Methods and Parameters in Java Methods in Java
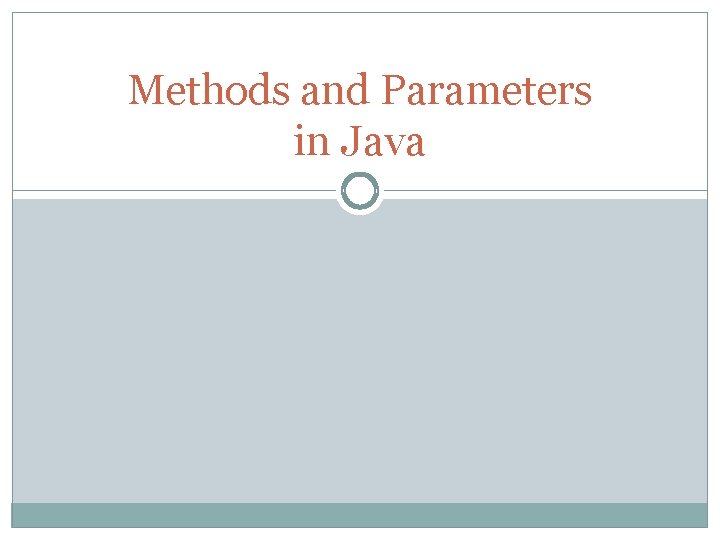
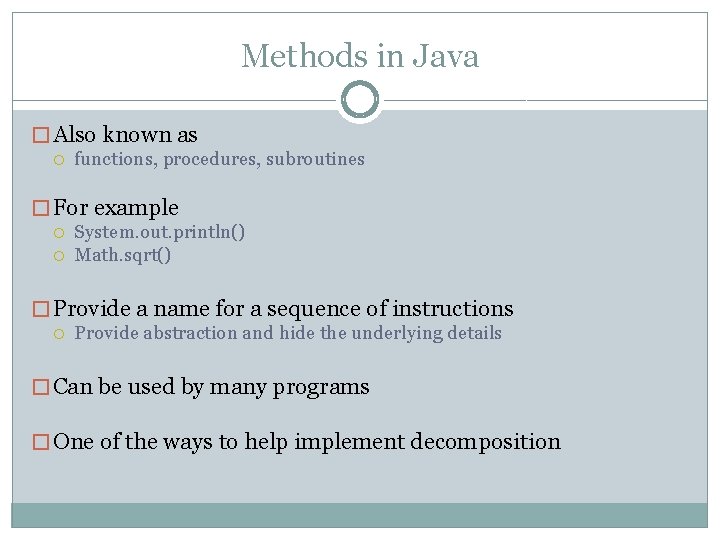
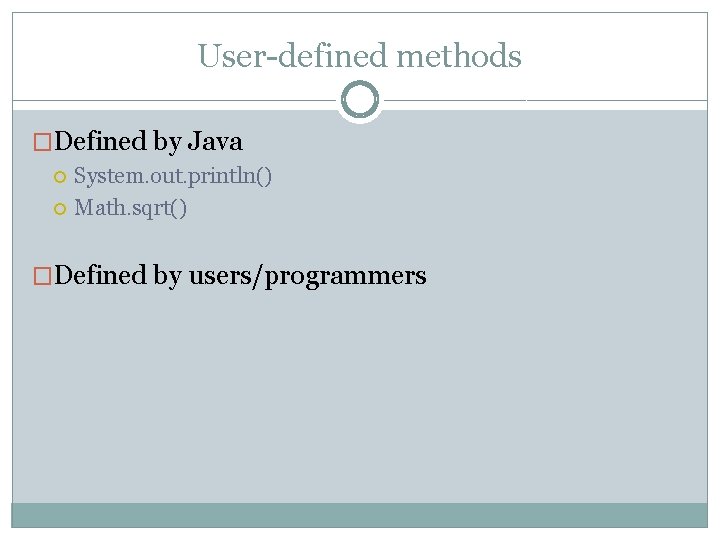
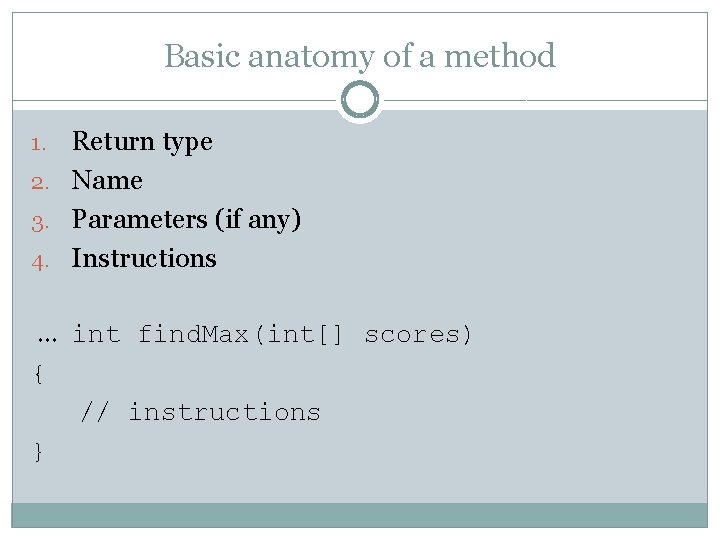
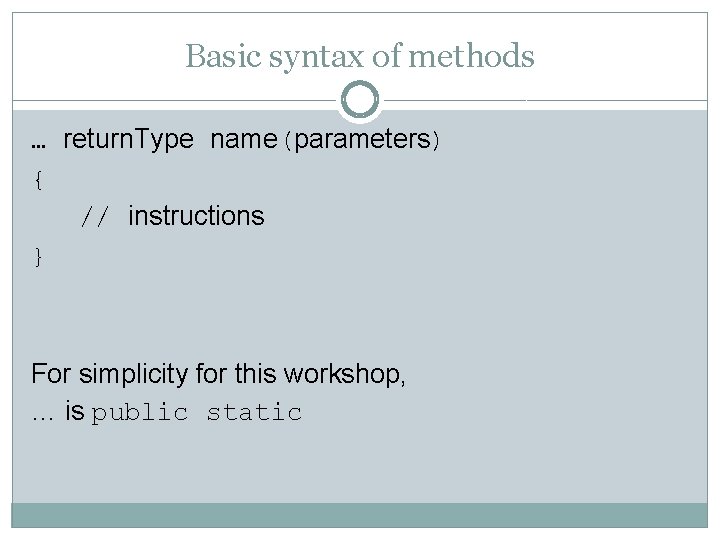
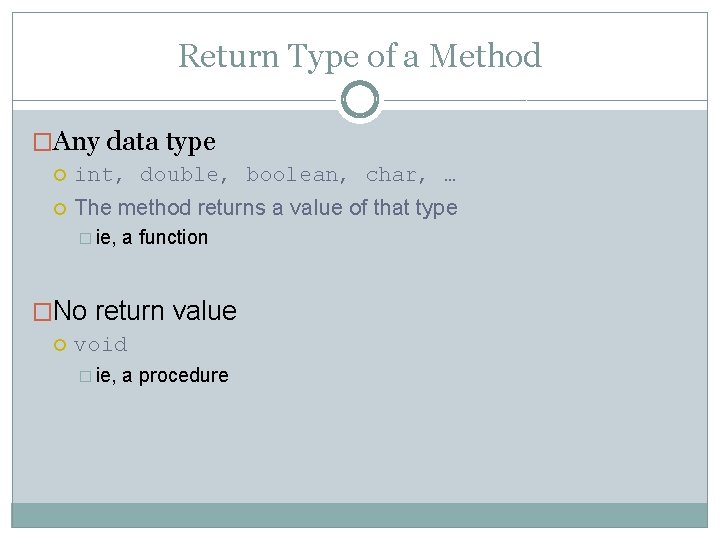
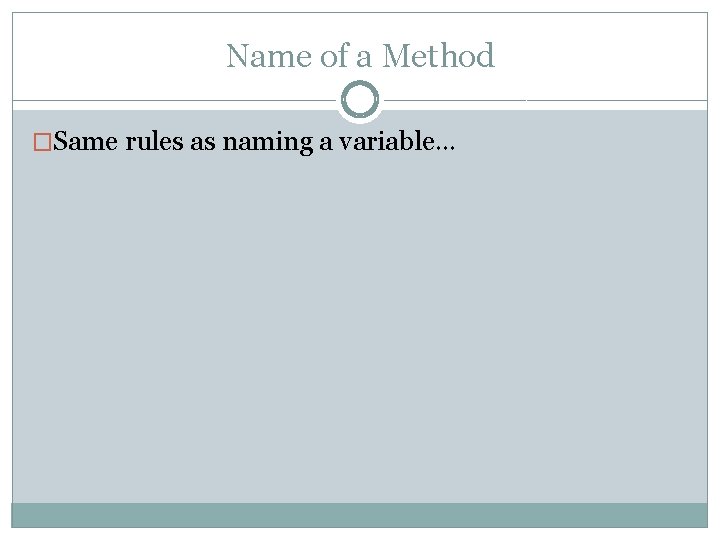
![Parameters of a Method �Type and name E. g. int find. Max(int[] scores) �More Parameters of a Method �Type and name E. g. int find. Max(int[] scores) �More](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-8.jpg)
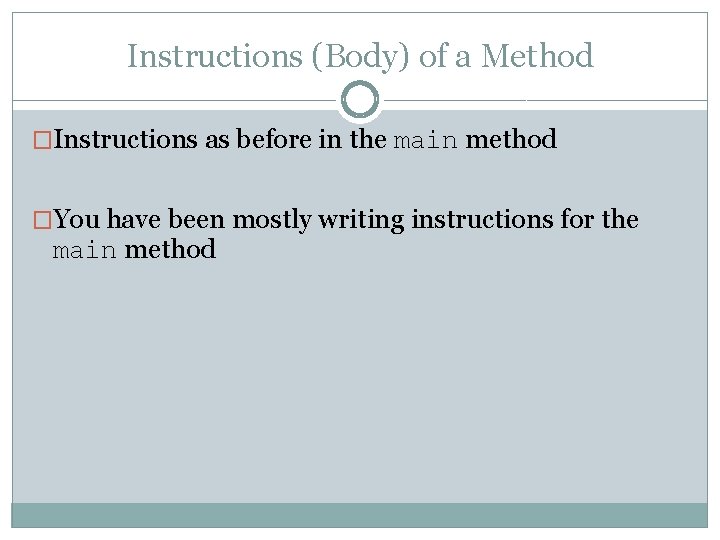
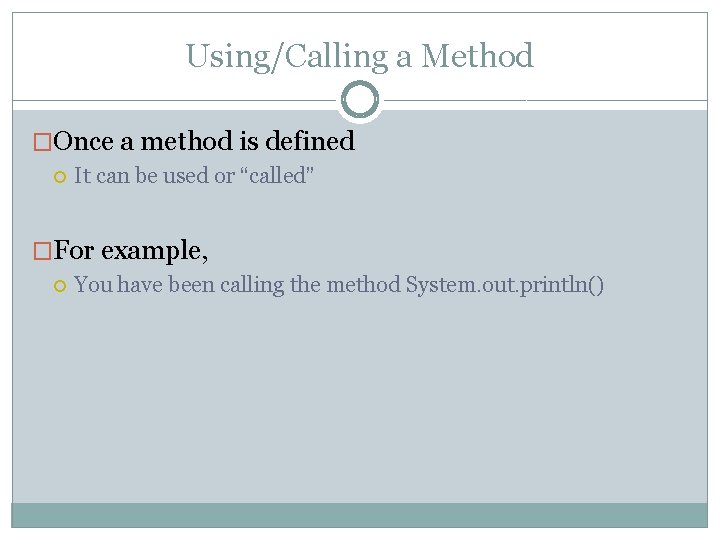
