Lecture 10 Programming Exceptionally CS 201 j Engineering
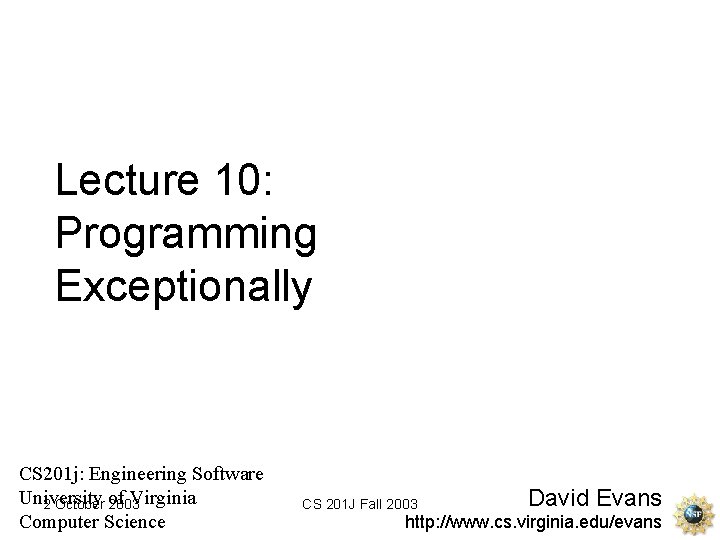
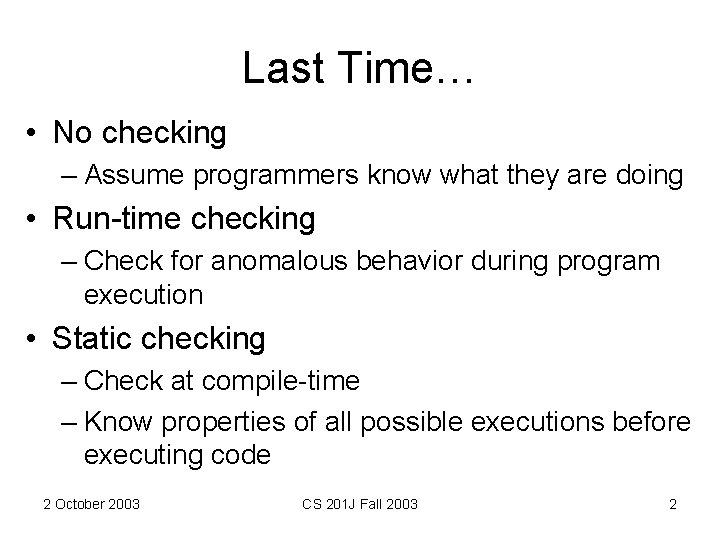
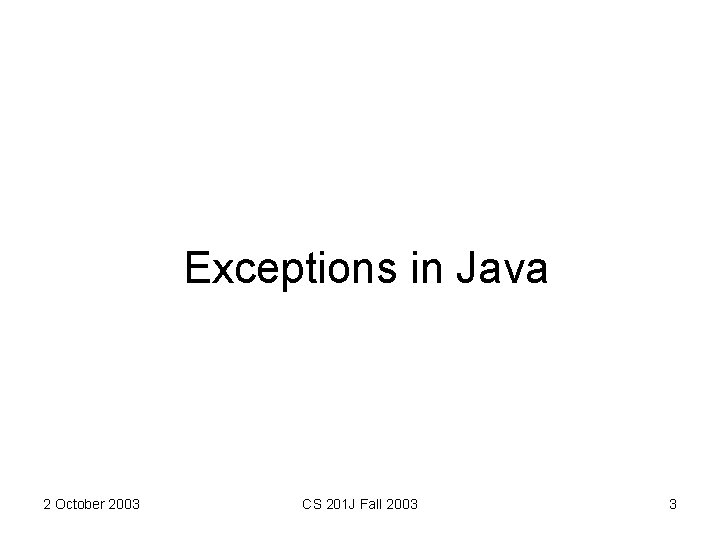
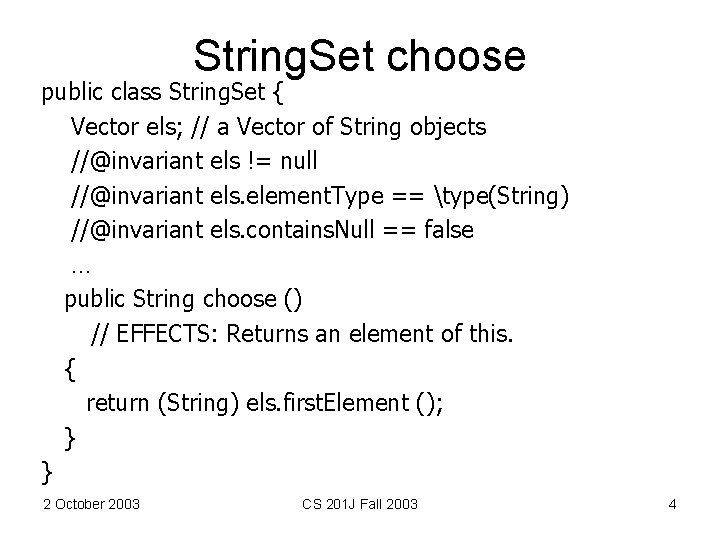
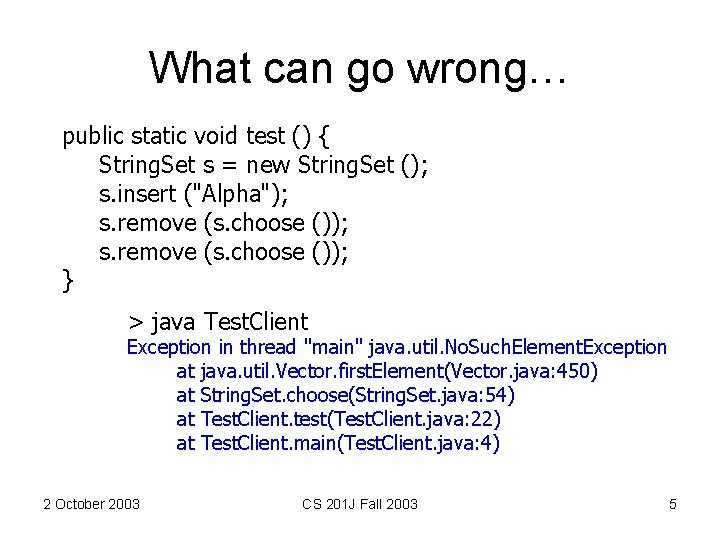
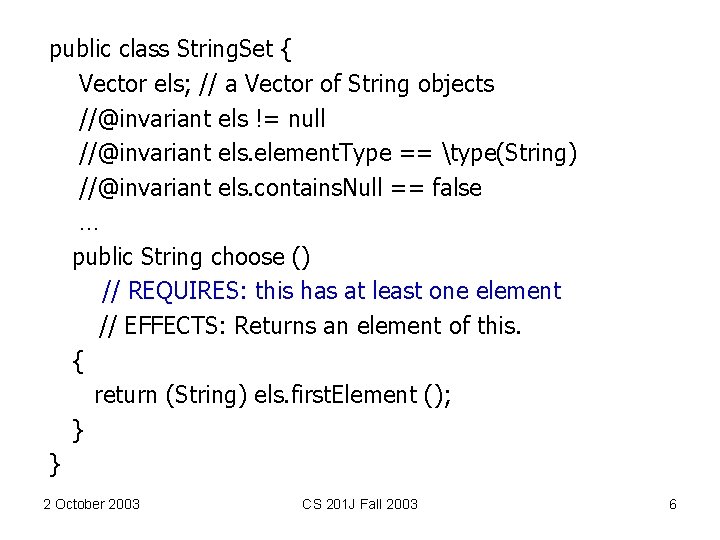
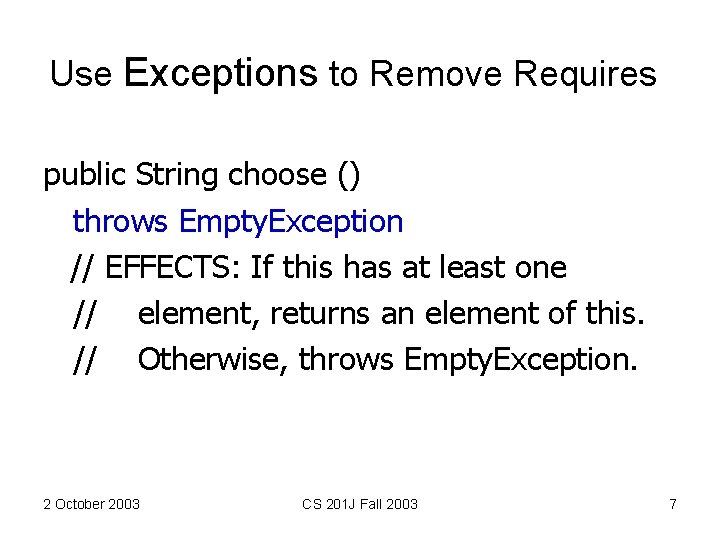
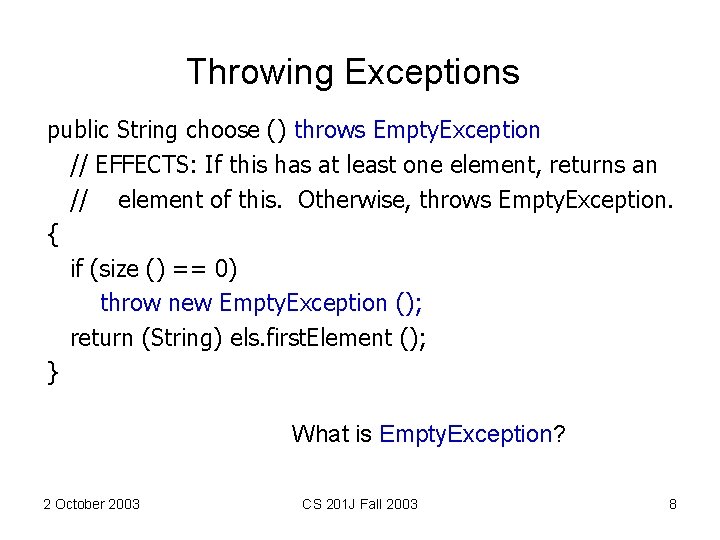
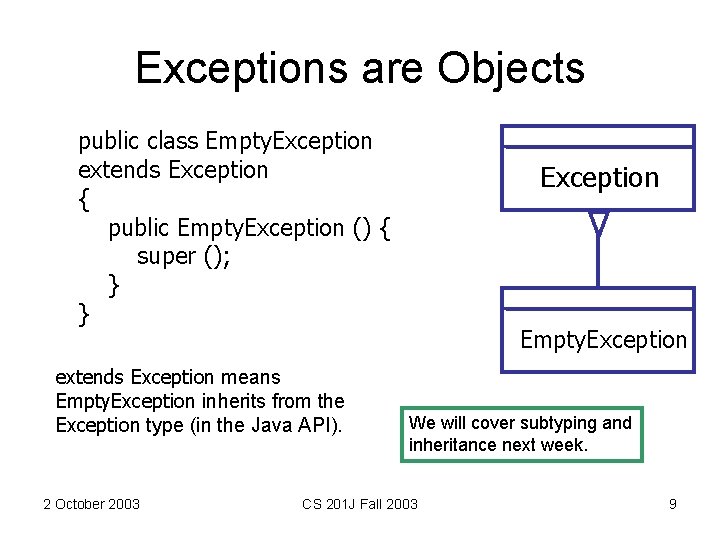
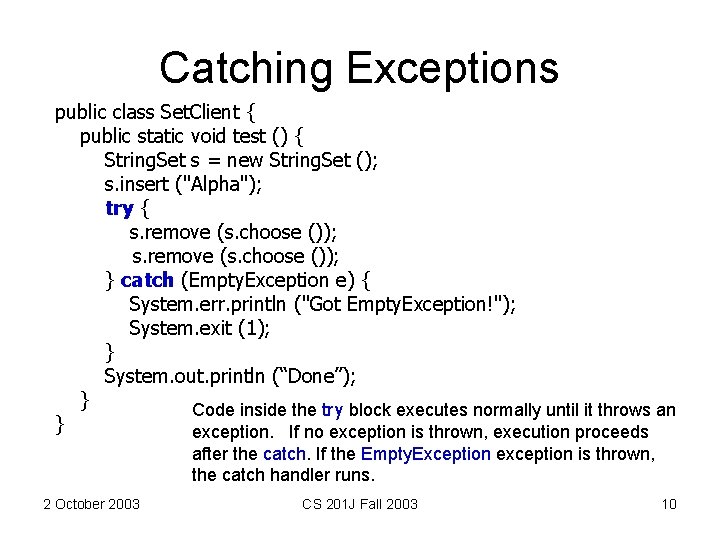
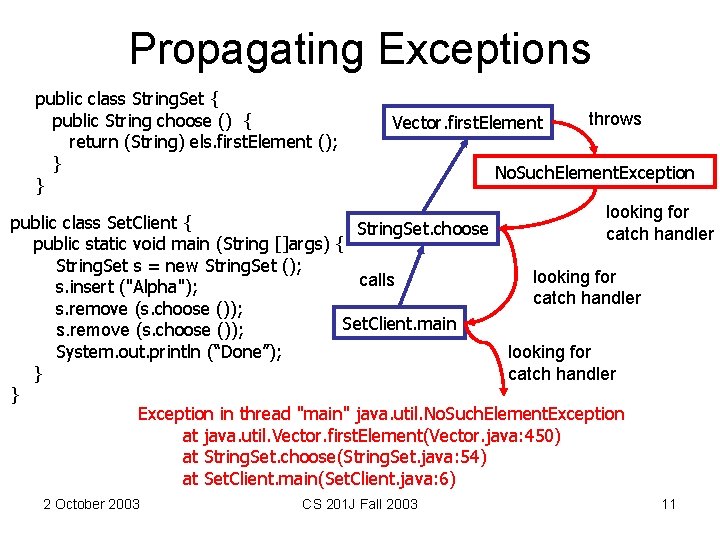
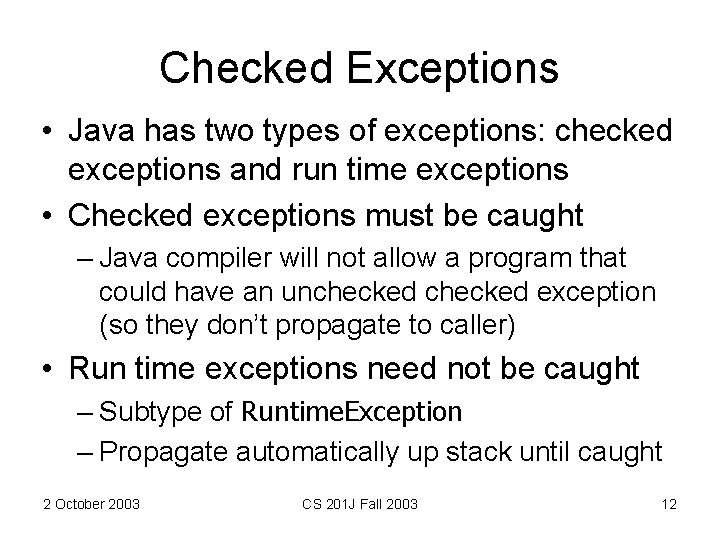
![Catching Exceptions public class Set. Client { public static void main (String args[]) { Catching Exceptions public class Set. Client { public static void main (String args[]) {](https://slidetodoc.com/presentation_image_h2/f476821706a29cf8a5d34e049deaf01e/image-13.jpg)
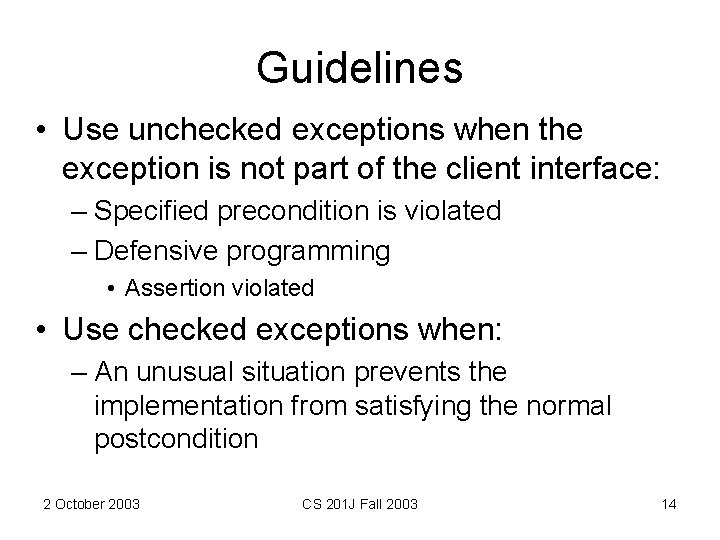
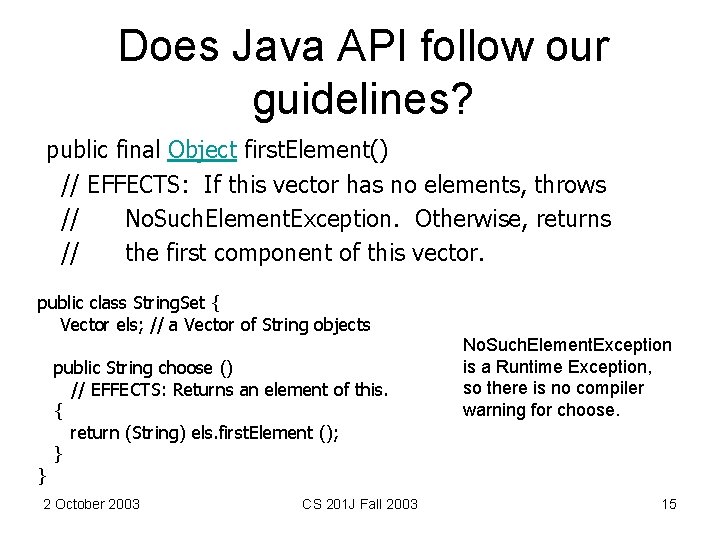
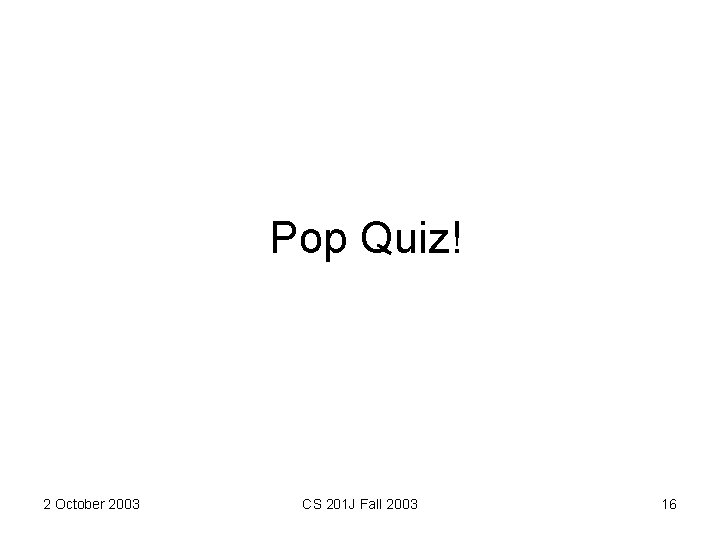
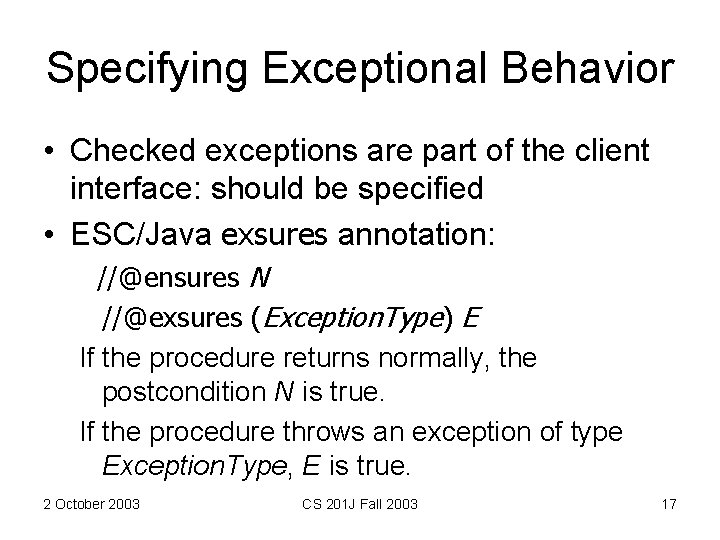
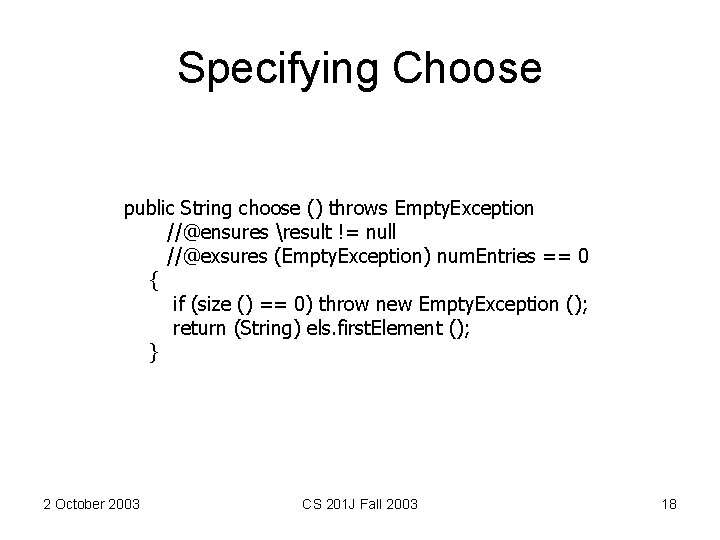
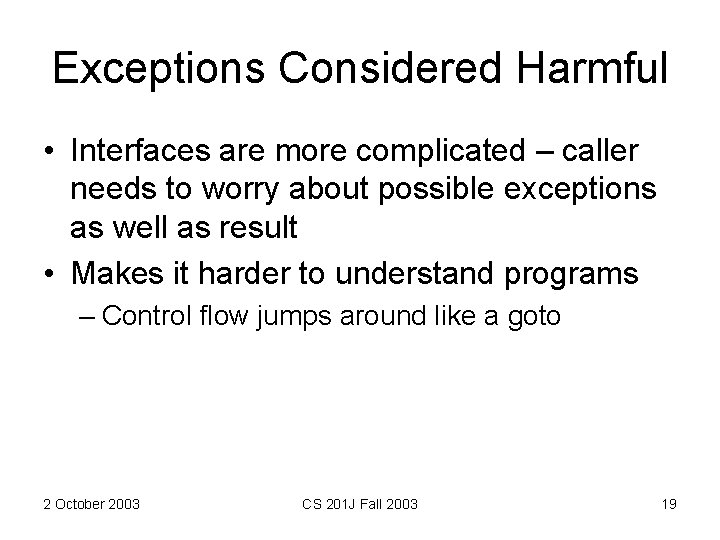
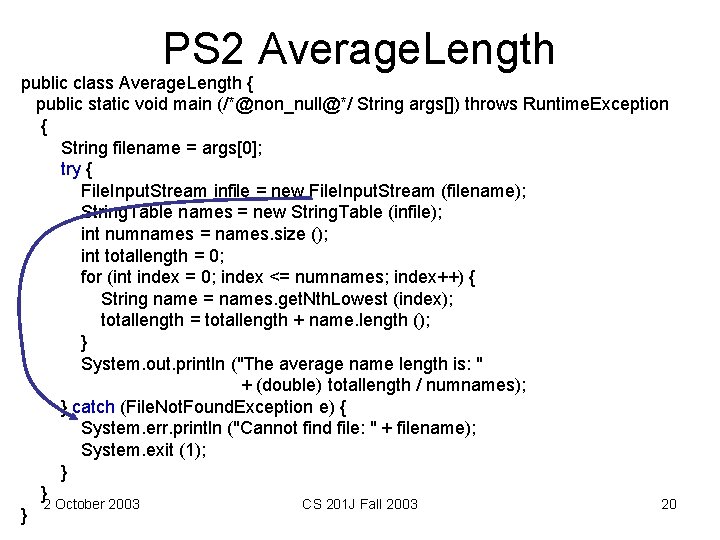
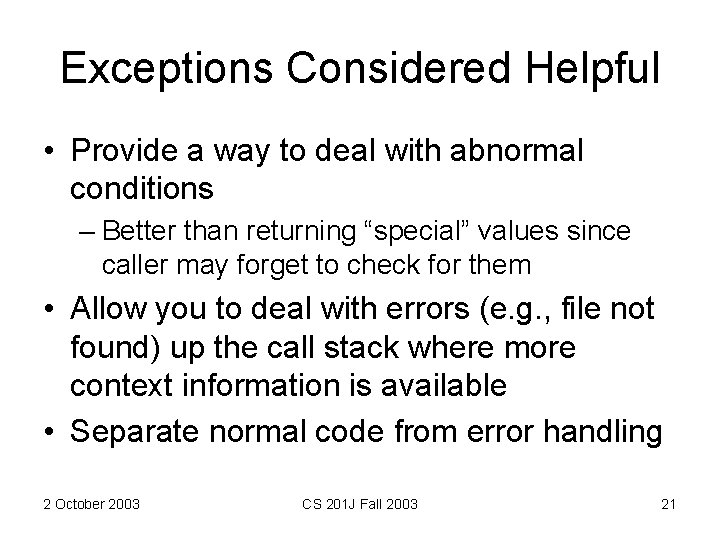
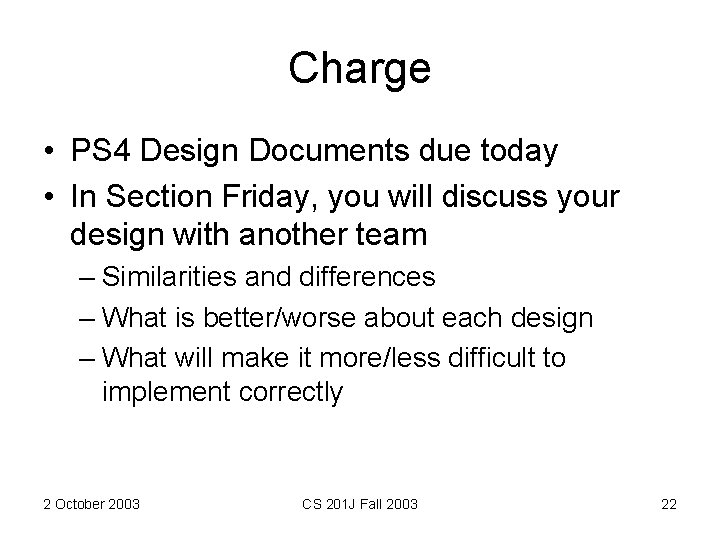
- Slides: 22
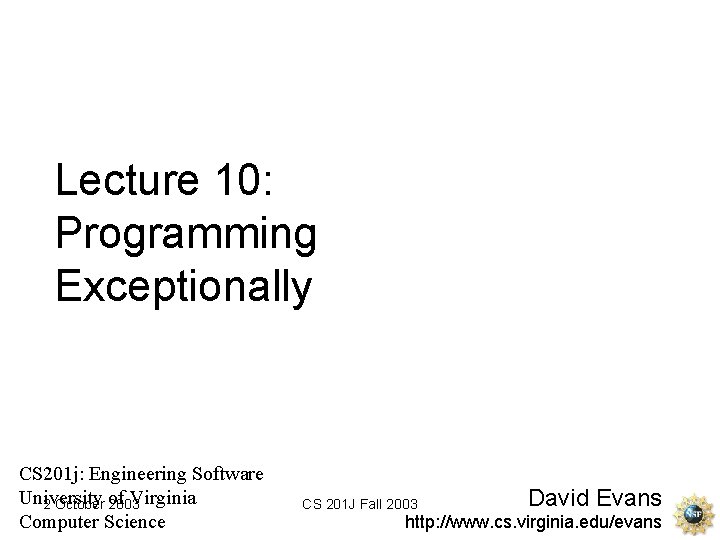
Lecture 10: Programming Exceptionally CS 201 j: Engineering Software University Virginia 2 October of 2003 Computer Science CS 201 J Fall 2003 David Evans http: //www. cs. virginia. edu/evans
