Java Methods TM Maria Litvin Gary Litvin An
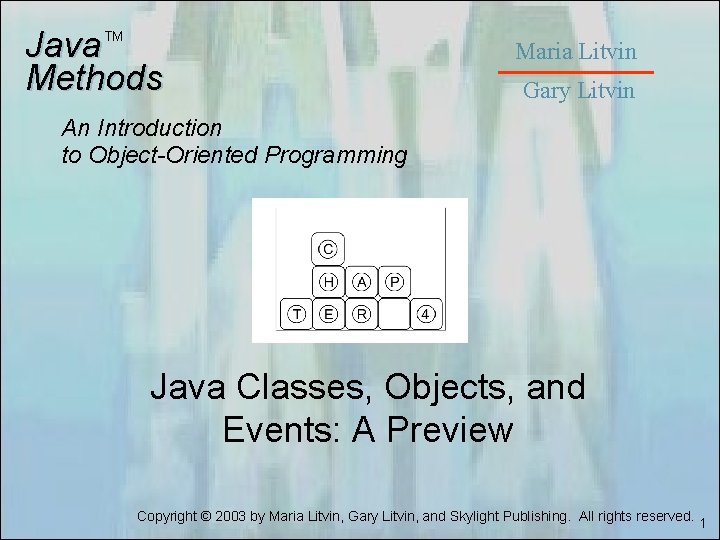
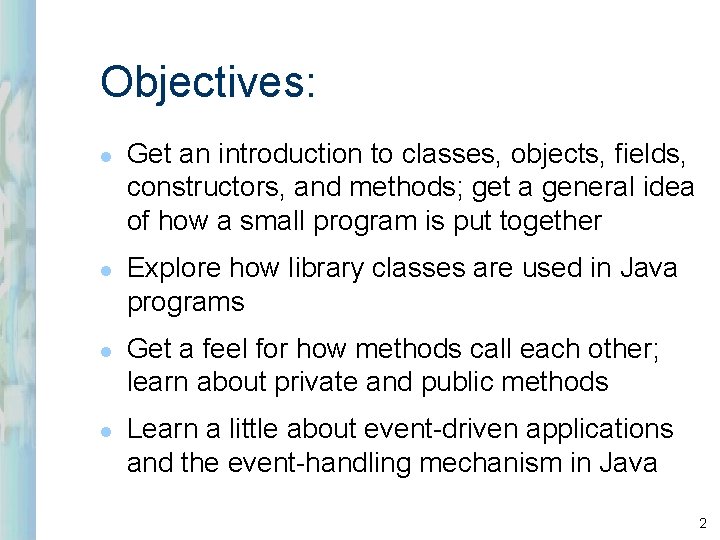
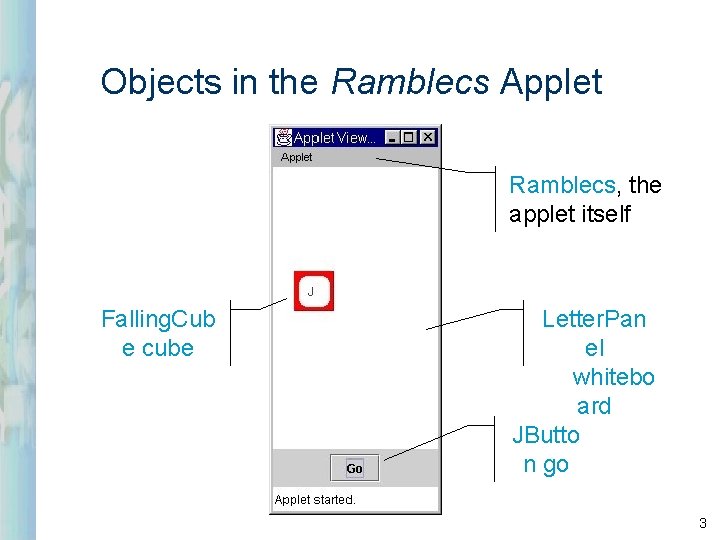
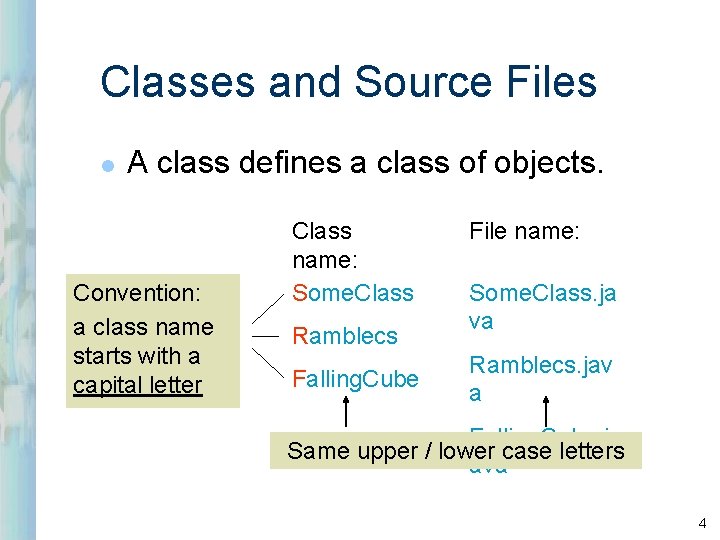
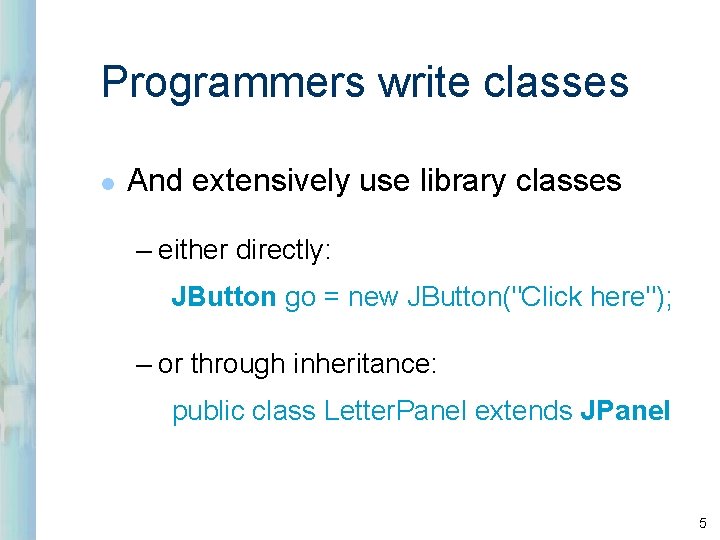
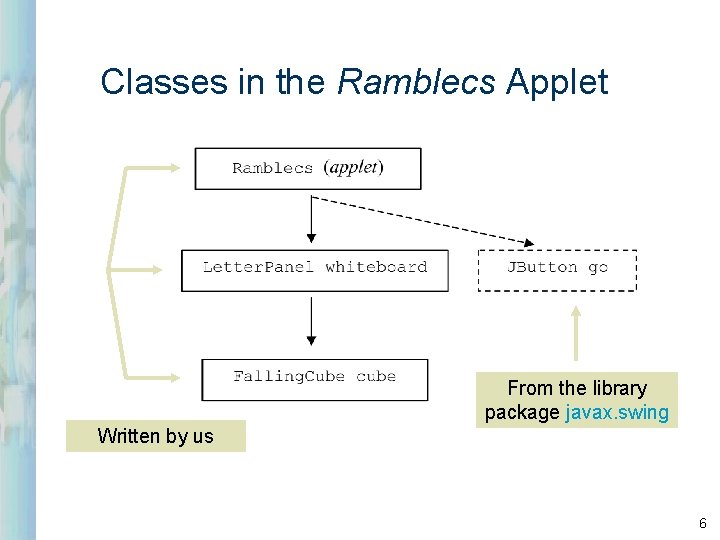
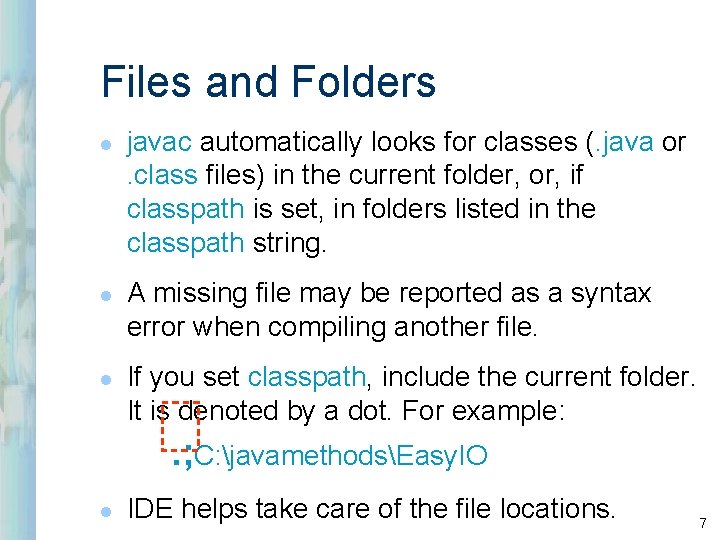
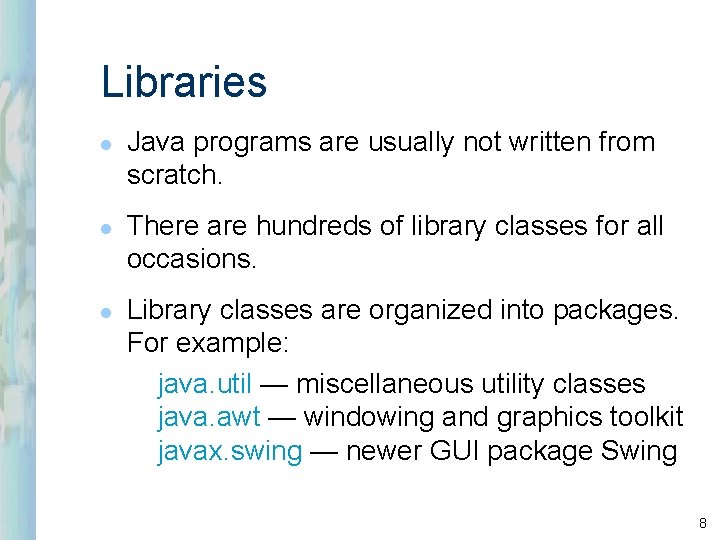
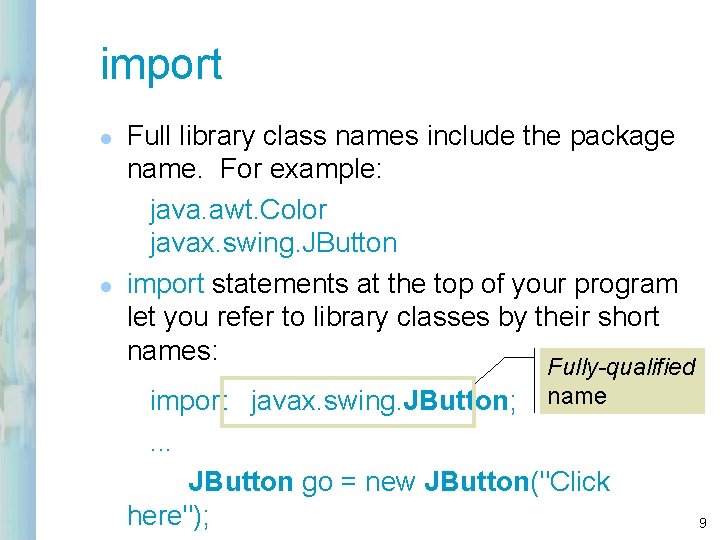
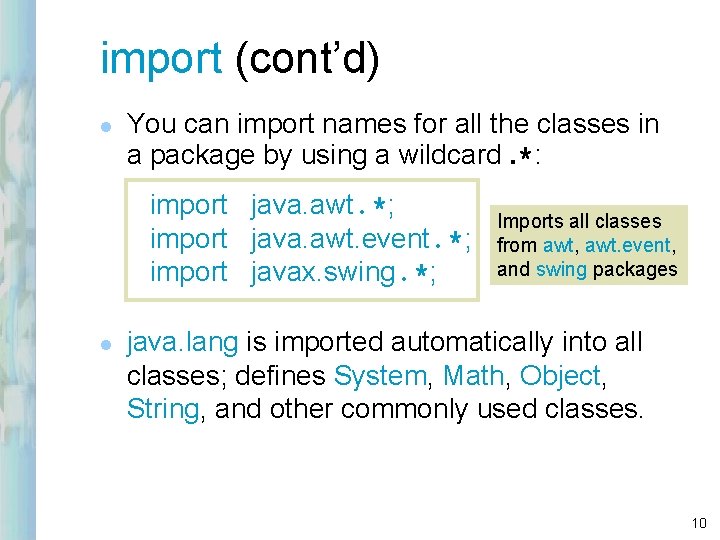
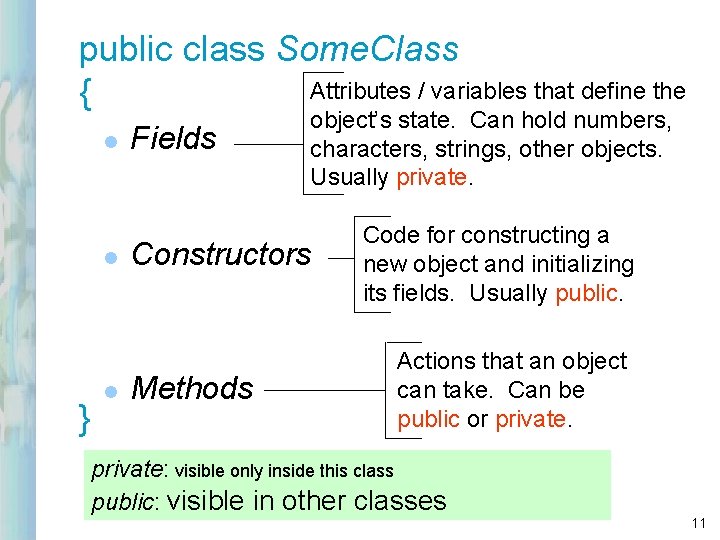
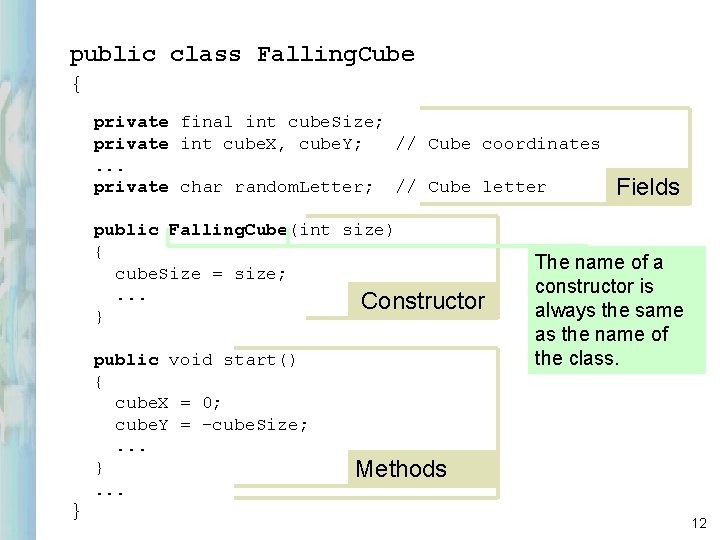
![Fields You name it! private (or public) [static] [final] Usually privatename; May be present: Fields You name it! private (or public) [static] [final] Usually privatename; May be present:](https://slidetodoc.com/presentation_image_h/17944643f8adf08eefe97ed7d2b57c08/image-13.jpg)
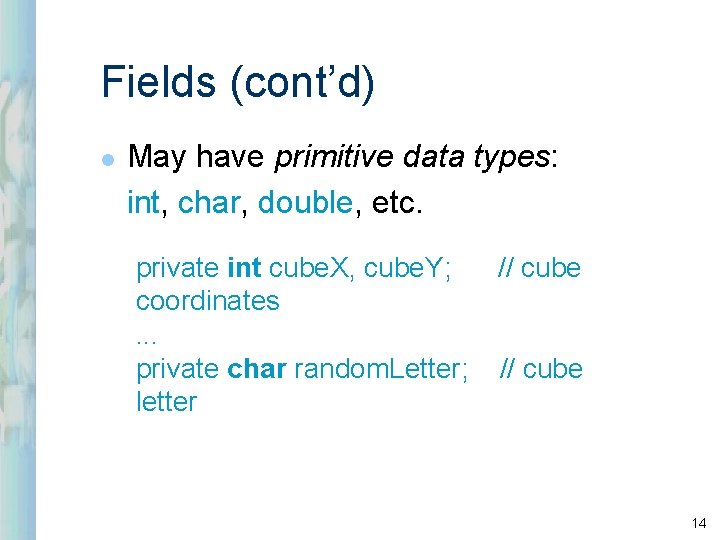
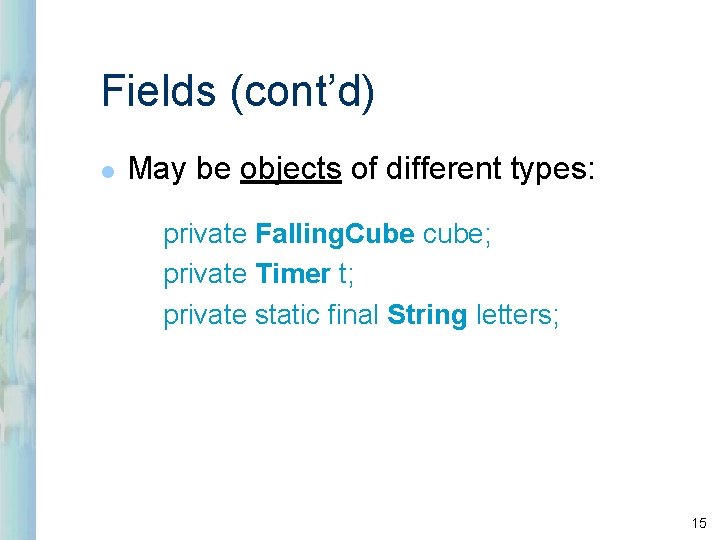
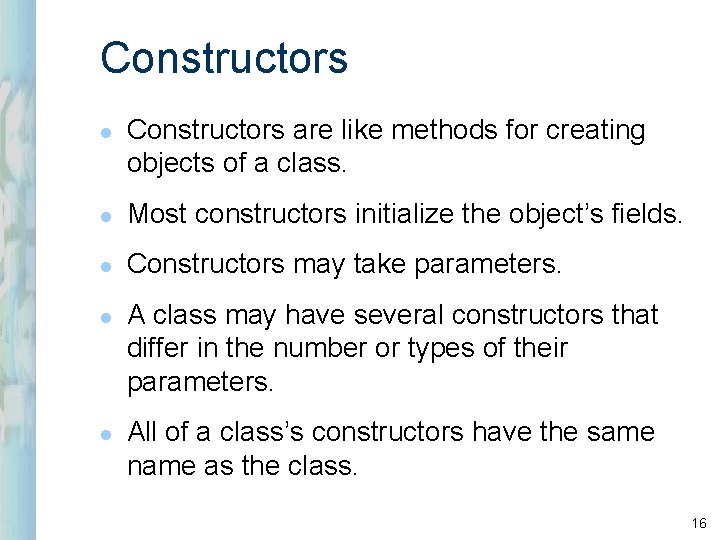
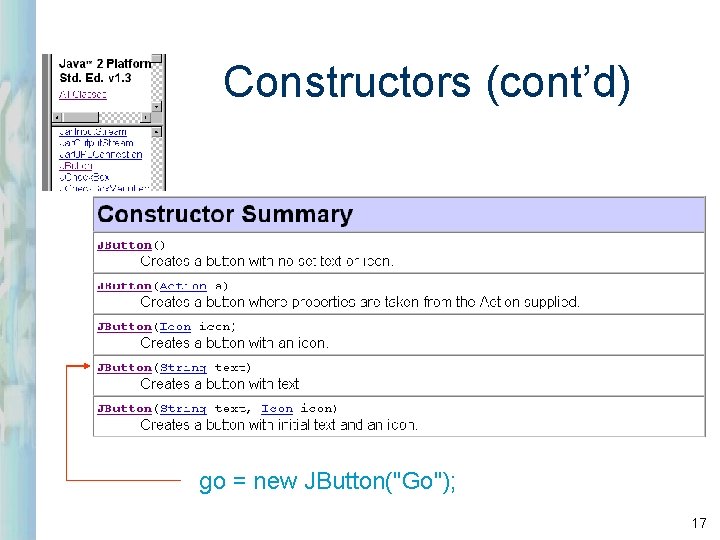
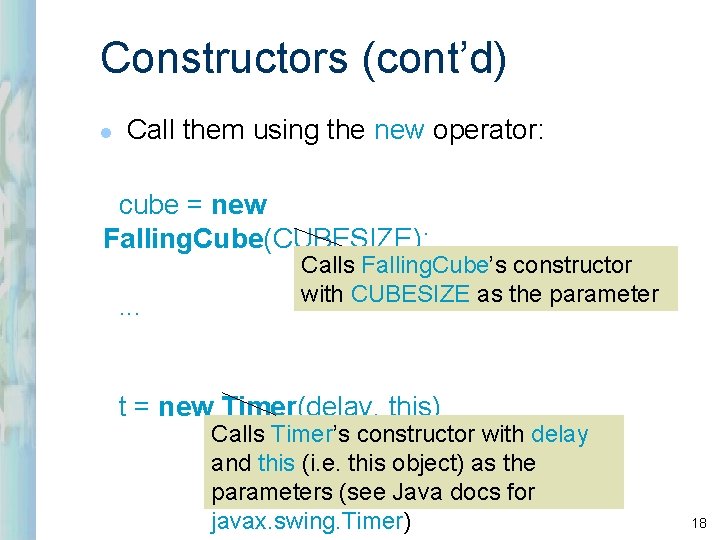
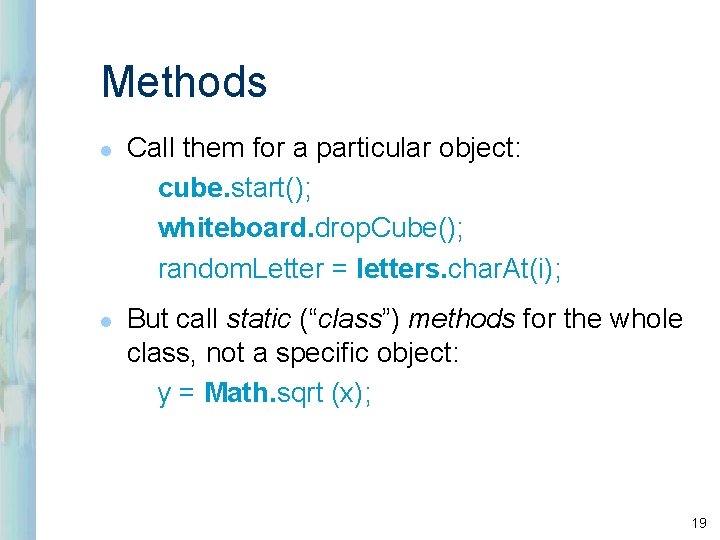
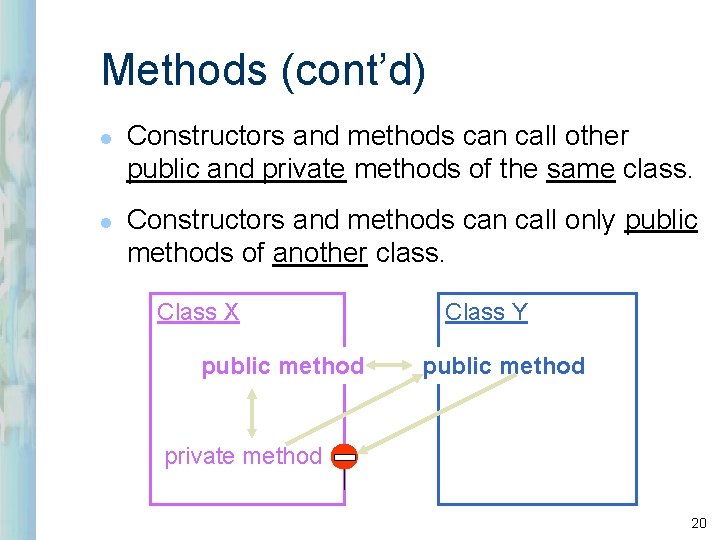
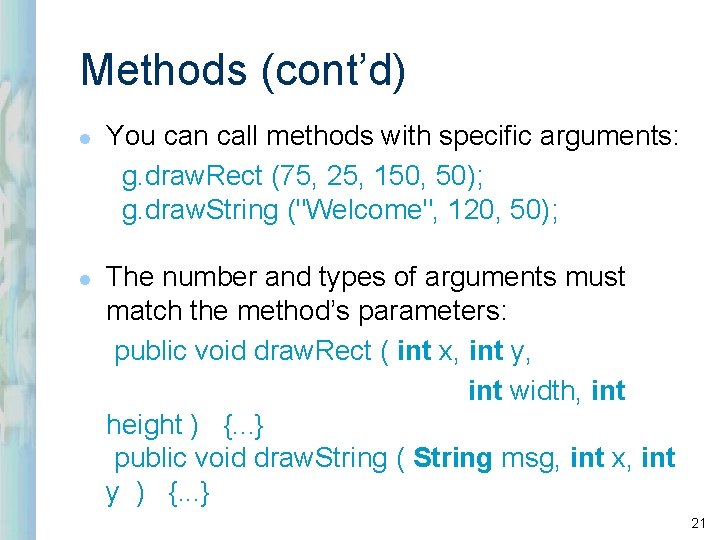
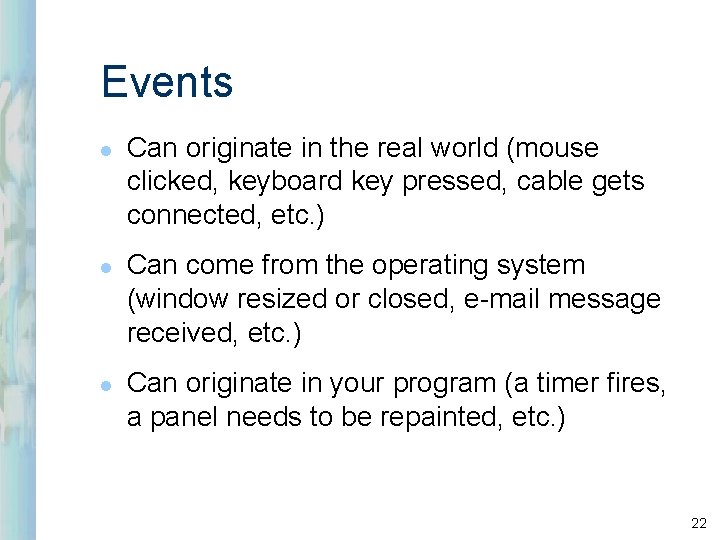
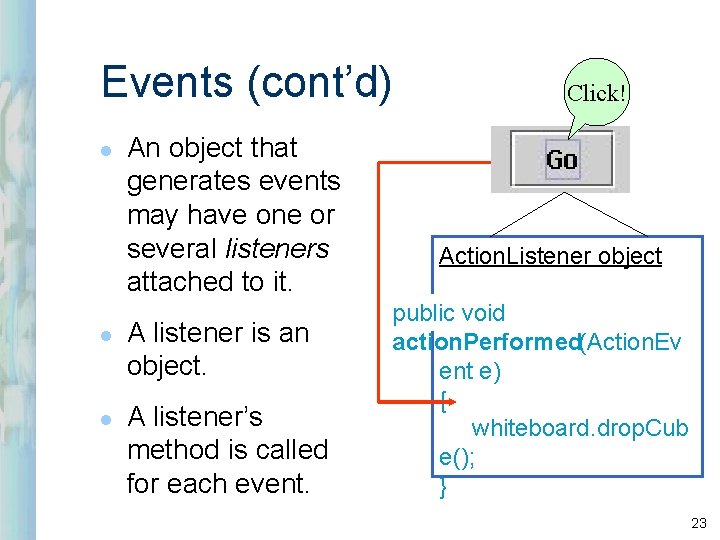
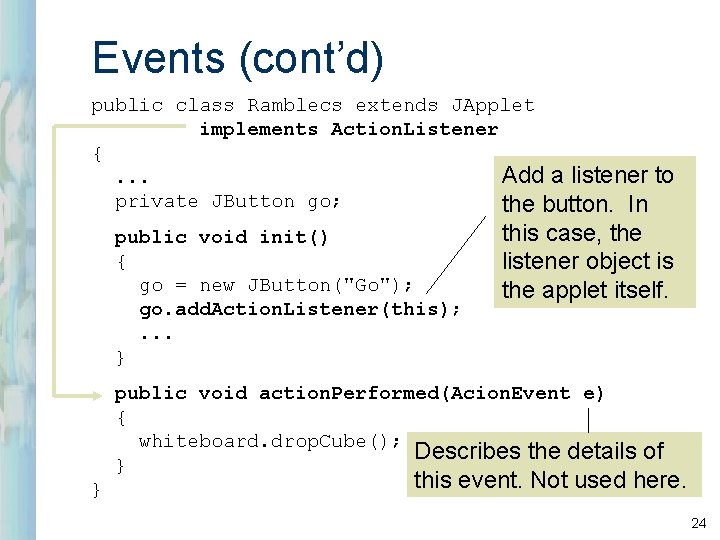
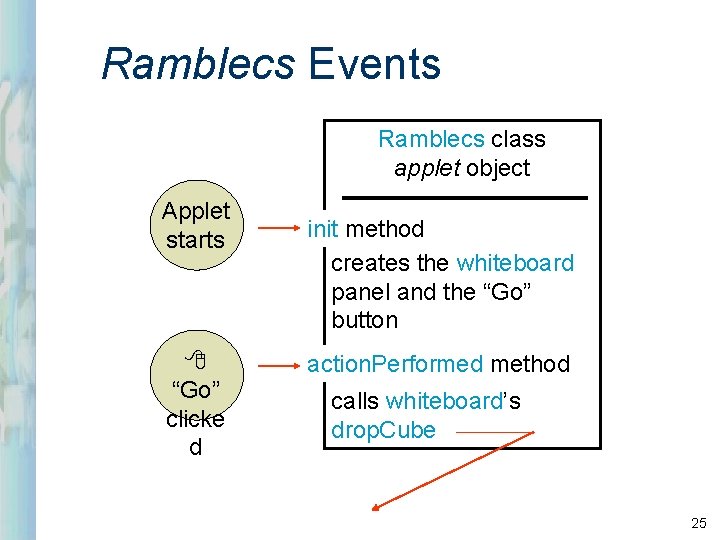
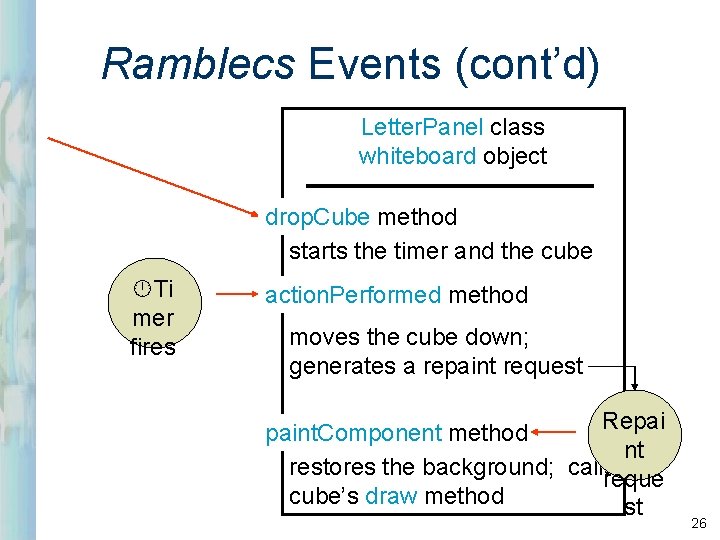
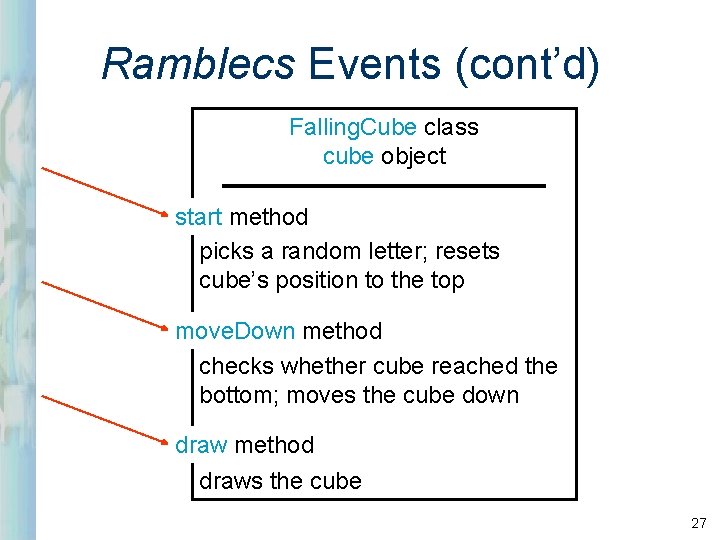
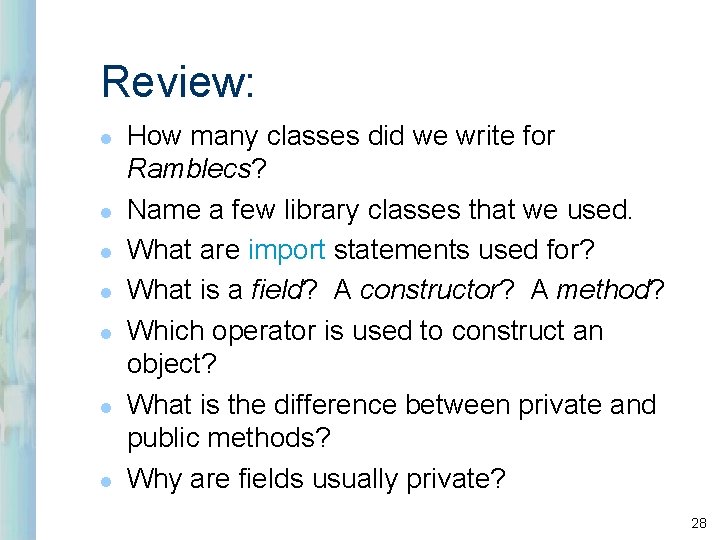
