IO Multiplexing What is IO multiplexing When an
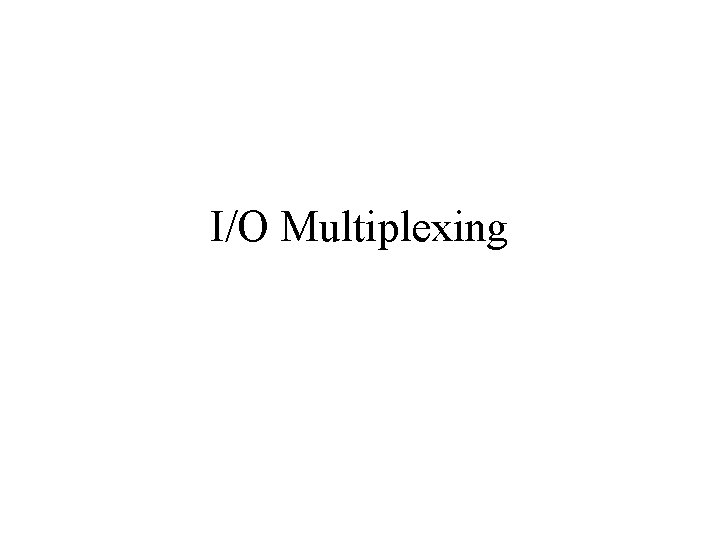
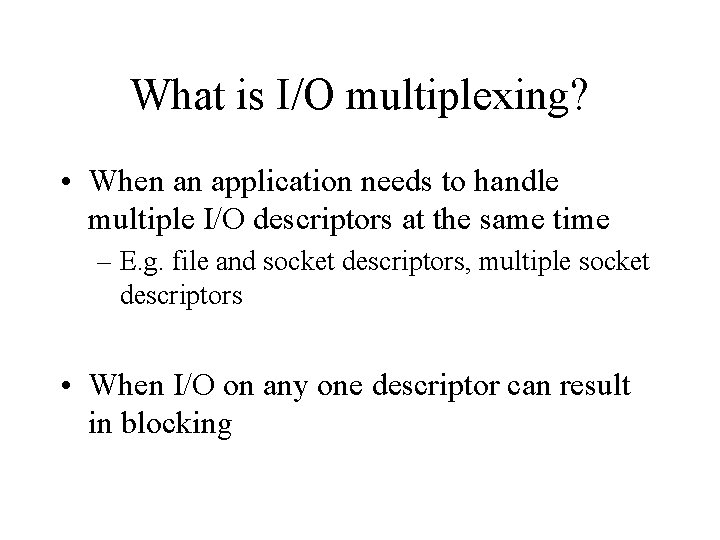
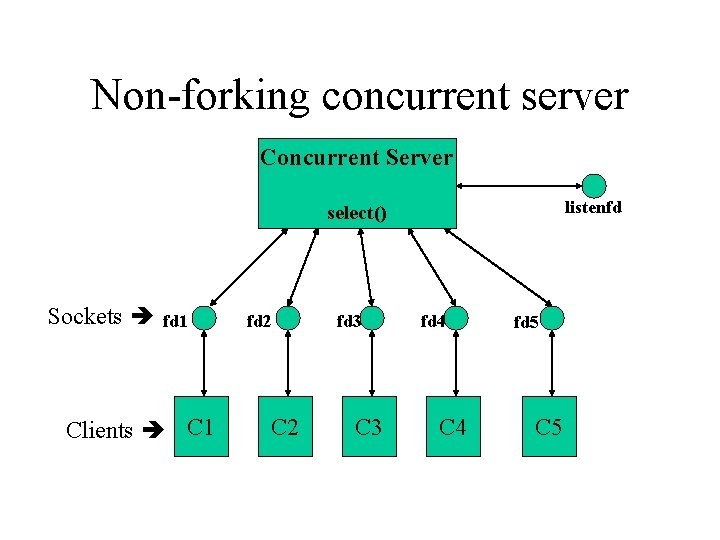
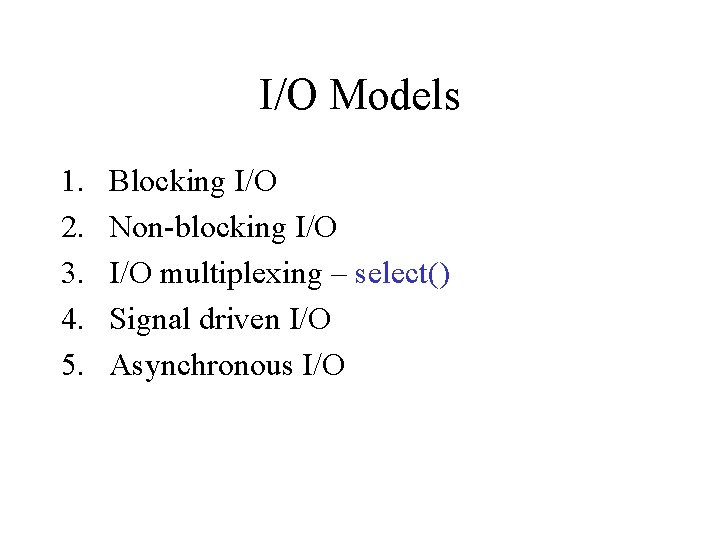
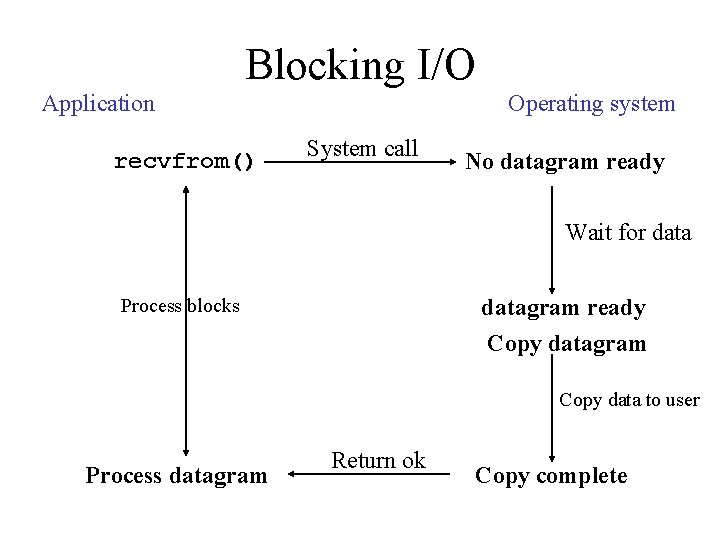
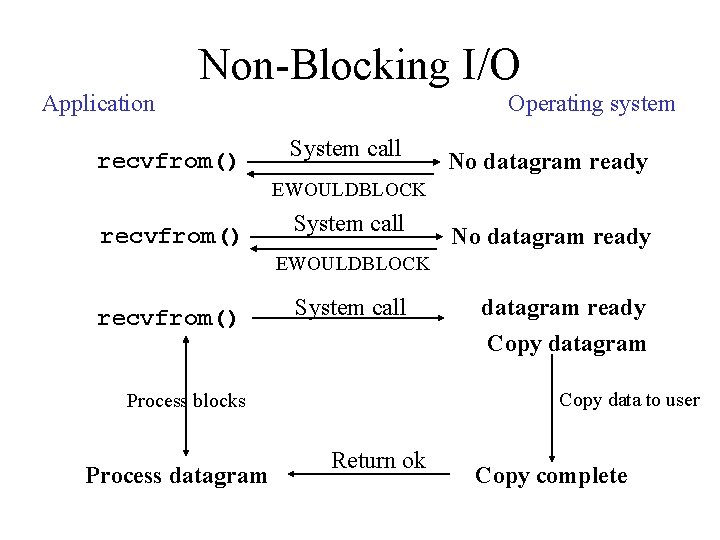
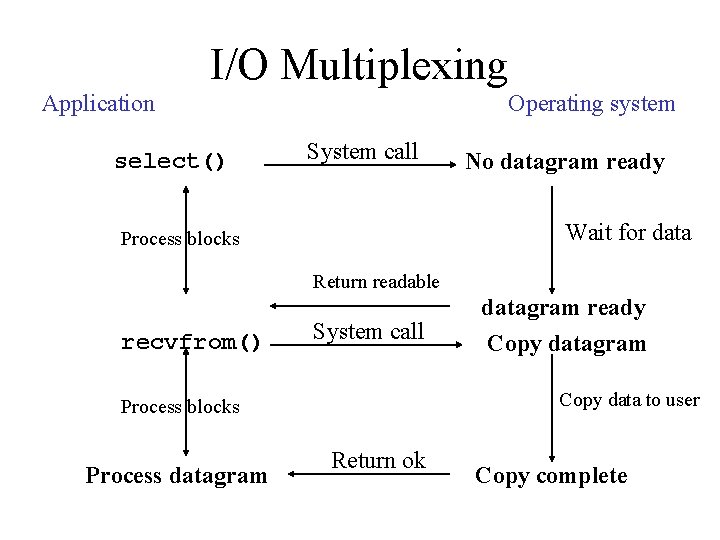
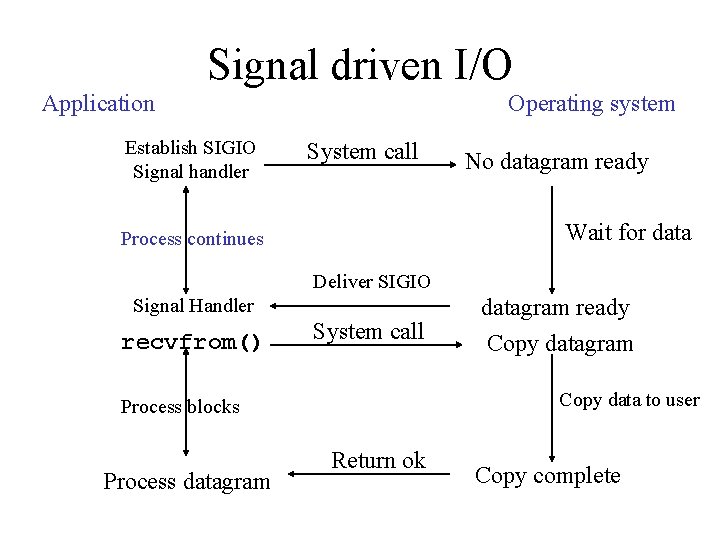
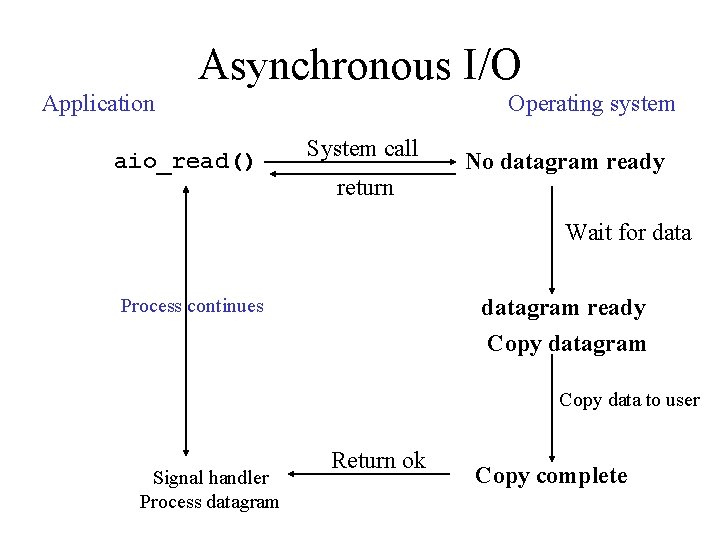
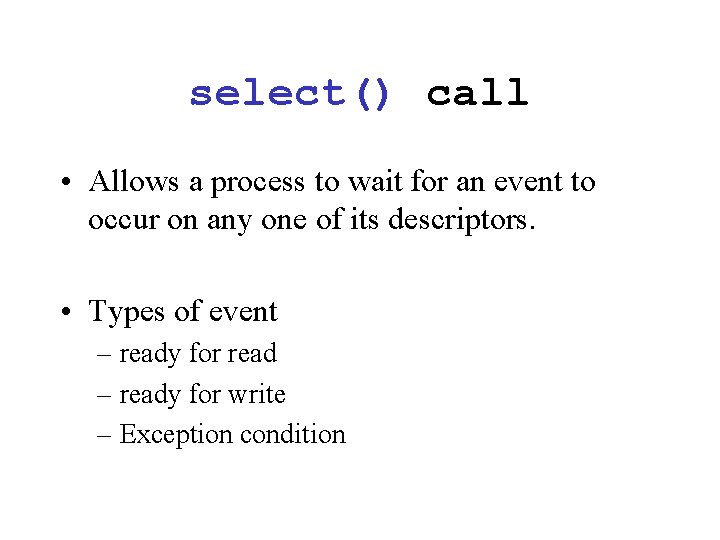
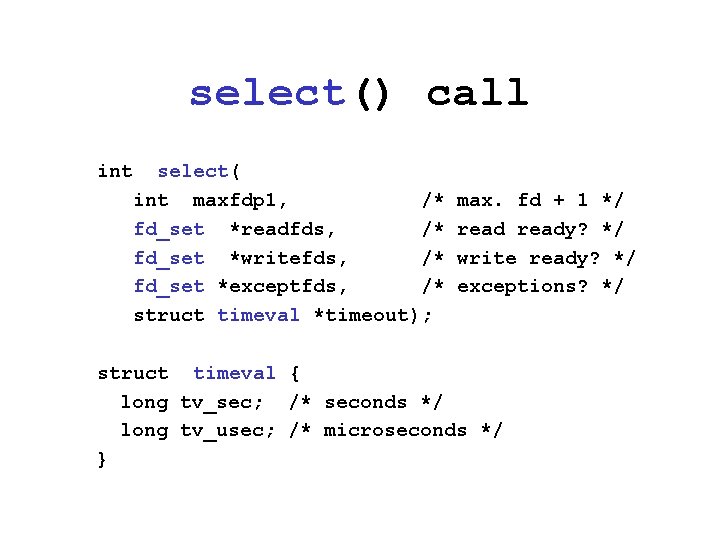
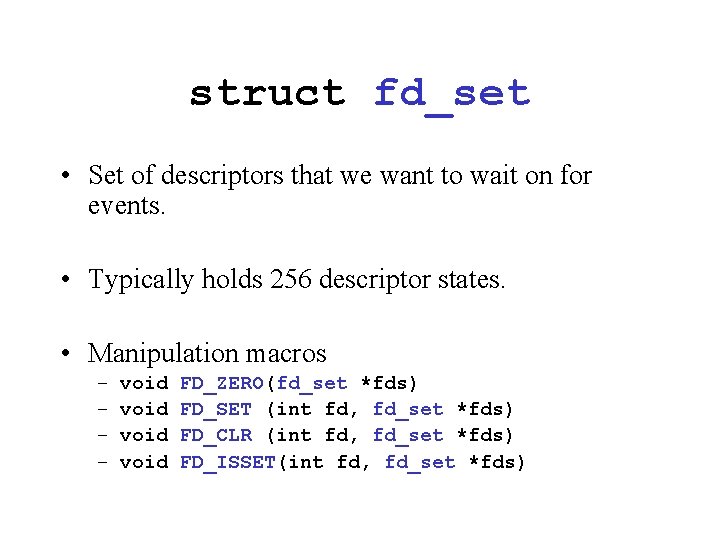
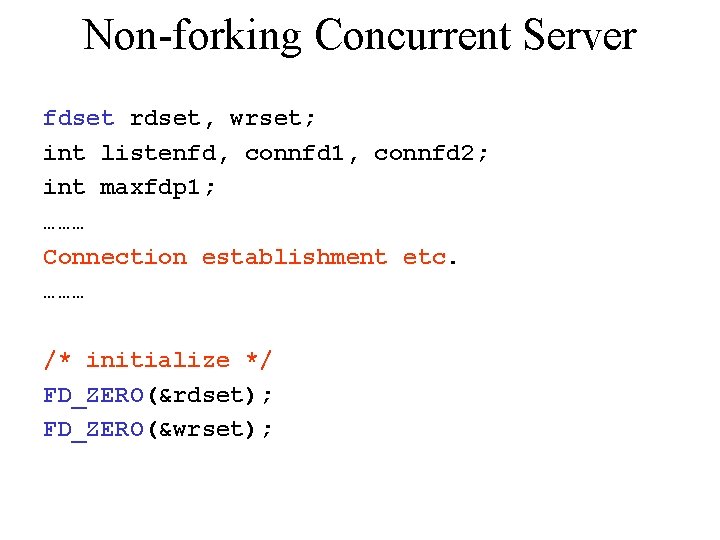
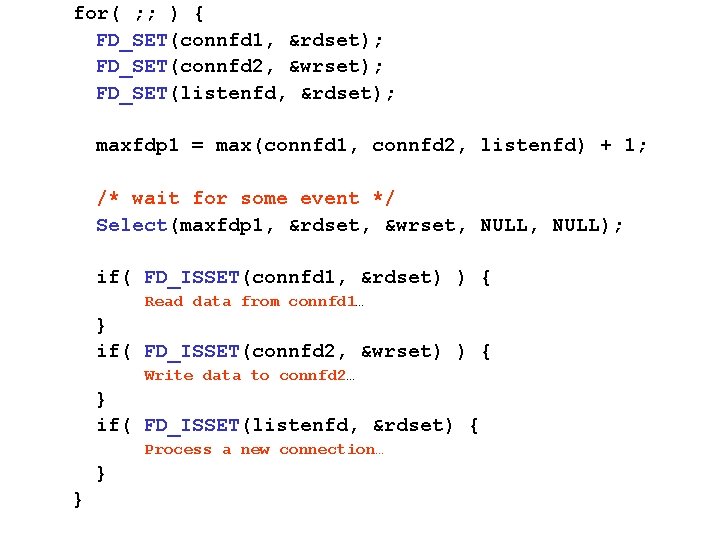
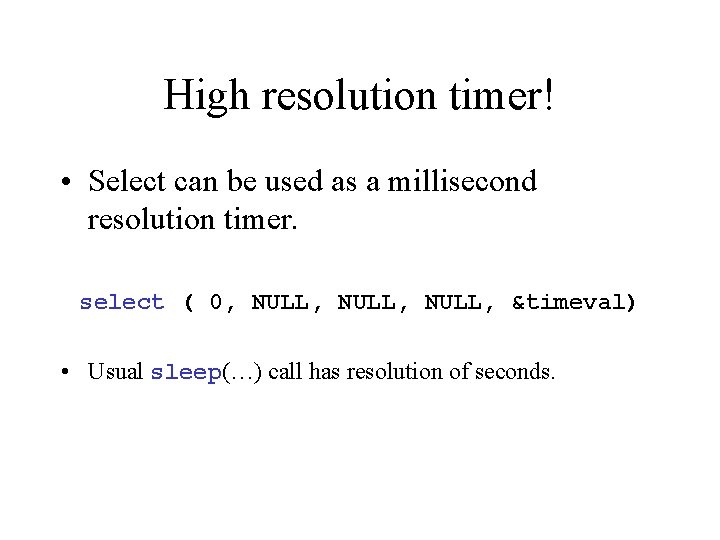