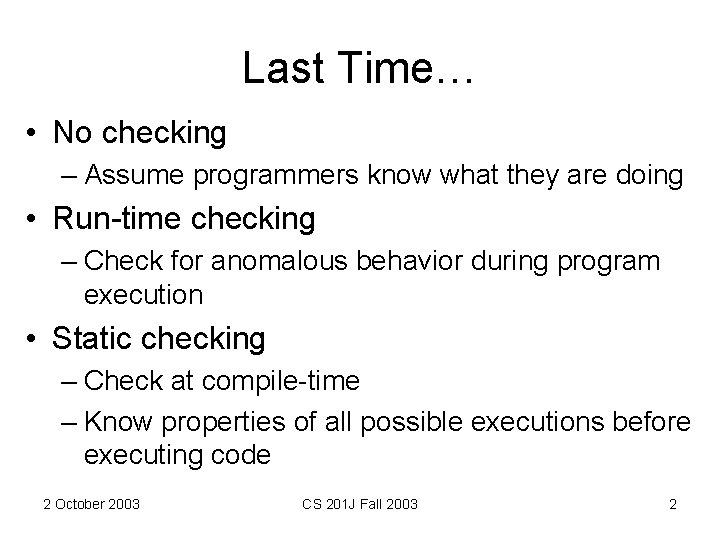
Last Time… • No checking – Assume programmers know what they are doing • Run-time checking – Check for anomalous behavior during program execution • Static checking – Check at compile-time – Know properties of all possible executions before executing code 2 October 2003 CS 201 J Fall 2003 2
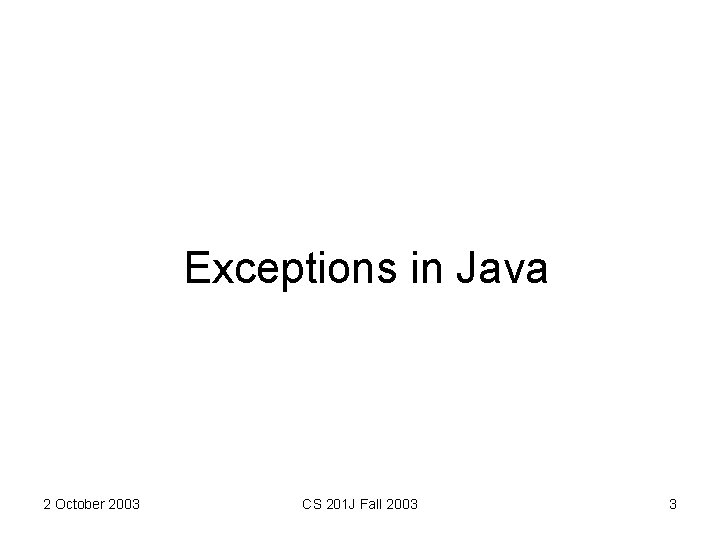
Exceptions in Java 2 October 2003 CS 201 J Fall 2003 3
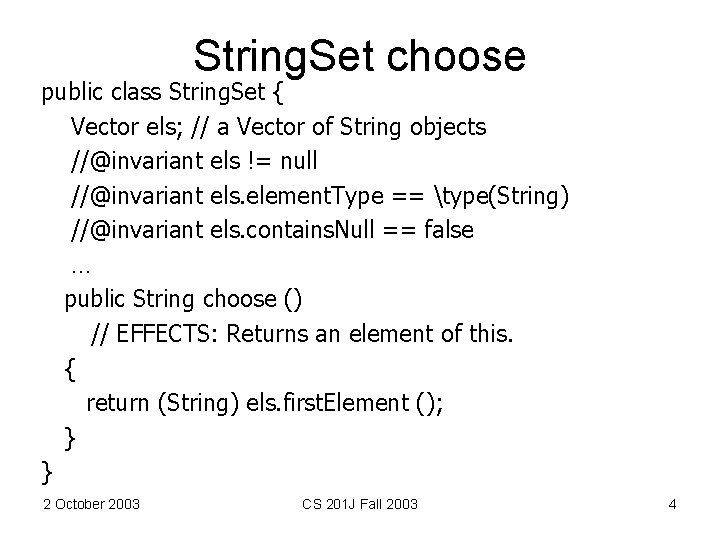
String. Set choose public class String. Set { Vector els; // a Vector of String objects //@invariant els != null //@invariant els. element. Type == type(String) //@invariant els. contains. Null == false … public String choose () // EFFECTS: Returns an element of this. { return (String) els. first. Element (); } } 2 October 2003 CS 201 J Fall 2003 4
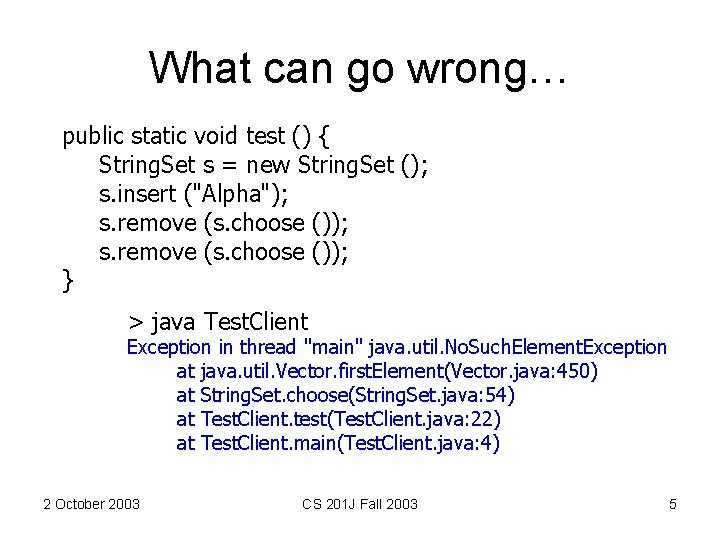
What can go wrong… public static void test () { String. Set s = new String. Set (); s. insert ("Alpha"); s. remove (s. choose ()); } > java Test. Client Exception in thread "main" java. util. No. Such. Element. Exception at java. util. Vector. first. Element(Vector. java: 450) at String. Set. choose(String. Set. java: 54) at Test. Client. test(Test. Client. java: 22) at Test. Client. main(Test. Client. java: 4) 2 October 2003 CS 201 J Fall 2003 5
