Parameter Passing Mechanisms Reference Parameters Function Call Server
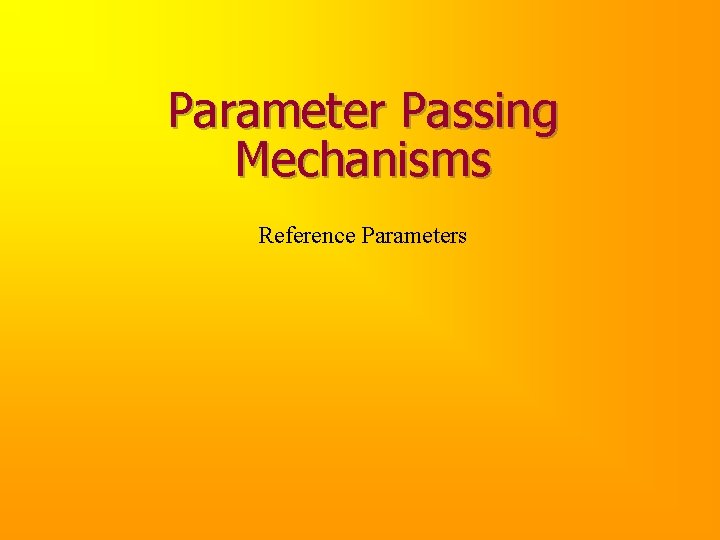
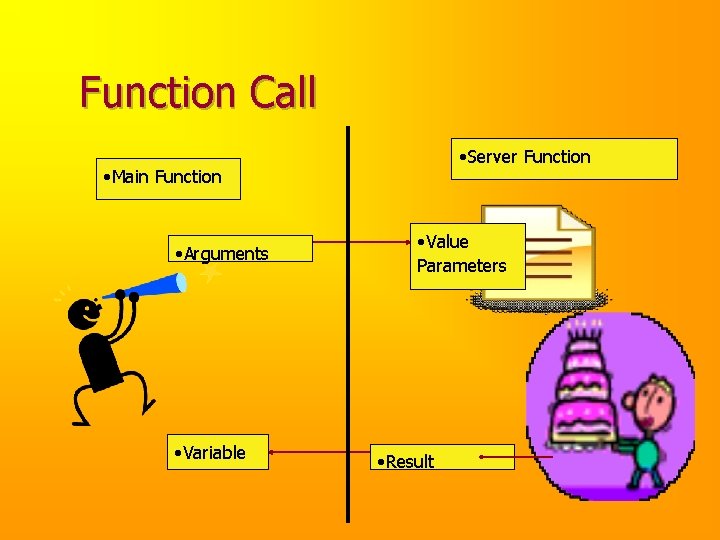
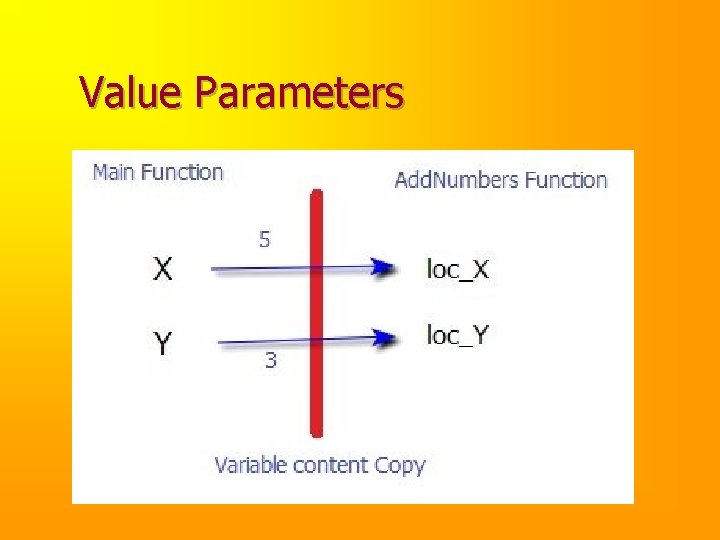
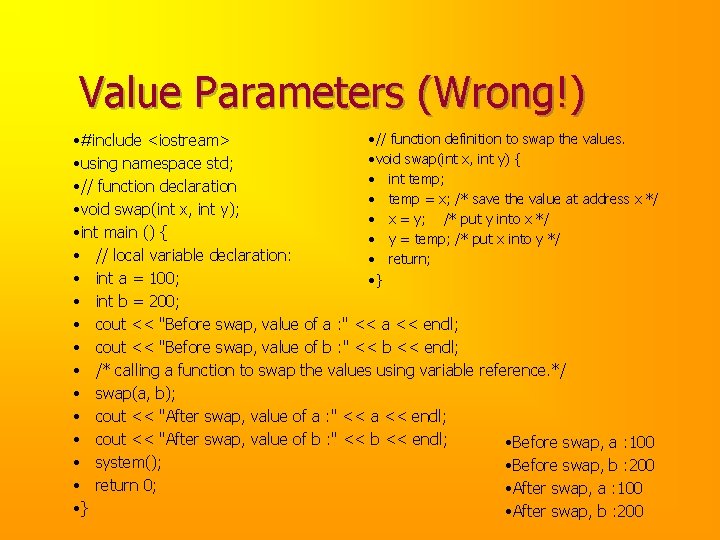
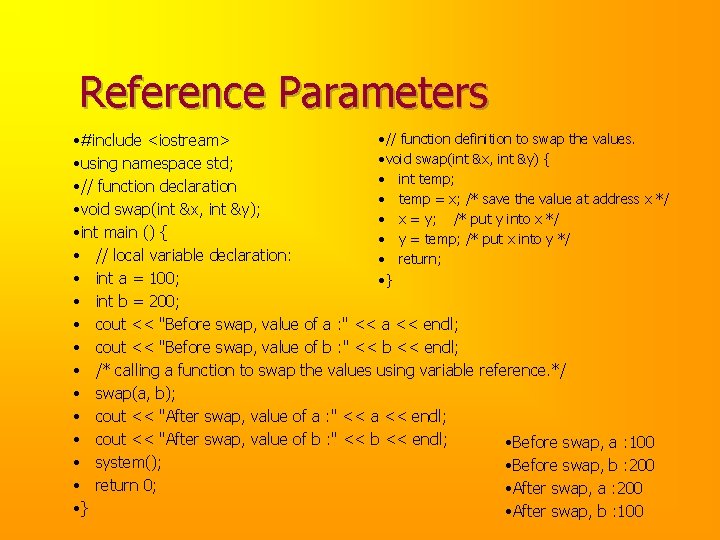
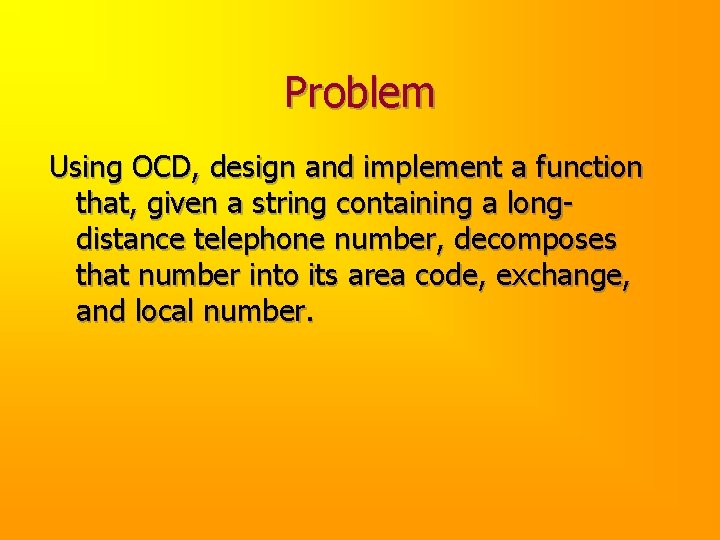
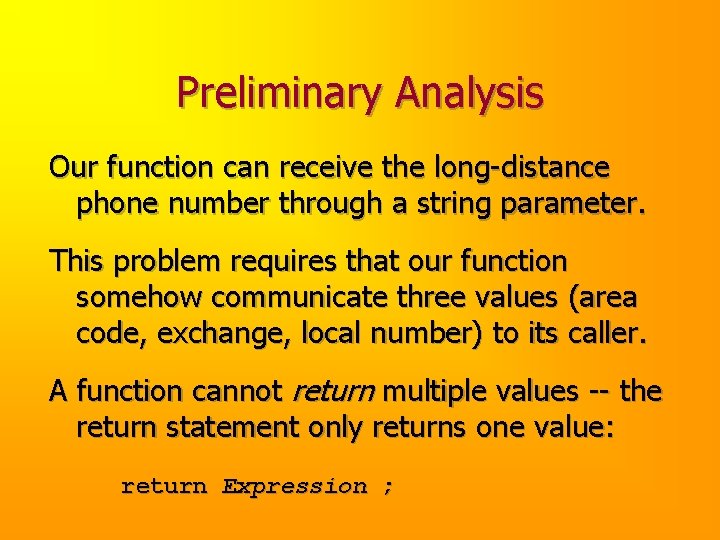
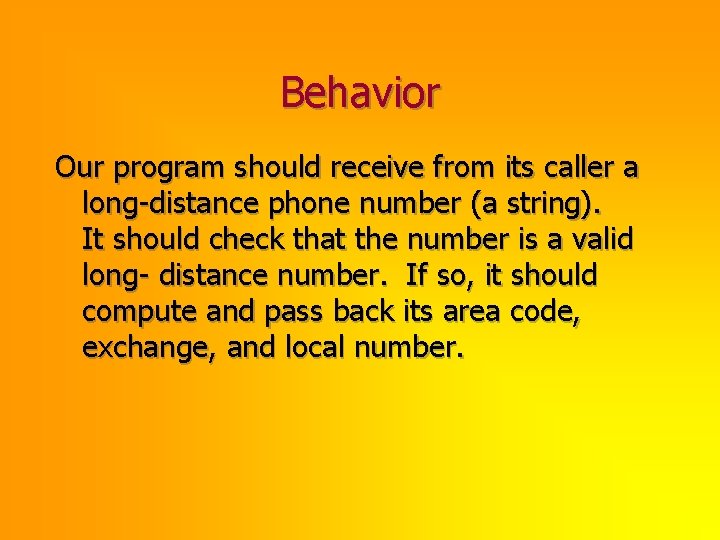
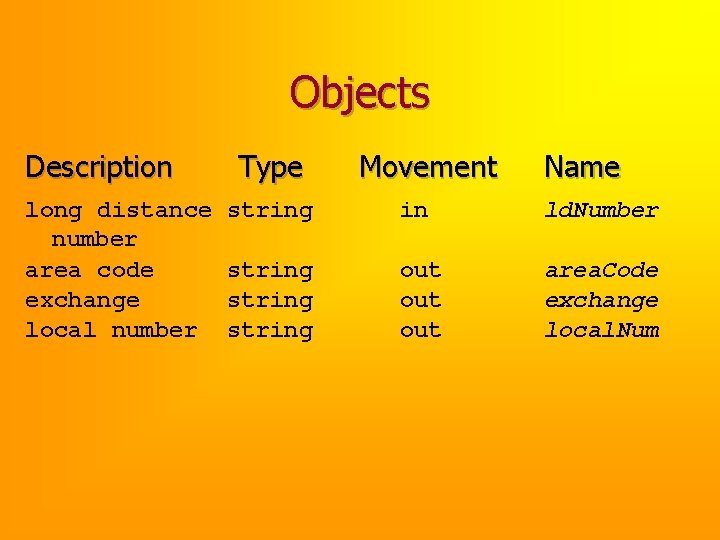
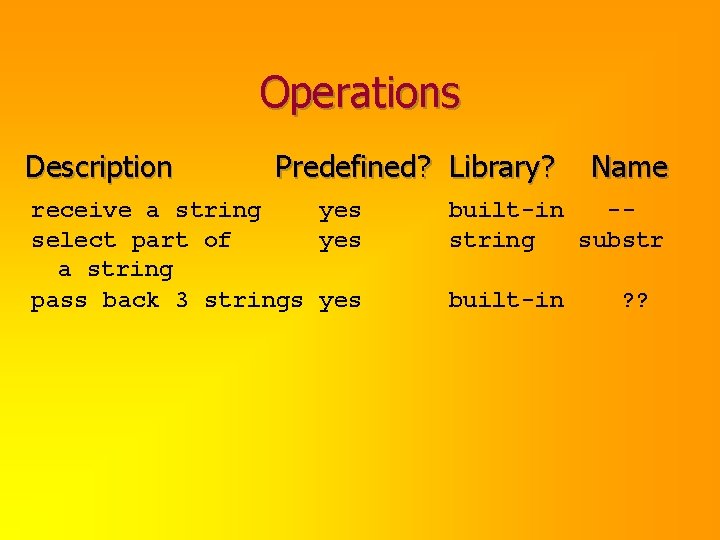
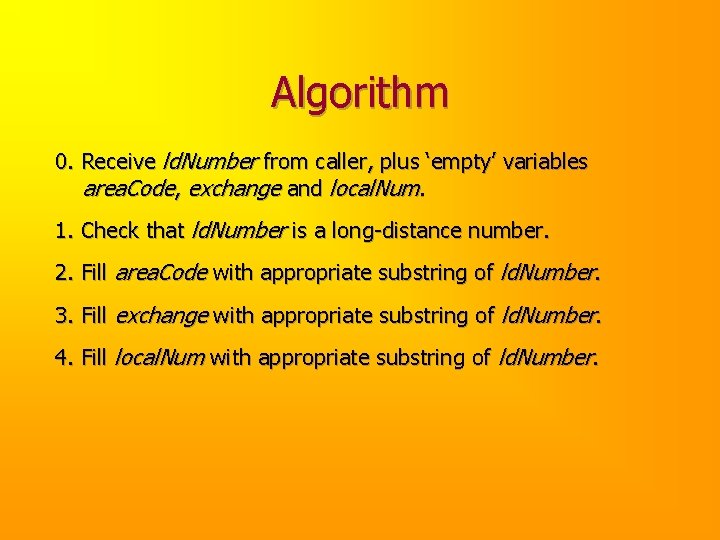
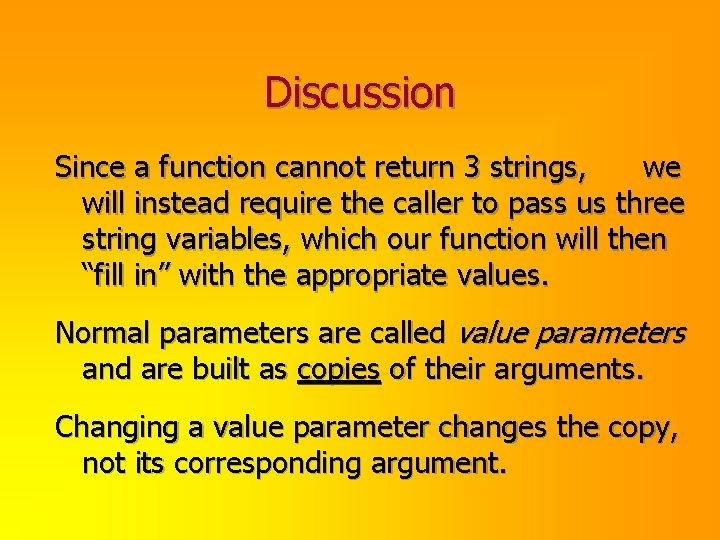
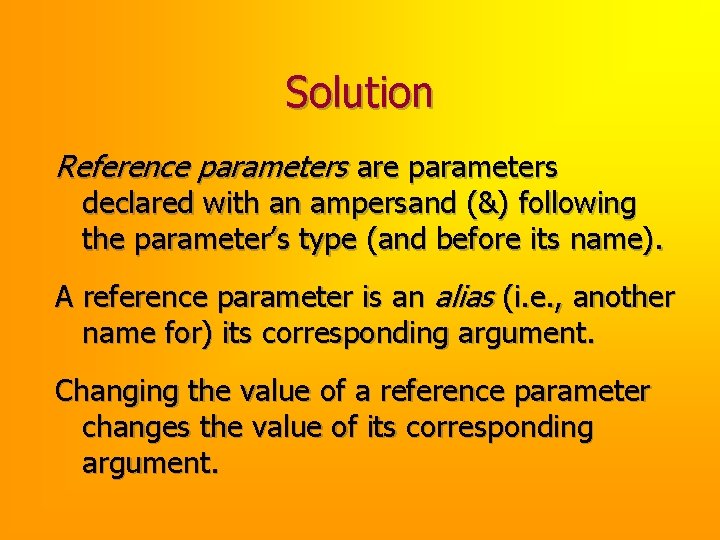
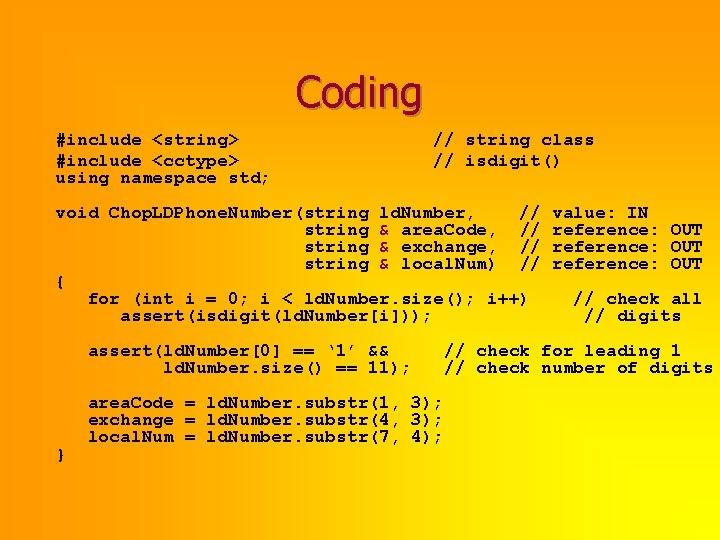
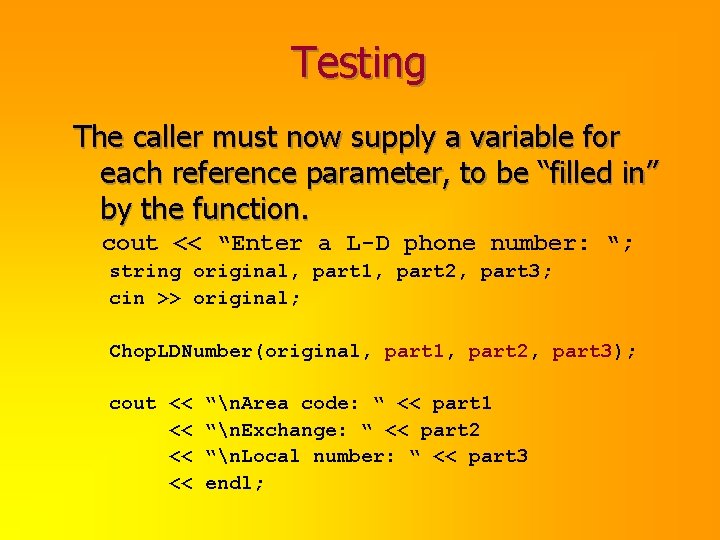
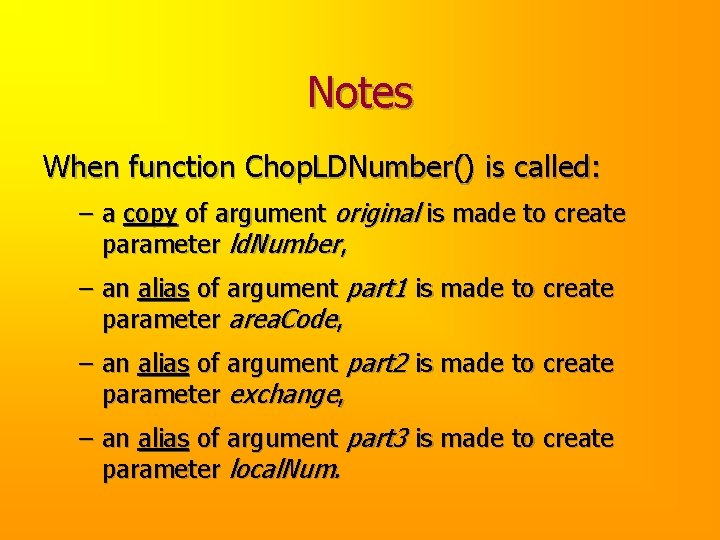
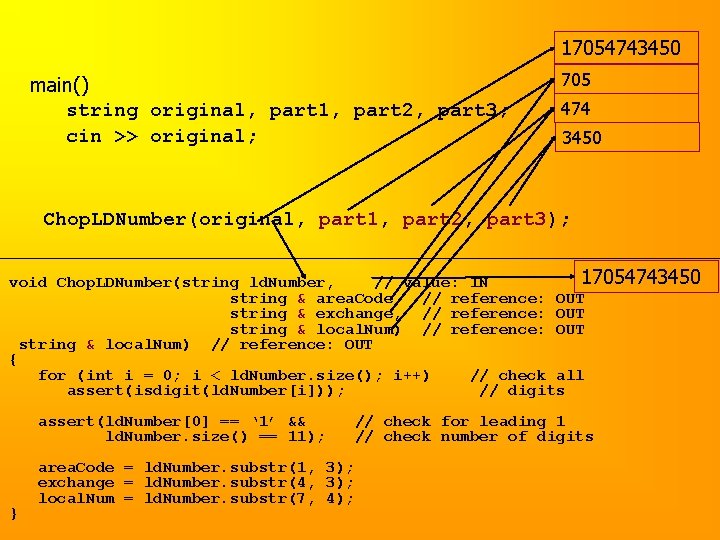
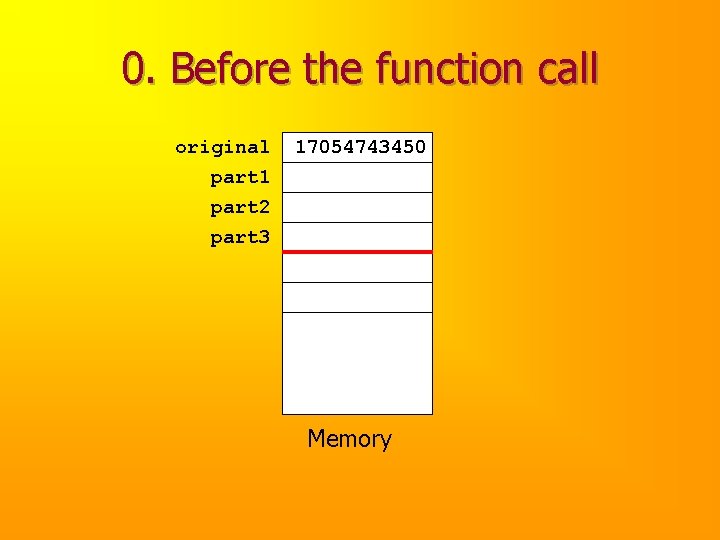
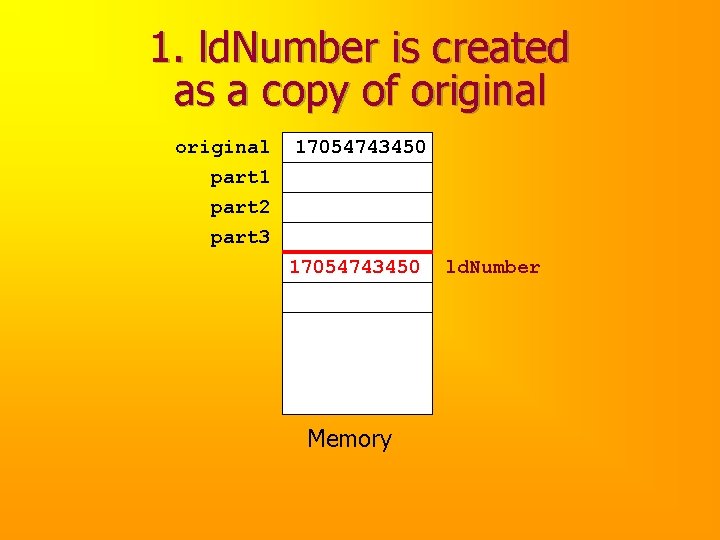
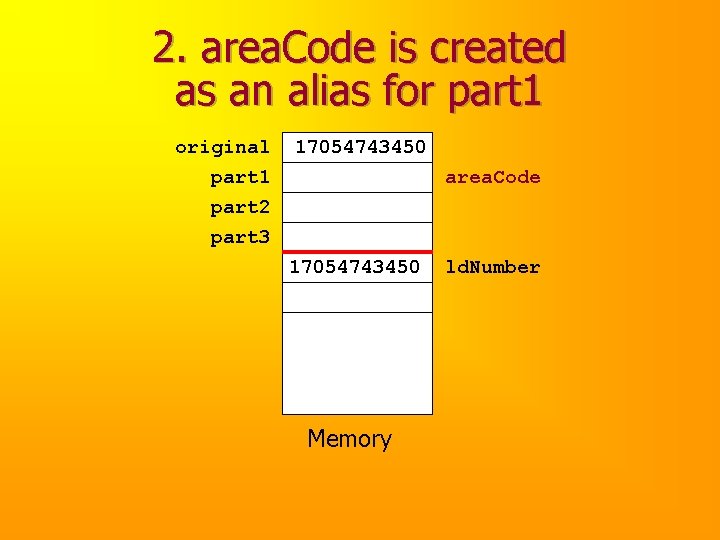
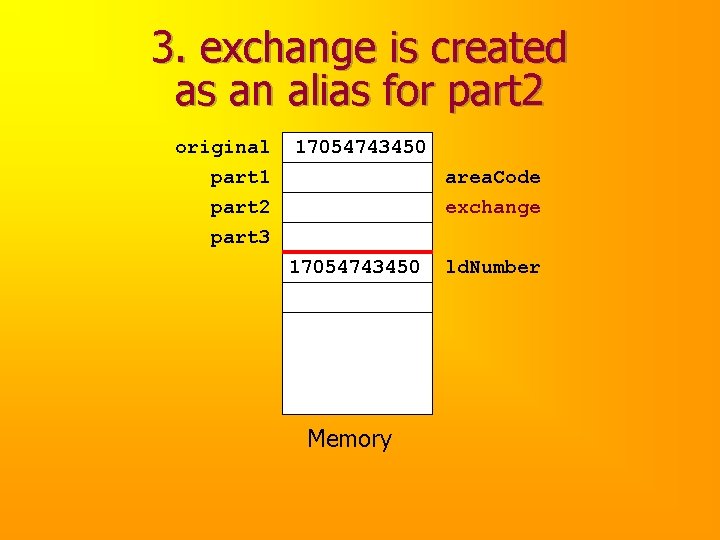
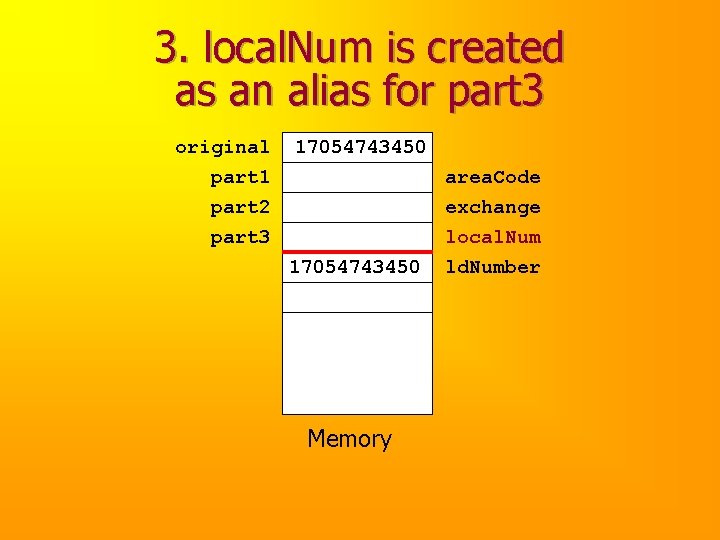
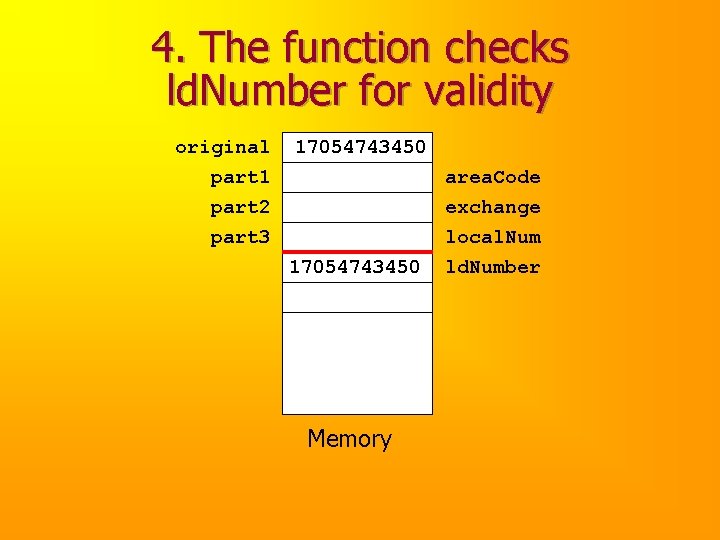
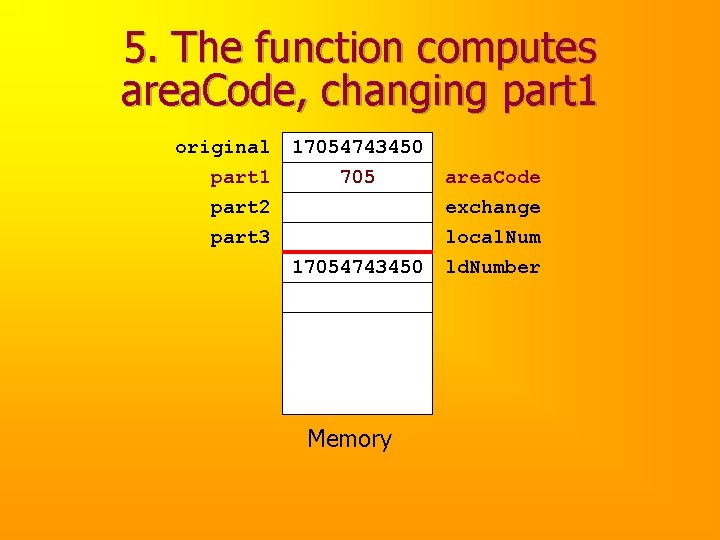
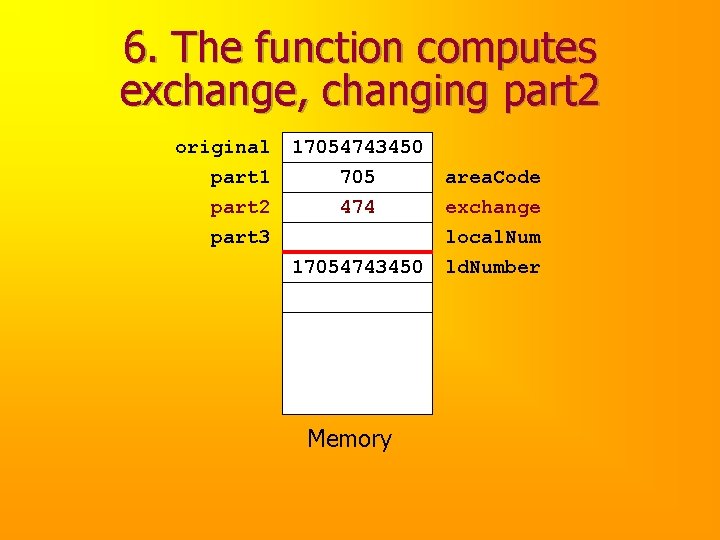
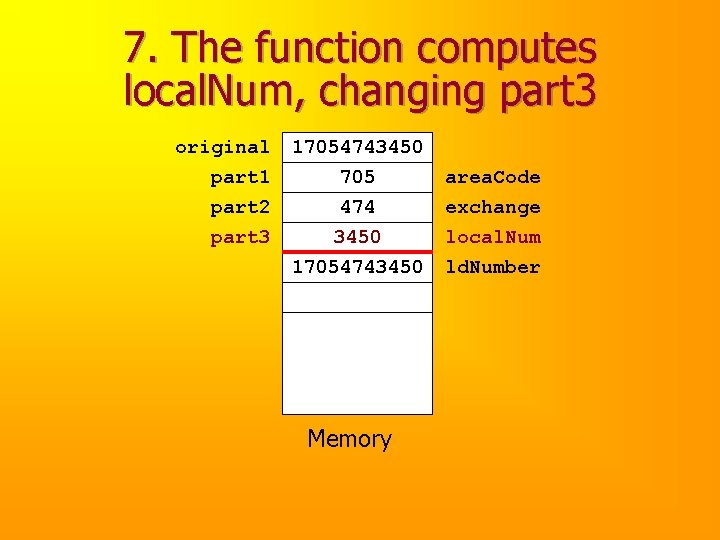
