Outline Announcements High Level Synchronization Constructs Simultaneous semaphores
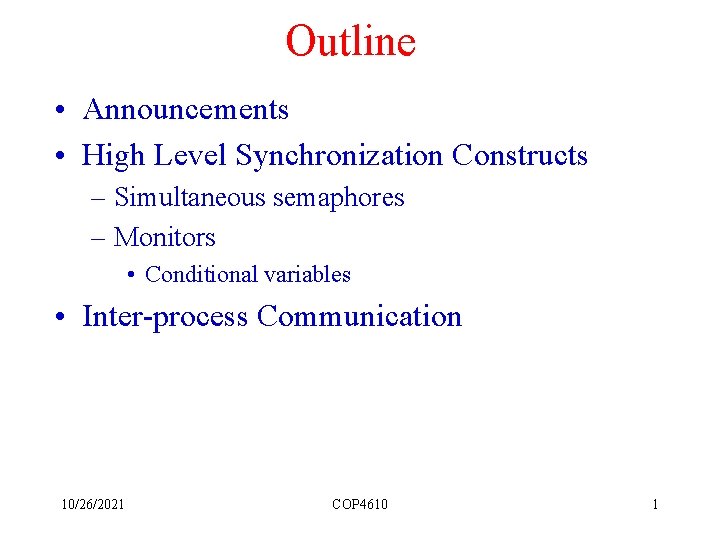
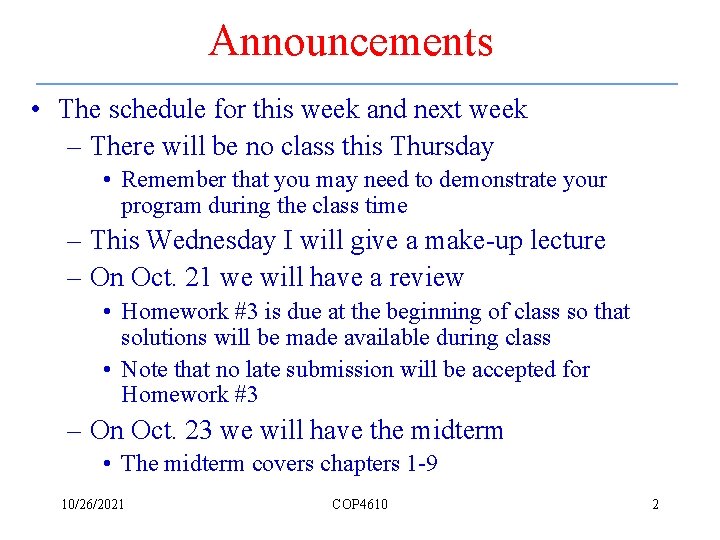
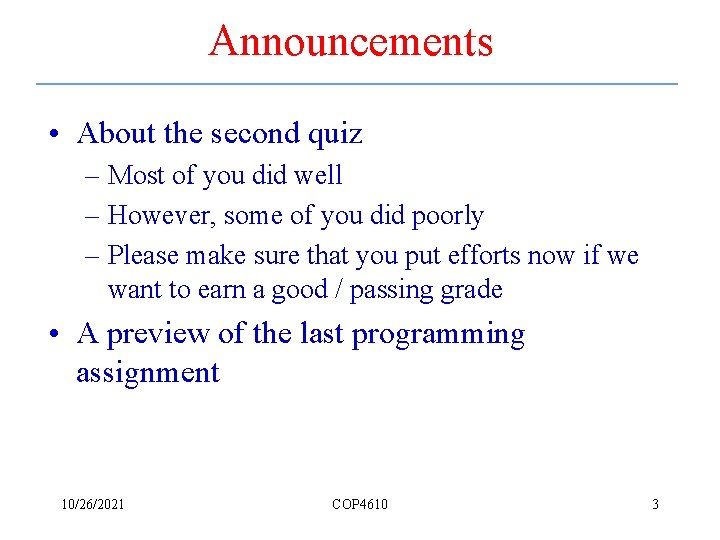
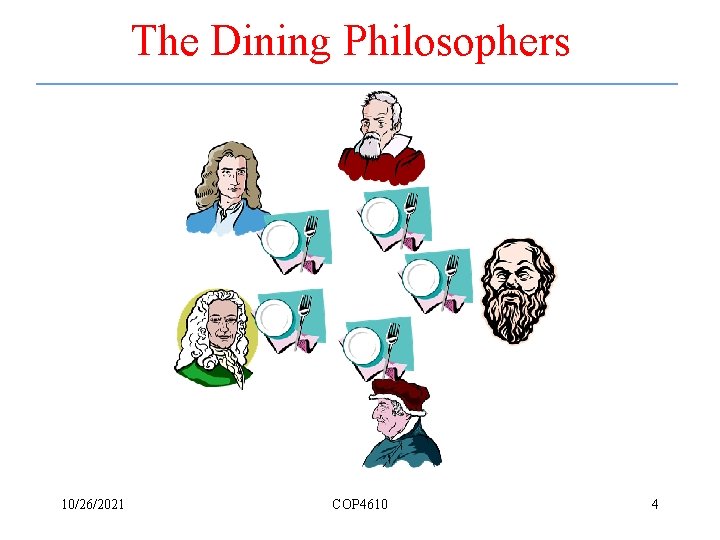
![First Try Solution philosopher(int i) { while(TRUE) { // Think // Eat P(fork[i]); P(fork[(i+1) First Try Solution philosopher(int i) { while(TRUE) { // Think // Eat P(fork[i]); P(fork[(i+1)](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-5.jpg)
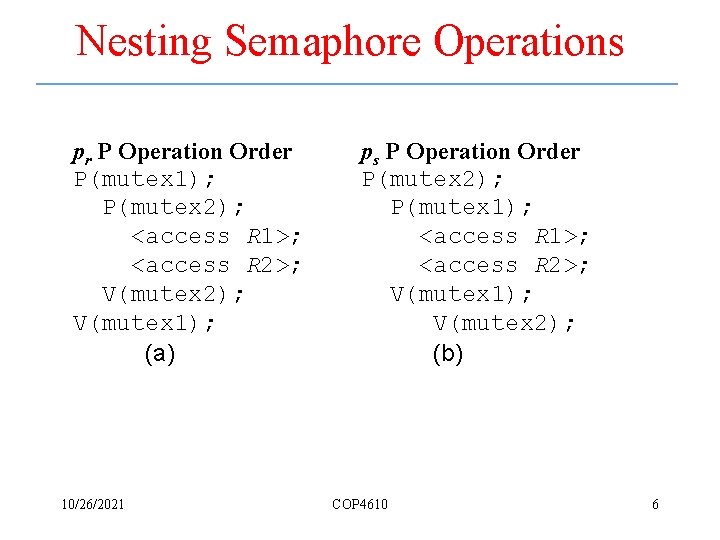
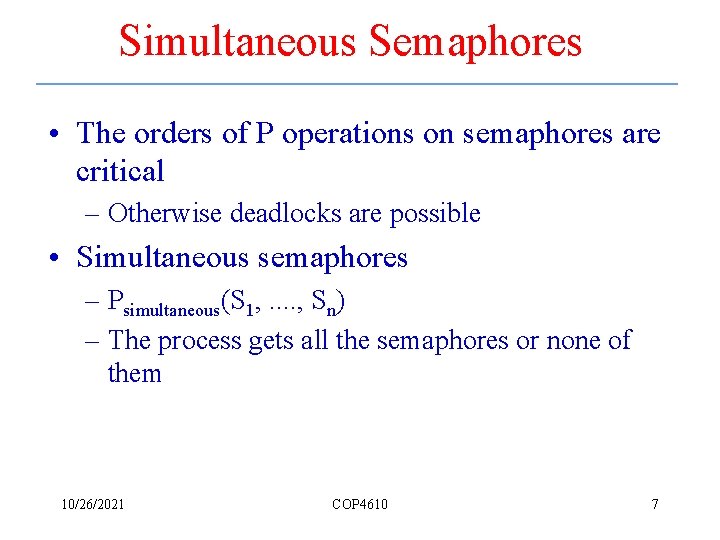
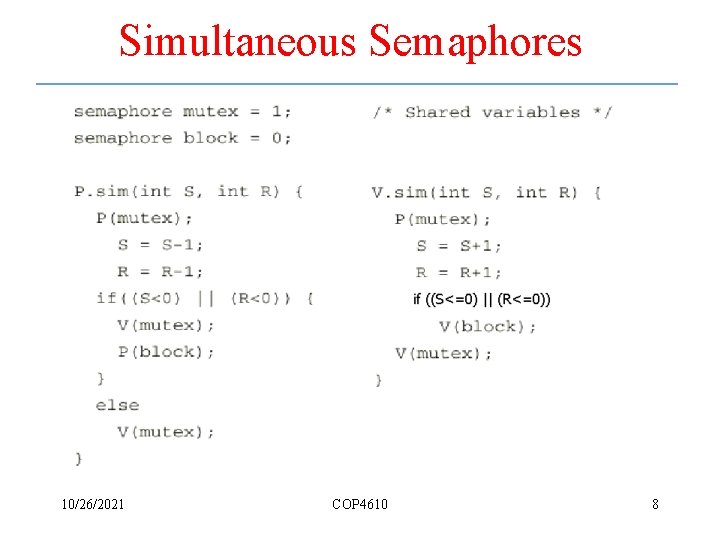
![A Solution philosopher(int i) { while(TRUE) { // Think // Eat } Psim(fork[i], fork[(i+1) A Solution philosopher(int i) { while(TRUE) { // Think // Eat } Psim(fork[i], fork[(i+1)](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-9.jpg)
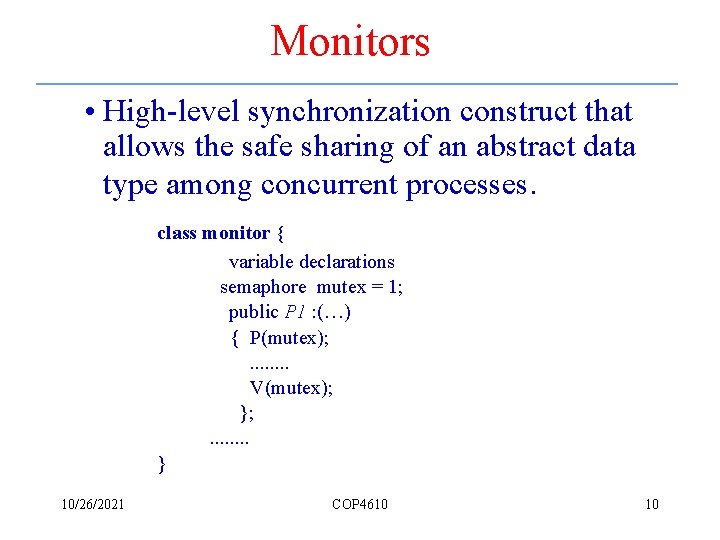
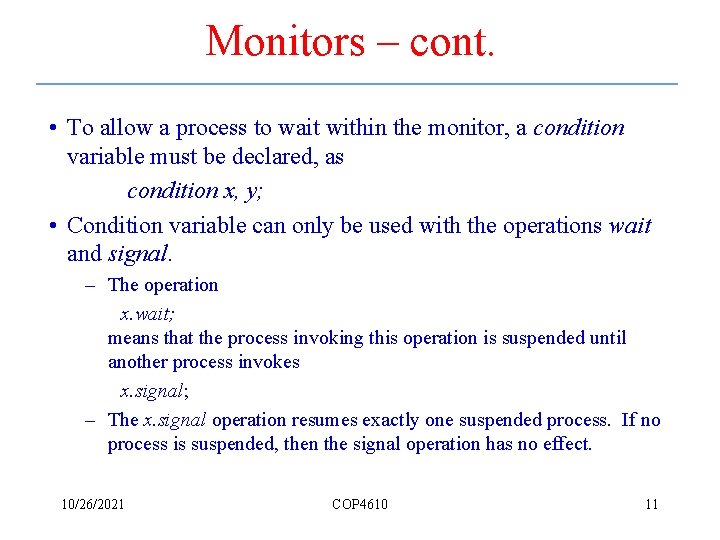
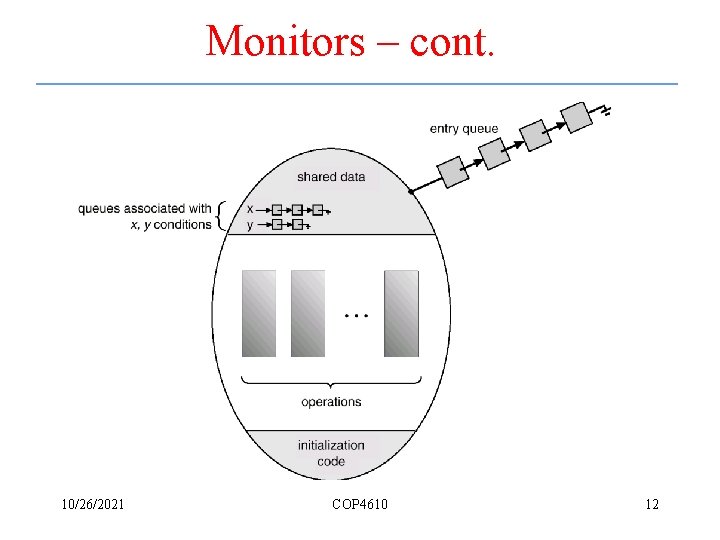
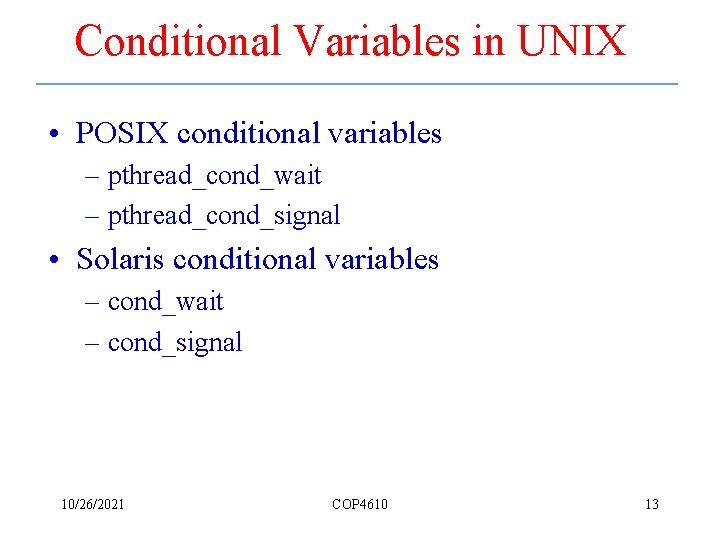
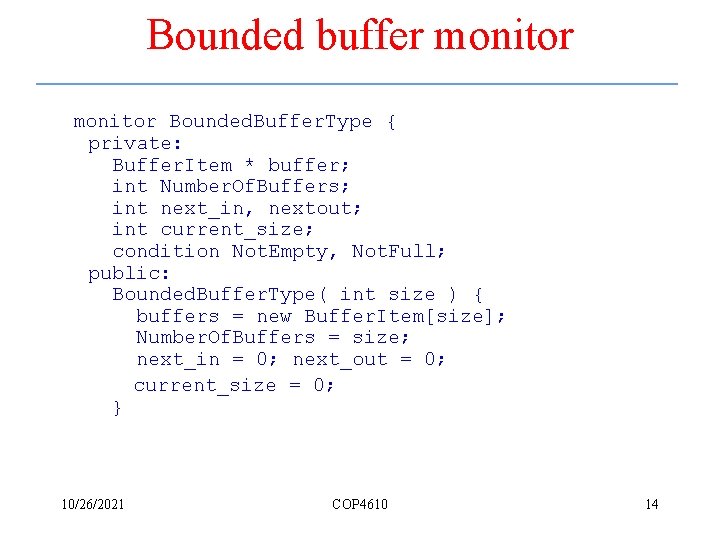
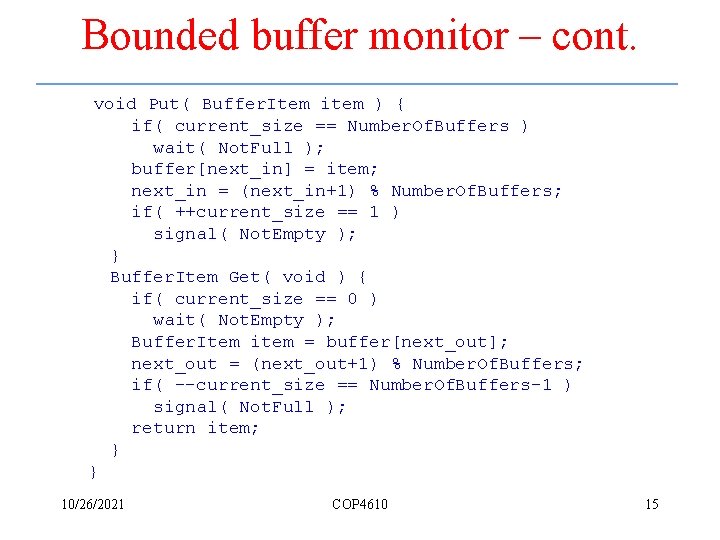
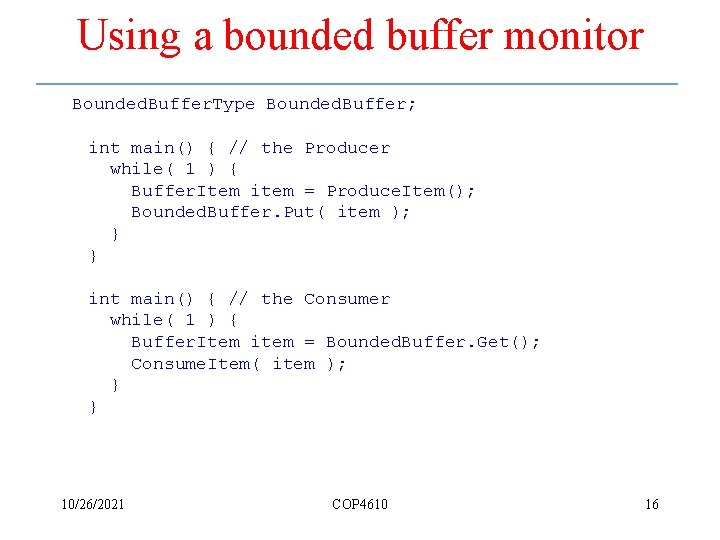
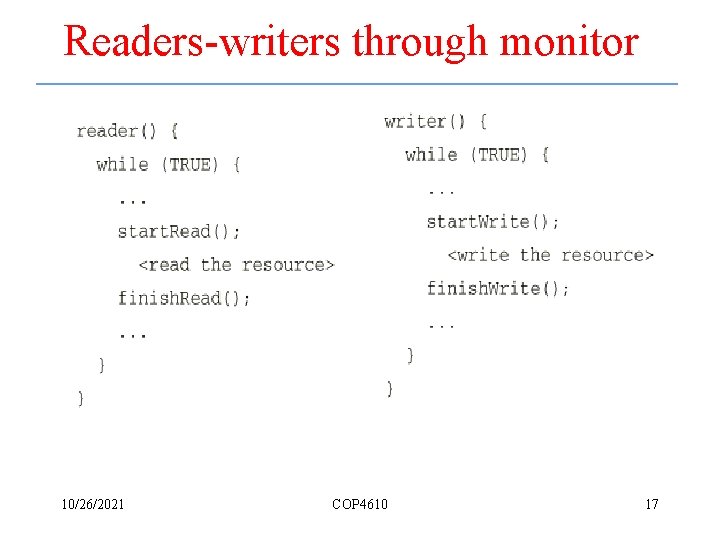
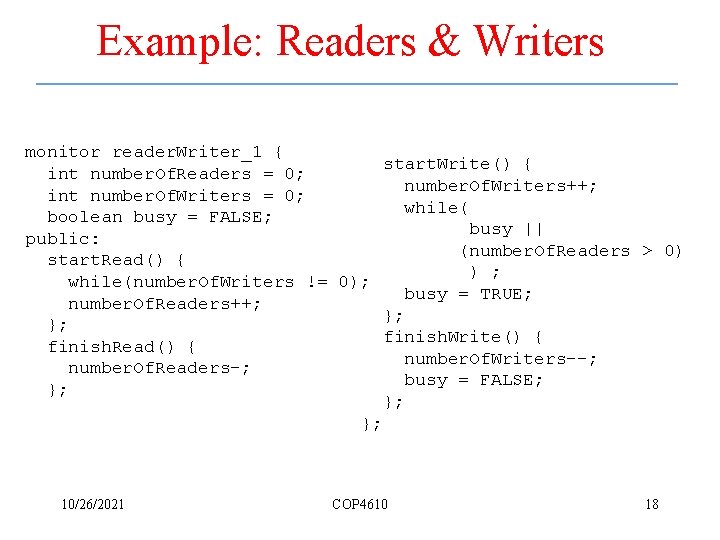
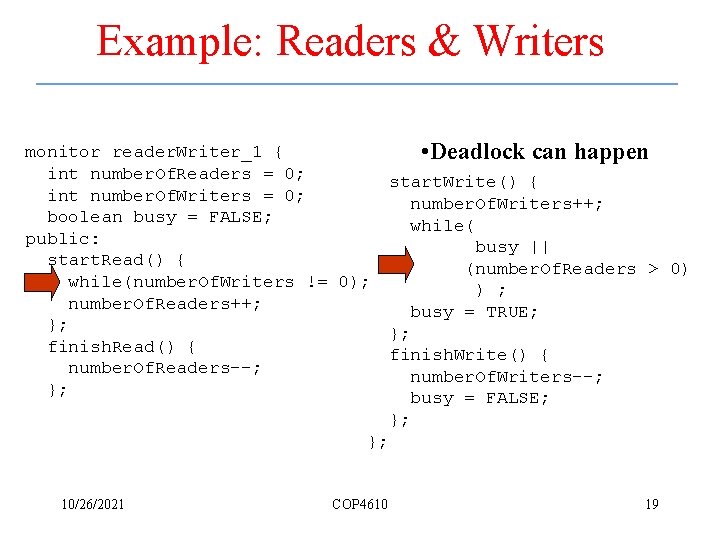
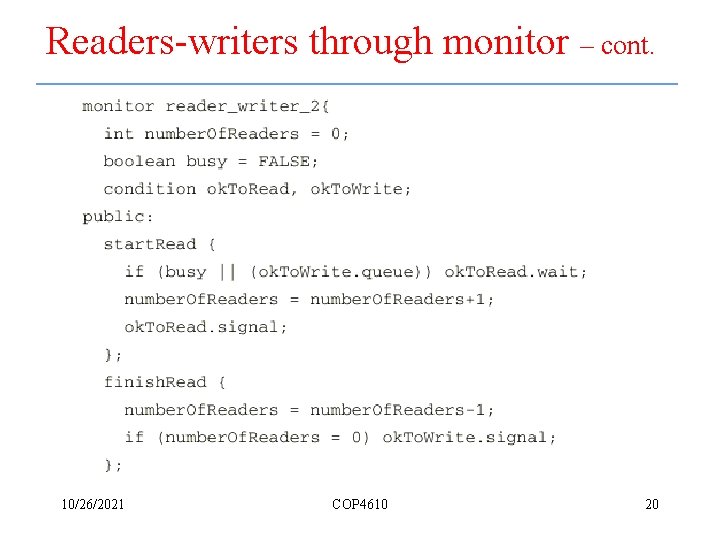
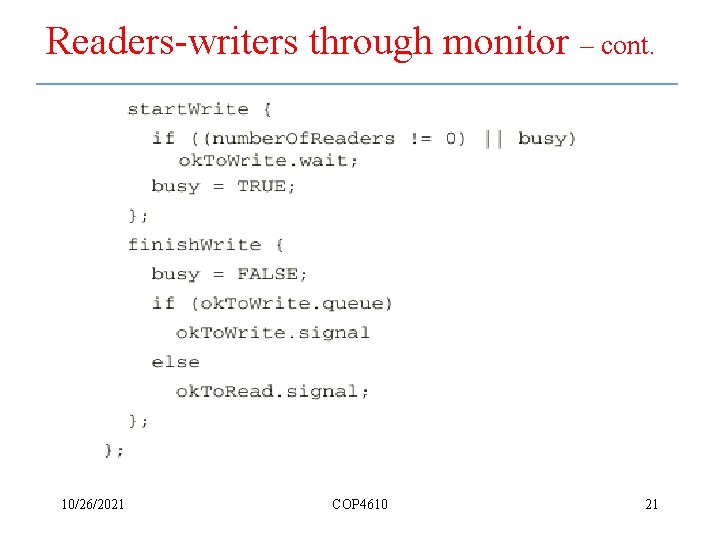
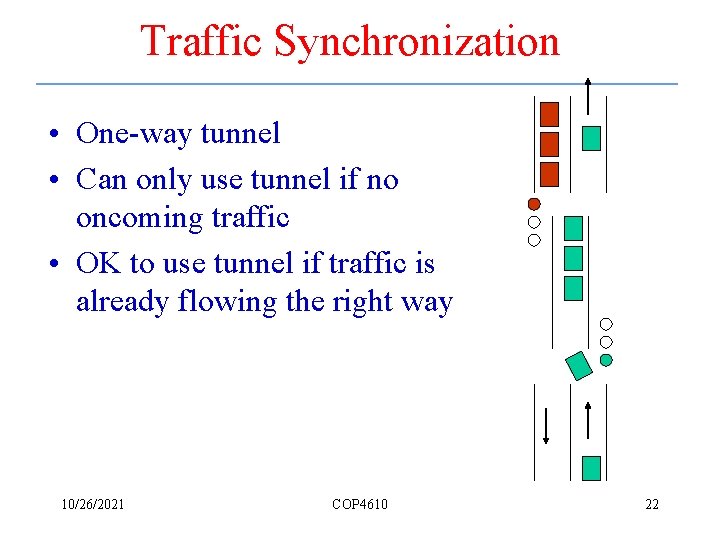
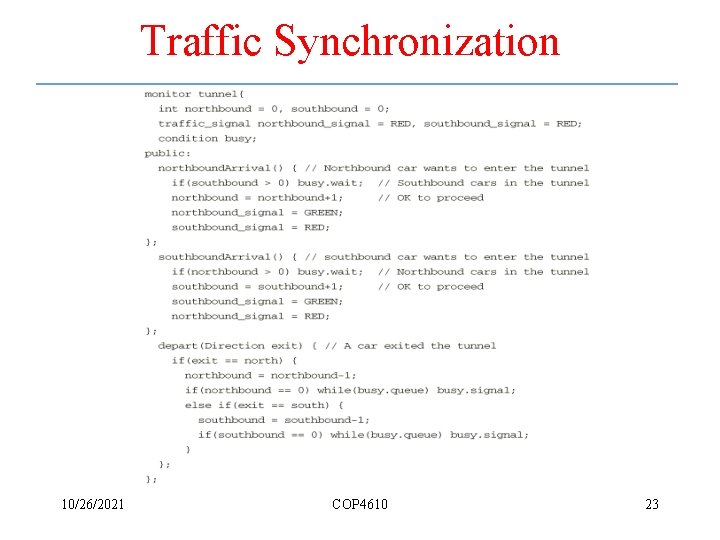
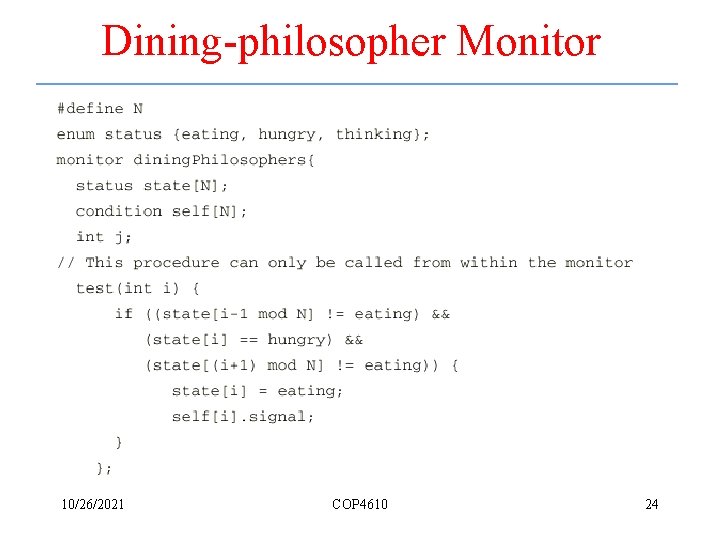
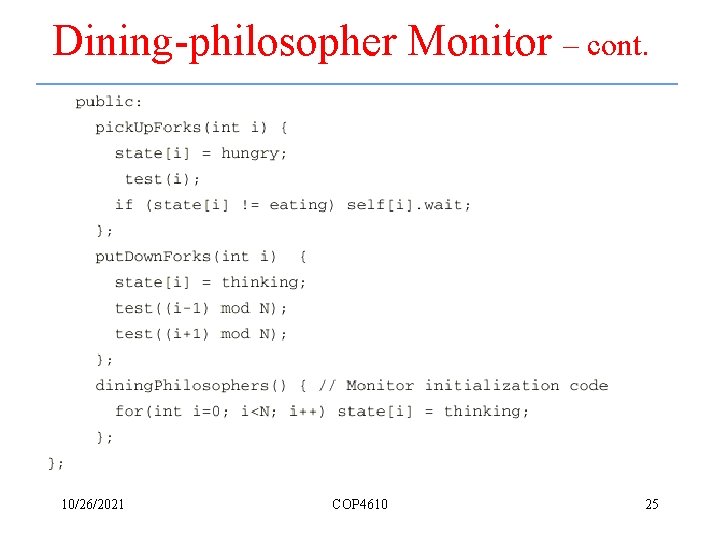
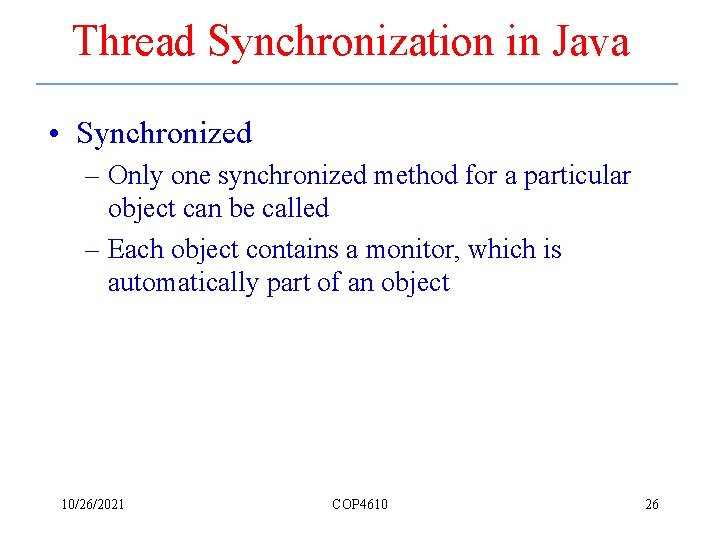
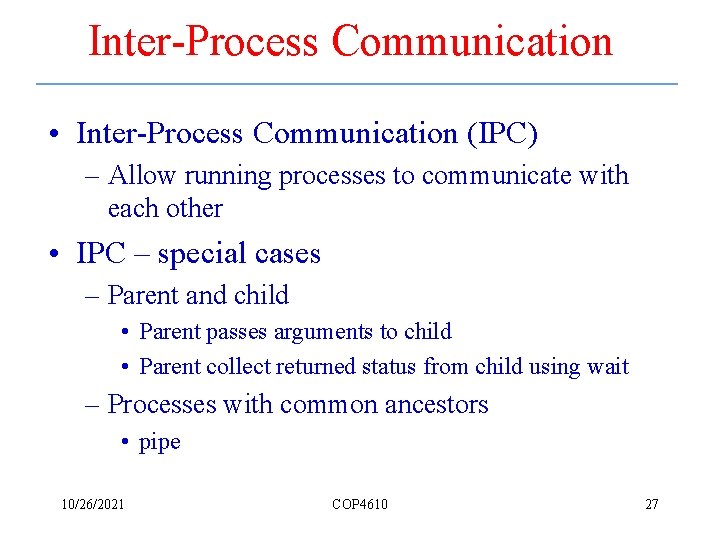
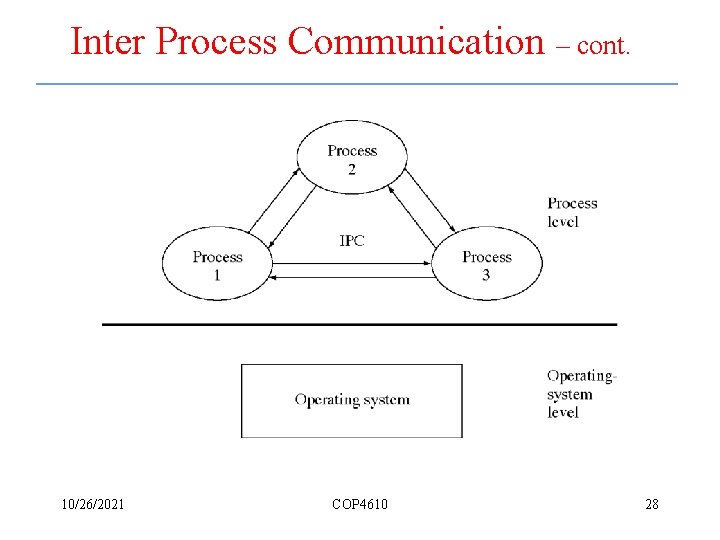
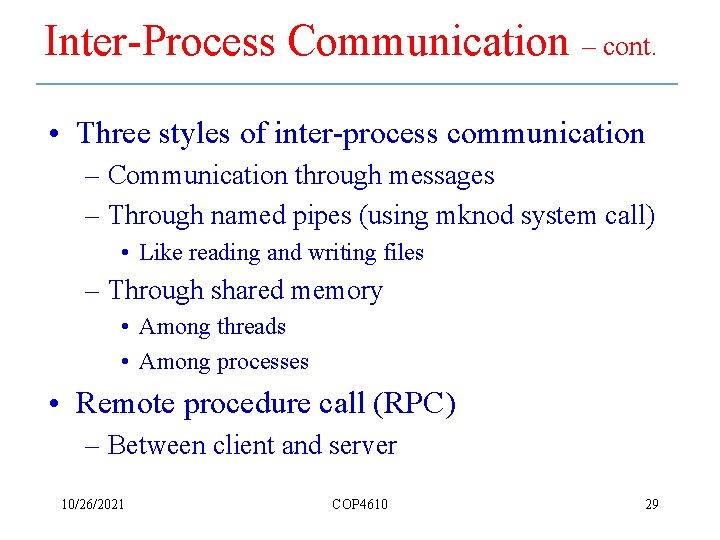
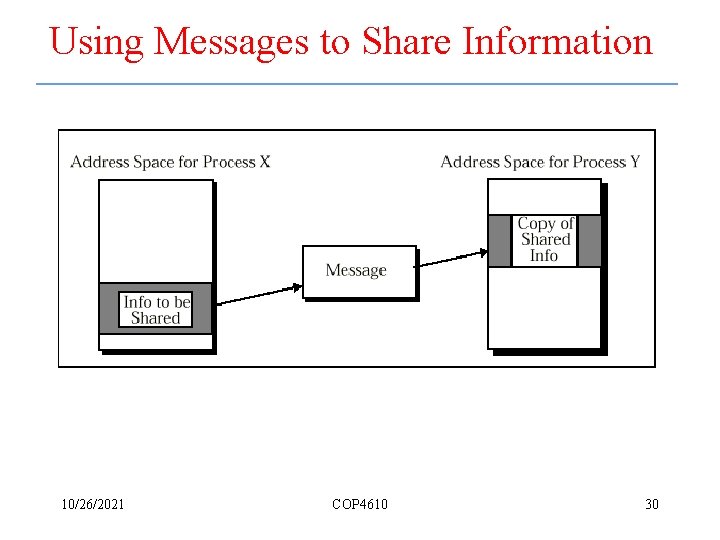
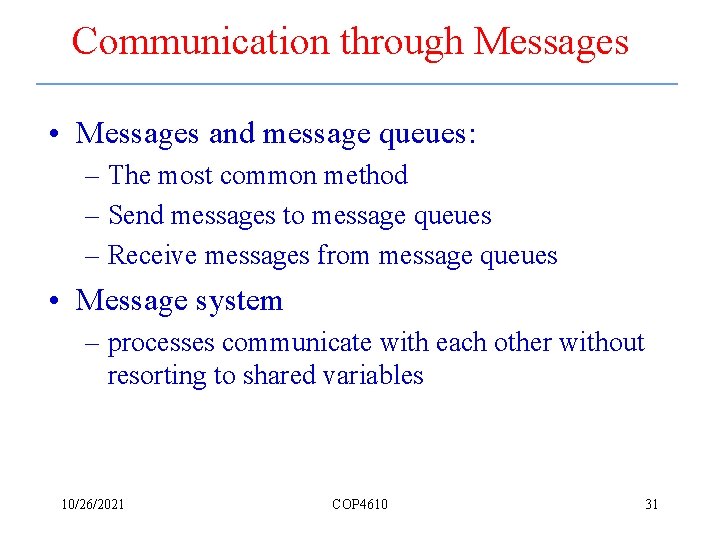
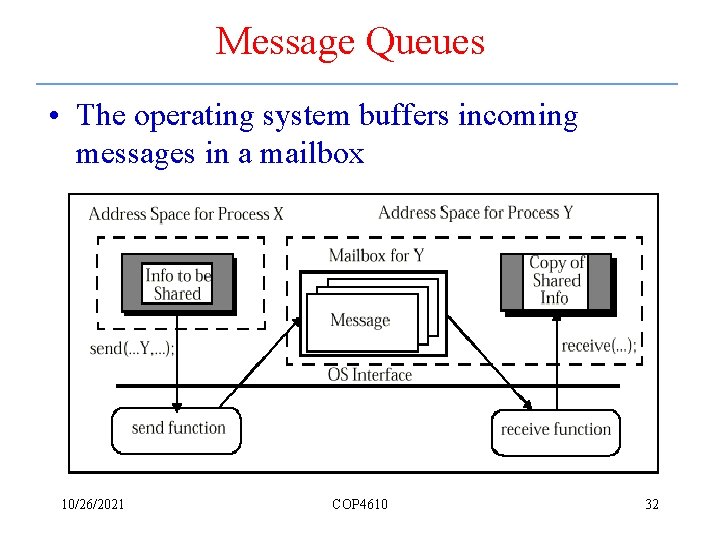
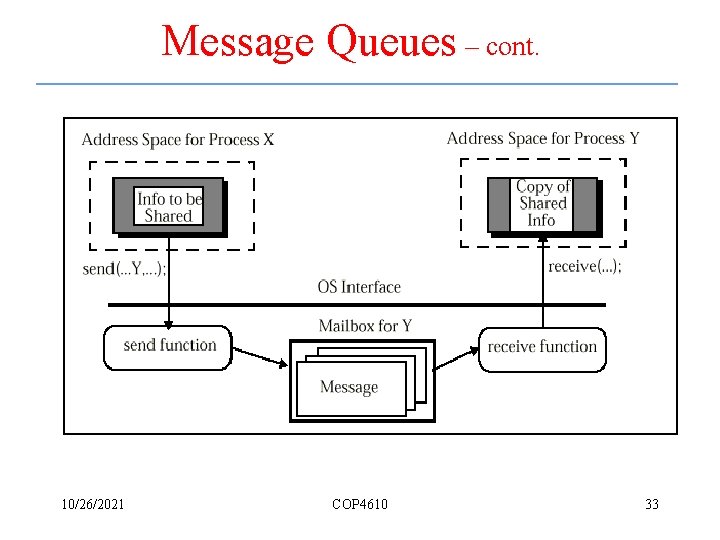
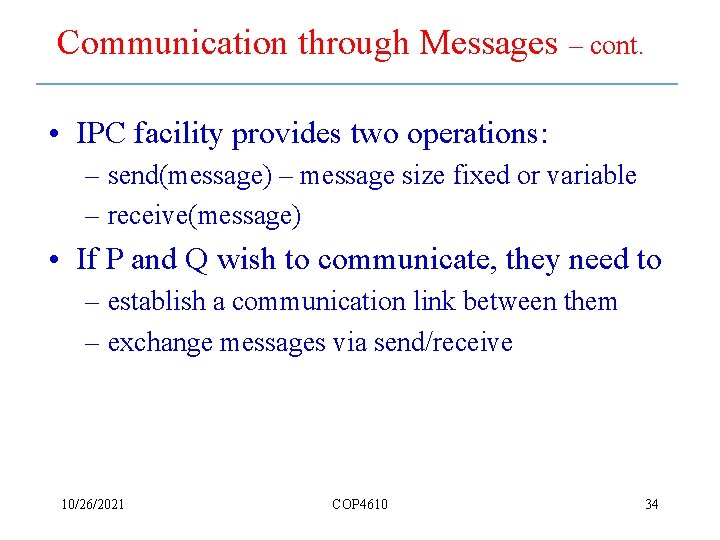
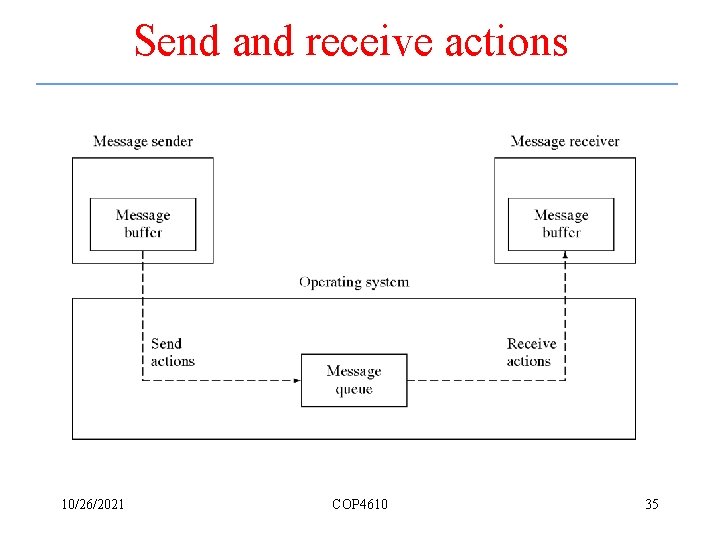
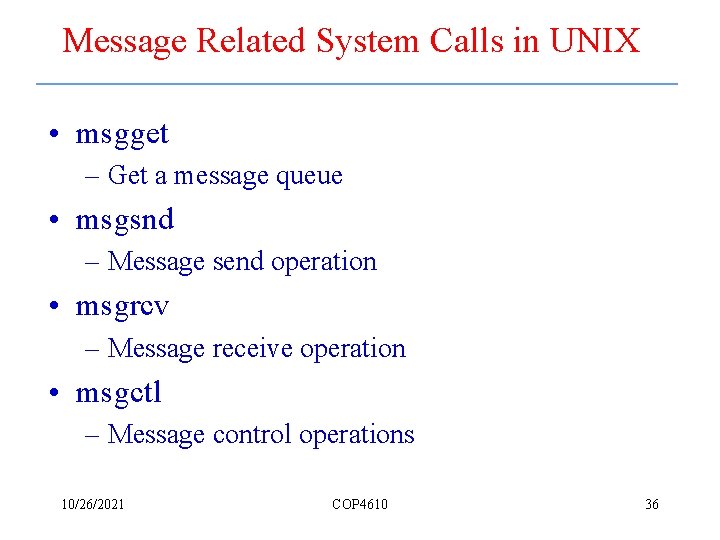
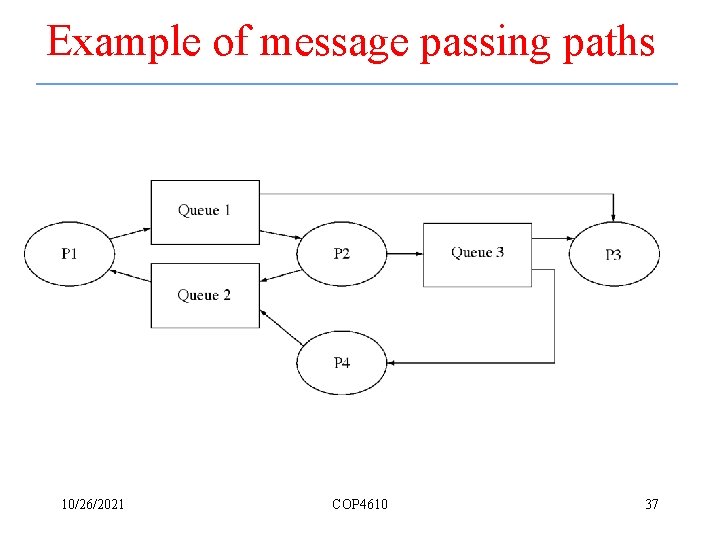
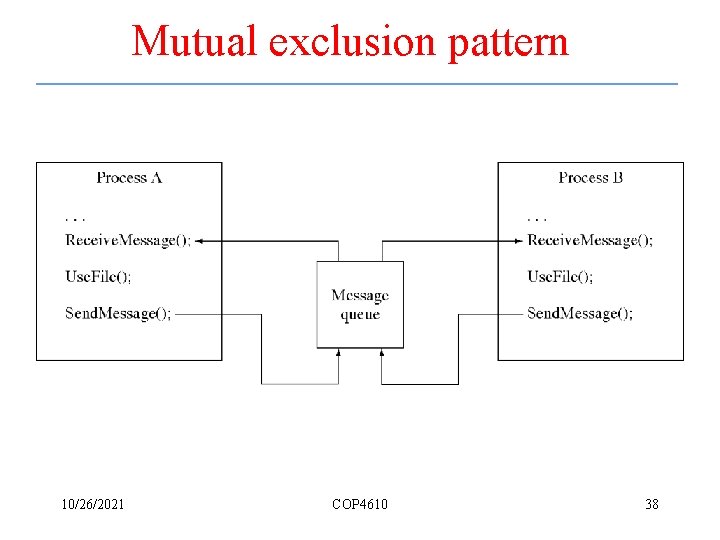
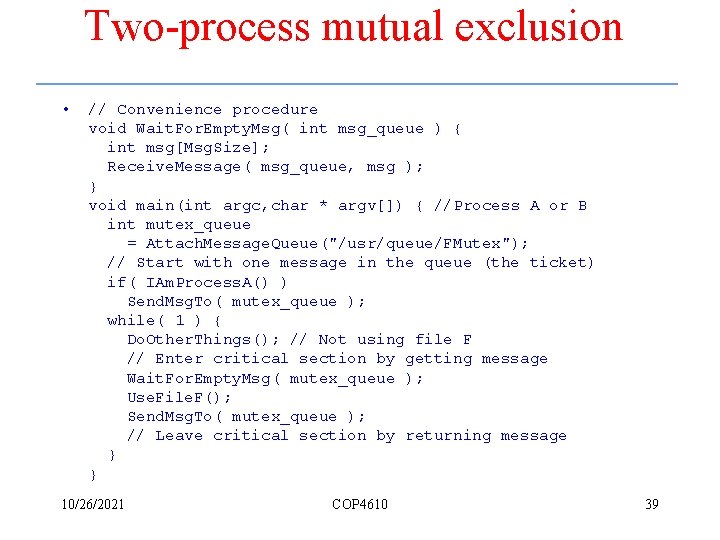
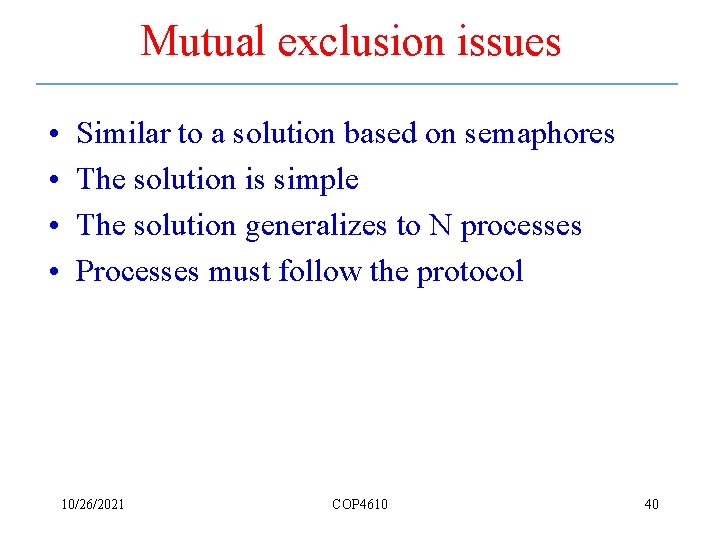
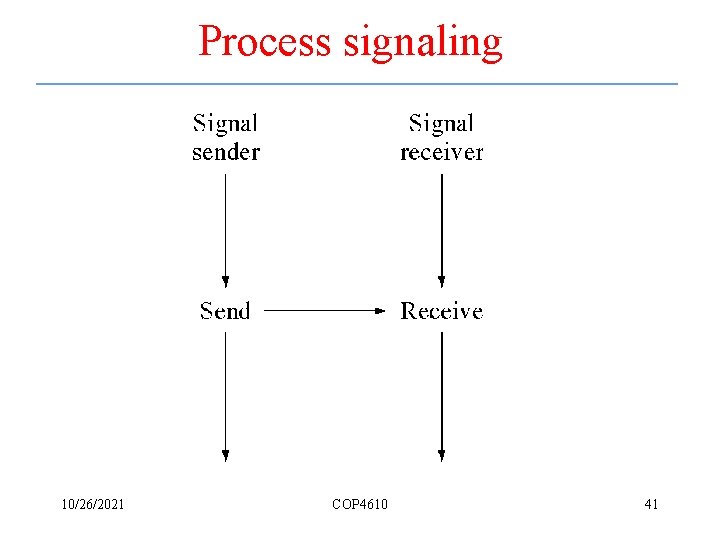
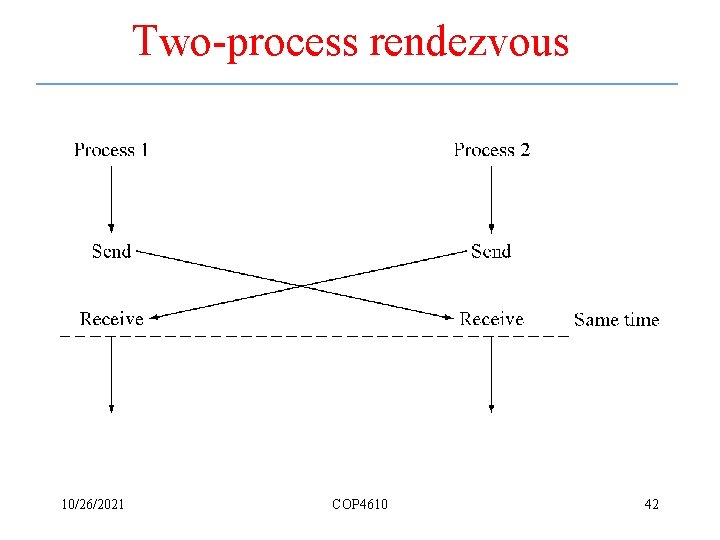
![Two-process rendezvous • void main( int argc, char *argv[ ] ) { // Game Two-process rendezvous • void main( int argc, char *argv[ ] ) { // Game](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-43.jpg)