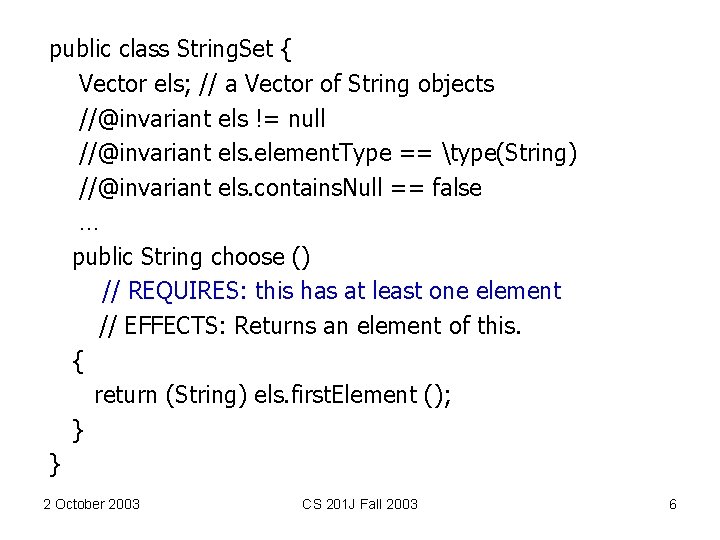
public class String. Set { Vector els; // a Vector of String objects //@invariant els != null //@invariant els. element. Type == type(String) //@invariant els. contains. Null == false … public String choose () // REQUIRES: this has at least one element // EFFECTS: Returns an element of this. { return (String) els. first. Element (); } } 2 October 2003 CS 201 J Fall 2003 6
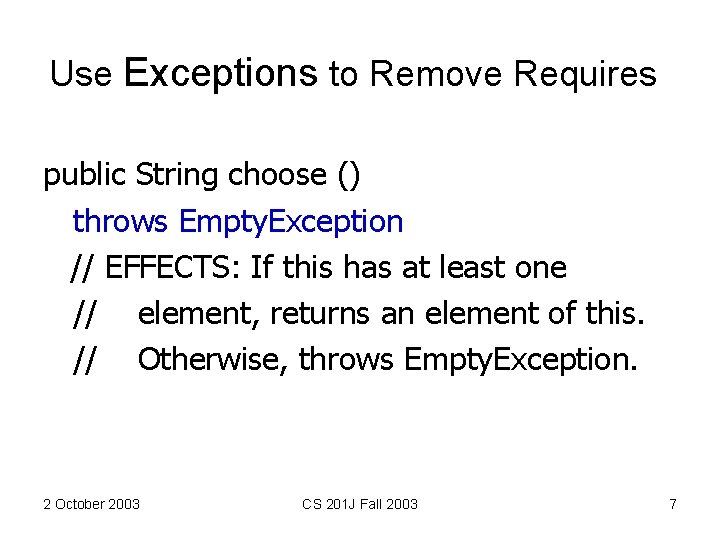
Use Exceptions to Remove Requires public String choose () throws Empty. Exception // EFFECTS: If this has at least one // element, returns an element of this. // Otherwise, throws Empty. Exception. 2 October 2003 CS 201 J Fall 2003 7
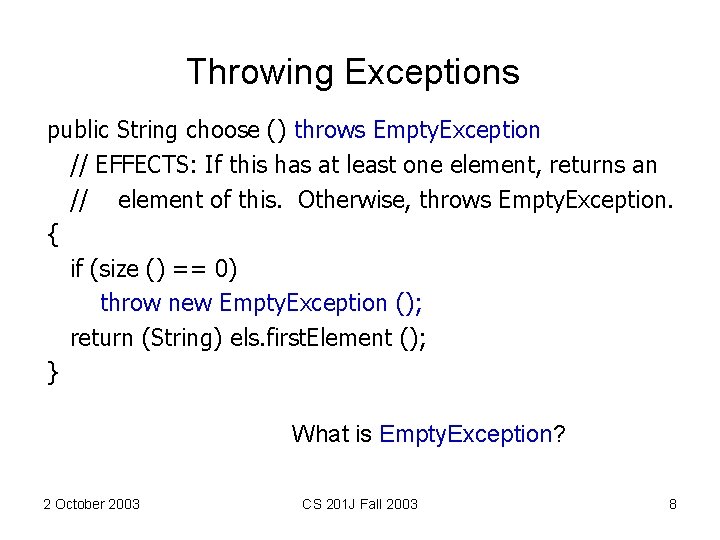
Throwing Exceptions public String choose () throws Empty. Exception // EFFECTS: If this has at least one element, returns an // element of this. Otherwise, throws Empty. Exception. { if (size () == 0) throw new Empty. Exception (); return (String) els. first. Element (); } What is Empty. Exception? 2 October 2003 CS 201 J Fall 2003 8
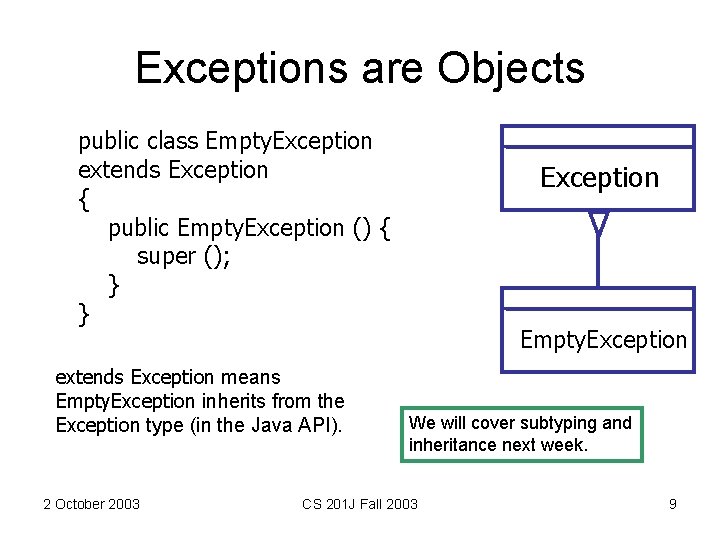
Exceptions are Objects public class Empty. Exception extends Exception { public Empty. Exception () { super (); } } extends Exception means Empty. Exception inherits from the Exception type (in the Java API). 2 October 2003 Exception Empty. Exception We will cover subtyping and inheritance next week. CS 201 J Fall 2003 9