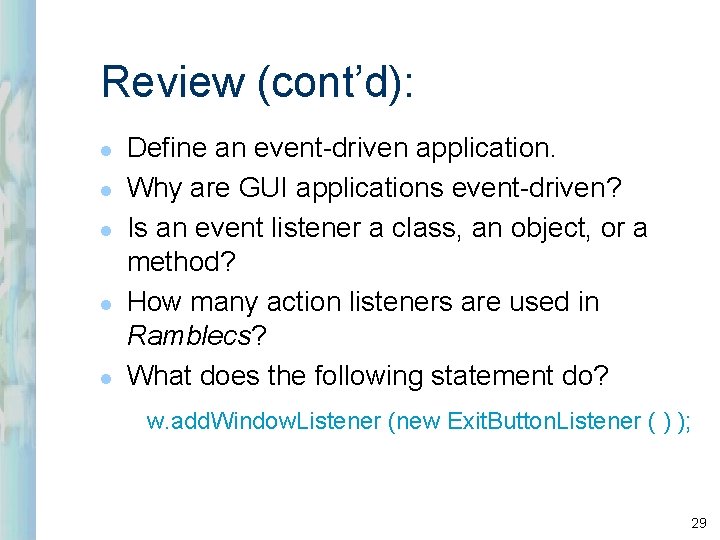
- Slides: 29
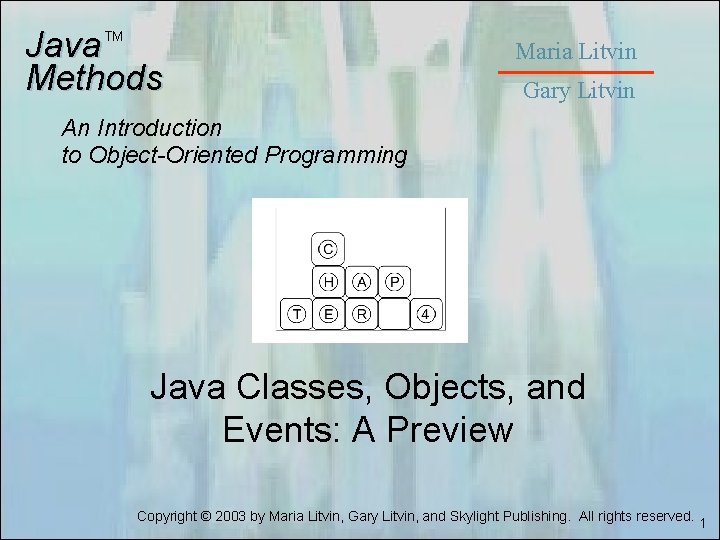
Java Methods TM Maria Litvin Gary Litvin An Introduction to Object-Oriented Programming Java Classes, Objects, and Events: A Preview Copyright © 2003 by Maria Litvin, Gary Litvin, and Skylight Publishing. All rights reserved. 1
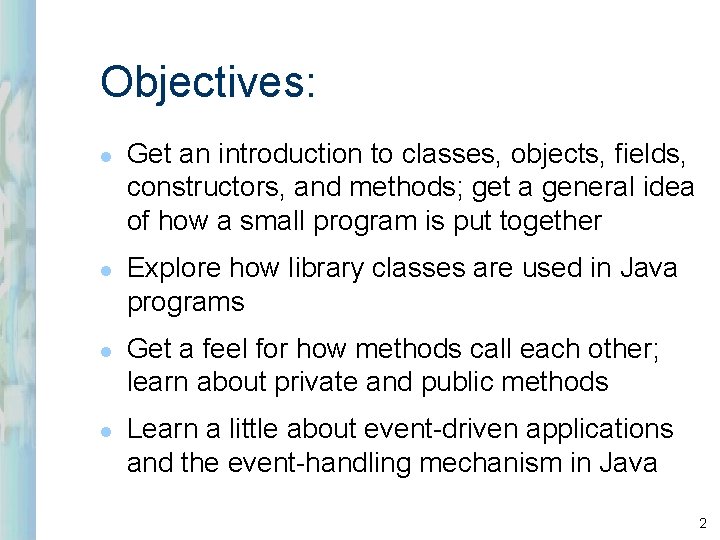
Objectives: l l Get an introduction to classes, objects, fields, constructors, and methods; get a general idea of how a small program is put together Explore how library classes are used in Java programs Get a feel for how methods call each other; learn about private and public methods Learn a little about event-driven applications and the event-handling mechanism in Java 2
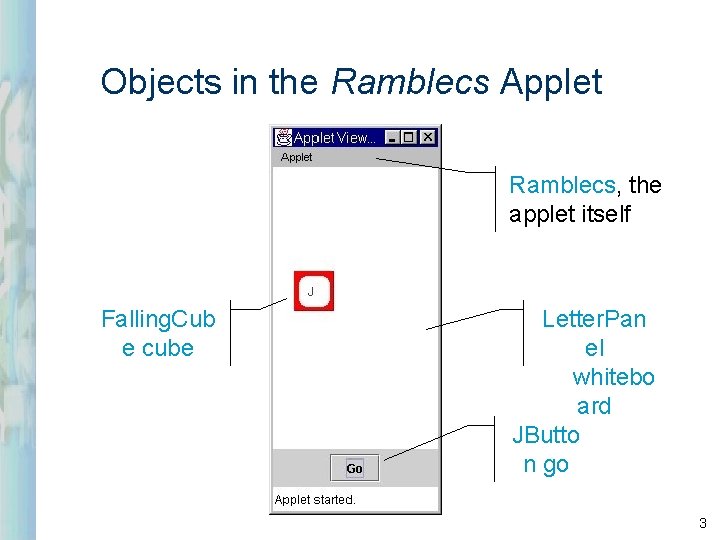
Objects in the Ramblecs Applet Ramblecs, the applet itself Falling. Cub e cube Letter. Pan el whitebo ard JButto n go 3
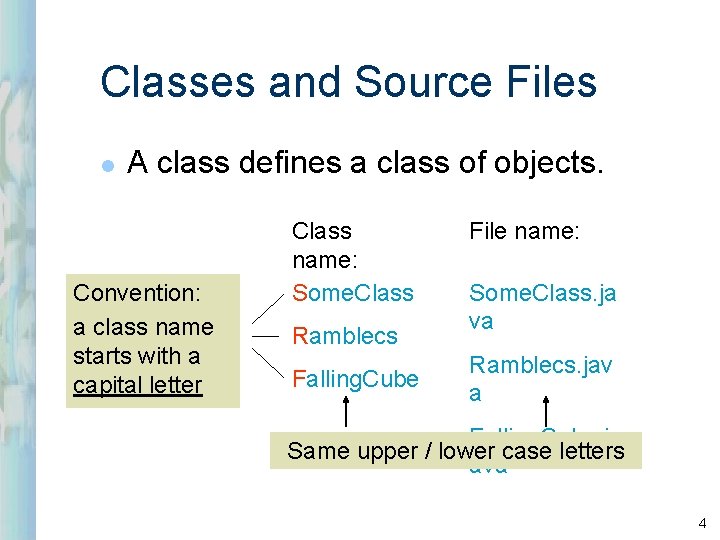
Classes and Source Files l A class defines a class of objects. Convention: a class name starts with a capital letter Class name: Some. Class Ramblecs Falling. Cube File name: Some. Class. ja va Ramblecs. jav a Falling. Cube. j Same upper / lower case letters ava 4
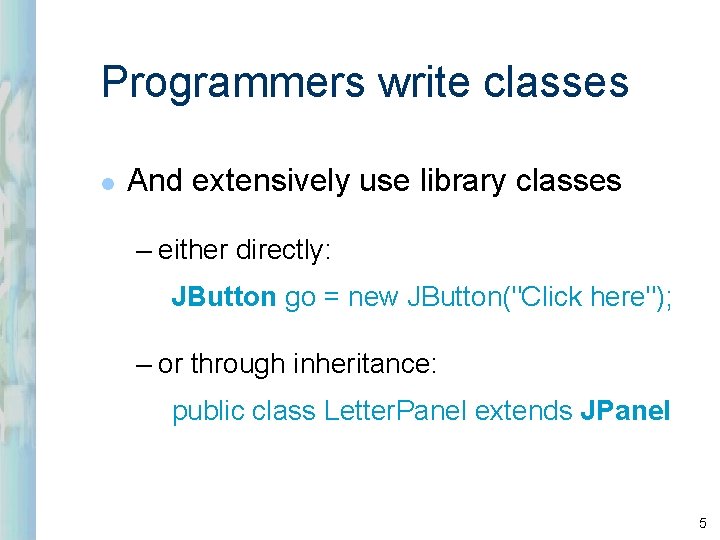
Programmers write classes l And extensively use library classes – either directly: JButton go = new JButton("Click here"); – or through inheritance: public class Letter. Panel extends JPanel 5
