Review of Instance Fields and Methods Each instance
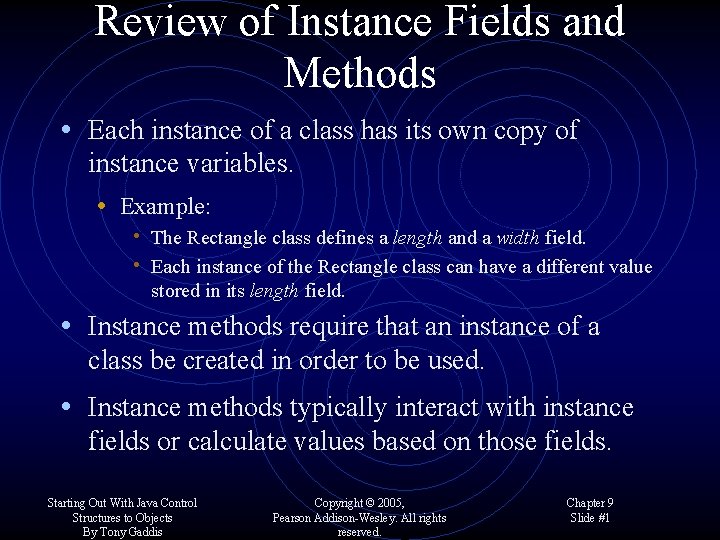
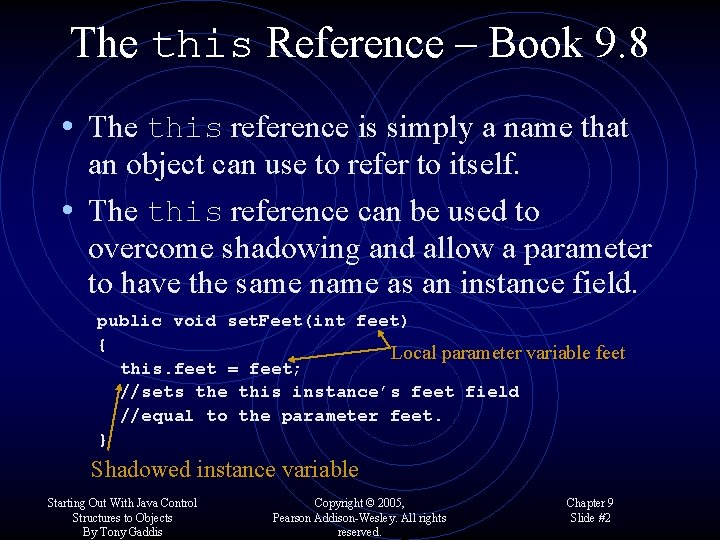
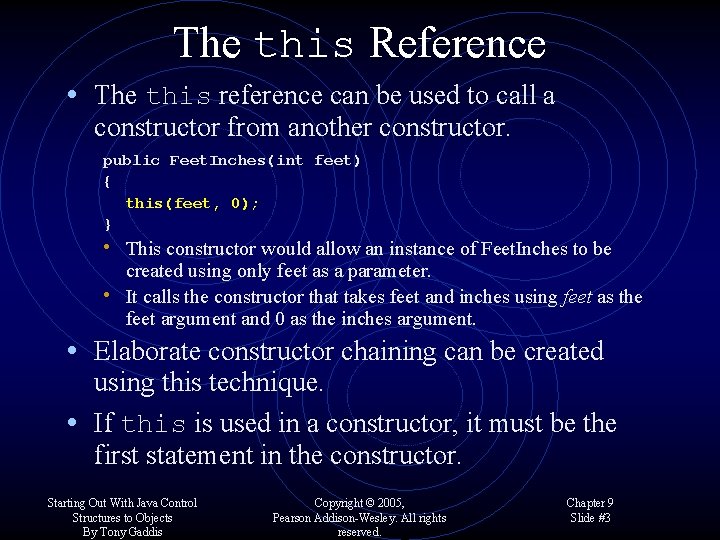
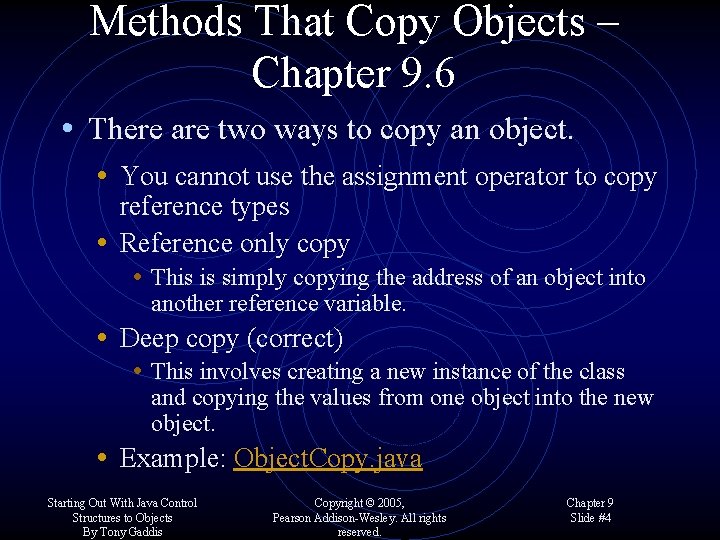
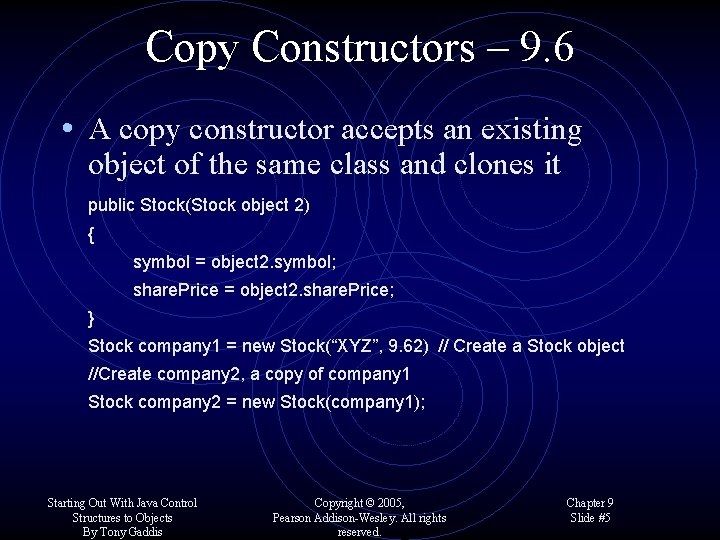
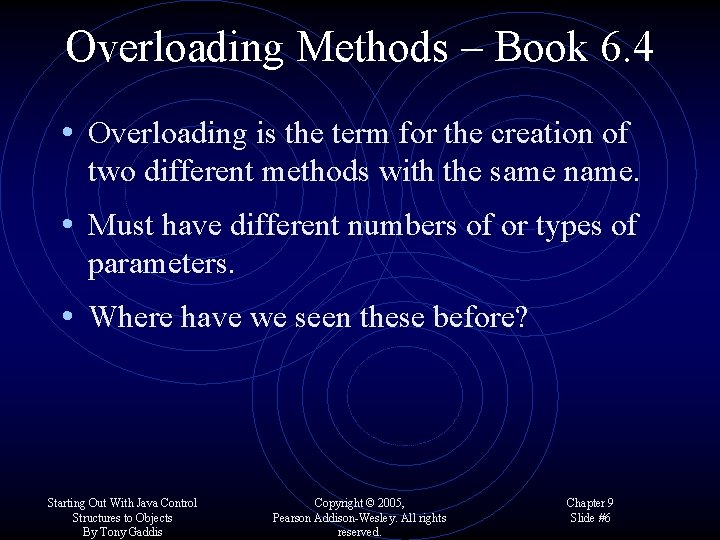
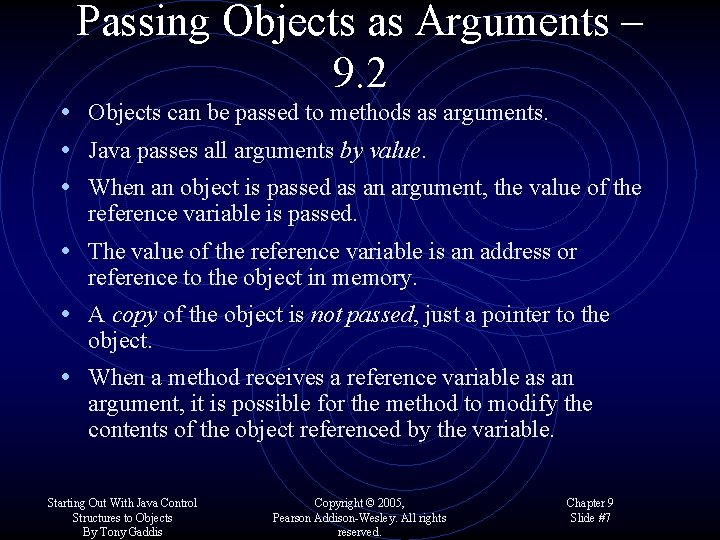
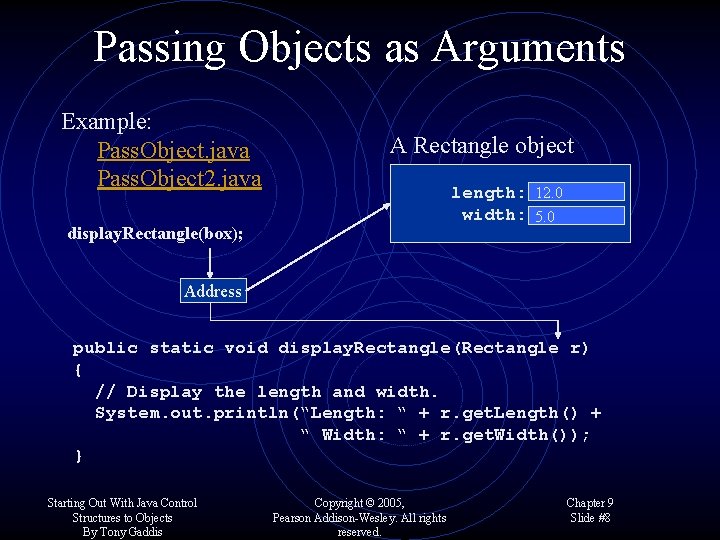
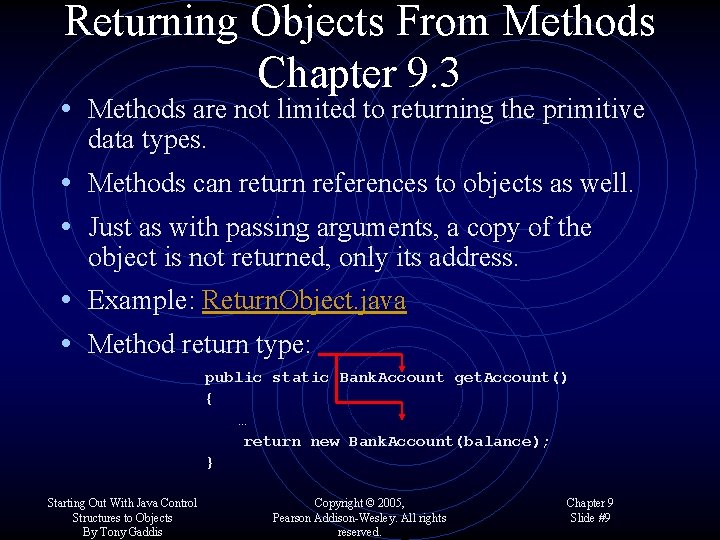
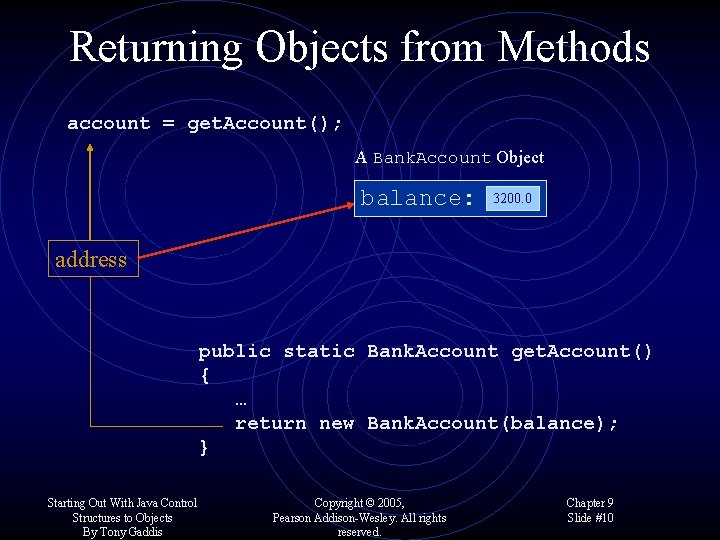
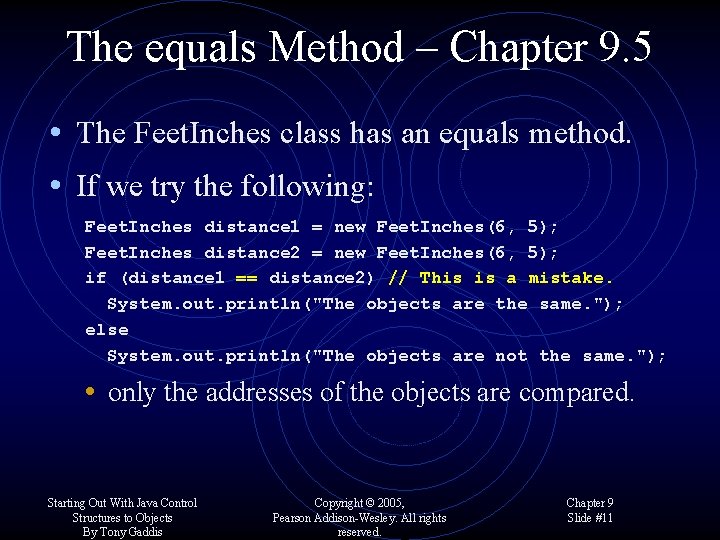
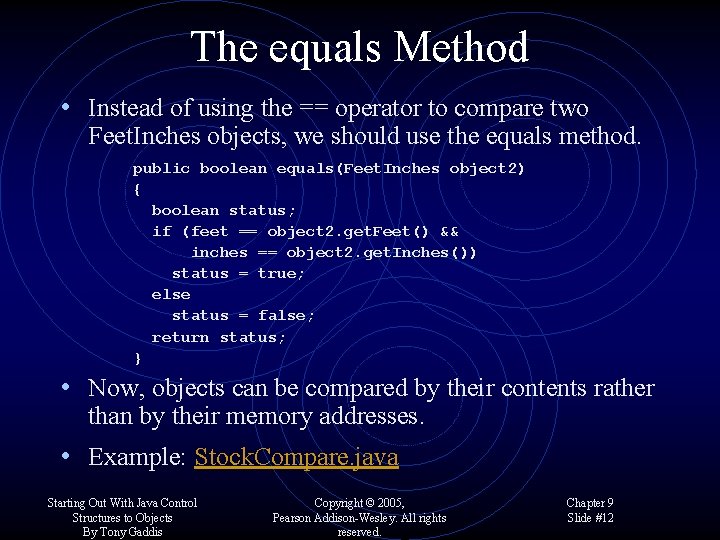
- Slides: 12
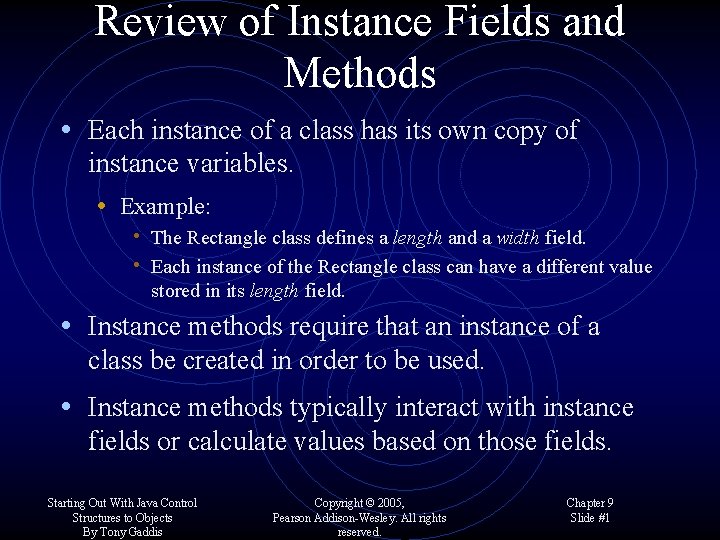
Review of Instance Fields and Methods • Each instance of a class has its own copy of instance variables. • Example: • The Rectangle class defines a length and a width field. • Each instance of the Rectangle class