Java programming Nested classes Nested classes A class
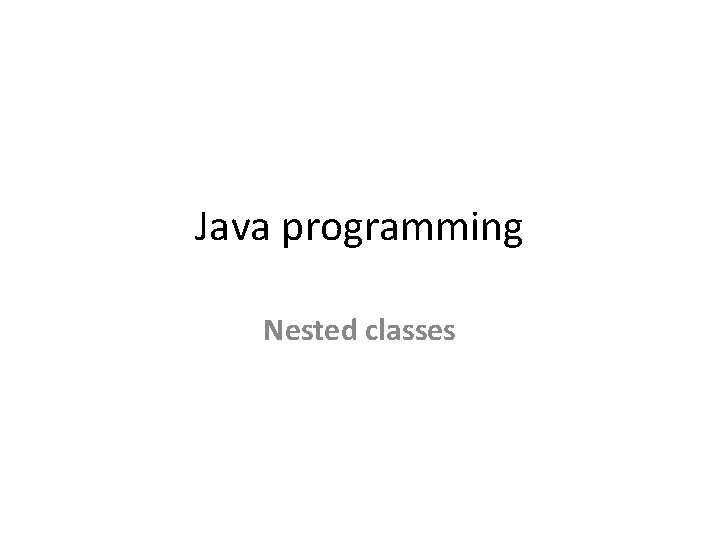
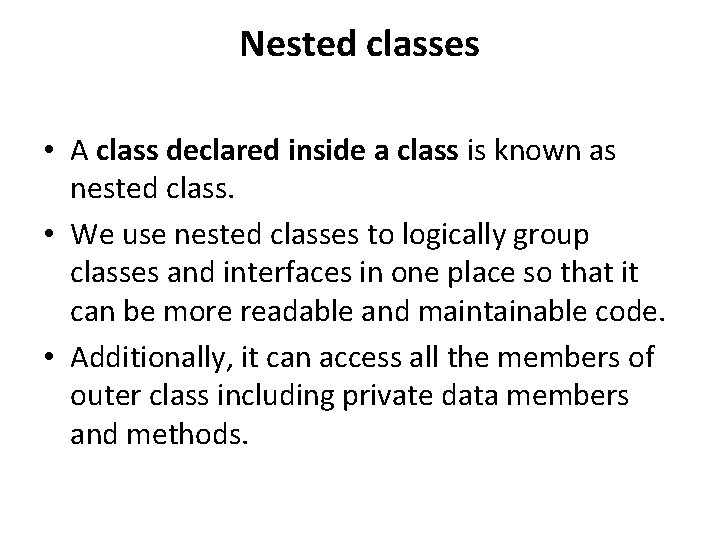
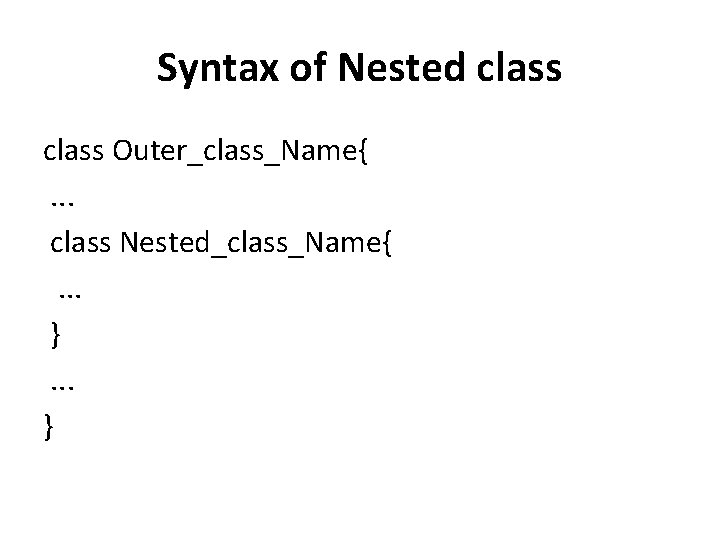
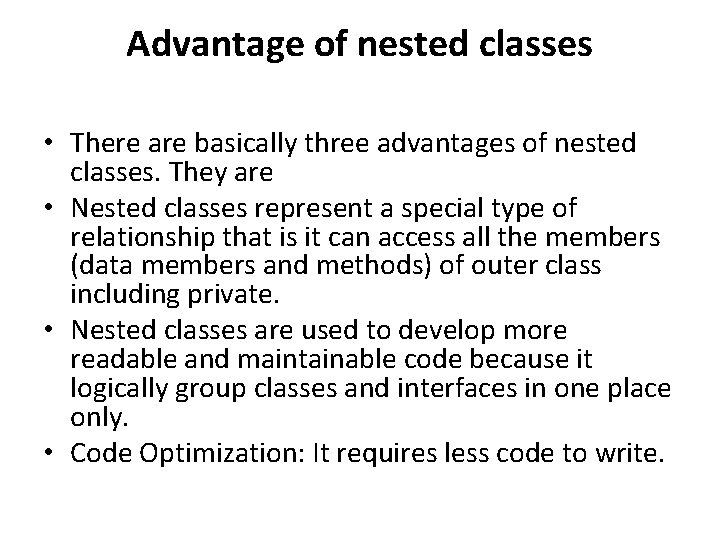
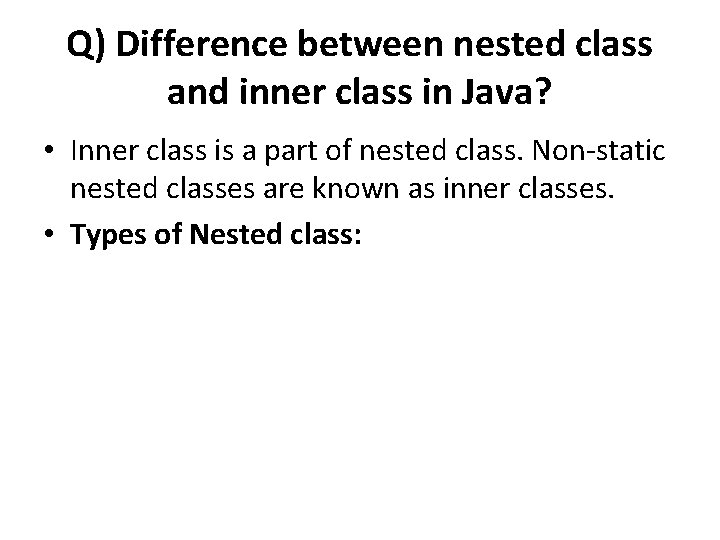
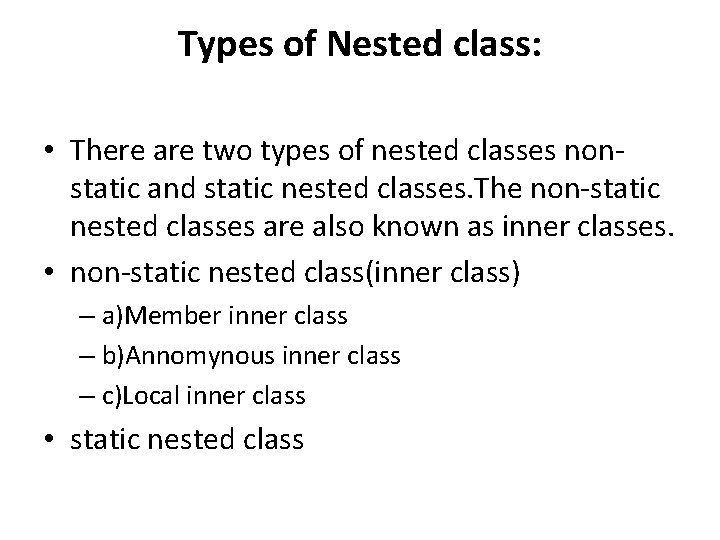
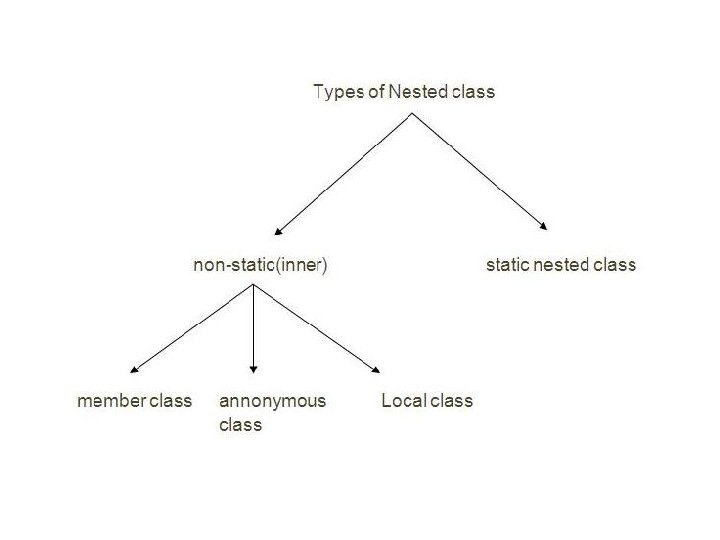
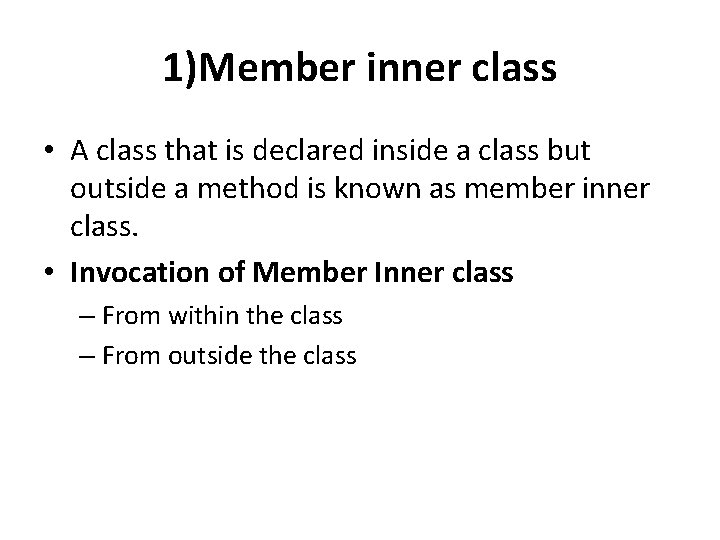
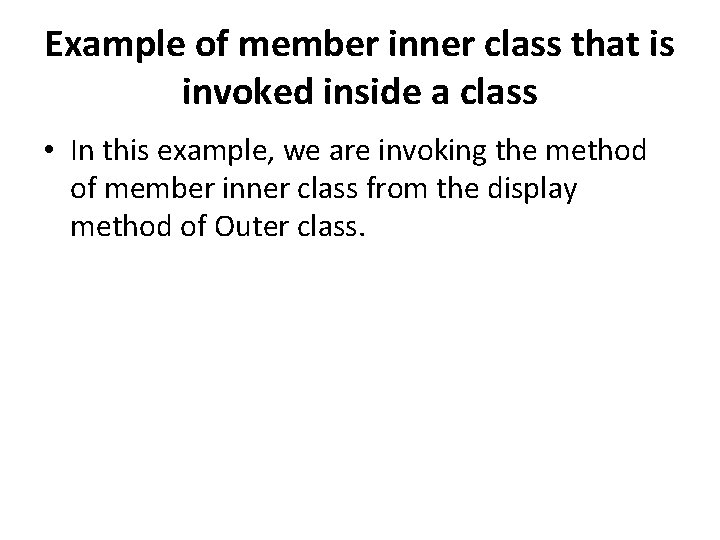
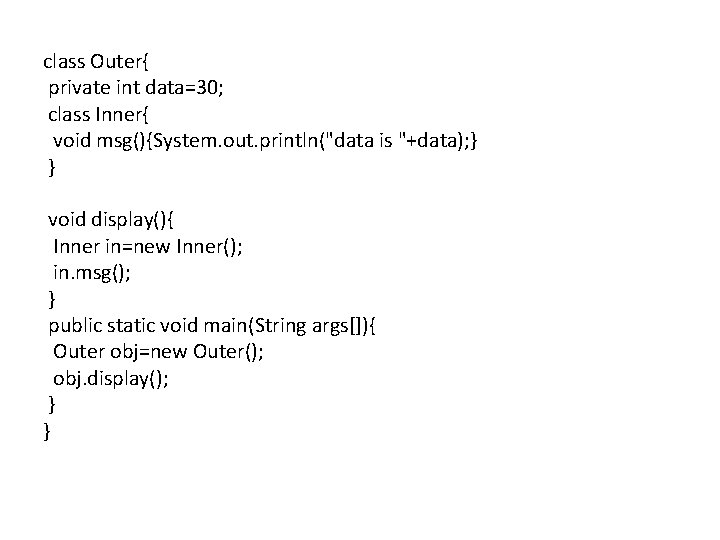
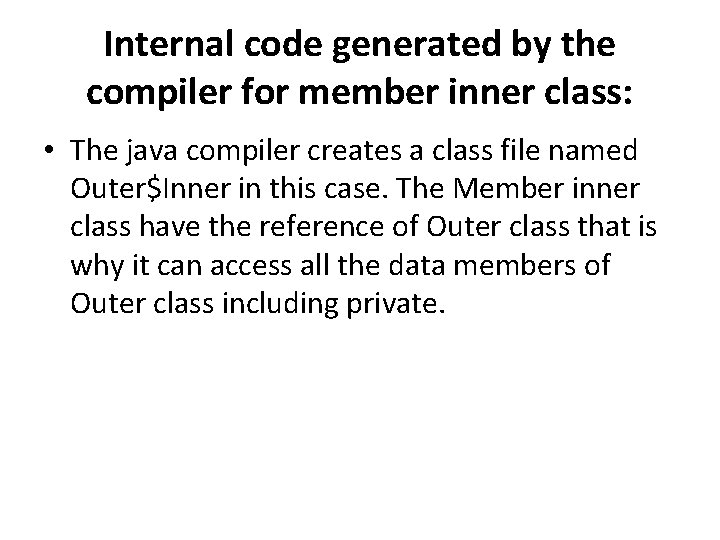
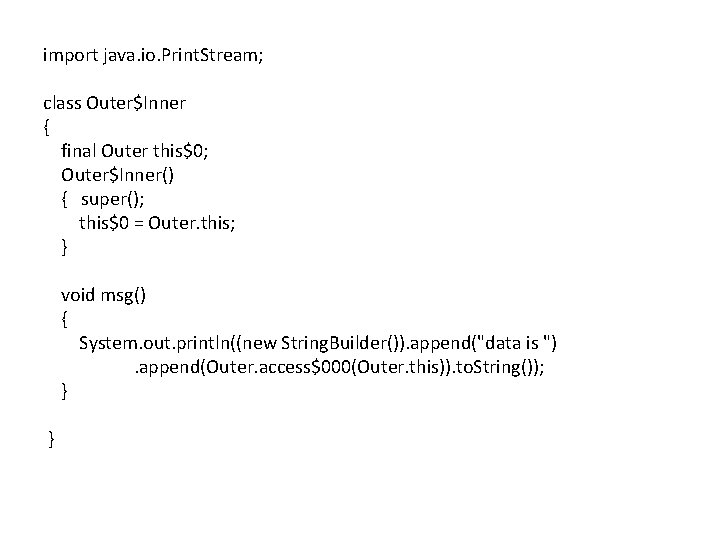
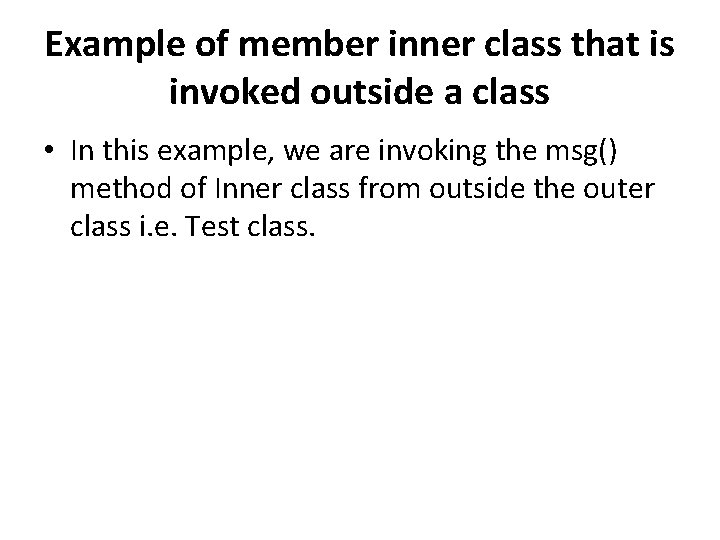
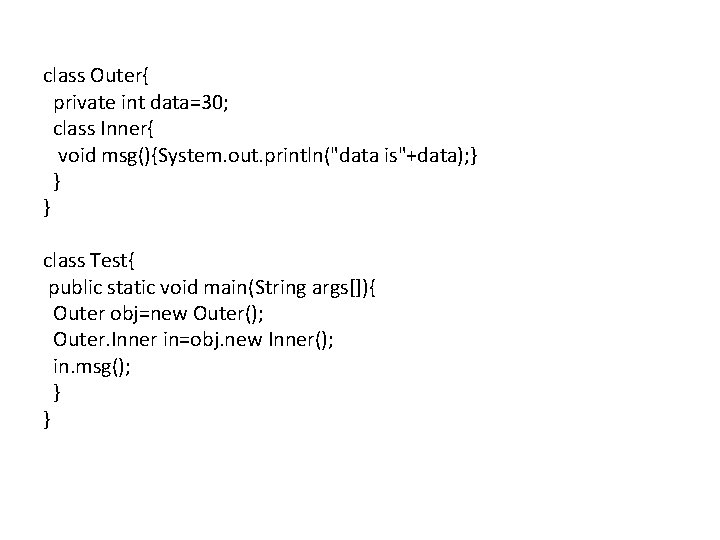
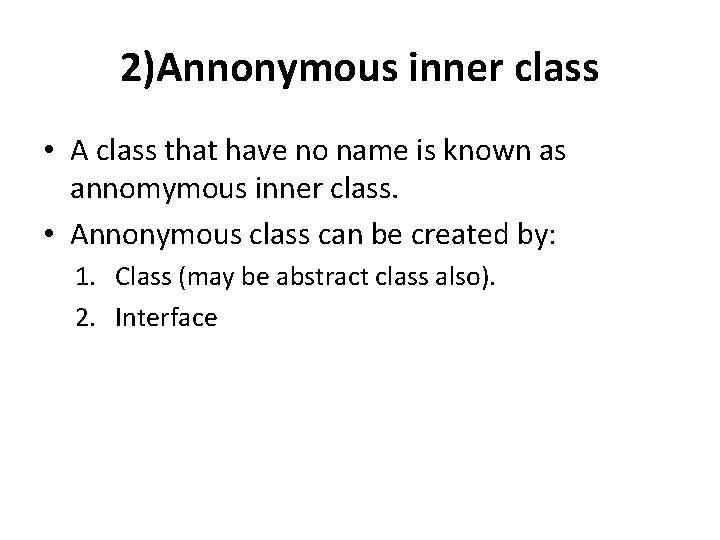