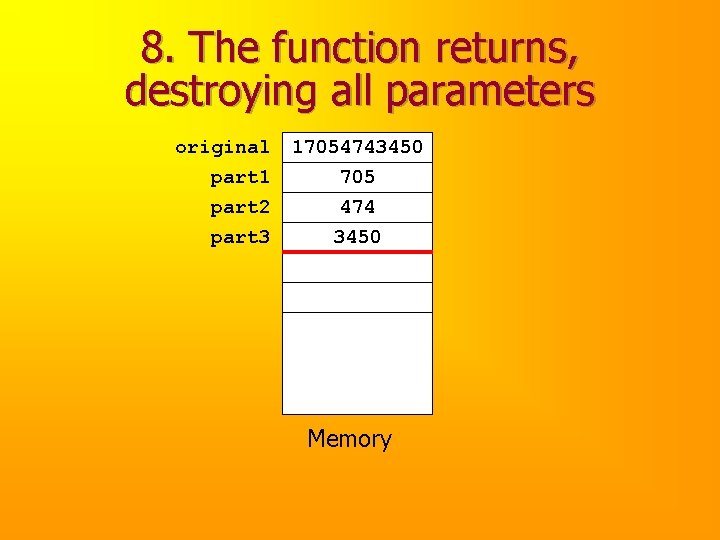
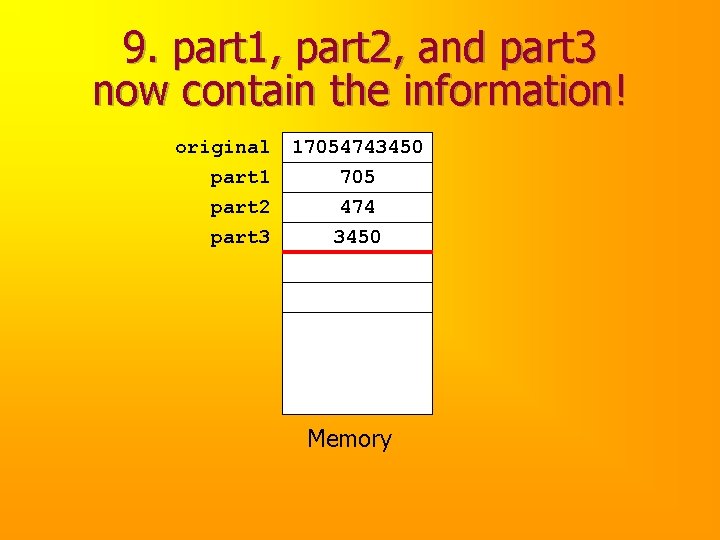
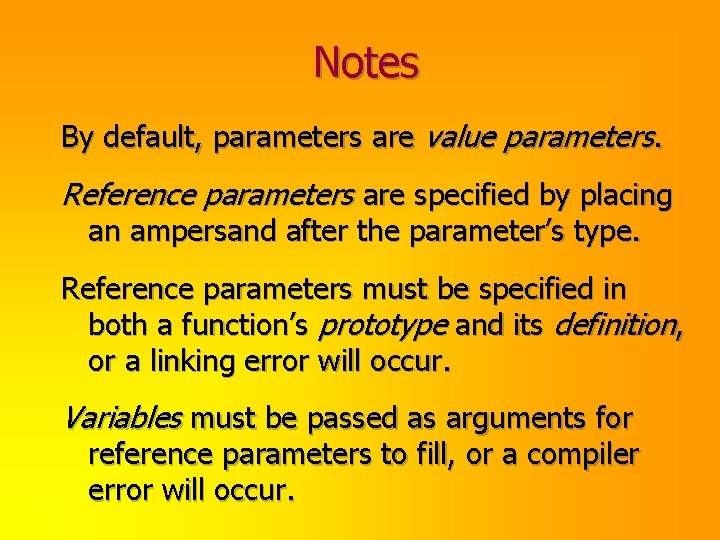
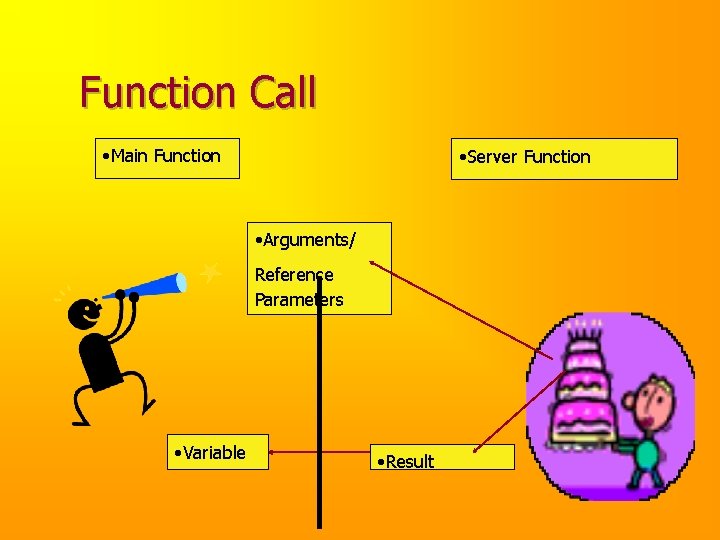
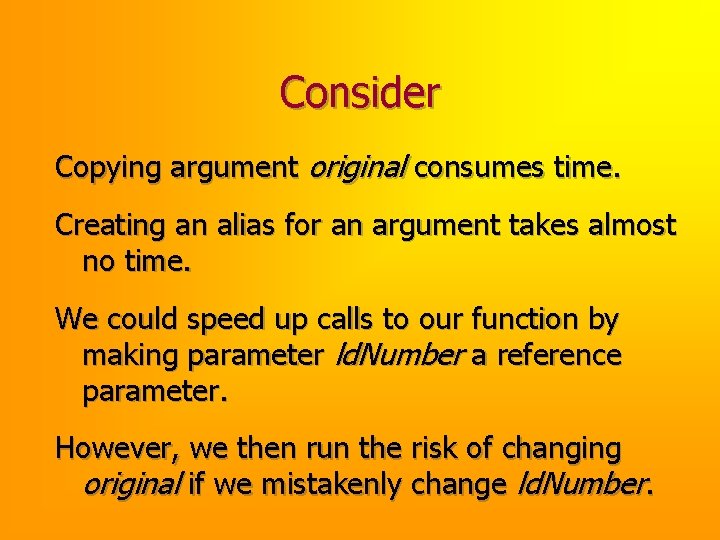
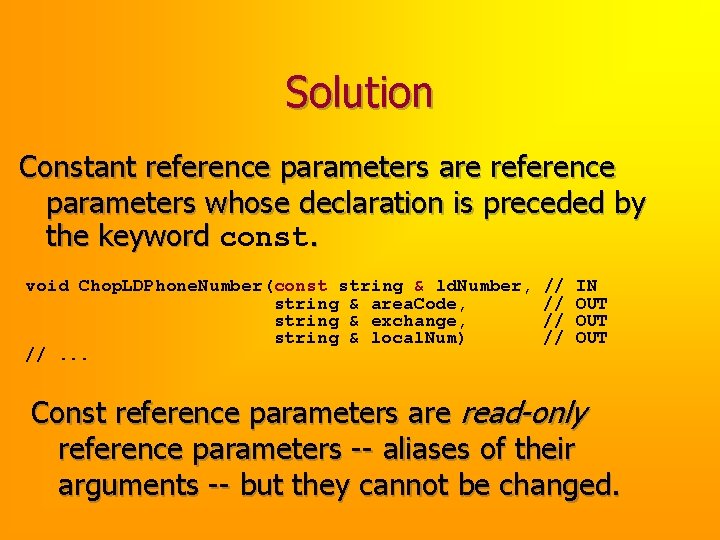
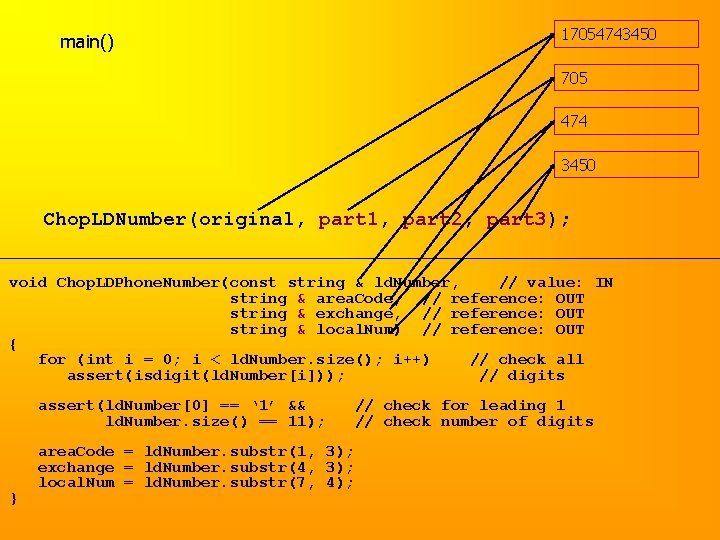
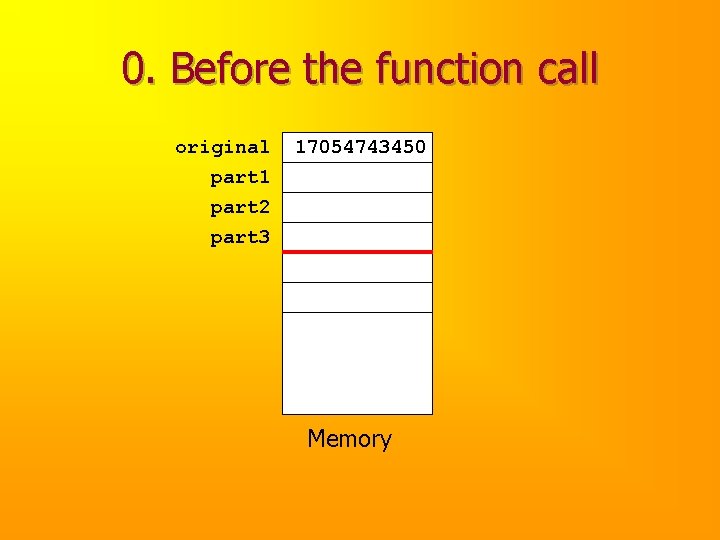
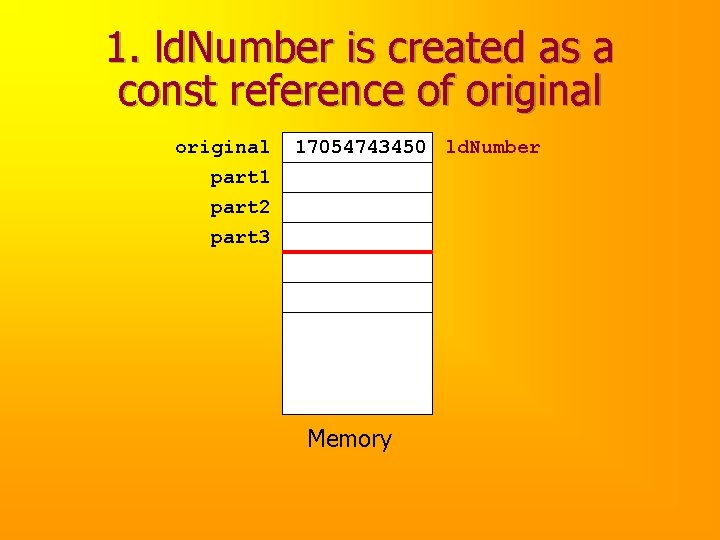
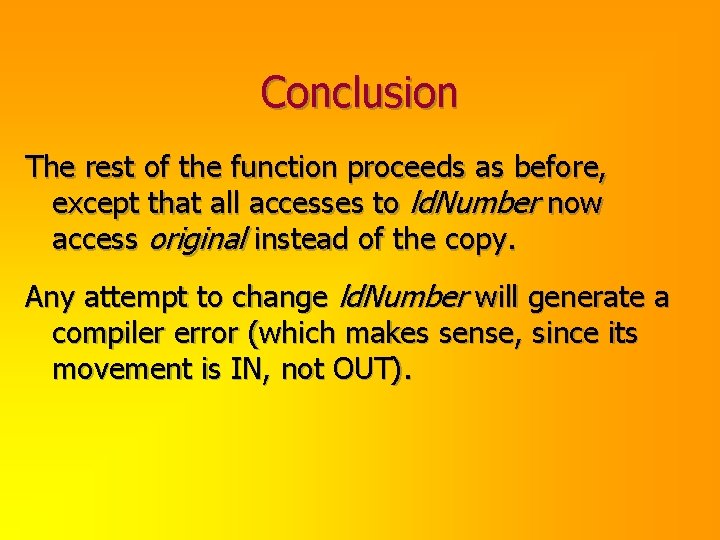
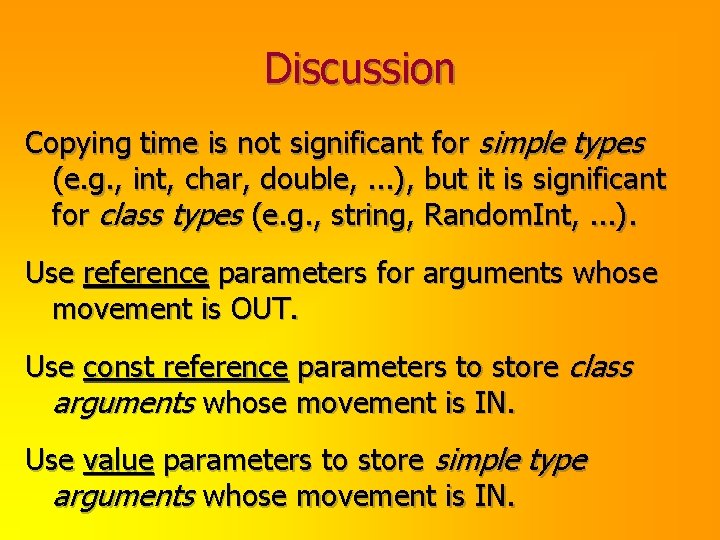
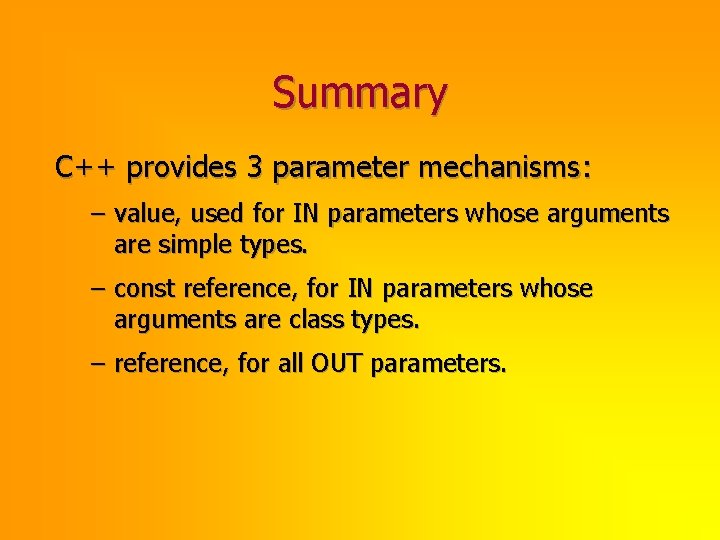
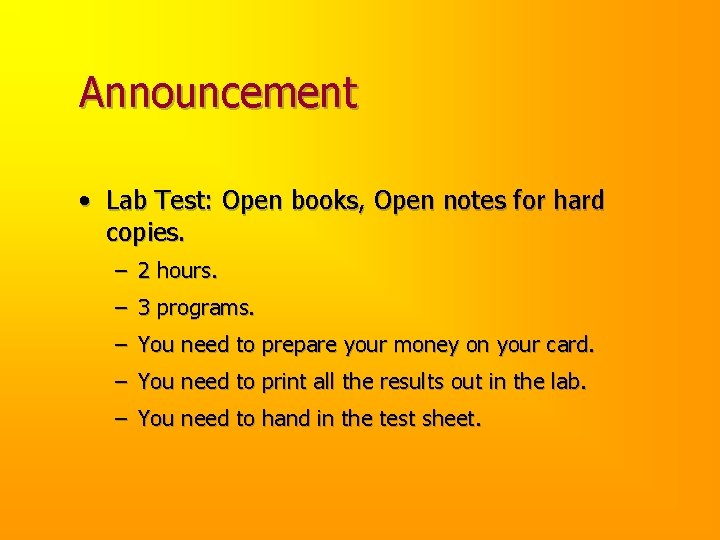
- Slides: 39
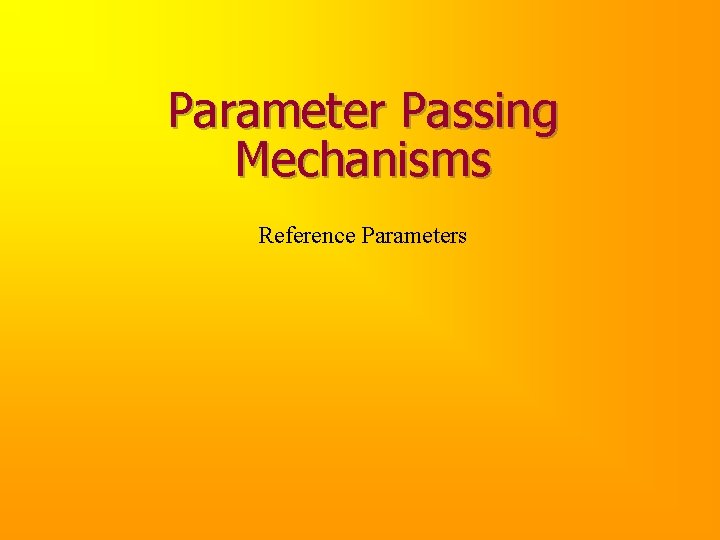
Parameter Passing Mechanisms Reference Parameters
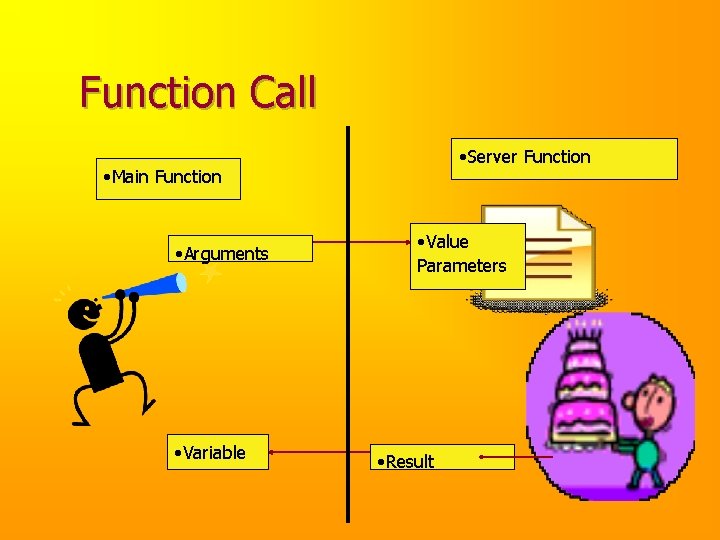
Function Call • Server Function • Main Function • Arguments • Variable • Value Parameters • Result
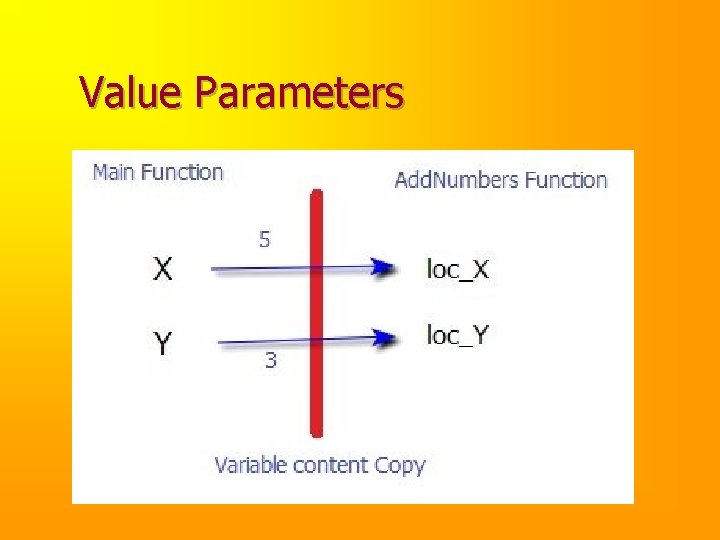
Value Parameters
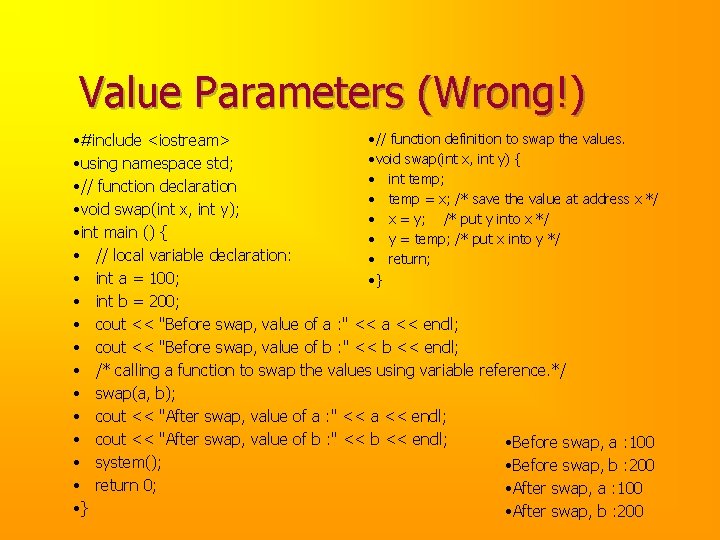
Value Parameters (Wrong!) • // function definition to swap the values. • #include <iostream> • void swap(int x, int y) { • using namespace std; • int temp; • // function declaration • temp = x; /* save the value at address x */ • void swap(int x, int y); • x = y; /* put y into x */ • int main () { • y = temp; /* put x into y */ • // local variable declaration: • return; • int a = 100; • } • int b = 200; • cout << "Before swap, value of a : " << a << endl; • cout << "Before swap, value of b : " << b << endl; • /* calling a function to swap the values using variable reference. */ • swap(a, b); • cout << "After swap, value of a : " << a << endl; • cout << "After swap, value of b : " << b << endl; • Before swap, a : 100 • system(); • Before swap, b : 200 • return 0; • After swap, a : 100 • } • After swap, b : 200
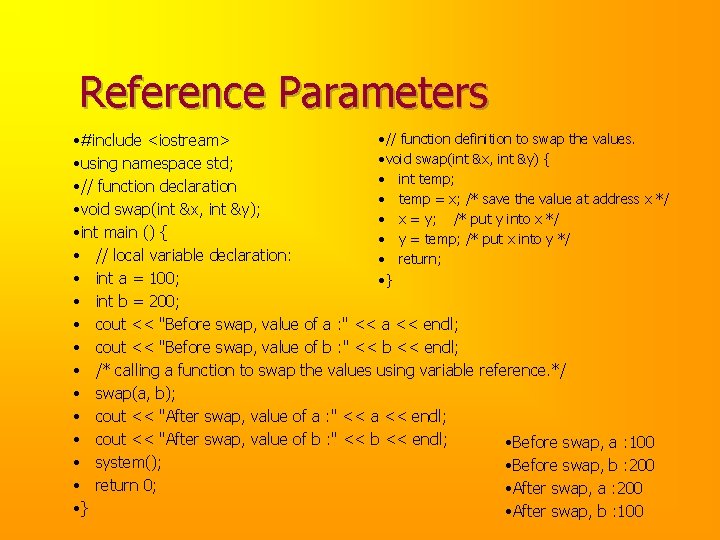
Reference Parameters • // function definition to swap the values. • #include <iostream> • void swap(int &x, int &y) { • using namespace std; • int temp; • // function declaration • temp = x; /* save the value at address x */ • void swap(int &x, int &y); • x = y; /* put y into x */ • int main () { • y = temp; /* put x into y */ • // local variable declaration: • return; • int a = 100; • } • int b = 200; • cout << "Before swap, value of a : " << a << endl; • cout << "Before swap, value of b : " << b << endl; • /* calling a function to swap the values using variable reference. */ • swap(a, b); • cout << "After swap, value of a : " << a << endl; • cout << "After swap, value of b : " << b << endl; • Before swap, a : 100 • system(); • Before swap, b : 200 • return 0; • After swap, a : 200 • } • After swap, b : 100
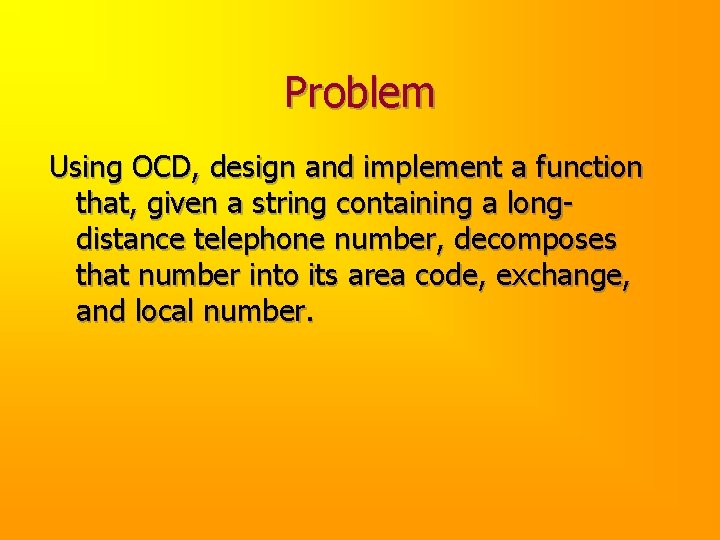
Problem Using OCD, design and implement a function that, given a string containing a longdistance telephone number, decomposes that number into its area code, exchange, and local number.
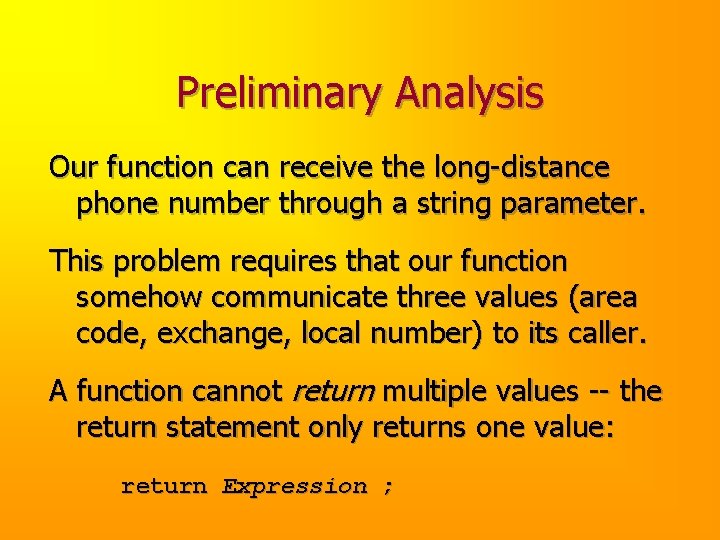
Preliminary Analysis Our function can receive the long-distance phone number through a string parameter. This problem requires that our function somehow communicate three values (area code, exchange, local number) to its caller. A function cannot return multiple values -- the return statement only returns one value: return Expression ;
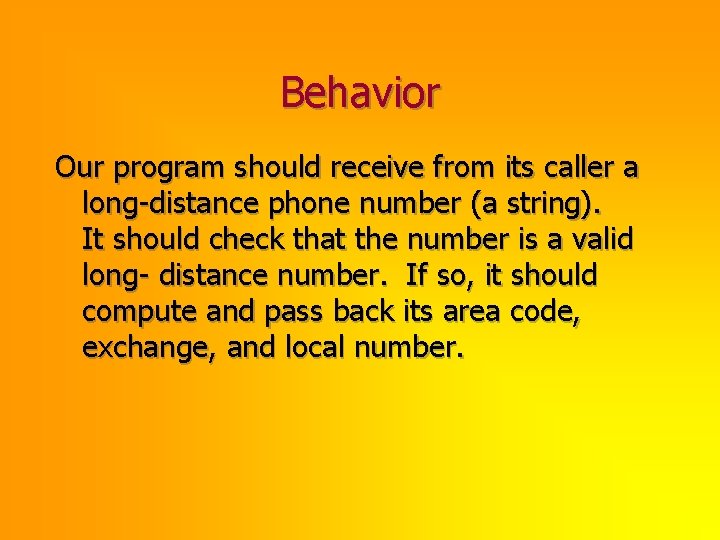
Behavior Our program should receive from its caller a long-distance phone number (a string). It should check that the number is a valid long- distance number. If so, it should compute and pass back its area code, exchange, and local number.
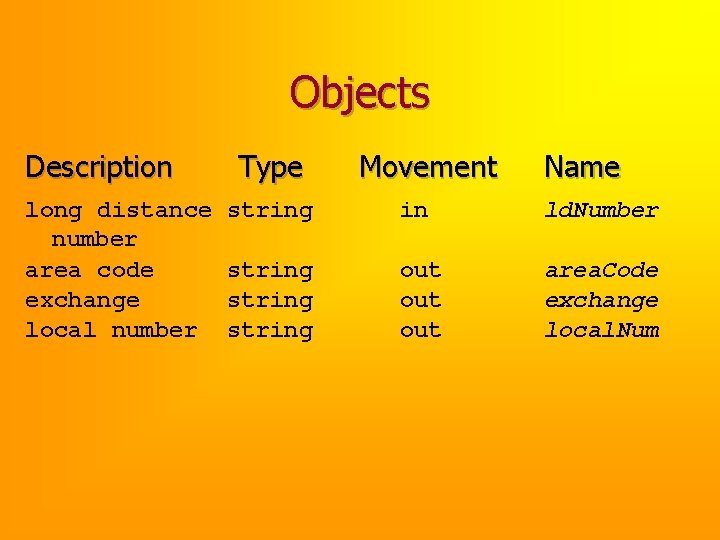
