Methods Why Write Methods Methods are commonly used
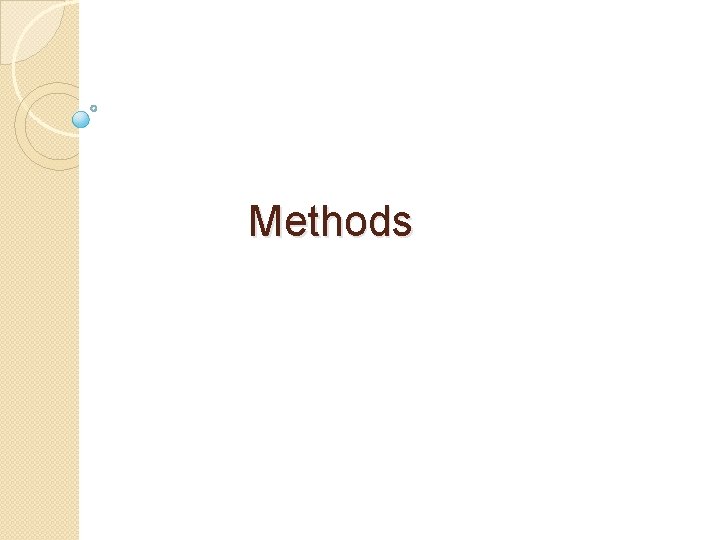
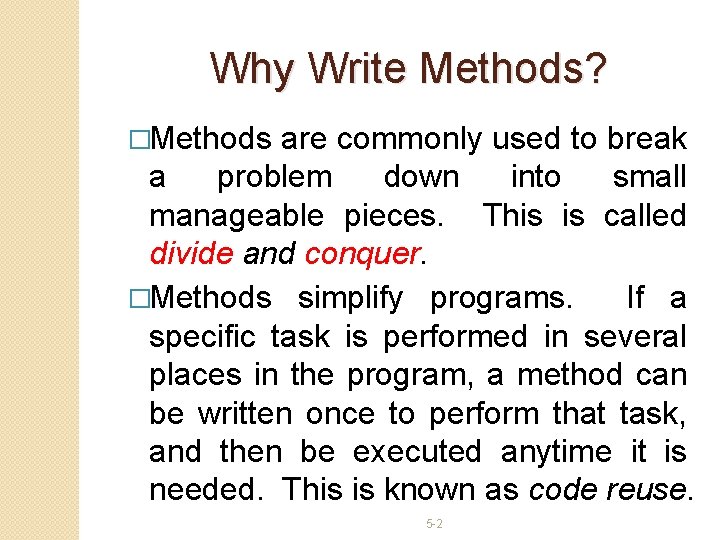
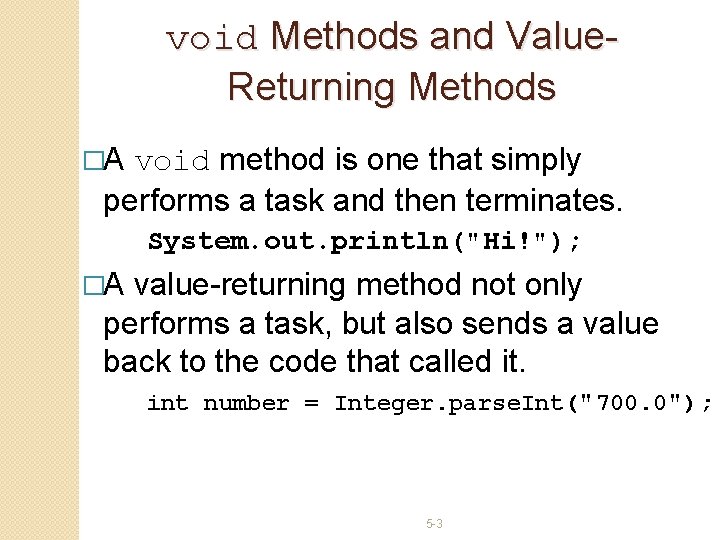
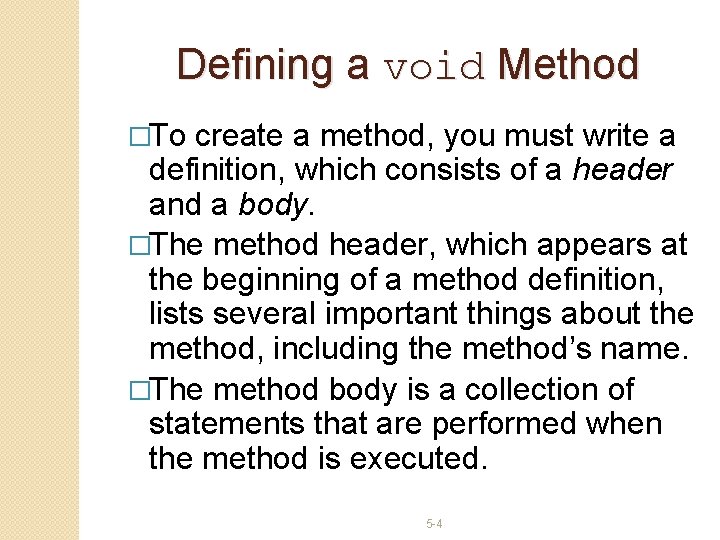
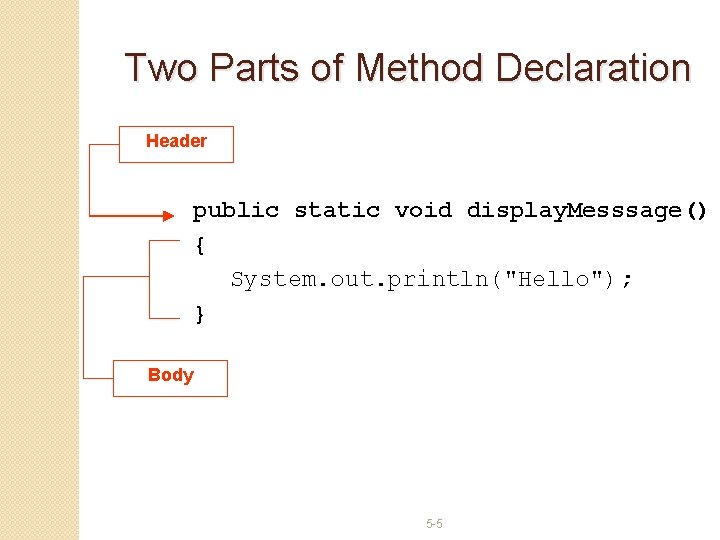
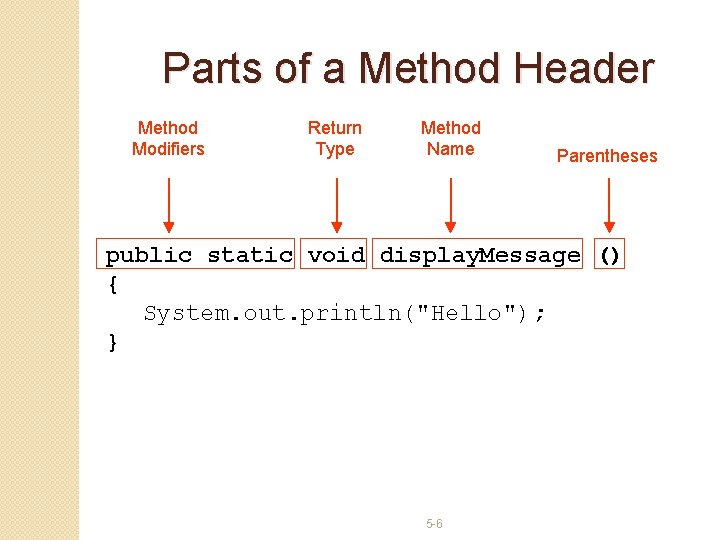
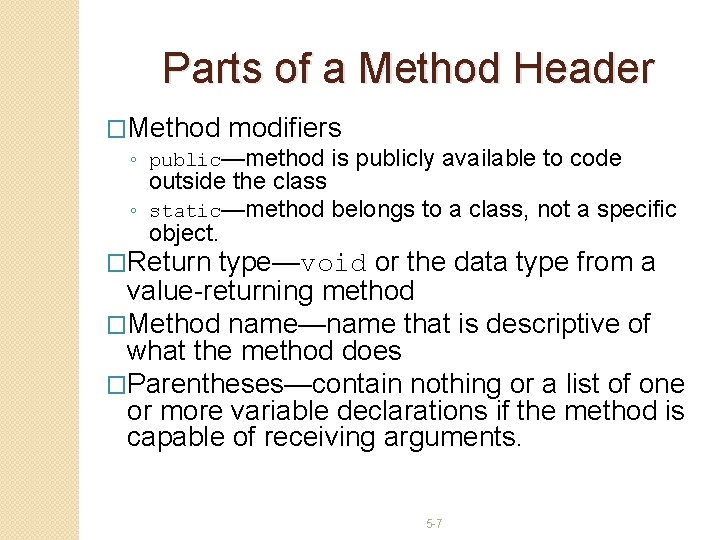
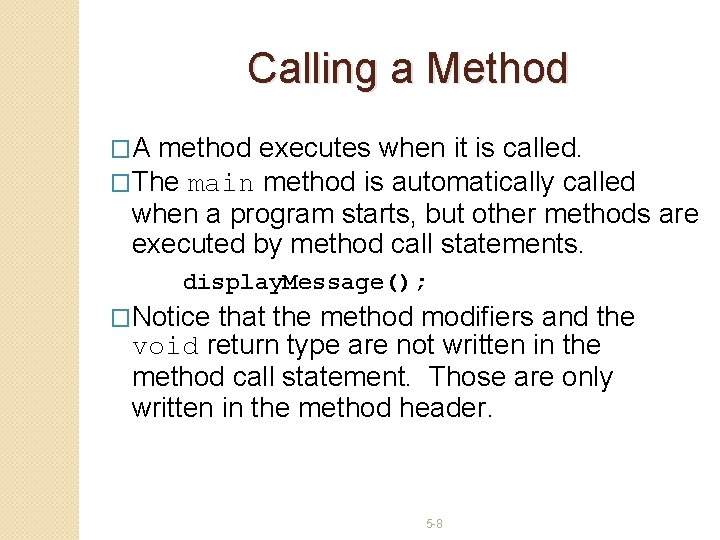
![Calling a Method public class Simple. Method { public static void main(String[] args) { Calling a Method public class Simple. Method { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-9.jpg)
![Hierarchical Method Calls public class Deep. And. Deeper { public static void main(String[] args) Hierarchical Method Calls public class Deep. And. Deeper { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-10.jpg)
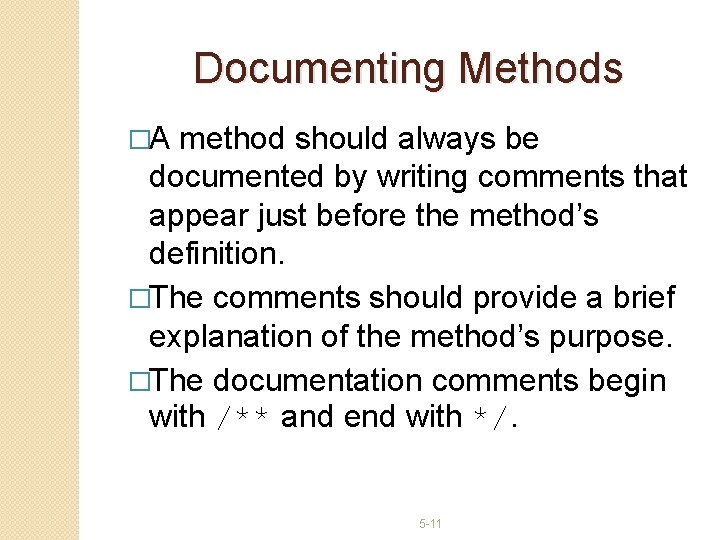
![Documenting Methods public class Simple. Method { public static void main(String[] args) { System. Documenting Methods public class Simple. Method { public static void main(String[] args) { System.](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-12.jpg)
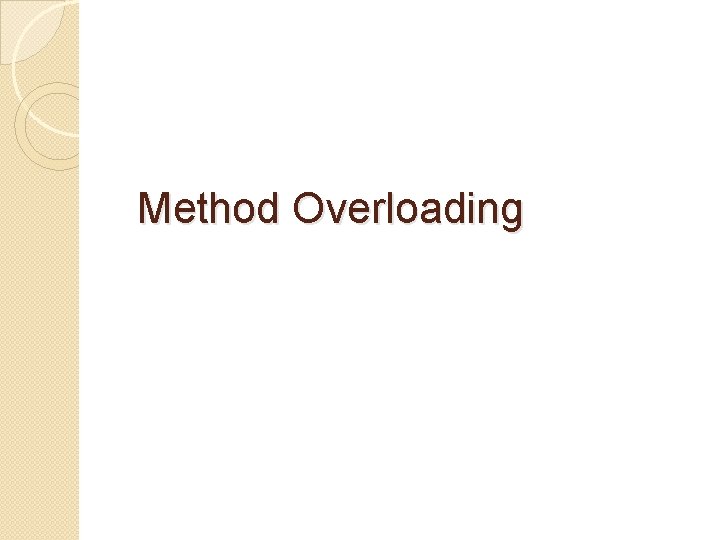
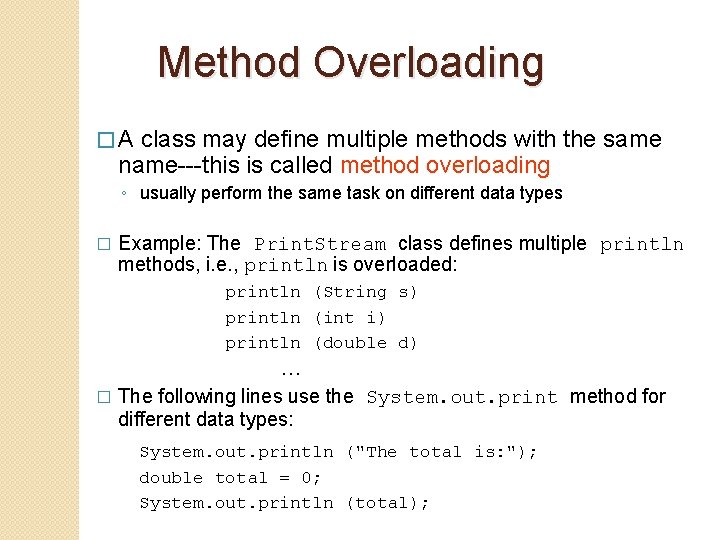
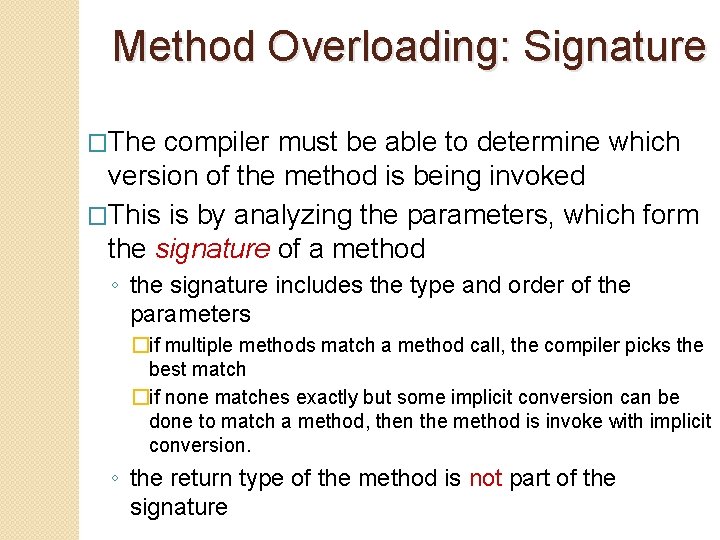
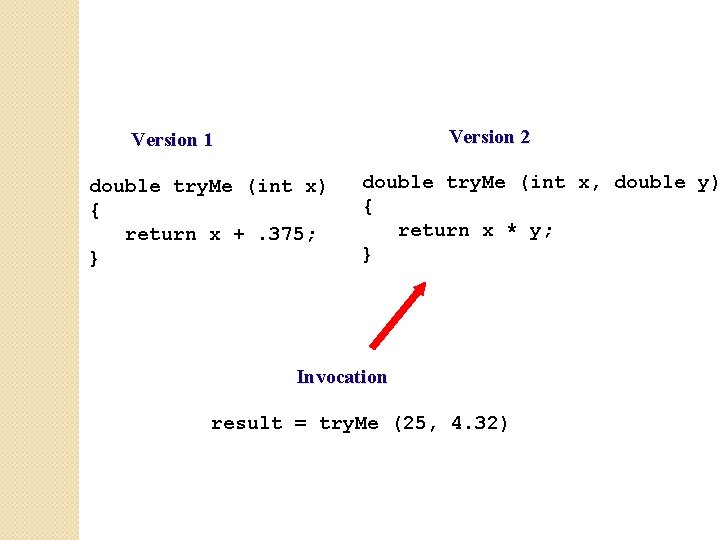
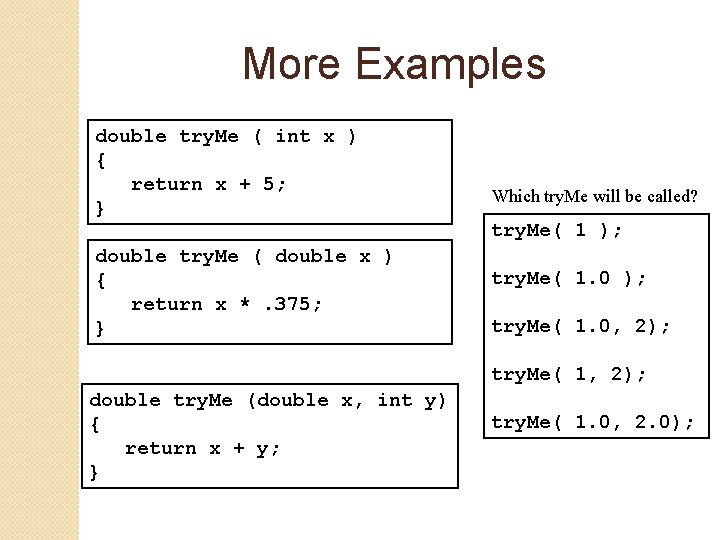
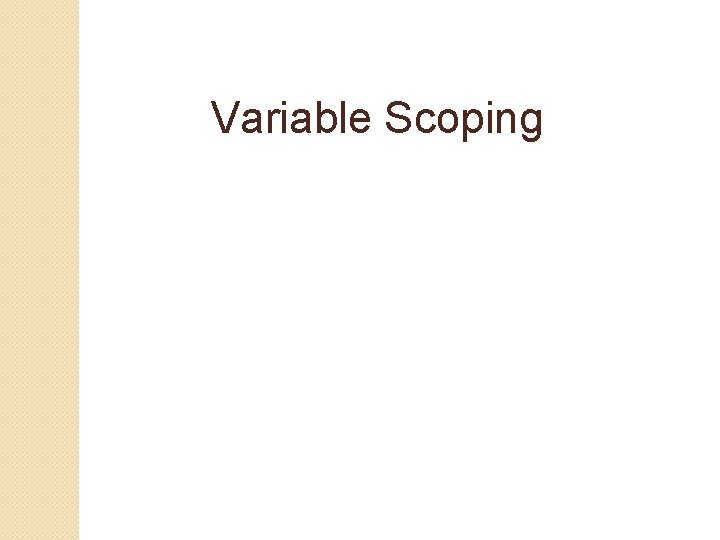
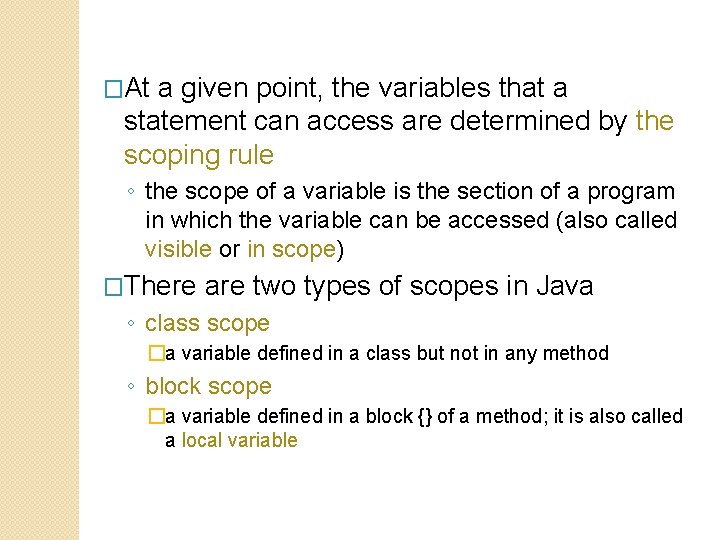
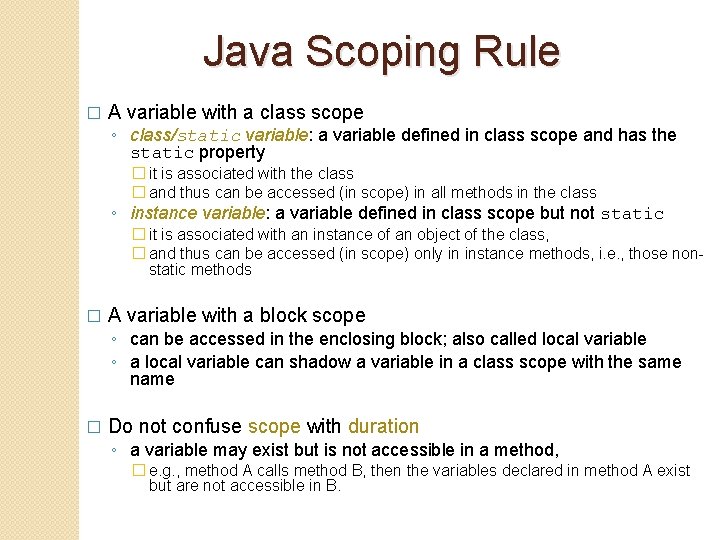
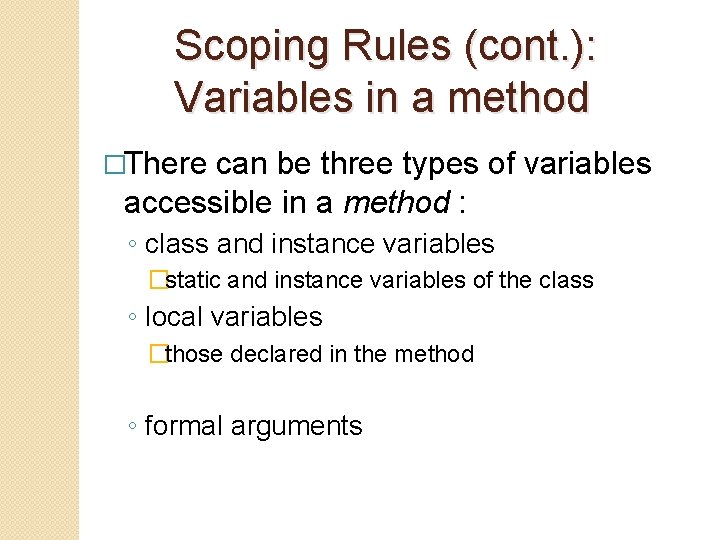
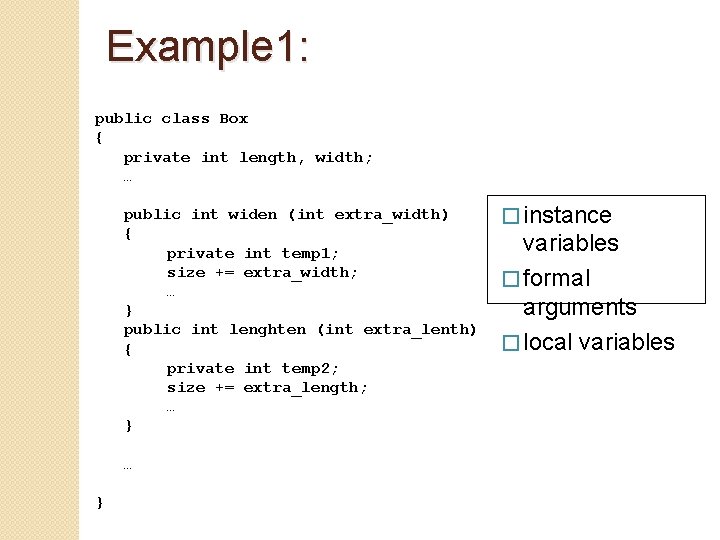
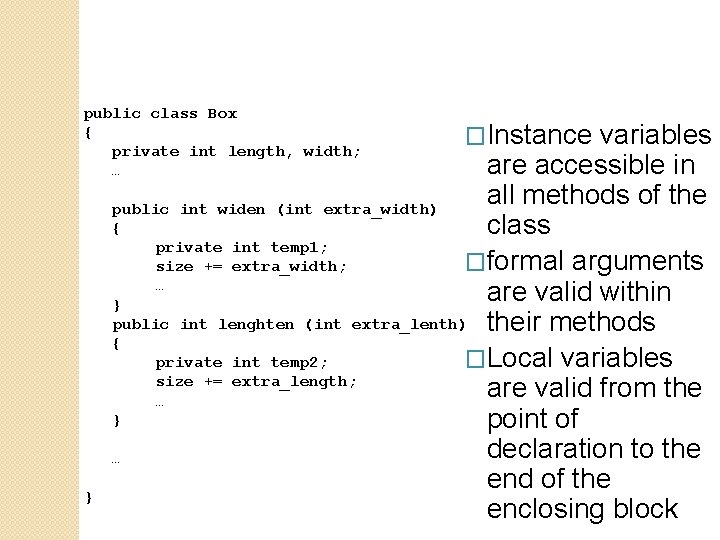
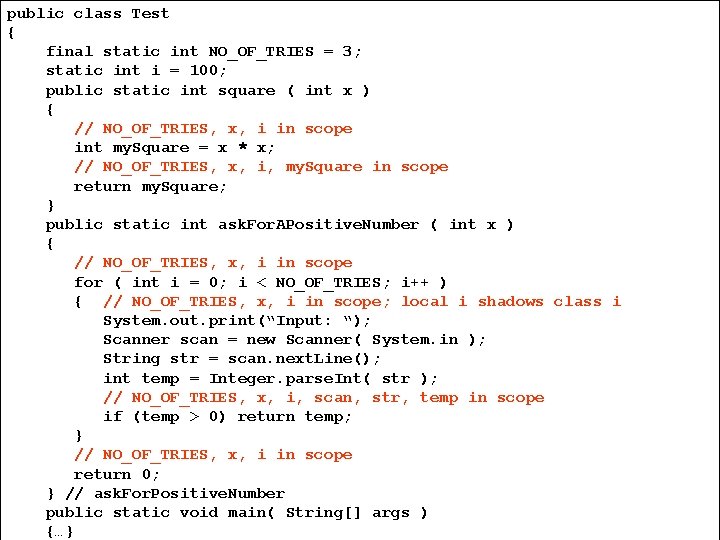
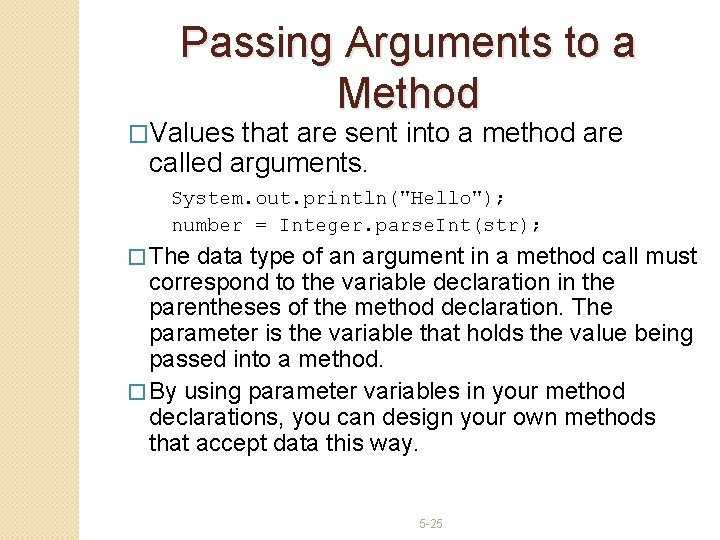
![Passing Arguments to a Method public class Pass. Arg { public static void main(String[] Passing Arguments to a Method public class Pass. Arg { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-26.jpg)
