Polymorphism Polymorphism An Introduction q One interface multiple
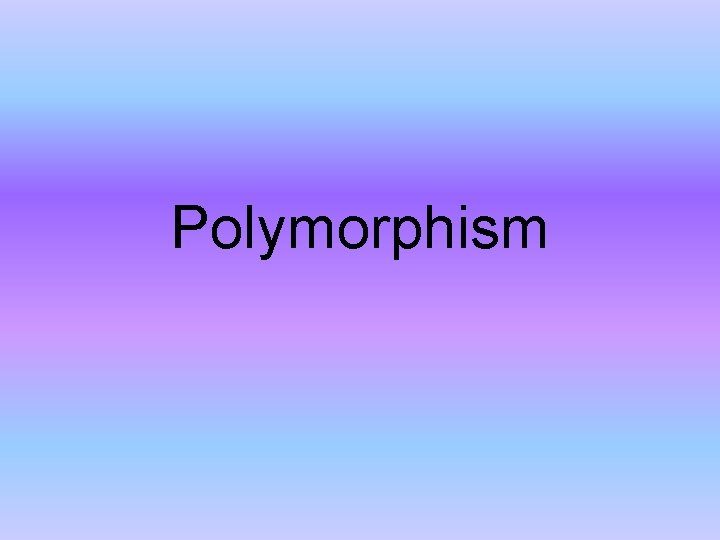
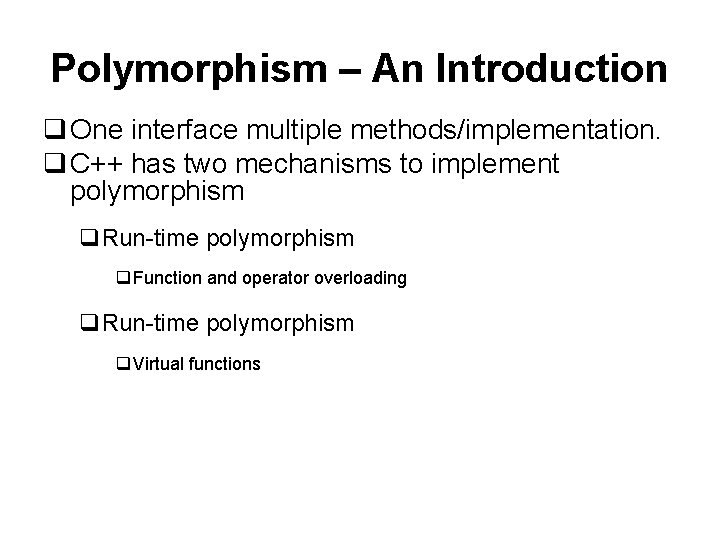
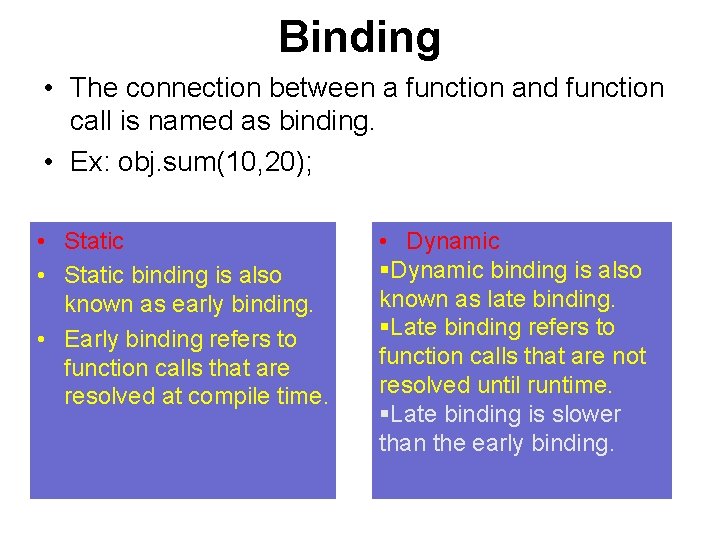
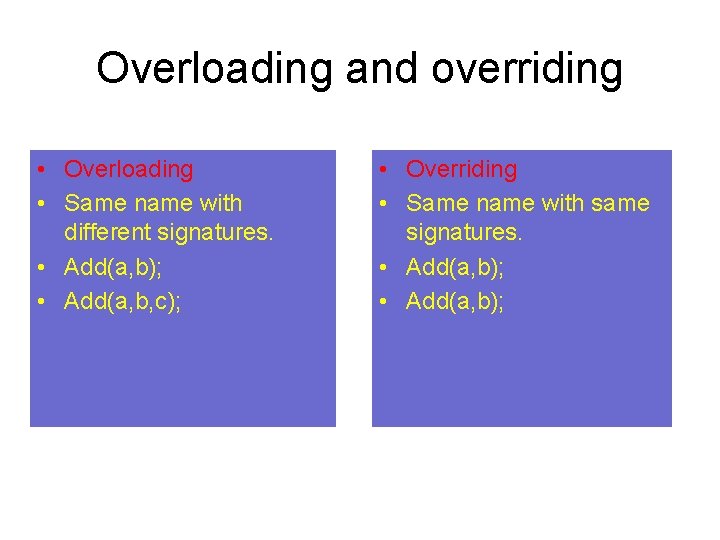
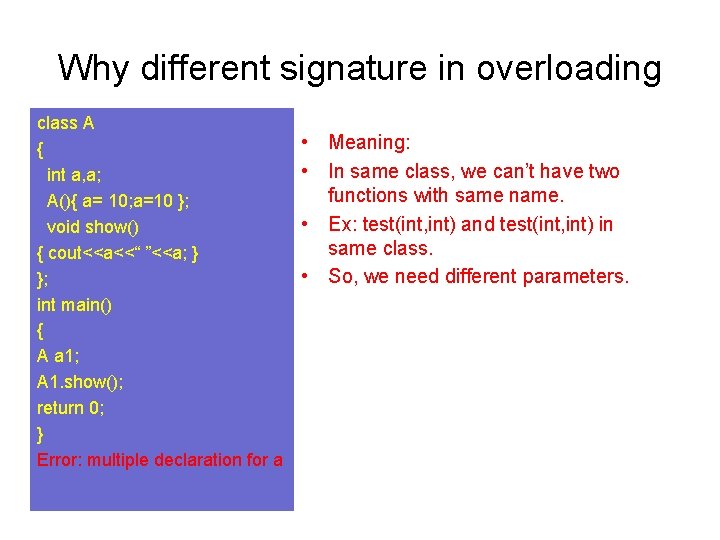
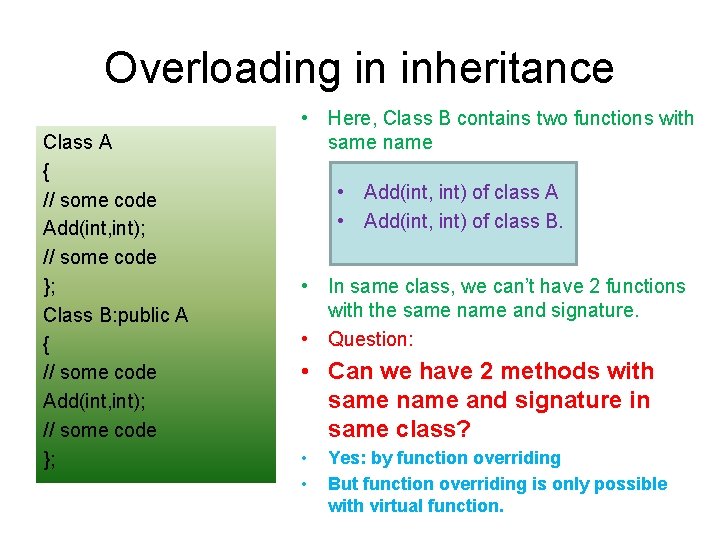
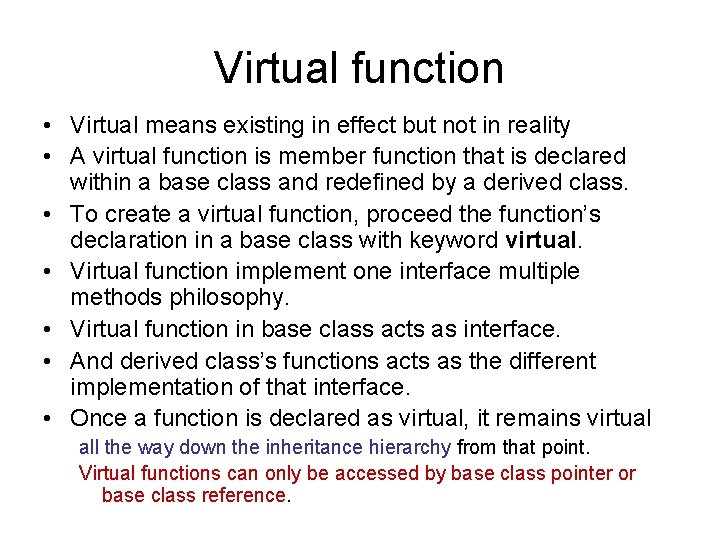
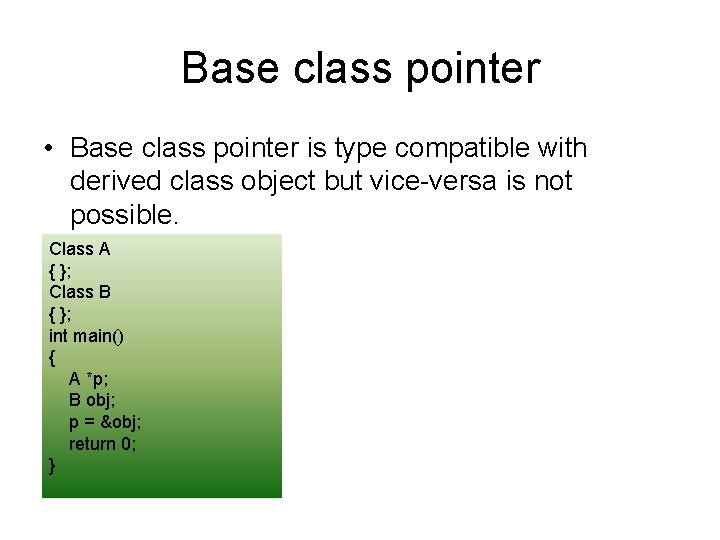
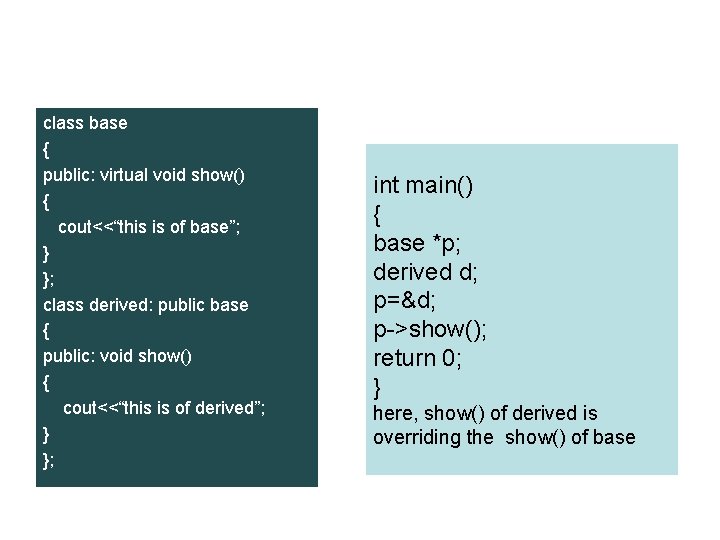
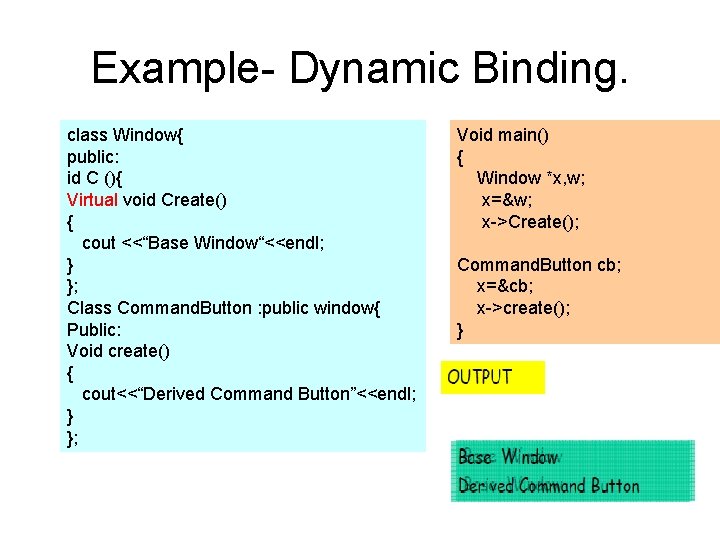
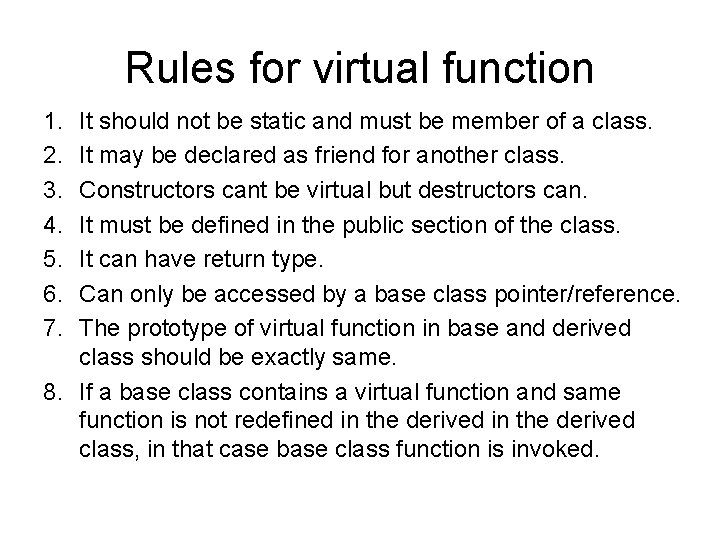
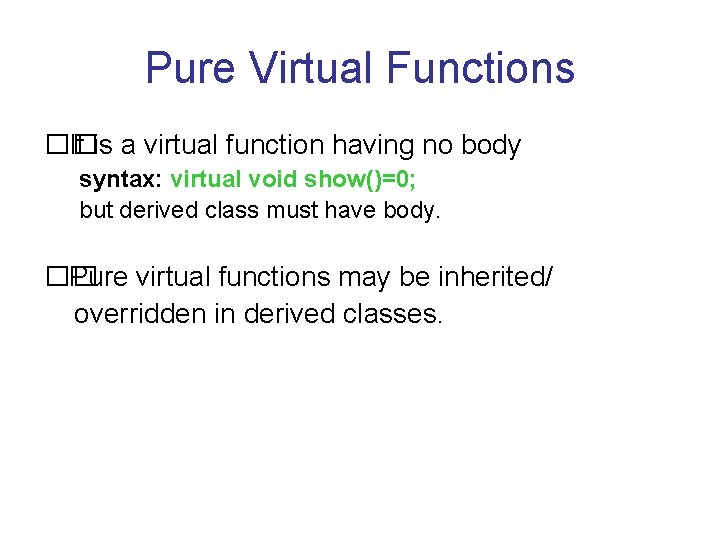