Objects Description long distance number area code exchange local number Type Movement Name string in ld. Number string out out area. Code exchange local. Num
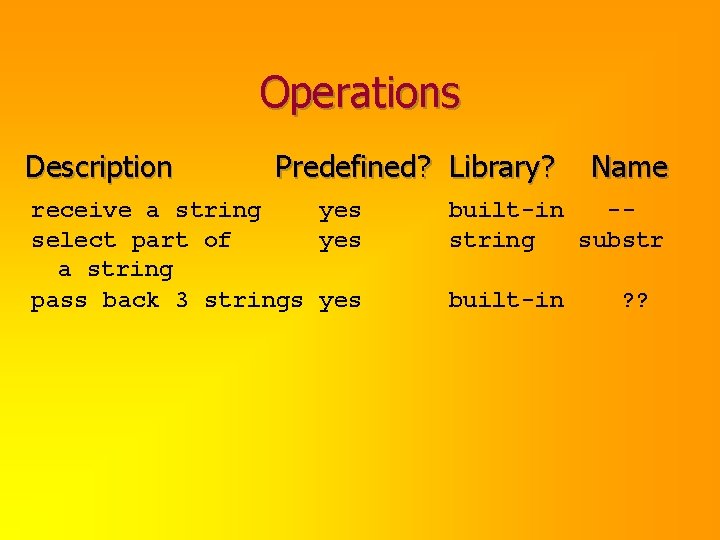
Operations Description Predefined? Library? receive a string yes select part of yes a string pass back 3 strings yes Name built-in -string substr built-in ? ?
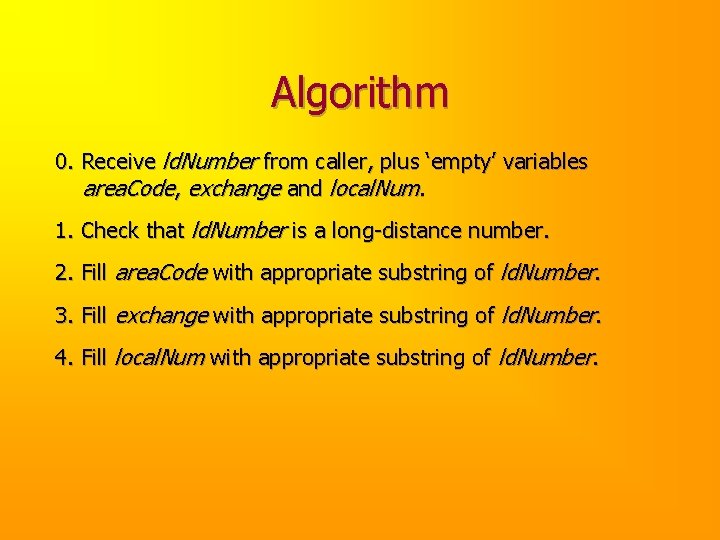
Algorithm 0. Receive ld. Number from caller, plus ‘empty’ variables area. Code, exchange and local. Num. 1. Check that ld. Number is a long-distance number. 2. Fill area. Code with appropriate substring of ld. Number. 3. Fill exchange with appropriate substring of ld. Number. 4. Fill local. Num with appropriate substring of ld. Number.
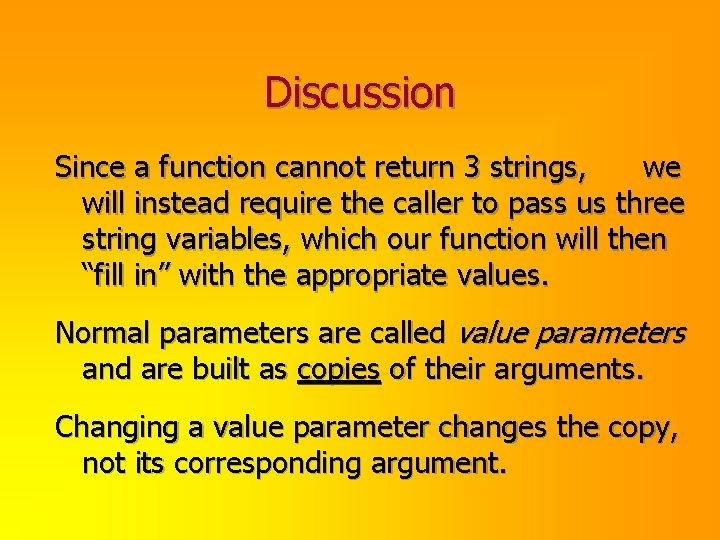
Discussion Since a function cannot return 3 strings, we will instead require the caller to pass us three string variables, which our function will then “fill in” with the appropriate values. Normal parameters are called value parameters and are built as copies of their arguments. Changing a value parameter changes the copy, not its corresponding argument.