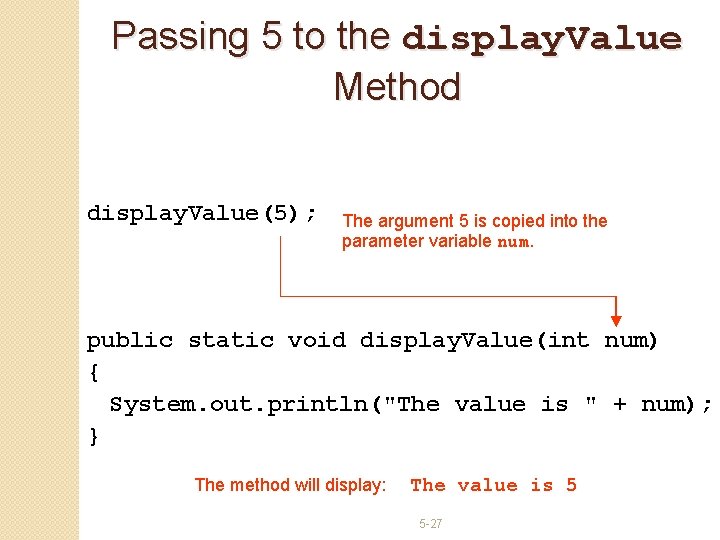
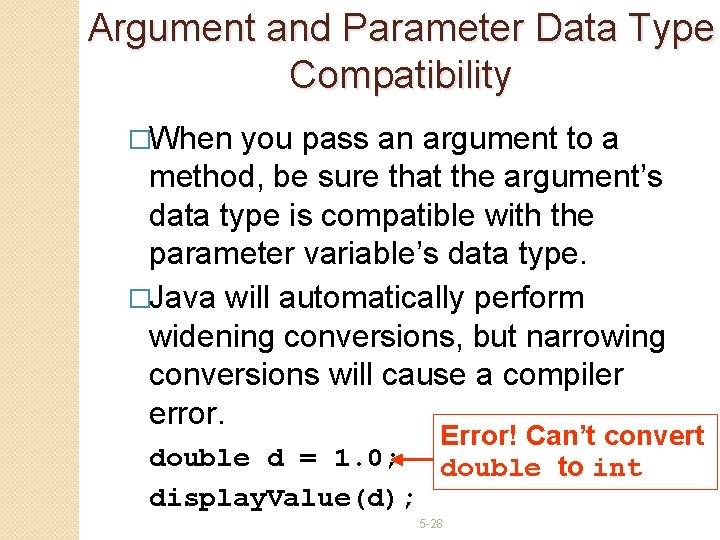
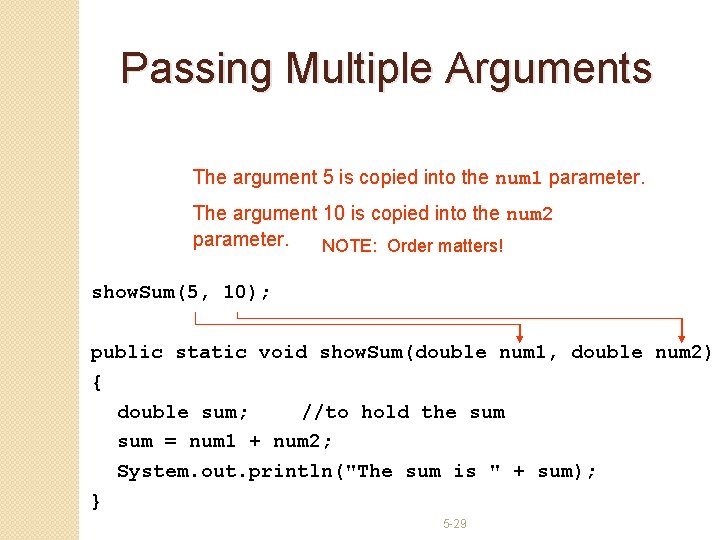
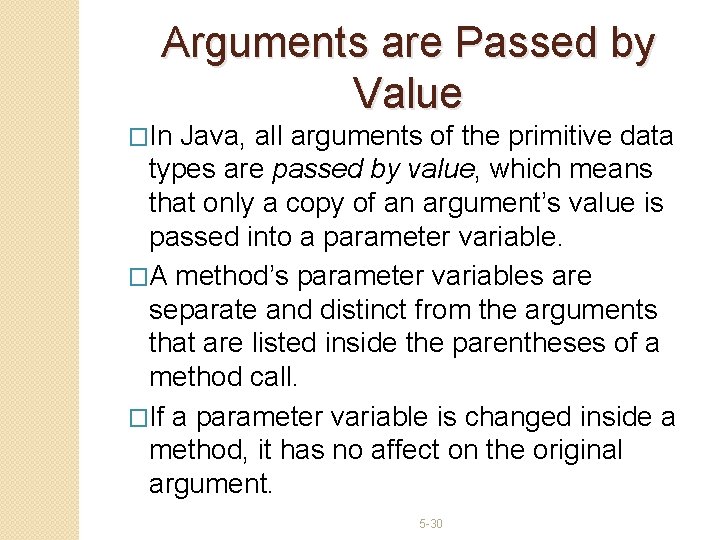
![public class Pass. Arg { public static void main(String[] args) { int number = public class Pass. Arg { public static void main(String[] args) { int number =](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-31.jpg)
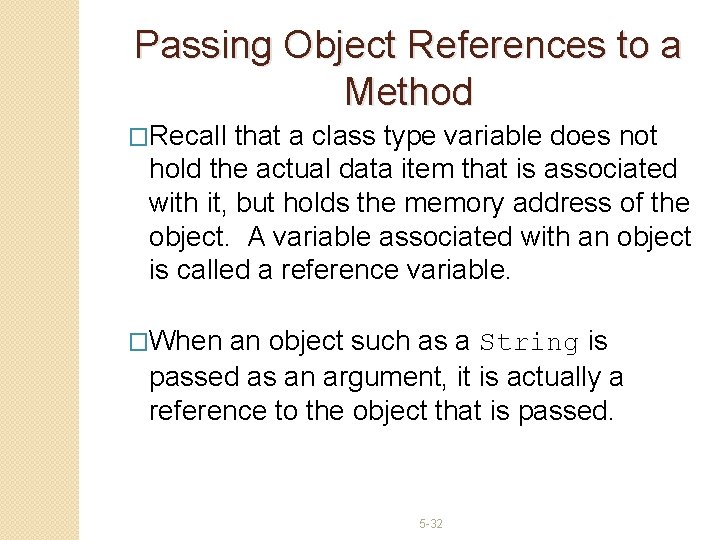
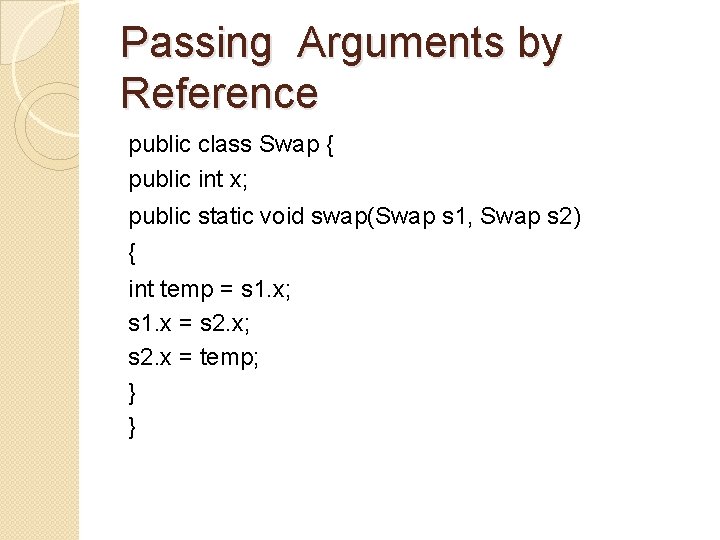
![class Swap. Test{ public static void main(String args[]) { Swap a 1 = new class Swap. Test{ public static void main(String args[]) { Swap a 1 = new](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-34.jpg)
![class Swap. Test{ public static void main(String args[]) { Swap a 1 = new class Swap. Test{ public static void main(String args[]) { Swap a 1 = new](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-35.jpg)
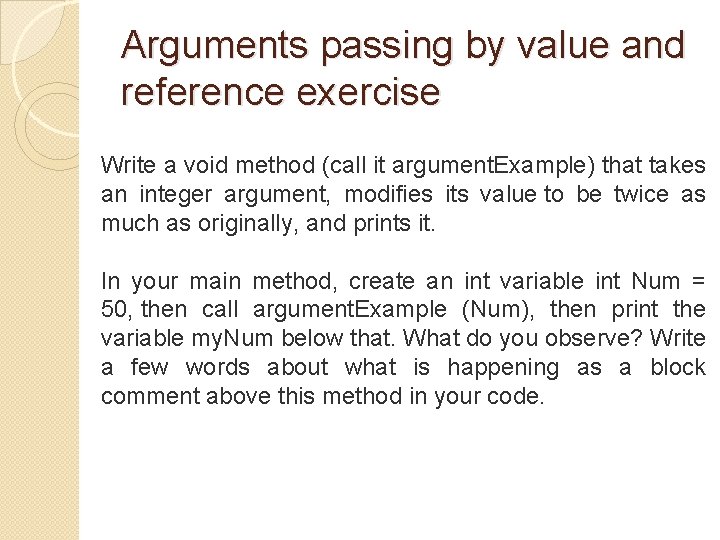
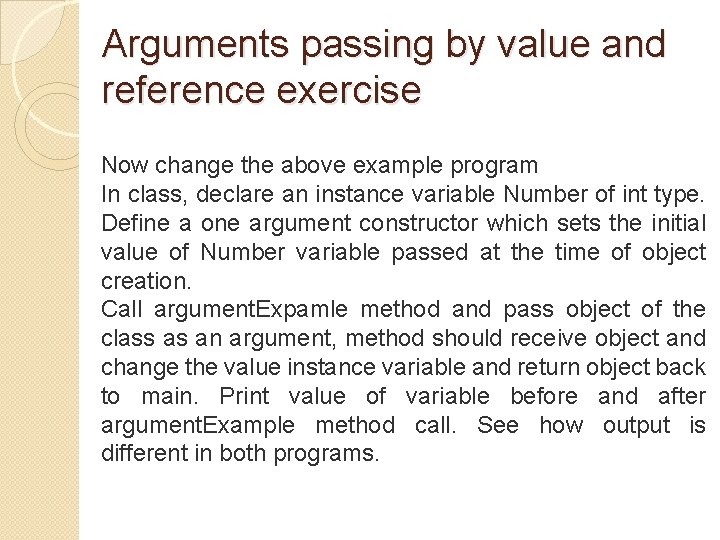
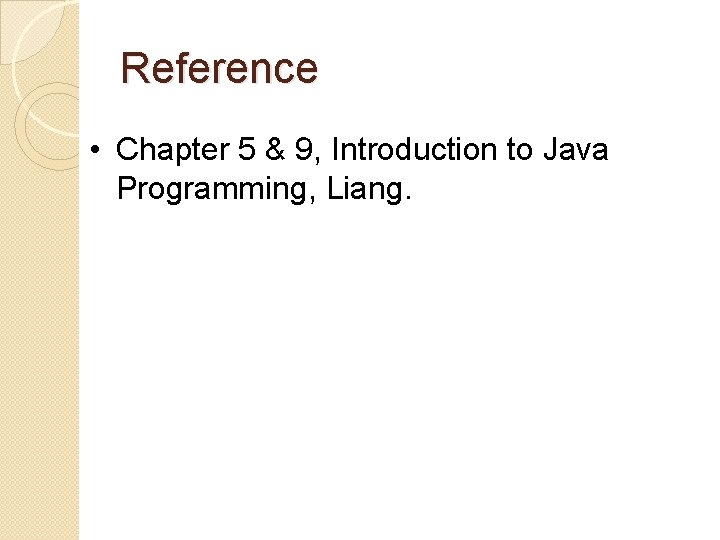
- Slides: 38
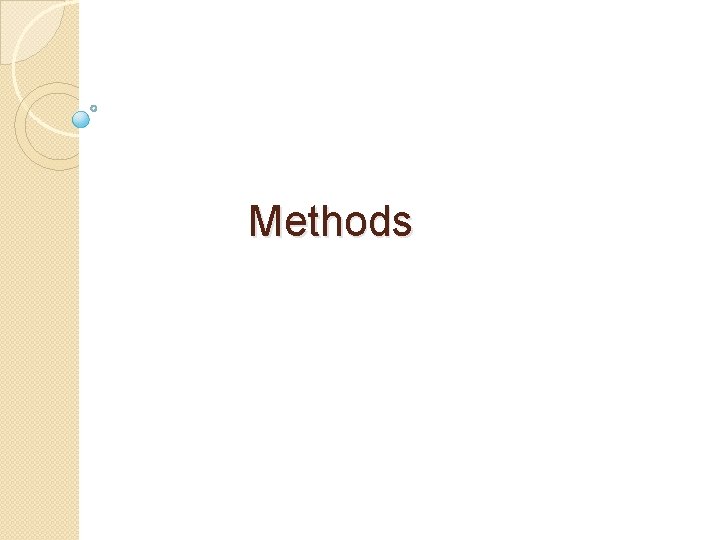
Methods
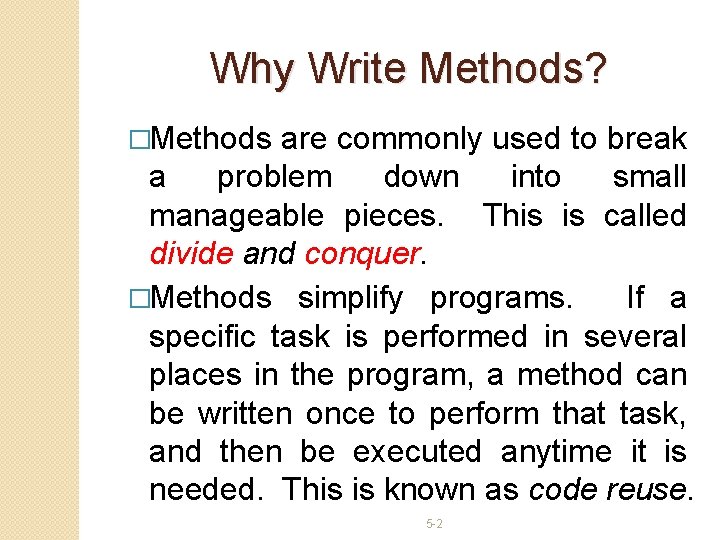
Why Write Methods? �Methods are commonly used to break a problem down into small manageable pieces. This is called divide and conquer. �Methods simplify programs. If a specific task is performed in several places in the program, a method can be written once to perform that task, and then be executed anytime it is needed. This is known as code reuse. 5 -2
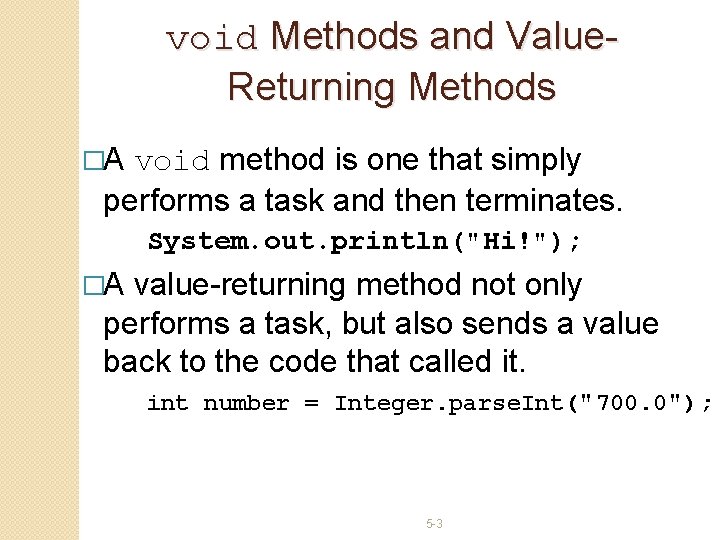