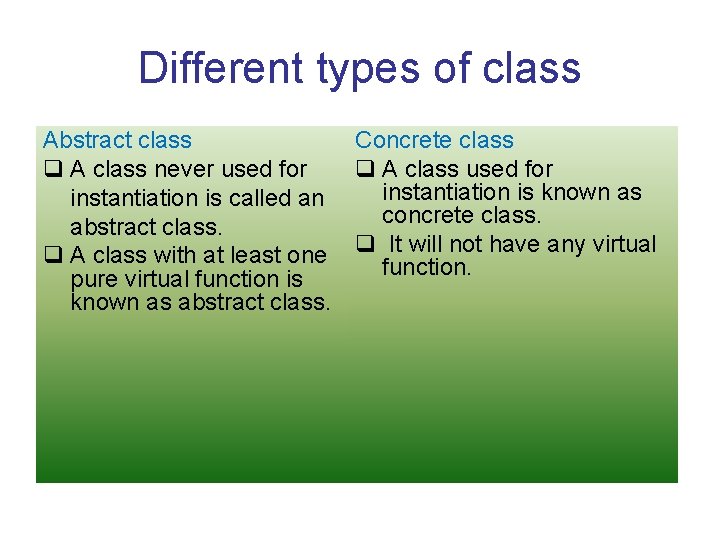
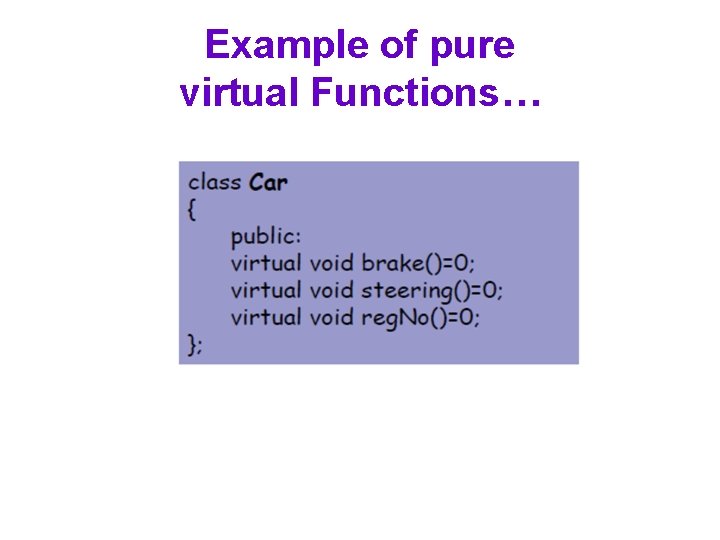
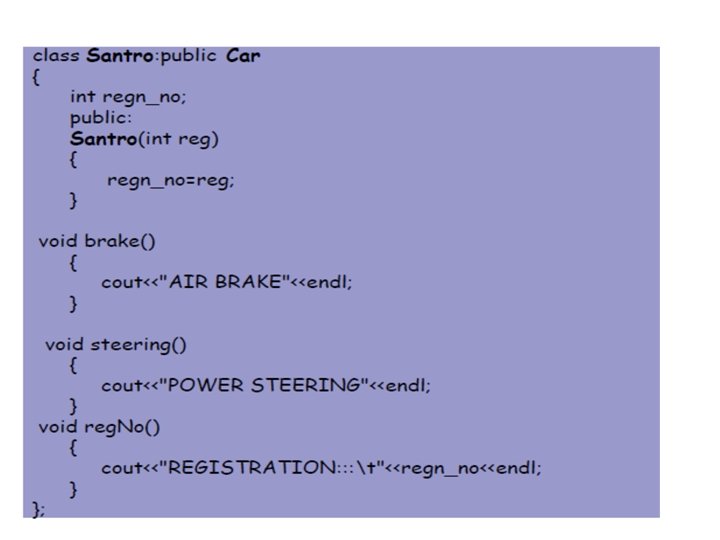
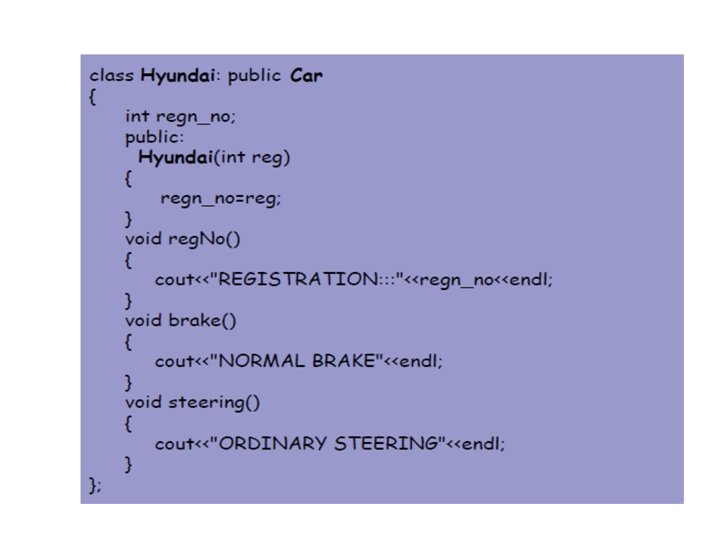
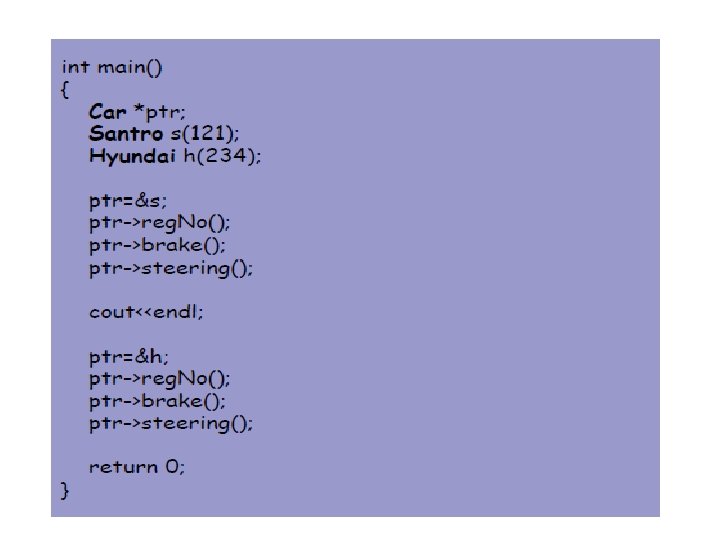
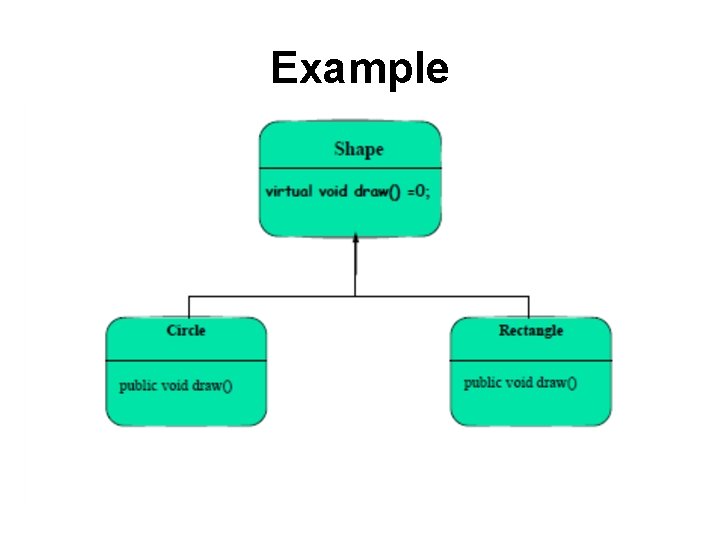
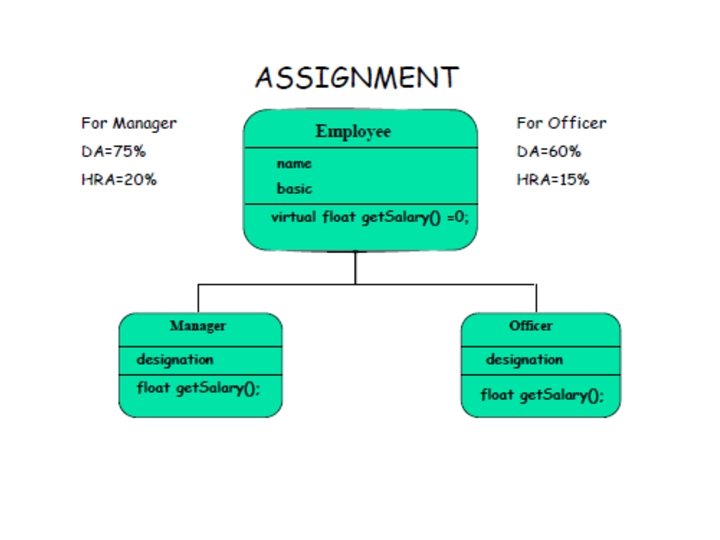
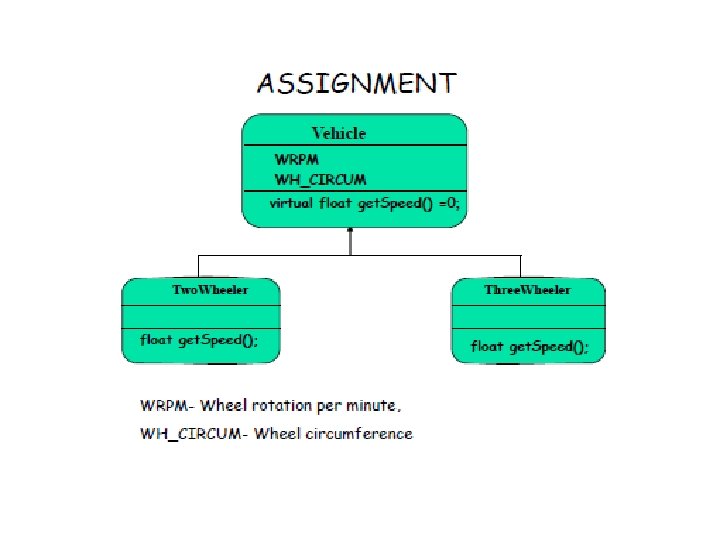
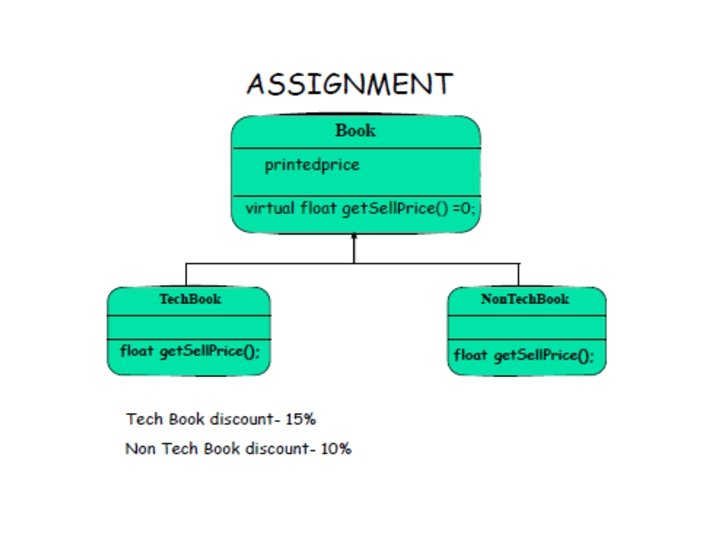
- Slides: 21
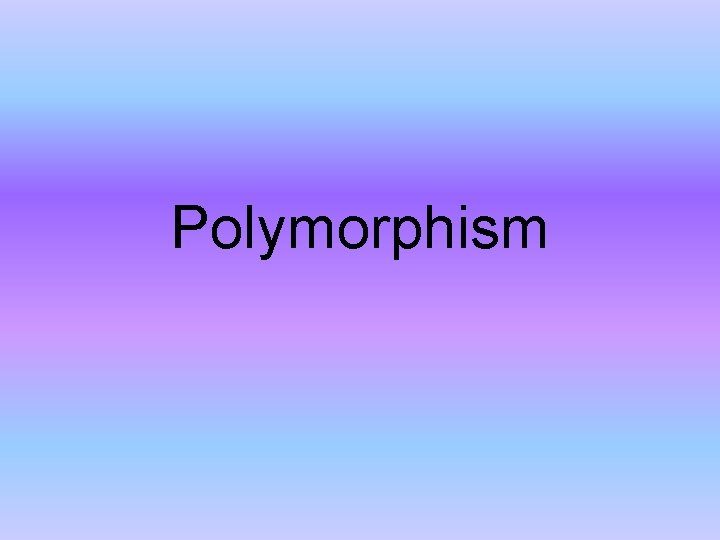
Polymorphism
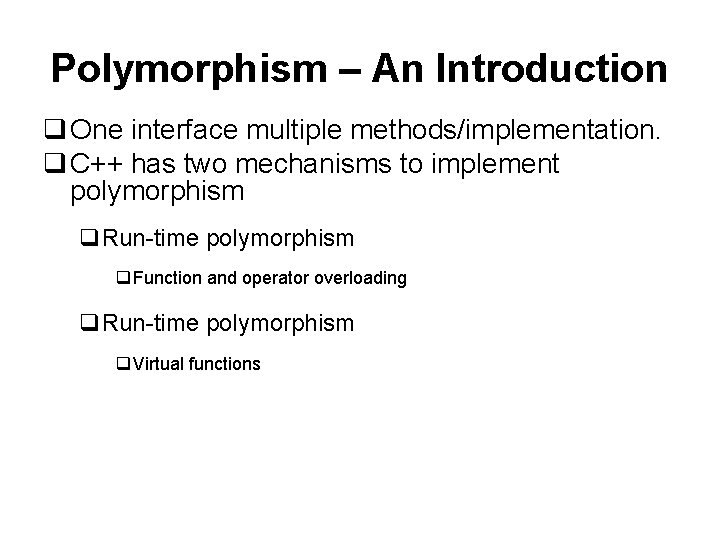
Polymorphism – An Introduction q One interface multiple methods/implementation. q C++ has two mechanisms to implement