can have a different value stored in its length field. • Instance methods require that an instance of a class be created in order to be used. • Instance methods typically interact with instance fields or calculate values based on those fields. Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #1
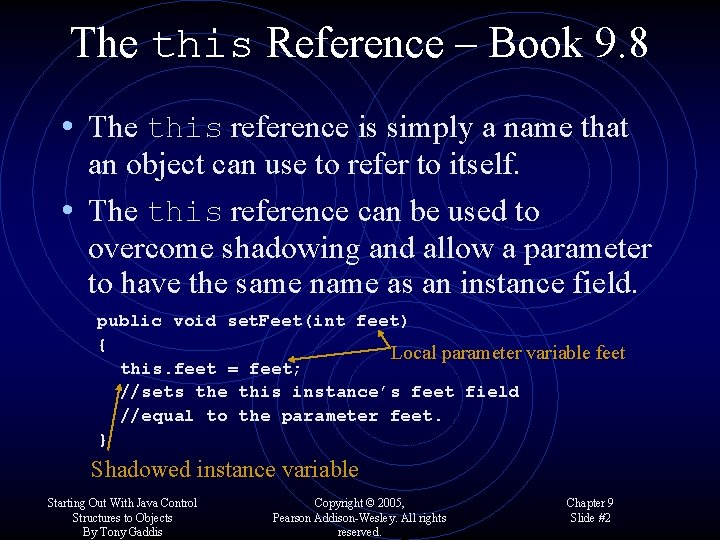
The this Reference – Book 9. 8 • The this reference is simply a name that an object can use to refer to itself. • The this reference can be used to overcome shadowing and allow a parameter to have the same name as an instance field. public void set. Feet(int feet) { Local parameter variable feet this. feet = feet; //sets the this instance’s feet field //equal to the parameter feet. } Shadowed instance variable Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #2
