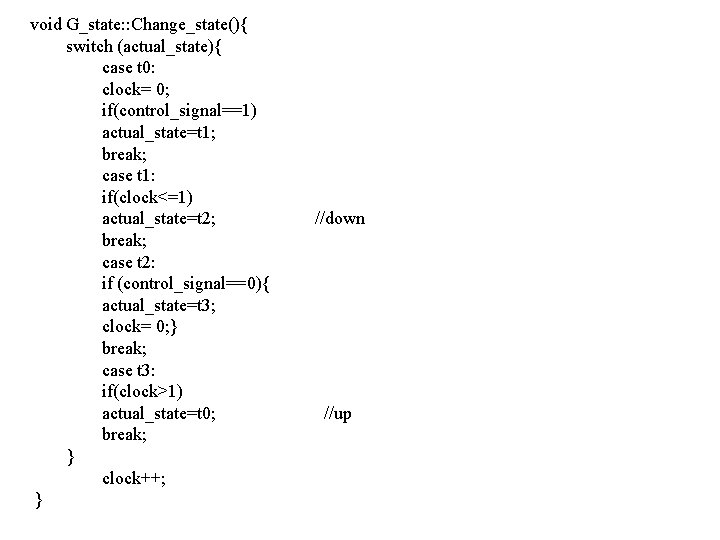
![Control_System *array 1[3]; State *array 2[3]; main(){ d 1=200; d 2=700; global_time=0; T_state *trs; Control_System *array 1[3]; State *array 2[3]; main(){ d 1=200; d 2=700; global_time=0; T_state *trs;](https://slidetodoc.com/presentation_image_h2/ca09cbb8f11293655f9a424fc2f8ebb2/image-49.jpg)
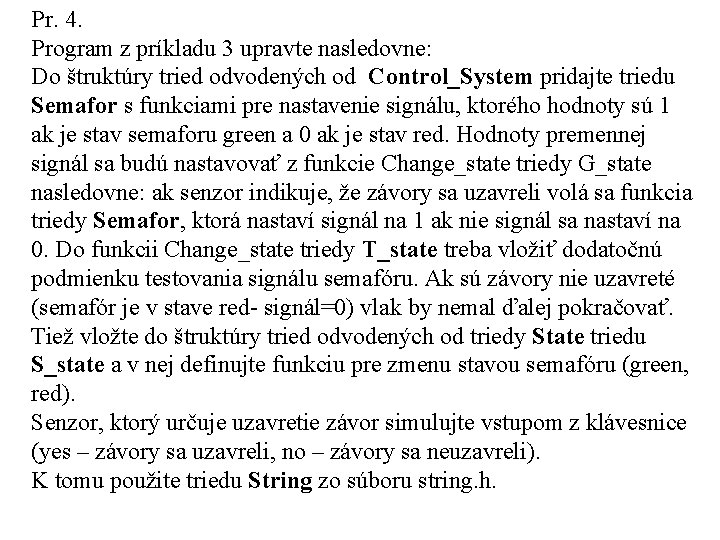
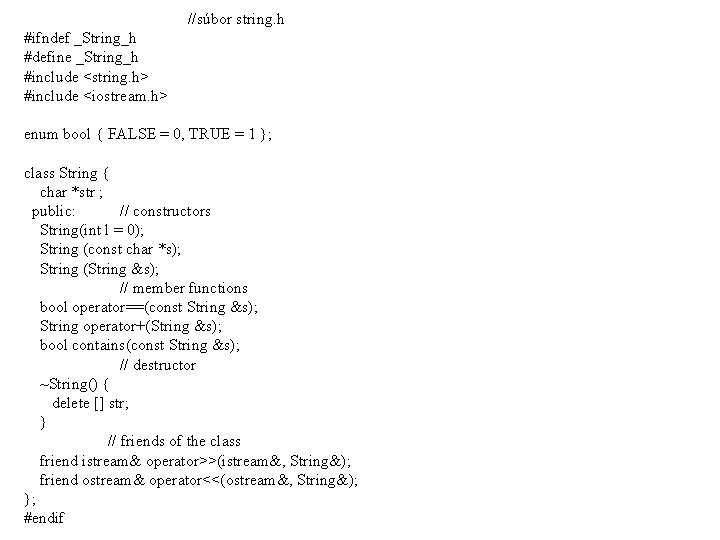
![#include "string. h" String: : String(int l ){ str = new char[l]; } String: #include "string. h" String: : String(int l ){ str = new char[l]; } String:](https://slidetodoc.com/presentation_image_h2/ca09cbb8f11293655f9a424fc2f8ebb2/image-52.jpg)
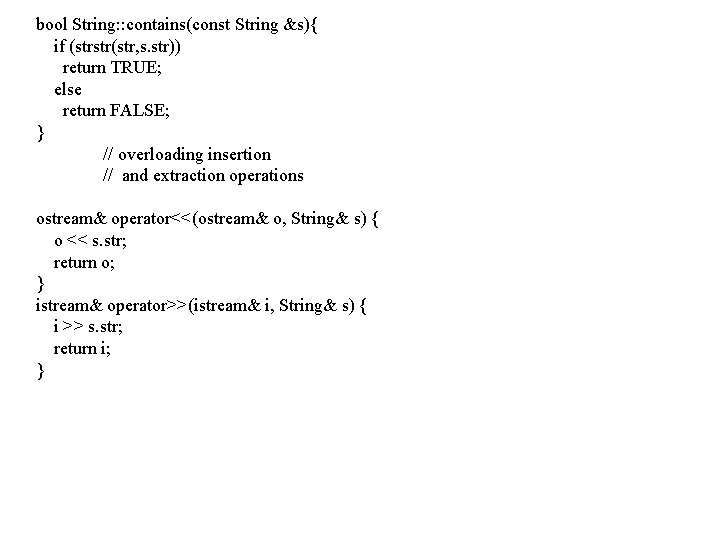
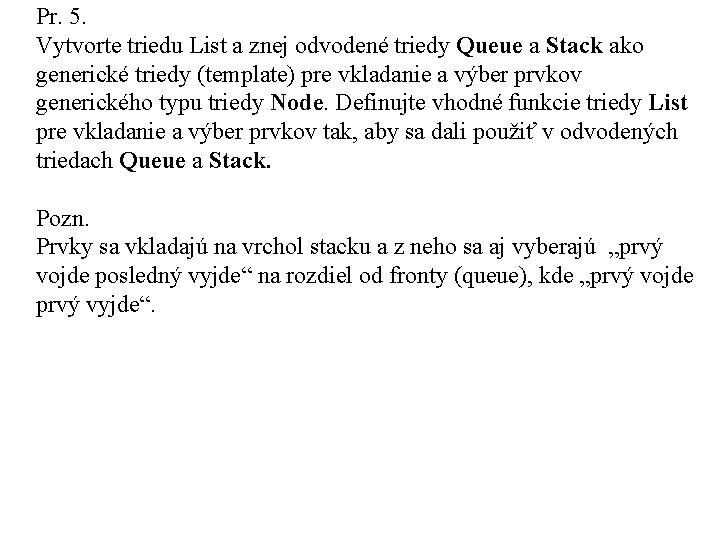
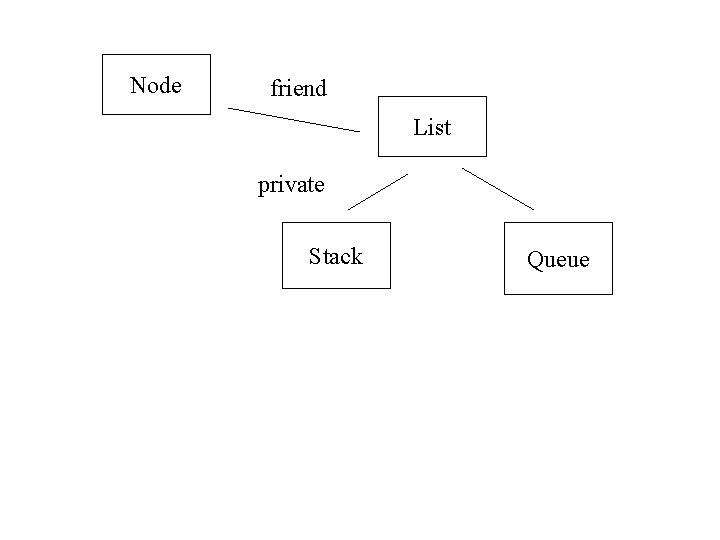
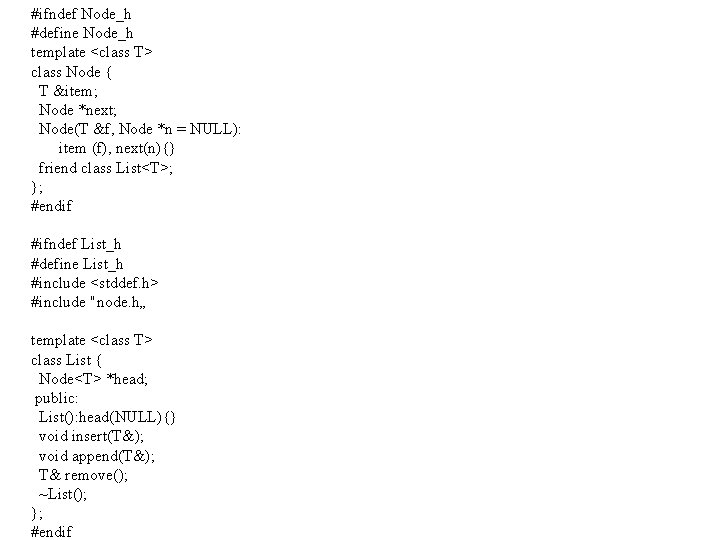
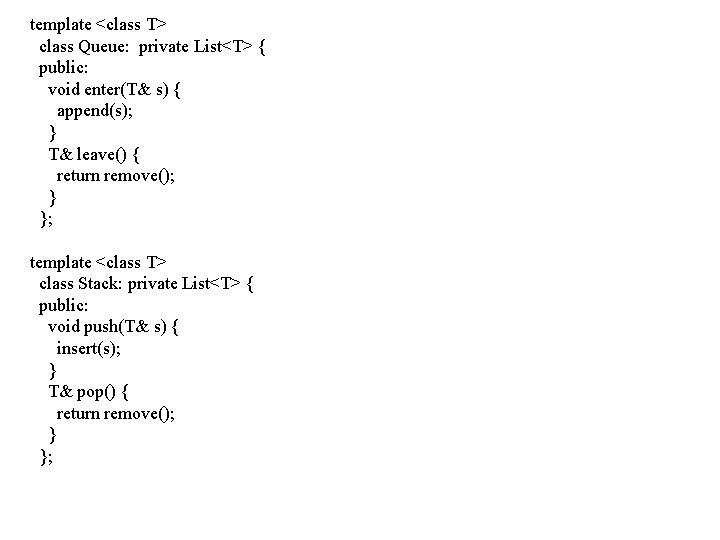
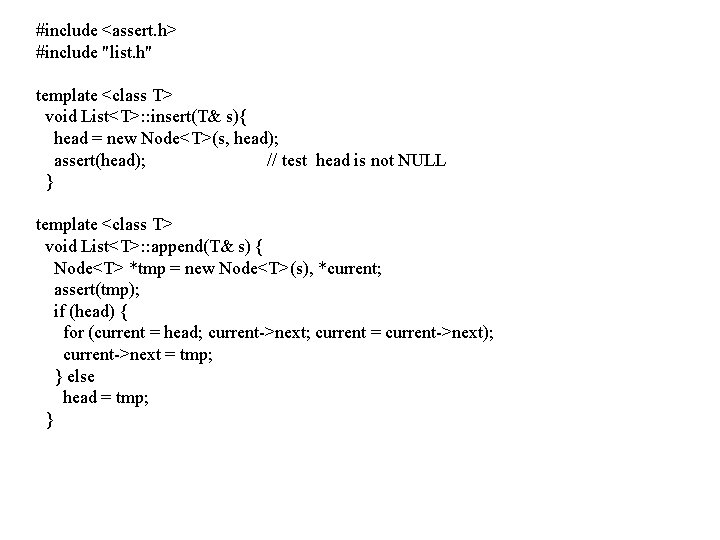
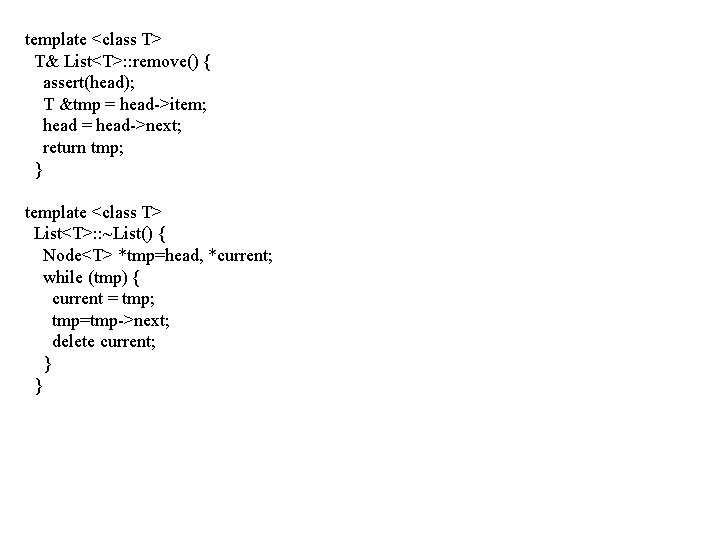
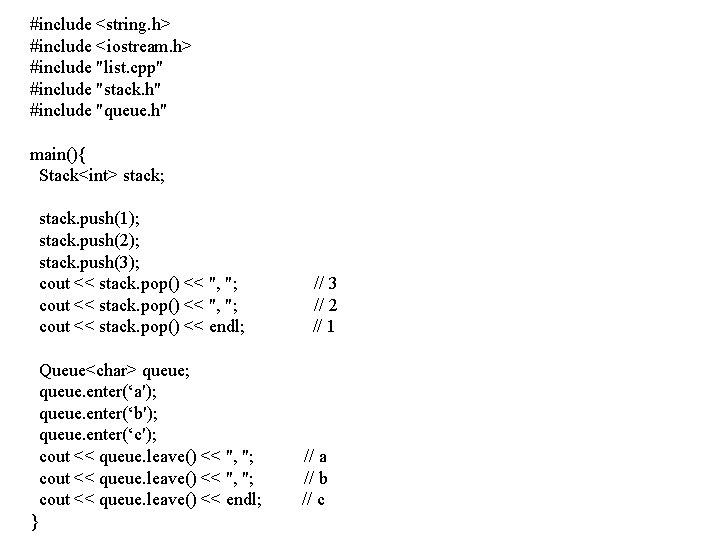
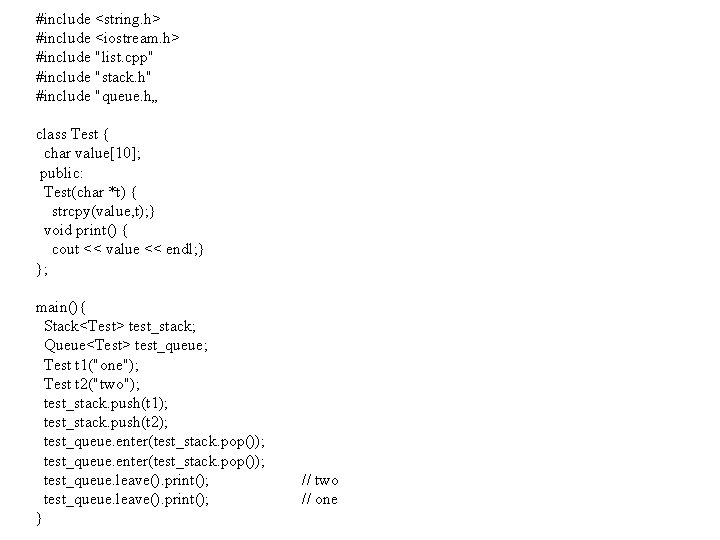
- Slides: 61
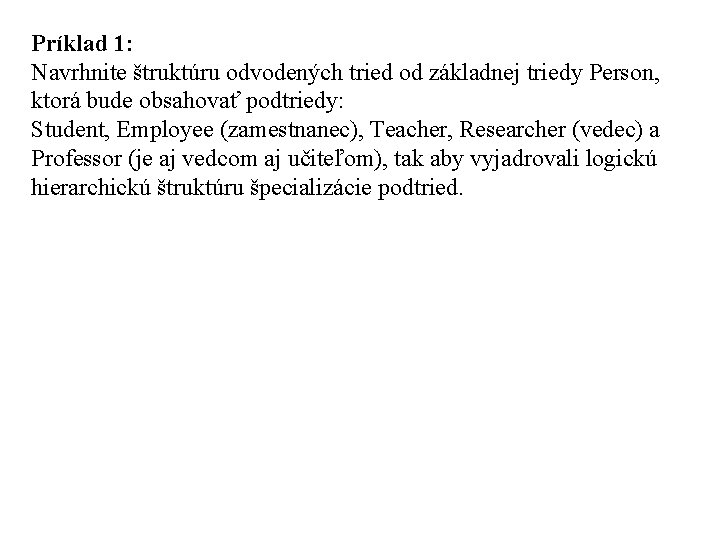
Príklad 1: Navrhnite štruktúru odvodených tried od základnej triedy Person, ktorá bude obsahovať podtriedy: Student, Employee (zamestnanec), Teacher, Researcher (vedec) a Professor (je aj vedcom aj učiteľom), tak aby vyjadrovali logickú hierarchickú štruktúru špecializácie podtried.