void Methods and Value. Returning Methods �A void method is one that simply performs a task and then terminates. System. out. println("Hi!"); �A value-returning method not only performs a task, but also sends a value back to the code that called it. int number = Integer. parse. Int("700. 0"); 5 -3
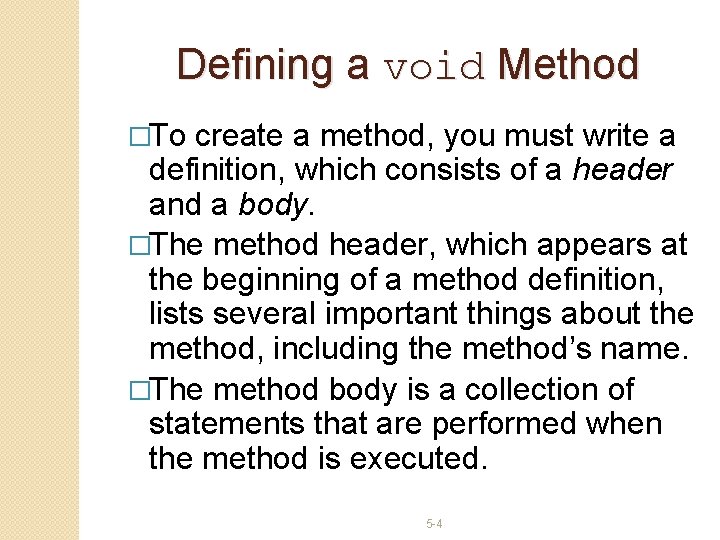
Defining a void Method �To create a method, you must write a definition, which consists of a header and a body. �The method header, which appears at the beginning of a method definition, lists several important things about the method, including the method’s name. �The method body is a collection of statements that are performed when the method is executed. 5 -4
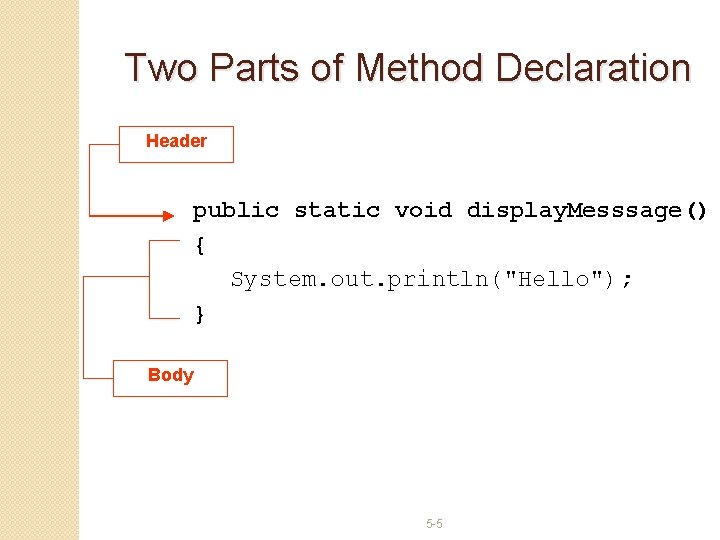
Two Parts of Method Declaration Header public static void display. Messsage() { System. out. println("Hello"); } Body 5 -5
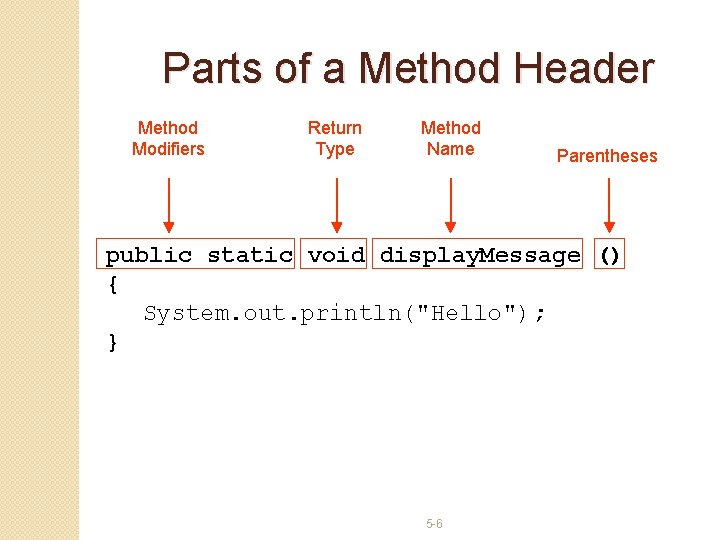
Parts of a Method Header Method Modifiers Return Type Method Name Parentheses public static void display. Message () { System. out. println("Hello"); } 5 -6
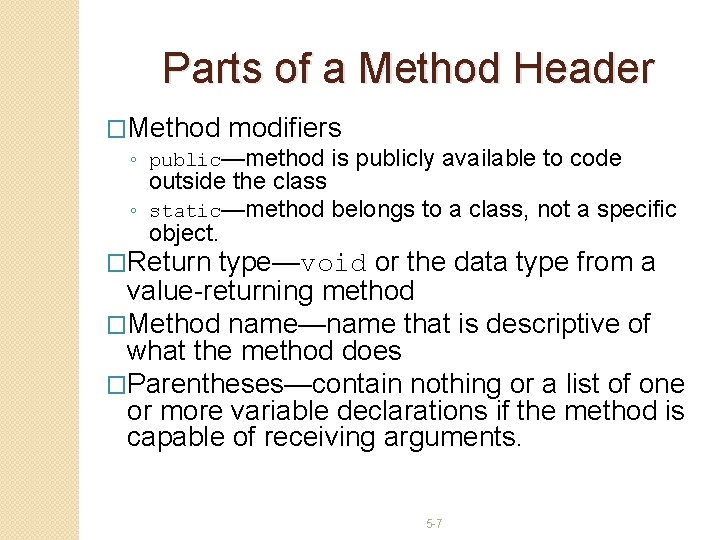
Parts of a Method Header �Method modifiers ◦ public—method is publicly available to code outside the class ◦ static—method belongs to a class, not a specific object. �Return type—void or the data type from a value-returning method �Method name—name that is descriptive of what the method does �Parentheses—contain nothing or a list of one or more variable declarations if the method is capable of receiving arguments. 5 -7
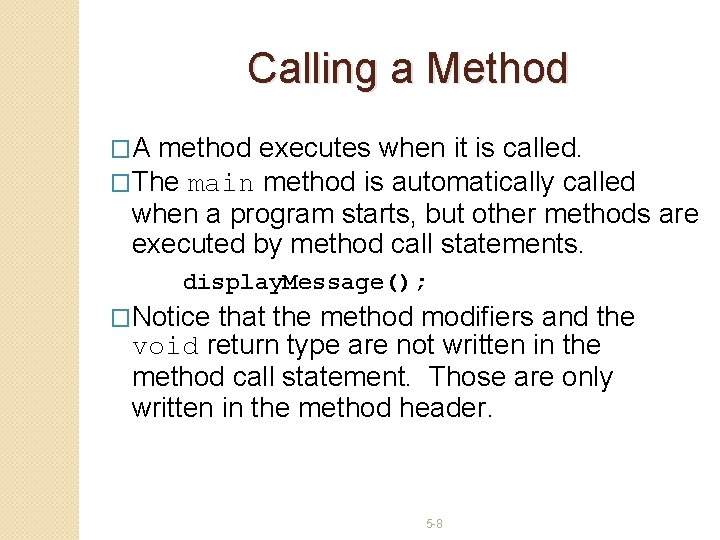
Calling a Method �A method executes when it is called. �The main method is automatically called when a program starts, but other methods are executed by method call statements. display. Message(); �Notice that the method modifiers and the void return type are not written in the method call statement. Those are only written in the method header. 5 -8