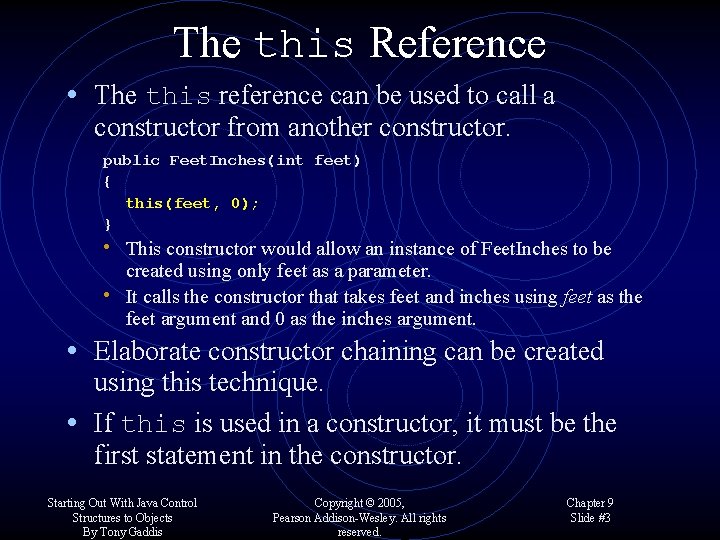
The this Reference • The this reference can be used to call a constructor from another constructor. public Feet. Inches(int feet) { this(feet, 0); } • This constructor would allow an instance of Feet. Inches to be created using only feet as a parameter. • It calls the constructor that takes feet and inches using feet as the feet argument and 0 as the inches argument. • Elaborate constructor chaining can be created using this technique. • If this is used in a constructor, it must be the first statement in the constructor. Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #3
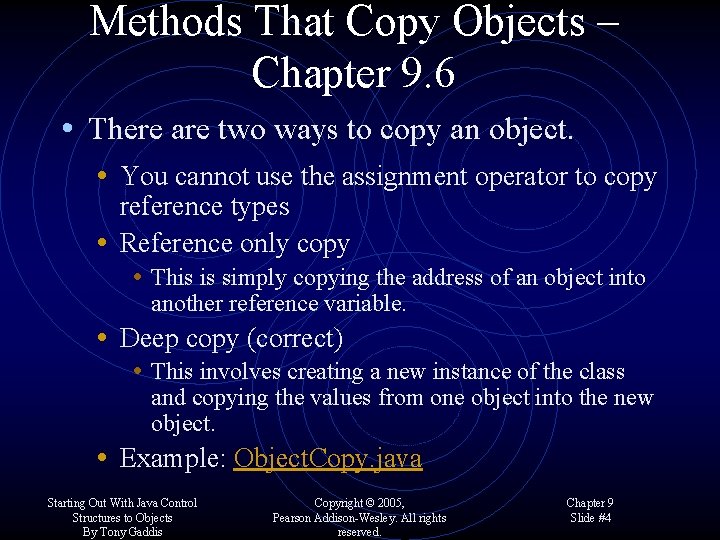
Methods That Copy Objects – Chapter 9. 