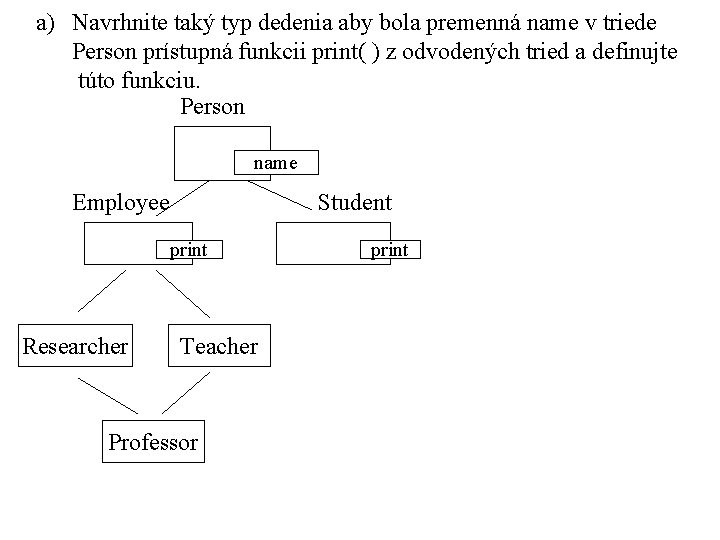
a) Navrhnite taký typ dedenia aby bola premenná name v triede Person prístupná funkcii print( ) z odvodených tried a definujte túto funkciu. Person name Employee Student print Researcher Teacher Professor print
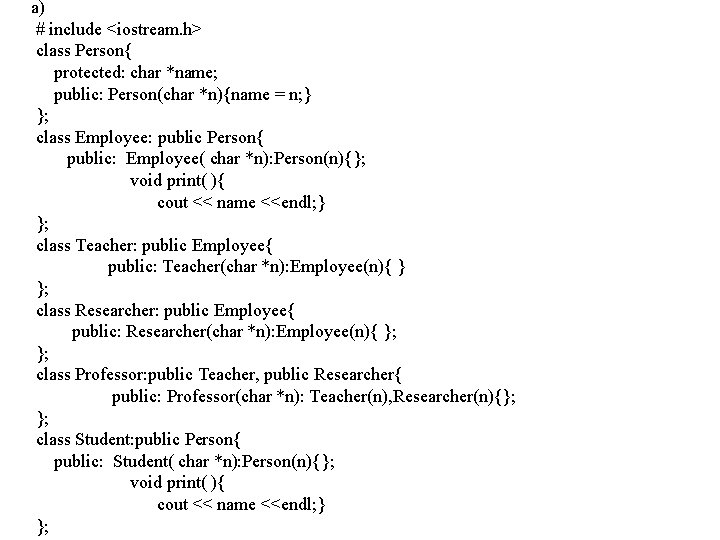
a) # include <iostream. h> class Person{ protected: char *name; public: Person(char *n){name = n; } }; class Employee: public Person{ public: Employee( char *n): Person(n){}; void print( ){ cout << name <<endl; } }; class Teacher: public Employee{ public: Teacher(char *n): Employee(n){ } }; class Researcher: public Employee{ public: Researcher(char *n): Employee(n){ }; }; class Professor: public Teacher, public Researcher{ public: Professor(char *n): Teacher(n), Researcher(n){}; }; class Student: public Person{ public: Student( char *n): Person(n){}; void print( ){ cout << name <<endl; } };
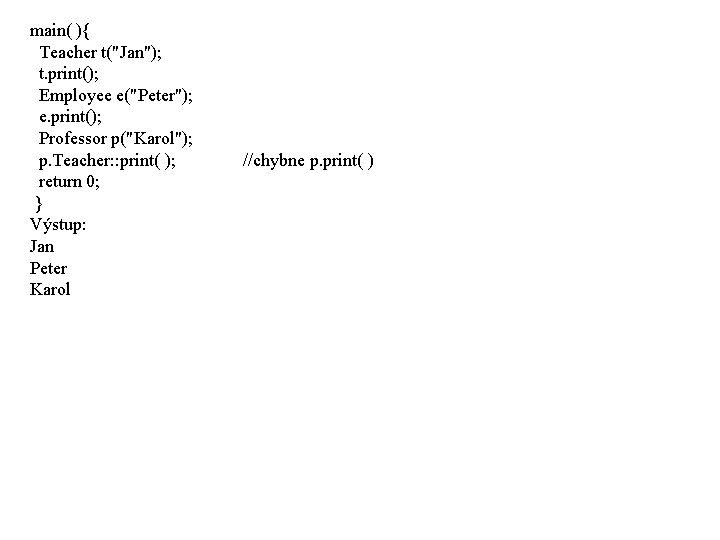
main( ){ Teacher t("Jan"); t. print(); Employee e("Peter"); e. print(); Professor p("Karol"); p. Teacher: : print( ); return 0; } Výstup: Jan Peter Karol //chybne p. print( )
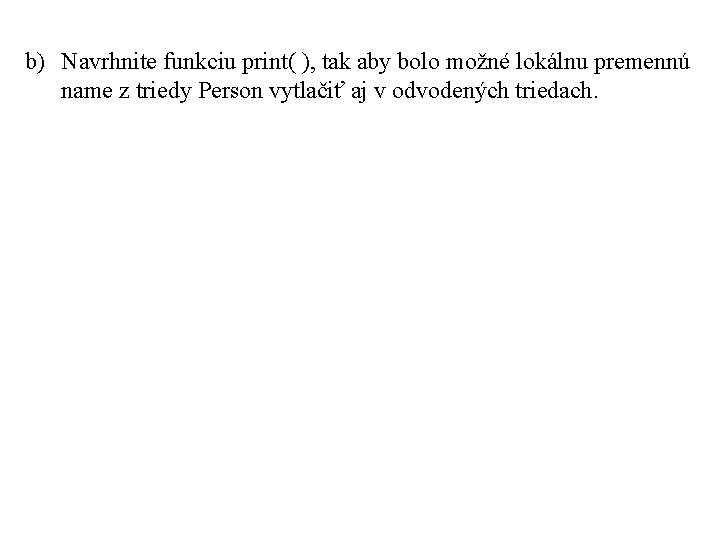
b) Navrhnite funkciu print( ), tak aby bolo možné lokálnu premennú name z triedy Person vytlačiť aj v odvodených triedach.
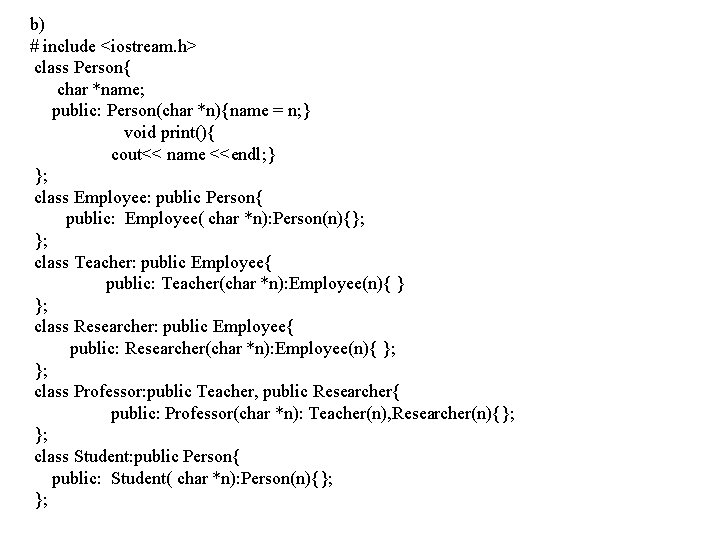
b) # include <iostream. h> class Person{ char *name; public: Person(char *n){name = n; } void print(){ cout<< name <<endl; } }; class Employee: public Person{ public: Employee( char *n): Person(n){}; }; class Teacher: public Employee{ public: Teacher(char *n): Employee(n){ } }; class Researcher: public Employee{ public: Researcher(char *n): Employee(n){ }; }; class Professor: public Teacher, public Researcher{ public: Professor(char *n): Teacher(n), Researcher(n){}; }; class Student: public Person{ public: Student( char *n): Person(n){}; };
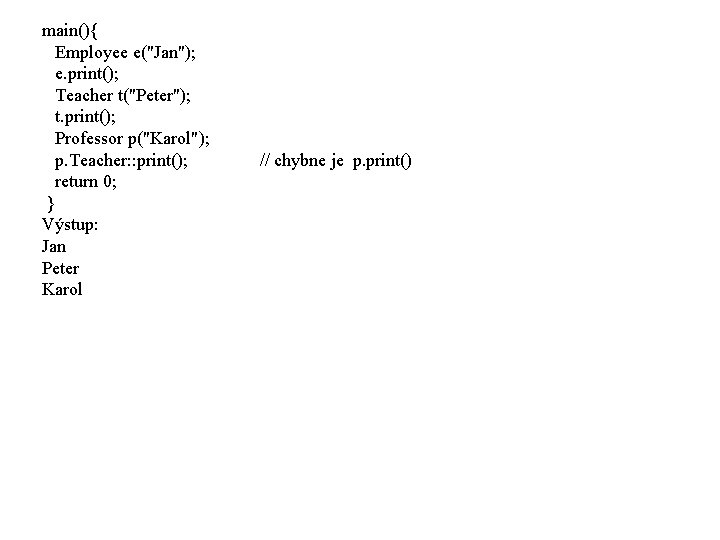
main(){ Employee e("Jan"); e. print(); Teacher t("Peter"); t. print(); Professor p("Karol"); p. Teacher: : print(); return 0; } Výstup: Jan Peter Karol // chybne je p. print()
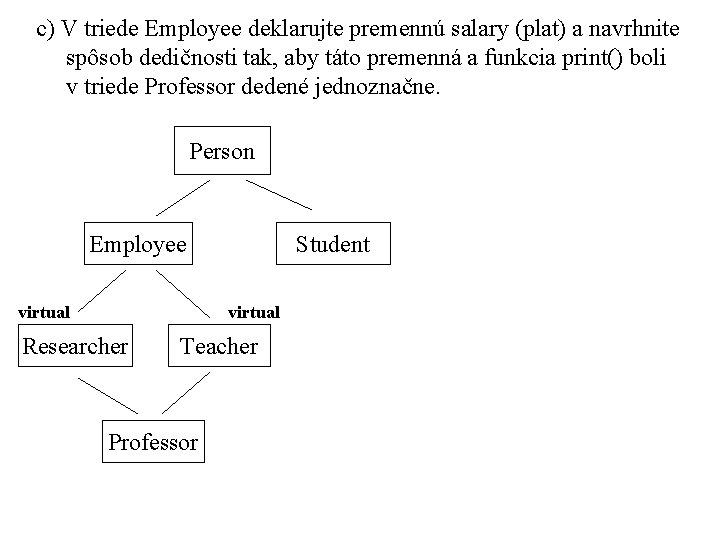
c) V triede Employee deklarujte premennú salary (plat) a navrhnite spôsob dedičnosti tak, aby táto premenná a funkcia print() boli v triede Professor dedené jednoznačne. Person Employee virtual Student virtual Researcher Teacher Professor
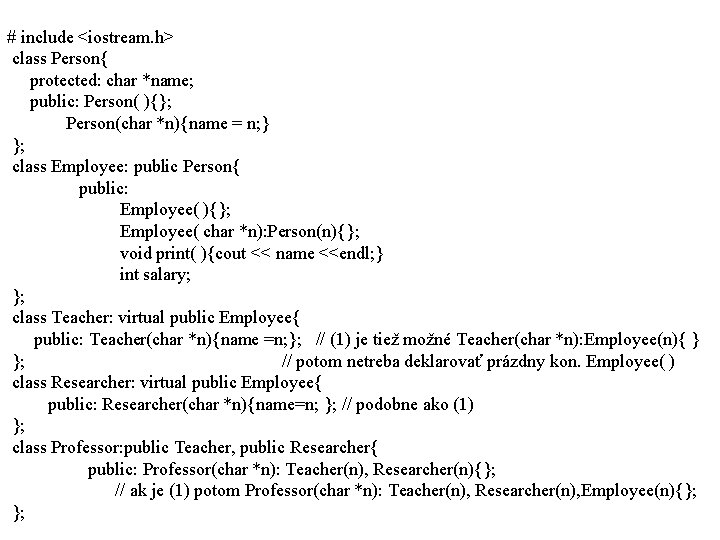