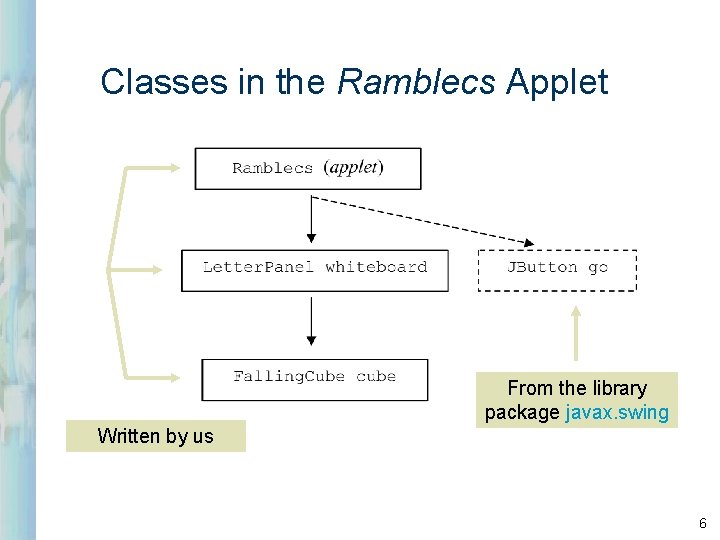
Classes in the Ramblecs Applet From the library package javax. swing Written by us 6
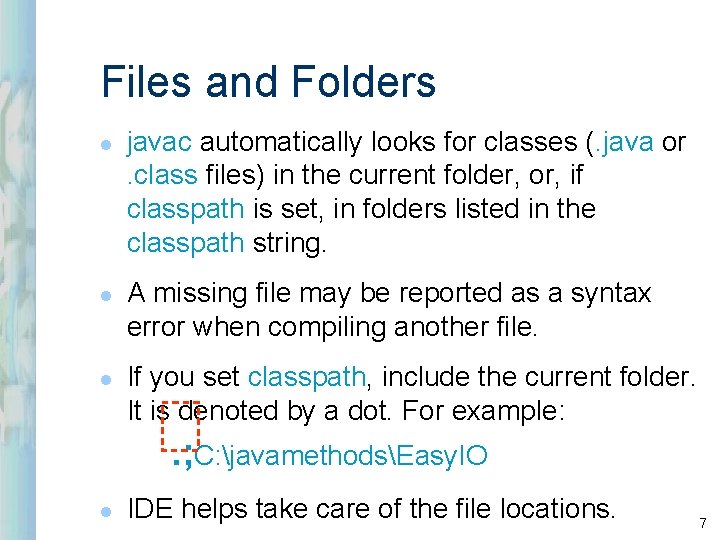
Files and Folders l l l javac automatically looks for classes (. java or. class files) in the current folder, or, if classpath is set, in folders listed in the classpath string. A missing file may be reported as a syntax error when compiling another file. If you set classpath, include the current folder. It is denoted by a dot. For example: . ; C: javamethodsEasy. IO l IDE helps take care of the file locations. 7
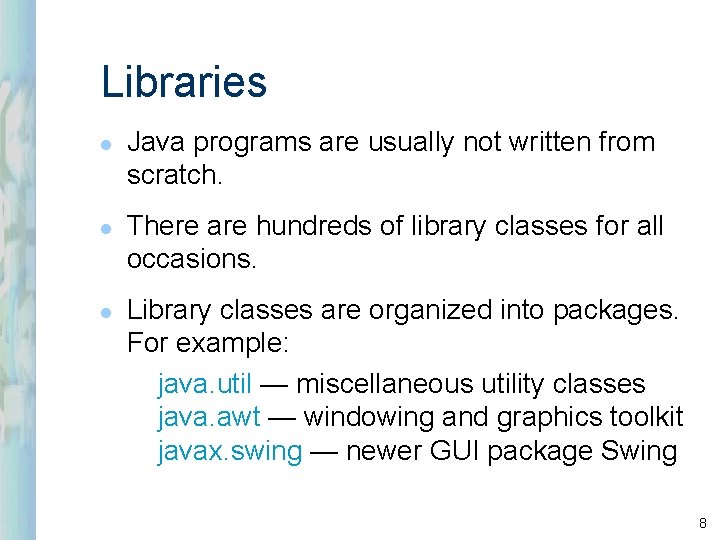
Libraries l l l Java programs are usually not written from scratch. There are hundreds of library classes for all occasions. Library classes are organized into packages. For example: java. util — miscellaneous utility classes java. awt — windowing and graphics toolkit javax. swing — newer GUI package Swing 8
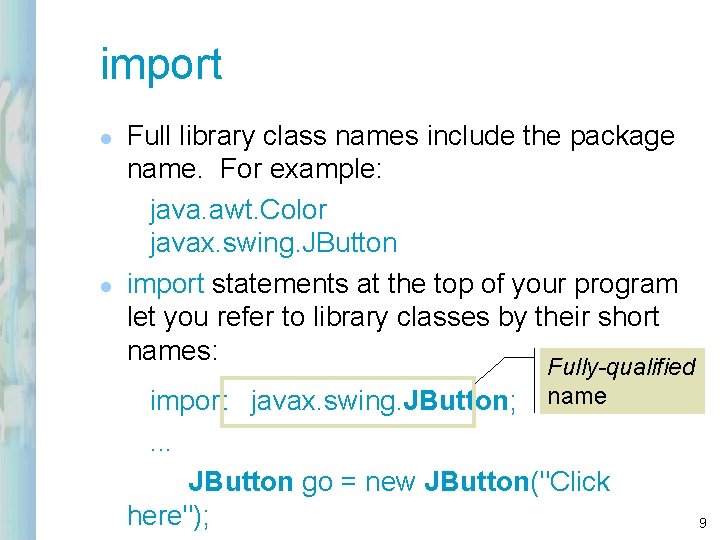
import l l Full library class names include the package name. For example: java. awt. Color javax. swing. JButton import statements at the top of your program let you refer to library classes by their short names: Fully-qualified name import javax. swing. JButton; . . . JButton go = new JButton("Click here"); 9
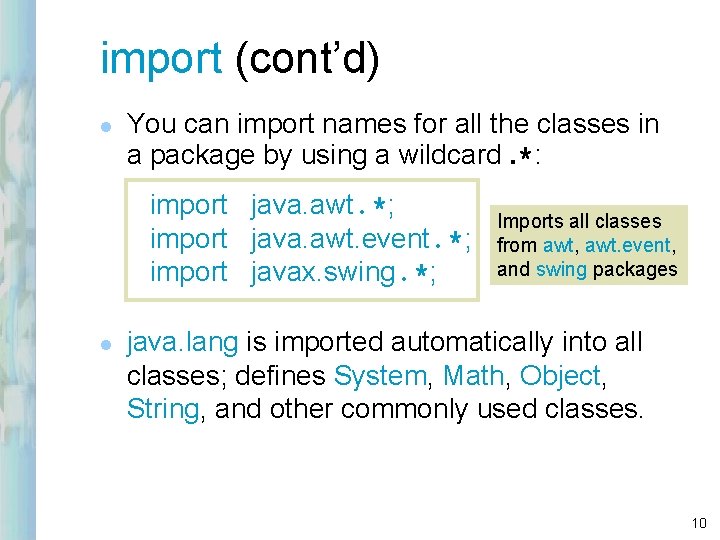