![Many-process rendezvous • void main(int argc, char *argv[ ]) { // Coordinator int msg[Msg. Many-process rendezvous • void main(int argc, char *argv[ ]) { // Coordinator int msg[Msg.](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-44.jpg)
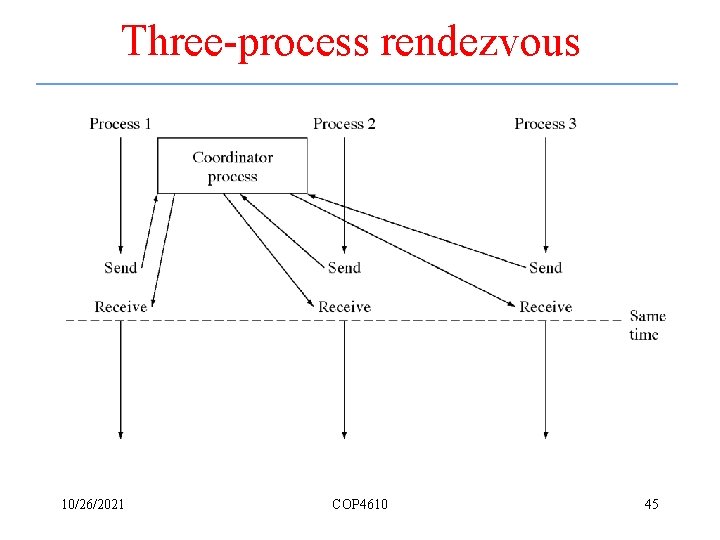
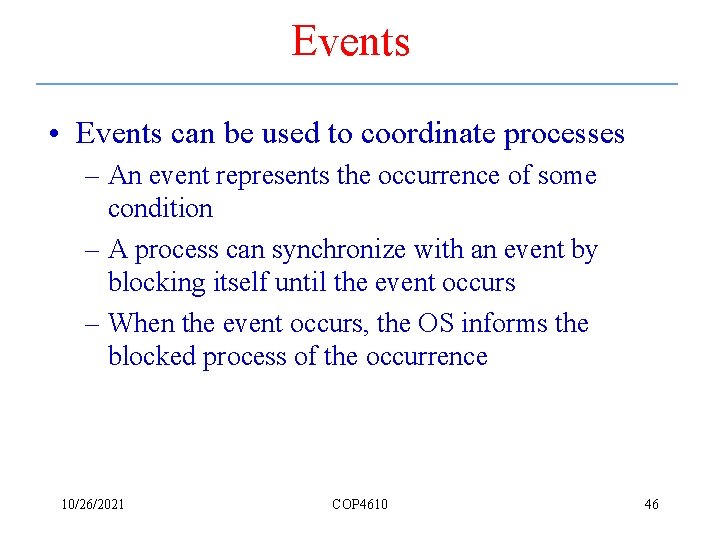
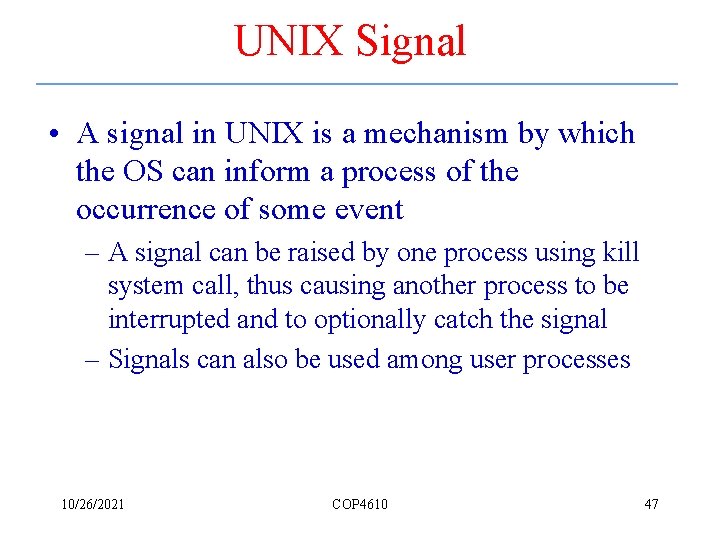
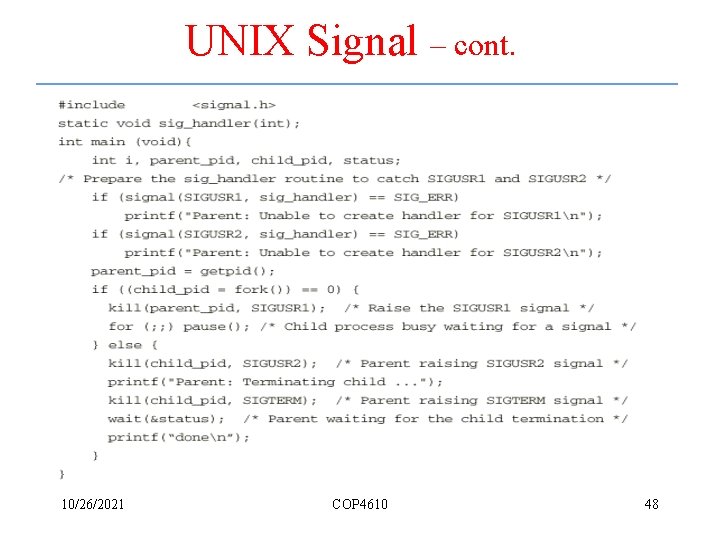
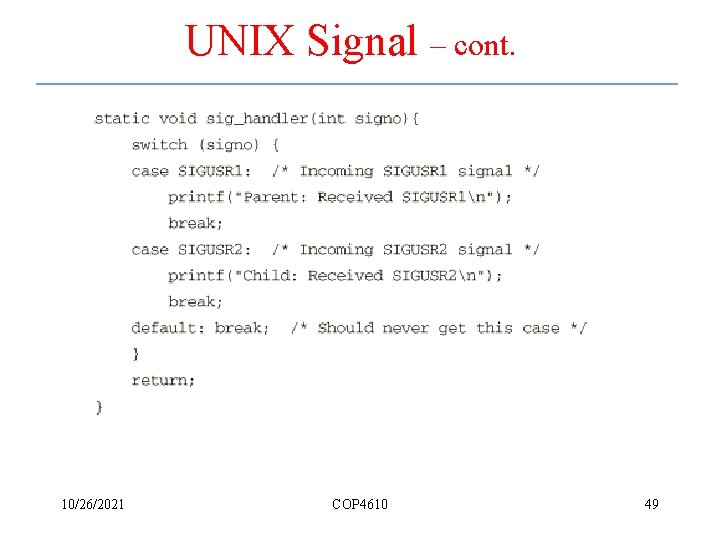
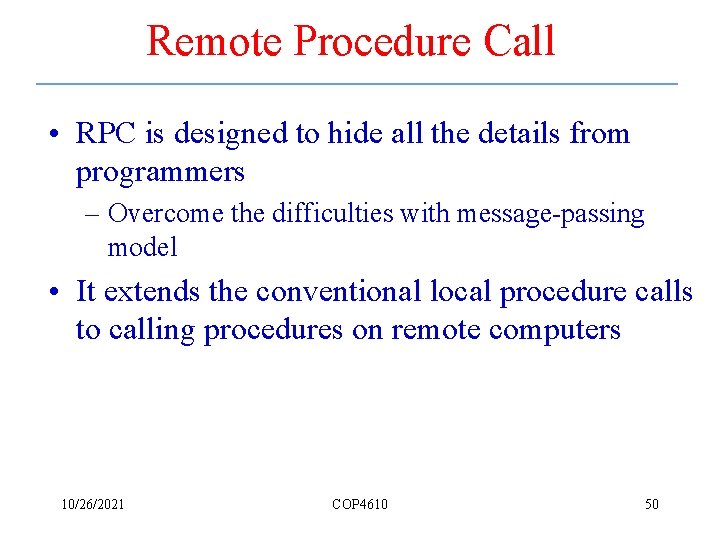
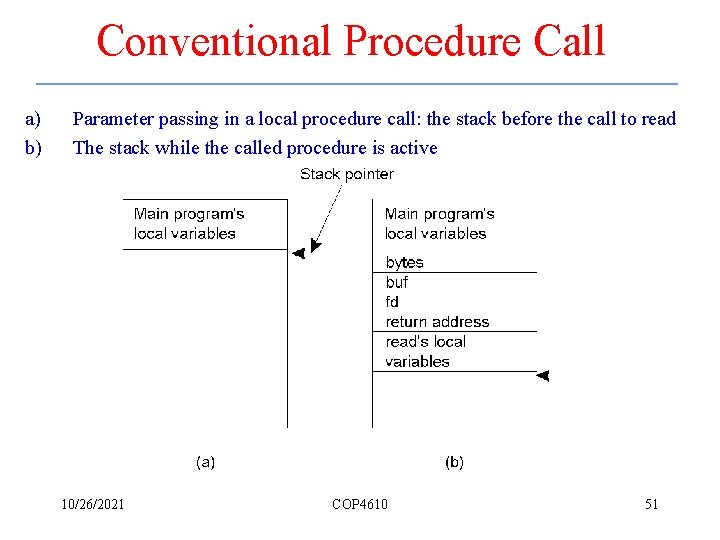
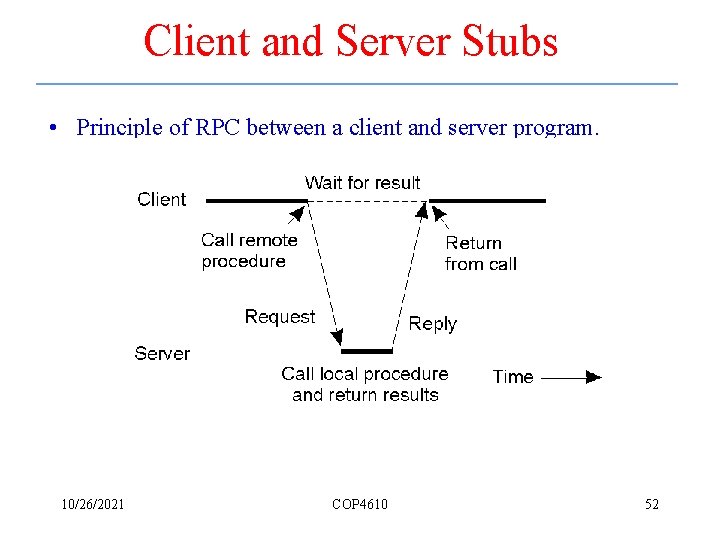
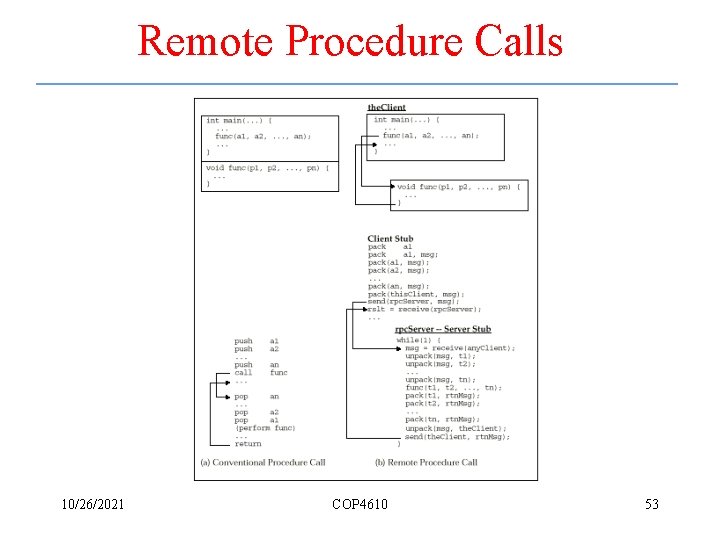
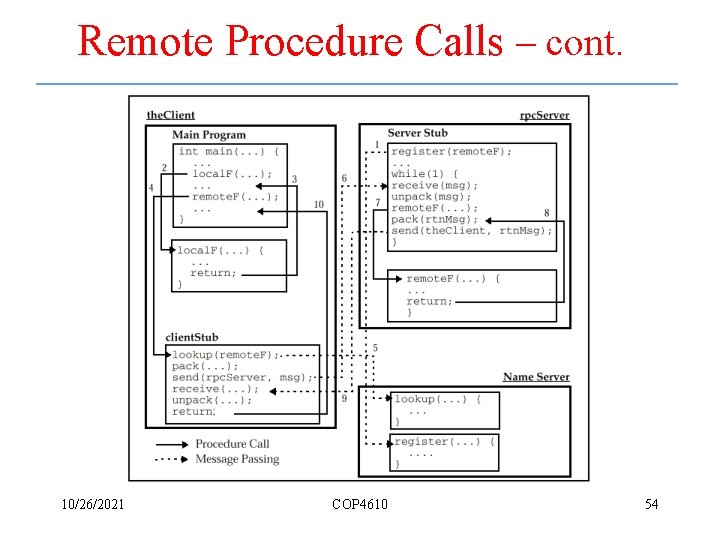
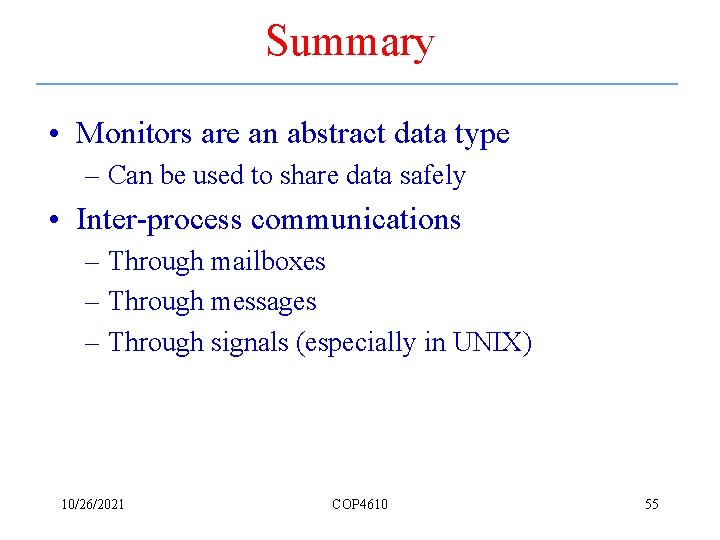
- Slides: 55
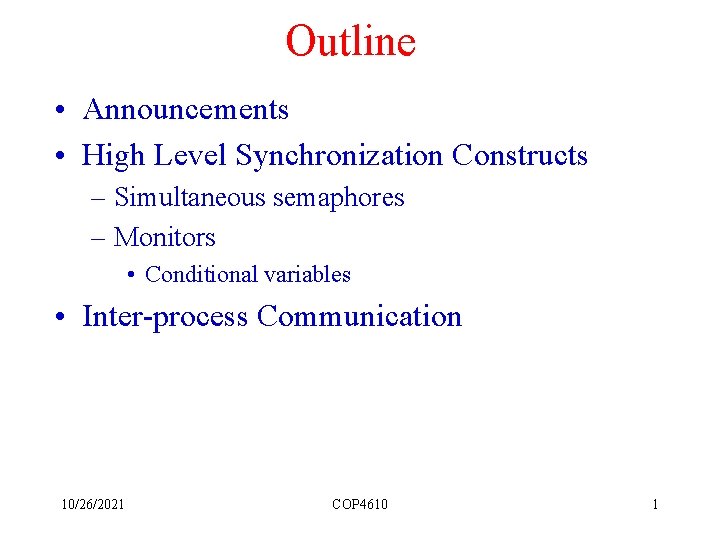
Outline • Announcements • High Level Synchronization Constructs – Simultaneous semaphores – Monitors • Conditional variables • Inter-process Communication 10/26/2021 COP 4610 1
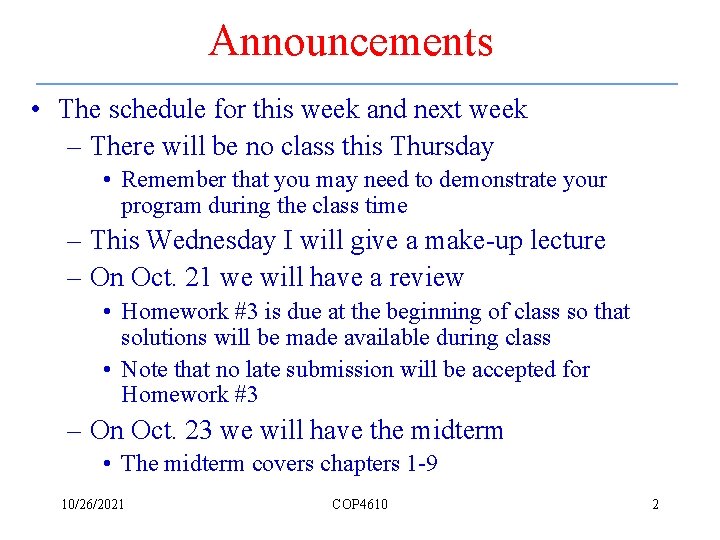
Announcements • The schedule for this week and next week – There will be no class this Thursday • Remember that you may need to demonstrate your program during the class time – This Wednesday I will give a make-up lecture – On Oct. 21 we will have a review • Homework #3 is due at the beginning of class so that solutions will be made available during class • Note that no late submission will be accepted for Homework #3 – On Oct. 23 we will have the midterm • The midterm covers chapters 1 -9 10/26/2021 COP 4610 2
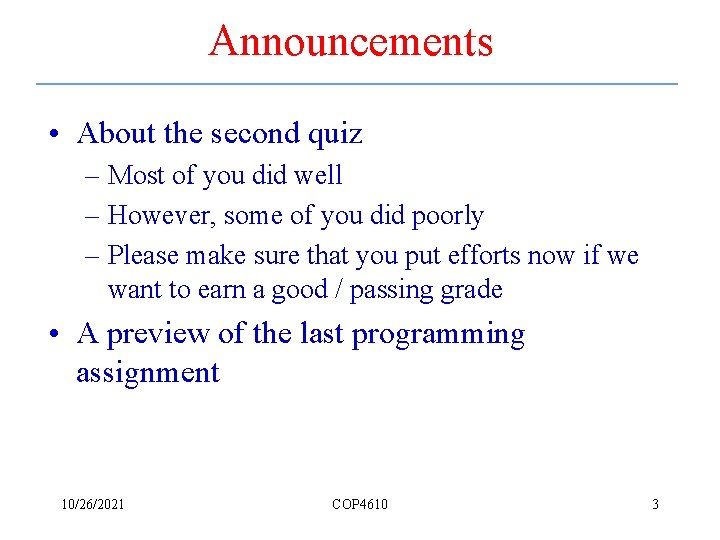
Announcements • About the second quiz – Most of you did well – However, some of you did poorly – Please make sure that you put efforts now if we want to earn a good / passing grade • A preview of the last programming assignment 10/26/2021 COP 4610 3
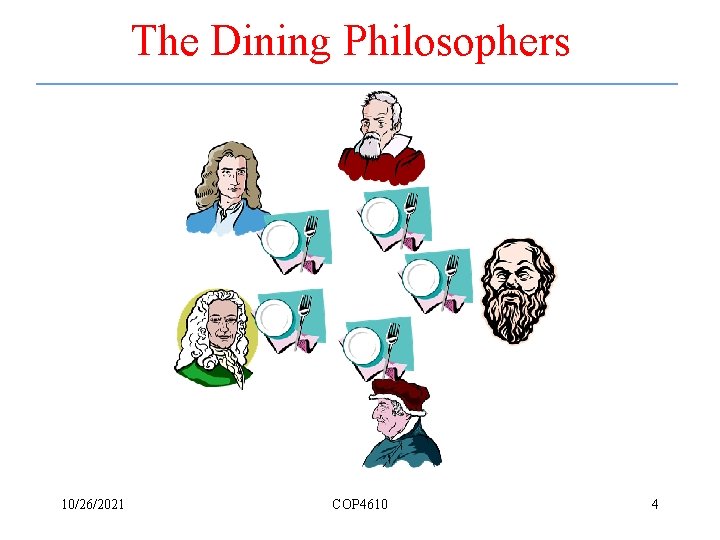
The Dining Philosophers 10/26/2021 COP 4610 4
![First Try Solution philosopherint i whileTRUE Think Eat Pforki Pforki1 First Try Solution philosopher(int i) { while(TRUE) { // Think // Eat P(fork[i]); P(fork[(i+1)](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-5.jpg)
First Try Solution philosopher(int i) { while(TRUE) { // Think // Eat P(fork[i]); P(fork[(i+1) mod 5]); eat(); V(fork[(i+1) mod 5]); V(fork[i]); } } semaphore fork[5] = (1, 1, 1); fork(philosopher, 1, 0); fork(philosopher, 1, 1); fork(philosopher, 1, 2); fork(philosopher, 1, 3); fork(philosopher, 1, 4); 10/26/2021 COP 4610 5