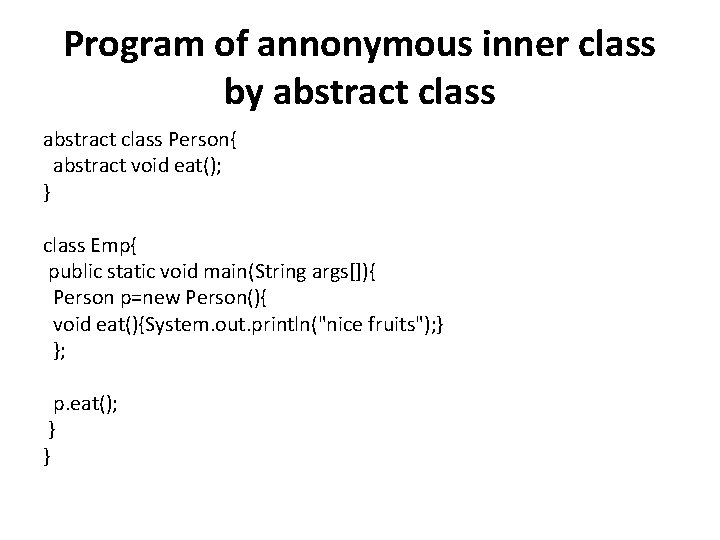
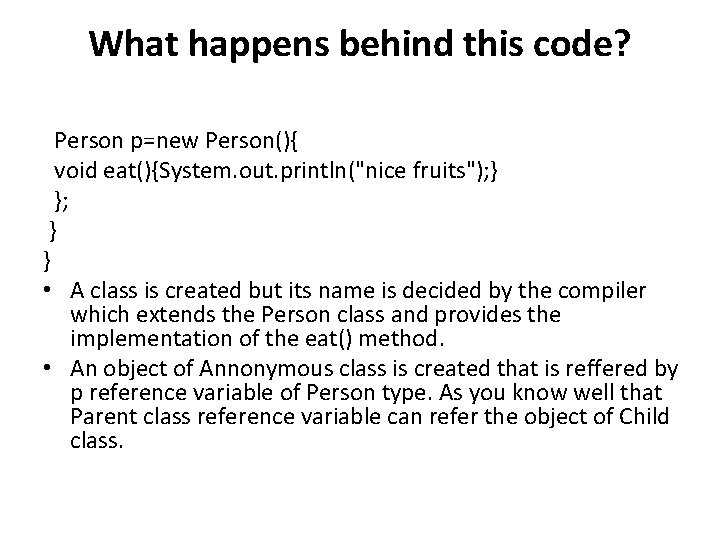
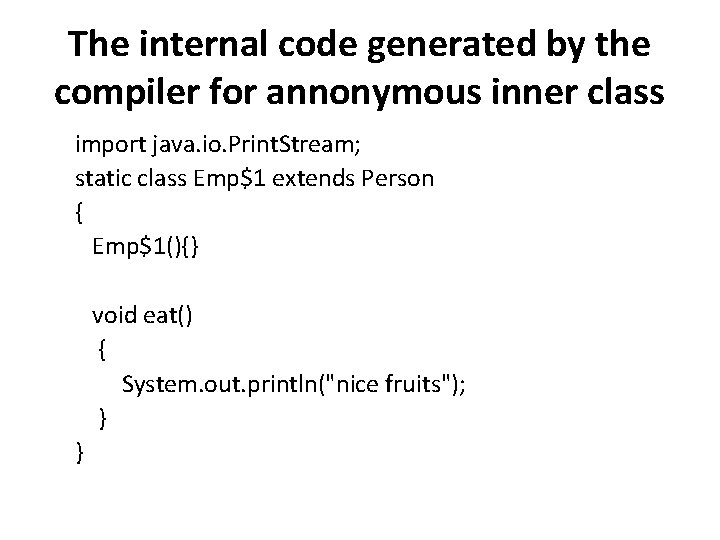
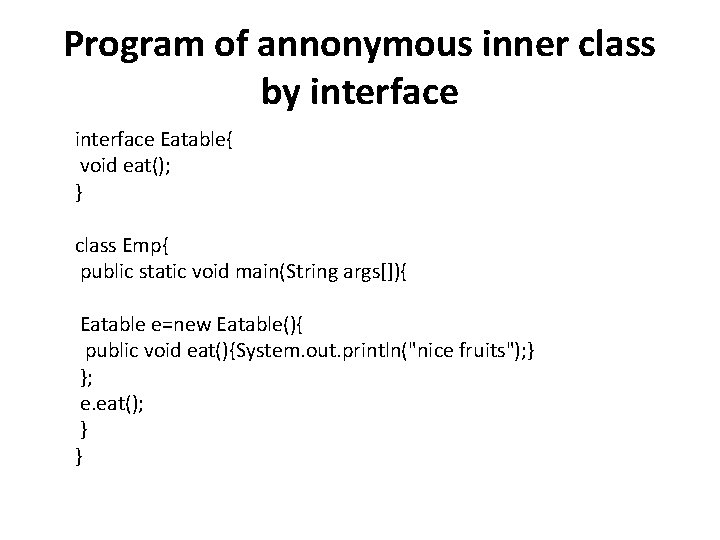
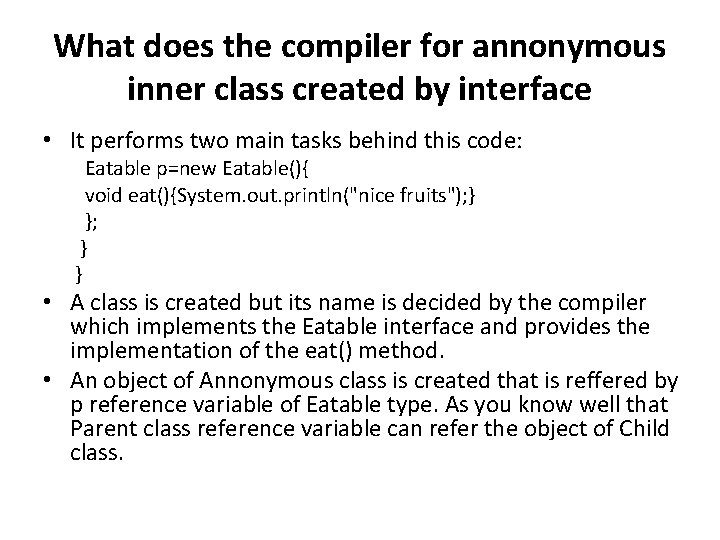
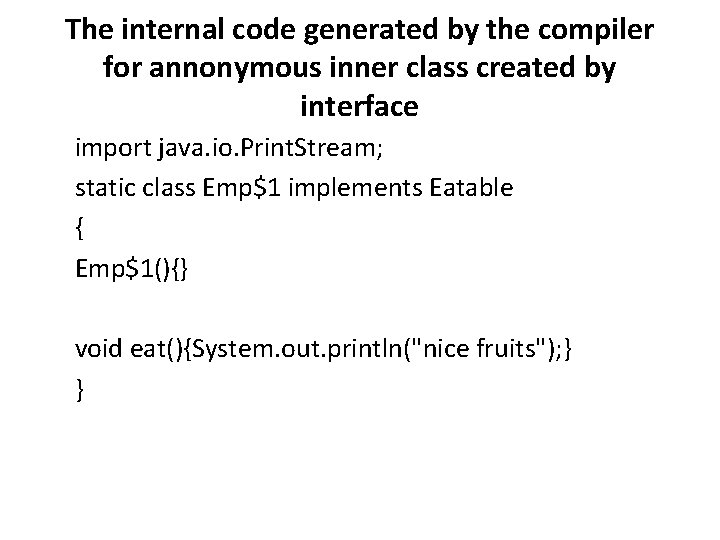
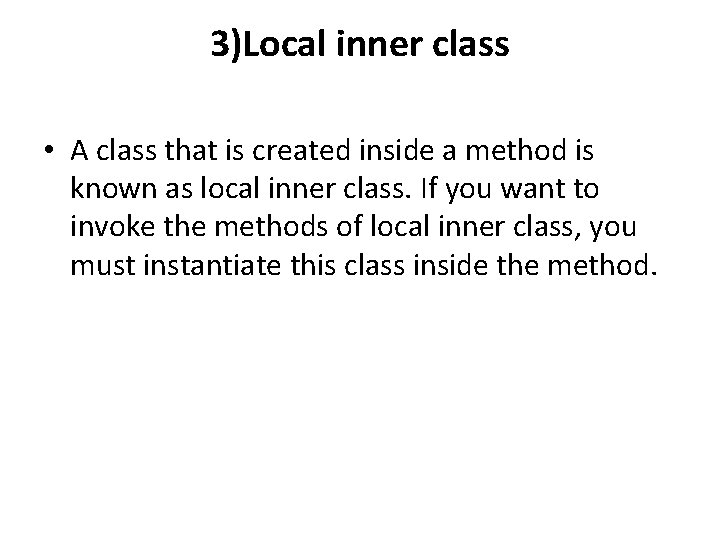
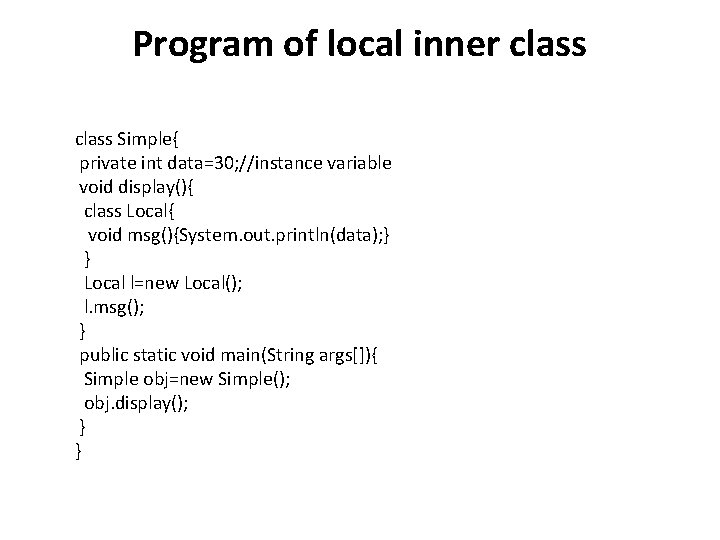
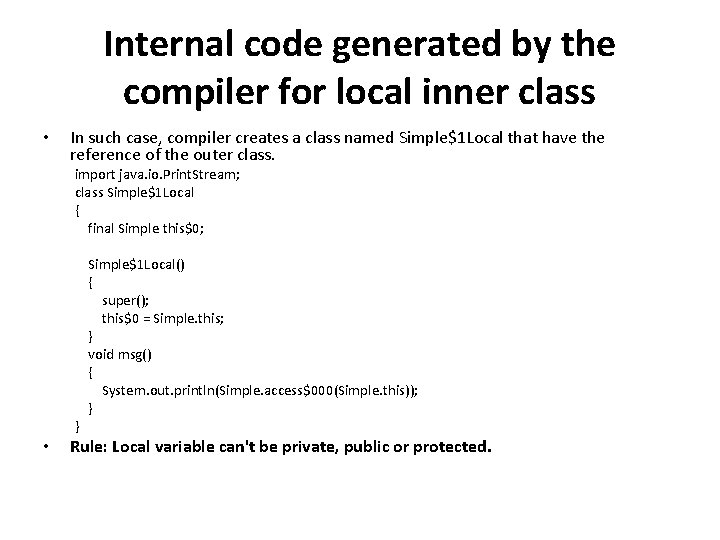
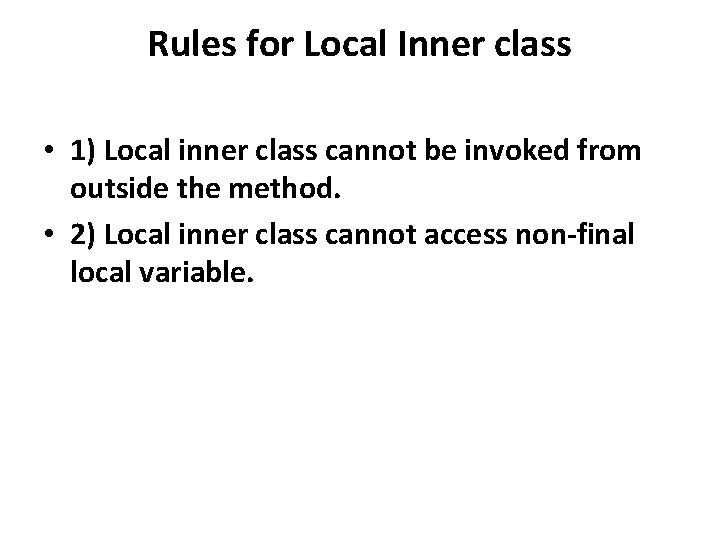
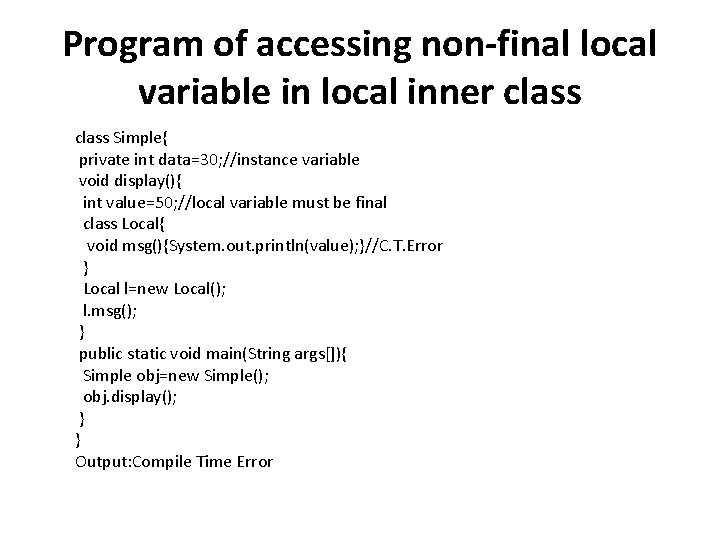
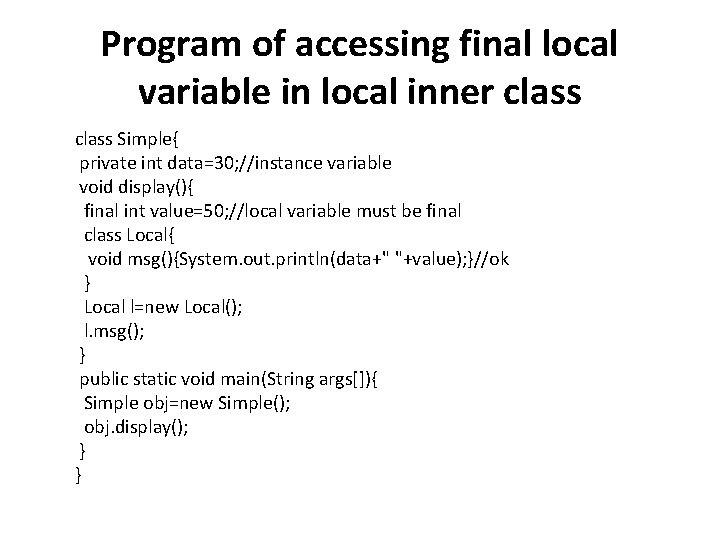
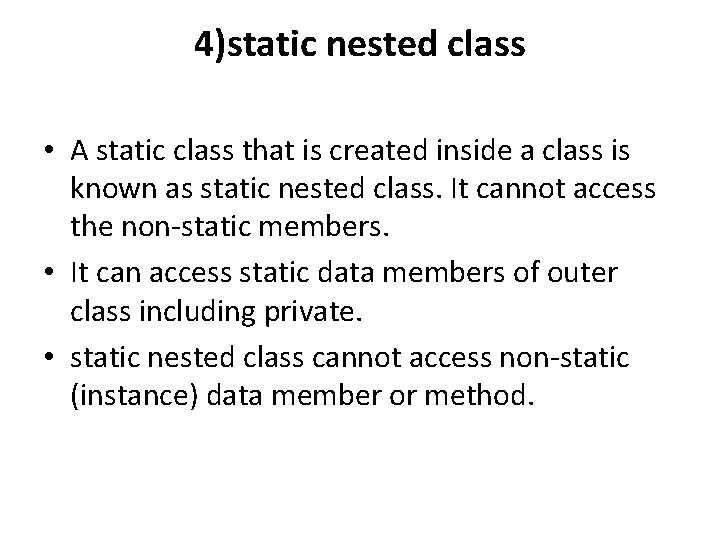
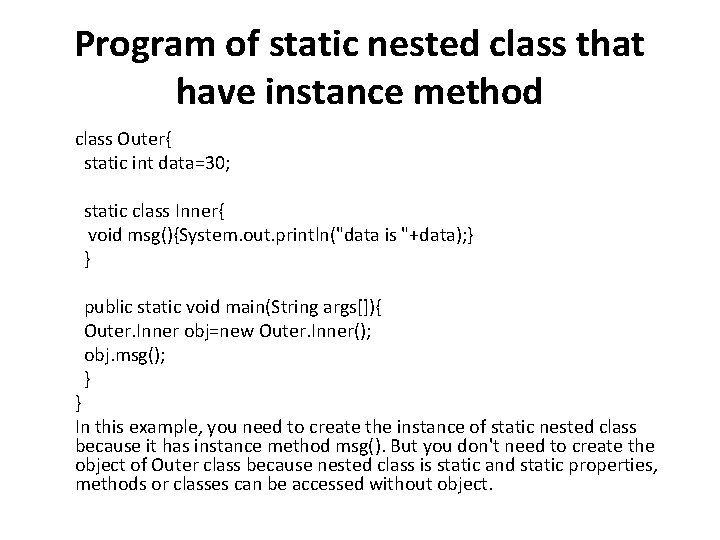
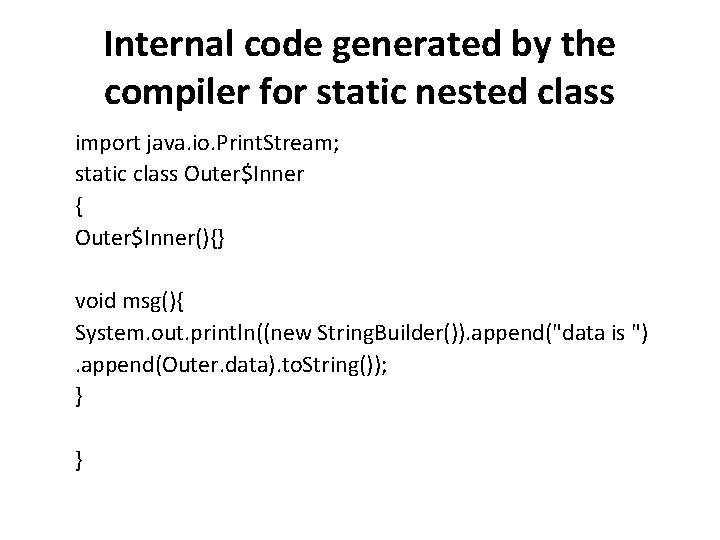
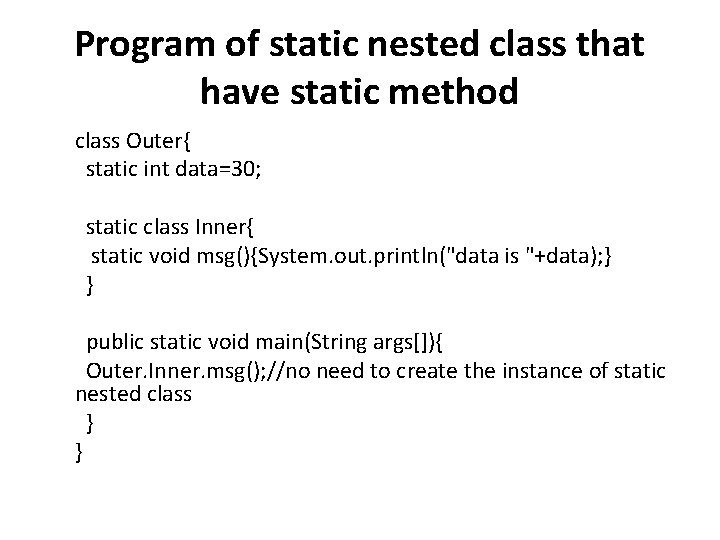
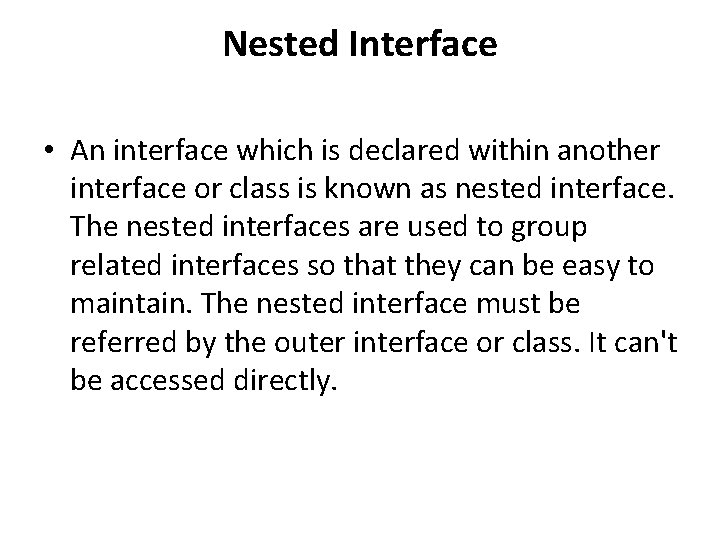