import (cont’d) l You can import names for all the classes in a package by using a wildcard. *: import java. awt. *; import java. awt. event. *; import javax. swing. *; l Imports all classes from awt, awt. event, and swing packages java. lang is imported automatically into all classes; defines System, Math, Object, String, and other commonly used classes. 10
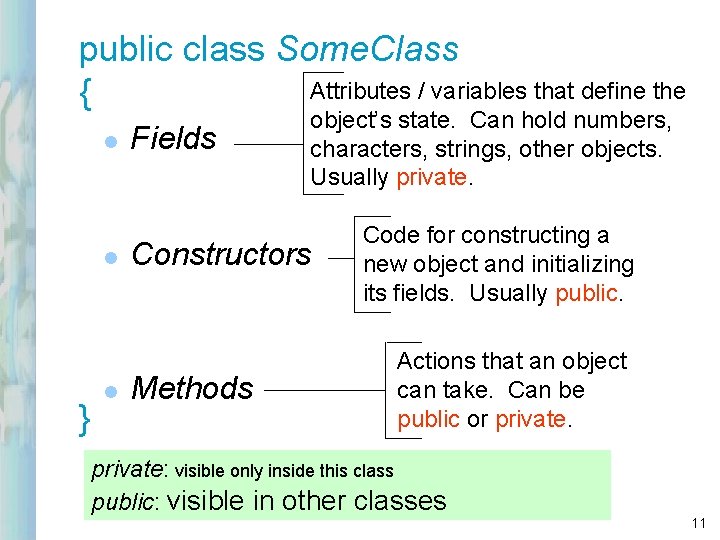
public class Some. Class Attributes / variables that define the { l l } l Fields object’s state. Can hold numbers, characters, strings, other objects. Usually private. Constructors Methods Code for constructing a new object and initializing its fields. Usually public. Actions that an object can take. Can be public or private: visible only inside this class public: visible in other classes 11
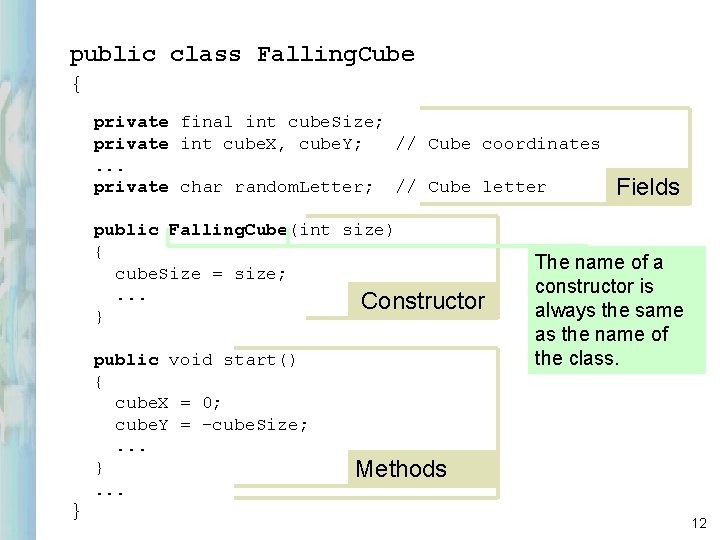
public class Falling. Cube { private final int cube. Size; private int cube. X, cube. Y; // Cube coordinates. . . private char random. Letter; // Cube letter public Falling. Cube(int size) { cube. Size = size; . . . Constructor } } public void start() { cube. X = 0; cube. Y = -cube. Size; . . . }. . . Fields The name of a constructor is always the same as the name of the class. Methods 12
![Fields You name it private or public static final Usually privatename May be present Fields You name it! private (or public) [static] [final] Usually privatename; May be present:](https://slidetodoc.com/presentation_image_h/17944643f8adf08eefe97ed7d2b57c08/image-13.jpg)