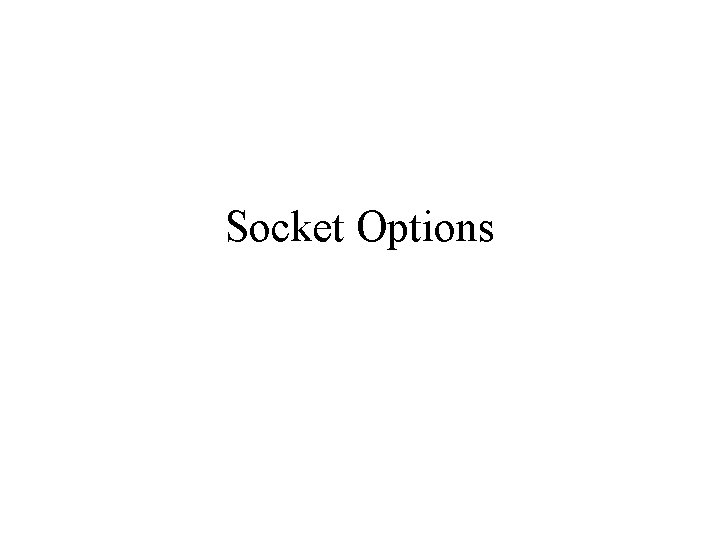
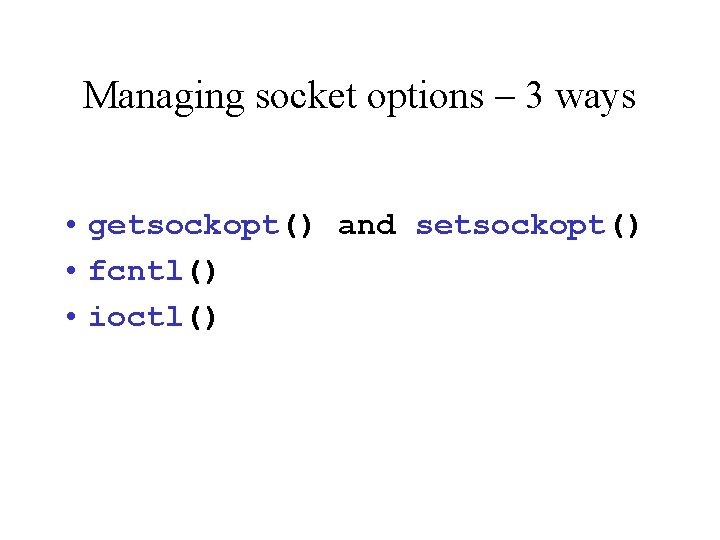
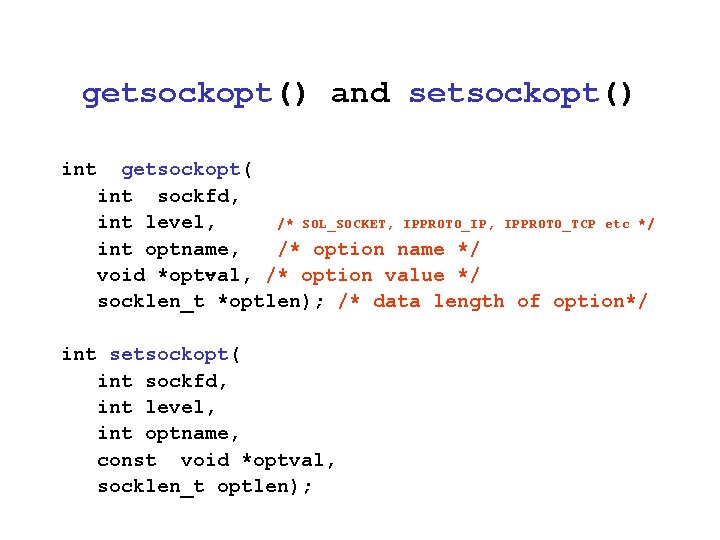
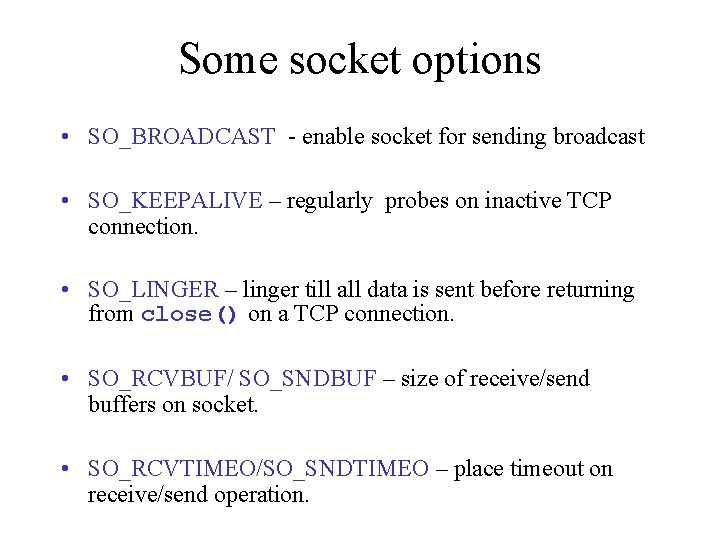
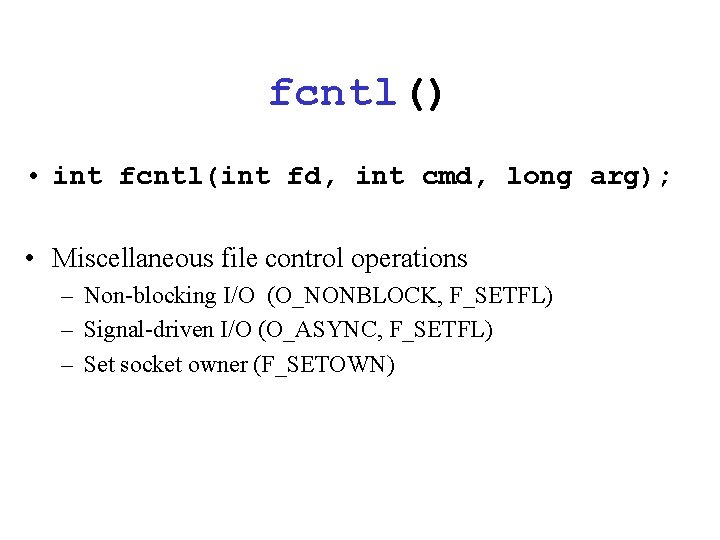
- Slides: 20
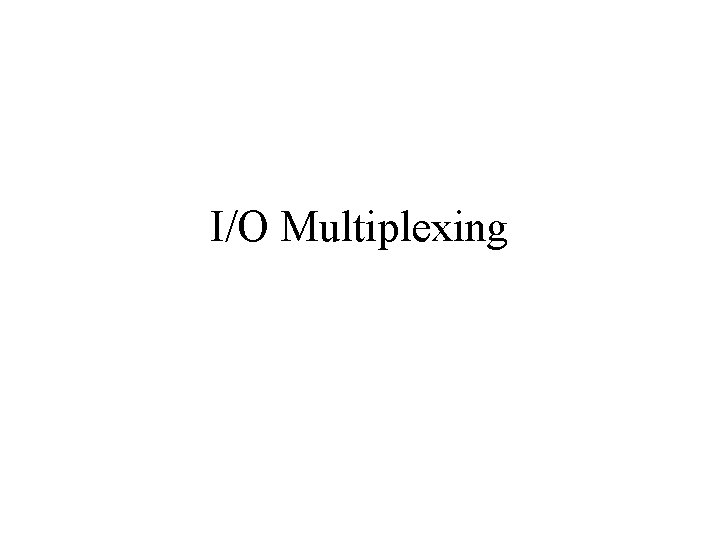
I/O Multiplexing
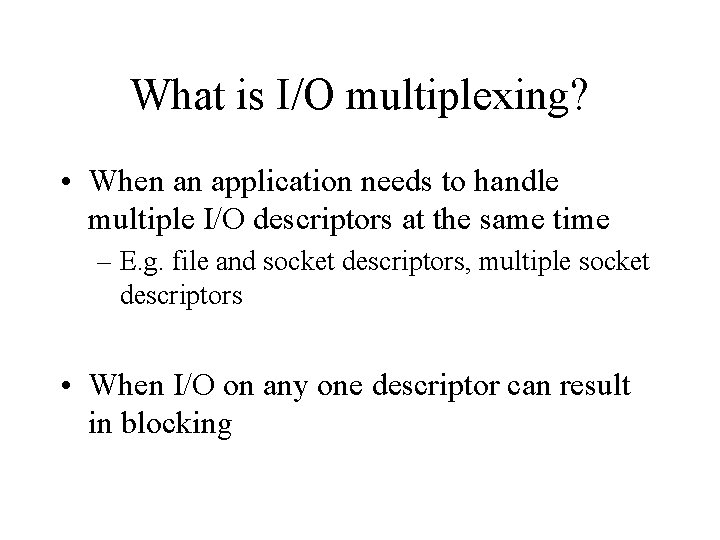
What is I/O multiplexing? • When an application needs to handle multiple I/O descriptors at the same time – E. g. file and socket descriptors, multiple socket descriptors • When I/O on any one descriptor can result in blocking
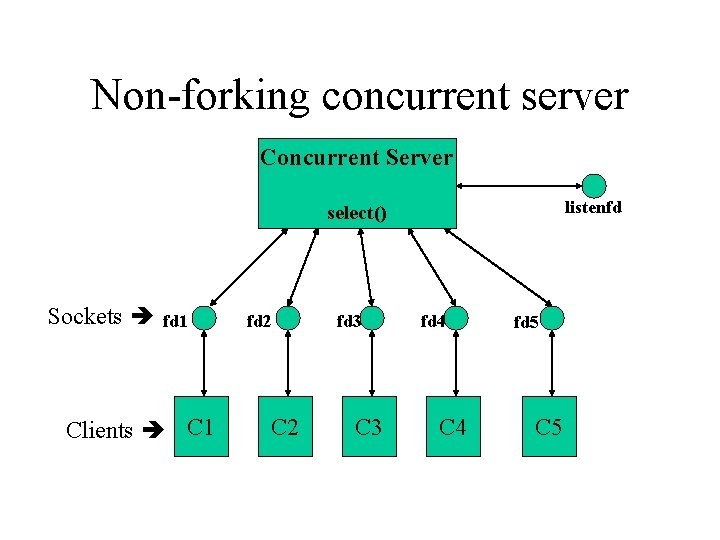