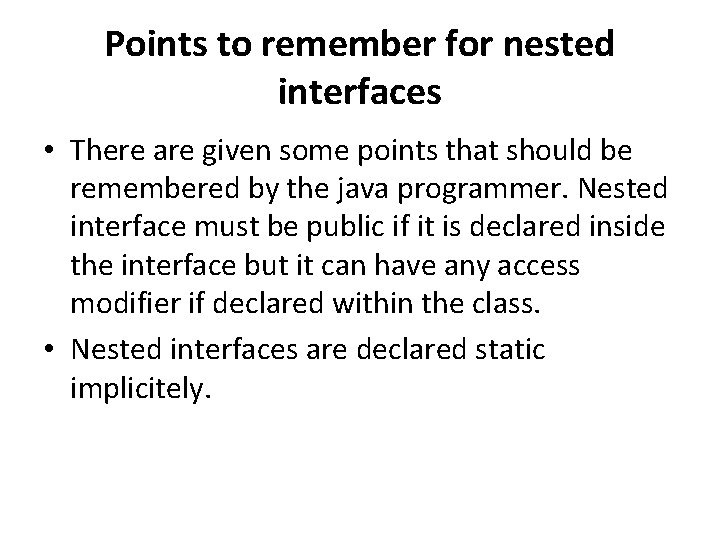
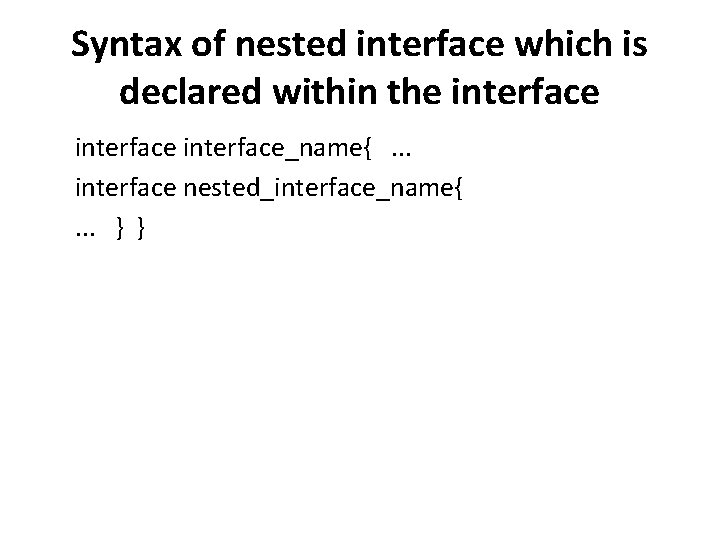
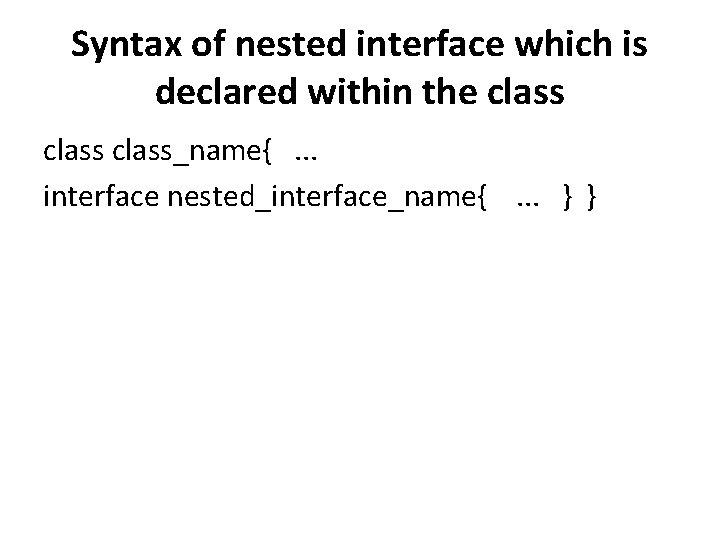
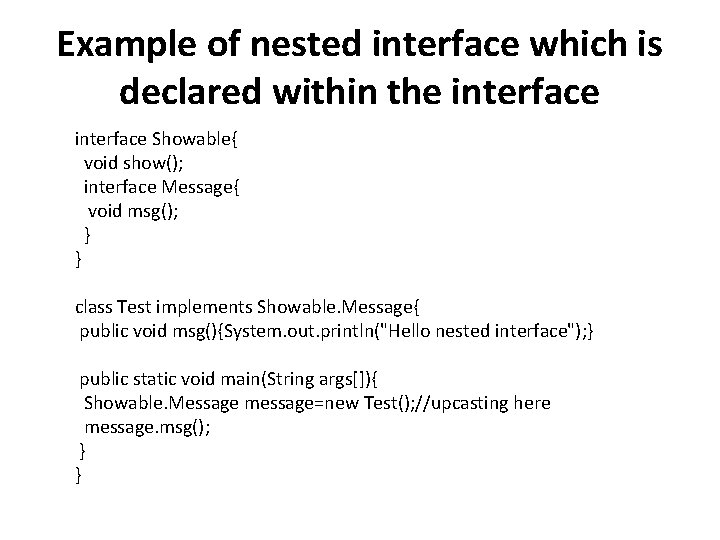
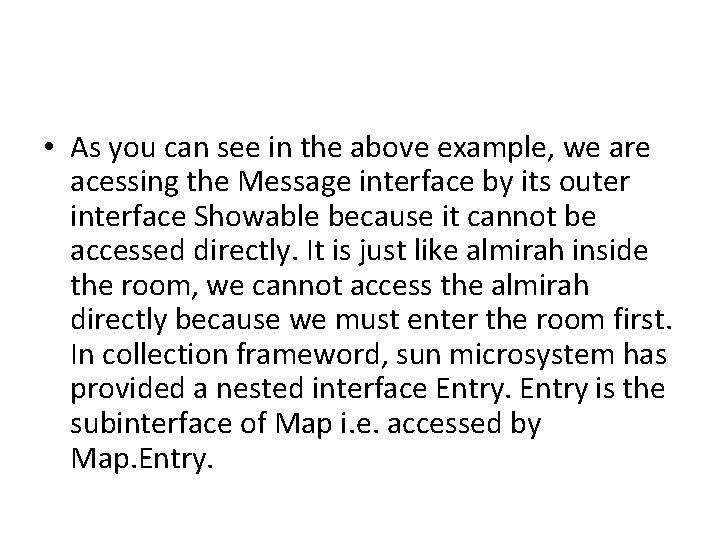
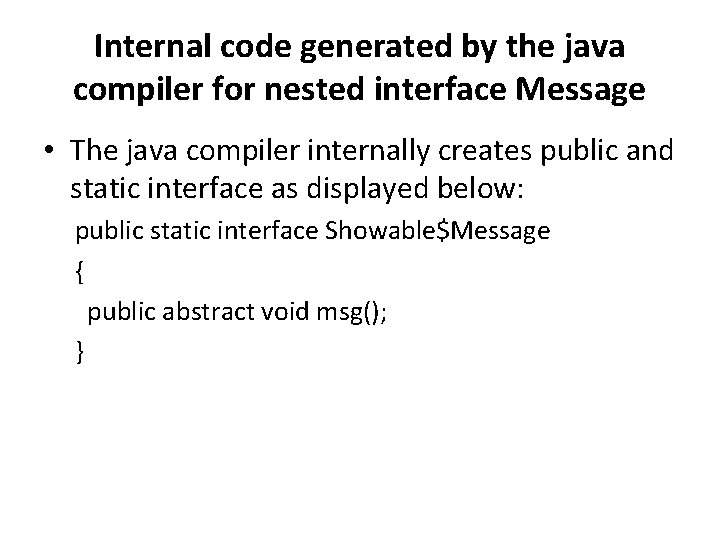
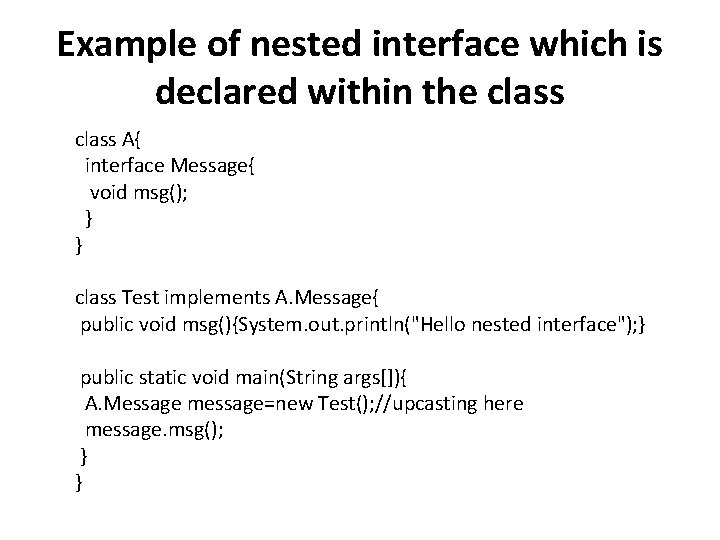
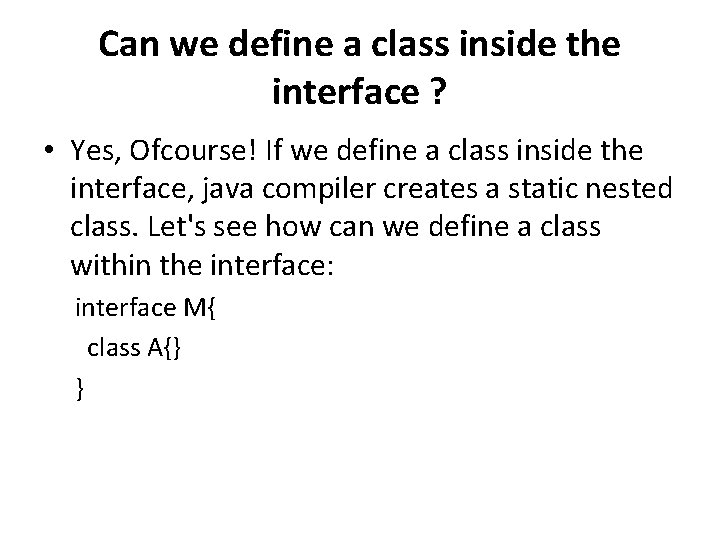
- Slides: 40
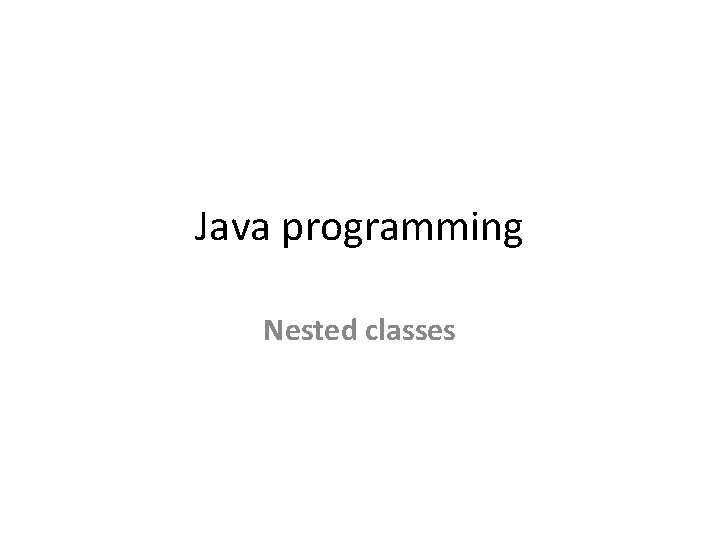
Java programming Nested classes
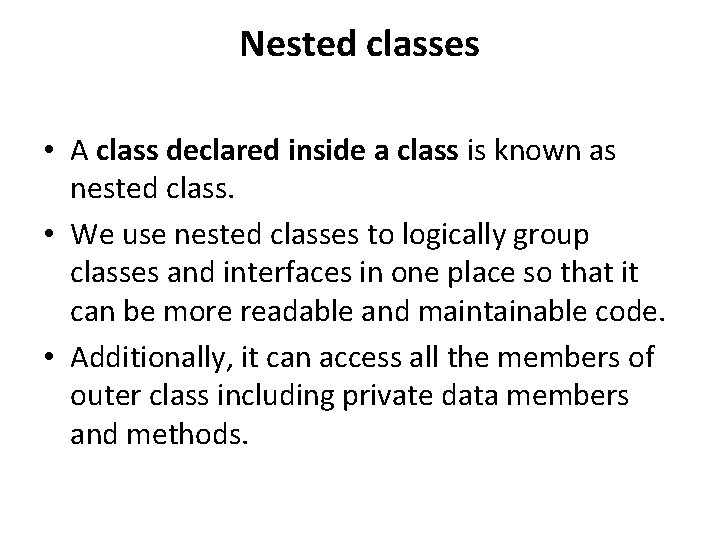
Nested classes • A class declared inside a class is known as nested class. • We use nested classes to logically group classes and interfaces in one place so that it can be more readable and maintainable code. • Additionally, it can access all the members of outer class including private data members and methods.
