Performance Analysis Space complexity Instance characteristics Time complexity
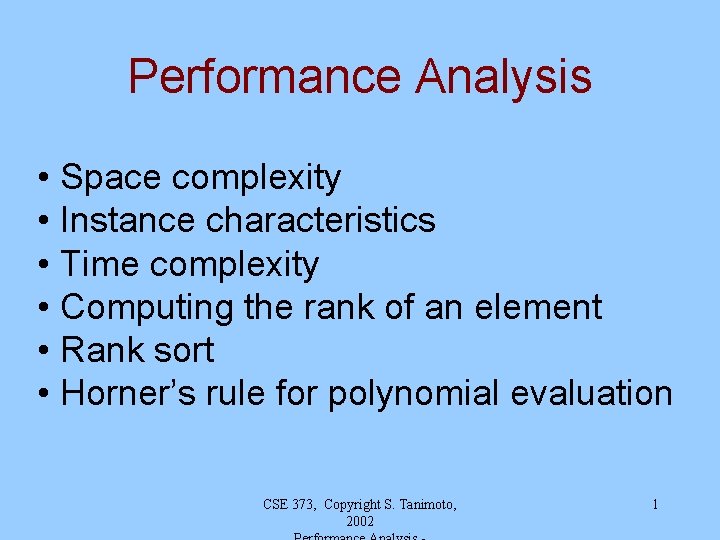
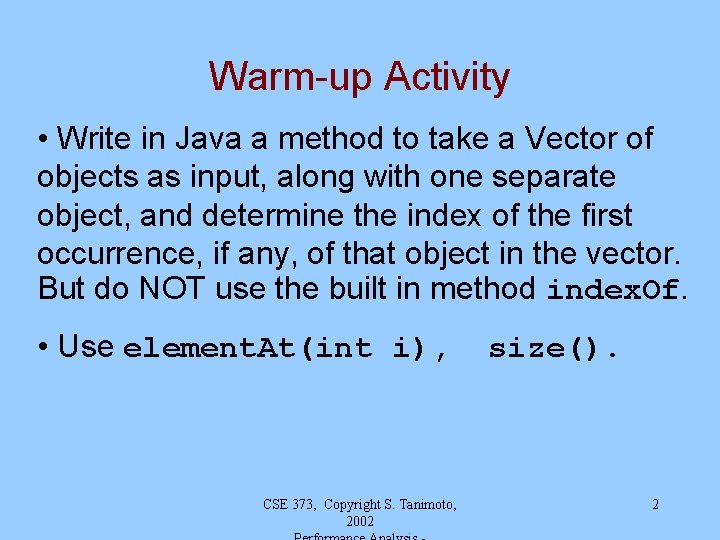
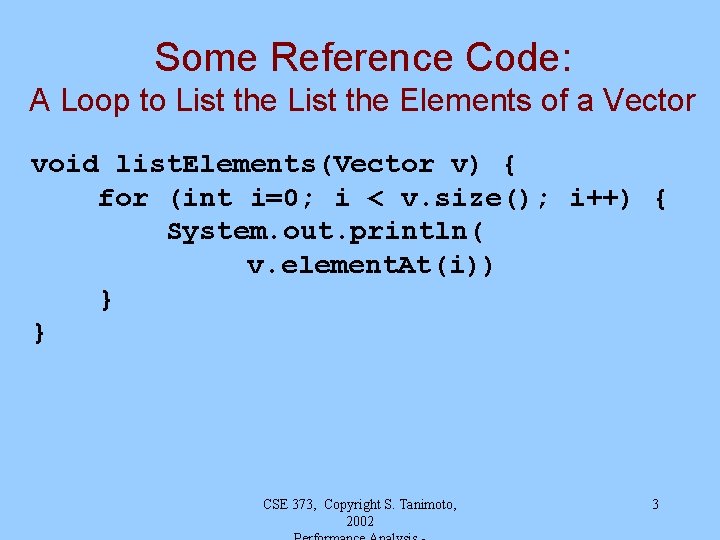
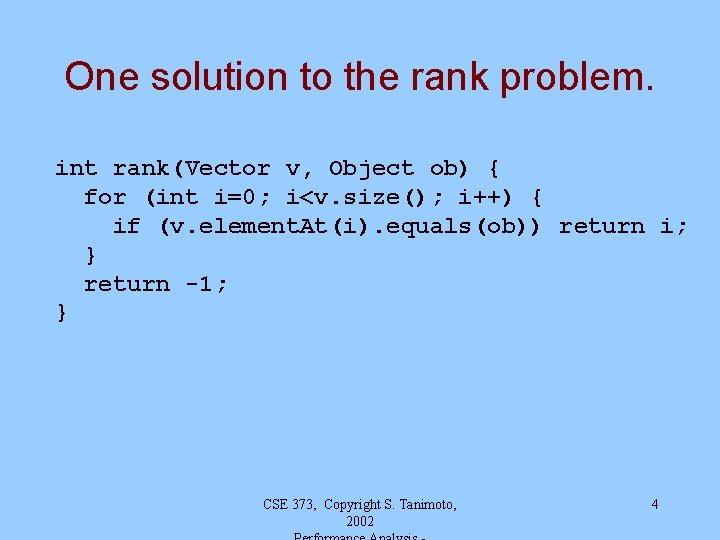
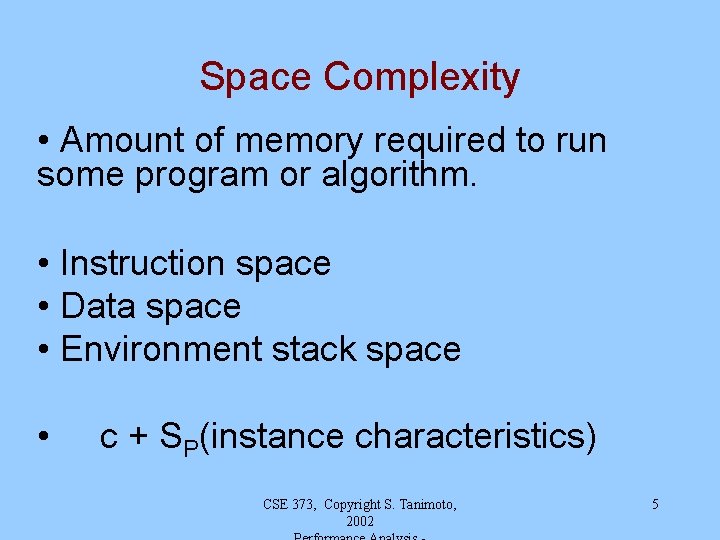
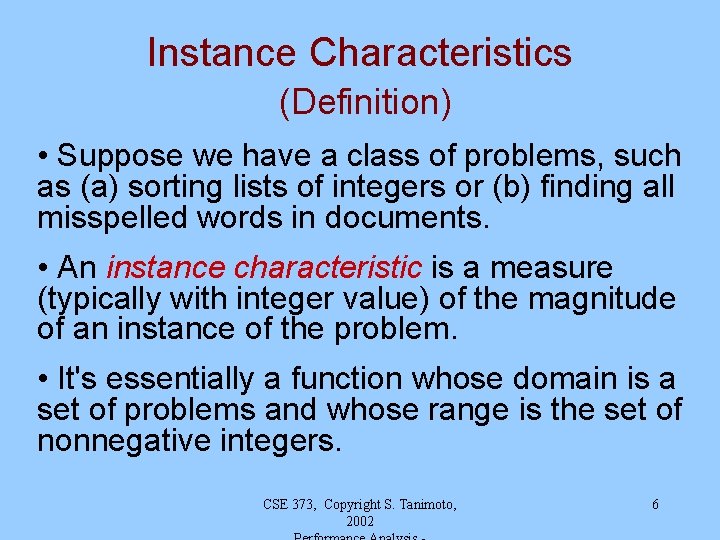
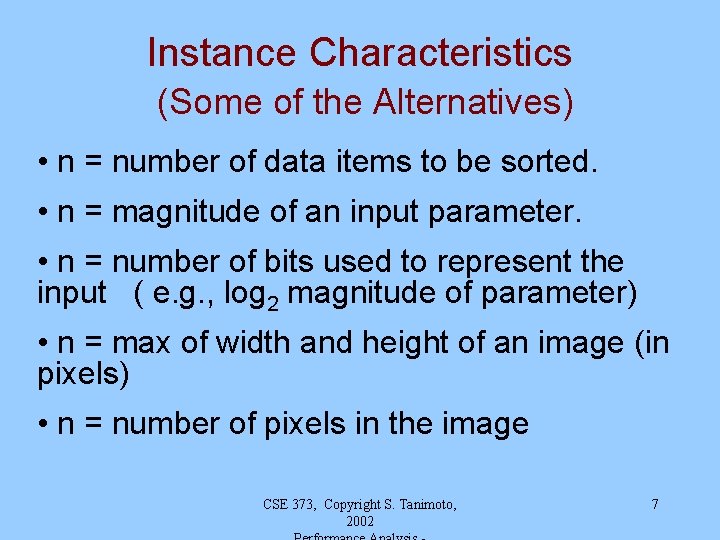
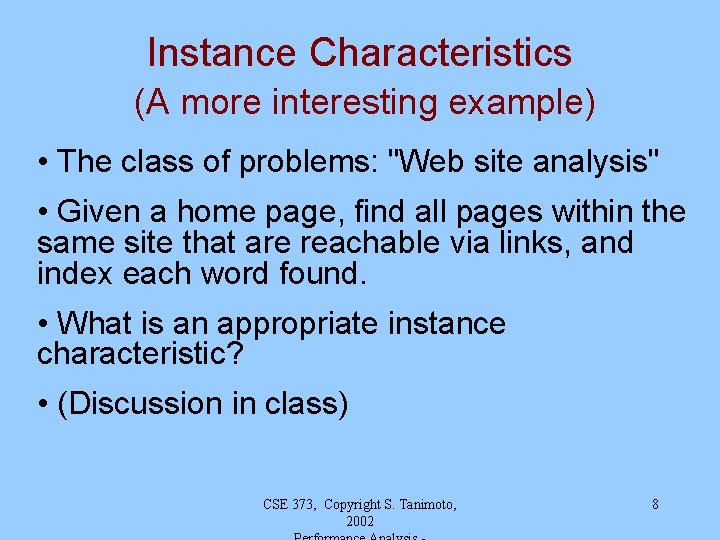
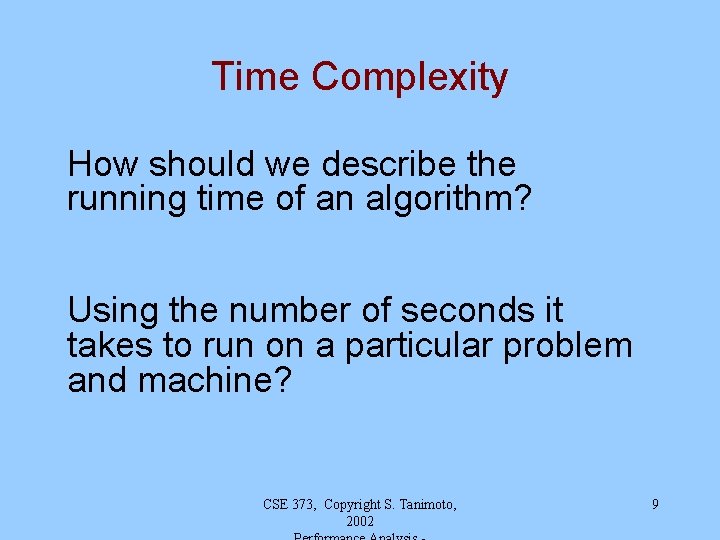
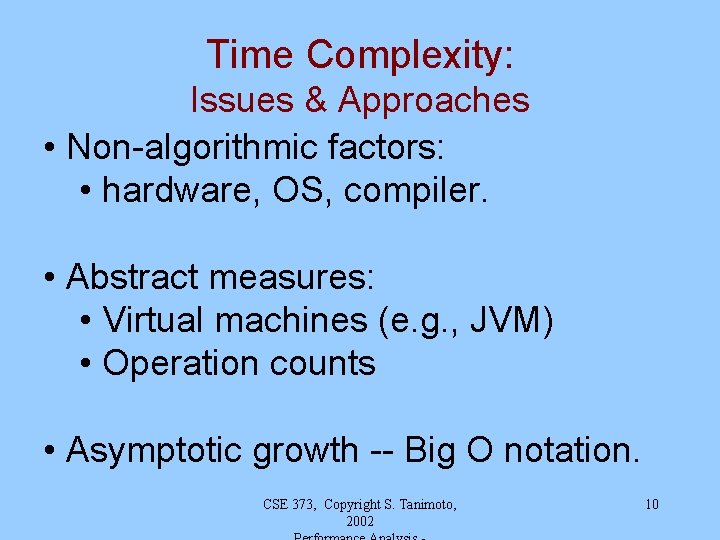
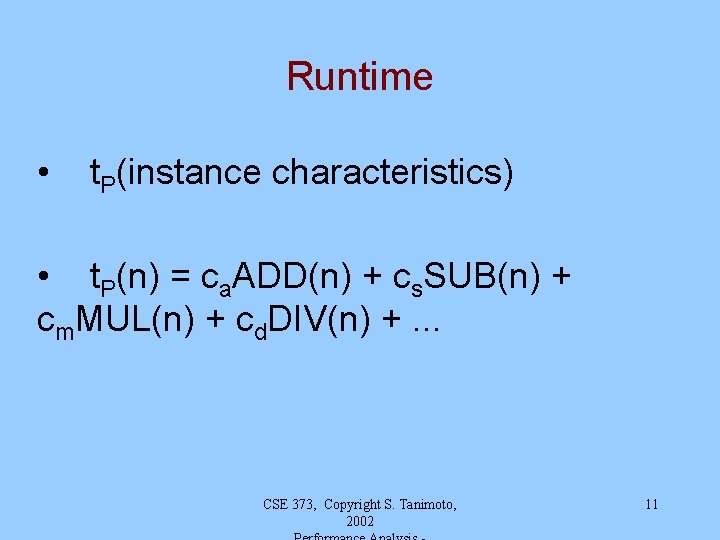
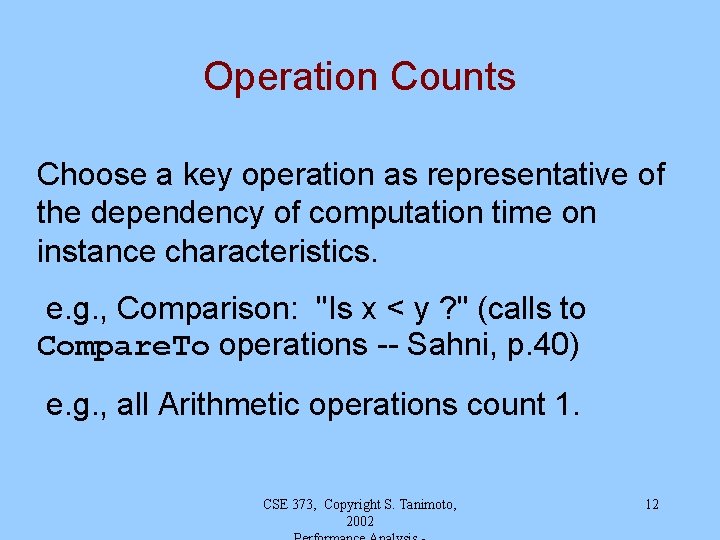
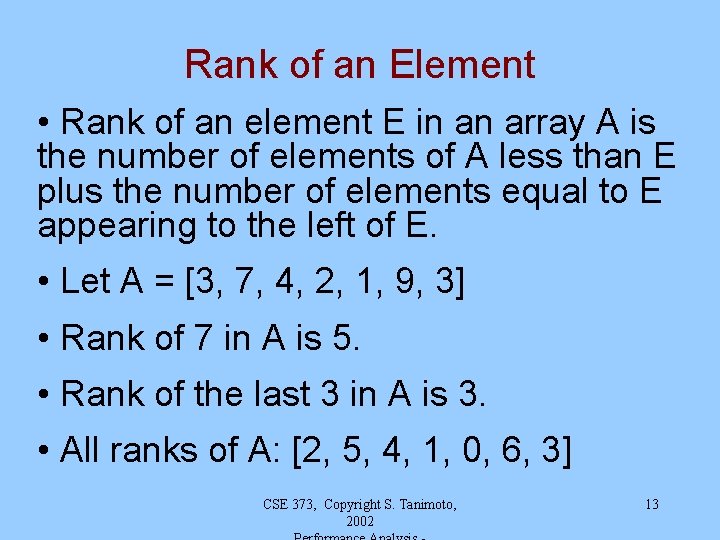
![Computing the Ranks public static void rank(Comparable [] a, int [] r) { if Computing the Ranks public static void rank(Comparable [] a, int [] r) { if](https://slidetodoc.com/presentation_image_h2/1cec4aa7ab4c586fcade097a6fda4dcb/image-14.jpg)
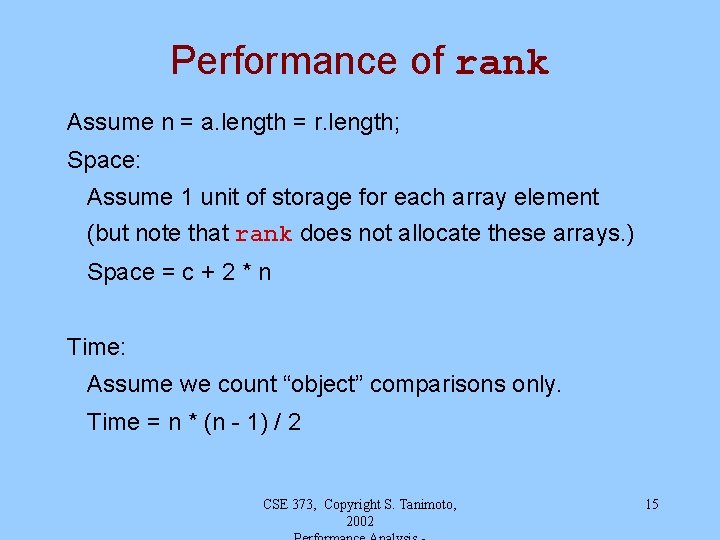
![Rearranging public static void rearrange(Comparable [] a, int [] r) { Comparable [] u Rearranging public static void rearrange(Comparable [] a, int [] r) { Comparable [] u](https://slidetodoc.com/presentation_image_h2/1cec4aa7ab4c586fcade097a6fda4dcb/image-16.jpg)