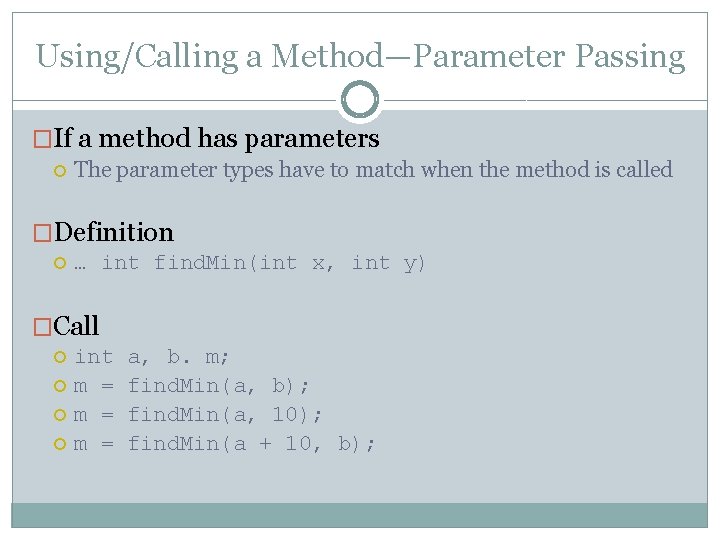
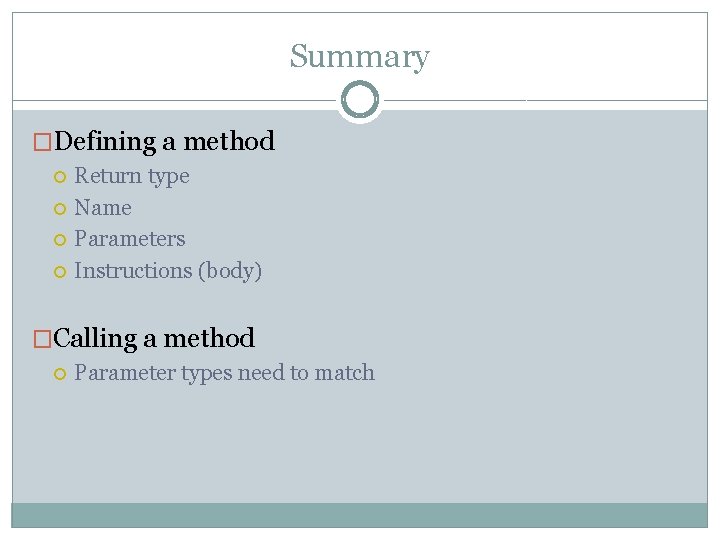
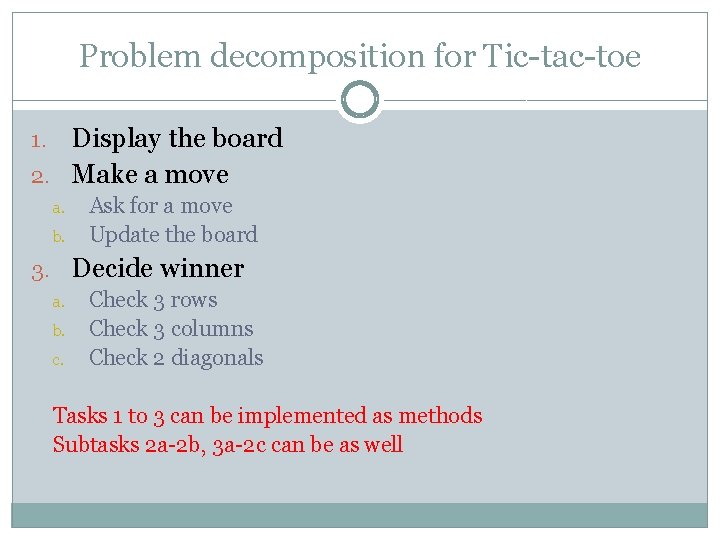
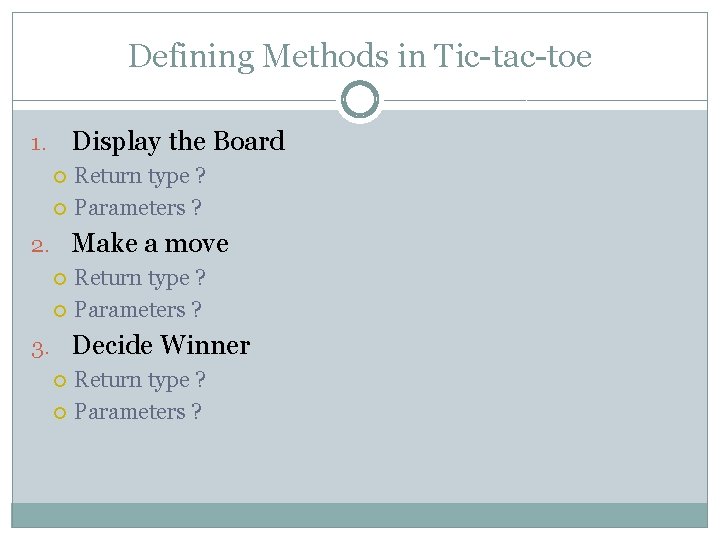
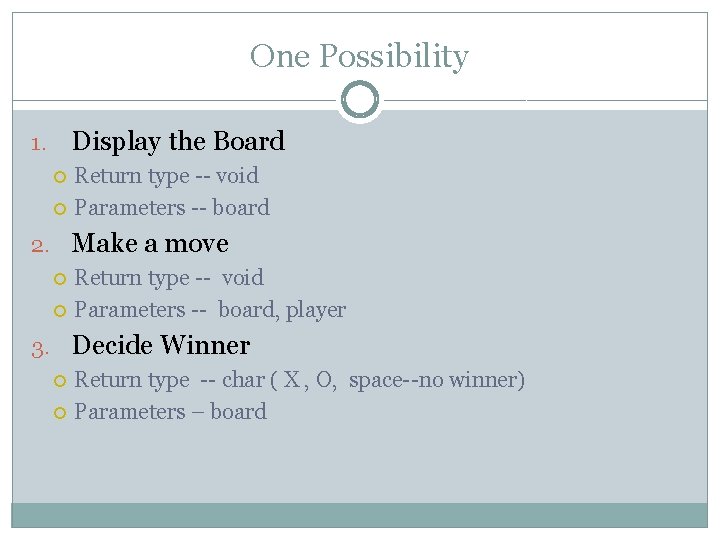
![Main method—first level in decomposition … main(…) { char[][] board = new char[3][3]; char Main method—first level in decomposition … main(…) { char[][] board = new char[3][3]; char](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-16.jpg)
![Main method—Refining it … main(…) { char[][] board = new char[3][3]; char player = Main method—Refining it … main(…) { char[][] board = new char[3][3]; char player =](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-17.jpg)