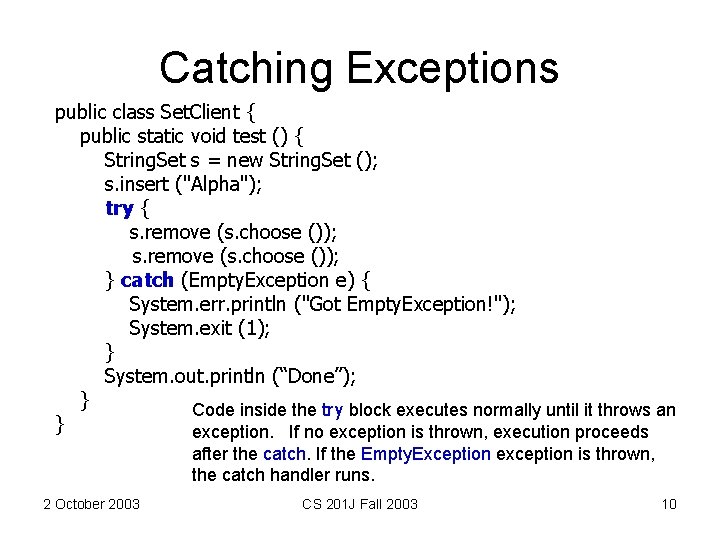
Catching Exceptions public class Set. Client { public static void test () { String. Set s = new String. Set (); s. insert ("Alpha"); try { s. remove (s. choose ()); } catch (Empty. Exception e) { System. err. println ("Got Empty. Exception!"); System. exit (1); } System. out. println (“Done”); } Code inside the try block executes normally until it throws an } exception. If no exception is thrown, execution proceeds after the catch. If the Empty. Exception exception is thrown, the catch handler runs. 2 October 2003 CS 201 J Fall 2003 10
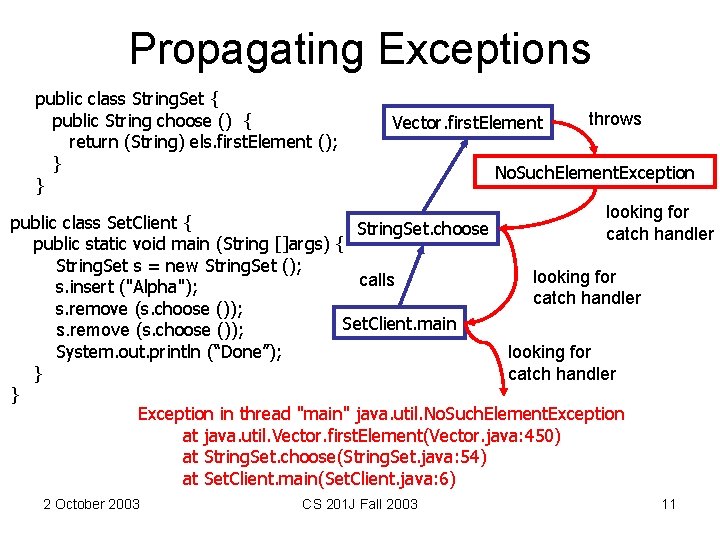
Propagating Exceptions public class String. Set { public String choose () { return (String) els. first. Element (); } } Vector. first. Element throws No. Such. Element. Exception looking for public class Set. Client { String. Set. choose catch handler public static void main (String []args) { String. Set s = new String. Set (); looking for calls s. insert ("Alpha"); catch handler s. remove (s. choose ()); Set. Client. main s. remove (s. choose ()); System. out. println (“Done”); looking for } catch handler } Exception in thread "main" java. util. No. Such. Element. Exception at java. util. Vector. first. Element(Vector. java: 450) at String. Set. choose(String. Set. java: 54) at Set. Client. main(Set. Client. java: 6) 2 October 2003 CS 201 J Fall 2003 11