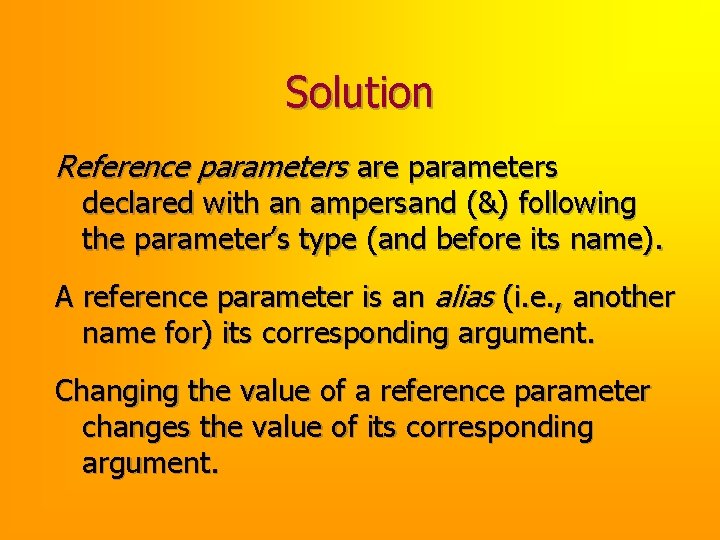
Solution Reference parameters are parameters declared with an ampersand (&) following the parameter’s type (and before its name). A reference parameter is an alias (i. e. , another name for) its corresponding argument. Changing the value of a reference parameter changes the value of its corresponding argument.
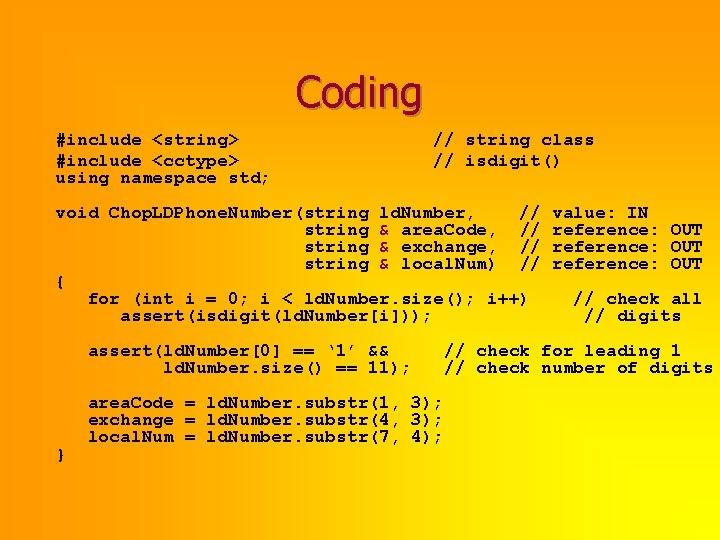
Coding #include <string> #include <cctype> using namespace std; // string class // isdigit() void Chop. LDPhone. Number(string ld. Number, // string & area. Code, // string & exchange, // string & local. Num) // { for (int i = 0; i < ld. Number. size(); i++) assert(isdigit(ld. Number[i])); assert(ld. Number[0] == ‘ 1’ && ld. Number. size() == 11); } value: IN reference: OUT // check all // digits area. Code = ld. Number. substr(1, 3); exchange = ld. Number. substr(4, 3); local. Num = ld. Number. substr(7, 4); // check for leading 1 // check number of digits
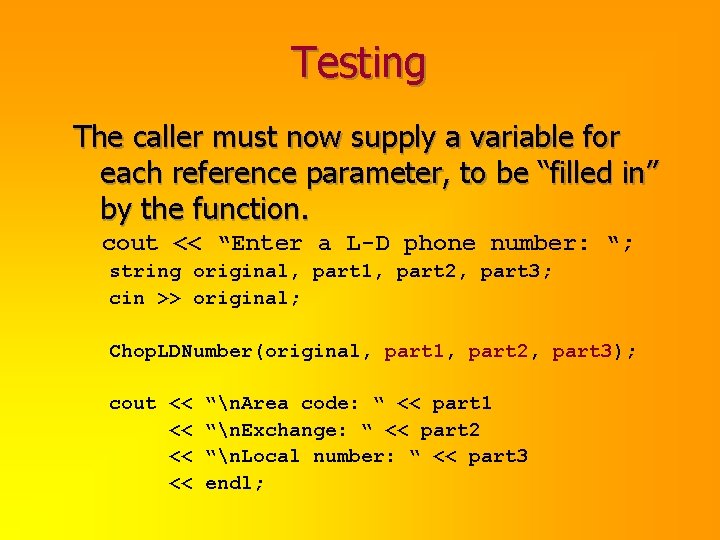
Testing The caller must now supply a variable for each reference parameter, to be “filled in” by the function. cout << “Enter a L-D phone number: “; string original, part 1, part 2, part 3; cin >> original; Chop. LDNumber(original, part 1, part 2, part 3); cout << << “n. Area code: “ << part 1 “n. Exchange: “ << part 2 “n. Local number: “ << part 3 endl;
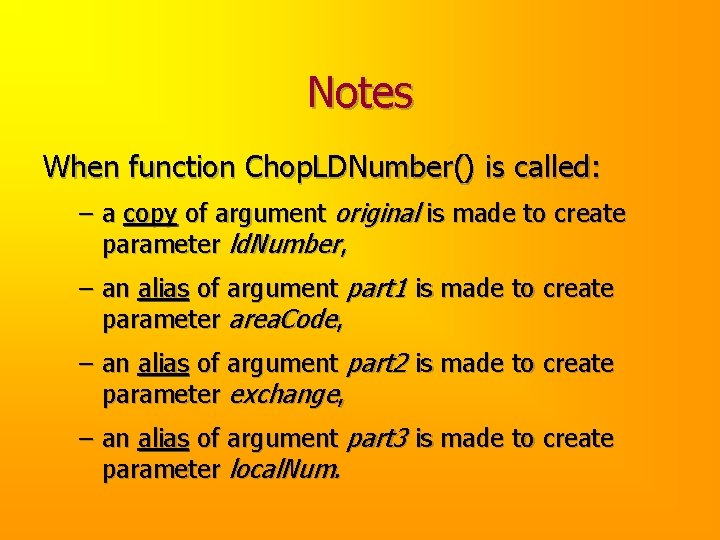
Notes When function Chop. LDNumber() is called: – a copy of argument original is made to create parameter ld. Number, – an alias of argument part 1 is made to create parameter area. Code, – an alias of argument part 2 is made to create parameter exchange, – an alias of argument part 3 is made to create parameter local. Num.
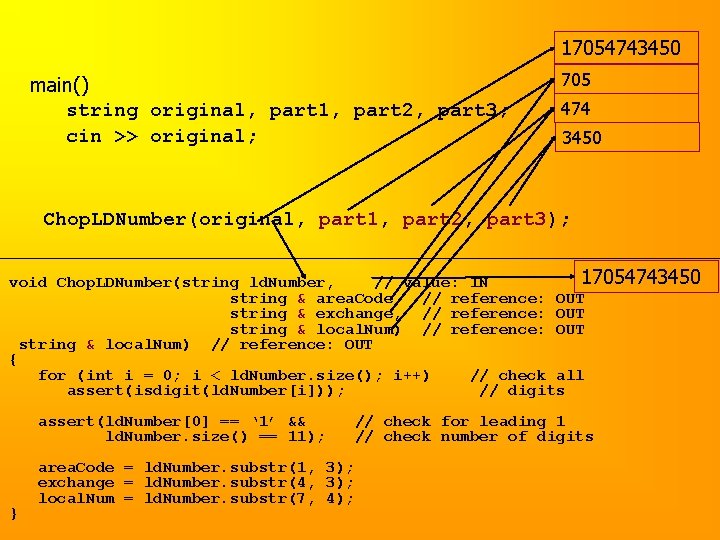
17054743450 main() string original, part 1, part 2, part 3; cin >> original; 705 474 3450 Chop. LDNumber(original, part 1, part 2, part 3); 17054743450 void Chop. LDNumber(string ld. Number, // value: IN string & area. Code, // reference: OUT string & exchange, // reference: OUT string & local. Num) // reference: OUT { for (int i = 0; i < ld. Number. size(); i++) // check all assert(isdigit(ld. Number[i])); // digits assert(ld. Number[0] == ‘ 1’ && ld. Number. size() == 11); } area. Code = ld. Number. substr(1, 3); exchange = ld. Number. substr(4, 3); local. Num = ld. Number. substr(7, 4); // check for leading 1 // check number of digits
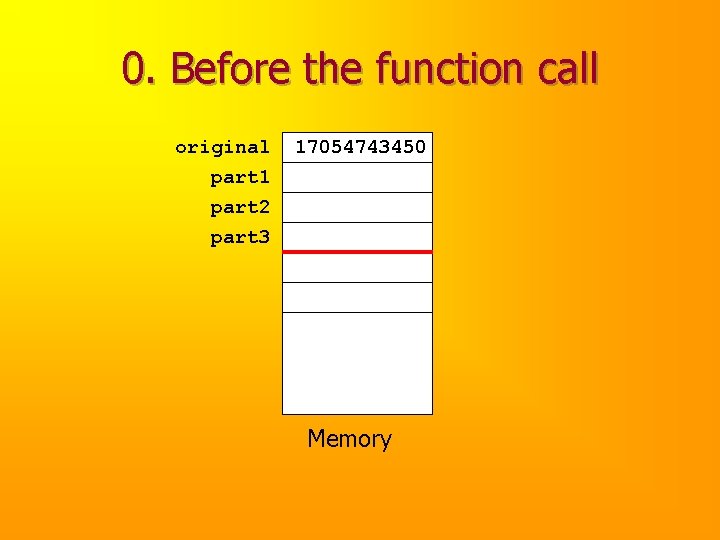
0. Before the function call original part 1 part 2 part 3 17054743450 Memory
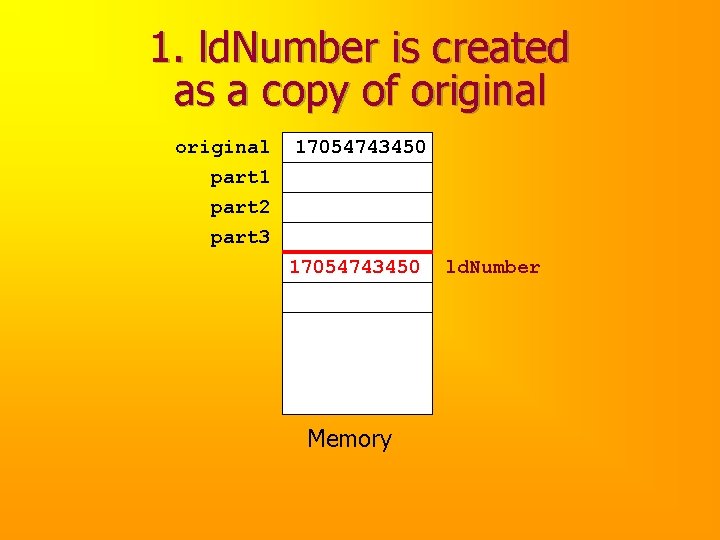