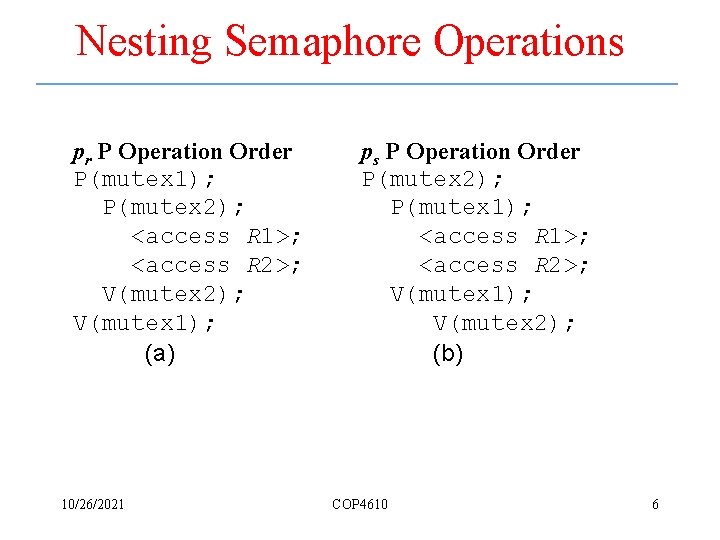
Nesting Semaphore Operations pr P Operation Order P(mutex 1); P(mutex 2); <access R 1>; <access R 2>; V(mutex 2); V(mutex 1); (a) 10/26/2021 ps P Operation Order P(mutex 2); P(mutex 1); <access R 1>; <access R 2>; V(mutex 1); V(mutex 2); (b) COP 4610 6
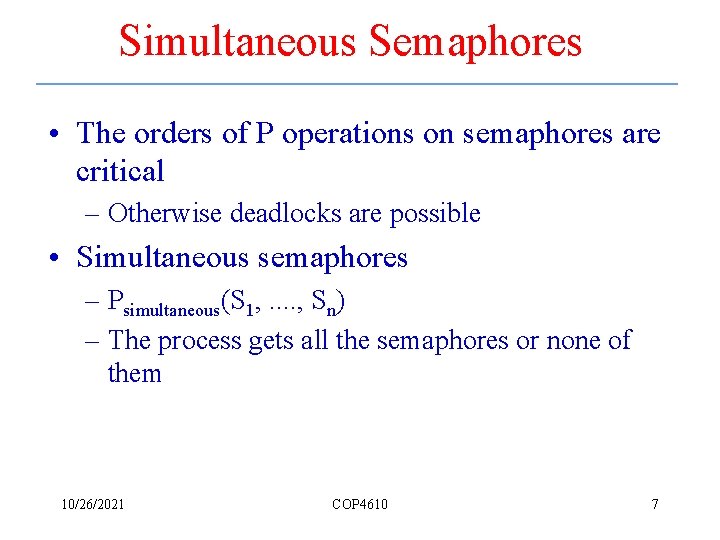
Simultaneous Semaphores • The orders of P operations on semaphores are critical – Otherwise deadlocks are possible • Simultaneous semaphores – Psimultaneous(S 1, . . , Sn) – The process gets all the semaphores or none of them 10/26/2021 COP 4610 7
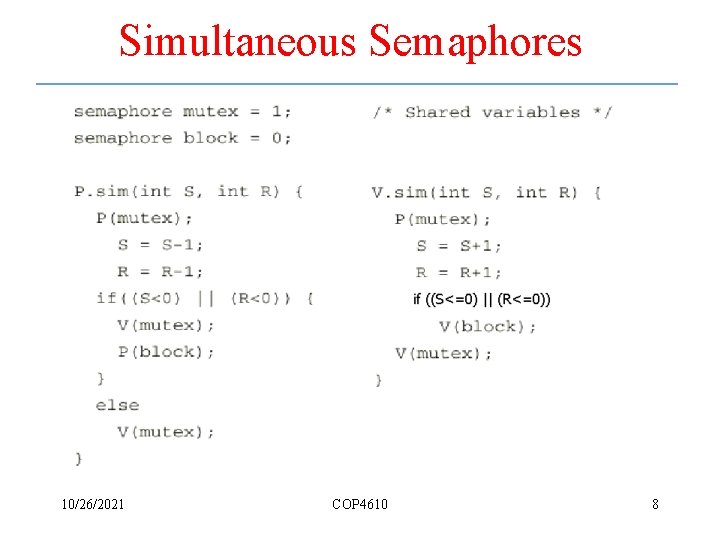
Simultaneous Semaphores 10/26/2021 COP 4610 8
![A Solution philosopherint i whileTRUE Think Eat Psimforki forki1 A Solution philosopher(int i) { while(TRUE) { // Think // Eat } Psim(fork[i], fork[(i+1)](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-9.jpg)
A Solution philosopher(int i) { while(TRUE) { // Think // Eat } Psim(fork[i], fork[(i+1) mod 5]); eat(); Vsim(fork[i], fork[(i+1) mod 5]); } semaphore fork[5] fork(philosopher, fork(philosopher, 10/26/2021 = (1, 1, 1); 1, 0); 1, 1); 1, 2); 1, 3); 1, 4); COP 4610 9
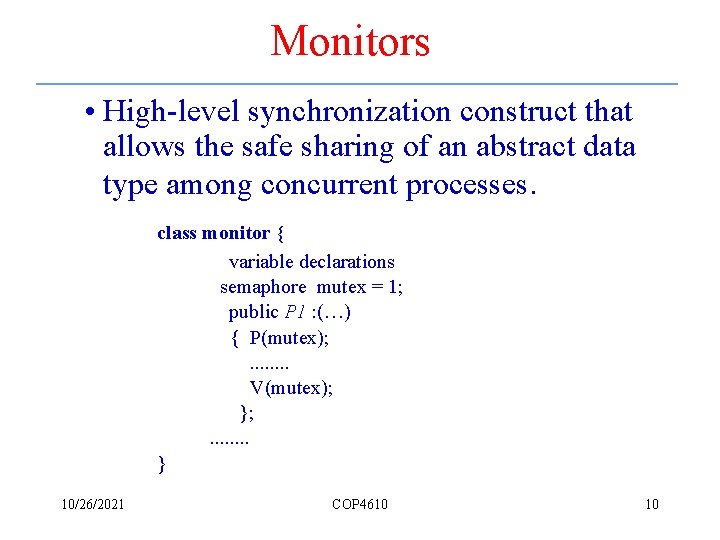
Monitors • High-level synchronization construct that allows the safe sharing of an abstract data type among concurrent processes. class monitor { variable declarations semaphore mutex = 1; public P 1 : (…) { P(mutex); . . . . V(mutex); }; . . . . } 10/26/2021 COP 4610 10
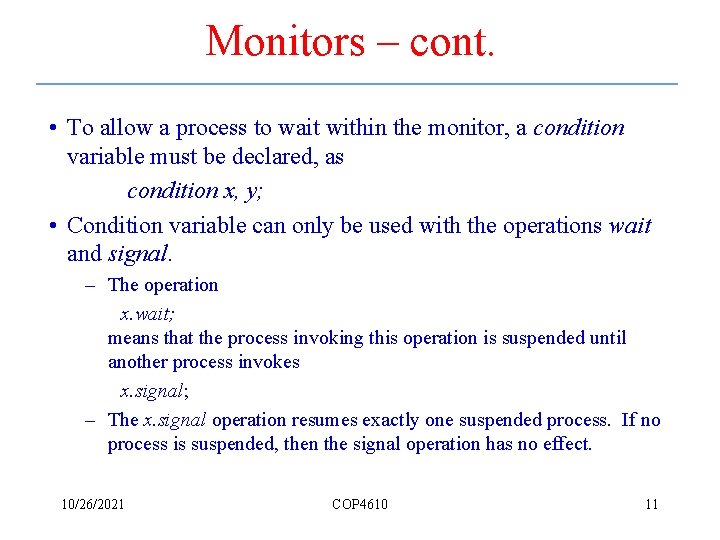
Monitors – cont. • To allow a process to wait within the monitor, a condition variable must be declared, as condition x, y; • Condition variable can only be used with the operations wait and signal. – The operation x. wait; means that the process invoking this operation is suspended until another process invokes x. signal; – The x. signal operation resumes exactly one suspended process. If no process is suspended, then the signal operation has no effect. 10/26/2021 COP 4610 11
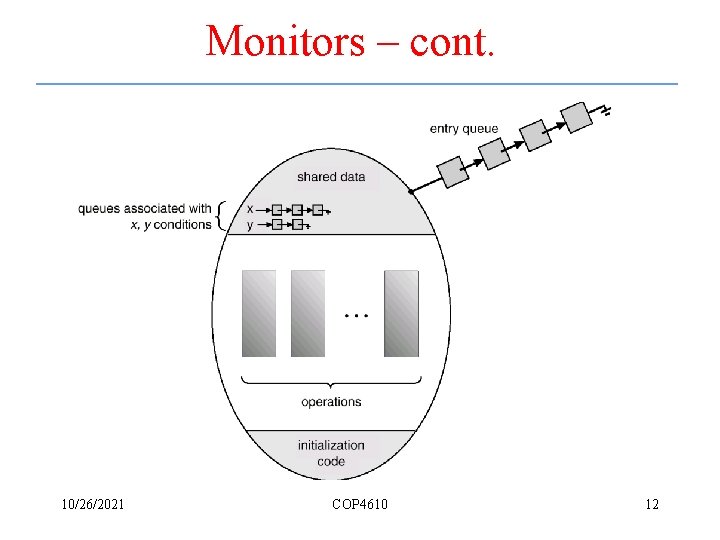
Monitors – cont. 10/26/2021 COP 4610 12
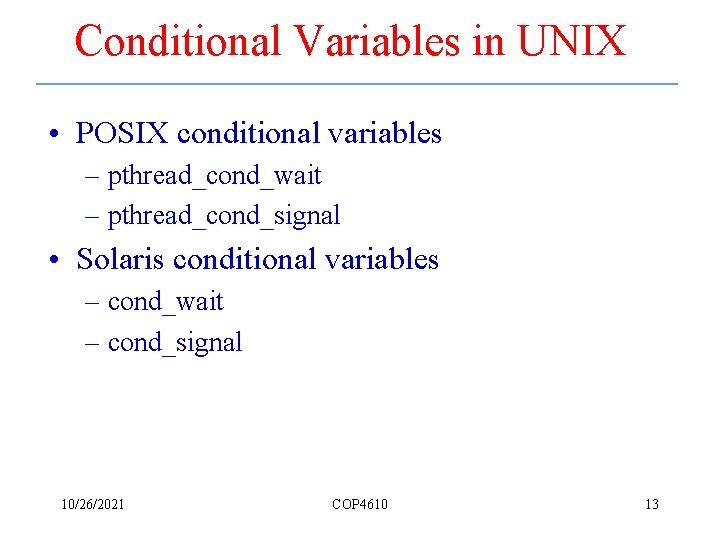
Conditional Variables in UNIX • POSIX conditional variables – pthread_cond_wait – pthread_cond_signal • Solaris conditional variables – cond_wait – cond_signal 10/26/2021 COP 4610 13
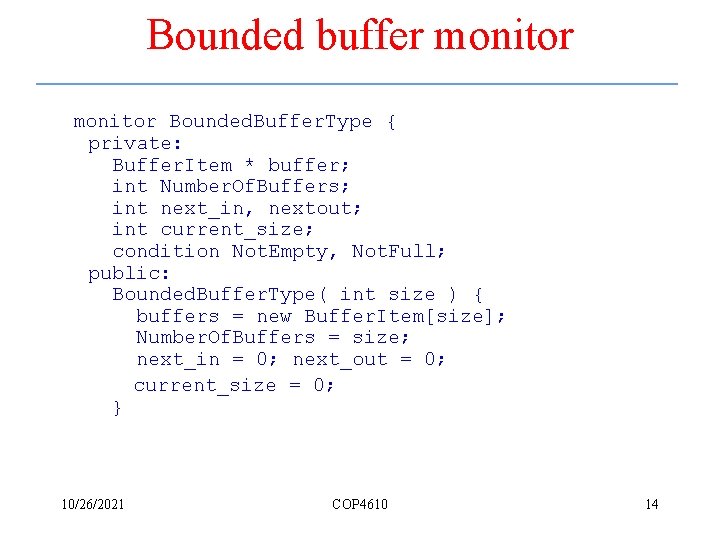