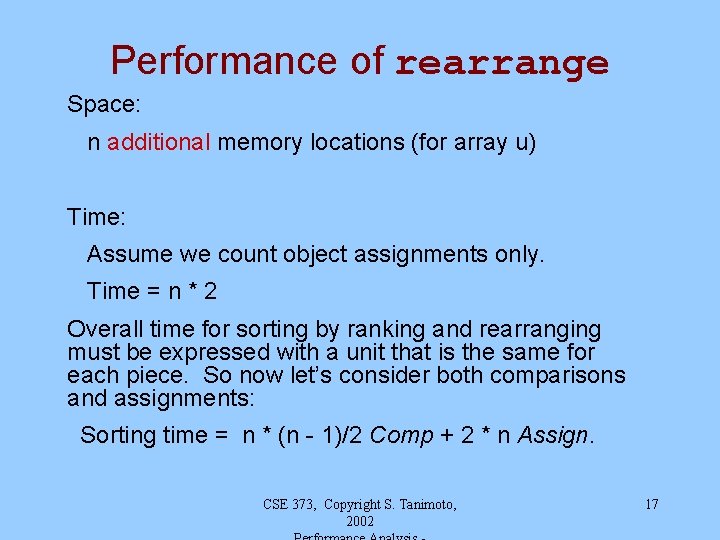
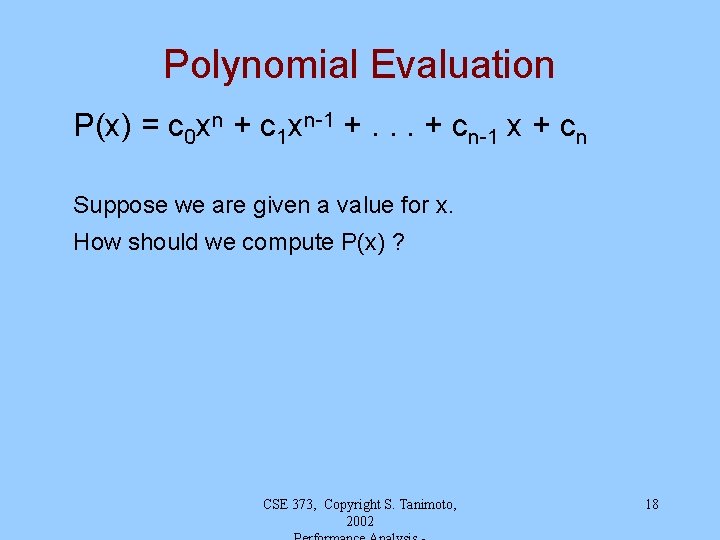
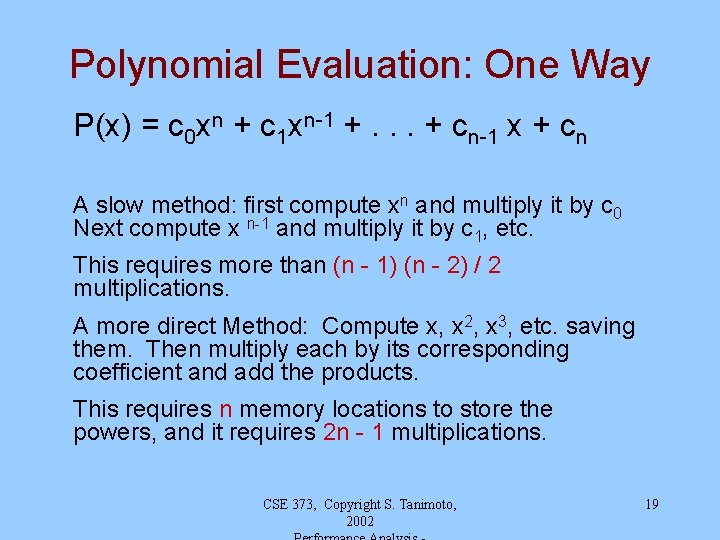
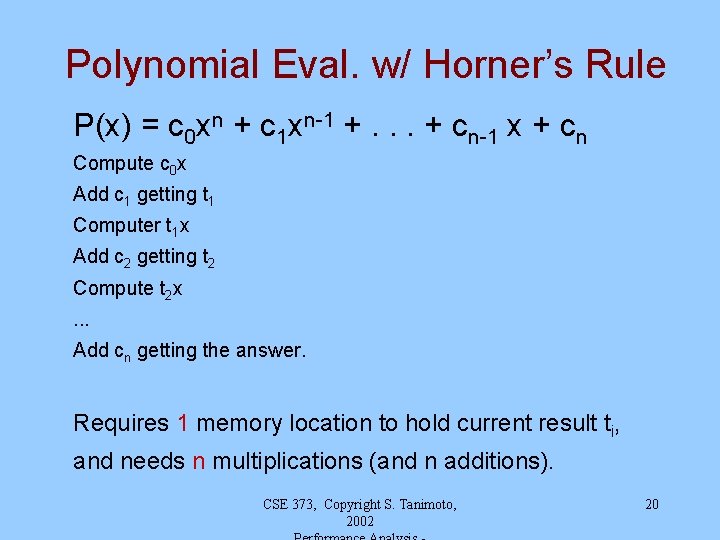
- Slides: 20
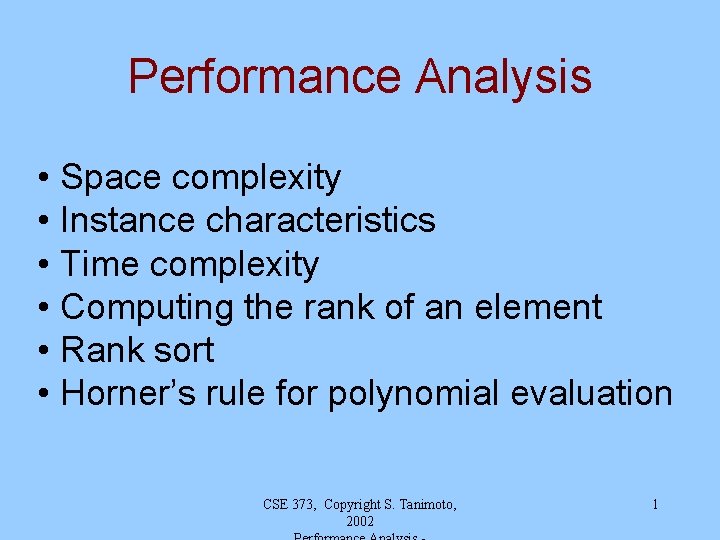
Performance Analysis • Space complexity • Instance characteristics • Time complexity • Computing the rank of an element • Rank sort • Horner’s rule for polynomial evaluation CSE 373, Copyright S. Tanimoto, 2002 1
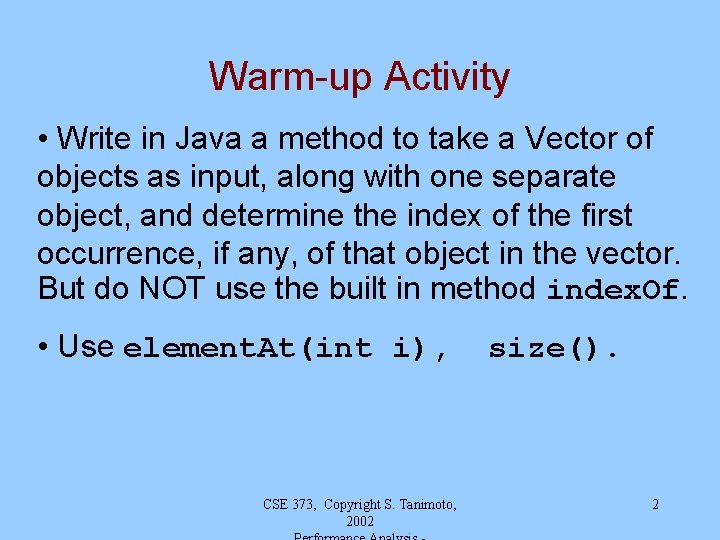
Warm-up Activity • Write in Java a method to take a Vector of objects as input, along with one separate object, and determine the index of the first occurrence, if any, of that object in the vector. But do NOT use the built in method index. Of. • Use element. At(int i), CSE 373, Copyright S. Tanimoto, 2002 size(). 2
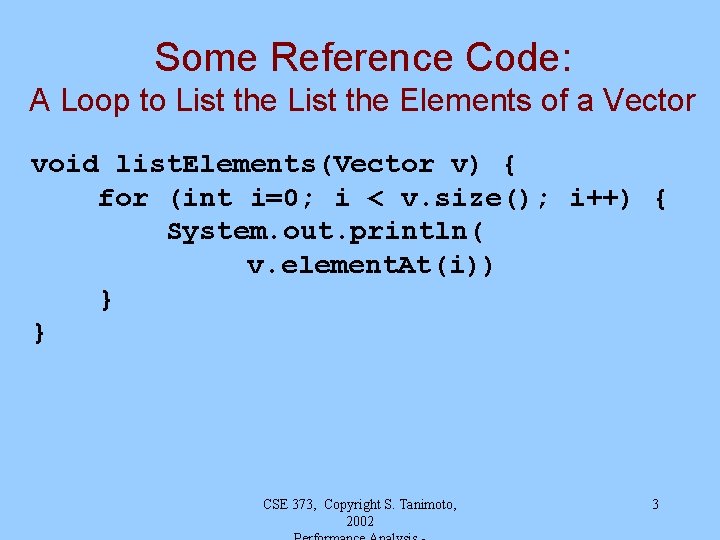
Some Reference Code: A Loop to List the Elements of a Vector void list. Elements(Vector v) { for (int i=0; i < v. size(); i++) { System. out. println( v. element. At(i)) } } CSE 373, Copyright S. Tanimoto, 2002 3
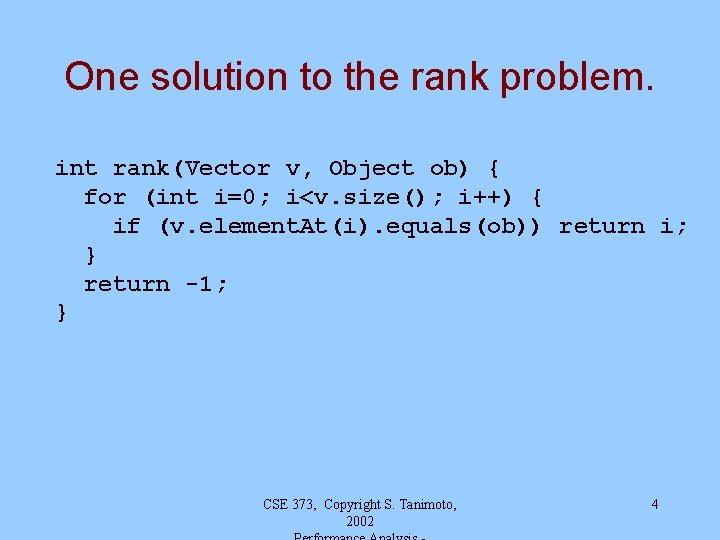