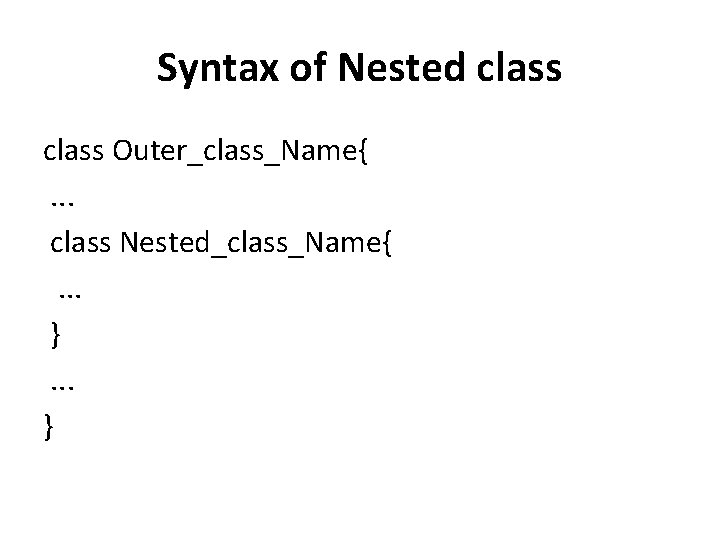
Syntax of Nested class Outer_class_Name{. . . class Nested_class_Name{. . . }
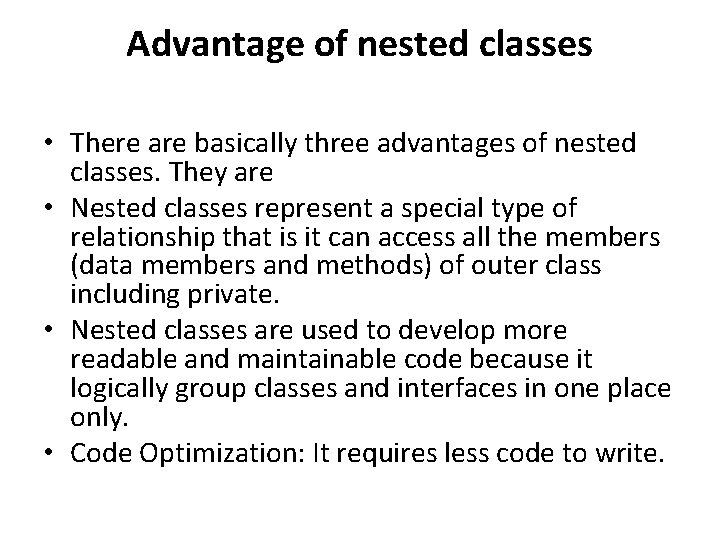
Advantage of nested classes • There are basically three advantages of nested classes. They are • Nested classes represent a special type of relationship that is it can access all the members (data members and methods) of outer class including private. • Nested classes are used to develop more readable and maintainable code because it logically group classes and interfaces in one place only. • Code Optimization: It requires less code to write.