Fields You name it! private (or public) [static] [final] Usually privatename; May be present: means the field is shared by all objects in the class datatype May be present: means the field is a constant int, double, etc. , or an object: String, JButton, Falling. Cube, Timer 13
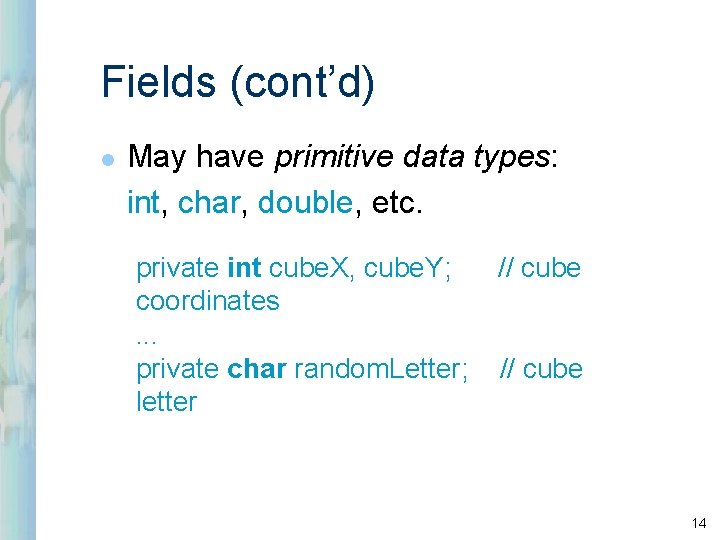
Fields (cont’d) l May have primitive data types: int, char, double, etc. private int cube. X, cube. Y; coordinates. . . private char random. Letter; letter // cube 14
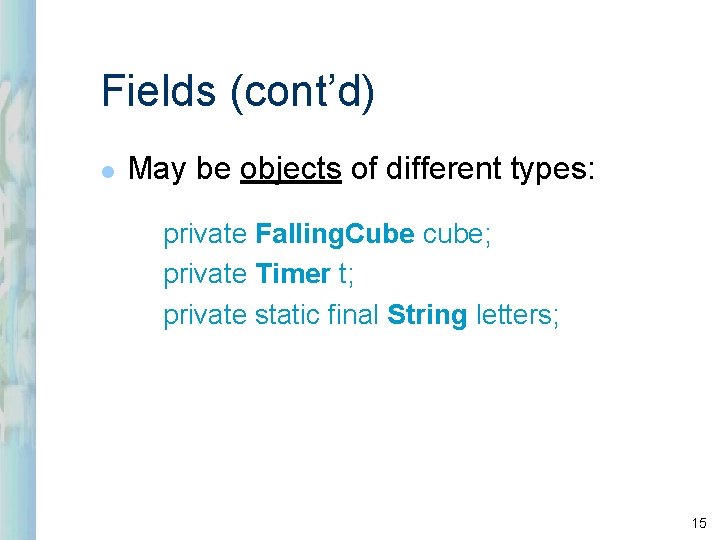
Fields (cont’d) l May be objects of different types: private Falling. Cube cube; private Timer t; private static final String letters; 15
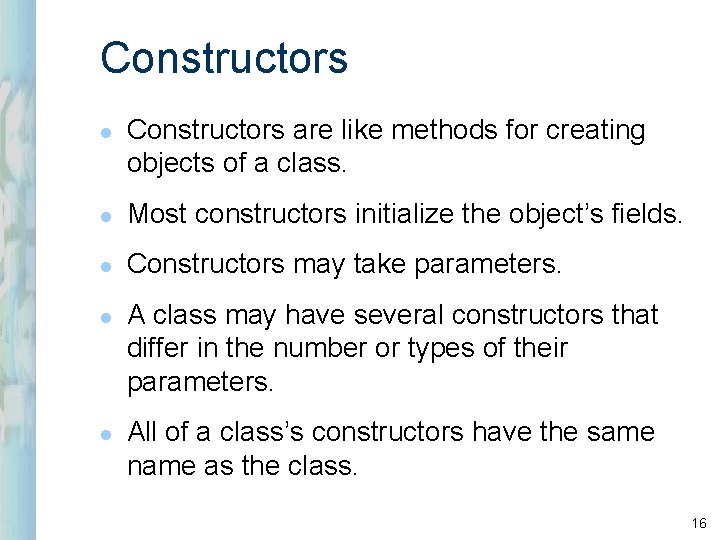
Constructors l Constructors are like methods for creating objects of a class. l Most constructors initialize the object’s fields. l Constructors may take parameters. l l A class may have several constructors that differ in the number or types of their parameters. All of a class’s constructors have the same name as the class. 16
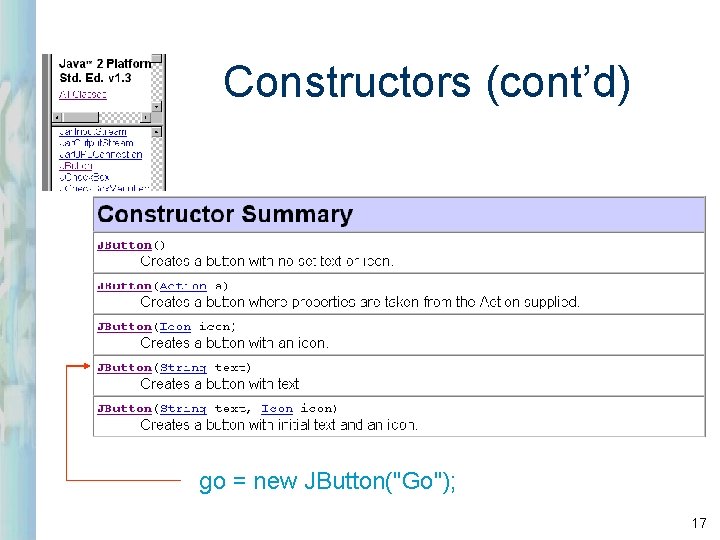
Constructors (cont’d) go = new JButton("Go"); 17
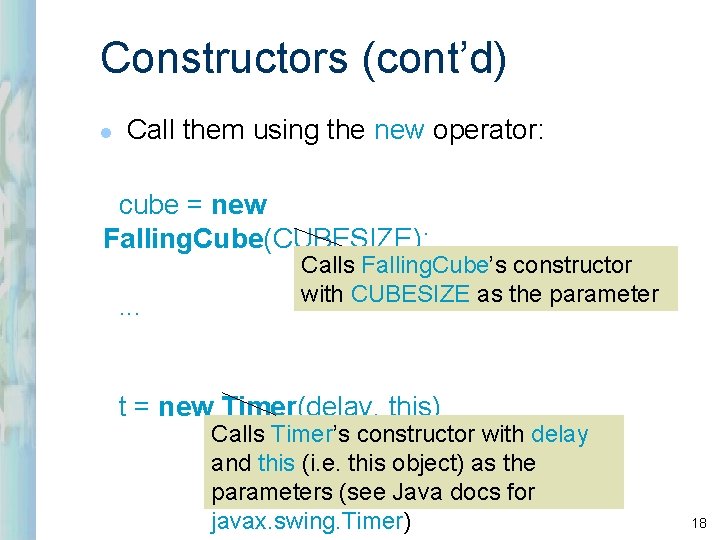
Constructors (cont’d) l Call them using the new operator: cube = new Falling. Cube(CUBESIZE); . . . Calls Falling. Cube’s constructor with CUBESIZE as the parameter t = new Timer(delay, this) Calls Timer’s constructor with delay and this (i. e. this object) as the parameters (see Java docs for javax. swing. Timer) 18