Bounded buffer monitor Bounded. Buffer. Type { private: Buffer. Item * buffer; int Number. Of. Buffers; int next_in, nextout; int current_size; condition Not. Empty, Not. Full; public: Bounded. Buffer. Type( int size ) { buffers = new Buffer. Item[size]; Number. Of. Buffers = size; next_in = 0; next_out = 0; current_size = 0; } 10/26/2021 COP 4610 14
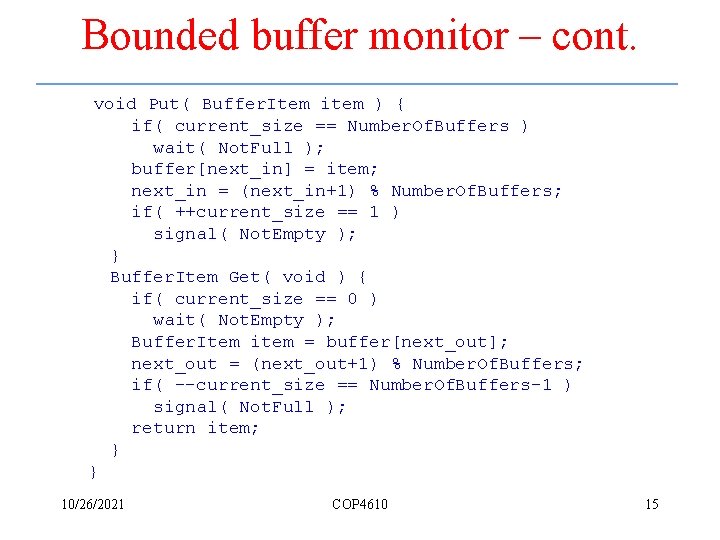
Bounded buffer monitor – cont. void Put( Buffer. Item item ) { if( current_size == Number. Of. Buffers ) wait( Not. Full ); buffer[next_in] = item; next_in = (next_in+1) % Number. Of. Buffers; if( ++current_size == 1 ) signal( Not. Empty ); } Buffer. Item Get( void ) { if( current_size == 0 ) wait( Not. Empty ); Buffer. Item item = buffer[next_out]; next_out = (next_out+1) % Number. Of. Buffers; if( --current_size == Number. Of. Buffers-1 ) signal( Not. Full ); return item; } } 10/26/2021 COP 4610 15
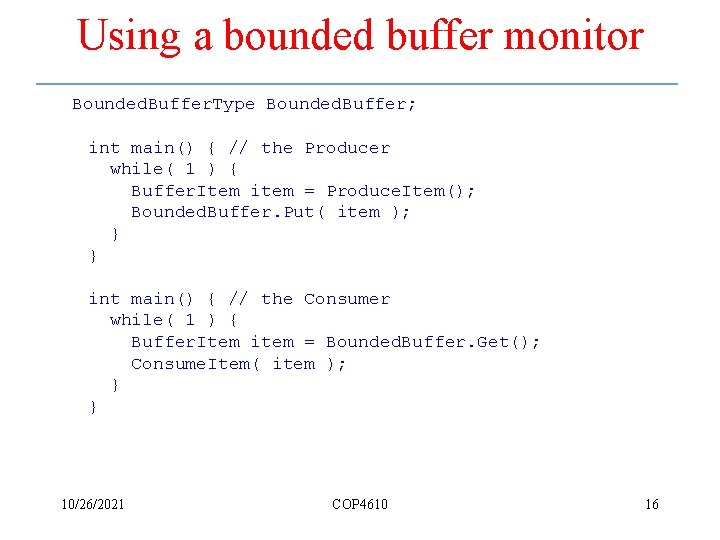
Using a bounded buffer monitor Bounded. Buffer. Type Bounded. Buffer; int main() { // the Producer while( 1 ) { Buffer. Item item = Produce. Item(); Bounded. Buffer. Put( item ); } } int main() { // the Consumer while( 1 ) { Buffer. Item item = Bounded. Buffer. Get(); Consume. Item( item ); } } 10/26/2021 COP 4610 16
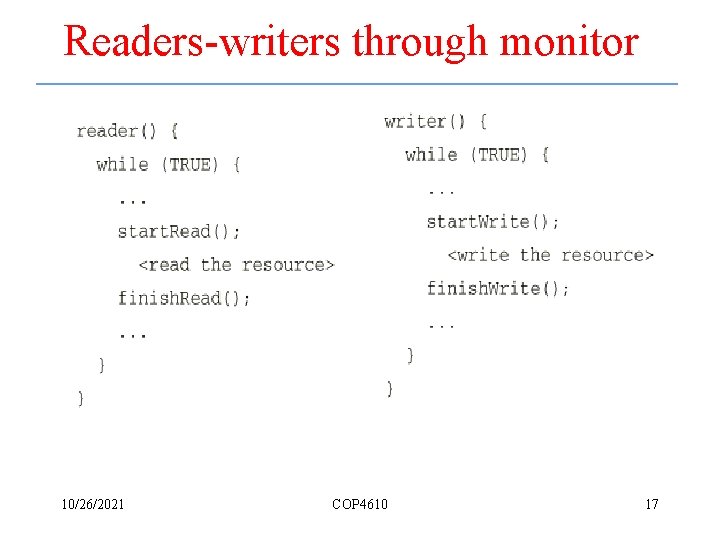
Readers-writers through monitor 10/26/2021 COP 4610 17
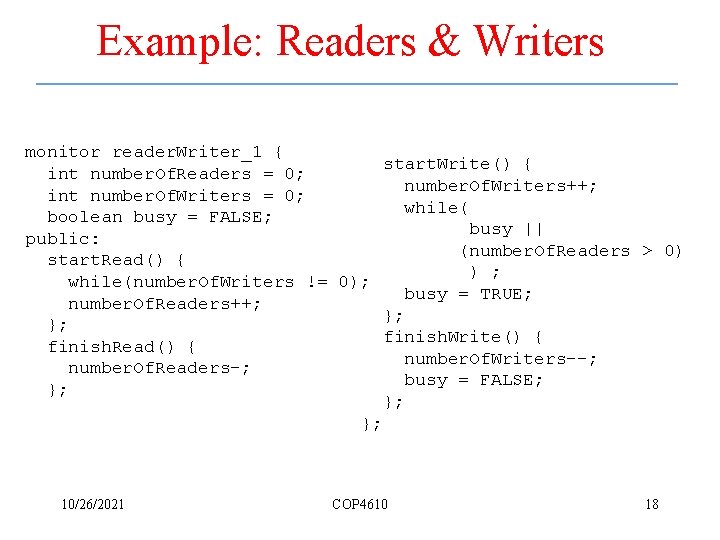
Example: Readers & Writers monitor reader. Writer_1 { start. Write() { int number. Of. Readers = 0; number. Of. Writers++; int number. Of. Writers = 0; while( boolean busy = FALSE; busy || public: (number. Of. Readers > 0) start. Read() { ) ; while(number. Of. Writers != 0); busy = TRUE; number. Of. Readers++; }; }; finish. Write() { finish. Read() { number. Of. Writers--; number. Of. Readers-; busy = FALSE; }; }; }; 10/26/2021 COP 4610 18
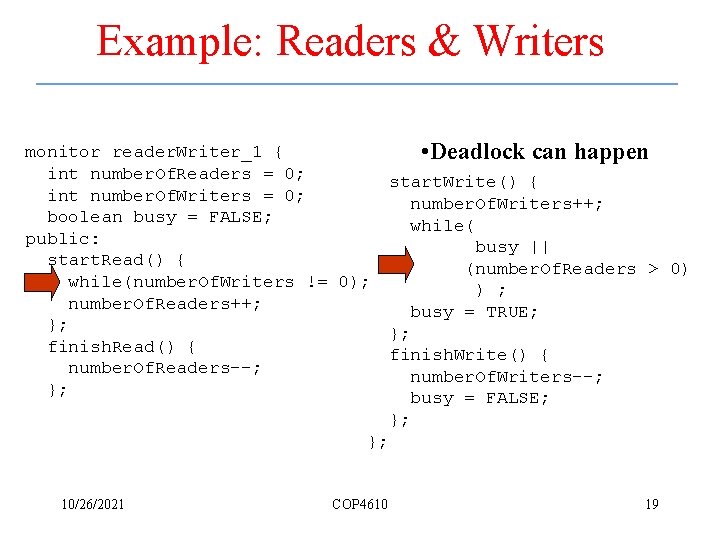
Example: Readers & Writers monitor reader. Writer_1 { • Deadlock can happen int number. Of. Readers = 0; start. Write() { int number. Of. Writers = 0; number. Of. Writers++; boolean busy = FALSE; while( public: busy || start. Read() { (number. Of. Readers > 0) while(number. Of. Writers != 0); ) ; number. Of. Readers++; busy = TRUE; }; }; finish. Read() { finish. Write() { number. Of. Readers--; number. Of. Writers--; }; busy = FALSE; }; }; 10/26/2021 COP 4610 19
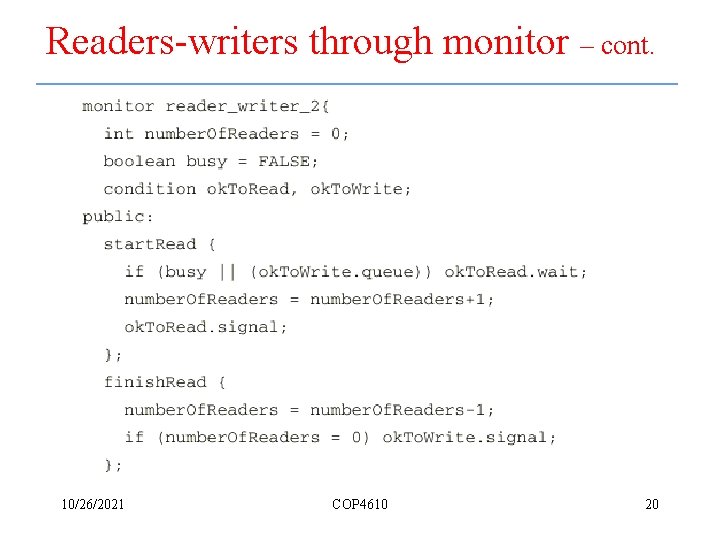
Readers-writers through monitor – cont. 10/26/2021 COP 4610 20
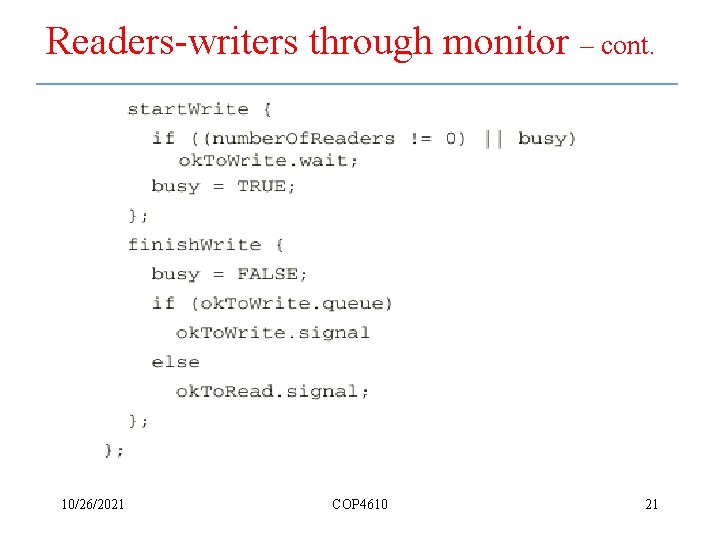
Readers-writers through monitor – cont. 10/26/2021 COP 4610 21