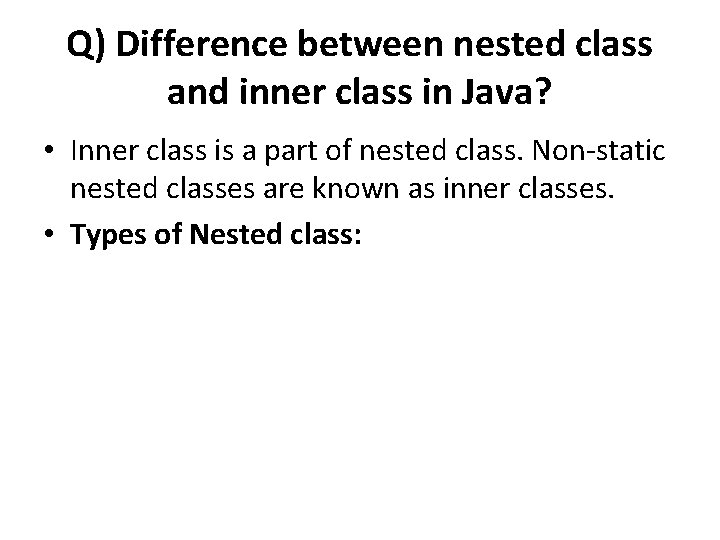
Q) Difference between nested class and inner class in Java? • Inner class is a part of nested class. Non-static nested classes are known as inner classes. • Types of Nested class:
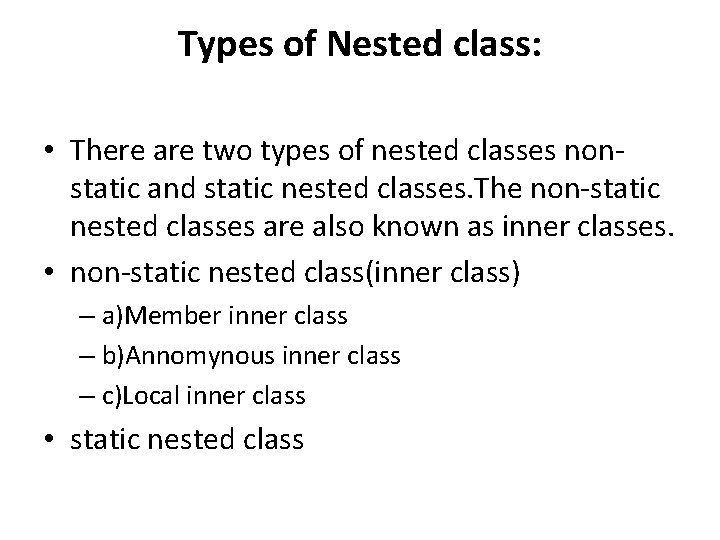
Types of Nested class: • There are two types of nested classes nonstatic and static nested classes. The non-static nested classes are also known as inner classes. • non-static nested class(inner class) – a)Member inner class – b)Annomynous inner class – c)Local inner class • static nested class
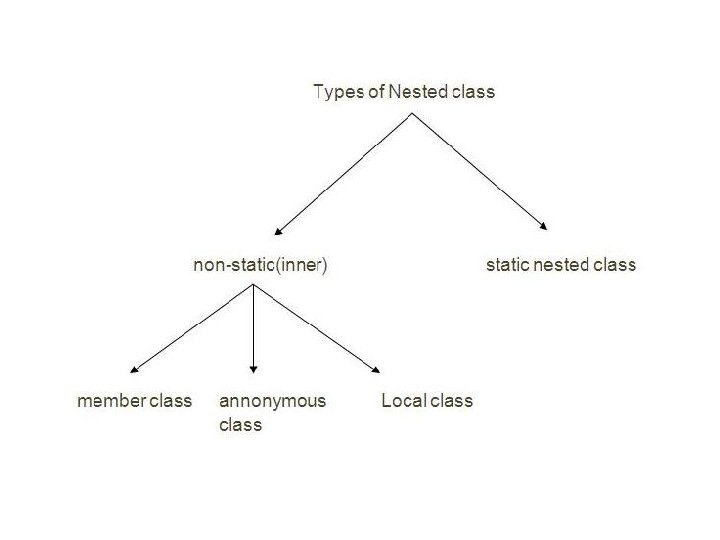
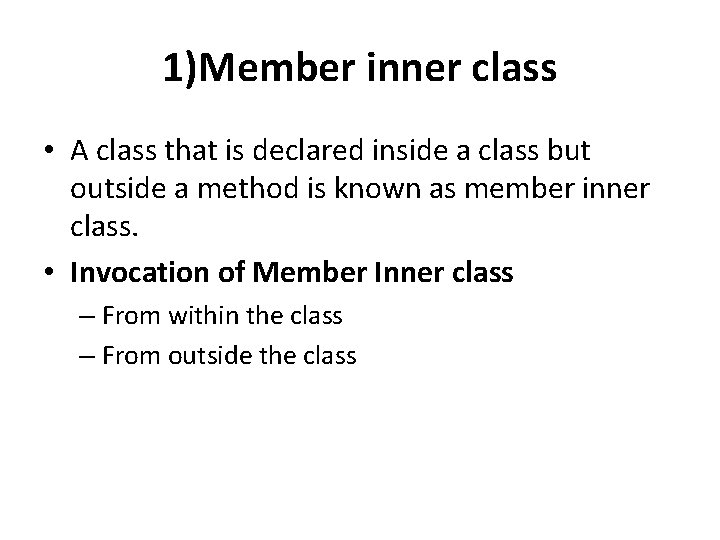
1)Member inner class • A class that is declared inside a class but outside a method is known as member inner class. • Invocation of Member Inner class – From within the class – From outside the class
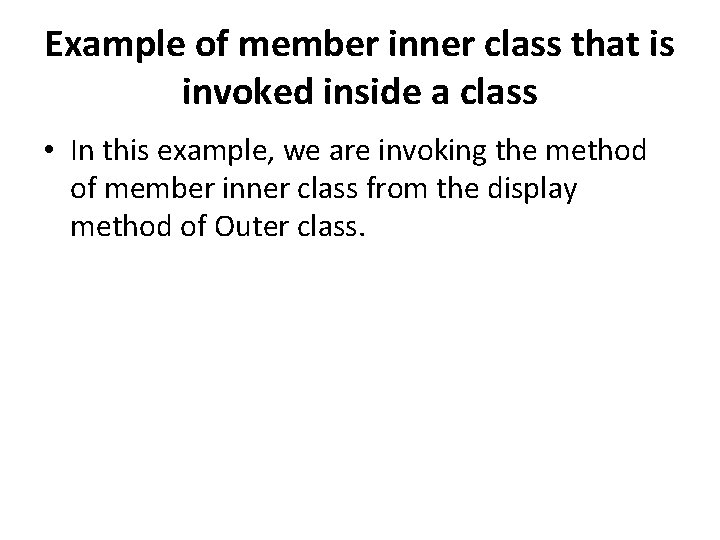
Example of member inner class that is invoked inside a class • In this example, we are invoking the method of member inner class from the display method of Outer class.
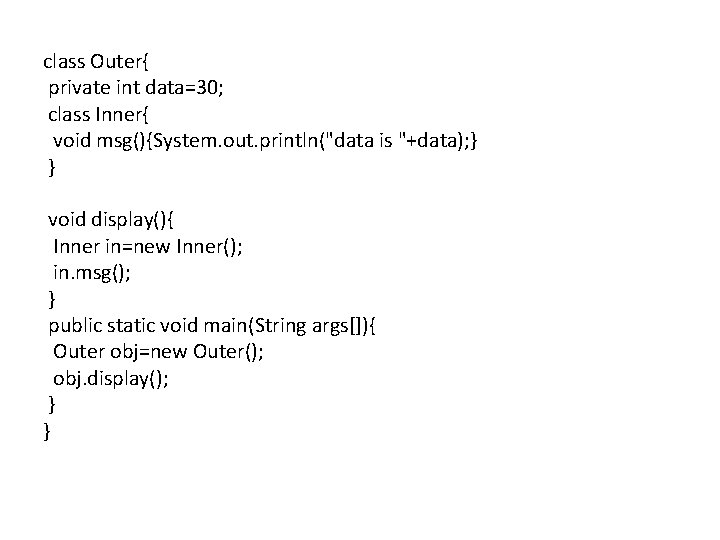
class Outer{ private int data=30; class Inner{ void msg(){System. out. println("data is "+data); } } void display(){ Inner in=new Inner(); in. msg(); } public static void main(String args[]){ Outer obj=new Outer(); obj. display(); } }
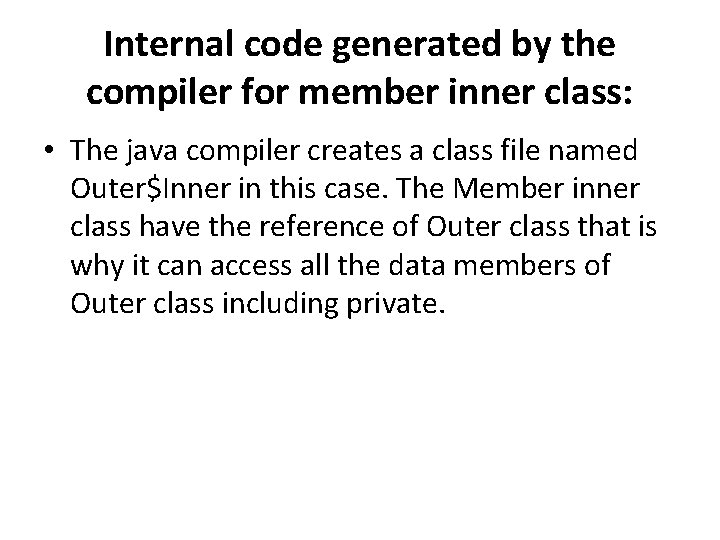
Internal code generated by the compiler for member inner class: • The java compiler creates a class file named Outer$Inner in this case. The Member inner class have the reference of Outer class that is why it can access all the data members of Outer class including private.
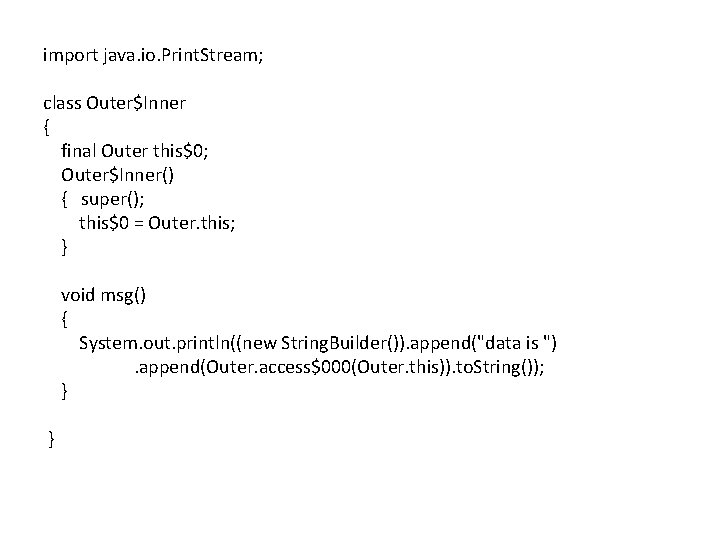
import java. io. Print. Stream; class Outer$Inner { final Outer this$0; Outer$Inner() { super(); this$0 = Outer. this; } void msg() { System. out. println((new String. Builder()). append("data is "). append(Outer. access$000(Outer. this)). to. String()); } }
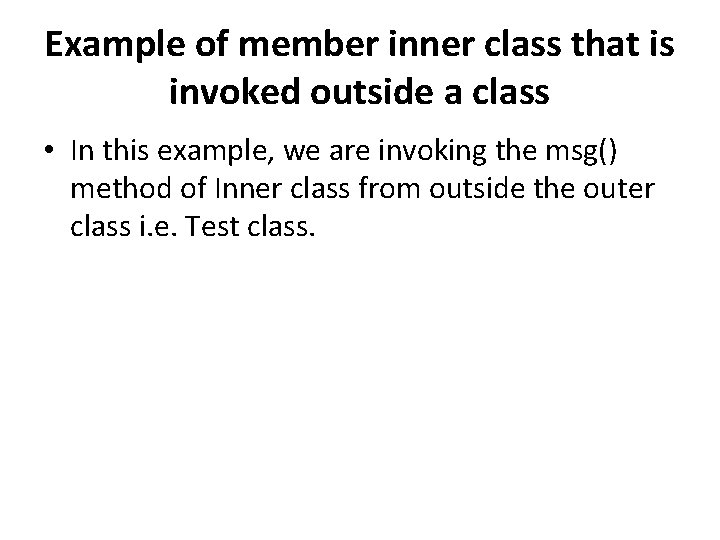
Example of member inner class that is invoked outside a class • In this example, we are invoking the msg() method of Inner class from outside the outer class i. e. Test class.