One solution to the rank problem. int rank(Vector v, Object ob) { for (int i=0; i<v. size(); i++) { if (v. element. At(i). equals(ob)) return i; } return -1; } CSE 373, Copyright S. Tanimoto, 2002 4
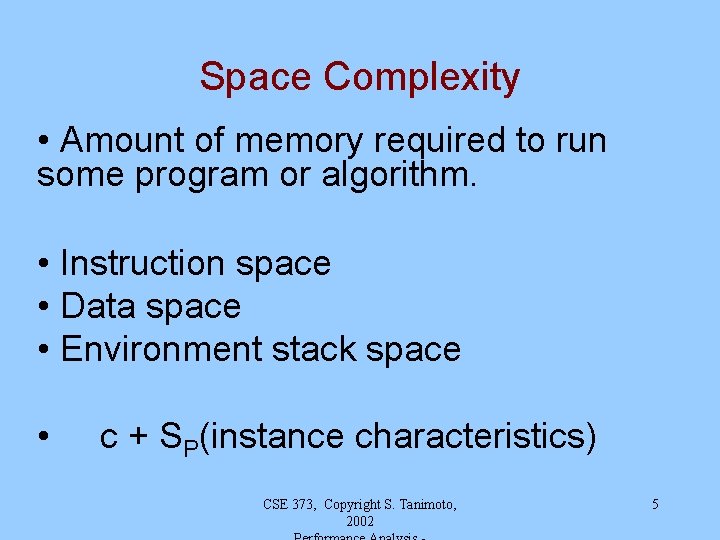
Space Complexity • Amount of memory required to run some program or algorithm. • Instruction space • Data space • Environment stack space • c + SP(instance characteristics) CSE 373, Copyright S. Tanimoto, 2002 5
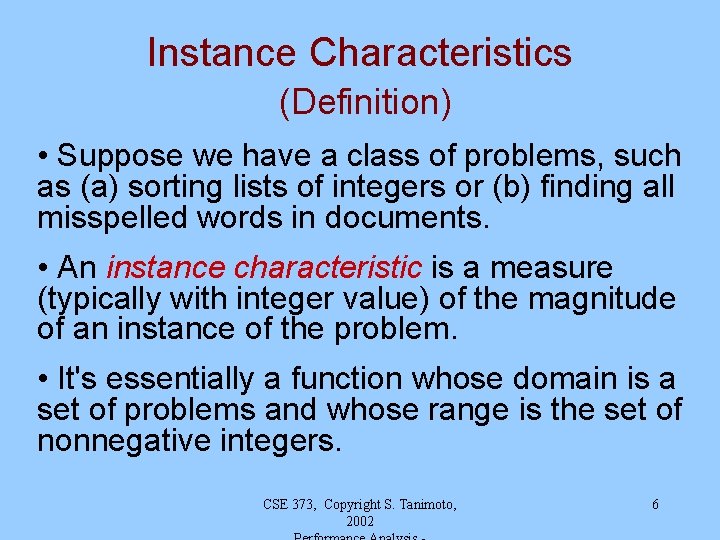
Instance Characteristics (Definition) • Suppose we have a class of problems, such as (a) sorting lists of integers or (b) finding all misspelled words in documents. • An instance characteristic is a measure (typically with integer value) of the magnitude of an instance of the problem. • It's essentially a function whose domain is a set of problems and whose range is the set of nonnegative integers. CSE 373, Copyright S. Tanimoto, 2002 6
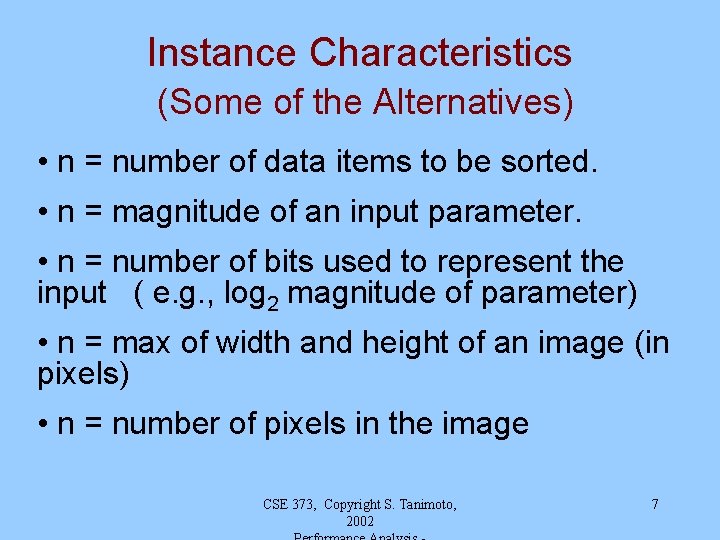