polymorphism q. Run-time polymorphism q Function and operator overloading q. Run-time polymorphism q Virtual functions
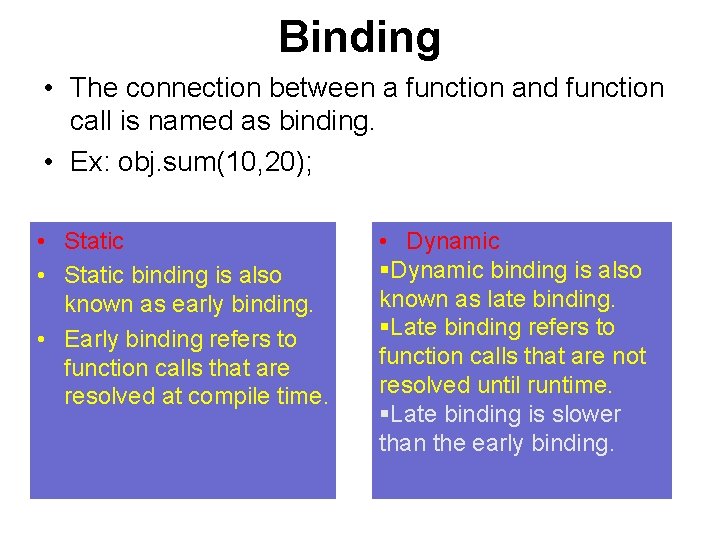
Binding • The connection between a function and function call is named as binding. • Ex: obj. sum(10, 20); • Static binding is also known as early binding. • Early binding refers to function calls that are resolved at compile time. • Dynamic §Dynamic binding is also known as late binding. §Late binding refers to function calls that are not resolved until runtime. §Late binding is slower than the early binding.