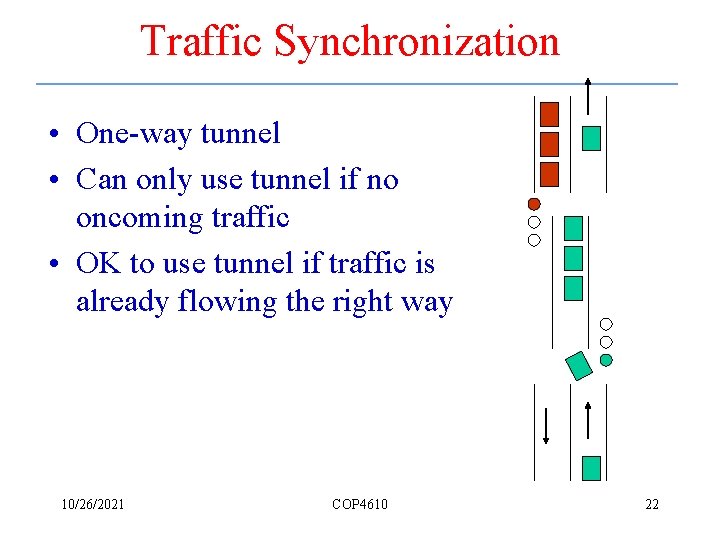
Traffic Synchronization • One-way tunnel • Can only use tunnel if no oncoming traffic • OK to use tunnel if traffic is already flowing the right way 10/26/2021 COP 4610 22
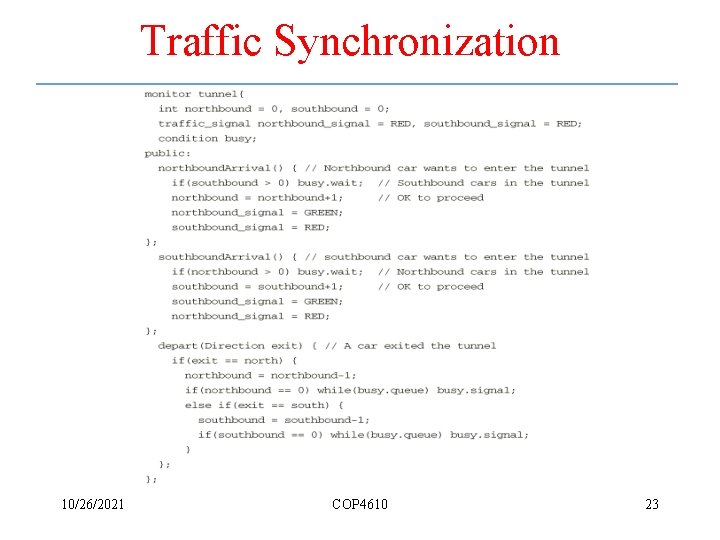
Traffic Synchronization 10/26/2021 COP 4610 23
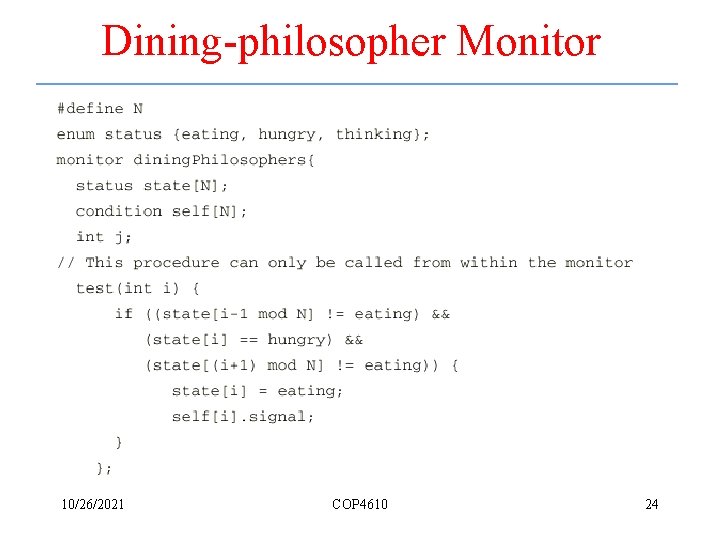
Dining-philosopher Monitor 10/26/2021 COP 4610 24
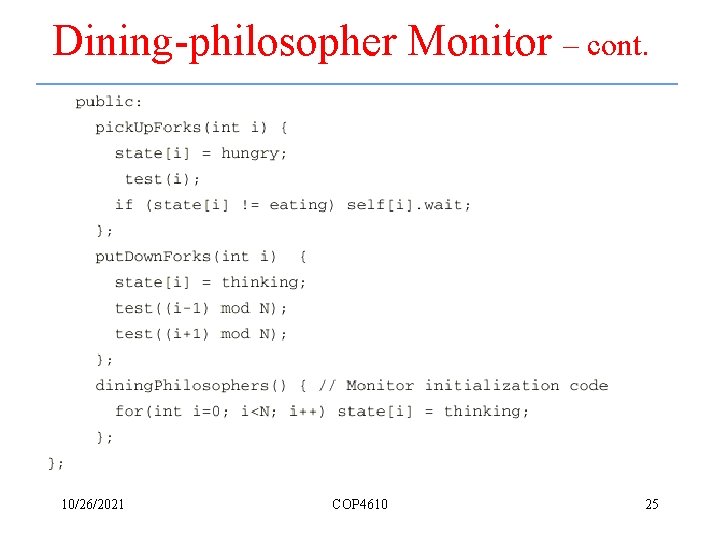
Dining-philosopher Monitor – cont. 10/26/2021 COP 4610 25
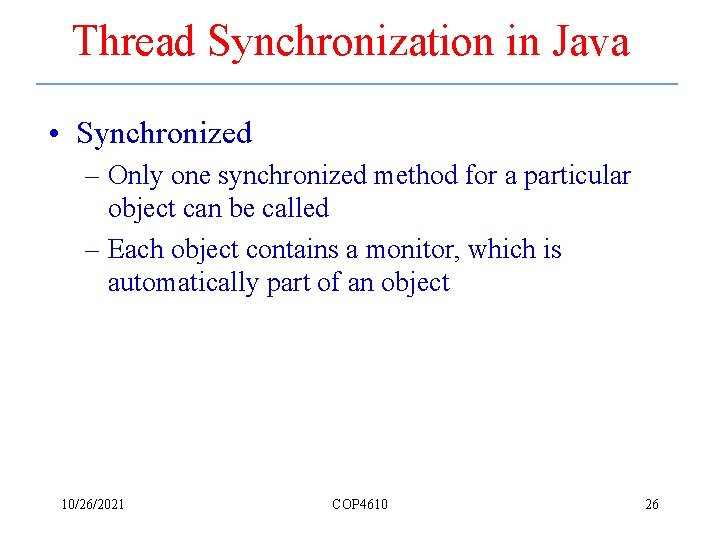
Thread Synchronization in Java • Synchronized – Only one synchronized method for a particular object can be called – Each object contains a monitor, which is automatically part of an object 10/26/2021 COP 4610 26
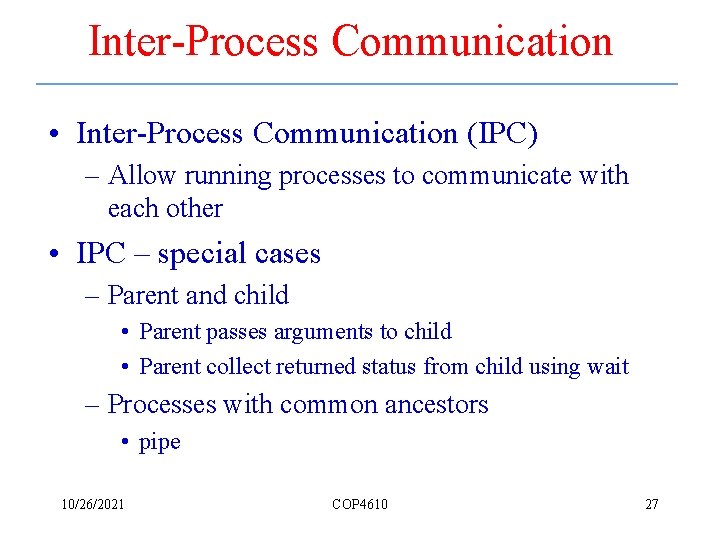
Inter-Process Communication • Inter-Process Communication (IPC) – Allow running processes to communicate with each other • IPC – special cases – Parent and child • Parent passes arguments to child • Parent collect returned status from child using wait – Processes with common ancestors • pipe 10/26/2021 COP 4610 27
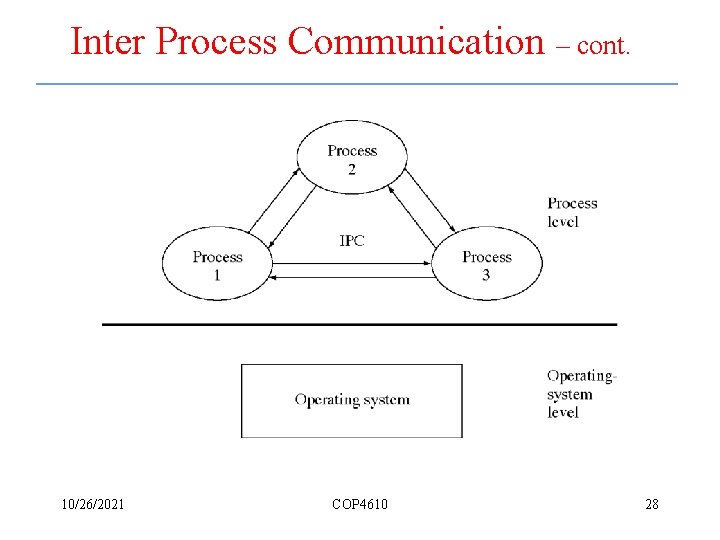
Inter Process Communication – cont. 10/26/2021 COP 4610 28
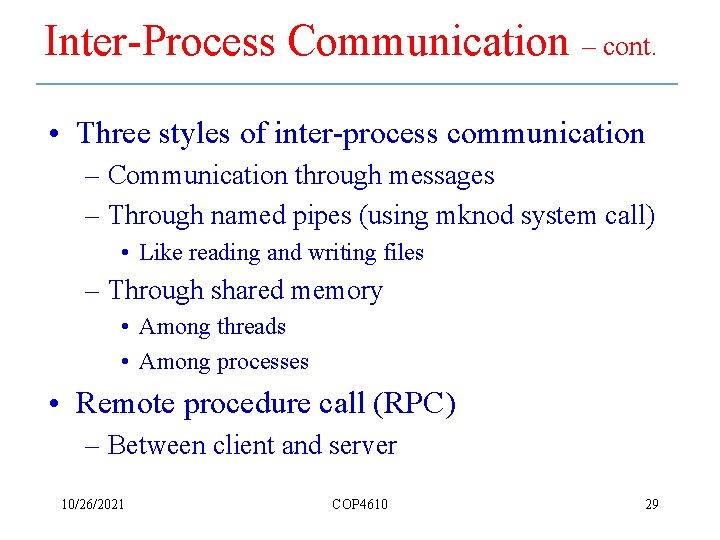
Inter-Process Communication – cont. • Three styles of inter-process communication – Communication through messages – Through named pipes (using mknod system call) • Like reading and writing files – Through shared memory • Among threads • Among processes • Remote procedure call (RPC) – Between client and server 10/26/2021 COP 4610 29
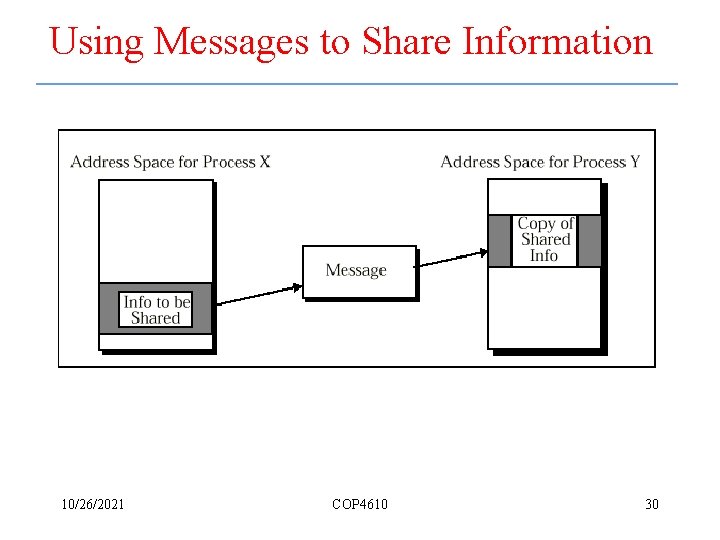
Using Messages to Share Information 10/26/2021 COP 4610 30
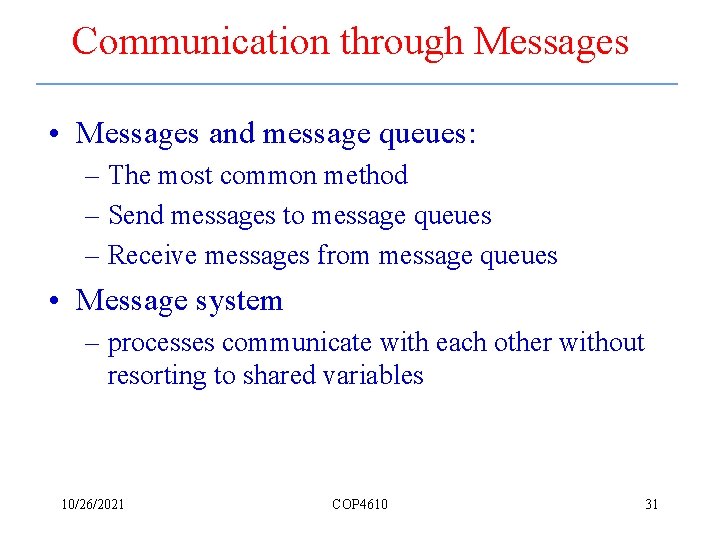
Communication through Messages • Messages and message queues: – The most common method – Send messages to message queues – Receive messages from message queues • Message system – processes communicate with each other without resorting to shared variables 10/26/2021 COP 4610 31
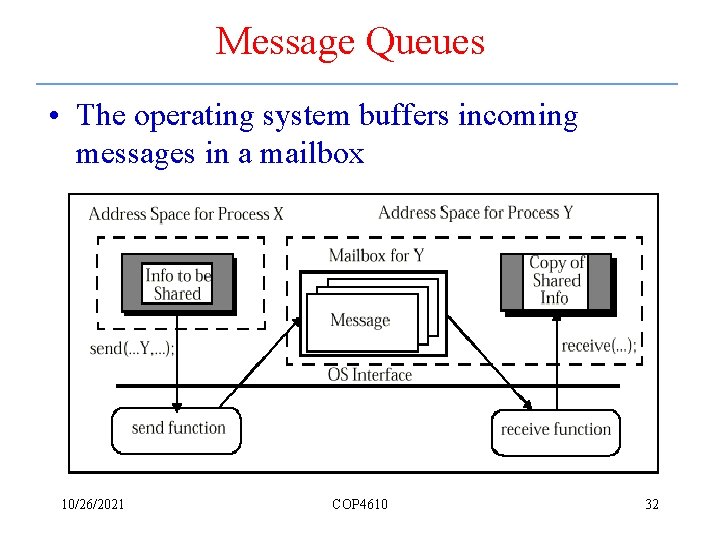
Message Queues • The operating system buffers incoming messages in a mailbox 10/26/2021 COP 4610 32
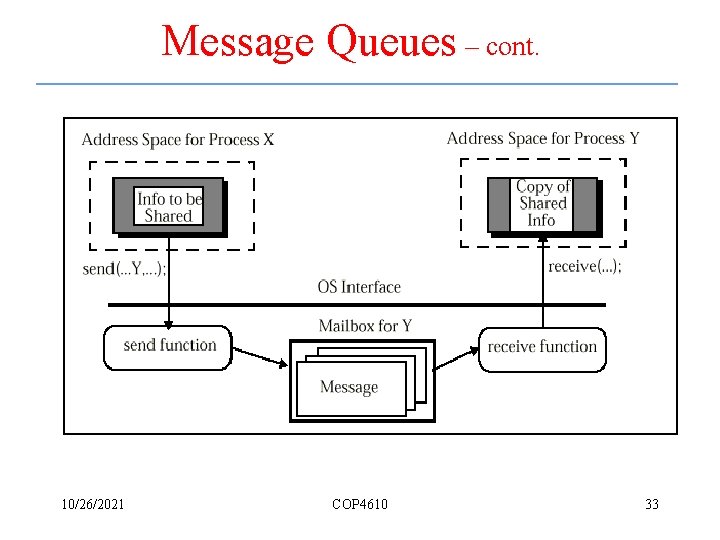
Message Queues – cont. 10/26/2021 COP 4610 33
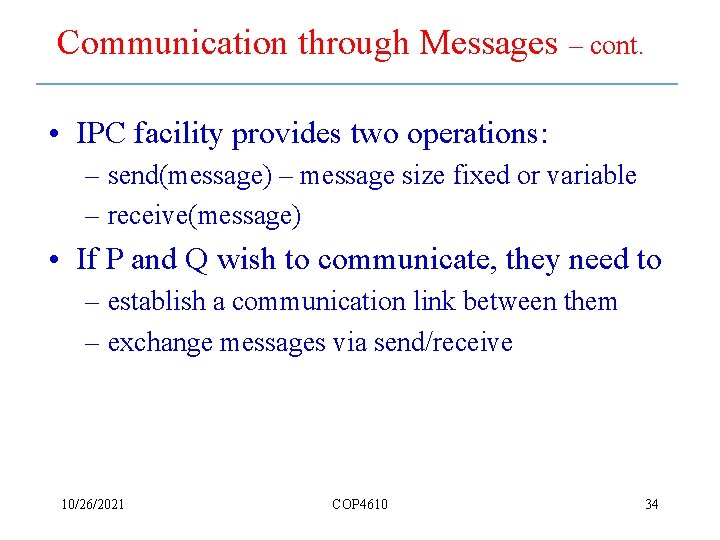