Non-forking concurrent server Concurrent Server listenfd select() Sockets fd 1 Clients C 1 fd 2 C 2 fd 3 C 3 fd 4 C 4 fd 5 C 5
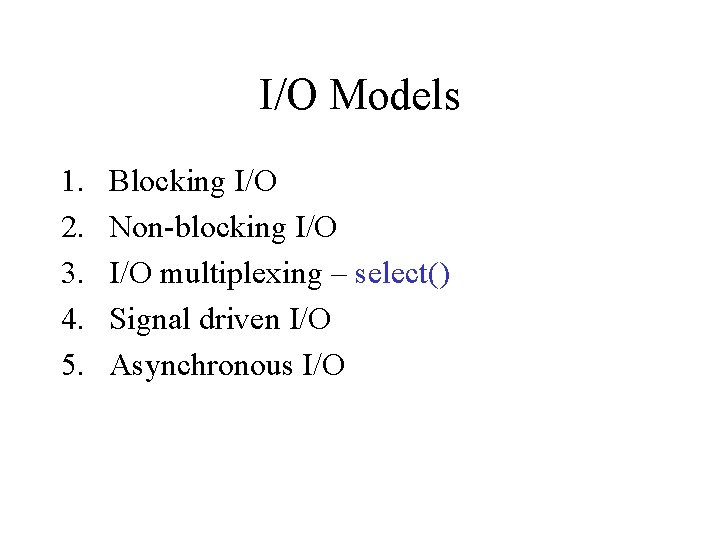
I/O Models 1. 2. 3. 4. 5. Blocking I/O Non-blocking I/O multiplexing – select() Signal driven I/O Asynchronous I/O
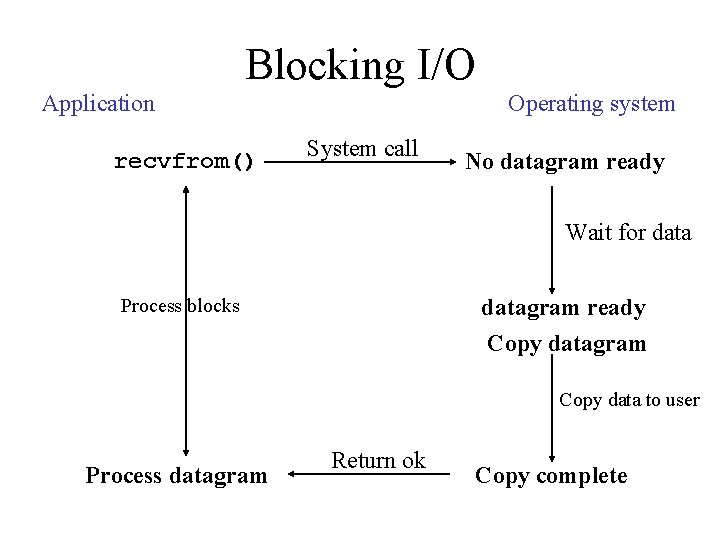
Blocking I/O Application recvfrom() Operating system System call No datagram ready Wait for datagram ready Copy datagram Process blocks Copy data to user Process datagram Return ok Copy complete
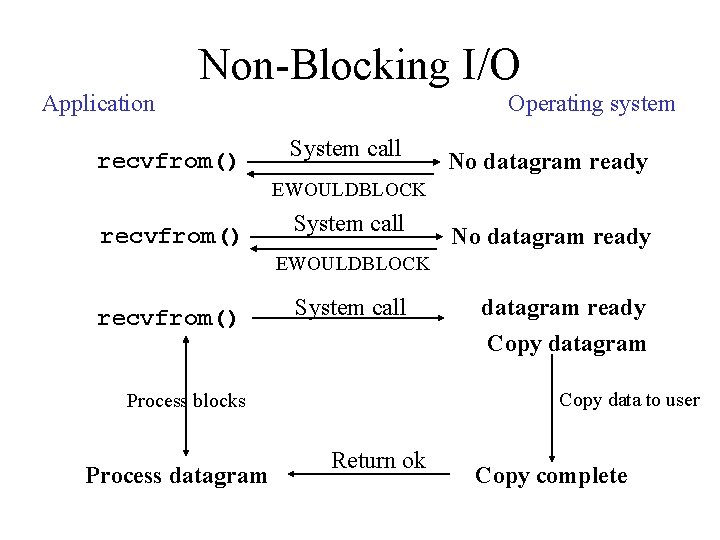
Non-Blocking I/O Application recvfrom() Operating system System call No datagram ready EWOULDBLOCK recvfrom() System call Copy data to user Process blocks Process datagram ready Copy datagram Return ok Copy complete