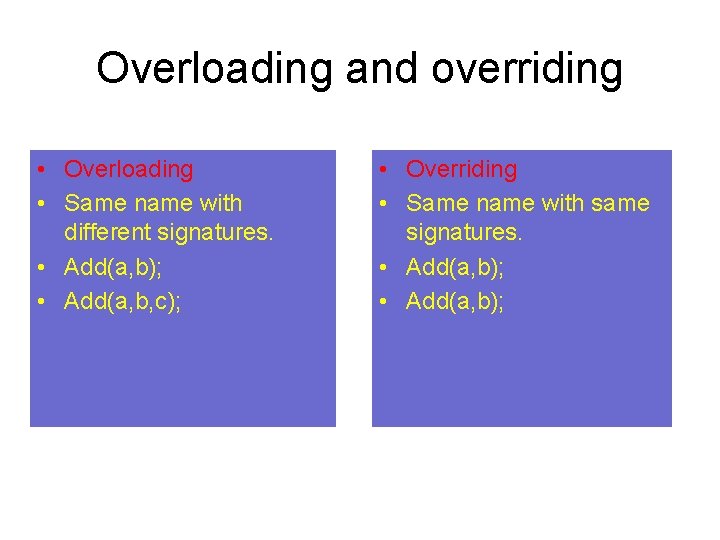
Overloading and overriding • Overloading • Same name with different signatures. • Add(a, b); • Add(a, b, c); • Overriding • Same name with same signatures. • Add(a, b);
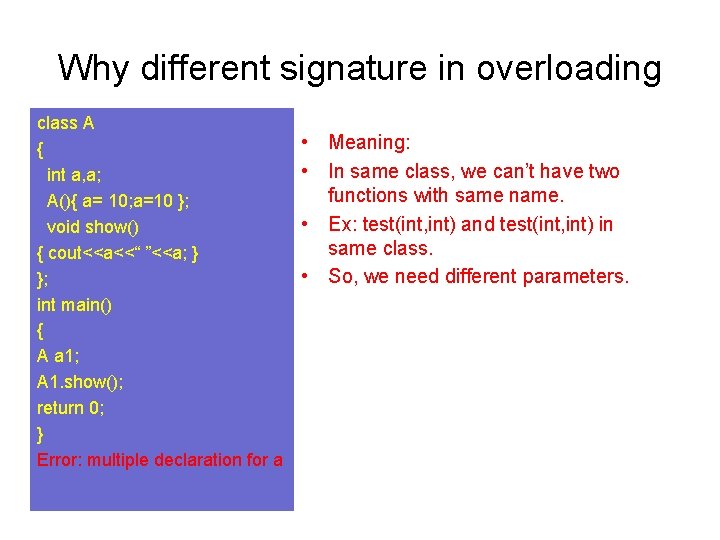
Why different signature in overloading class A { int a, a; A(){ a= 10; a=10 }; void show() { cout<<a<<“ ”<<a; } }; int main() { A a 1; A 1. show(); return 0; } Error: multiple declaration for a • Meaning: • In same class, we can’t have two functions with same name. • Ex: test(int, int) and test(int, int) in same class. • So, we need different parameters.