Communication through Messages – cont. • IPC facility provides two operations: – send(message) – message size fixed or variable – receive(message) • If P and Q wish to communicate, they need to – establish a communication link between them – exchange messages via send/receive 10/26/2021 COP 4610 34
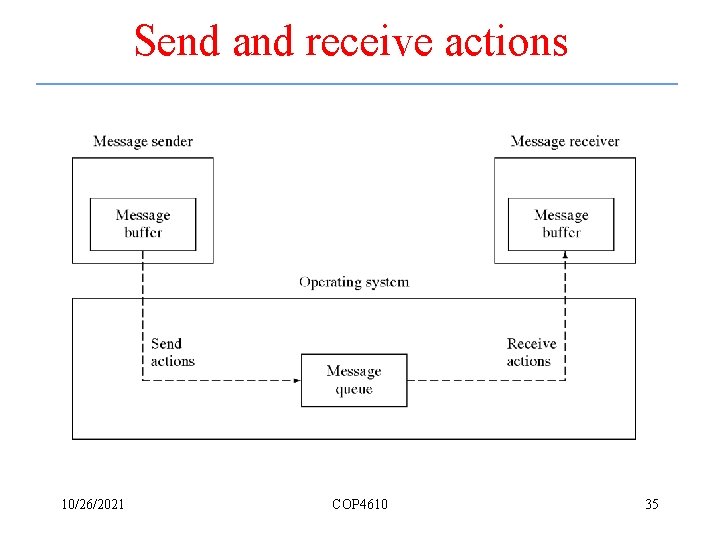
Send and receive actions 10/26/2021 COP 4610 35
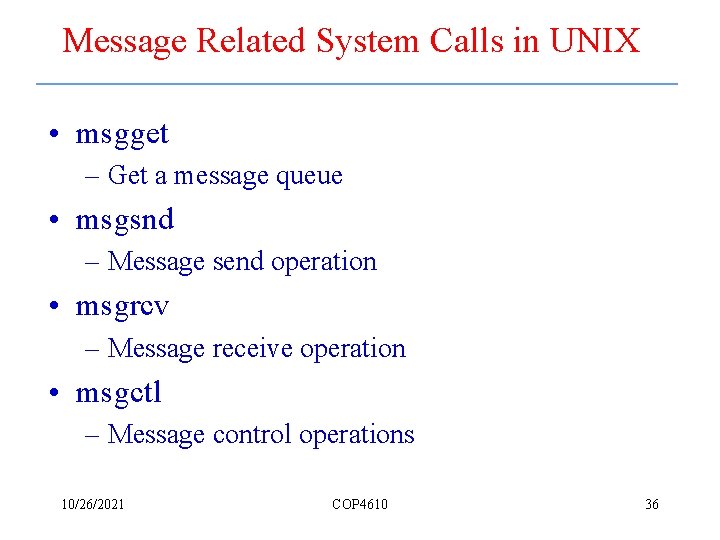
Message Related System Calls in UNIX • msgget – Get a message queue • msgsnd – Message send operation • msgrcv – Message receive operation • msgctl – Message control operations 10/26/2021 COP 4610 36
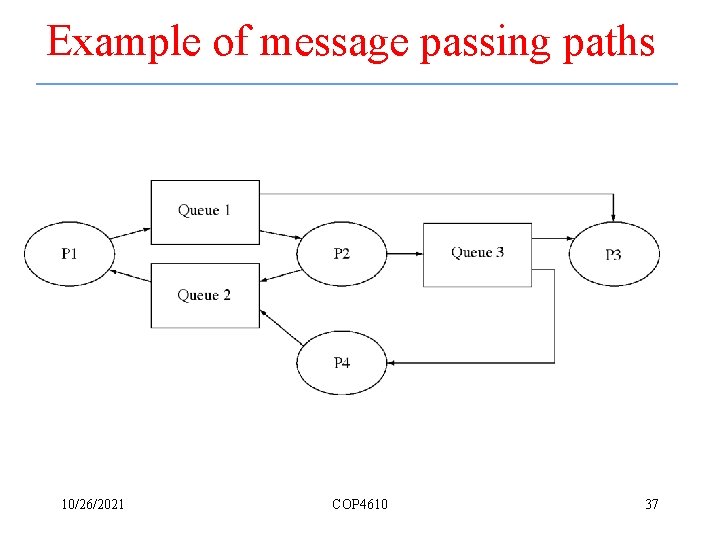
Example of message passing paths 10/26/2021 COP 4610 37
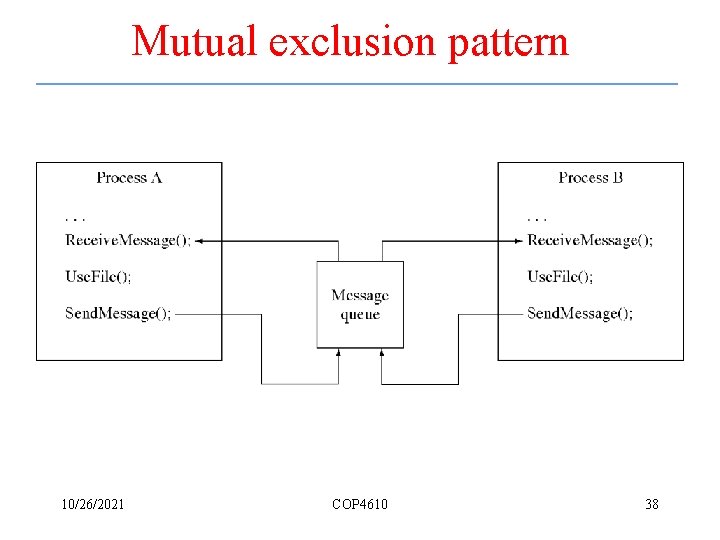
Mutual exclusion pattern 10/26/2021 COP 4610 38
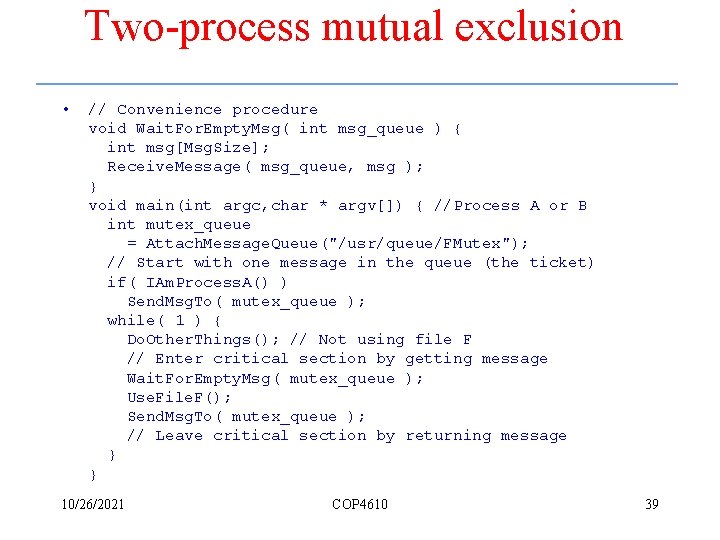
Two-process mutual exclusion • // Convenience procedure void Wait. For. Empty. Msg( int msg_queue ) { int msg[Msg. Size]; Receive. Message( msg_queue, msg ); } void main(int argc, char * argv[]) { //Process A or B int mutex_queue = Attach. Message. Queue("/usr/queue/FMutex"); // Start with one message in the queue (the ticket) if( IAm. Process. A() ) Send. Msg. To( mutex_queue ); while( 1 ) { Do. Other. Things(); // Not using file F // Enter critical section by getting message Wait. For. Empty. Msg( mutex_queue ); Use. File. F(); Send. Msg. To( mutex_queue ); // Leave critical section by returning message } } 10/26/2021 COP 4610 39
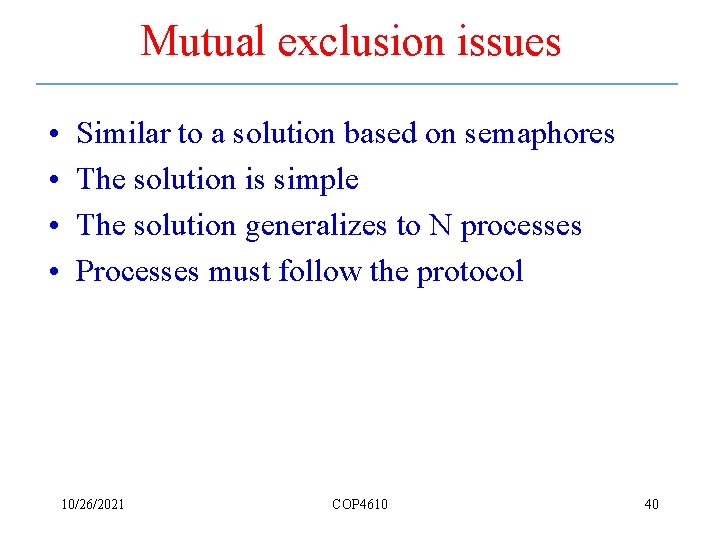
Mutual exclusion issues • • Similar to a solution based on semaphores The solution is simple The solution generalizes to N processes Processes must follow the protocol 10/26/2021 COP 4610 40
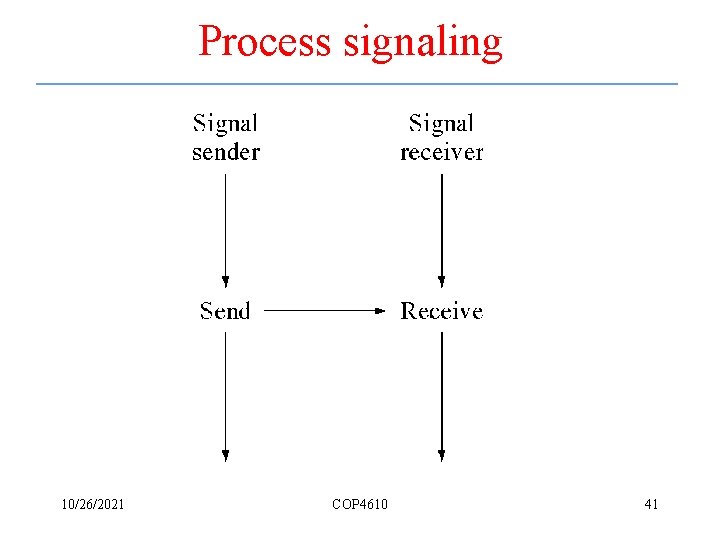
Process signaling 10/26/2021 COP 4610 41
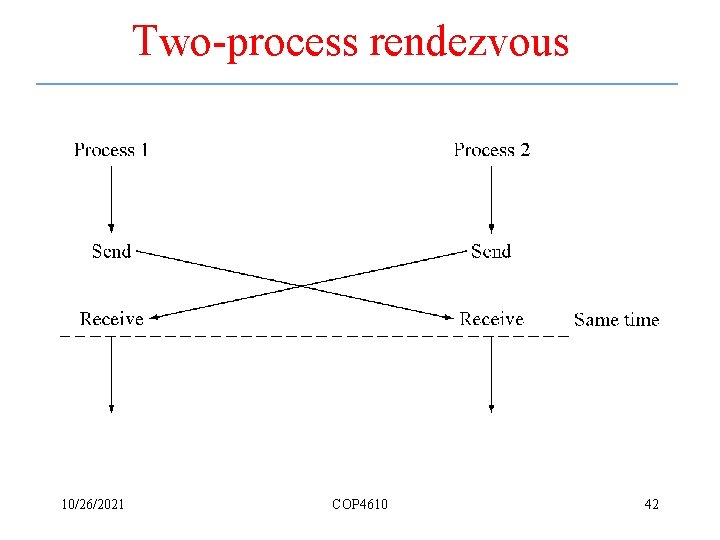
Two-process rendezvous 10/26/2021 COP 4610 42
![Twoprocess rendezvous void main int argc char argv Game Two-process rendezvous • void main( int argc, char *argv[ ] ) { // Game](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-43.jpg)
Two-process rendezvous • void main( int argc, char *argv[ ] ) { // Game Player A int b_queue = Attach. Message. Queue("/usr/queue/gpb"); Send. Msg. To( b_queue, Ready. To. Start ); int a_queue = Attach. Message. Queue("/usr/queue/gpa"); Wait. For. Empty. Msg( a_queue ); } void main( int argc, char *argv[ ] ) { // Game Player B int a_queue = Attach. Message. Queue("/usr/queue/gpa"); Send. Msg. To( a_queue, Ready. To. Start ); int b_queue = Attach. Message. Queue("/usr/queue/gpb"); Wait. For. Empty. Msg( b_queue ); } 10/26/2021 COP 4610 43