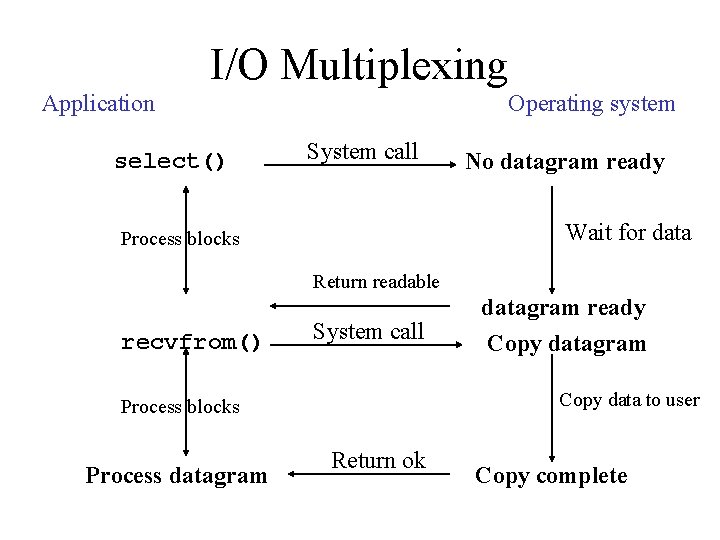
I/O Multiplexing Application select() Operating system System call No datagram ready Wait for data Process blocks Return readable recvfrom() System call Copy data to user Process blocks Process datagram ready Copy datagram Return ok Copy complete
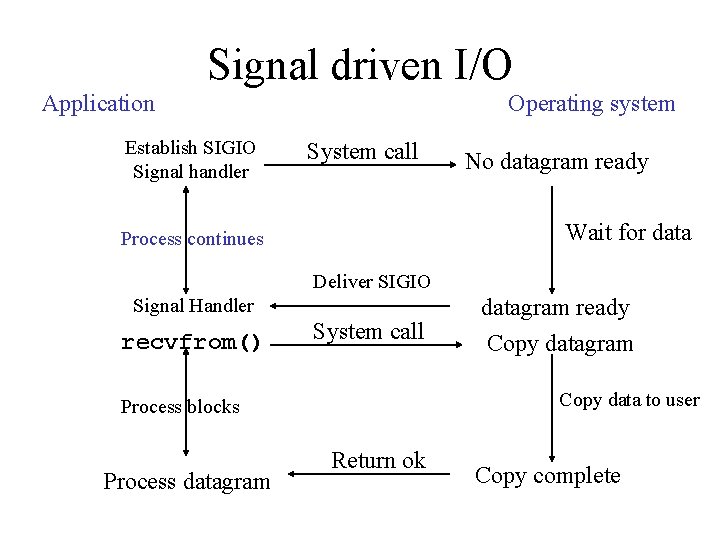
Signal driven I/O Application Establish SIGIO Signal handler Operating system System call No datagram ready Wait for data Process continues Deliver SIGIO Signal Handler recvfrom() System call Copy data to user Process blocks Process datagram ready Copy datagram Return ok Copy complete