![Main method (high-level & readable) … main(…) { char[][] board = new char[3][3]; char Main method (high-level & readable) … main(…) { char[][] board = new char[3][3]; char](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-18.jpg)
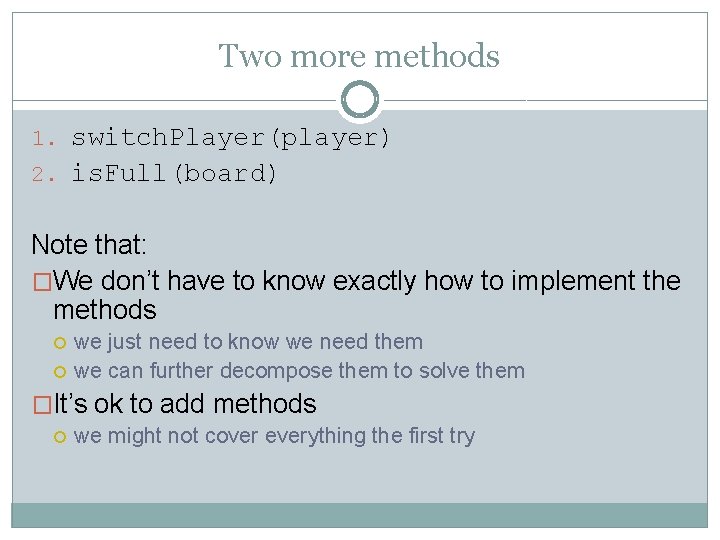
- Slides: 19
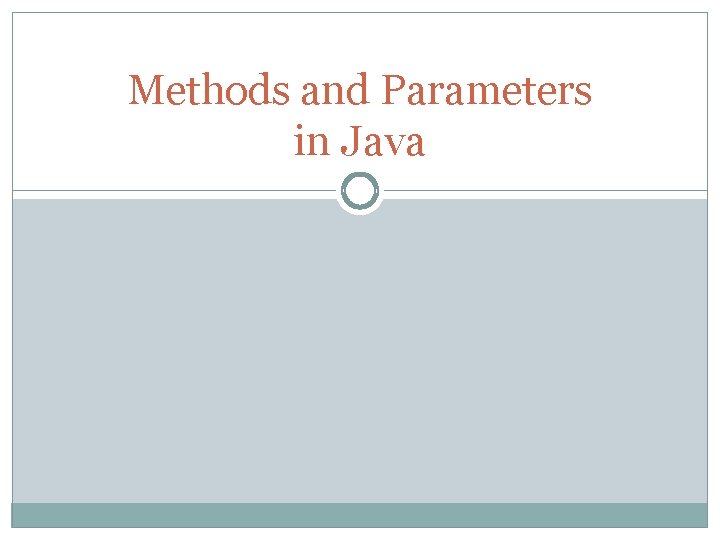
Methods and Parameters in Java
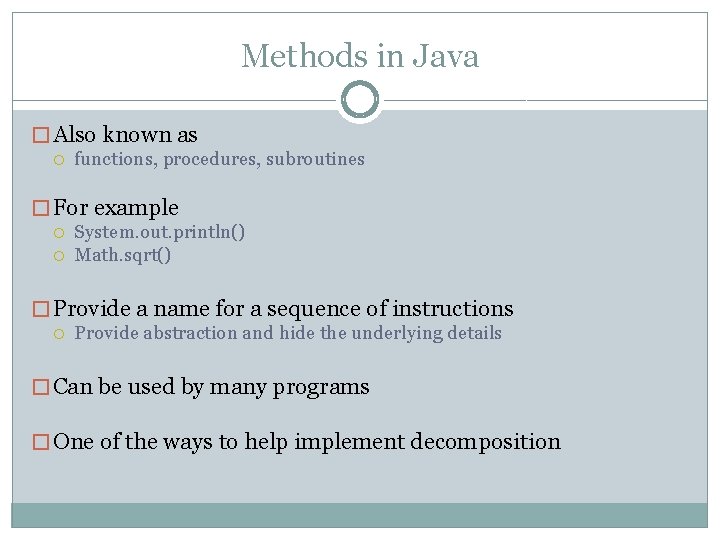
Methods in Java � Also known as functions, procedures, subroutines � For example System. out. println() Math. sqrt() � Provide a name for a sequence of instructions Provide abstraction and hide the underlying details � Can be used by many programs � One of the ways to help implement decomposition