![Calling a Method public class Simple Method public static void mainString args Calling a Method public class Simple. Method { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-9.jpg)
Calling a Method public class Simple. Method { public static void main(String[] args) { System. out. println(“Hello from the main method!”); display. Message(); System. out. println(“Back in the main method. ”); } public static void display. Message() { System. out. println(“Hello from the display. Message method!”); } } 5 -9
![Hierarchical Method Calls public class Deep And Deeper public static void mainString args Hierarchical Method Calls public class Deep. And. Deeper { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-10.jpg)
Hierarchical Method Calls public class Deep. And. Deeper { public static void main(String[] args) { System. out. println(“I am starting in main. ”); 1 deep(); 2 System. out. println(“Now I am back in the main method. ”); 7 } public static void deep()2 { System. out. println(“I am now in deep. ”); 3 deeper(); 4 System. out. println(“Now I am back in deep. ”); 6 } public static void deeper() 4 { System. out. println(“I am now in deeper. ”); 5 } } 5 -10
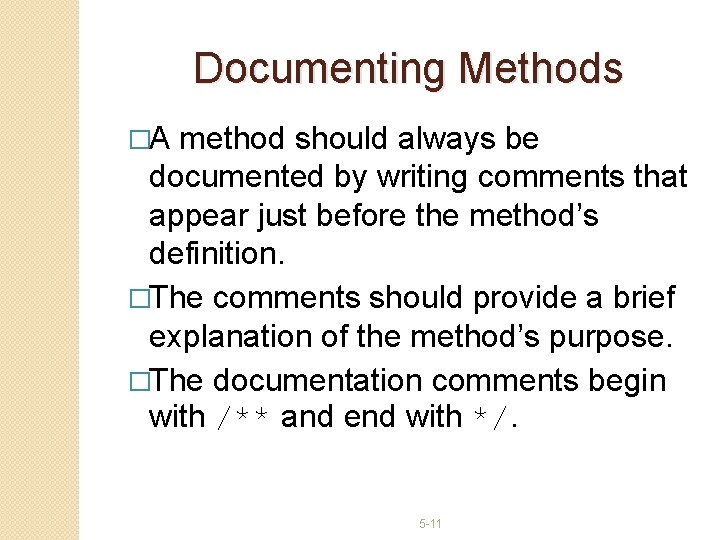
Documenting Methods �A method should always be documented by writing comments that appear just before the method’s definition. �The comments should provide a brief explanation of the method’s purpose. �The documentation comments begin with /** and end with */. 5 -11
![Documenting Methods public class Simple Method public static void mainString args System Documenting Methods public class Simple. Method { public static void main(String[] args) { System.](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-12.jpg)