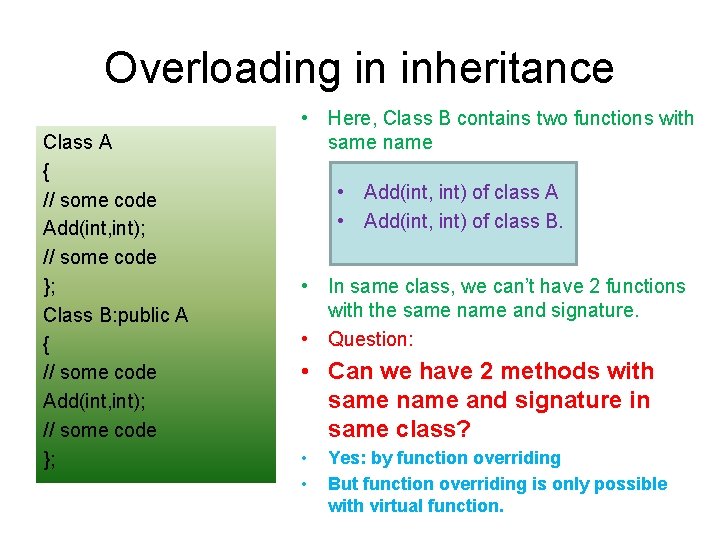
Overloading in inheritance Class A { // some code Add(int, int); // some code }; Class B: public A { // some code Add(int, int); // some code }; • Here, Class B contains two functions with same name • Add(int, int) of class A • Add(int, int) of class B. • In same class, we can’t have 2 functions with the same name and signature. • Question: • Can we have 2 methods with same name and signature in same class? • • Yes: by function overriding But function overriding is only possible with virtual function.