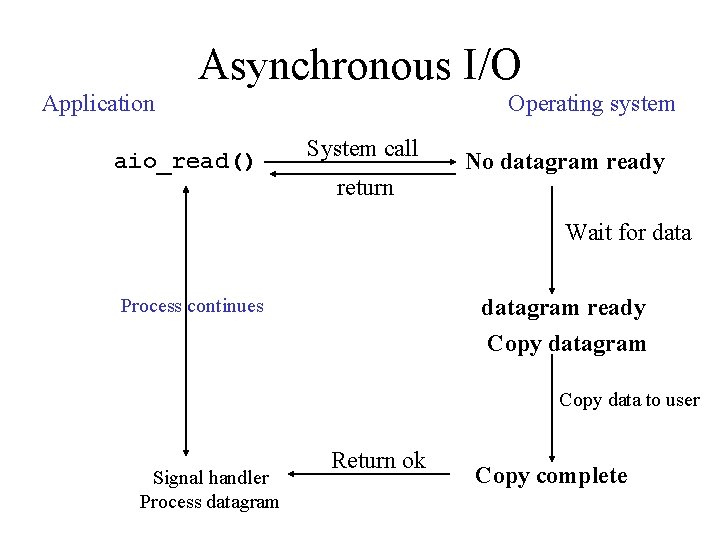
Asynchronous I/O Application aio_read() Operating system System call return No datagram ready Wait for datagram ready Copy datagram Process continues Copy data to user Signal handler Process datagram Return ok Copy complete
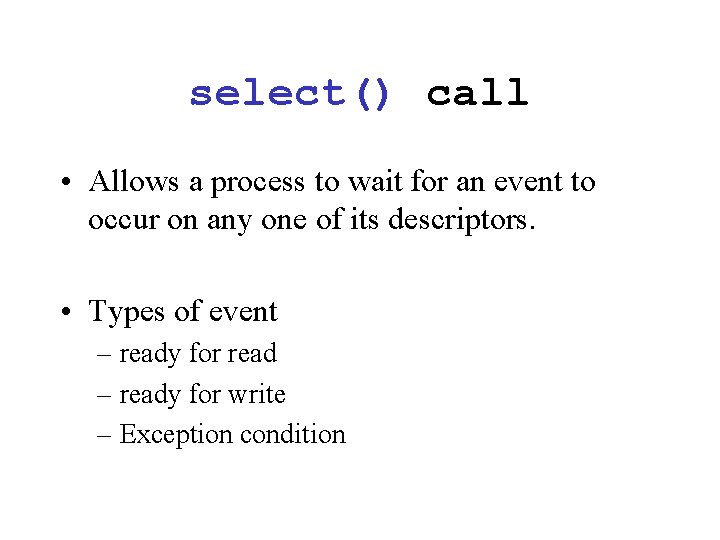
select() call • Allows a process to wait for an event to occur on any one of its descriptors. • Types of event – ready for read – ready for write – Exception condition
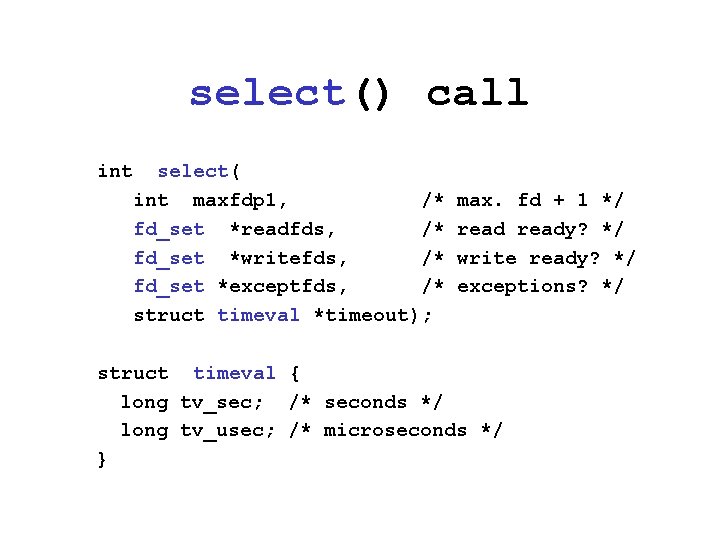
select() call int select( int maxfdp 1, /* fd_set *readfds, /* fd_set *writefds, /* fd_set *exceptfds, /* struct