# include <iostream. h> class Person{ protected: char *name; public: Person( ){}; Person(char *n){name = n; } }; class Employee: public Person{ public: Employee( ){}; Employee( char *n): Person(n){}; void print( ){cout << name <<endl; } int salary; }; class Teacher: virtual public Employee{ public: Teacher(char *n){name =n; }; // (1) je tiež možné Teacher(char *n): Employee(n){ } }; // potom netreba deklarovať prázdny kon. Employee( ) class Researcher: virtual public Employee{ public: Researcher(char *n){name=n; }; // podobne ako (1) }; class Professor: public Teacher, public Researcher{ public: Professor(char *n): Teacher(n), Researcher(n){}; // ak je (1) potom Professor(char *n): Teacher(n), Researcher(n), Employee(n){}; };
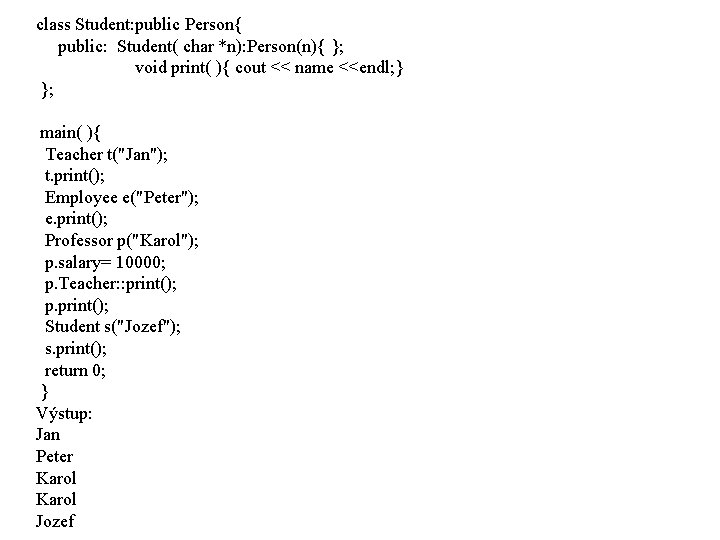
class Student: public Person{ public: Student( char *n): Person(n){ }; void print( ){ cout << name <<endl; } }; main( ){ Teacher t("Jan"); t. print(); Employee e("Peter"); e. print(); Professor p("Karol"); p. salary= 10000; p. Teacher: : print(); p. print(); Student s("Jozef"); s. print(); return 0; } Výstup: Jan Peter Karol Jozef
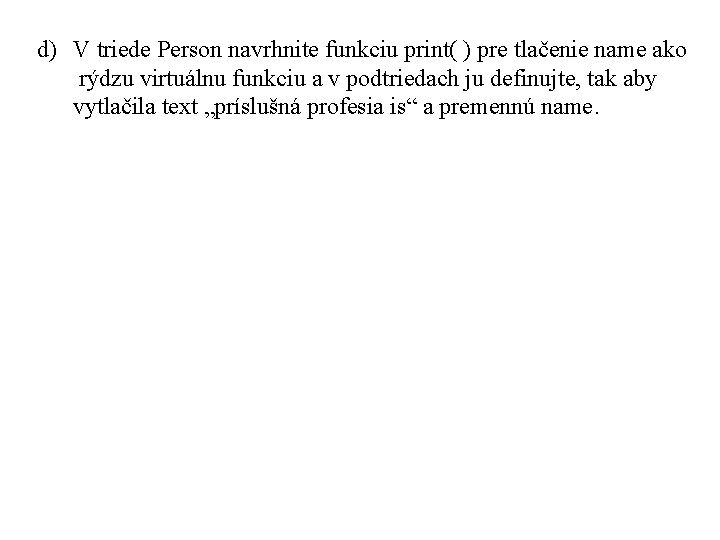
d) V triede Person navrhnite funkciu print( ) pre tlačenie name ako rýdzu virtuálnu funkciu a v podtriedach ju definujte, tak aby vytlačila text „príslušná profesia is“ a premennú name.
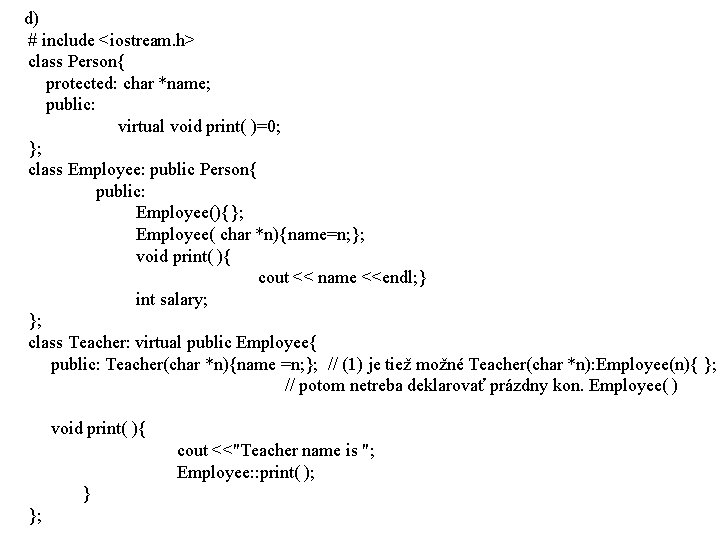
d) # include <iostream. h> class Person{ protected: char *name; public: virtual void print( )=0; }; class Employee: public Person{ public: Employee(){}; Employee( char *n){name=n; }; void print( ){ cout << name <<endl; } int salary; }; class Teacher: virtual public Employee{ public: Teacher(char *n){name =n; }; // (1) je tiež možné Teacher(char *n): Employee(n){ }; // potom netreba deklarovať prázdny kon. Employee( ) void print( ){ cout <<"Teacher name is "; Employee: : print( ); } };
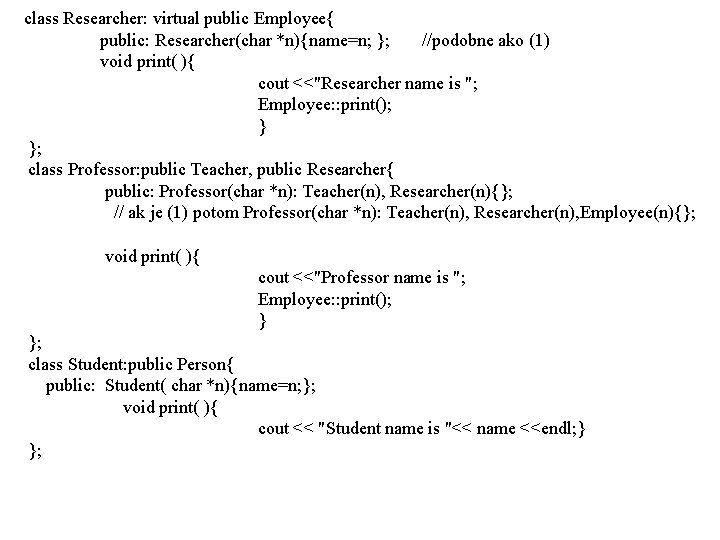
class Researcher: virtual public Employee{ public: Researcher(char *n){name=n; }; //podobne ako (1) void print( ){ cout <<"Researcher name is "; Employee: : print(); } }; class Professor: public Teacher, public Researcher{ public: Professor(char *n): Teacher(n), Researcher(n){}; // ak je (1) potom Professor(char *n): Teacher(n), Researcher(n), Employee(n){}; void print( ){ cout <<"Professor name is "; Employee: : print(); } }; class Student: public Person{ public: Student( char *n){name=n; }; void print( ){ cout << "Student name is "<< name <<endl; } };
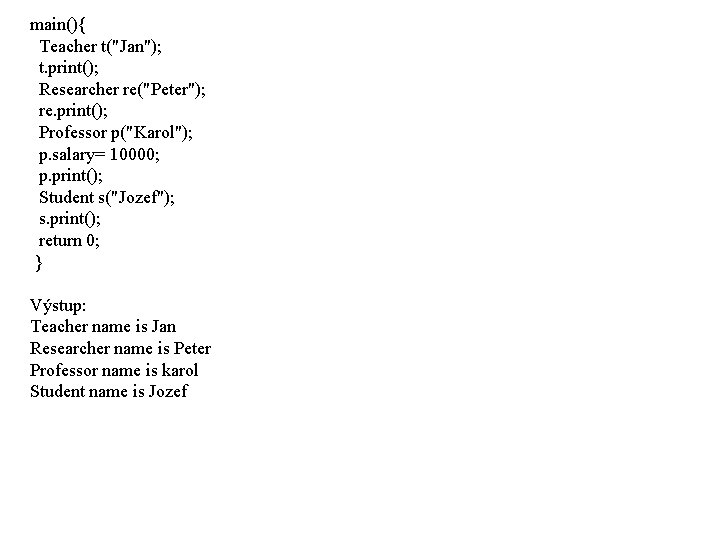
main(){ Teacher t("Jan"); t. print(); Researcher re("Peter"); re. print(); Professor p("Karol"); p. salary= 10000; p. print(); Student s("Jozef"); s. print(); return 0; } Výstup: Teacher name is Jan Researcher name is Peter Professor name is karol Student name is Jozef
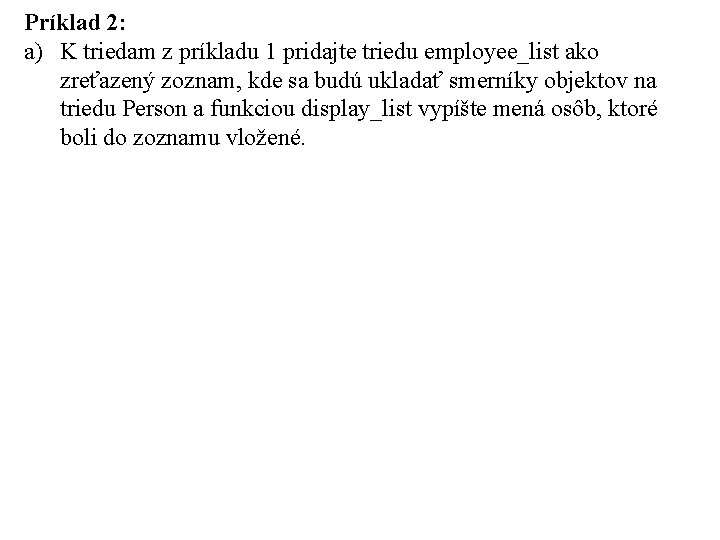
Príklad 2: a) K triedam z príkladu 1 pridajte triedu employee_list ako zreťazený zoznam, kde sa budú ukladať smerníky objektov na triedu Person a funkciou display_list vypíšte mená osôb, ktoré boli do zoznamu vložené.