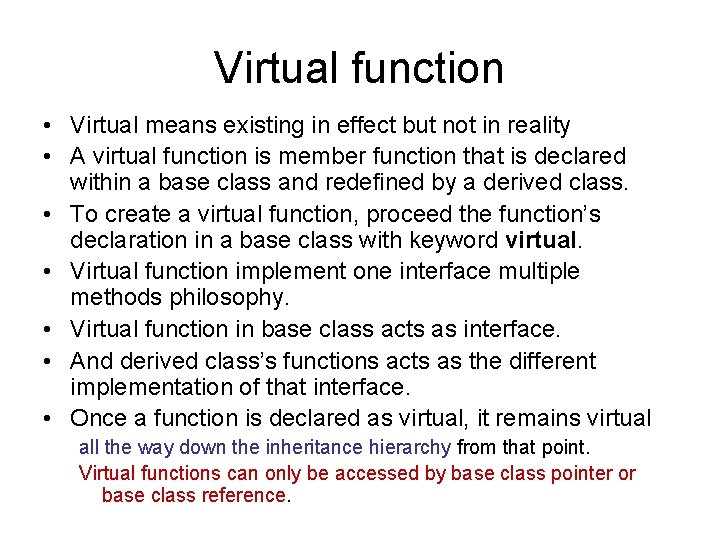
Virtual function • Virtual means existing in effect but not in reality • A virtual function is member function that is declared within a base class and redefined by a derived class. • To create a virtual function, proceed the function’s declaration in a base class with keyword virtual. • Virtual function implement one interface multiple methods philosophy. • Virtual function in base class acts as interface. • And derived class’s functions acts as the different implementation of that interface. • Once a function is declared as virtual, it remains virtual all the way down the inheritance hierarchy from that point. Virtual functions can only be accessed by base class pointer or base class reference.
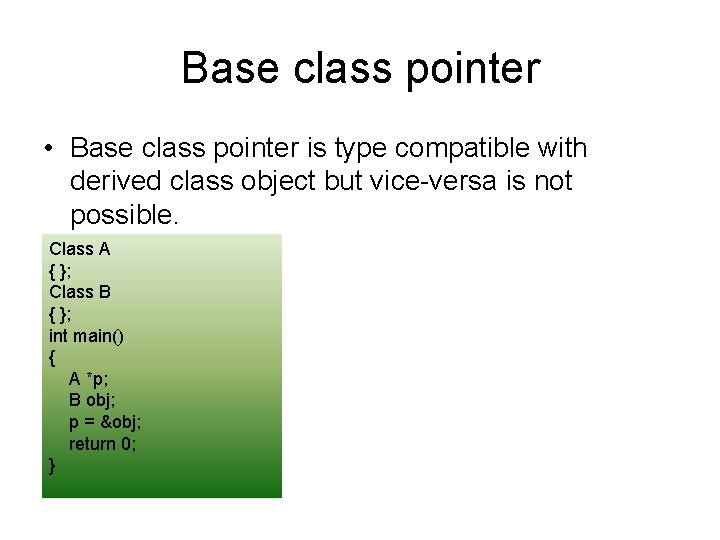
Base class pointer • Base class pointer is type compatible with derived class object but vice-versa is not possible. Class A { }; Class B { }; int main() { A *p; B obj; p = &obj; return 0; }
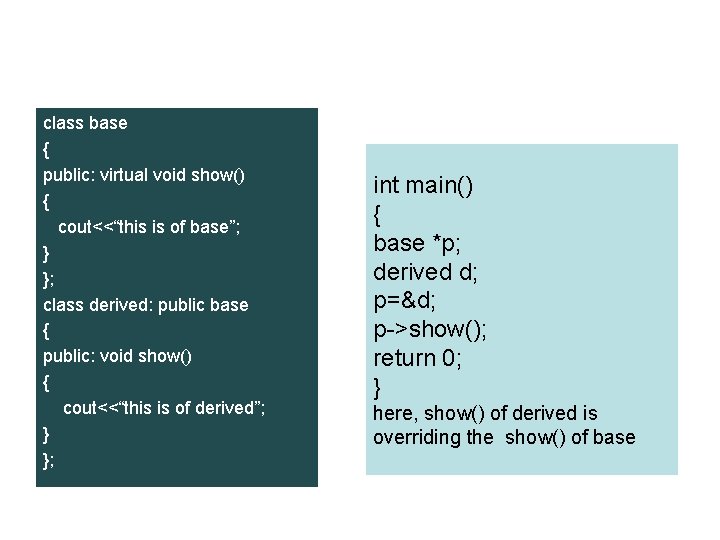
class base { public: virtual void show() { cout<<“this is of base”; } }; class derived: public base { public: void show() { cout<<“this is of derived”; } }; int main() { base *p; derived d; p=&d; p->show(); return 0; } here, show() of derived is overriding the show() of base
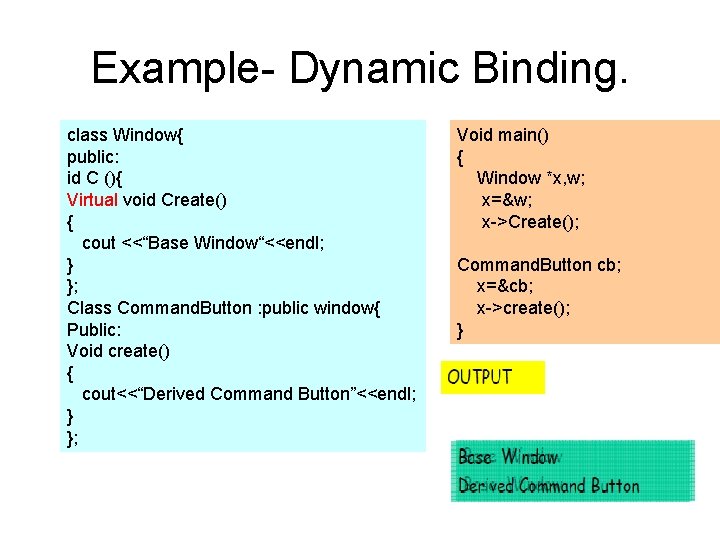
Example- Dynamic Binding. class Window{ public: id C (){ Virtual void Create() { cout <<“Base Window“<<endl; } }; Class Command. Button : public window{ Public: Void create() { cout<<“Derived Command Button”<<endl; } }; Void main() { Window *x, w; x=&w; x->Create(); Command. Button cb; x=&cb; x->create(); }
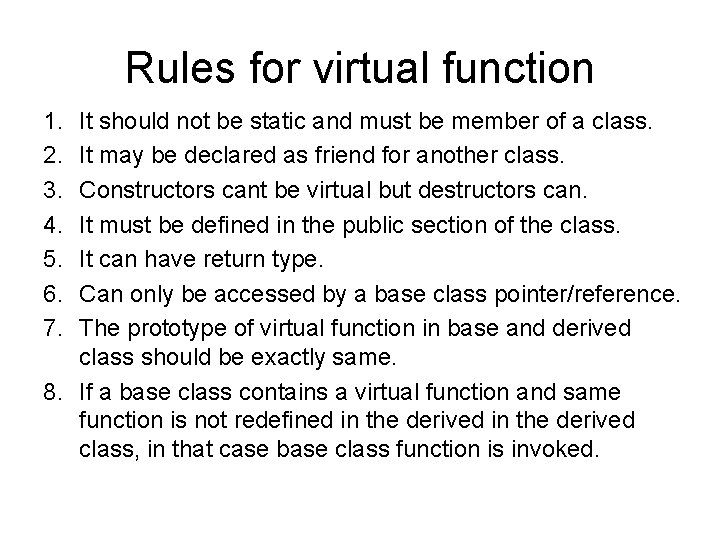
Rules for virtual function 1. 2. 3. 4. 5. 6. 7. It should not be static and must be member of a class. It may be declared as friend for another class. Constructors cant be virtual but destructors can. It must be defined in the public section of the class. It can have return type. Can only be accessed by a base class pointer/reference. The prototype of virtual function in base and derived class should be exactly same. 8. If a base class contains a virtual function and same function is not redefined in the derived class, in that case base class function is invoked.