timeval *timeout); max. fd + 1 */ ready? */ write ready? */ exceptions? */ struct timeval { long tv_sec; /* seconds */ long tv_usec; /* microseconds */ }
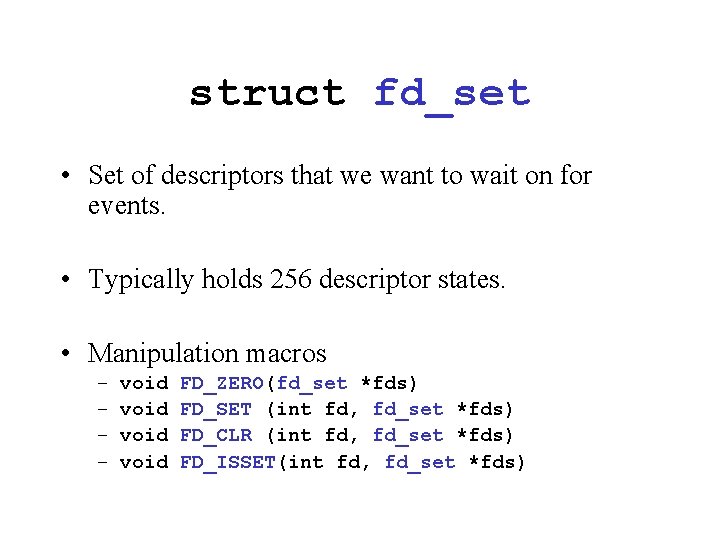
struct fd_set • Set of descriptors that we want to wait on for events. • Typically holds 256 descriptor states. • Manipulation macros – – void FD_ZERO(fd_set *fds) FD_SET (int fd, fd_set *fds) FD_CLR (int fd, fd_set *fds) FD_ISSET(int fd, fd_set *fds)
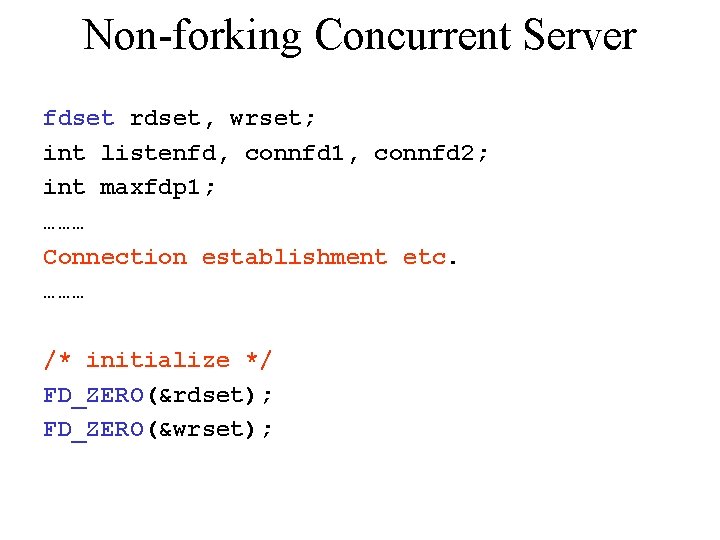
Non-forking Concurrent Server fdset rdset, wrset; int listenfd, connfd 1, connfd 2; int maxfdp 1; ……… Connection establishment etc. ……… /* initialize */ FD_ZERO(&rdset); FD_ZERO(&wrset);