Instance Characteristics (Some of the Alternatives) • n = number of data items to be sorted. • n = magnitude of an input parameter. • n = number of bits used to represent the input ( e. g. , log 2 magnitude of parameter) • n = max of width and height of an image (in pixels) • n = number of pixels in the image CSE 373, Copyright S. Tanimoto, 2002 7
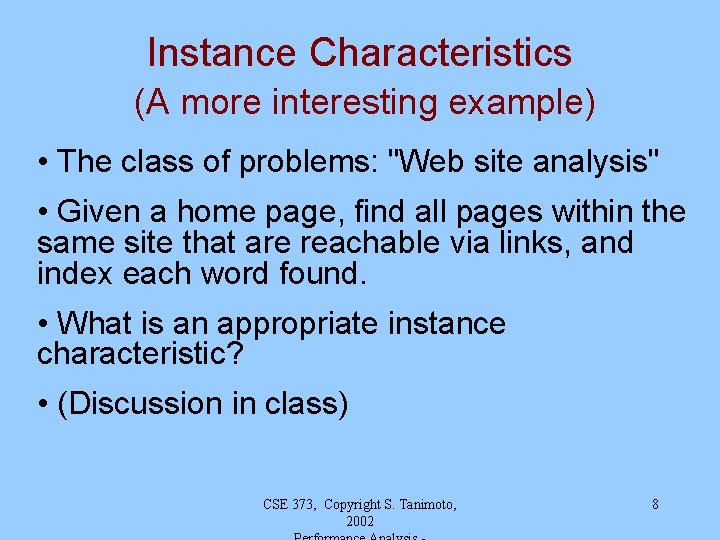
Instance Characteristics (A more interesting example) • The class of problems: "Web site analysis" • Given a home page, find all pages within the same site that are reachable via links, and index each word found. • What is an appropriate instance characteristic? • (Discussion in class) CSE 373, Copyright S. Tanimoto, 2002 8
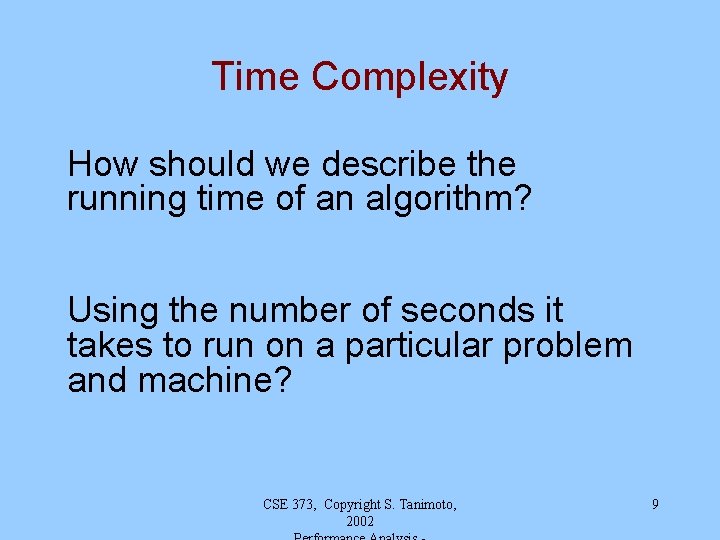
Time Complexity How should we describe the running time of an algorithm? Using the number of seconds it takes to run on a particular problem and machine? CSE 373, Copyright S. Tanimoto, 2002 9
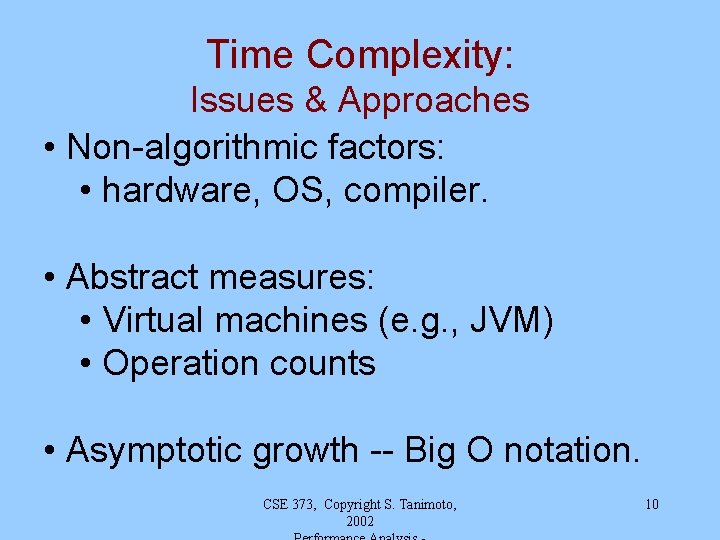
Time Complexity: Issues & Approaches • Non-algorithmic factors: • hardware, OS, compiler. • Abstract measures: • Virtual machines (e. g. , JVM) • Operation counts • Asymptotic growth -- Big O notation. CSE 373, Copyright S. Tanimoto, 2002 10
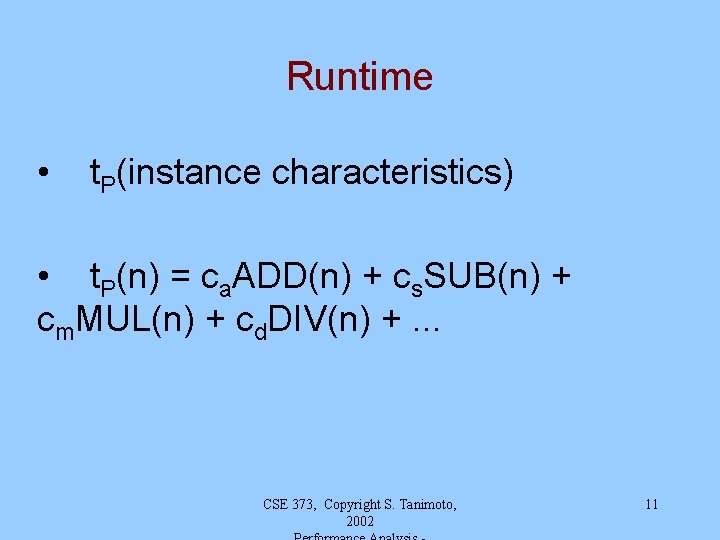