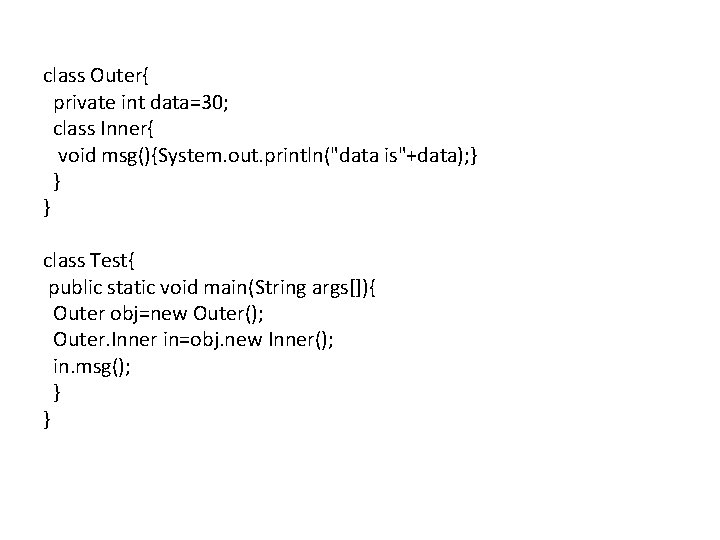
class Outer{ private int data=30; class Inner{ void msg(){System. out. println("data is"+data); } } } class Test{ public static void main(String args[]){ Outer obj=new Outer(); Outer. Inner in=obj. new Inner(); in. msg(); } }
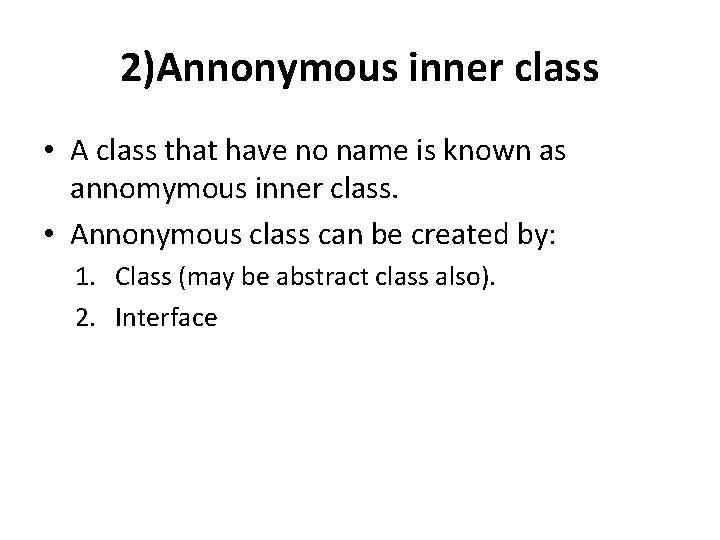
2)Annonymous inner class • A class that have no name is known as annomymous inner class. • Annonymous class can be created by: 1. Class (may be abstract class also). 2. Interface
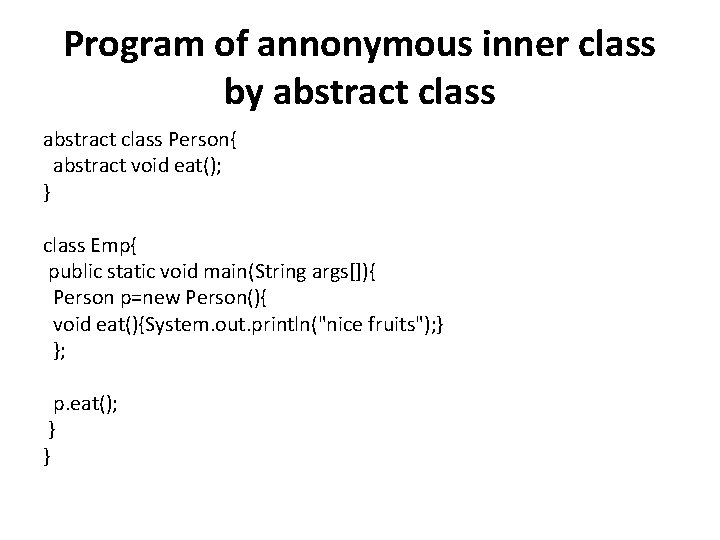
Program of annonymous inner class by abstract class Person{ abstract void eat(); } class Emp{ public static void main(String args[]){ Person p=new Person(){ void eat(){System. out. println("nice fruits"); } }; p. eat(); } }
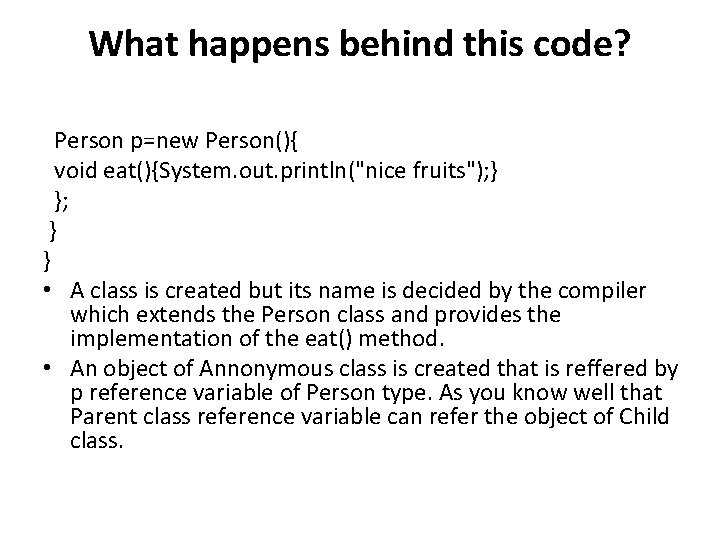
What happens behind this code? Person p=new Person(){ void eat(){System. out. println("nice fruits"); } } • A class is created but its name is decided by the compiler which extends the Person class and provides the implementation of the eat() method. • An object of Annonymous class is created that is reffered by p reference variable of Person type. As you know well that Parent class reference variable can refer the object of Child class.
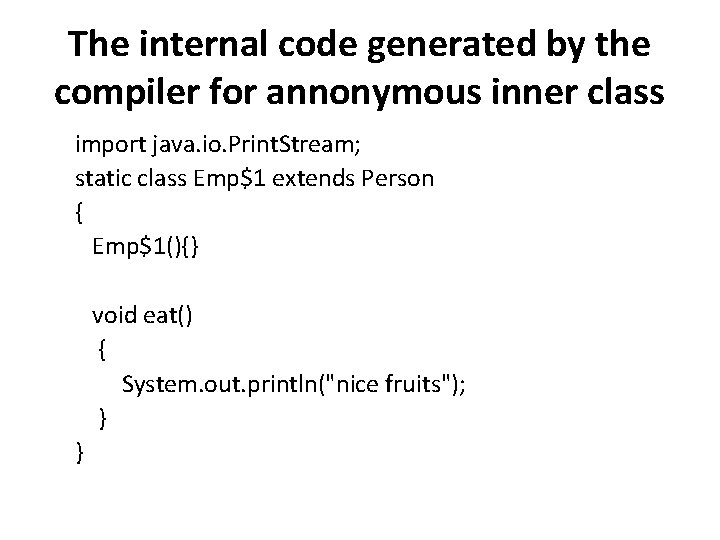
The internal code generated by the compiler for annonymous inner class import java. io. Print. Stream; static class Emp$1 extends Person { Emp$1(){} void eat() { System. out. println("nice fruits"); } }
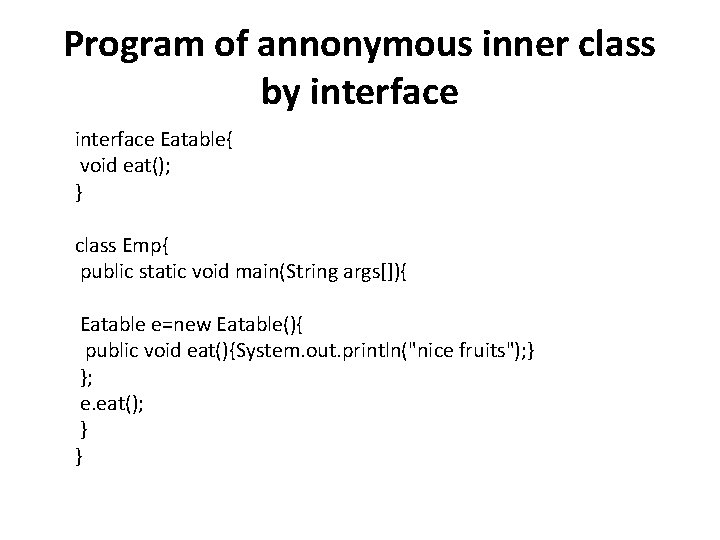
Program of annonymous inner class by interface Eatable{ void eat(); } class Emp{ public static void main(String args[]){ Eatable e=new Eatable(){ public void eat(){System. out. println("nice fruits"); } }; e. eat(); } }
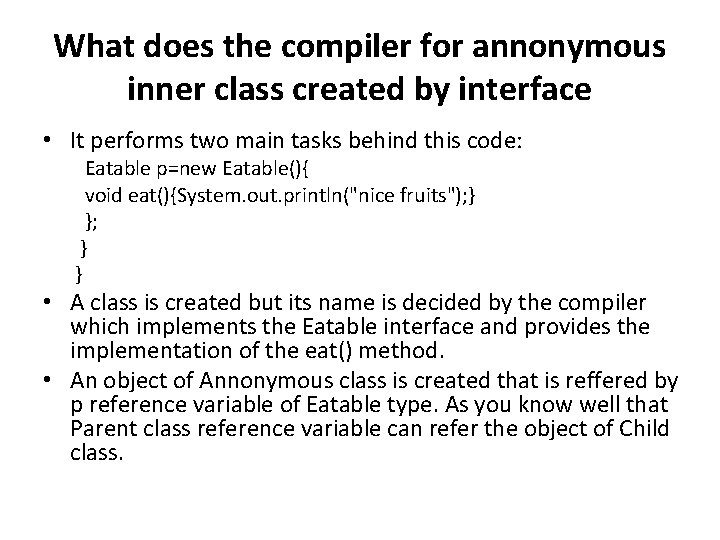
What does the compiler for annonymous inner class created by interface • It performs two main tasks behind this code: Eatable p=new Eatable(){ void eat(){System. out. println("nice fruits"); } } • A class is created but its name is decided by the compiler which implements the Eatable interface and provides the implementation of the eat() method. • An object of Annonymous class is created that is reffered by p reference variable of Eatable type. As you know well that Parent class reference variable can refer the object of Child class.
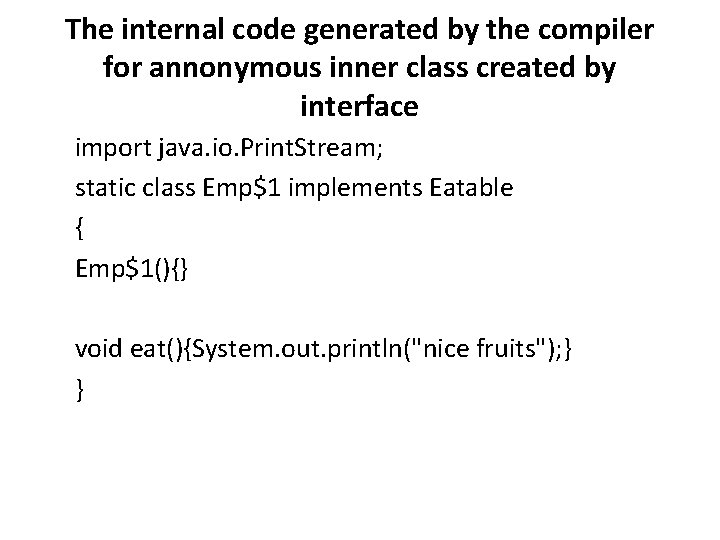
The internal code generated by the compiler for annonymous inner class created by interface import java. io. Print. Stream; static class Emp$1 implements Eatable { Emp$1(){} void eat(){System. out. println("nice fruits"); } }
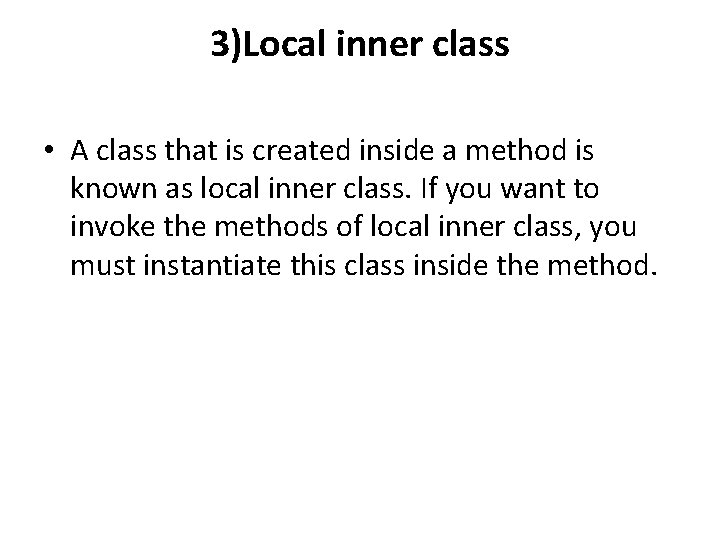
3)Local inner class • A class that is created inside a method is known as local inner class. If you want to invoke the methods of local inner class, you must instantiate this class inside the method.