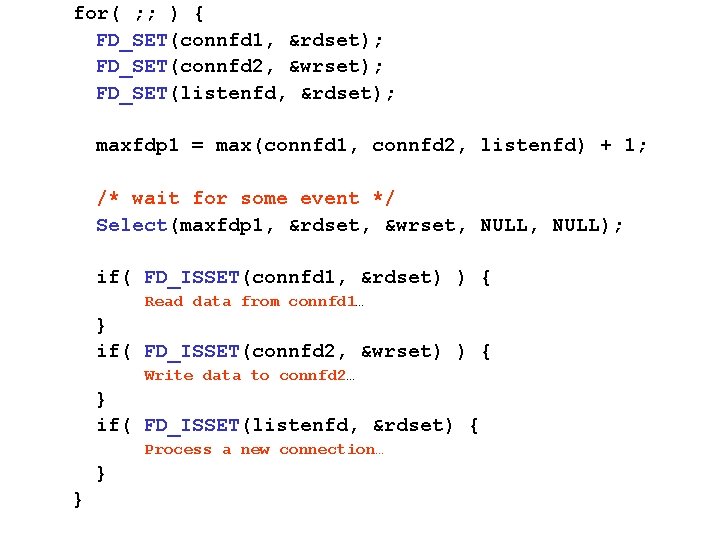
for( ; ; ) { FD_SET(connfd 1, &rdset); FD_SET(connfd 2, &wrset); FD_SET(listenfd, &rdset); maxfdp 1 = max(connfd 1, connfd 2, listenfd) + 1; /* wait for some event */ Select(maxfdp 1, &rdset, &wrset, NULL); if( FD_ISSET(connfd 1, &rdset) ) { Read data from connfd 1… } if( FD_ISSET(connfd 2, &wrset) ) { Write data to connfd 2… } if( FD_ISSET(listenfd, &rdset) { Process a new connection… } }
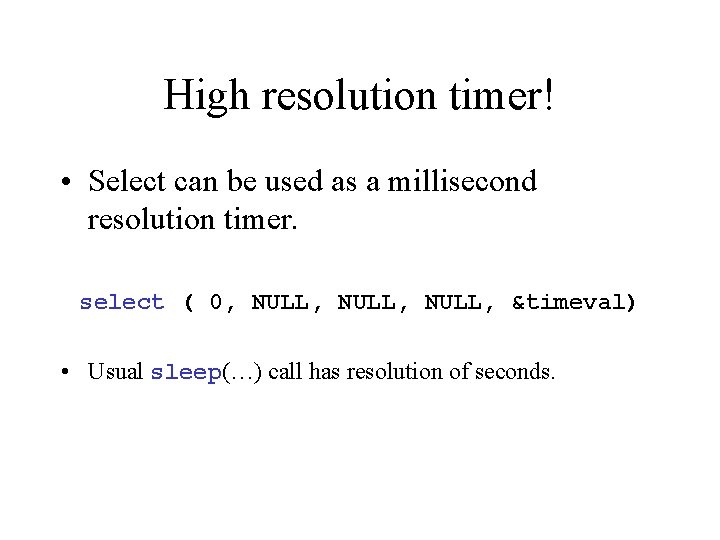
High resolution timer! • Select can be used as a millisecond resolution timer. select ( 0, NULL, &timeval) • Usual sleep(…) call has resolution of seconds.