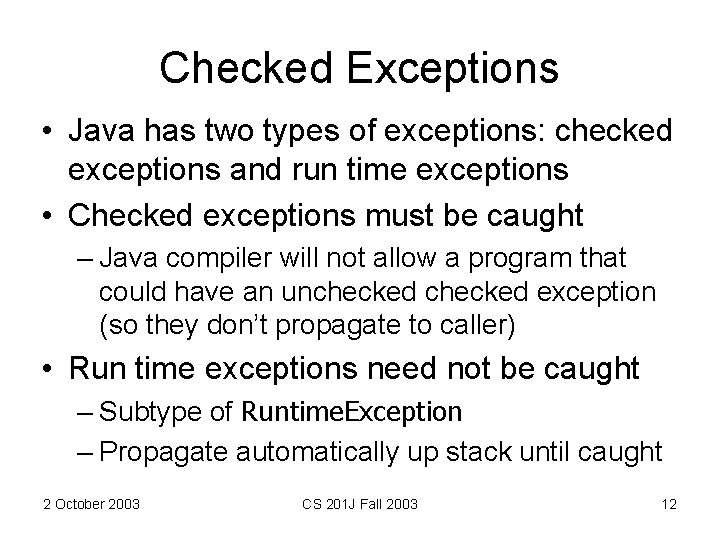
Checked Exceptions • Java has two types of exceptions: checked exceptions and run time exceptions • Checked exceptions must be caught – Java compiler will not allow a program that could have an unchecked exception (so they don’t propagate to caller) • Run time exceptions need not be caught – Subtype of Runtime. Exception – Propagate automatically up stack until caught 2 October 2003 CS 201 J Fall 2003 12
![Catching Exceptions public class Set Client public static void main String args Catching Exceptions public class Set. Client { public static void main (String args[]) {](https://slidetodoc.com/presentation_image_h2/f476821706a29cf8a5d34e049deaf01e/image-13.jpg)
Catching Exceptions public class Set. Client { public static void main (String args[]) { String. Set s = new String. Set (); s. insert ("Alpha"); System. out. println (s. choose ()); } } > javac Set. Client. java: 5: unreported exception Empty. Exception; must be caught or declared to be thrown 2 October 2003 CS 201 J Fall 2003 13
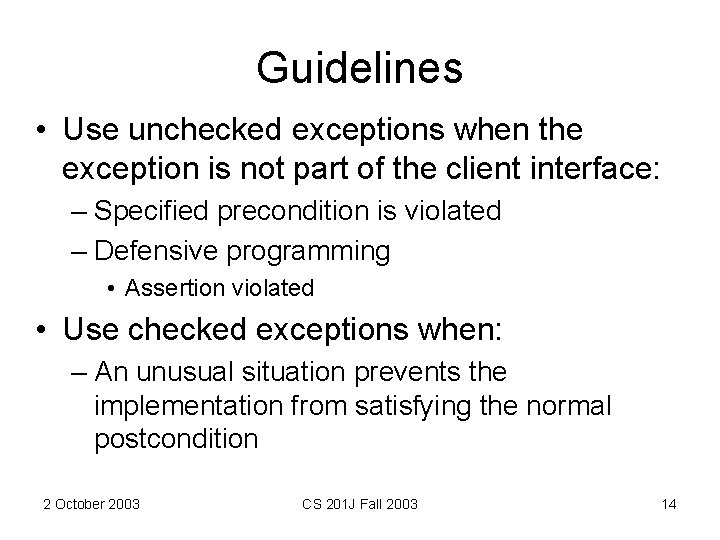