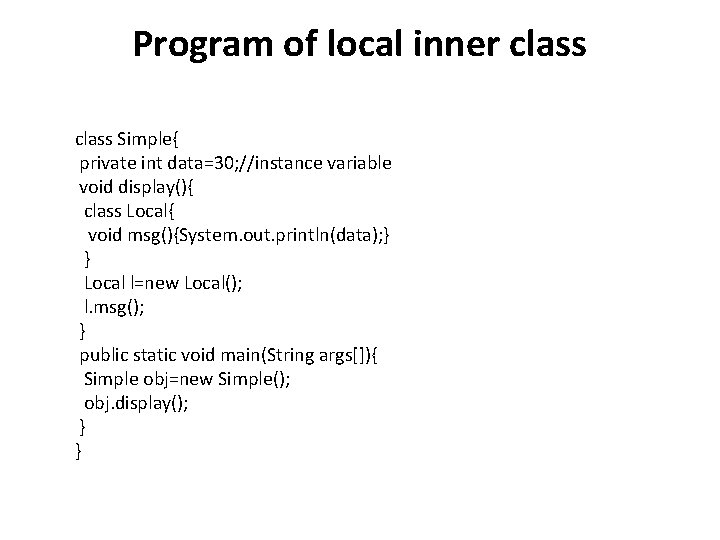
Program of local inner class Simple{ private int data=30; //instance variable void display(){ class Local{ void msg(){System. out. println(data); } } Local l=new Local(); l. msg(); } public static void main(String args[]){ Simple obj=new Simple(); obj. display(); } }
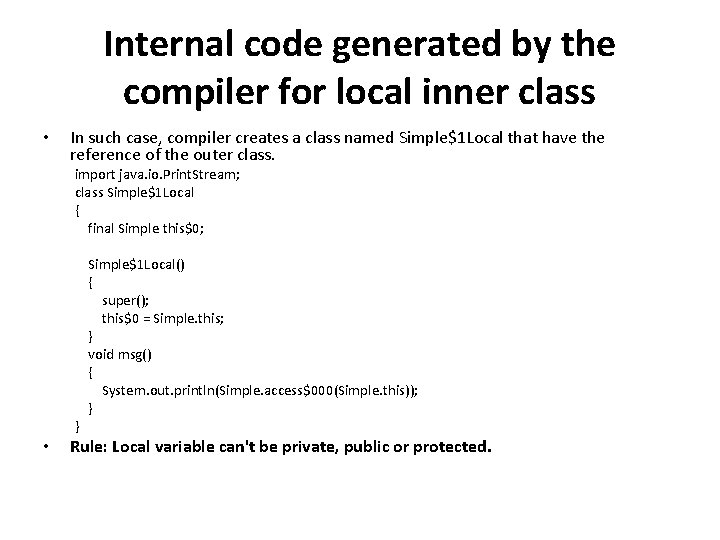
Internal code generated by the compiler for local inner class • In such case, compiler creates a class named Simple$1 Local that have the reference of the outer class. import java. io. Print. Stream; class Simple$1 Local { final Simple this$0; } • Simple$1 Local() { super(); this$0 = Simple. this; } void msg() { System. out. println(Simple. access$000(Simple. this)); } Rule: Local variable can't be private, public or protected.
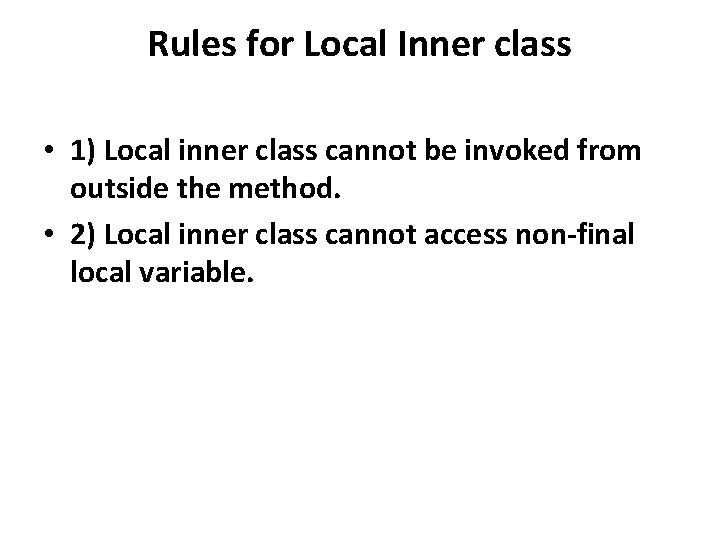
Rules for Local Inner class • 1) Local inner class cannot be invoked from outside the method. • 2) Local inner class cannot access non-final local variable.
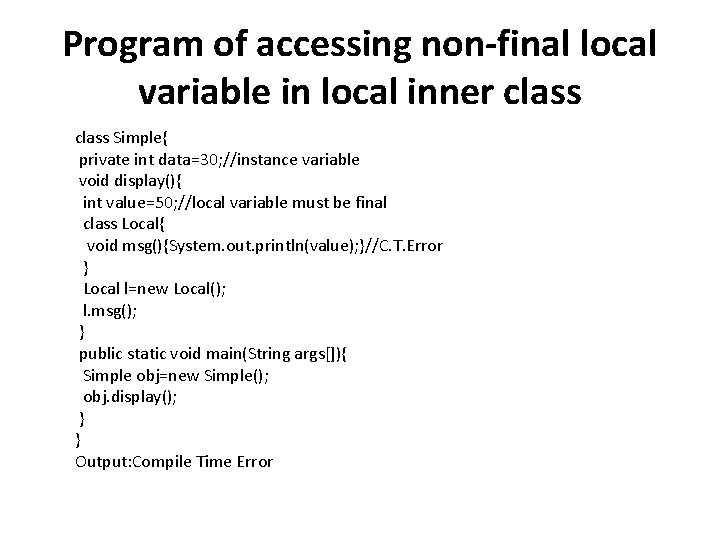
Program of accessing non-final local variable in local inner class Simple{ private int data=30; //instance variable void display(){ int value=50; //local variable must be final class Local{ void msg(){System. out. println(value); }//C. T. Error } Local l=new Local(); l. msg(); } public static void main(String args[]){ Simple obj=new Simple(); obj. display(); } } Output: Compile Time Error
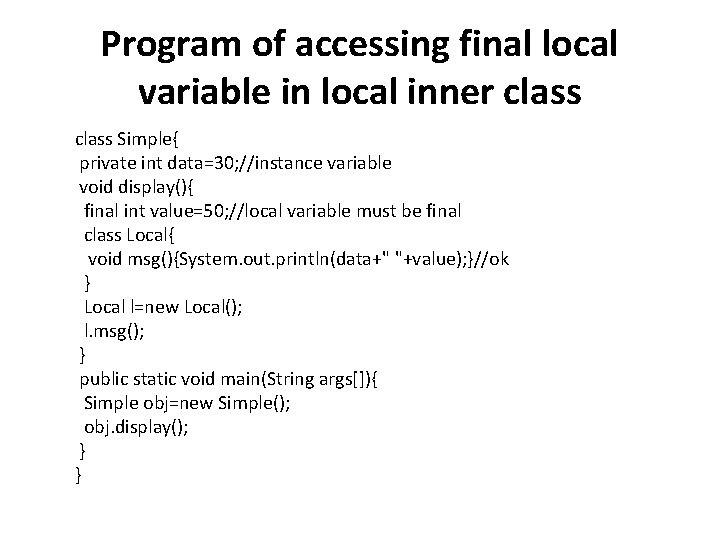
Program of accessing final local variable in local inner class Simple{ private int data=30; //instance variable void display(){ final int value=50; //local variable must be final class Local{ void msg(){System. out. println(data+" "+value); }//ok } Local l=new Local(); l. msg(); } public static void main(String args[]){ Simple obj=new Simple(); obj. display(); } }
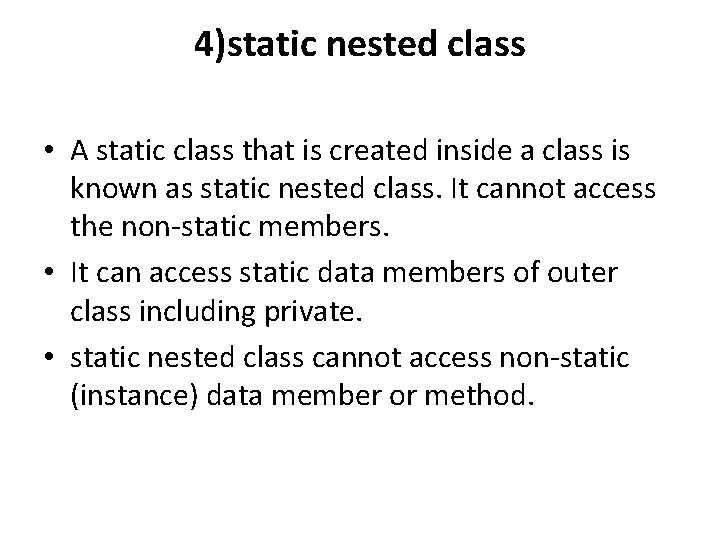
4)static nested class • A static class that is created inside a class is known as static nested class. It cannot access the non-static members. • It can access static data members of outer class including private. • static nested class cannot access non-static (instance) data member or method.
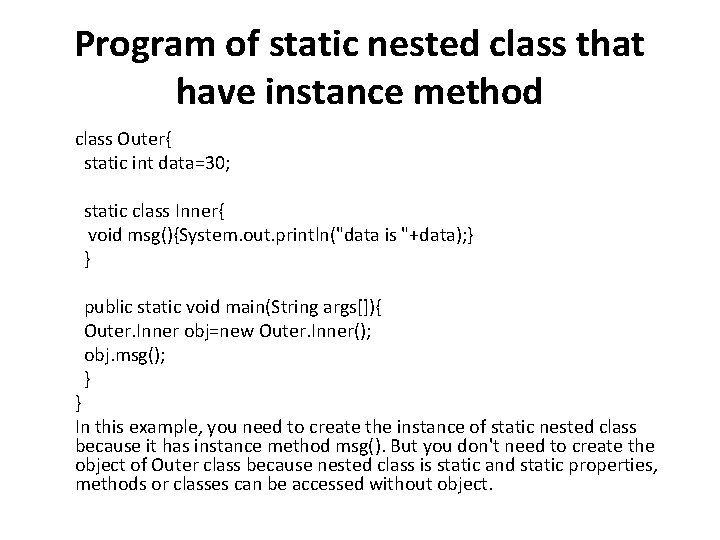
Program of static nested class that have instance method class Outer{ static int data=30; static class Inner{ void msg(){System. out. println("data is "+data); } } public static void main(String args[]){ Outer. Inner obj=new Outer. Inner(); obj. msg(); } } In this example, you need to create the instance of static nested class because it has instance method msg(). But you don't need to create the object of Outer class because nested class is static and static properties, methods or classes can be accessed without object.
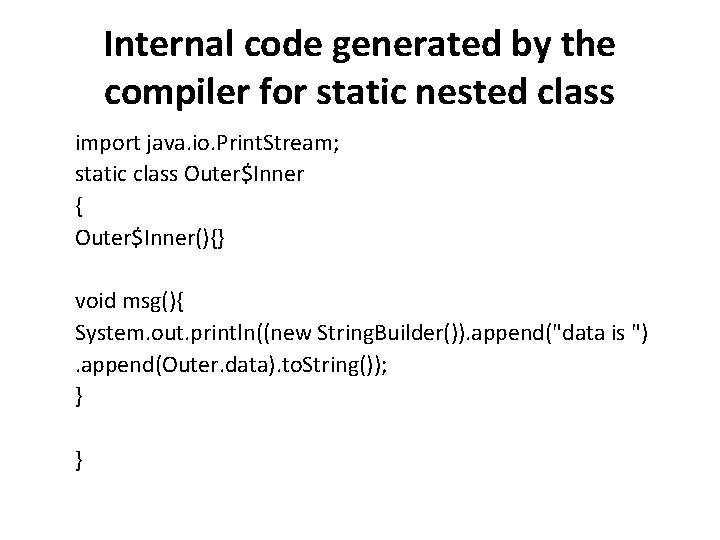
Internal code generated by the compiler for static nested class import java. io. Print. Stream; static class Outer$Inner { Outer$Inner(){} void msg(){ System. out. println((new String. Builder()). append("data is "). append(Outer. data). to. String()); } }
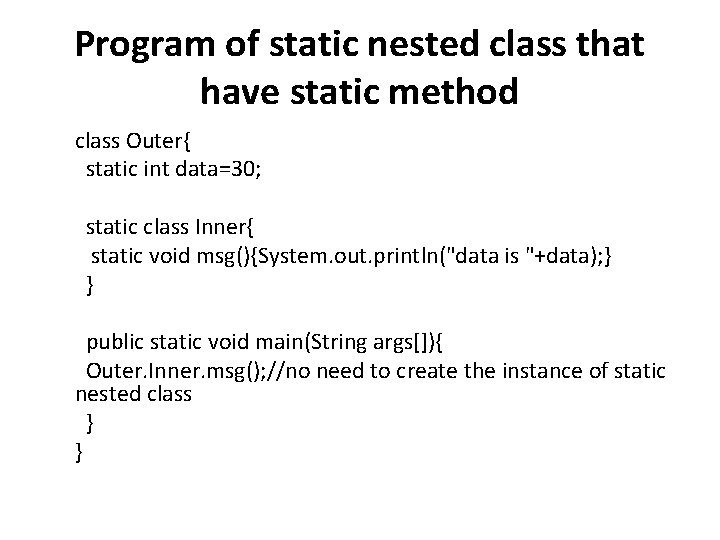
Program of static nested class that have static method class Outer{ static int data=30; static class Inner{ static void msg(){System. out. println("data is "+data); } } public static void main(String args[]){ Outer. Inner. msg(); //no need to create the instance of static nested class } }
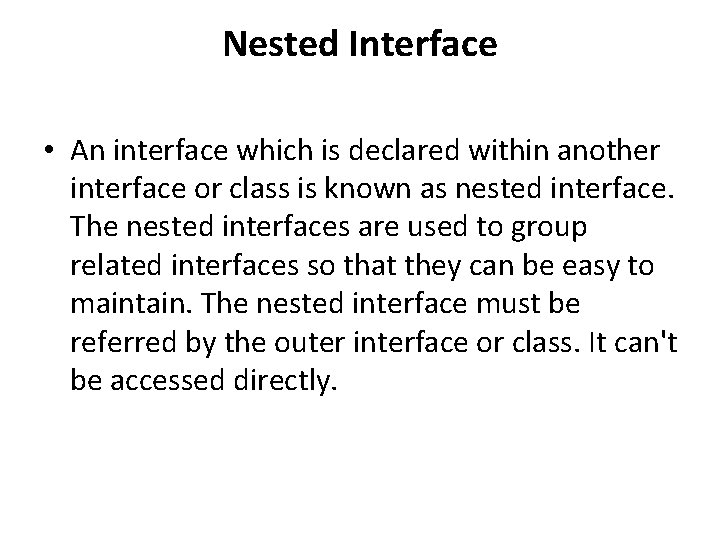
Nested Interface • An interface which is declared within another interface or class is known as nested interface. The nested interfaces are used to group related interfaces so that they can be easy to maintain. The nested interface must be referred by the outer interface or class. It can't be accessed directly.