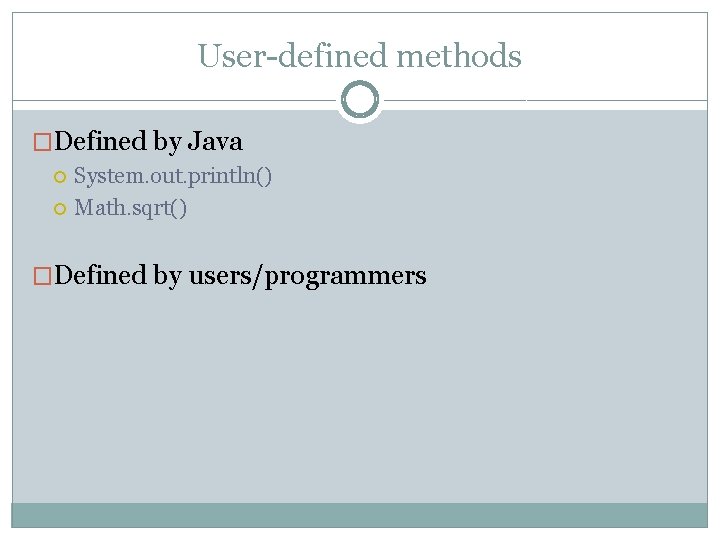
User-defined methods �Defined by Java System. out. println() Math. sqrt() �Defined by users/programmers
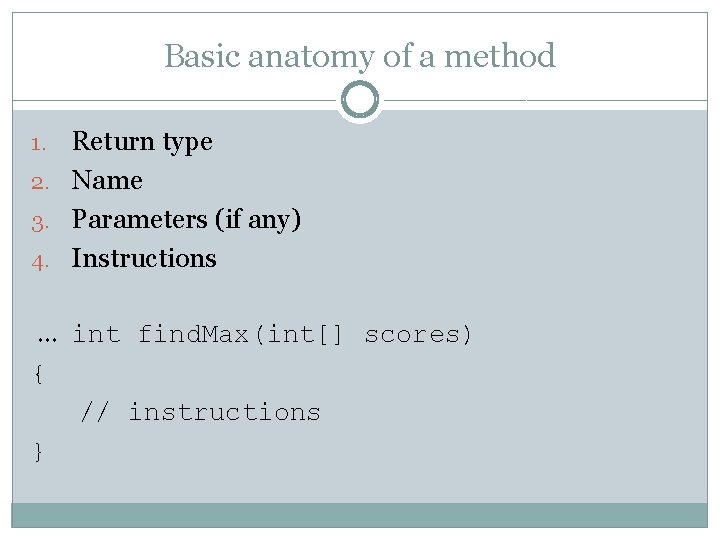
Basic anatomy of a method Return type 2. Name 3. Parameters (if any) 4. Instructions 1. … int find. Max(int[] scores) { // instructions }
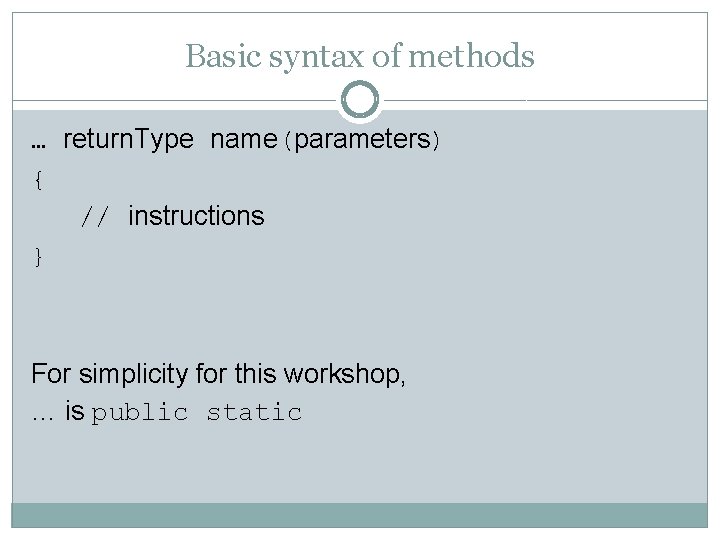
Basic syntax of methods … return. Type name(parameters) { // instructions } For simplicity for this workshop, … is public static
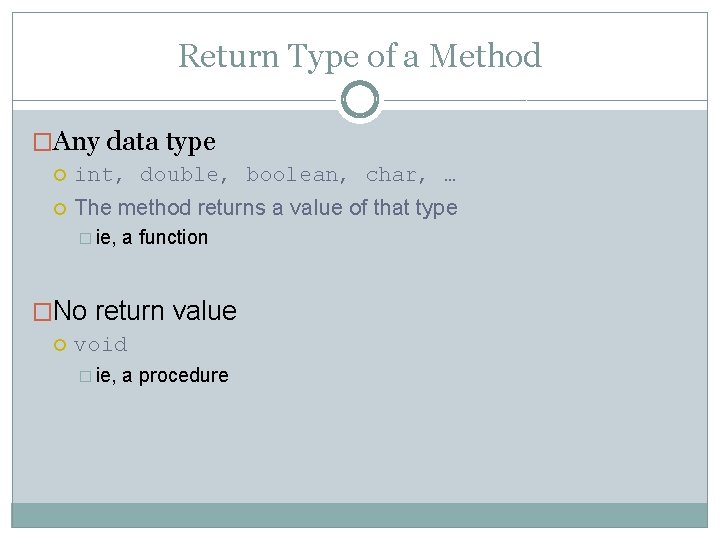