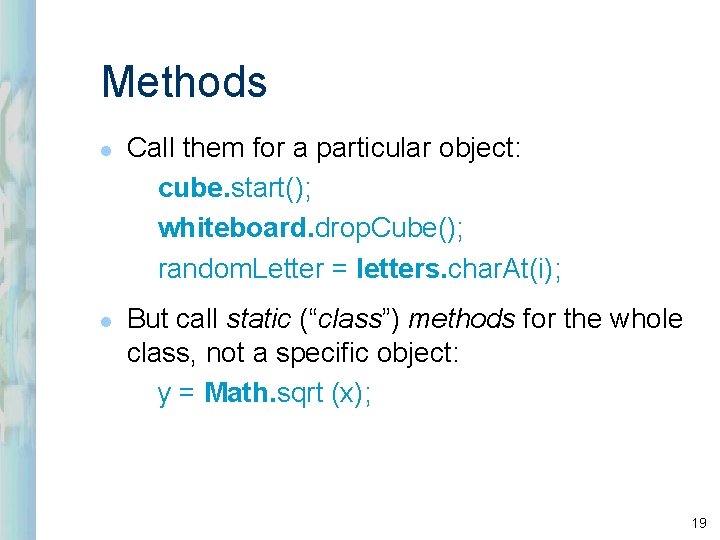
Methods l l Call them for a particular object: cube. start(); whiteboard. drop. Cube(); random. Letter = letters. char. At(i); But call static (“class”) methods for the whole class, not a specific object: y = Math. sqrt (x); 19
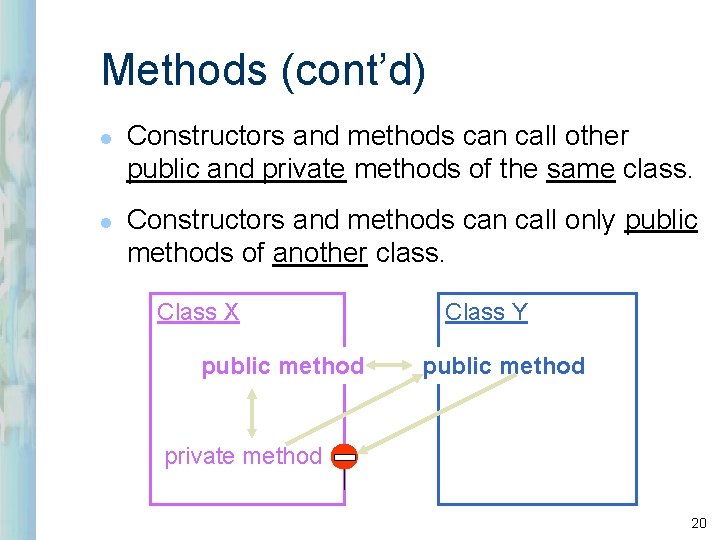
Methods (cont’d) l l Constructors and methods can call other public and private methods of the same class. Constructors and methods can call only public methods of another class. Class X public method Class Y public method private method 20
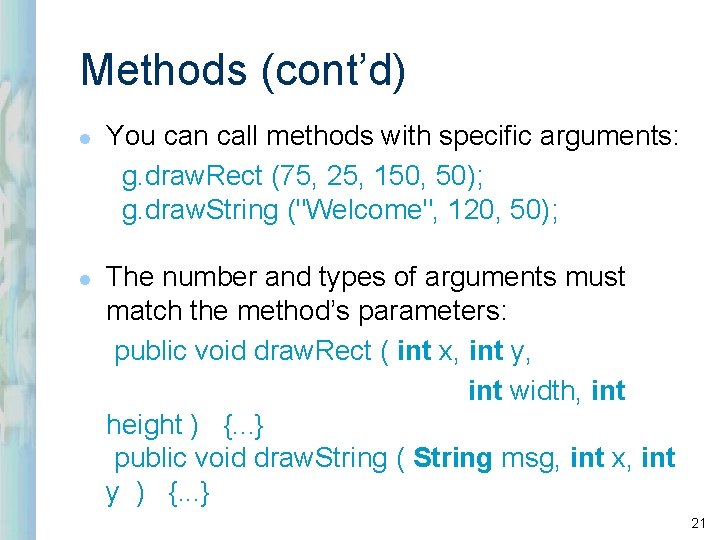
Methods (cont’d) l l You can call methods with specific arguments: g. draw. Rect (75, 25, 150, 50); g. draw. String ("Welcome", 120, 50); The number and types of arguments must match the method’s parameters: public void draw. Rect ( int x, int y, int width, int height ) {. . . } public void draw. String ( String msg, int x, int y ) {. . . } 21
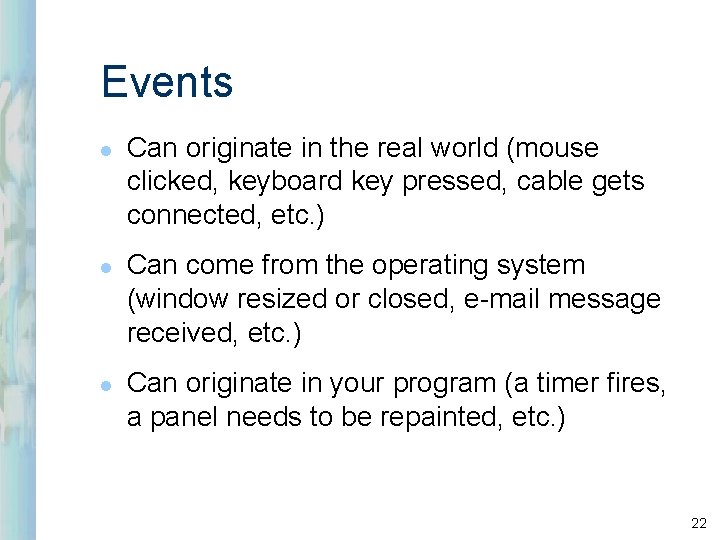
Events l l l Can originate in the real world (mouse clicked, keyboard key pressed, cable gets connected, etc. ) Can come from the operating system (window resized or closed, e-mail message received, etc. ) Can originate in your program (a timer fires, a panel needs to be repainted, etc. ) 22
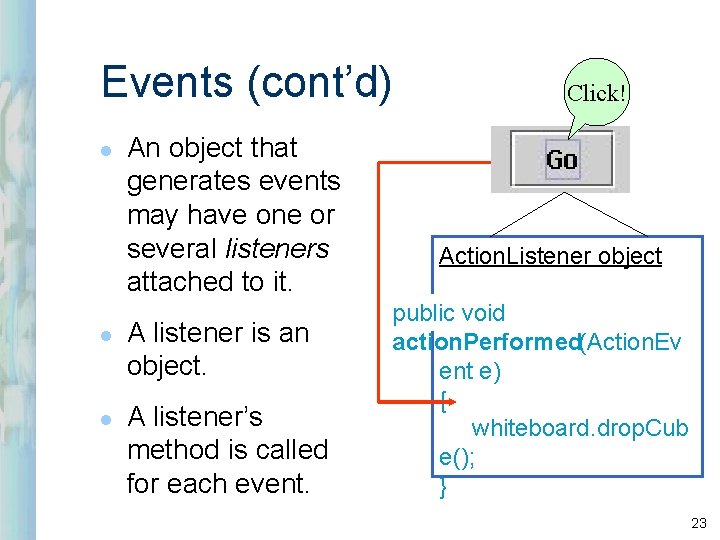