Guidelines • Use unchecked exceptions when the exception is not part of the client interface: – Specified precondition is violated – Defensive programming • Assertion violated • Use checked exceptions when: – An unusual situation prevents the implementation from satisfying the normal postcondition 2 October 2003 CS 201 J Fall 2003 14
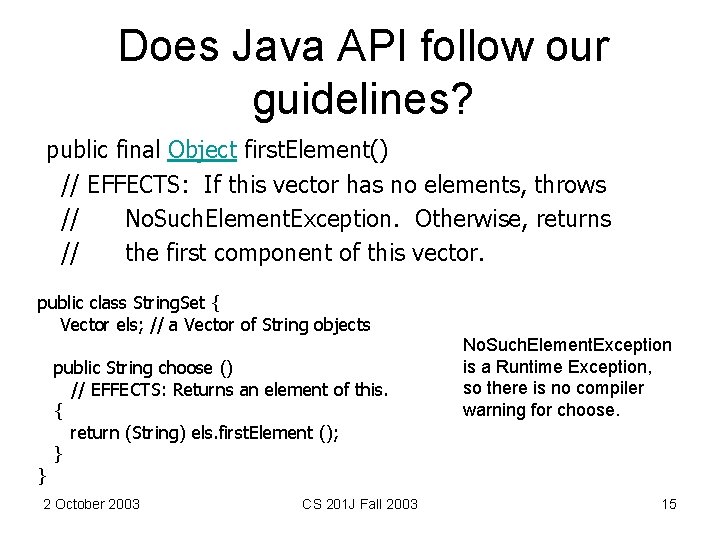
Does Java API follow our guidelines? public final Object first. Element() // EFFECTS: If this vector has no elements, throws // No. Such. Element. Exception. Otherwise, returns // the first component of this vector. public class String. Set { Vector els; // a Vector of String objects } public String choose () // EFFECTS: Returns an element of this. { return (String) els. first. Element (); } 2 October 2003 CS 201 J Fall 2003 No. Such. Element. Exception is a Runtime Exception, so there is no compiler warning for choose. 15
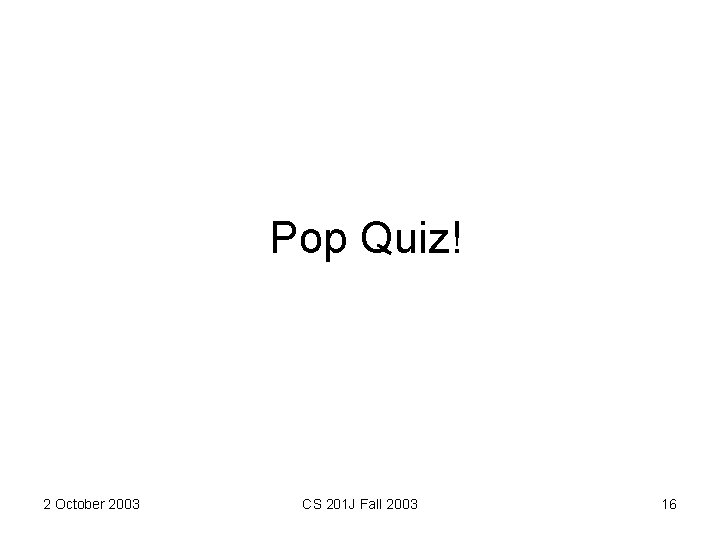
Pop Quiz! 2 October 2003 CS 201 J Fall 2003 16
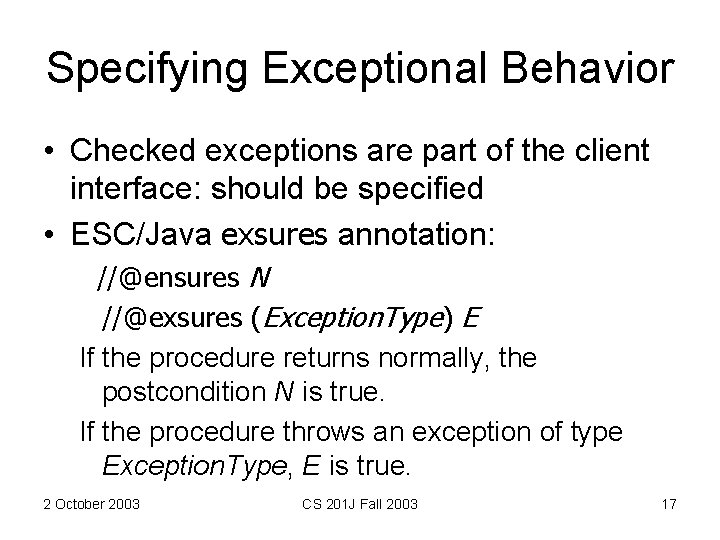
Specifying Exceptional Behavior • Checked exceptions are part of the client interface: should be specified • ESC/Java exsures annotation: //@ensures N //@exsures (Exception. Type) E If the procedure returns normally, the postcondition N is true. If the procedure throws an exception of type Exception. Type, E is true. 2 October 2003 CS 201 J Fall 2003 17
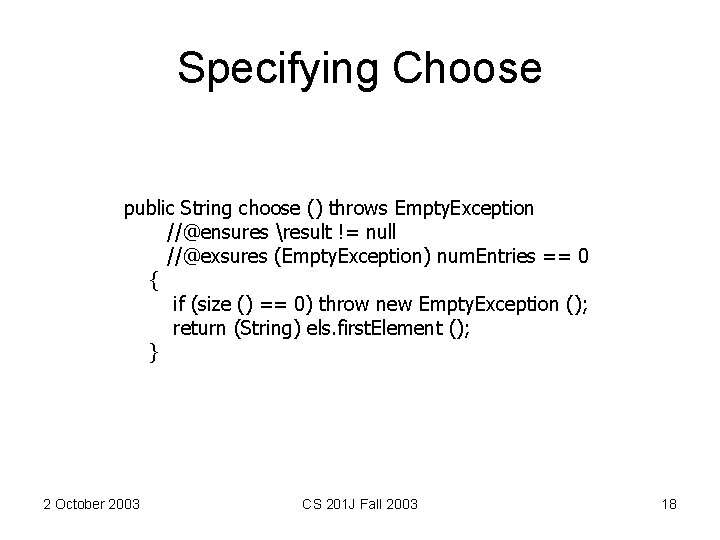