Documenting Methods public class Simple. Method { public static void main(String[] args) { System. out. println(“Hello from the main method!”); display. Message(); System. out. println(“Back in the main method. ”); } /** The display. Message method displays a greeting */ public static void display. Message() { System. out. println(“Hello from the display. Message method!”); } } 5 -12
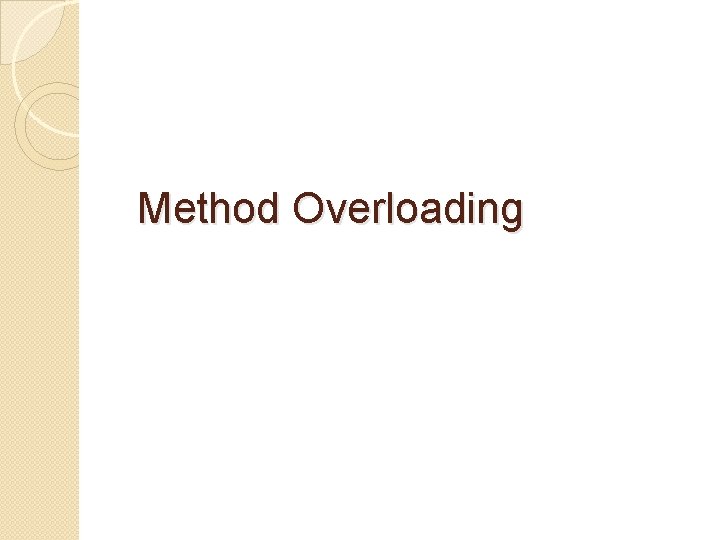
Method Overloading
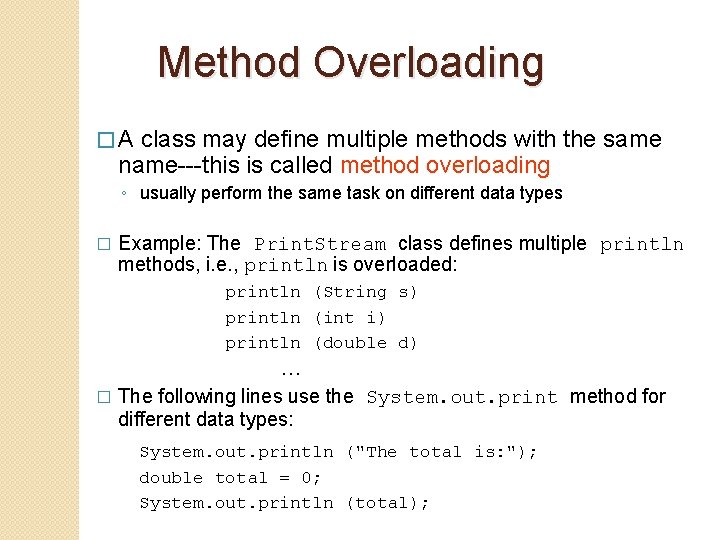
Method Overloading �A class may define multiple methods with the same name---this is called method overloading ◦ usually perform the same task on different data types � Example: The Print. Stream class defines multiple println methods, i. e. , println is overloaded: println (String s) println (int i) println (double d) … � The following lines use the System. out. print method for different data types: System. out. println ("The total is: "); double total = 0; System. out. println (total);
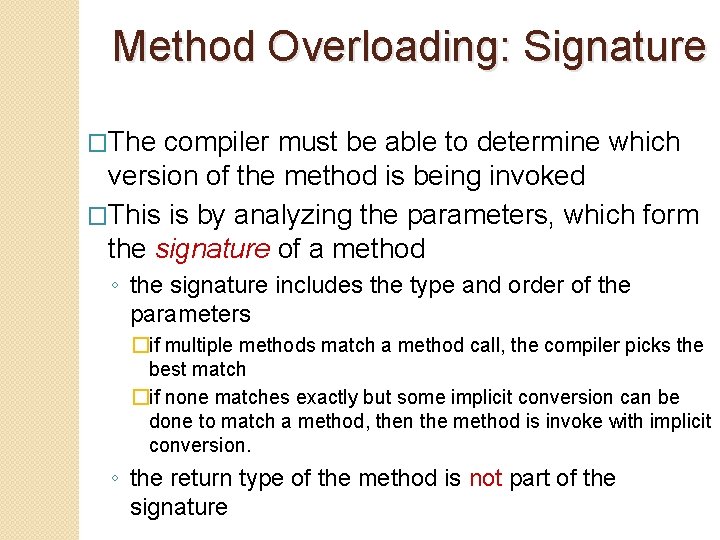
Method Overloading: Signature �The compiler must be able to determine which version of the method is being invoked �This is by analyzing the parameters, which form the signature of a method ◦ the signature includes the type and order of the parameters �if multiple methods match a method call, the compiler picks the best match �if none matches exactly but some implicit conversion can be done to match a method, then the method is invoke with implicit conversion. ◦ the return type of the method is not part of the signature
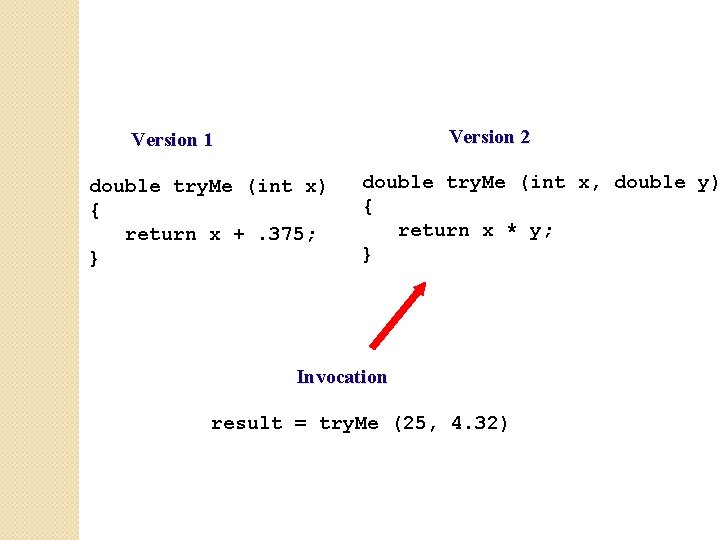
Version 2 Version 1 double try. Me (int x) { return x +. 375; } double try. Me (int x, double y) { return x * y; } Invocation result = try. Me (25, 4. 32)