Return Type of a Method �Any data type int, double, boolean, char, … The method returns a value of that type � ie, a function �No return value void � ie, a procedure
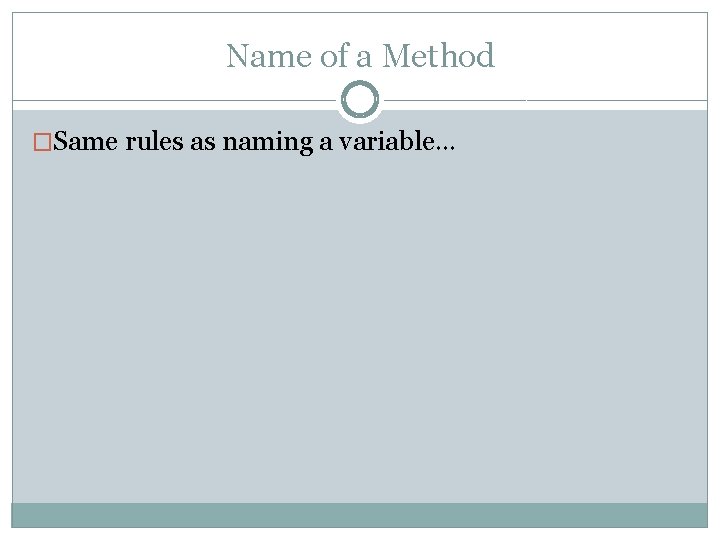
Name of a Method �Same rules as naming a variable…
![Parameters of a Method Type and name E g int find Maxint scores More Parameters of a Method �Type and name E. g. int find. Max(int[] scores) �More](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-8.jpg)
Parameters of a Method �Type and name E. g. int find. Max(int[] scores) �More than one parameter E. g. int find. Min(int x, int y)
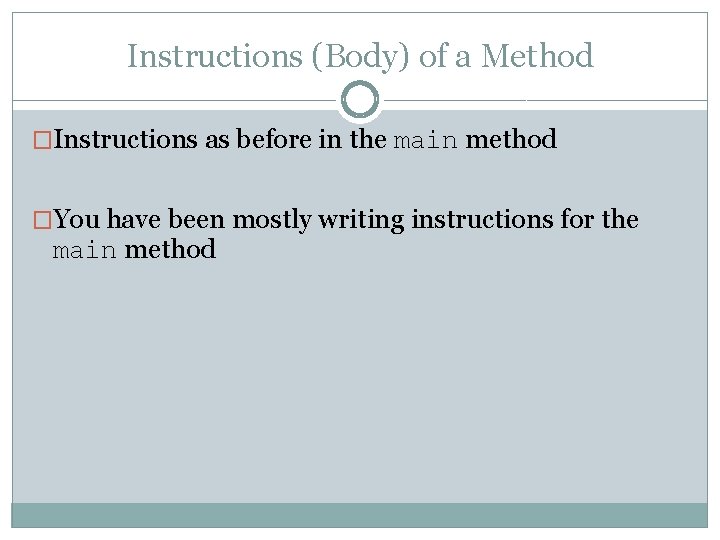