6 • There are two ways to copy an object. • You cannot use the assignment operator to copy reference types • Reference only copy • This is simply copying the address of an object into another reference variable. • Deep copy (correct) • This involves creating a new instance of the class and copying the values from one object into the new object. • Example: Object. Copy. java Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #4
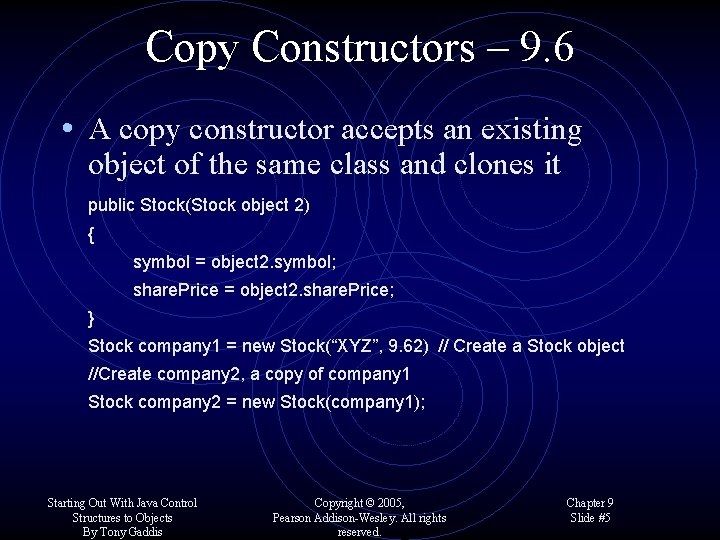
Copy Constructors – 9. 6 • A copy constructor accepts an existing object of the same class and clones it public Stock(Stock object 2) { symbol = object 2. symbol; share. Price = object 2. share. Price; } Stock company 1 = new Stock(“XYZ”, 9. 62) // Create a Stock object //Create company 2, a copy of company 1 Stock company 2 = new Stock(company 1); Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #5
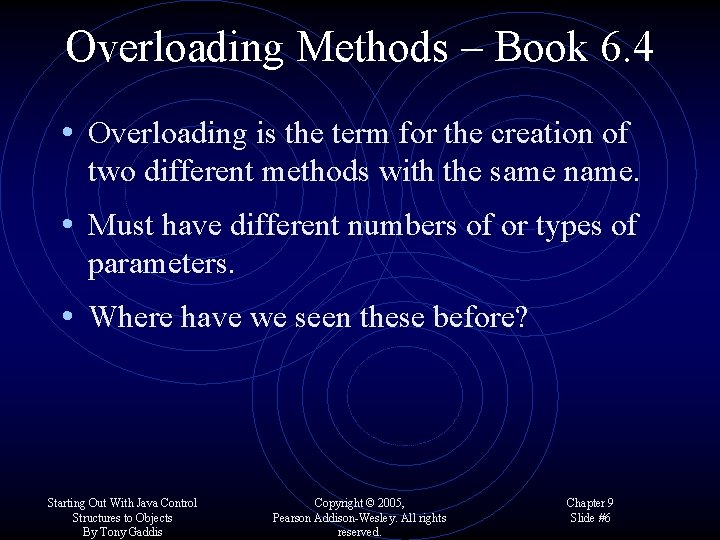