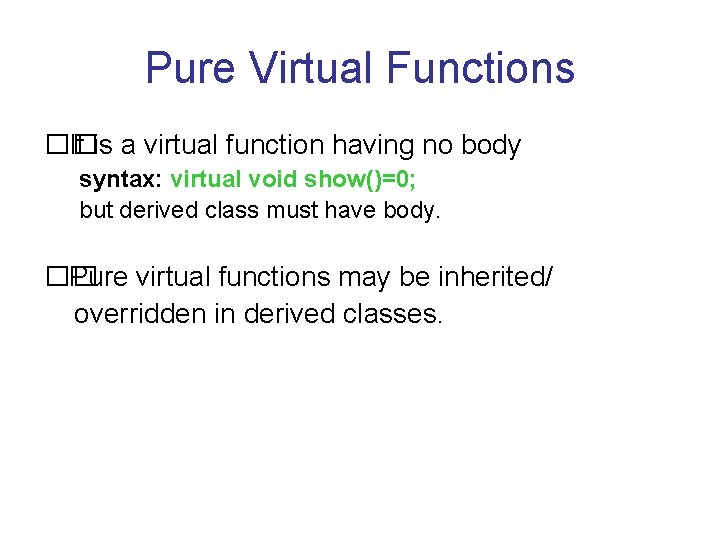
Pure Virtual Functions �� It is a virtual function having no body syntax: virtual void show()=0; but derived class must have body. �� Pure virtual functions may be inherited/ overridden in derived classes.
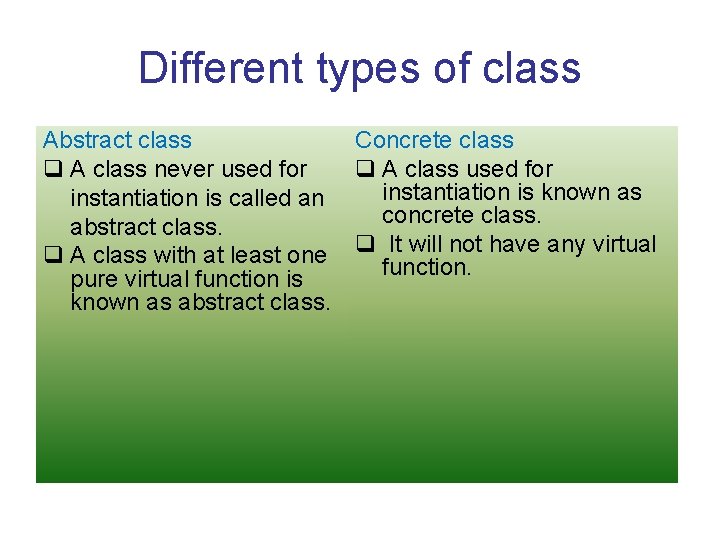
Different types of class Abstract class Concrete class q A class never used for q A class used for instantiation is known as instantiation is called an concrete class. abstract class. It will not have any virtual q A class with at least one q function. pure virtual function is known as abstract class.
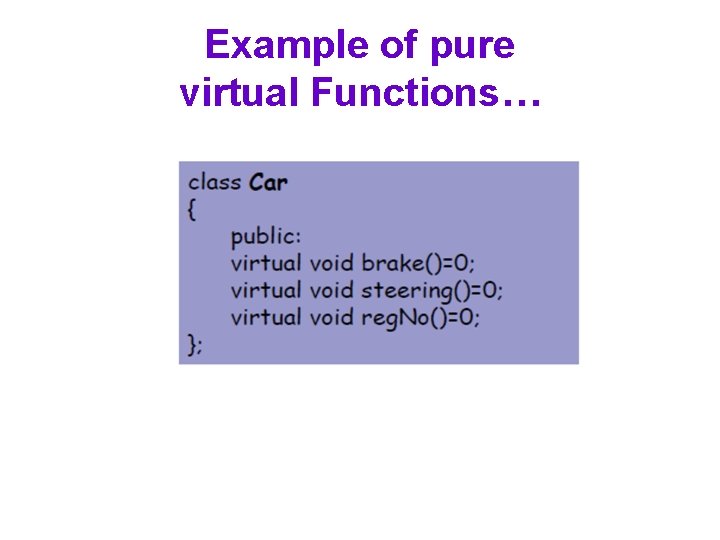
Example of pure virtual Functions…
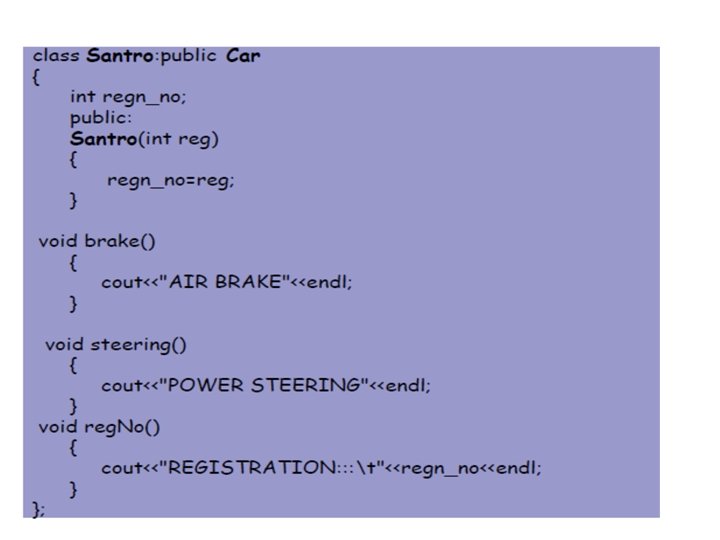
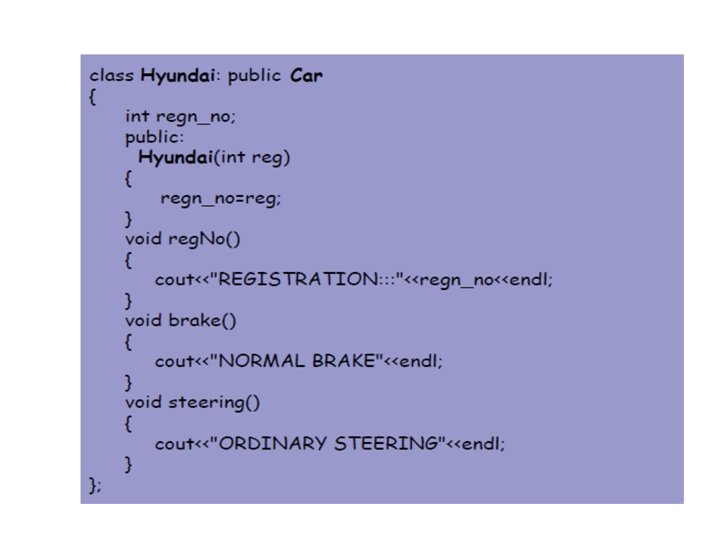
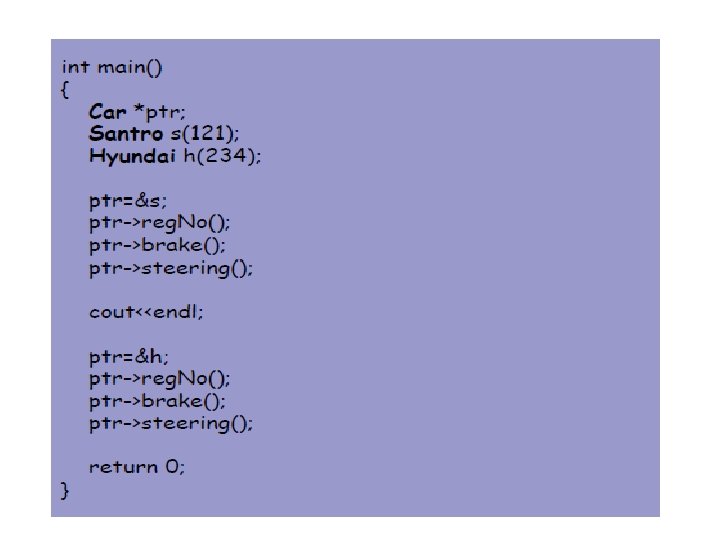
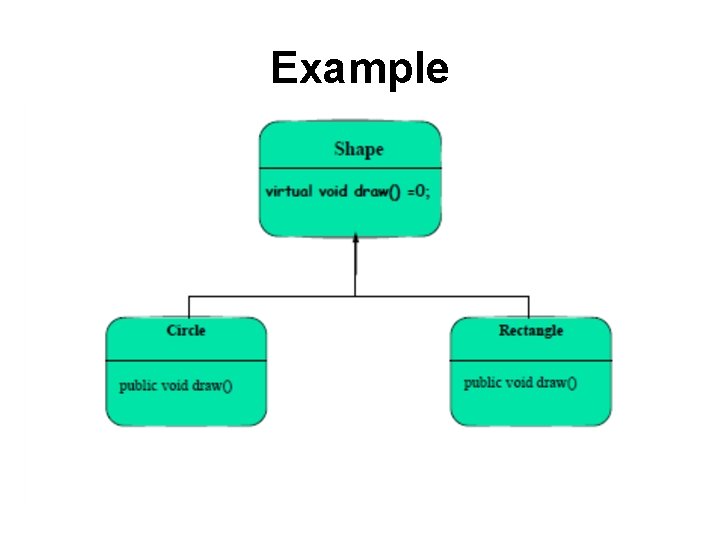