![Manyprocess rendezvous void mainint argc char argv Coordinator int msgMsg Many-process rendezvous • void main(int argc, char *argv[ ]) { // Coordinator int msg[Msg.](https://slidetodoc.com/presentation_image_h2/ec44d5d37756ae1c54a069c9f909a86d/image-44.jpg)
Many-process rendezvous • void main(int argc, char *argv[ ]) { // Coordinator int msg[Msg. Size]; int player[Number. Of. Players], coordinator_queue = Attach. Message. Queue( "/usr/queue/coordq" ); for( i = 0; i < Number. Of. Players; ++i ) { Receive. Message( coordinator_queue, msg ); player[i] = msg[1]; } for( i = 0; i < Number. Of. Players; ++i ) Send. Msg. To( player[i], Begin. Playing ); } void main( int argc, char *argv[ ] ) { // Player I int coordinator_queue = Attach. Message. Queue( "/usr/queue/coordq" ); char qname[32]; sprintf( qname, "/usr/queue/%s", argv[1] ); int my_queue = Attach. Message. Queue( qname ); Send. Msg. To(coordinator_queue, Ready. To. Start, my_queue); Wait. For. Empty. Msg( my_queue ); } 10/26/2021 COP 4610 44
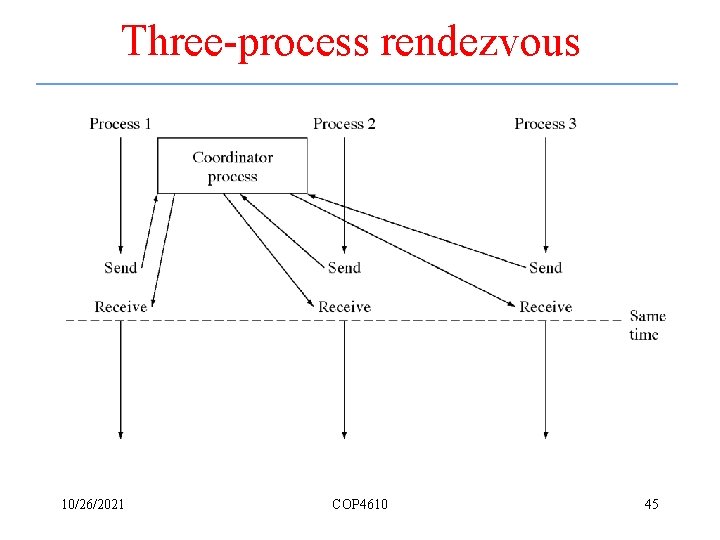
Three-process rendezvous 10/26/2021 COP 4610 45
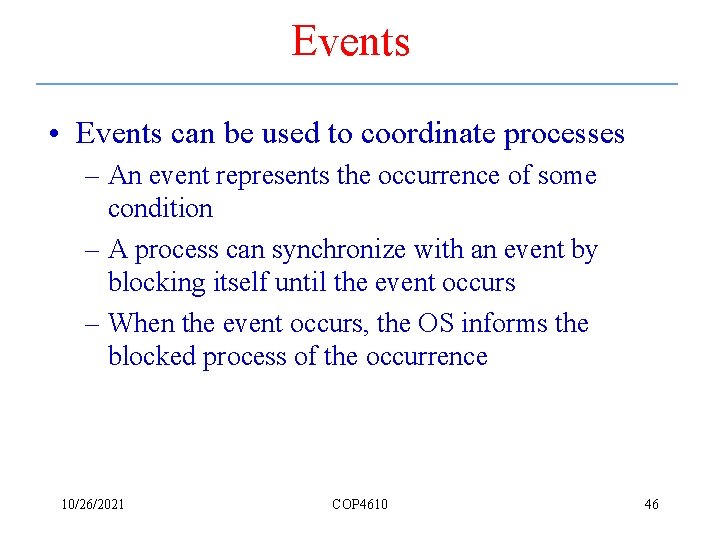
Events • Events can be used to coordinate processes – An event represents the occurrence of some condition – A process can synchronize with an event by blocking itself until the event occurs – When the event occurs, the OS informs the blocked process of the occurrence 10/26/2021 COP 4610 46
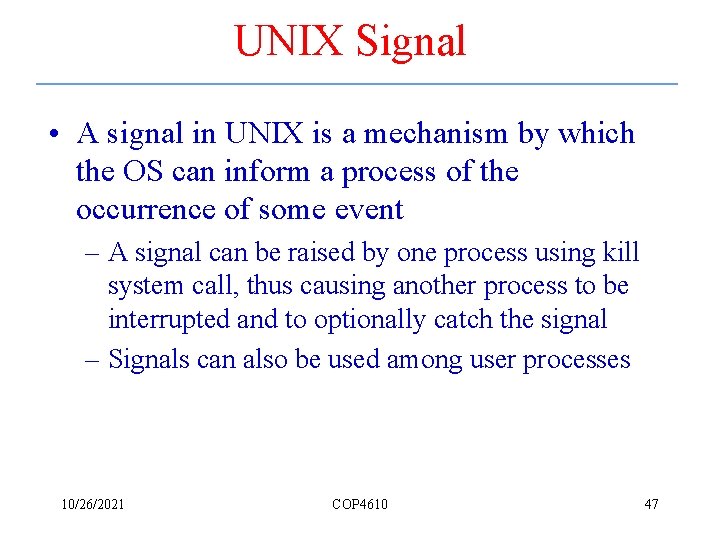
UNIX Signal • A signal in UNIX is a mechanism by which the OS can inform a process of the occurrence of some event – A signal can be raised by one process using kill system call, thus causing another process to be interrupted and to optionally catch the signal – Signals can also be used among user processes 10/26/2021 COP 4610 47
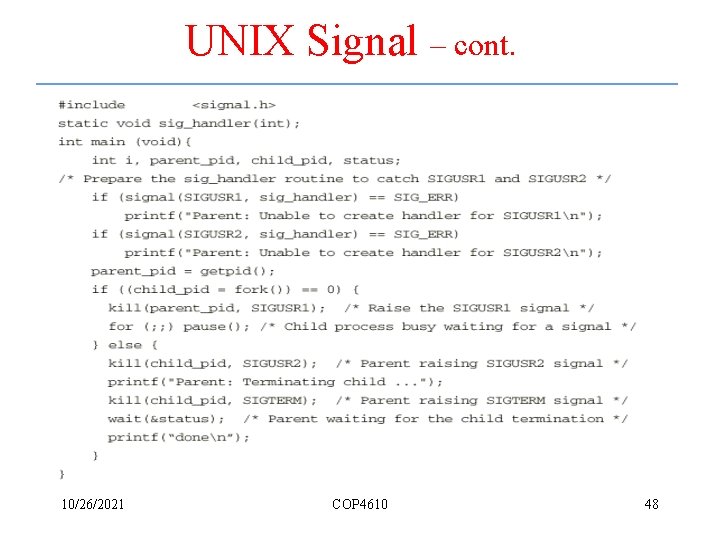
UNIX Signal – cont. 10/26/2021 COP 4610 48
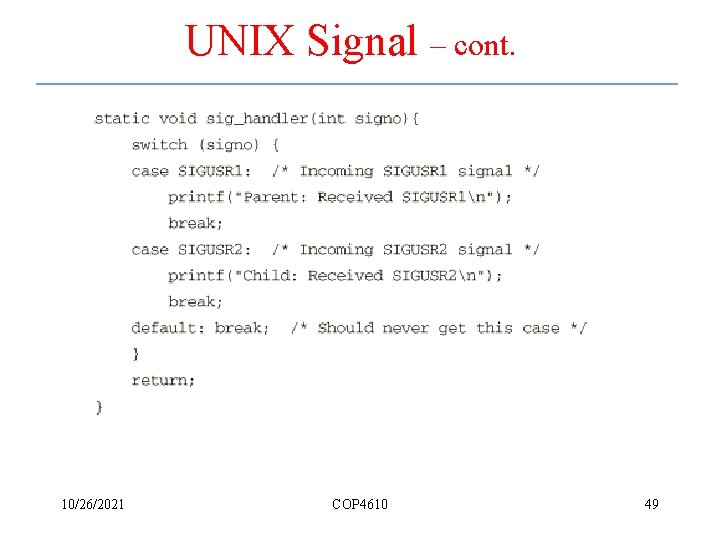
UNIX Signal – cont. 10/26/2021 COP 4610 49
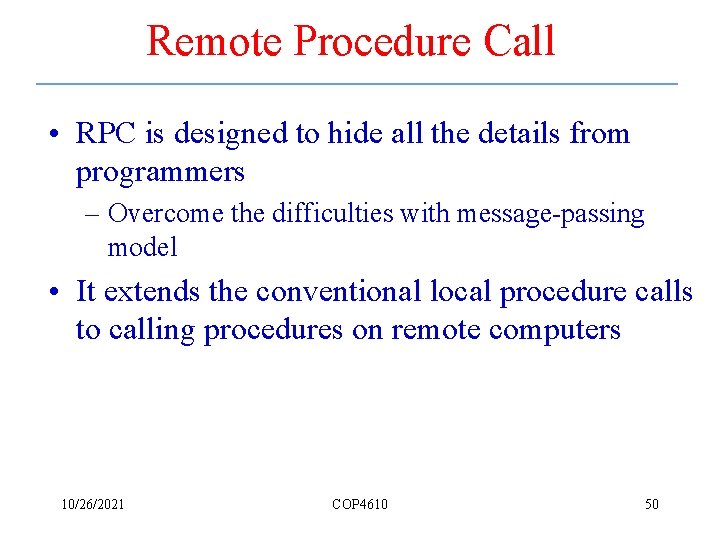
Remote Procedure Call • RPC is designed to hide all the details from programmers – Overcome the difficulties with message-passing model • It extends the conventional local procedure calls to calling procedures on remote computers 10/26/2021 COP 4610 50
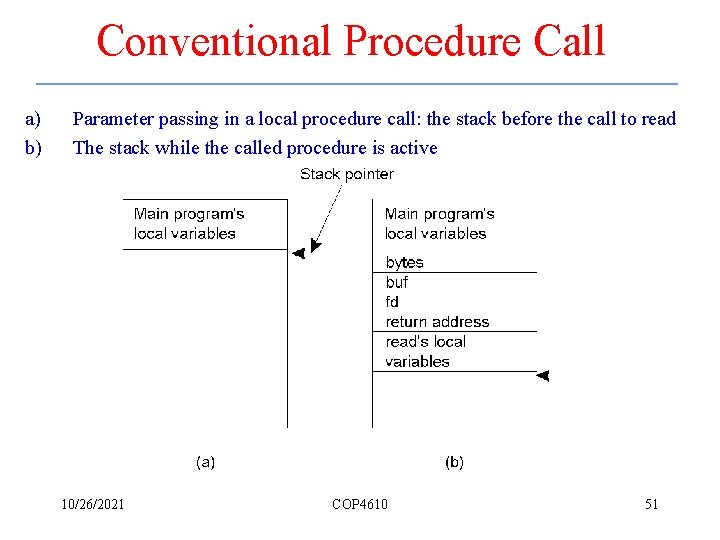