Overloading Methods – Book 6. 4 • Overloading is the term for the creation of two different methods with the same name. • Must have different numbers of or types of parameters. • Where have we seen these before? Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #6
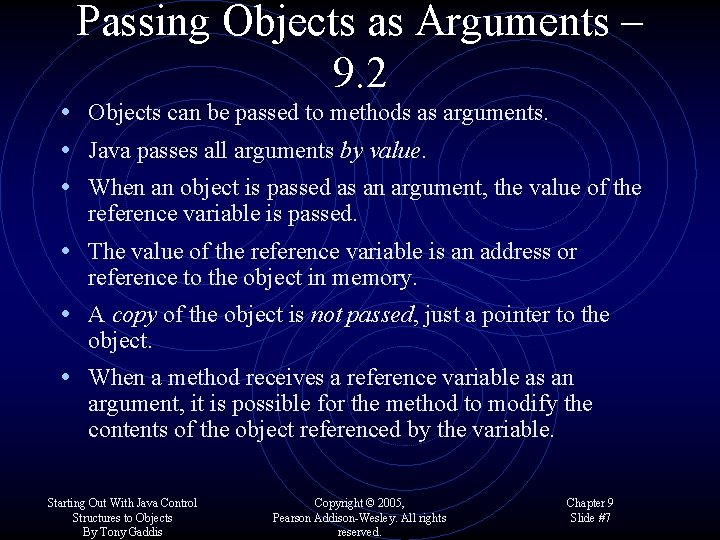
Passing Objects as Arguments – 9. 2 • Objects can be passed to methods as arguments. • Java passes all arguments by value. • When an object is passed as an argument, the value of the reference variable is passed. • The value of the reference variable is an address or reference to the object in memory. • A copy of the object is not passed, just a pointer to the object. • When a method receives a reference variable as an argument, it is possible for the method to modify the contents of the object referenced by the variable. Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #7