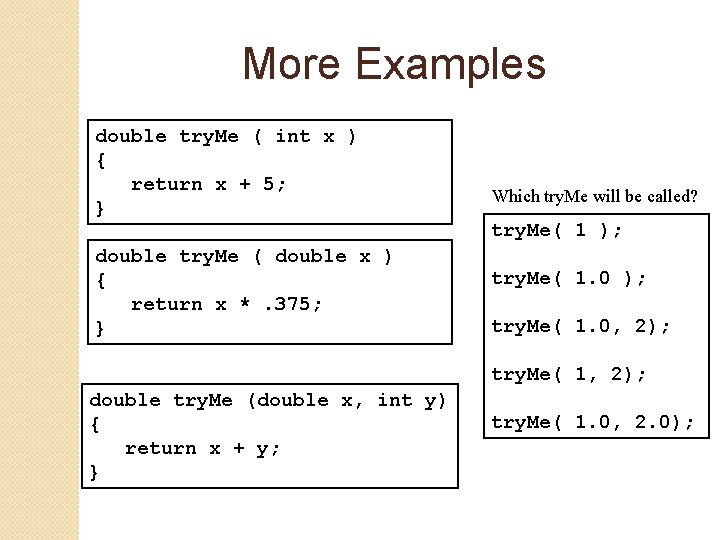
More Examples double try. Me ( int x ) { return x + 5; } double try. Me ( double x ) { return x *. 375; } Which try. Me will be called? try. Me( 1 ); try. Me( 1. 0, 2); try. Me( 1, 2); double try. Me (double x, int y) { return x + y; } try. Me( 1. 0, 2. 0);
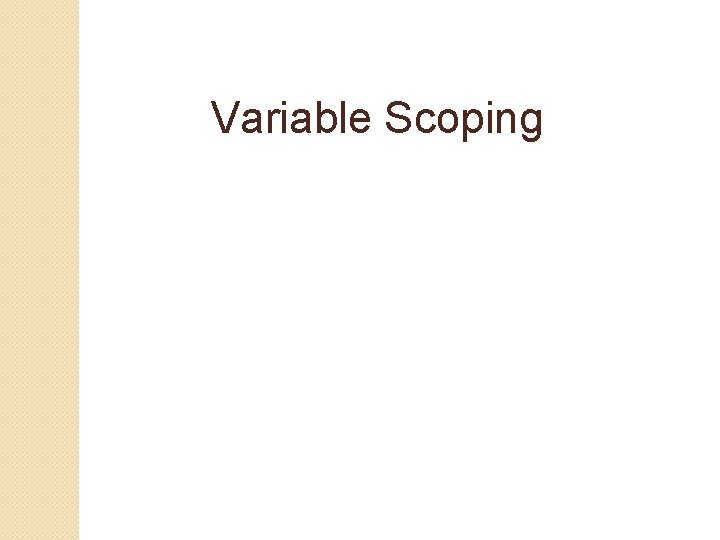
Variable Scoping
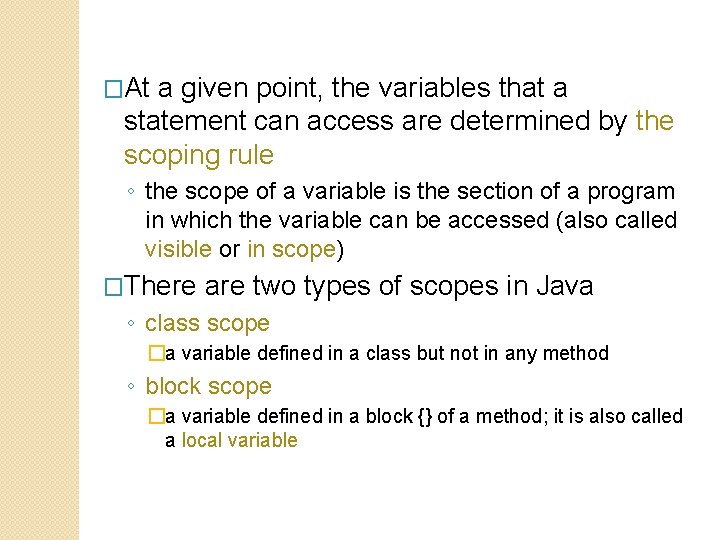
�At a given point, the variables that a statement can access are determined by the scoping rule ◦ the scope of a variable is the section of a program in which the variable can be accessed (also called visible or in scope) �There are two types of scopes in Java ◦ class scope �a variable defined in a class but not in any method ◦ block scope �a variable defined in a block {} of a method; it is also called a local variable
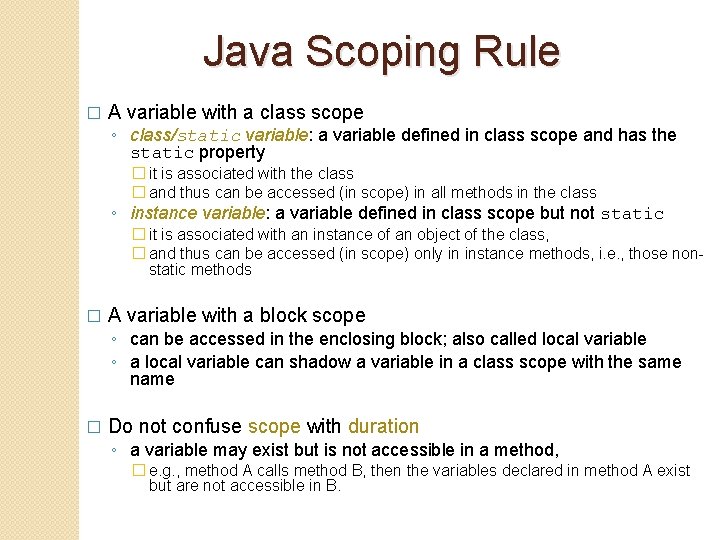
Java Scoping Rule � A variable with a class scope ◦ class/static variable: a variable defined in class scope and has the static property � it is associated with the class � and thus can be accessed (in scope) in all methods in the class ◦ instance variable: a variable defined in class scope but not static � it is associated with an instance of an object of the class, � and thus can be accessed (in scope) only in instance methods, i. e. , those nonstatic methods � A variable with a block scope ◦ can be accessed in the enclosing block; also called local variable ◦ a local variable can shadow a variable in a class scope with the same name � Do not confuse scope with duration ◦ a variable may exist but is not accessible in a method, � e. g. , method A calls method B, then the variables declared in method A exist but are not accessible in B.