Runtime • t. P(instance characteristics) • t. P(n) = ca. ADD(n) + cs. SUB(n) + cm. MUL(n) + cd. DIV(n) +. . . CSE 373, Copyright S. Tanimoto, 2002 11
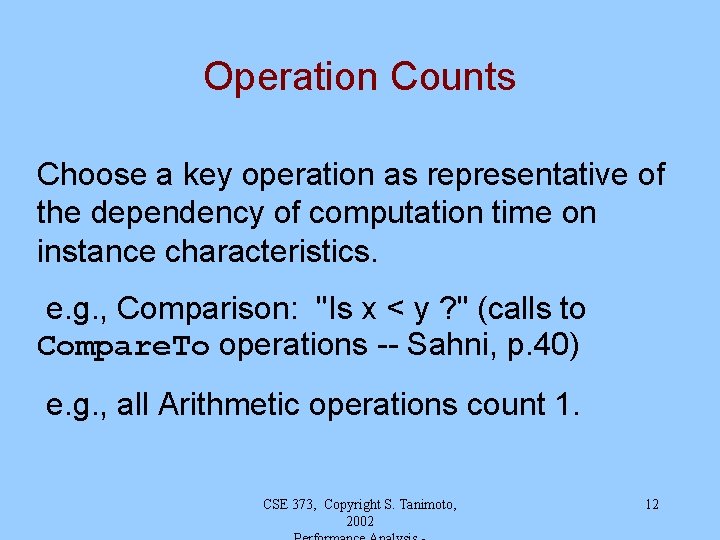
Operation Counts Choose a key operation as representative of the dependency of computation time on instance characteristics. e. g. , Comparison: "Is x < y ? " (calls to Compare. To operations -- Sahni, p. 40) e. g. , all Arithmetic operations count 1. CSE 373, Copyright S. Tanimoto, 2002 12
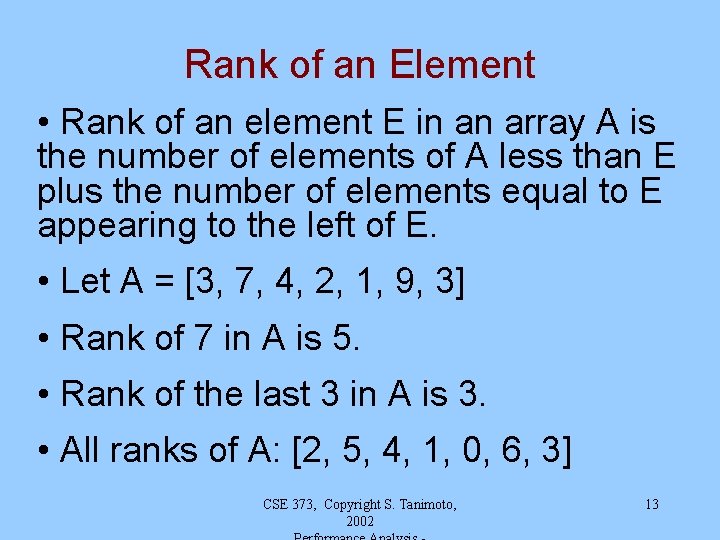
Rank of an Element • Rank of an element E in an array A is the number of elements of A less than E plus the number of elements equal to E appearing to the left of E. • Let A = [3, 7, 4, 2, 1, 9, 3] • Rank of 7 in A is 5. • Rank of the last 3 in A is 3. • All ranks of A: [2, 5, 4, 1, 0, 6, 3] CSE 373, Copyright S. Tanimoto, 2002 13
![Computing the Ranks public static void rankComparable a int r if Computing the Ranks public static void rank(Comparable [] a, int [] r) { if](https://slidetodoc.com/presentation_image_h2/1cec4aa7ab4c586fcade097a6fda4dcb/image-14.jpg)