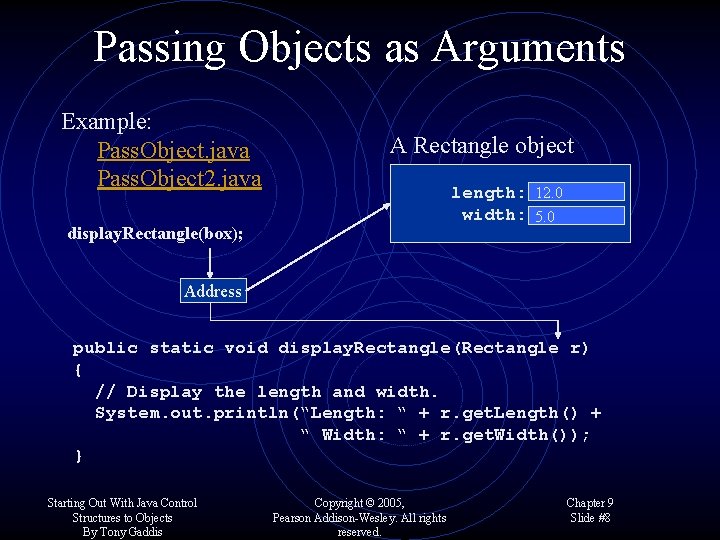
Passing Objects as Arguments Example: Pass. Object. java Pass. Object 2. java A Rectangle object length: 12. 0 width: 5. 0 display. Rectangle(box); Address public static void display. Rectangle(Rectangle r) { // Display the length and width. System. out. println(“Length: “ + r. get. Length() + “ Width: “ + r. get. Width()); } Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #8
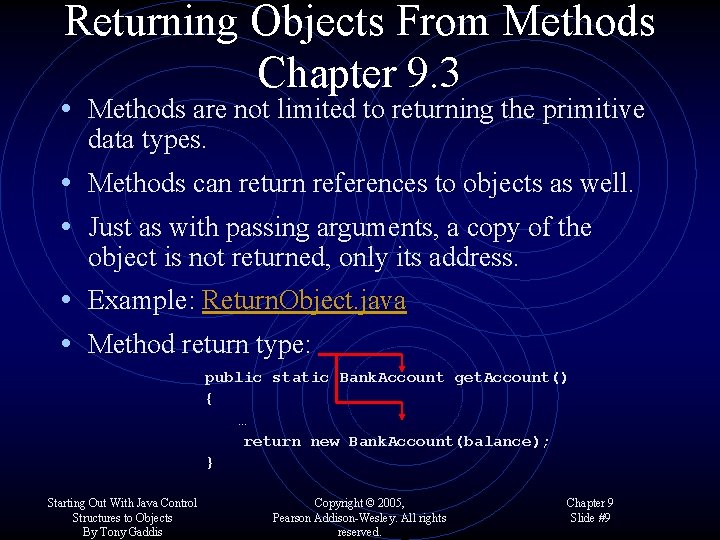
Returning Objects From Methods Chapter 9. 3 • Methods are not limited to returning the primitive data types. • Methods can return references to objects as well. • Just as with passing arguments, a copy of the object is not returned, only its address. • Example: Return. Object. java • Method return type: public static Bank. Account get. Account() { … return new Bank. Account(balance); } Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #9
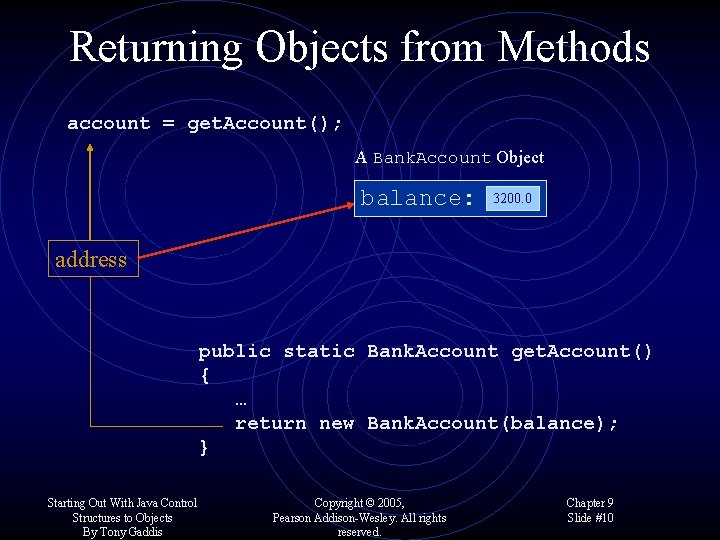
Returning Objects from Methods account = get. Account(); A Bank. Account Object balance: 3200. 0 address public static Bank. Account get. Account() { … return new Bank. Account(balance); } Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #10
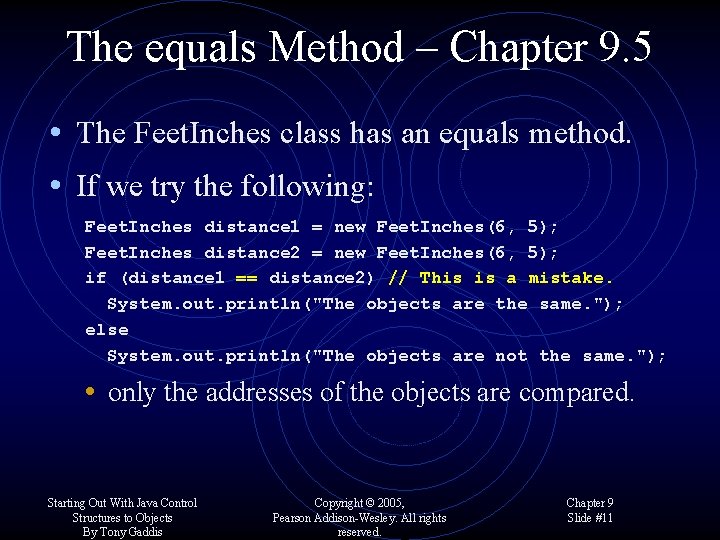
The equals Method – Chapter 9. 5 • The Feet. Inches class has an equals method. • If we try the following: Feet. Inches distance 1 = new Feet. Inches(6, 5); Feet. Inches distance 2 = new Feet. Inches(6, 5); if (distance 1 == distance 2) // This is a mistake. System. out. println("The objects