Conventional Procedure Call a) b) Parameter passing in a local procedure call: the stack before the call to read The stack while the called procedure is active 10/26/2021 COP 4610 51
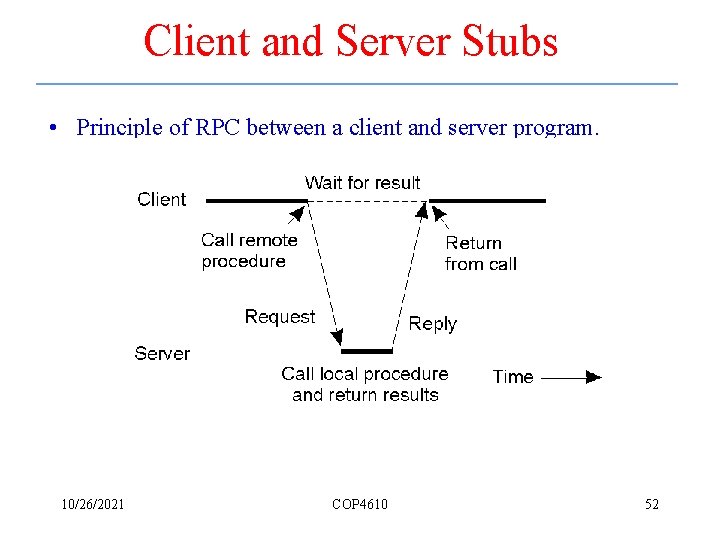
Client and Server Stubs • Principle of RPC between a client and server program. 10/26/2021 COP 4610 52
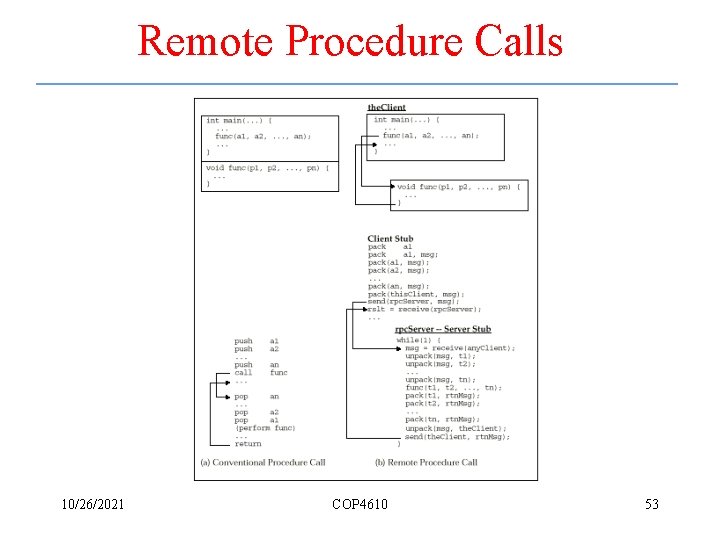
Remote Procedure Calls 10/26/2021 COP 4610 53
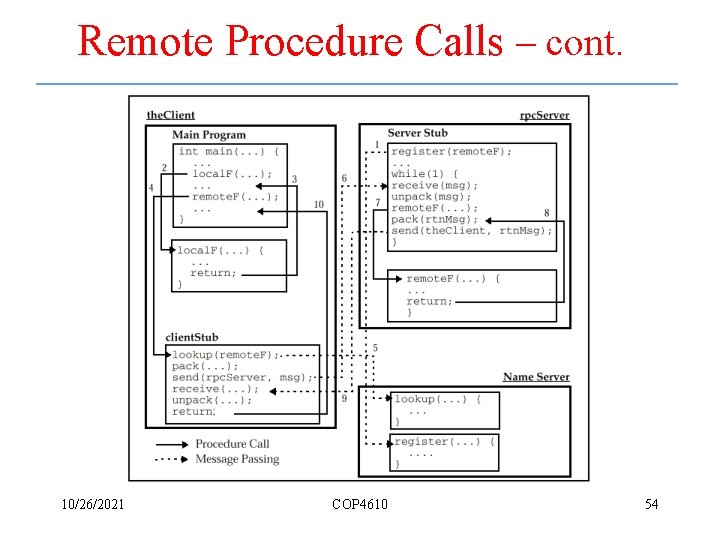
Remote Procedure Calls – cont. 10/26/2021 COP 4610 54
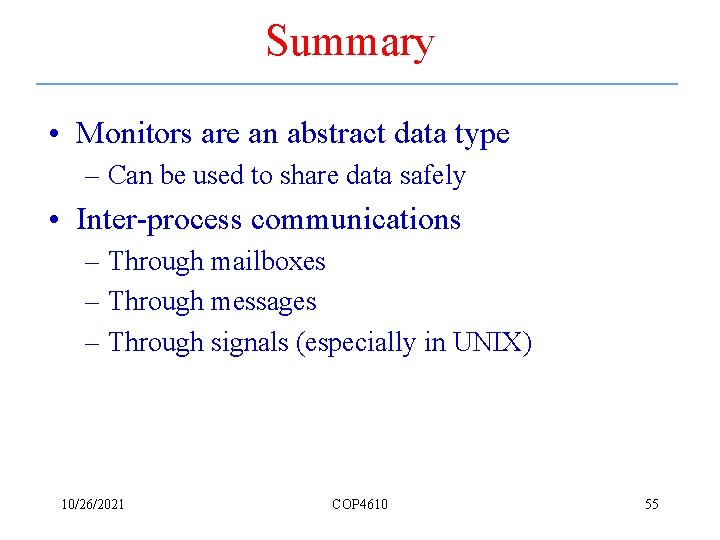
Summary • Monitors are an abstract data type – Can be used to share data safely • Inter-process communications – Through mailboxes – Through messages – Through signals (especially in UNIX) 10/26/2021 COP 4610 55
High level synchronization construct
High level outline
Little book of semaphores
Disadvantages of semaphore
The main disadvantage of mutex lock is
Pvu official announcement
R/announcements!
Semaphores provide a primitive yet powerful and flexible
Sleeping barber problem using semaphores in c
Church announcements
Types of semaphore
Test and set
What did mildred regret losing in the fire
Semaphores
Kayl announcements
Semaphores and monitors
General announcements
Non synthesizable constructs in verilog
Concepts constructs and variables
Procedural constructs
Php language constructs
The calvin cycle constructs
Sending and receiving signals are special constructs of
Control flow constructs
Cfir constructs
Encapsulation constructs in ppl
Low-level thinking in high-level shading languages
Quote sandwich example
Fast clock to slow clock synchronization
Process synchronization in os
Data synchronization in tally
Multiprocessor synchronization
What is lean synchronization
Soft-reconfiguration inbound
Lock free synchronization
Classical problem of synchronization in operating system
Synchronization tool in os
The bounded buffer problem in operating system
Basic synchronization principles
Windchill workspace synchronization
Cuda global synchronization
Process synchronization definition
Time frequency domain
Pthread synchronization
Shared memory in unix
Synchronization primitives c#
Synchronization algorithms and concurrent programming
Process synchronization definition
Linux kernel synchronization
Kernel synchronization in linux
Synchronization in distributed systems
Wait free synchronization
Synchronization tools in operating system
Supply chain synchronization
Synchronization in computer architecture
User123haru