Computing the Ranks public static void rank(Comparable [] a, int [] r) { if (r. length < a. length) throw new Illegal. Argument. Exception ("Rank array too short"); for (int i = 0; i < a. length; i++) r[i] = 0; for (int i = 1; i < a. length; i++) { for (int j = 0; j < i; j++) if (a[j]. compare. To(a[i])) <= 0) r[i]++; else r[j]++; } //(Sahni, p. 79) CSE 373, Copyright S. Tanimoto, 2002 14
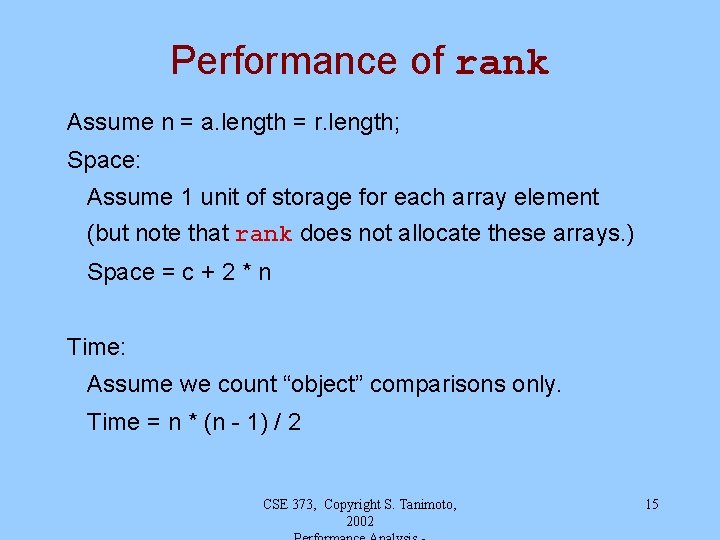
Performance of rank Assume n = a. length = r. length; Space: Assume 1 unit of storage for each array element (but note that rank does not allocate these arrays. ) Space = c + 2 * n Time: Assume we count “object” comparisons only. Time = n * (n - 1) / 2 CSE 373, Copyright S. Tanimoto, 2002 15
![Rearranging public static void rearrangeComparable a int r Comparable u Rearranging public static void rearrange(Comparable [] a, int [] r) { Comparable [] u](https://slidetodoc.com/presentation_image_h2/1cec4aa7ab4c586fcade097a6fda4dcb/image-16.jpg)
Rearranging public static void rearrange(Comparable [] a, int [] r) { Comparable [] u = new Comparable [a. length]; // Sort into u using ranks: for (int i = 0; i < a. length; i++) { u[r[i]] = a[i]; // Copy items back to array a. for (int i = 0; i < a. length; i++) { a[i] = u[i]; } //(Sahni, p. 80) CSE 373, Copyright S. Tanimoto, 2002 16