are the same. "); else System. out. println("The objects are not the same. "); • only the addresses of the objects are compared. Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #11
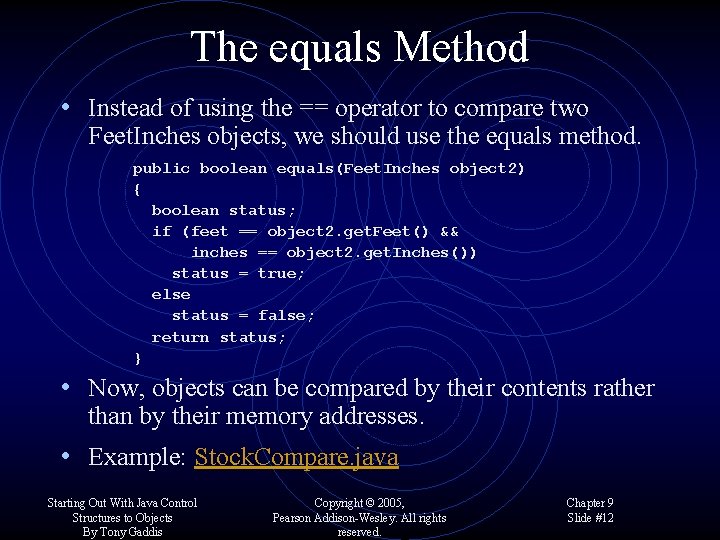
The equals Method • Instead of using the == operator to compare two Feet. Inches objects, we should use the equals method. public boolean equals(Feet. Inches object 2) { boolean status; if (feet == object 2. get. Feet() && inches == object 2. get. Inches()) status = true; else status = false; return status; } • Now, objects can be compared by their contents rather than by their memory addresses. • Example: Stock. Compare. java Starting Out With Java Control Structures to Objects By Tony Gaddis Copyright © 2005, Pearson Addison-Wesley. All rights reserved. Chapter 9 Slide #12