1. ld. Number is created as a copy of original part 1 part 2 part 3 17054743450 Memory ld. Number
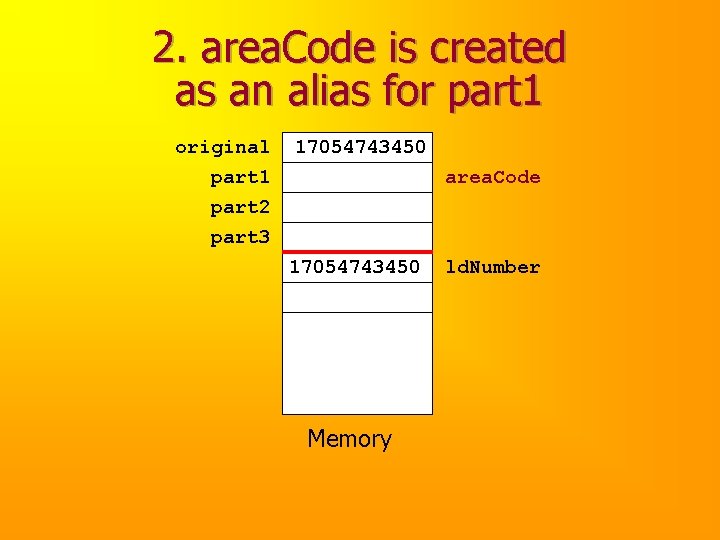
2. area. Code is created as an alias for part 1 original part 1 part 2 part 3 17054743450 area. Code 17054743450 Memory ld. Number
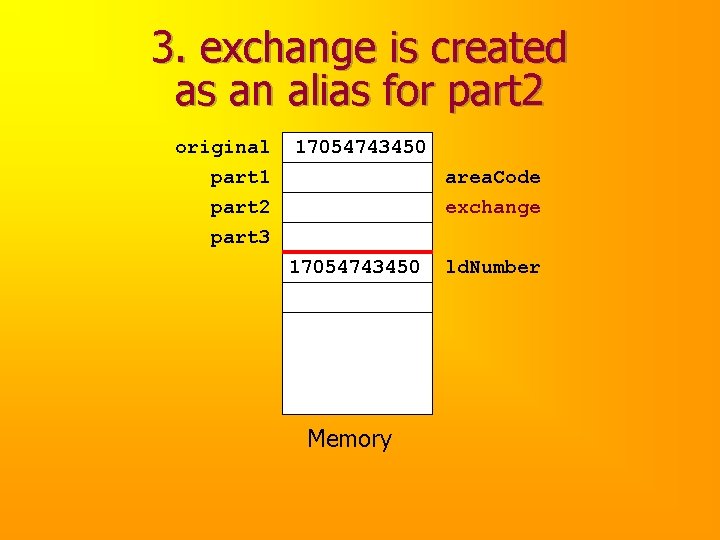
3. exchange is created as an alias for part 2 original part 1 part 2 part 3 17054743450 area. Code exchange 17054743450 Memory ld. Number
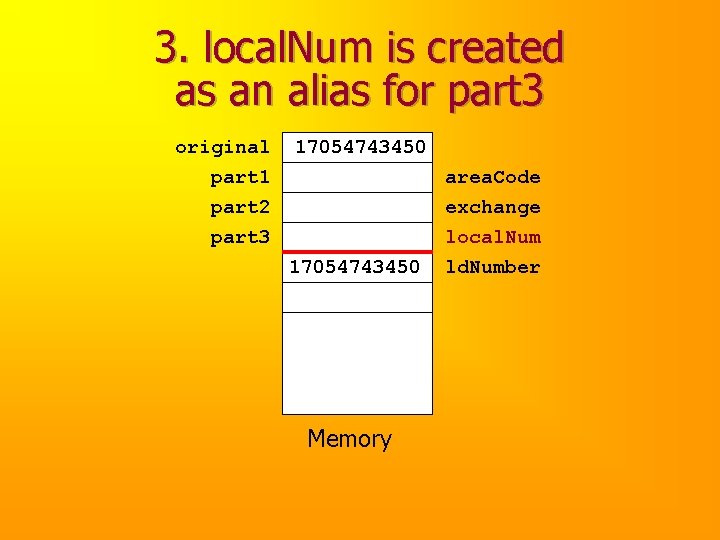
3. local. Num is created as an alias for part 3 original part 1 part 2 part 3 17054743450 Memory area. Code exchange local. Num ld. Number
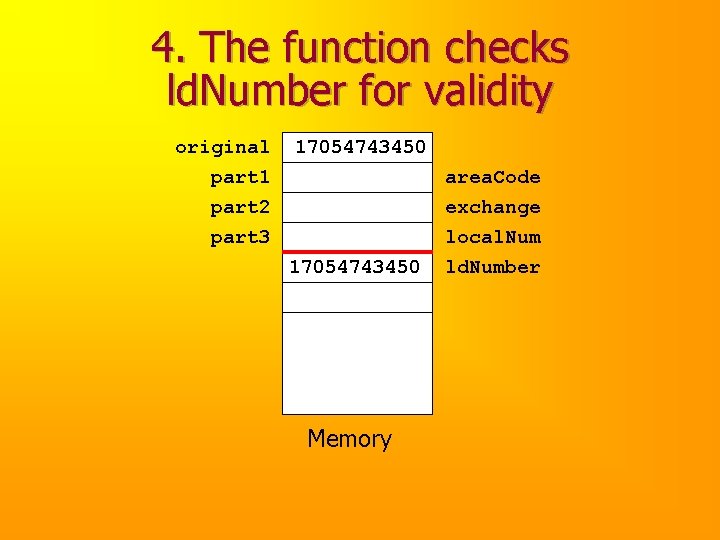
4. The function checks ld. Number for validity original part 1 part 2 part 3 17054743450 Memory area. Code exchange local. Num ld. Number
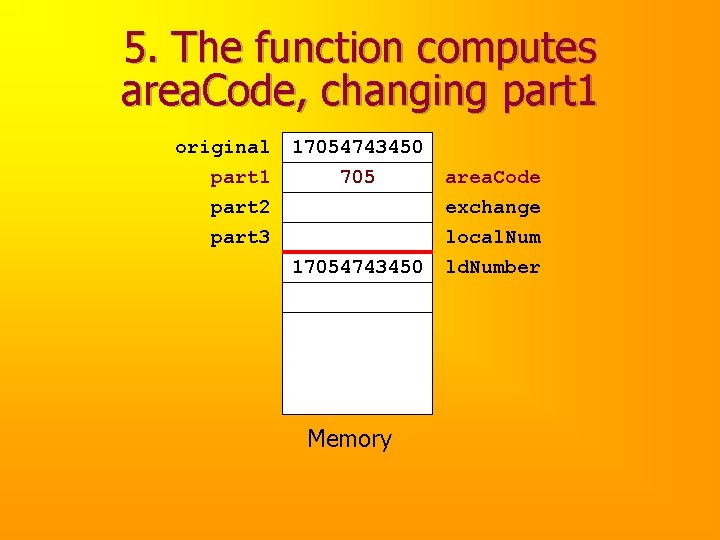
5. The function computes area. Code, changing part 1 original part 1 part 2 part 3 17054743450 705 17054743450 Memory area. Code exchange local. Num ld. Number
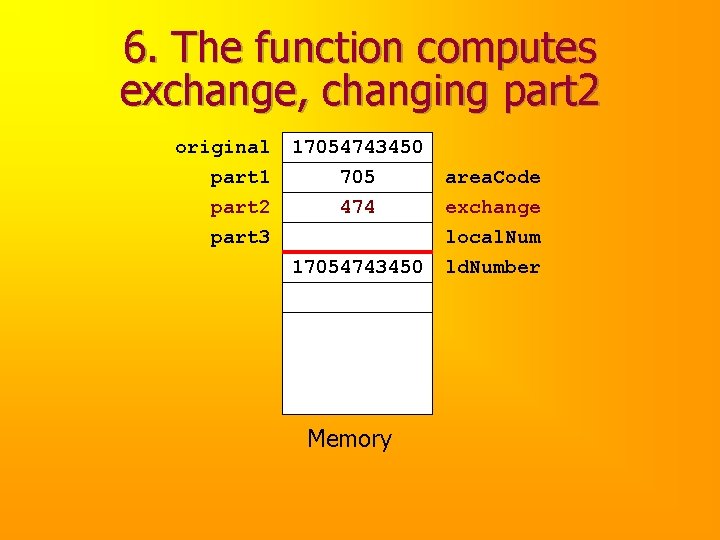
6. The function computes exchange, changing part 2 original part 1 part 2 part 3 17054743450 705 474 17054743450 Memory area. Code exchange local. Num ld. Number
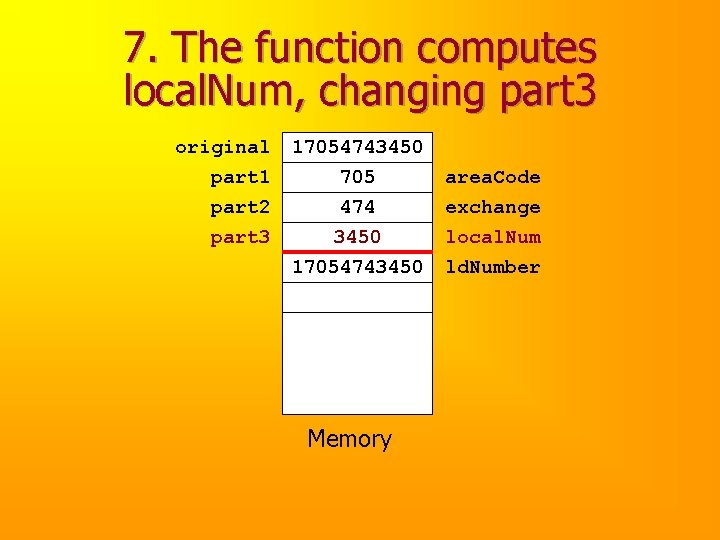
7. The function computes local. Num, changing part 3 original part 1 part 2 part 3 17054743450 705 474 3450 17054743450 Memory area. Code exchange local. Num ld. Number
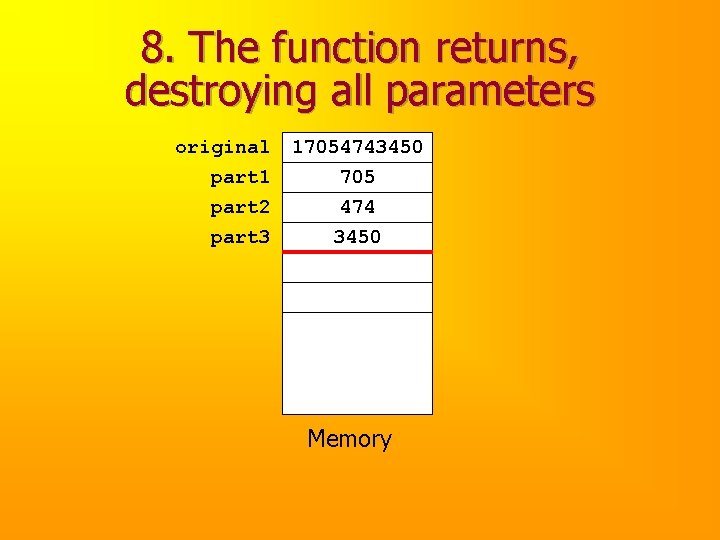
8. The function returns, destroying all parameters original part 1 part 2 part 3 17054743450 705 474 3450 Memory
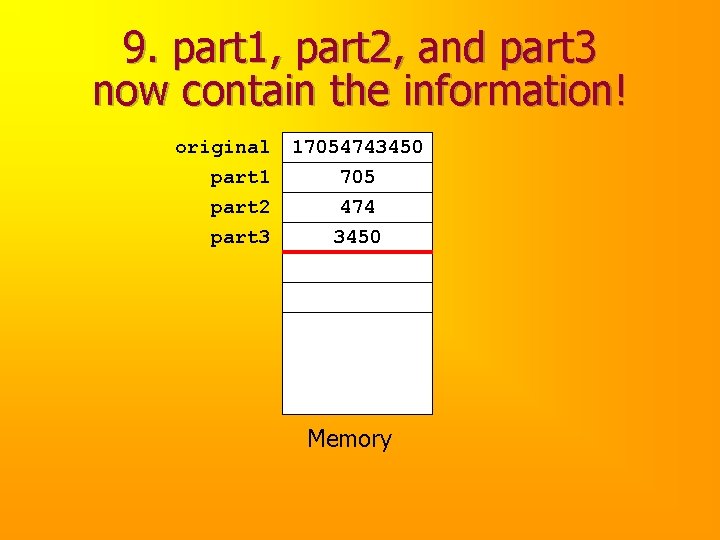
9. part 1, part 2, and part 3 now contain the information! original part 1 part 2 part 3 17054743450 705 474 3450 Memory
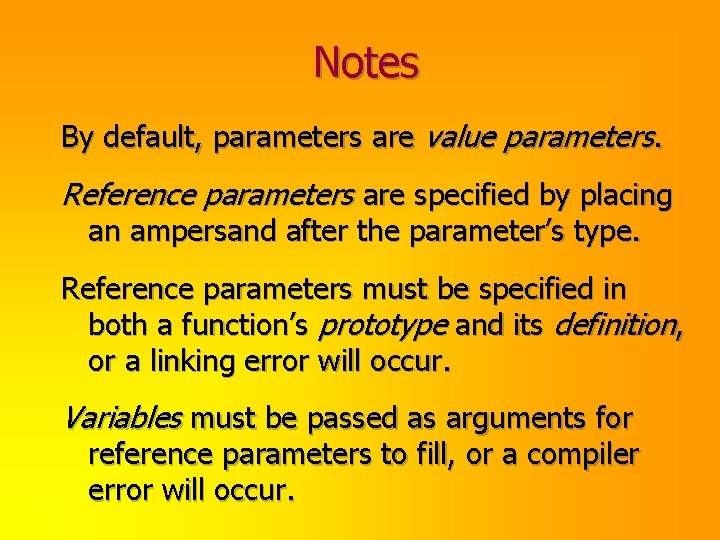
Notes By default, parameters are value parameters. Reference parameters are specified by placing an ampersand after the parameter’s type. Reference parameters must be specified in both a function’s prototype and its definition, or a linking error will occur. Variables must be passed as arguments for reference parameters to fill, or a compiler error will occur.
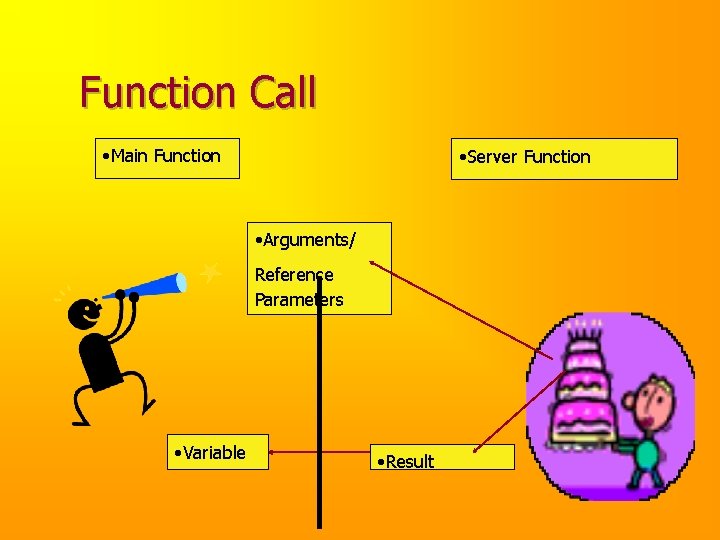
Function Call • Main Function • Server Function • Arguments/ Reference Parameters • Variable • Result
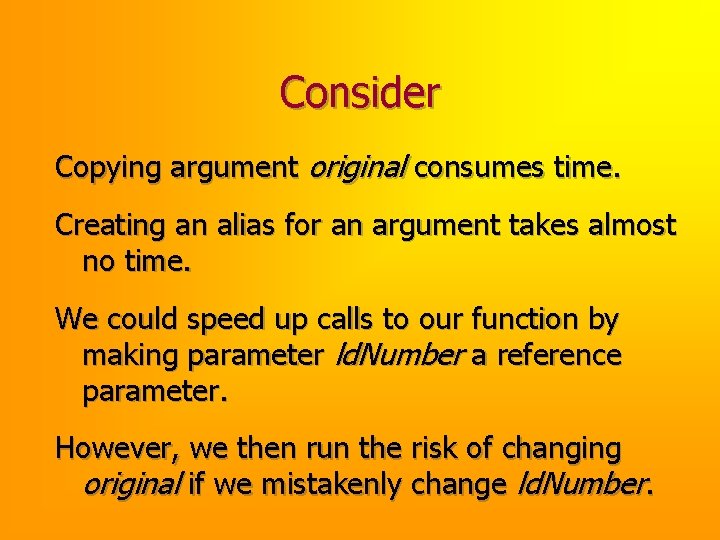
Consider Copying argument original consumes time. Creating an alias for an argument takes almost no time. We could speed up calls to our function by making parameter ld. Number a reference parameter. However, we then run the risk of changing original if we mistakenly change ld. Number.