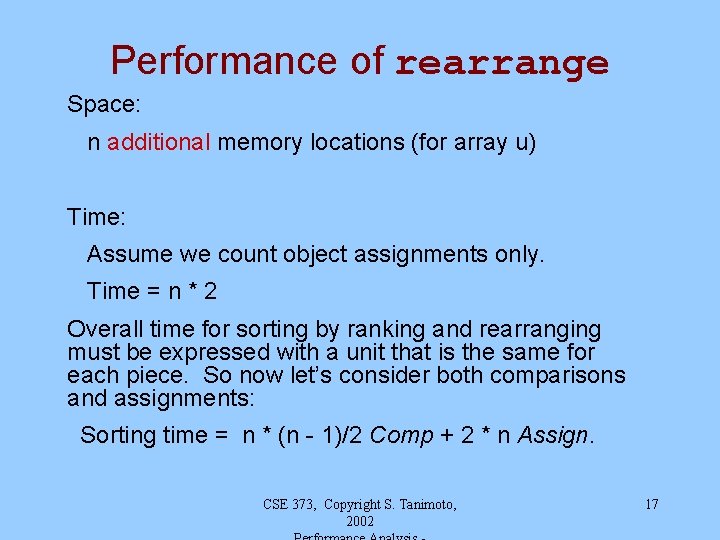
Performance of rearrange Space: n additional memory locations (for array u) Time: Assume we count object assignments only. Time = n * 2 Overall time for sorting by ranking and rearranging must be expressed with a unit that is the same for each piece. So now let’s consider both comparisons and assignments: Sorting time = n * (n - 1)/2 Comp + 2 * n Assign. CSE 373, Copyright S. Tanimoto, 2002 17
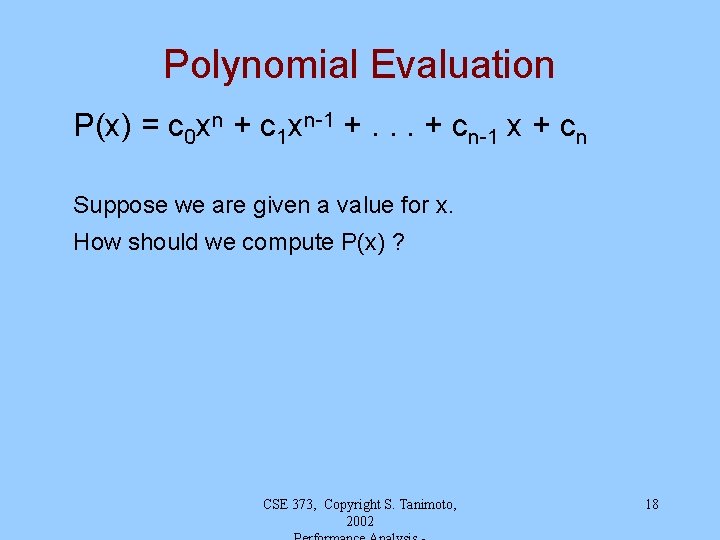
Polynomial Evaluation P(x) = c 0 xn + c 1 xn-1 +. . . + cn-1 x + cn Suppose we are given a value for x. How should we compute P(x) ? CSE 373, Copyright S. Tanimoto, 2002 18
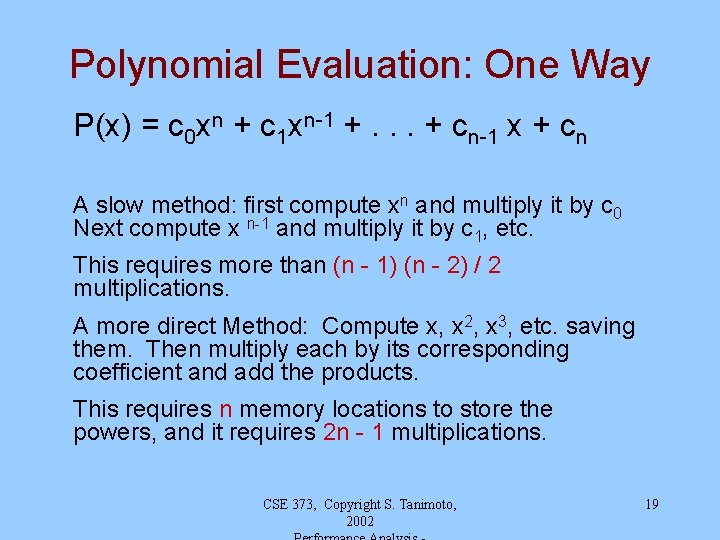
Polynomial Evaluation: One Way P(x) = c 0 xn + c 1 xn-1 +. . . + cn-1 x + cn A slow method: first compute xn and multiply it by c 0 Next compute x n-1 and multiply it by c 1, etc. This requires more than (n - 1) (n - 2) / 2 multiplications. A more direct Method: Compute x, x 2, x 3, etc. saving them. Then