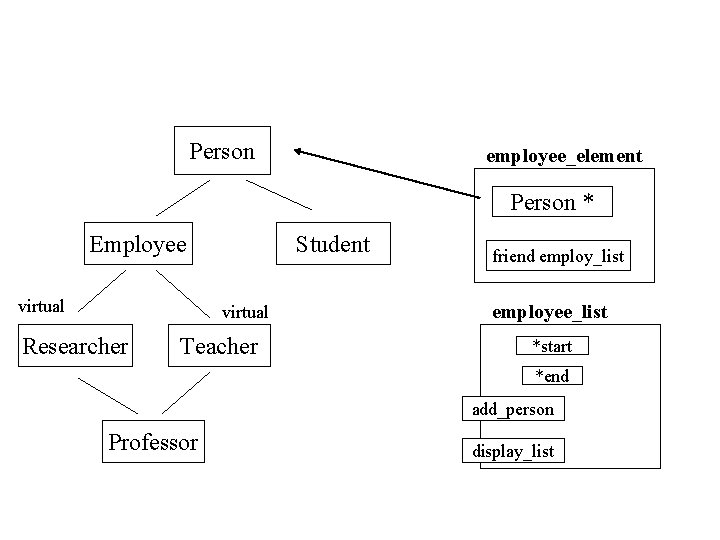
Person employee_element Person * Employee virtual Student virtual Researcher Teacher friend employ_list employee_list *start *end add_person Professor display_list
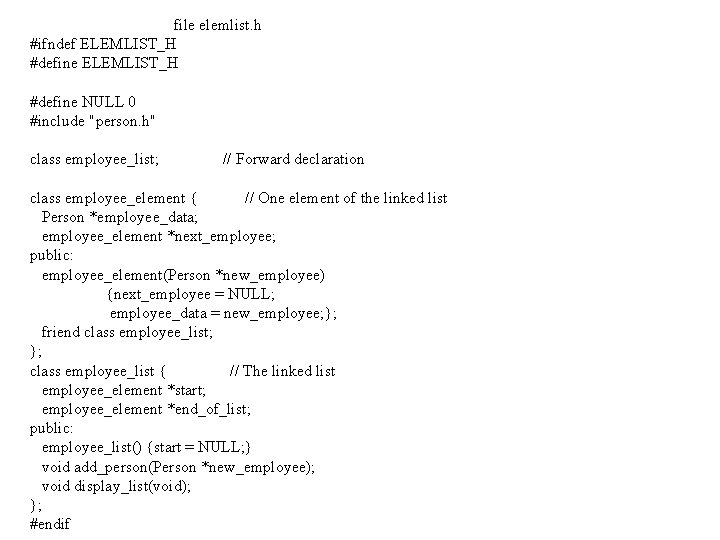
file elemlist. h #ifndef ELEMLIST_H #define NULL 0 #include "person. h" class employee_list; // Forward declaration class employee_element { // One element of the linked list Person *employee_data; employee_element *next_employee; public: employee_element(Person *new_employee) {next_employee = NULL; employee_data = new_employee; }; friend class employee_list; }; class employee_list { // The linked list employee_element *start; employee_element *end_of_list; public: employee_list() {start = NULL; } void add_person(Person *new_employee); void display_list(void); }; #endif
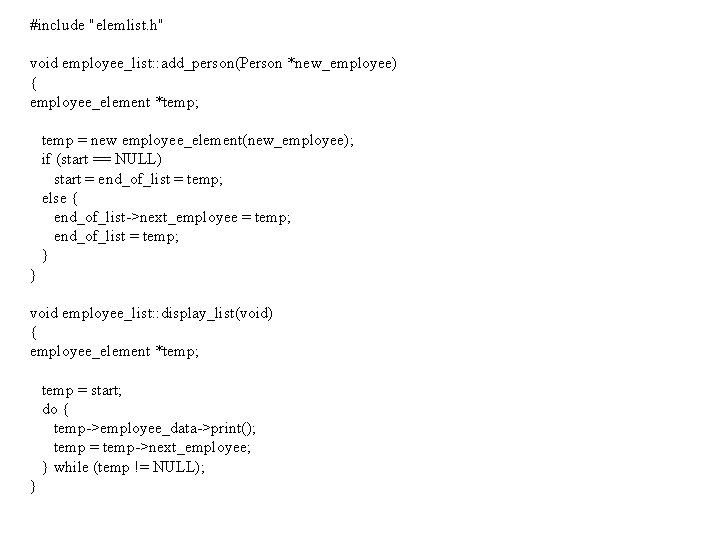
#include "elemlist. h" void employee_list: : add_person(Person *new_employee) { employee_element *temp; temp = new employee_element(new_employee); if (start == NULL) start = end_of_list = temp; else { end_of_list->next_employee = temp; end_of_list = temp; } } void employee_list: : display_list(void) { employee_element *temp; temp = start; do { temp->employee_data->print(); temp = temp->next_employee; } while (temp != NULL); }
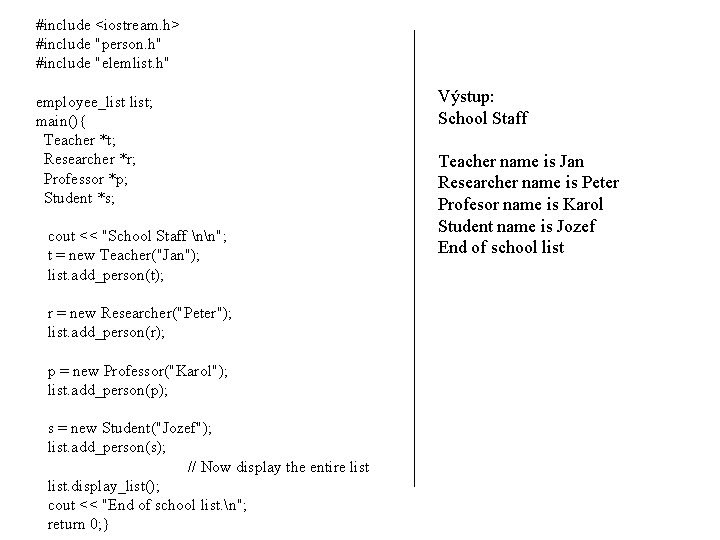
#include <iostream. h> #include "person. h" #include "elemlist. h" employee_list; main(){ Teacher *t; Researcher *r; Professor *p; Student *s; cout << "School Staff nn"; t = new Teacher("Jan"); list. add_person(t); r = new Researcher("Peter"); list. add_person(r); p = new Professor("Karol"); list. add_person(p); s = new Student("Jozef"); list. add_person(s); // Now display the entire list. display_list(); cout << "End of school list. n"; return 0; } Výstup: School Staff Teacher name is Jan Researcher name is Peter Profesor name is Karol Student name is Jozef End of school list
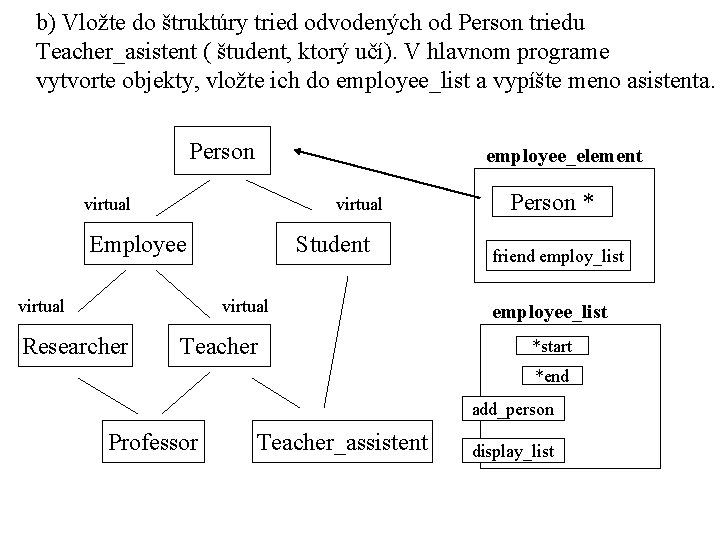
b) Vložte do štruktúry tried odvodených od Person triedu Teacher_asistent ( študent, ktorý učí). V hlavnom programe vytvorte objekty, vložte ich do employee_list a vypíšte meno asistenta. Person employee_element virtual Employee virtual Student virtual Researcher Teacher Person * friend employ_list employee_list *start *end add_person Professor Teacher_assistent display_list
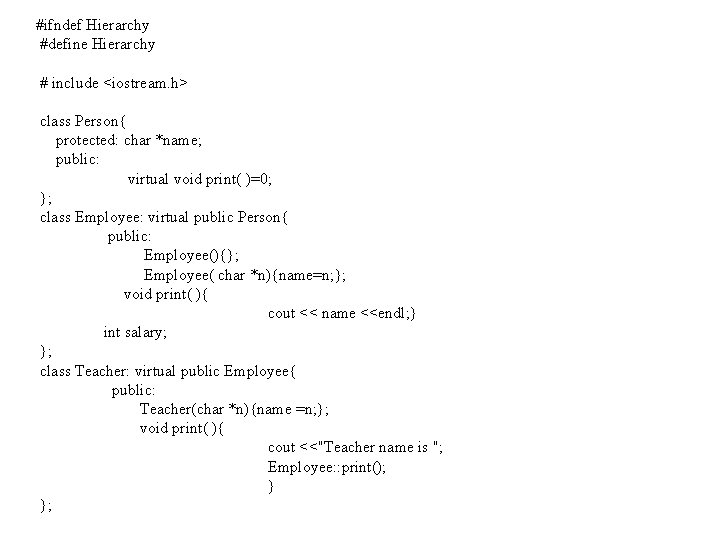
#ifndef Hierarchy #define Hierarchy # include <iostream. h> class Person{ protected: char *name; public: virtual void print( )=0; }; class Employee: virtual public Person{ public: Employee(){}; Employee( char *n){name=n; }; void print( ){ cout << name <<endl; } int salary; }; class Teacher: virtual public Employee{ public: Teacher(char *n){name =n; }; void print( ){ cout <<"Teacher name is "; Employee: : print(); } };
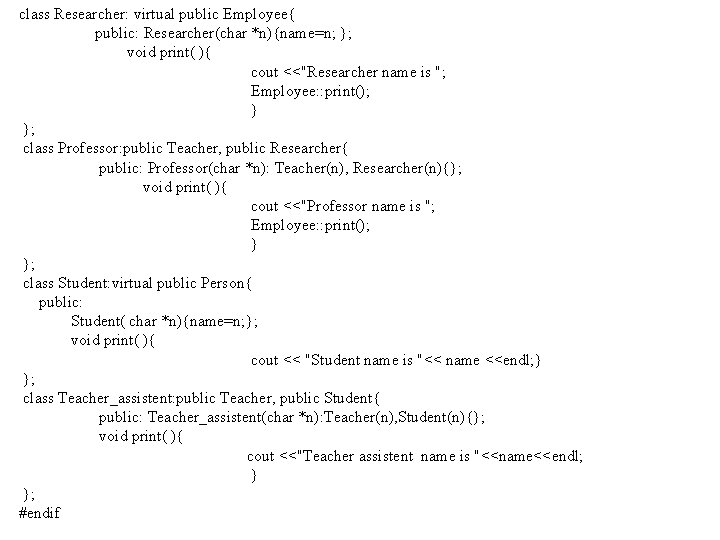
class Researcher: virtual public Employee{ public: Researcher(char *n){name=n; }; void print( ){ cout <<"Researcher name is "; Employee: : print(); } }; class Professor: public Teacher, public Researcher{ public: Professor(char *n): Teacher(n), Researcher(n){}; void print( ){ cout <<"Professor name is "; Employee: : print(); } }; class Student: virtual public Person{ public: Student( char *n){name=n; }; void print( ){ cout << "Student name is "<< name <<endl; } }; class Teacher_assistent: public Teacher, public Student{ public: Teacher_assistent(char *n): Teacher(n), Student(n){}; void print( ){ cout <<"Teacher assistent name is "<<name<<endl; } }; #endif
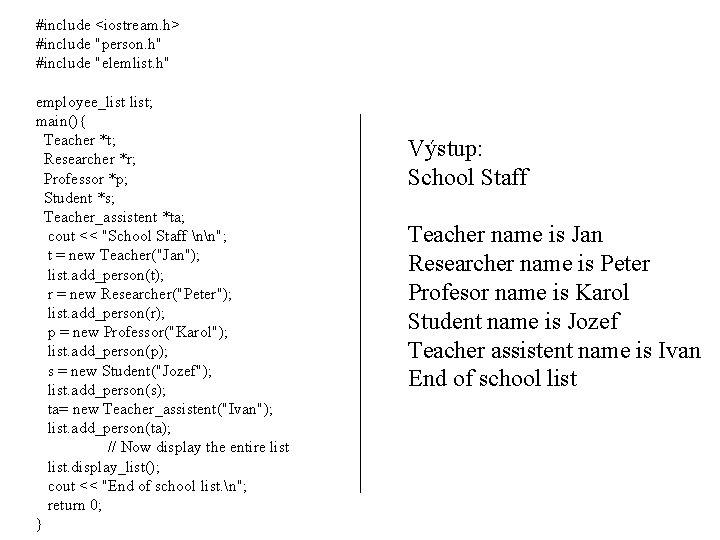
#include <iostream. h> #include "person. h" #include "elemlist. h" employee_list; main(){ Teacher *t; Researcher *r; Professor *p; Student *s; Teacher_assistent *ta; cout << "School Staff nn"; t = new Teacher("Jan"); list. add_person(t); r = new Researcher("Peter"); list. add_person(r); p = new Professor("Karol"); list. add_person(p); s = new Student("Jozef"); list. add_person(s); ta= new Teacher_assistent("Ivan"); list. add_person(ta); // Now display the entire list. display_list(); cout << "End of school list. n"; return 0; } Výstup: School Staff Teacher name is Jan Researcher name is Peter Profesor name is Karol Student name is Jozef Teacher assistent name is Ivan End of school list
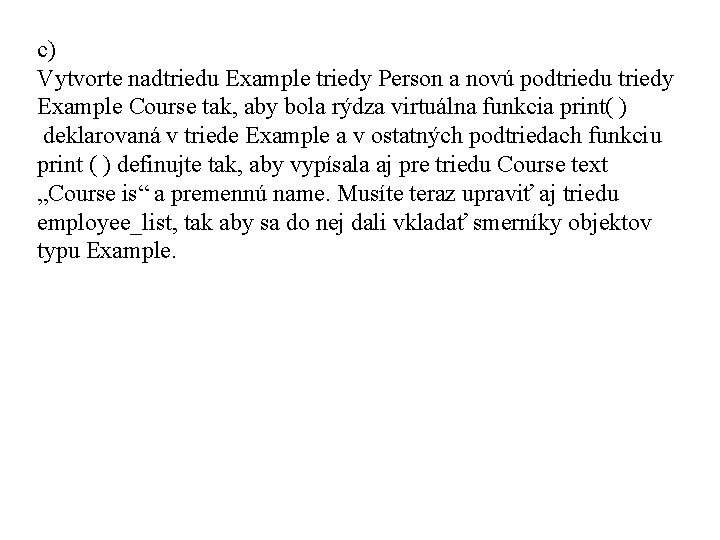
c) Vytvorte nadtriedu Example triedy Person a novú podtriedu triedy Example Course tak, aby bola rýdza virtuálna funkcia print( ) deklarovaná v triede Example a v ostatných podtriedach funkciu print ( ) definujte tak, aby vypísala aj pre triedu Course text „Course is“ a premennú name. Musíte teraz upraviť aj triedu employee_list, tak aby sa do nej dali vkladať smerníky objektov typu Example.