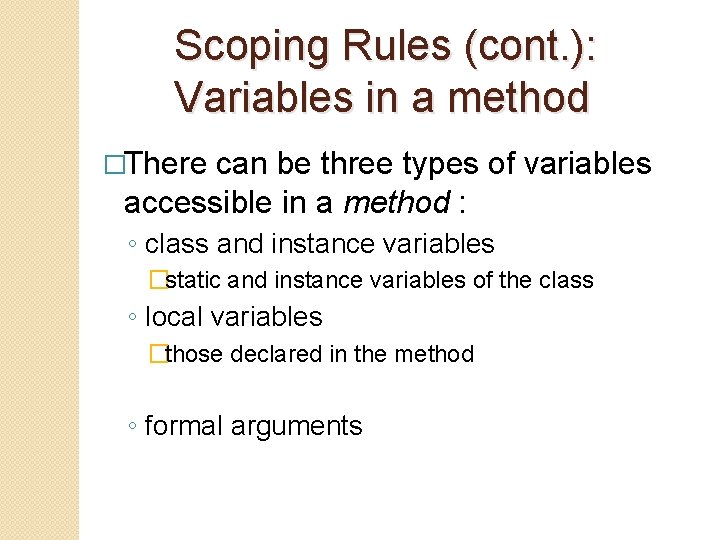
Scoping Rules (cont. ): Variables in a method �There can be three types of variables accessible in a method : ◦ class and instance variables �static and instance variables of the class ◦ local variables �those declared in the method ◦ formal arguments
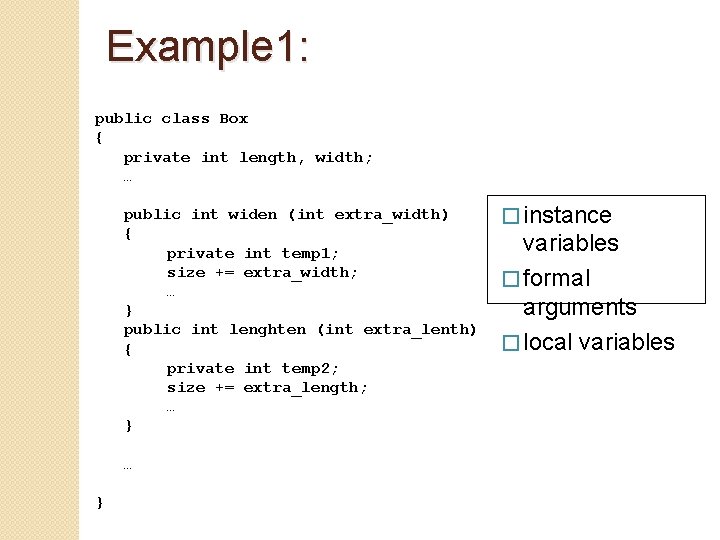
Example 1: public class Box { private int length, width; … public int widen (int extra_width) { private int temp 1; size += extra_width; … } public int lenghten (int extra_lenth) { private int temp 2; size += extra_length; … } � instance variables � formal arguments � local variables
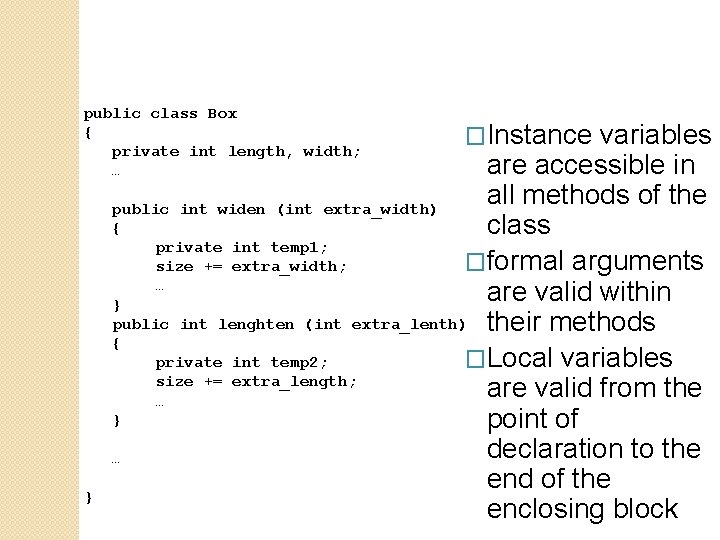
public class Box { private int length, width; … } �Instance variables are accessible in all methods of the public int widen (int extra_width) { class private int temp 1; �formal arguments size += extra_width; … are valid within } public int lenghten (int extra_lenth) their methods { �Local variables private int temp 2; size += extra_length; are valid from the … } point of declaration to the … end of the enclosing block
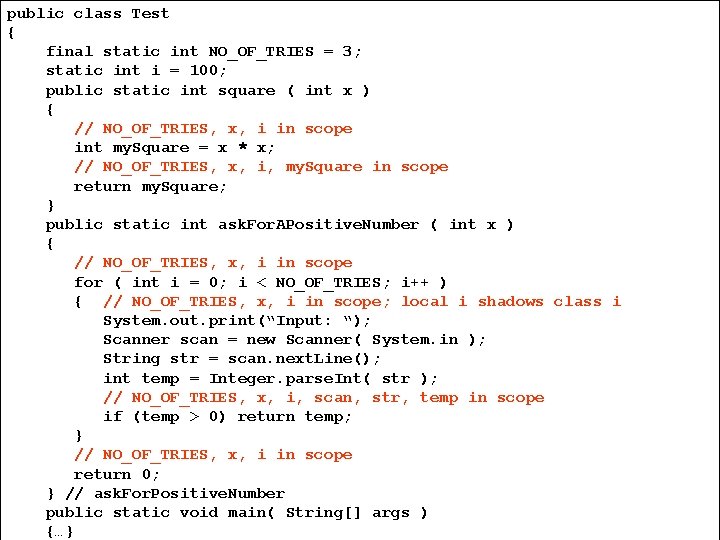
public class Test { final static int NO_OF_TRIES = 3; static int i = 100; public static int square ( int x ) { // NO_OF_TRIES, x, i in scope int my. Square = x * x; // NO_OF_TRIES, x, i, my. Square in scope return my. Square; } public static int ask. For. APositive. Number ( int x ) { // NO_OF_TRIES, x, i in scope for ( int i = 0; i < NO_OF_TRIES; i++ ) { // NO_OF_TRIES, x, i in scope; local i shadows class i System. out. print(“Input: “); Scanner scan = new Scanner( System. in ); String str = scan. next. Line(); int temp = Integer. parse. Int( str ); // NO_OF_TRIES, x, i, scan, str, temp in scope if (temp > 0) return temp; } // NO_OF_TRIES, x, i in scope return 0; } // ask. For. Positive. Number public static void main( String[] args ) {…}
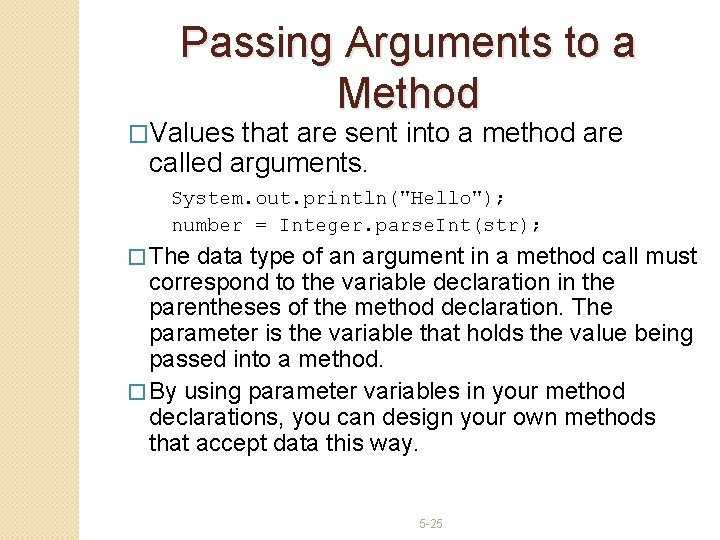
Passing Arguments to a Method �Values that are sent into a method are called arguments. System. out. println("Hello"); number = Integer. parse. Int(str); � The data type of an argument in a method call must correspond to the variable declaration in the parentheses of the method declaration. The parameter is the variable that holds the value being passed into a method. � By using parameter variables in your method declarations, you can design your own methods that accept data this way. 5 -25
![Passing Arguments to a Method public class Pass Arg public static void mainString Passing Arguments to a Method public class Pass. Arg { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-26.jpg)
Passing Arguments to a Method public class Pass. Arg { public static void main(String[] args) { int x = 10; display. Value(x); display. Value(x * 4); } public static void display. Value(int num) { System. out. println(“The value is: ” + num); } } 5 -26
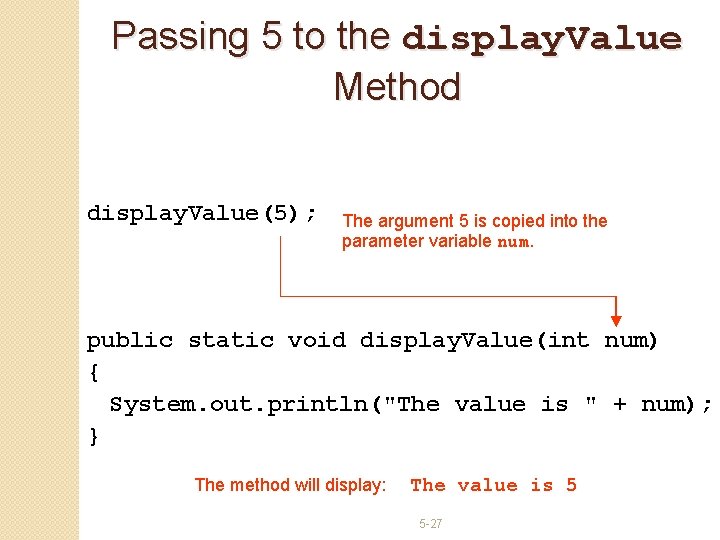
Passing 5 to the display. Value Method display. Value(5); The argument 5 is copied into the parameter variable num. public static void display. Value(int num) { System. out. println("The value is " + num); } The method will display: The value is 5 5 -27
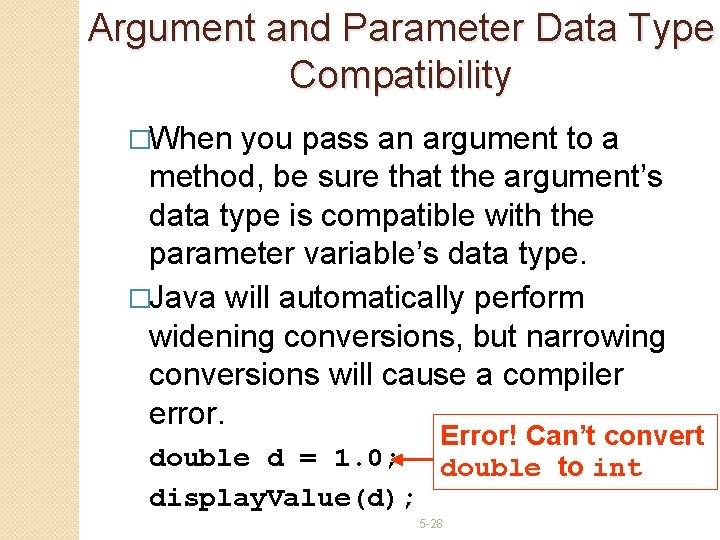
Argument and Parameter Data Type Compatibility �When you pass an argument to a method, be sure that the argument’s data type is compatible with the parameter variable’s data type. �Java will automatically perform widening conversions, but narrowing conversions will cause a compiler error. double d = 1. 0; display. Value(d); Error! Can’t convert double to int 5 -28
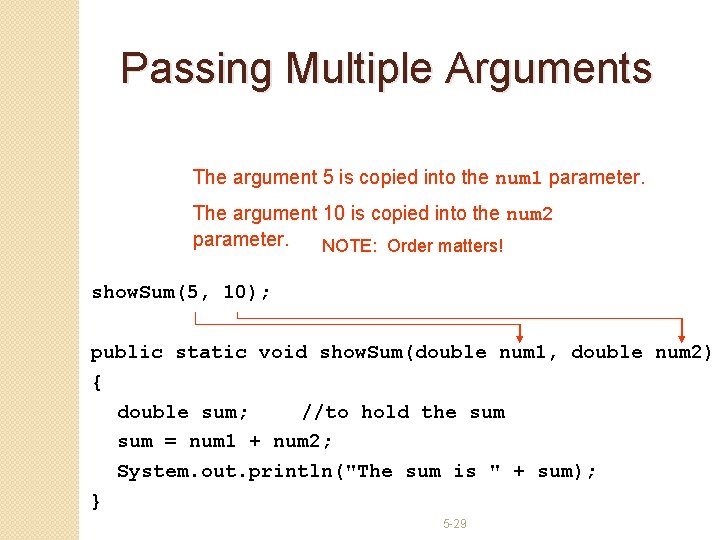
Passing Multiple Arguments The argument 5 is copied into the num 1 parameter. The argument 10 is copied into the num 2 parameter. NOTE: Order matters! show. Sum(5, 10); public static void show. Sum(double num 1, double num 2) { double sum; //to hold the sum = num 1 + num 2; System. out. println("The sum is " + sum); } 5 -29
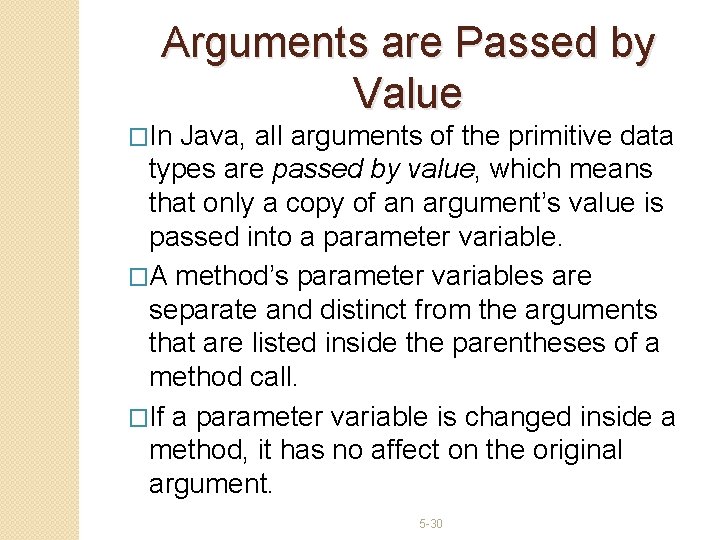
Arguments are Passed by Value �In Java, all arguments of the primitive data types are passed by value, which means that only a copy of an argument’s value is passed into a parameter variable. �A method’s parameter variables are separate and distinct from the arguments that are listed inside the parentheses of a method call. �If a parameter variable is changed inside a method, it has no affect on the original argument. 5 -30
![public class Pass Arg public static void mainString args int number public class Pass. Arg { public static void main(String[] args) { int number =](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-31.jpg)