multiply each by its corresponding coefficient and add the products. This requires n memory locations to store the powers, and it requires 2 n - 1 multiplications. CSE 373, Copyright S. Tanimoto, 2002 19
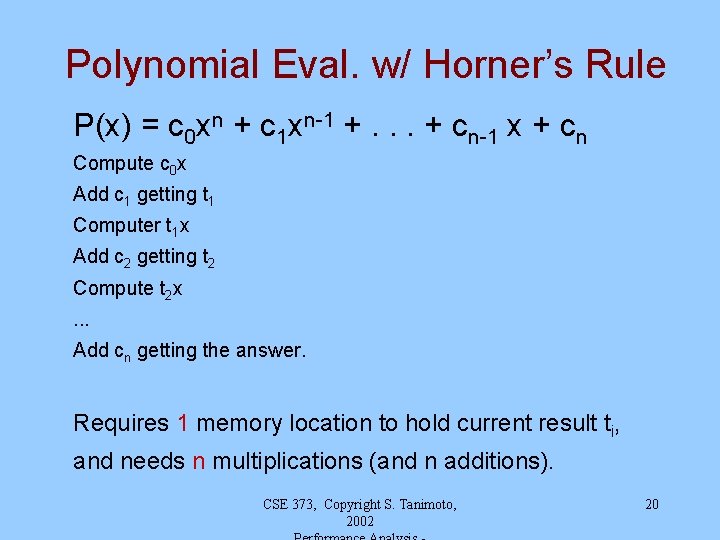
Polynomial Eval. w/ Horner’s Rule P(x) = c 0 xn + c 1 xn-1 +. . . + cn-1 x + cn Compute c 0 x Add c 1 getting t 1 Computer t 1 x Add c 2 getting t 2 Compute t 2 x. . . Add cn getting the answer. Requires 1 memory location to hold current result ti, and needs n multiplications (and n additions). CSE 373, Copyright S. Tanimoto, 2002 20
For loop space complexity
The blind search algorithms are.
Space complexity bfs
Space complexity bfs
Bfs
Space complexity of insertion sort
Instance variables
Instance de socialisation
L'enfant sauvage socialisation
Instance weighting for domain adaptation in nlp
Hadoop web services
Table instance chart
Instance of a class
[email protected]
Logical data independence in dbms
Dicom file structure
Database instance in dbms
Ec2 instance purchasing options
Instance based learning in machine learning
Instance meaning
Oracle instance architecture