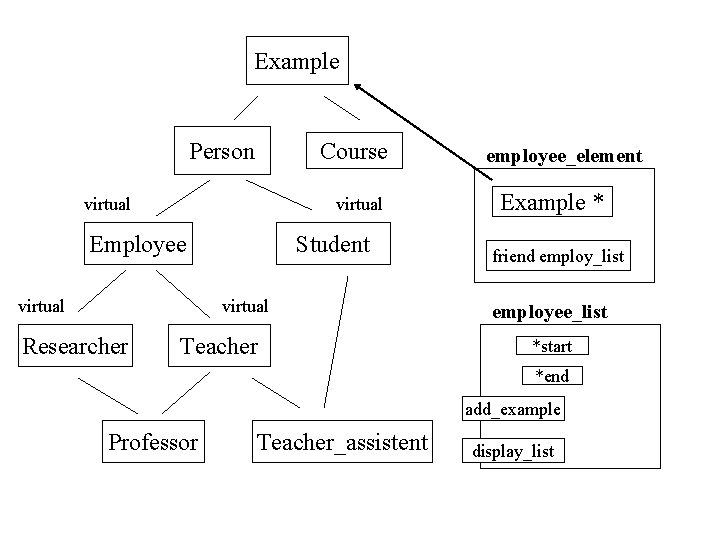
Example Person Course virtual Employee virtual Student virtual Researcher Teacher employee_element Example * friend employ_list employee_list *start *end add_example Professor Teacher_assistent display_list
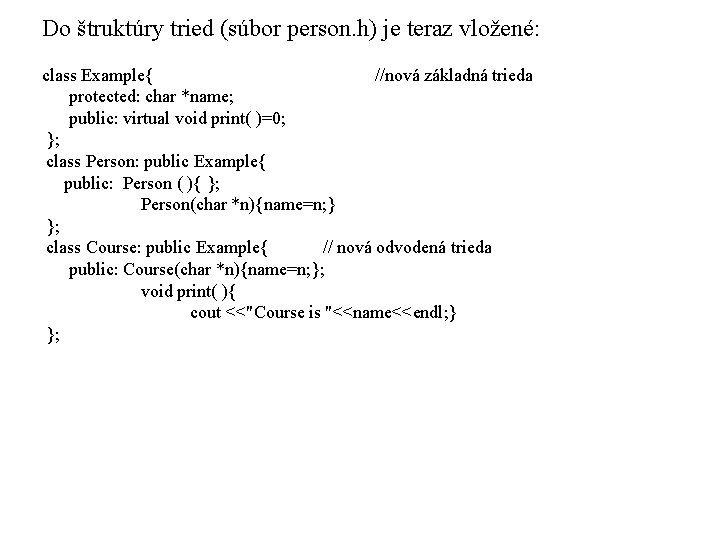
Do štruktúry tried (súbor person. h) je teraz vložené: class Example{ //nová základná trieda protected: char *name; public: virtual void print( )=0; }; class Person: public Example{ public: Person ( ){ }; Person(char *n){name=n; } }; class Course: public Example{ // nová odvodená trieda public: Course(char *n){name=n; }; void print( ){ cout <<"Course is "<<name<<endl; } };
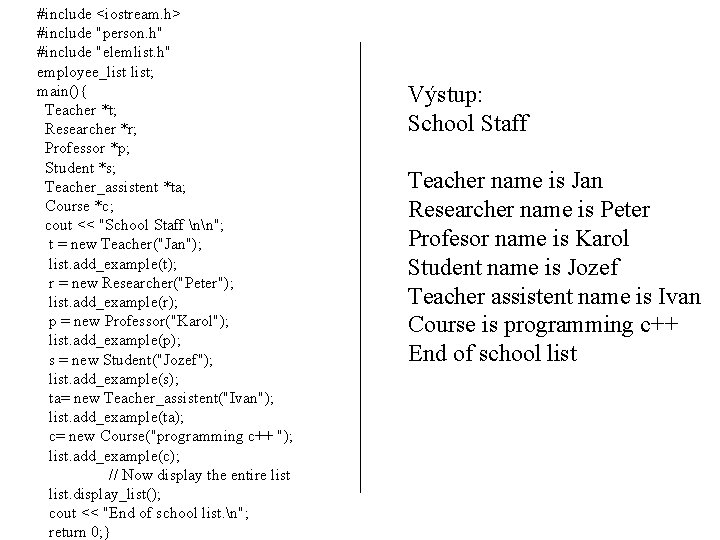
#include <iostream. h> #include "person. h" #include "elemlist. h" employee_list; main(){ Teacher *t; Researcher *r; Professor *p; Student *s; Teacher_assistent *ta; Course *c; cout << "School Staff nn"; t = new Teacher("Jan"); list. add_example(t); r = new Researcher("Peter"); list. add_example(r); p = new Professor("Karol"); list. add_example(p); s = new Student("Jozef"); list. add_example(s); ta= new Teacher_assistent("Ivan"); list. add_example(ta); c= new Course("programming c++ "); list. add_example(c); // Now display the entire list. display_list(); cout << "End of school list. n"; return 0; } Výstup: School Staff Teacher name is Jan Researcher name is Peter Profesor name is Karol Student name is Jozef Teacher assistent name is Ivan Course is programming c++ End of school list
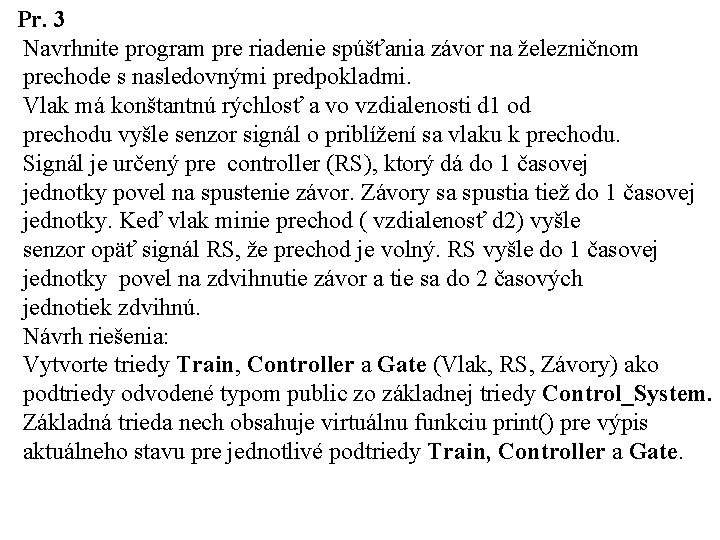
Pr. 3 Navrhnite program pre riadenie spúšťania závor na železničnom prechode s nasledovnými predpokladmi. Vlak má konštantnú rýchlosť a vo vzdialenosti d 1 od prechodu vyšle senzor signál o priblížení sa vlaku k prechodu. Signál je určený pre controller (RS), ktorý dá do 1 časovej jednotky povel na spustenie závor. Závory sa spustia tiež do 1 časovej jednotky. Keď vlak minie prechod ( vzdialenosť d 2) vyšle senzor opäť signál RS, že prechod je volný. RS vyšle do 1 časovej jednotky povel na zdvihnutie závor a tie sa do 2 časových jednotiek zdvihnú. Návrh riešenia: Vytvorte triedy Train, Controller a Gate (Vlak, RS, Závory) ako podtriedy odvodené typom public zo základnej triedy Control_System. Základná trieda nech obsahuje virtuálnu funkciu print() pre výpis aktuálneho stavu pre jednotlivé podtriedy Train, Controller a Gate.
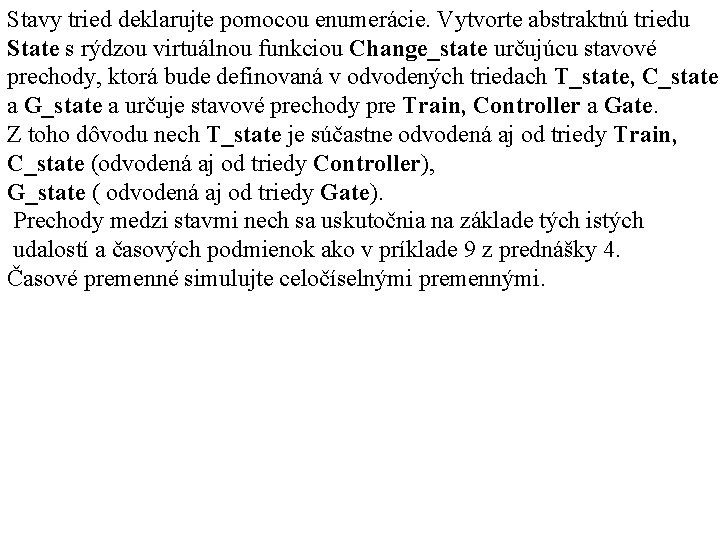
Stavy tried deklarujte pomocou enumerácie. Vytvorte abstraktnú triedu State s rýdzou virtuálnou funkciou Change_state určujúcu stavové prechody, ktorá bude definovaná v odvodených triedach T_state, C_state a G_state a určuje stavové prechody pre Train, Controller a Gate. Z toho dôvodu nech T_state je súčastne odvodená aj od triedy Train, C_state (odvodená aj od triedy Controller), G_state ( odvodená aj od triedy Gate). Prechody medzi stavmi nech sa uskutočnia na základe tých istých udalostí a časových podmienok ako v príklade 9 z prednášky 4. Časové premenné simulujte celočíselnými premennými.
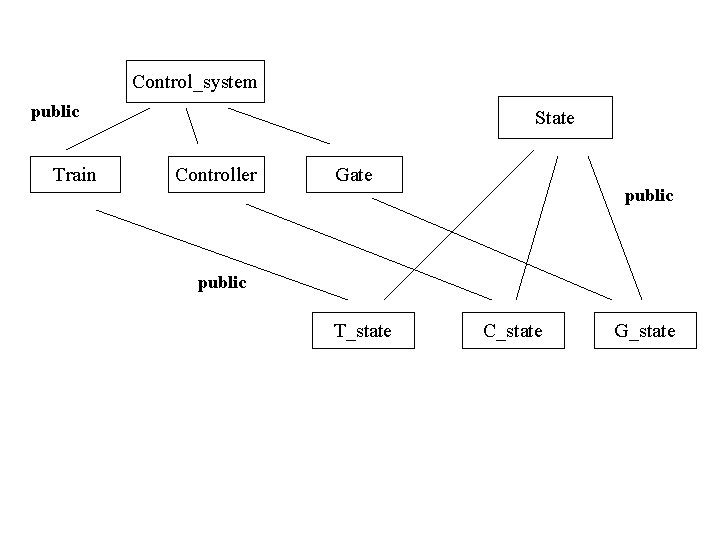
Control_system public Train State Controller Gate public T_state C_state G_state
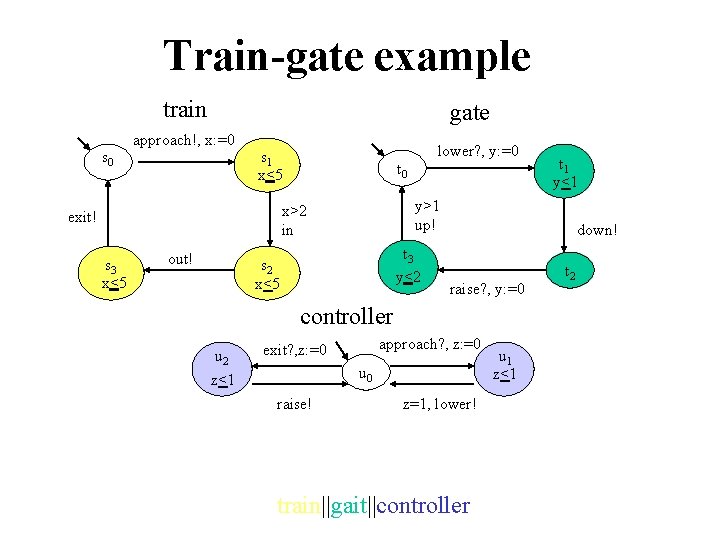
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 up! x>2 in exit! s 3 x<5 out! t 3 y<2 s 2 x<5 down! raise? , y: =0 controller u 2 z<1 approach? , z: =0 exit? , z: =0 u 0 raise! t 1 y<1 z=1, lower! train||gait||controller u 1 z<1 t 2
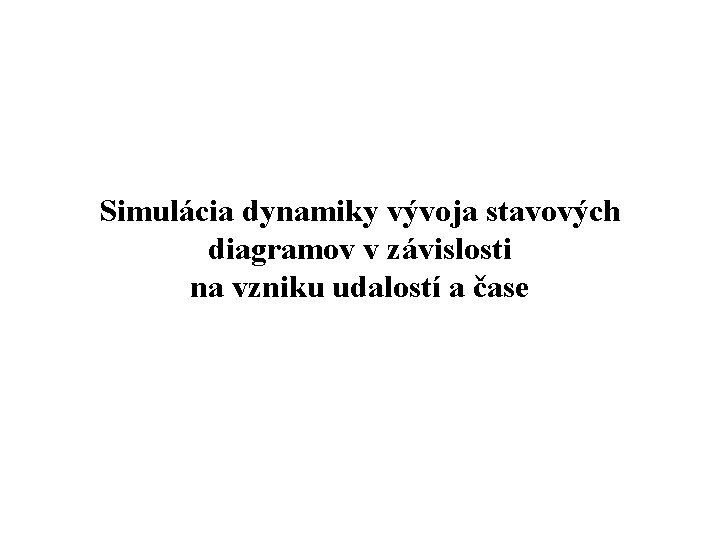
Simulácia dynamiky vývoja stavových diagramov v závislosti na vzniku udalostí a čase
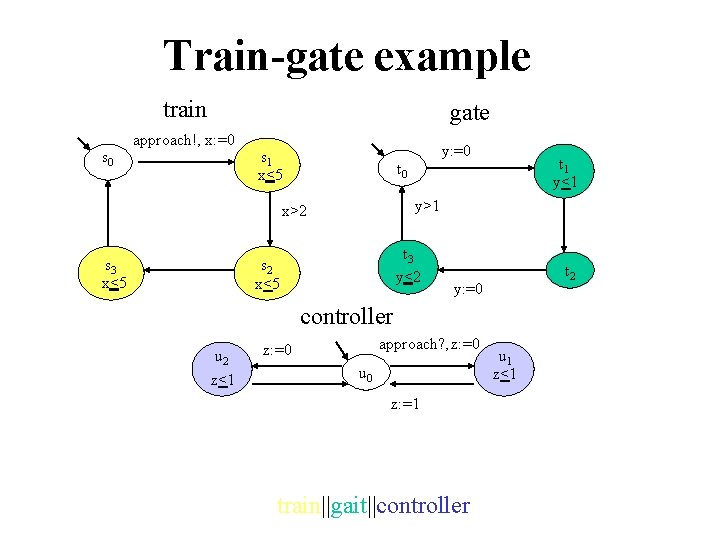
Train-gate example train s 0 gate approach!, x: =0 y: =0 s 1 x<5 y>1 x>2 s 3 x<5 t 1 y<1 t 0 t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 approach? , z: =0 u 0 z: =1 train||gait||controller u 1 z<1
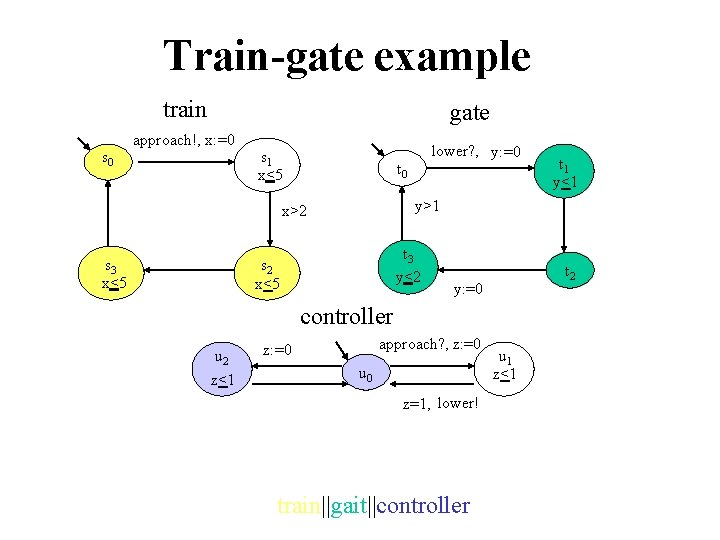
Train-gate example train s 0 gate approach!, x: =0 s 1 x<5 t 0 t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 t 1 y<1 y>1 x>2 s 3 x<5 lower? , y: =0 approach? , z: =0 u 0 z=1, lower! train||gait||controller u 1 z<1
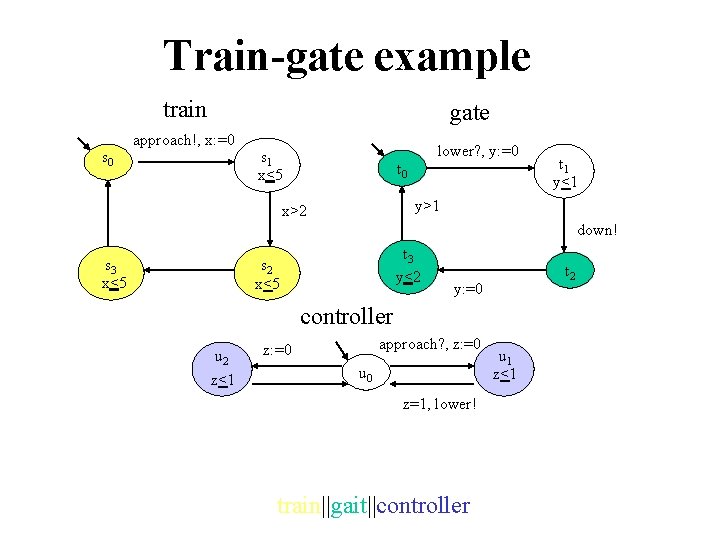
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 t 1 y<1 y>1 x>2 down! s 3 x<5 t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 approach? , z: =0 u 0 z=1, lower! train||gait||controller u 1 z<1