Instructions (Body) of a Method �Instructions as before in the main method �You have been mostly writing instructions for the main method
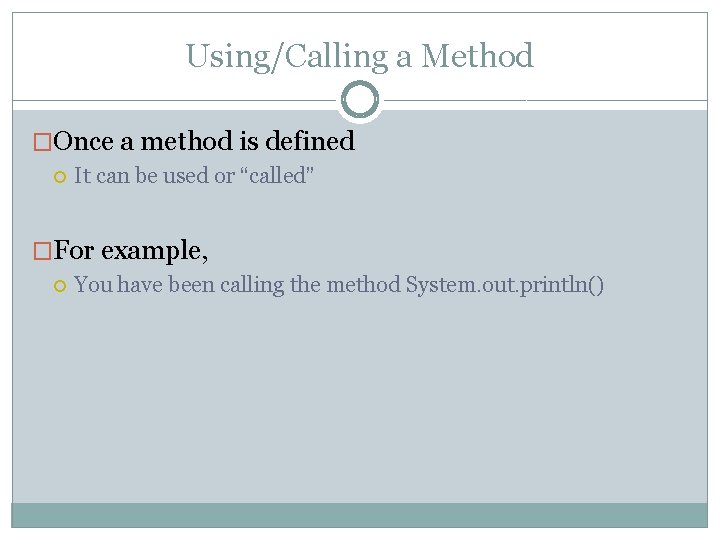
Using/Calling a Method �Once a method is defined It can be used or “called” �For example, You have been calling the method System. out. println()
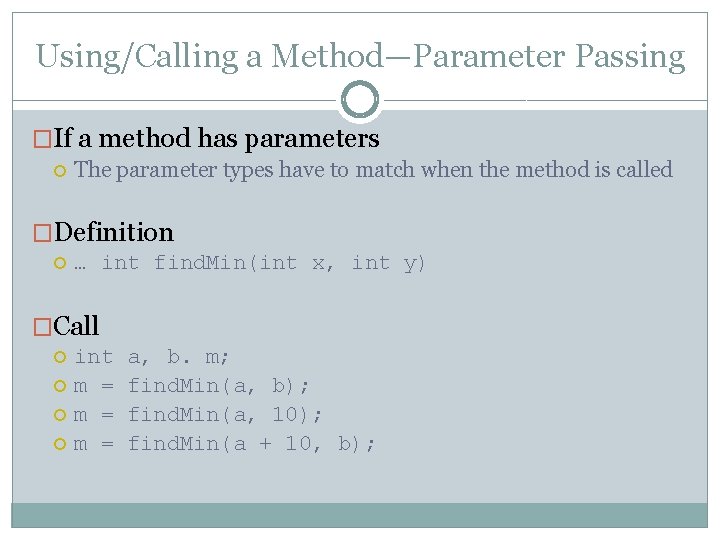
Using/Calling a Method—Parameter Passing �If a method has parameters The parameter types have to match when the method is called �Definition … int find. Min(int x, int y) �Call int m = m = a, b. m; find. Min(a, b); find. Min(a, 10); find. Min(a + 10, b);
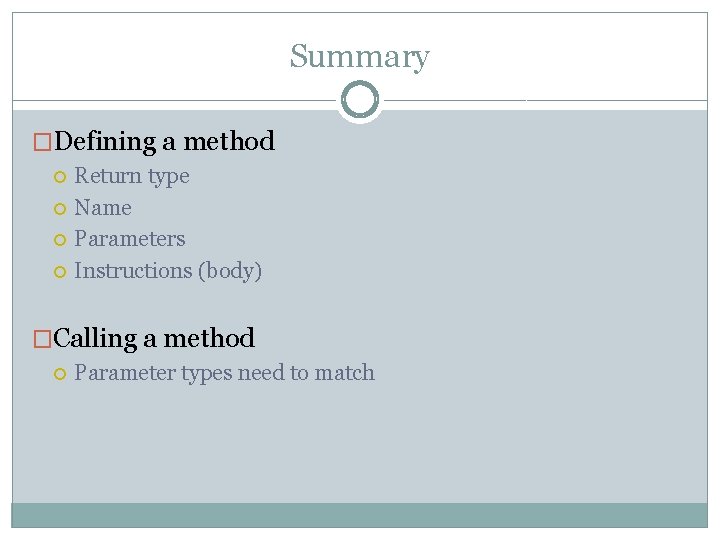