Specifying Choose public String choose () throws Empty. Exception //@ensures result != null //@exsures (Empty. Exception) num. Entries == 0 { if (size () == 0) throw new Empty. Exception (); return (String) els. first. Element (); } 2 October 2003 CS 201 J Fall 2003 18
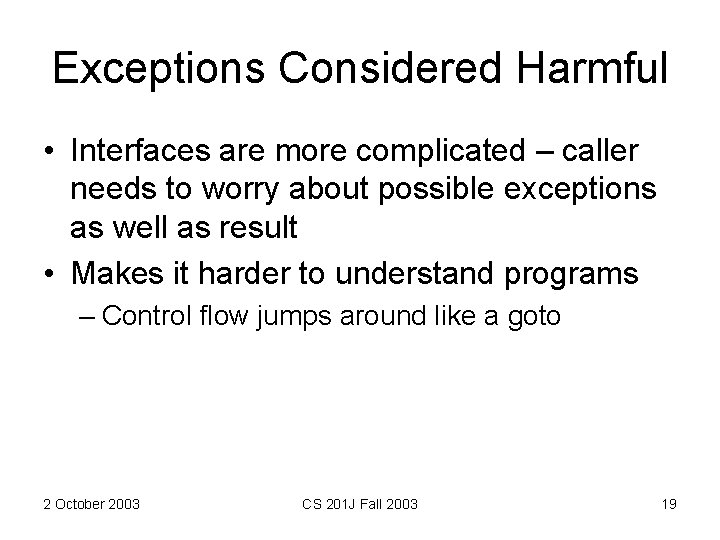
Exceptions Considered Harmful • Interfaces are more complicated – caller needs to worry about possible exceptions as well as result • Makes it harder to understand programs – Control flow jumps around like a goto 2 October 2003 CS 201 J Fall 2003 19
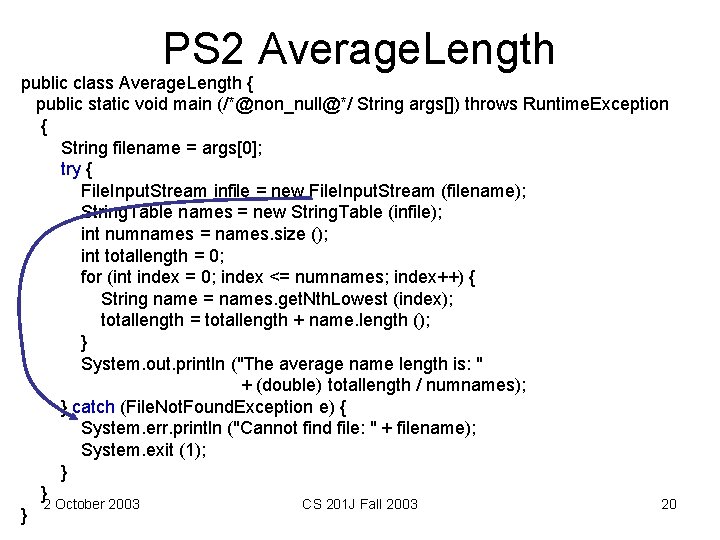
PS 2 Average. Length public class Average. Length { public static void main (/*@non_null@*/ String args[]) throws Runtime. Exception { String filename = args[0]; try { File. Input. Stream infile = new File. Input. Stream (filename); String. Table names = new String. Table (infile); int numnames = names. size (); int totallength = 0; for (int index = 0; index <= numnames; index++) { String name = names. get. Nth. Lowest (index); totallength = totallength + name. length (); } System. out. println ("The average name length is: " + (double) totallength / numnames); } catch (File. Not. Found. Exception e) { System. err. println ("Cannot find file: " + filename); System. exit (1); } } 2 October 2003 CS 201 J Fall 2003 20 }
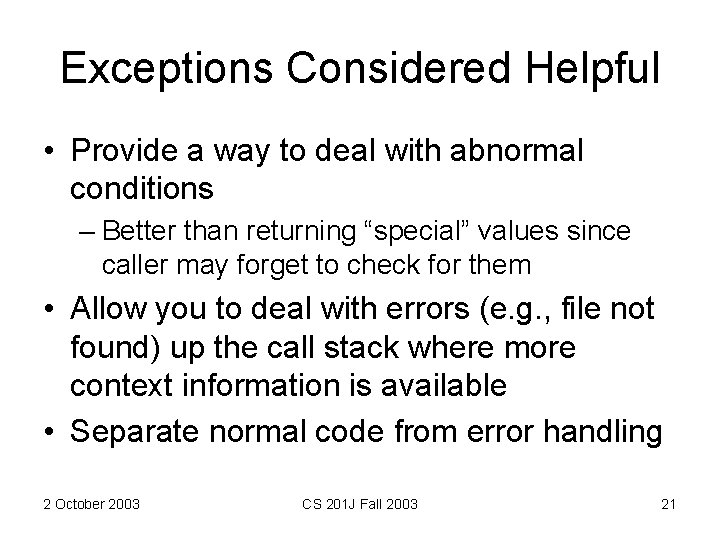