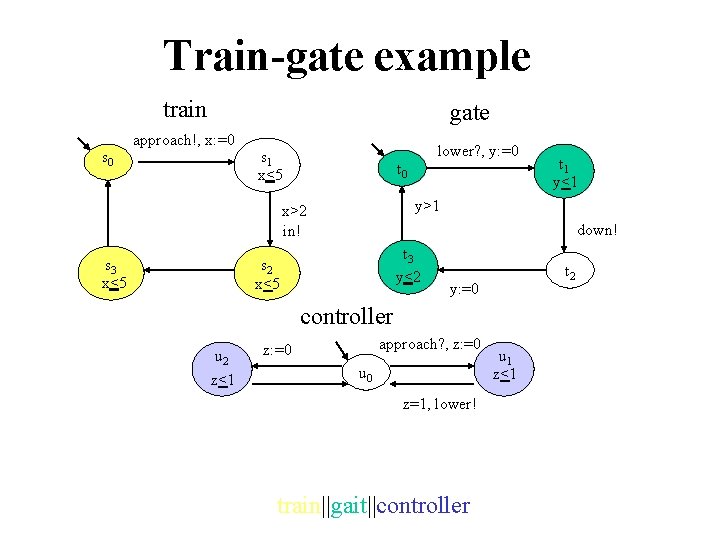
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 x>2 in! s 3 x<5 down! t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 t 1 y<1 approach? , z: =0 u 0 z=1, lower! train||gait||controller u 1 z<1
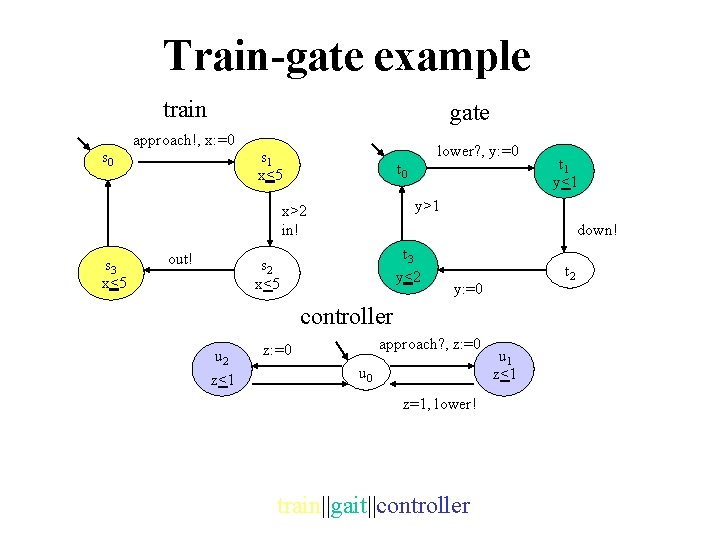
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 x>2 in! s 3 x<5 out! down! t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 t 1 y<1 approach? , z: =0 u 0 z=1, lower! train||gait||controller u 1 z<1
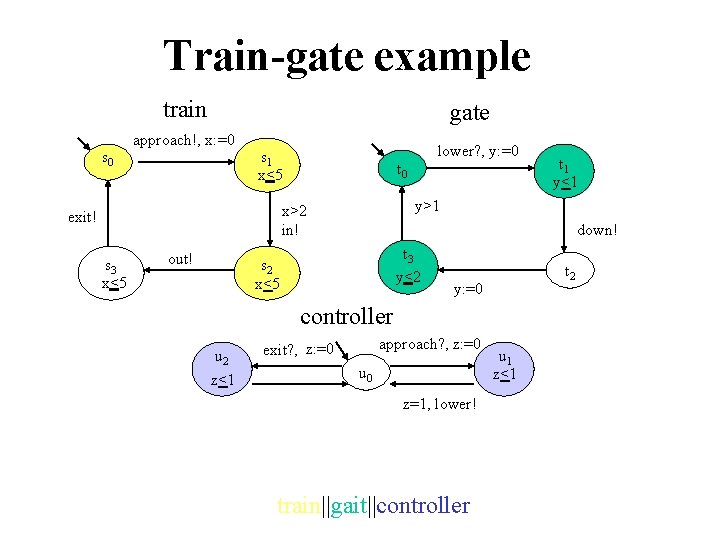
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 x>2 in! exit! s 3 x<5 out! down! t 3 y<2 s 2 x<5 t 2 y: =0 controller u 2 z<1 t 1 y<1 approach? , z: =0 exit? , z: =0 u 0 z=1, lower! train||gait||controller u 1 z<1
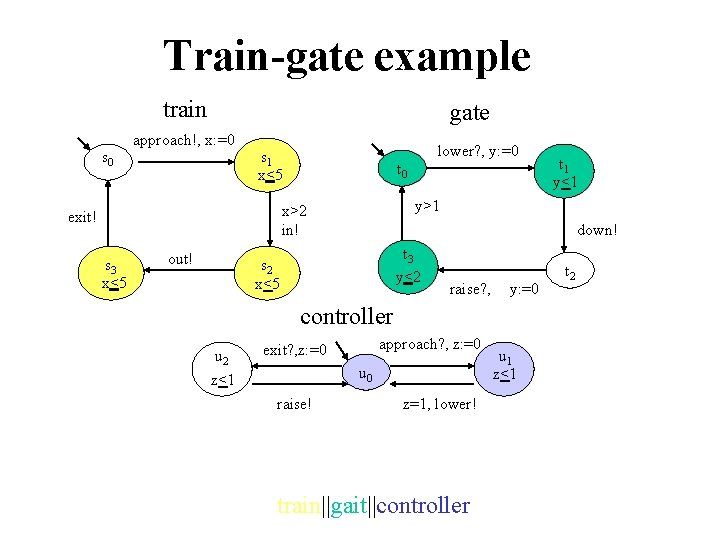
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 x>2 in! exit! s 3 x<5 out! down! t 3 y<2 s 2 x<5 raise? , y: =0 controller u 2 z<1 approach? , z: =0 exit? , z: =0 u 0 raise! t 1 y<1 z=1, lower! train||gait||controller u 1 z<1 t 2
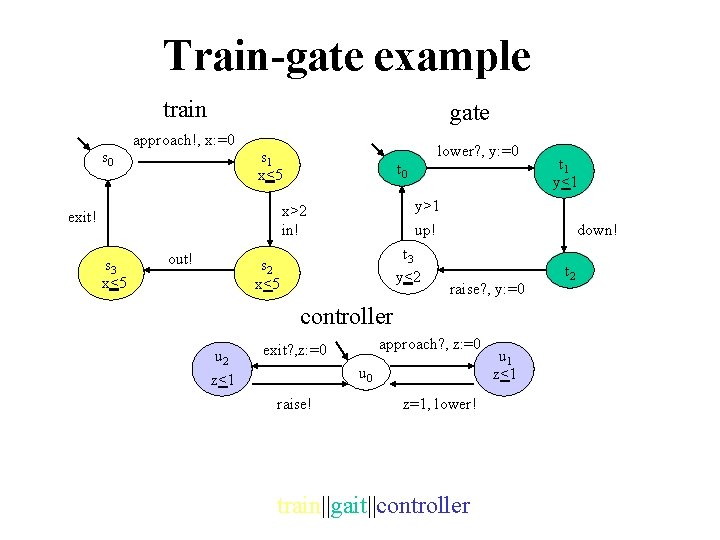
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 up! x>2 in! exit! s 3 x<5 out! t 3 y<2 s 2 x<5 down! raise? , y: =0 controller u 2 z<1 approach? , z: =0 exit? , z: =0 u 0 raise! t 1 y<1 z=1, lower! train||gait||controller u 1 z<1 t 2
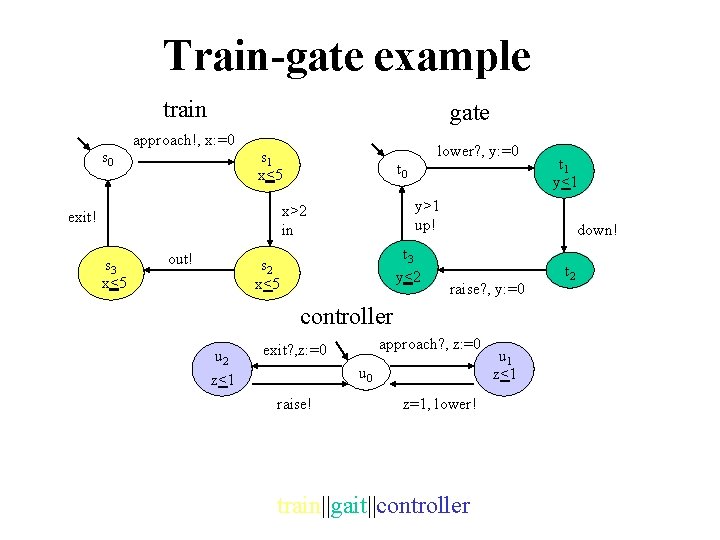
Train-gate example train s 0 gate approach!, x: =0 lower? , y: =0 s 1 x<5 t 0 y>1 up! x>2 in exit! s 3 x<5 out! t 3 y<2 s 2 x<5 down! raise? , y: =0 controller u 2 z<1 approach? , z: =0 exit? , z: =0 u 0 raise! t 1 y<1 z=1, lower! train||gait||controller u 1 z<1 t 2
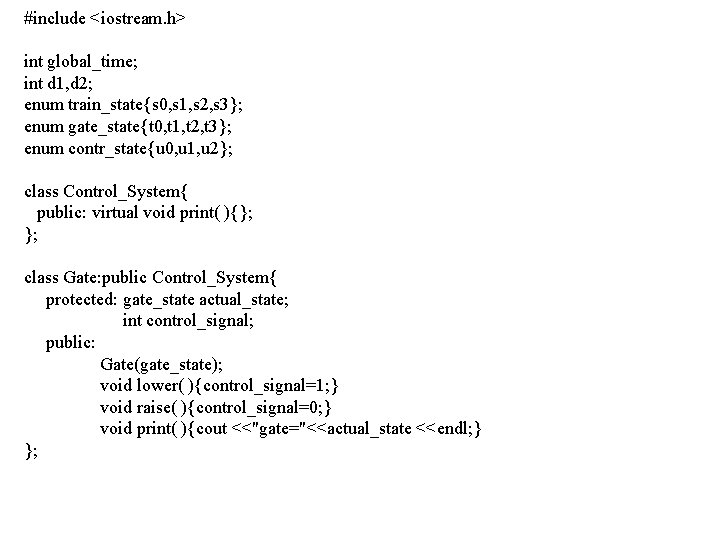
#include <iostream. h> int global_time; int d 1, d 2; enum train_state{s 0, s 1, s 2, s 3}; enum gate_state{t 0, t 1, t 2, t 3}; enum contr_state{u 0, u 1, u 2}; class Control_System{ public: virtual void print( ){}; }; class Gate: public Control_System{ protected: gate_state actual_state; int control_signal; public: Gate(gate_state); void lower( ){control_signal=1; } void raise( ){control_signal=0; } void print( ){cout <<"gate="<<actual_state <<endl; } };
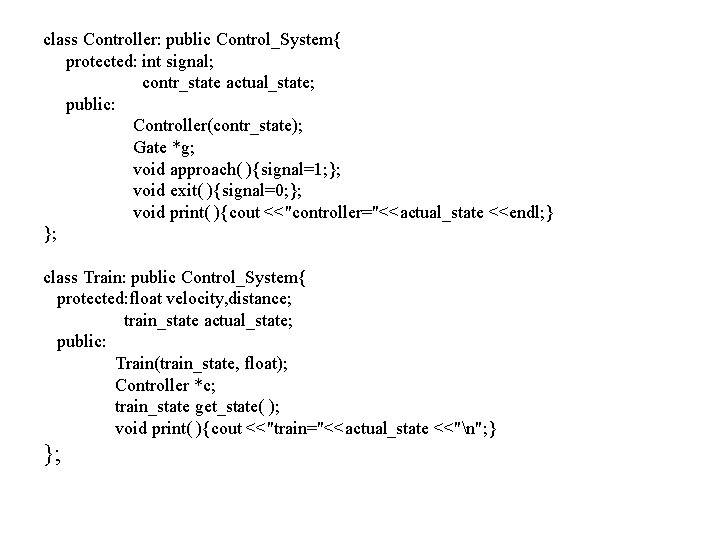
class Controller: public Control_System{ protected: int signal; contr_state actual_state; public: Controller(contr_state); Gate *g; void approach( ){signal=1; }; void exit( ){signal=0; }; void print( ){cout <<"controller="<<actual_state <<endl; } }; class Train: public Control_System{ protected: float velocity, distance; train_state actual_state; public: Train(train_state, float); Controller *c; train_state get_state( ); void print( ){cout <<"train="<<actual_state <<"n"; } };
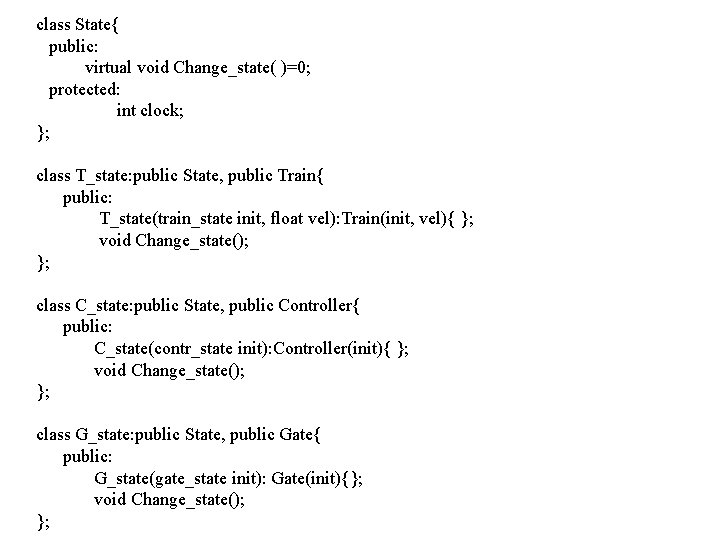
class State{ public: virtual void Change_state( )=0; protected: int clock; }; class T_state: public State, public Train{ public: T_state(train_state init, float vel): Train(init, vel){ }; void Change_state(); }; class C_state: public State, public Controller{ public: C_state(contr_state init): Controller(init){ }; void Change_state(); }; class G_state: public State, public Gate{ public: G_state(gate_state init): Gate(init){}; void Change_state(); };
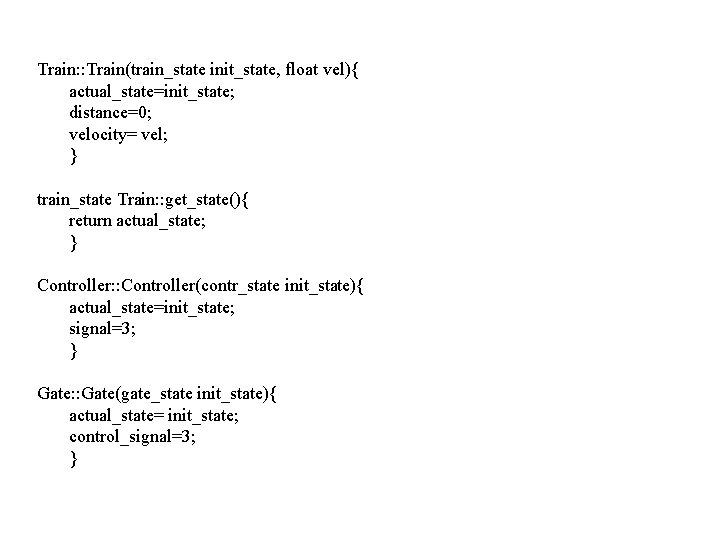
Train: : Train(train_state init_state, float vel){ actual_state=init_state; distance=0; velocity= vel; } train_state Train: : get_state(){ return actual_state; } Controller: : Controller(contr_state init_state){ actual_state=init_state; signal=3; } Gate: : Gate(gate_state init_state){ actual_state= init_state; control_signal=3; }
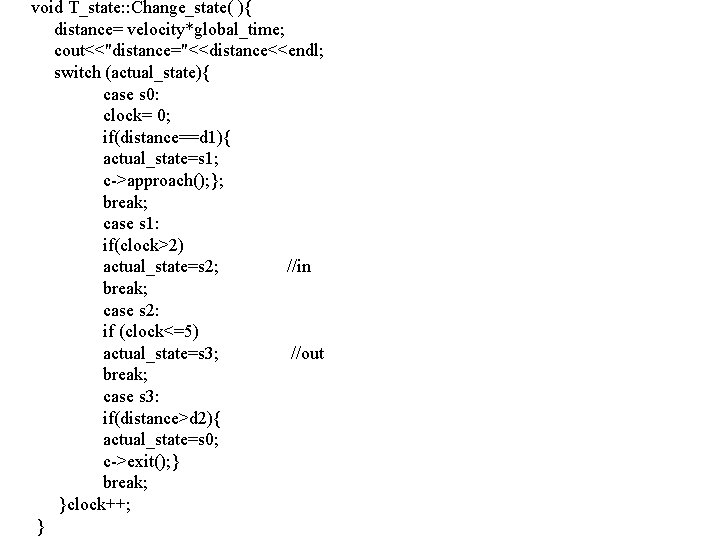
void T_state: : Change_state( ){ distance= velocity*global_time; cout<<"distance="<<distance<<endl; switch (actual_state){ case s 0: clock= 0; if(distance==d 1){ actual_state=s 1; c->approach(); }; break; case s 1: if(clock>2) actual_state=s 2; //in break; case s 2: if (clock<=5) actual_state=s 3; //out break; case s 3: if(distance>d 2){ actual_state=s 0; c->exit(); } break; }clock++; }
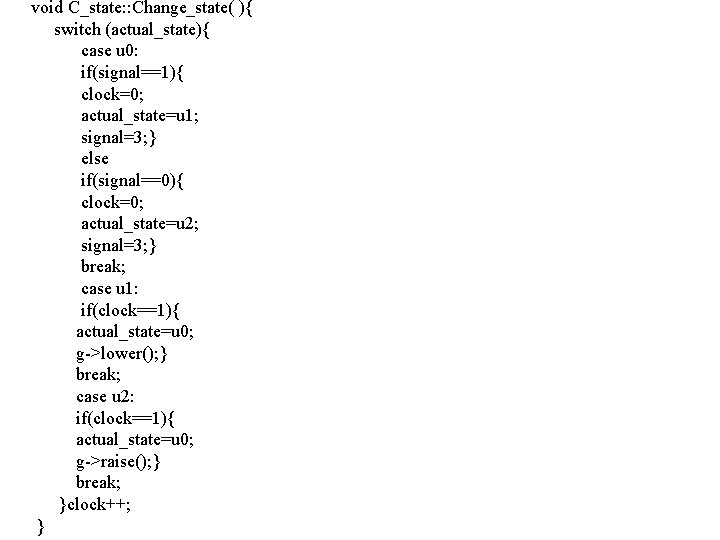
void C_state: : Change_state( ){ switch (actual_state){ case u 0: if(signal==1){ clock=0; actual_state=u 1; signal=3; } else if(signal==0){ clock=0; actual_state=u 2; signal=3; } break; case u 1: if(clock==1){ actual_state=u 0; g->lower(); } break; case u 2: if(clock==1){ actual_state=u 0; g->raise(); } break; }clock++; }