Summary �Defining a method Return type Name Parameters Instructions (body) �Calling a method Parameter types need to match
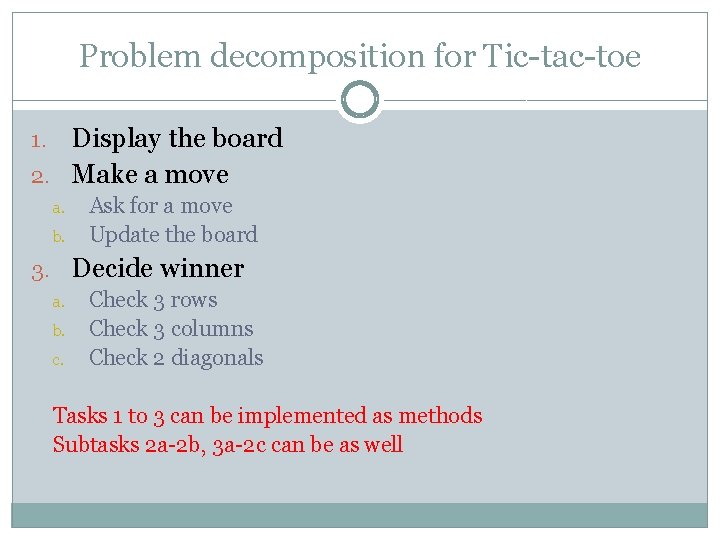
Problem decomposition for Tic-tac-toe Display the board 2. Make a move 1. a. b. Ask for a move Update the board 3. Decide winner a. b. c. Check 3 rows Check 3 columns Check 2 diagonals Tasks 1 to 3 can be implemented as methods Subtasks 2 a-2 b, 3 a-2 c can be as well
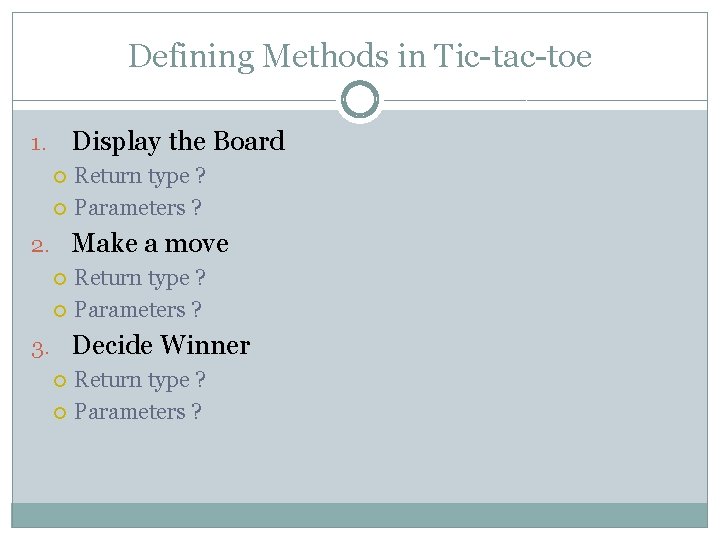
Defining Methods in Tic-tac-toe Display the Board 1. Return type ? Parameters ? 2. Make a move Return type ? Parameters ? 3. Decide Winner Return type ? Parameters ?