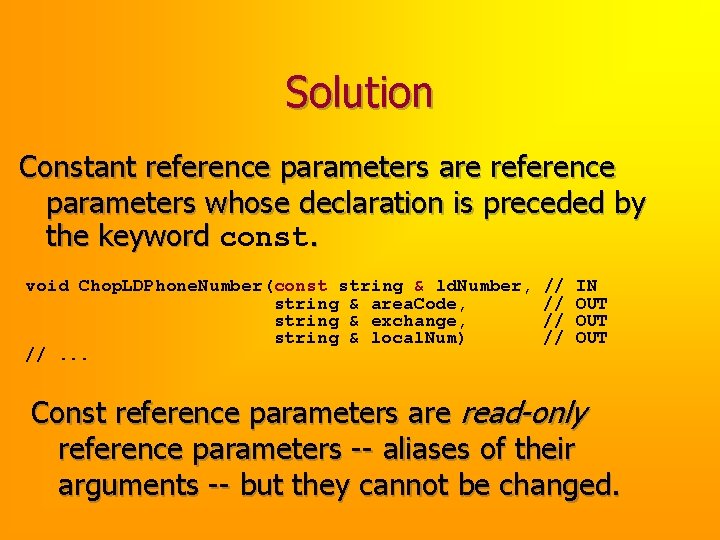
Solution Constant reference parameters are reference parameters whose declaration is preceded by the keyword const. void Chop. LDPhone. Number(const string & ld. Number, string & area. Code, string & exchange, string & local. Num) //. . . // // IN OUT OUT Const reference parameters are read-only reference parameters -- aliases of their arguments -- but they cannot be changed.
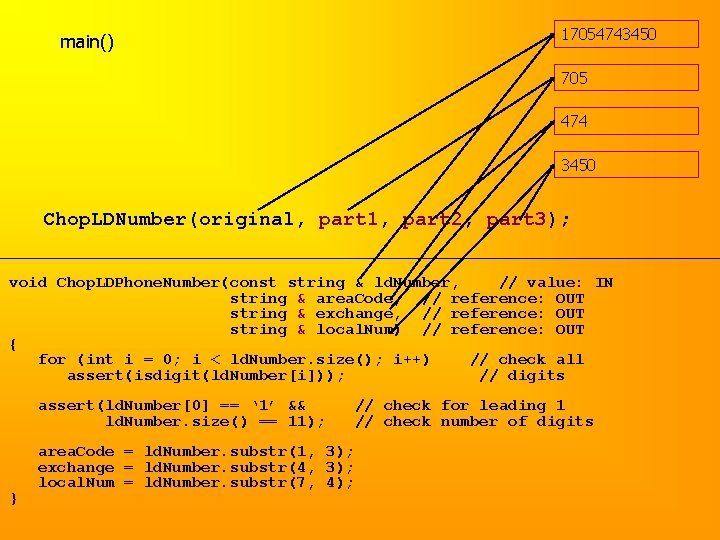
17054743450 main() 705 474 3450 Chop. LDNumber(original, part 1, part 2, part 3); void Chop. LDPhone. Number(const string & ld. Number, // value: IN string & area. Code, // reference: OUT string & exchange, // reference: OUT string & local. Num) // reference: OUT { for (int i = 0; i < ld. Number. size(); i++) // check all assert(isdigit(ld. Number[i])); // digits assert(ld. Number[0] == ‘ 1’ && ld. Number. size() == 11); } area. Code = ld. Number. substr(1, 3); exchange = ld. Number. substr(4, 3); local. Num = ld. Number. substr(7, 4); // check for leading 1 // check number of digits
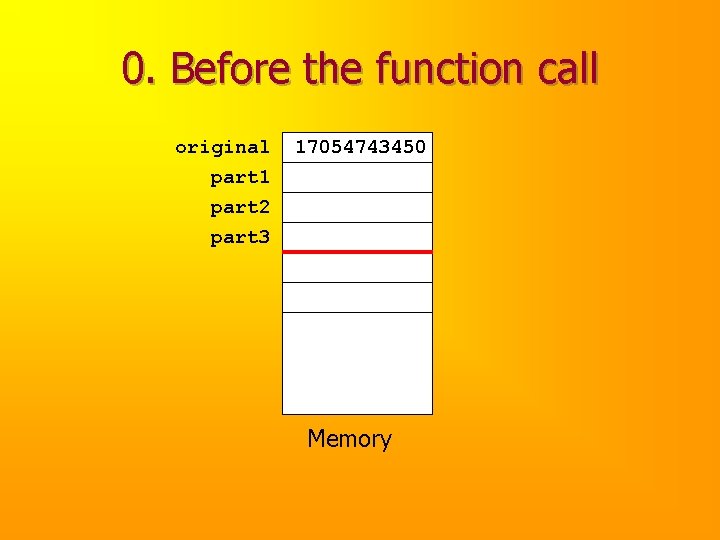
0. Before the function call original part 1 part 2 part 3 17054743450 Memory
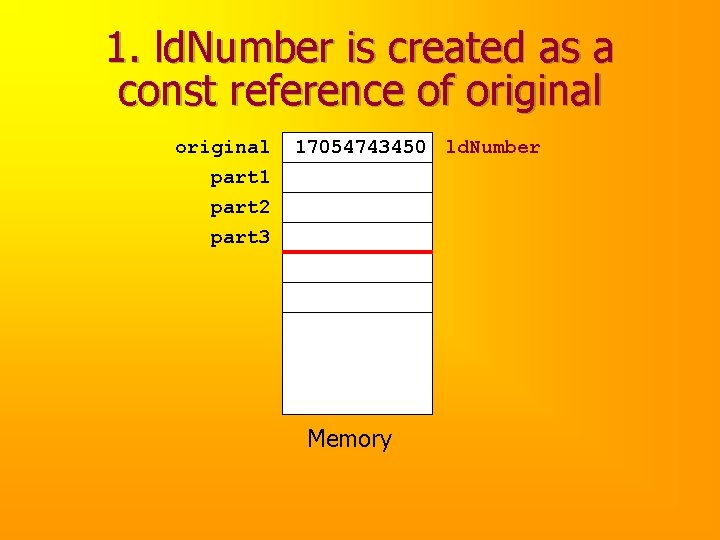
1. ld. Number is created as a const reference of original part 1 part 2 part 3 17054743450 ld. Number Memory
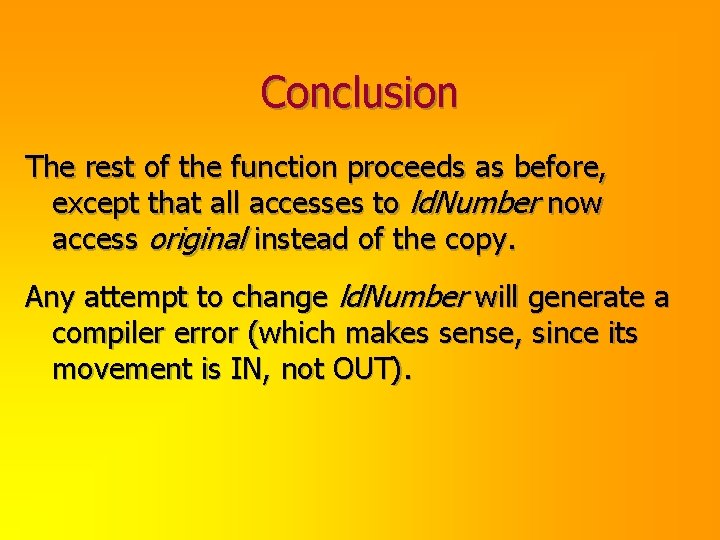
Conclusion The rest of the function proceeds as before, except that all accesses to ld. Number now access original instead of the copy. Any attempt to change ld. Number will generate a compiler error (which makes sense, since its movement is IN, not OUT).
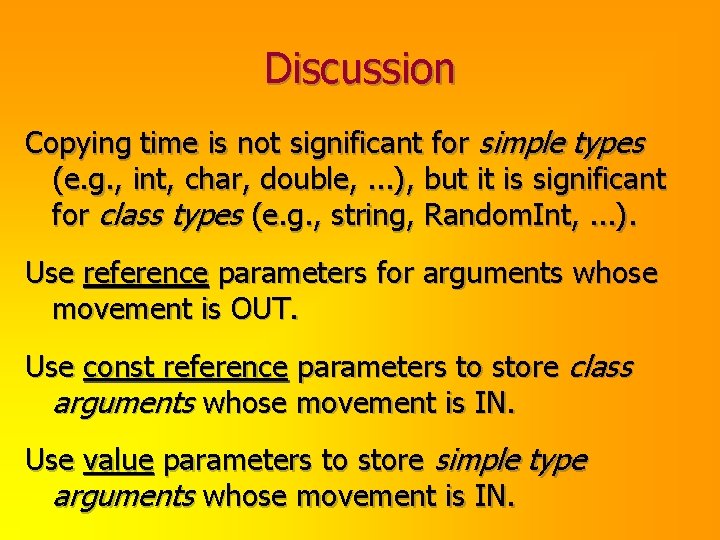
Discussion Copying time is not significant for simple types (e. g. , int, char, double, . . . ), but it is significant for class types (e. g. , string, Random. Int, . . . ). Use reference parameters for arguments whose movement is OUT. Use const reference parameters to store class arguments whose movement is IN. Use value parameters to store simple type arguments whose movement is IN.
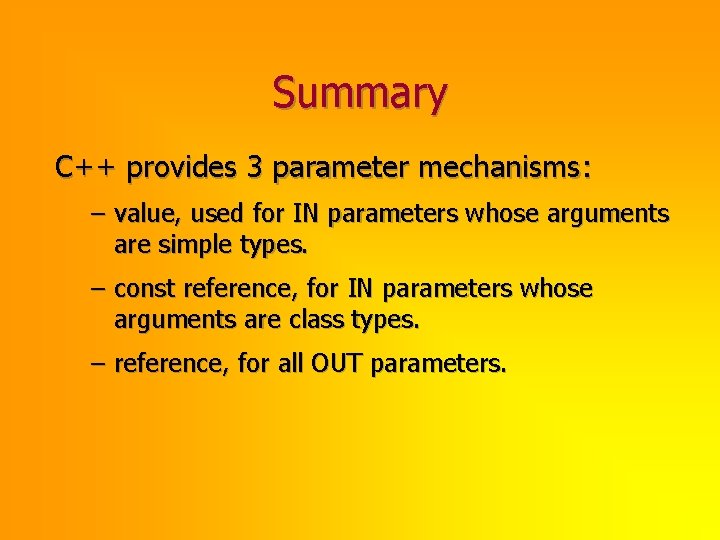
Summary C++ provides 3 parameter mechanisms: – value, used for IN parameters whose arguments are simple types. – const reference, for IN parameters whose arguments are class types. – reference, for all OUT parameters.
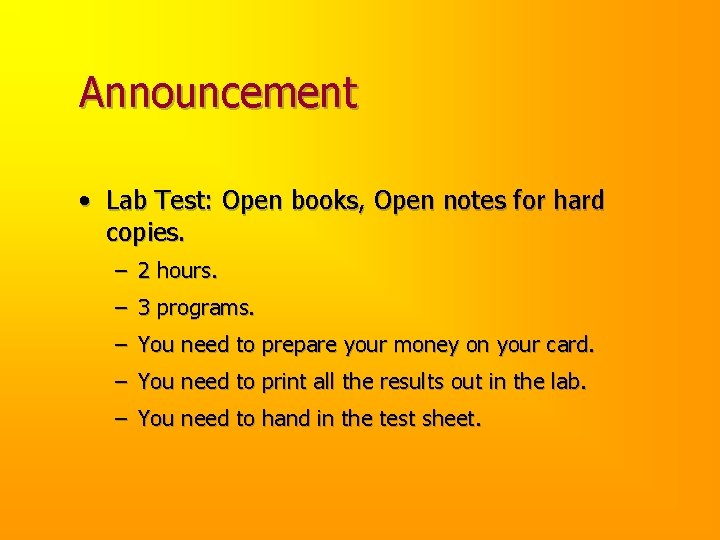
Announcement • Lab Test: Open books, Open notes for hard copies. – 2 hours. – 3 programs. – You need to prepare your money on your card. – You need to print all the results out in the lab. – You need to hand in the test sheet.