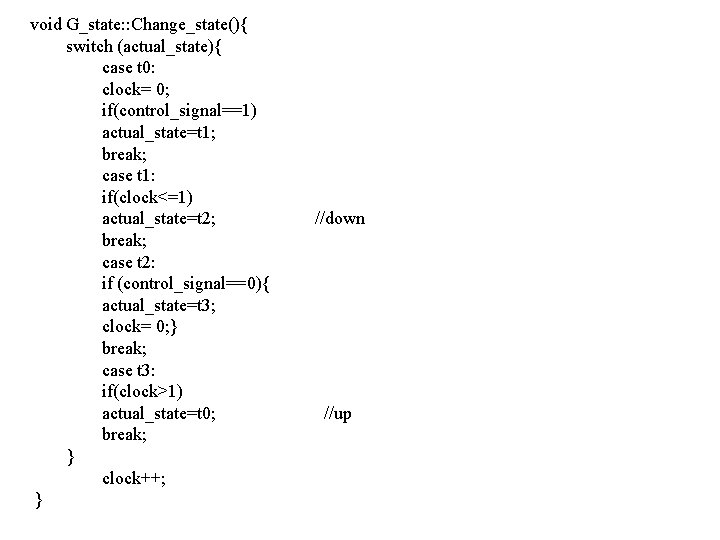
void G_state: : Change_state(){ switch (actual_state){ case t 0: clock= 0; if(control_signal==1) actual_state=t 1; break; case t 1: if(clock<=1) actual_state=t 2; break; case t 2: if (control_signal==0){ actual_state=t 3; clock= 0; } break; case t 3: if(clock>1) actual_state=t 0; break; } clock++; } //down //up
![ControlSystem array 13 State array 23 main d 1200 d 2700 globaltime0 Tstate trs Control_System *array 1[3]; State *array 2[3]; main(){ d 1=200; d 2=700; global_time=0; T_state *trs;](https://slidetodoc.com/presentation_image_h2/ca09cbb8f11293655f9a424fc2f8ebb2/image-49.jpg)
Control_System *array 1[3]; State *array 2[3]; main(){ d 1=200; d 2=700; global_time=0; T_state *trs; trs= new T_state(s 0, 100); array 1[1]=trs; array 2[1]=trs; C_state *cons; cons= new C_state(u 0); array 1[2]=cons; array 2[2]=cons; G_state *gts; gts= new G_state(t 0); array 1[3]=gts; array 2[3]=gts; trs->c= cons; cons->g= gts; do{ for(int i=1; i<4; i++){ array 2[i]->Change_state(); array 1[i]->print(); } global_time++; cout<<"time="<<global_time<<"n"; }while (global_time<12); delete [] array 1; delete [] array 2; return 0; }
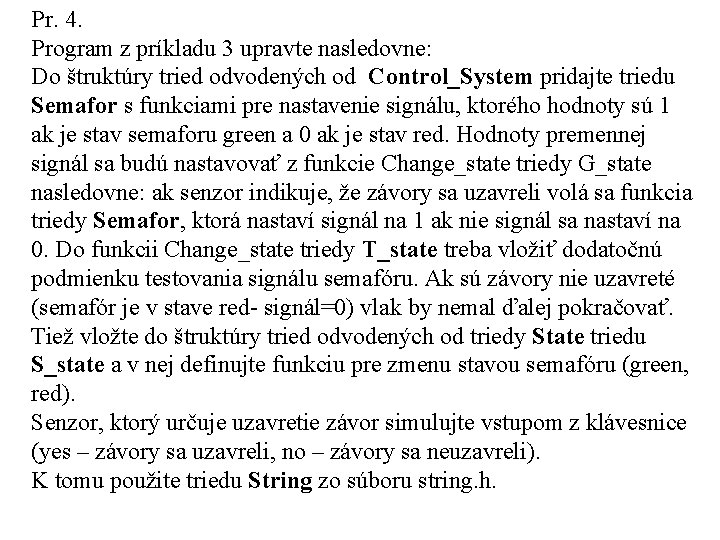
Pr. 4. Program z príkladu 3 upravte nasledovne: Do štruktúry tried odvodených od Control_System pridajte triedu Semafor s funkciami pre nastavenie signálu, ktorého hodnoty sú 1 ak je stav semaforu green a 0 ak je stav red. Hodnoty premennej signál sa budú nastavovať z funkcie Change_state triedy G_state nasledovne: ak senzor indikuje, že závory sa uzavreli volá sa funkcia triedy Semafor, ktorá nastaví signál na 1 ak nie signál sa nastaví na 0. Do funkcii Change_state triedy T_state treba vložiť dodatočnú podmienku testovania signálu semafóru. Ak sú závory nie uzavreté (semafór je v stave red- signál=0) vlak by nemal ďalej pokračovať. Tiež vložte do štruktúry tried odvodených od triedy State triedu S_state a v nej definujte funkciu pre zmenu stavou semafóru (green, red). Senzor, ktorý určuje uzavretie závor simulujte vstupom z klávesnice (yes – závory sa uzavreli, no – závory sa neuzavreli). K tomu použite triedu String zo súboru string. h.
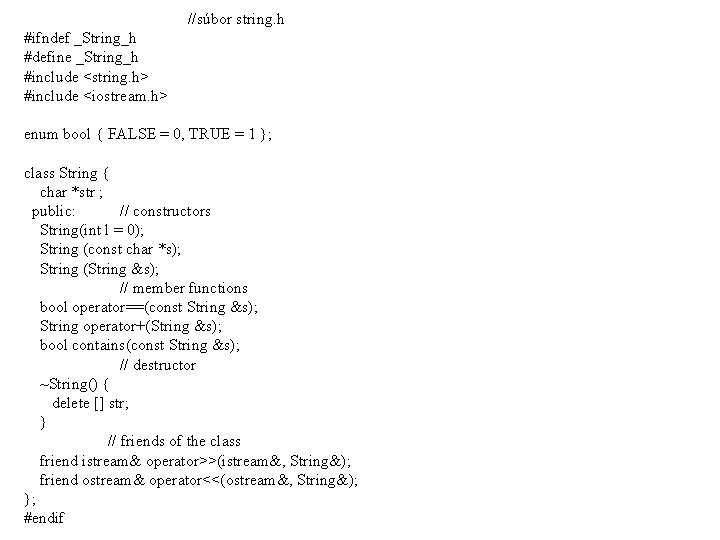
//súbor string. h #ifndef _String_h #define _String_h #include <string. h> #include <iostream. h> enum bool { FALSE = 0, TRUE = 1 }; class String { char *str ; public: // constructors String(int l = 0); String (const char *s); String (String &s); // member functions bool operator==(const String &s); String operator+(String &s); bool contains(const String &s); // destructor ~String() { delete [] str; } // friends of the class friend istream& operator>>(istream&, String&); friend ostream& operator<<(ostream&, String&); }; #endif
![include string h String Stringint l str new charl String #include "string. h" String: : String(int l ){ str = new char[l]; } String:](https://slidetodoc.com/presentation_image_h2/ca09cbb8f11293655f9a424fc2f8ebb2/image-52.jpg)
#include "string. h" String: : String(int l ){ str = new char[l]; } String: : String (const char *s){ int len = strlen(s); str = new char[len + 1]; strcpy(str, s); } String: : String (String &s){ int len = strlen(s. str); str = new char[len + 1]; strcpy(str, s. str); } // member functions bool String: : operator==(const String &s){ return (bool) !(strcmp(str, s. str)); } String: : operator+(String &s){ char *tmp = new char [strlen(str) + strlen(s. str) + 1]; strcpy(tmp, str); strcat(tmp, s. str); return *new String(tmp); }
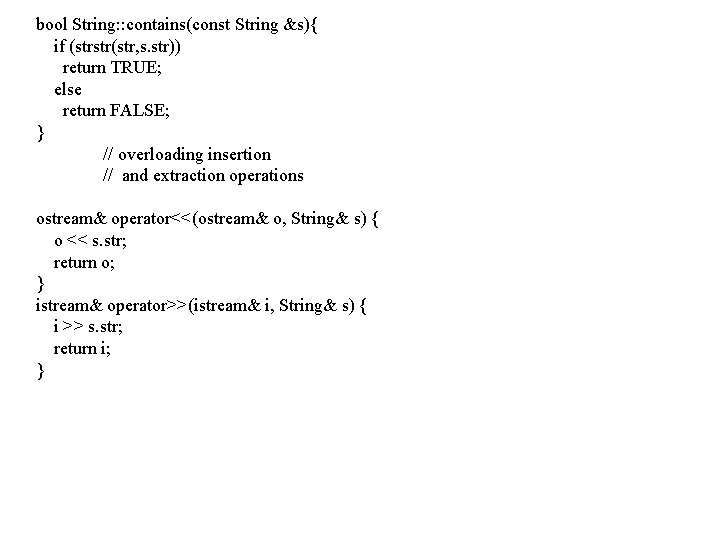
bool String: : contains(const String &s){ if (strstr(str, s. str)) return TRUE; else return FALSE; } // overloading insertion // and extraction operations ostream& operator<<(ostream& o, String& s) { o << s. str; return o; } istream& operator>>(istream& i, String& s) { i >> s. str; return i; }
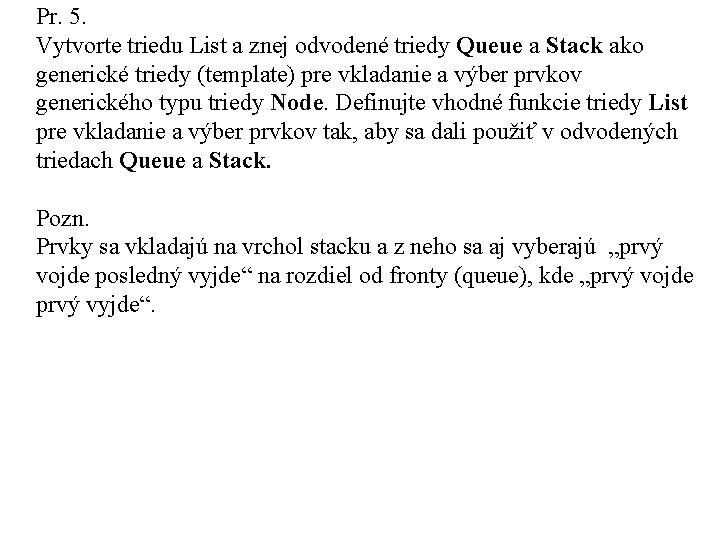
Pr. 5. Vytvorte triedu List a znej odvodené triedy Queue a Stack ako generické triedy (template) pre vkladanie a výber prvkov generického typu triedy Node. Definujte vhodné funkcie triedy List pre vkladanie a výber prvkov tak, aby sa dali použiť v odvodených triedach Queue a Stack. Pozn. Prvky sa vkladajú na vrchol stacku a z neho sa aj vyberajú „prvý vojde posledný vyjde“ na rozdiel od fronty (queue), kde „prvý vojde prvý vyjde“.