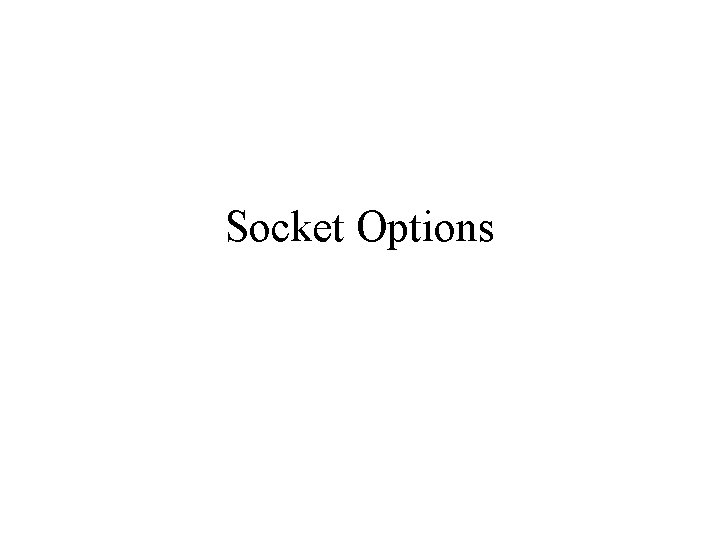
Socket Options
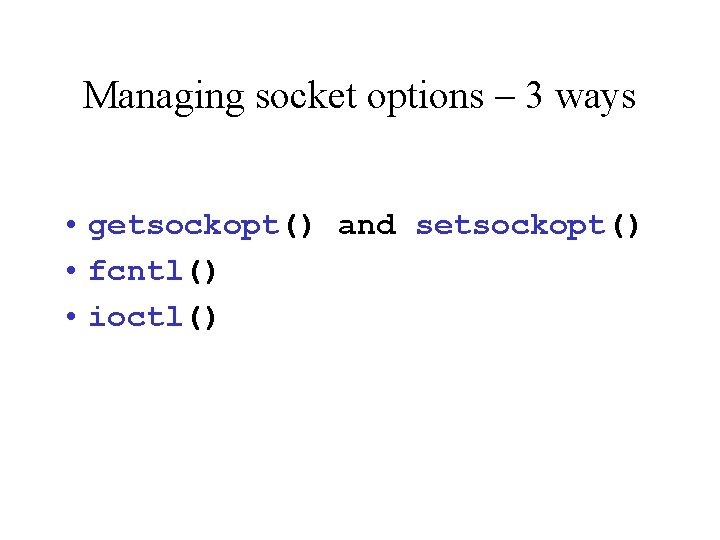
Managing socket options – 3 ways • getsockopt() and setsockopt() • fcntl() • ioctl()
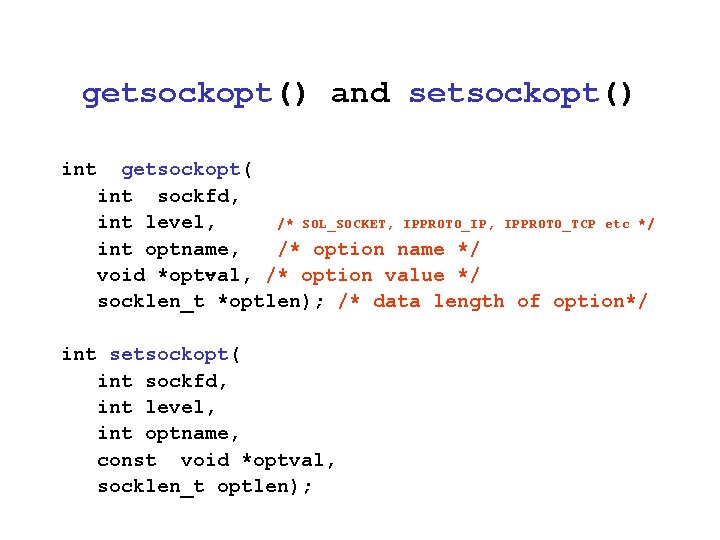
getsockopt() and setsockopt() int getsockopt( int sockfd, int level, /* SOL_SOCKET, IPPROTO_IP, IPPROTO_TCP etc */ int optname, /* option name */ void *opt val, /* option value */ socklen_t *optlen); /* data length of option*/ int setsockopt( int sockfd, int level, int optname, const void *optval, socklen_t optlen);
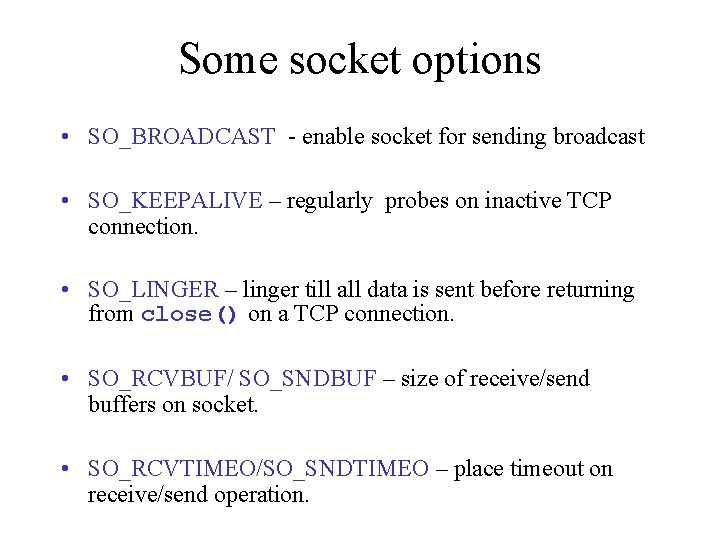
Some socket options • SO_BROADCAST - enable socket for sending broadcast • SO_KEEPALIVE – regularly probes on inactive TCP connection. • SO_LINGER – linger till all data is sent before returning from close() on a TCP connection. • SO_RCVBUF/ SO_SNDBUF – size of receive/send buffers on socket. • SO_RCVTIMEO/SO_SNDTIMEO – place timeout on receive/send operation.
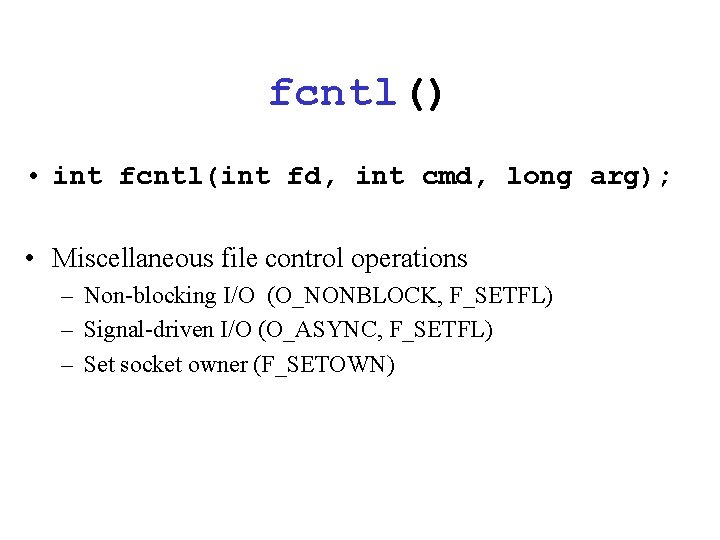
fcntl() • int fcntl(int fd, int cmd, long arg); • Miscellaneous file control operations – Non-blocking I/O (O_NONBLOCK, F_SETFL) – Signal-driven I/O (O_ASYNC, F_SETFL) – Set socket owner (F_SETOWN)
Vertical align
Upward multiplexing
Multiplexing and demultiplexing in transport layer
Io multiplexing
Code division multiplexing with example
Upward multiplexing
Pengertian multiplexing
Define statistical time division multiplexing.
Sonet multiplexing
Spatial multiplexing
Bandwidth utilization multiplexing and spreading
Sdh multiplexing structure
Multiplexing adalah
Internet access techniques
What is multiplexing and demultiplexing
Statistical multiplexing example
Difference between multiplexing and multiple access
Long term evolution - advanced
Multilevel multiplexing
Block diagram of time division multiplexing
Rtp multiplexing