Exceptions Considered Helpful • Provide a way to deal with abnormal conditions – Better than returning “special” values since caller may forget to check for them • Allow you to deal with errors (e. g. , file not found) up the call stack where more context information is available • Separate normal code from error handling 2 October 2003 CS 201 J Fall 2003 21
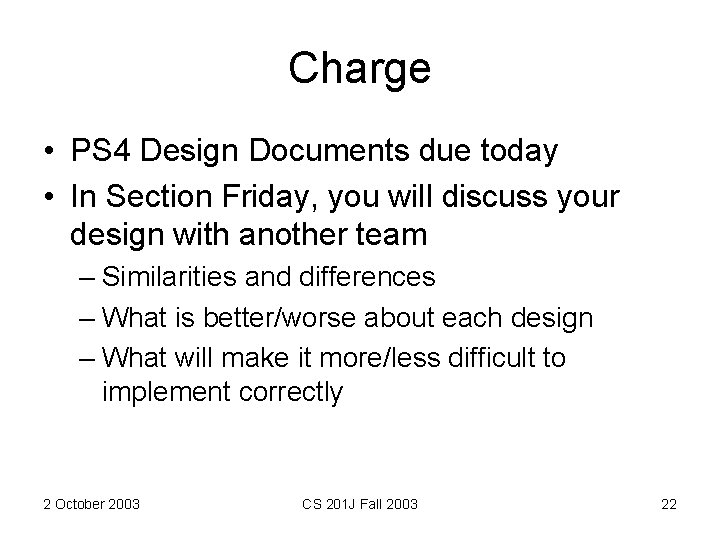
Charge • PS 4 Design Documents due today • In Section Friday, you will discuss your design with another team – Similarities and differences – What is better/worse about each design – What will make it more/less difficult to implement correctly 2 October 2003 CS 201 J Fall 2003 22
What makes london olympic 2012 exceptionally sensational
01:640:244 lecture notes - lecture 15: plat, idah, farad
Sustainable engineering ktu syllabus
C programming lecture
Money-time relationship and equivalence
Requirement analysis in software engineering notes
Foundation engineering lecture notes
Descriptive ethics
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
What is system programing
Integer programming vs linear programming
Definisi integer
Extreme programming in software engineering
Mechanical engineering programming
Nf p 40-201
Mcb 201
Math 201 bryant and stratton
Seramikler hangi bağ çeşidine sahiptir
Toon company
Fst 201
Complex numbers in electrical circuits
Engr 201