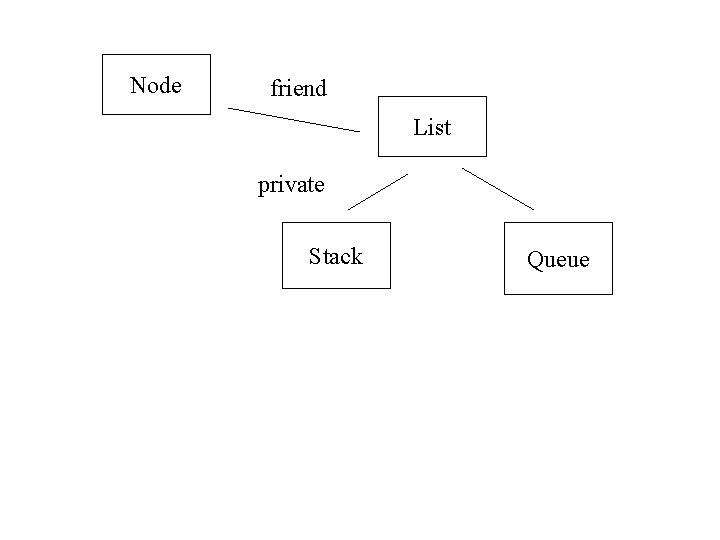
Node friend List private Stack Queue
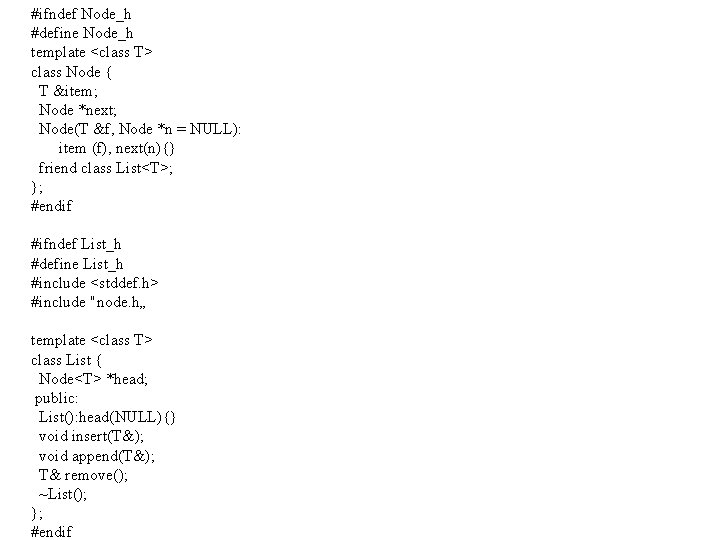
#ifndef Node_h #define Node_h template <class T> class Node { T &item; Node *next; Node(T &f, Node *n = NULL): item (f), next(n){} friend class List<T>; }; #endif #ifndef List_h #define List_h #include <stddef. h> #include "node. h„ template <class T> class List { Node<T> *head; public: List(): head(NULL){} void insert(T&); void append(T&); T& remove(); ~List(); }; #endif
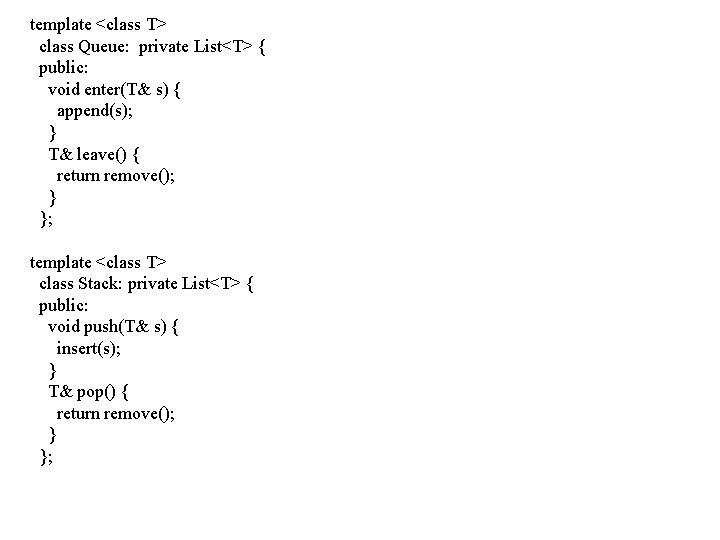
template <class T> class Queue: private List<T> { public: void enter(T& s) { append(s); } T& leave() { return remove(); } }; template <class T> class Stack: private List<T> { public: void push(T& s) { insert(s); } T& pop() { return remove(); } };
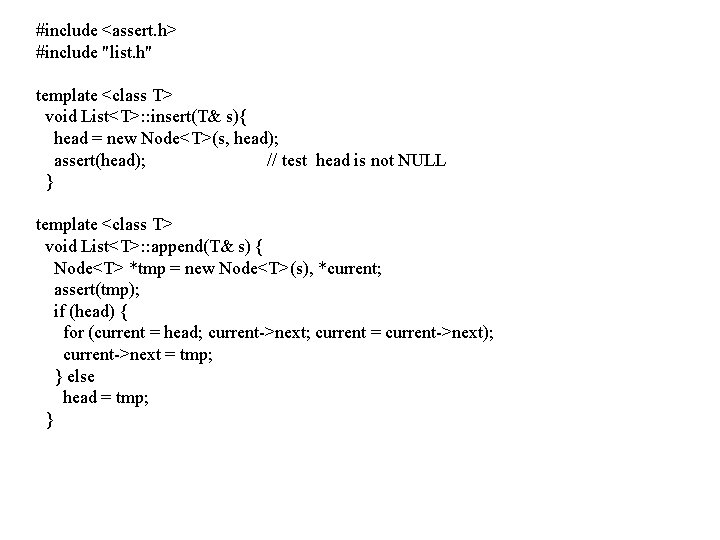
#include <assert. h> #include "list. h" template <class T> void List<T>: : insert(T& s){ head = new Node<T>(s, head); assert(head); // test head is not NULL } template <class T> void List<T>: : append(T& s) { Node<T> *tmp = new Node<T>(s), *current; assert(tmp); if (head) { for (current = head; current->next; current = current->next); current->next = tmp; } else head = tmp; }
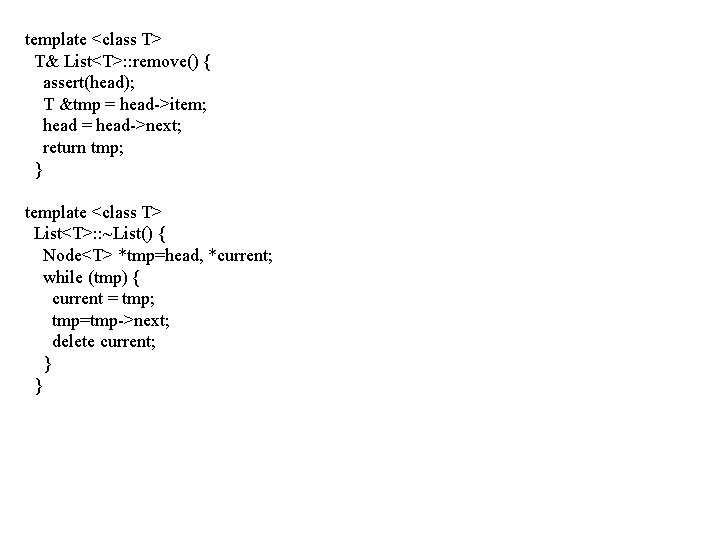
template <class T> T& List<T>: : remove() { assert(head); T &tmp = head->item; head = head->next; return tmp; } template <class T> List<T>: : ~List() { Node<T> *tmp=head, *current; while (tmp) { current = tmp; tmp=tmp->next; delete current; } }
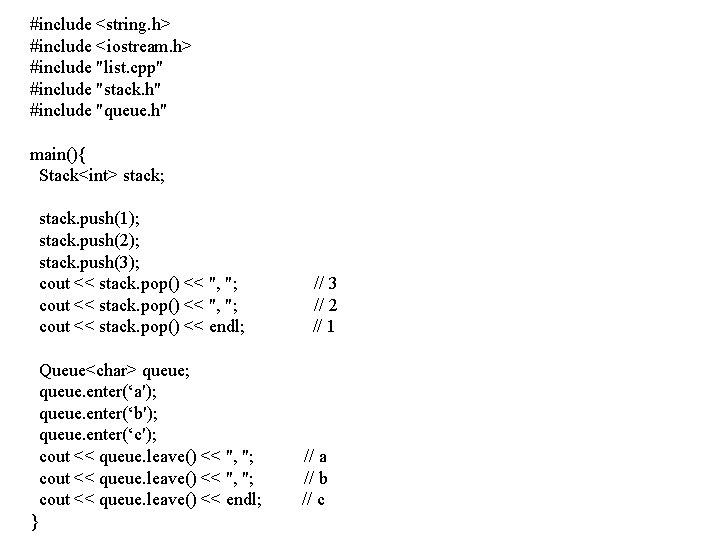
#include <string. h> #include <iostream. h> #include "list. cpp" #include "stack. h" #include "queue. h" main(){ Stack<int> stack; stack. push(1); stack. push(2); stack. push(3); cout << stack. pop() << ", "; cout << stack. pop() << endl; Queue<char> queue; queue. enter(‘a'); queue. enter(‘b'); queue. enter(‘c'); cout << queue. leave() << ", "; cout << queue. leave() << endl; } // 3 // 2 // 1 // a // b // c
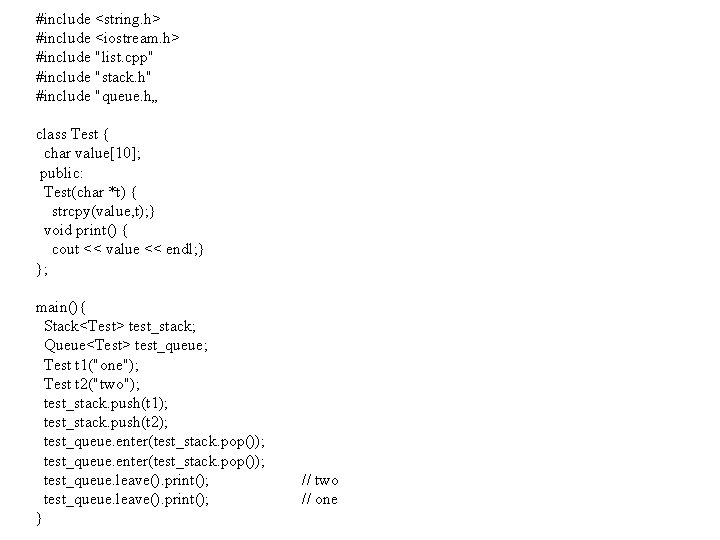
#include <string. h> #include <iostream. h> #include "list. cpp" #include "stack. h" #include "queue. h„ class Test { char value[10]; public: Test(char *t) { strcpy(value, t); } void print() { cout << value << endl; } }; main(){ Stack<Test> test_stack; Queue<Test> test_queue; Test t 1("one"); Test t 2("two"); test_stack. push(t 1); test_stack. push(t 2); test_queue. enter(test_stack. pop()); test_queue. leave(). print(); } // two // one
Gaussovo rozdelenie
Graf nepriamej úmernosti
Hustota zlata g/cm3
Prklad
Prklad
Prklada
Prklad
Elephant poem
Propaganda definition
The old man tried to stop
Why shouldn't juveniles be tried as adults
I tried poems
Tried people
Played tried smiled wanted
The old man tried to stop
I'll be a friend to jesus
Complaints were made by teachers and administrators also
Ever tried ever failed no matter
Jamie tried to solve an equation step by step.
Unpack 3. hali
Gabe tried to use the law of cosines
Helping verb
Pune, india, has tried to increase its rainfall by
Supporting areas of maxilla
Was caused by breathing impure
The old man tried to stop
Who tried to warn caesar
Mr tongue twister tried to
In a hurricane the eye wall represents