public class Pass. Arg { public static void main(String[] args) { int number = 99; Program output Number is 99 I am changing the value Now the value is 0 Number is 99 System. out. println(“Number is “ + number); change. Me(number); System. out. println(“Number is “ + number); Only a copy of an argument’s value is passed into a parameter variable } public static void change. Me(int my. Value) { System. out. println(“I am changing the value. ”); my. Value = 0; System. out. println(“Now the value is “ + my. Value); } } 5 -31
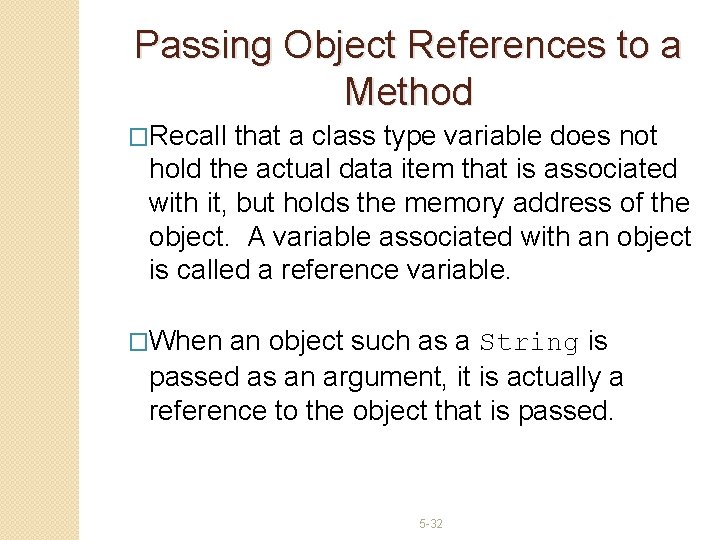
Passing Object References to a Method �Recall that a class type variable does not hold the actual data item that is associated with it, but holds the memory address of the object. A variable associated with an object is called a reference variable. �When an object such as a String is passed as an argument, it is actually a reference to the object that is passed. 5 -32
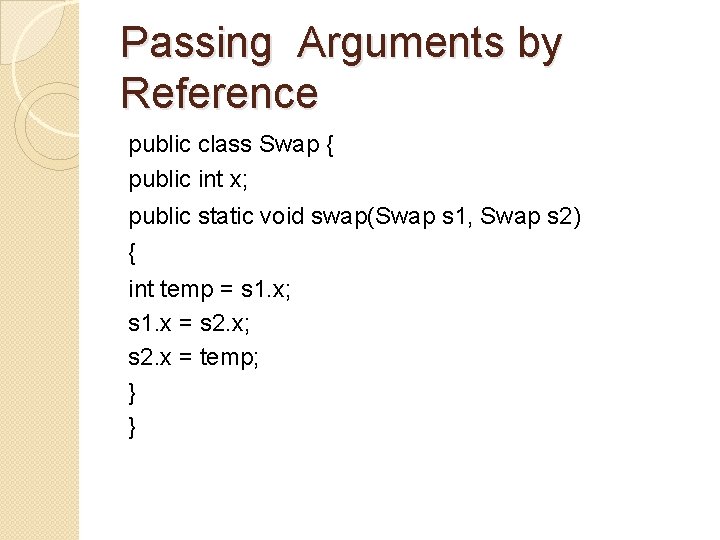
Passing Arguments by Reference public class Swap { public int x; public static void swap(Swap s 1, Swap s 2) { int temp = s 1. x; s 1. x = s 2. x; s 2. x = temp; } }
![class Swap Test public static void mainString args Swap a 1 new class Swap. Test{ public static void main(String args[]) { Swap a 1 = new](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-34.jpg)
class Swap. Test{ public static void main(String args[]) { Swap a 1 = new Swap(); // creating object Swap a 2 = new Swap(); a 1. x = 2; // assigning value to data field a 2. x = 5; System. out. println("Before swap"); System. out. println("a 1. x="+a 1. x+" a 2. x="+a 2. x); Swap. swap(a 1. x, a 2. x); System. out. println("After swap"); System. out. println("a 1. x="+a 1. x+" a 2. x="+a 2. x); } } Output: ? ?
![class Swap Test public static void mainString args Swap a 1 new class Swap. Test{ public static void main(String args[]) { Swap a 1 = new](https://slidetodoc.com/presentation_image_h2/70eacb41ed7f5396709cc4acd9308d7d/image-35.jpg)
class Swap. Test{ public static void main(String args[]) { Swap a 1 = new Swap(); // creating object Swap a 2 = new Swap(); a 1. x = 2; // assigning value to data field a 2. x = 5; System. out. println("Before swap"); • Output: System. out. println("a 1. x="+a 1. x+" Before swap a 2. x="+a 2. x); Swap. swap(a 1. x, a 2. x); a 1. x=2 a 2. x=5 After swap System. out. println("After swap"); a 1. x=5 a 2. x=2 System. out. println("a 1. x="+a 1. x+" a 2. x="+a 2. x); } }
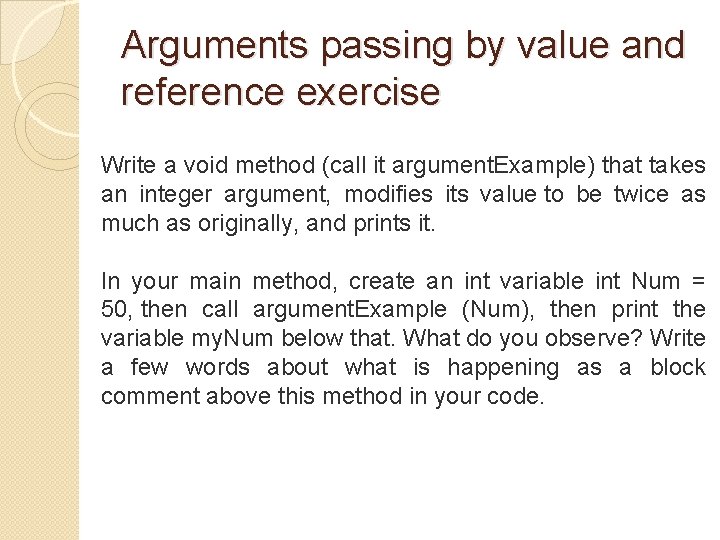
Arguments passing by value and reference exercise Write a void method (call it argument. Example) that takes an integer argument, modifies its value to be twice as much as originally, and prints it. In your main method, create an int variable int Num = 50, then call argument. Example (Num), then print the variable my. Num below that. What do you observe? Write a few words about what is happening as a block comment above this method in your code.
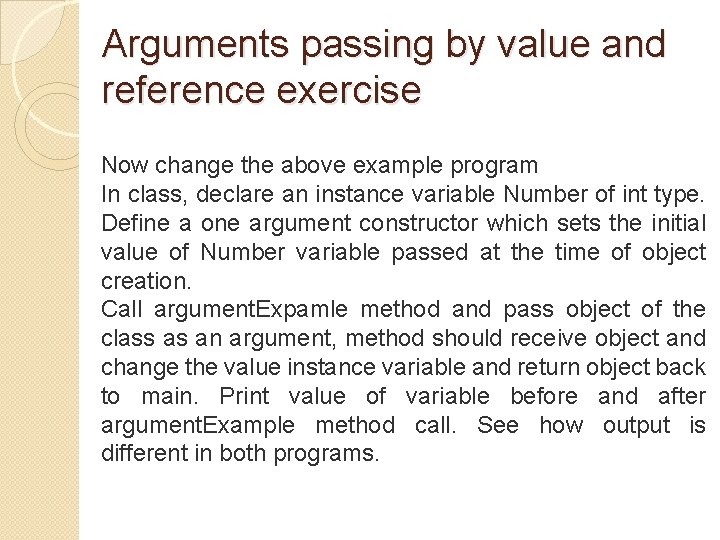
Arguments passing by value and reference exercise Now change the above example program In class, declare an instance variable Number of int type. Define a one argument constructor which sets the initial value of Number variable passed at the time of object creation. Call argument. Expamle method and pass object of the class as an argument, method should receive object and change the value instance variable and return object back to main. Print value of variable before and after argument. Example method call. See how output is different in both programs.
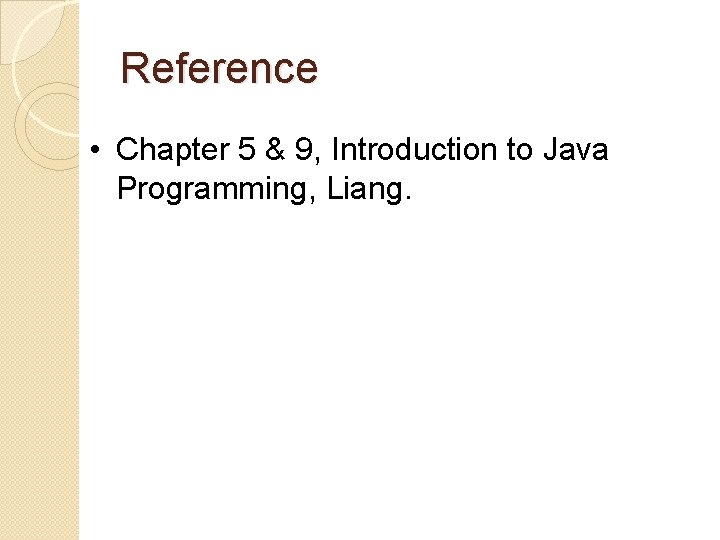
Reference • Chapter 5 & 9, Introduction to Java Programming, Liang.
Insidan region jh
Methods are commonly used to:
Hey hey bye bye
Sectional drawing examples
Constrains two faces, edges, points, or axes together.
Computer graphics models are now commonly used for making
Hair roller placement
Booster hose is designed to be carried:
Classification of clutch
A depressant drug drivers ed
What is the purpose of the
3 components of narrative paragraph
Most commonly used system
Before using a pneumatically powered
Don't ask why why why
Section 17-3 practice commonly abused drugs
Commonly damaged areas of a vehicle during hoisting
Signing naturally 5:9 answers
Sodium hydroxide relaxers
Altered cognition in older adults is commonly attributed to
History is the witness that ______ passing of time.
Virtual memory is commonly implemented by
Multinational financial management meaning
Pacm1101
Types of optical storage
Circle graph called
Agaricus commonly known as
What is the collection of web page
Sodium hydroxide relaxers are commonly called
A commonly cited hazard for stairways and or ladders is
Commonly confused words paragraph
Effected vs affected
Abused children commonly exhibit
Commonly confused words jeopardy
What are poriferans
Commonly misspelled homophones
Financial difficulties are commonly caused by overspending
Commonly misused homonyms
Karakteristik write through didalam write policy