Example
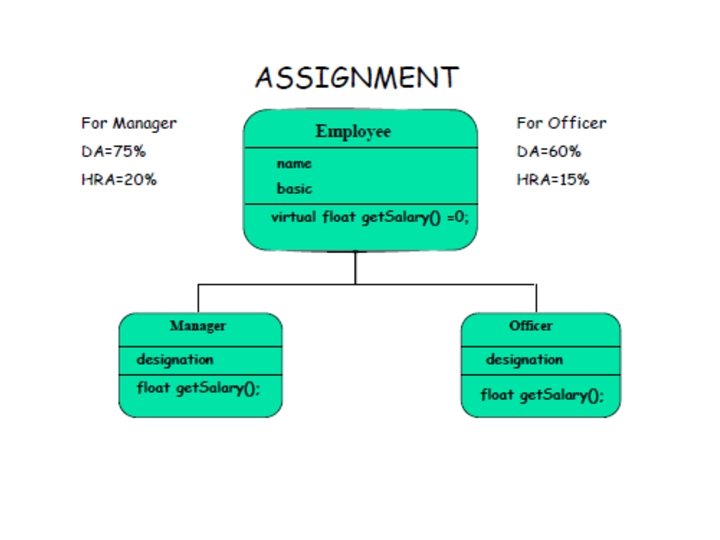
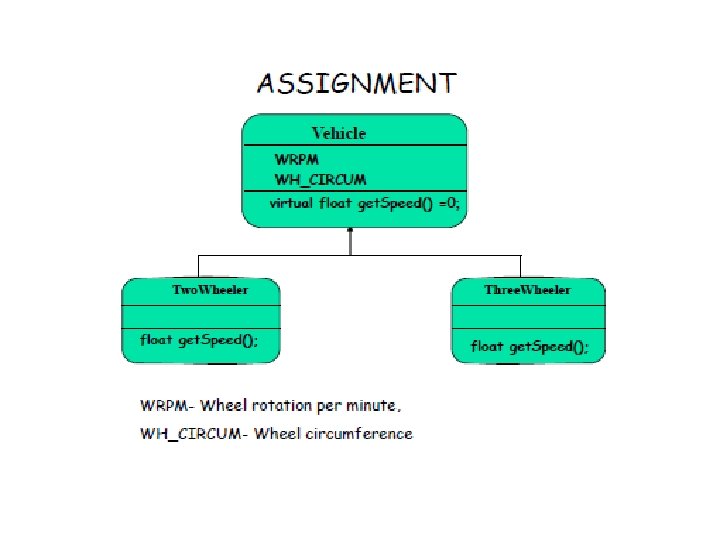
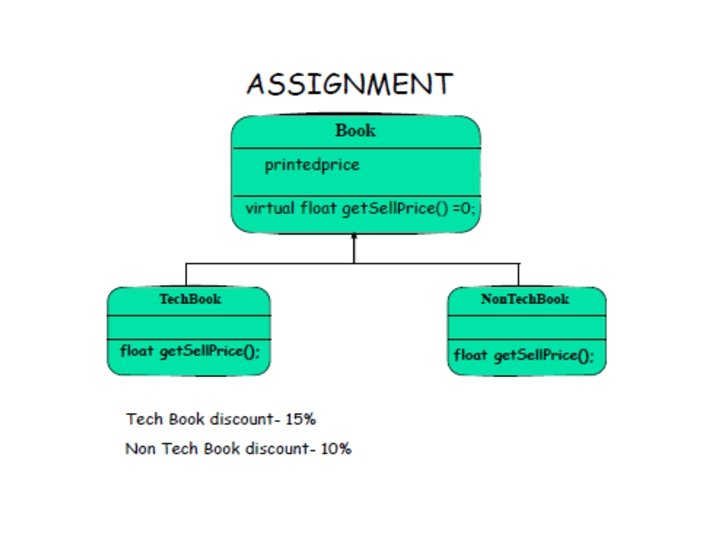
Java interface polymorphism
What is interface in java
User led through interaction via series of questions
Office interface vs industrial interface
Interface------------ an interface *
Multiple document interface
Multiple-document interface
Delayed multiple baseline design
Shared memory mimd architecture
One god one empire one emperor
One one one little puppy run
One king one law one faith
Byzantine definition
One ford
See one do one teach one
See one, do one, teach one
Twelfth night speeches
See one do one teach one
Asean tourism strategic plan
Graphic organizer with the aims of la liga filipina
Function of graphical user interface
Control word format of 8255