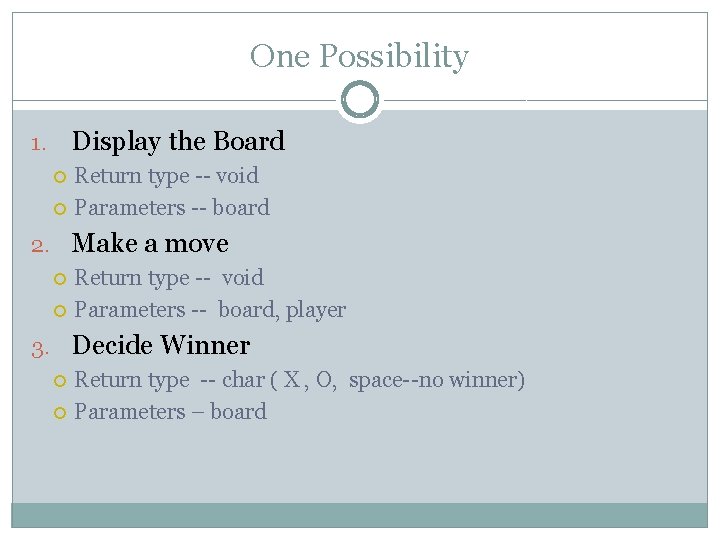
One Possibility Display the Board 1. Return type -- void Parameters -- board 2. Make a move Return type -- void Parameters -- board, player 3. Decide Winner Return type -- char ( X , O, space--no winner) Parameters – board
![Main methodfirst level in decomposition main char board new char33 char Main method—first level in decomposition … main(…) { char[][] board = new char[3][3]; char](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-16.jpg)
Main method—first level in decomposition … main(…) { char[][] board = new char[3][3]; char player = ’X’, winner = ’ ’; // initialize the board … display. Board(board); make. AMove(board, player); winner = decide. Winner(board); }
![Main methodRefining it main char board new char33 char player Main method—Refining it … main(…) { char[][] board = new char[3][3]; char player =](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-17.jpg)
Main method—Refining it … main(…) { char[][] board = new char[3][3]; char player = ’X’, winner = ’ ’; // initialize the board … do { display. Board(board); make. AMove(board, player); player = switch. Player(player); winner = decide. Winner(board); } while (…); // no winner and board is not full }
![Main method highlevel readable main char board new char33 char Main method (high-level & readable) … main(…) { char[][] board = new char[3][3]; char](https://slidetodoc.com/presentation_image_h2/e612c1d5d5de2a14784c743bf529ffff/image-18.jpg)
Main method (high-level & readable) … main(…) { char[][] board = new char[3][3]; char player = ’X’, winner = ’ ’; // initialize the board … do { display. Board(board); make. AMove(board, player); player = switch. Player(player); winner = decide. Winner(board); } while (winner == ’ ’ && !is. Full(board)); // no winner and board is not full }
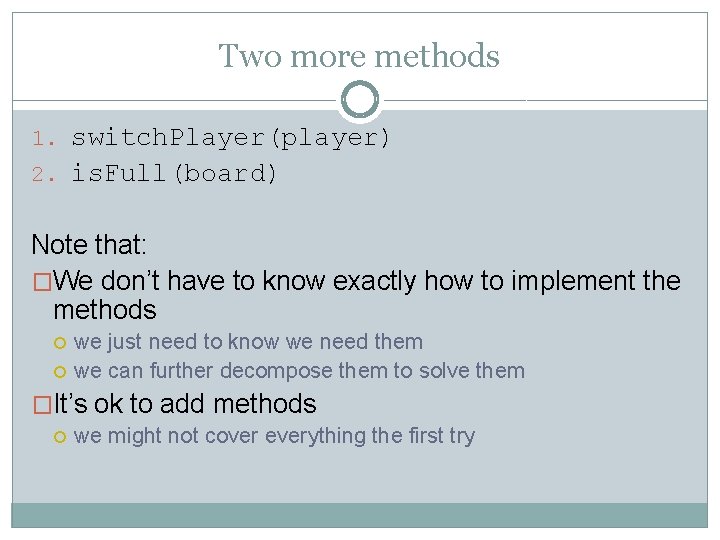
Two more methods 1. switch. Player(player) 2. is. Full(board) Note that: �We don’t have to know exactly how to implement the methods we just need to know we need them we can further decompose them to solve them �It’s ok to add methods we might not cover everything the first try
Mutator and accessor methods in java
Mutator and accessor methods in java
Mutator and accessor methods in java
Overloading methods and constructors in java
Indirect methods of contoring uses how many methods
Lesson 4 parameters and return make
Code.org unit 7 lesson 3
Common critical values
Lesson 3 parameters and return practice 7
Extrinsic parameters of food
Instrumentation and control systems ppt
Measurement and control of bioprocess parameters
Entropy order parameters and complexity
Java string methods
User defined functions in java
Java string methods
Jav msit
Undo subst command
Lennard-jones parameters table
Y parameter