Events (cont’d) l l l An object that generates events may have one or several listeners attached to it. A listener is an object. A listener’s method is called for each event. Click! Action. Listener object public void action. Performed(Action. Ev ent e) { whiteboard. drop. Cub e(); } 23
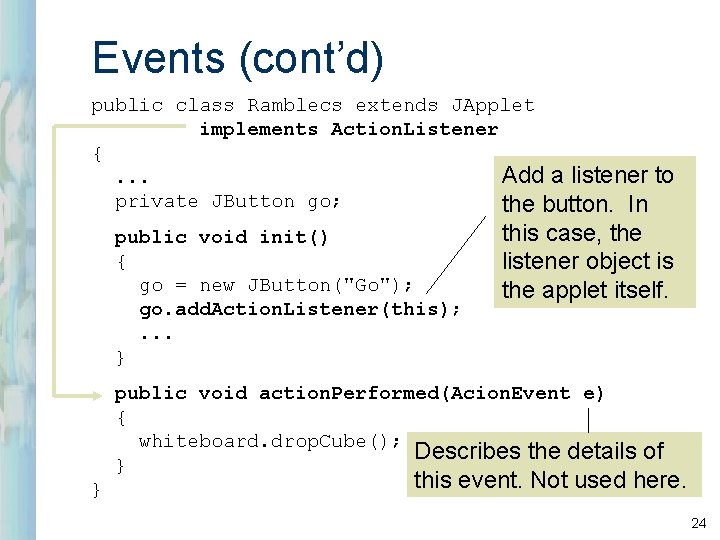
Events (cont’d) public class Ramblecs extends JApplet implements Action. Listener { Add a listener. . . private JButton go; the button. In public void init() { go = new JButton("Go"); go. add. Action. Listener(this); . . . } to this case, the listener object is the applet itself. public void action. Performed(Acion. Event e) { whiteboard. drop. Cube(); Describes the details } } of this event. Not used here. 24
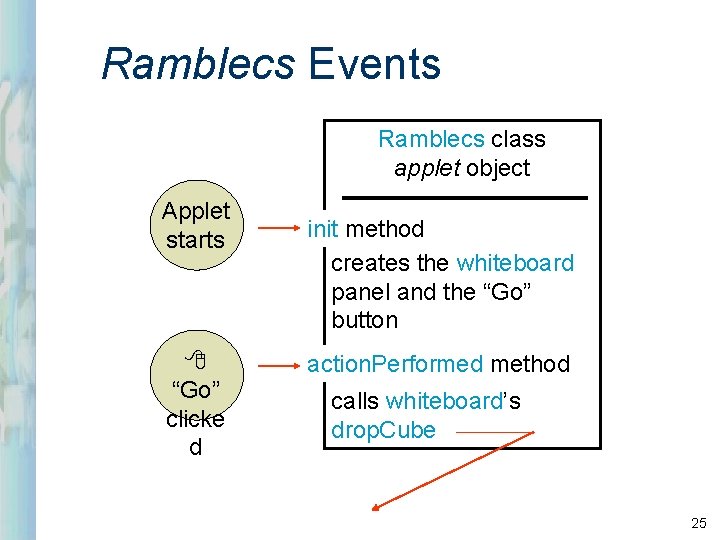
Ramblecs Events Ramblecs class applet object Applet starts “Go” clicke d init method creates the whiteboard panel and the “Go” button action. Performed method calls whiteboard’s drop. Cube 25
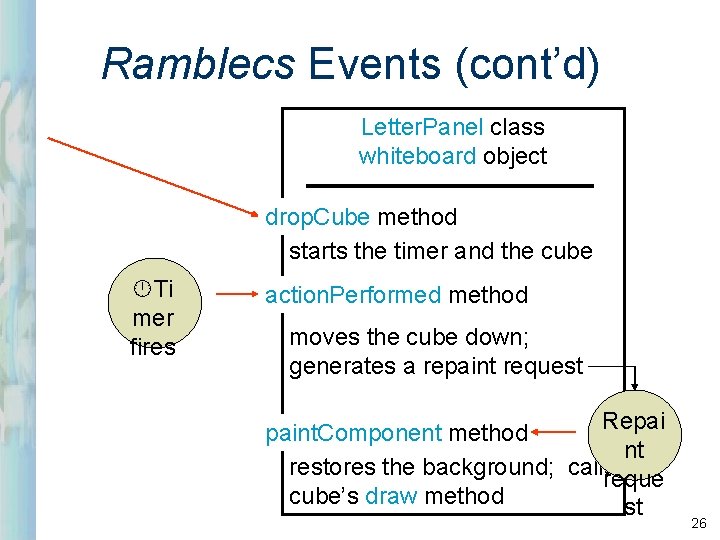
Ramblecs Events (cont’d) Letter. Panel class whiteboard object drop. Cube method starts the timer and the cube Ti mer fires action. Performed method moves the cube down; generates a repaint request Repai paint. Component method nt restores the background; calls reque cube’s draw method st 26
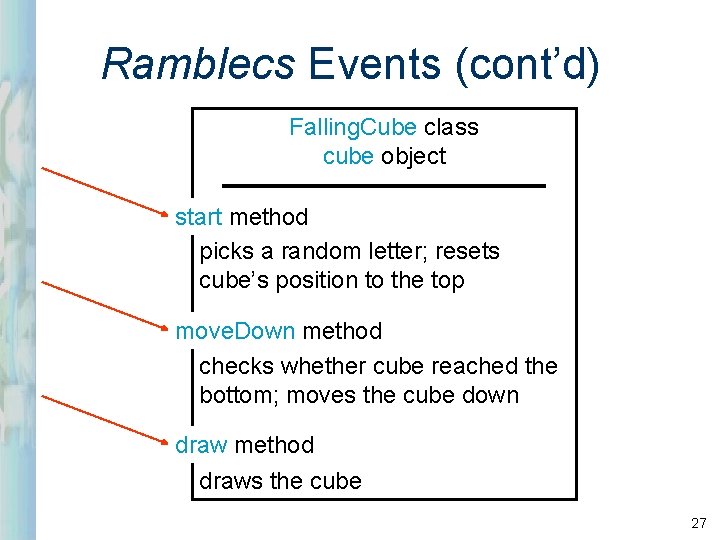
Ramblecs Events (cont’d) Falling. Cube class cube object start method picks a random letter; resets cube’s position to the top move. Down method checks whether cube reached the bottom; moves the cube down draw method draws the cube 27