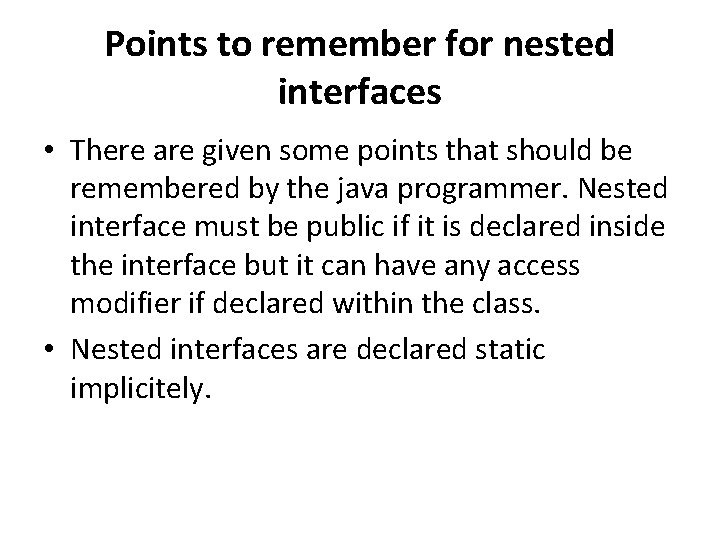
Points to remember for nested interfaces • There are given some points that should be remembered by the java programmer. Nested interface must be public if it is declared inside the interface but it can have any access modifier if declared within the class. • Nested interfaces are declared static implicitely.
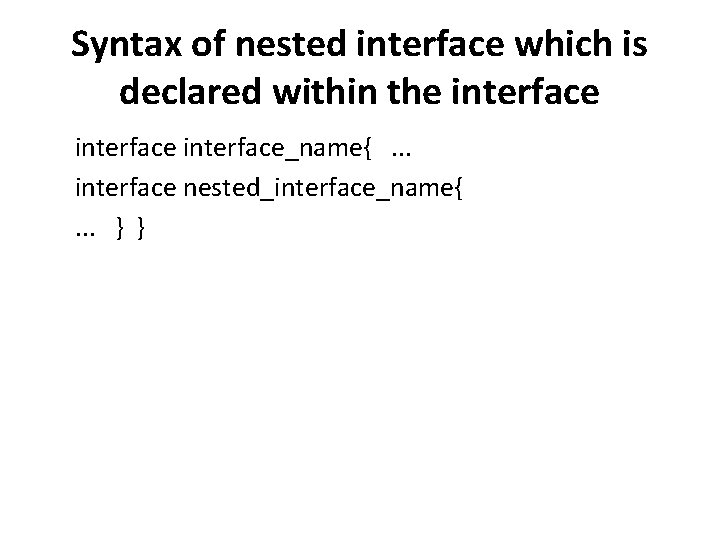
Syntax of nested interface which is declared within the interface_name{. . . interface nested_interface_name{. . . } }
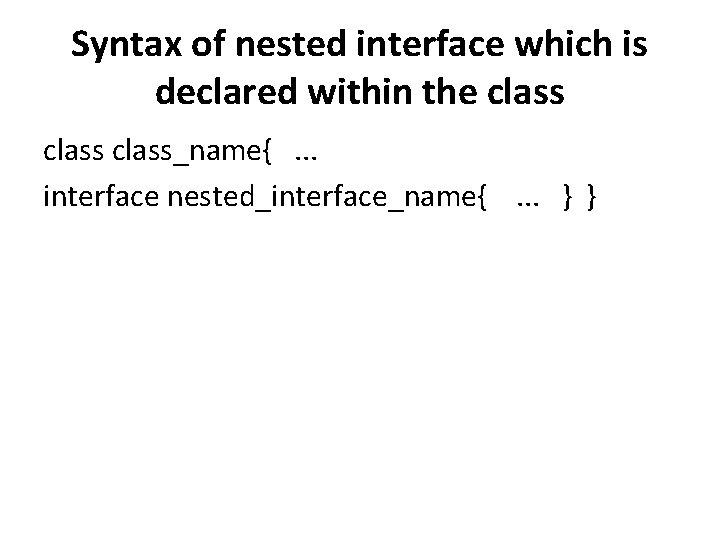
Syntax of nested interface which is declared within the class_name{. . . interface nested_interface_name{. . . } }
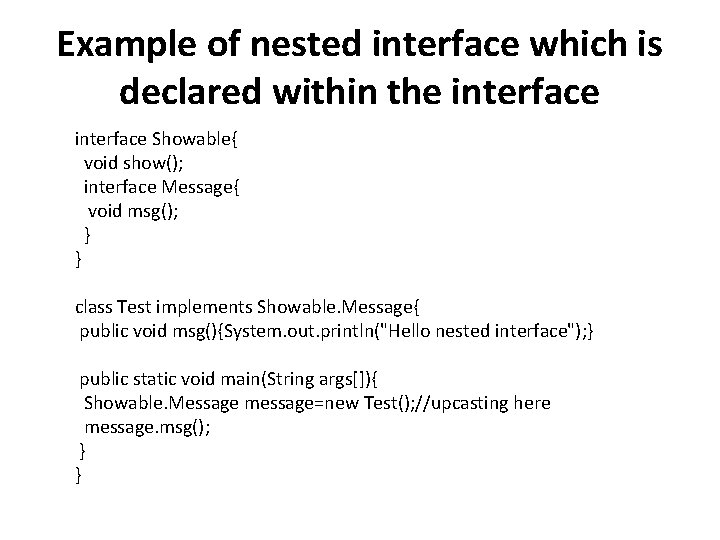
Example of nested interface which is declared within the interface Showable{ void show(); interface Message{ void msg(); } } class Test implements Showable. Message{ public void msg(){System. out. println("Hello nested interface"); } public static void main(String args[]){ Showable. Message message=new Test(); //upcasting here message. msg(); } }
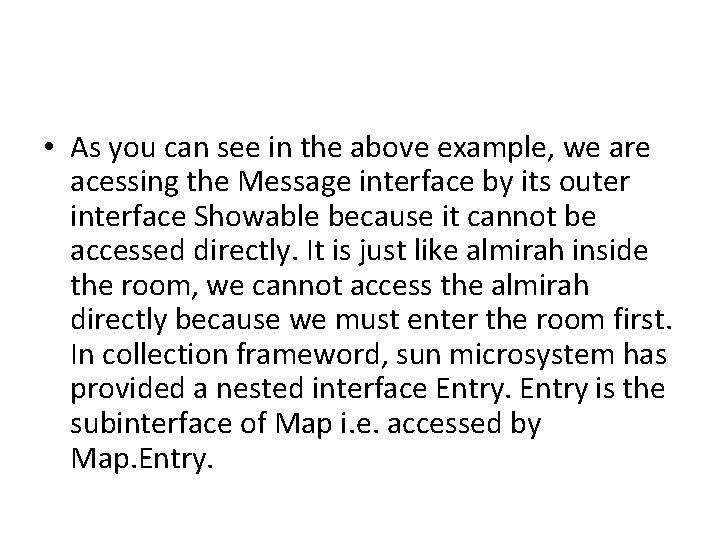
• As you can see in the above example, we are acessing the Message interface by its outer interface Showable because it cannot be accessed directly. It is just like almirah inside the room, we cannot access the almirah directly because we must enter the room first. In collection frameword, sun microsystem has provided a nested interface Entry is the subinterface of Map i. e. accessed by Map. Entry.
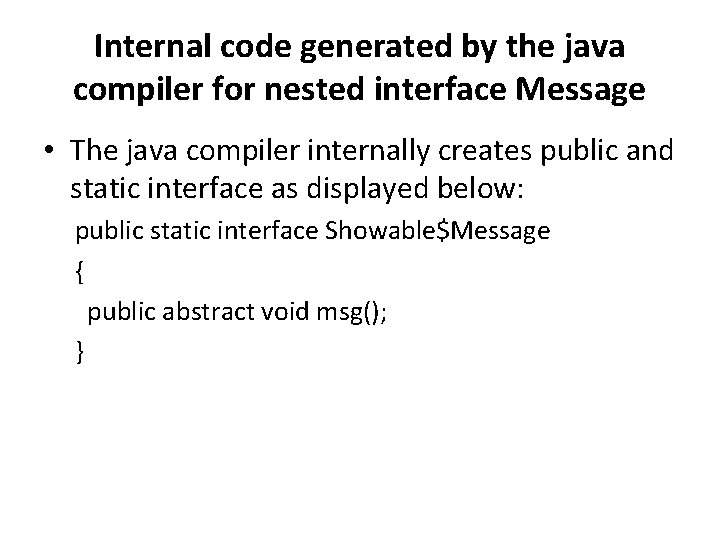
Internal code generated by the java compiler for nested interface Message • The java compiler internally creates public and static interface as displayed below: public static interface Showable$Message { public abstract void msg(); }
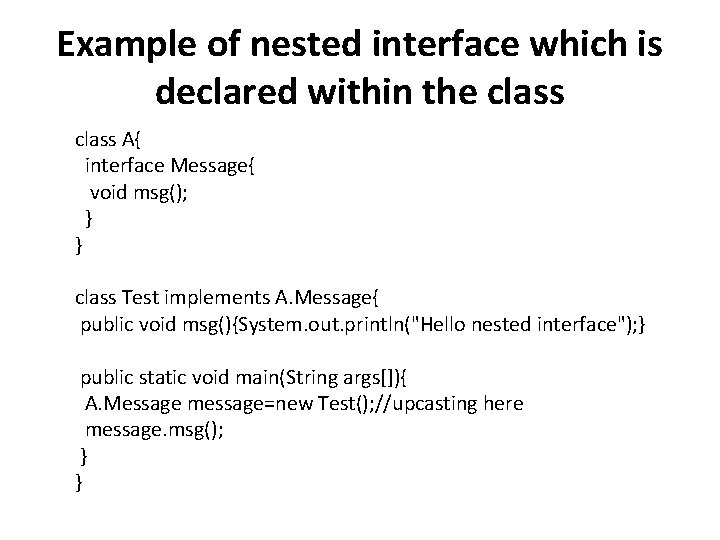
Example of nested interface which is declared within the class A{ interface Message{ void msg(); } } class Test implements A. Message{ public void msg(){System. out. println("Hello nested interface"); } public static void main(String args[]){ A. Message message=new Test(); //upcasting here message. msg(); } }
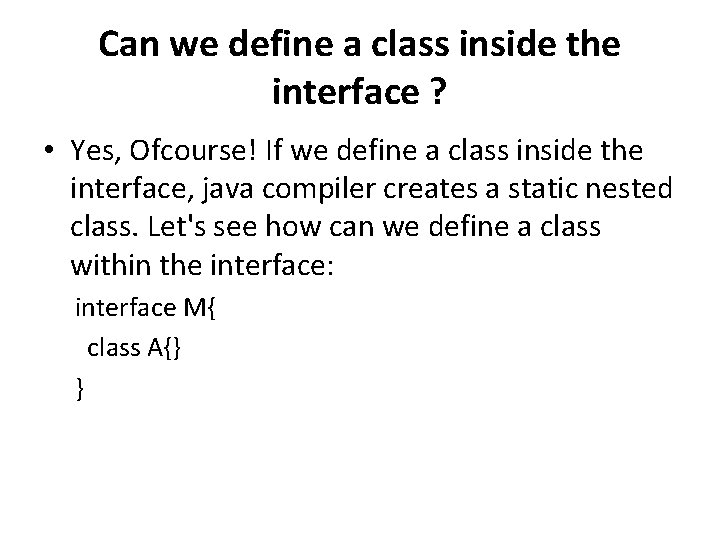
Can we define a class inside the interface ? • Yes, Ofcourse! If we define a class inside the interface, java compiler creates a static nested class. Let's see how can we define a class within the interface: interface M{ class A{} }
Matlab break command
Nested loops java
Subclasse e classe de palavras
Pre ap classes vs regular classes
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
Definition of system programming
Linear vs integer programming
Definisi linear
List button
Adapter class java
Isomerism
Interfaces
Writing classes java
Abstract classes
Java script classes
Udp socket programming in java
Parallel programming java
Object oriented programming exercises java
Java introduction to problem solving and programming
Java event driven programming example
Daniel liang introduction to java programming
Java asynchronous programming
Contoh program terstruktur
Arne kutzner
Khan academy java script
Event driven programming in java
Defensive programming java
Java refresher course
Java games programming
Java programming symbols
Java introduction to problem solving and programming
Elementary programming in java
Java database programming
Java asynchronous programming
Conclusion of java programming
Java programming
Elementary programming in java
Basic elements of java
Advanced programming in java
Java language