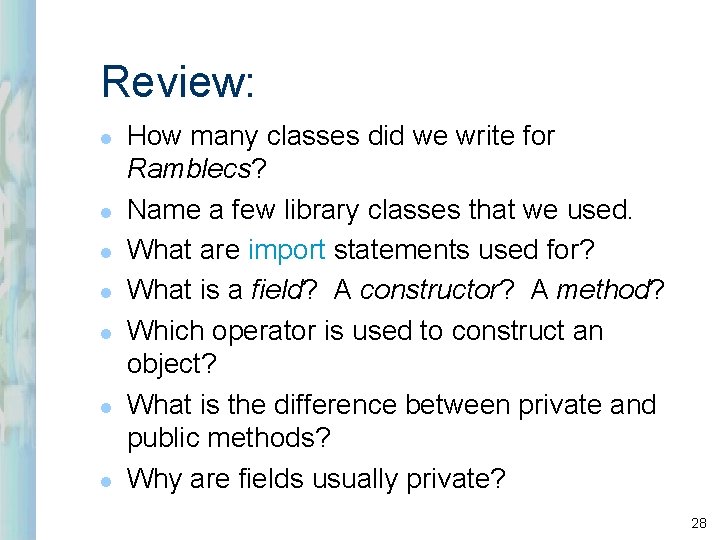
Review: l l l l How many classes did we write for Ramblecs? Name a few library classes that we used. What are import statements used for? What is a field? A constructor? A method? Which operator is used to construct an object? What is the difference between private and public methods? Why are fields usually private? 28
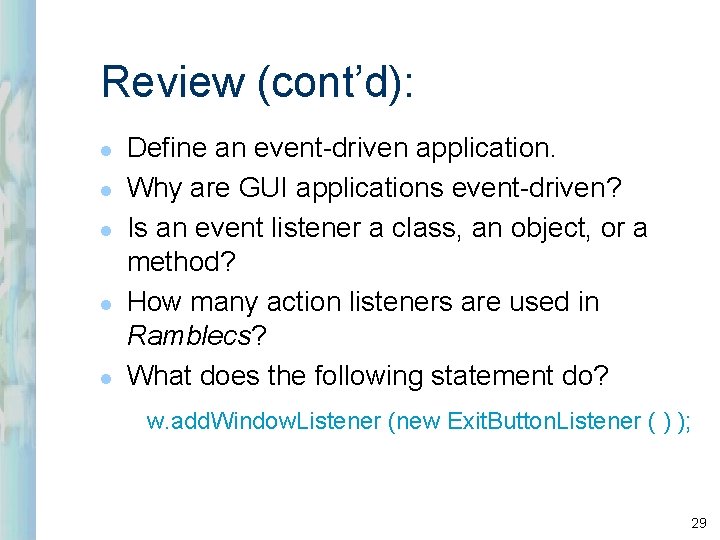
Review (cont’d): l l l Define an event-driven application. Why are GUI applications event-driven? Is an event listener a class, an object, or a method? How many action listeners are used in Ramblecs? What does the following statement do? w. add. Window. Listener (new Exit. Button. Listener ( ) ); 29
Gary litvin
Gary litvin
Root node
Gary litvin
Maria litvin
Wax pattern fabrication
Maria felicia de jesús sacramentado maría teresa arminda
Mutator and accessor methods in java
Mutator and accessor methods in java
Mutator and accessor methods in java
Java string methods
User defined functions in java
Method signature consists of
Java string methods
Method
Import java.util.*
Java import java.util.*
Import java.awt.* import java.applet.*
Import.java.util.scanner;
Import java.io.* import java.util.*
Gcd java
Java random
Import java.io.* in java
Public class
Java import java.io.*
Perbedaan antara java swing dan awt adalah
Import java.awt.* import java.awt.event.*
Language
Rmi javatpoint
Gary fish maine